An Introduction to Programming with C Sixth Edition
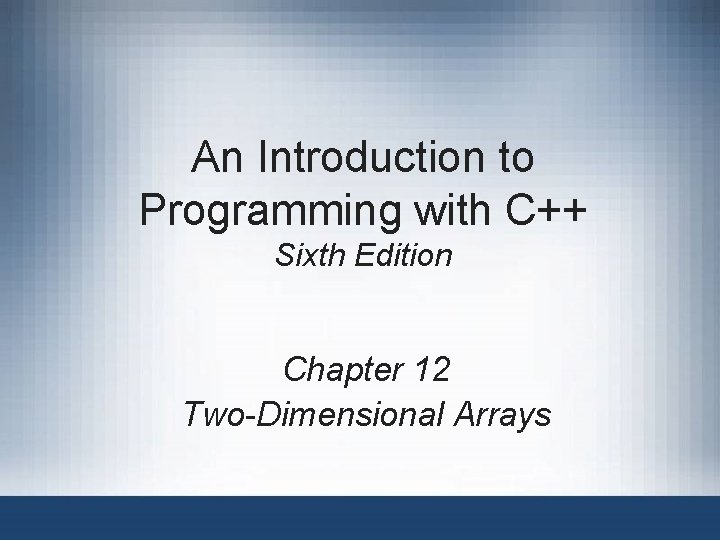
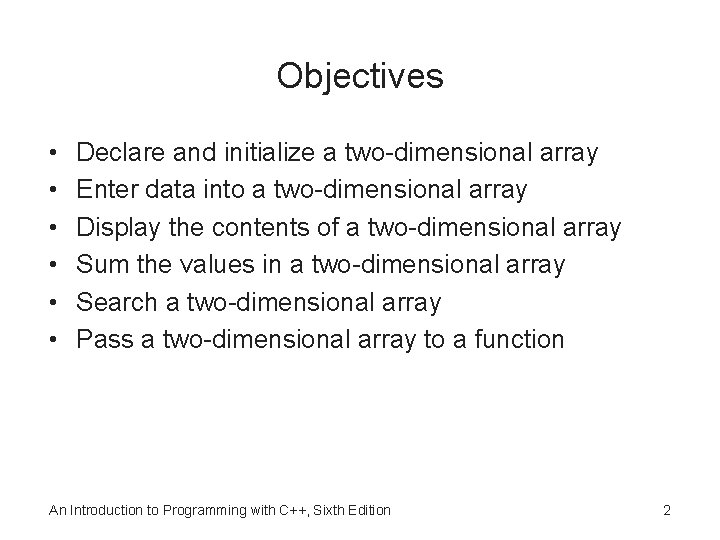
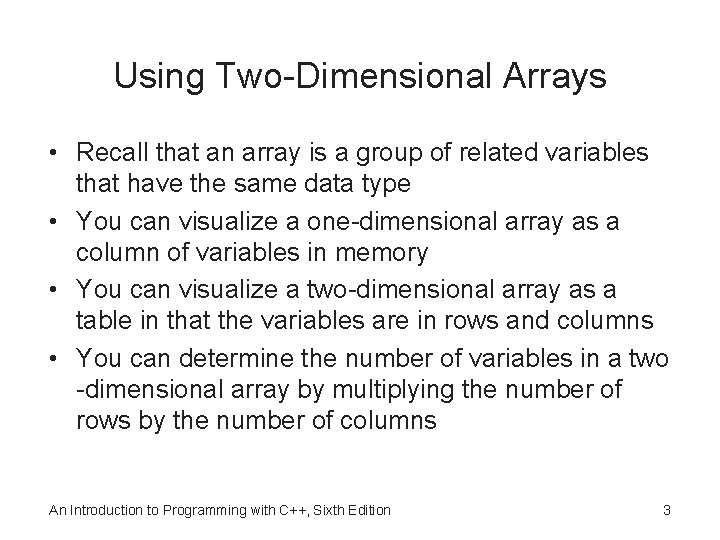
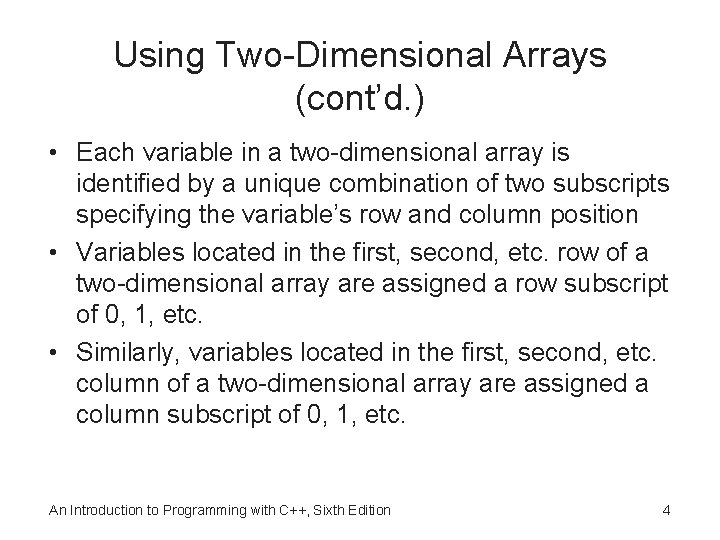
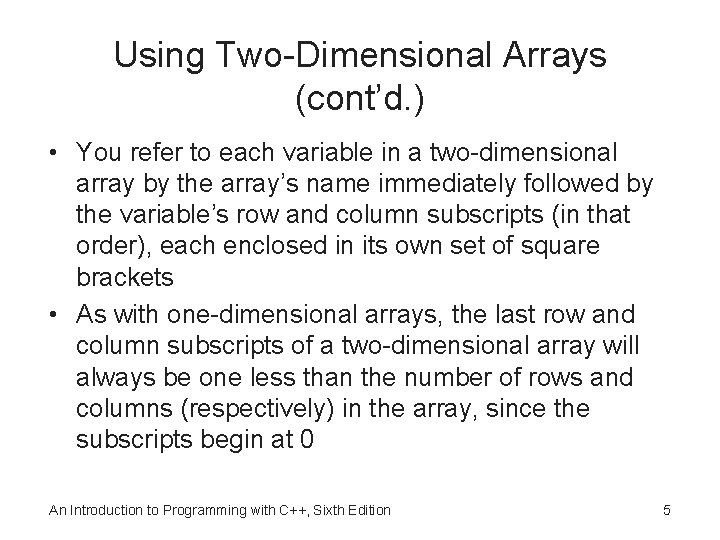
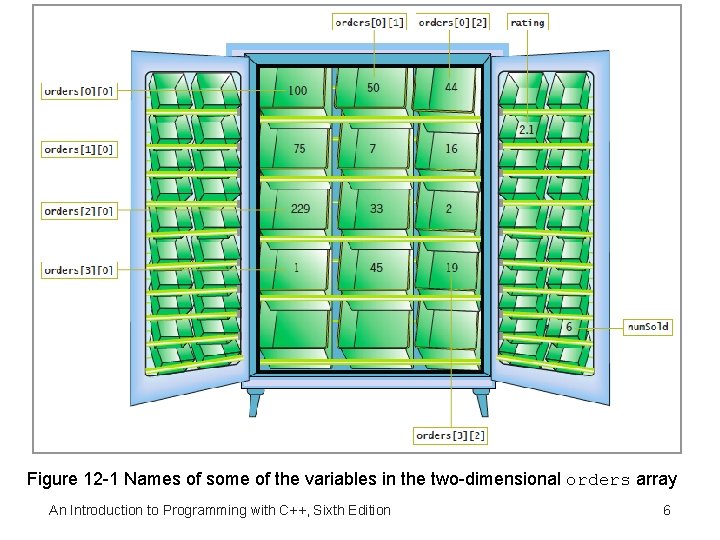
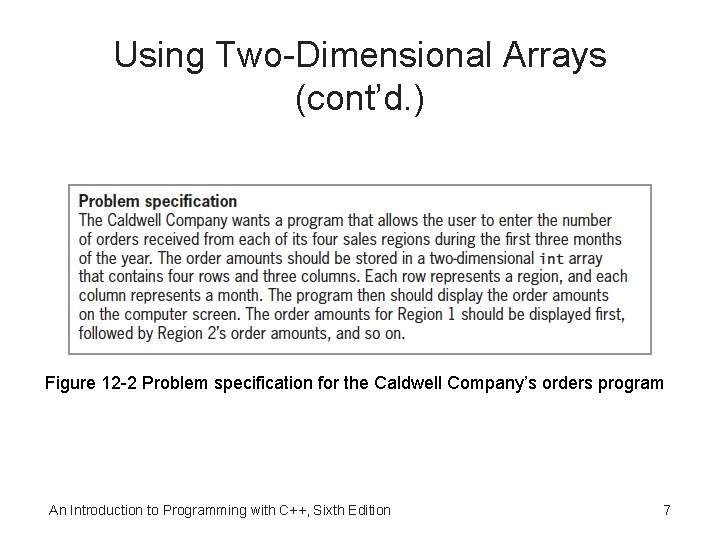
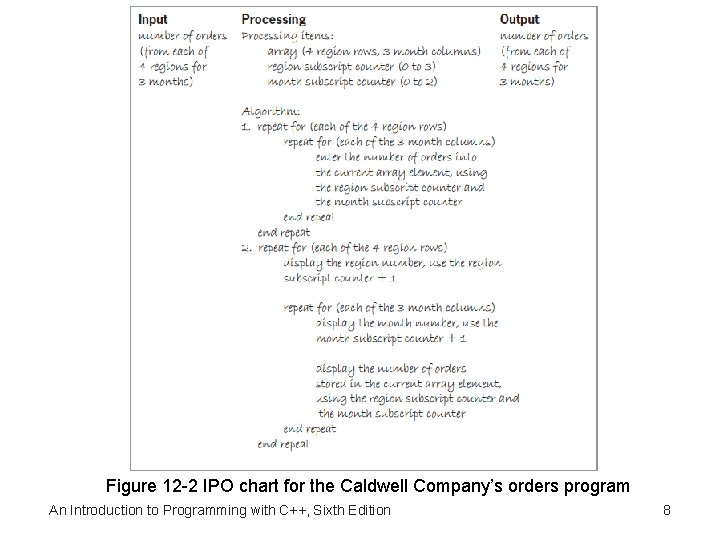
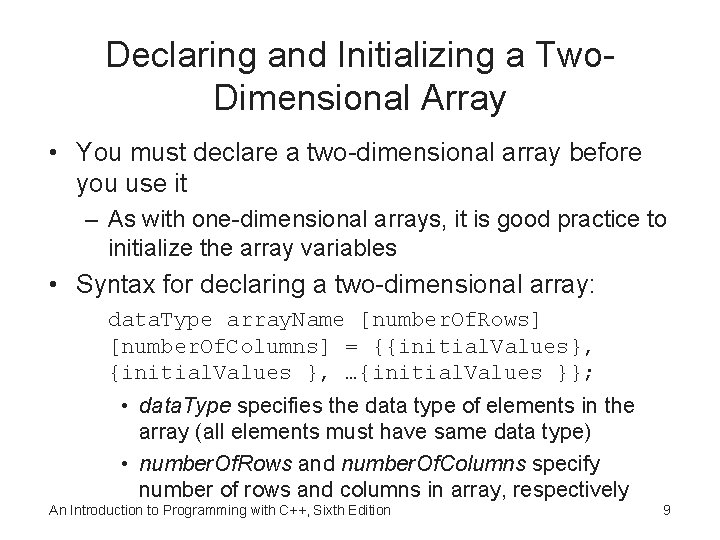
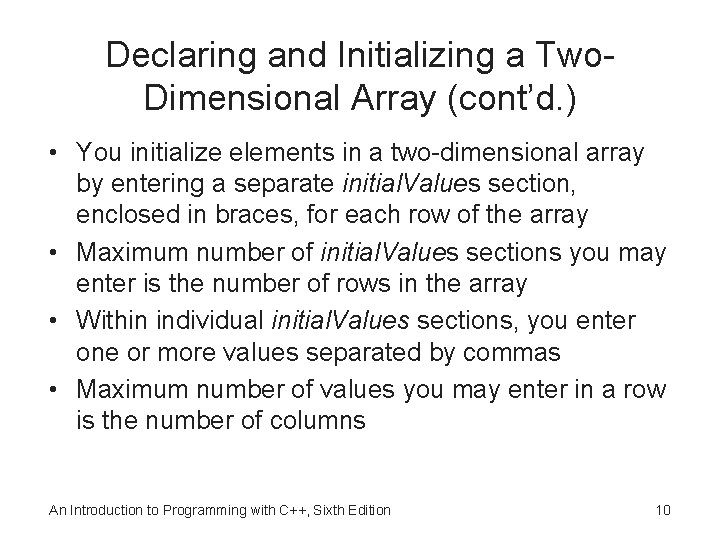
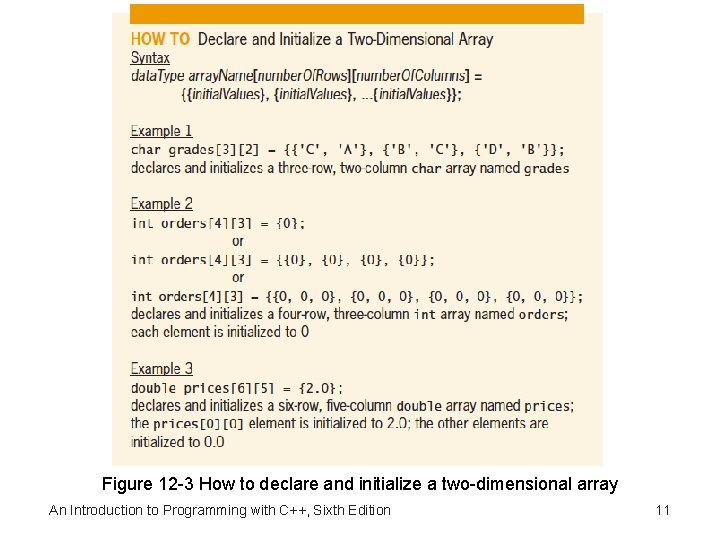
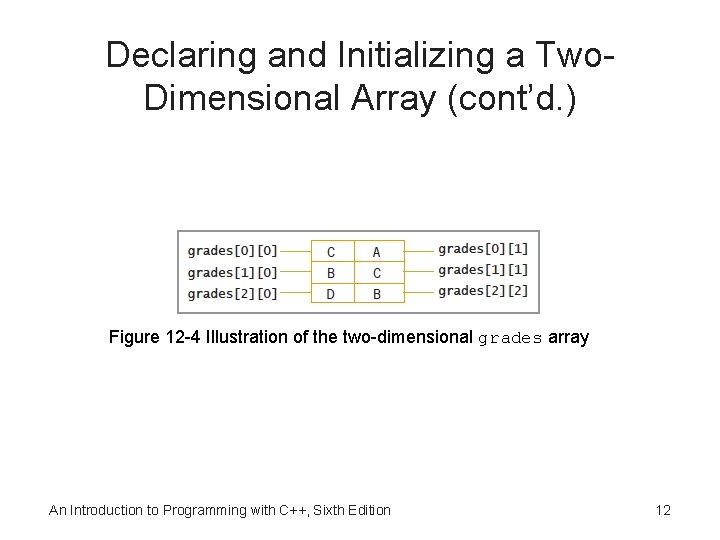
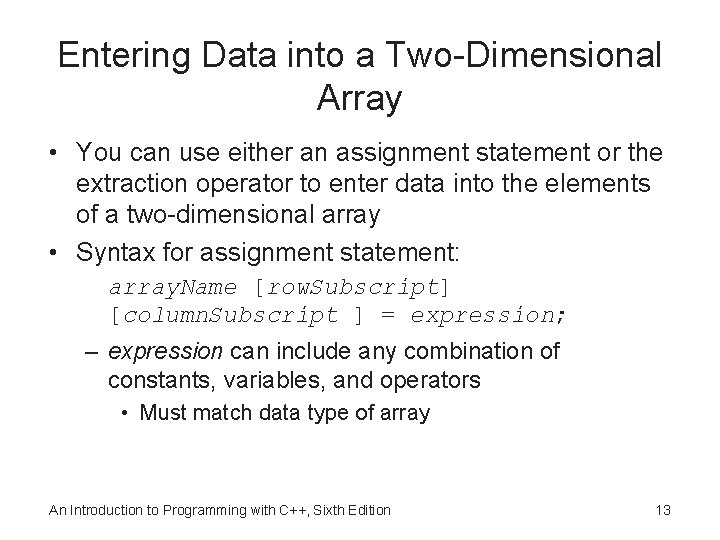
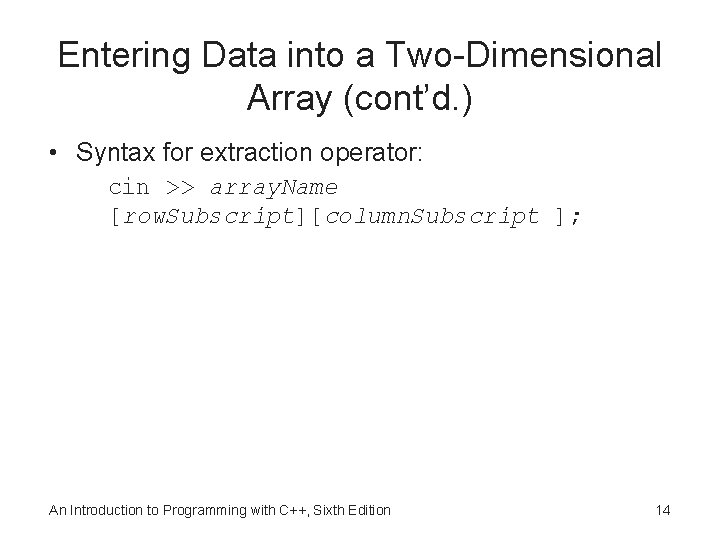
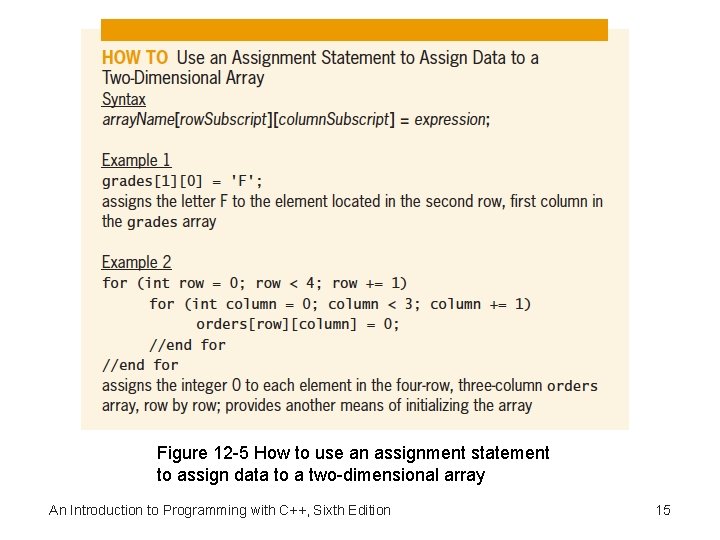
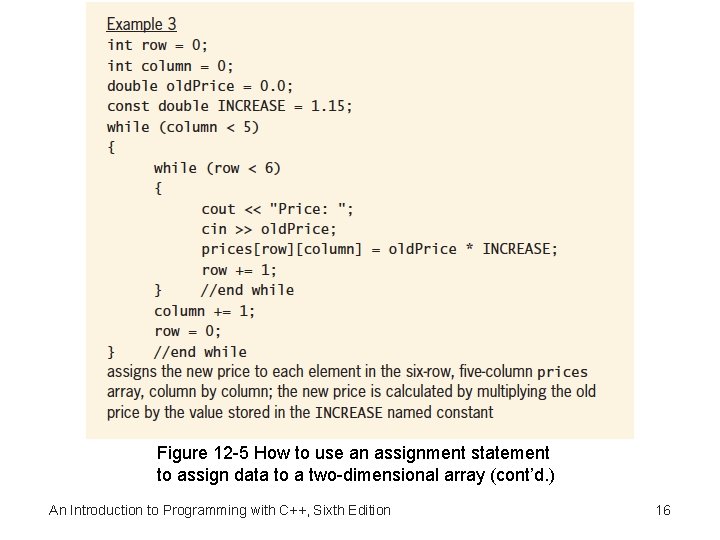
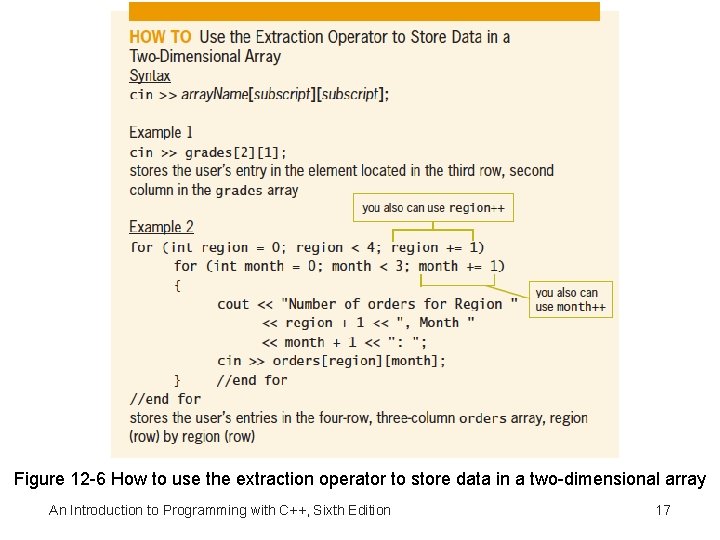
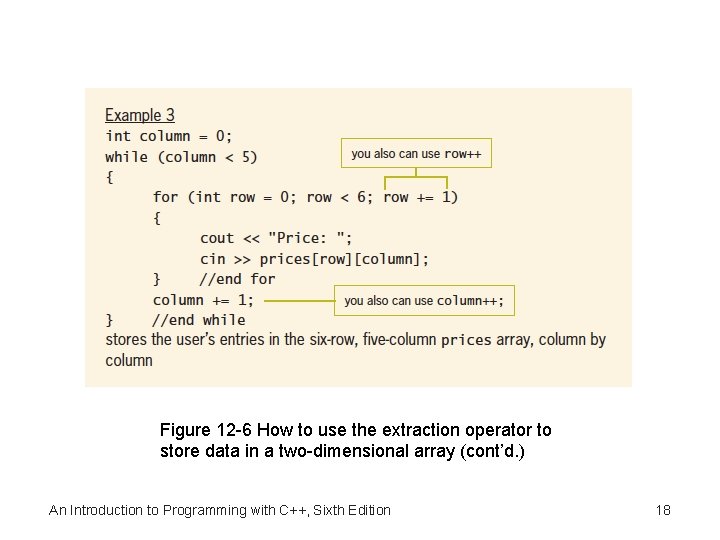
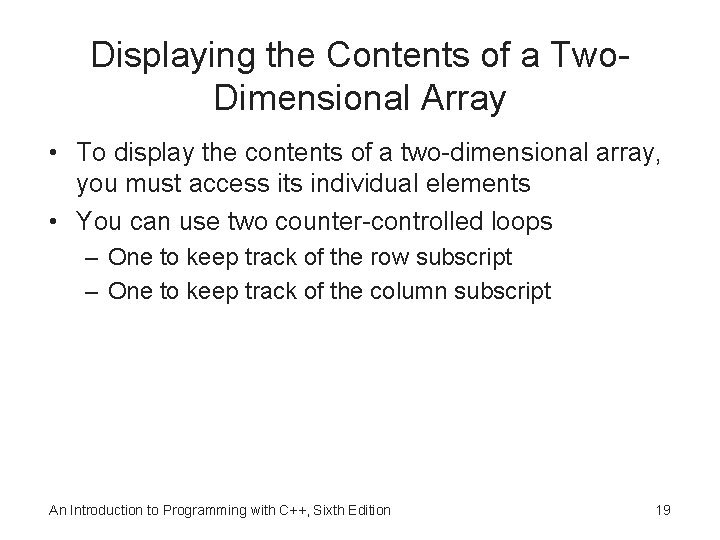
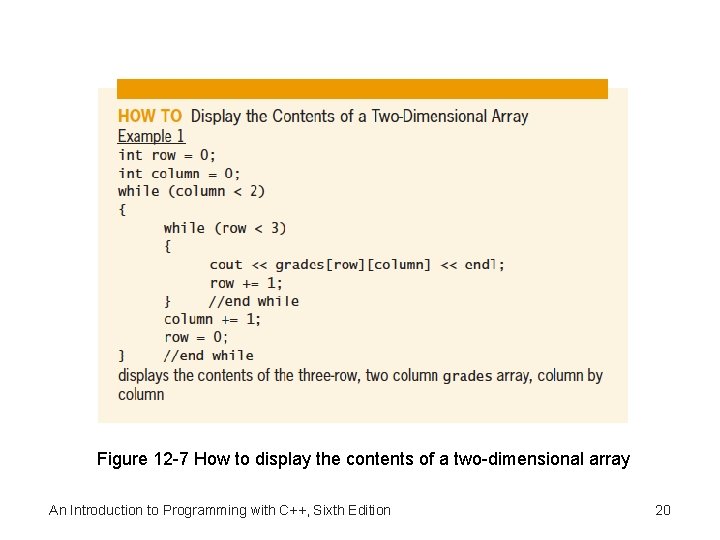
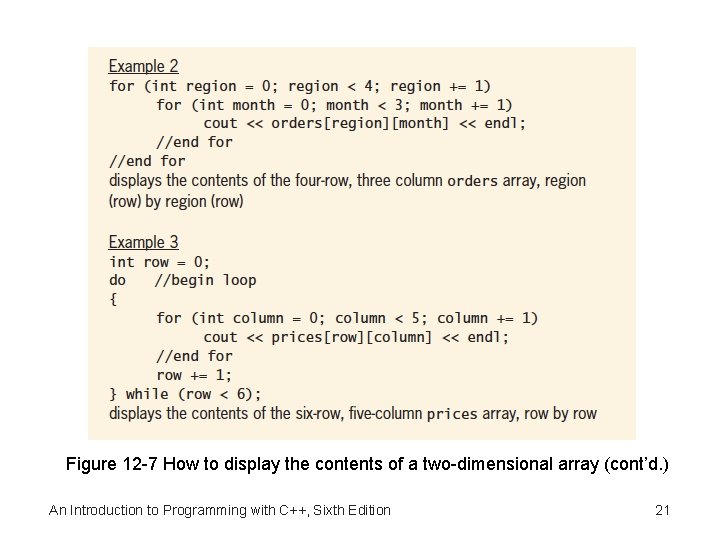
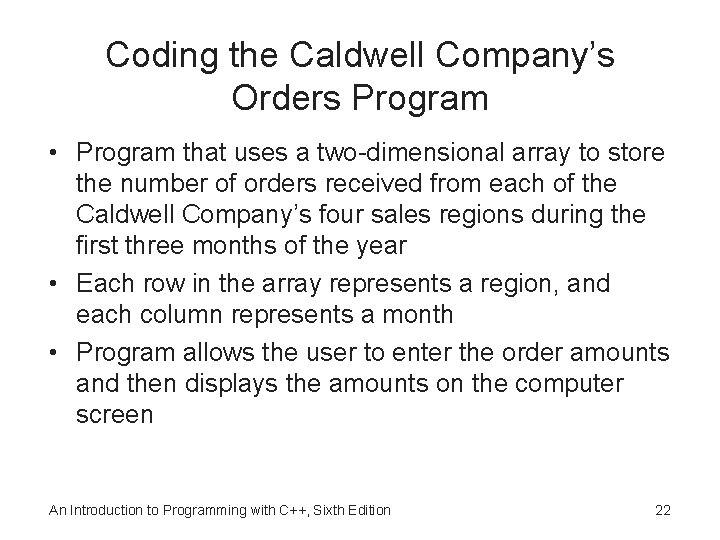
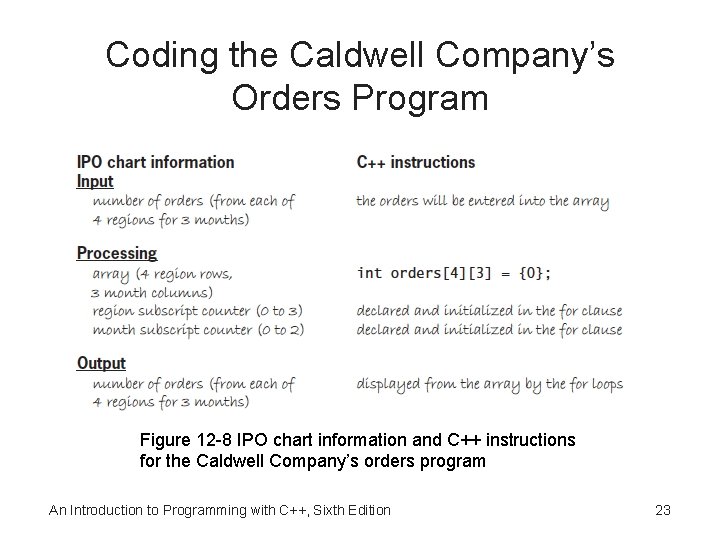
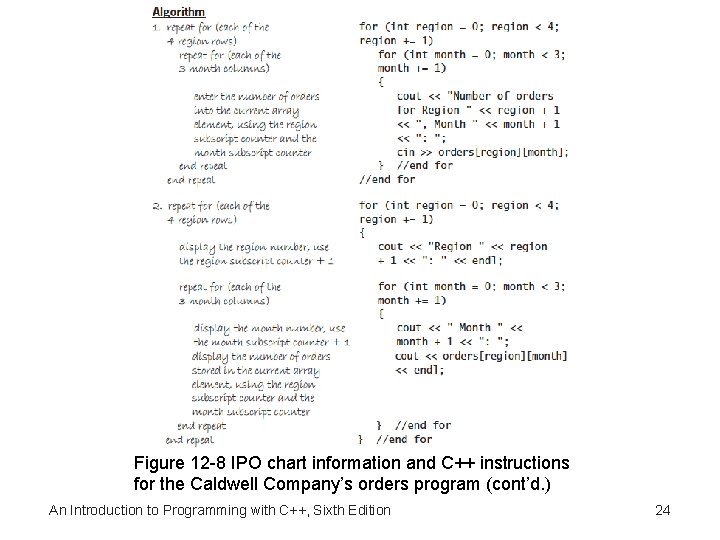
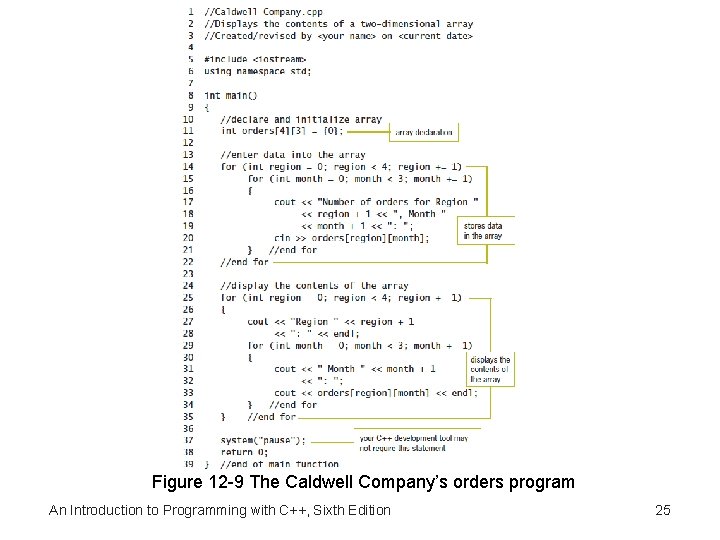
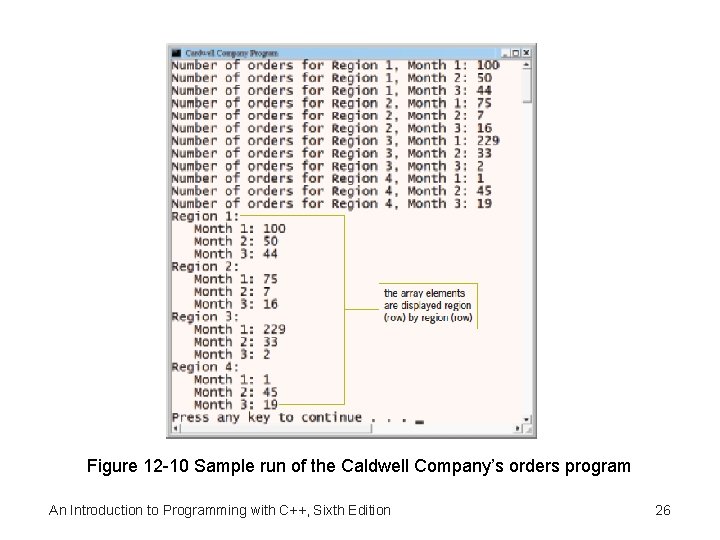
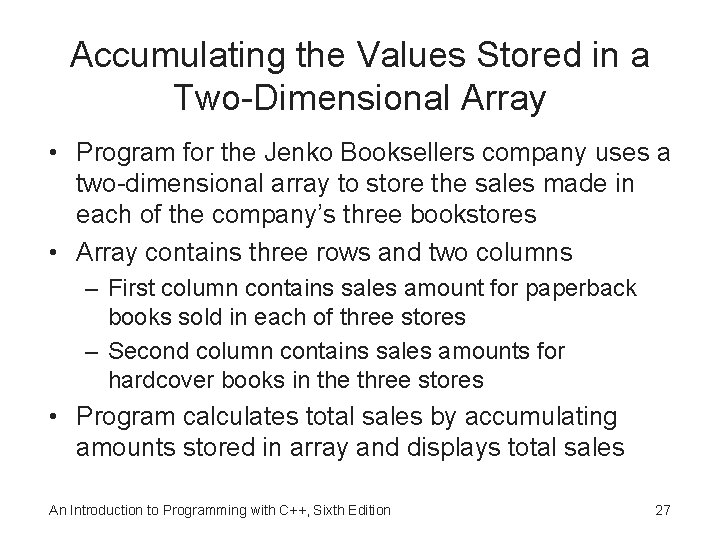
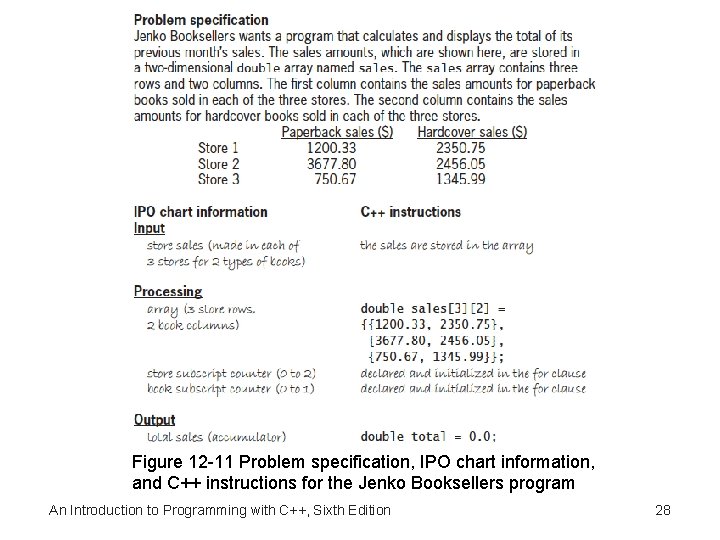
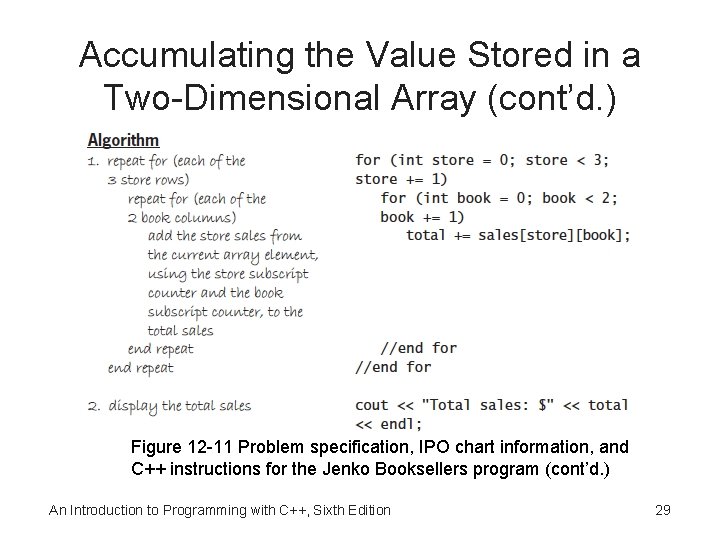
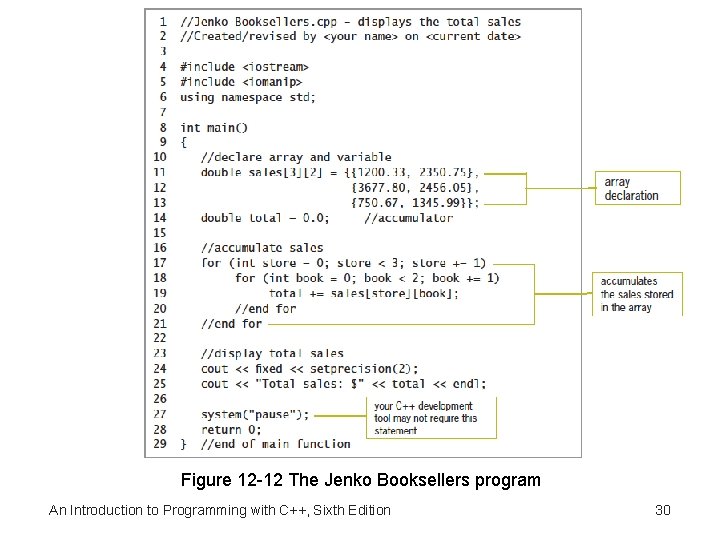
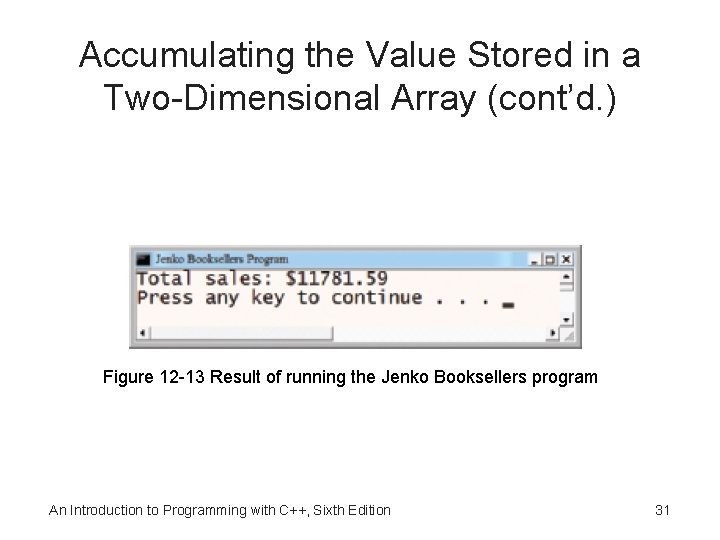
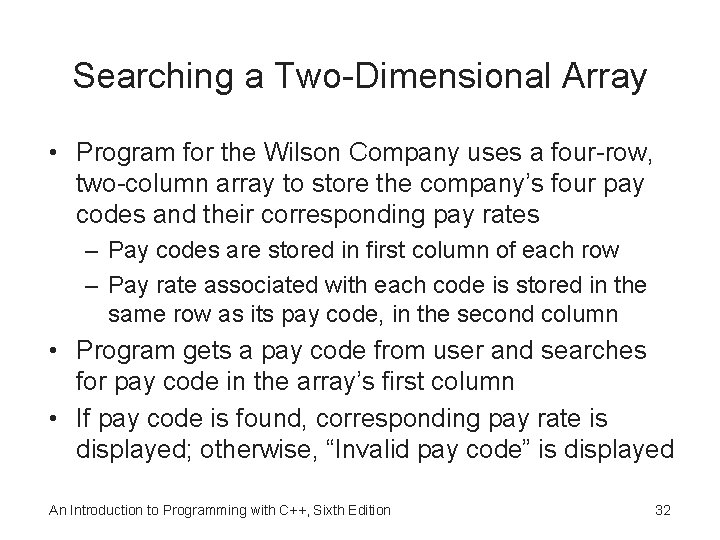
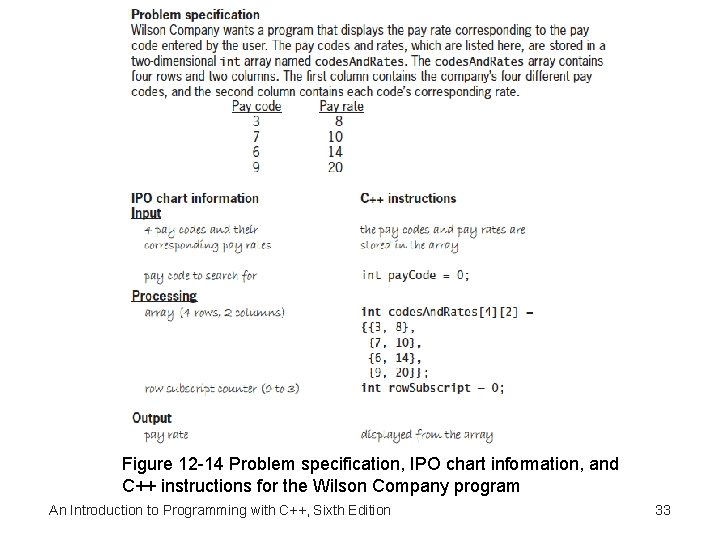
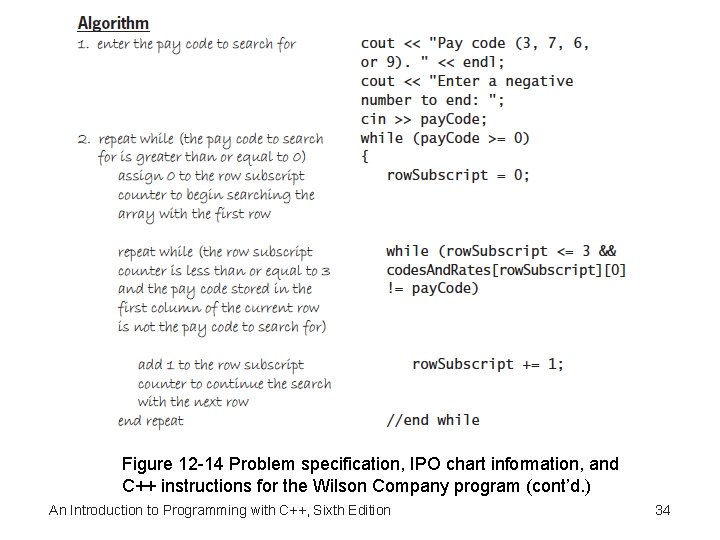
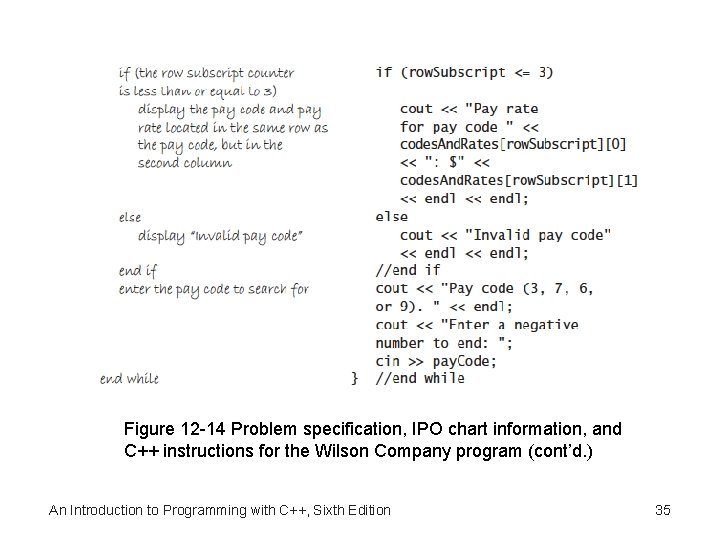
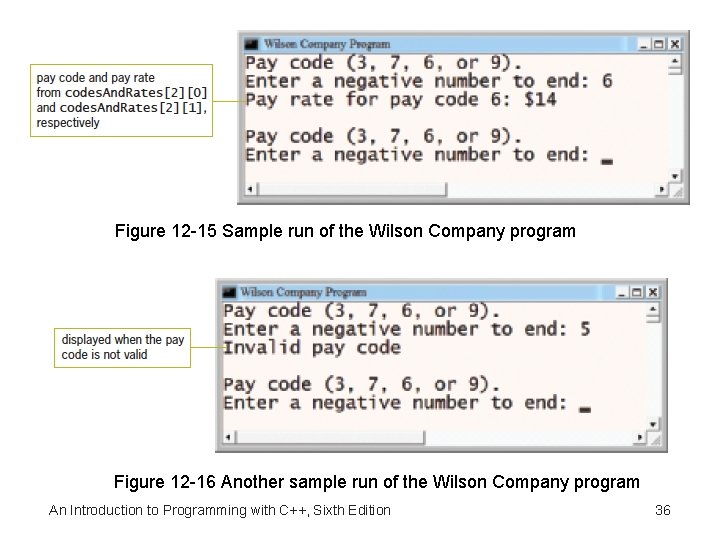
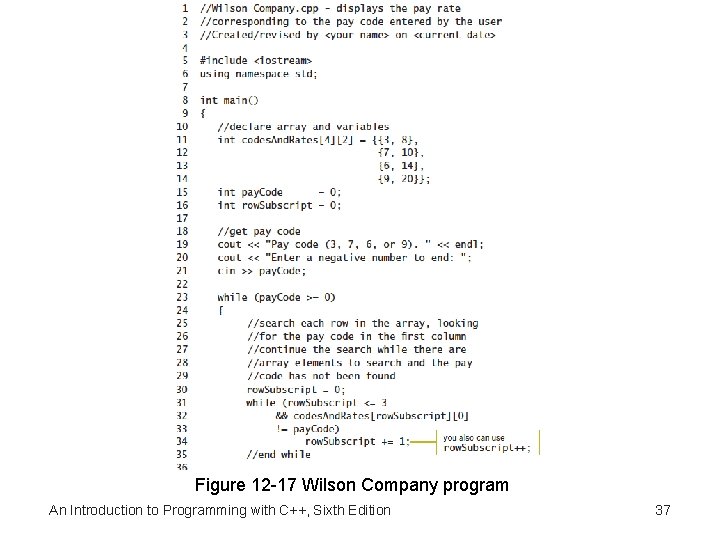
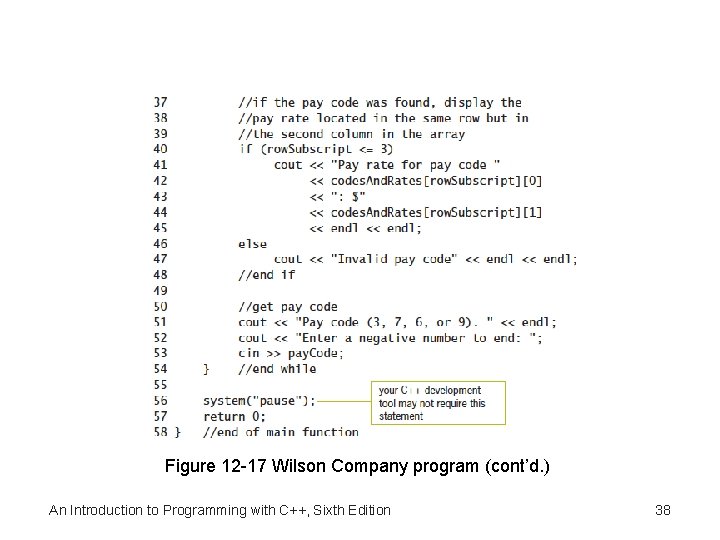
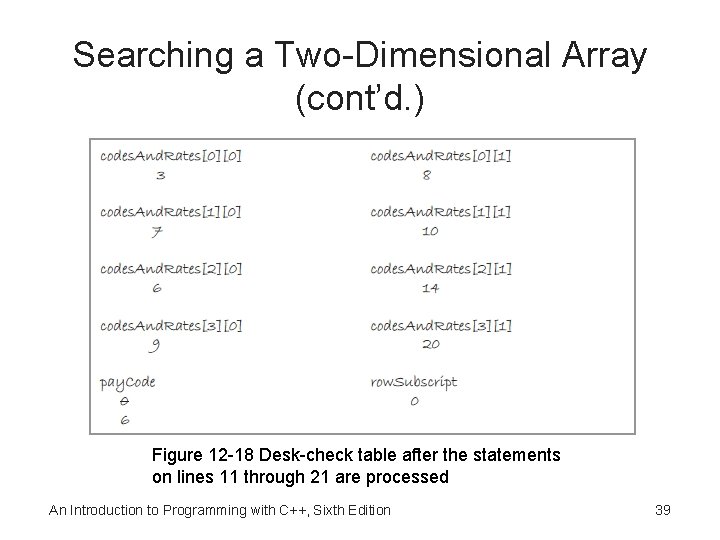
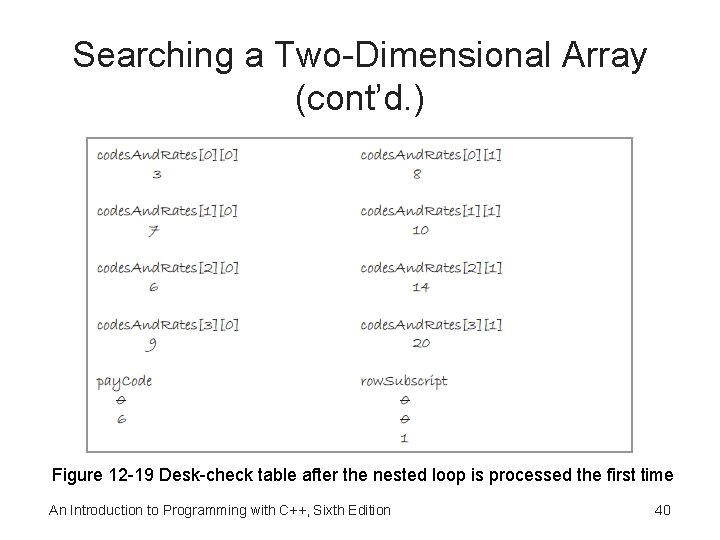
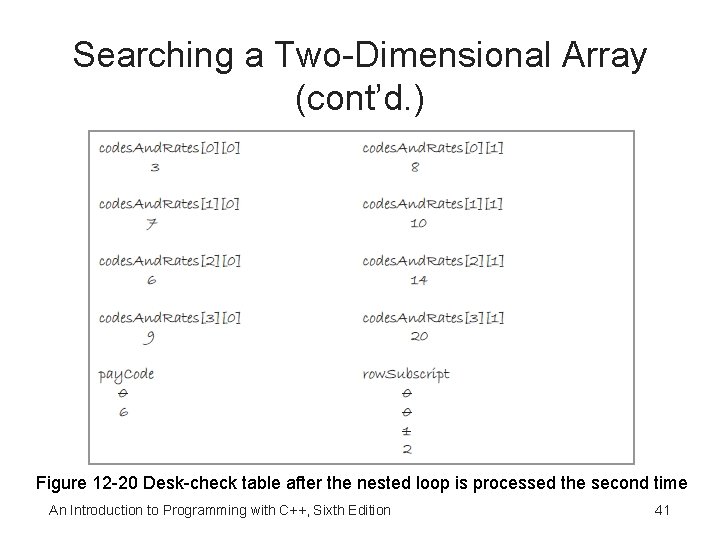
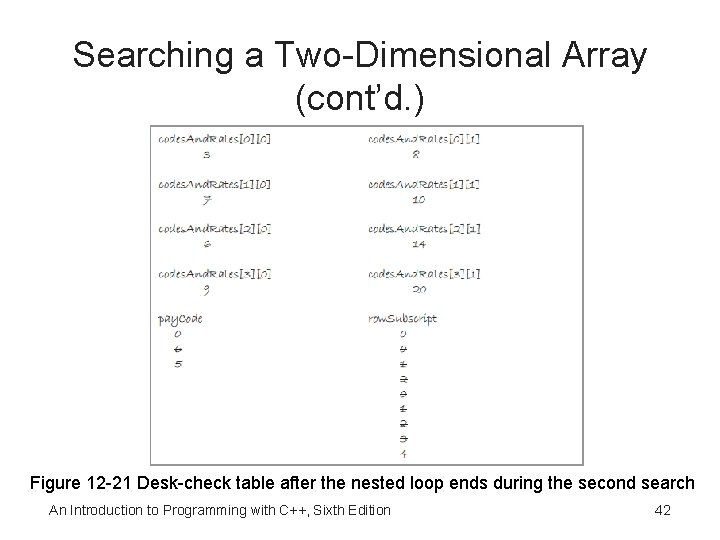
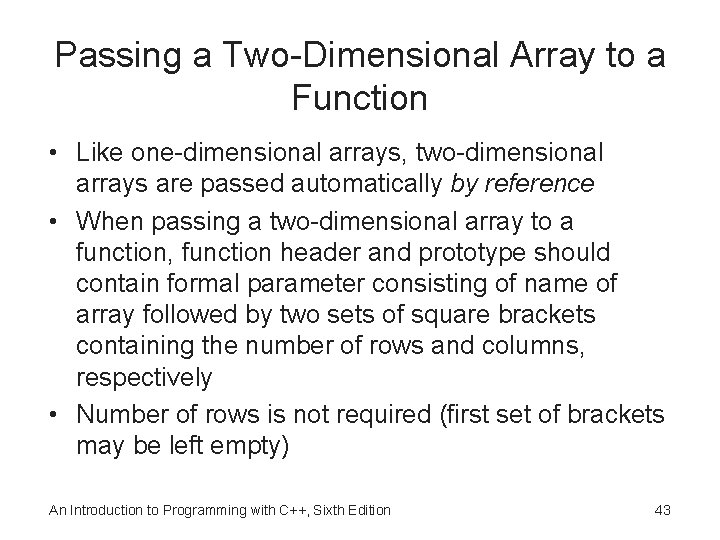
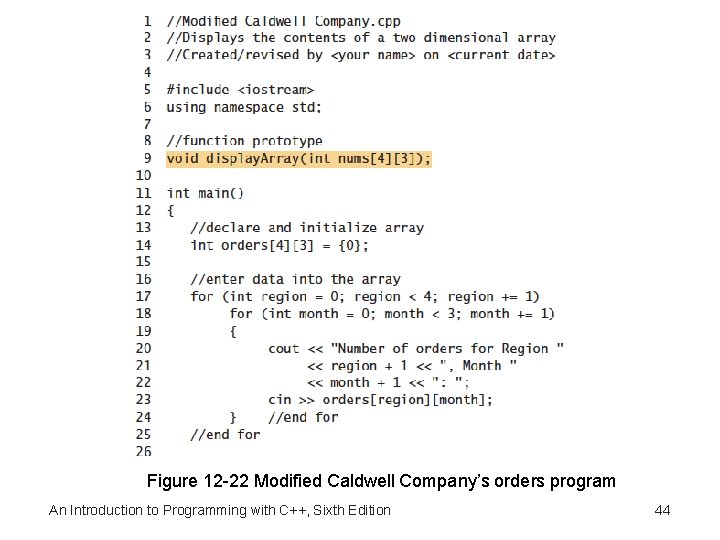
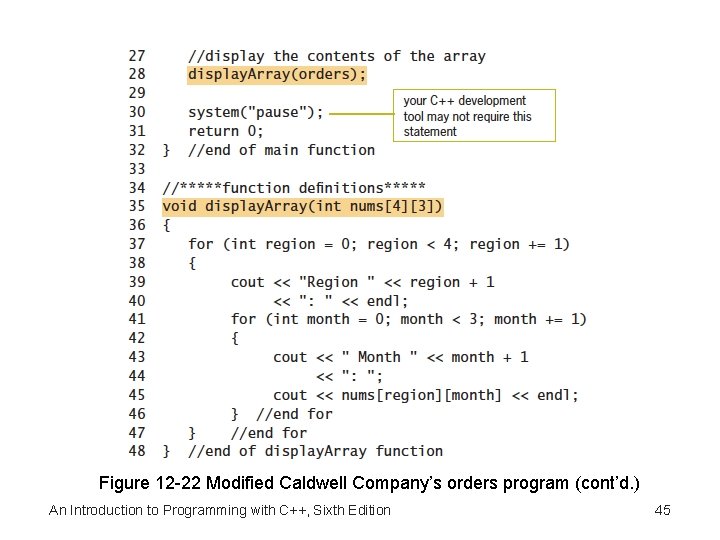
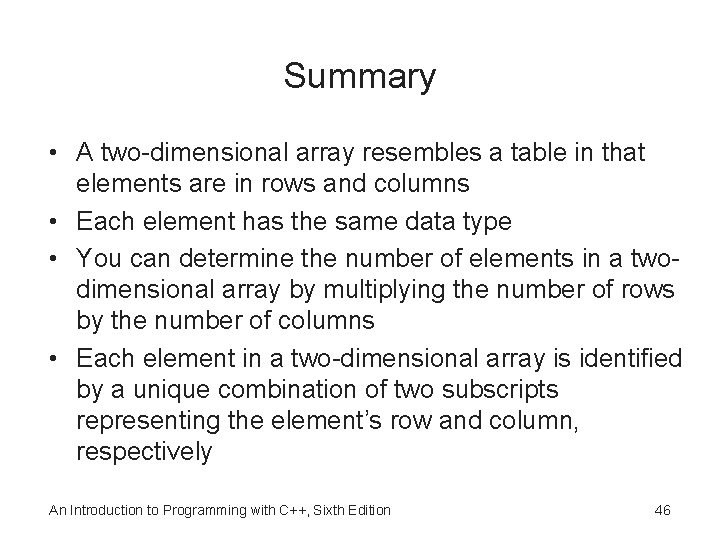
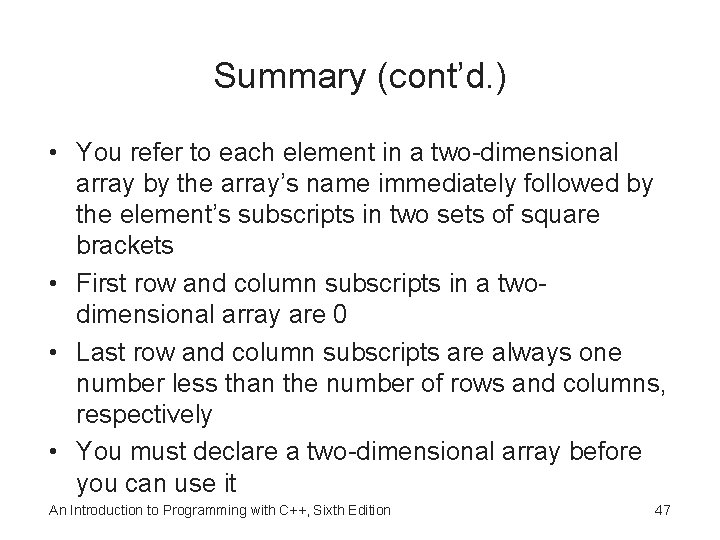
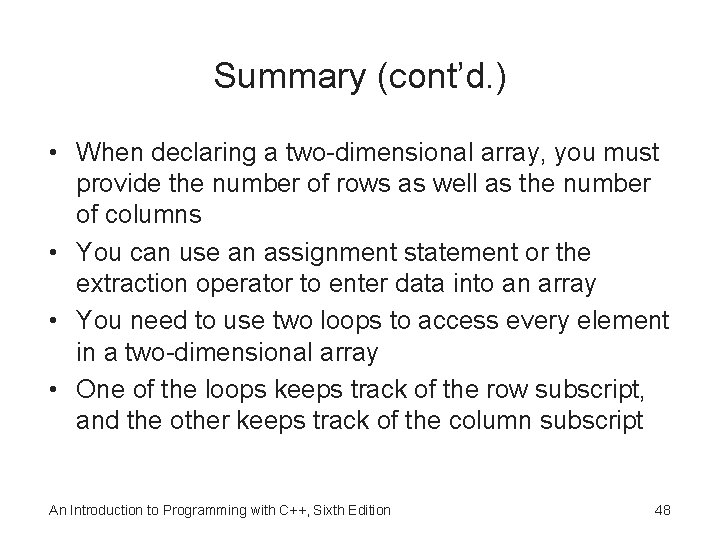
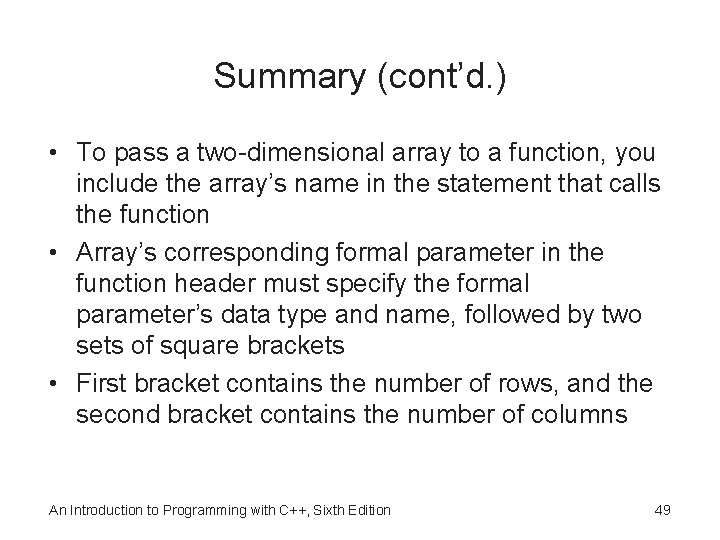
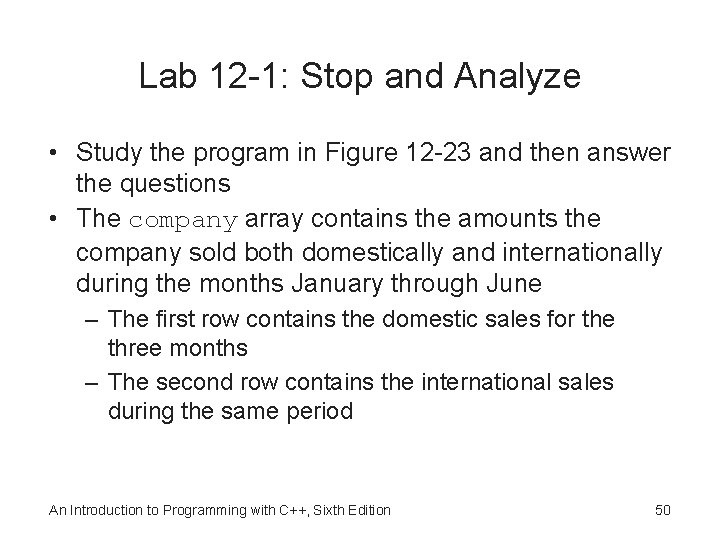
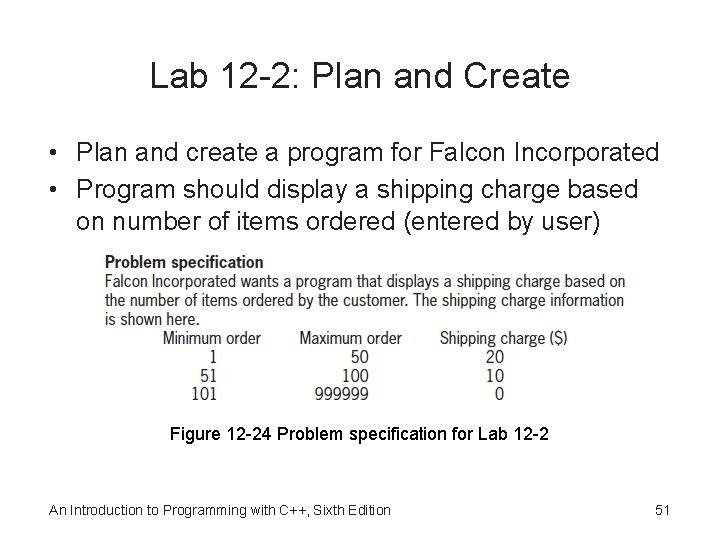
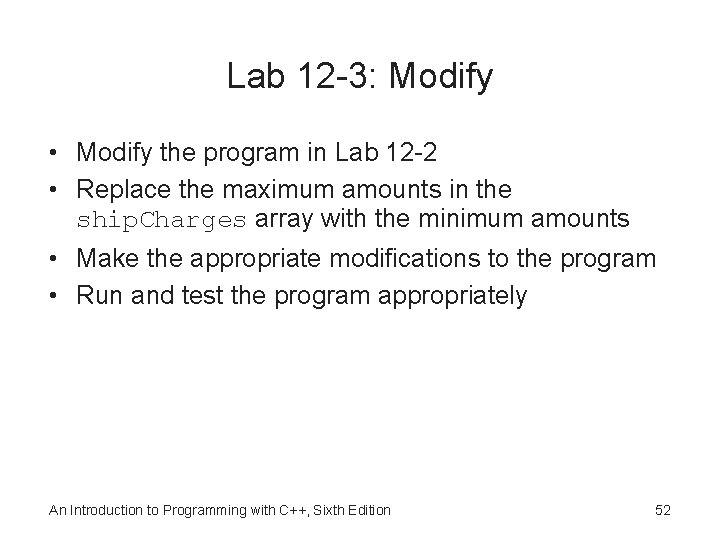
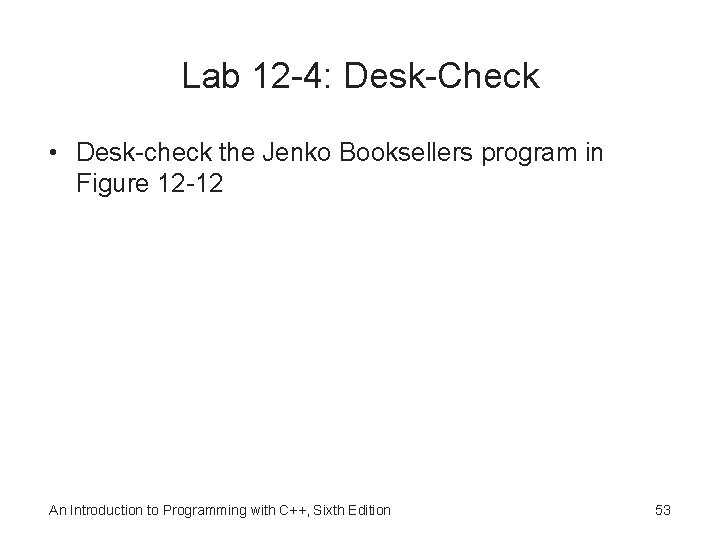
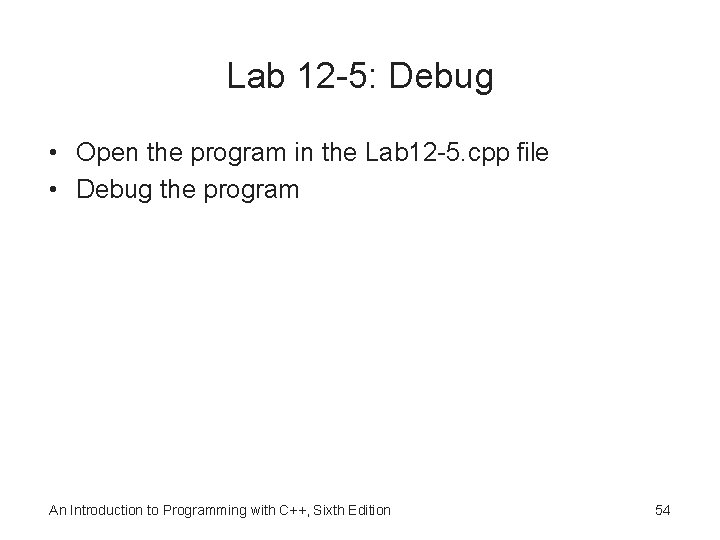
- Slides: 54
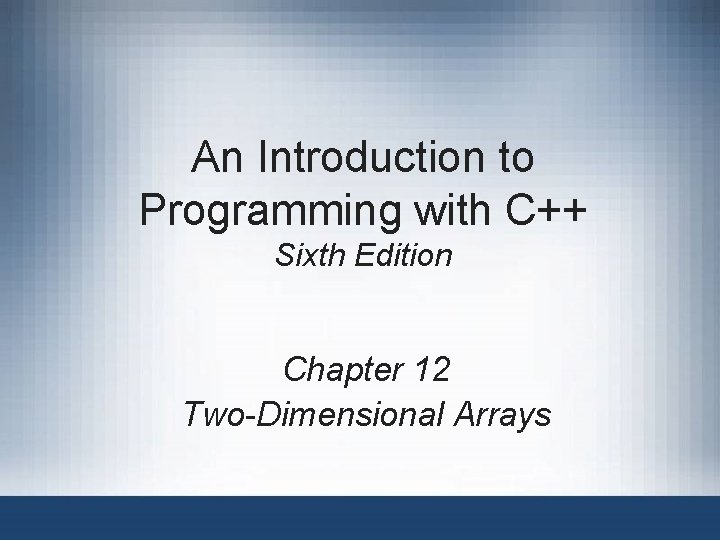
An Introduction to Programming with C++ Sixth Edition Chapter 12 Two-Dimensional Arrays
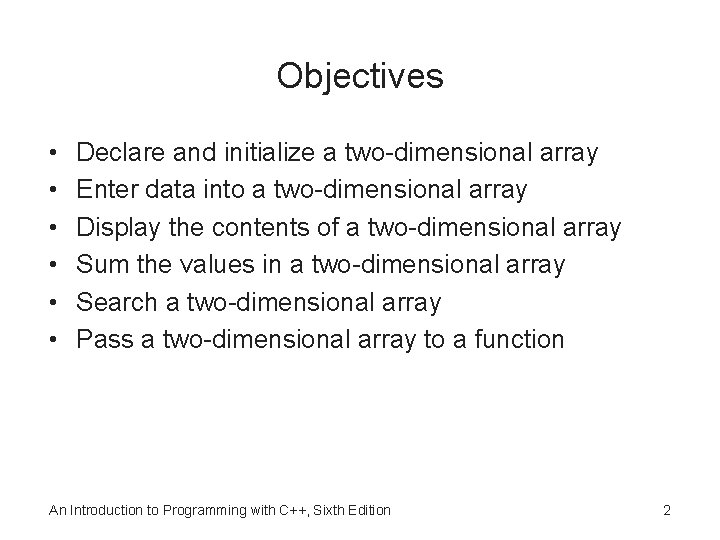
