Chapter 7 Queues Chien Chin Chen Department of
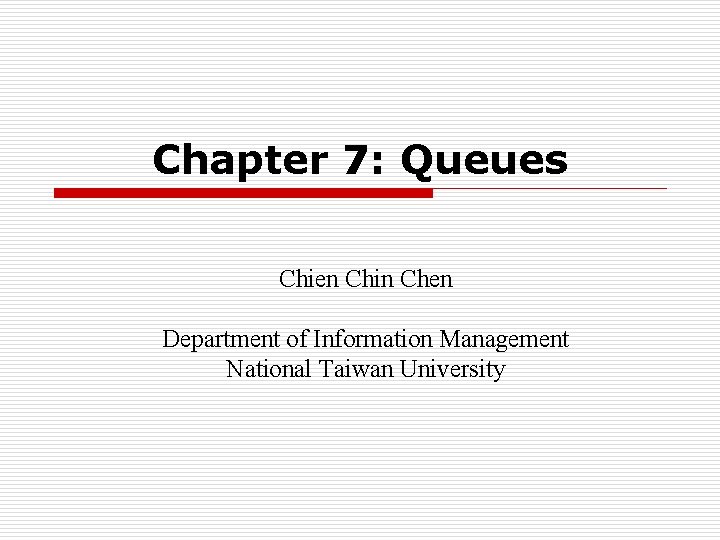
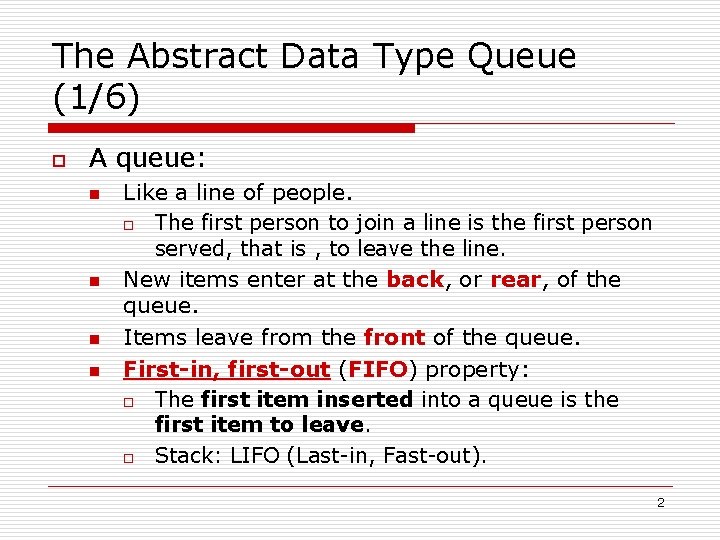
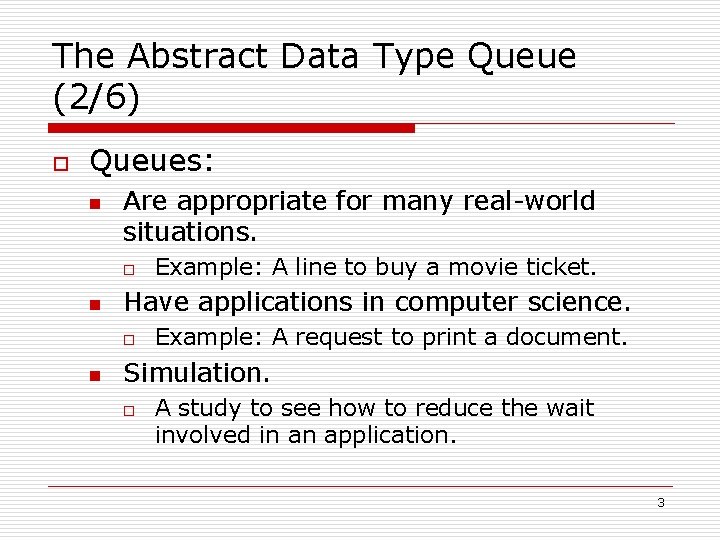
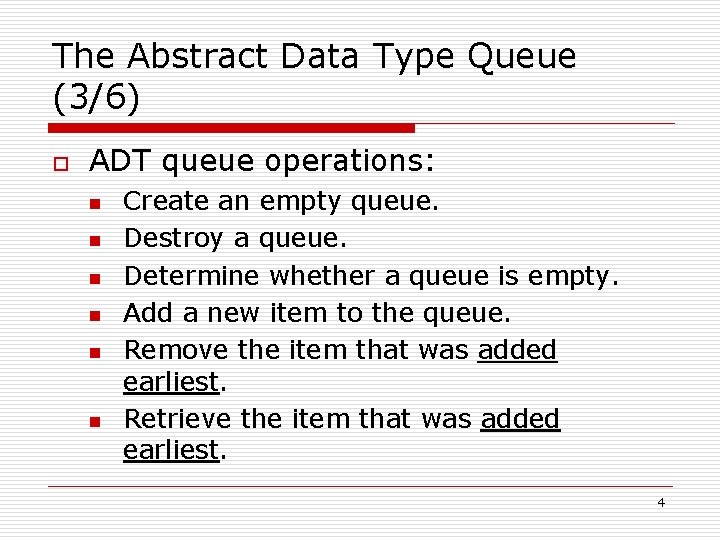
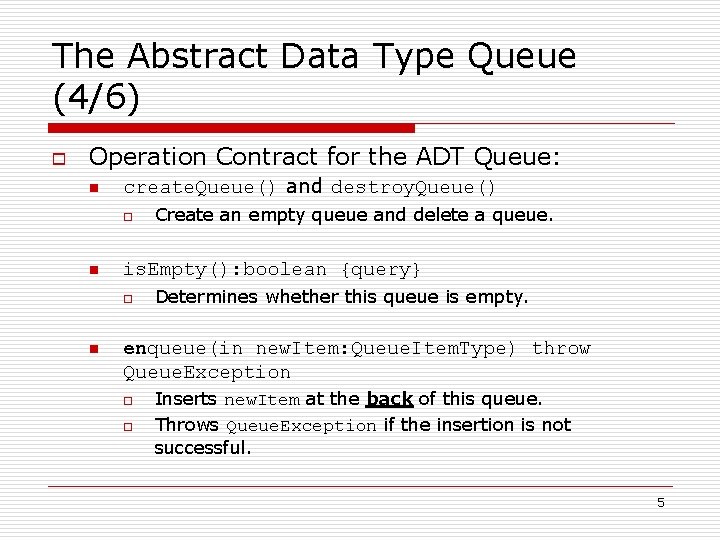
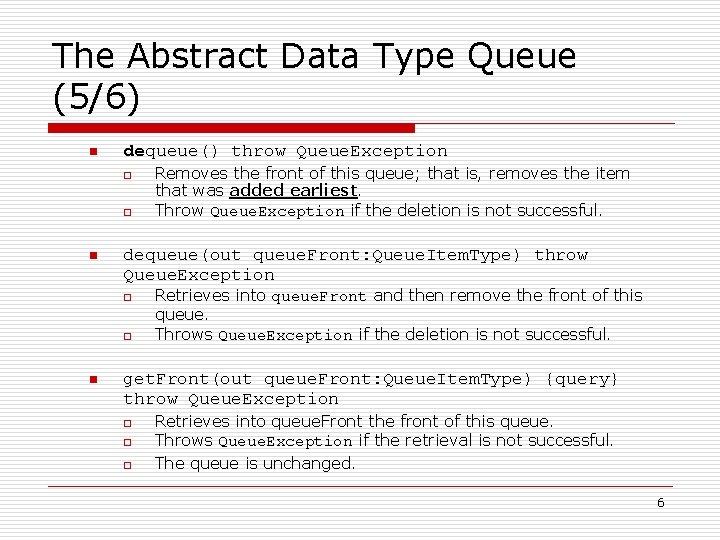
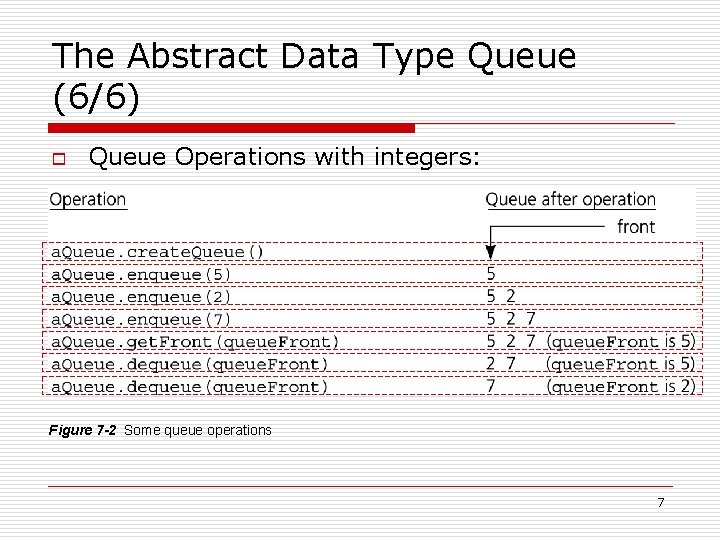
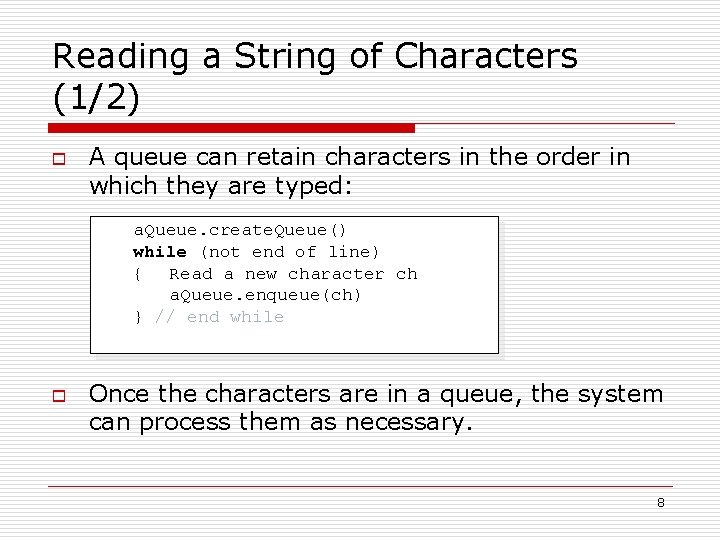
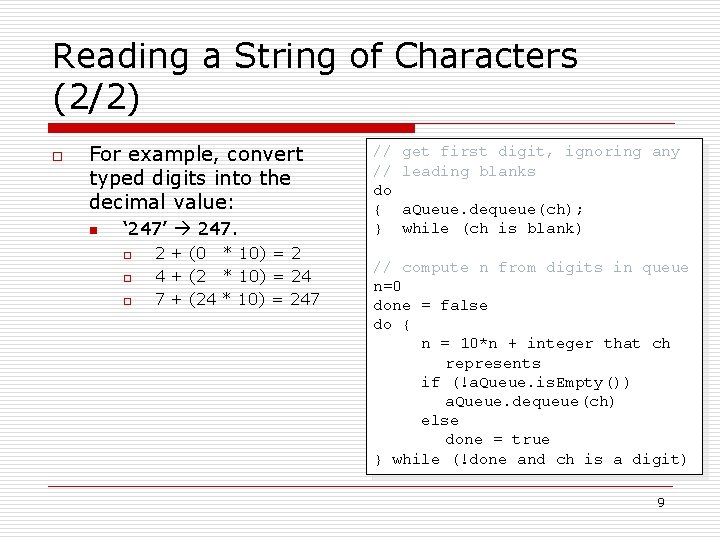
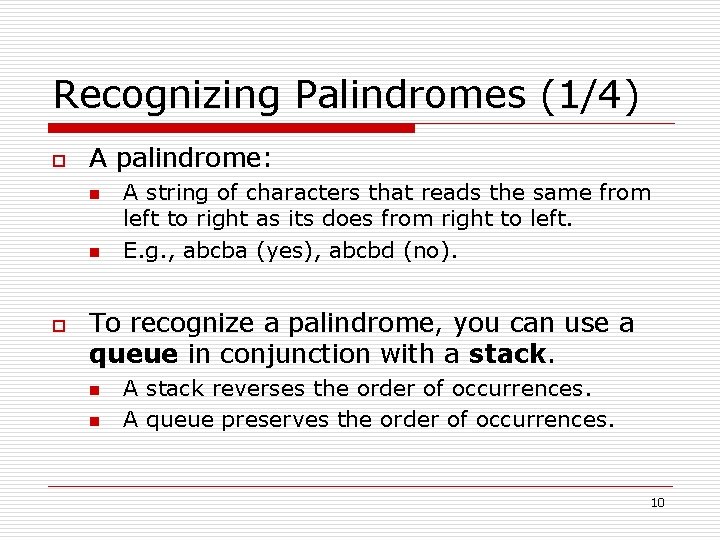
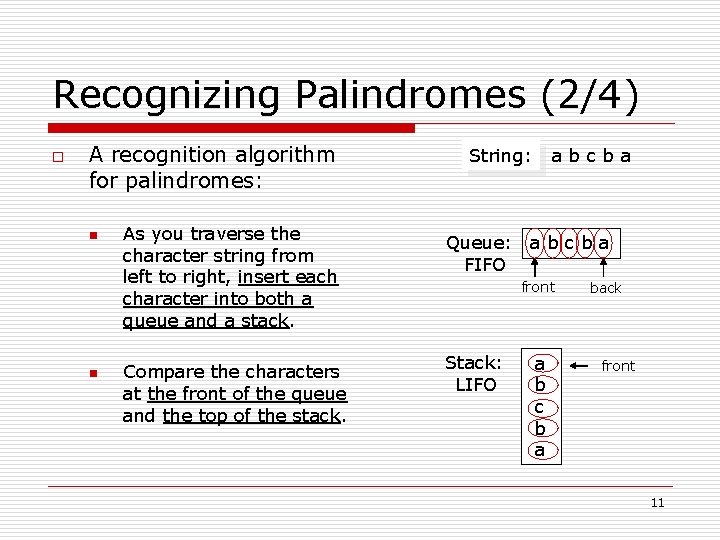
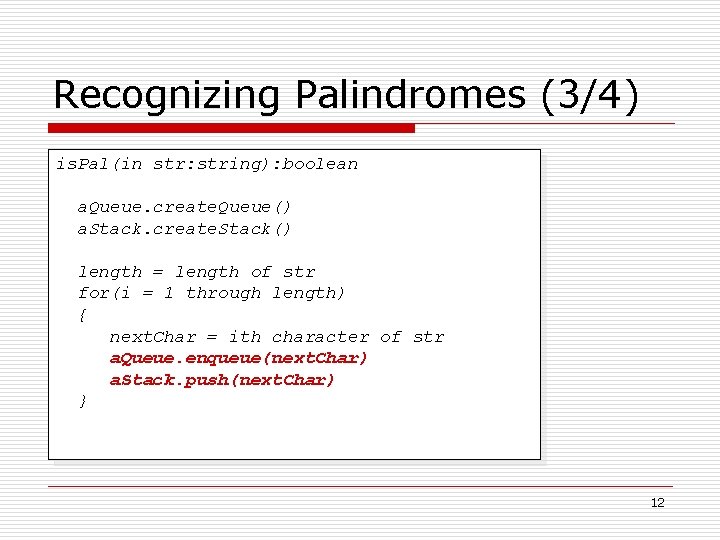
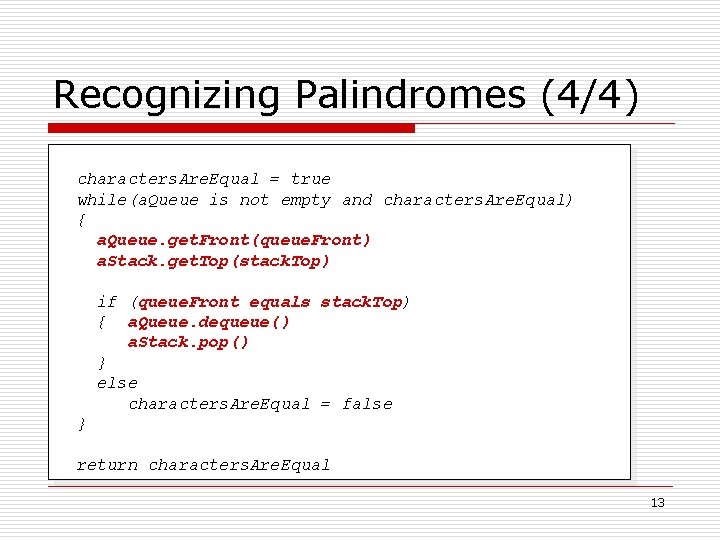
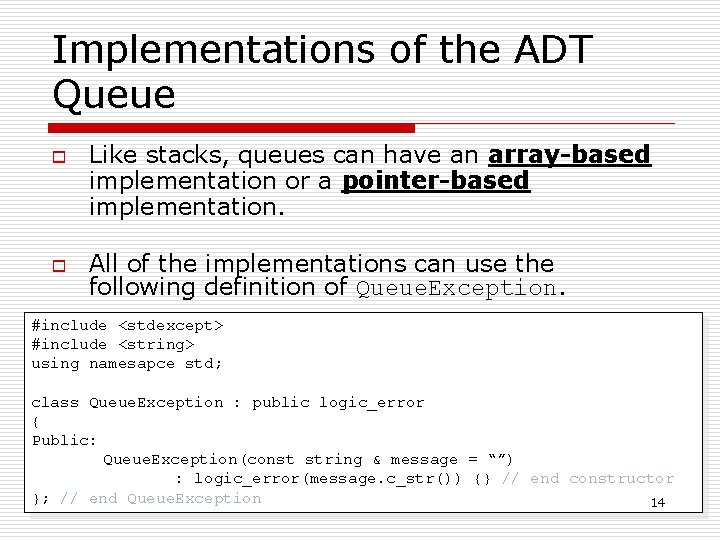
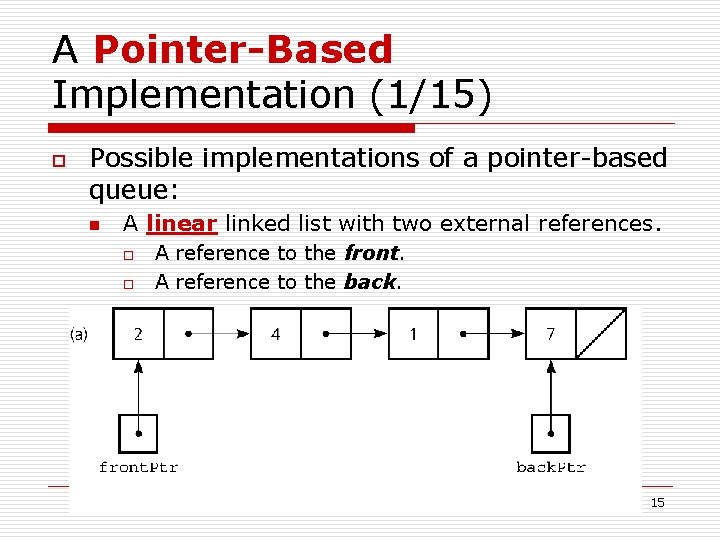
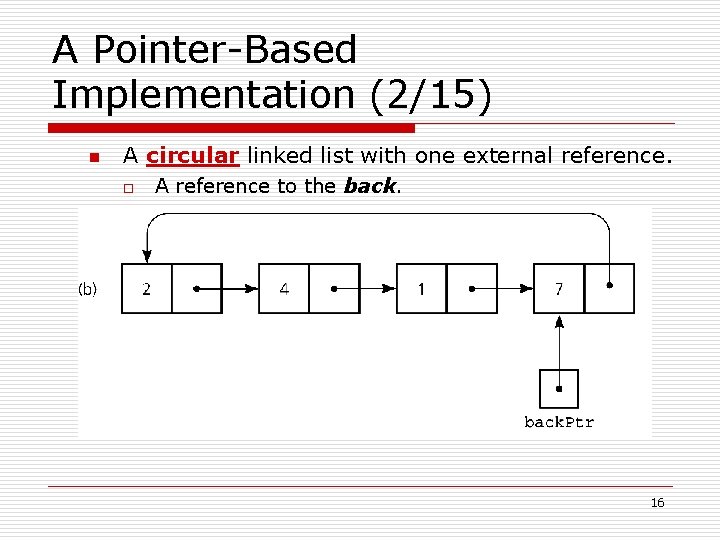
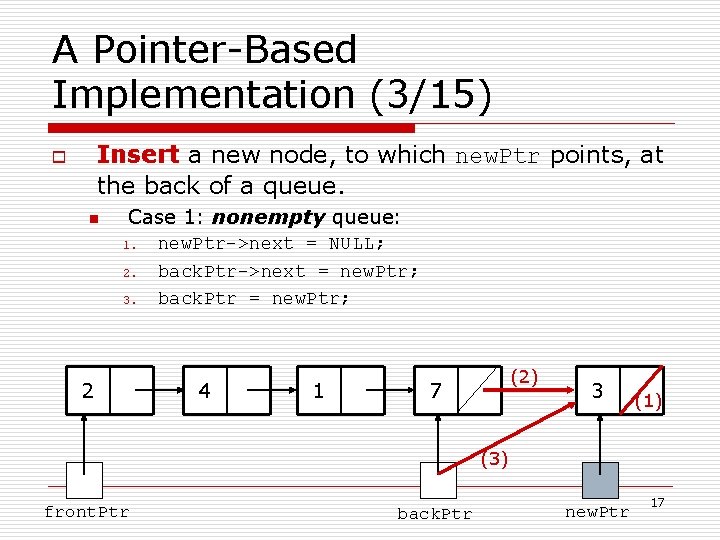
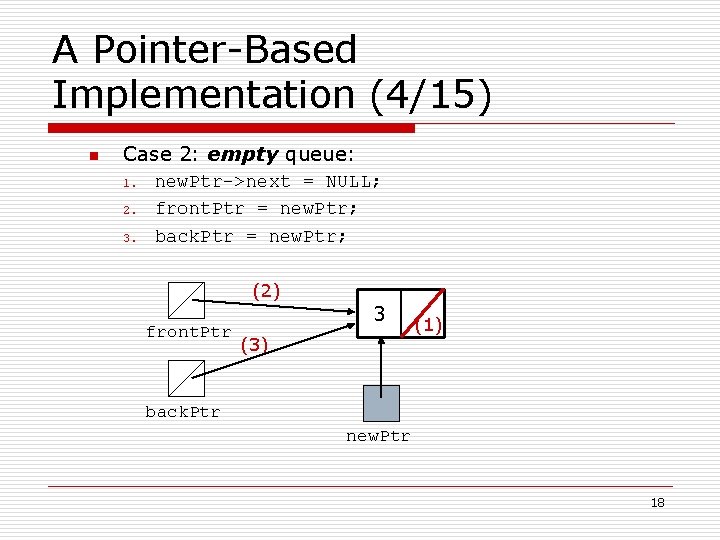
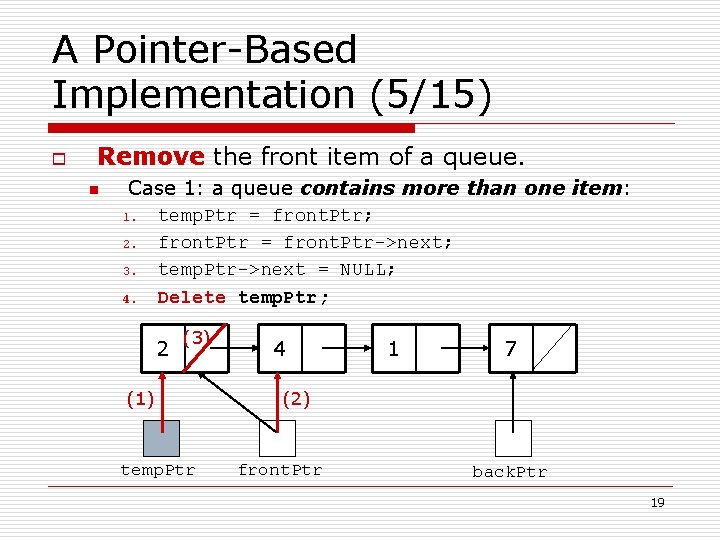
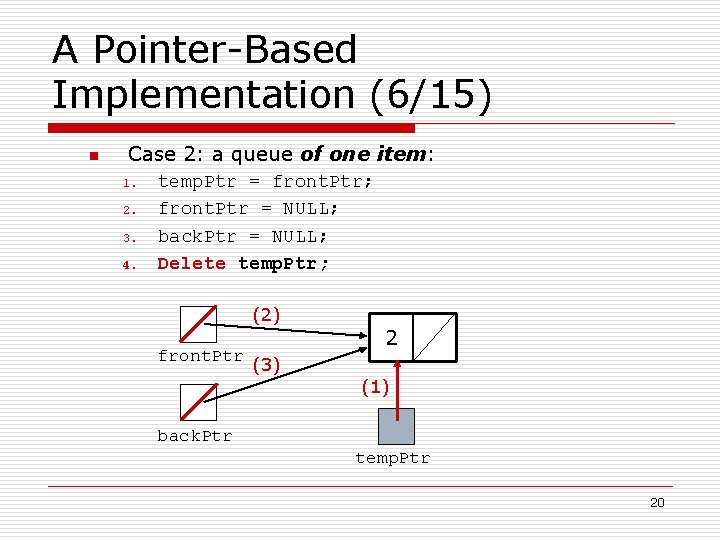
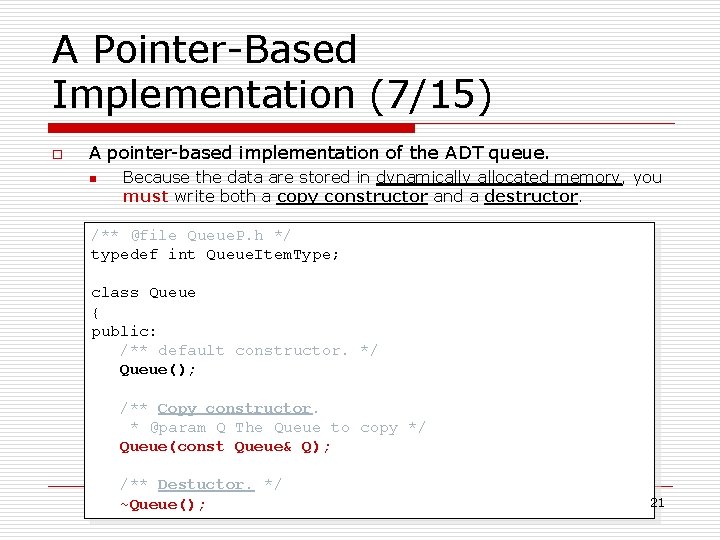
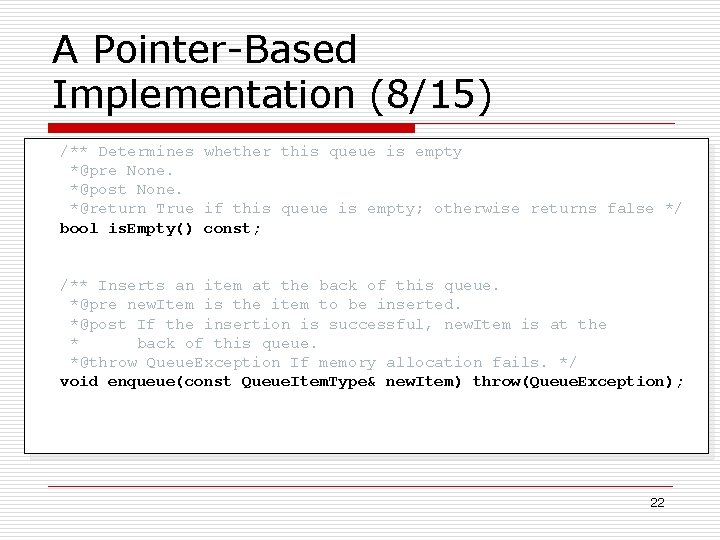
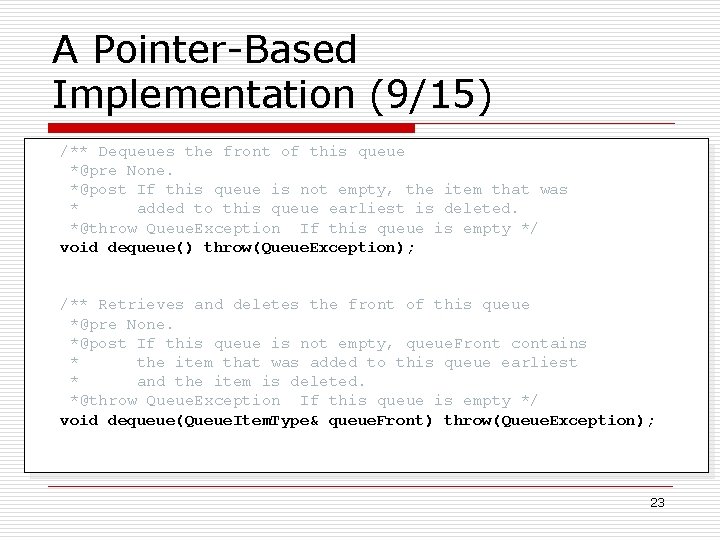
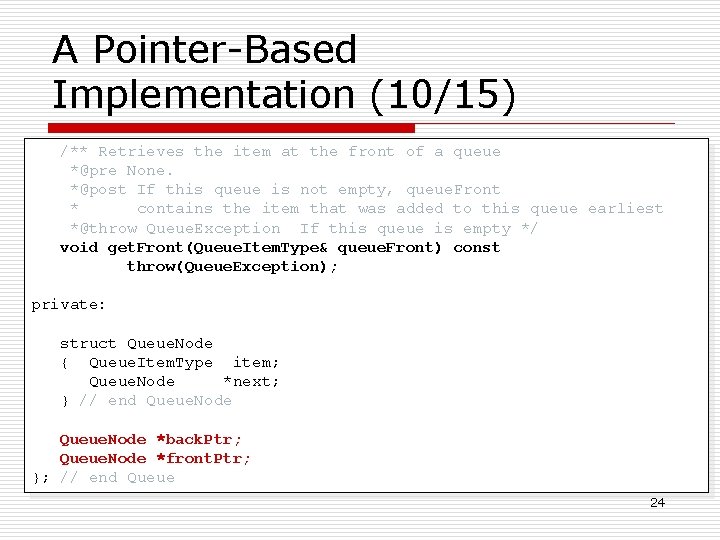
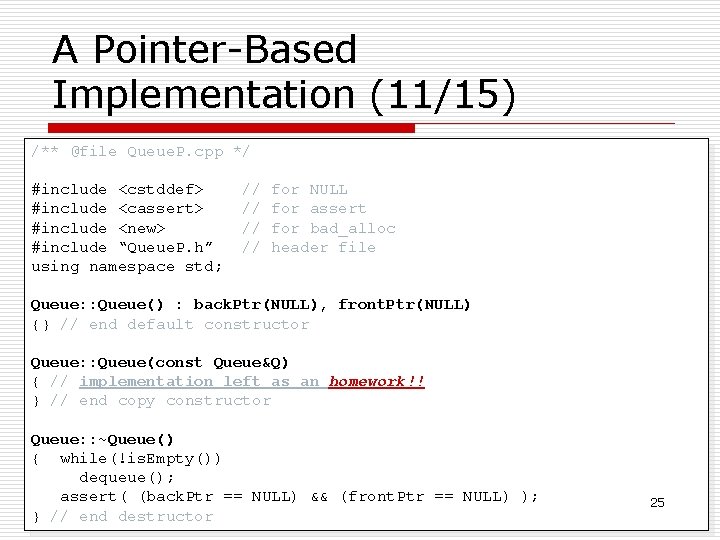
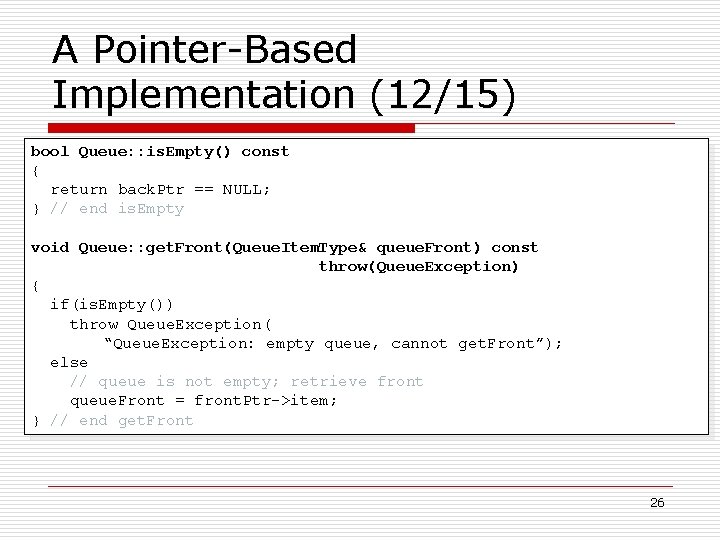
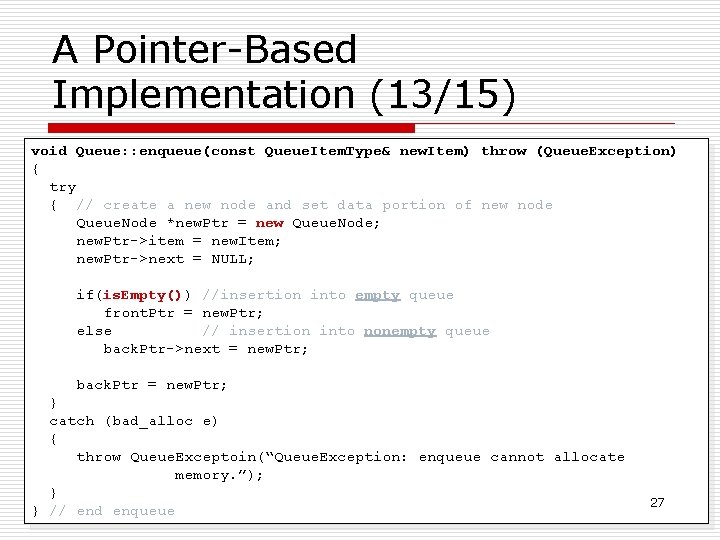
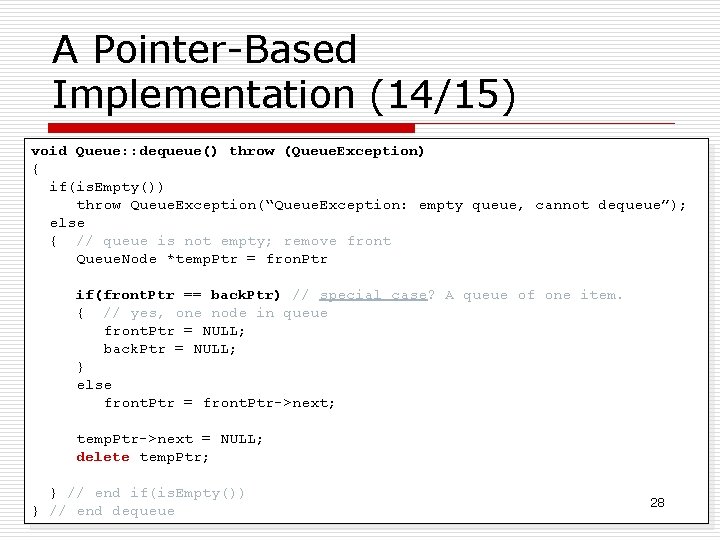
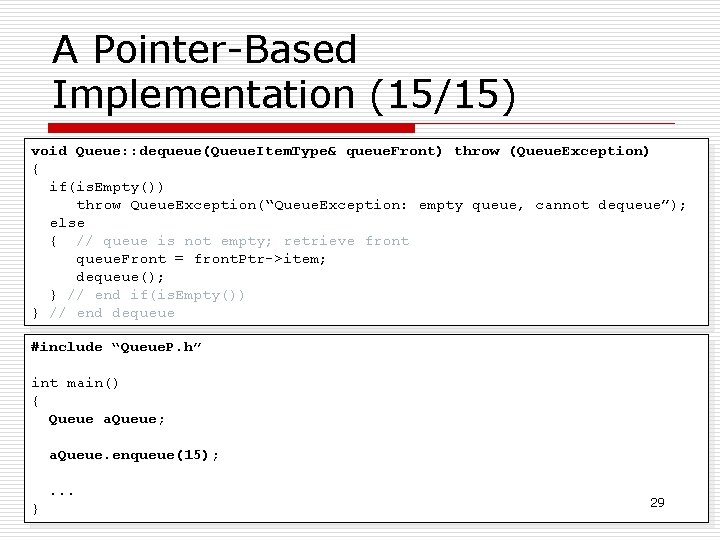
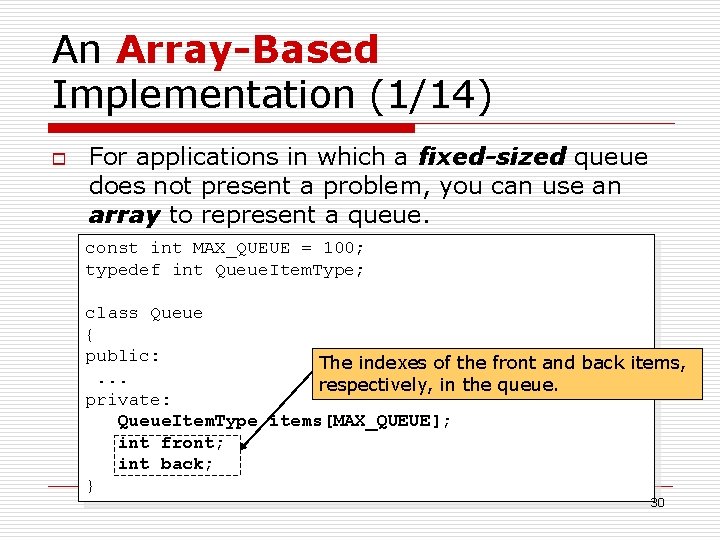
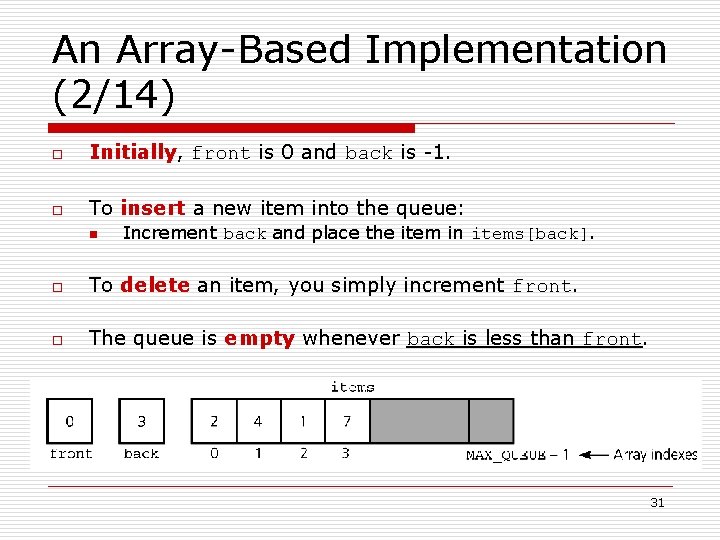
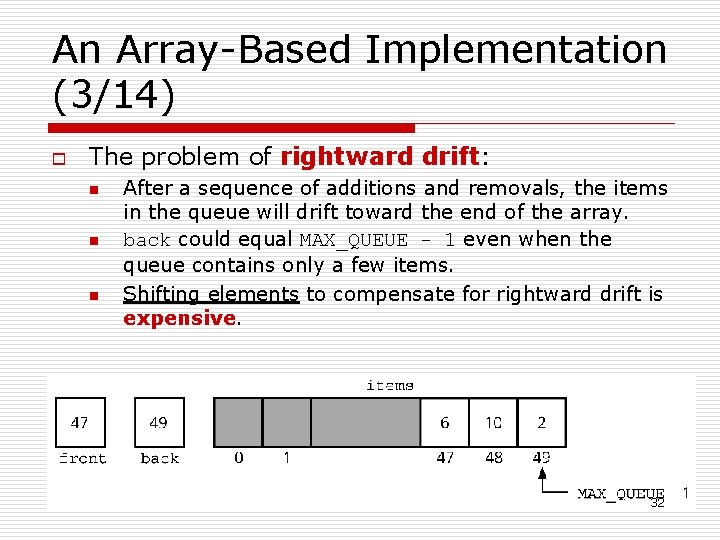
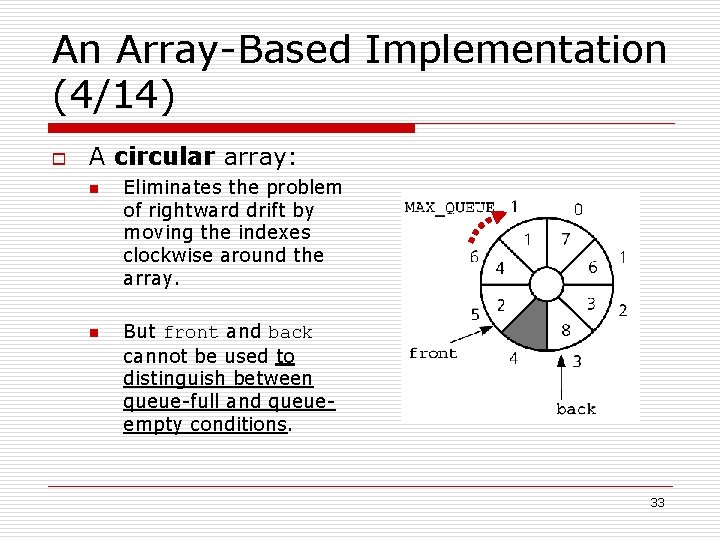
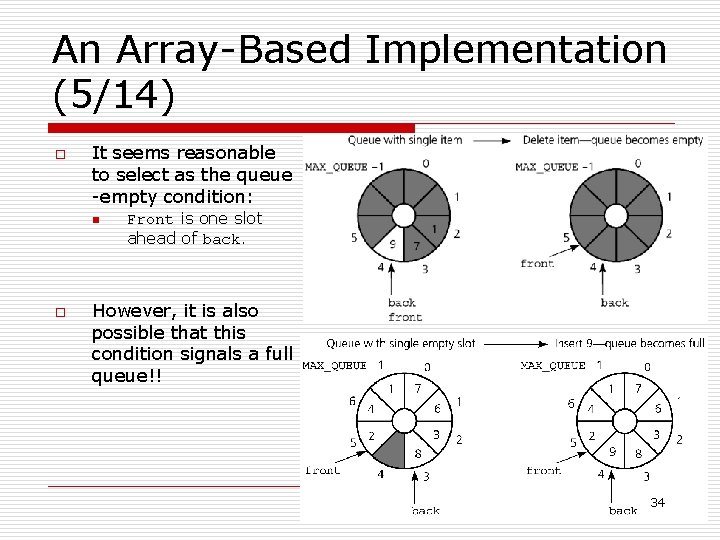
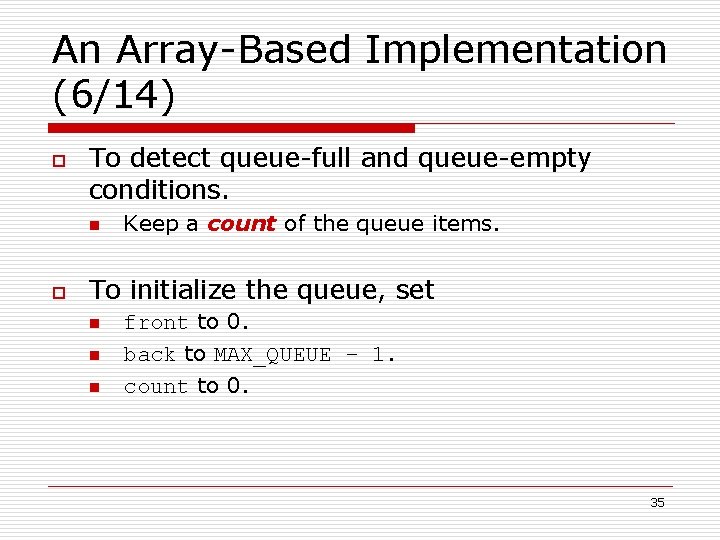
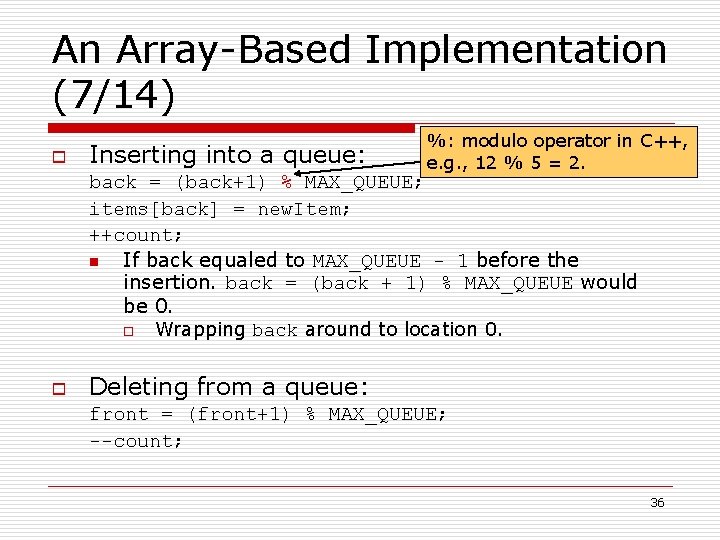
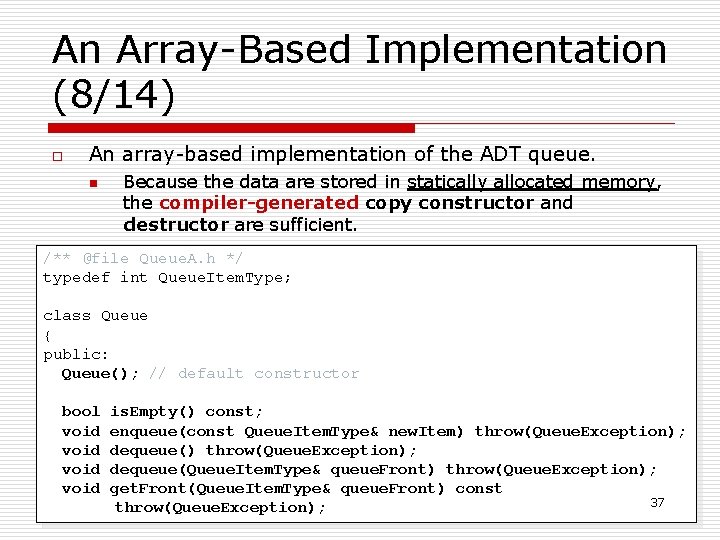
![An Array-Based Implementation (9/14) private: Queue. Item. Type items[MAX_QUEUE]; int front; int back; int An Array-Based Implementation (9/14) private: Queue. Item. Type items[MAX_QUEUE]; int front; int back; int](https://slidetodoc.com/presentation_image/afe928bd2f7d8e4fe93626d61bb9298c/image-38.jpg)
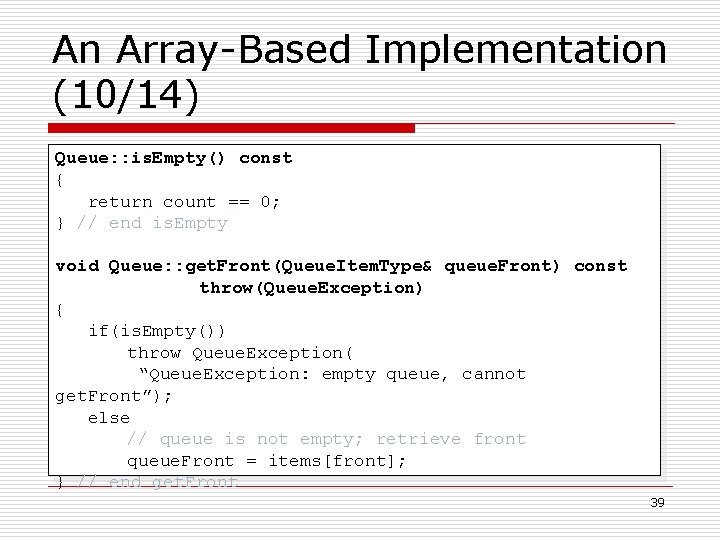
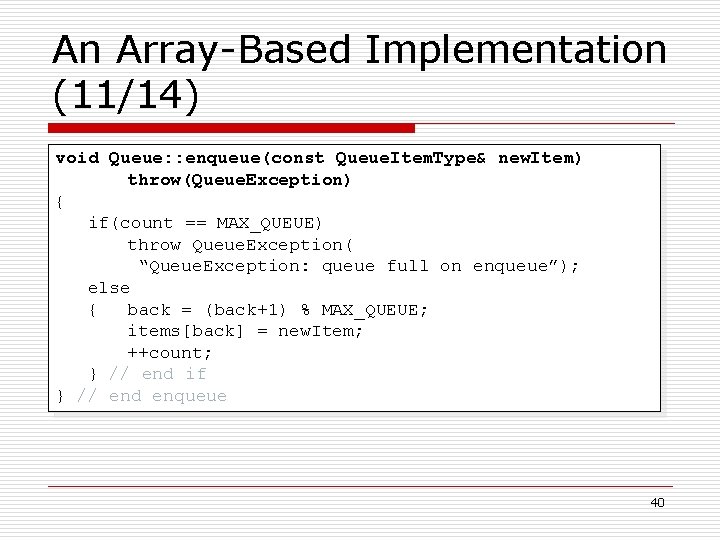
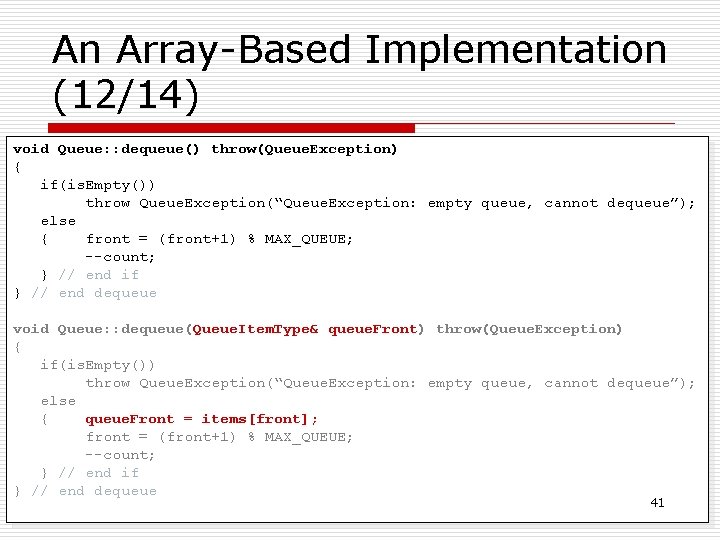
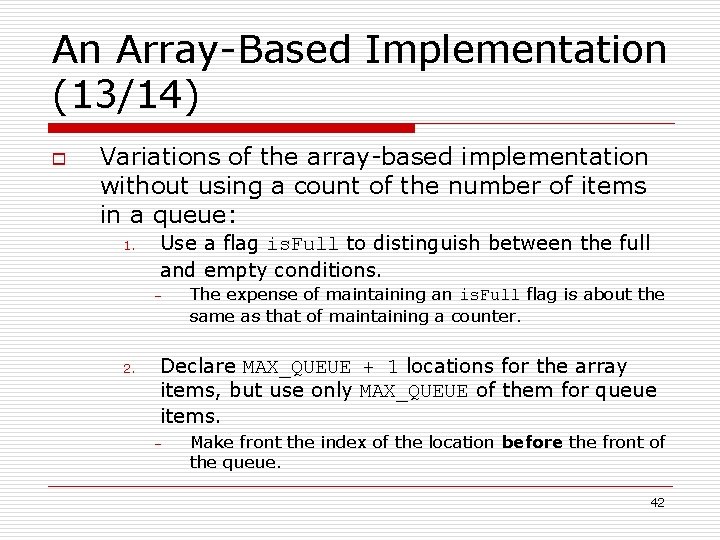
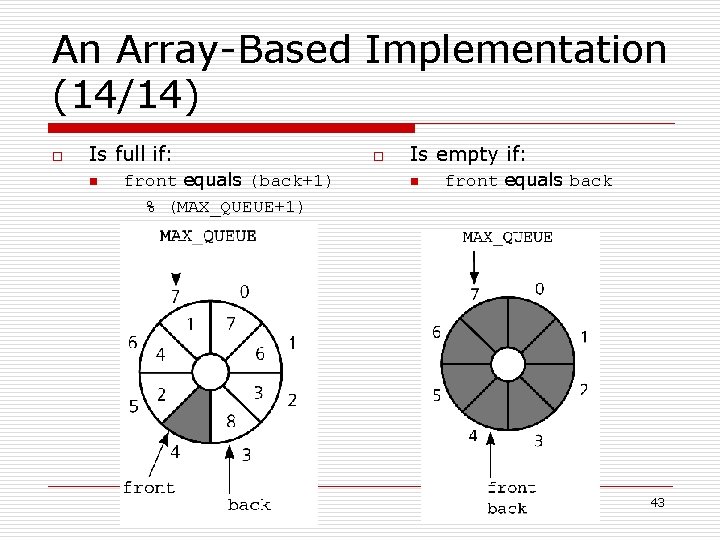
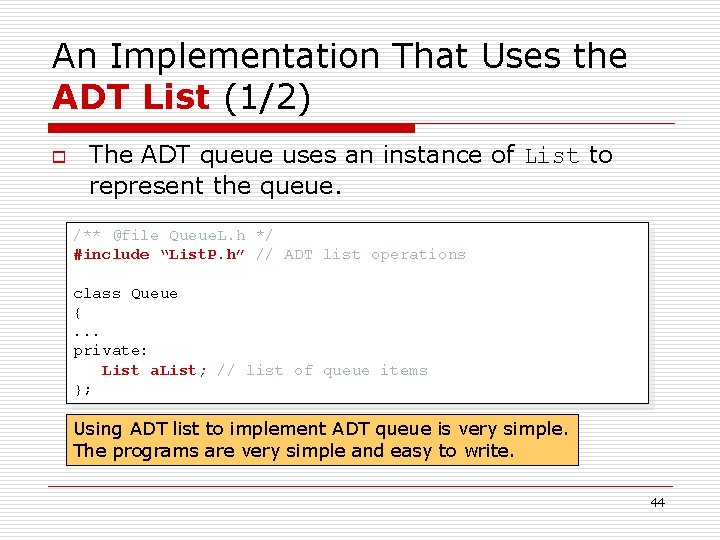
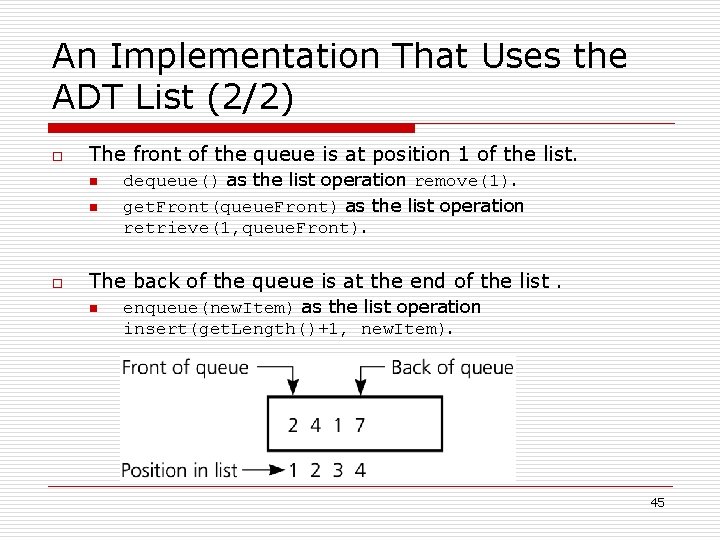
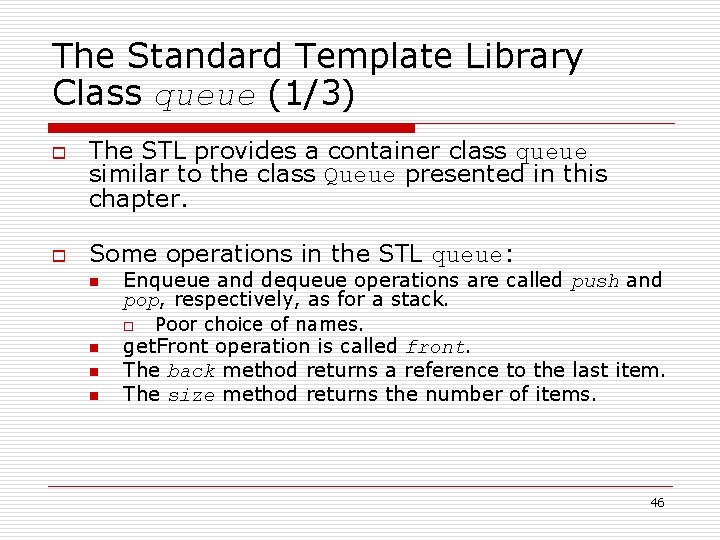
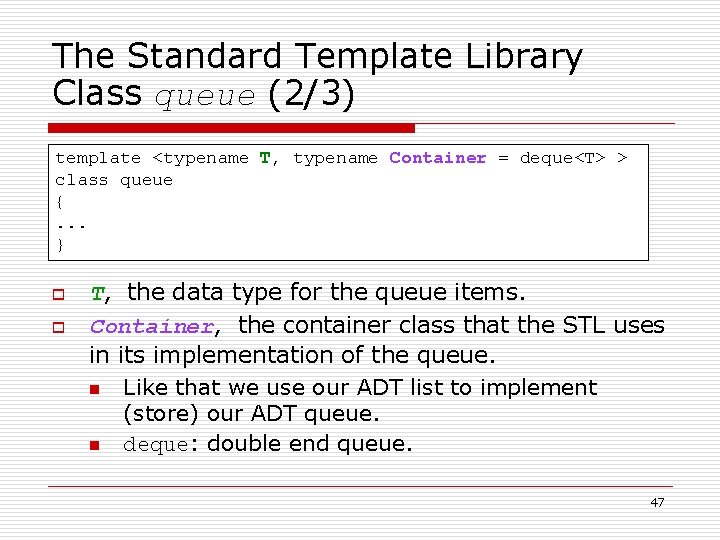
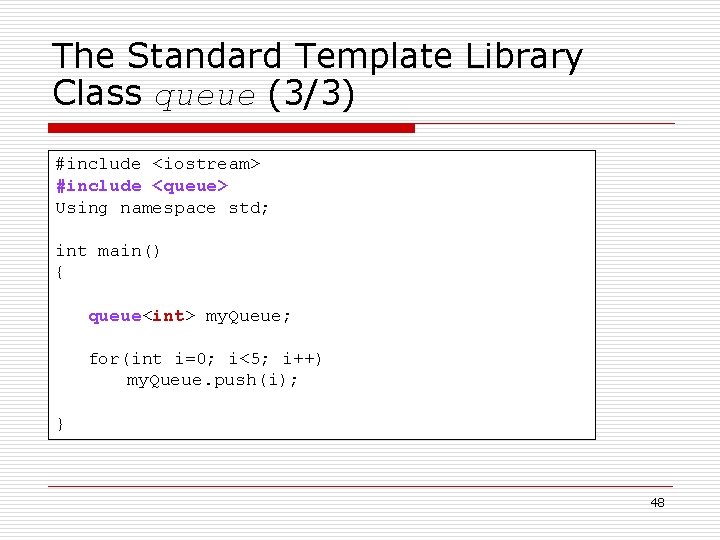
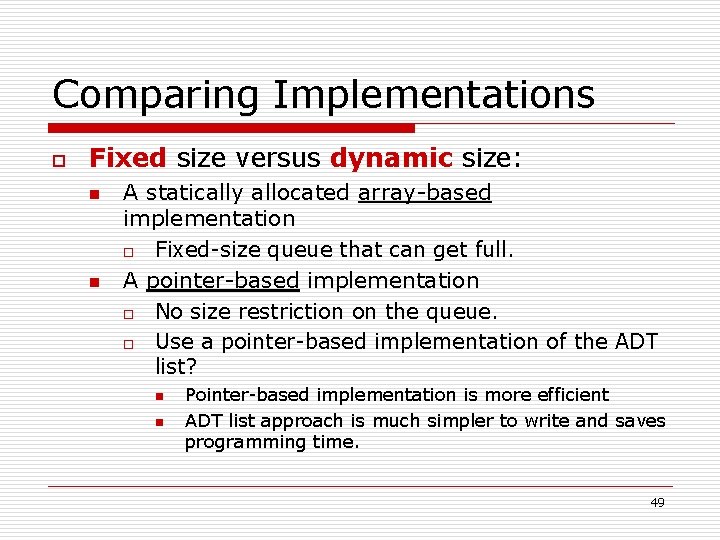
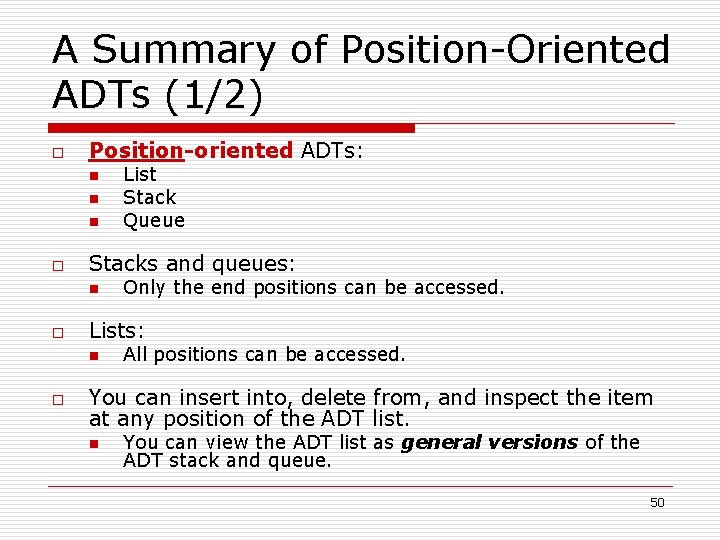
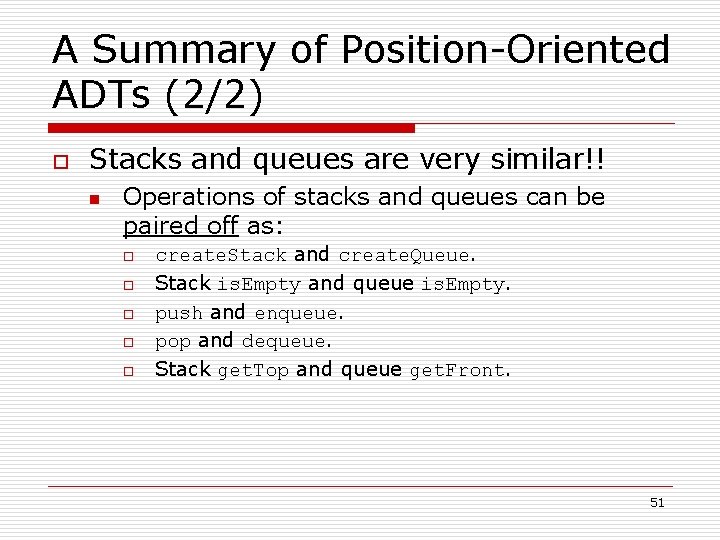
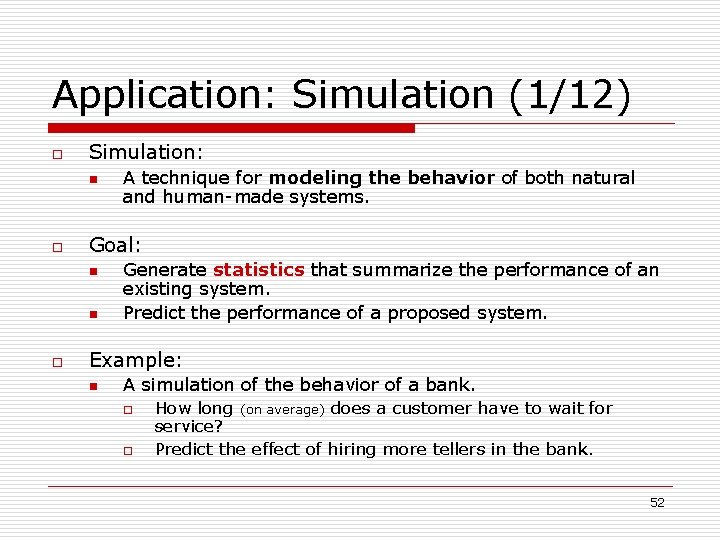
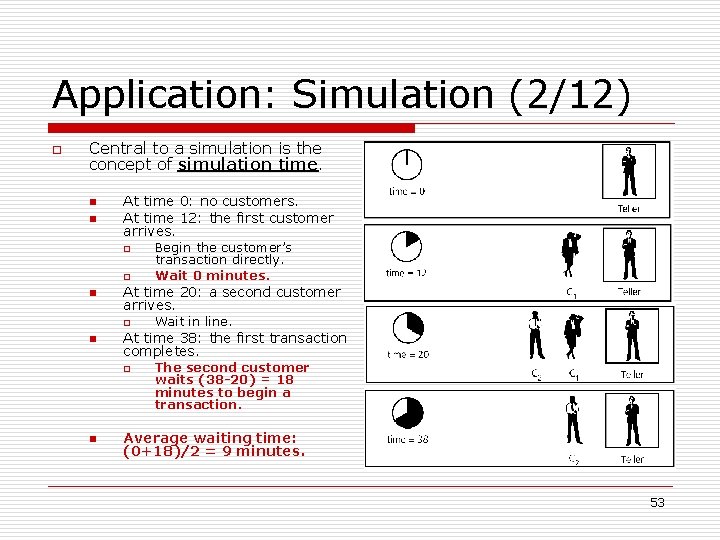
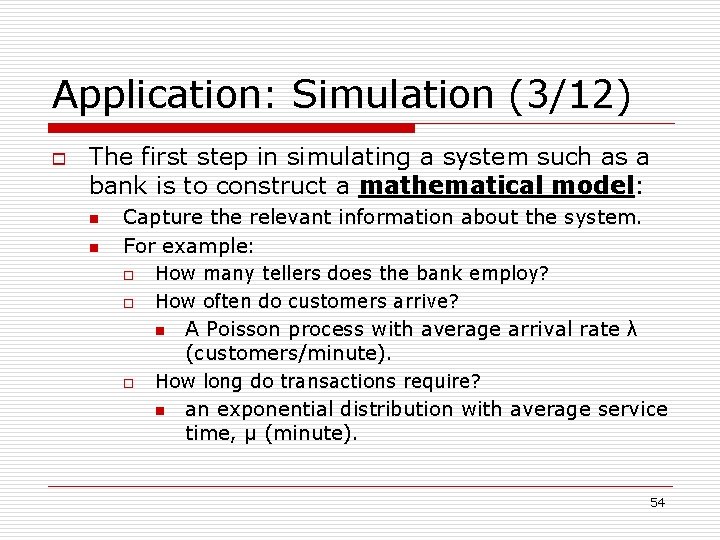
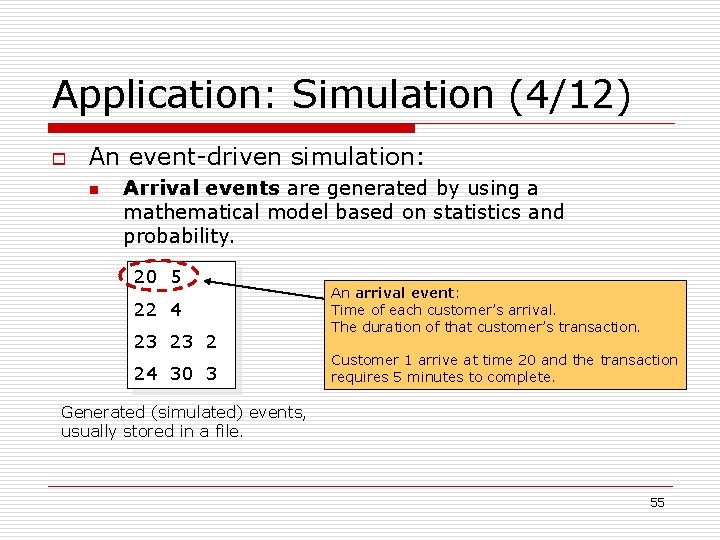
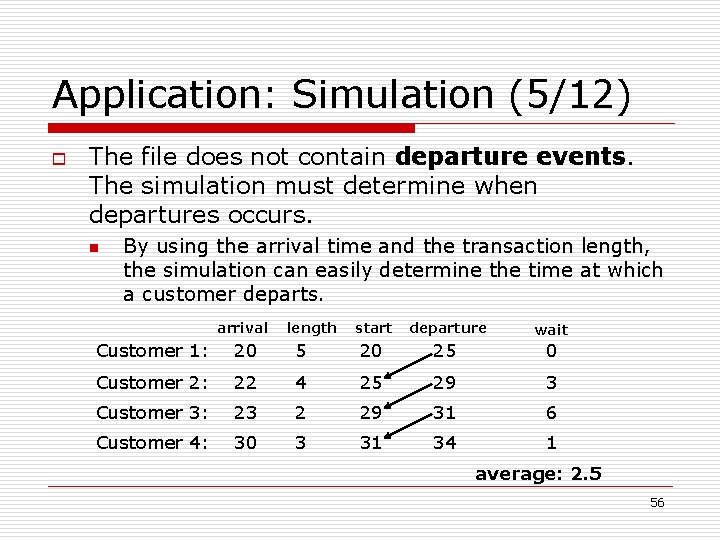
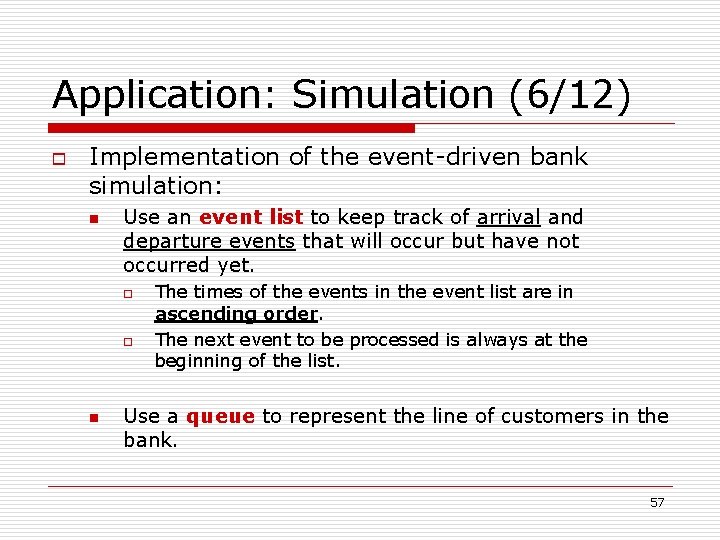
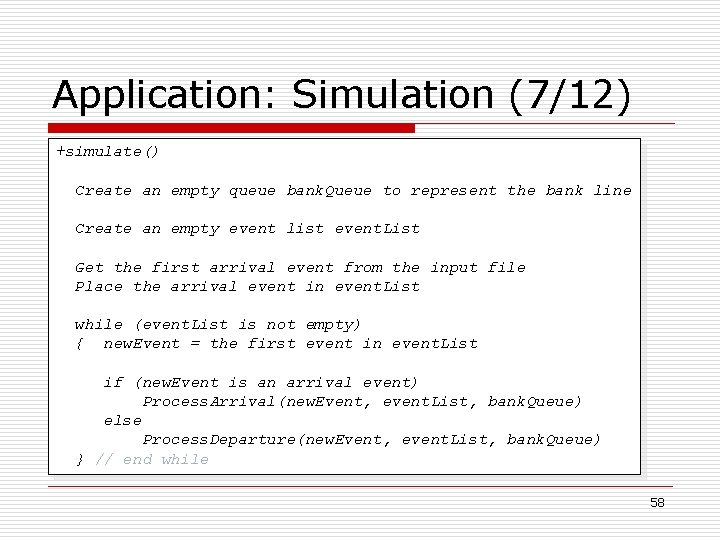
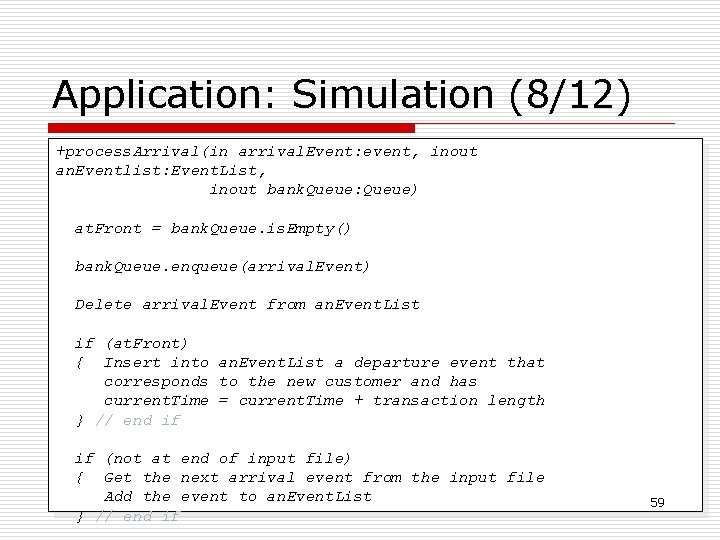
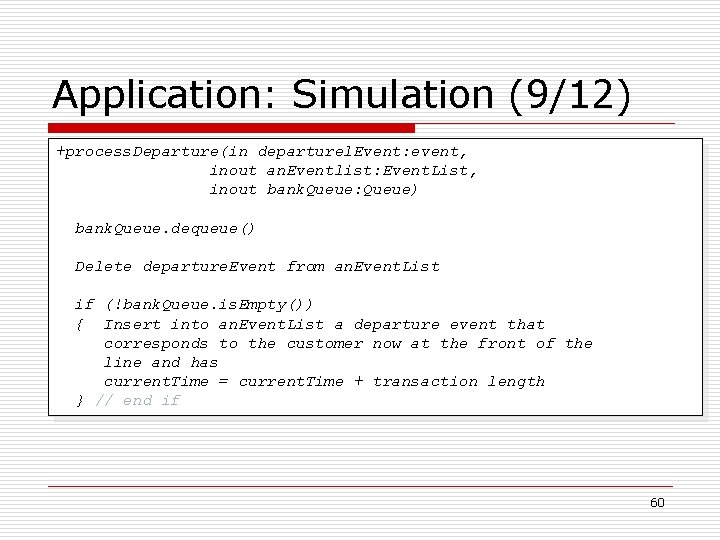
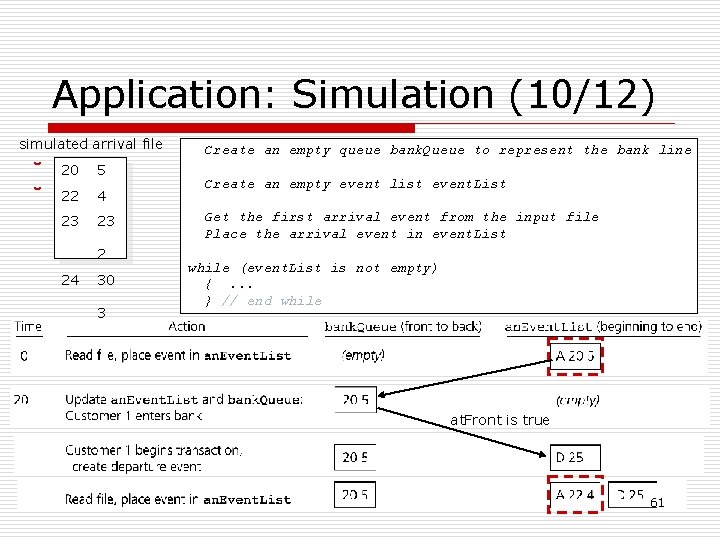
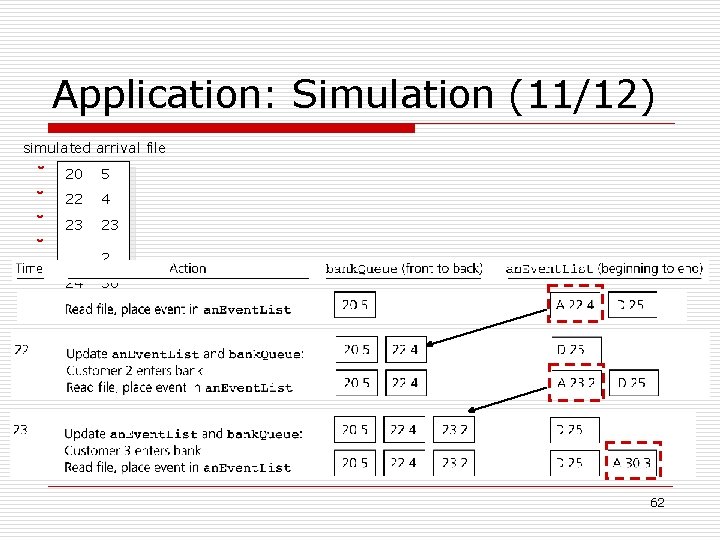
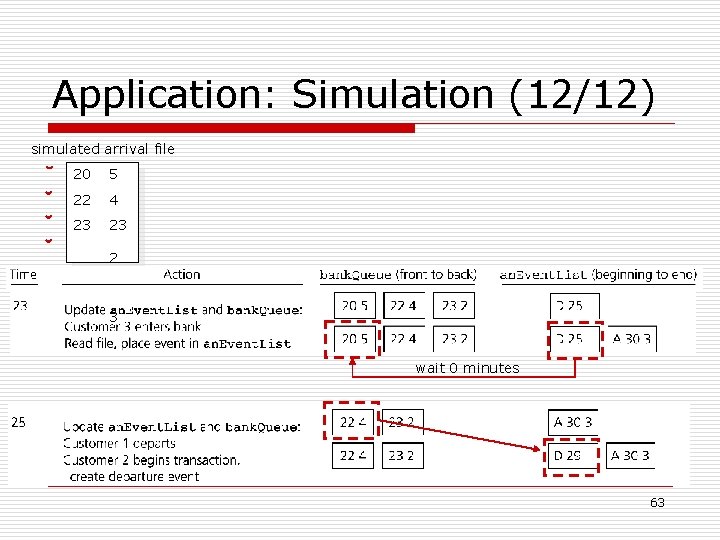
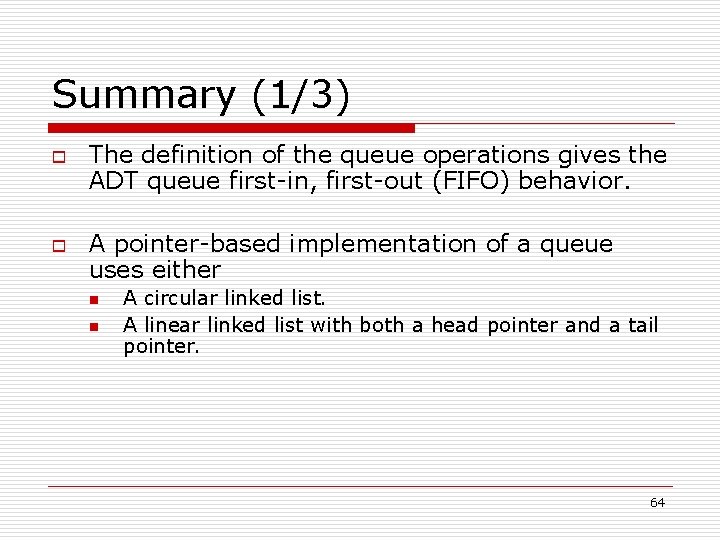
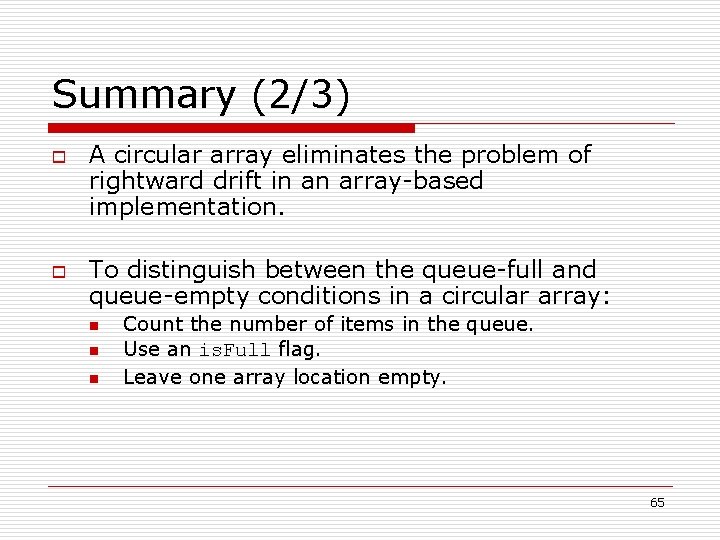
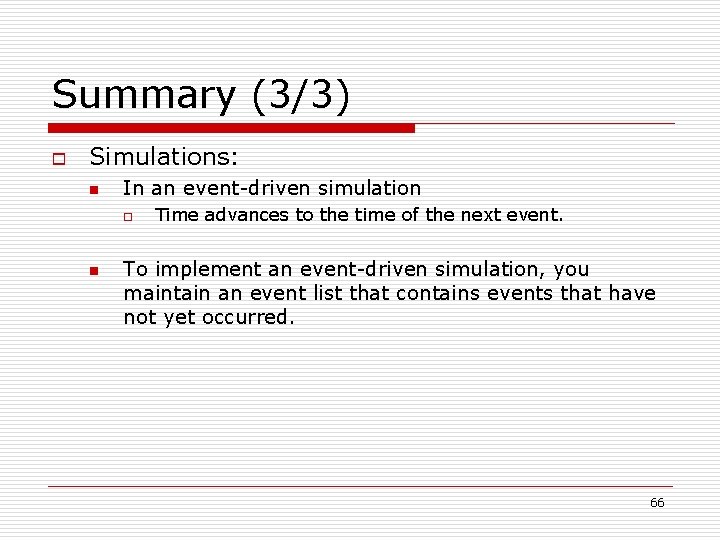
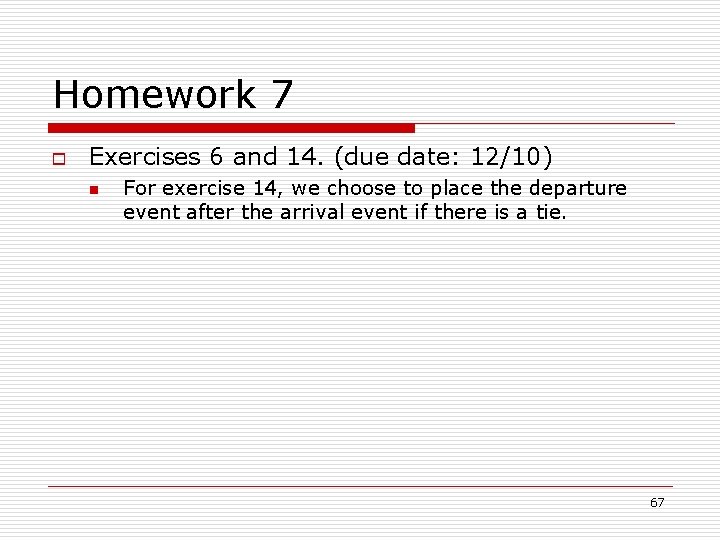
- Slides: 67
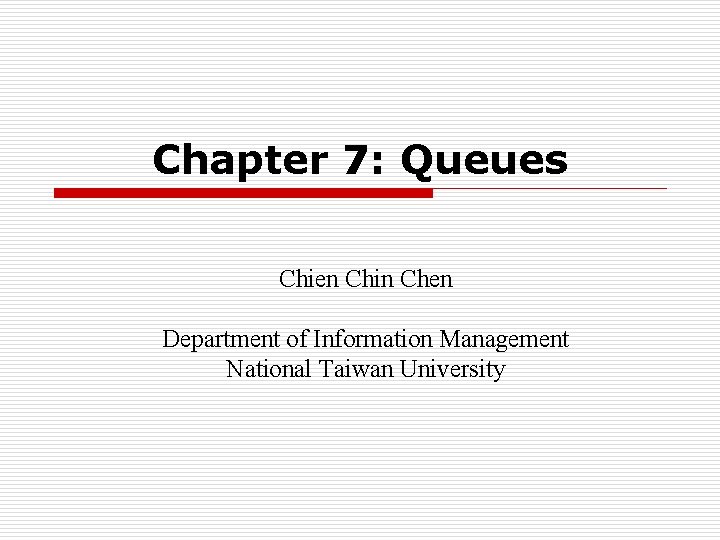
Chapter 7: Queues Chien Chin Chen Department of Information Management National Taiwan University
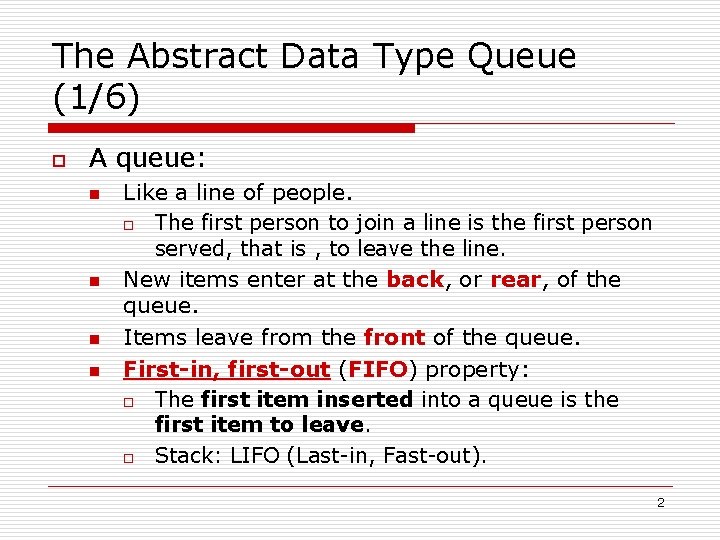
The Abstract Data Type Queue (1/6) o A queue: n n Like a line of people. o The first person to join a line is the first person served, that is , to leave the line. New items enter at the back, or rear, of the queue. Items leave from the front of the queue. First-in, first-out (FIFO) property: o The first item inserted into a queue is the first item to leave. o Stack: LIFO (Last-in, Fast-out). 2
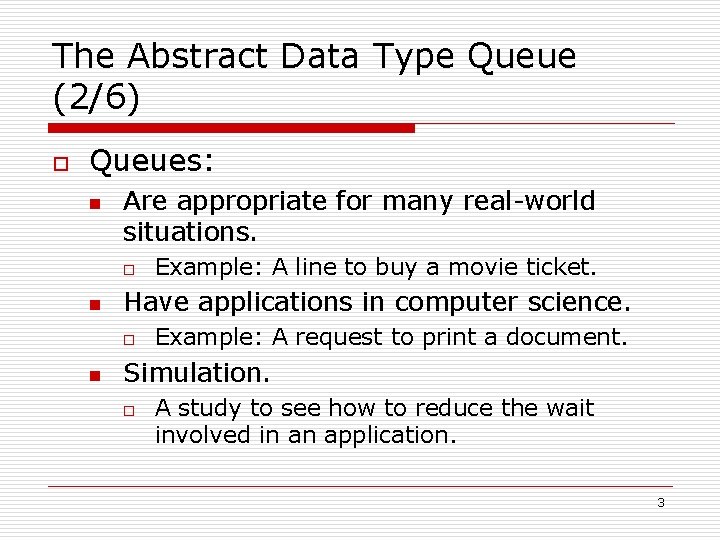
The Abstract Data Type Queue (2/6) o Queues: n Are appropriate for many real-world situations. o n Have applications in computer science. o n Example: A line to buy a movie ticket. Example: A request to print a document. Simulation. o A study to see how to reduce the wait involved in an application. 3
