Chapter 10 Getting Started with Graphics Programming Graphics
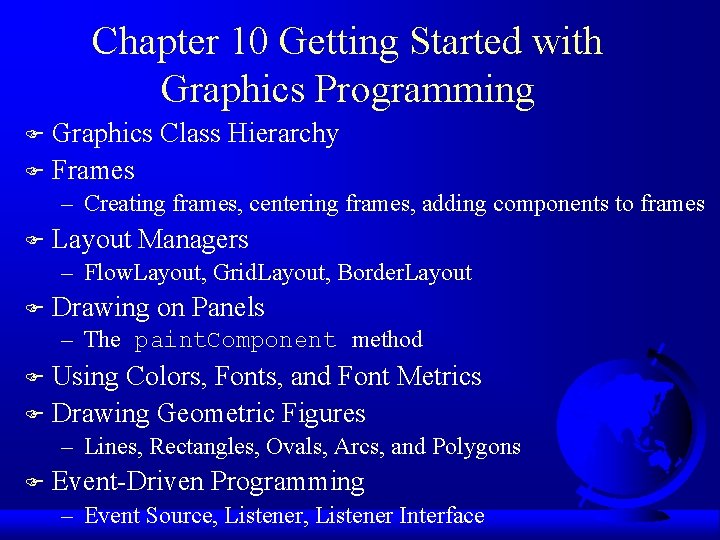
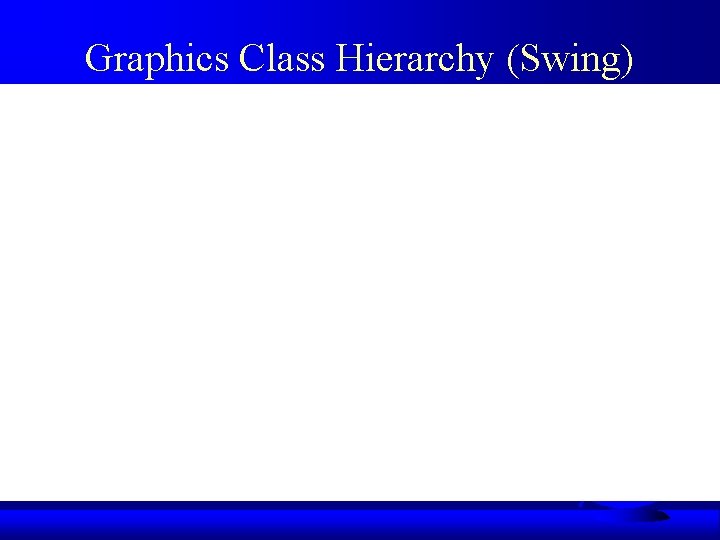
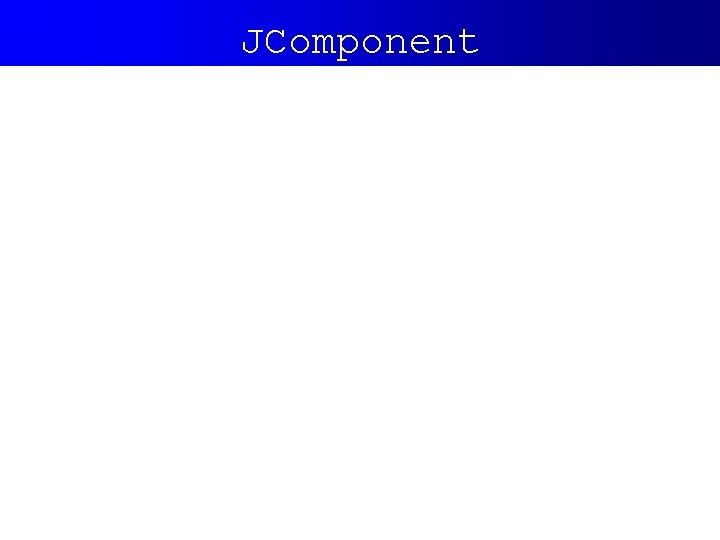
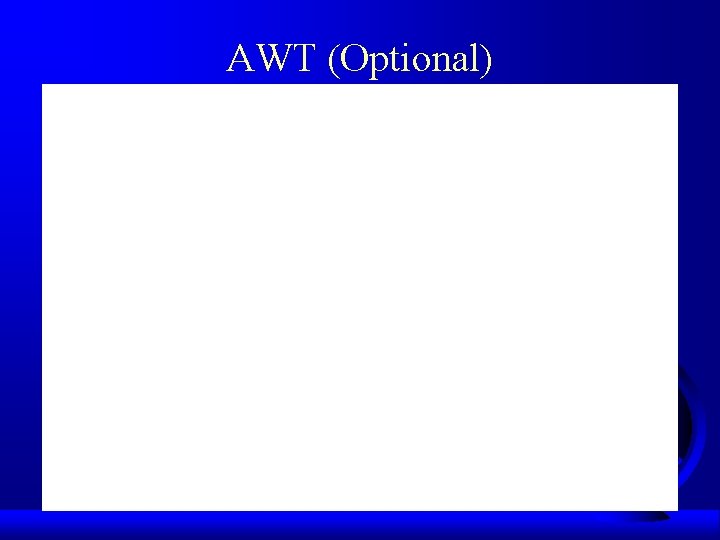
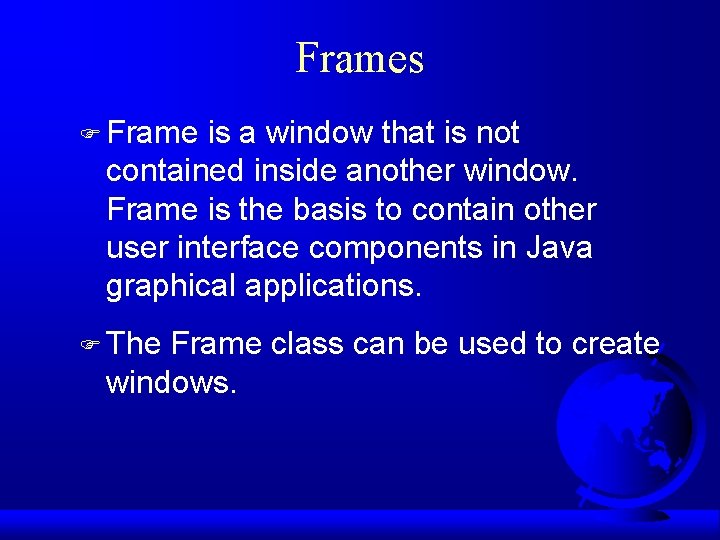
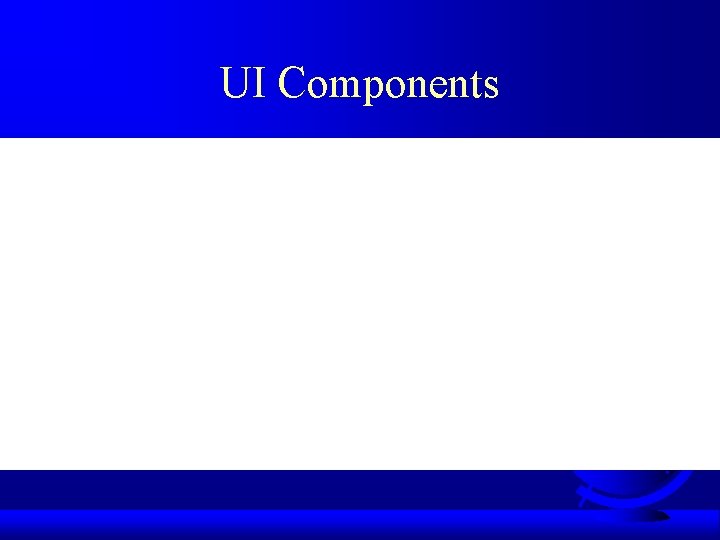
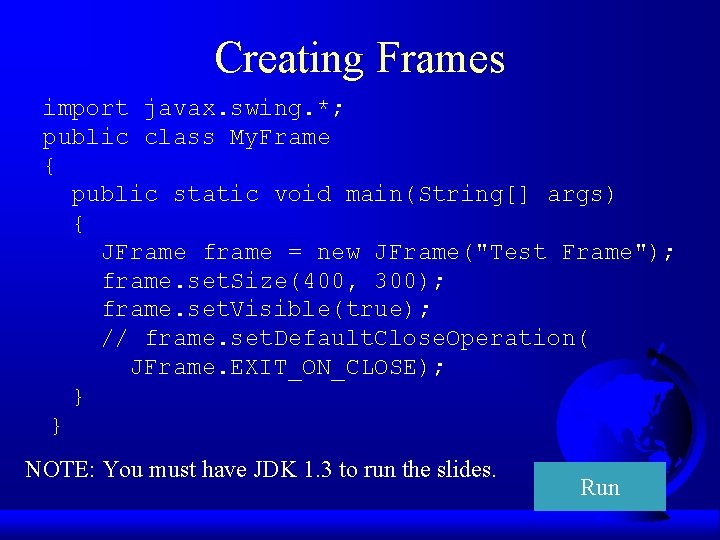
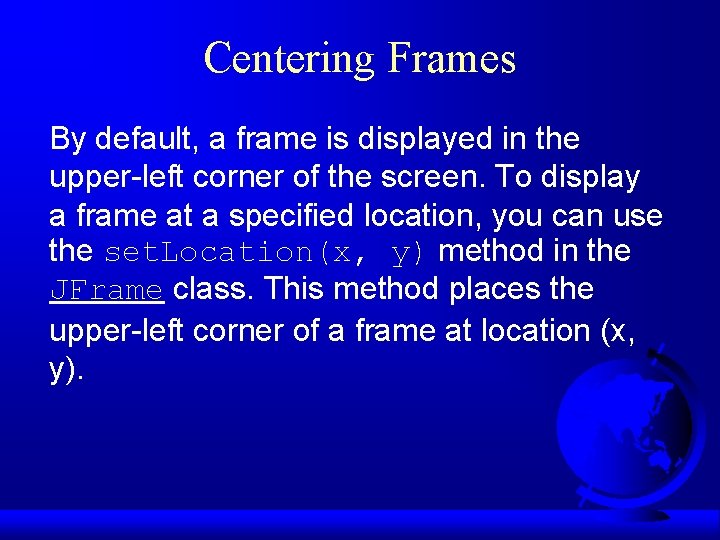
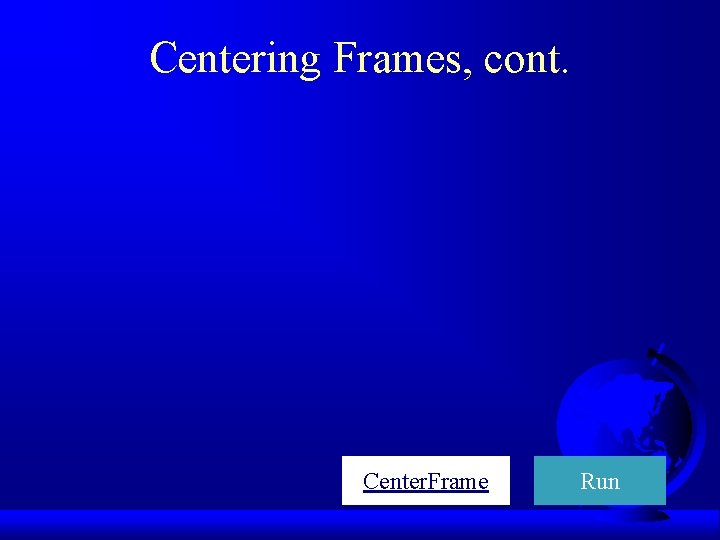
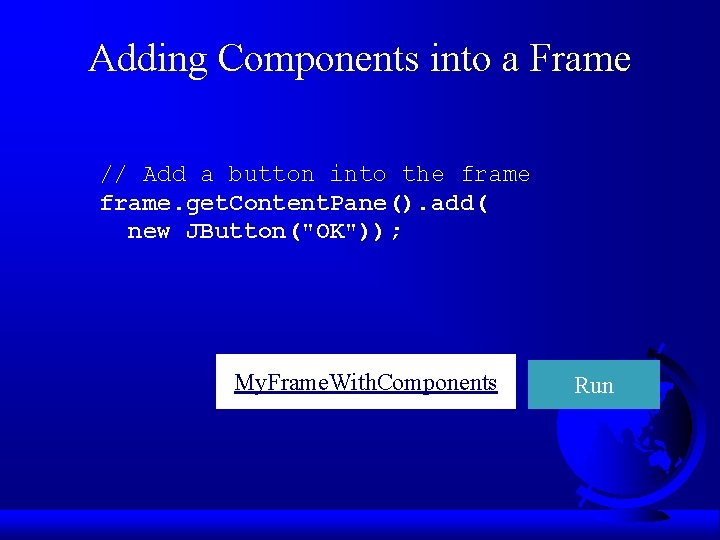
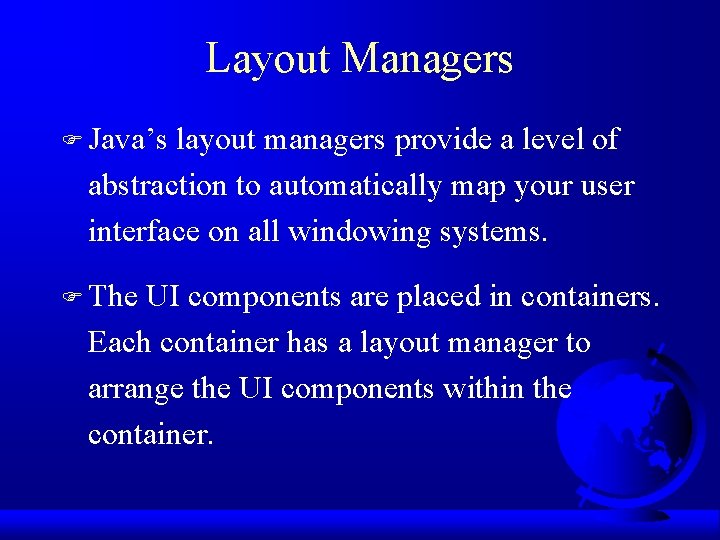
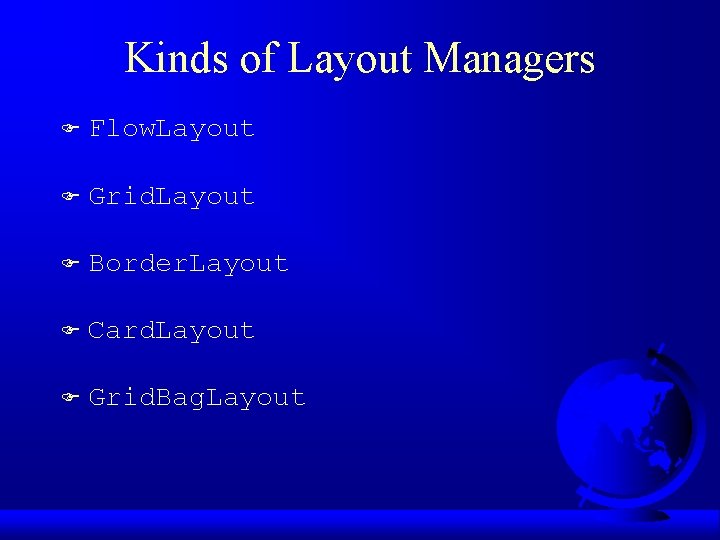
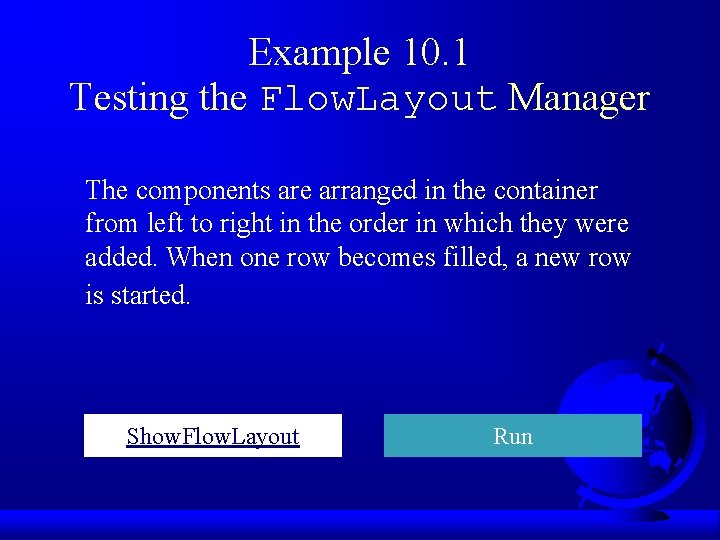
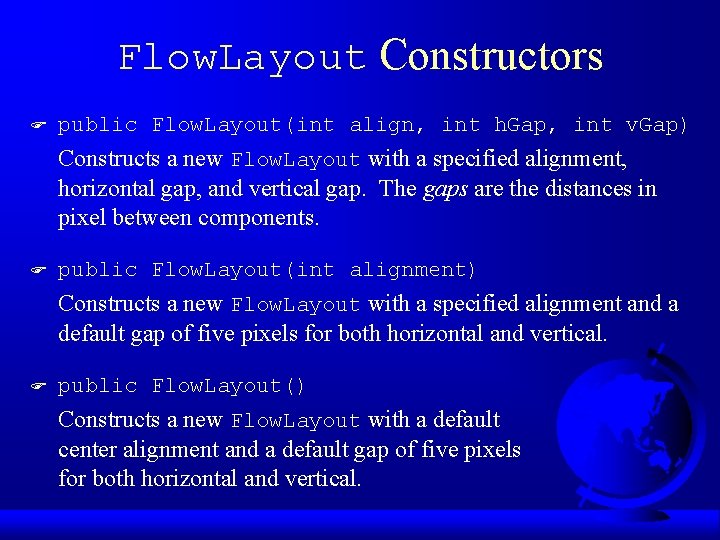
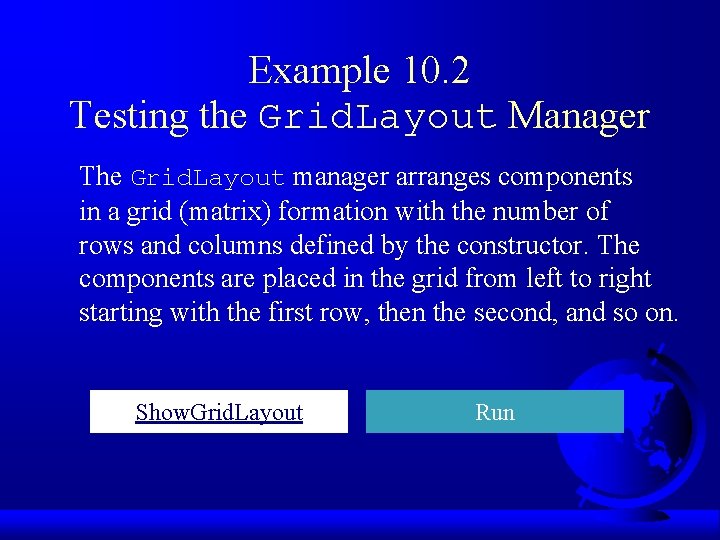
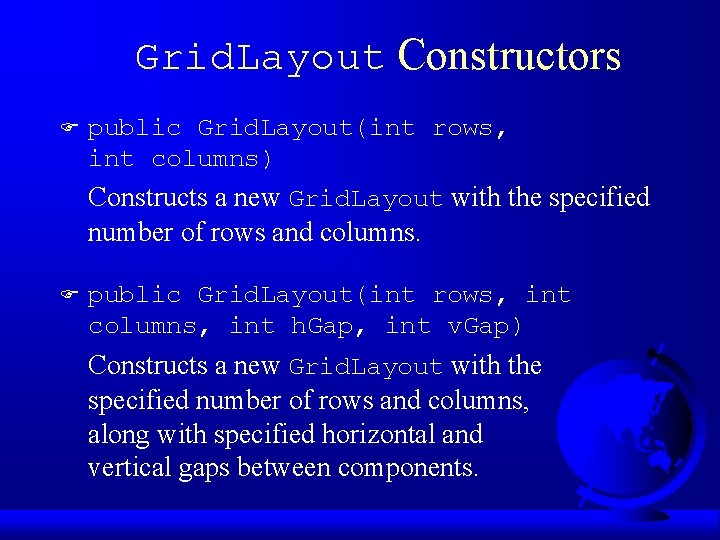
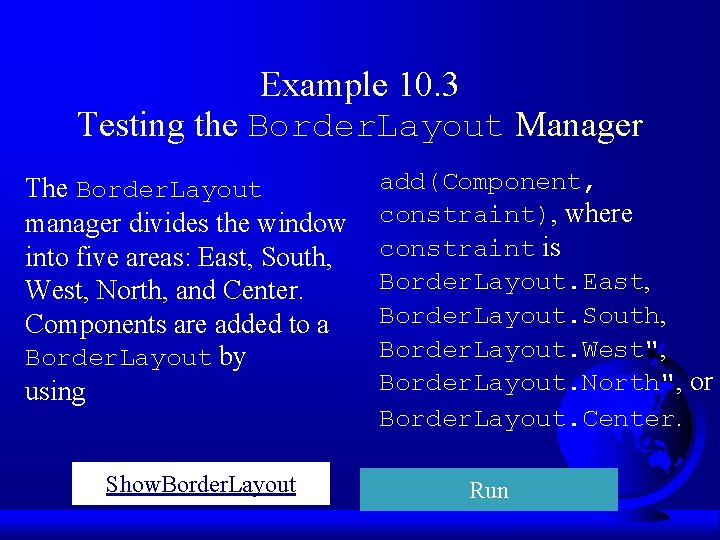