Objectives • • • Declare and initialize a two-dimensional array Enter data into a two-dimensional array Display the contents of a two-dimensional array Sum the values in a two-dimensional array Search a two-dimensional array Pass a two-dimensional array to a function An Introduction to Programming with C++, Sixth Edition 2
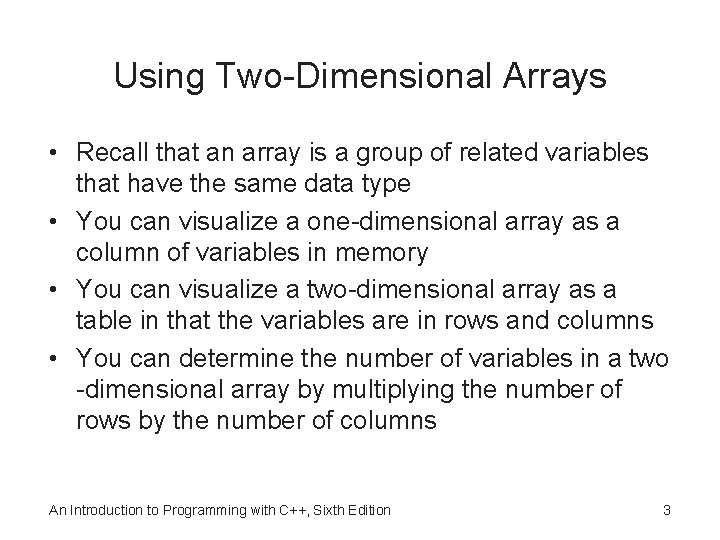
Using Two-Dimensional Arrays • Recall that an array is a group of related variables that have the same data type • You can visualize a one-dimensional array as a column of variables in memory • You can visualize a two-dimensional array as a table in that the variables are in rows and columns • You can determine the number of variables in a two -dimensional array by multiplying the number of rows by the number of columns An Introduction to Programming with C++, Sixth Edition 3
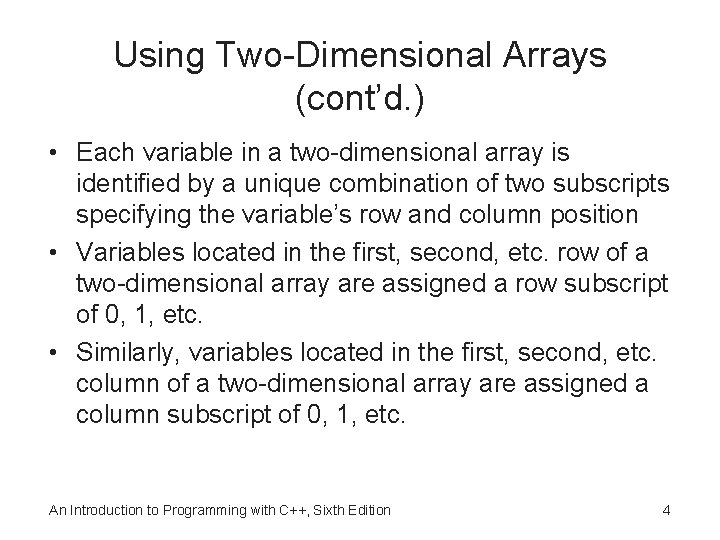
Using Two-Dimensional Arrays (cont’d. ) • Each variable in a two-dimensional array is identified by a unique combination of two subscripts specifying the variable’s row and column position • Variables located in the first, second, etc. row of a two-dimensional array are assigned a row subscript of 0, 1, etc. • Similarly, variables located in the first, second, etc. column of a two-dimensional array are assigned a column subscript of 0, 1, etc. An Introduction to Programming with C++, Sixth Edition 4
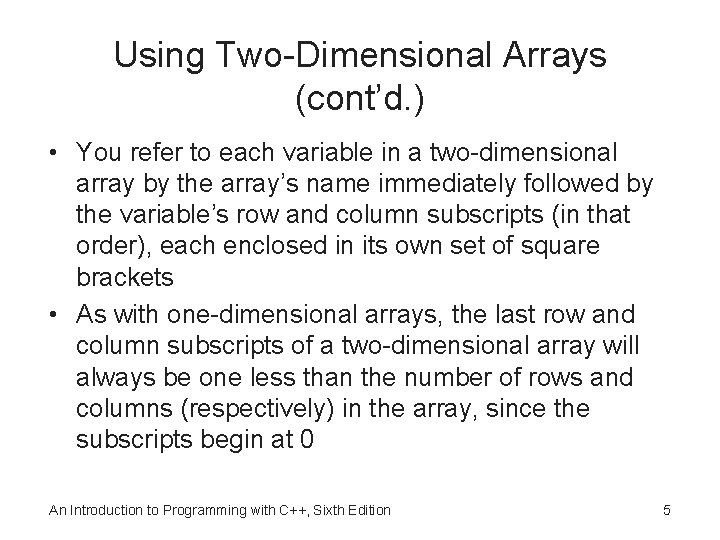
Using Two-Dimensional Arrays (cont’d. ) • You refer to each variable in a two-dimensional array by the array’s name immediately followed by the variable’s row and column subscripts (in that order), each enclosed in its own set of square brackets • As with one-dimensional arrays, the last row and column subscripts of a two-dimensional array will always be one less than the number of rows and columns (respectively) in the array, since the subscripts begin at 0 An Introduction to Programming with C++, Sixth Edition 5
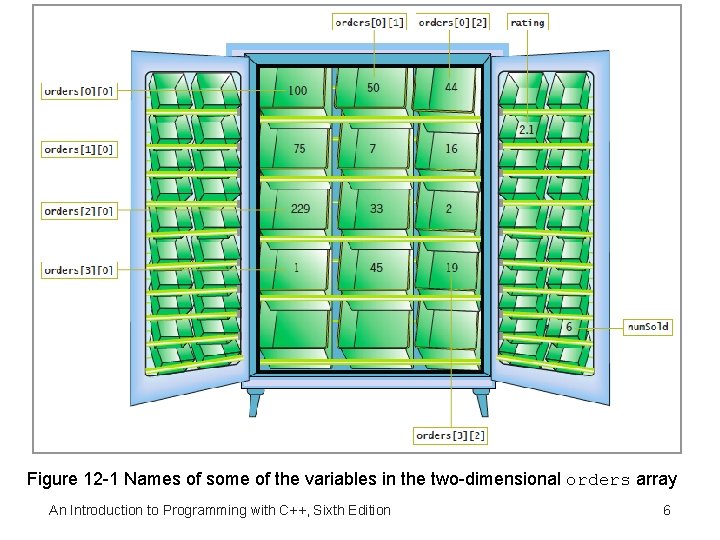
Figure 12 -1 Names of some of the variables in the two-dimensional orders array An Introduction to Programming with C++, Sixth Edition 6
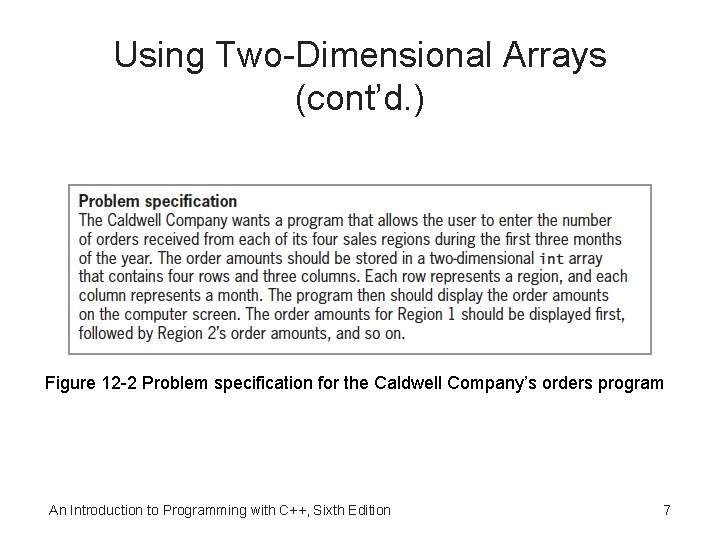
Using Two-Dimensional Arrays (cont’d. ) Figure 12 -2 Problem specification for the Caldwell Company’s orders program An Introduction to Programming with C++, Sixth Edition 7
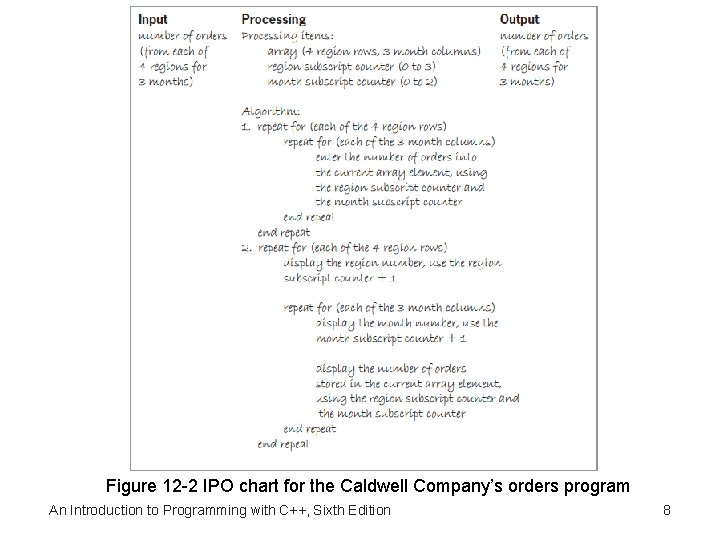
Figure 12 -2 IPO chart for the Caldwell Company’s orders program An Introduction to Programming with C++, Sixth Edition 8
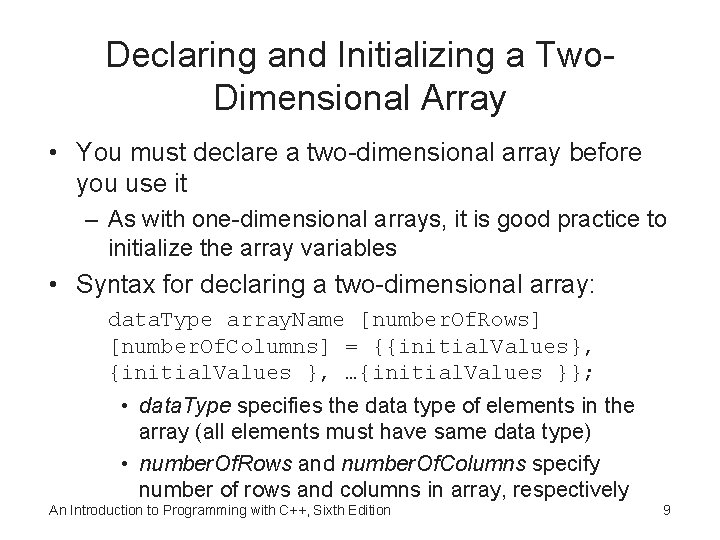
