Queues Chapter 5 Queue Definition A queue is
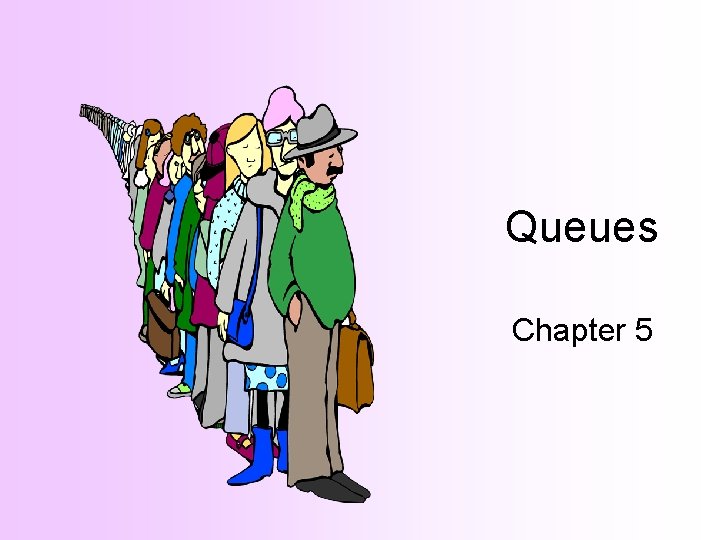
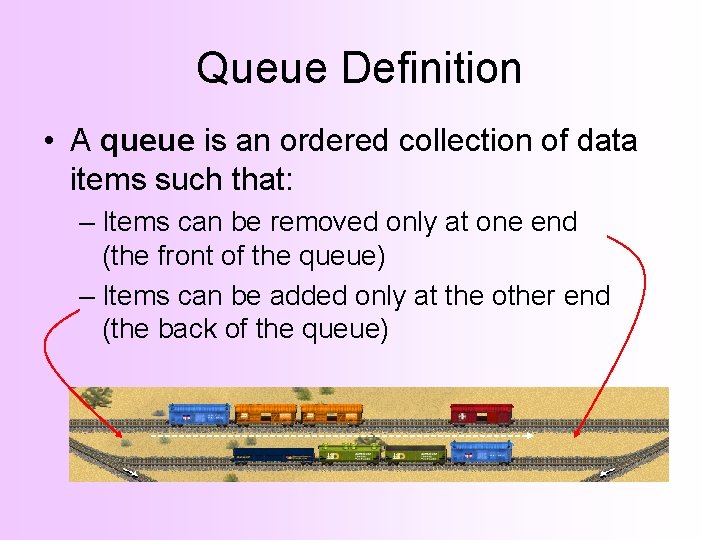
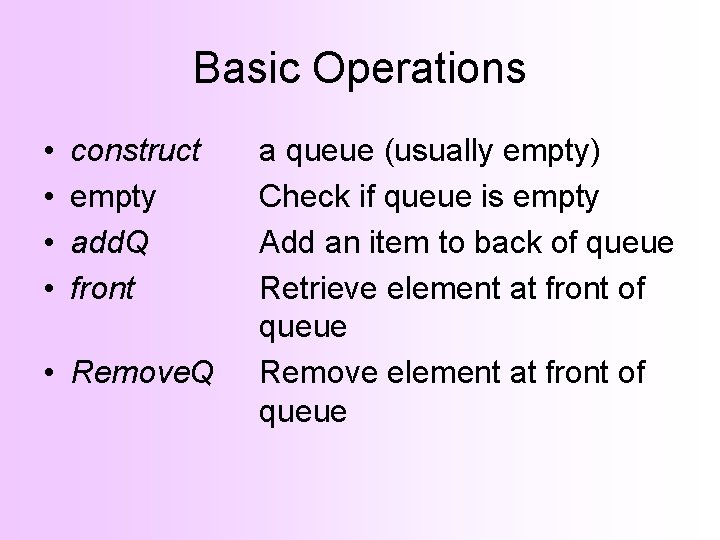
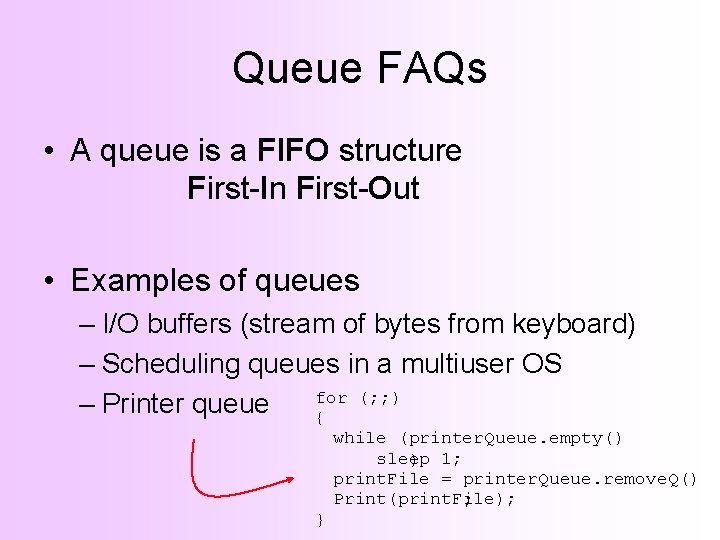
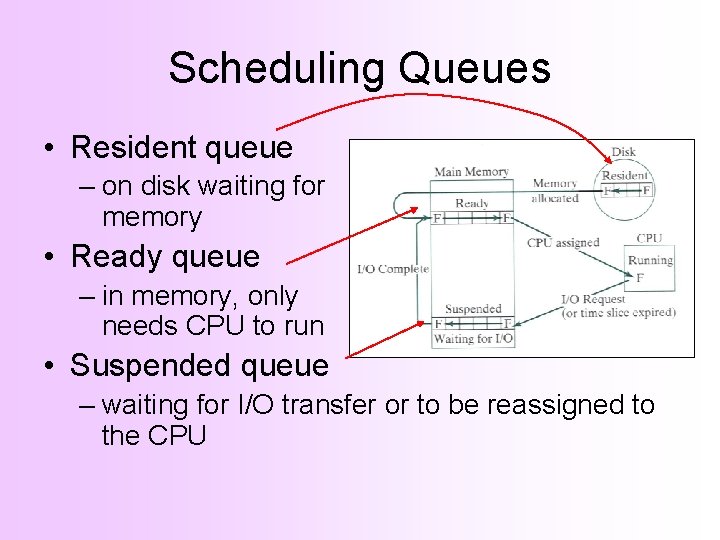
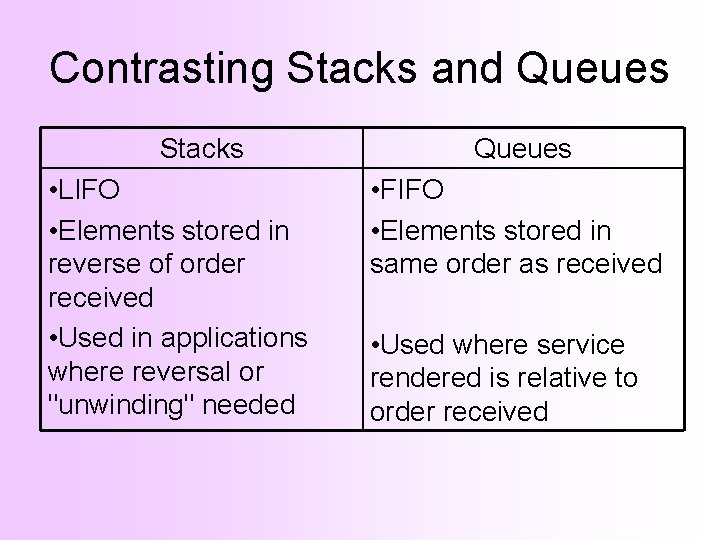
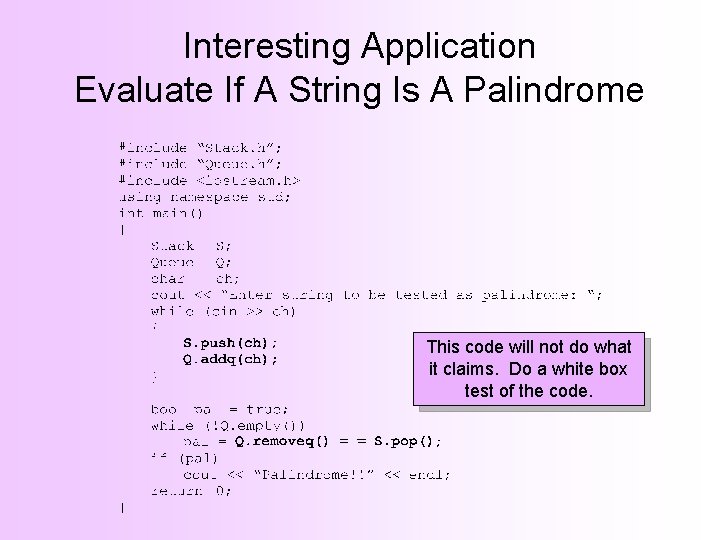
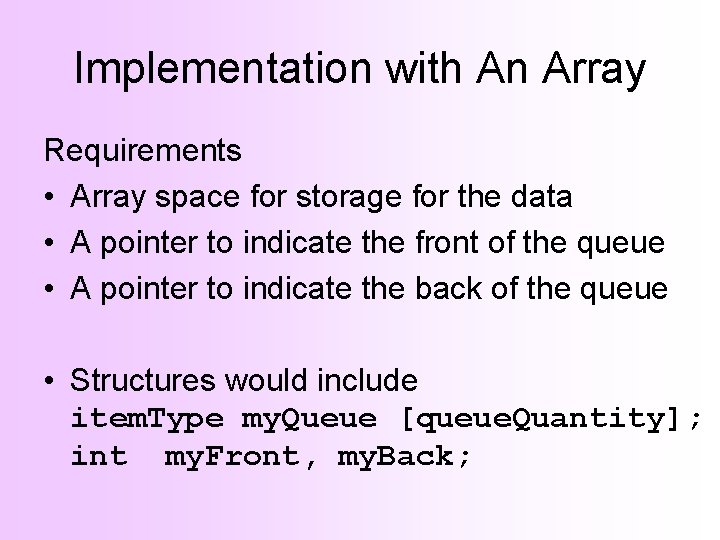
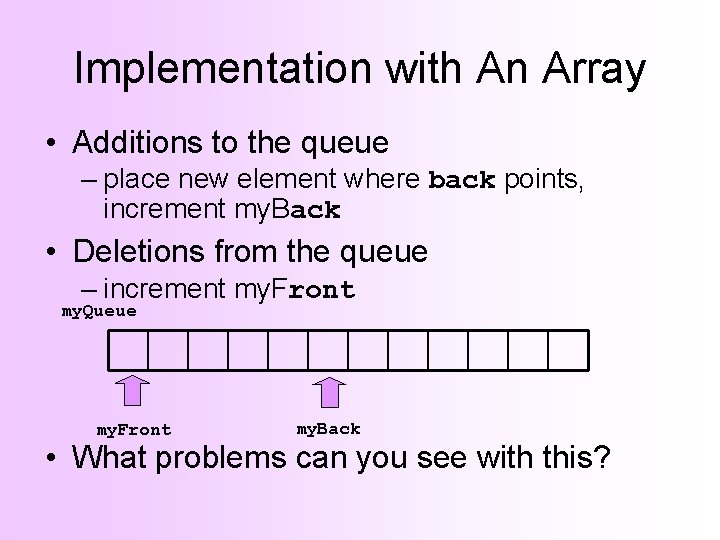
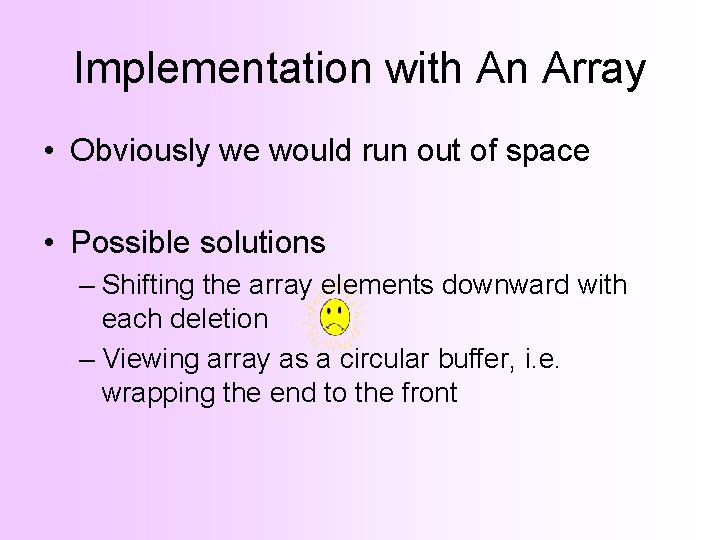
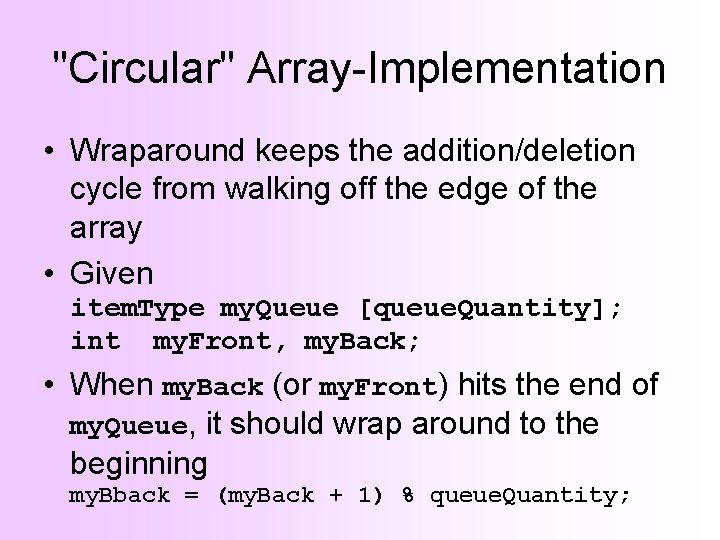
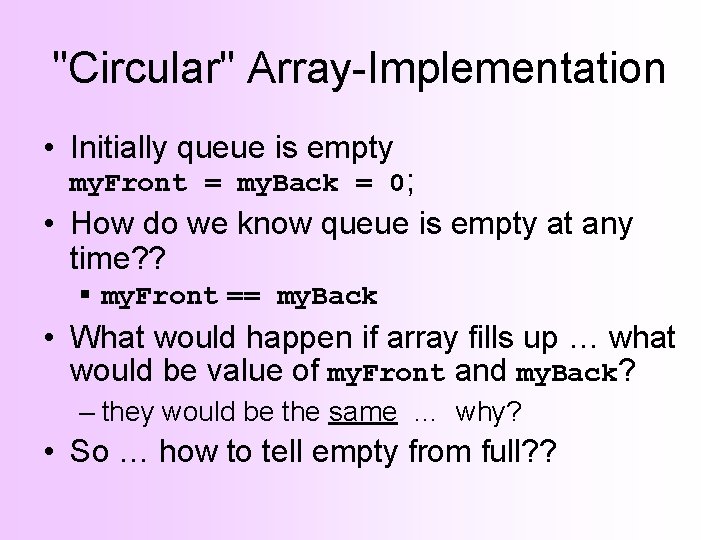