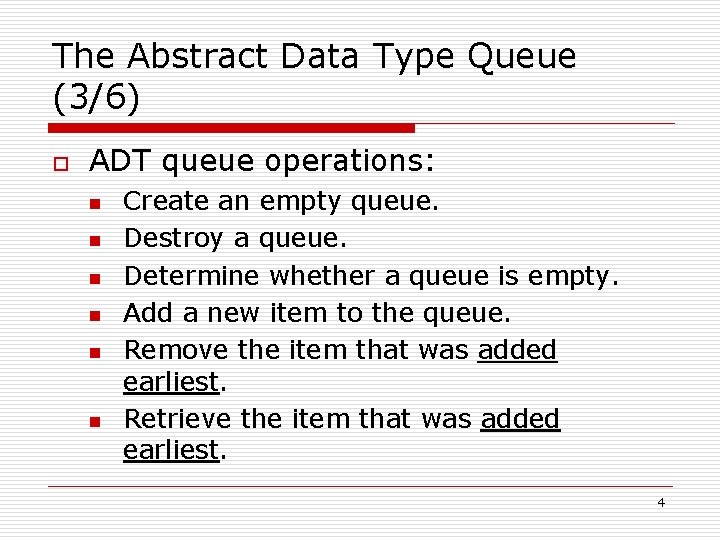
The Abstract Data Type Queue (3/6) o ADT queue operations: n n n Create an empty queue. Destroy a queue. Determine whether a queue is empty. Add a new item to the queue. Remove the item that was added earliest. Retrieve the item that was added earliest. 4
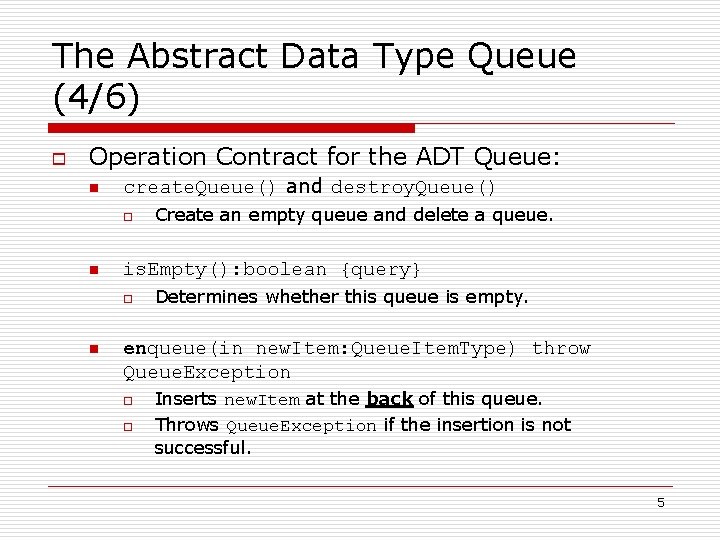
The Abstract Data Type Queue (4/6) o Operation Contract for the ADT Queue: n create. Queue() and destroy. Queue() o n is. Empty(): boolean {query} o n Create an empty queue and delete a queue. Determines whether this queue is empty. enqueue(in new. Item: Queue. Item. Type) throw Queue. Exception o o Inserts new. Item at the back of this queue. Throws Queue. Exception if the insertion is not successful. 5
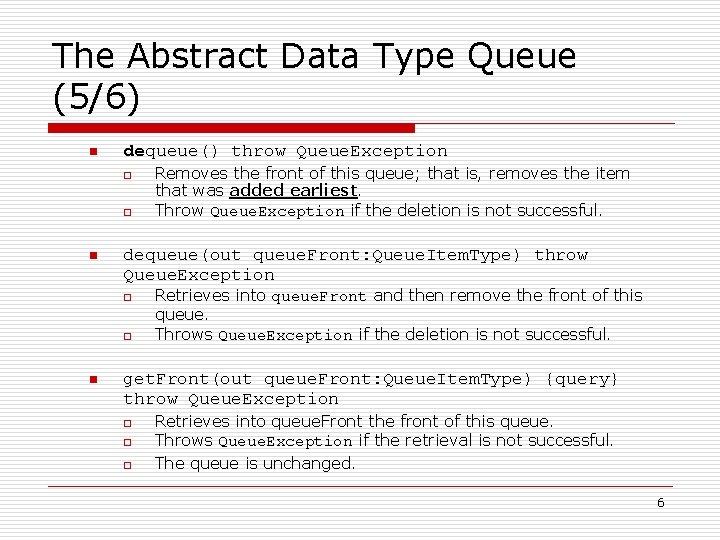
The Abstract Data Type Queue (5/6) n dequeue() throw Queue. Exception o o n dequeue(out queue. Front: Queue. Item. Type) throw Queue. Exception o o n Removes the front of this queue; that is, removes the item that was added earliest. Throw Queue. Exception if the deletion is not successful. Retrieves into queue. Front and then remove the front of this queue. Throws Queue. Exception if the deletion is not successful. get. Front(out queue. Front: Queue. Item. Type) {query} throw Queue. Exception o o o Retrieves into queue. Front the front of this queue. Throws Queue. Exception if the retrieval is not successful. The queue is unchanged. 6
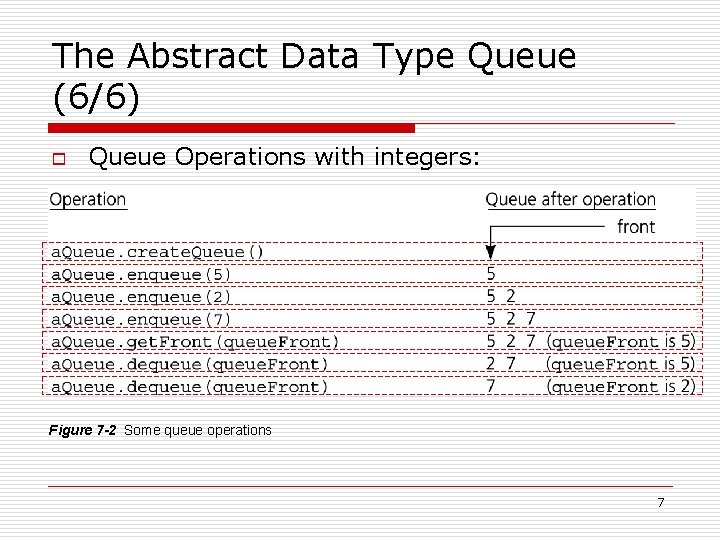
The Abstract Data Type Queue (6/6) o Queue Operations with integers: Figure 7 -2 Some queue operations 7
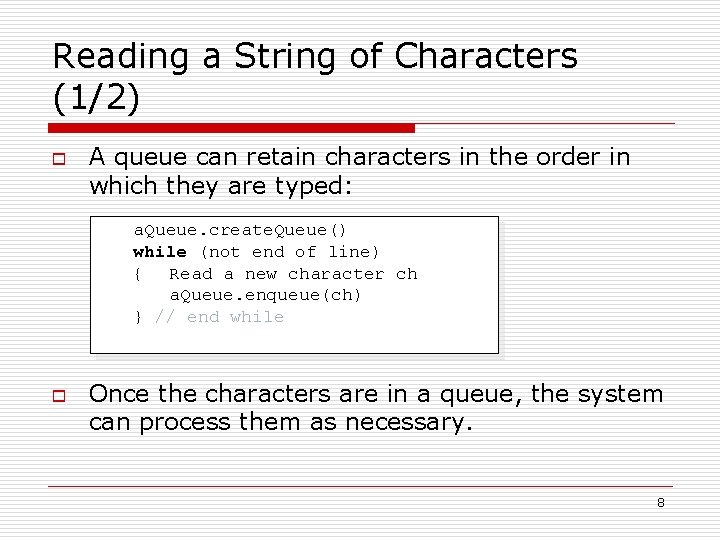
Reading a String of Characters (1/2) o A queue can retain characters in the order in which they are typed: a. Queue. create. Queue() while (not end of line) { Read a new character ch a. Queue. enqueue(ch) } // end while o Once the characters are in a queue, the system can process them as necessary. 8
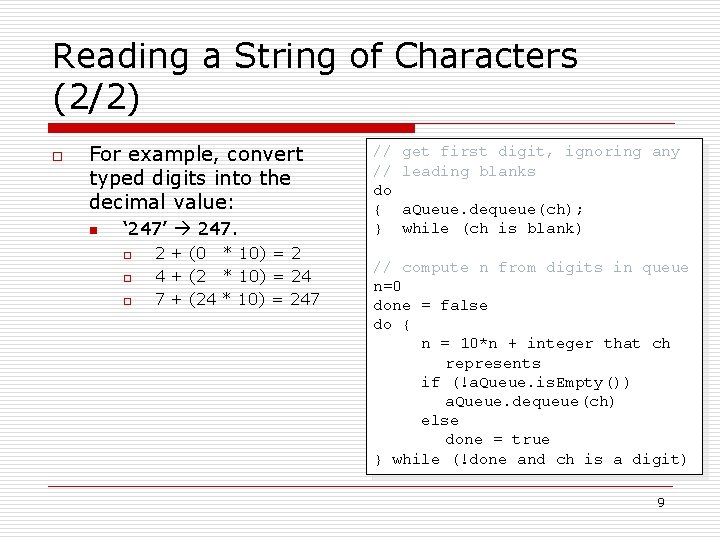
Reading a String of Characters (2/2) o For example, convert typed digits into the decimal value: n ‘ 247’ 247. o o o 2 + (0 * 10) = 2 4 + (2 * 10) = 24 7 + (24 * 10) = 247 // // do { } get first digit, ignoring any leading blanks a. Queue. dequeue(ch); while (ch is blank) // compute n from digits in queue n=0 done = false do { n = 10*n + integer that ch represents if (!a. Queue. is. Empty()) a. Queue. dequeue(ch) else done = true } while (!done and ch is a digit) 9
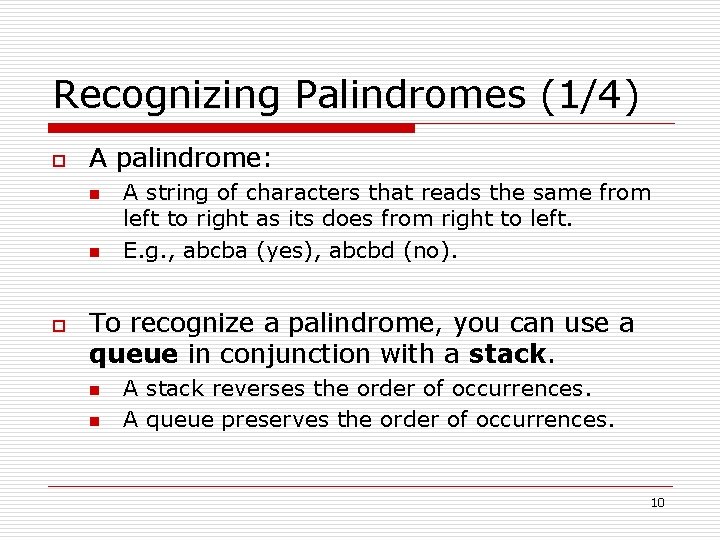
Recognizing Palindromes (1/4) o A palindrome: n n o A string of characters that reads the same from left to right as its does from right to left. E. g. , abcba (yes), abcbd (no). To recognize a palindrome, you can use a queue in conjunction with a stack. n n A stack reverses the order of occurrences. A queue preserves the order of occurrences. 10
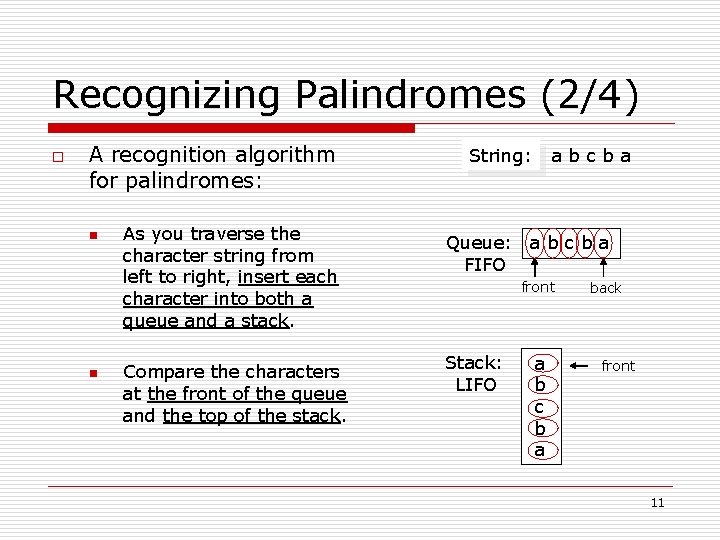
Recognizing Palindromes (2/4) o A recognition algorithm for palindromes: n n As you traverse the character string from left to right, insert each character into both a queue and a stack. Compare the characters at the front of the queue and the top of the stack. abc ba String: Queue: a b c b a FIFO front Stack: LIFO a b c b a back front 11
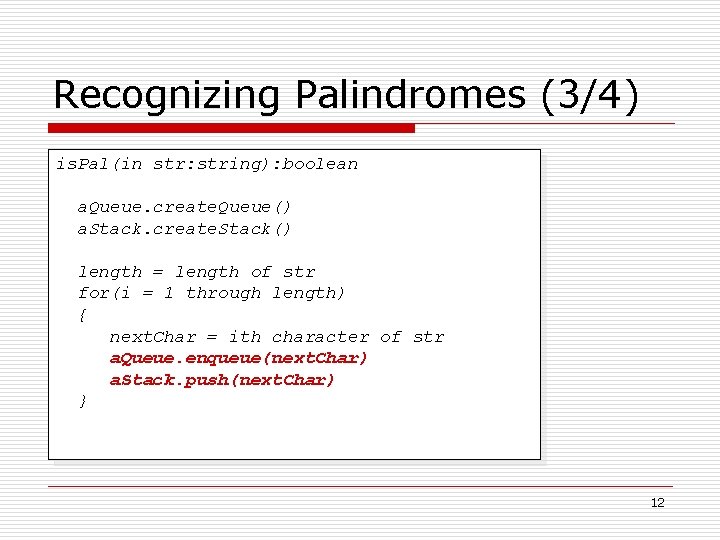
Recognizing Palindromes (3/4) is. Pal(in str: string): boolean a. Queue. create. Queue() a. Stack. create. Stack() length = length of str for(i = 1 through length) { next. Char = ith character of str a. Queue. enqueue(next. Char) a. Stack. push(next. Char) } 12
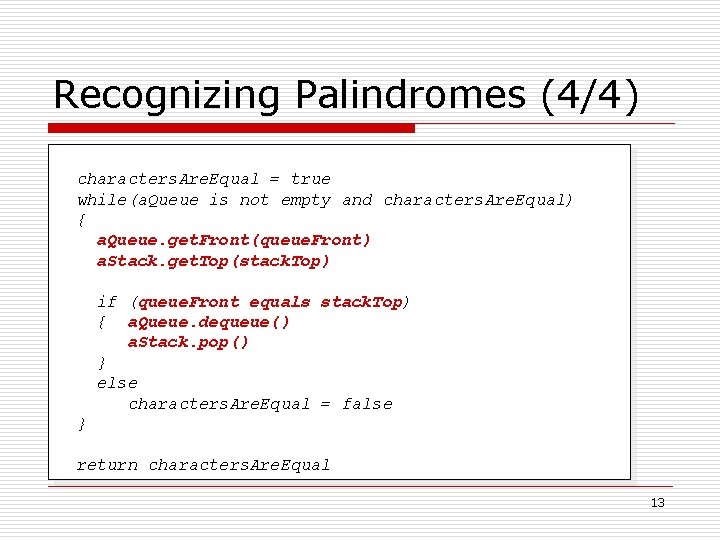
Recognizing Palindromes (4/4) characters. Are. Equal = true while(a. Queue is not empty and characters. Are. Equal) { a. Queue. get. Front(queue. Front) a. Stack. get. Top(stack. Top) if (queue. Front equals stack. Top) { a. Queue. dequeue() a. Stack. pop() } else characters. Are. Equal = false } return characters. Are. Equal 13