Declaring and Initializing a Two. Dimensional Array • You must declare a two-dimensional array before you use it – As with one-dimensional arrays, it is good practice to initialize the array variables • Syntax for declaring a two-dimensional array: data. Type array. Name [number. Of. Rows] [number. Of. Columns] = {{initial. Values}, {initial. Values }, …{initial. Values }}; • data. Type specifies the data type of elements in the array (all elements must have same data type) • number. Of. Rows and number. Of. Columns specify number of rows and columns in array, respectively An Introduction to Programming with C++, Sixth Edition 9
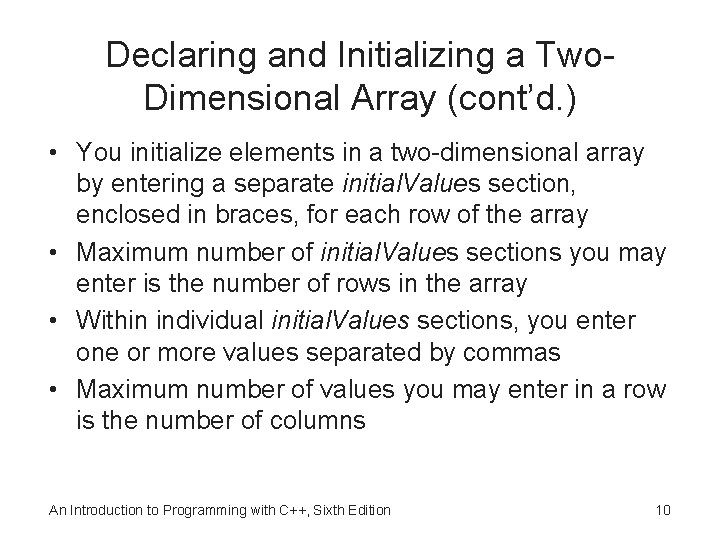
Declaring and Initializing a Two. Dimensional Array (cont’d. ) • You initialize elements in a two-dimensional array by entering a separate initial. Values section, enclosed in braces, for each row of the array • Maximum number of initial. Values sections you may enter is the number of rows in the array • Within individual initial. Values sections, you enter one or more values separated by commas • Maximum number of values you may enter in a row is the number of columns An Introduction to Programming with C++, Sixth Edition 10
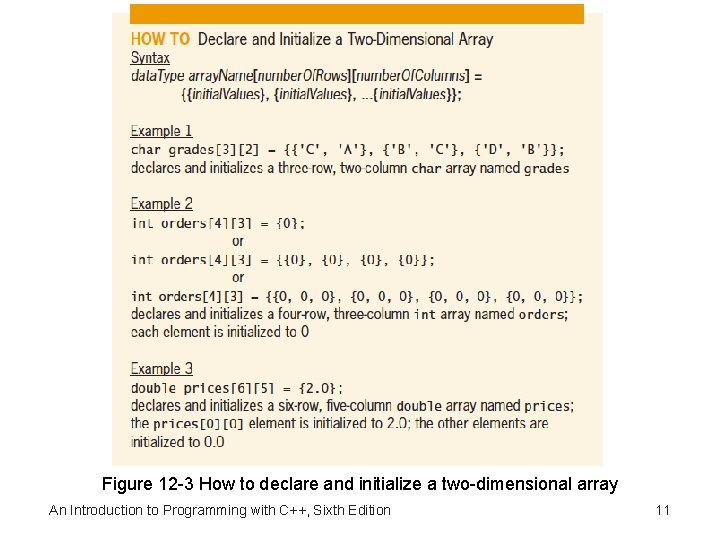
Figure 12 -3 How to declare and initialize a two-dimensional array An Introduction to Programming with C++, Sixth Edition 11
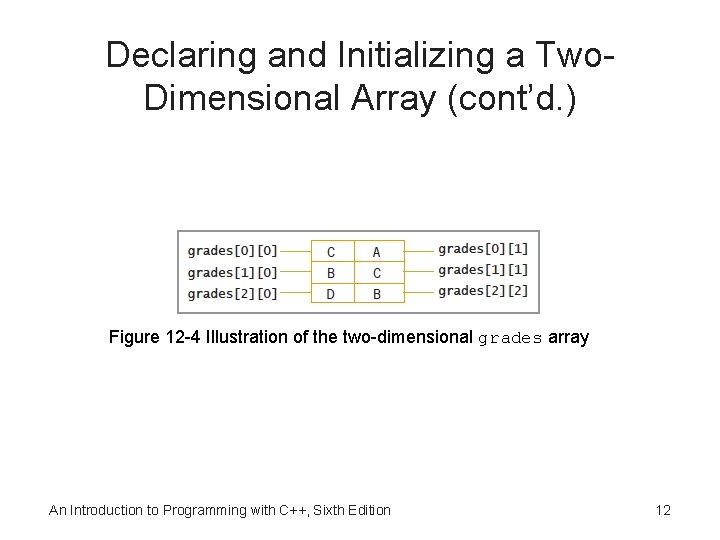
Declaring and Initializing a Two. Dimensional Array (cont’d. ) Figure 12 -4 Illustration of the two-dimensional grades array An Introduction to Programming with C++, Sixth Edition 12
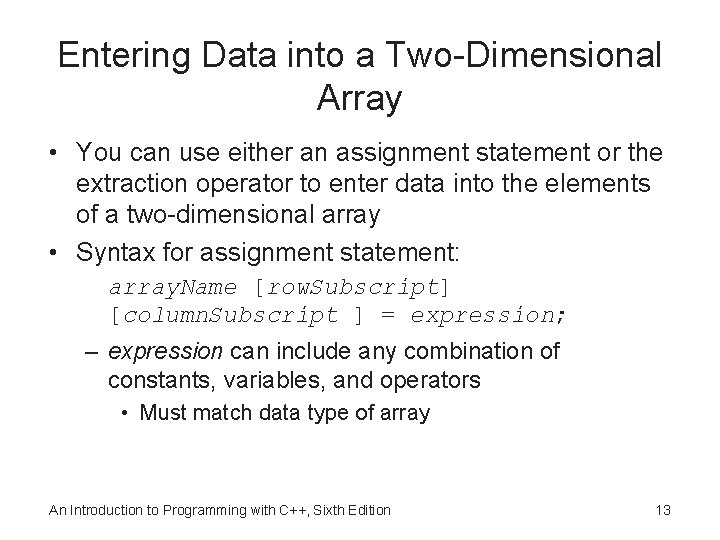
Entering Data into a Two-Dimensional Array • You can use either an assignment statement or the extraction operator to enter data into the elements of a two-dimensional array • Syntax for assignment statement: array. Name [row. Subscript] [column. Subscript ] = expression; – expression can include any combination of constants, variables, and operators • Must match data type of array An Introduction to Programming with C++, Sixth Edition 13
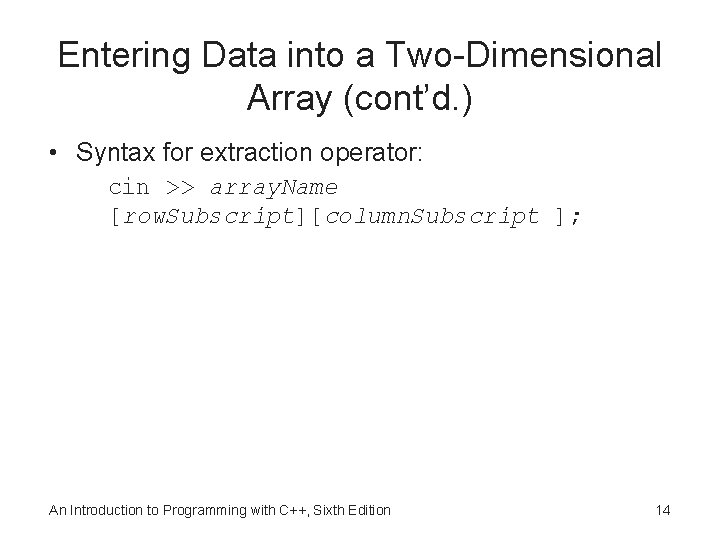
Entering Data into a Two-Dimensional Array (cont’d. ) • Syntax for extraction operator: cin >> array. Name [row. Subscript][column. Subscript ]; An Introduction to Programming with C++, Sixth Edition 14
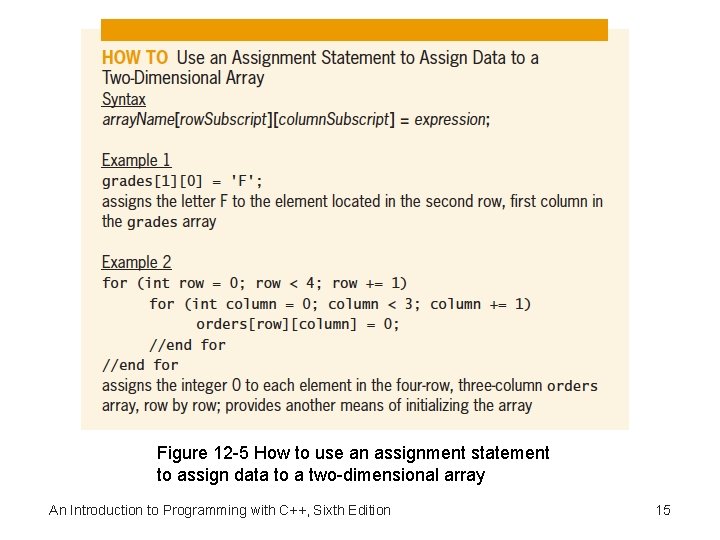
Figure 12 -5 How to use an assignment statement to assign data to a two-dimensional array An Introduction to Programming with C++, Sixth Edition 15
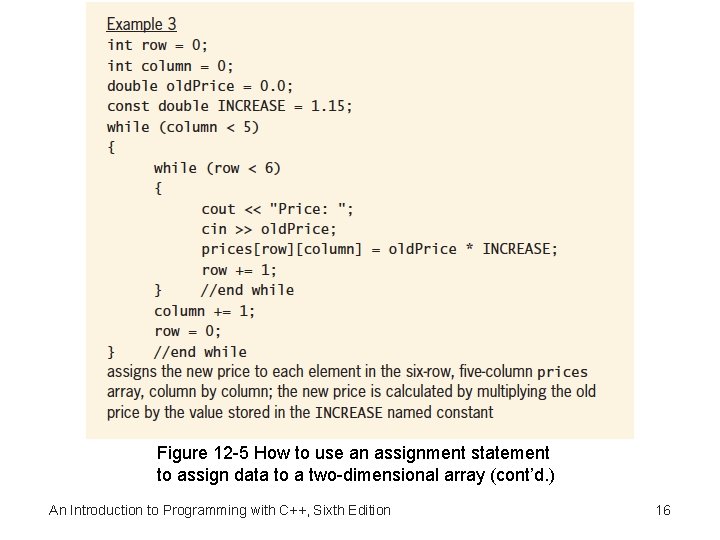
Figure 12 -5 How to use an assignment statement to assign data to a two-dimensional array (cont’d. ) An Introduction to Programming with C++, Sixth Edition 16
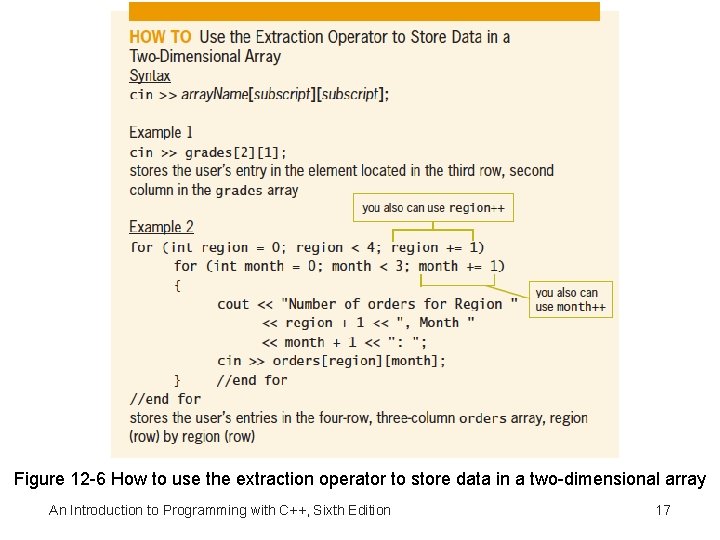