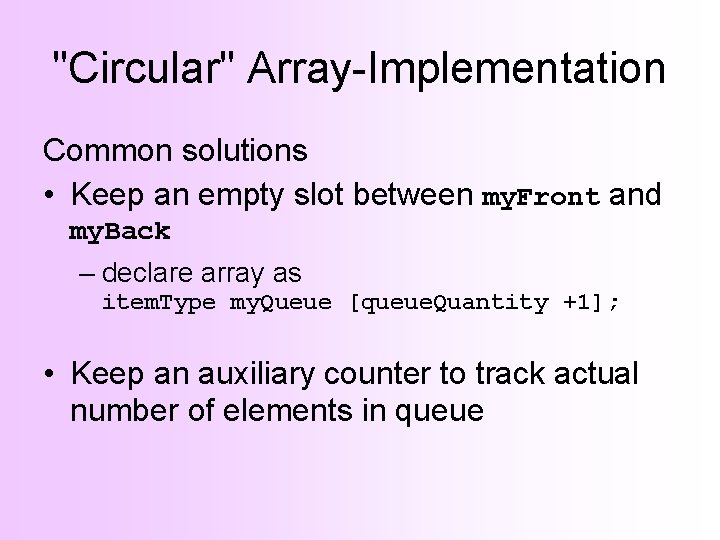
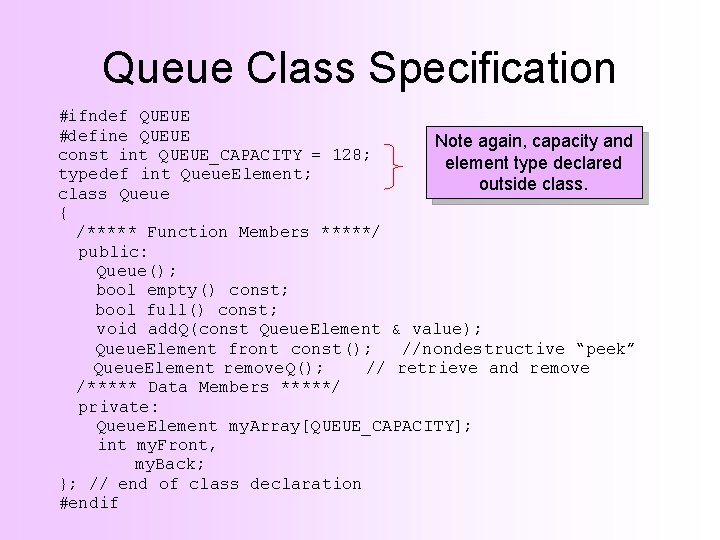
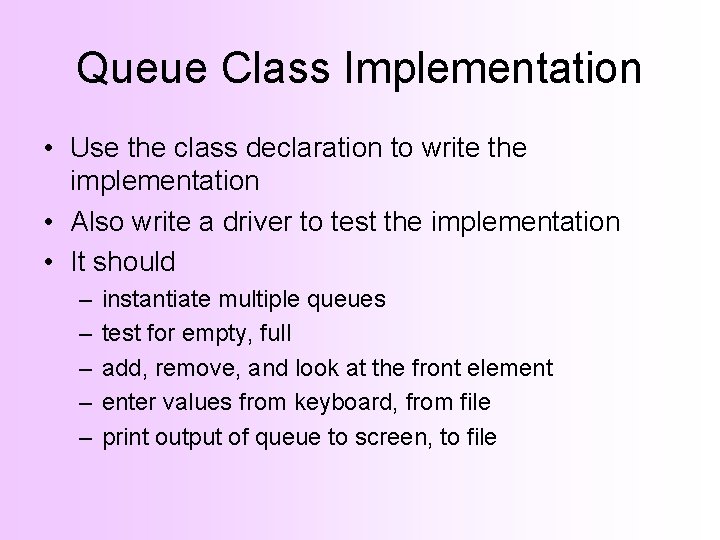
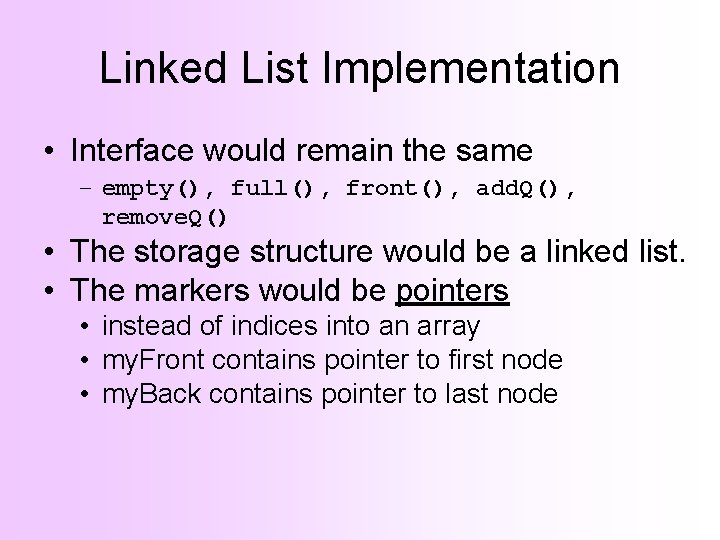
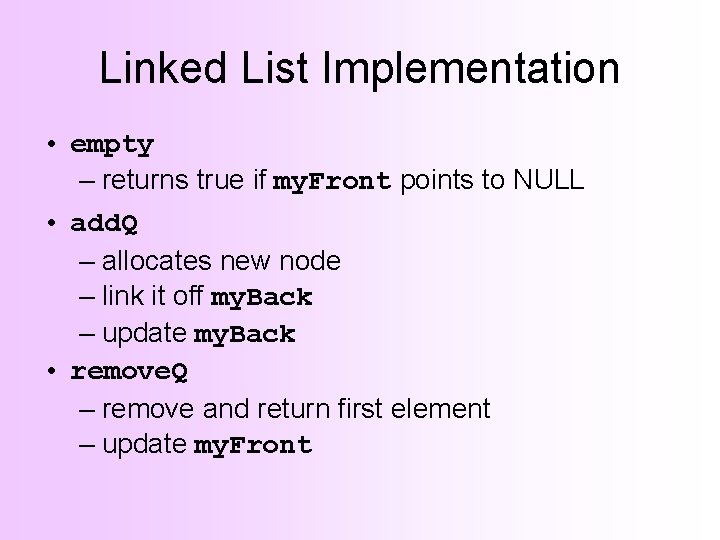
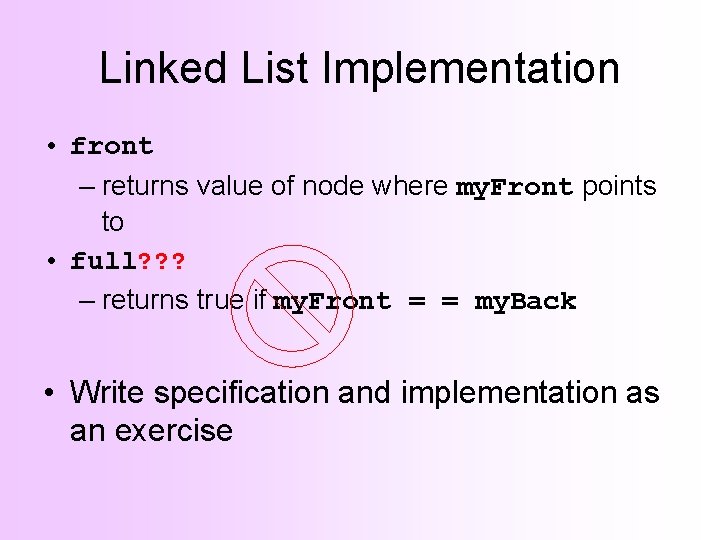
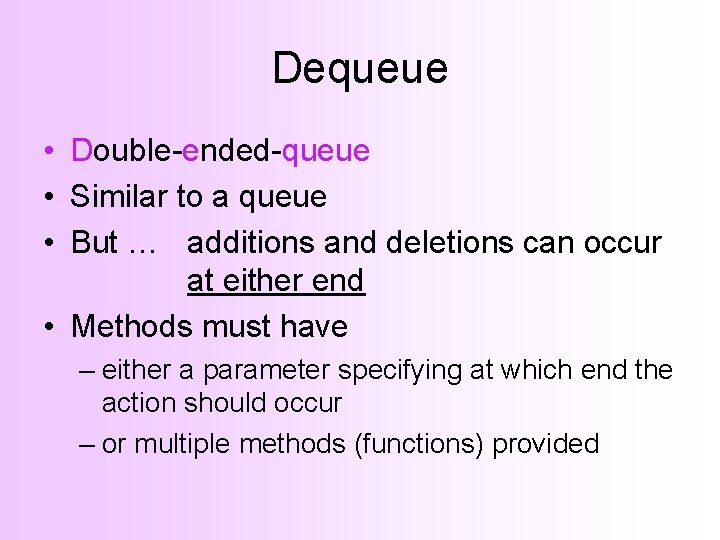
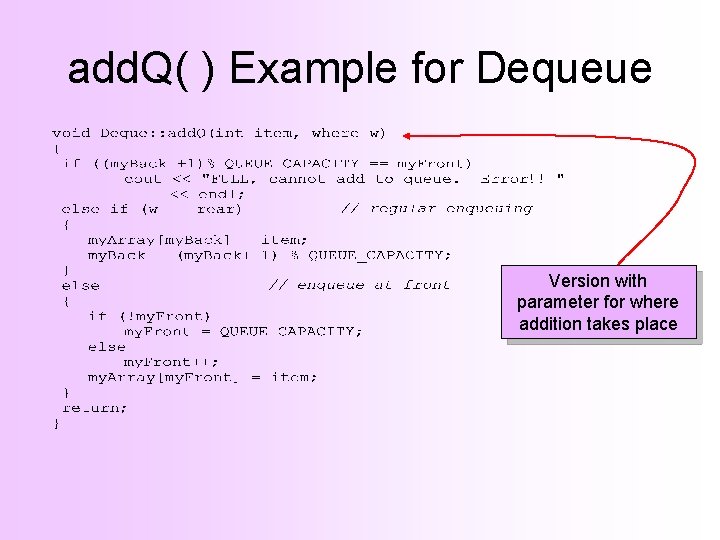
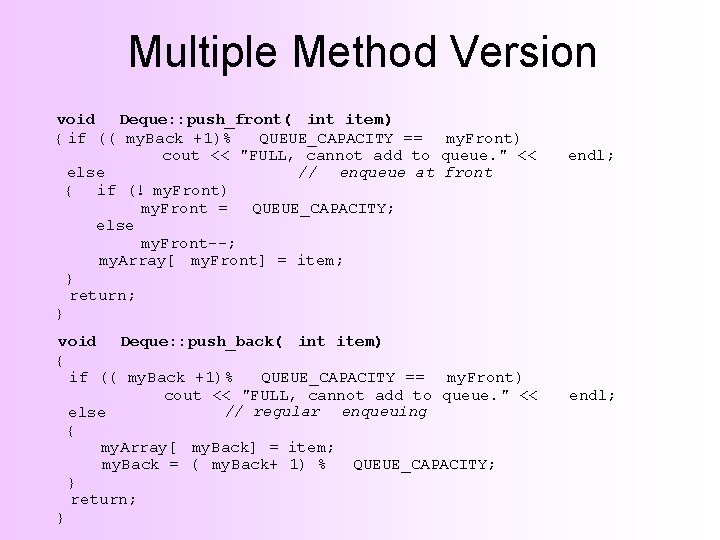
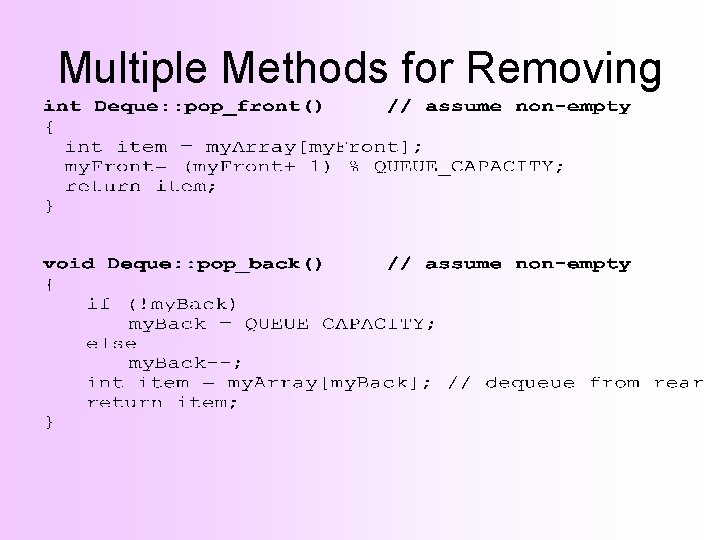
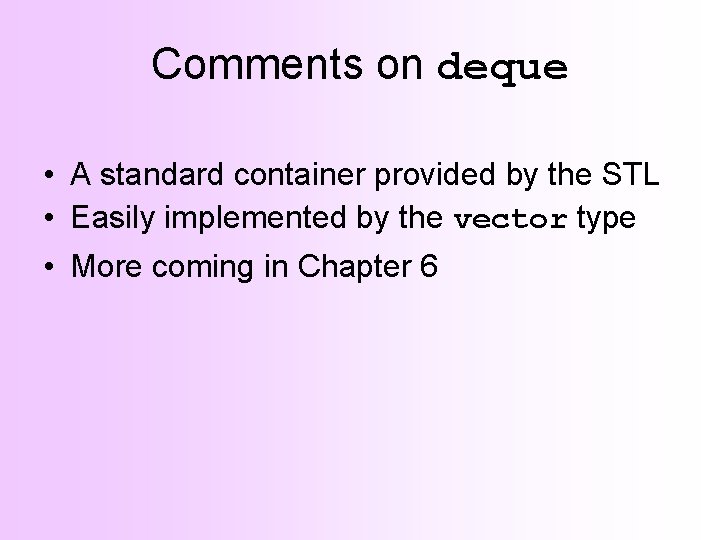
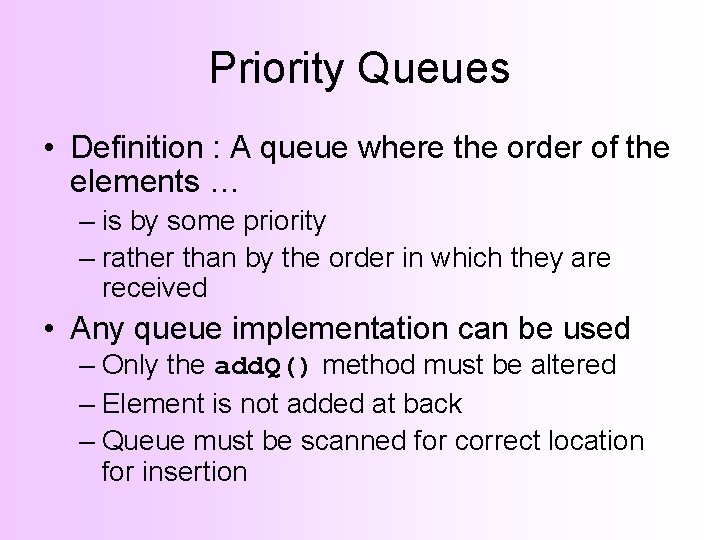
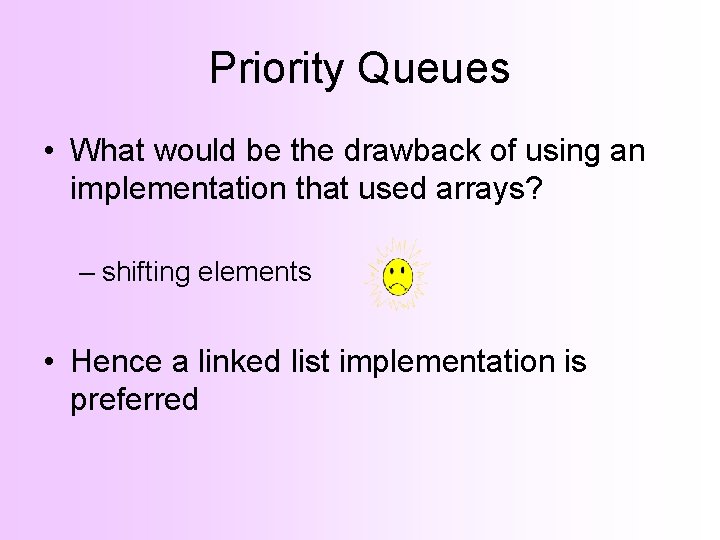
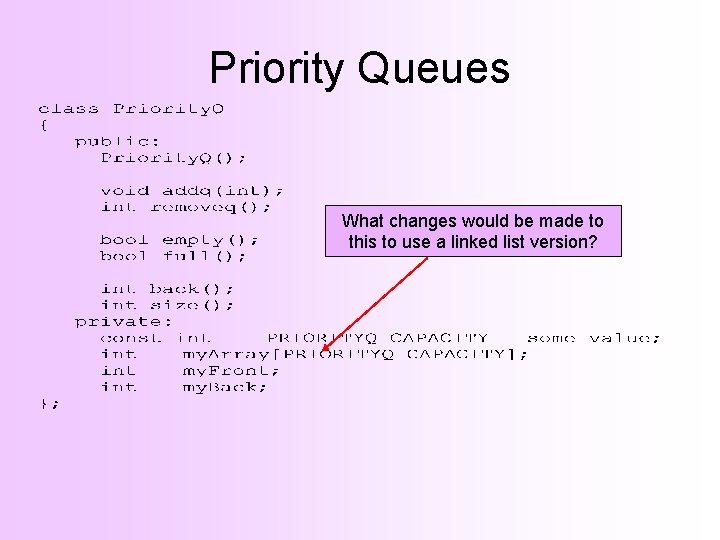