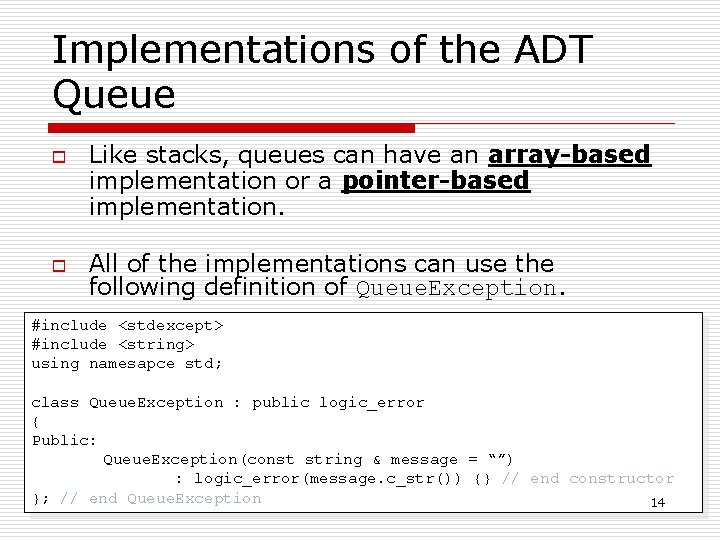
Implementations of the ADT Queue o o Like stacks, queues can have an array-based implementation or a pointer-based implementation. All of the implementations can use the following definition of Queue. Exception. #include <stdexcept> #include <string> using namesapce std; class Queue. Exception : public logic_error { Public: Queue. Exception(const string & message = “”) : logic_error(message. c_str()) {} // end constructor }; // end Queue. Exception 14
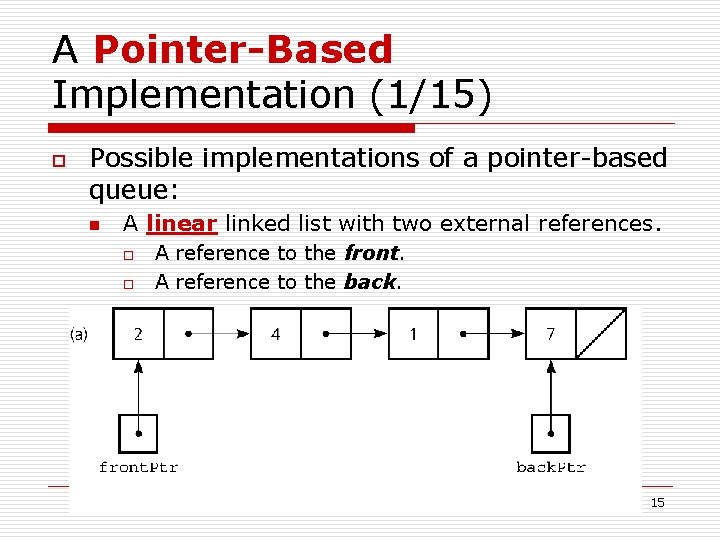
A Pointer-Based Implementation (1/15) o Possible implementations of a pointer-based queue: n A linear linked list with two external references. o o A reference to the front. A reference to the back. 15
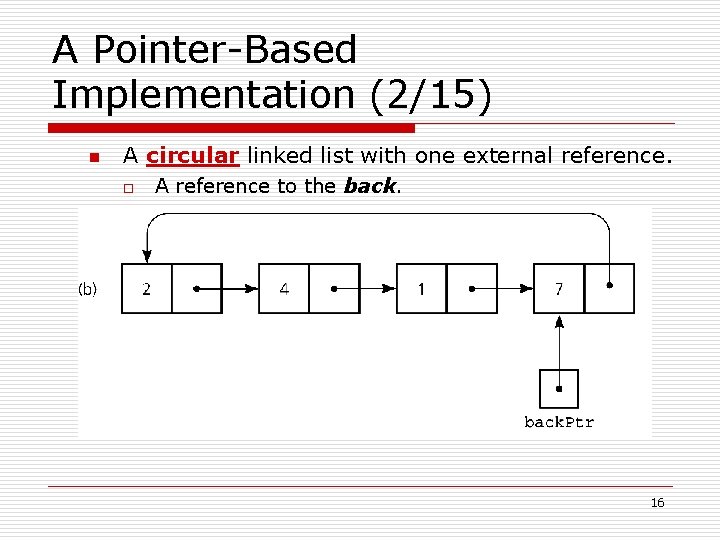
A Pointer-Based Implementation (2/15) n A circular linked list with one external reference. o A reference to the back. 16
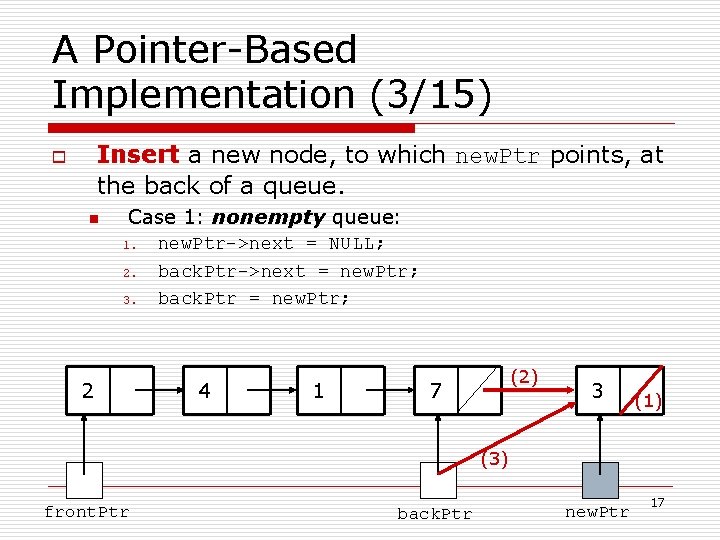
A Pointer-Based Implementation (3/15) Insert a new node, to which new. Ptr points, at the back of a queue. o n Case 1: nonempty queue: 1. 2. 3. 2 new. Ptr->next = NULL; back. Ptr->next = new. Ptr; back. Ptr = new. Ptr; 4 1 (2) 7 3 (1) (3) front. Ptr back. Ptr new. Ptr 17
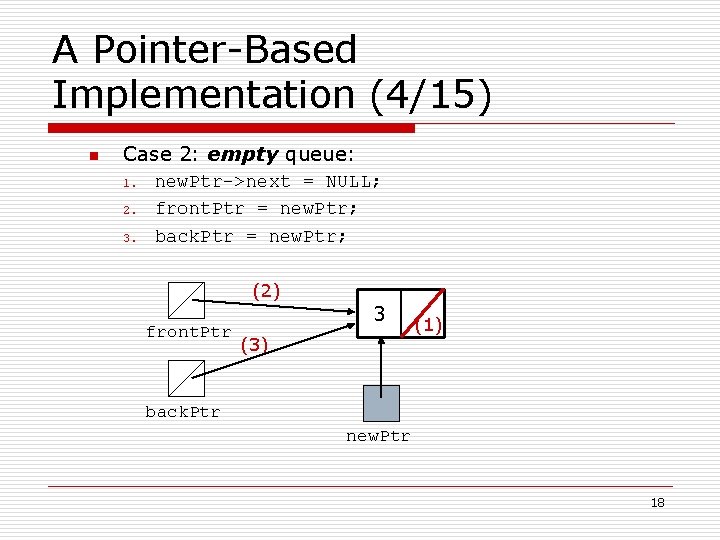
A Pointer-Based Implementation (4/15) n Case 2: empty queue: 1. 2. 3. new. Ptr->next = NULL; front. Ptr = new. Ptr; back. Ptr = new. Ptr; (2) front. Ptr 3 (3) (1) back. Ptr new. Ptr 18
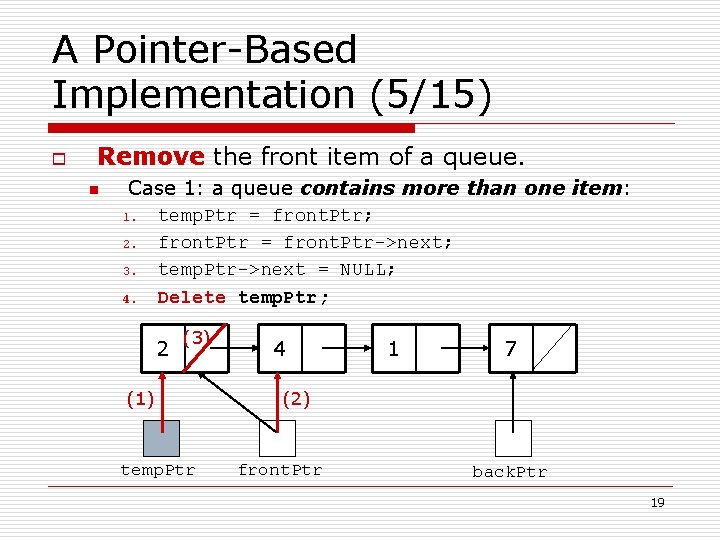
A Pointer-Based Implementation (5/15) o Remove the front item of a queue. n Case 1: a queue contains more than one item: 1. 2. 3. 4. temp. Ptr = front. Ptr; front. Ptr = front. Ptr->next; temp. Ptr->next = NULL; Delete temp. Ptr; 2 (3) (1) temp. Ptr 4 1 7 (2) front. Ptr back. Ptr 19
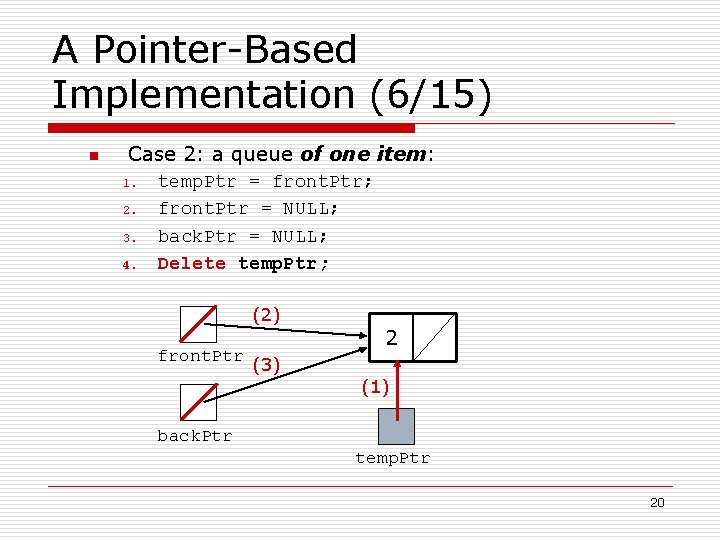
A Pointer-Based Implementation (6/15) n Case 2: a queue of one item: 1. 2. 3. 4. temp. Ptr = front. Ptr; front. Ptr = NULL; back. Ptr = NULL; Delete temp. Ptr; (2) front. Ptr 2 (3) (1) back. Ptr temp. Ptr 20
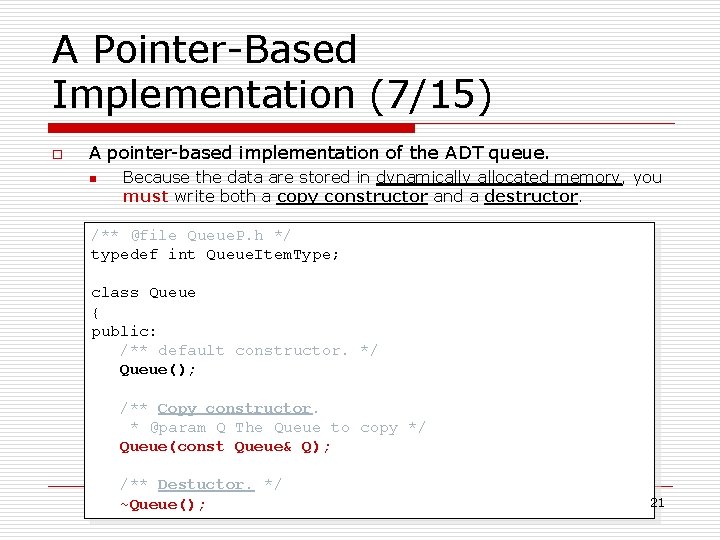
A Pointer-Based Implementation (7/15) o A pointer-based implementation of the ADT queue. n Because the data are stored in dynamically allocated memory, you must write both a copy constructor and a destructor. /** @file Queue. P. h */ typedef int Queue. Item. Type; class Queue { public: /** default constructor. */ Queue(); /** Copy constructor. * @param Q The Queue to copy */ Queue(const Queue& Q); /** Destuctor. */ ~Queue(); 21
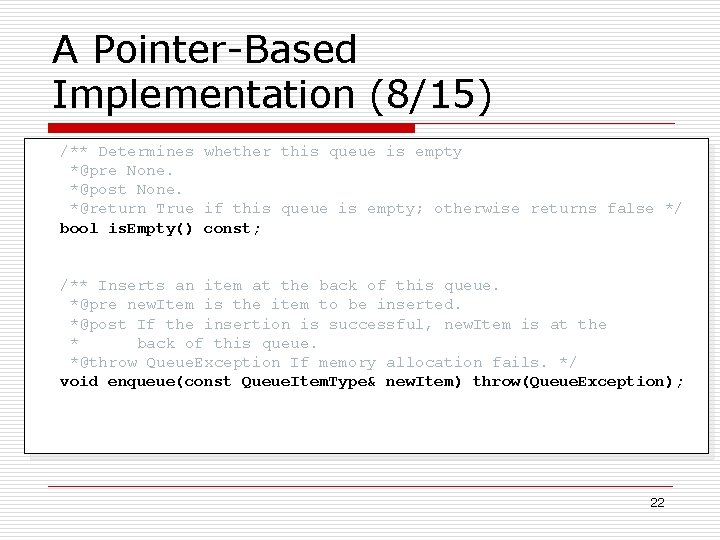
A Pointer-Based Implementation (8/15) /** Determines whether this queue is empty *@pre None. *@post None. *@return True if this queue is empty; otherwise returns false */ bool is. Empty() const; /** Inserts an item at the back of this queue. *@pre new. Item is the item to be inserted. *@post If the insertion is successful, new. Item is at the * back of this queue. *@throw Queue. Exception If memory allocation fails. */ void enqueue(const Queue. Item. Type& new. Item) throw(Queue. Exception); 22
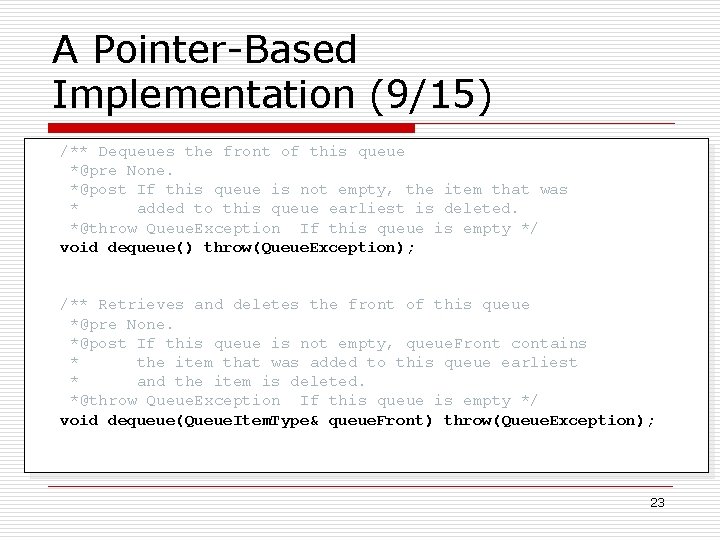
A Pointer-Based Implementation (9/15) /** Dequeues the front of this queue *@pre None. *@post If this queue is not empty, the item that was * added to this queue earliest is deleted. *@throw Queue. Exception If this queue is empty */ void dequeue() throw(Queue. Exception); /** Retrieves and deletes the front of this queue *@pre None. *@post If this queue is not empty, queue. Front contains * the item that was added to this queue earliest * and the item is deleted. *@throw Queue. Exception If this queue is empty */ void dequeue(Queue. Item. Type& queue. Front) throw(Queue. Exception); 23
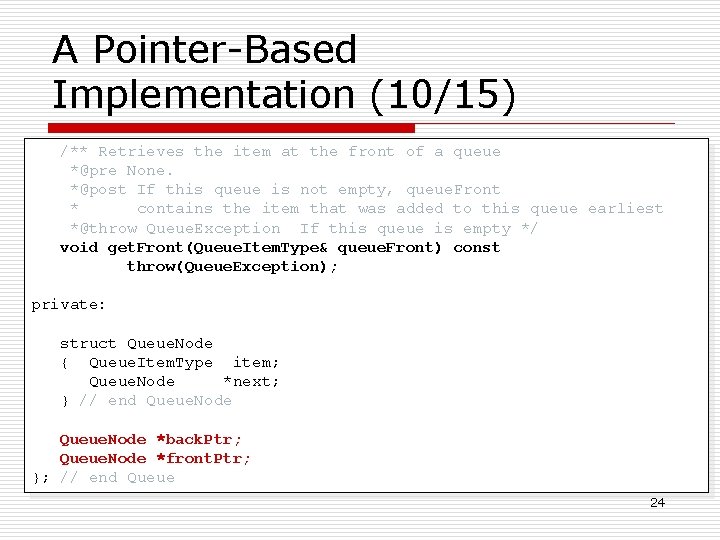