Figure 12 -6 How to use the extraction operator to store data in a two-dimensional array An Introduction to Programming with C++, Sixth Edition 17
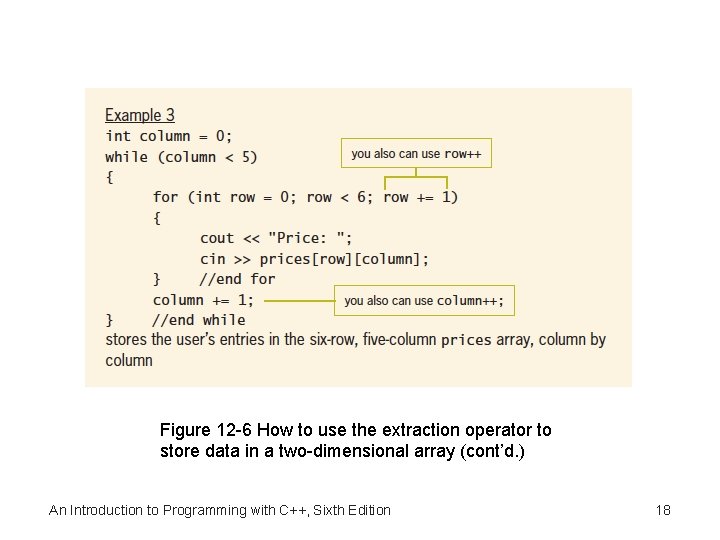
Figure 12 -6 How to use the extraction operator to store data in a two-dimensional array (cont’d. ) An Introduction to Programming with C++, Sixth Edition 18
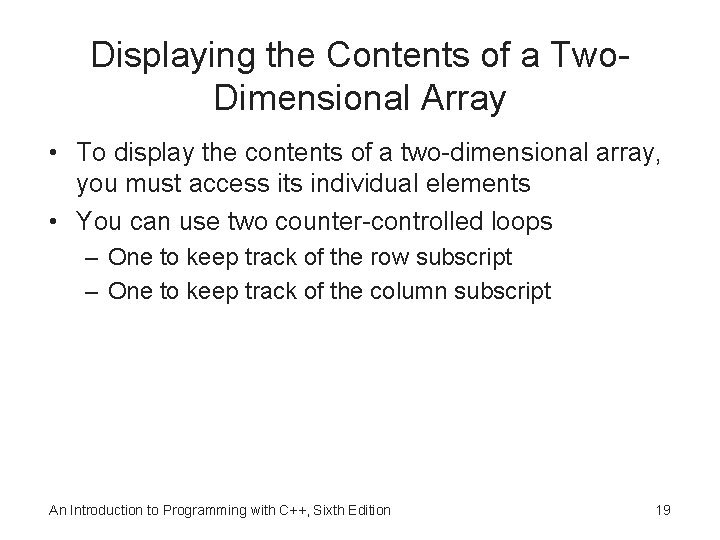
Displaying the Contents of a Two. Dimensional Array • To display the contents of a two-dimensional array, you must access its individual elements • You can use two counter-controlled loops – One to keep track of the row subscript – One to keep track of the column subscript An Introduction to Programming with C++, Sixth Edition 19
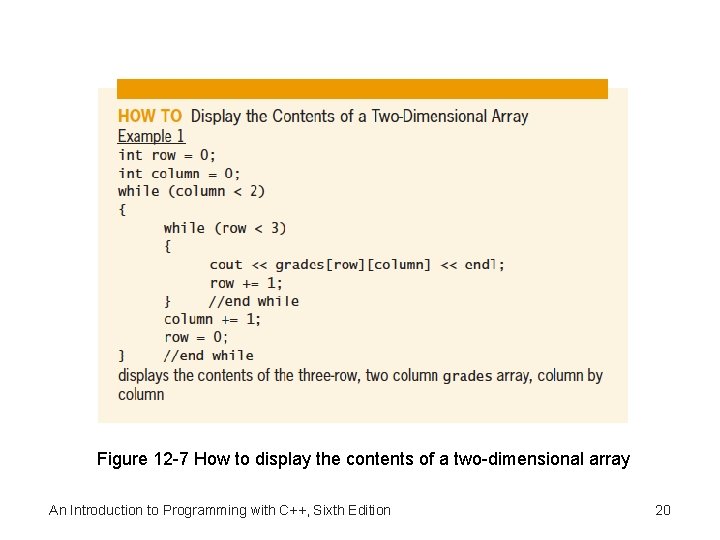
Figure 12 -7 How to display the contents of a two-dimensional array An Introduction to Programming with C++, Sixth Edition 20
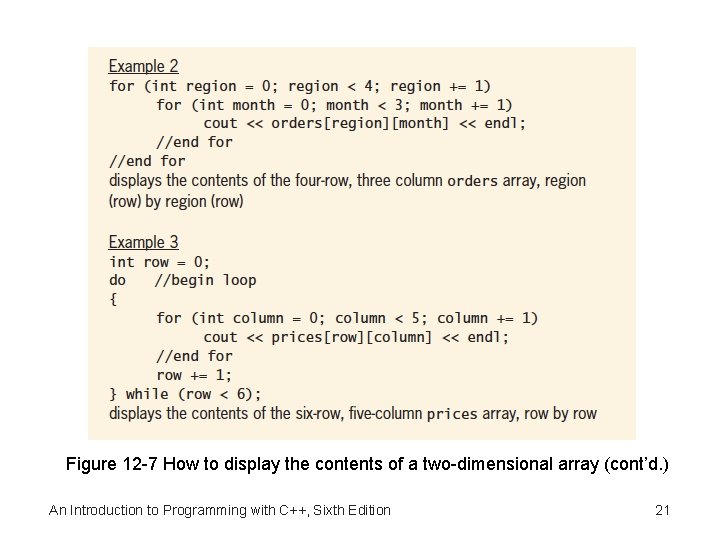
Figure 12 -7 How to display the contents of a two-dimensional array (cont’d. ) An Introduction to Programming with C++, Sixth Edition 21
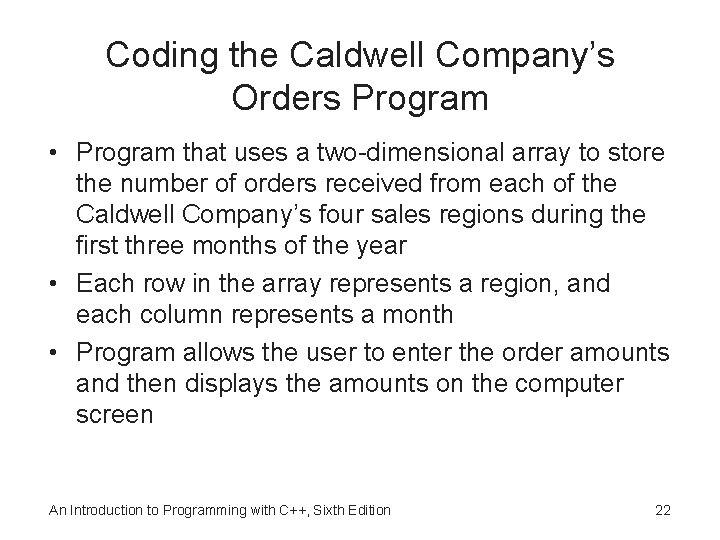
Coding the Caldwell Company’s Orders Program • Program that uses a two-dimensional array to store the number of orders received from each of the Caldwell Company’s four sales regions during the first three months of the year • Each row in the array represents a region, and each column represents a month • Program allows the user to enter the order amounts and then displays the amounts on the computer screen An Introduction to Programming with C++, Sixth Edition 22
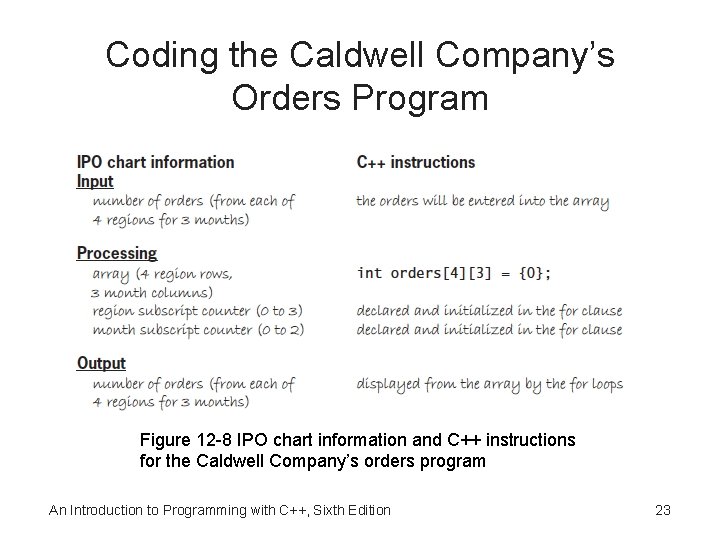
Coding the Caldwell Company’s Orders Program Figure 12 -8 IPO chart information and C++ instructions for the Caldwell Company’s orders program An Introduction to Programming with C++, Sixth Edition 23
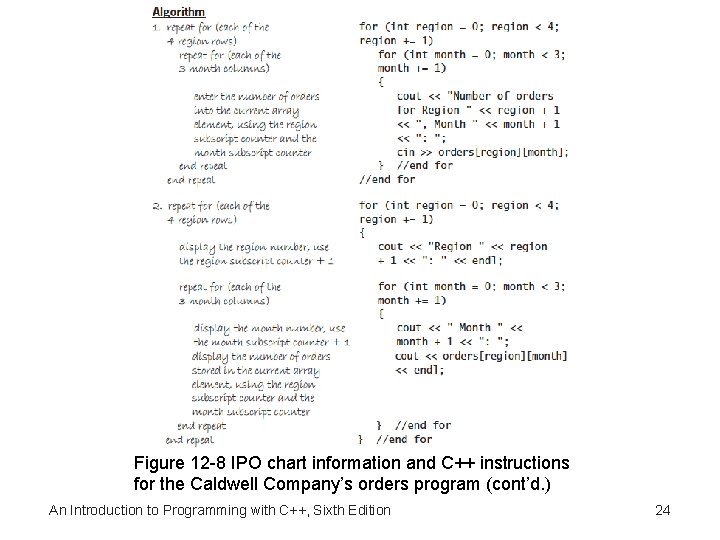
Figure 12 -8 IPO chart information and C++ instructions for the Caldwell Company’s orders program (cont’d. ) An Introduction to Programming with C++, Sixth Edition 24
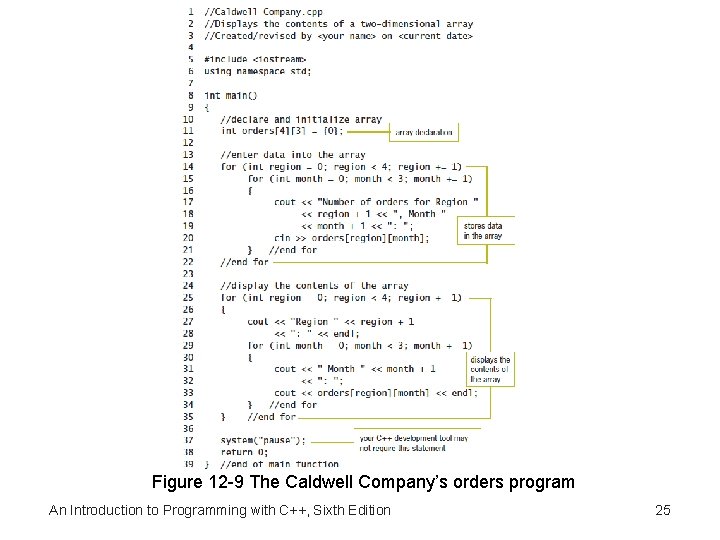
Figure 12 -9 The Caldwell Company’s orders program An Introduction to Programming with C++, Sixth Edition 25
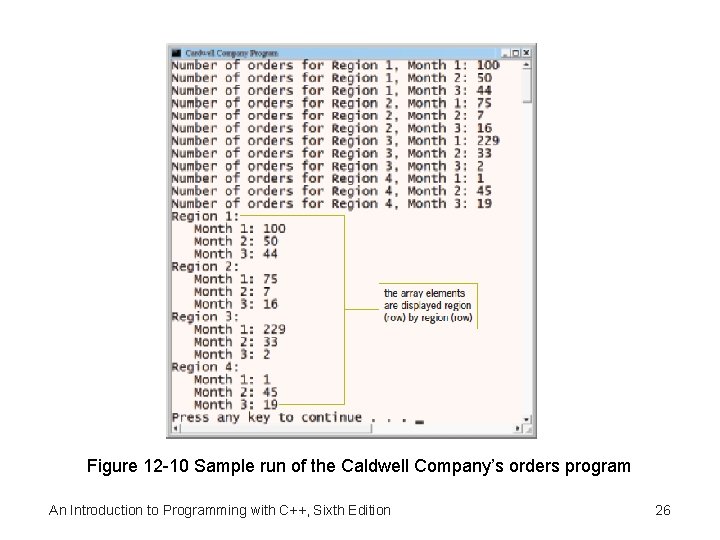
Figure 12 -10 Sample run of the Caldwell Company’s orders program An Introduction to Programming with C++, Sixth Edition 26
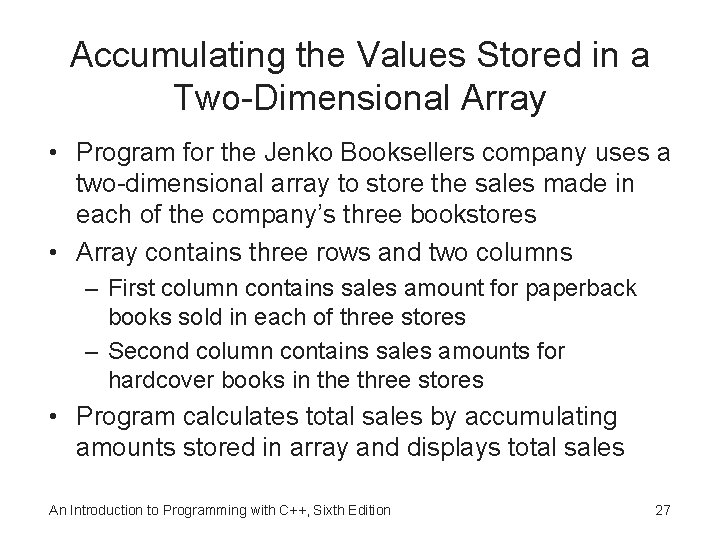
