Common Lisp LISTS Mitthgskolan 10242020 1 Lists are
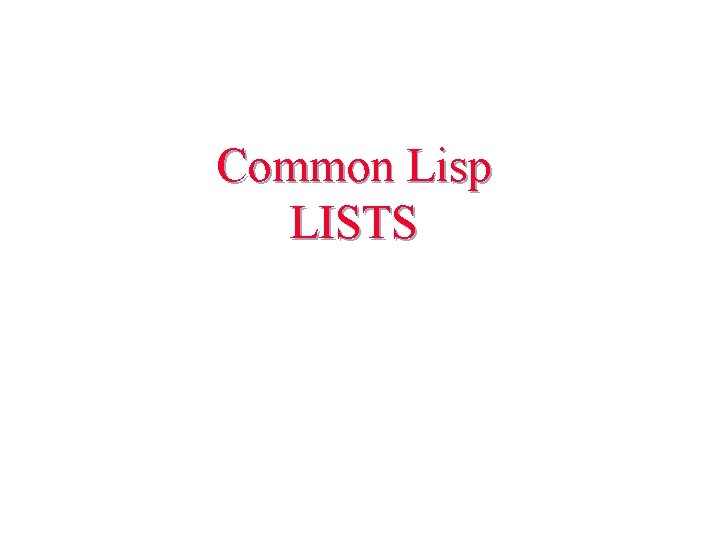
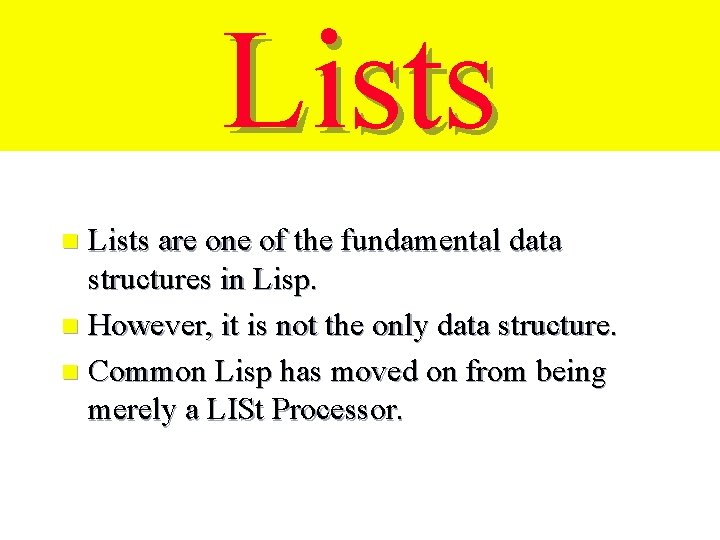
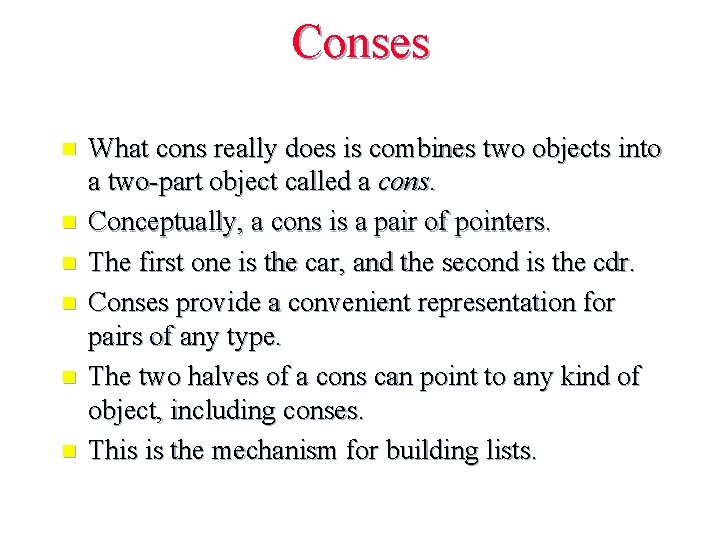
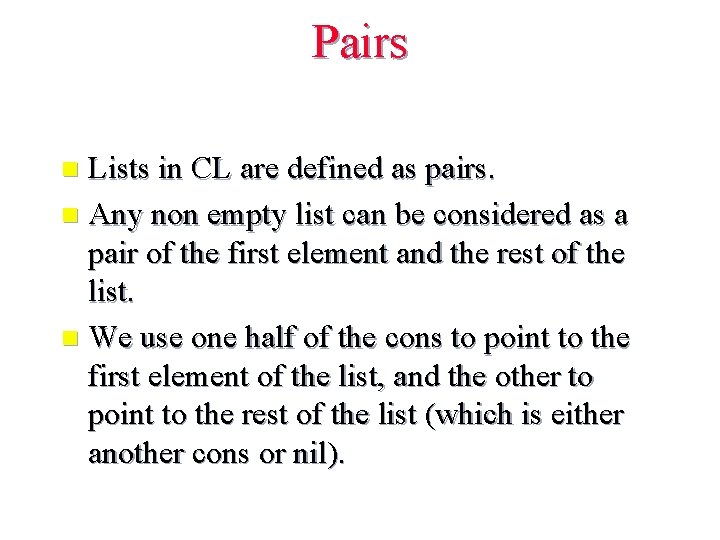
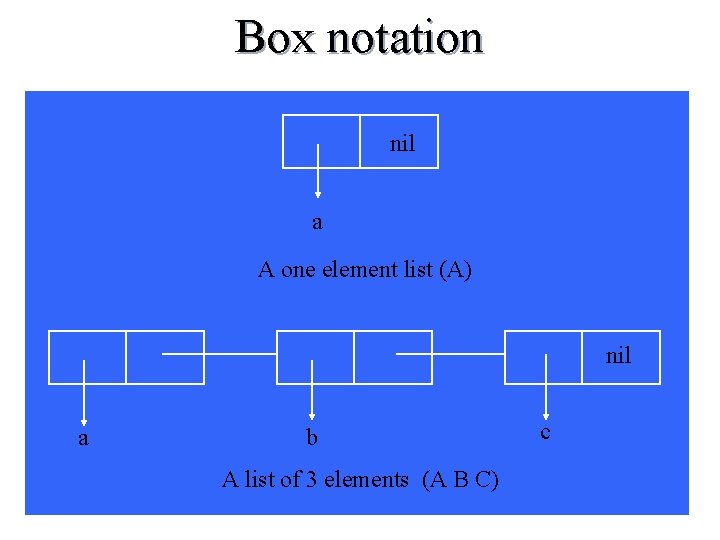
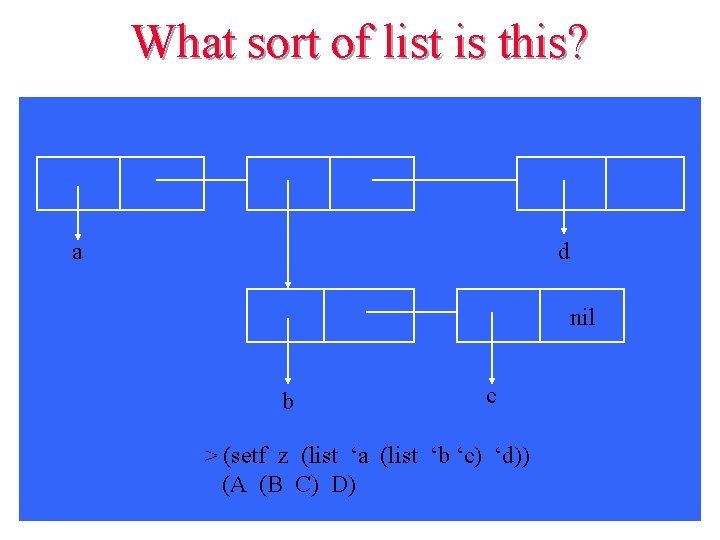
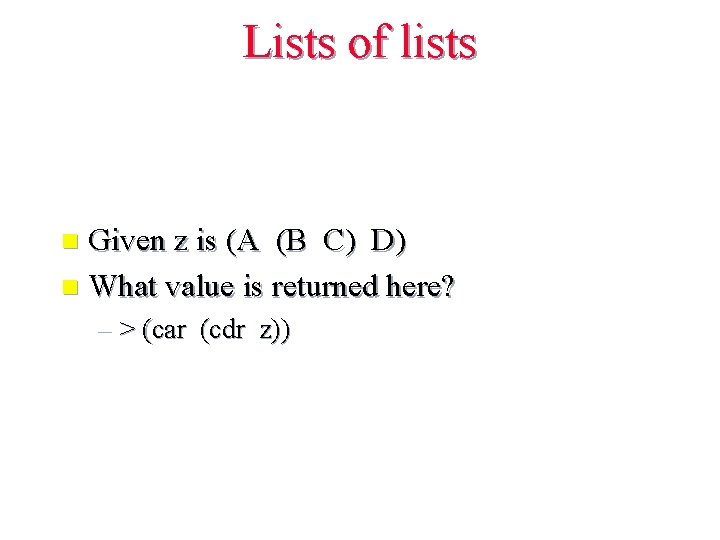
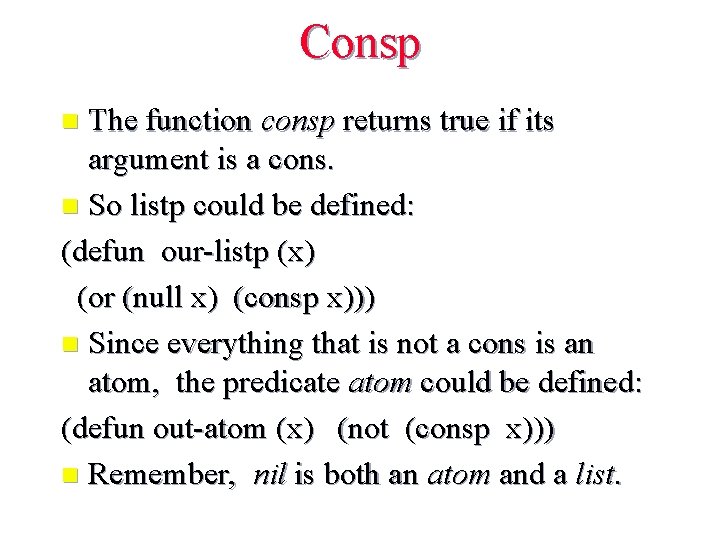
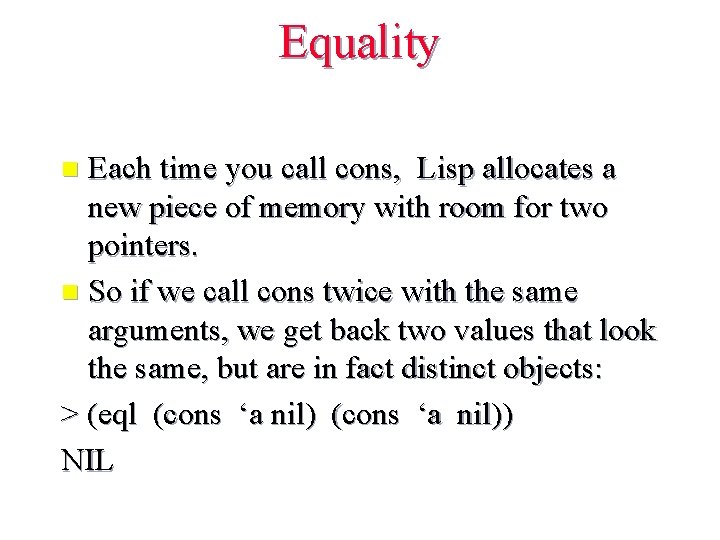
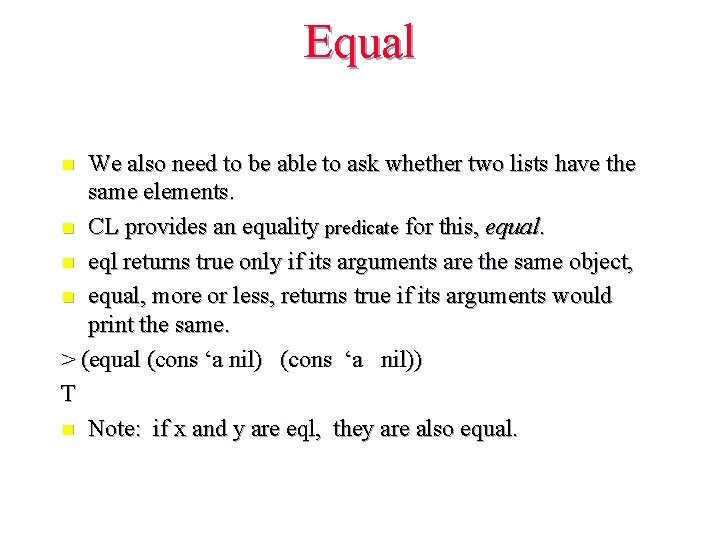
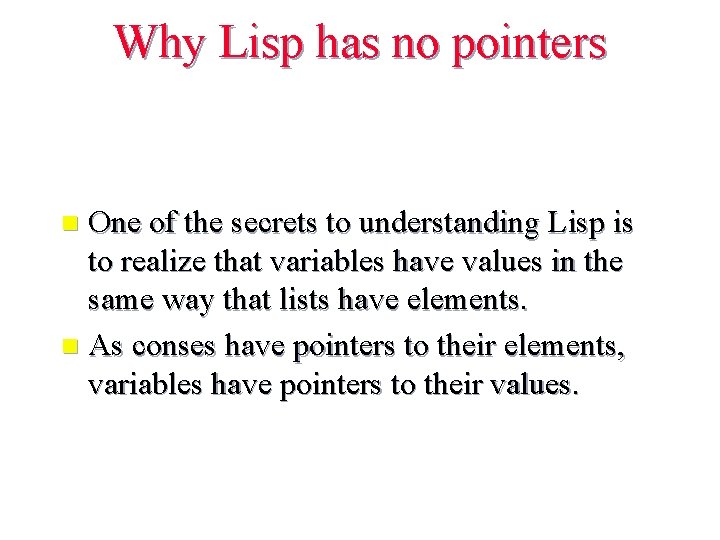
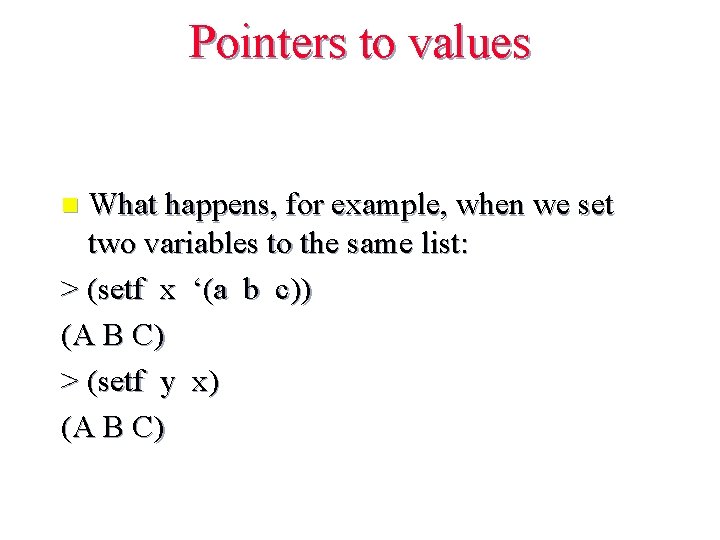
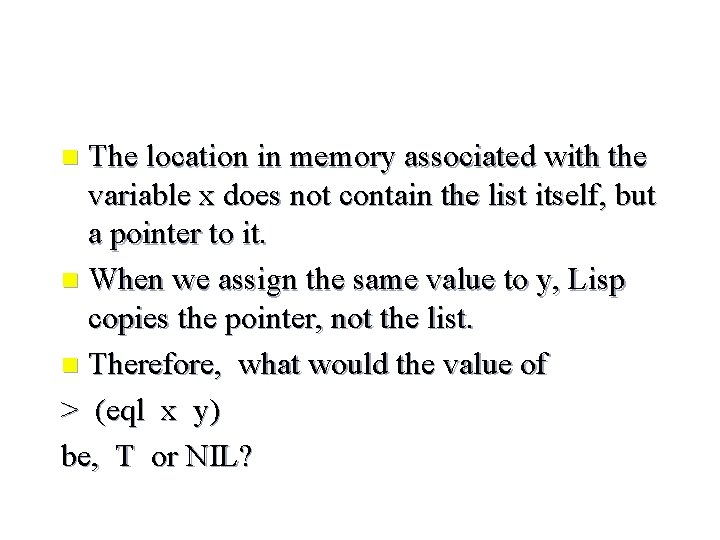
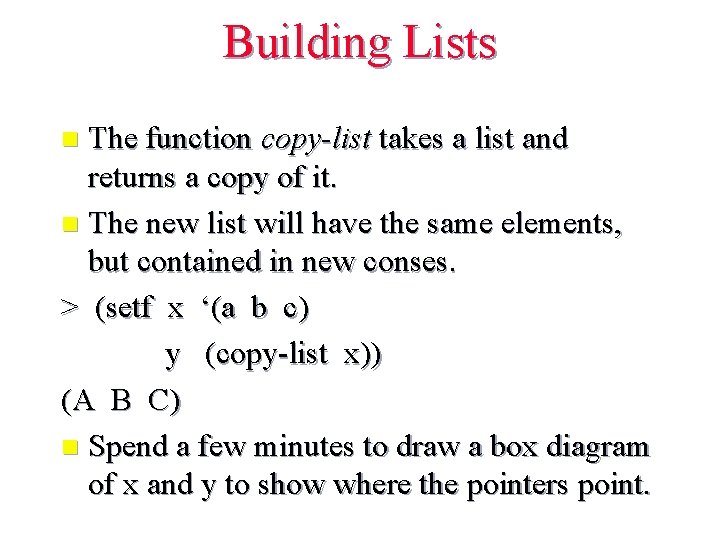
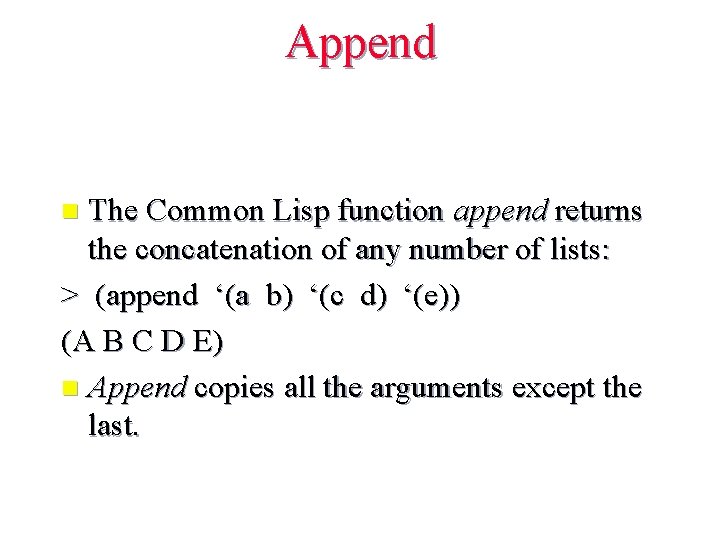
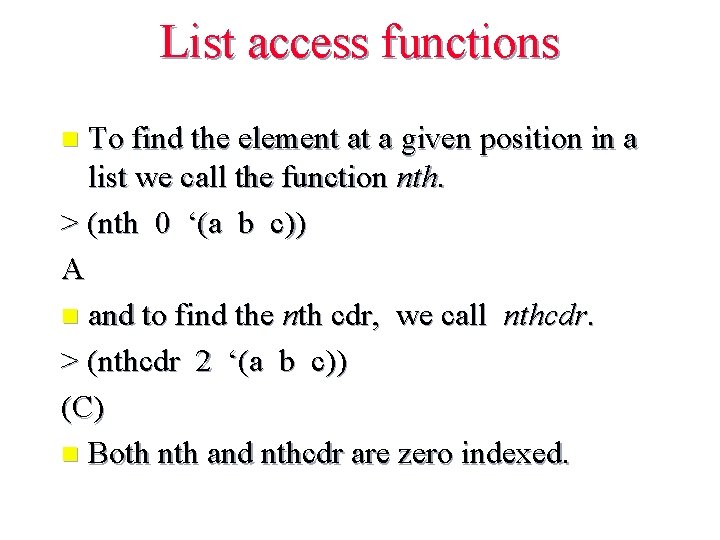
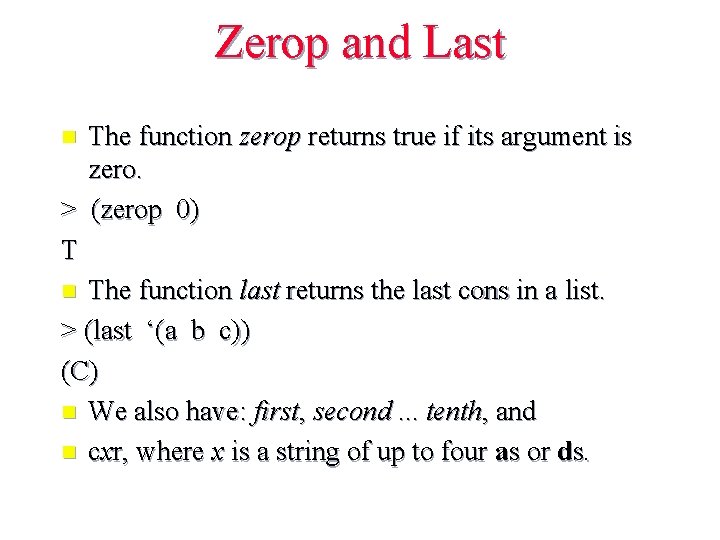
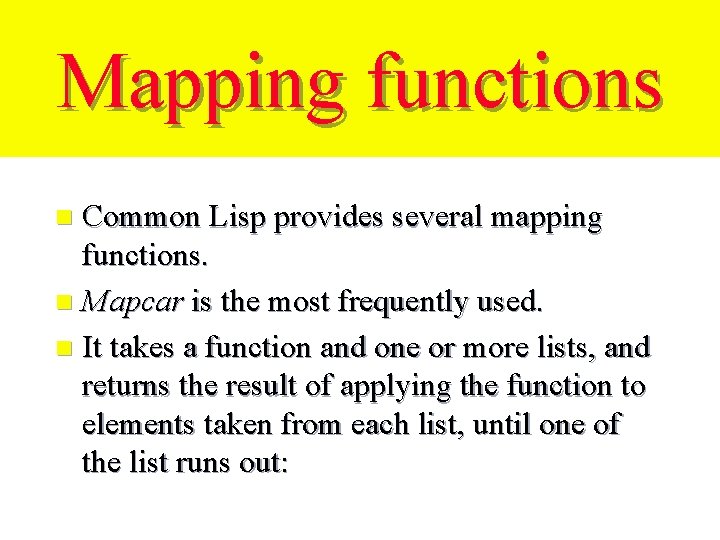
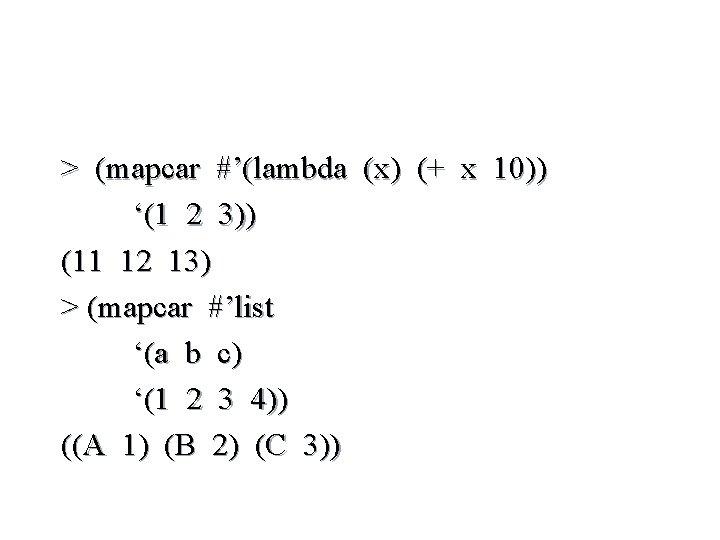
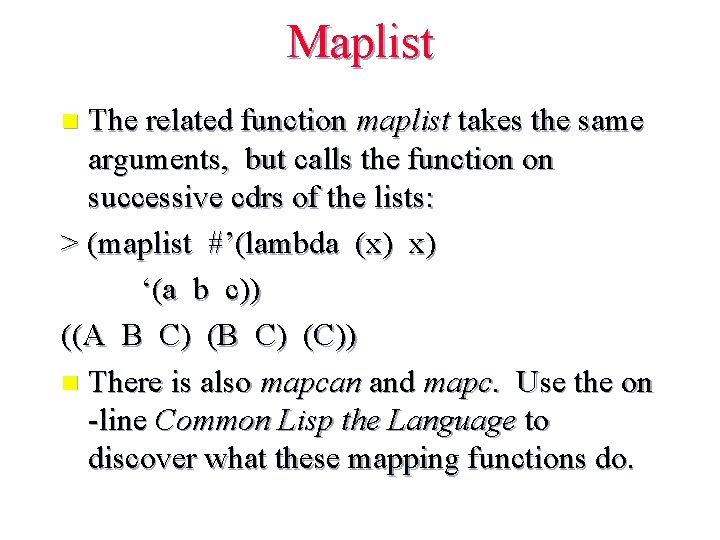
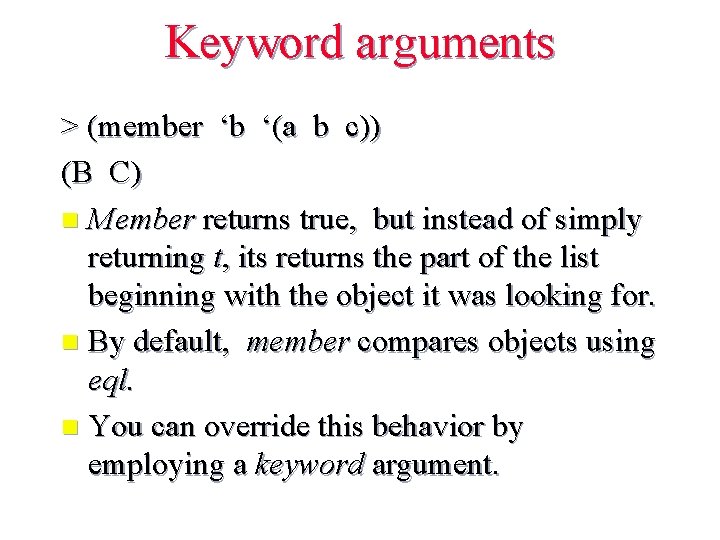
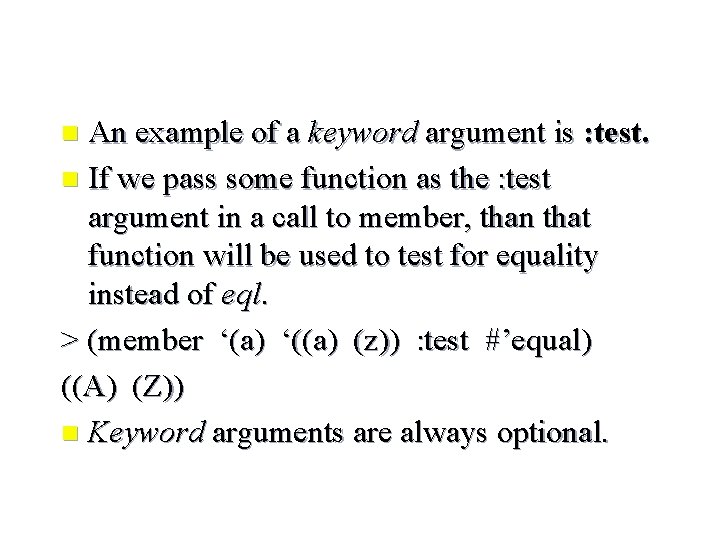
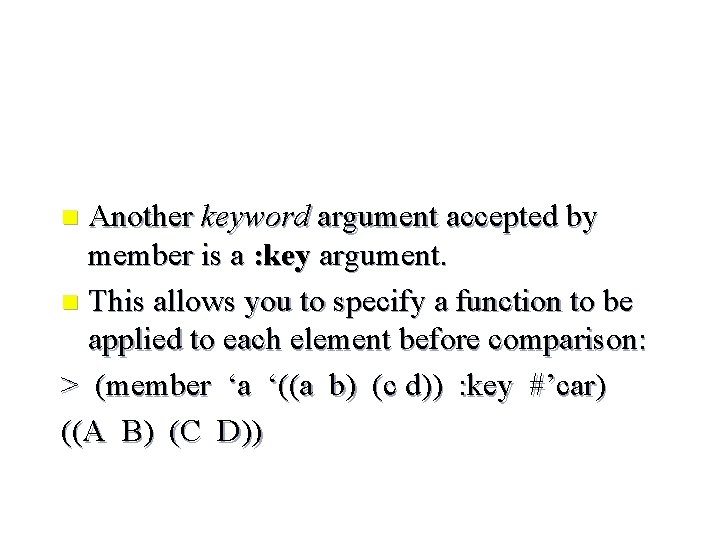
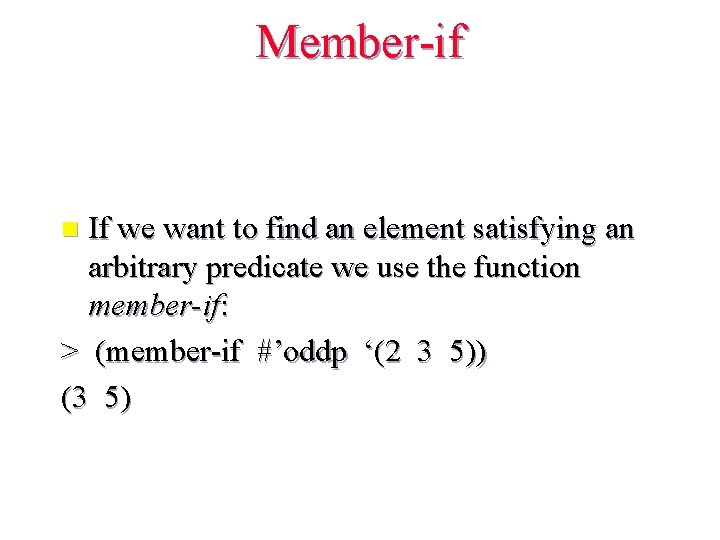
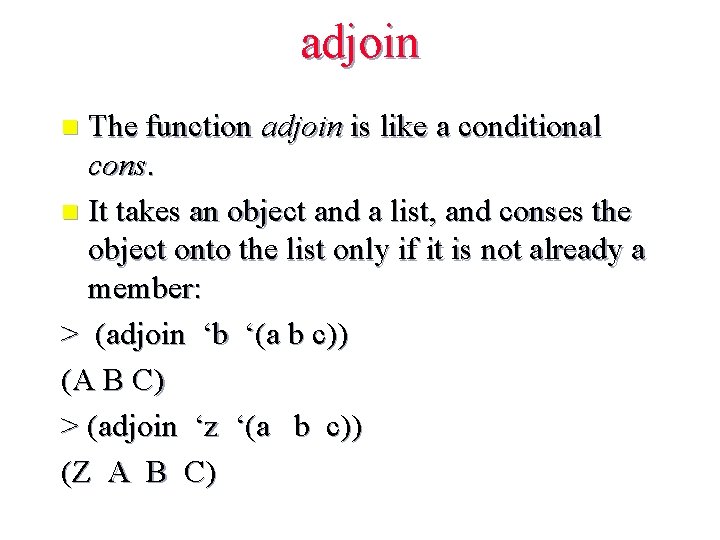
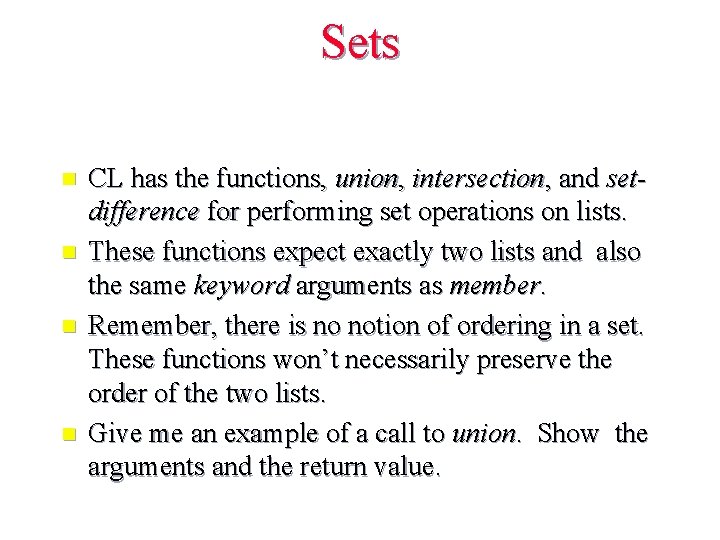
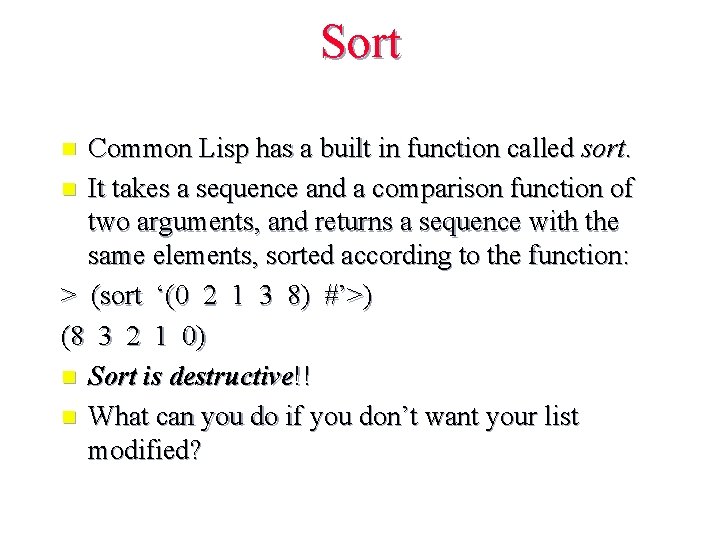
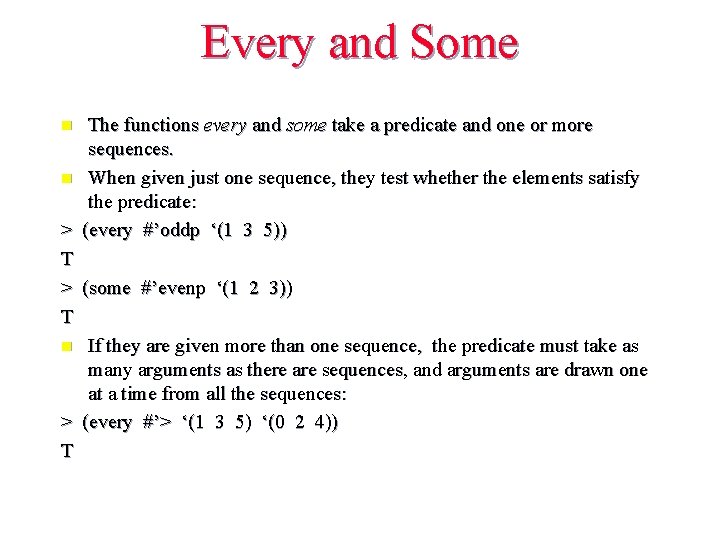
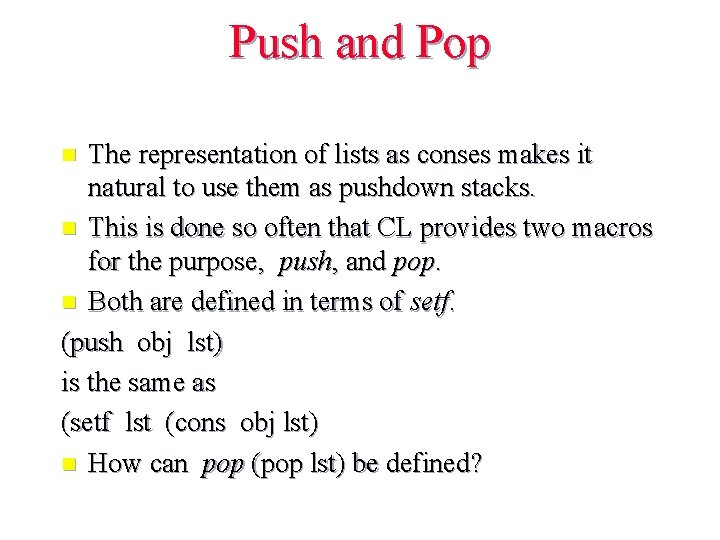
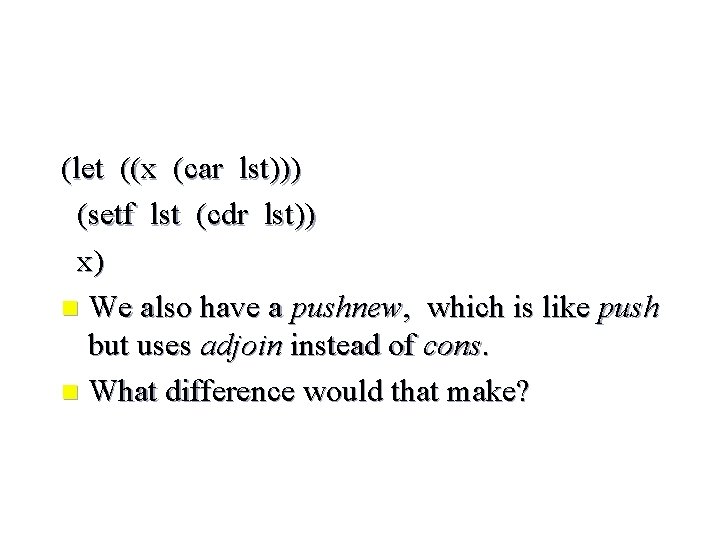
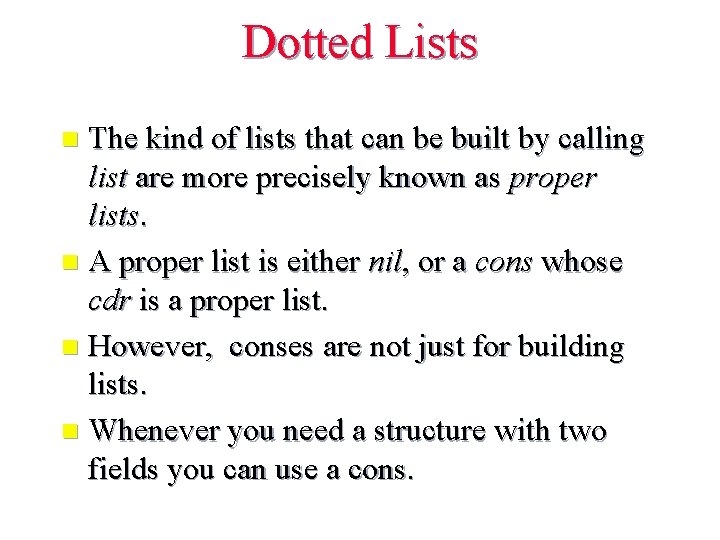
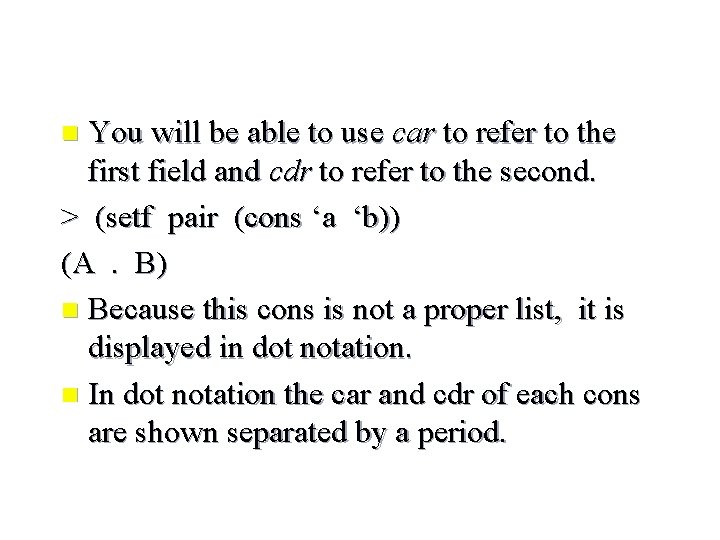