A Pointer-Based Implementation (10/15) /** Retrieves the item at the front of a queue *@pre None. *@post If this queue is not empty, queue. Front * contains the item that was added to this queue earliest *@throw Queue. Exception If this queue is empty */ void get. Front(Queue. Item. Type& queue. Front) const throw(Queue. Exception); private: struct Queue. Node { Queue. Item. Type item; Queue. Node *next; } // end Queue. Node *back. Ptr; Queue. Node *front. Ptr; }; // end Queue 24
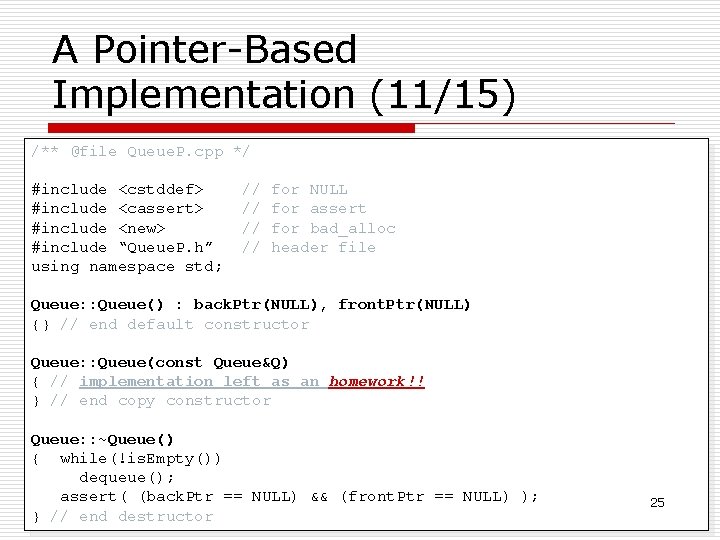
A Pointer-Based Implementation (11/15) /** @file Queue. P. cpp */ #include <cstddef> #include <cassert> #include <new> #include “Queue. P. h” using namespace std; // // for NULL for assert for bad_alloc header file Queue: : Queue() : back. Ptr(NULL), front. Ptr(NULL) {} // end default constructor Queue: : Queue(const Queue&Q) { // implementation left as an homework!! } // end copy constructor Queue: : ~Queue() { while(!is. Empty()) dequeue(); assert( (back. Ptr == NULL) && (front. Ptr == NULL) ); } // end destructor 25
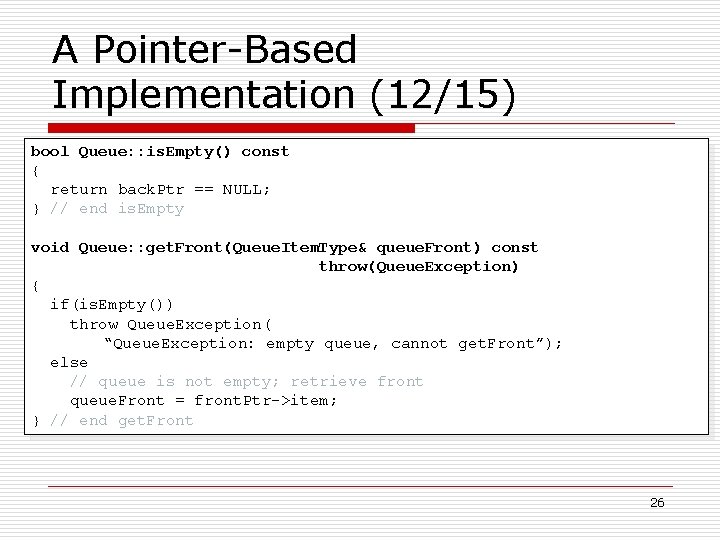
A Pointer-Based Implementation (12/15) bool Queue: : is. Empty() const { return back. Ptr == NULL; } // end is. Empty void Queue: : get. Front(Queue. Item. Type& queue. Front) const throw(Queue. Exception) { if(is. Empty()) throw Queue. Exception( “Queue. Exception: empty queue, cannot get. Front”); else // queue is not empty; retrieve front queue. Front = front. Ptr->item; } // end get. Front 26
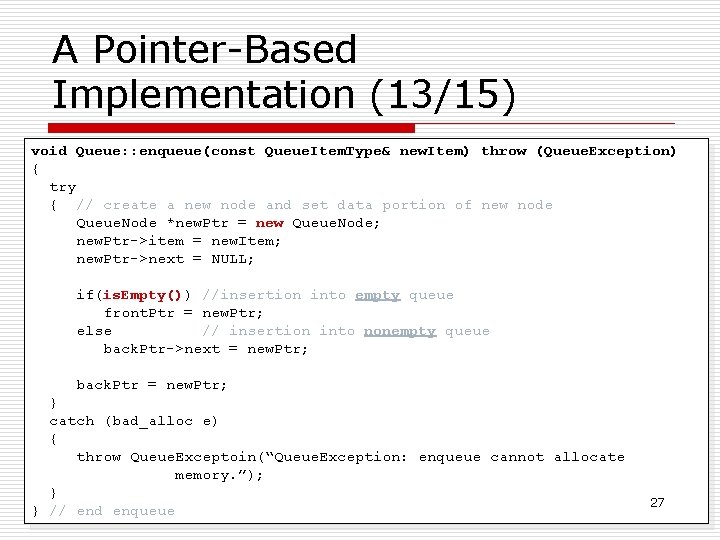
A Pointer-Based Implementation (13/15) void Queue: : enqueue(const Queue. Item. Type& new. Item) throw (Queue. Exception) { try { // create a new node and set data portion of new node Queue. Node *new. Ptr = new Queue. Node; new. Ptr->item = new. Item; new. Ptr->next = NULL; if(is. Empty()) //insertion into empty queue front. Ptr = new. Ptr; else // insertion into nonempty queue back. Ptr->next = new. Ptr; back. Ptr = new. Ptr; } catch (bad_alloc e) { throw Queue. Exceptoin(“Queue. Exception: enqueue cannot allocate memory. ”); } } // end enqueue 27
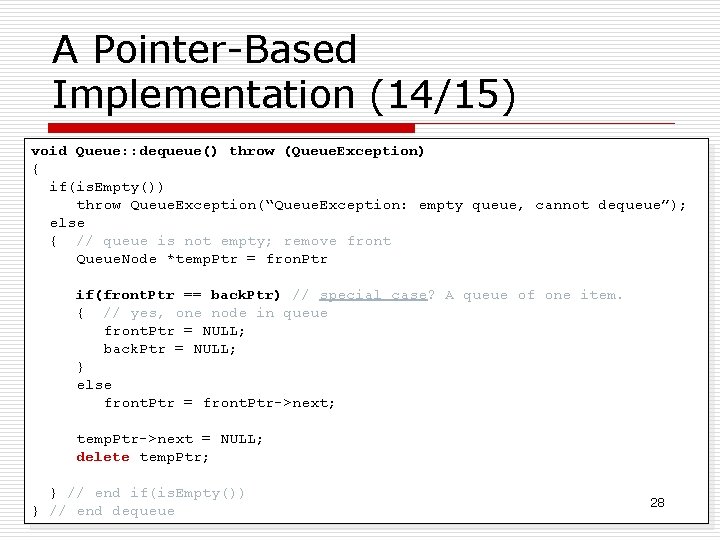
A Pointer-Based Implementation (14/15) void Queue: : dequeue() throw (Queue. Exception) { if(is. Empty()) throw Queue. Exception(“Queue. Exception: empty queue, cannot dequeue”); else { // queue is not empty; remove front Queue. Node *temp. Ptr = fron. Ptr if(front. Ptr == back. Ptr) // special case? A queue of one item. { // yes, one node in queue front. Ptr = NULL; back. Ptr = NULL; } else front. Ptr = front. Ptr->next; temp. Ptr->next = NULL; delete temp. Ptr; } // end if(is. Empty()) } // end dequeue 28
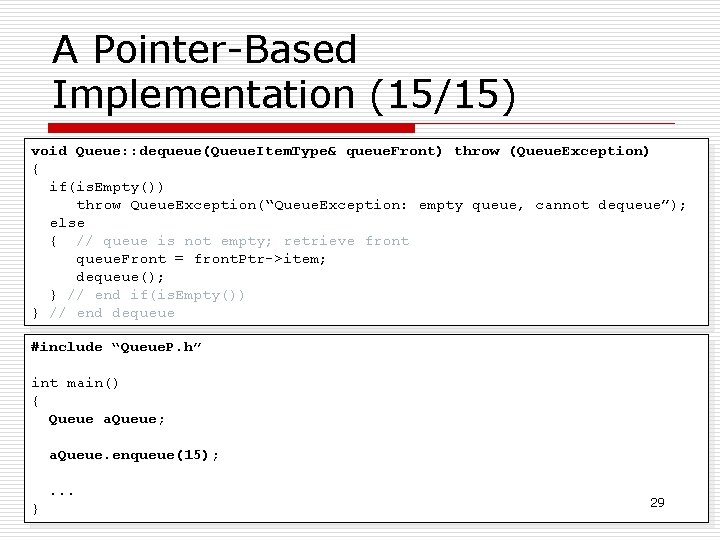
A Pointer-Based Implementation (15/15) void Queue: : dequeue(Queue. Item. Type& queue. Front) throw (Queue. Exception) { if(is. Empty()) throw Queue. Exception(“Queue. Exception: empty queue, cannot dequeue”); else { // queue is not empty; retrieve front queue. Front = front. Ptr->item; dequeue(); } // end if(is. Empty()) } // end dequeue #include “Queue. P. h” int main() { Queue a. Queue; a. Queue. enqueue(15); . . . } 29
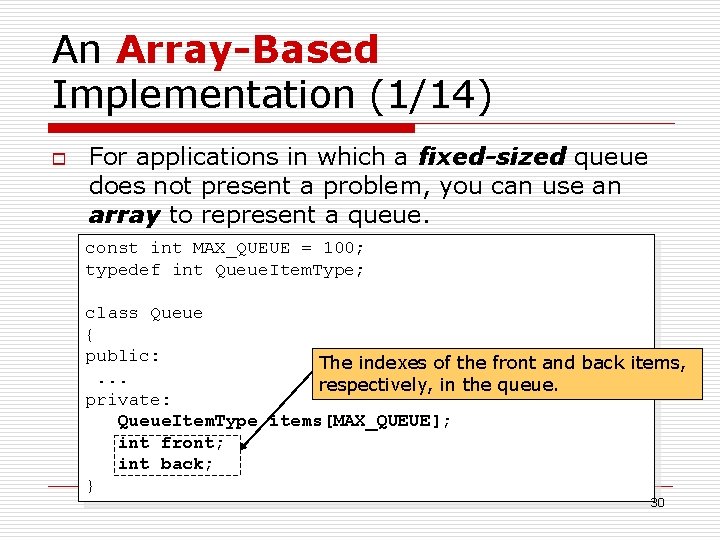
An Array-Based Implementation (1/14) o For applications in which a fixed-sized queue does not present a problem, you can use an array to represent a queue. const int MAX_QUEUE = 100; typedef int Queue. Item. Type; class Queue { public: The indexes of the front and back items, . . . respectively, in the queue. private: Queue. Item. Type items[MAX_QUEUE]; int front; int back; } 30
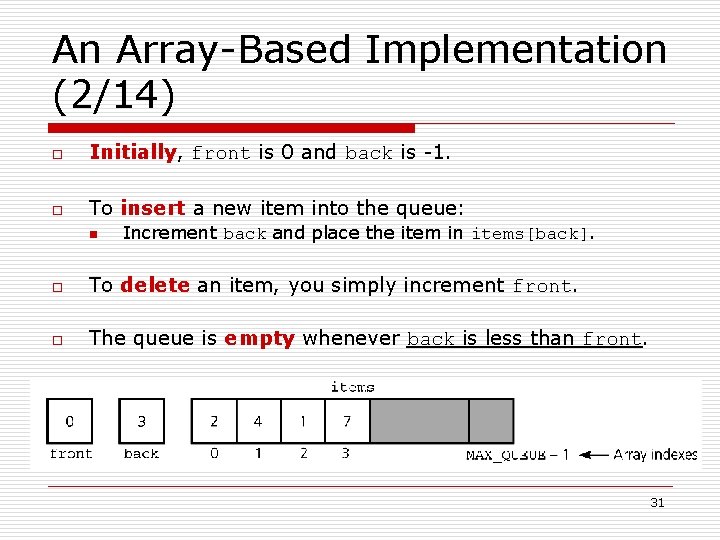
An Array-Based Implementation (2/14) o Initially, front is 0 and back is -1. o To insert a new item into the queue: n Increment back and place the item in items[back]. o To delete an item, you simply increment front. o The queue is empty whenever back is less than front. 31
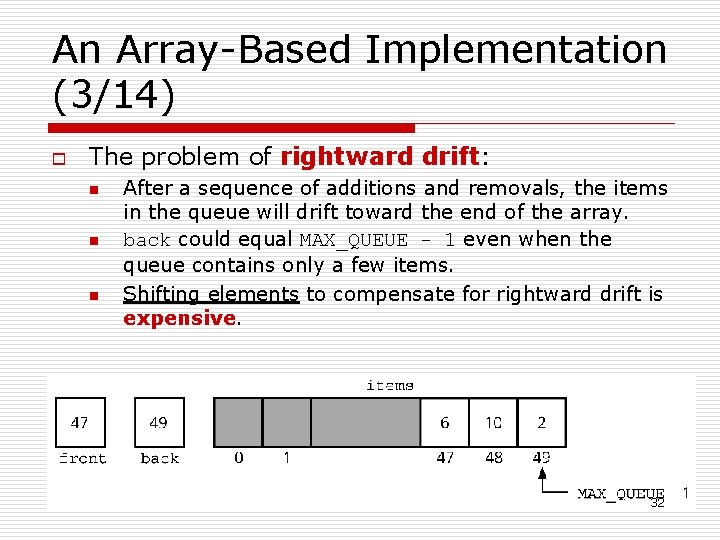