Accumulating the Values Stored in a Two-Dimensional Array • Program for the Jenko Booksellers company uses a two-dimensional array to store the sales made in each of the company’s three bookstores • Array contains three rows and two columns – First column contains sales amount for paperback books sold in each of three stores – Second column contains sales amounts for hardcover books in the three stores • Program calculates total sales by accumulating amounts stored in array and displays total sales An Introduction to Programming with C++, Sixth Edition 27
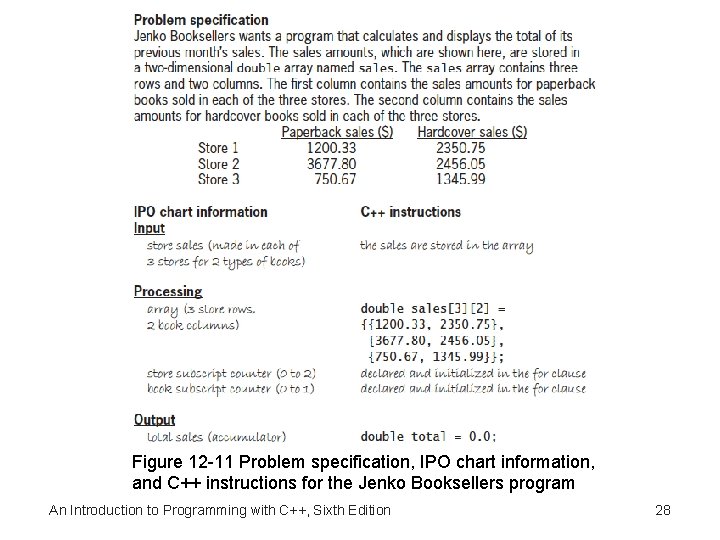
Figure 12 -11 Problem specification, IPO chart information, and C++ instructions for the Jenko Booksellers program An Introduction to Programming with C++, Sixth Edition 28
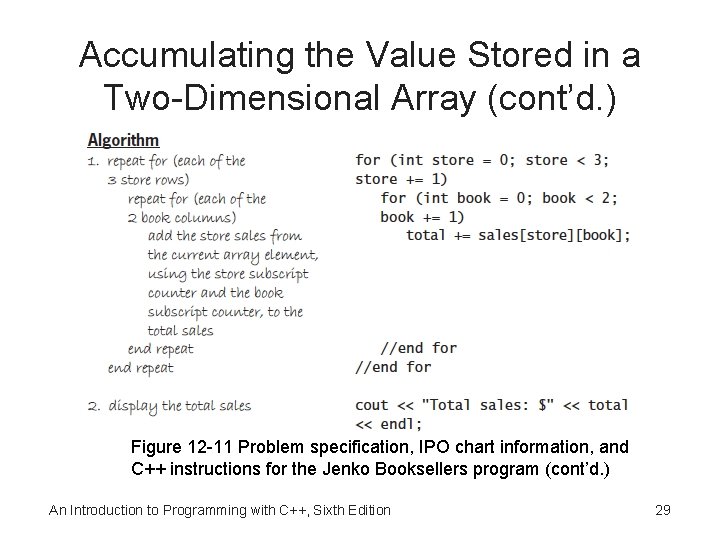
Accumulating the Value Stored in a Two-Dimensional Array (cont’d. ) Figure 12 -11 Problem specification, IPO chart information, and C++ instructions for the Jenko Booksellers program (cont’d. ) An Introduction to Programming with C++, Sixth Edition 29
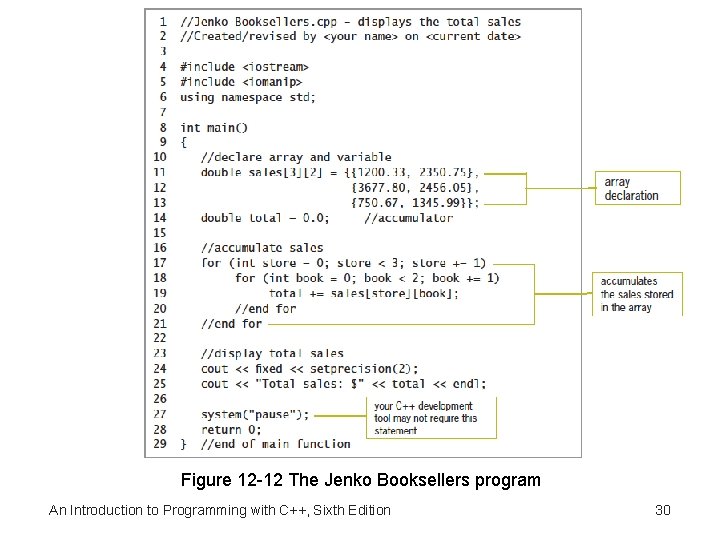
Figure 12 -12 The Jenko Booksellers program An Introduction to Programming with C++, Sixth Edition 30
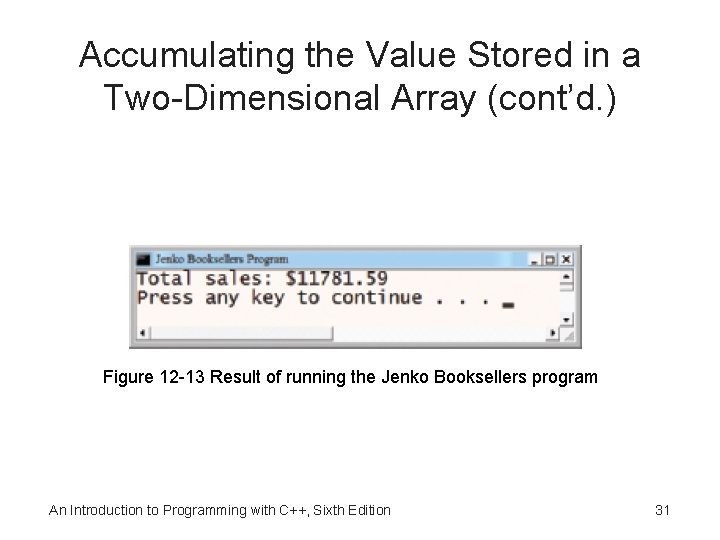
Accumulating the Value Stored in a Two-Dimensional Array (cont’d. ) Figure 12 -13 Result of running the Jenko Booksellers program An Introduction to Programming with C++, Sixth Edition 31
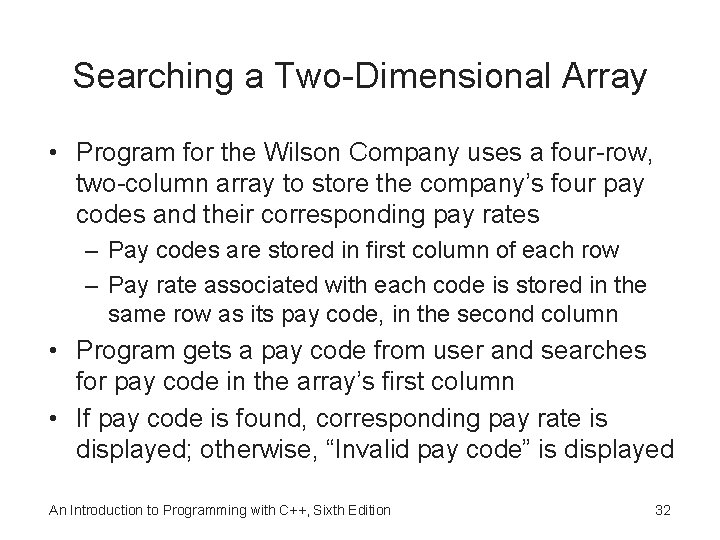
Searching a Two-Dimensional Array • Program for the Wilson Company uses a four-row, two-column array to store the company’s four pay codes and their corresponding pay rates – Pay codes are stored in first column of each row – Pay rate associated with each code is stored in the same row as its pay code, in the second column • Program gets a pay code from user and searches for pay code in the array’s first column • If pay code is found, corresponding pay rate is displayed; otherwise, “Invalid pay code” is displayed An Introduction to Programming with C++, Sixth Edition 32
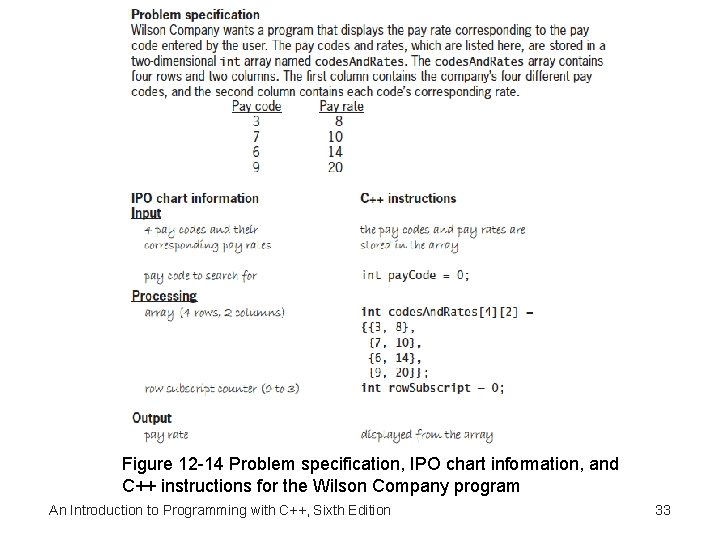
Figure 12 -14 Problem specification, IPO chart information, and C++ instructions for the Wilson Company program An Introduction to Programming with C++, Sixth Edition 33
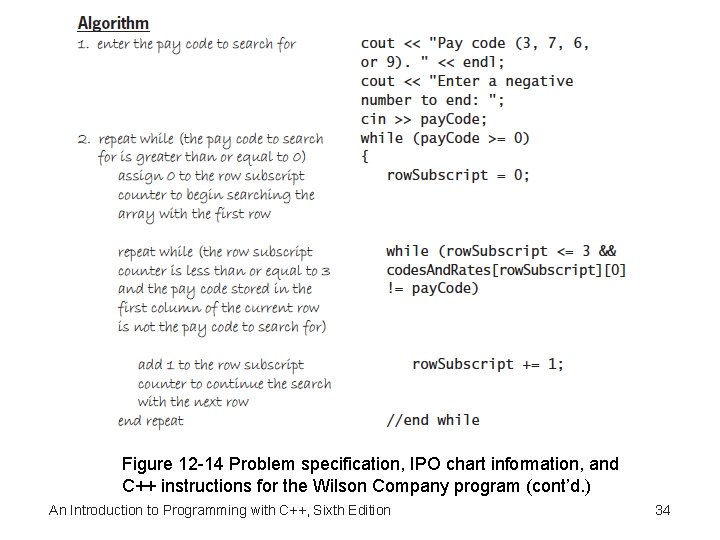
Figure 12 -14 Problem specification, IPO chart information, and C++ instructions for the Wilson Company program (cont’d. ) An Introduction to Programming with C++, Sixth Edition 34
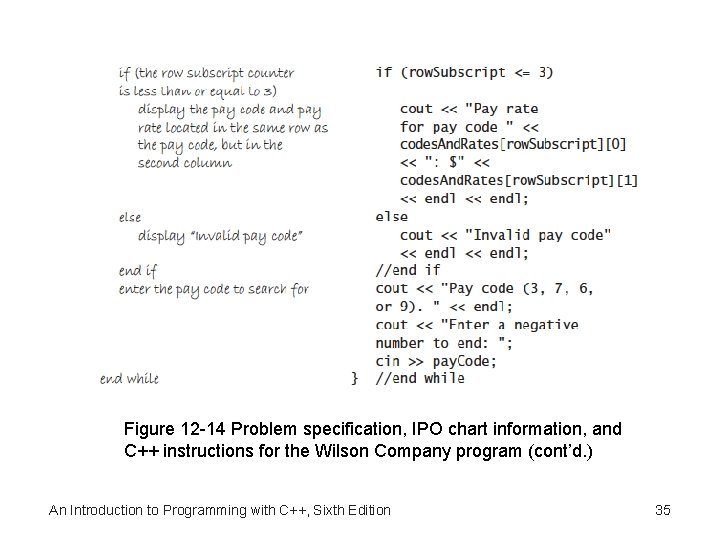
Figure 12 -14 Problem specification, IPO chart information, and C++ instructions for the Wilson Company program (cont’d. ) An Introduction to Programming with C++, Sixth Edition 35
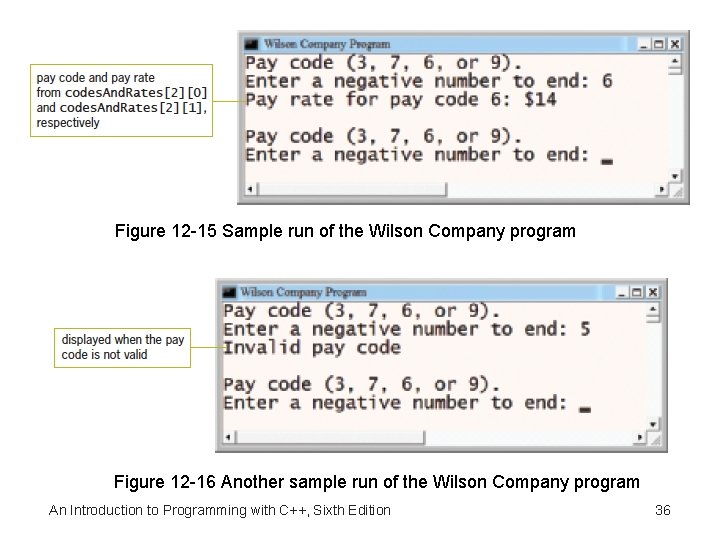
Figure 12 -15 Sample run of the Wilson Company program Figure 12 -16 Another sample run of the Wilson Company program An Introduction to Programming with C++, Sixth Edition 36
