What is LISP is one of the oldest
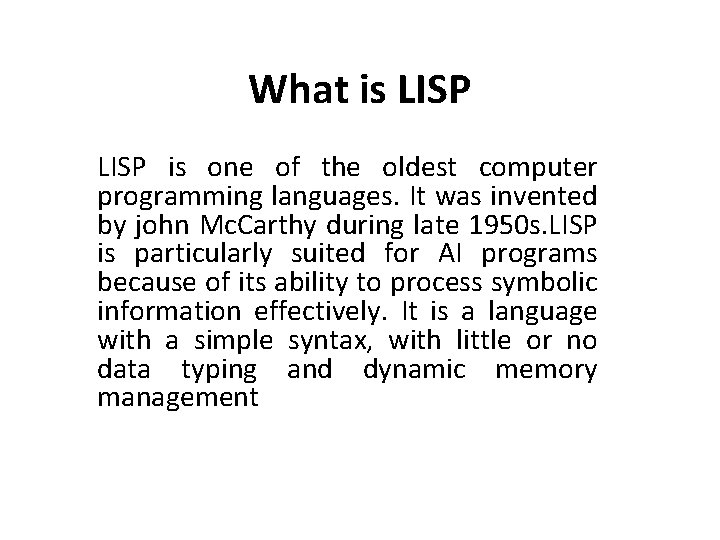
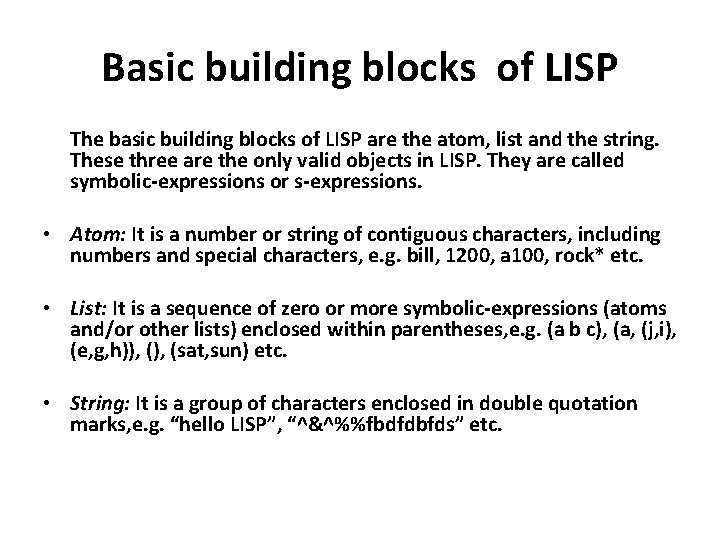
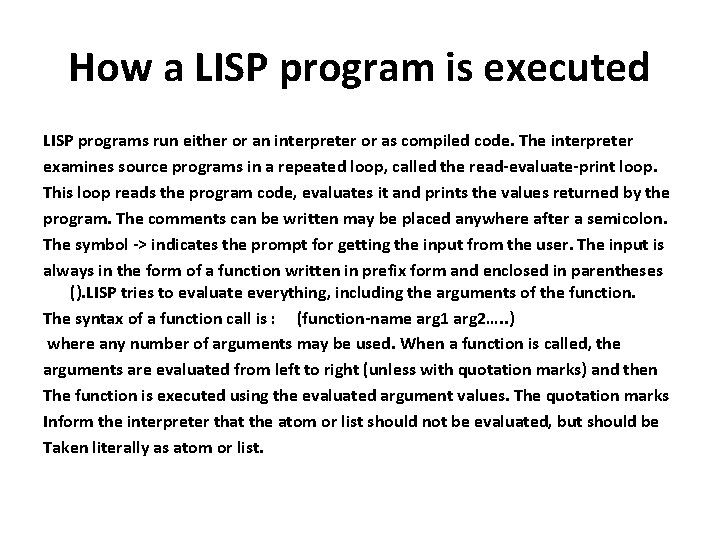
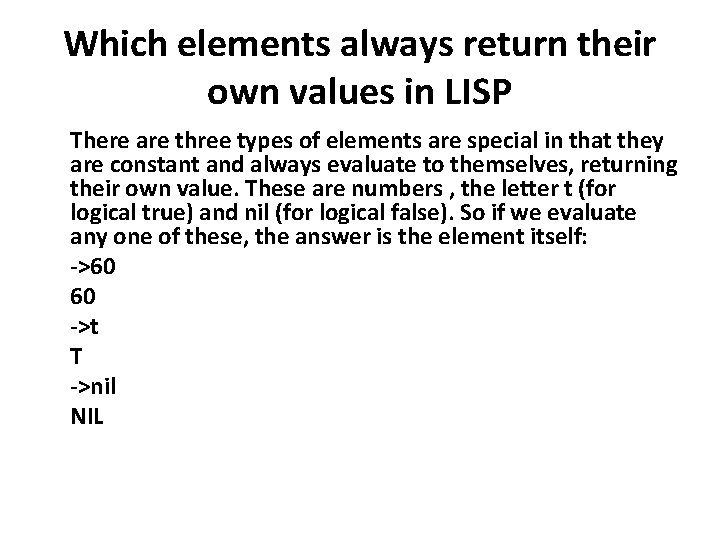
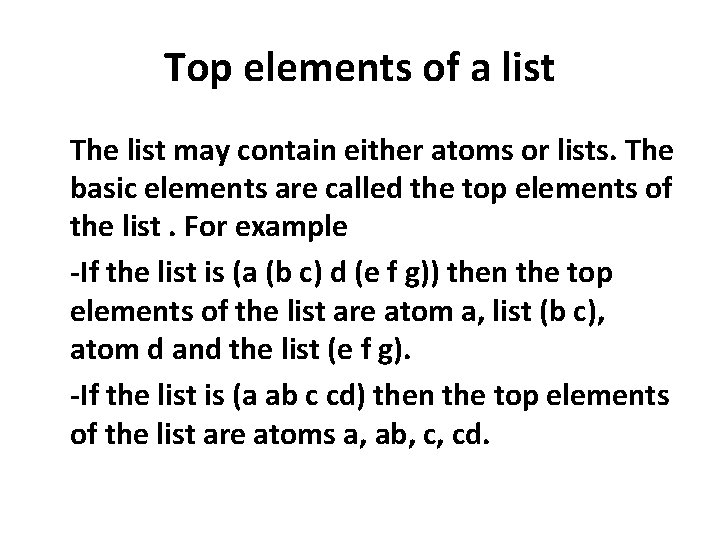
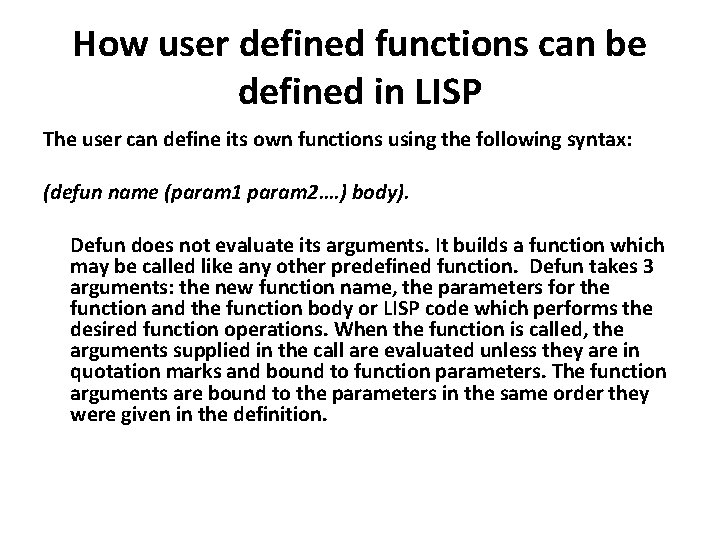
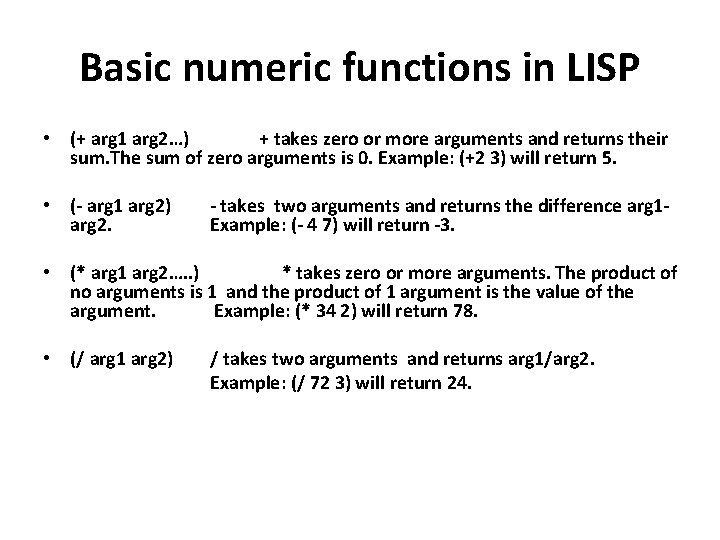
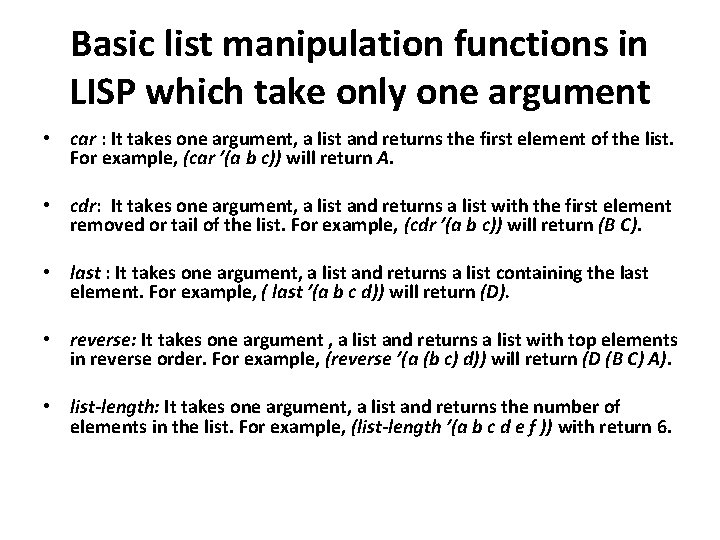
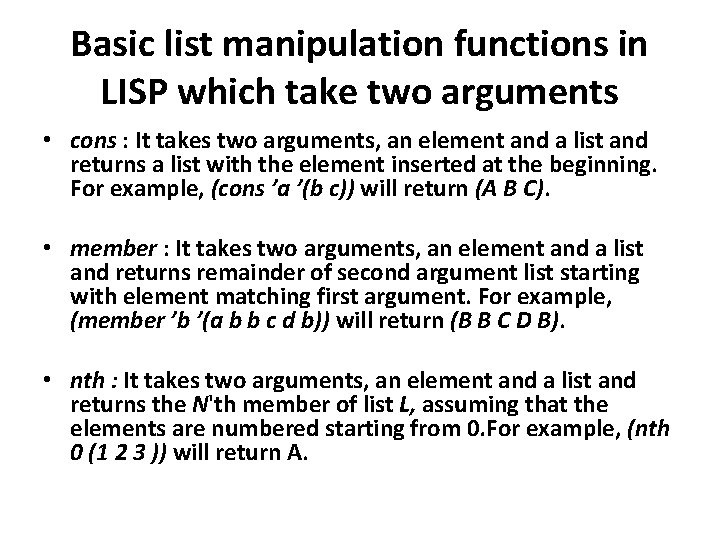
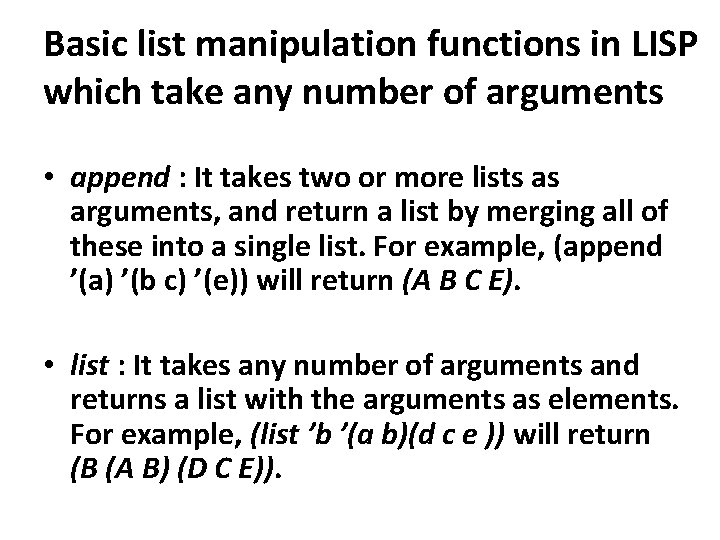
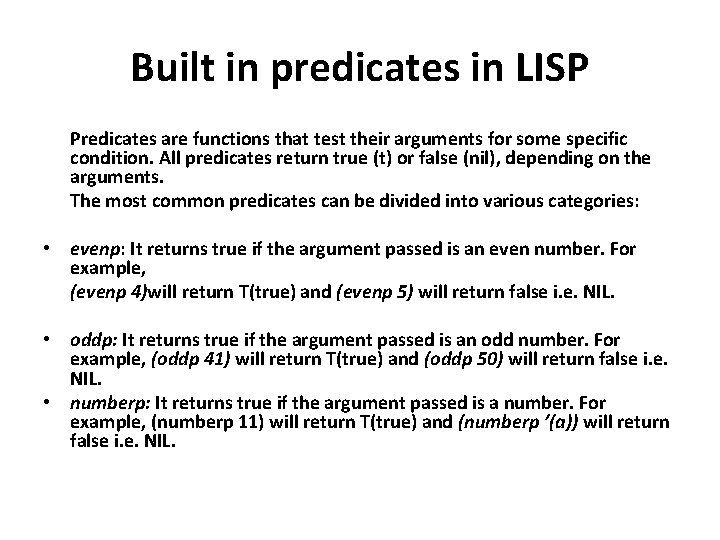
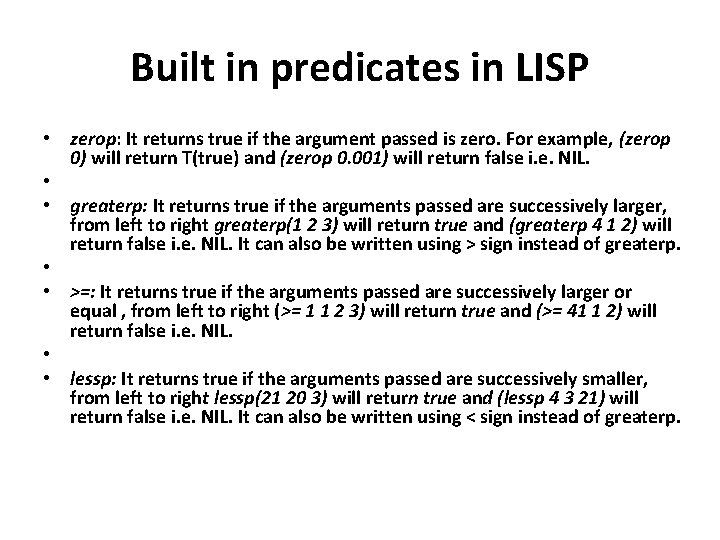
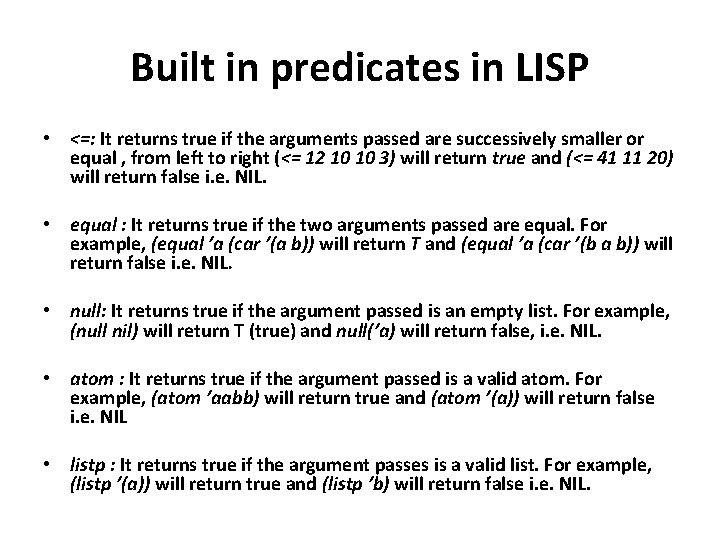
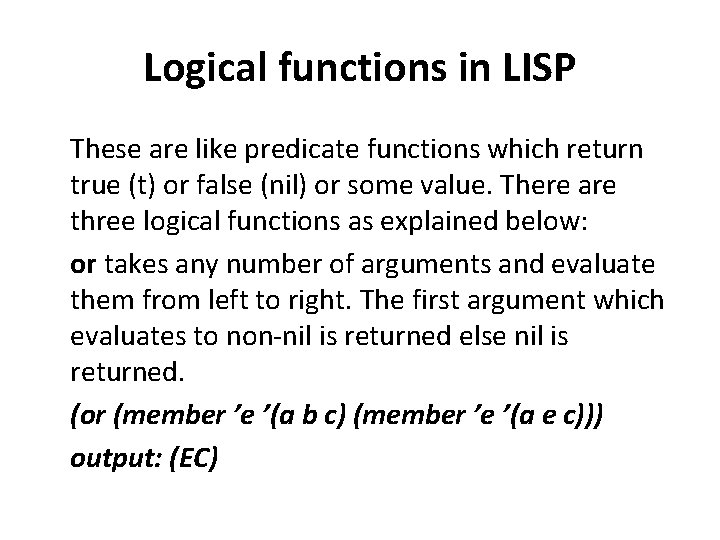
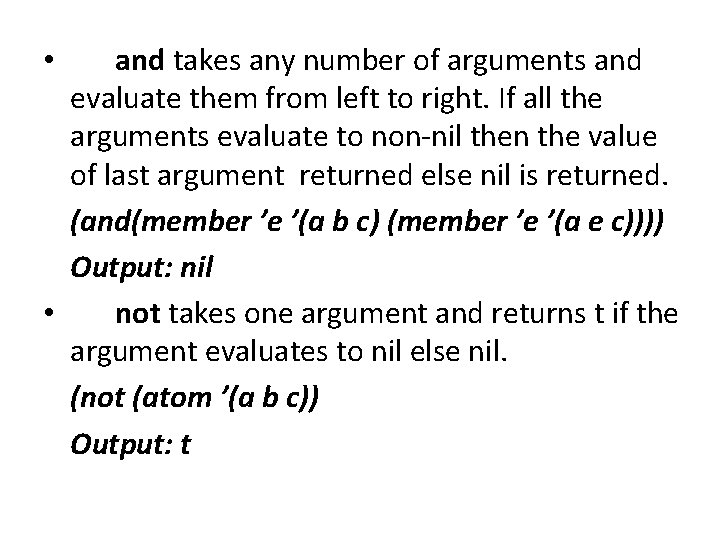
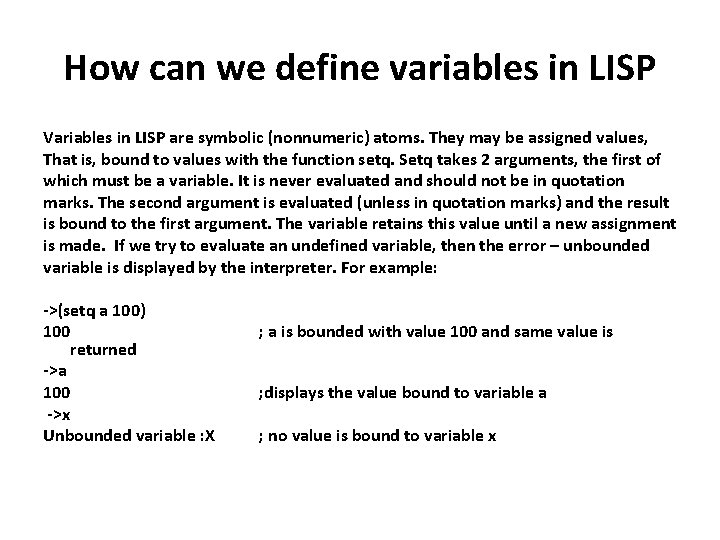
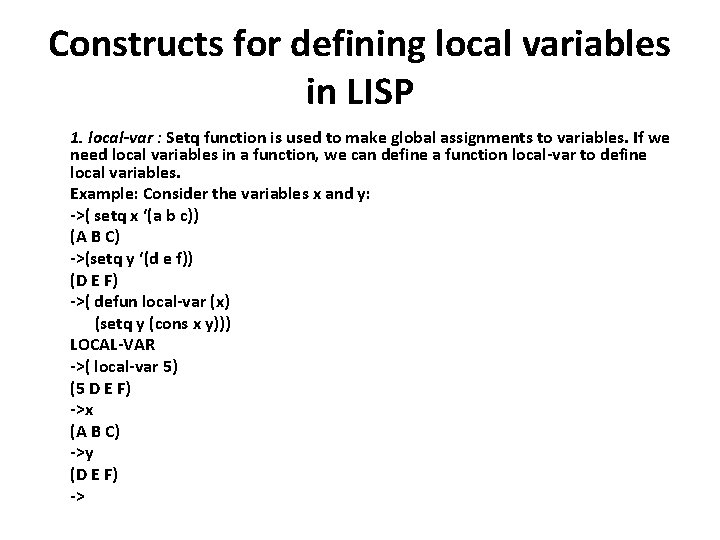
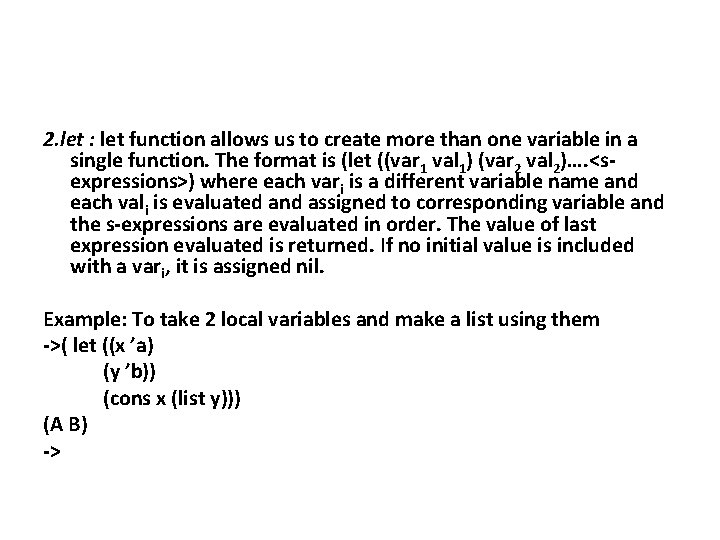
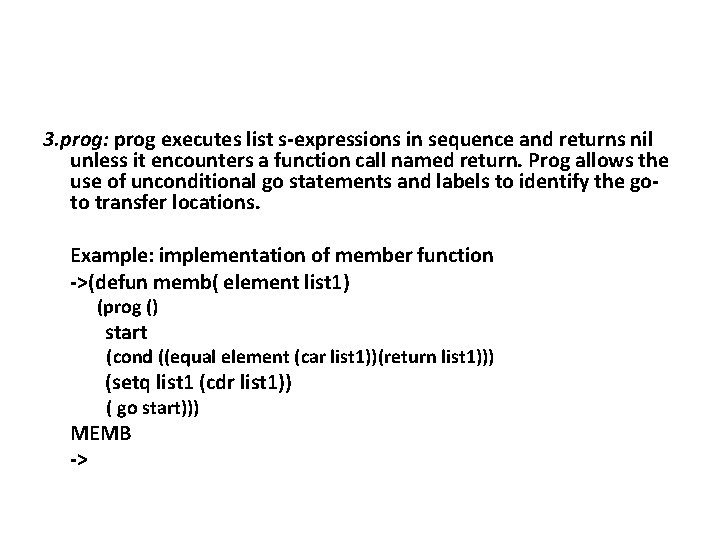
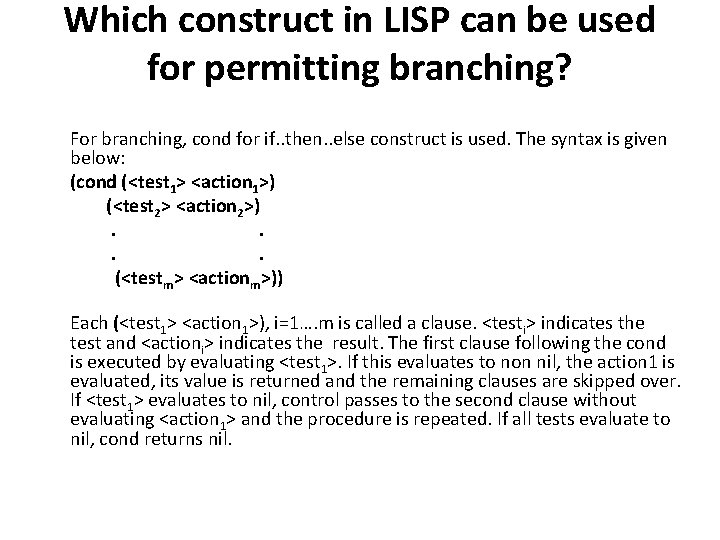
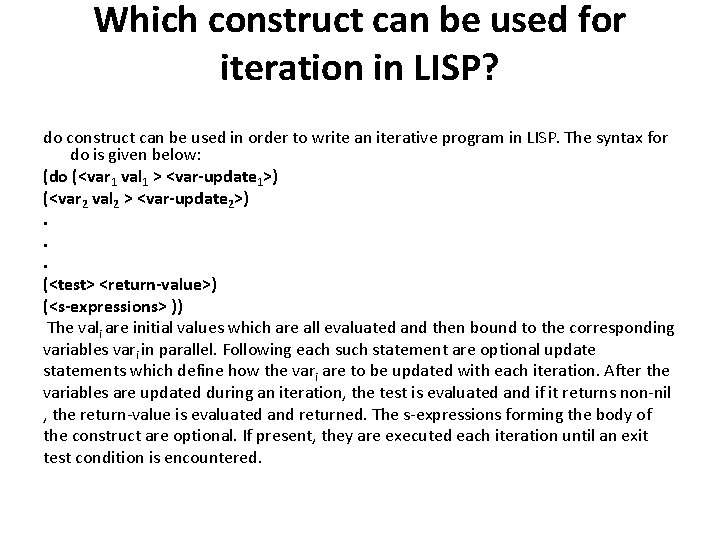
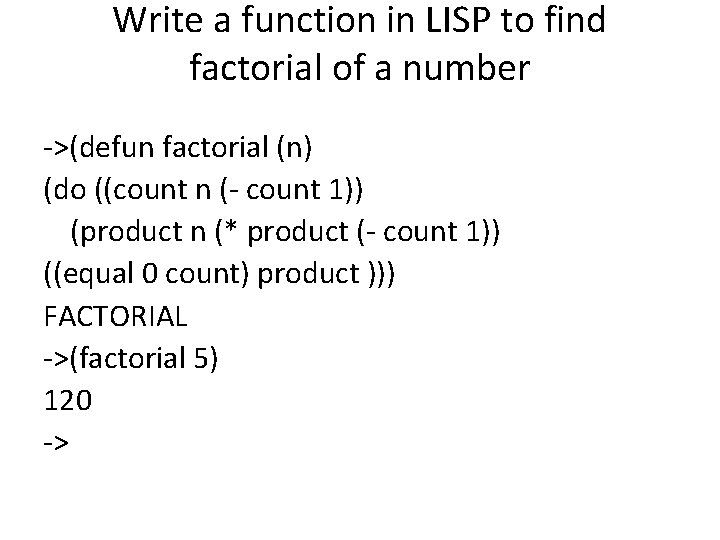
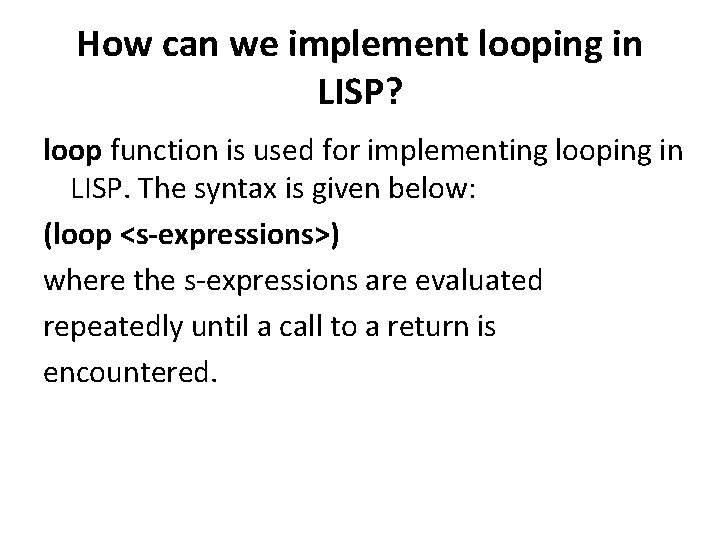
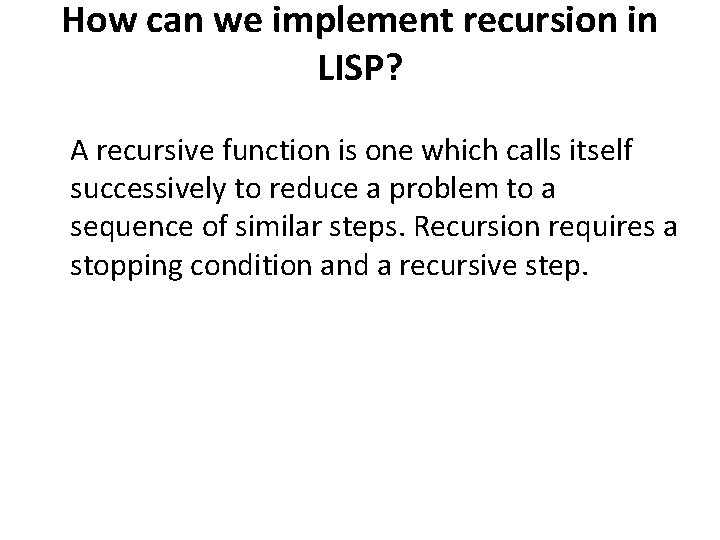
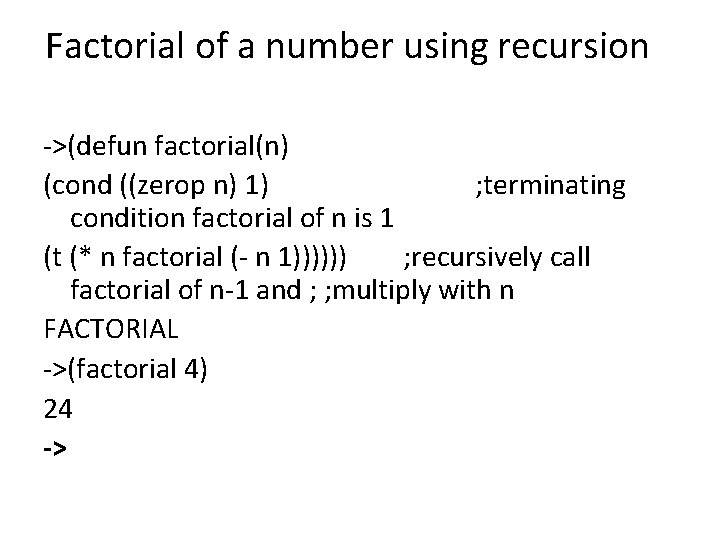
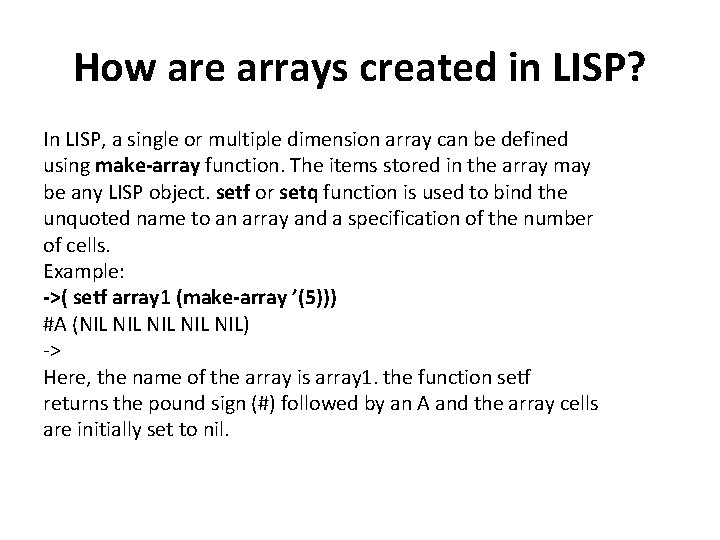
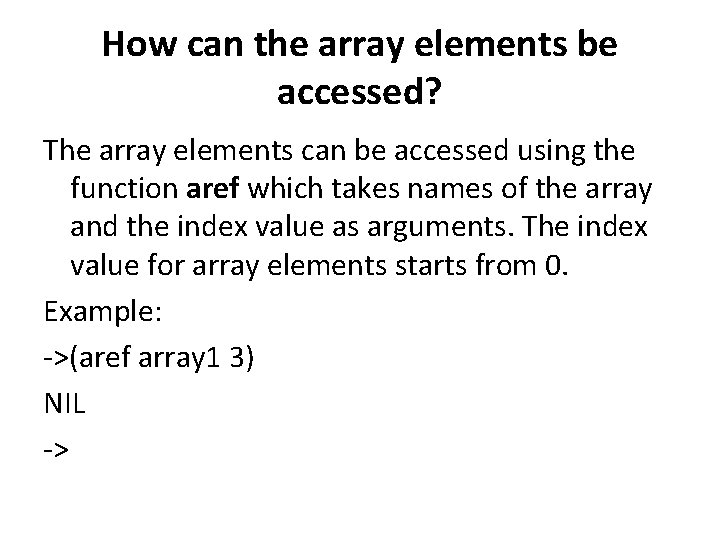
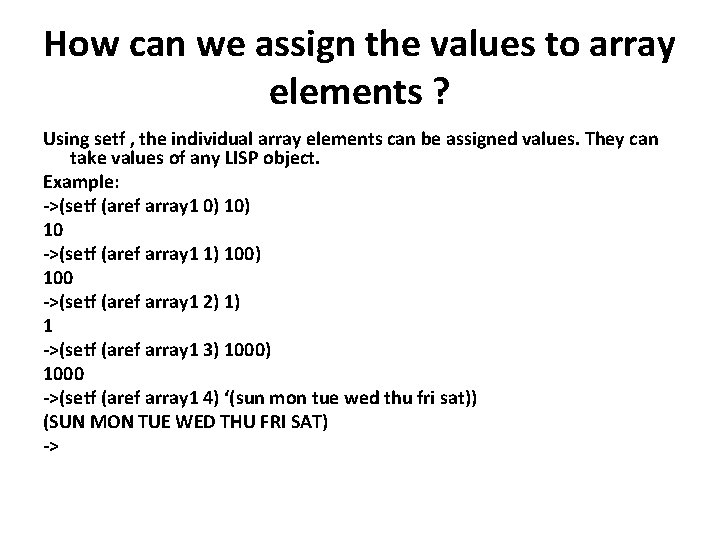
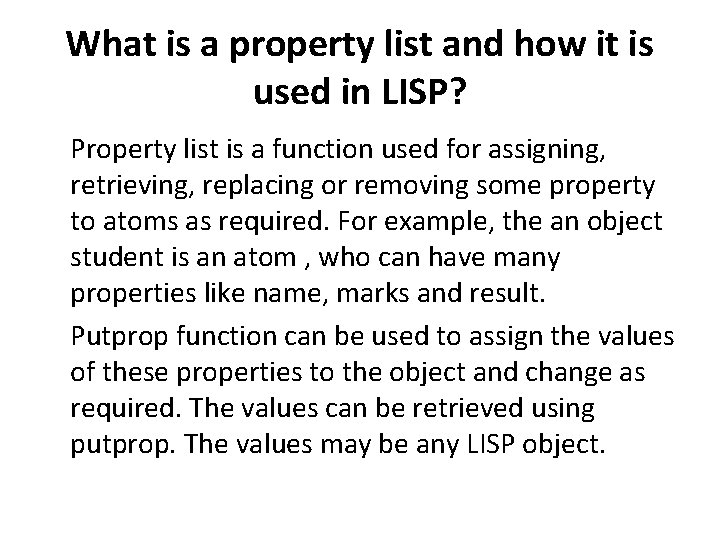
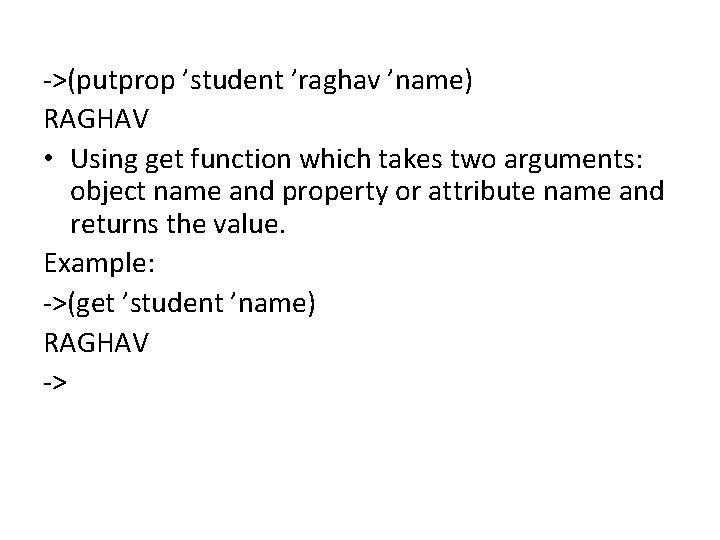