An Array-Based Implementation (3/14) o The problem of rightward drift: n n n After a sequence of additions and removals, the items in the queue will drift toward the end of the array. back could equal MAX_QUEUE - 1 even when the queue contains only a few items. Shifting elements to compensate for rightward drift is expensive. 32
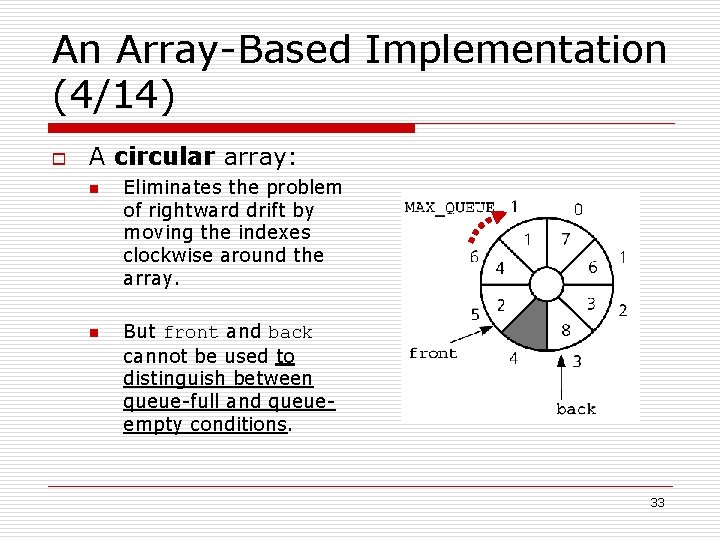
An Array-Based Implementation (4/14) o A circular array: n n Eliminates the problem of rightward drift by moving the indexes clockwise around the array. But front and back cannot be used to distinguish between queue-full and queueempty conditions. 33
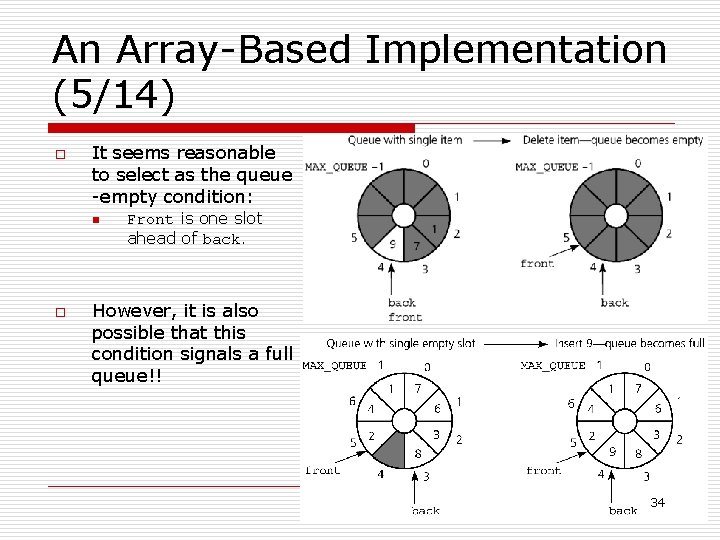
An Array-Based Implementation (5/14) o It seems reasonable to select as the queue -empty condition: n o Front is one slot ahead of back. However, it is also possible that this condition signals a full queue!! 34
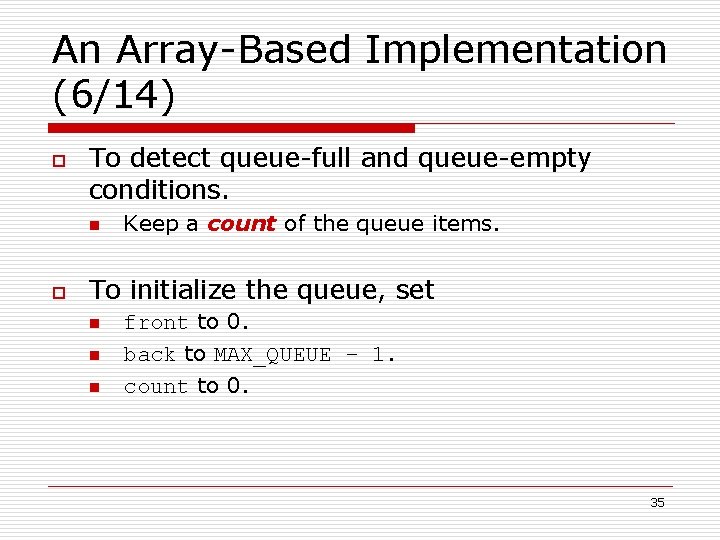
An Array-Based Implementation (6/14) o To detect queue-full and queue-empty conditions. n o Keep a count of the queue items. To initialize the queue, set n n n front to 0. back to MAX_QUEUE – 1. count to 0. 35
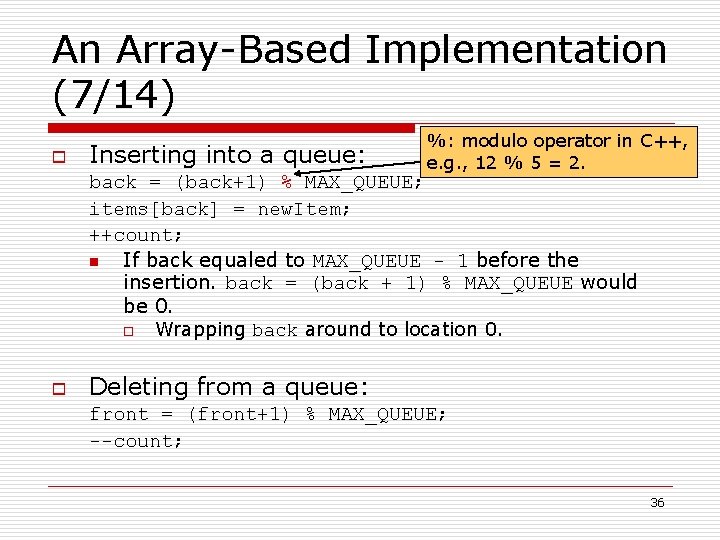
An Array-Based Implementation (7/14) o Inserting into a queue: %: modulo operator in C++, e. g. , 12 % 5 = 2. back = (back+1) % MAX_QUEUE; items[back] = new. Item; ++count; n If back equaled to MAX_QUEUE - 1 before the insertion. back = (back + 1) % MAX_QUEUE would be 0. o o Wrapping back around to location 0. Deleting from a queue: front = (front+1) % MAX_QUEUE; --count; 36
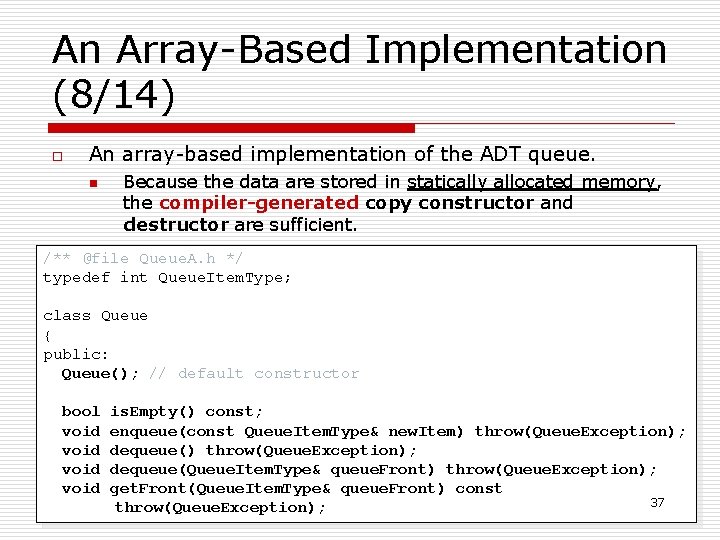
An Array-Based Implementation (8/14) o An array-based implementation of the ADT queue. n Because the data are stored in statically allocated memory, the compiler-generated copy constructor and destructor are sufficient. /** @file Queue. A. h */ typedef int Queue. Item. Type; class Queue { public: Queue(); // default constructor bool void is. Empty() const; enqueue(const Queue. Item. Type& new. Item) throw(Queue. Exception); dequeue(Queue. Item. Type& queue. Front) throw(Queue. Exception); get. Front(Queue. Item. Type& queue. Front) const 37 throw(Queue. Exception);
![An ArrayBased Implementation 914 private Queue Item Type itemsMAXQUEUE int front int back int An Array-Based Implementation (9/14) private: Queue. Item. Type items[MAX_QUEUE]; int front; int back; int](https://slidetodoc.com/presentation_image/afe928bd2f7d8e4fe93626d61bb9298c/image-38.jpg)
An Array-Based Implementation (9/14) private: Queue. Item. Type items[MAX_QUEUE]; int front; int back; int count; }; // end Queue /** @file Queue. A. cpp */ #include “Queue. A. h” Queue: : Queue() : front(0), back(MAX_QUEUE-1), count(0) { } // end default constructor 38
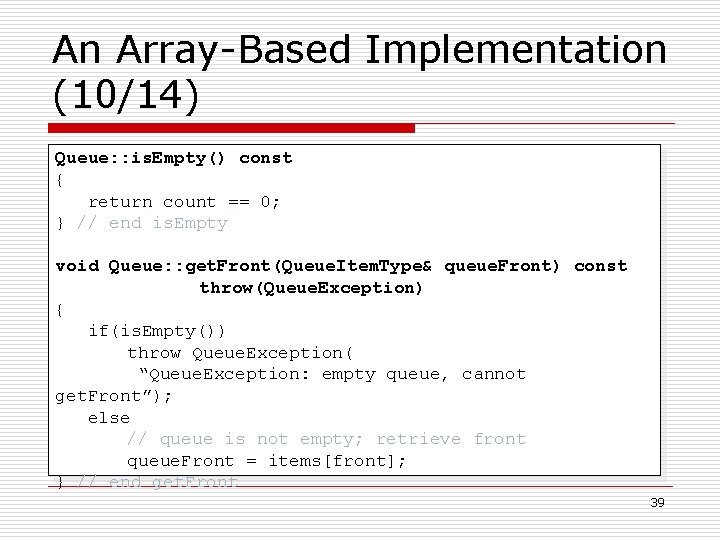
An Array-Based Implementation (10/14) Queue: : is. Empty() const { return count == 0; } // end is. Empty void Queue: : get. Front(Queue. Item. Type& queue. Front) const throw(Queue. Exception) { if(is. Empty()) throw Queue. Exception( “Queue. Exception: empty queue, cannot get. Front”); else // queue is not empty; retrieve front queue. Front = items[front]; } // end get. Front 39
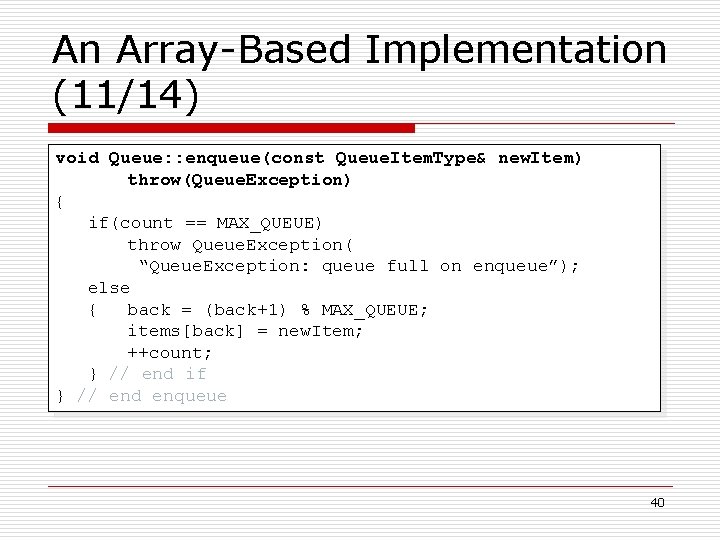
An Array-Based Implementation (11/14) void Queue: : enqueue(const Queue. Item. Type& new. Item) throw(Queue. Exception) { if(count == MAX_QUEUE) throw Queue. Exception( “Queue. Exception: queue full on enqueue”); else { back = (back+1) % MAX_QUEUE; items[back] = new. Item; ++count; } // end if } // end enqueue 40
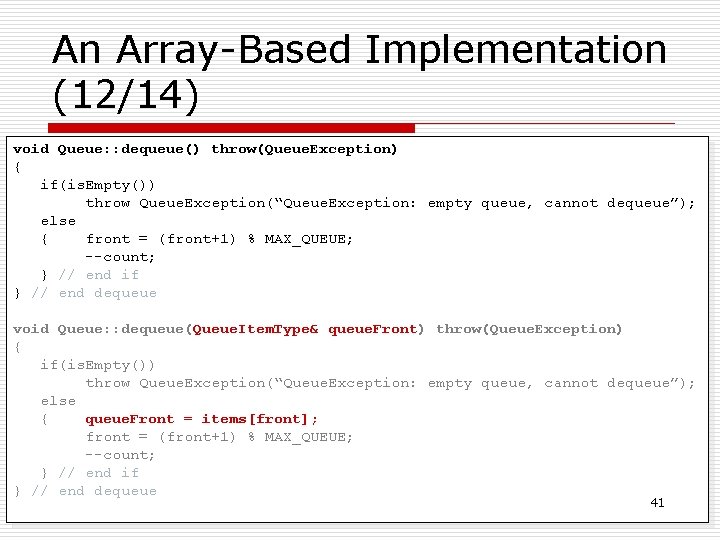
An Array-Based Implementation (12/14) void Queue: : dequeue() throw(Queue. Exception) { if(is. Empty()) throw Queue. Exception(“Queue. Exception: empty queue, cannot dequeue”); else { front = (front+1) % MAX_QUEUE; --count; } // end if } // end dequeue void Queue: : dequeue(Queue. Item. Type& queue. Front) throw(Queue. Exception) { if(is. Empty()) throw Queue. Exception(“Queue. Exception: empty queue, cannot dequeue”); else { queue. Front = items[front]; front = (front+1) % MAX_QUEUE; --count; } // end if } // end dequeue 41