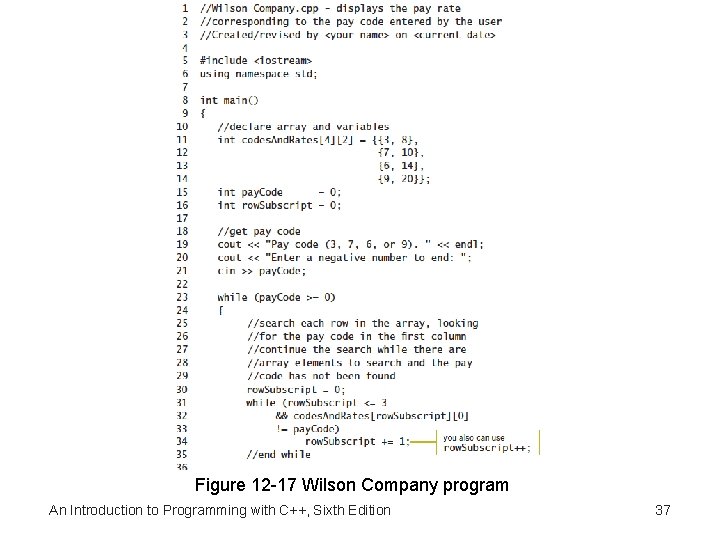
Figure 12 -17 Wilson Company program An Introduction to Programming with C++, Sixth Edition 37
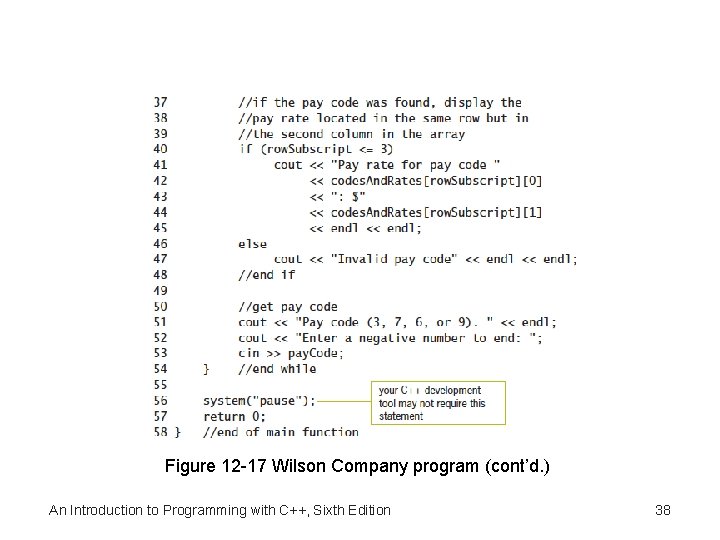
Figure 12 -17 Wilson Company program (cont’d. ) An Introduction to Programming with C++, Sixth Edition 38
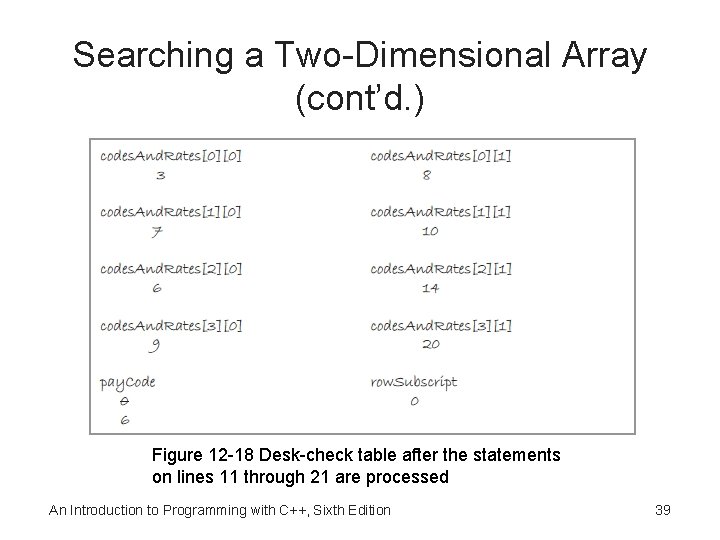
Searching a Two-Dimensional Array (cont’d. ) Figure 12 -18 Desk-check table after the statements on lines 11 through 21 are processed An Introduction to Programming with C++, Sixth Edition 39
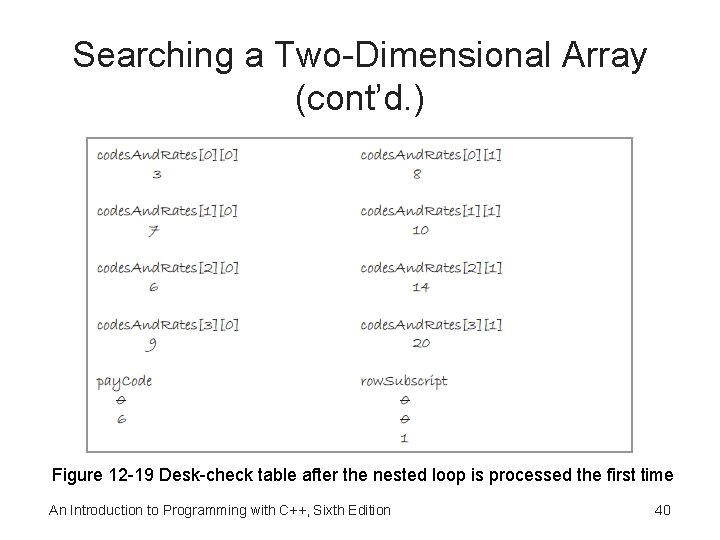
Searching a Two-Dimensional Array (cont’d. ) Figure 12 -19 Desk-check table after the nested loop is processed the first time An Introduction to Programming with C++, Sixth Edition 40
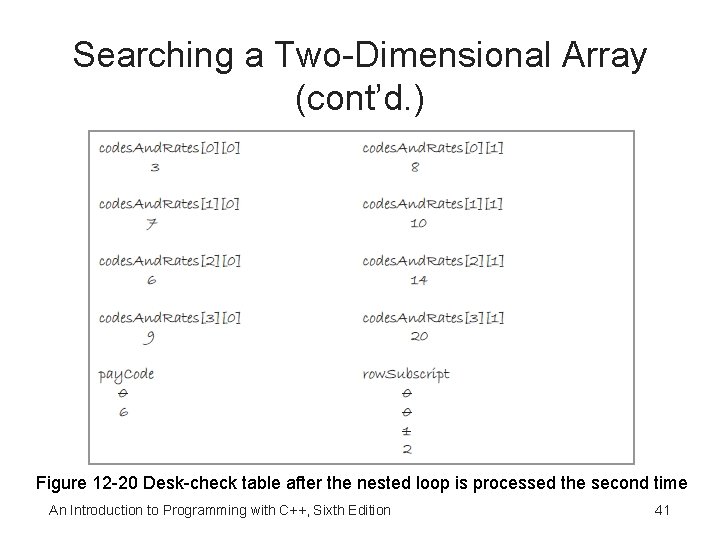
Searching a Two-Dimensional Array (cont’d. ) Figure 12 -20 Desk-check table after the nested loop is processed the second time An Introduction to Programming with C++, Sixth Edition 41
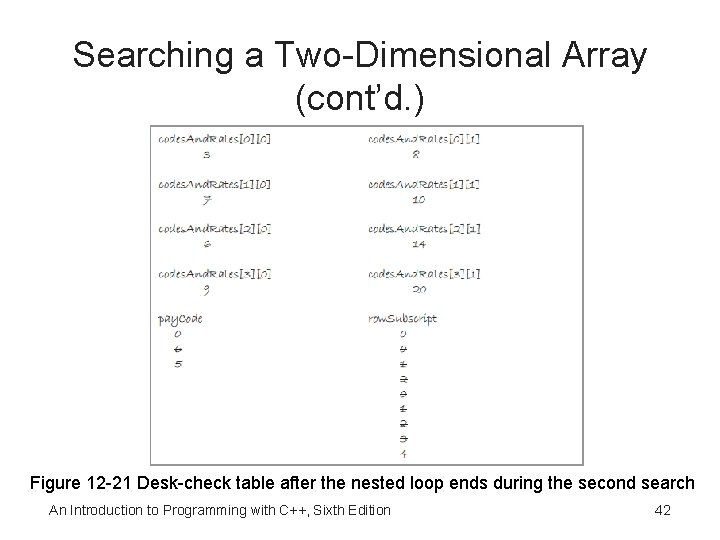
Searching a Two-Dimensional Array (cont’d. ) Figure 12 -21 Desk-check table after the nested loop ends during the second search An Introduction to Programming with C++, Sixth Edition 42
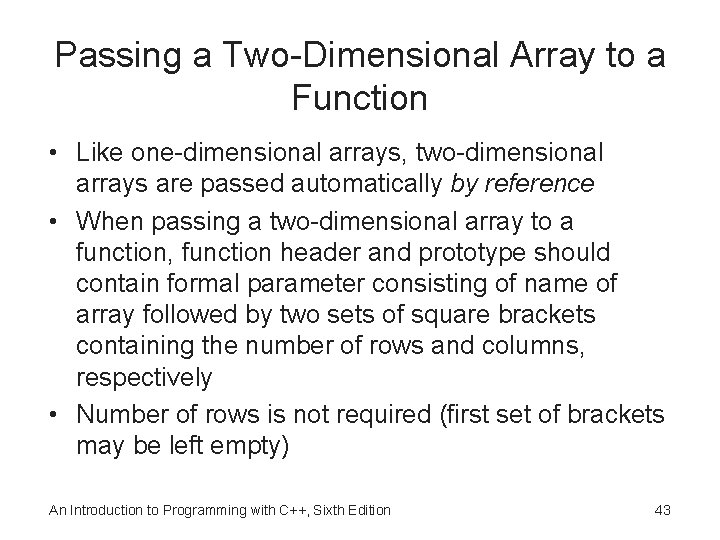
Passing a Two-Dimensional Array to a Function • Like one-dimensional arrays, two-dimensional arrays are passed automatically by reference • When passing a two-dimensional array to a function, function header and prototype should contain formal parameter consisting of name of array followed by two sets of square brackets containing the number of rows and columns, respectively • Number of rows is not required (first set of brackets may be left empty) An Introduction to Programming with C++, Sixth Edition 43
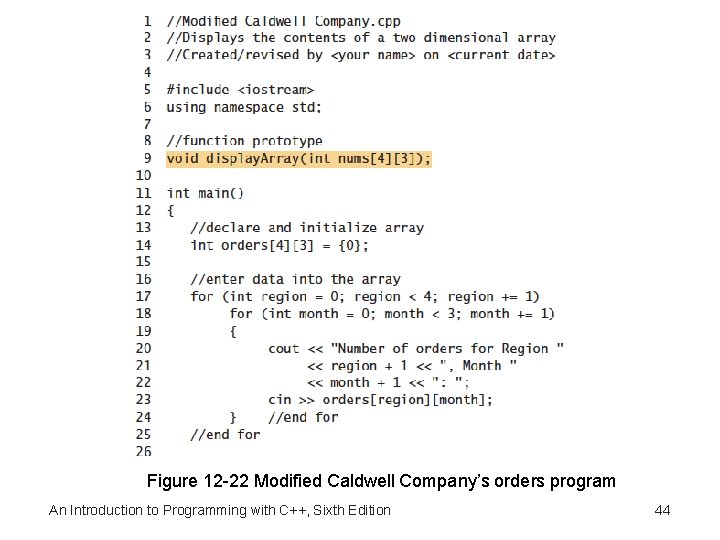
Figure 12 -22 Modified Caldwell Company’s orders program An Introduction to Programming with C++, Sixth Edition 44
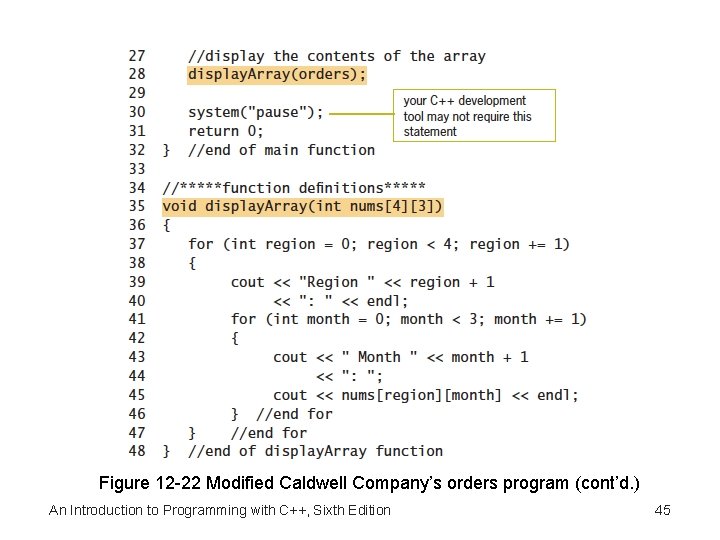
Figure 12 -22 Modified Caldwell Company’s orders program (cont’d. ) An Introduction to Programming with C++, Sixth Edition 45
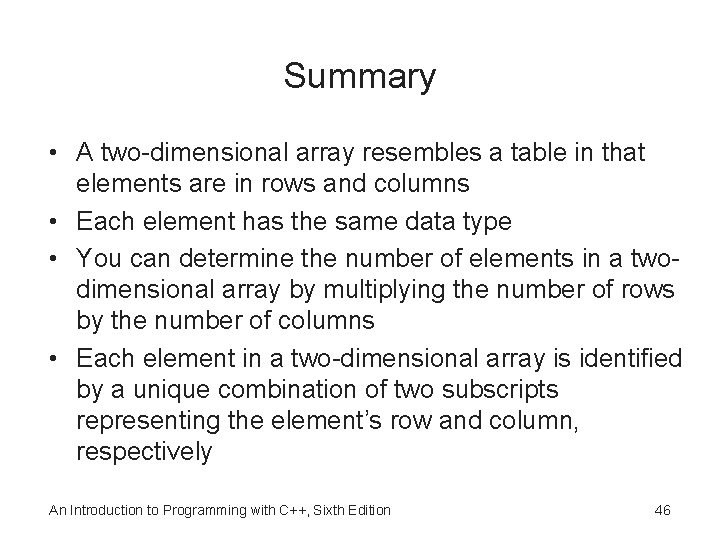
Summary • A two-dimensional array resembles a table in that elements are in rows and columns • Each element has the same data type • You can determine the number of elements in a twodimensional array by multiplying the number of rows by the number of columns • Each element in a two-dimensional array is identified by a unique combination of two subscripts representing the element’s row and column, respectively An Introduction to Programming with C++, Sixth Edition 46