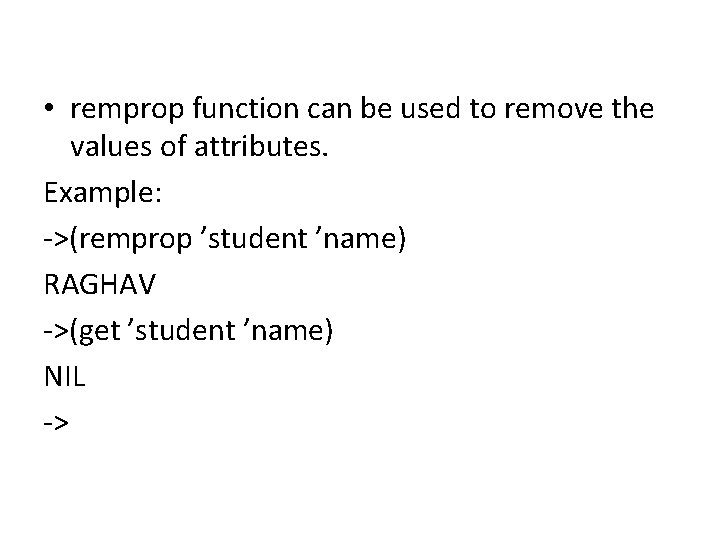
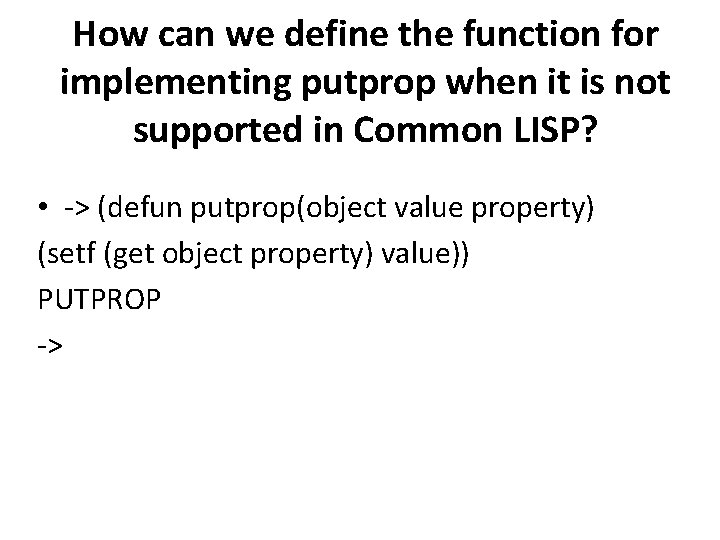
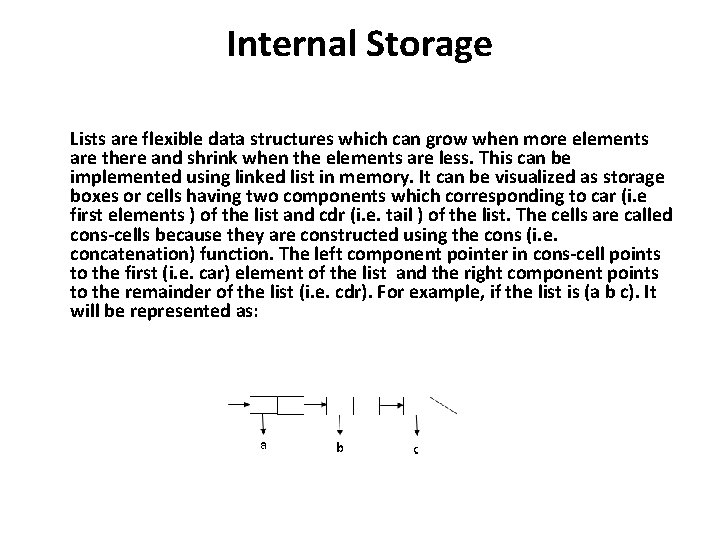
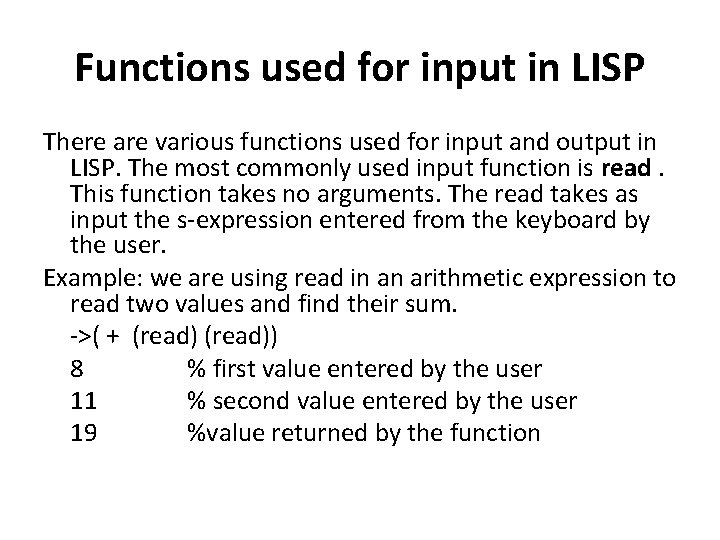
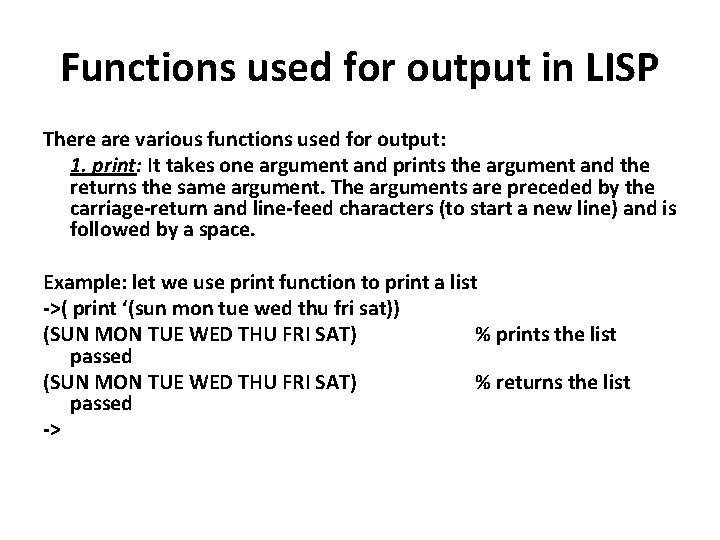
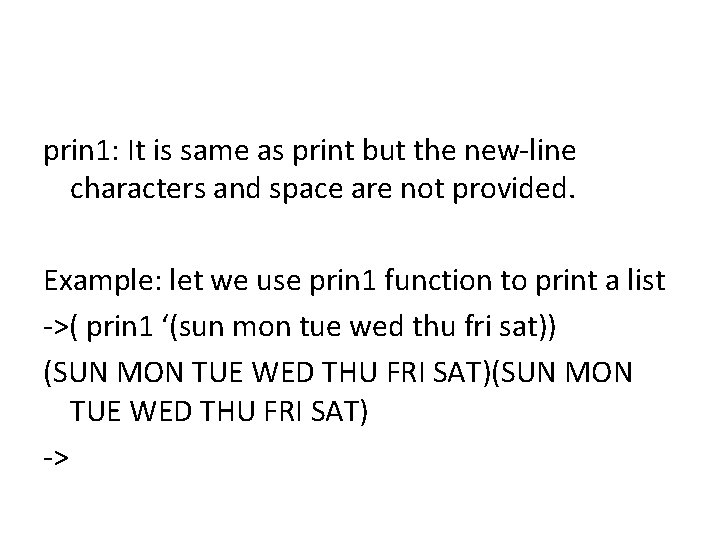
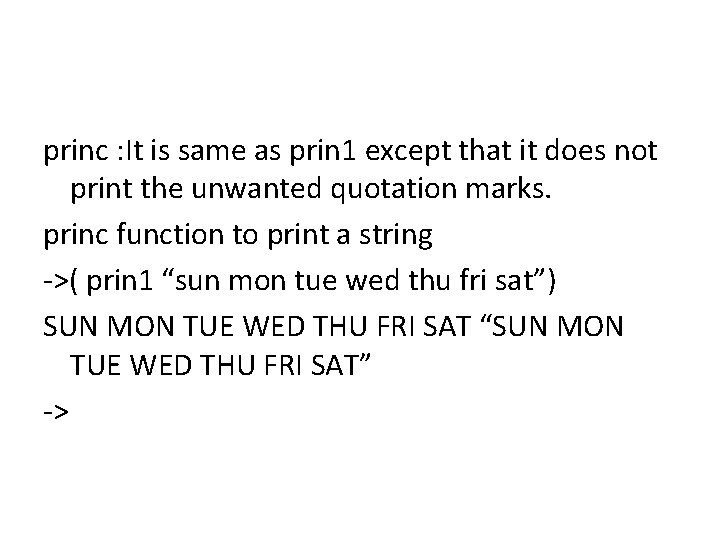
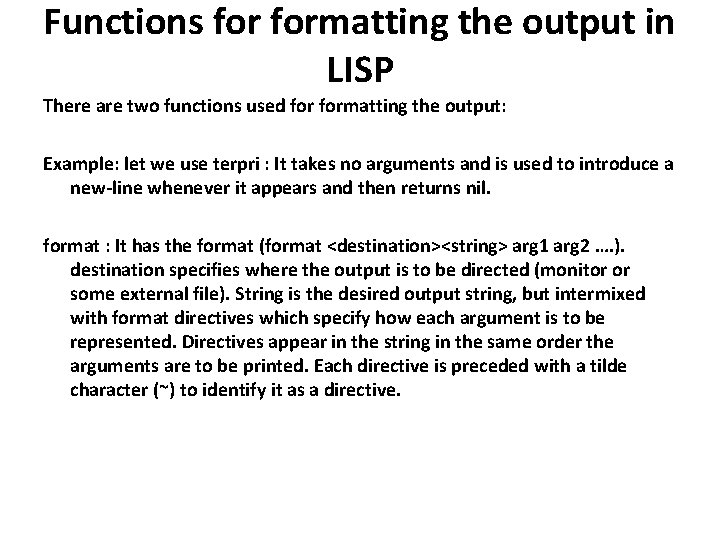
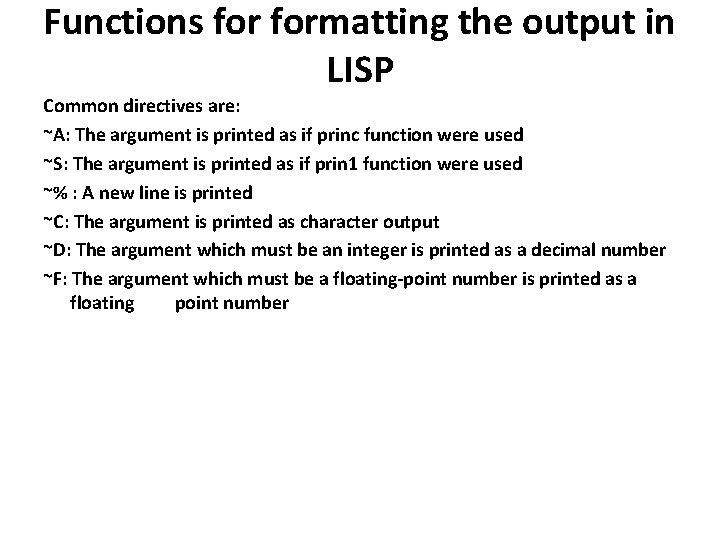
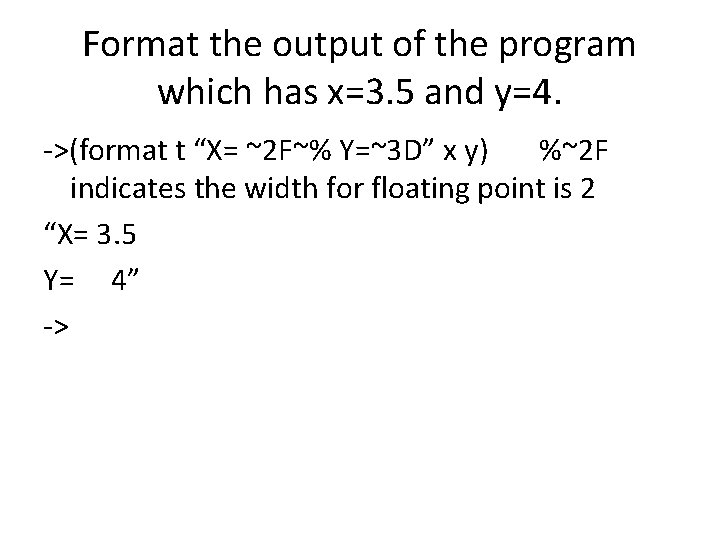
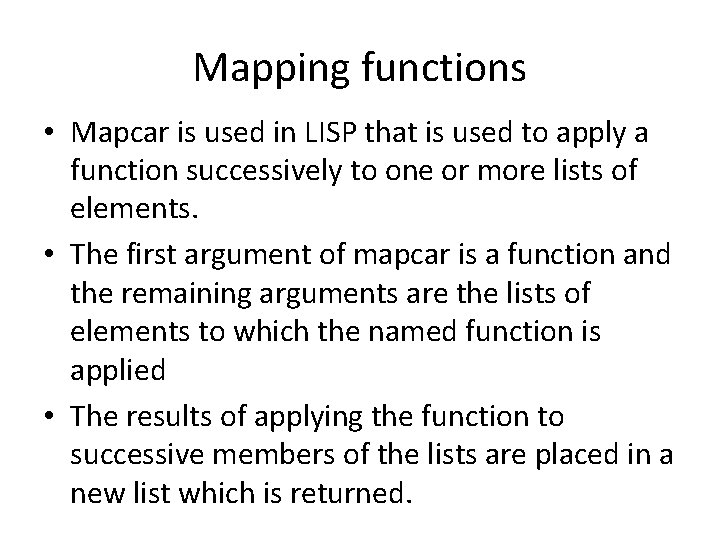
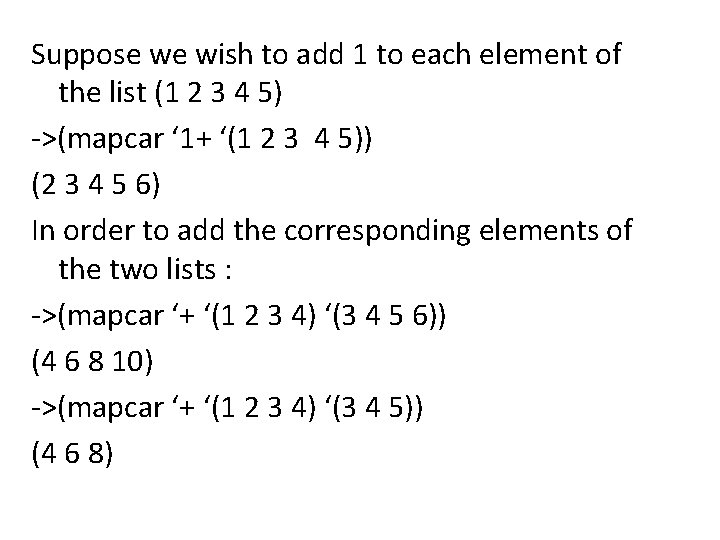
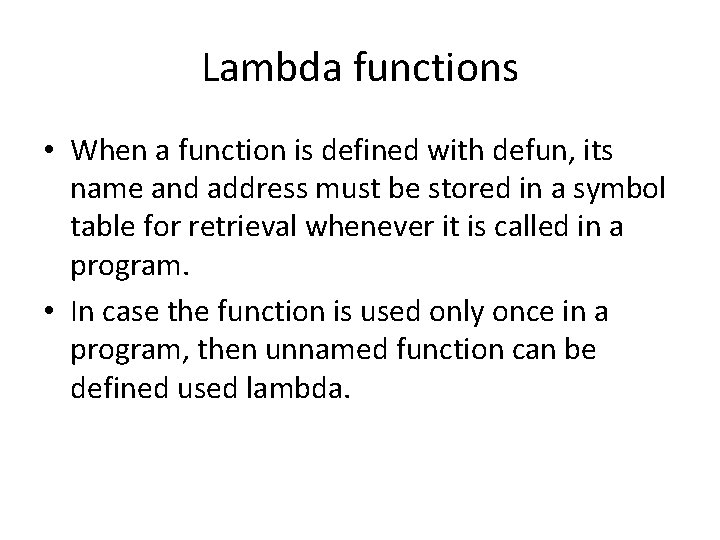
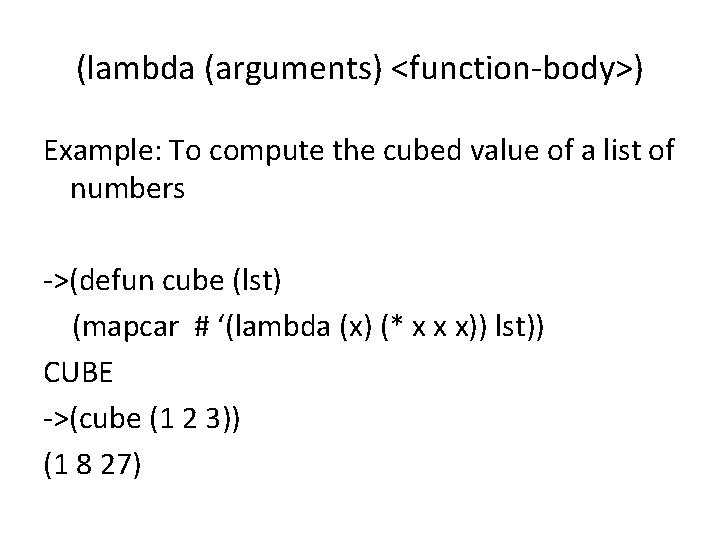
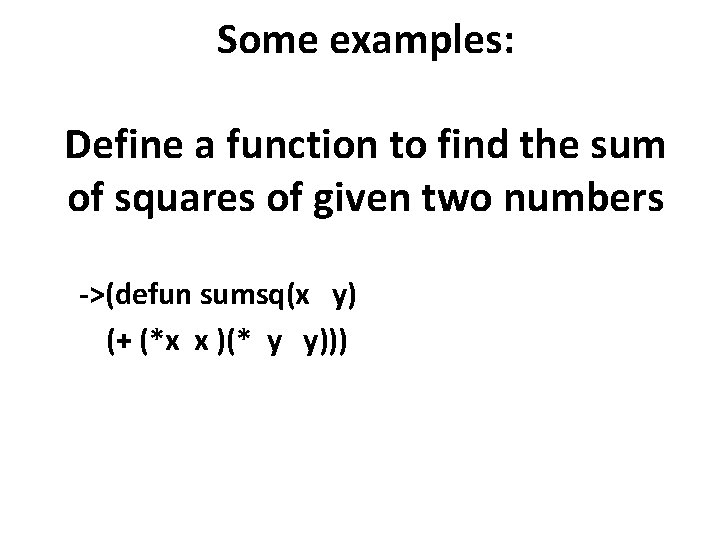
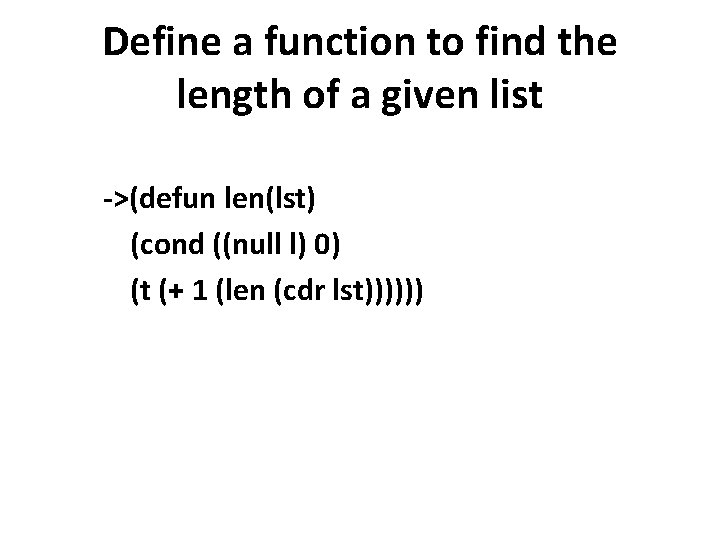
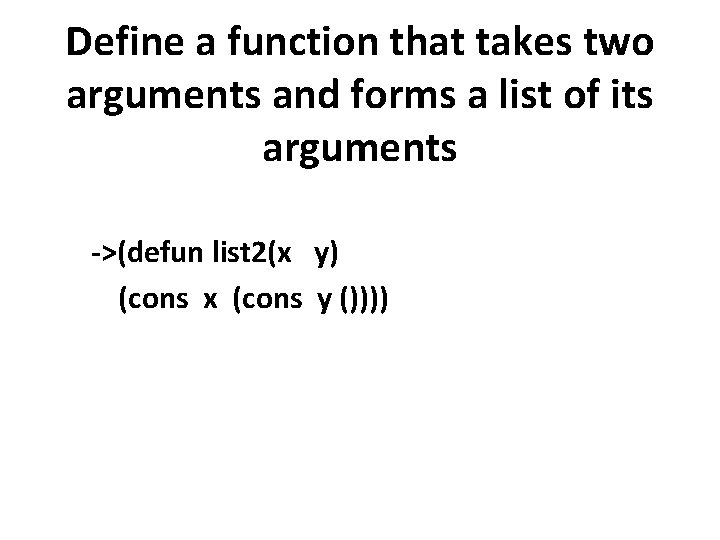
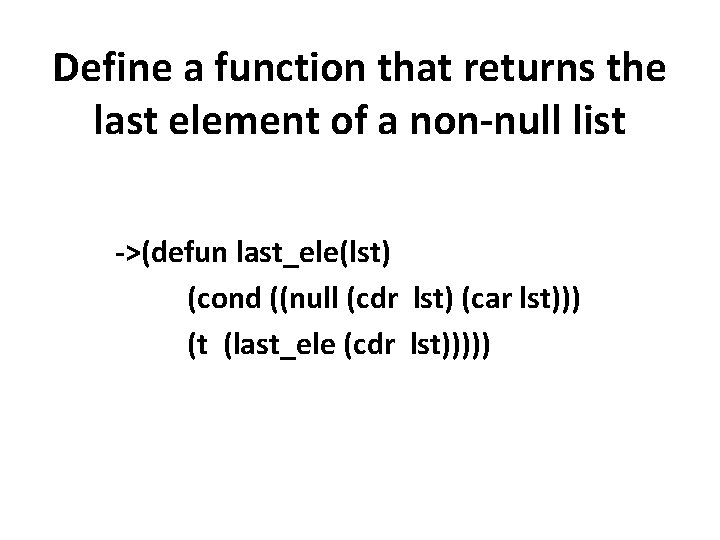
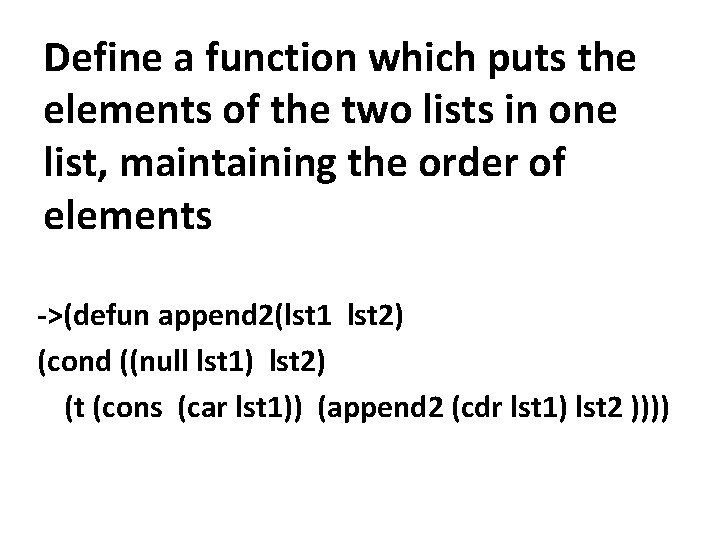
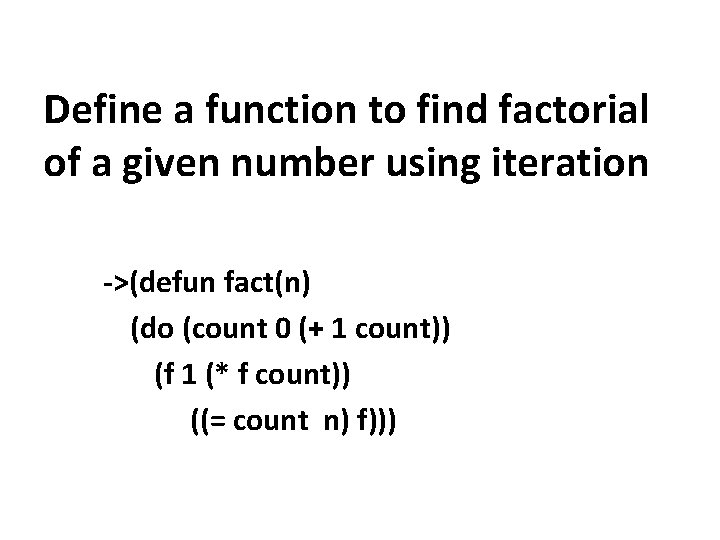
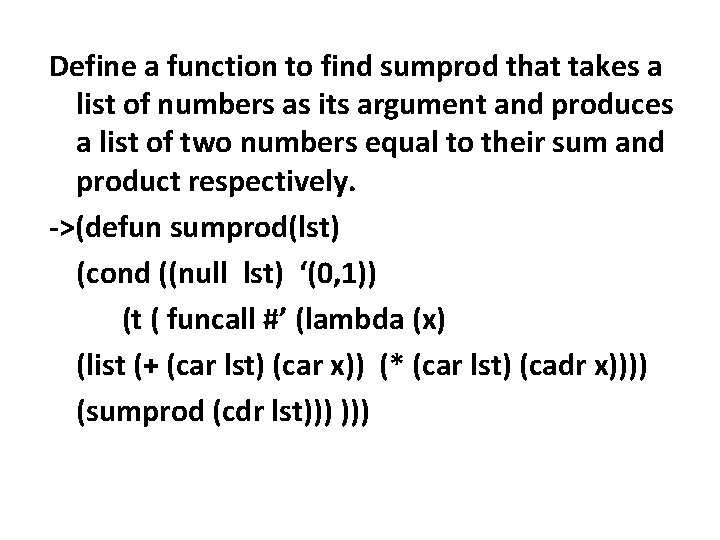
- Slides: 51
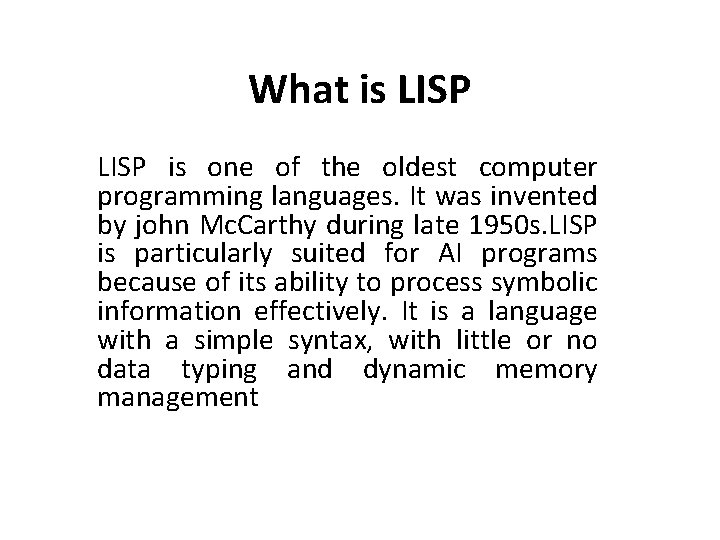
What is LISP is one of the oldest computer programming languages. It was invented by john Mc. Carthy during late 1950 s. LISP is particularly suited for AI programs because of its ability to process symbolic information effectively. It is a language with a simple syntax, with little or no data typing and dynamic memory management