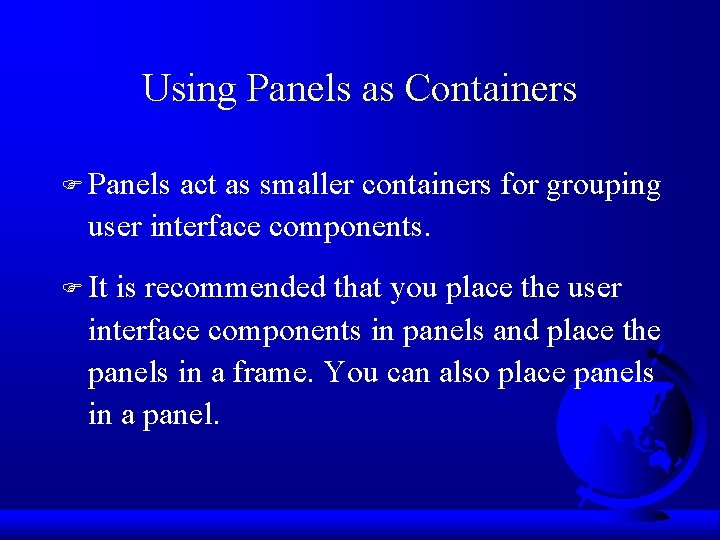
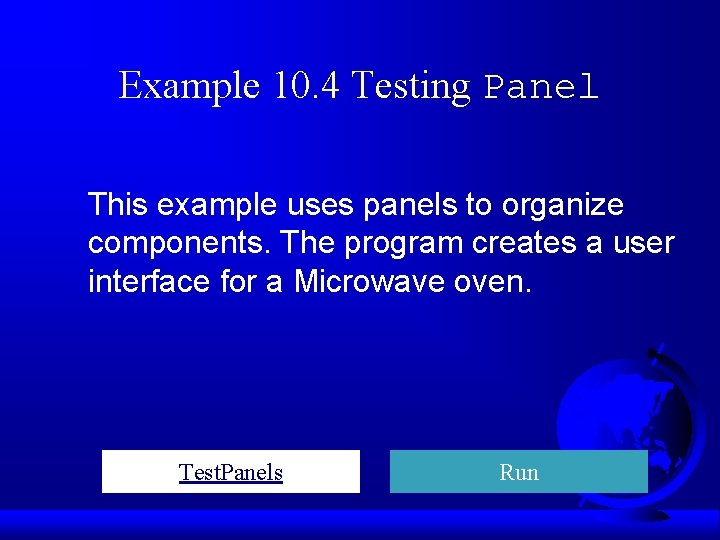
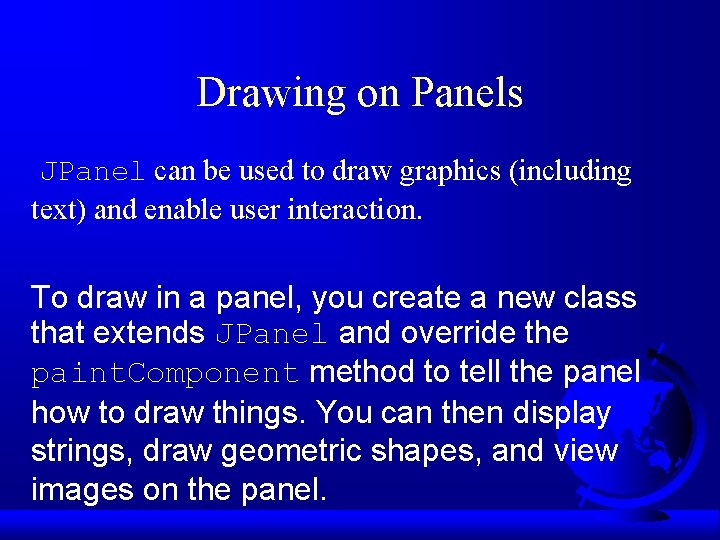
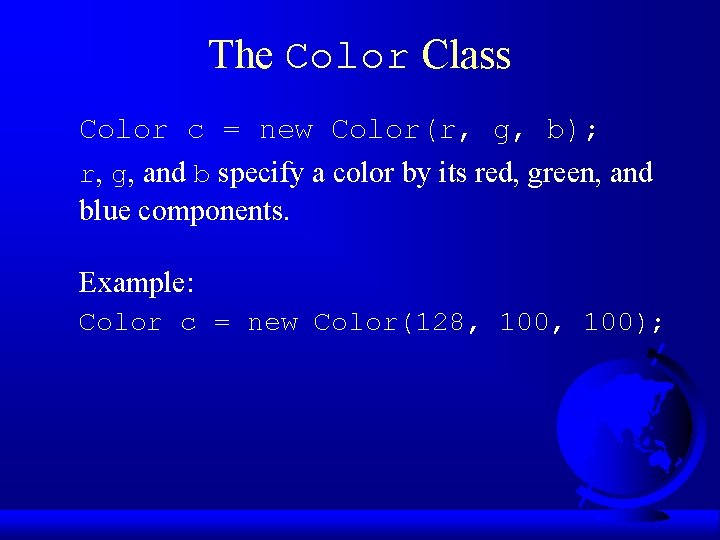
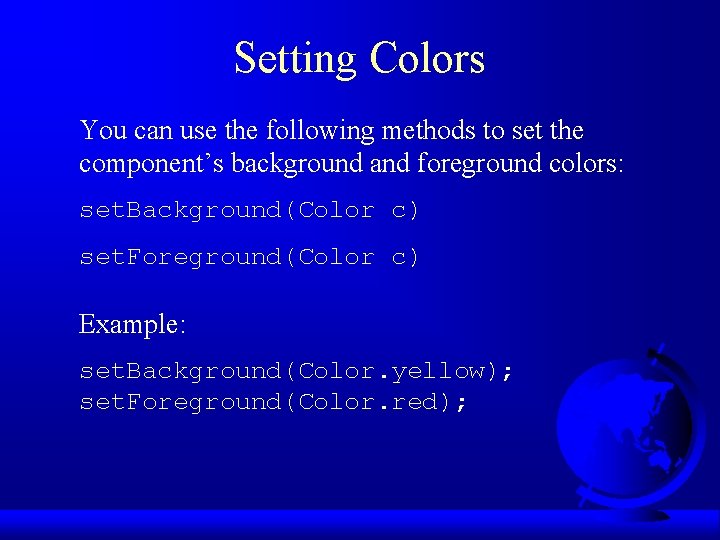
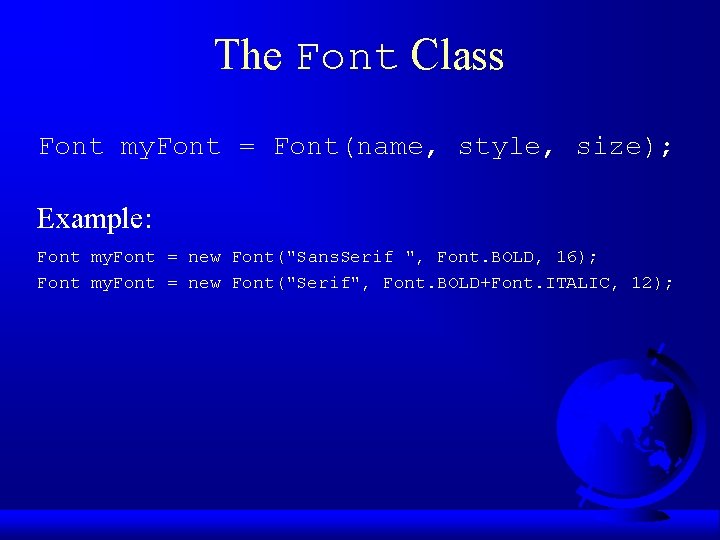
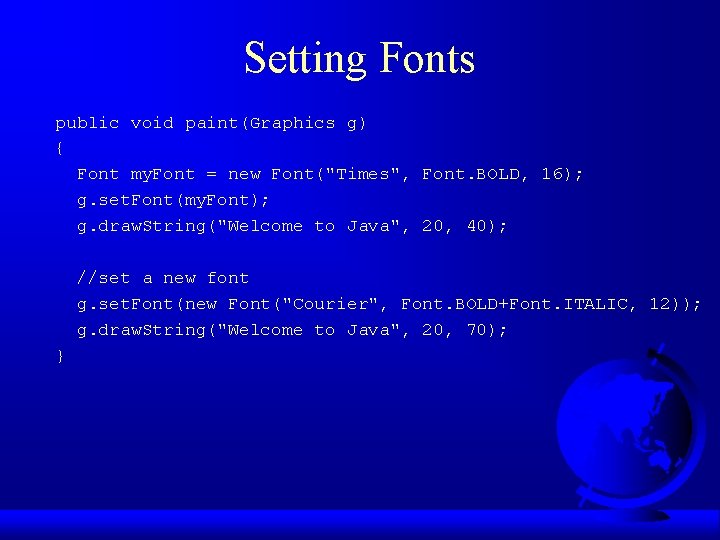
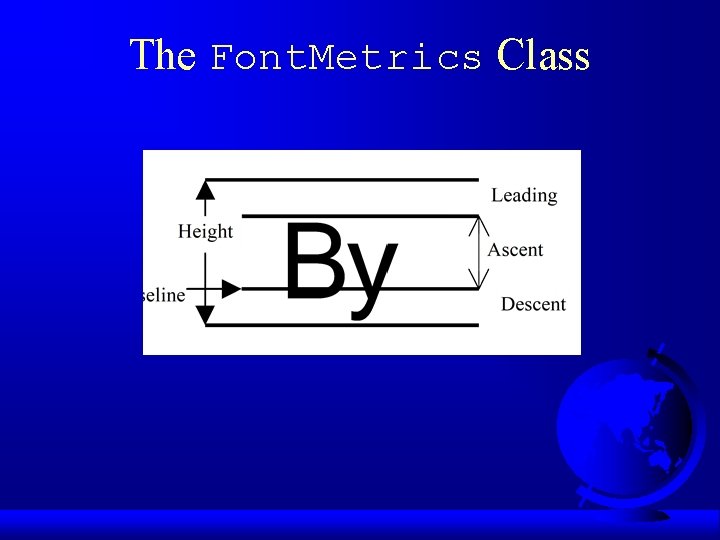
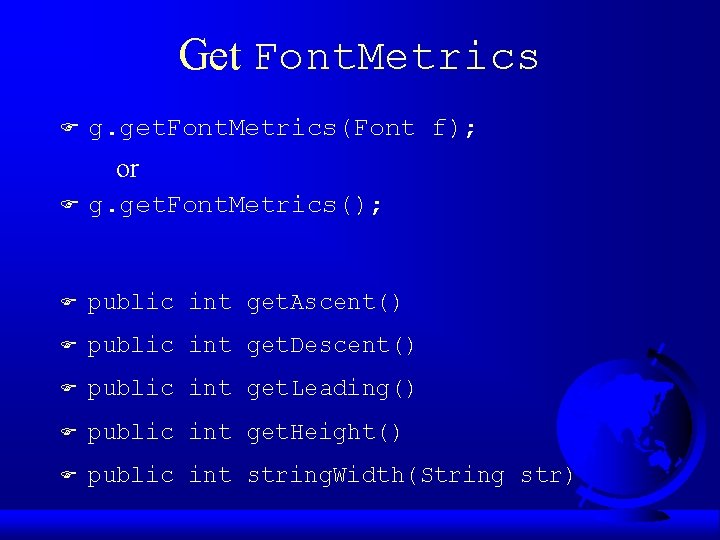
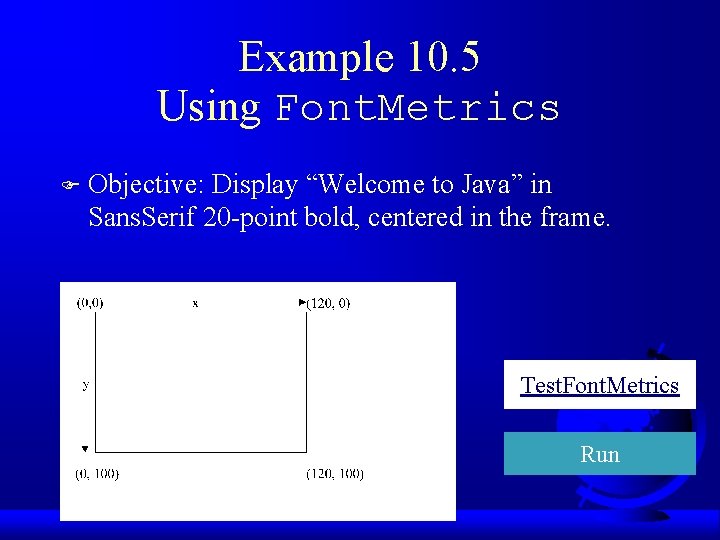
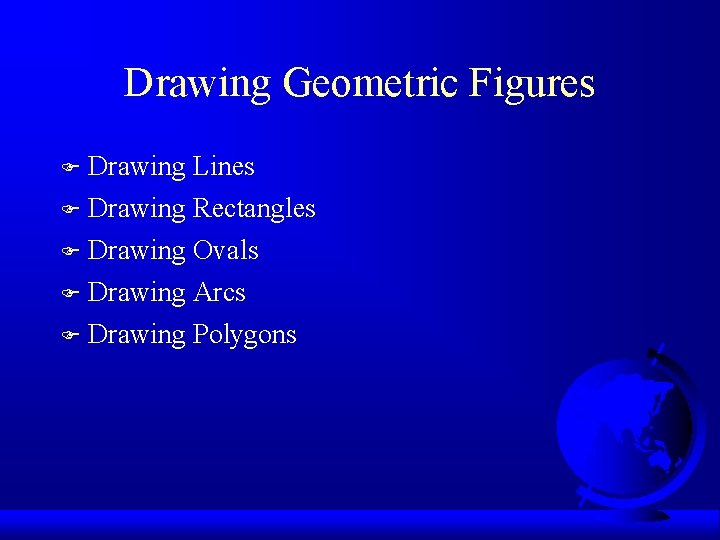
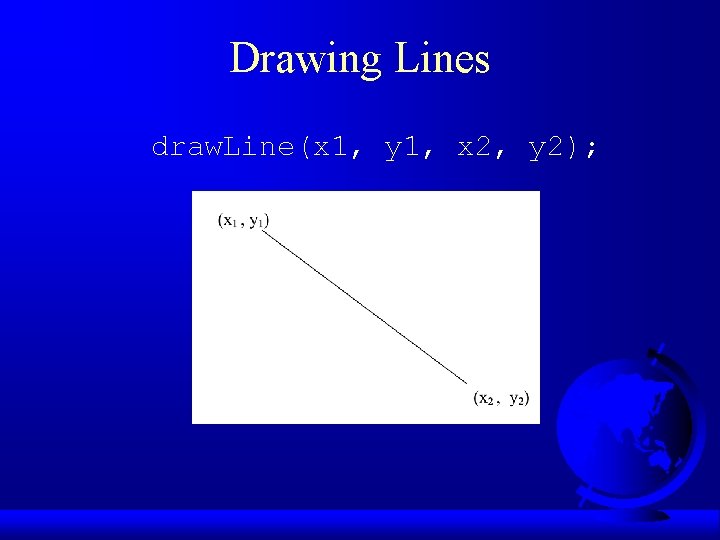
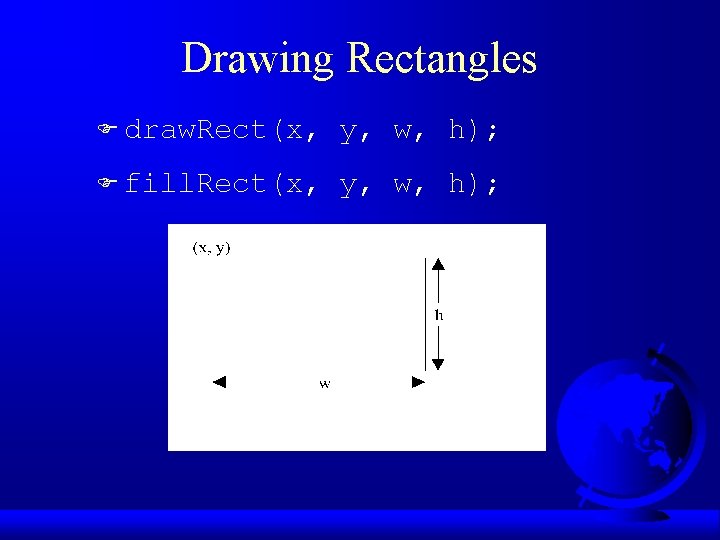
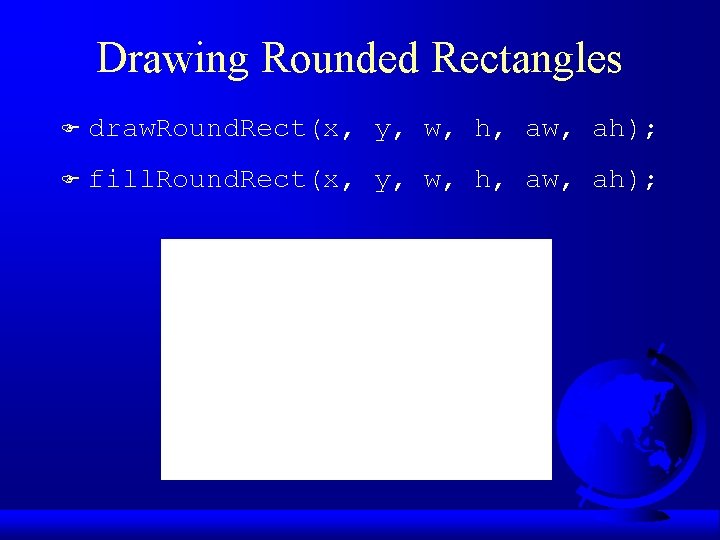
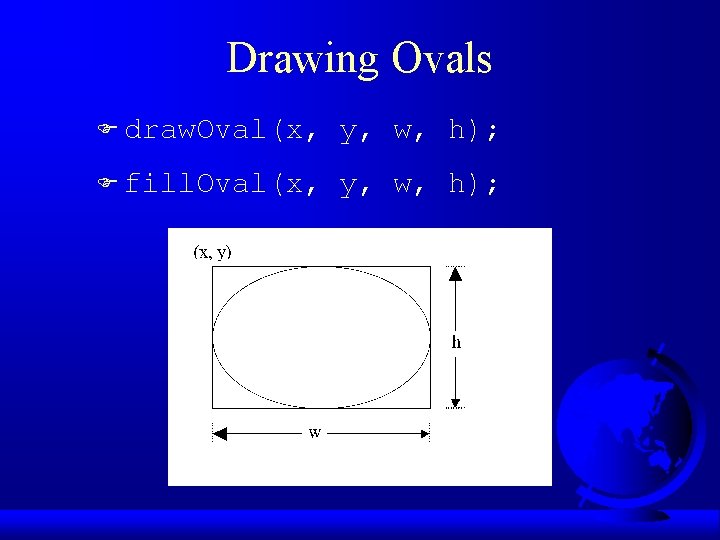
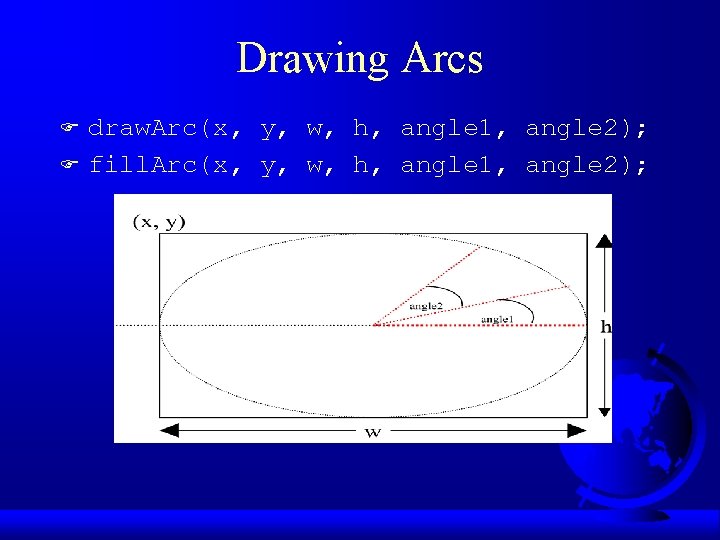