- Slides: 26
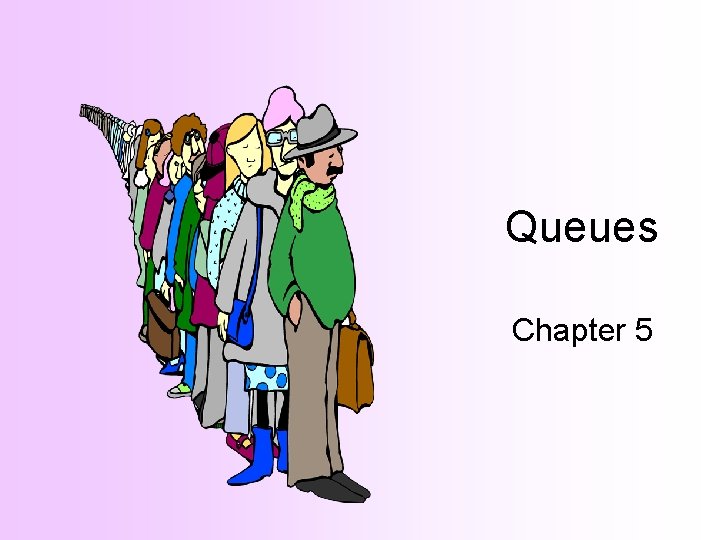
Queues Chapter 5
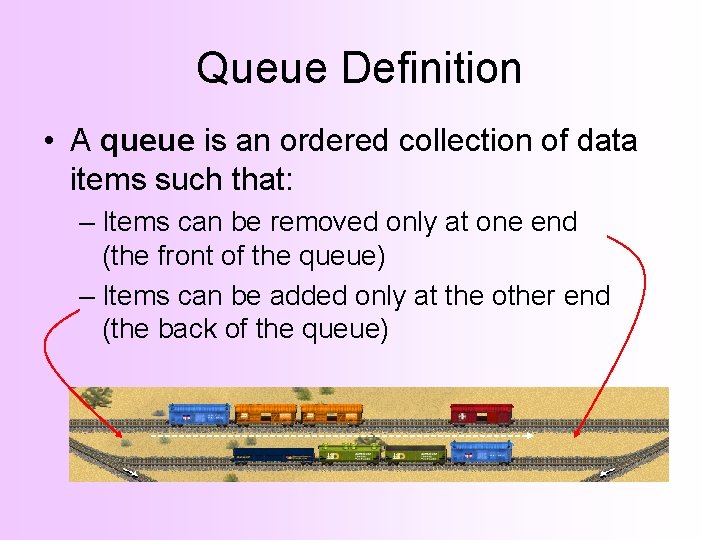
Queue Definition • A queue is an ordered collection of data items such that: – Items can be removed only at one end (the front of the queue) – Items can be added only at the other end (the back of the queue)
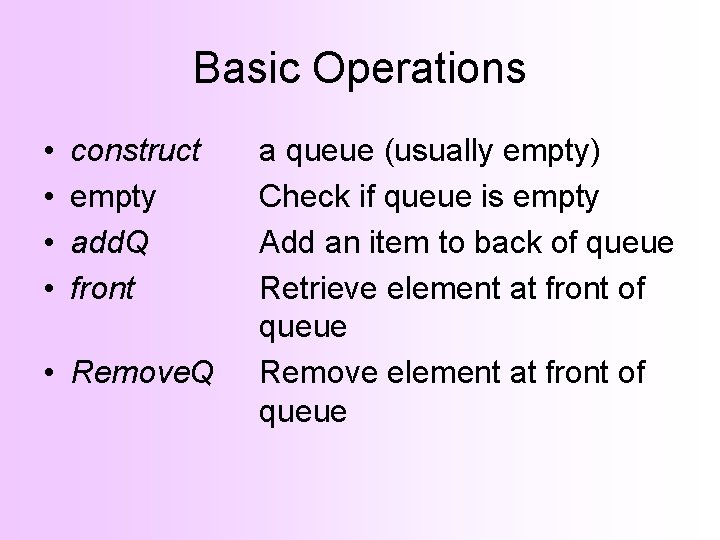
Basic Operations • • construct empty add. Q front • Remove. Q a queue (usually empty) Check if queue is empty Add an item to back of queue Retrieve element at front of queue Remove element at front of queue