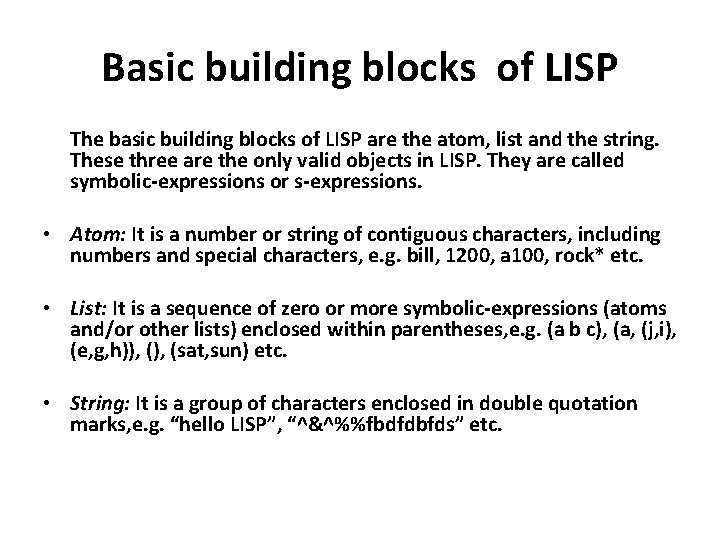
Basic building blocks of LISP The basic building blocks of LISP are the atom, list and the string. These three are the only valid objects in LISP. They are called symbolic-expressions or s-expressions. • Atom: It is a number or string of contiguous characters, including numbers and special characters, e. g. bill, 1200, a 100, rock* etc. • List: It is a sequence of zero or more symbolic-expressions (atoms and/or other lists) enclosed within parentheses, e. g. (a b c), (a, (j, i), (e, g, h)), (sat, sun) etc. • String: It is a group of characters enclosed in double quotation marks, e. g. “hello LISP”, “^&^%%fbdfdbfds” etc.
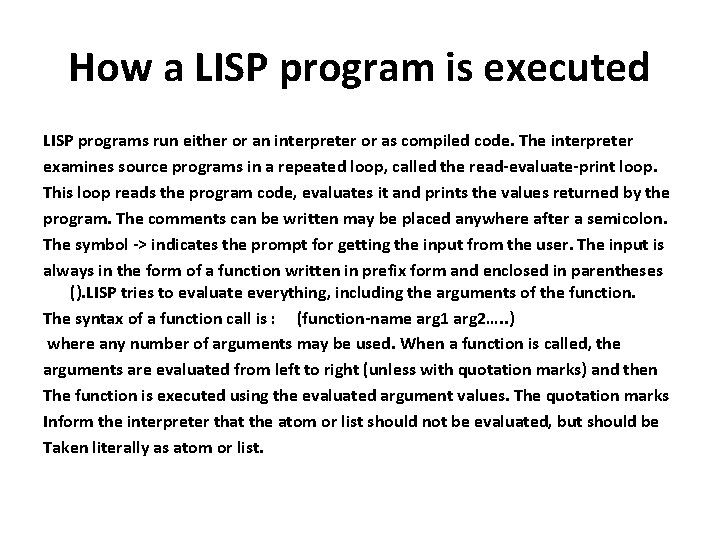
How a LISP program is executed LISP programs run either or an interpreter or as compiled code. The interpreter examines source programs in a repeated loop, called the read-evaluate-print loop. This loop reads the program code, evaluates it and prints the values returned by the program. The comments can be written may be placed anywhere after a semicolon. The symbol -> indicates the prompt for getting the input from the user. The input is always in the form of a function written in prefix form and enclosed in parentheses (). LISP tries to evaluate