![Drawing Polygons int[] x = {40, 70, 60, 45, 20}; int[] y = {20, Drawing Polygons int[] x = {40, 70, 60, 45, 20}; int[] y = {20,](https://slidetodoc.com/presentation_image/7995e938e89268e5376fb8afc6923bb9/image-34.jpg)
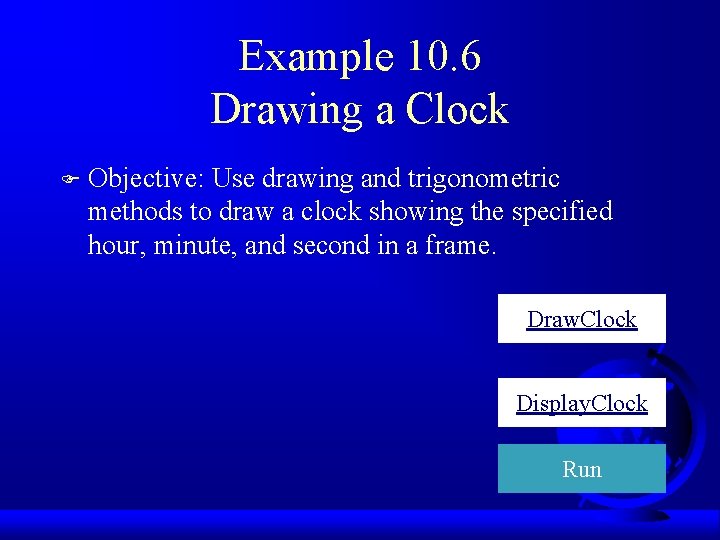
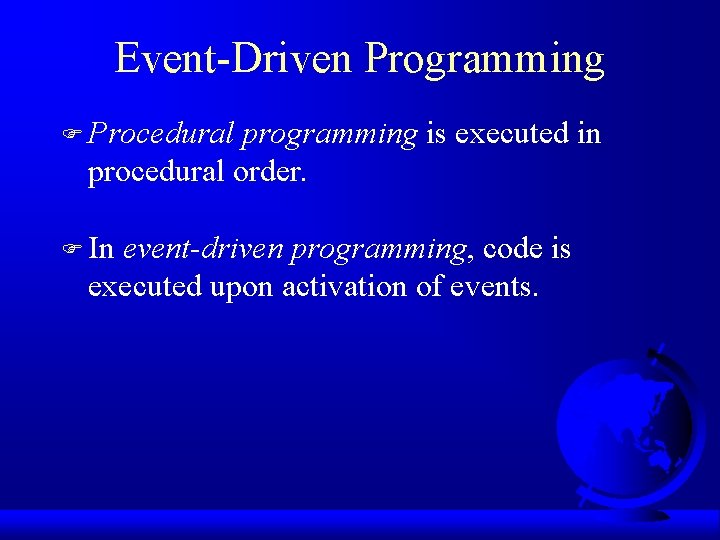
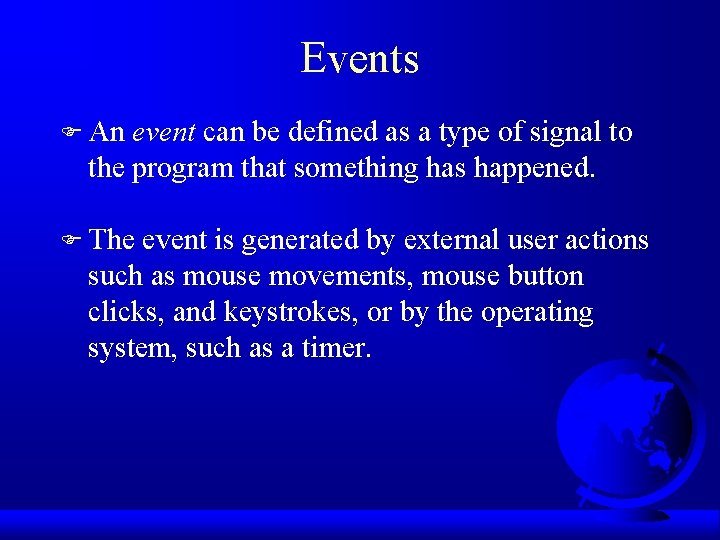
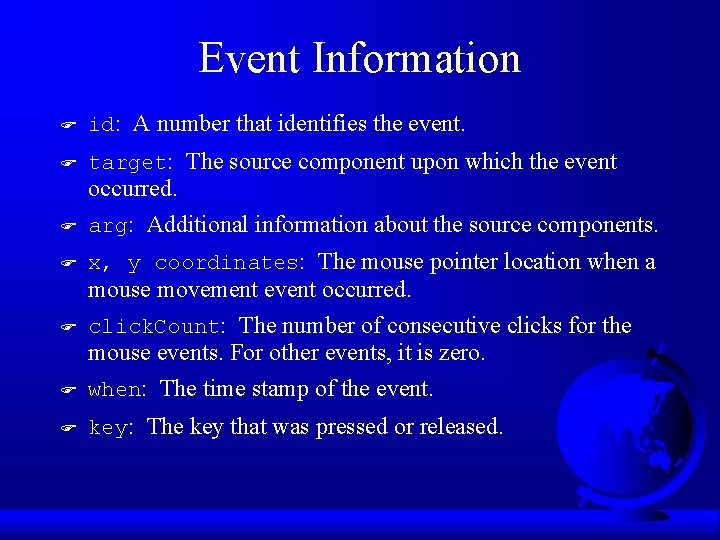
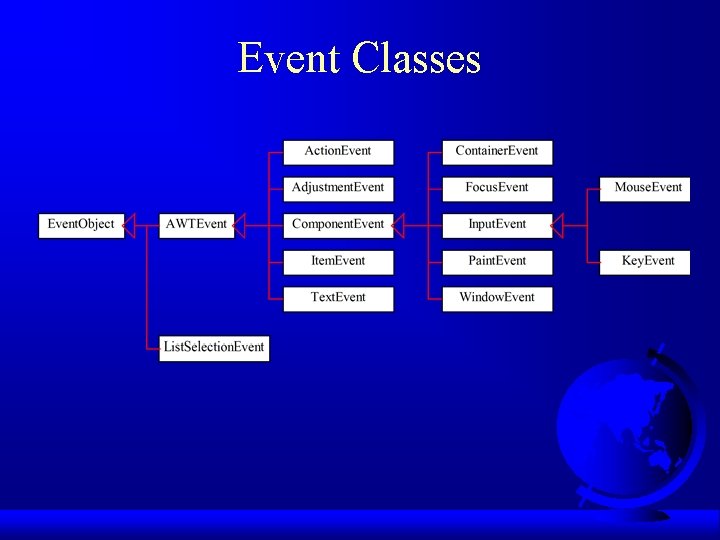
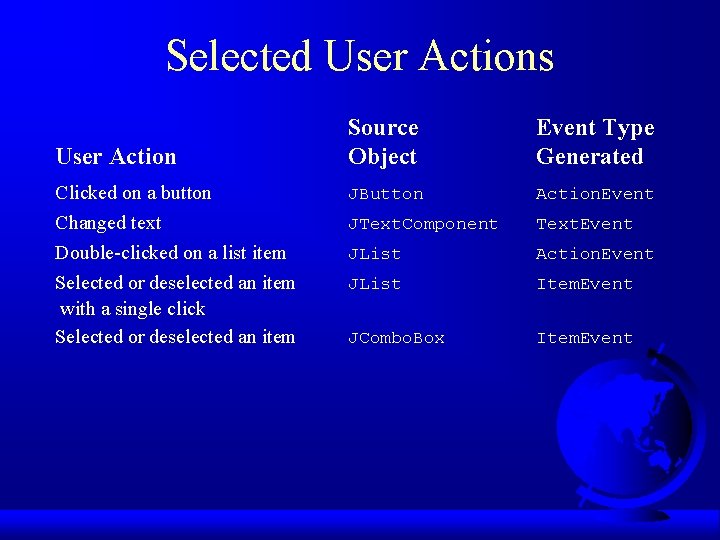
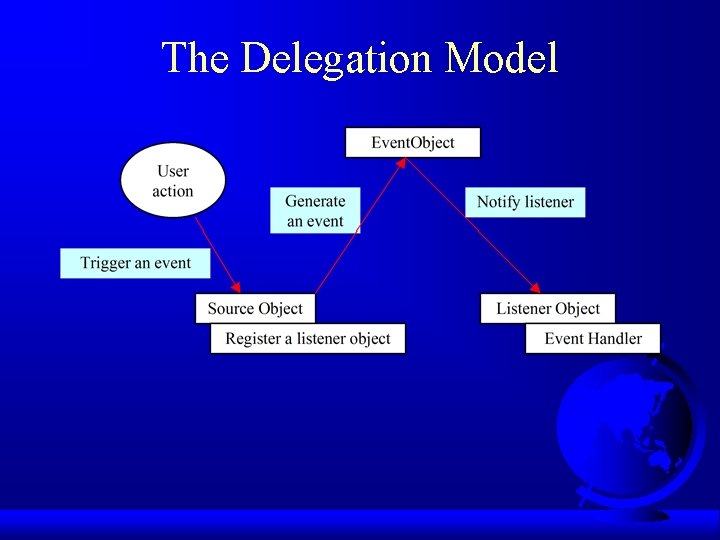
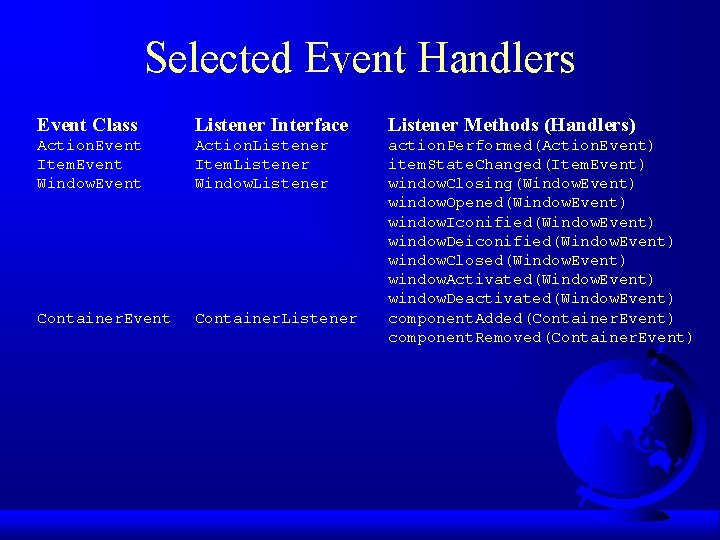
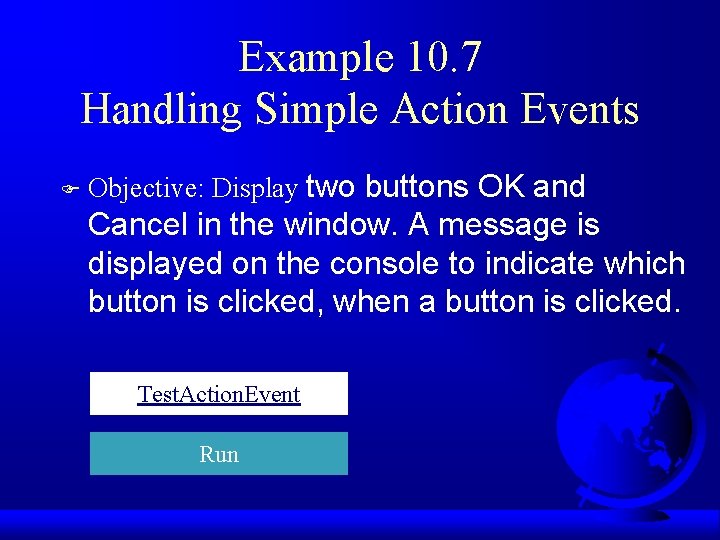
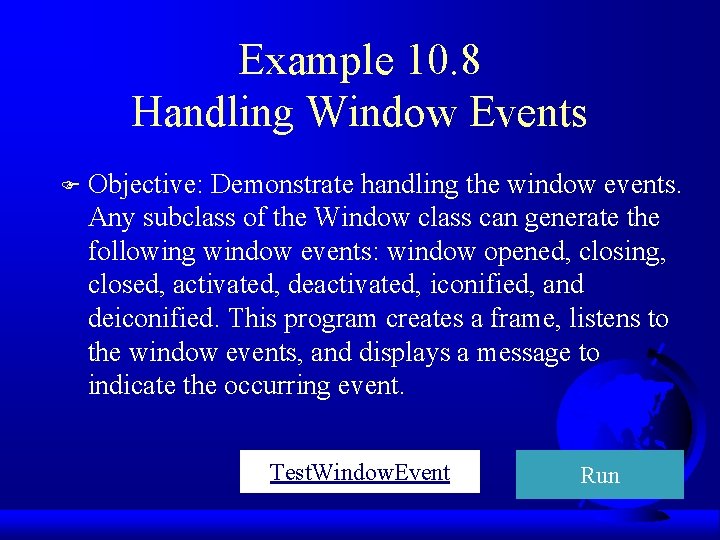
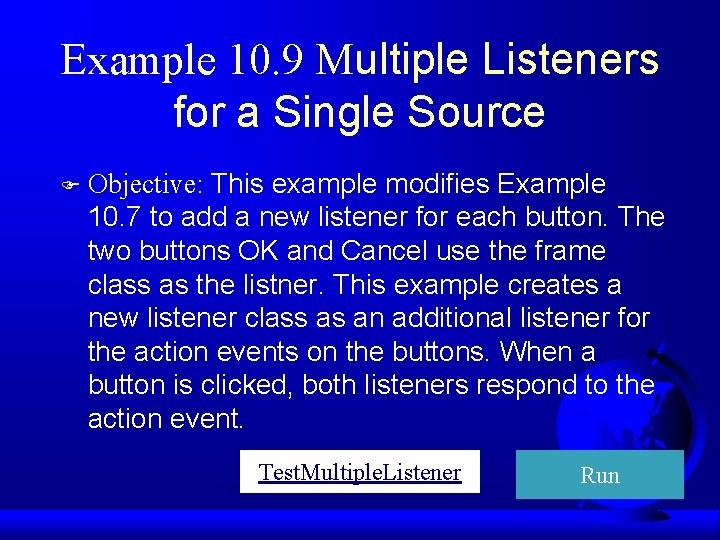
- Slides: 45
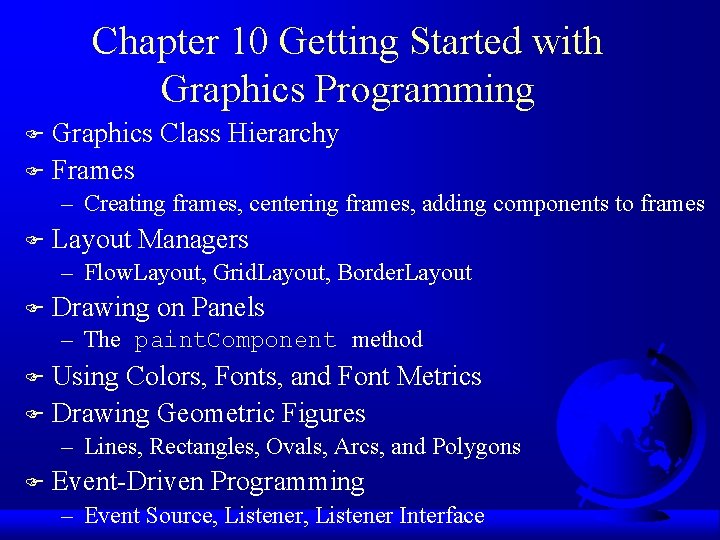
Chapter 10 Getting Started with Graphics Programming Graphics Class Hierarchy F Frames F – Creating frames, centering frames, adding