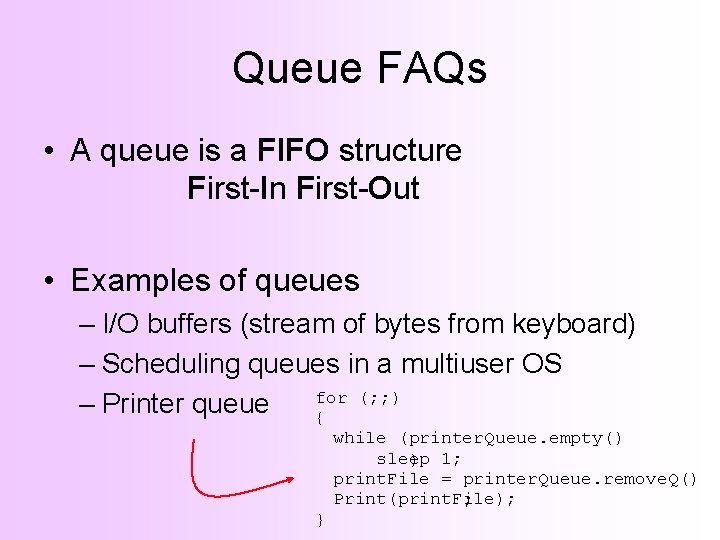
Queue FAQs • A queue is a FIFO structure First-In First-Out • Examples of queues – I/O buffers (stream of bytes from keyboard) – Scheduling queues in a multiuser OS for (; ; ) – Printer queue { } while (printer. Queue. empty() sleep ) 1; print. File = printer. Queue. remove. Q() Print(print. File); ;
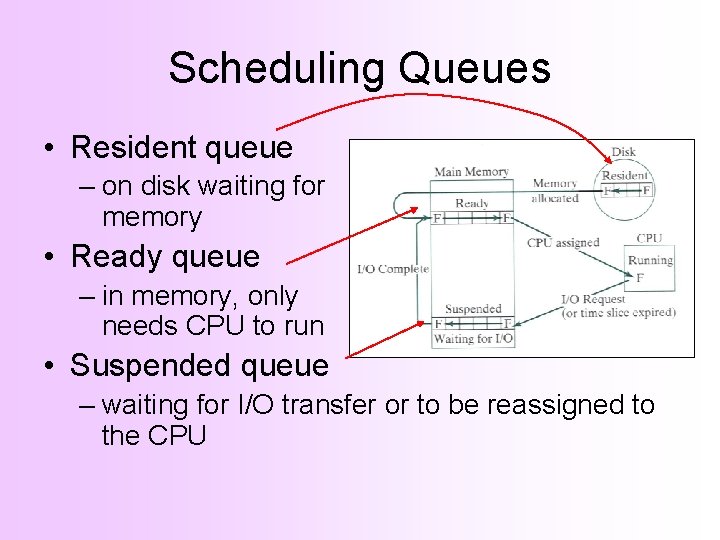
Scheduling Queues • Resident queue – on disk waiting for memory • Ready queue – in memory, only needs CPU to run • Suspended queue – waiting for I/O transfer or to be reassigned to the CPU