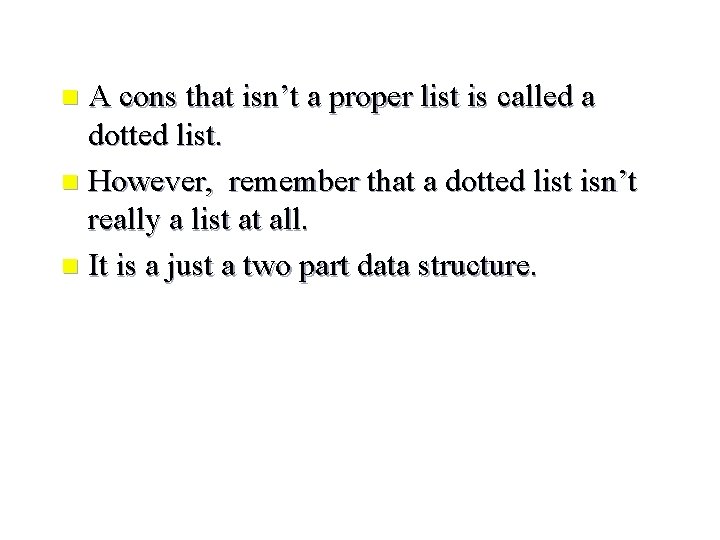
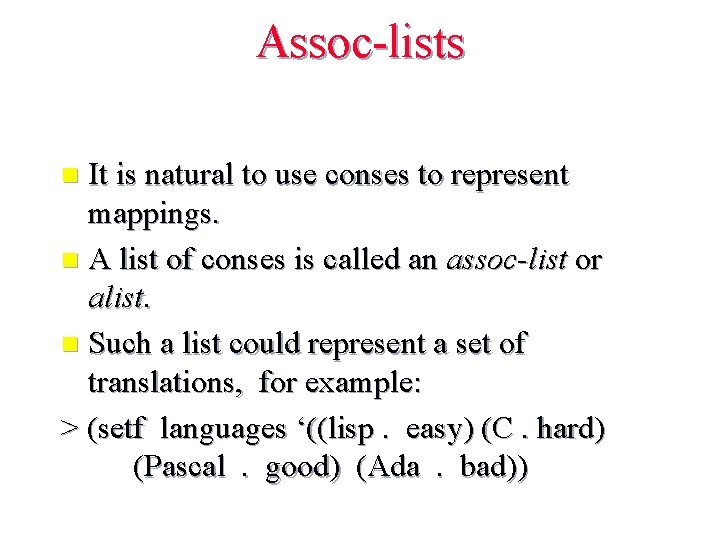
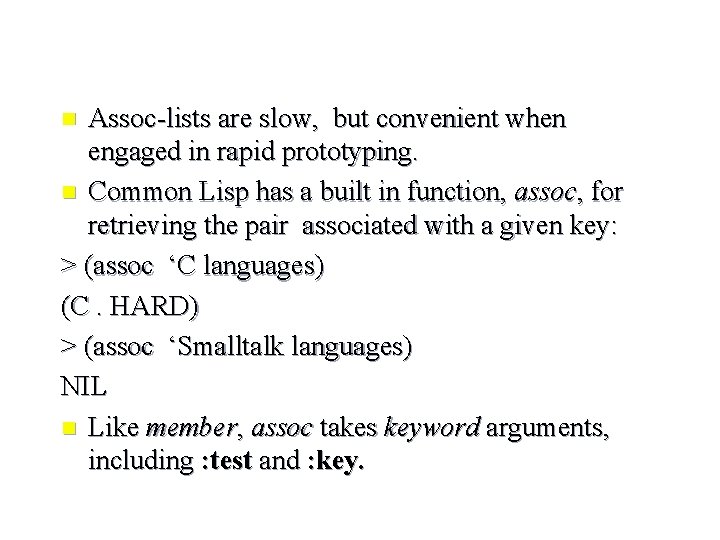
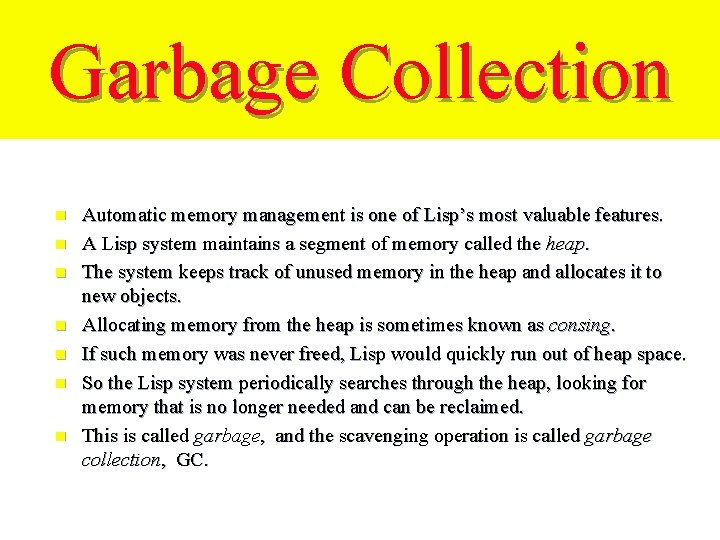
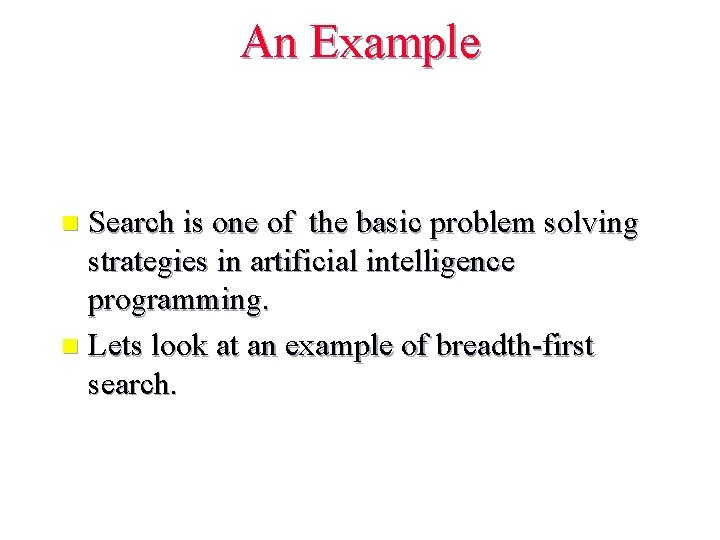
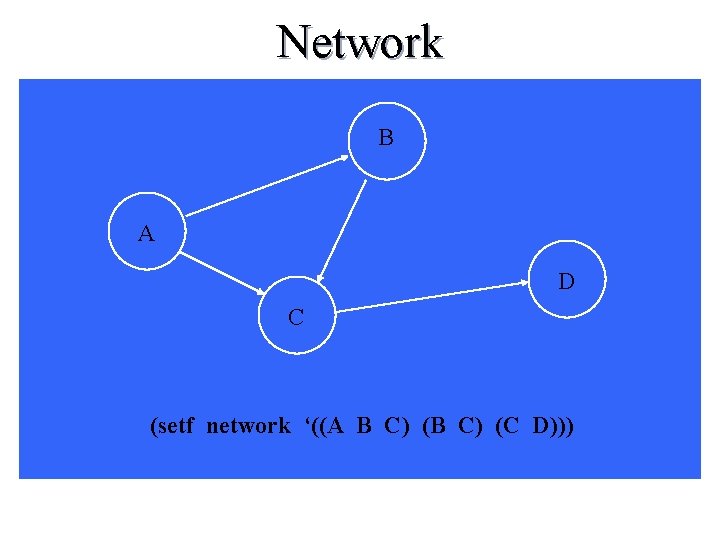
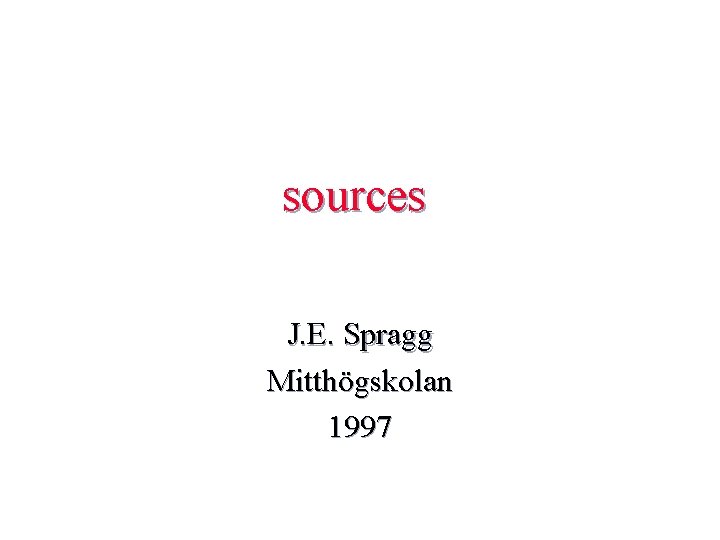
- Slides: 39
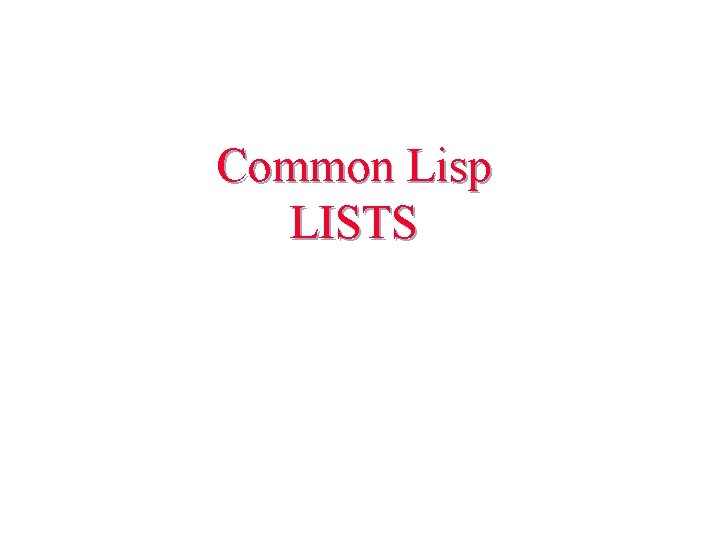
Common Lisp LISTS Mitthögskolan 10/24/2020 1
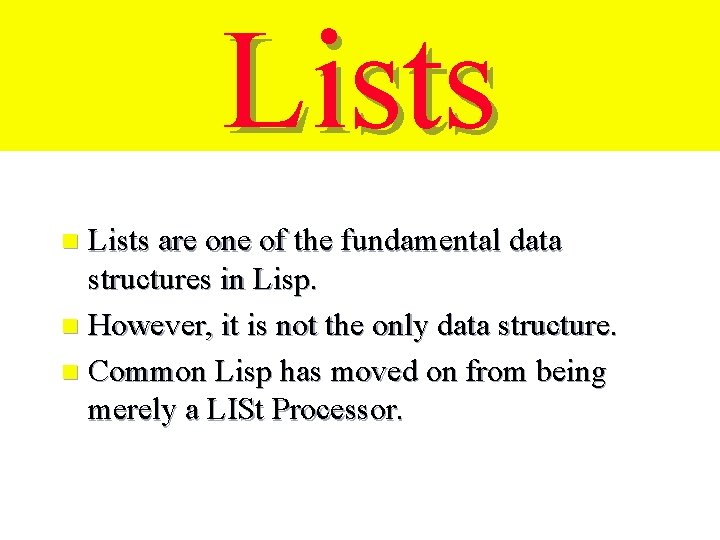
Lists are one of the fundamental data structures in Lisp. n However, it is not the only data structure. n Common Lisp has moved on from being merely a LISt Processor. n Mitthögskolan 10/24/2020 2
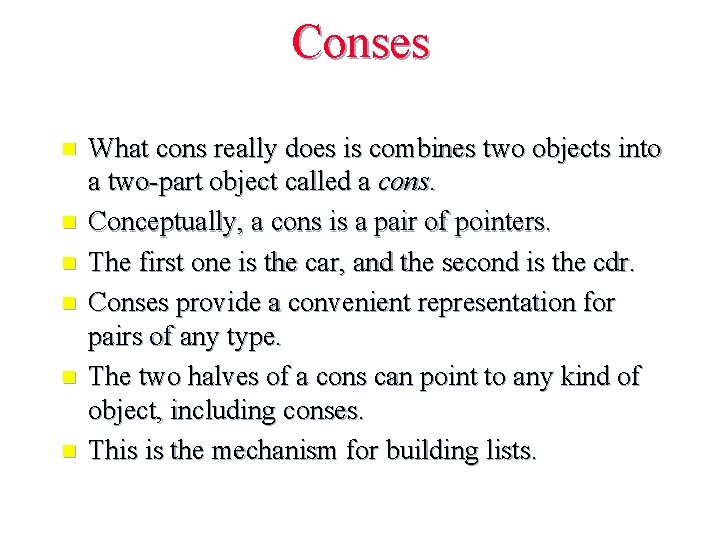
Conses n n n What cons really does is combines two objects into a two-part object called a cons. Conceptually, a cons is a pair of pointers. The first one is the car, and the second is the cdr. Conses provide a convenient representation for pairs of any type. The two halves of a cons can point to any kind of object, including conses. This is the mechanism for building lists. Mitthögskolan 10/24/2020 3
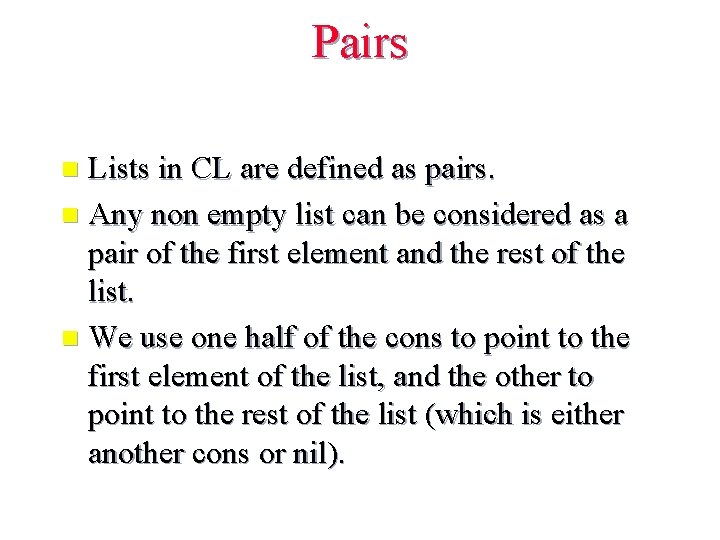
Pairs Lists in CL are defined as pairs. n Any non empty list can be considered as a pair of the first element and the rest of the list. n We use one half of the cons to point to the first element of the list, and the other to point to the rest of the list (which is either another cons or nil). n Mitthögskolan 10/24/2020 4