everything, including the arguments of the function. The syntax of a function call is : (function-name arg 1 arg 2…. . ) where any number of arguments may be used. When a function is called, the arguments are evaluated from left to right (unless with quotation marks) and then The function is executed using the evaluated argument values. The quotation marks Inform the interpreter that the atom or list should not be evaluated, but should be Taken literally as atom or list.
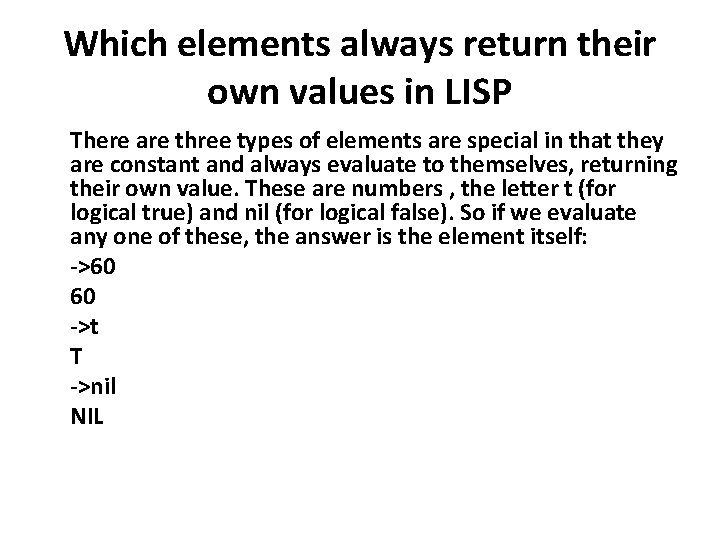
Which elements always return their own values in LISP There are three types of elements are special in that they are constant and always evaluate to themselves, returning their own value. These are numbers , the letter t (for logical true) and nil (for logical false). So if we evaluate any one of these, the answer is the element itself: ->60 60 ->t T ->nil NIL
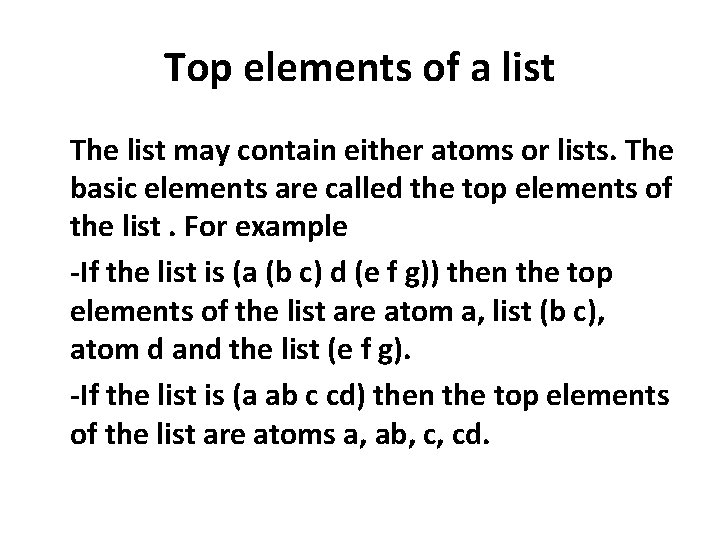
Top elements of a list The list may contain either atoms or lists. The basic elements are called the top elements of the list. For example -If the list is (a (b c) d (e f g)) then the top elements of the list are atom a, list (b c), atom d and the list (e f g). -If the list is (a ab c cd) then the top elements of the list are atoms a, ab, c, cd.