components to frames F Layout Managers – Flow. Layout, Grid. Layout, Border. Layout F Drawing on Panels – The paint. Component method Using Colors, Fonts, and Font Metrics F Drawing Geometric Figures F – Lines, Rectangles, Ovals, Arcs, and Polygons F Event-Driven Programming – Event Source, Listener Interface
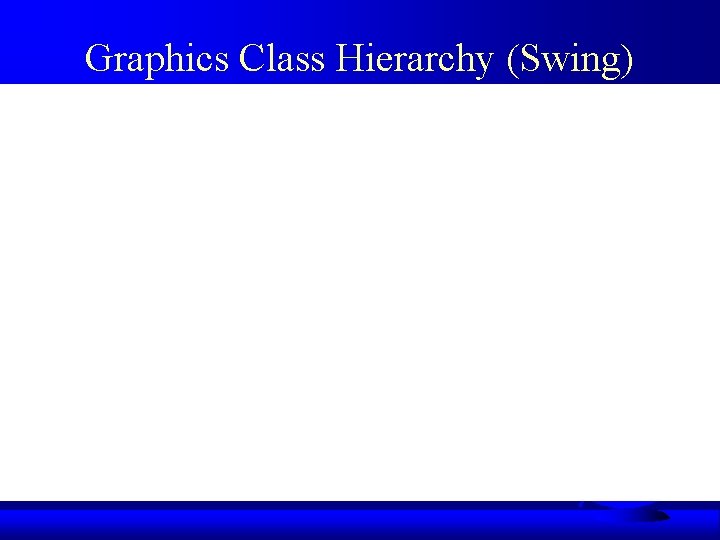
Graphics Class Hierarchy (Swing)
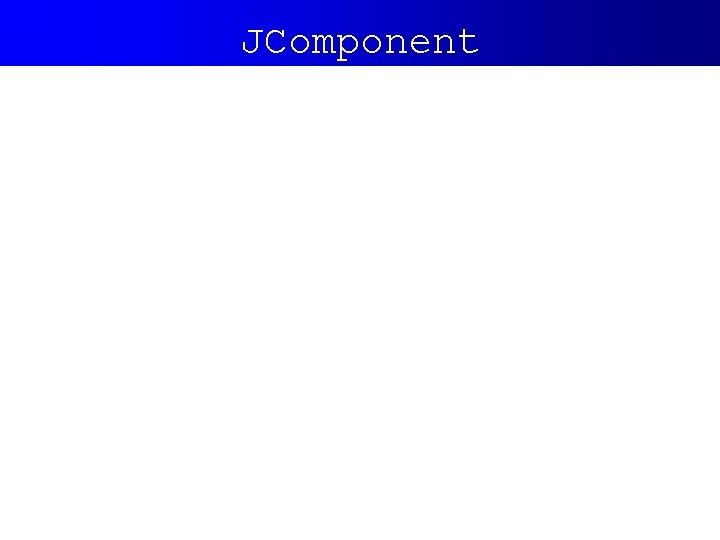
JComponent
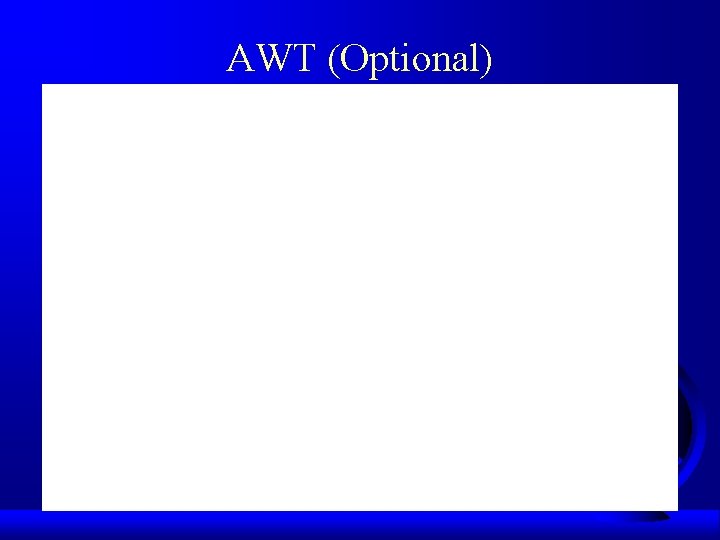
AWT (Optional)
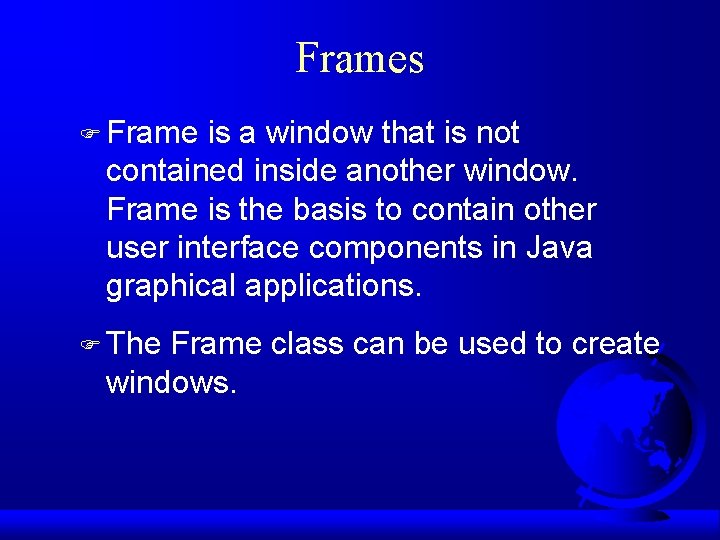
Frames F Frame is a window that is not contained inside another window. Frame is the basis to contain other user interface components in Java graphical applications. F The Frame class can be used to create windows.