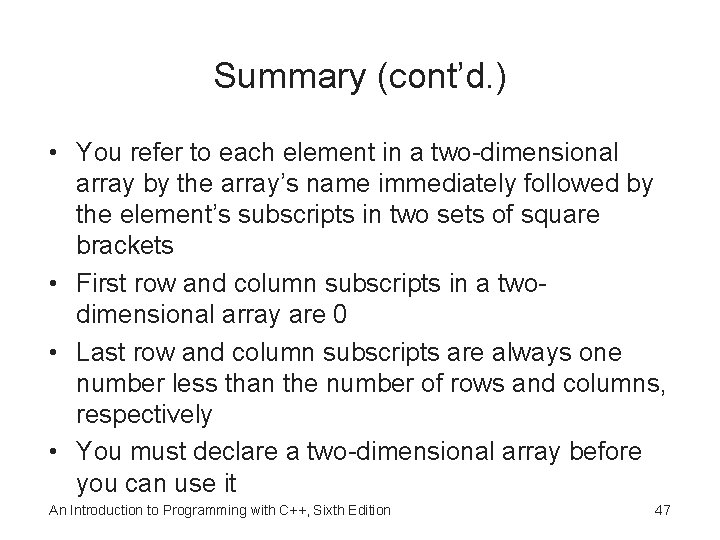
Summary (cont’d. ) • You refer to each element in a two-dimensional array by the array’s name immediately followed by the element’s subscripts in two sets of square brackets • First row and column subscripts in a twodimensional array are 0 • Last row and column subscripts are always one number less than the number of rows and columns, respectively • You must declare a two-dimensional array before you can use it An Introduction to Programming with C++, Sixth Edition 47
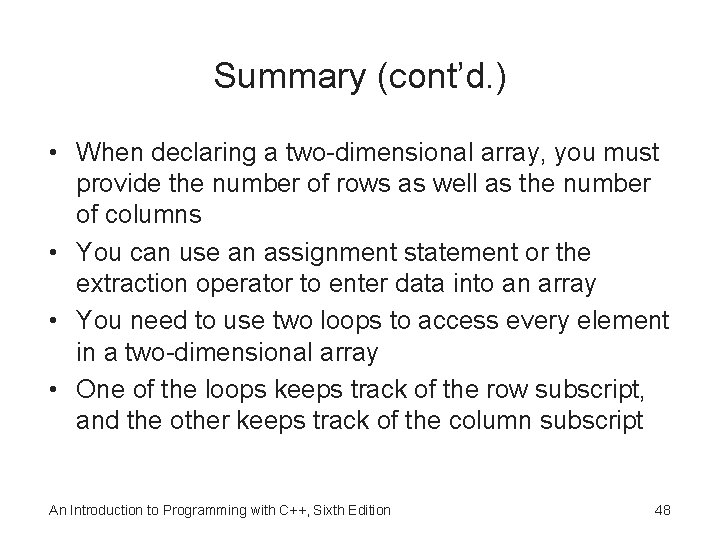
Summary (cont’d. ) • When declaring a two-dimensional array, you must provide the number of rows as well as the number of columns • You can use an assignment statement or the extraction operator to enter data into an array • You need to use two loops to access every element in a two-dimensional array • One of the loops keeps track of the row subscript, and the other keeps track of the column subscript An Introduction to Programming with C++, Sixth Edition 48
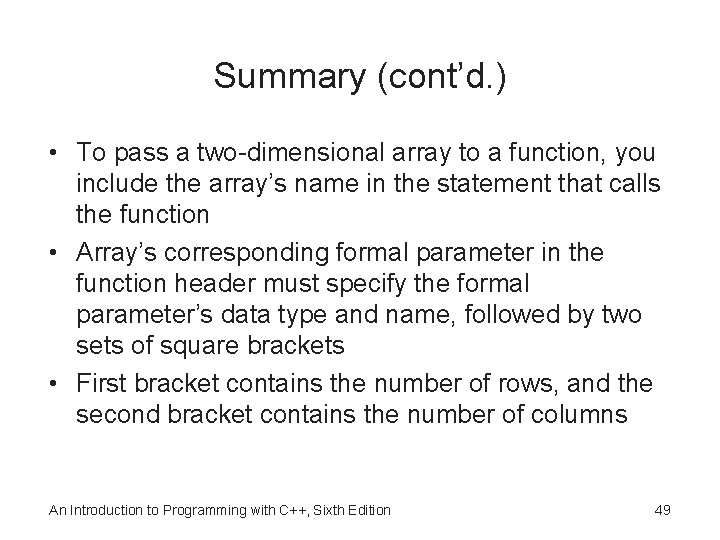
Summary (cont’d. ) • To pass a two-dimensional array to a function, you include the array’s name in the statement that calls the function • Array’s corresponding formal parameter in the function header must specify the formal parameter’s data type and name, followed by two sets of square brackets • First bracket contains the number of rows, and the second bracket contains the number of columns An Introduction to Programming with C++, Sixth Edition 49
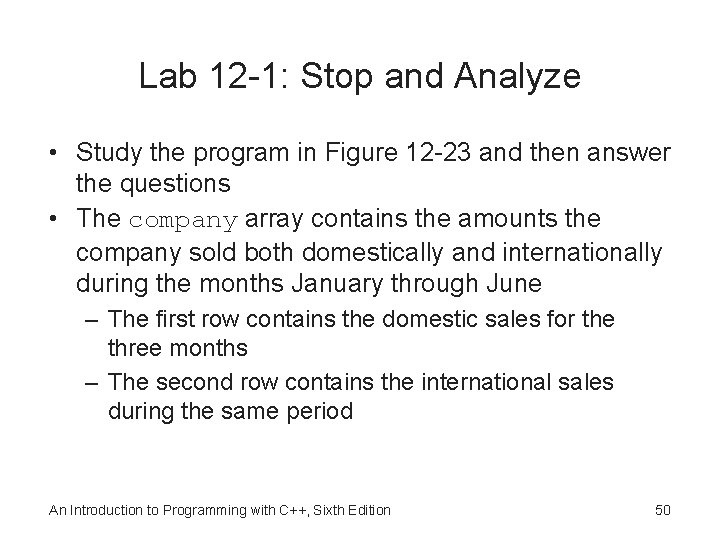
Lab 12 -1: Stop and Analyze • Study the program in Figure 12 -23 and then answer the questions • The company array contains the amounts the company sold both domestically and internationally during the months January through June – The first row contains the domestic sales for the three months – The second row contains the international sales during the same period An Introduction to Programming with C++, Sixth Edition 50
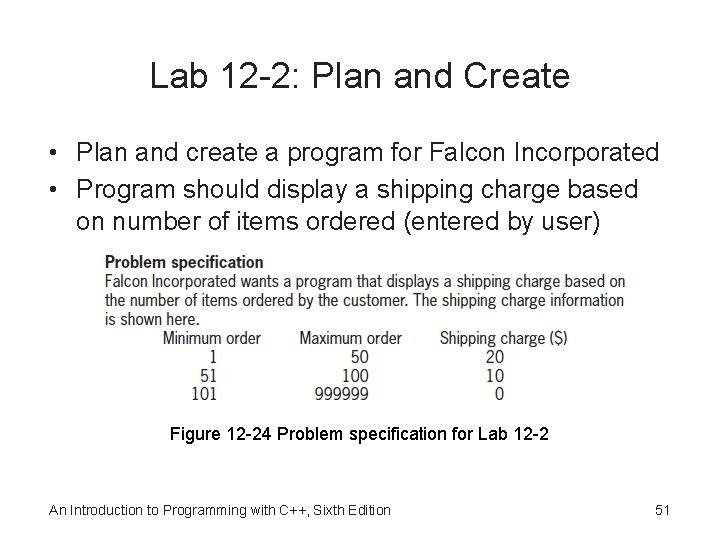
Lab 12 -2: Plan and Create • Plan and create a program for Falcon Incorporated • Program should display a shipping charge based on number of items ordered (entered by user) Figure 12 -24 Problem specification for Lab 12 -2 An Introduction to Programming with C++, Sixth Edition 51
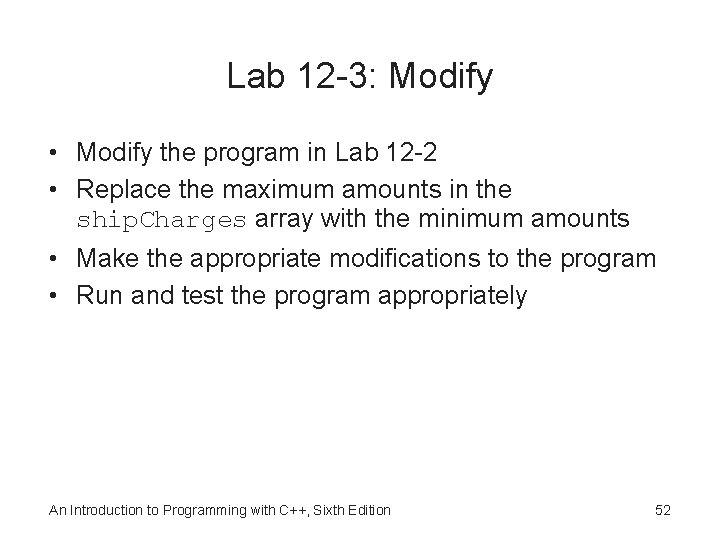
Lab 12 -3: Modify • Modify the program in Lab 12 -2 • Replace the maximum amounts in the ship. Charges array with the minimum amounts • Make the appropriate modifications to the program • Run and test the program appropriately An Introduction to Programming with C++, Sixth Edition 52