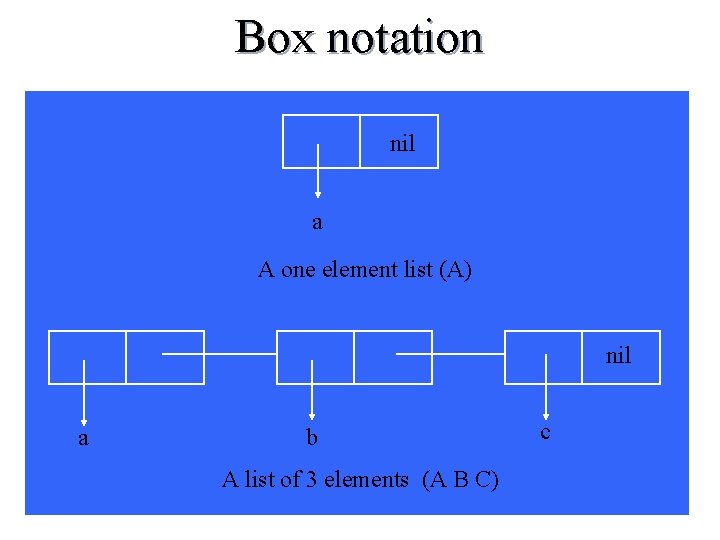
Box notation nil a A one element list (A) nil a c b A list of 3 elements (A B C) Mitthögskolan 10/24/2020 5
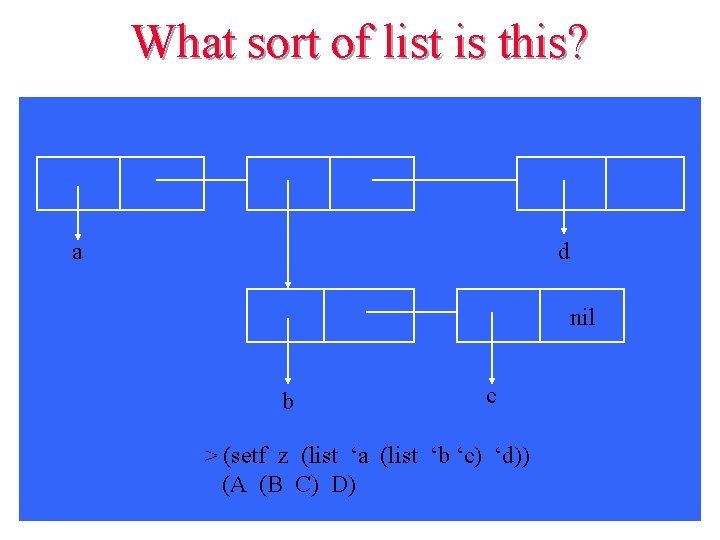
What sort of list is this? a d nil c b > (setf z (list ‘a (list ‘b ‘c) ‘d)) (A (B C) D) Mitthögskolan 10/24/2020 6
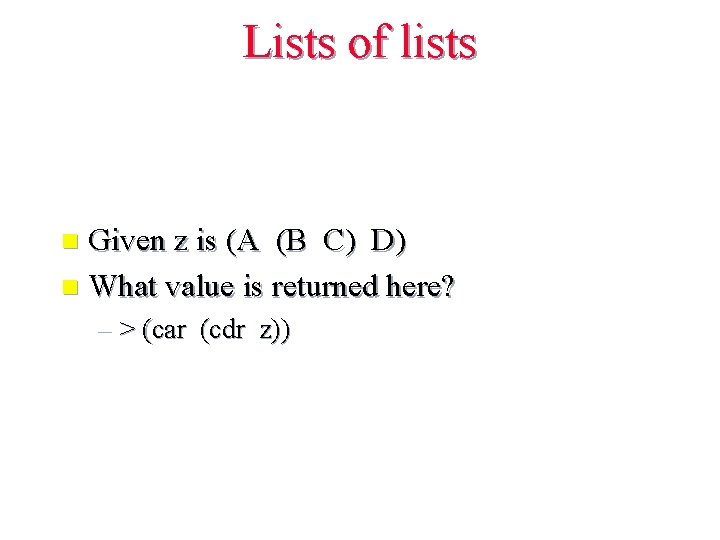
Lists of lists Given z is (A (B C) D) n What value is returned here? n – > (car (cdr z)) Mitthögskolan 10/24/2020 7
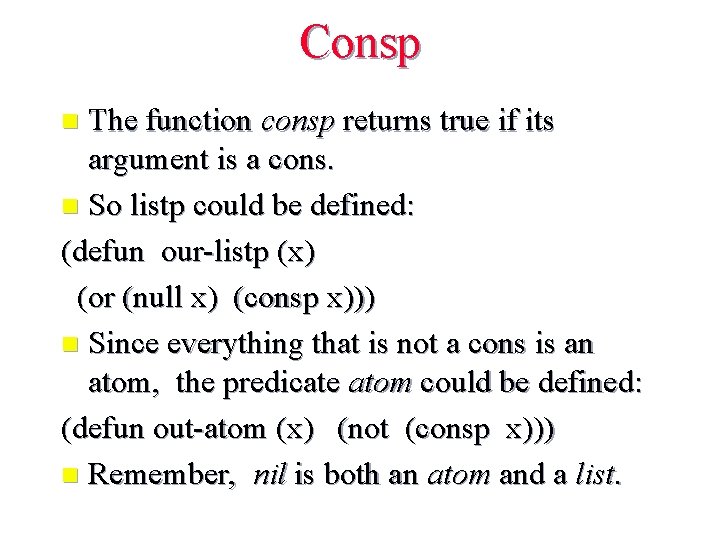
Consp The function consp returns true if its argument is a cons. n So listp could be defined: (defun our-listp (x) (or (null x) (consp x))) n Since everything that is not a cons is an atom, the predicate atom could be defined: (defun out-atom (x) (not (consp x))) n Remember, nil is both an atom and a list. n Mitthögskolan 10/24/2020 8
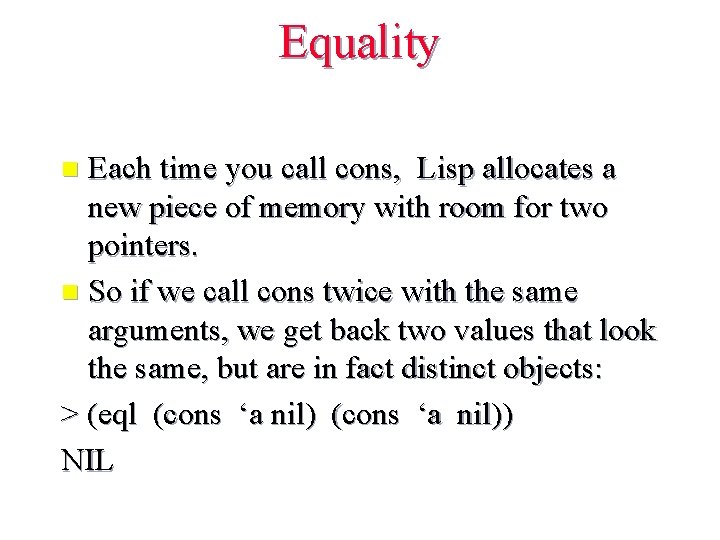
Equality Each time you call cons, Lisp allocates a new piece of memory with room for two pointers. n So if we call cons twice with the same arguments, we get back two values that look the same, but are in fact distinct objects: > (eql (cons ‘a nil)) NIL n Mitthögskolan 10/24/2020 9
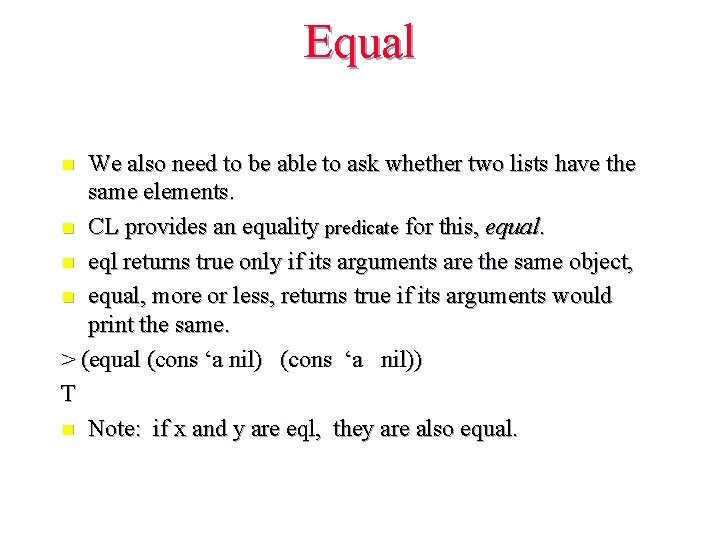