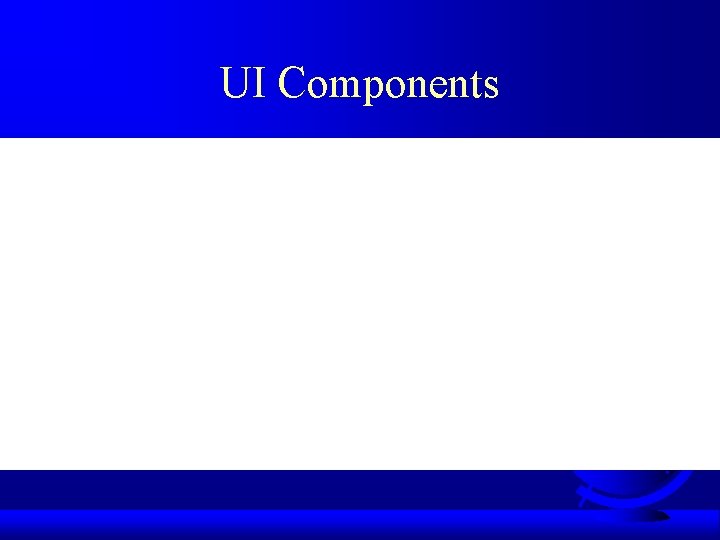
UI Components
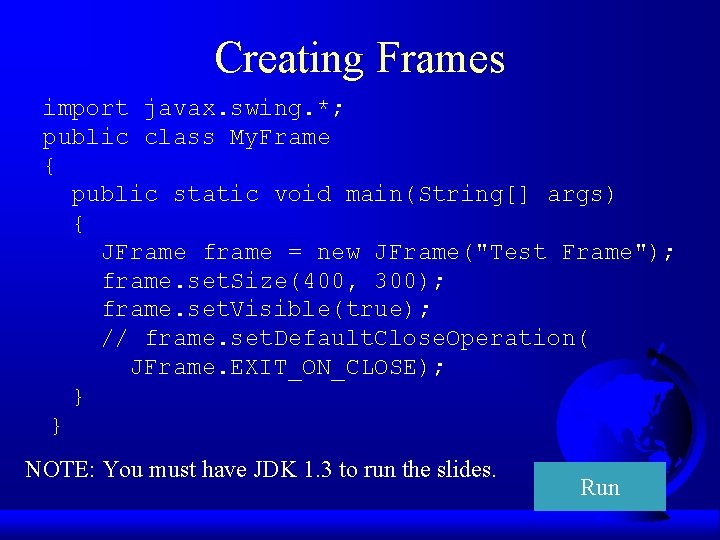
Creating Frames import javax. swing. *; public class My. Frame { public static void main(String[] args) { JFrame frame = new JFrame("Test Frame"); frame. set. Size(400, 300); frame. set. Visible(true); // frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE); } } NOTE: You must have JDK 1. 3 to run the slides. Run
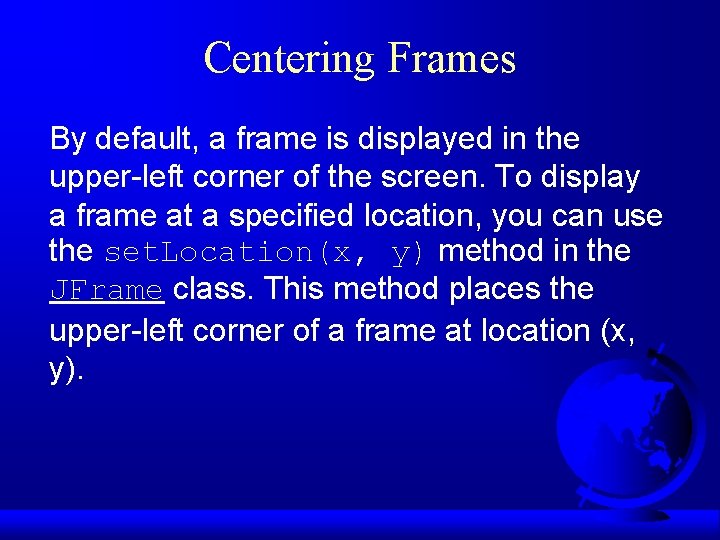
Centering Frames By default, a frame is displayed in the upper-left corner of the screen. To display a frame at a specified location, you can use the set. Location(x, y) method in the JFrame class. This method places the upper-left corner of a frame at location (x, y).
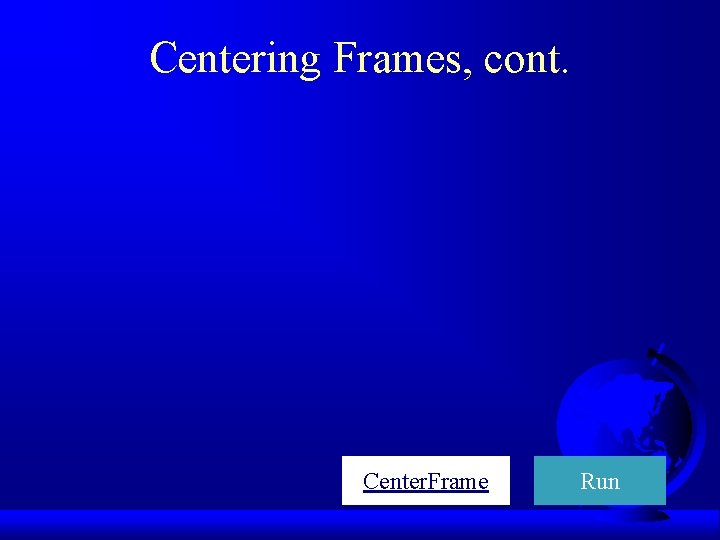
Centering Frames, cont. Center. Frame Run
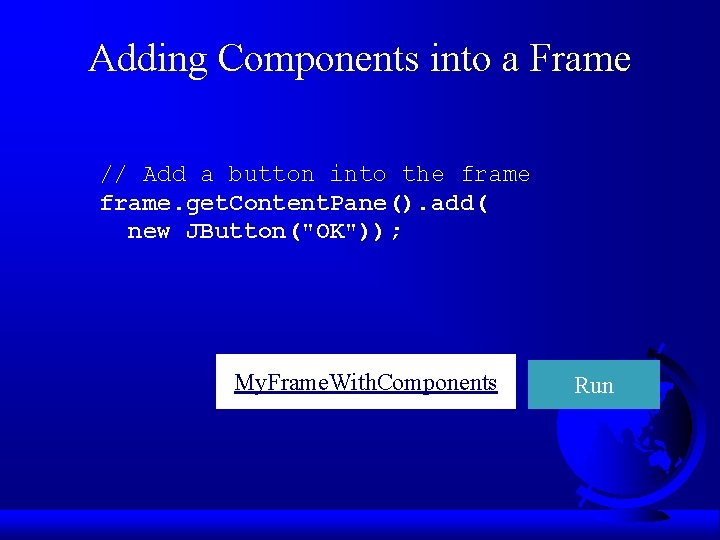
Adding Components into a Frame // Add a button into the frame. get. Content. Pane(). add( new JButton("OK")); My. Frame. With. Components Run
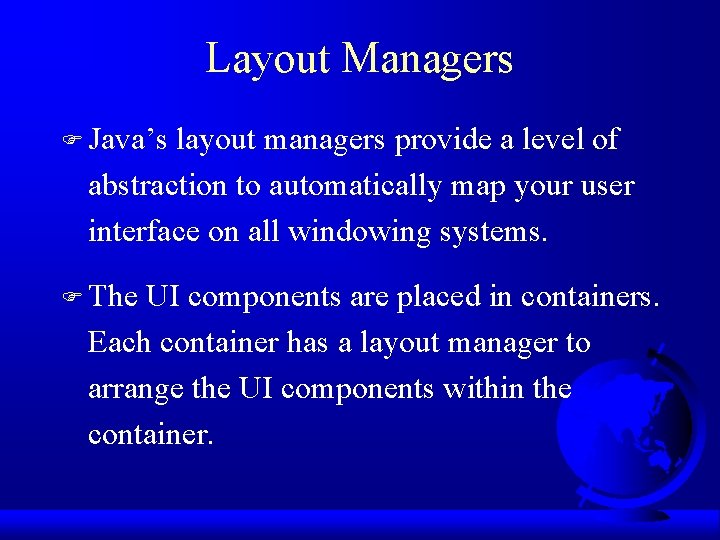
Layout Managers F Java’s layout managers provide a level of abstraction to automatically map your user interface on all windowing systems. F The UI components are placed in containers. Each container has a layout manager to arrange the UI components within the container.
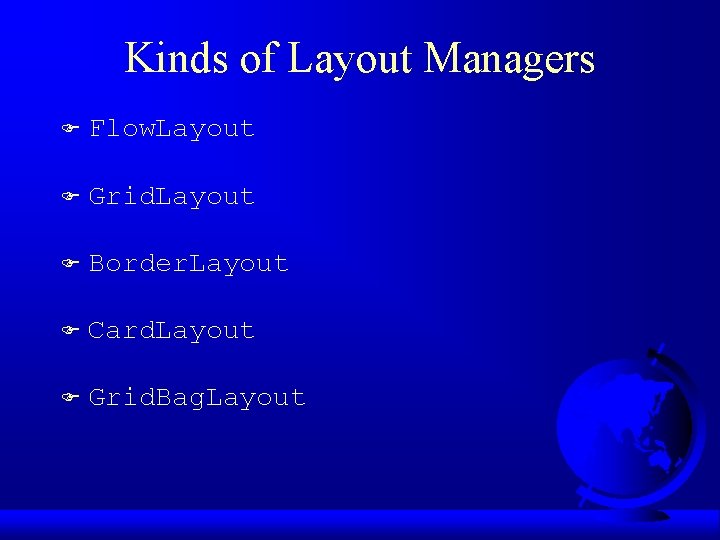
Kinds of Layout Managers F Flow. Layout F Grid. Layout F Border. Layout F Card. Layout F Grid. Bag. Layout
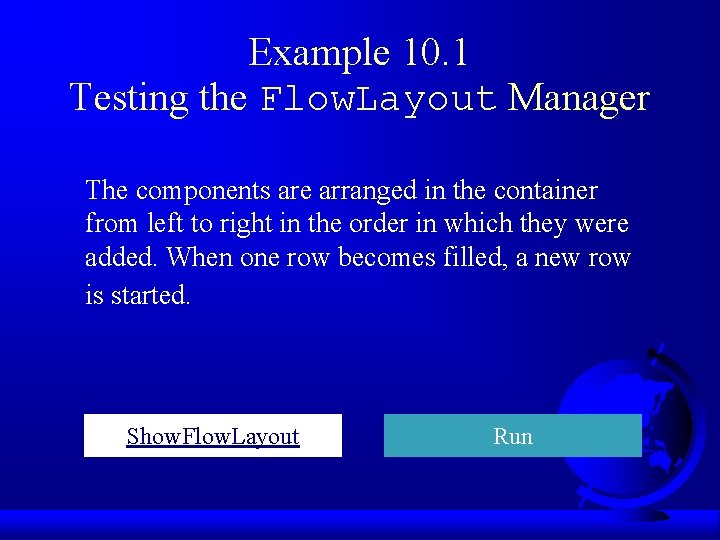
Example 10. 1 Testing the Flow. Layout Manager The components are arranged in the container from left to right in the order in which they were added. When one row becomes filled, a new row is started. Show. Flow. Layout Run
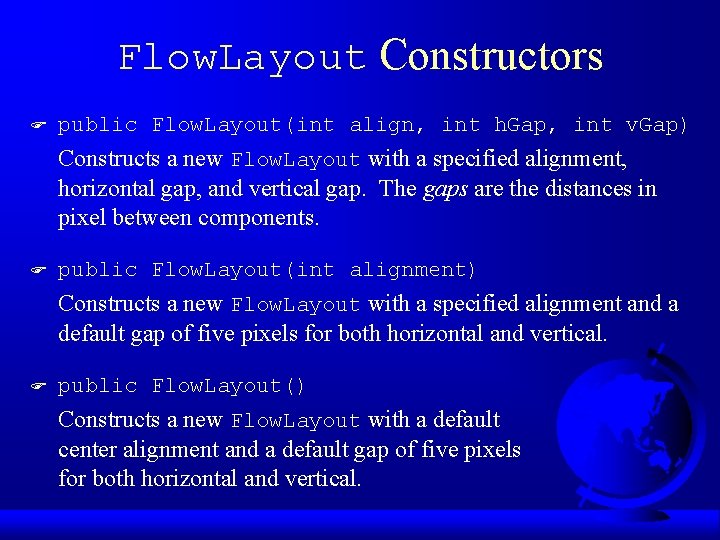
Flow. Layout Constructors F public Flow. Layout(int align, int h. Gap, int v. Gap) Constructs a new Flow. Layout with a specified alignment, horizontal gap, and vertical gap. The gaps are the distances in pixel between components. F public Flow. Layout(int alignment) Constructs a new Flow. Layout with a specified alignment and a default gap of five pixels for both horizontal and vertical. F public Flow. Layout() Constructs a new Flow. Layout with a default center alignment and a default gap of five pixels for both horizontal and vertical.