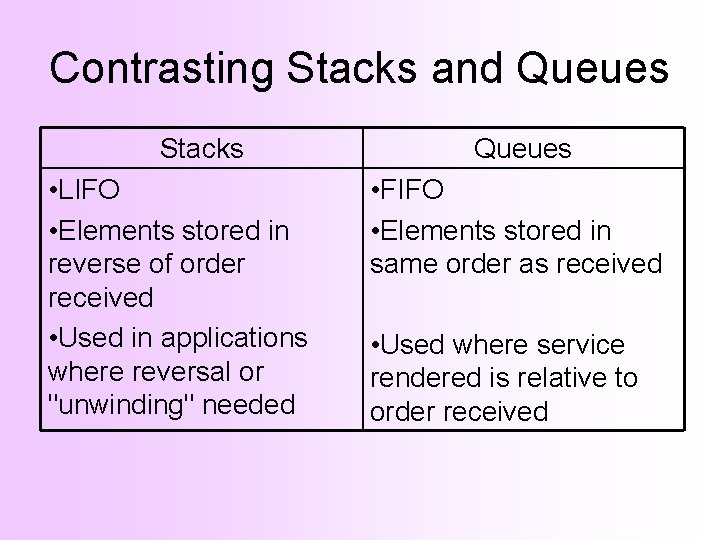
Contrasting Stacks and Queues Stacks • LIFO • Elements stored in reverse of order received • Used in applications where reversal or "unwinding" needed Queues • FIFO • Elements stored in same order as received • Used where service rendered is relative to order received
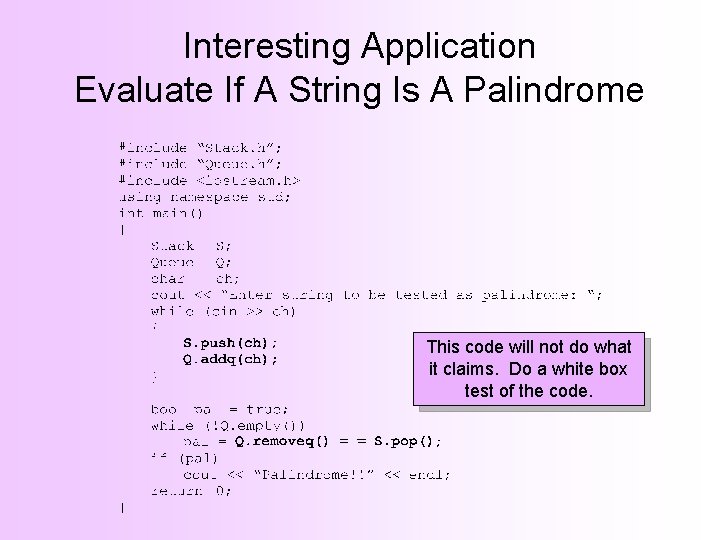
Interesting Application Evaluate If A String Is A Palindrome This code will not do what it claims. Do a white box test of the code.