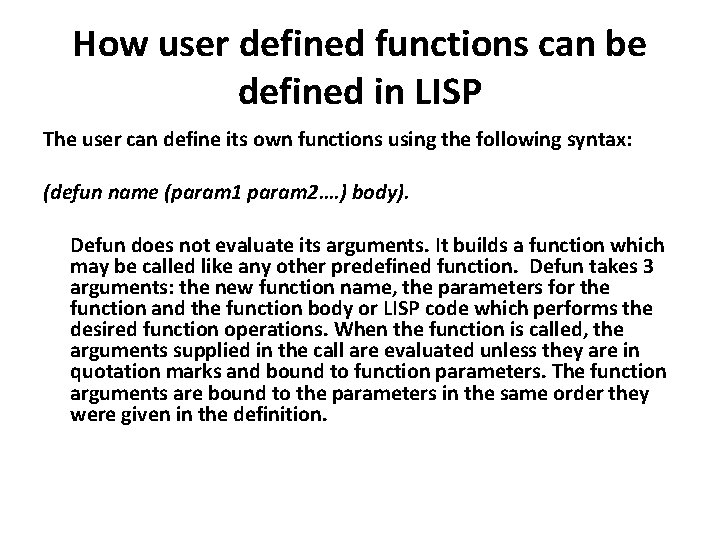
How user defined functions can be defined in LISP The user can define its own functions using the following syntax: (defun name (param 1 param 2…. ) body). Defun does not evaluate its arguments. It builds a function which may be called like any other predefined function. Defun takes 3 arguments: the new function name, the parameters for the function and the function body or LISP code which performs the desired function operations. When the function is called, the arguments supplied in the call are evaluated unless they are in quotation marks and bound to function parameters. The function arguments are bound to the parameters in the same order they were given in the definition.
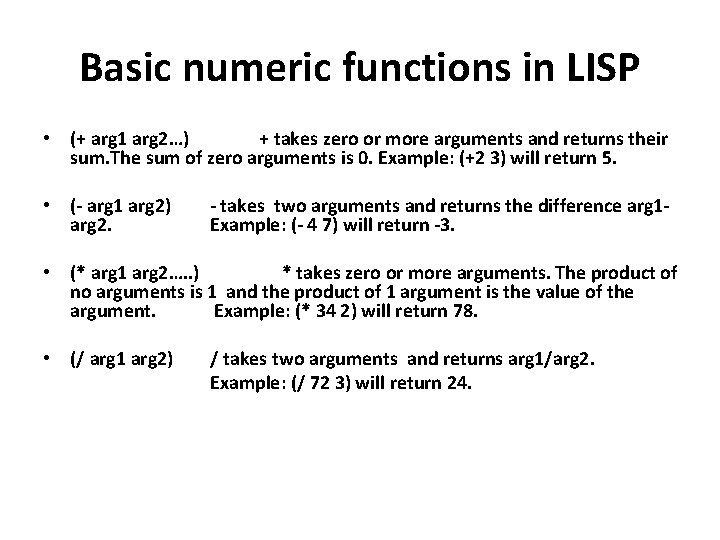
Basic numeric functions in LISP • (+ arg 1 arg 2…) + takes zero or more arguments and returns their sum. The sum of zero arguments is 0. Example: (+2 3) will return 5. • (- arg 1 arg 2) - takes two arguments and returns the difference arg 1 arg 2. Example: (- 4 7) will return -3. • (* arg 1 arg 2…. . ) * takes zero or more arguments. The product of no arguments is 1 and the product of 1 argument is the value of the argument. Example: (* 34 2) will return 78. • (/ arg 1 arg 2) / takes two arguments and returns arg 1/arg 2. Example: (/ 72 3) will return 24.
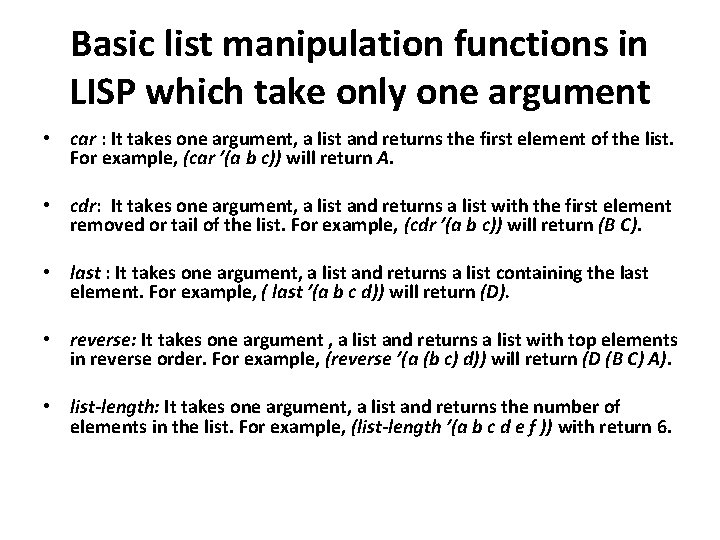
Basic list manipulation functions in LISP which take only one argument • car : It takes one argument, a list and returns the first element of the list. For example, (car ’(a b c)) will return A. • cdr: It takes one argument, a list and returns a list with the first element removed or tail of the list. For example, (cdr ’(a b c)) will return (B C). • last : It takes one argument, a list and returns a list containing the last element. For example, ( last ’(a b c d)) will return (D). • reverse: It takes one argument , a list and returns a list with top elements in reverse order. For example, (reverse ’(a (b c) d)) will return (D (B C) A). • list-length: It takes one argument, a list and returns the number of elements in the list. For example, (list-length ’(a b c d e f )) with return 6.
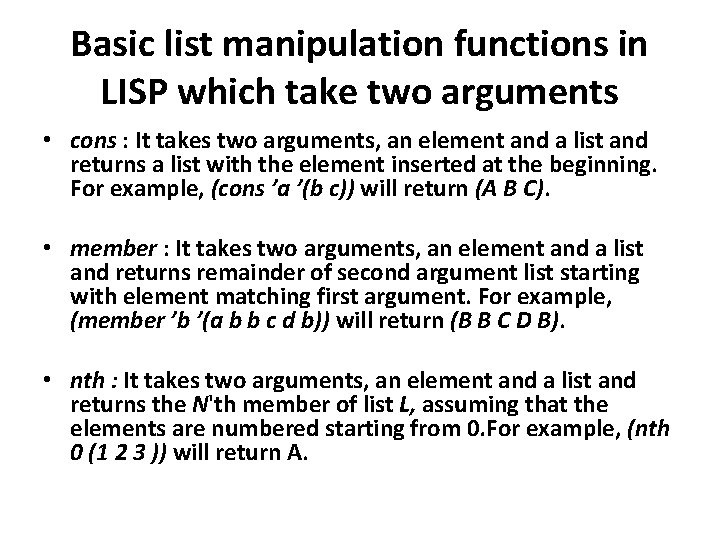
Basic list manipulation functions in LISP which take two arguments • cons : It takes two arguments, an element and a list and returns a list with the element inserted at the beginning. For example, (cons ’a ’(b c)) will return (A B C). • member : It takes two arguments, an element and a list and returns remainder of second argument list starting with element matching first argument. For example, (member ’b ’(a b b c d b)) will return (B B C D B). • nth : It takes two arguments, an element and a list and returns the N'th member of list L, assuming that the elements are numbered starting from 0. For example, (nth 0 (1 2 3 )) will return A.
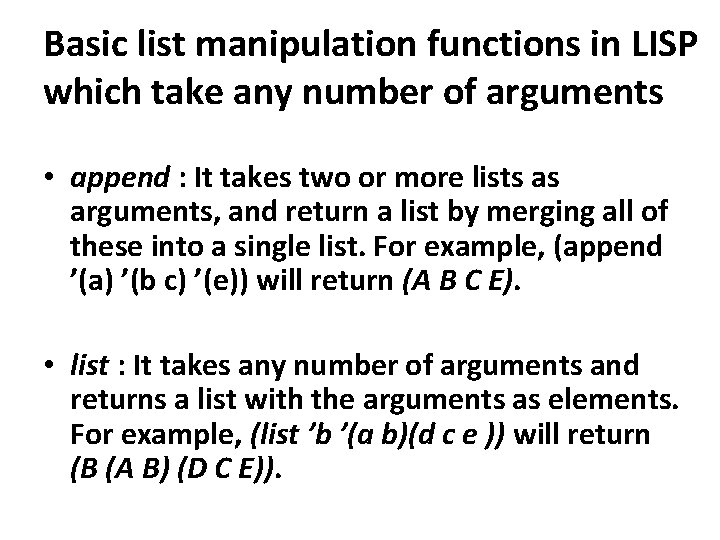
Basic list manipulation functions in LISP which take any number of arguments • append : It takes two or more lists as arguments, and return a list by merging all of these into a single list. For example, (append ’(a) ’(b c) ’(e)) will return (A B C E). • list : It takes any number of arguments and returns a list with the arguments as elements. For example, (list ’b ’(a b)(d c e )) will return (B (A B) (D C E)).
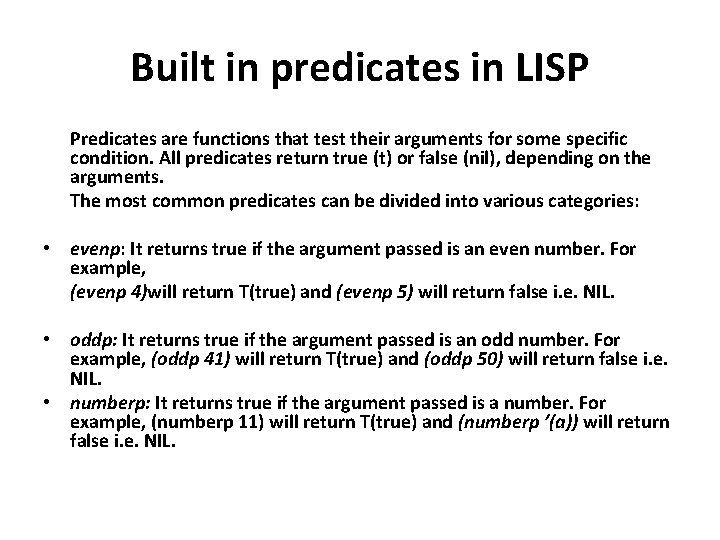
Built in predicates in LISP Predicates are functions that test their arguments for some specific condition. All predicates return true (t) or false (nil), depending on the arguments. The most common predicates can be divided into various categories: • evenp: It returns true if the argument passed is an even number. For example, (evenp 4)will return T(true) and (evenp 5) will return false i. e. NIL. • oddp: It returns true if the argument passed is an odd number. For example, (oddp 41) will return T(true) and (oddp 50) will return false i. e. NIL. • numberp: It returns true if the argument passed is a number. For example, (numberp 11) will return T(true) and (numberp ’(a)) will return false i. e. NIL.
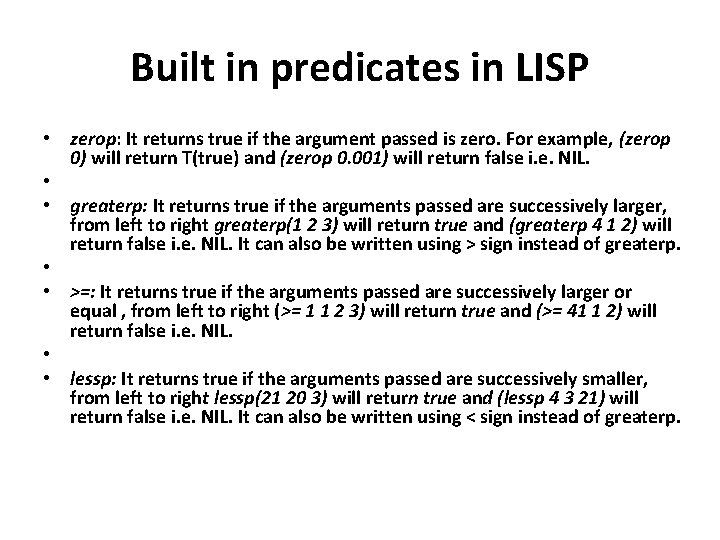
Built in predicates in LISP • zerop: It returns true if the argument passed is zero. For example, (zerop 0) will return T(true) and (zerop 0. 001) will return false i. e. NIL. • • greaterp: It returns true if the arguments passed are successively larger, from left to right greaterp(1 2 3) will return true and (greaterp 4 1 2) will return false i. e. NIL. It can also be written using > sign instead of greaterp. • • >=: It returns true if the arguments passed are successively larger or equal , from left to right (>= 1 1 2 3) will return true and (>= 41 1 2) will return false i. e. NIL. • • lessp: It returns true if the arguments passed are successively smaller, from left to right lessp(21 20 3) will return true and (lessp 4 3 21) will return false i. e. NIL. It can also be written using < sign instead of greaterp.
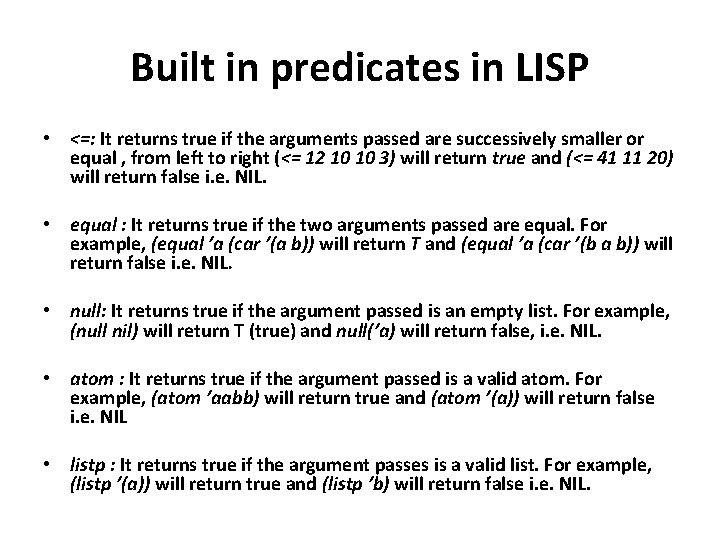
Built in predicates in LISP • <=: It returns true if the arguments passed are successively smaller or equal , from left to right (<= 12 10 10 3) will return true and (<= 41 11 20) will return false i. e. NIL. • equal : It returns true if the two arguments passed are equal. For example, (equal ’a (car ’(a b)) will return T and (equal ’a (car ’(b a b)) will return false i. e. NIL. • null: It returns true if the argument passed is an empty list. For example, (null nil) will return T (true) and null(’a) will return false, i. e. NIL. • atom : It returns true if the argument passed is a valid atom. For example, (atom ’aabb) will return true and (atom ’(a)) will return false i. e. NIL • listp : It returns true if the argument passes is a valid list. For example, (listp ’(a)) will return true and (listp ’b) will return false i. e. NIL.