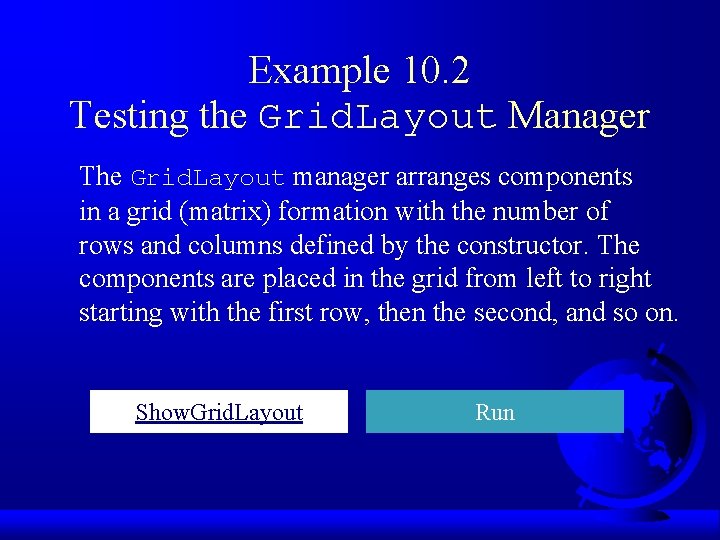
Example 10. 2 Testing the Grid. Layout Manager The Grid. Layout manager arranges components in a grid (matrix) formation with the number of rows and columns defined by the constructor. The components are placed in the grid from left to right starting with the first row, then the second, and so on. Show. Grid. Layout Run
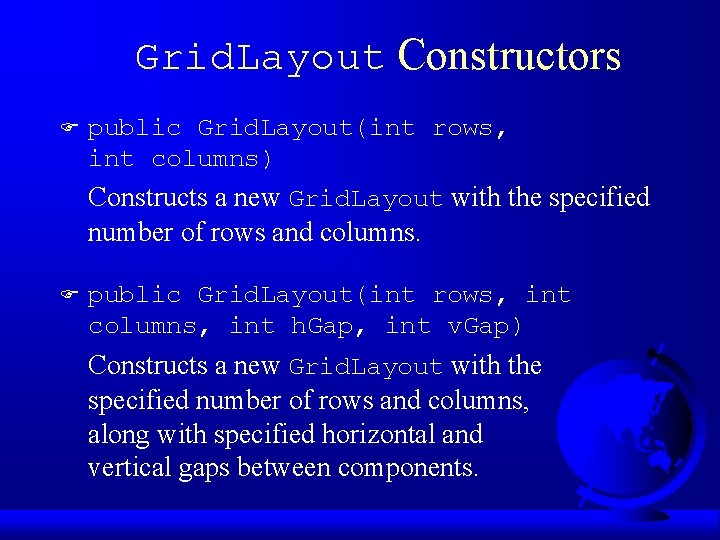
Grid. Layout Constructors F public Grid. Layout(int rows, int columns) Constructs a new Grid. Layout with the specified number of rows and columns. F public Grid. Layout(int rows, int columns, int h. Gap, int v. Gap) Constructs a new Grid. Layout with the specified number of rows and columns, along with specified horizontal and vertical gaps between components.
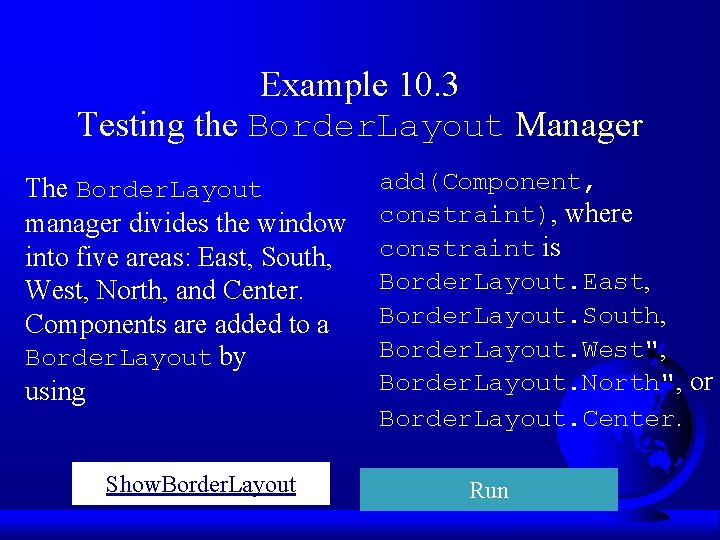
Example 10. 3 Testing the Border. Layout Manager The Border. Layout manager divides the window into five areas: East, South, West, North, and Center. Components are added to a Border. Layout by using Show. Border. Layout add(Component, constraint), where constraint is Border. Layout. East, Border. Layout. South, Border. Layout. West", Border. Layout. North", or Border. Layout. Center. Run
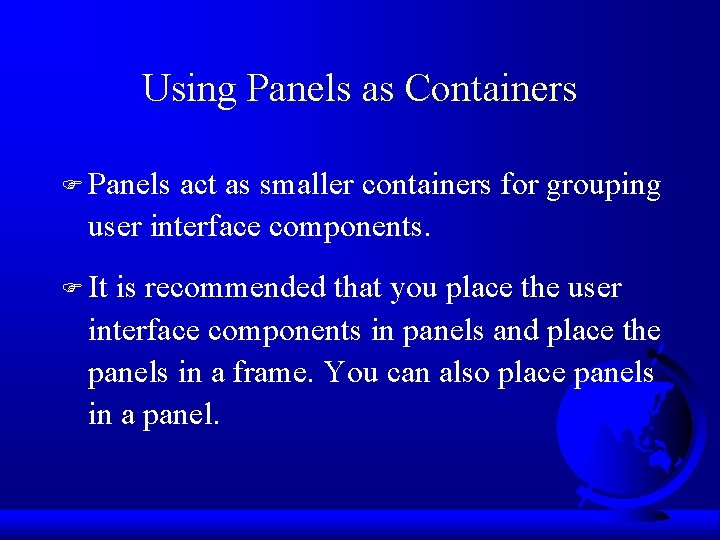
Using Panels as Containers F Panels act as smaller containers for grouping user interface components. F It is recommended that you place the user interface components in panels and place the panels in a frame. You can also place panels in a panel.
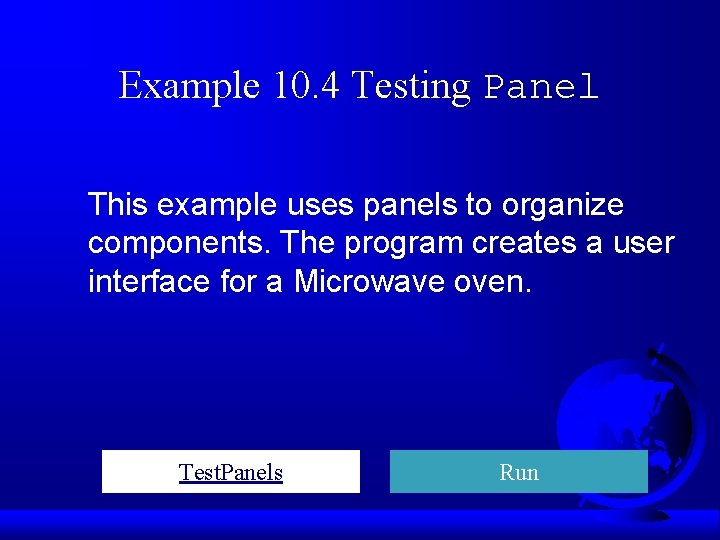
Example 10. 4 Testing Panel This example uses panels to organize components. The program creates a user interface for a Microwave oven. Test. Panels Run
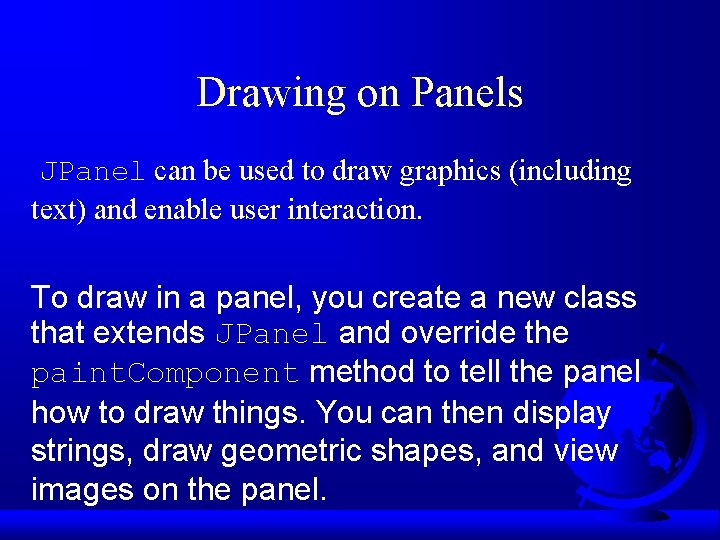
Drawing on Panels JPanel can be used to draw graphics (including text) and enable user interaction. To draw in a panel, you create a new class that extends JPanel and override the paint. Component method to tell the panel how to draw things. You can then display strings, draw geometric shapes, and view images on the panel.
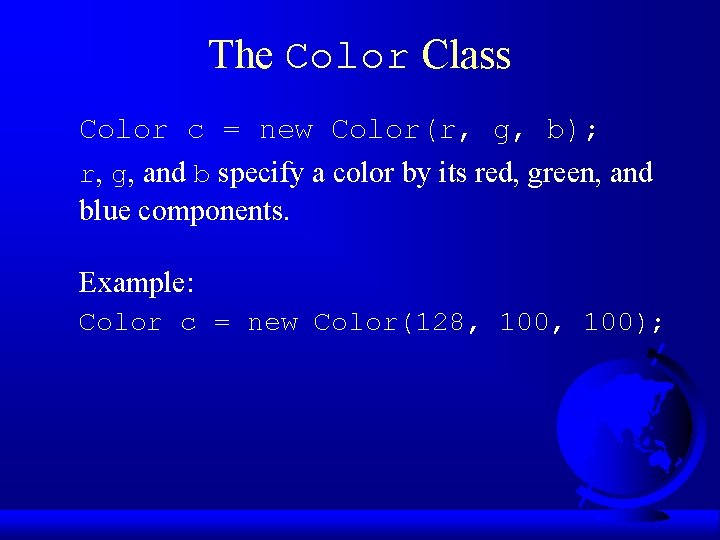
The Color Class Color c = new Color(r, g, b); r, g, and b specify a color by its red, green, and blue components. Example: Color c = new Color(128, 100);
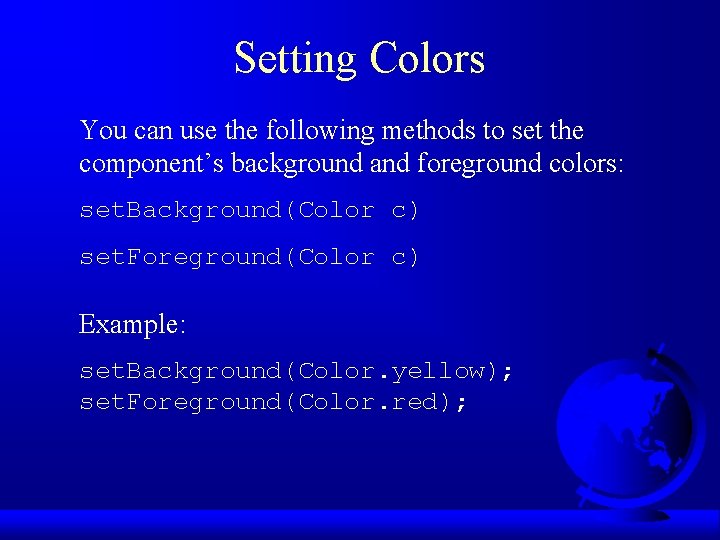
Setting Colors You can use the following methods to set the component’s background and foreground colors: set. Background(Color c) set. Foreground(Color c) Example: set. Background(Color. yellow); set. Foreground(Color. red);
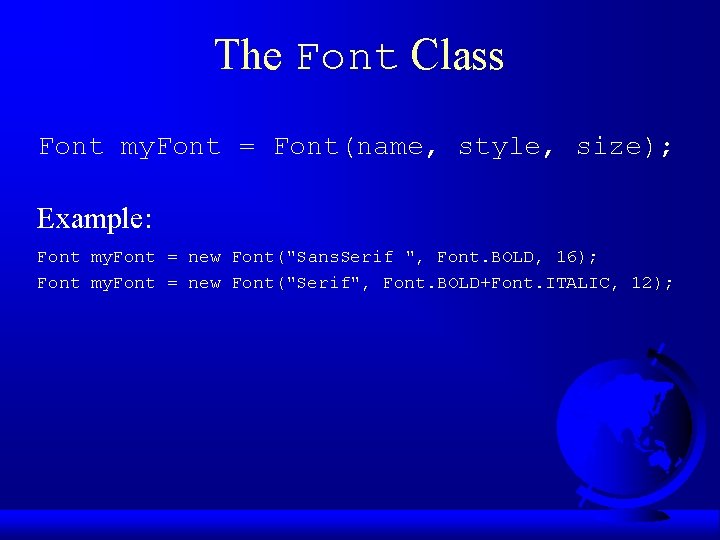