Equal We also need to be able to ask whether two lists have the same elements. n CL provides an equality predicate for this, equal. n eql returns true only if its arguments are the same object, n equal, more or less, returns true if its arguments would print the same. > (equal (cons ‘a nil)) T n Note: if x and y are eql, they are also equal. n Mitthögskolan 10/24/2020 10
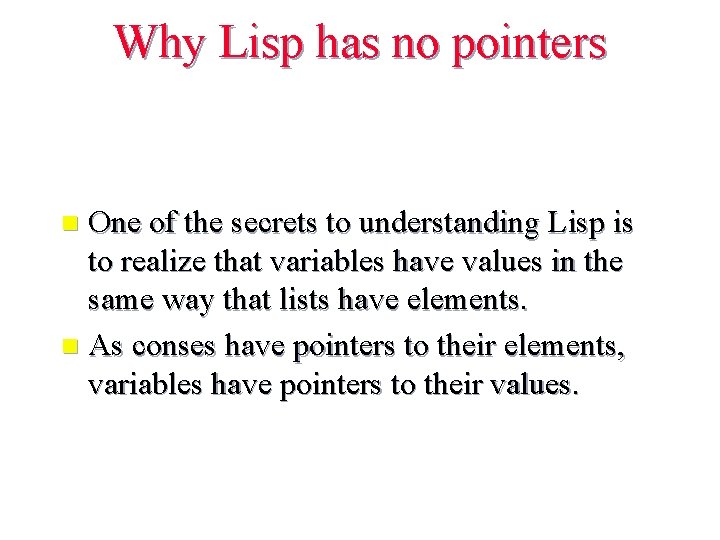
Why Lisp has no pointers One of the secrets to understanding Lisp is to realize that variables have values in the same way that lists have elements. n As conses have pointers to their elements, variables have pointers to their values. n Mitthögskolan 10/24/2020 11
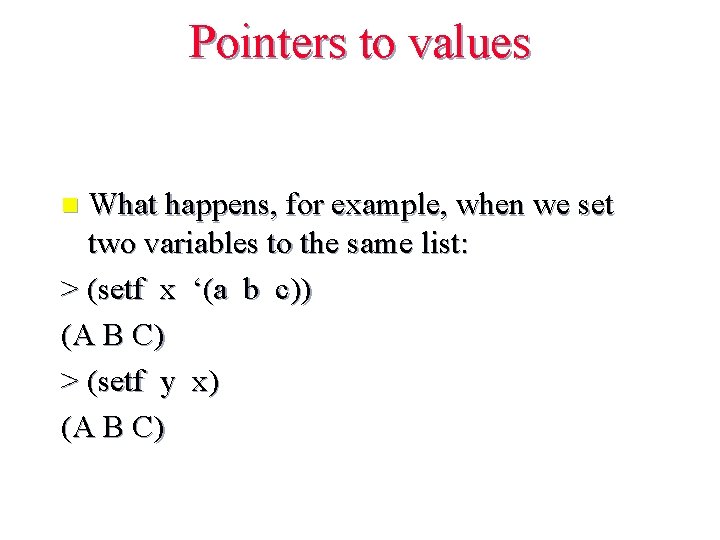
Pointers to values What happens, for example, when we set two variables to the same list: > (setf x ‘(a b c)) (A B C) > (setf y x) (A B C) n Mitthögskolan 10/24/2020 12
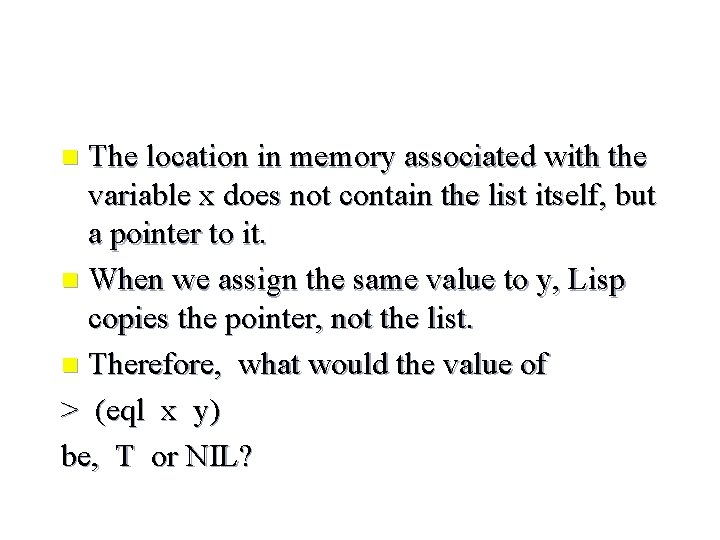
The location in memory associated with the variable x does not contain the list itself, but a pointer to it. n When we assign the same value to y, Lisp copies the pointer, not the list. n Therefore, what would the value of > (eql x y) be, T or NIL? n Mitthögskolan 10/24/2020 13
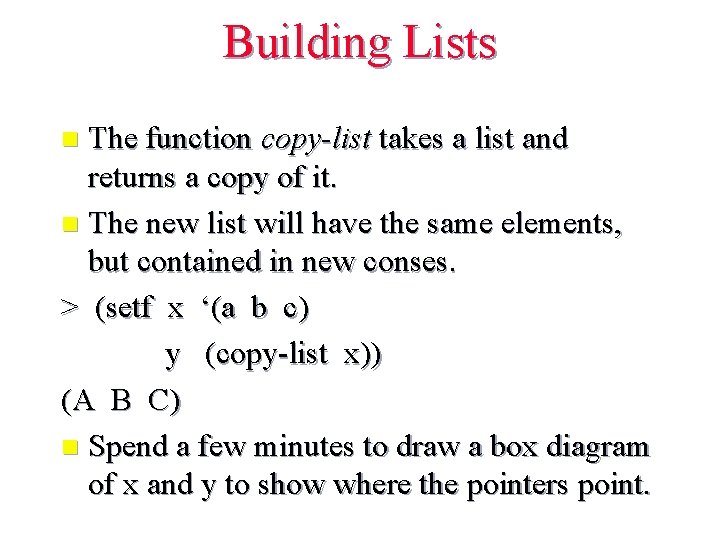
Building Lists The function copy-list takes a list and returns a copy of it. n The new list will have the same elements, but contained in new conses. > (setf x ‘(a b c) y (copy-list x)) (A B C) n Spend a few minutes to draw a box diagram of x and y to show where the pointers point. n Mitthögskolan 10/24/2020 14