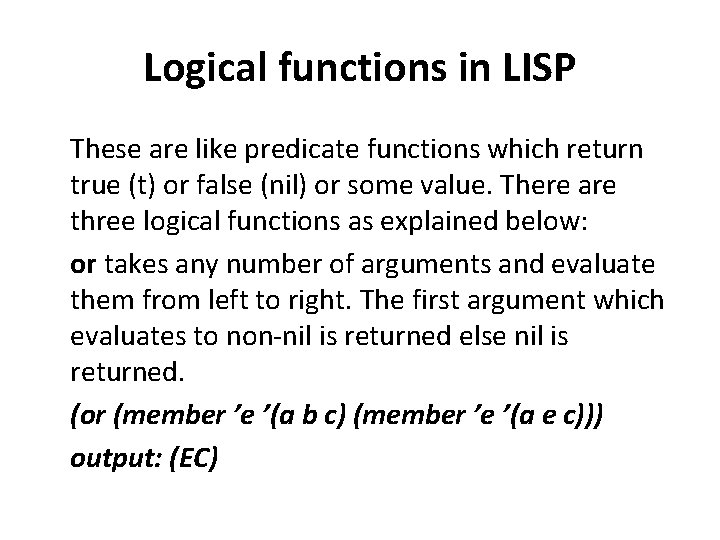
Logical functions in LISP These are like predicate functions which return true (t) or false (nil) or some value. There are three logical functions as explained below: or takes any number of arguments and evaluate them from left to right. The first argument which evaluates to non-nil is returned else nil is returned. (or (member ’e ’(a b c) (member ’e ’(a e c))) output: (EC)
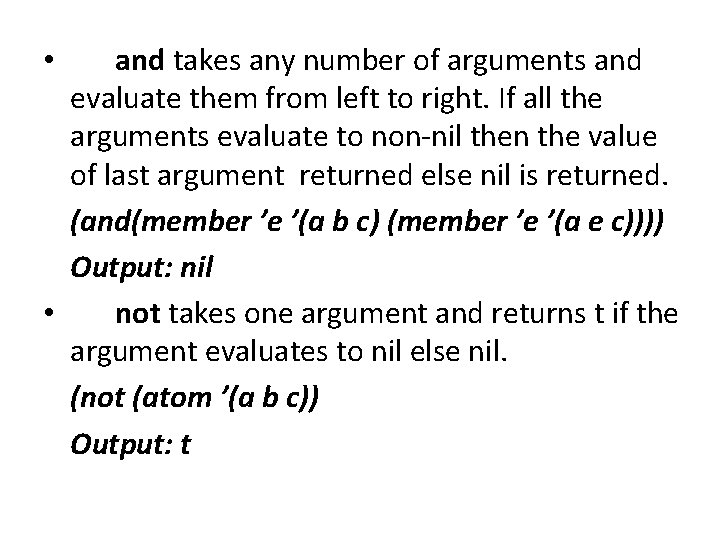
and takes any number of arguments and evaluate them from left to right. If all the arguments evaluate to non-nil then the value of last argument returned else nil is returned. (and(member ’e ’(a b c) (member ’e ’(a e c)))) Output: nil • not takes one argument and returns t if the argument evaluates to nil else nil. (not (atom ’(a b c)) Output: t •
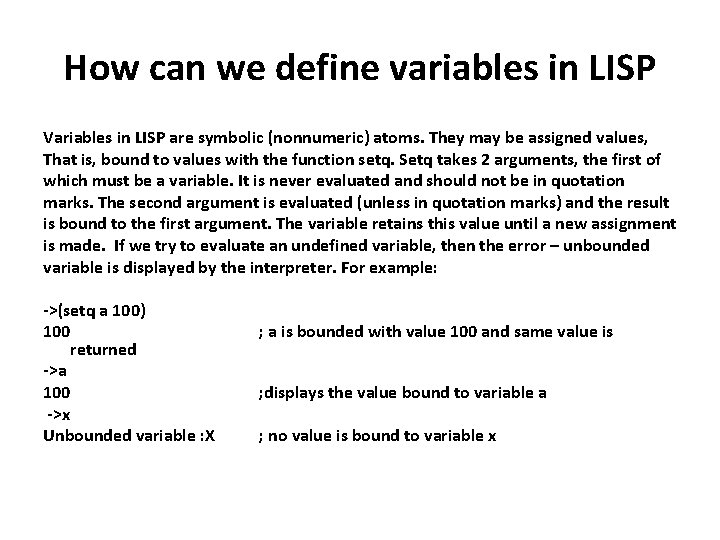
How can we define variables in LISP Variables in LISP are symbolic (nonnumeric) atoms. They may be assigned values, That is, bound to values with the function setq. Setq takes 2 arguments, the first of which must be a variable. It is never evaluated and should not be in quotation marks. The second argument is evaluated (unless in quotation marks) and the result is bound to the first argument. The variable retains this value until a new assignment is made. If we try to evaluate an undefined variable, then the error – unbounded variable is displayed by the interpreter. For example: ->(setq a 100) 100 returned ->a 100 ->x Unbounded variable : X ; a is bounded with value 100 and same value is ; displays the value bound to variable a ; no value is bound to variable x
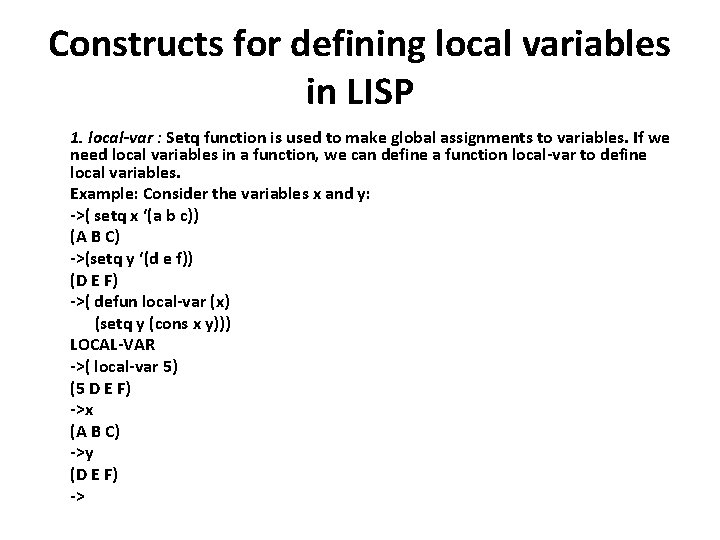
Constructs for defining local variables in LISP 1. local-var : Setq function is used to make global assignments to variables. If we need local variables in a function, we can define a function local-var to define local variables. Example: Consider the variables x and y: ->( setq x ‘(a b c)) (A B C) ->(setq y ‘(d e f)) (D E F) ->( defun local-var (x) (setq y (cons x y))) LOCAL-VAR ->( local-var 5) (5 D E F) ->x (A B C) ->y (D E F) ->
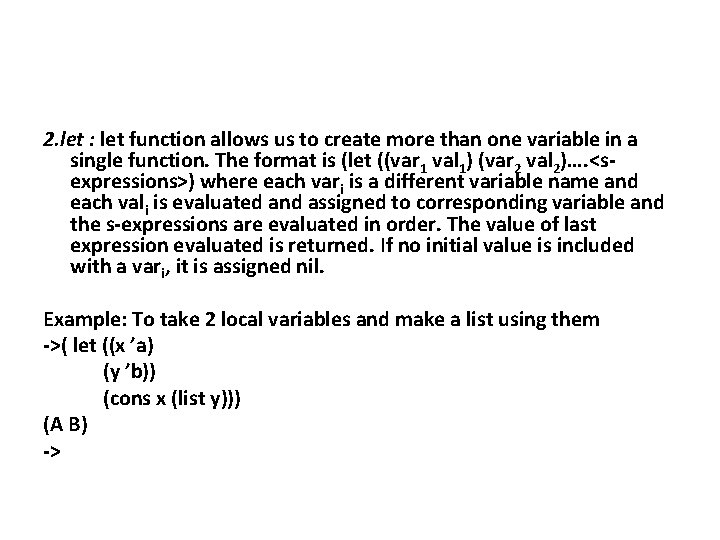
2. let : let function allows us to create more than one variable in a single function. The format is (let ((var 1 val 1) (var 2 val 2)…. <sexpressions>) where each vari is a different variable name and each vali is evaluated and assigned to corresponding variable and the s-expressions are evaluated in order. The value of last expression evaluated is returned. If no initial value is included with a vari, it is assigned nil. Example: To take 2 local variables and make a list using them ->( let ((x ’a) (y ’b)) (cons x (list y))) (A B) ->
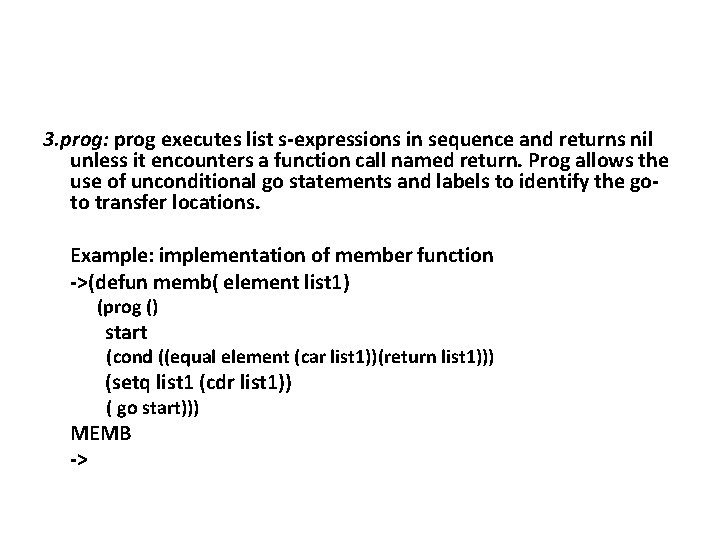
3. prog: prog executes list s-expressions in sequence and returns nil unless it encounters a function call named return. Prog allows the use of unconditional go statements and labels to identify the goto transfer locations. Example: implementation of member function ->(defun memb( element list 1) (prog () start (cond ((equal element (car list 1))(return list 1))) (setq list 1 (cdr list 1)) ( go start))) MEMB ->
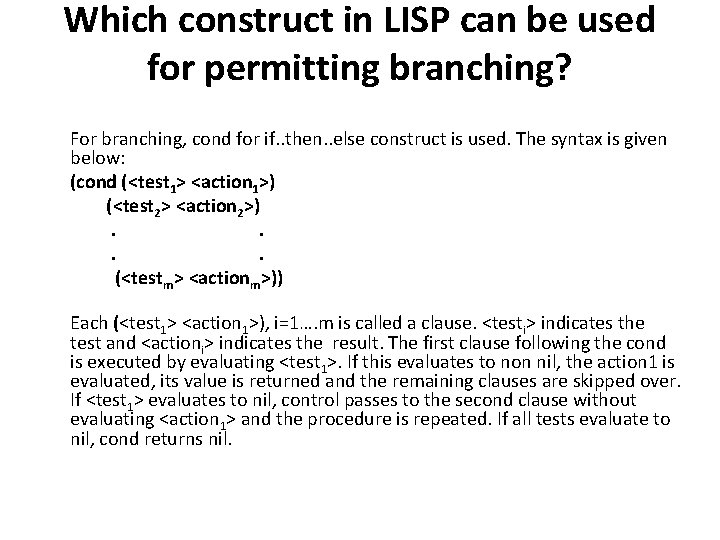
Which construct in LISP can be used for permitting branching? For branching, cond for if. . then. . else construct is used. The syntax is given below: (cond (<test 1> <action 1>) (<test 2> <action 2>) . . (<testm> <actionm>)) Each (<test 1> <action 1>), i=1…. m is called a clause. <testi> indicates the test and <actioni> indicates the result. The first clause following the cond is executed by evaluating <test 1>. If this evaluates to non nil, the action 1 is evaluated, its value is returned and the remaining clauses are skipped over. If <test 1> evaluates to nil, control passes to the second clause without evaluating <action 1> and the procedure is repeated. If all tests evaluate to nil, cond returns nil.
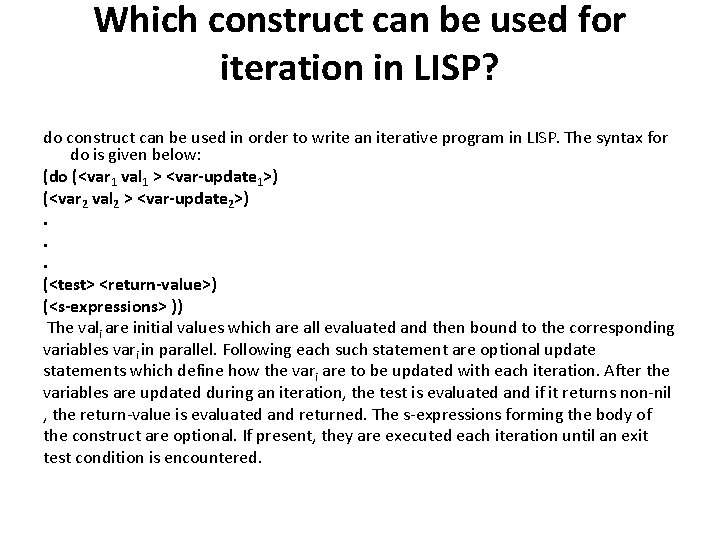
Which construct can be used for iteration in LISP? do construct can be used in order to write an iterative program in LISP. The syntax for do is given below: (do (<var 1 val 1 > <var-update 1>) (<var 2 val 2 > <var-update 2>). . . (<test> <return-value>) (<s-expressions> )) The vali are initial values which are all evaluated and then bound to the corresponding variables vari in parallel. Following each such statement are optional update statements which define how the vari are to be updated with each iteration. After the variables are updated during an iteration, the test is evaluated and if it returns non-nil , the return-value is evaluated and returned. The s-expressions forming the body of the construct are optional. If present, they are executed each iteration until an exit test condition is encountered.
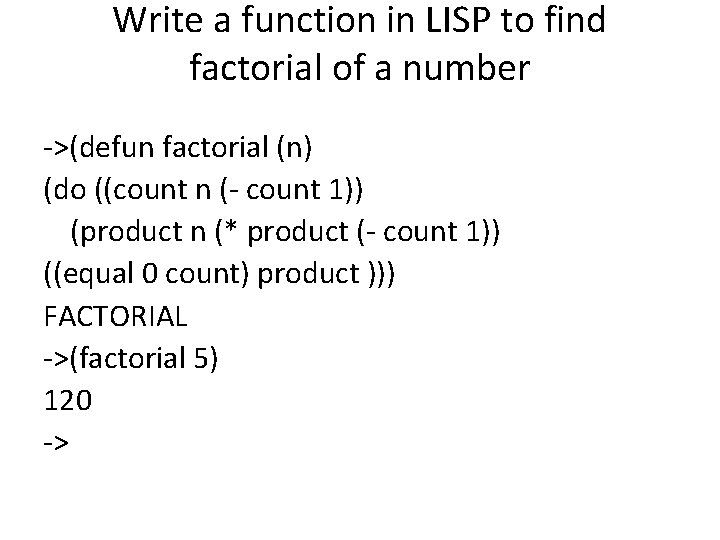
Write a function in LISP to find factorial of a number ->(defun factorial (n) (do ((count n (- count 1)) (product n (* product (- count 1)) ((equal 0 count) product ))) FACTORIAL ->(factorial 5) 120 ->
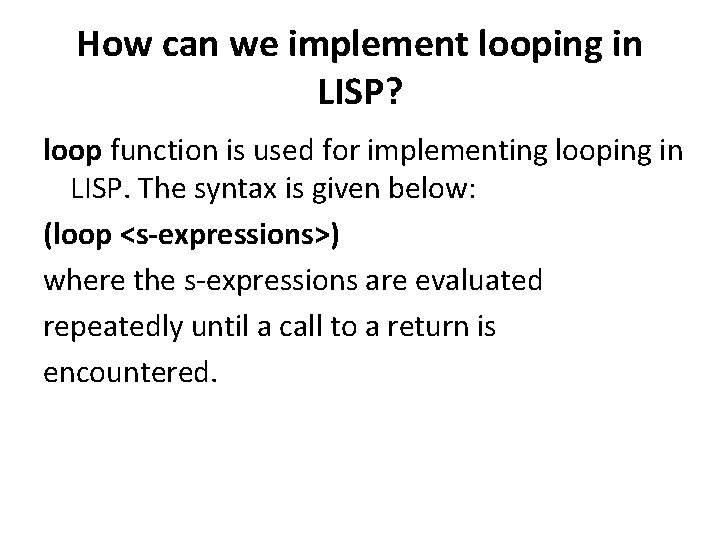
How can we implement looping in LISP? loop function is used for implementing looping in LISP. The syntax is given below: (loop <s-expressions>) where the s-expressions are evaluated repeatedly until a call to a return is encountered.
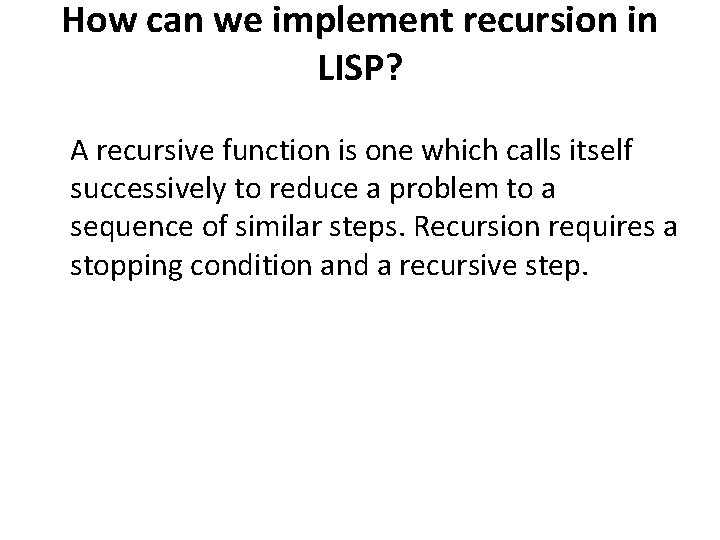
How can we implement recursion in LISP? A recursive function is one which calls itself successively to reduce a problem to a sequence of similar steps. Recursion requires a stopping condition and a recursive step.
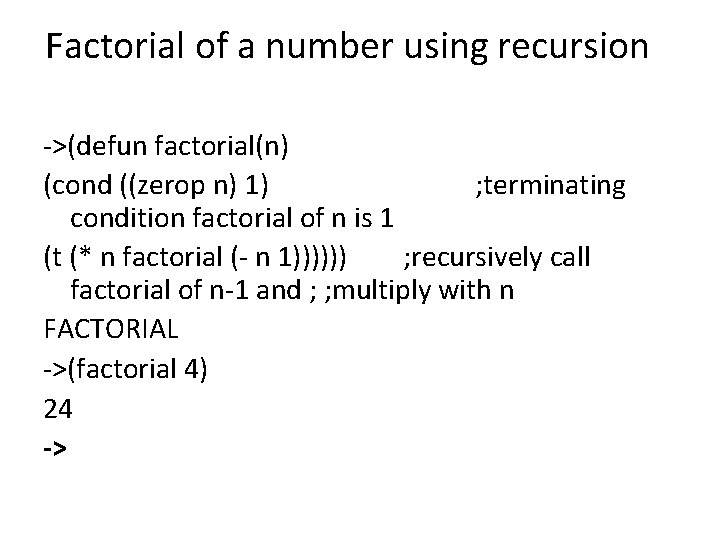
Factorial of a number using recursion ->(defun factorial(n) (cond ((zerop n) 1) ; terminating condition factorial of n is 1 (t (* n factorial (- n 1)))))) ; recursively call factorial of n-1 and ; ; multiply with n FACTORIAL ->(factorial 4) 24 ->
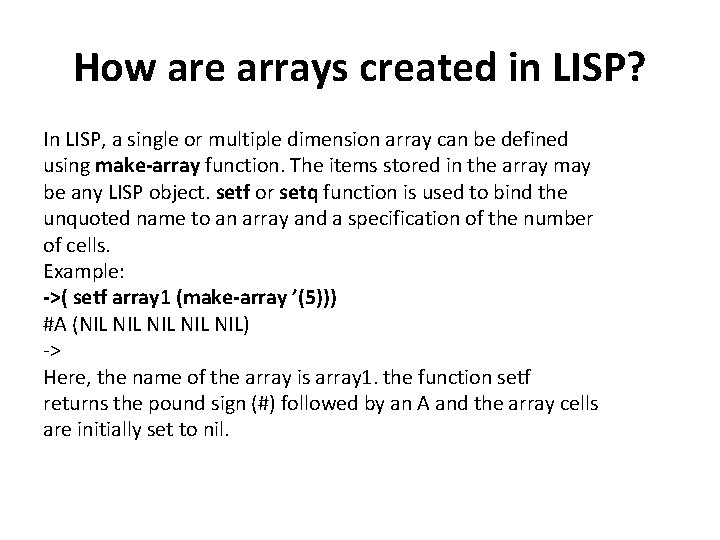
How are arrays created in LISP? In LISP, a single or multiple dimension array can be defined using make-array function. The items stored in the array may be any LISP object. setf or setq function is used to bind the unquoted name to an array and a specification of the number of cells. Example: ->( setf array 1 (make-array ’(5))) #A (NIL NIL NIL) -> Here, the name of the array is array 1. the function setf returns the pound sign (#) followed by an A and the array cells are initially set to nil.