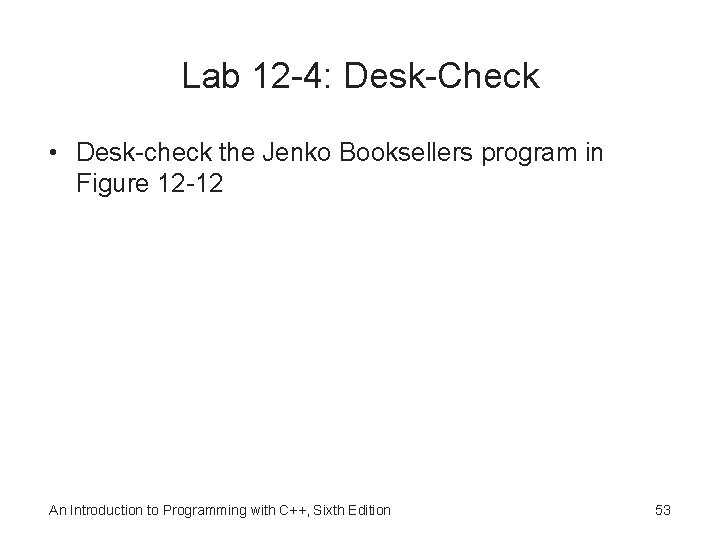
Lab 12 -4: Desk-Check • Desk-check the Jenko Booksellers program in Figure 12 -12 An Introduction to Programming with C++, Sixth Edition 53
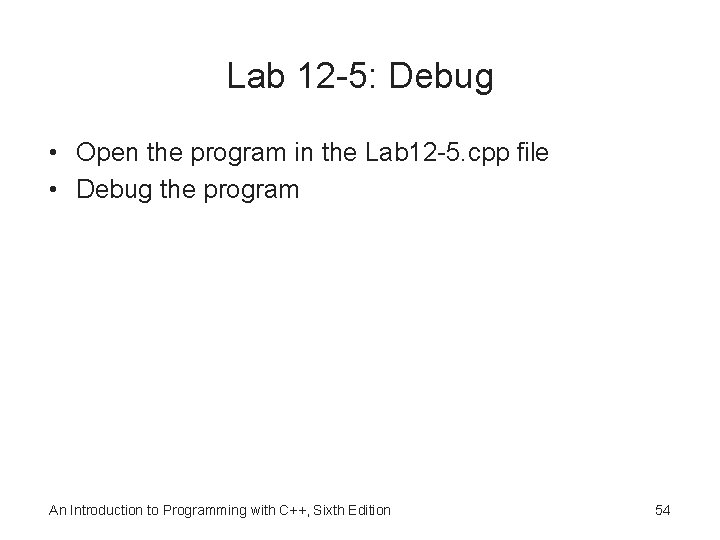
Lab 12 -5: Debug • Open the program in the Lab 12 -5. cpp file • Debug the program An Introduction to Programming with C++, Sixth Edition 54
Mmodation
The sixth sick sheik's sixth sheep's sick lyrics
Introduction to java programming 10th edition quizzes
Biochemistry sixth edition 2007 w.h. freeman and company
Computer architecture a quantitative approach sixth edition
Automotive technology sixth edition
Automotive technology sixth edition
Apa sixth edition
Computer architecture a quantitative approach sixth edition
Precalculus sixth edition
Principles of economics sixth edition
Computer architecture a quantitative approach sixth edition
Using mis 10th edition
Chapter 1
Prelude to programming 6th edition
Prelude to programming 6th edition
Prelude to programming 6th edition
Java programming enterprise edition course
Expert systems: principles and programming, fourth edition
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Windows 10 system programming, part 1
Integer programming vs linear programming
Definisi linear
Marketing an introduction 6th canadian edition
Introduction to teaching: becoming a professional
Introduction to sociology 9th edition
Introduction to radar systems skolnik 3rd edition pdf
Introduction to information systems 6th edition
Introduction to information systems 6th edition
Microbiology an introduction 9th edition
Introduction to information systems 3rd edition
Introduction to information systems 5th edition
Food and beverage directors expect a pour cost of
Introduction to hospitality 7th edition
Introduction to hospitality 7th edition
Introduction to algorithms 2nd ed
Introduction to information systems 3rd edition
Introduction to algorithms 2nd edition
Introduction to algorithms 2nd edition
Human genetics concepts and applications 10th edition
Kinicki: management: a practical introduction 3rd edition
Introduction to information systems 3rd edition
Introduction to server side programming
Problem solving
Introduction to programming languages
Elementary programming in java
An introduction to parallel programming peter pacheco
Introduction to visual basic programming
What does plc stands for
Java introduction to problem solving and programming
Introduction to windows programming
Introduction to programming
Csc 102
A web based introduction to programming