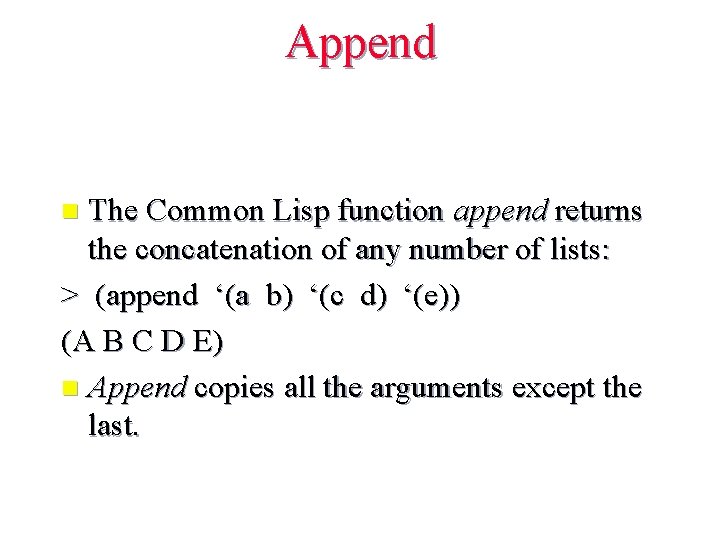
Append The Common Lisp function append returns the concatenation of any number of lists: > (append ‘(a b) ‘(c d) ‘(e)) (A B C D E) n Append copies all the arguments except the last. n Mitthögskolan 10/24/2020 15
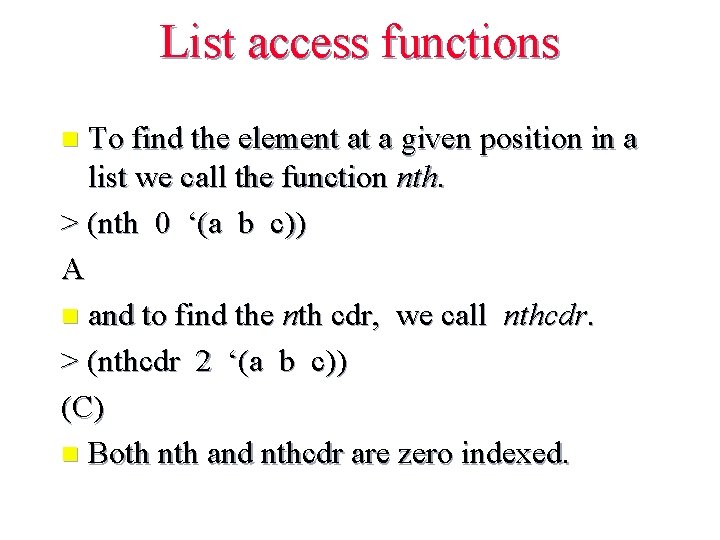
List access functions To find the element at a given position in a list we call the function nth. > (nth 0 ‘(a b c)) A n and to find the nth cdr, we call nthcdr. > (nthcdr 2 ‘(a b c)) (C) n Both nth and nthcdr are zero indexed. n Mitthögskolan 10/24/2020 16
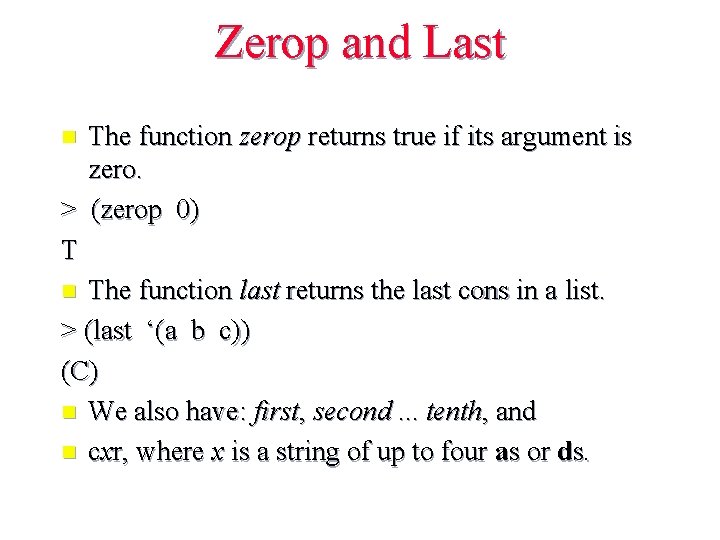
Zerop and Last The function zerop returns true if its argument is zero. > (zerop 0) T n The function last returns the last cons in a list. > (last ‘(a b c)) (C) n We also have: first, second. . . tenth, and n cxr, where x is a string of up to four as or ds. n Mitthögskolan 10/24/2020 17
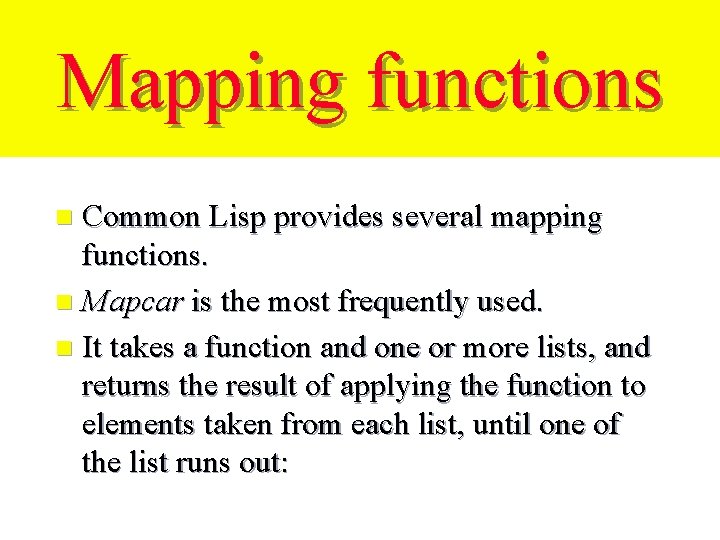
Mapping functions Common Lisp provides several mapping functions. n Mapcar is the most frequently used. n It takes a function and one or more lists, and returns the result of applying the function to elements taken from each list, until one of the list runs out: n Mitthögskolan 10/24/2020 18
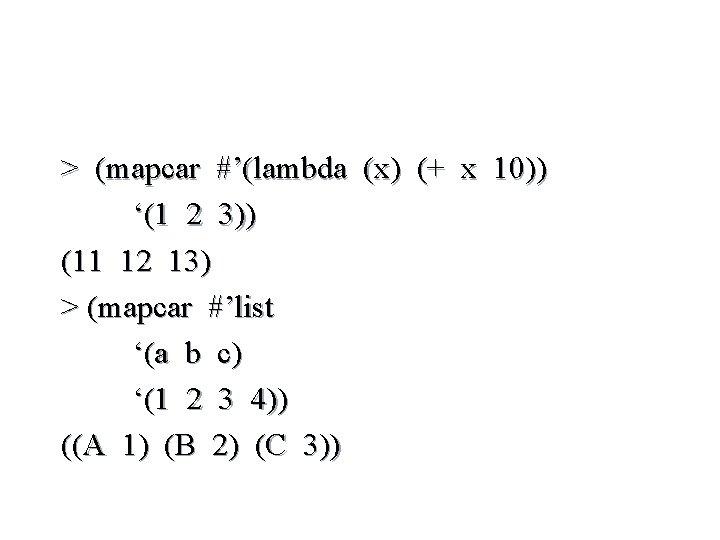