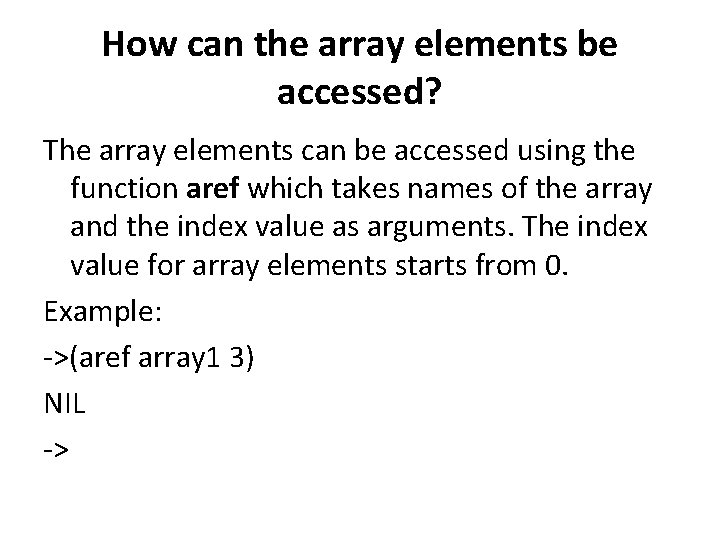
How can the array elements be accessed? The array elements can be accessed using the function aref which takes names of the array and the index value as arguments. The index value for array elements starts from 0. Example: ->(aref array 1 3) NIL ->
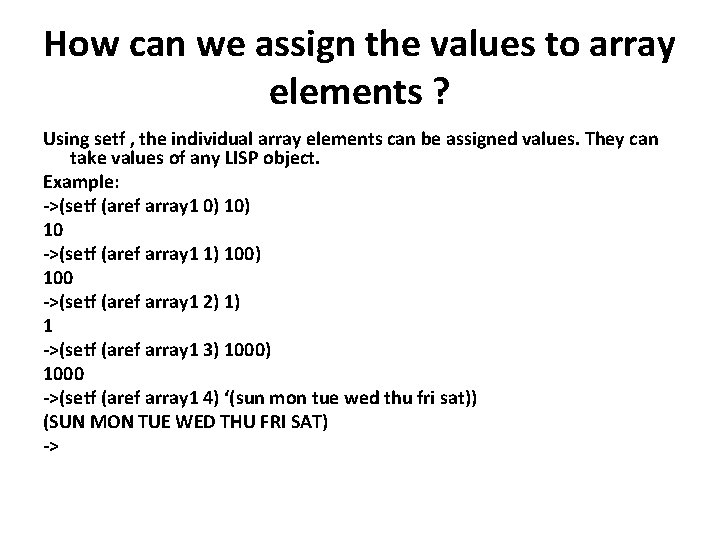
How can we assign the values to array elements ? Using setf , the individual array elements can be assigned values. They can take values of any LISP object. Example: ->(setf (aref array 1 0) 10 ->(setf (aref array 1 1) 100 ->(setf (aref array 1 2) 1) 1 ->(setf (aref array 1 3) 1000 ->(setf (aref array 1 4) ‘(sun mon tue wed thu fri sat)) (SUN MON TUE WED THU FRI SAT) ->
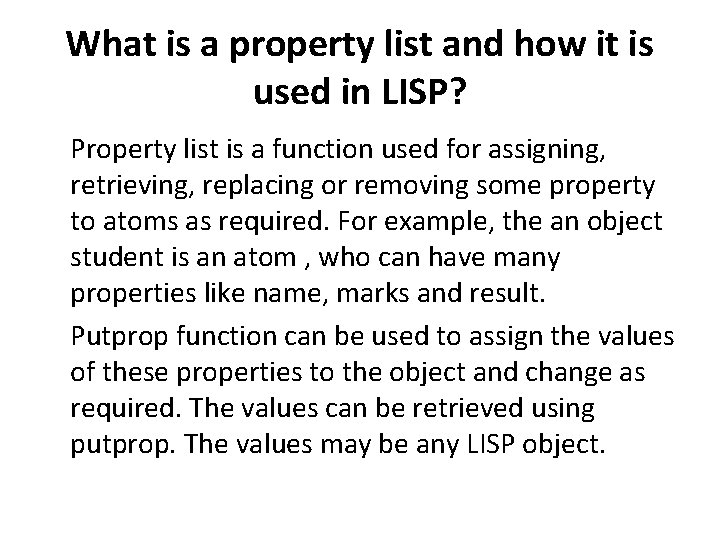
What is a property list and how it is used in LISP? Property list is a function used for assigning, retrieving, replacing or removing some property to atoms as required. For example, the an object student is an atom , who can have many properties like name, marks and result. Putprop function can be used to assign the values of these properties to the object and change as required. The values can be retrieved using putprop. The values may be any LISP object.
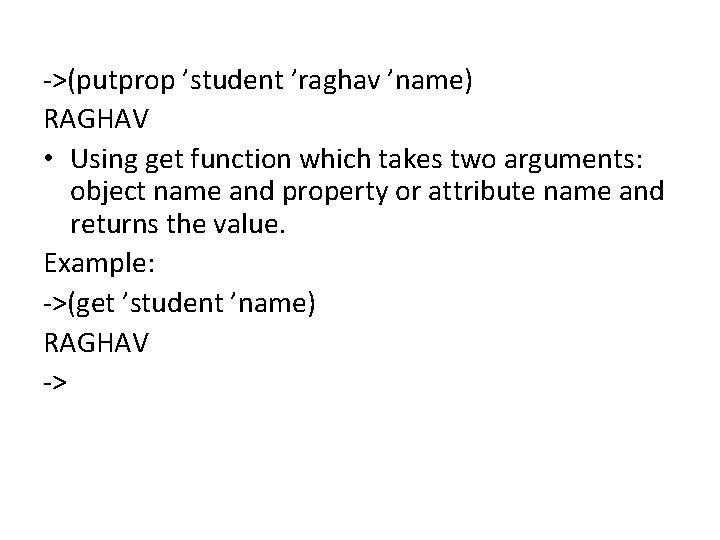
->(putprop ’student ’raghav ’name) RAGHAV • Using get function which takes two arguments: object name and property or attribute name and returns the value. Example: ->(get ’student ’name) RAGHAV ->
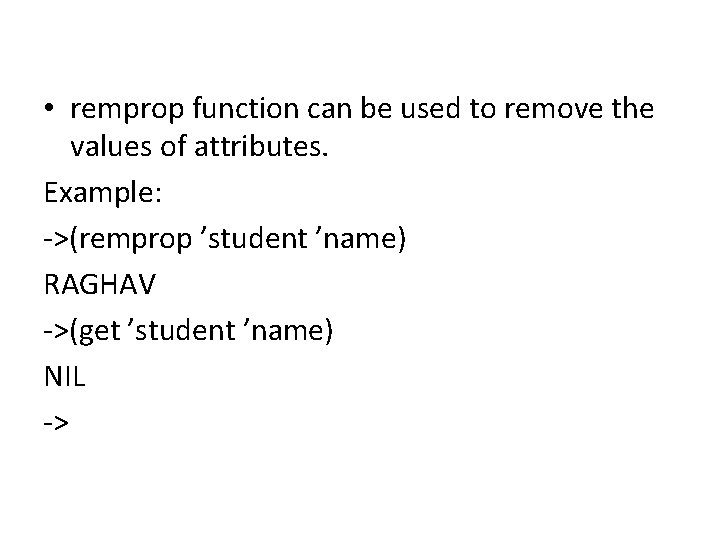
• remprop function can be used to remove the values of attributes. Example: ->(remprop ’student ’name) RAGHAV ->(get ’student ’name) NIL ->
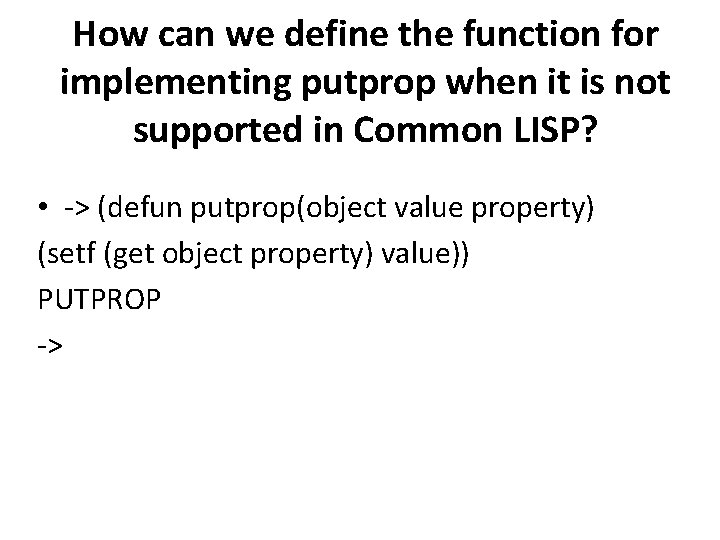
How can we define the function for implementing putprop when it is not supported in Common LISP? • -> (defun putprop(object value property) (setf (get object property) value)) PUTPROP ->
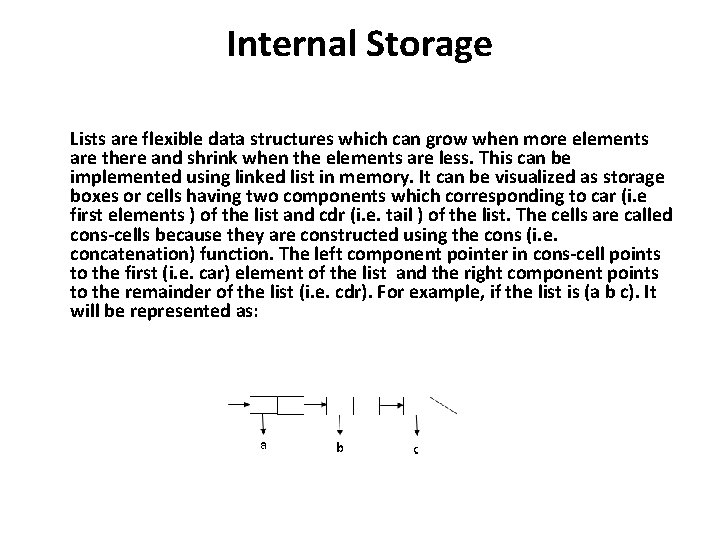
Internal Storage Lists are flexible data structures which can grow when more elements are there and shrink when the elements are less. This can be implemented using linked list in memory. It can be visualized as storage boxes or cells having two components which corresponding to car (i. e first elements ) of the list and cdr (i. e. tail ) of the list. The cells are called cons-cells because they are constructed using the cons (i. e. concatenation) function. The left component pointer in cons-cell points to the first (i. e. car) element of the list and the right component points to the remainder of the list (i. e. cdr). For example, if the list is (a b c). It will be represented as:
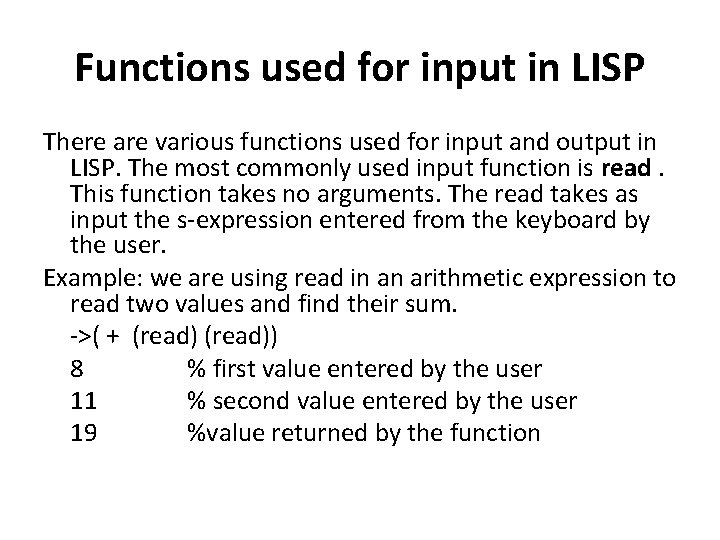
Functions used for input in LISP There are various functions used for input and output in LISP. The most commonly used input function is read. This function takes no arguments. The read takes as input the s-expression entered from the keyboard by the user. Example: we are using read in an arithmetic expression to read two values and find their sum. ->( + (read)) 8 % first value entered by the user 11 % second value entered by the user 19 %value returned by the function
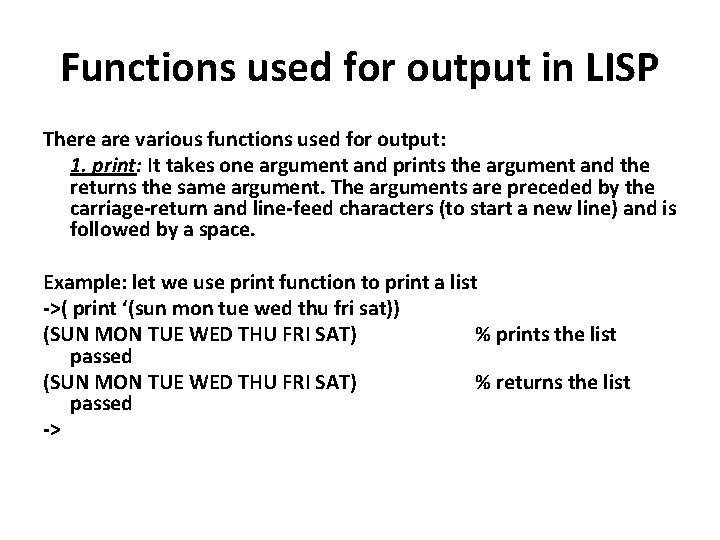
Functions used for output in LISP There are various functions used for output: 1. print: It takes one argument and prints the argument and the returns the same argument. The arguments are preceded by the carriage-return and line-feed characters (to start a new line) and is followed by a space. Example: let we use print function to print a list ->( print ‘(sun mon tue wed thu fri sat)) (SUN MON TUE WED THU FRI SAT) % prints the list passed (SUN MON TUE WED THU FRI SAT) % returns the list passed ->
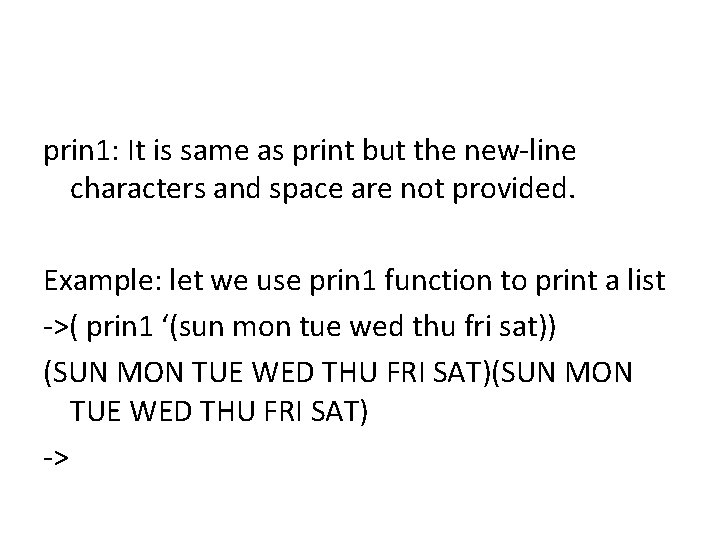
prin 1: It is same as print but the new-line characters and space are not provided. Example: let we use prin 1 function to print a list ->( prin 1 ‘(sun mon tue wed thu fri sat)) (SUN MON TUE WED THU FRI SAT) ->
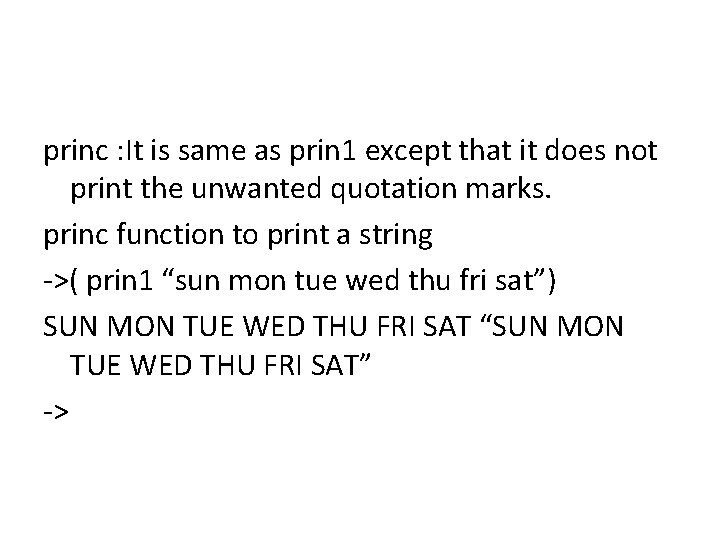
princ : It is same as prin 1 except that it does not print the unwanted quotation marks. princ function to print a string ->( prin 1 “sun mon tue wed thu fri sat”) SUN MON TUE WED THU FRI SAT “SUN MON TUE WED THU FRI SAT” ->
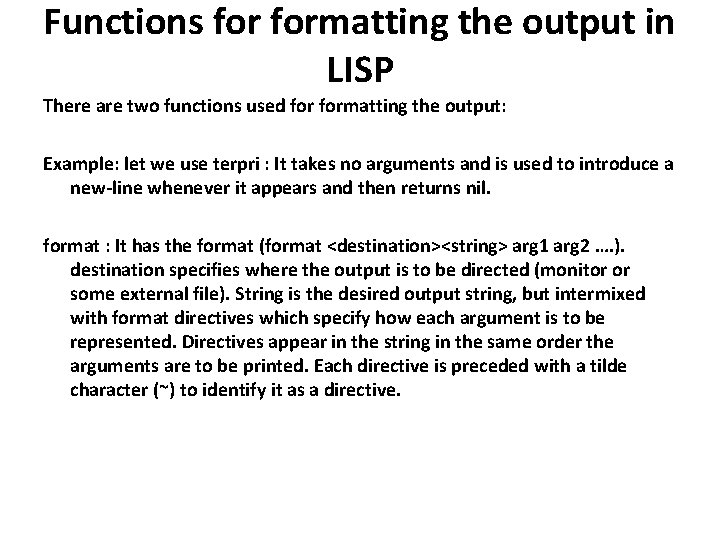
Functions formatting the output in LISP There are two functions used formatting the output: Example: let we use terpri : It takes no arguments and is used to introduce a new-line whenever it appears and then returns nil. format : It has the format (format <destination><string> arg 1 arg 2 …. ). destination specifies where the output is to be directed (monitor or some external file). String is the desired output string, but intermixed with format directives which specify how each argument is to be represented. Directives appear in the string in the same order the arguments are to be printed. Each directive is preceded with a tilde character (~) to identify it as a directive.