The Font Class Font my. Font = Font(name, style, size); Example: Font my. Font = new Font("Sans. Serif ", Font. BOLD, 16); Font my. Font = new Font("Serif", Font. BOLD+Font. ITALIC, 12);
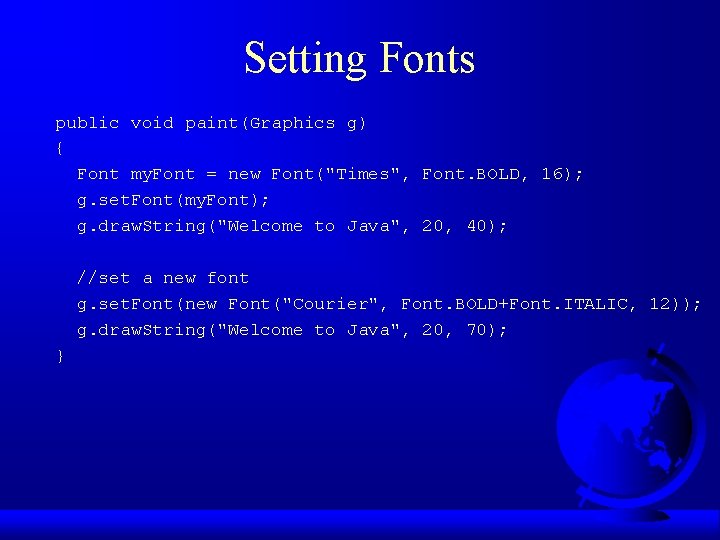
Setting Fonts public void paint(Graphics g) { Font my. Font = new Font("Times", Font. BOLD, 16); g. set. Font(my. Font); g. draw. String("Welcome to Java", 20, 40); //set a new font g. set. Font(new Font("Courier", Font. BOLD+Font. ITALIC, 12)); g. draw. String("Welcome to Java", 20, 70); }
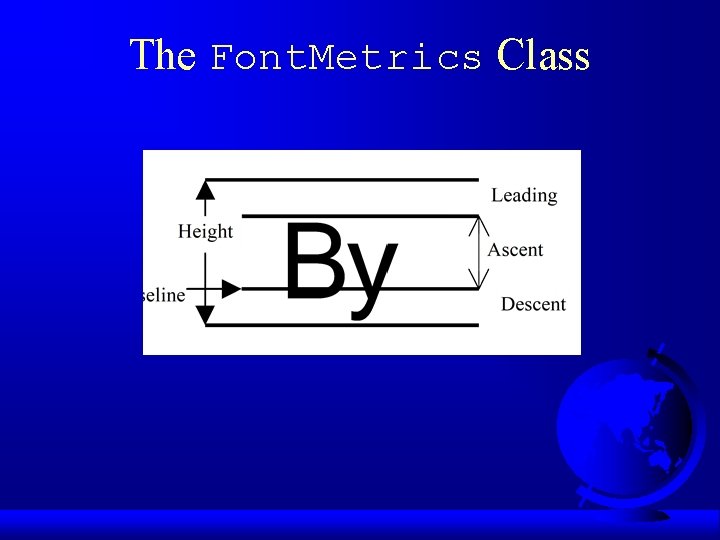
The Font. Metrics Class
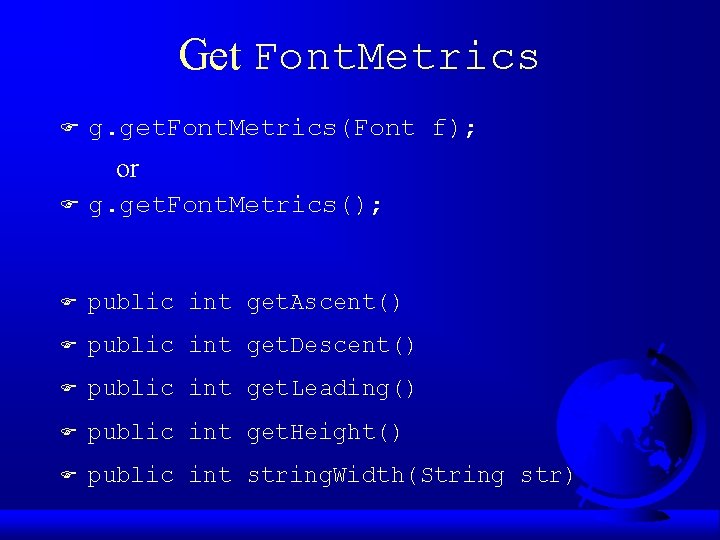
Get Font. Metrics F g. get. Font. Metrics(Font f); or F g. get. Font. Metrics(); F public int get. Ascent() F public int get. Descent() F public int get. Leading() F public int get. Height() F public int string. Width(String str)
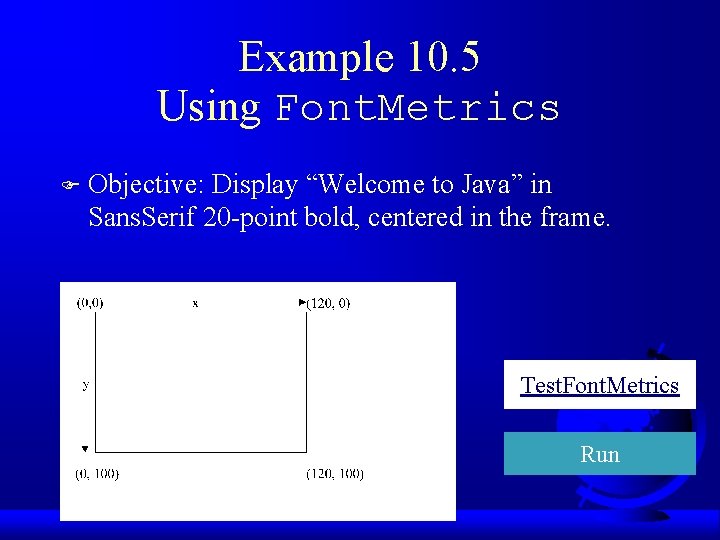
Example 10. 5 Using Font. Metrics F Objective: Display “Welcome to Java” in Sans. Serif 20 -point bold, centered in the frame. Test. Font. Metrics Run
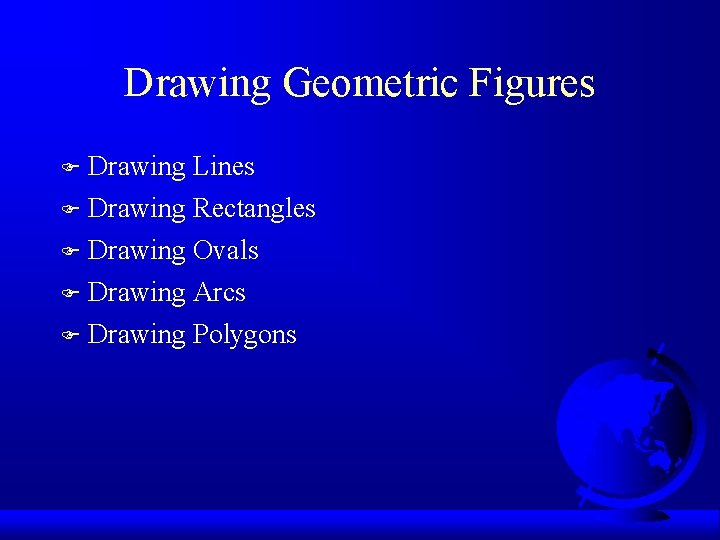
Drawing Geometric Figures F Drawing Lines F Drawing Rectangles Drawing Ovals F Drawing Arcs F Drawing Polygons F
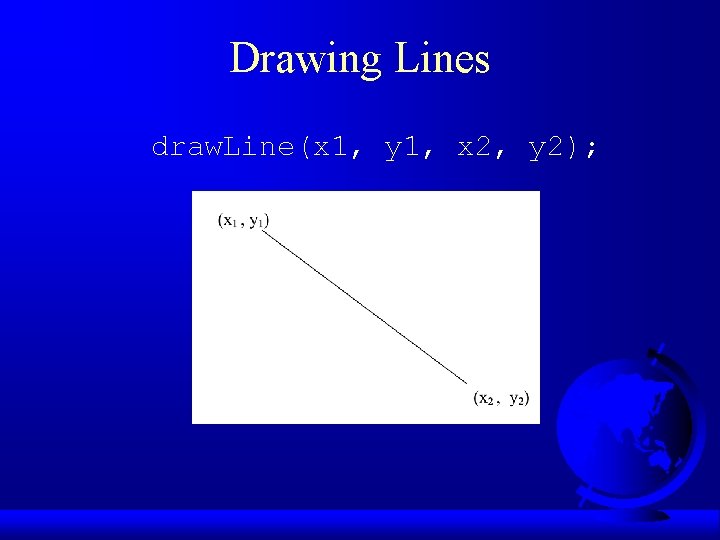
Drawing Lines draw. Line(x 1, y 1, x 2, y 2);
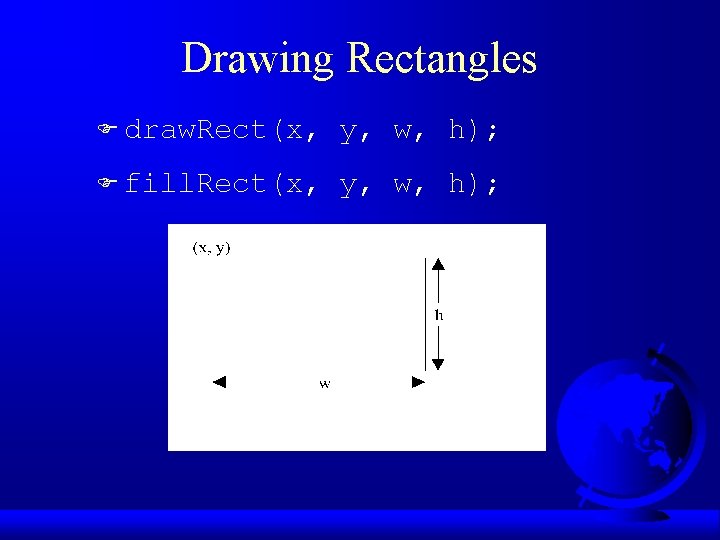
Drawing Rectangles F draw. Rect(x, y, w, h); F fill. Rect(x, y, w, h);
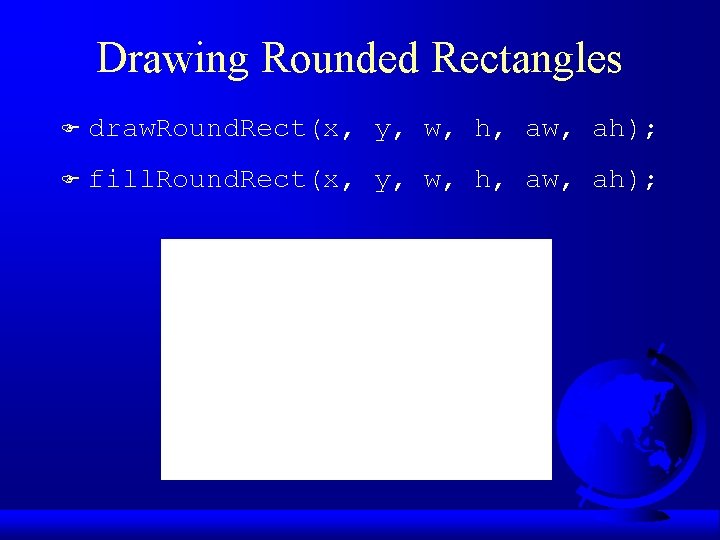
Drawing Rounded Rectangles F draw. Round. Rect(x, y, w, h, aw, ah); F fill. Round. Rect(x, y, w, h, aw, ah);
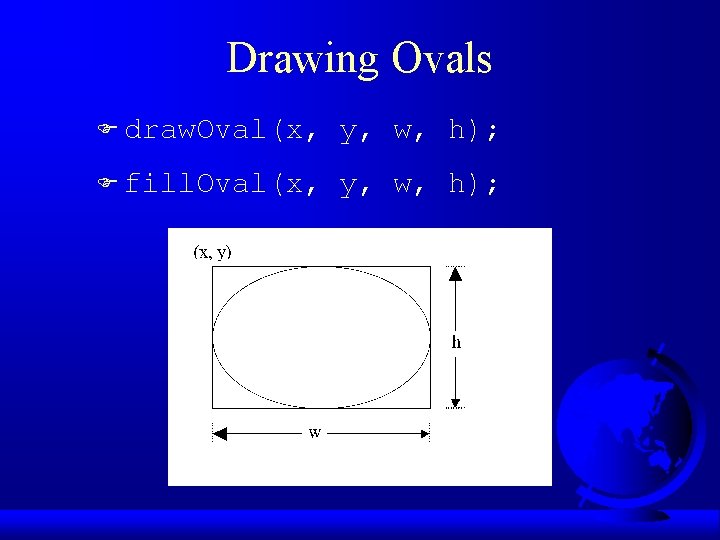
Drawing Ovals F draw. Oval(x, y, w, h); F fill. Oval(x, y, w, h);
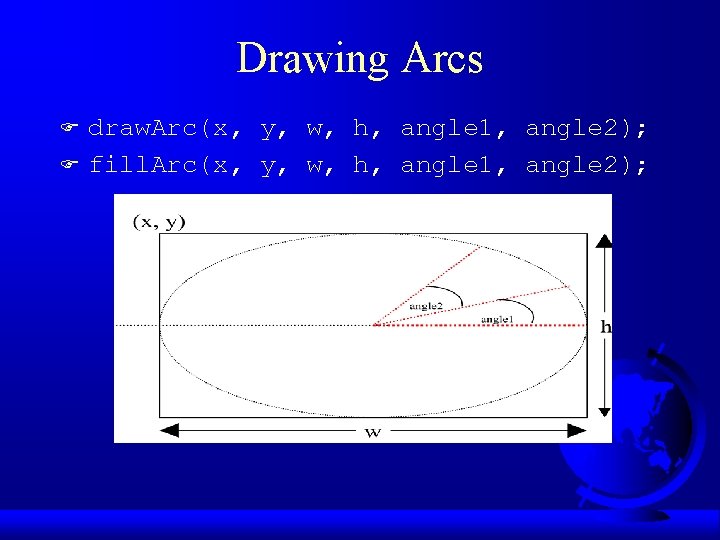
Drawing Arcs F F draw. Arc(x, y, w, h, angle 1, angle 2); fill. Arc(x, y, w, h, angle 1, angle 2);
![Drawing Polygons int x 40 70 60 45 20 int y 20 Drawing Polygons int[] x = {40, 70, 60, 45, 20}; int[] y = {20,](https://slidetodoc.com/presentation_image/7995e938e89268e5376fb8afc6923bb9/image-34.jpg)
Drawing Polygons int[] x = {40, 70, 60, 45, 20}; int[] y = {20, 40, 80, 45, 60}; g. draw. Polygon(x, y, x. length); g. fill. Polygon(x, y, x. length);
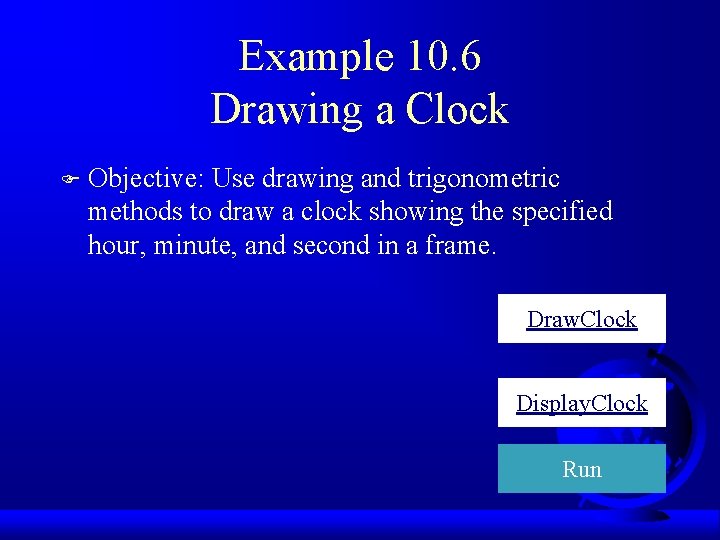
Example 10. 6 Drawing a Clock F Objective: Use drawing and trigonometric methods to draw a clock showing the specified hour, minute, and second in a frame. Draw. Clock Display. Clock Run
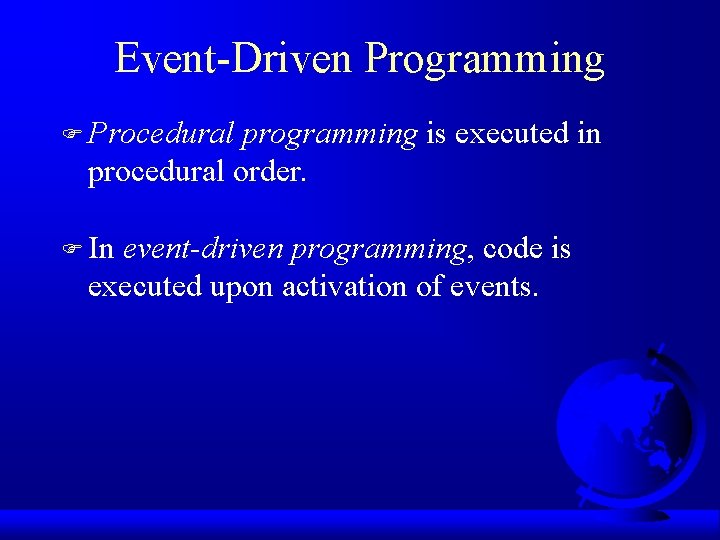
Event-Driven Programming F Procedural programming is executed in procedural order. F In event-driven programming, code is executed upon activation of events.