> (mapcar #’(lambda (x) (+ x 10)) ‘(1 2 3)) (11 12 13) > (mapcar #’list ‘(a b c) ‘(1 2 3 4)) ((A 1) (B 2) (C 3)) Mitthögskolan 10/24/2020 19
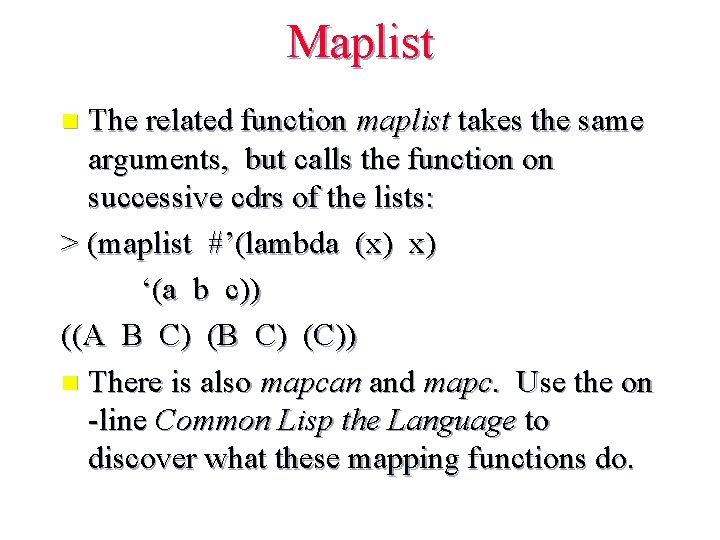
Maplist The related function maplist takes the same arguments, but calls the function on successive cdrs of the lists: > (maplist #’(lambda (x) x) ‘(a b c)) ((A B C) (C)) n There is also mapcan and mapc. Use the on -line Common Lisp the Language to discover what these mapping functions do. n Mitthögskolan 10/24/2020 20
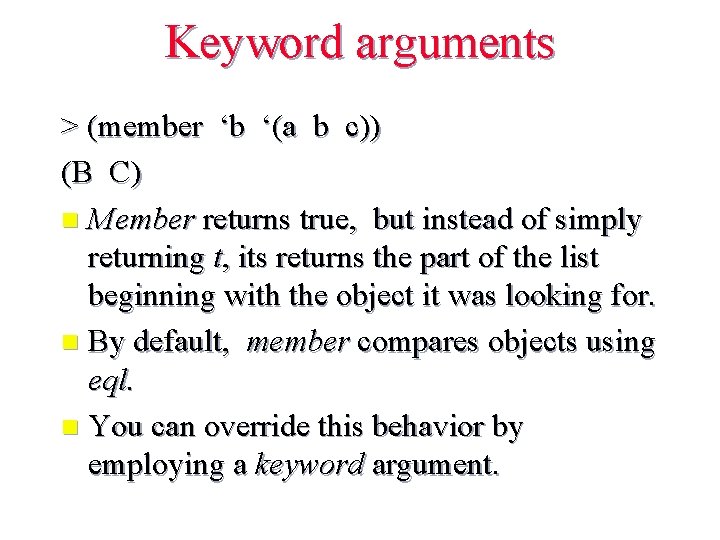
Keyword arguments > (member ‘b ‘(a b c)) (B C) n Member returns true, but instead of simply returning t, its returns the part of the list beginning with the object it was looking for. n By default, member compares objects using eql. n You can override this behavior by employing a keyword argument. Mitthögskolan 10/24/2020 21
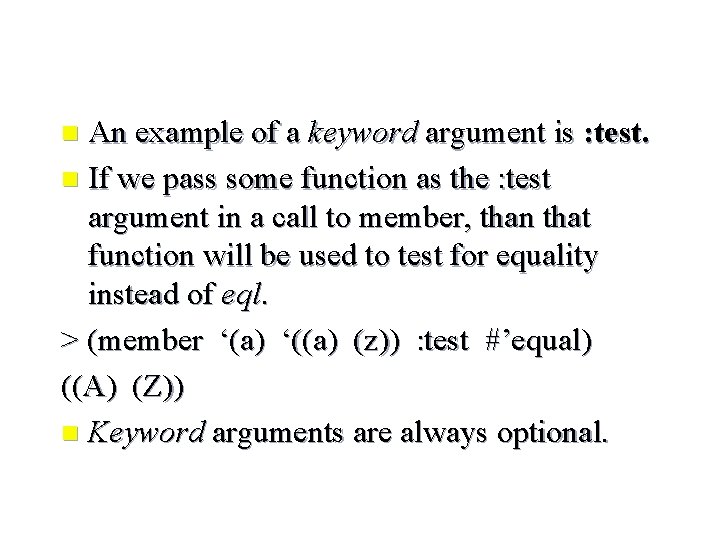
An example of a keyword argument is : test. n If we pass some function as the : test argument in a call to member, than that function will be used to test for equality instead of eql. > (member ‘(a) ‘((a) (z)) : test #’equal) ((A) (Z)) n Keyword arguments are always optional. n Mitthögskolan 10/24/2020 22
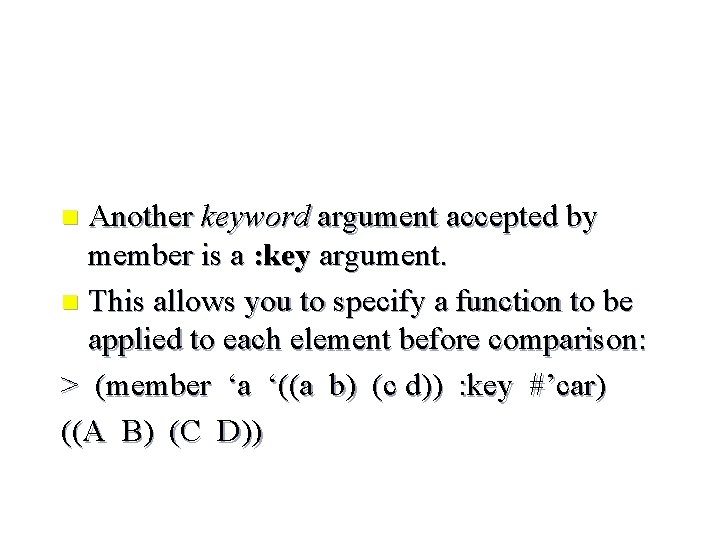