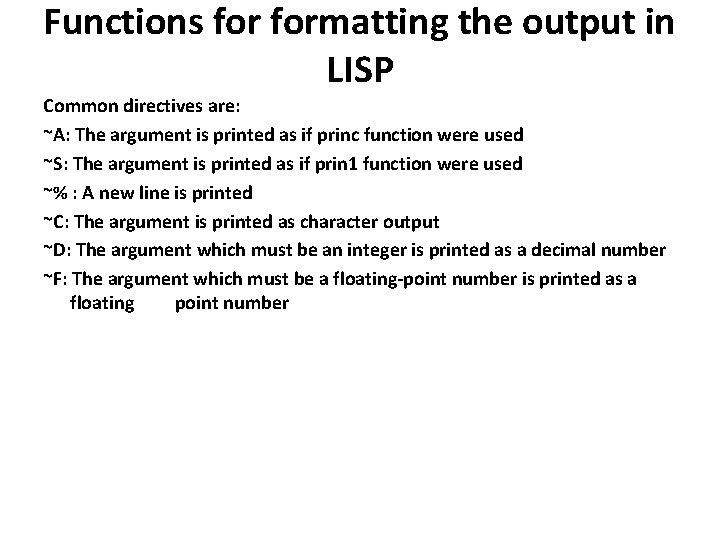
Functions formatting the output in LISP Common directives are: ~A: The argument is printed as if princ function were used ~S: The argument is printed as if prin 1 function were used ~% : A new line is printed ~C: The argument is printed as character output ~D: The argument which must be an integer is printed as a decimal number ~F: The argument which must be a floating-point number is printed as a floating point number
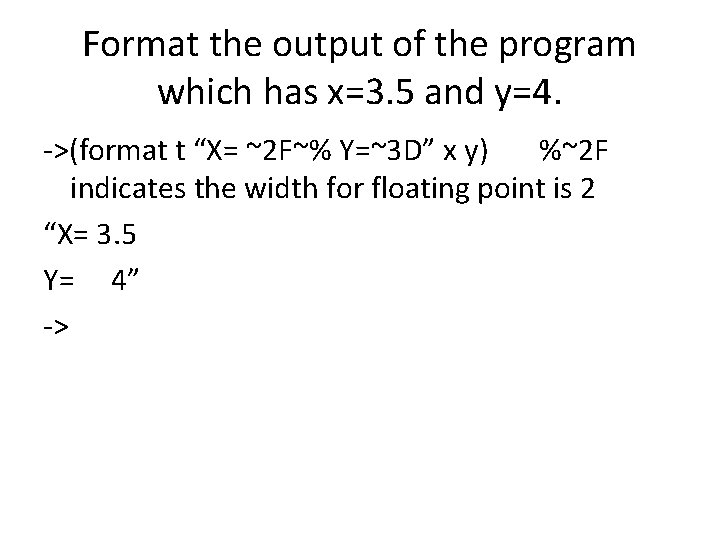
Format the output of the program which has x=3. 5 and y=4. ->(format t “X= ~2 F~% Y=~3 D” x y) %~2 F indicates the width for floating point is 2 “X= 3. 5 Y= 4” ->
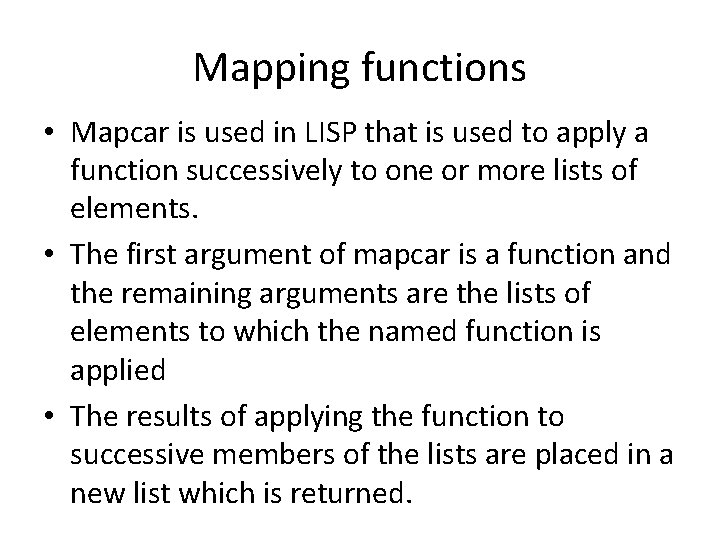
Mapping functions • Mapcar is used in LISP that is used to apply a function successively to one or more lists of elements. • The first argument of mapcar is a function and the remaining arguments are the lists of elements to which the named function is applied • The results of applying the function to successive members of the lists are placed in a new list which is returned.
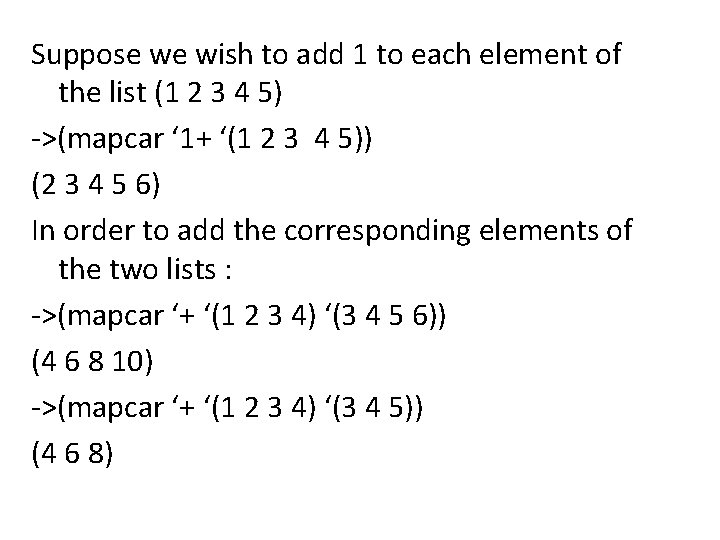
Suppose we wish to add 1 to each element of the list (1 2 3 4 5) ->(mapcar ‘ 1+ ‘(1 2 3 4 5)) (2 3 4 5 6) In order to add the corresponding elements of the two lists : ->(mapcar ‘+ ‘(1 2 3 4) ‘(3 4 5 6)) (4 6 8 10) ->(mapcar ‘+ ‘(1 2 3 4) ‘(3 4 5)) (4 6 8)
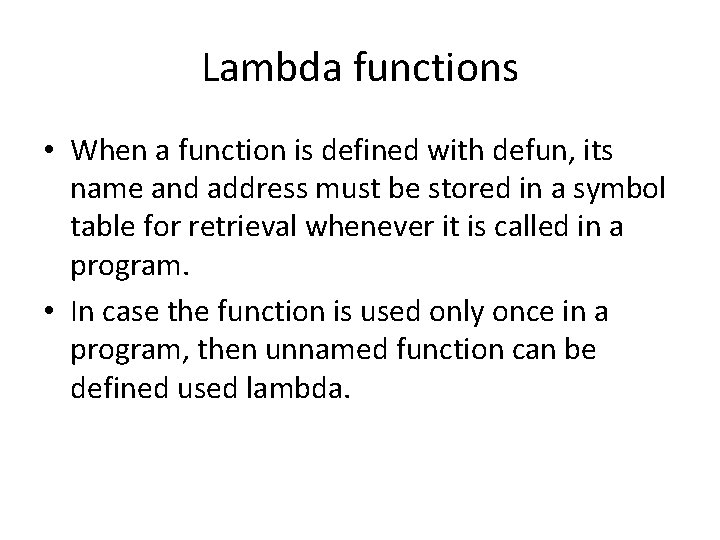
Lambda functions • When a function is defined with defun, its name and address must be stored in a symbol table for retrieval whenever it is called in a program. • In case the function is used only once in a program, then unnamed function can be defined used lambda.
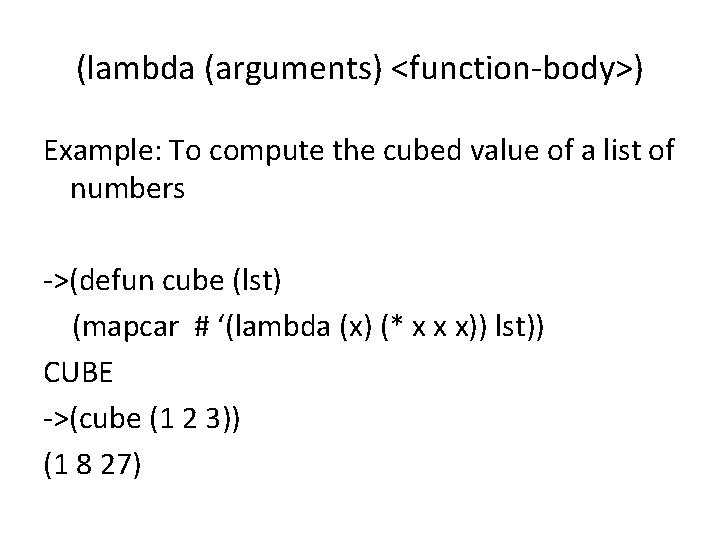
(lambda (arguments) <function-body>) Example: To compute the cubed value of a list of numbers ->(defun cube (lst) (mapcar # ‘(lambda (x) (* x x x)) lst)) CUBE ->(cube (1 2 3)) (1 8 27)
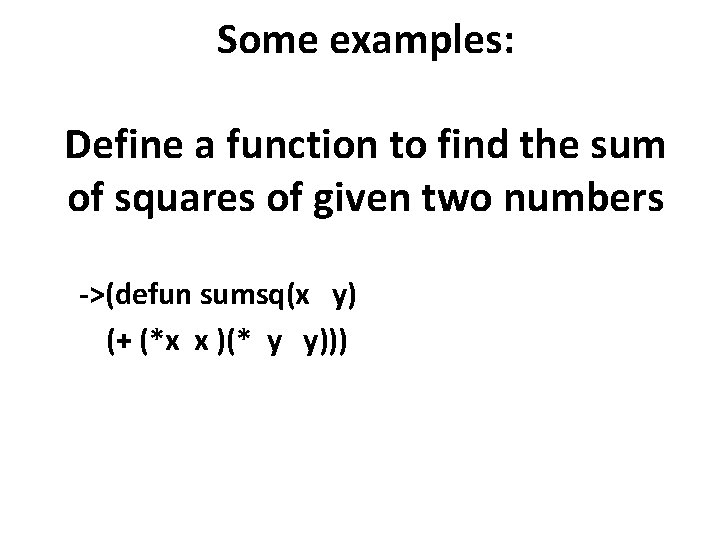
Some examples: Define a function to find the sum of squares of given two numbers ->(defun sumsq(x y) (+ (*x x )(* y y)))
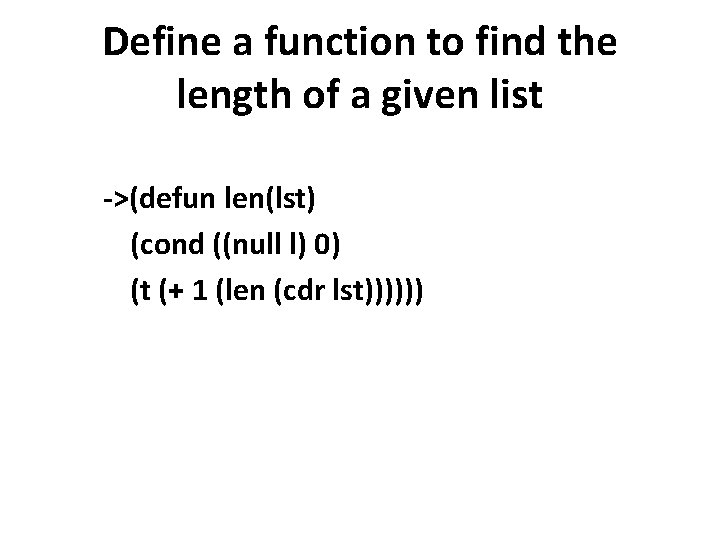
Define a function to find the length of a given list ->(defun len(lst) (cond ((null l) 0) (t (+ 1 (len (cdr lst))))))
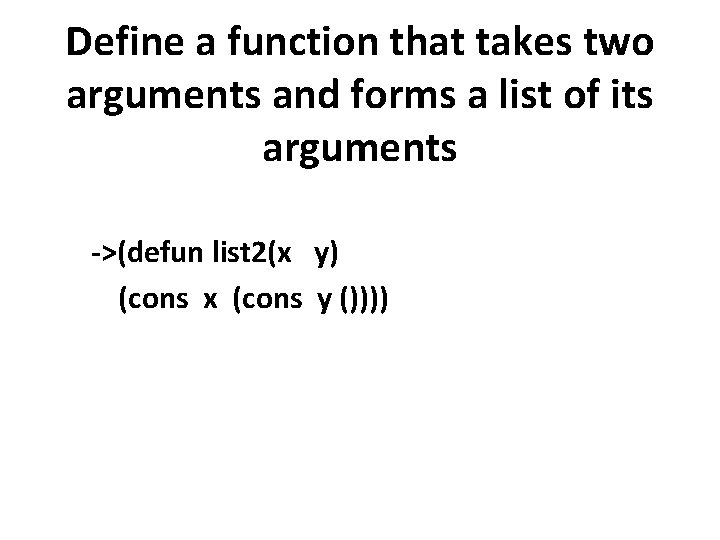
Define a function that takes two arguments and forms a list of its arguments ->(defun list 2(x y) (cons x (cons y ())))
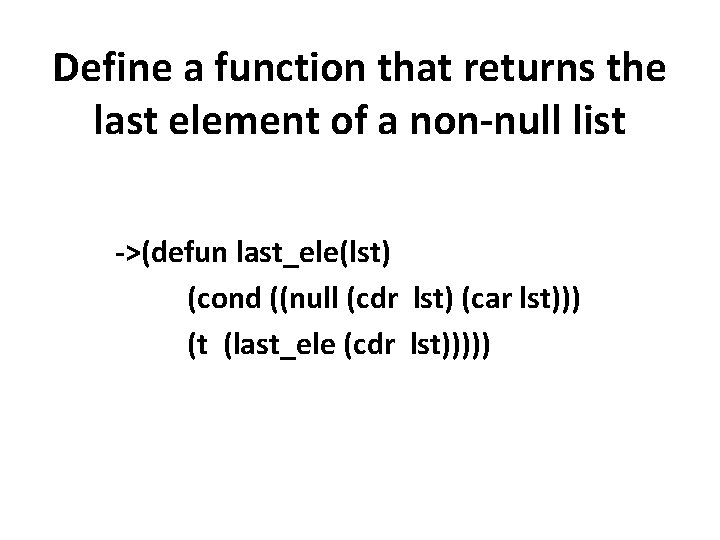
Define a function that returns the last element of a non-null list ->(defun last_ele(lst) (cond ((null (cdr lst) (car lst))) (t (last_ele (cdr lst)))))
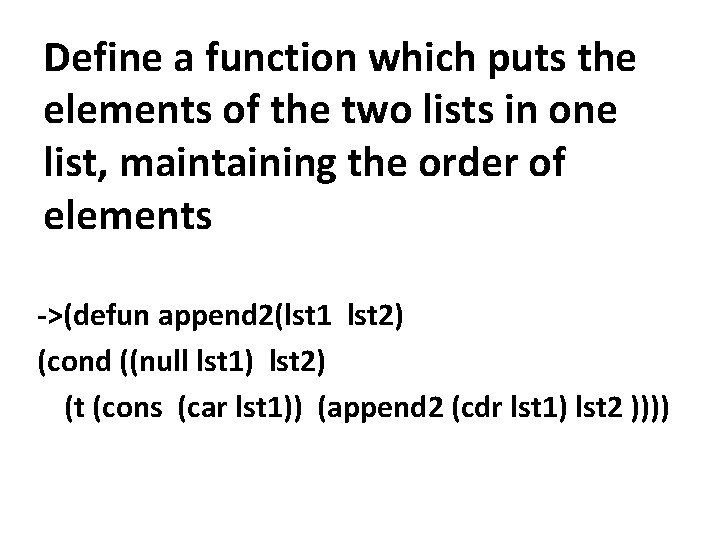
Define a function which puts the elements of the two lists in one list, maintaining the order of elements ->(defun append 2(lst 1 lst 2) (cond ((null lst 1) lst 2) (t (cons (car lst 1)) (append 2 (cdr lst 1) lst 2 ))))
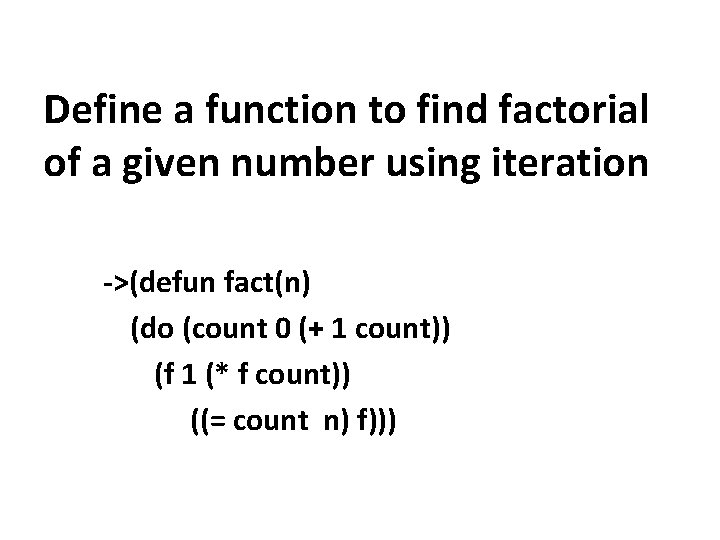
Define a function to find factorial of a given number using iteration ->(defun fact(n) (do (count 0 (+ 1 count)) (f 1 (* f count)) ((= count n) f)))
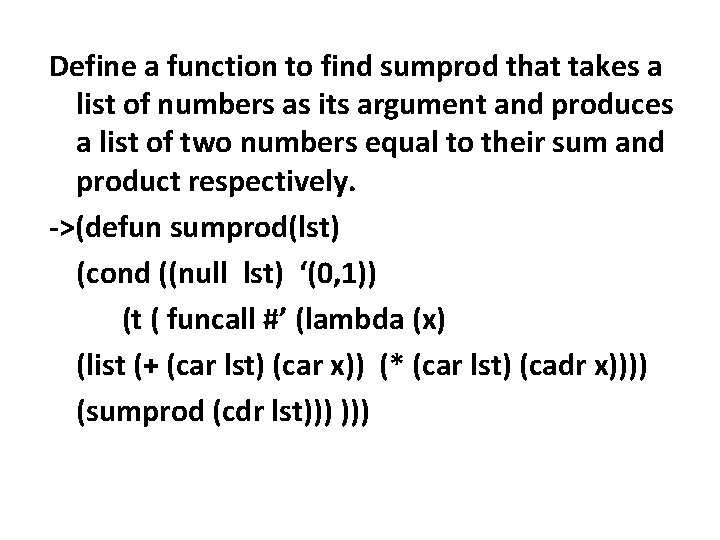
Define a function to find sumprod that takes a list of numbers as its argument and produces a list of two numbers equal to their sum and product respectively. ->(defun sumprod(lst) (cond ((null lst) ‘(0, 1)) (t ( funcall #’ (lambda (x) (list (+ (car lst) (car x)) (* (car lst) (cadr x)))) (sumprod (cdr lst)))