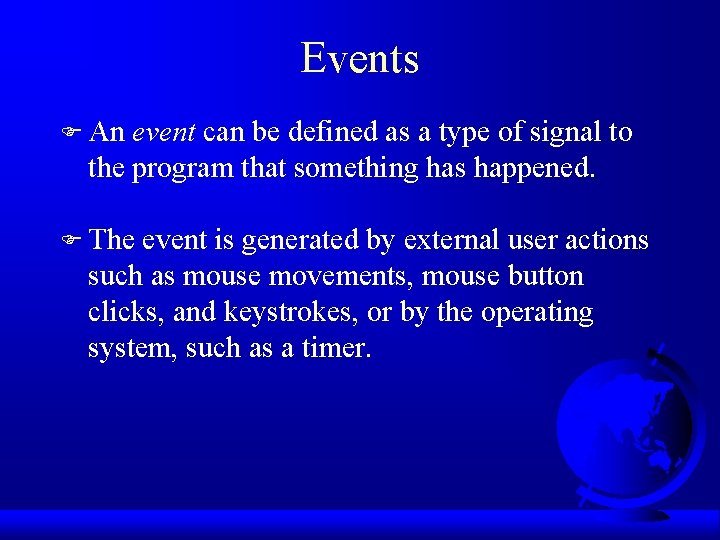
Events F An event can be defined as a type of signal to the program that something has happened. F The event is generated by external user actions such as mouse movements, mouse button clicks, and keystrokes, or by the operating system, such as a timer.
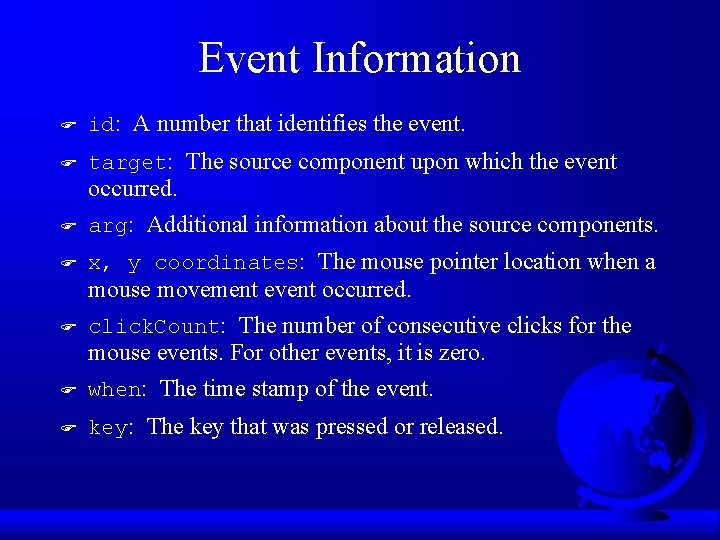
Event Information F id: A number that identifies the event. F target: The source component upon which the event occurred. F arg: Additional information about the source components. F x, y coordinates: The mouse pointer location when a mouse movement event occurred. F click. Count: The number of consecutive clicks for the mouse events. For other events, it is zero. F when: The time stamp of the event. F key: The key that was pressed or released.
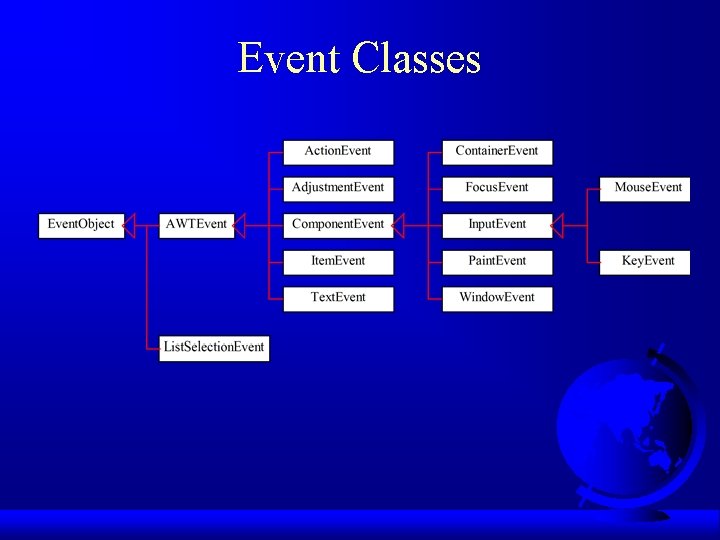
Event Classes
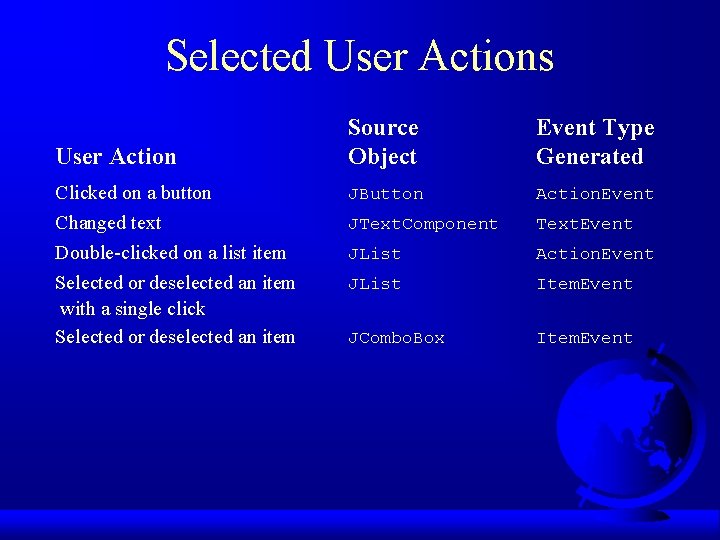
Selected User Actions User Action Clicked on a button Changed text Double-clicked on a list item Selected or deselected an item with a single click Selected or deselected an item Source Object Event Type Generated JButton Action. Event JText. Component Text. Event JList Action. Event JList Item. Event JCombo. Box Item. Event
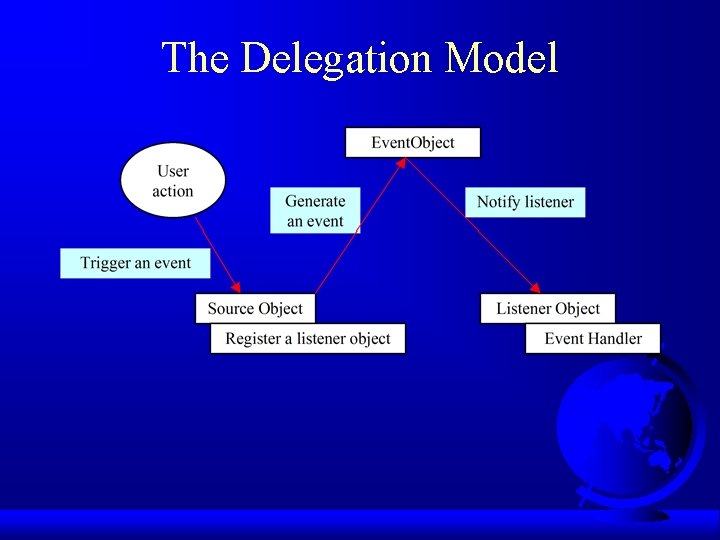
The Delegation Model
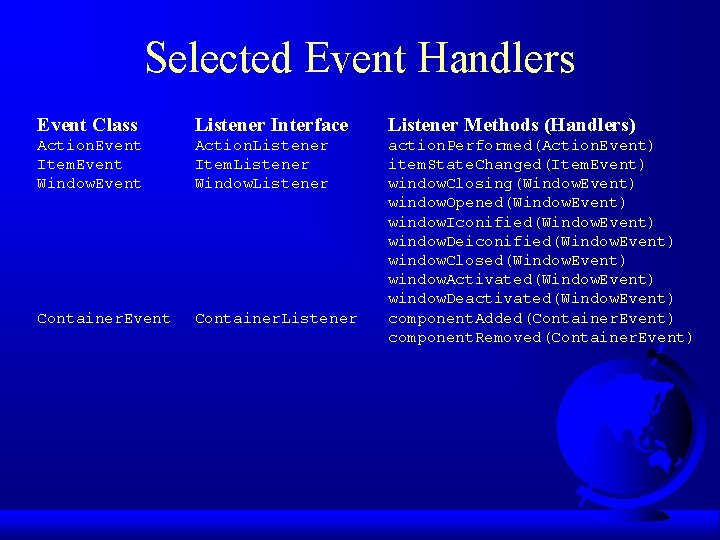
Selected Event Handlers Event Class Listener Interface Listener Methods (Handlers) Action. Event Item. Event Window. Event Action. Listener Item. Listener Window. Listener Container. Event Container. Listener action. Performed(Action. Event) item. State. Changed(Item. Event) window. Closing(Window. Event) window. Opened(Window. Event) window. Iconified(Window. Event) window. Deiconified(Window. Event) window. Closed(Window. Event) window. Activated(Window. Event) window. Deactivated(Window. Event) component. Added(Container. Event) component. Removed(Container. Event)
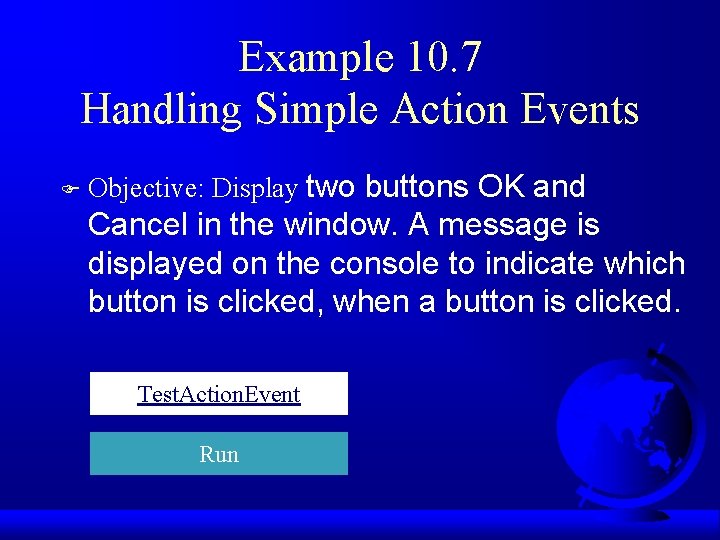
Example 10. 7 Handling Simple Action Events F Objective: Display two buttons OK and Cancel in the window. A message is displayed on the console to indicate which button is clicked, when a button is clicked. Test. Action. Event Run
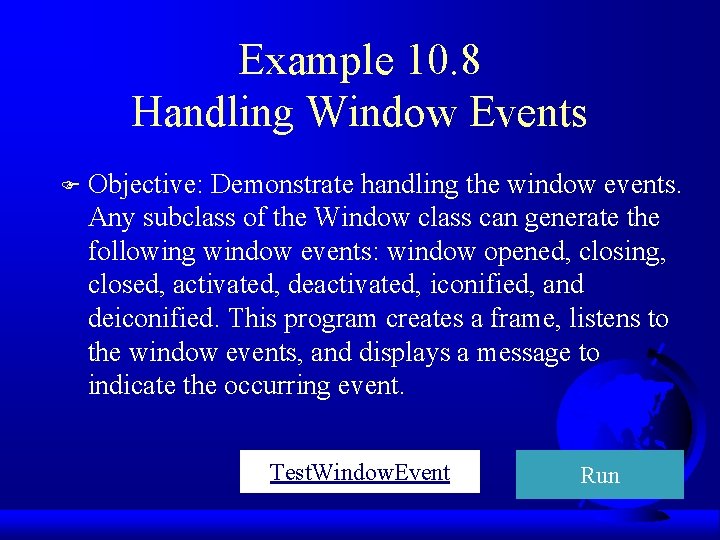
Example 10. 8 Handling Window Events F Objective: Demonstrate handling the window events. Any subclass of the Window class can generate the following window events: window opened, closing, closed, activated, deactivated, iconified, and deiconified. This program creates a frame, listens to the window events, and displays a message to indicate the occurring event. Test. Window. Event Run
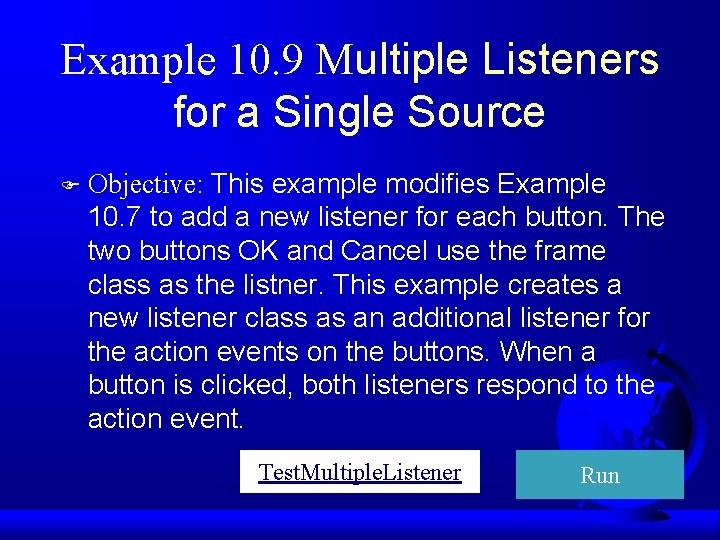
Example 10. 9 Multiple Listeners for a Single Source This example modifies Example 10. 7 to add a new listener for each button. The two buttons OK and Cancel use the frame class as the listner. This example creates a new listener class as an additional listener for the action events on the buttons. When a button is clicked, both listeners respond to the action event. F Objective: Test. Multiple. Listener Run
The secret to getting ahead is getting started
Getting started with vivado
Unix for bioinformatics
Splunke
Rancher getting started
Getting started with excel
Microsoft outlook 2010 tutorial
Getting started with xilinx fpga
Lua getting started
Unit 1 hobbies
Unit 1 local environment
Unit 1 getting started
Infuecers gone wild
Hi3ms
Perl read_file
Getting started with ft8
Unit 2 listen and read
Unit 1 getting started
Poll everywhere register
Android development getting started
Getting started with access
Getting started with eclipse
Mathematica getting started
Perbedaan linear programming dan integer programming
Greedy vs dynamic
Windows 10 system programming, part 1
Linear vs integer programming
Programing adalah
Chapter 5 lesson 4 getting help
Chapter 5 mental and emotional problems answer key
Realitykit example
Programming raster display system in computer graphics
Math for graphics programming
Programming graphics
Hand held computer
Computer graphics chapter 1 ppt
When was globalization started
When urdu hindi controversy emerged in subcontinent
Khalid al dossary
Ra 10912 meaning
Who founded samaritan's purse
Let's start with introduction
What started the cold war
Herbalife scientist
What is maitree event
Classical music has less complicated texture