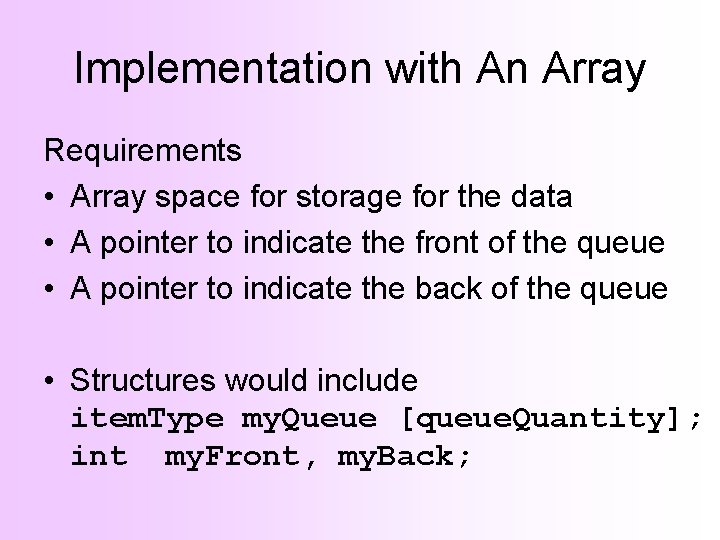
Implementation with An Array Requirements • Array space for storage for the data • A pointer to indicate the front of the queue • A pointer to indicate the back of the queue • Structures would include item. Type my. Queue [queue. Quantity]; int my. Front, my. Back;
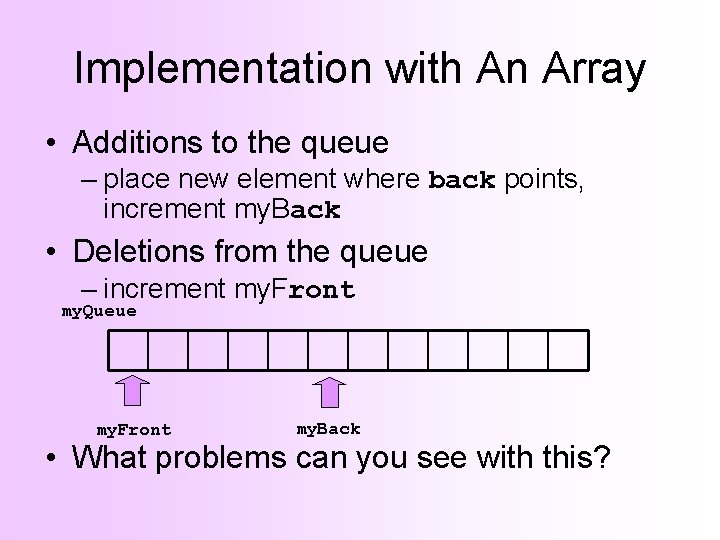
Implementation with An Array • Additions to the queue – place new element where back points, increment my. Back • Deletions from the queue – increment my. Front my. Queue my. Front my. Back • What problems can you see with this?
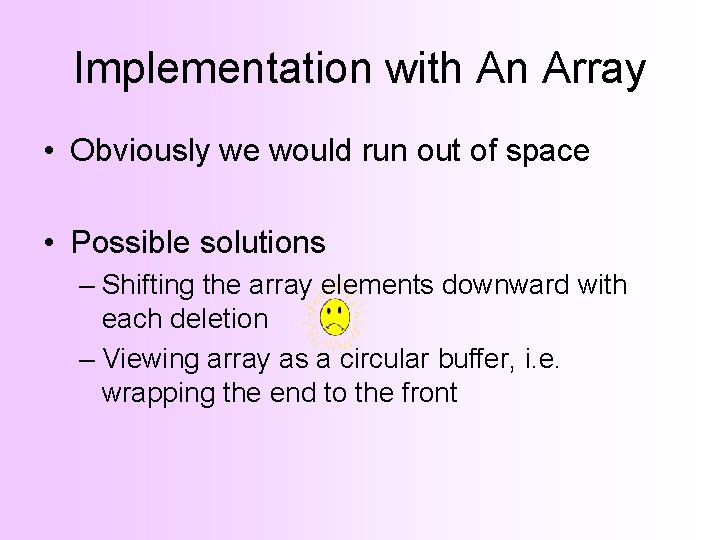
Implementation with An Array • Obviously we would run out of space • Possible solutions – Shifting the array elements downward with each deletion – Viewing array as a circular buffer, i. e. wrapping the end to the front
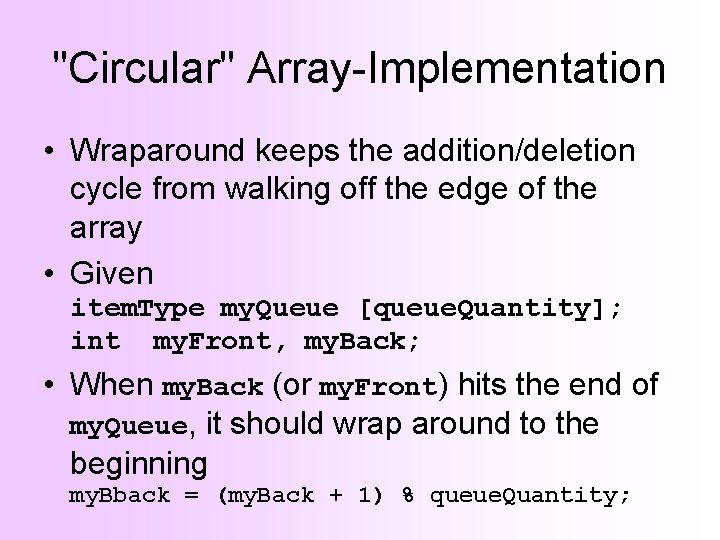
"Circular" Array-Implementation • Wraparound keeps the addition/deletion cycle from walking off the edge of the array • Given item. Type my. Queue [queue. Quantity]; int my. Front, my. Back; • When my. Back (or my. Front) hits the end of my. Queue, it should wrap around to the beginning my. Bback = (my. Back + 1) % queue. Quantity;
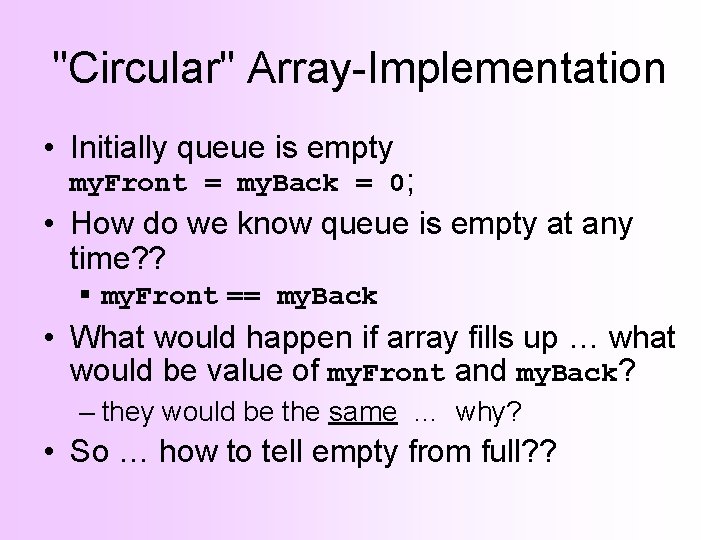
"Circular" Array-Implementation • Initially queue is empty my. Front = my. Back = 0; • How do we know queue is empty at any time? ? § my. Front == my. Back • What would happen if array fills up … what would be value of my. Front and my. Back? – they would be the same … why? • So … how to tell empty from full? ?
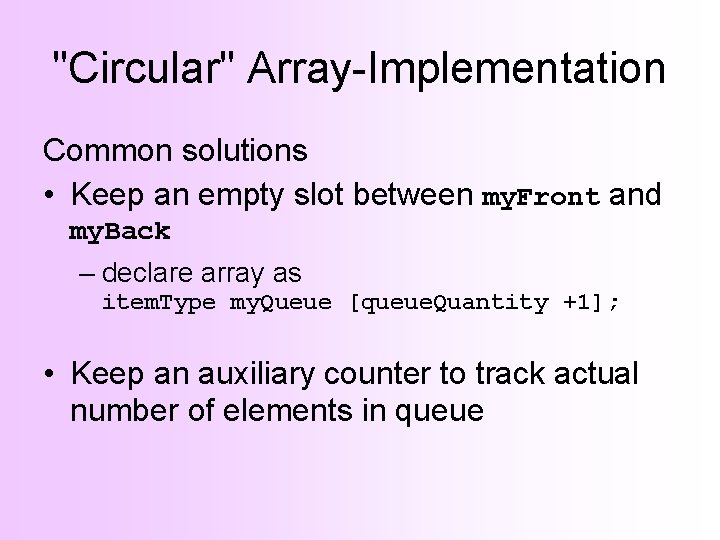
"Circular" Array-Implementation Common solutions • Keep an empty slot between my. Front and my. Back – declare array as item. Type my. Queue [queue. Quantity +1]; • Keep an auxiliary counter to track actual number of elements in queue