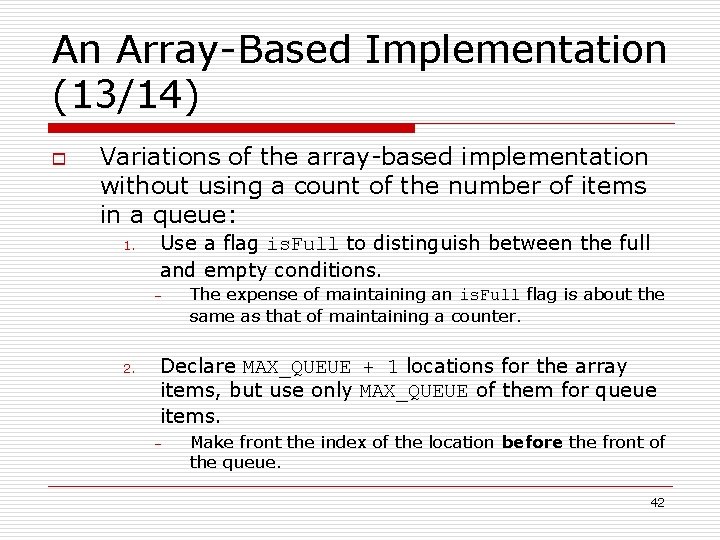
An Array-Based Implementation (13/14) o Variations of the array-based implementation without using a count of the number of items in a queue: 1. Use a flag is. Full to distinguish between the full and empty conditions. – 2. The expense of maintaining an is. Full flag is about the same as that of maintaining a counter. Declare MAX_QUEUE + 1 locations for the array items, but use only MAX_QUEUE of them for queue items. – Make front the index of the location before the front of the queue. 42
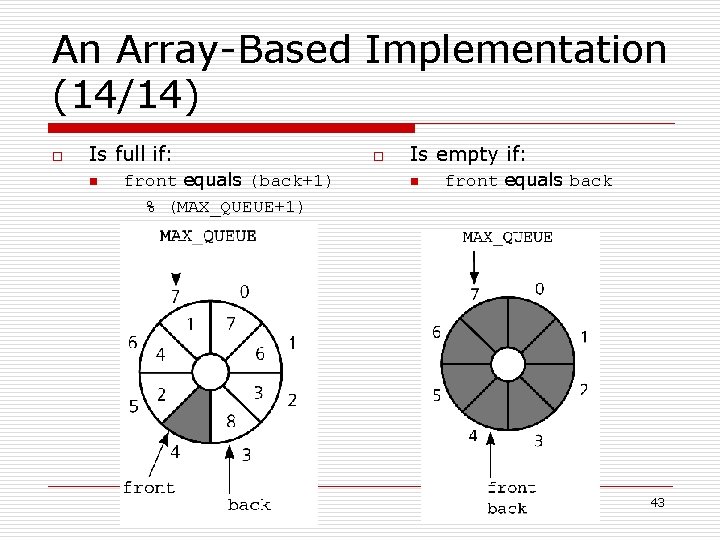
An Array-Based Implementation (14/14) o Is full if: n front equals (back+1) % (MAX_QUEUE+1) o Is empty if: n front equals back 43
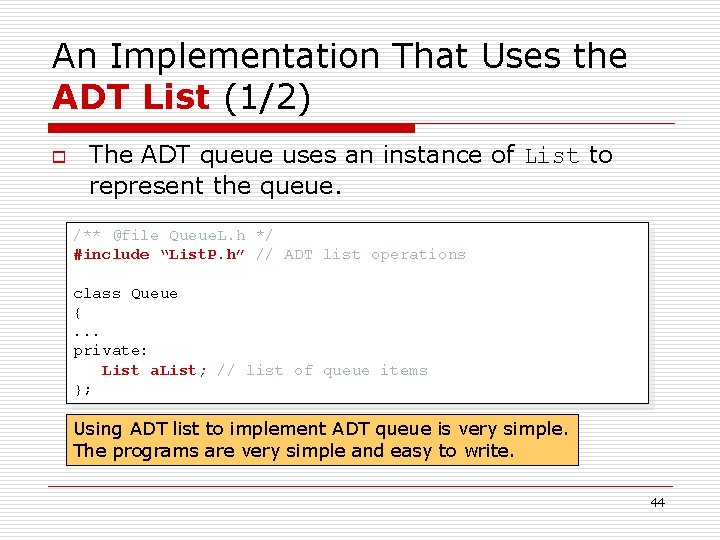
An Implementation That Uses the ADT List (1/2) o The ADT queue uses an instance of List to represent the queue. /** @file Queue. L. h */ #include “List. P. h” // ADT list operations class Queue {. . . private: List a. List; // list of queue items }; Using ADT list to implement ADT queue is very simple. The programs are very simple and easy to write. 44
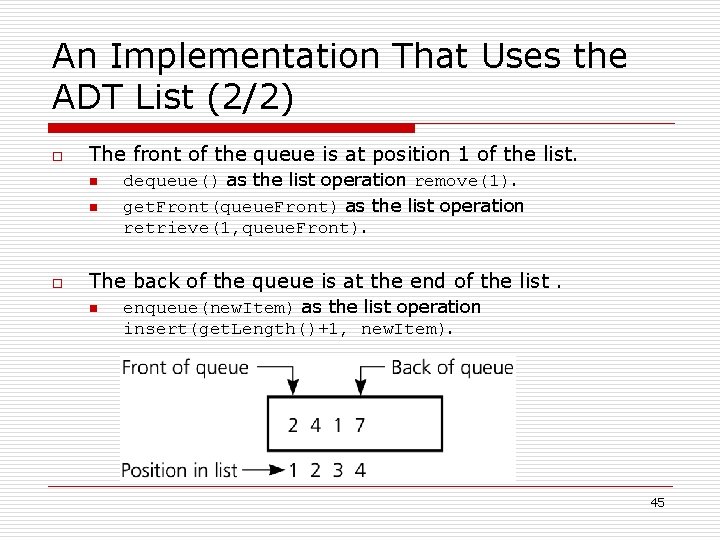
An Implementation That Uses the ADT List (2/2) o The front of the queue is at position 1 of the list. n n o dequeue() as the list operation remove(1). get. Front(queue. Front) as the list operation retrieve(1, queue. Front). The back of the queue is at the end of the list. n enqueue(new. Item) as the list operation insert(get. Length()+1, new. Item). 45
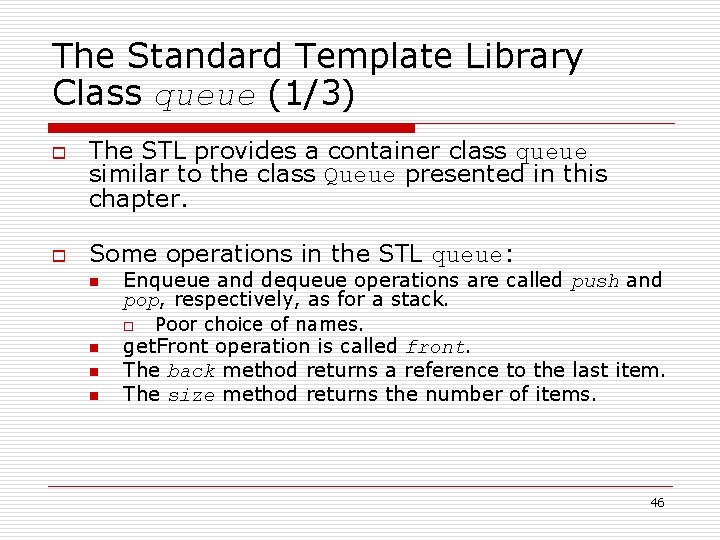
The Standard Template Library Class queue (1/3) o o The STL provides a container class queue similar to the class Queue presented in this chapter. Some operations in the STL queue: n Enqueue and dequeue operations are called push and pop, respectively, as for a stack. o n n n Poor choice of names. get. Front operation is called front. The back method returns a reference to the last item. The size method returns the number of items. 46
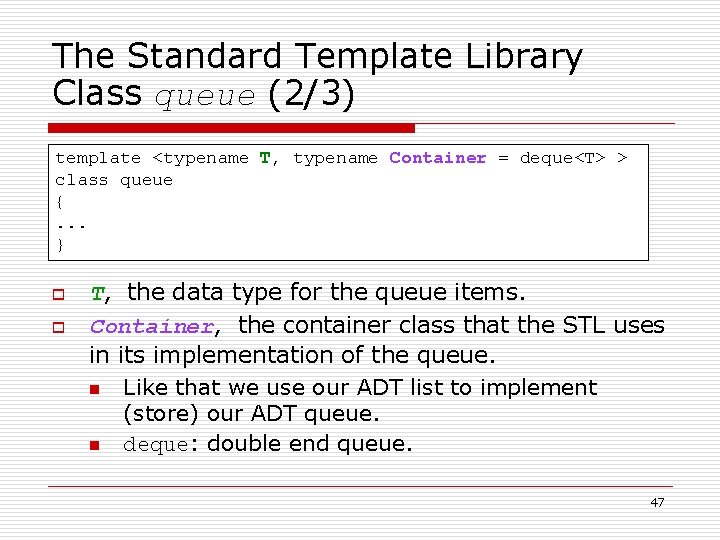
The Standard Template Library Class queue (2/3) template <typename T, typename Container = deque<T> > class queue {. . . } o o T, the data type for the queue items. Container, the container class that the STL uses in its implementation of the queue. n Like that we use our ADT list to implement (store) our ADT queue. n deque: double end queue. 47
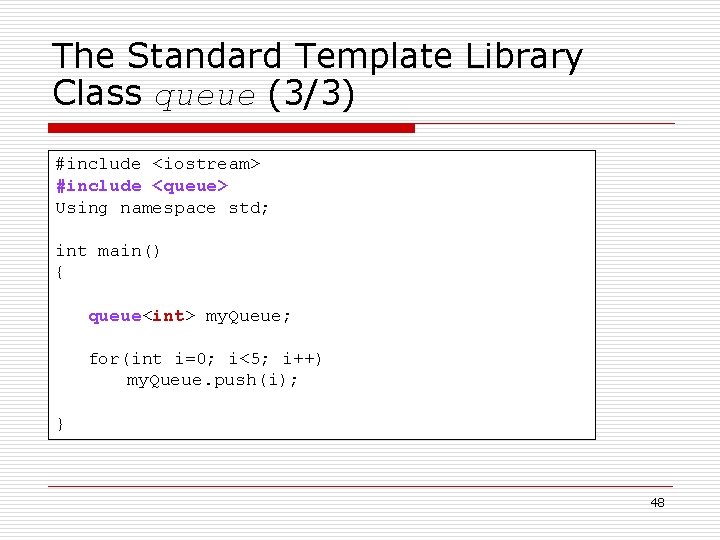
The Standard Template Library Class queue (3/3) #include <iostream> #include <queue> Using namespace std; int main() { queue<int> my. Queue; for(int i=0; i<5; i++) my. Queue. push(i); } 48
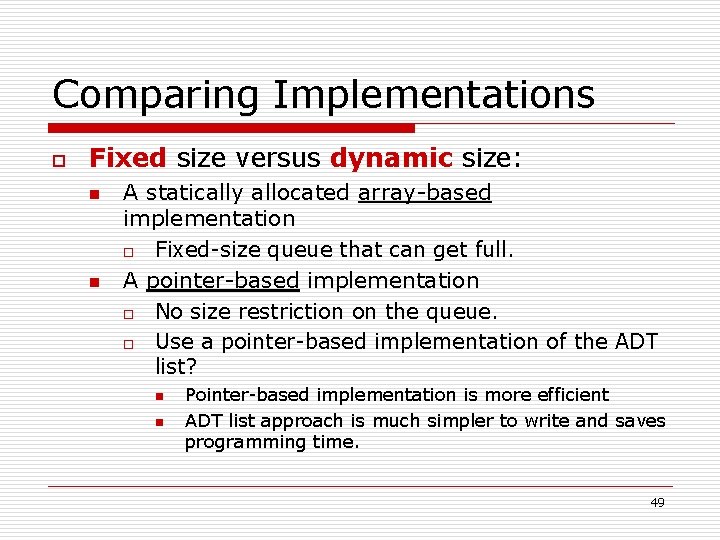
Comparing Implementations o Fixed size versus dynamic size: n n A statically allocated array-based implementation o Fixed-size queue that can get full. A pointer-based implementation o No size restriction on the queue. o Use a pointer-based implementation of the ADT list? n n Pointer-based implementation is more efficient ADT list approach is much simpler to write and saves programming time. 49
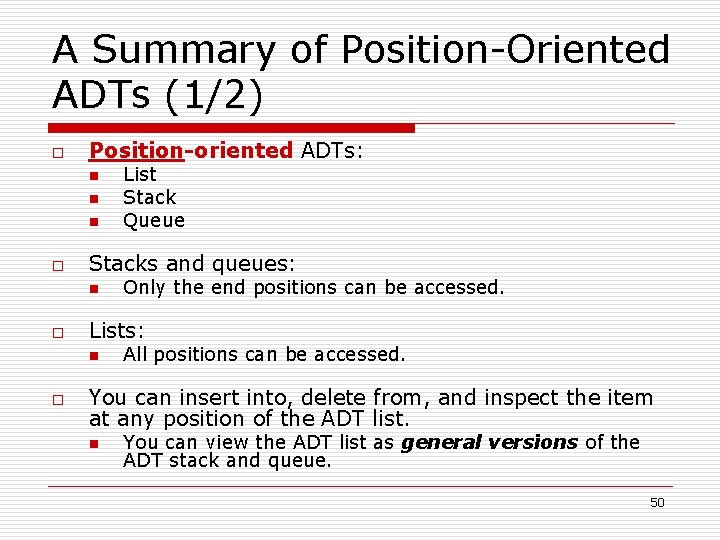
A Summary of Position-Oriented ADTs (1/2) o Position-oriented ADTs: n n n o Stacks and queues: n o Only the end positions can be accessed. Lists: n o List Stack Queue All positions can be accessed. You can insert into, delete from, and inspect the item at any position of the ADT list. n You can view the ADT list as general versions of the ADT stack and queue. 50
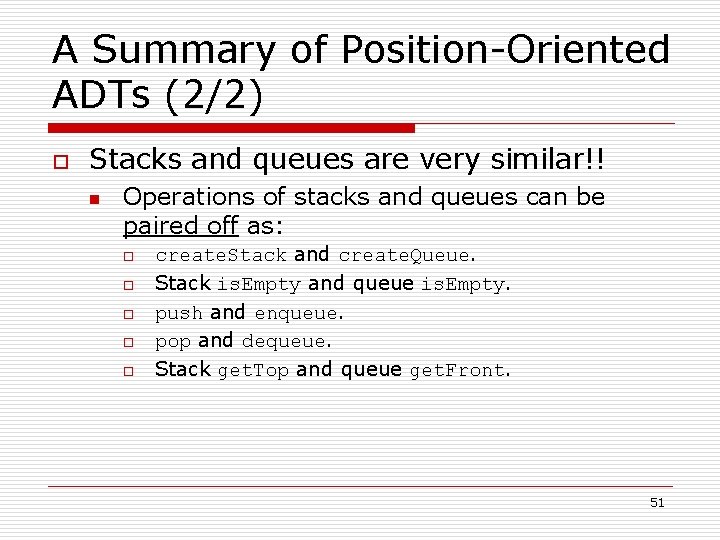
A Summary of Position-Oriented ADTs (2/2) o Stacks and queues are very similar!! n Operations of stacks and queues can be paired off as: o o o create. Stack and create. Queue. Stack is. Empty and queue is. Empty. push and enqueue. pop and dequeue. Stack get. Top and queue get. Front. 51