Another keyword argument accepted by member is a : key argument. n This allows you to specify a function to be applied to each element before comparison: > (member ‘a ‘((a b) (c d)) : key #’car) ((A B) (C D)) n Mitthögskolan 10/24/2020 23
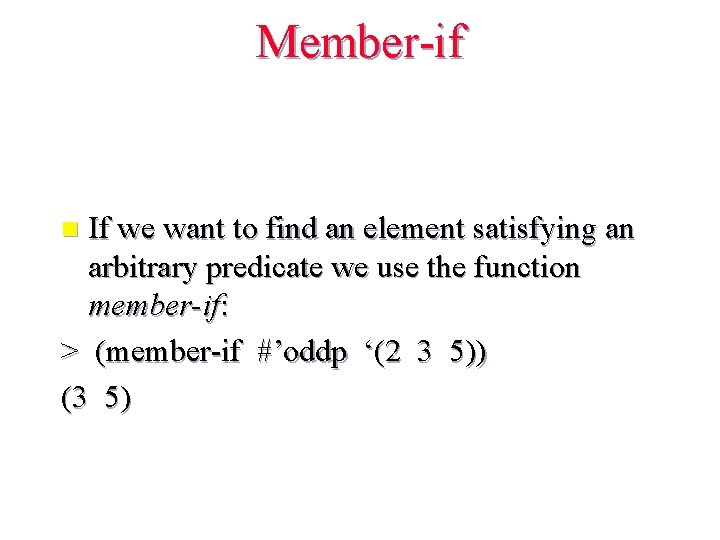
Member-if If we want to find an element satisfying an arbitrary predicate we use the function member-if: > (member-if #’oddp ‘(2 3 5)) (3 5) n Mitthögskolan 10/24/2020 24
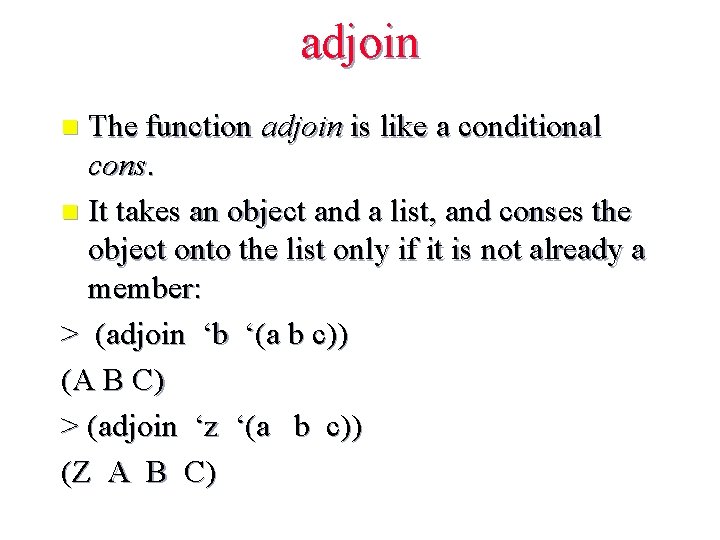
adjoin The function adjoin is like a conditional cons. n It takes an object and a list, and conses the object onto the list only if it is not already a member: > (adjoin ‘b ‘(a b c)) (A B C) > (adjoin ‘z ‘(a b c)) (Z A B C) n Mitthögskolan 10/24/2020 25
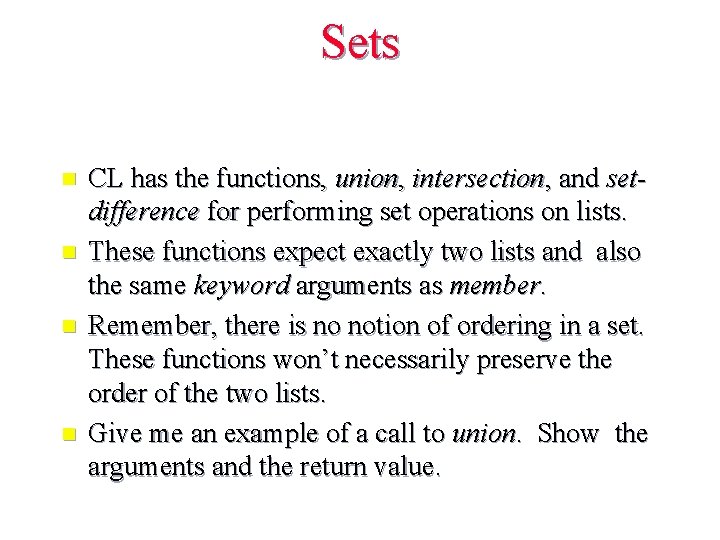
Sets n n CL has the functions, union, intersection, and setdifference for performing set operations on lists. These functions expect exactly two lists and also the same keyword arguments as member. Remember, there is no notion of ordering in a set. These functions won’t necessarily preserve the order of the two lists. Give me an example of a call to union. Show the arguments and the return value. Mitthögskolan 10/24/2020 26
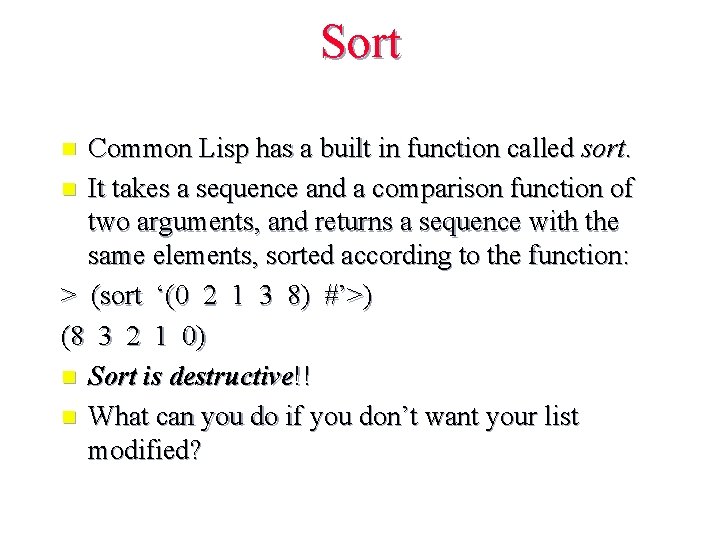
Sort Common Lisp has a built in function called sort. n It takes a sequence and a comparison function of two arguments, and returns a sequence with the same elements, sorted according to the function: > (sort ‘(0 2 1 3 8) #’>) (8 3 2 1 0) n Sort is destructive!! n What can you do if you don’t want your list modified? n Mitthögskolan 10/24/2020 27