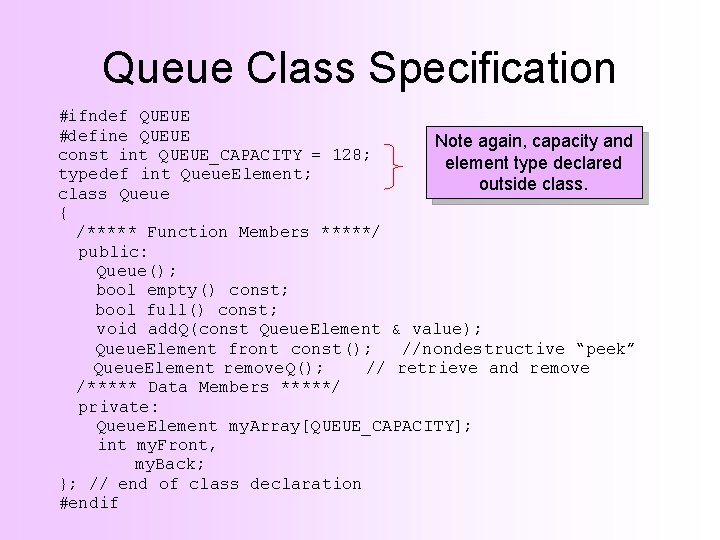
Queue Class Specification #ifndef QUEUE #define QUEUE Note again, capacity and const int QUEUE_CAPACITY = 128; element type declared typedef int Queue. Element; outside class Queue { /***** Function Members *****/ public: Queue(); bool empty() const; bool full() const; void add. Q(const Queue. Element & value); Queue. Element front const(); //nondestructive “peek” Queue. Element remove. Q(); // retrieve and remove /***** Data Members *****/ private: Queue. Element my. Array[QUEUE_CAPACITY]; int my. Front, my. Back; }; // end of class declaration #endif
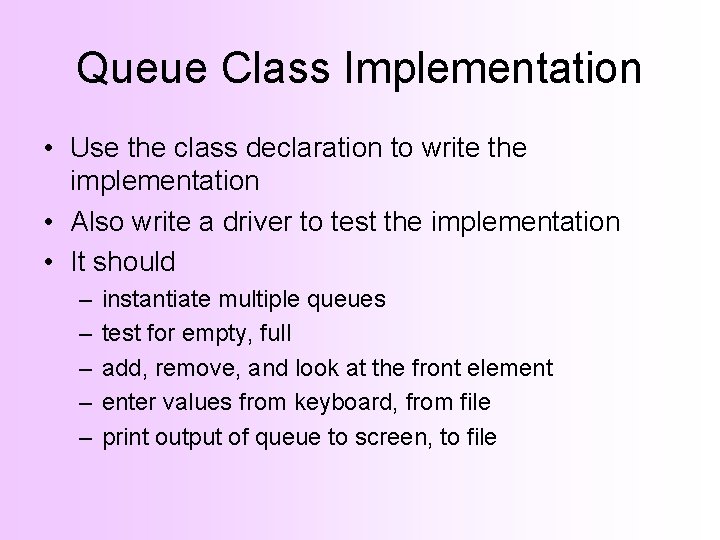
Queue Class Implementation • Use the class declaration to write the implementation • Also write a driver to test the implementation • It should – – – instantiate multiple queues test for empty, full add, remove, and look at the front element enter values from keyboard, from file print output of queue to screen, to file
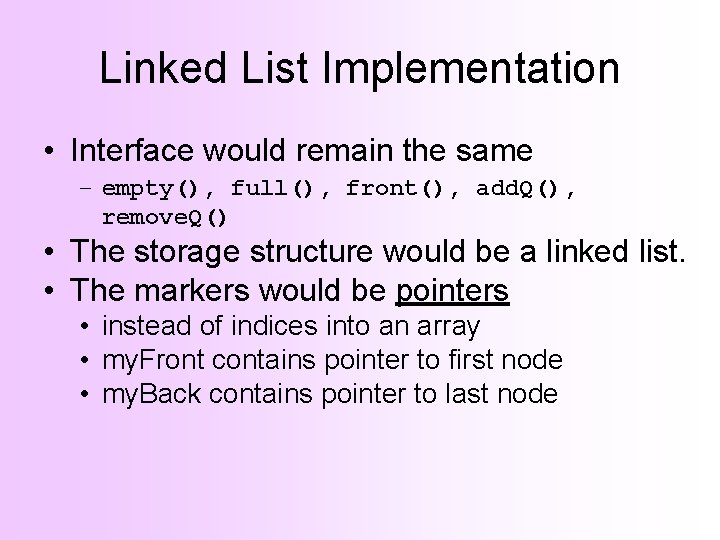
Linked List Implementation • Interface would remain the same – empty(), full(), front(), add. Q(), remove. Q() • The storage structure would be a linked list. • The markers would be pointers • instead of indices into an array • my. Front contains pointer to first node • my. Back contains pointer to last node
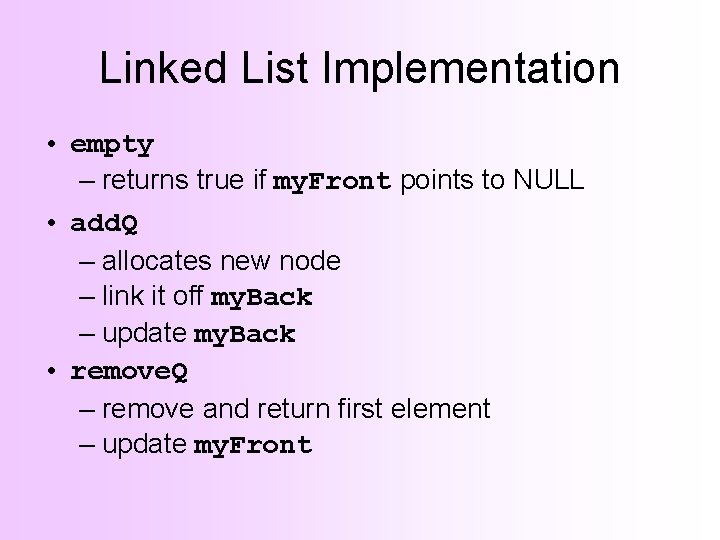
Linked List Implementation • empty – returns true if my. Front points to NULL • add. Q – allocates new node – link it off my. Back – update my. Back • remove. Q – remove and return first element – update my. Front
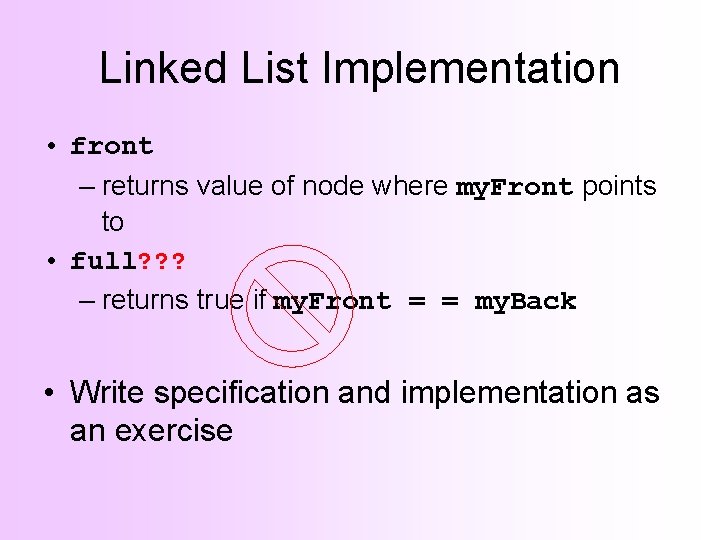
Linked List Implementation • front – returns value of node where my. Front points to • full? ? ? – returns true if my. Front = = my. Back • Write specification and implementation as an exercise
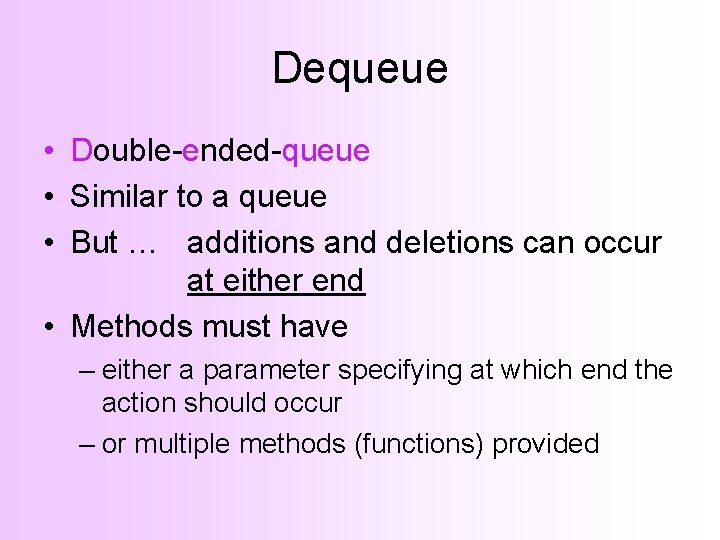