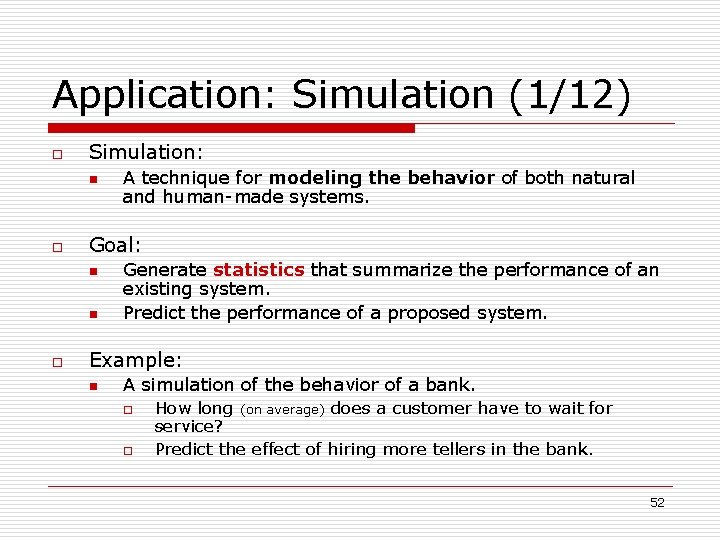
Application: Simulation (1/12) o Simulation: n o Goal: n n o A technique for modeling the behavior of both natural and human-made systems. Generate statistics that summarize the performance of an existing system. Predict the performance of a proposed system. Example: n A simulation of the behavior of a bank. o o How long (on average) does a customer have to wait for service? Predict the effect of hiring more tellers in the bank. 52
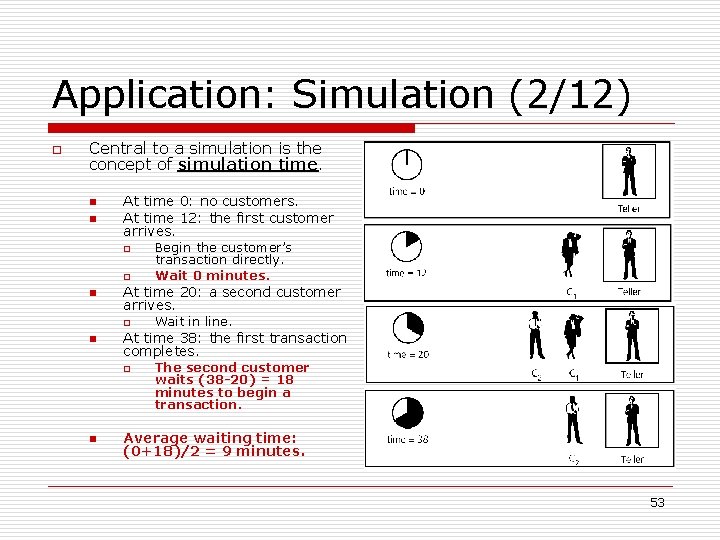
Application: Simulation (2/12) o Central to a simulation is the concept of simulation time. n n At time 0: no customers. At time 12: the first customer arrives. o o n At time 20: a second customer arrives. o n Wait in line. At time 38: the first transaction completes. o n Begin the customer’s transaction directly. Wait 0 minutes. The second customer waits (38 -20) = 18 minutes to begin a transaction. Average waiting time: (0+18)/2 = 9 minutes. 53
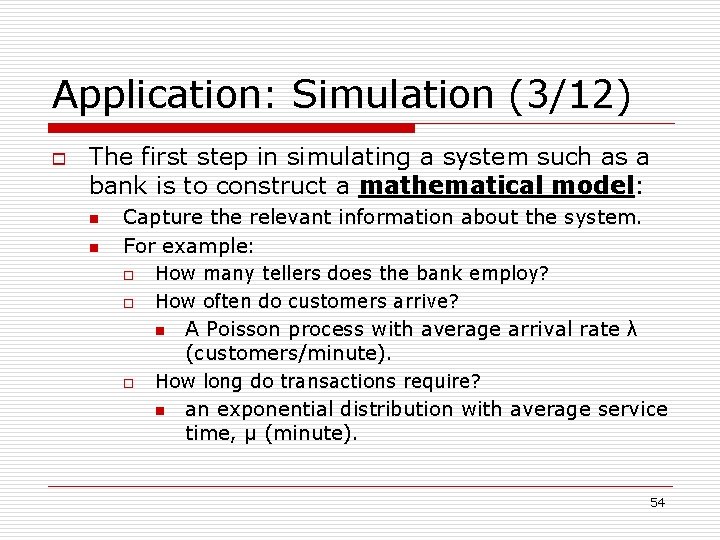
Application: Simulation (3/12) o The first step in simulating a system such as a bank is to construct a mathematical model: n n Capture the relevant information about the system. For example: o o How many tellers does the bank employ? How often do customers arrive? n o A Poisson process with average arrival rate λ (customers/minute). How long do transactions require? n an exponential distribution with average service time, μ (minute). 54
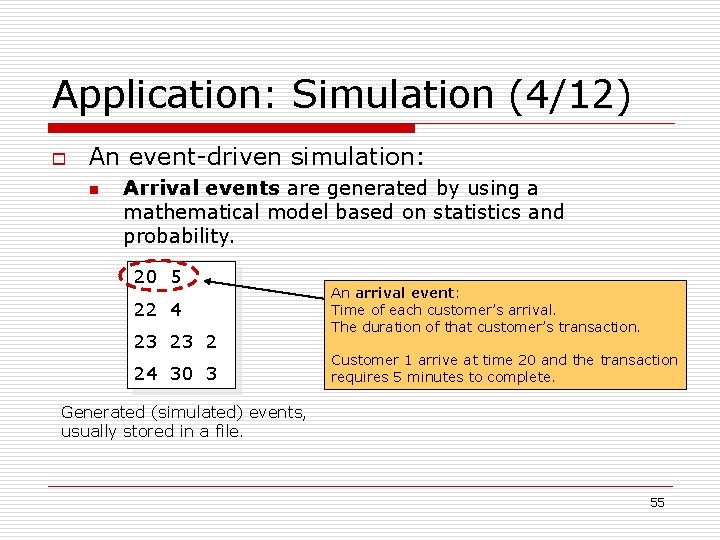
Application: Simulation (4/12) o An event-driven simulation: n Arrival events are generated by using a mathematical model based on statistics and probability. 20 5 22 4 23 23 2 24 30 3 An arrival event: Time of each customer’s arrival. The duration of that customer’s transaction. Customer 1 arrive at time 20 and the transaction requires 5 minutes to complete. Generated (simulated) events, usually stored in a file. 55
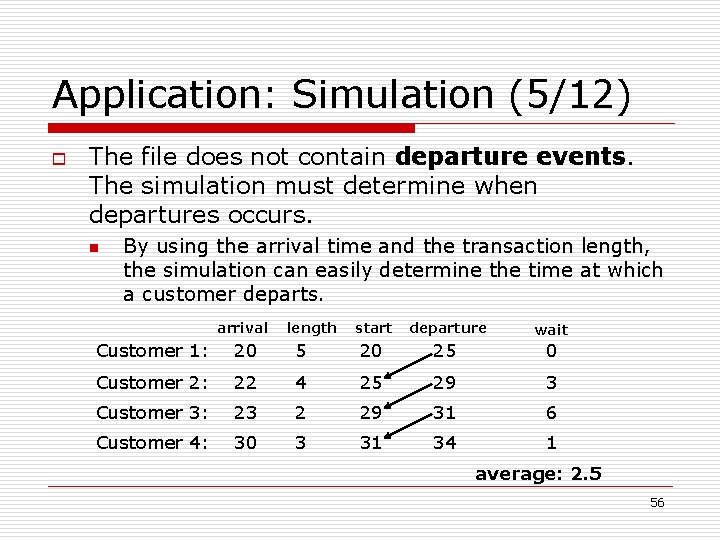
Application: Simulation (5/12) o The file does not contain departure events. The simulation must determine when departures occurs. n By using the arrival time and the transaction length, the simulation can easily determine the time at which a customer departs. arrival length start departure wait Customer 1: 20 5 20 25 0 Customer 2: 22 4 25 29 3 Customer 3: 23 2 29 31 6 Customer 4: 30 3 31 34 1 average: 2. 5 56
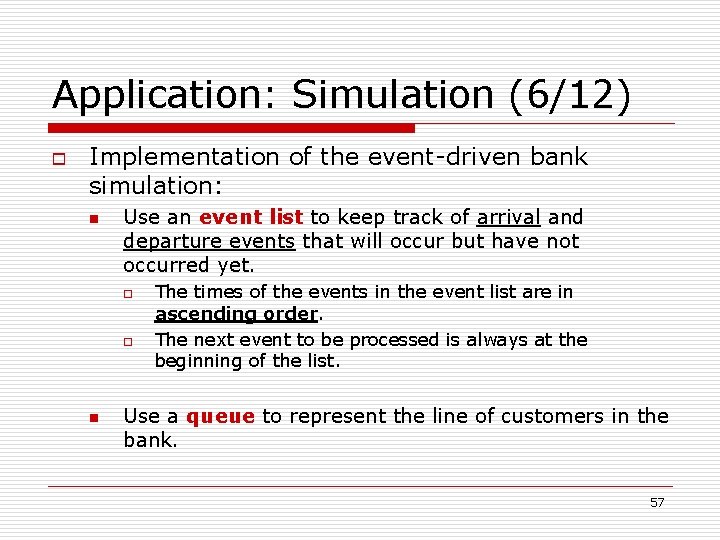
Application: Simulation (6/12) o Implementation of the event-driven bank simulation: n Use an event list to keep track of arrival and departure events that will occur but have not occurred yet. o o n The times of the events in the event list are in ascending order. The next event to be processed is always at the beginning of the list. Use a queue to represent the line of customers in the bank. 57
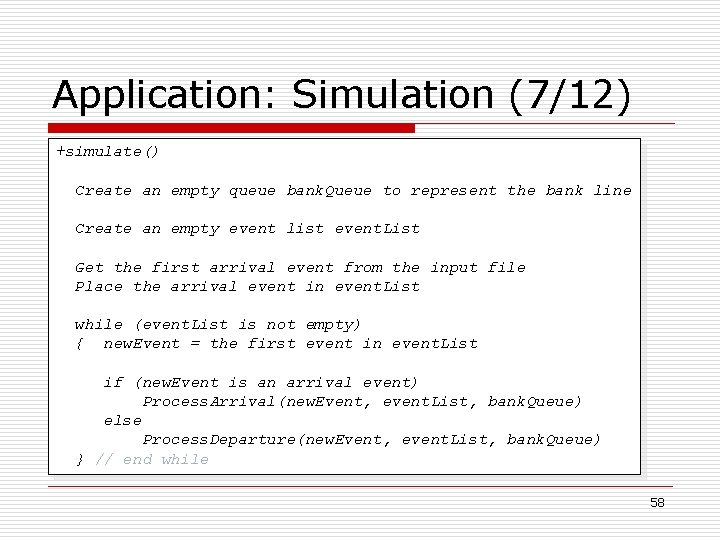
Application: Simulation (7/12) +simulate() Create an empty queue bank. Queue to represent the bank line Create an empty event list event. List Get the first arrival event from the input file Place the arrival event in event. List while (event. List is not empty) { new. Event = the first event in event. List if (new. Event is an arrival event) Process. Arrival(new. Event, event. List, bank. Queue) else Process. Departure(new. Event, event. List, bank. Queue) } // end while 58
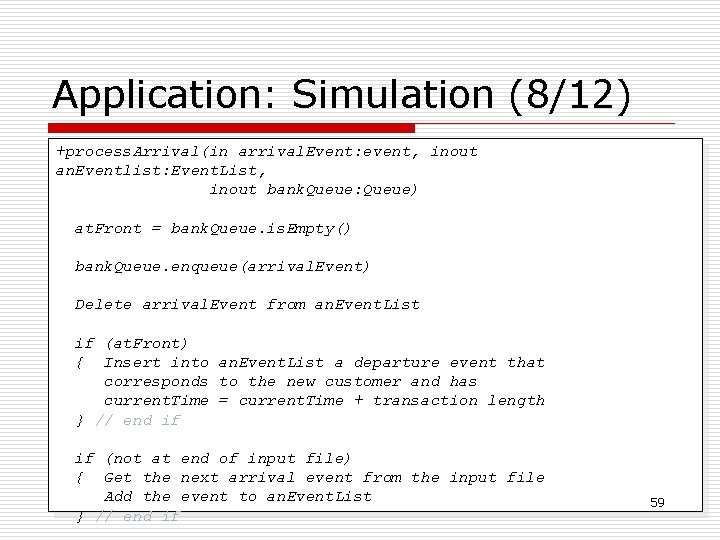
Application: Simulation (8/12) +process. Arrival(in arrival. Event: event, inout an. Eventlist: Event. List, inout bank. Queue: Queue) at. Front = bank. Queue. is. Empty() bank. Queue. enqueue(arrival. Event) Delete arrival. Event from an. Event. List if (at. Front) { Insert into an. Event. List a departure event that corresponds to the new customer and has current. Time = current. Time + transaction length } // end if if (not at end of input file) { Get the next arrival event from the input file Add the event to an. Event. List } // end if 59