Dequeue • Double-ended-queue • Similar to a queue • But … additions and deletions can occur at either end • Methods must have – either a parameter specifying at which end the action should occur – or multiple methods (functions) provided
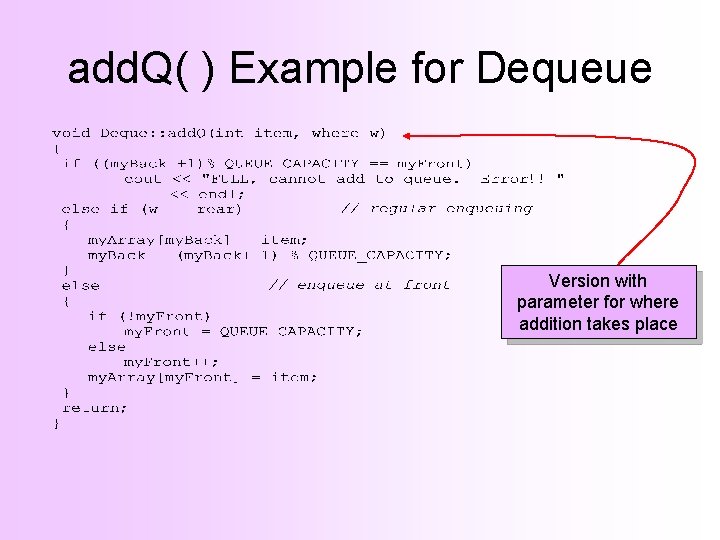
add. Q( ) Example for Dequeue Version with parameter for where addition takes place
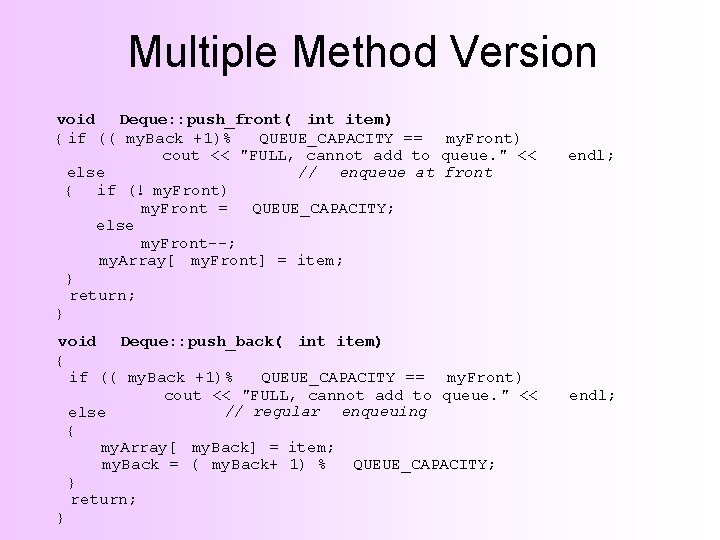
Multiple Method Version void Deque: : push_front( int item) { if (( my. Back +1)% QUEUE_CAPACITY == my. Front) cout << "FULL, cannot add to queue. " << // enqueue at front else { if (! my. Front) my. Front = QUEUE_CAPACITY; else my. Front--; my. Array[ my. Front] = item; } return; } void Deque: : push_back( int item) { if (( my. Back +1)% QUEUE_CAPACITY == my. Front) cout << "FULL, cannot add to queue. " << // regular enqueuing else { my. Array[ my. Back] = item; my. Back = ( my. Back+ 1) % QUEUE_CAPACITY; } return; } endl;
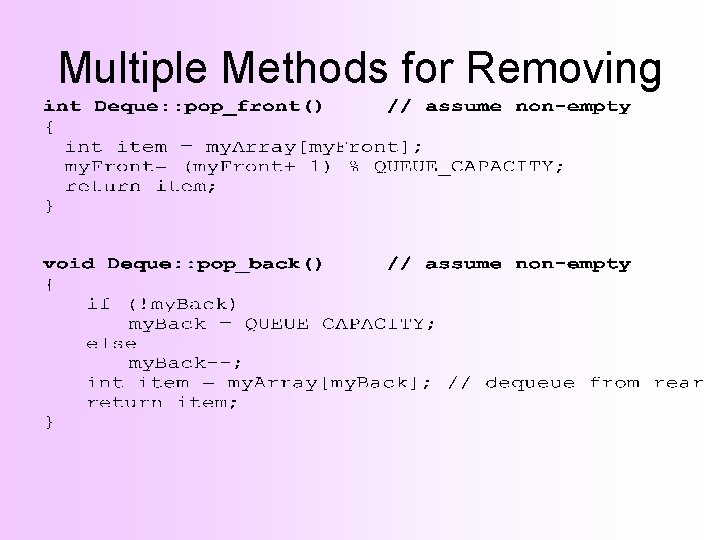
Multiple Methods for Removing
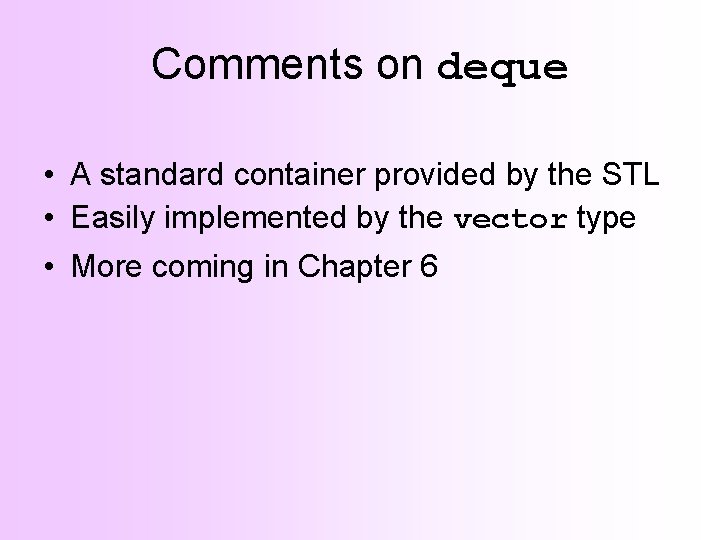
Comments on deque • A standard container provided by the STL • Easily implemented by the vector type • More coming in Chapter 6
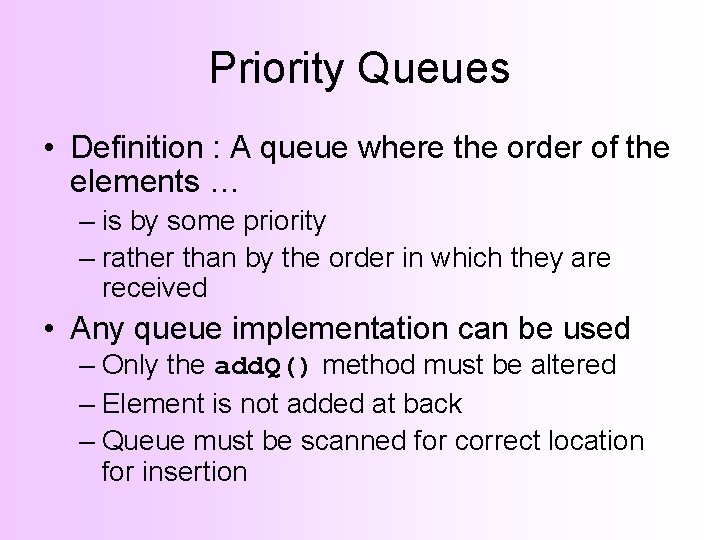
Priority Queues • Definition : A queue where the order of the elements … – is by some priority – rather than by the order in which they are received • Any queue implementation can be used – Only the add. Q() method must be altered – Element is not added at back – Queue must be scanned for correct location for insertion
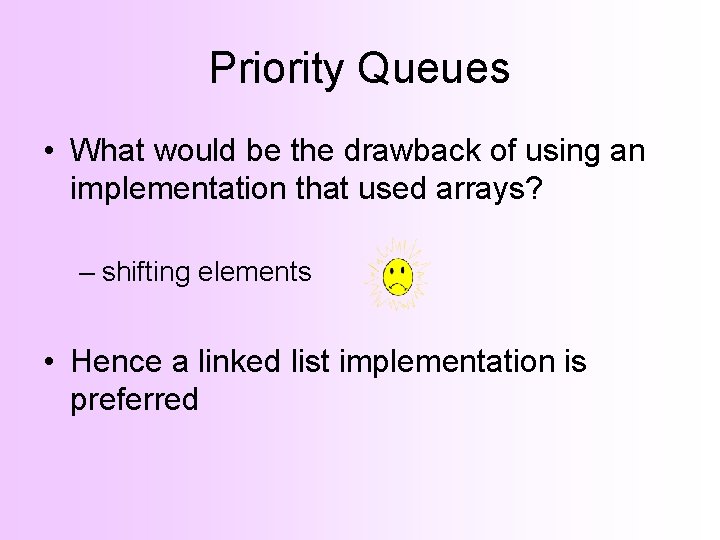
Priority Queues • What would be the drawback of using an implementation that used arrays? – shifting elements • Hence a linked list implementation is preferred
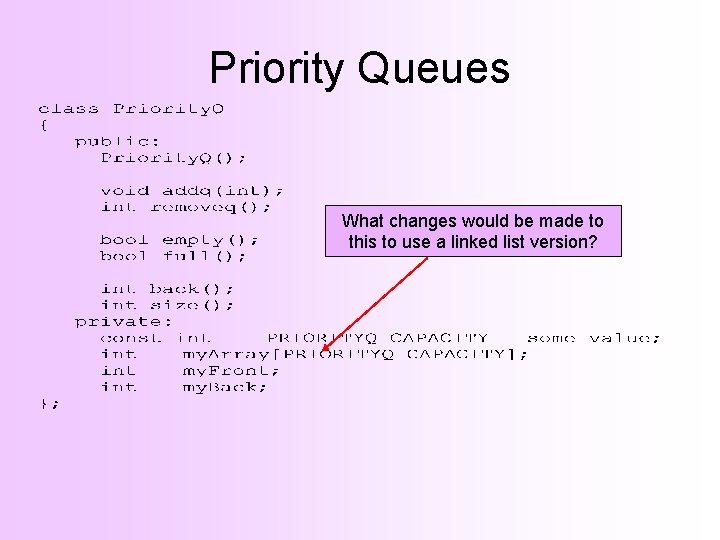
Priority Queues What changes would be made to this to use a linked list version?