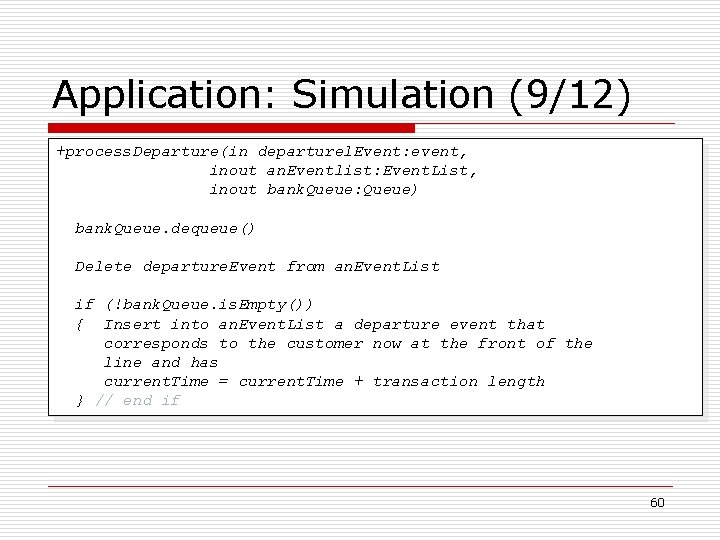
Application: Simulation (9/12) +process. Departure(in departurel. Event: event, inout an. Eventlist: Event. List, inout bank. Queue: Queue) bank. Queue. dequeue() Delete departure. Event from an. Event. List if (!bank. Queue. is. Empty()) { Insert into an. Event. List a departure event that corresponds to the customer now at the front of the line and has current. Time = current. Time + transaction length } // end if 60
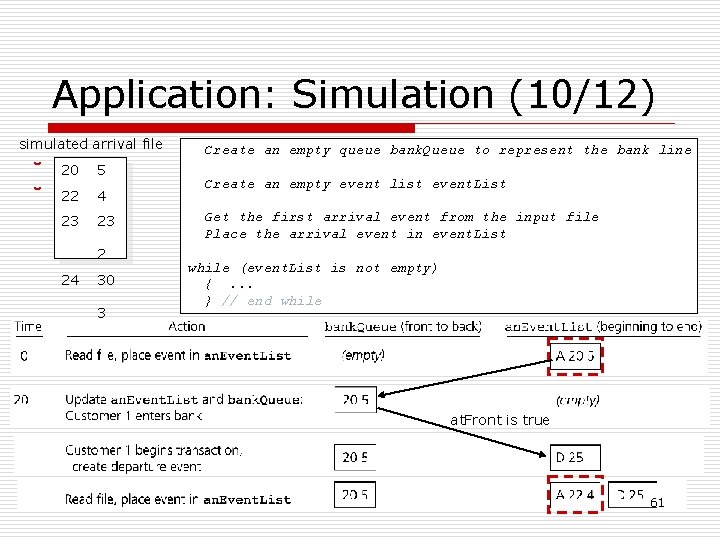
Application: Simulation (10/12) simulated arrival file ˇ 20 ˇ 22 23 5 4 23 2 24 30 3 Create an empty queue bank. Queue to represent the bank line Create an empty event list event. List Get the first arrival event from the input file Place the arrival event in event. List while (event. List is not empty) {. . . } // end while at. Front is true 61
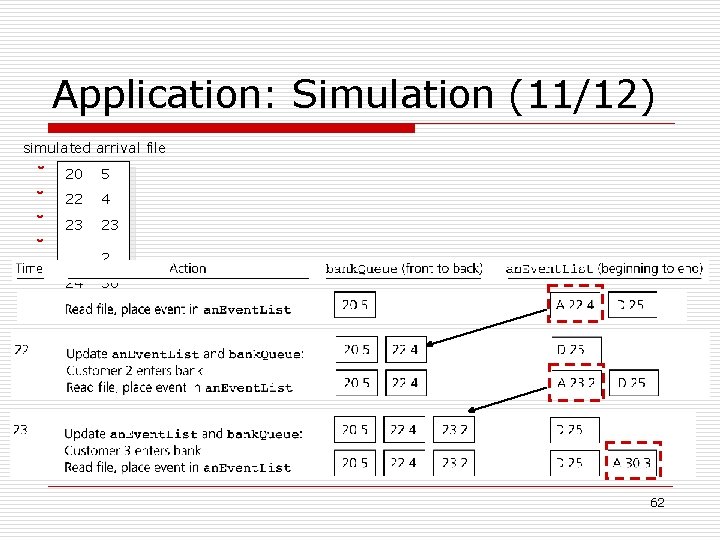
Application: Simulation (11/12) simulated arrival file ˇ 20 ˇ 22 ˇ 23 ˇ 24 5 4 23 2 30 3 62
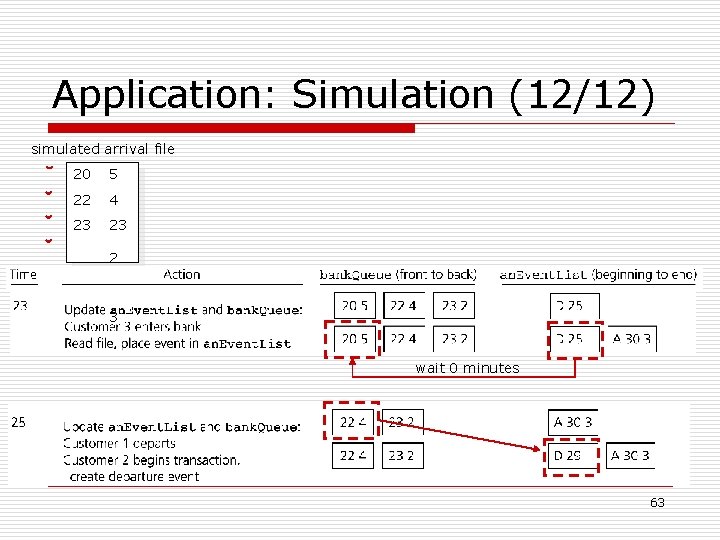
Application: Simulation (12/12) simulated arrival file ˇ 20 ˇ 22 ˇ 23 ˇ 24 5 4 23 2 30 3 wait 0 minutes 63
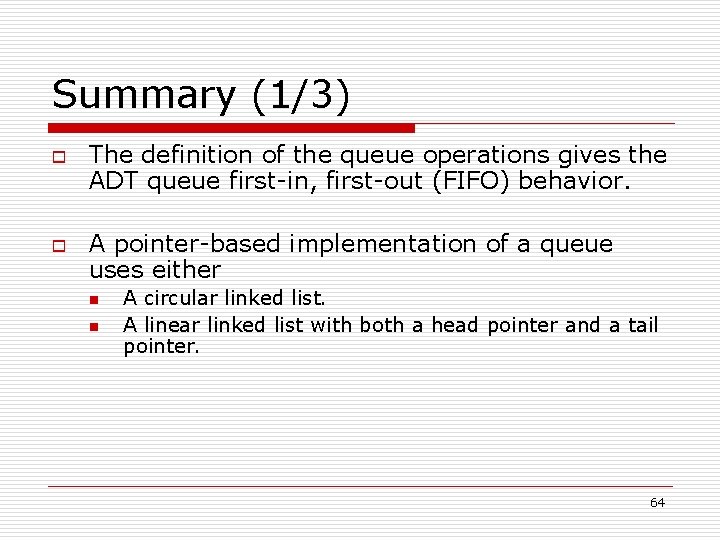
Summary (1/3) o o The definition of the queue operations gives the ADT queue first-in, first-out (FIFO) behavior. A pointer-based implementation of a queue uses either n n A circular linked list. A linear linked list with both a head pointer and a tail pointer. 64
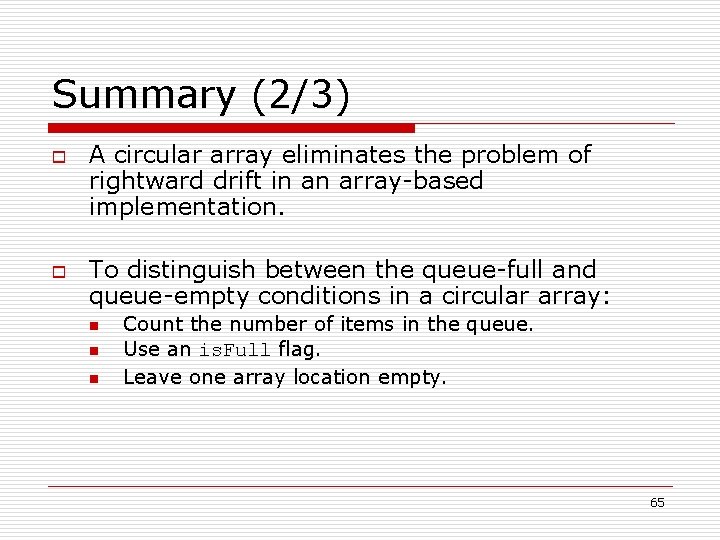
Summary (2/3) o o A circular array eliminates the problem of rightward drift in an array-based implementation. To distinguish between the queue-full and queue-empty conditions in a circular array: n n n Count the number of items in the queue. Use an is. Full flag. Leave one array location empty. 65
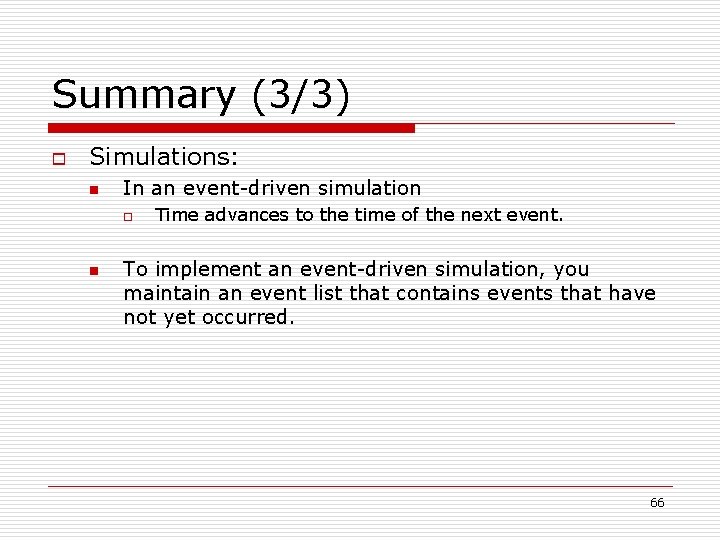
Summary (3/3) o Simulations: n In an event-driven simulation o n Time advances to the time of the next event. To implement an event-driven simulation, you maintain an event list that contains events that have not yet occurred. 66
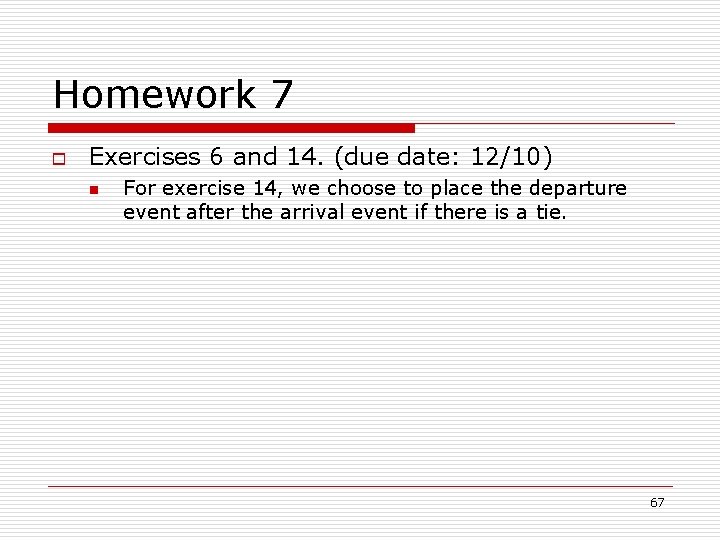
Homework 7 o Exercises 6 and 14. (due date: 12/10) n For exercise 14, we choose to place the departure event after the arrival event if there is a tie. 67
Brenda chin mei chen
Chen chen berlin
Python stack and queue
Priority queues: quiz
Java stacks and queues
Representation of queues
Exercises on stacks and queues
Message queues in unix
Priority queue adt
Pipes in rtos
Applications of priority queues
Struktur data queue sering digunakan untuk
Queue head tail
Mgh
Java stacks and queues
Chien de garde chapter 4
Máu chiên bò chúa không ưng
Brassai
Roussette chien de mer
Joanne chien
Sang de licorne harry potter
Un chien andalou
Ton animal
Position chien de fusil
Máu chiên bò chúa không ưng
Chúa chiến thắng khải hoàn
Chúa chiên lành người thương dẫn tôi đi
Dyssocialisation primaire chien
Eric chien symantec
Glande zygomatique chien
Programme de la tlvision ce soir
Sơ đồ chiến dịch điện biên phủ
Pancreatite anatomia
Zaira masood
Conduite à tenir devant une morsure de chien ppt
Chiến lược marketing của lg
Album photo chien
Chiến lược so st wo wt của vinamilk
Chiến thắng biên giới thu đông 1950 violet
Raymond marcillac et son chien
Walmart thất bại ở nhật
Biti's
Chiến lược xuyên quốc gia của ikea
Lepto chien
Anagramme aigle
Andy chien
Socialisation du chien
Cholangite lymphocytaire chat
Chiến thắng mtao mxây
Teyu chien
Morphophysiologie
Jai un chien
Test qi chien
French feminine and masculine nouns
Chien-chung shen
Narcolepsie cheval
Are freckles recessive
Cristen chin model
Profesi menurut chin yakobus
Head tilt chin lift jaw thrust
Bài hát ngoài đồng lúa chín thơm
Head tilt chin lift
Mento posterior face presentation
Chin reflex
Drawidzi
Head tilt chin lift jaw thrust
What are the 5 elements of hair design
Chin genetics