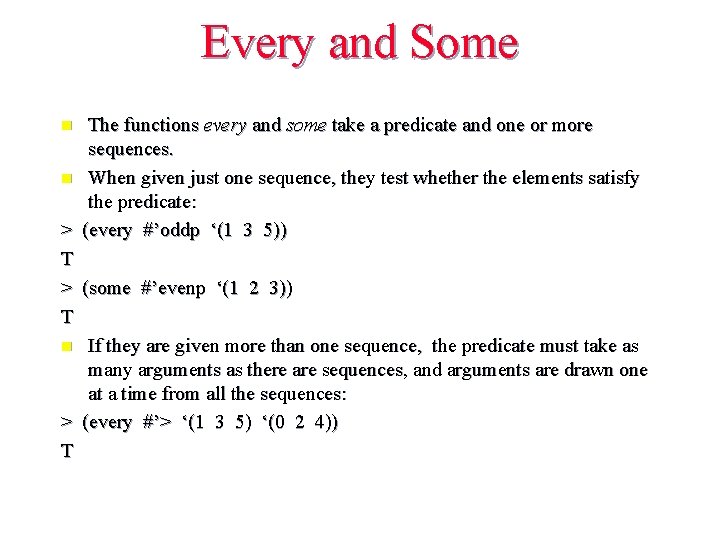
Every and Some The functions every and some take a predicate and one or more sequences. n When given just one sequence, they test whether the elements satisfy the predicate: > (every #’oddp ‘(1 3 5)) T > (some #’evenp ‘(1 2 3)) T n If they are given more than one sequence, the predicate must take as many arguments as there are sequences, and arguments are drawn one at a time from all the sequences: > (every #’> ‘(1 3 5) ‘(0 2 4)) T n Mitthögskolan 10/24/2020 28
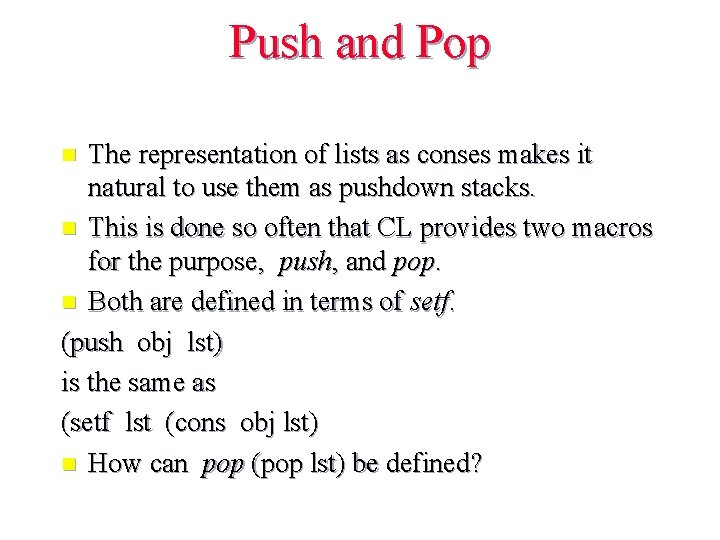
Push and Pop The representation of lists as conses makes it natural to use them as pushdown stacks. n This is done so often that CL provides two macros for the purpose, push, and pop. n Both are defined in terms of setf. (push obj lst) is the same as (setf lst (cons obj lst) n How can pop (pop lst) be defined? n Mitthögskolan 10/24/2020 29
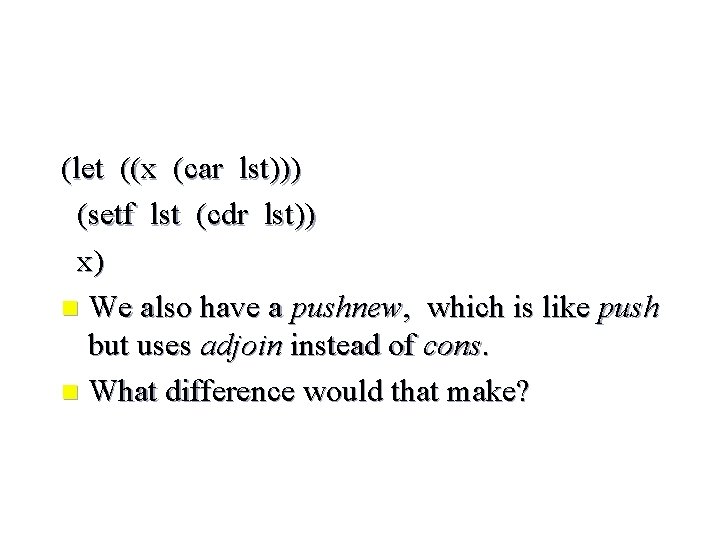
(let ((x (car lst))) (setf lst (cdr lst)) x) n We also have a pushnew, which is like push but uses adjoin instead of cons. n What difference would that make? Mitthögskolan 10/24/2020 30
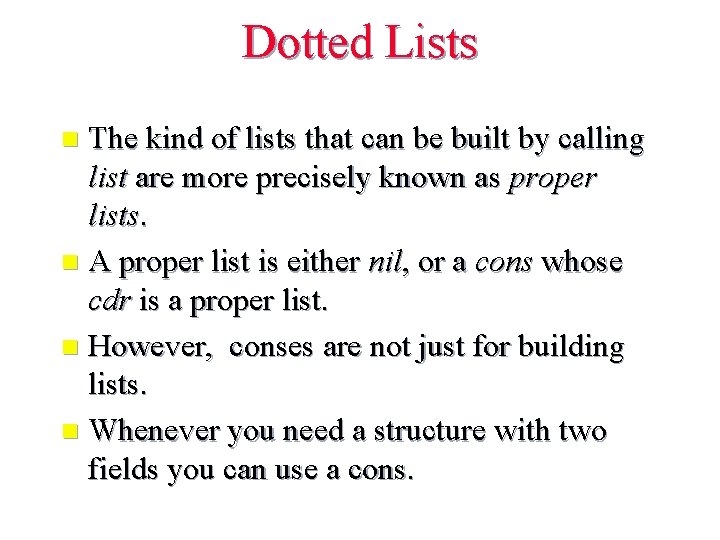
Dotted Lists The kind of lists that can be built by calling list are more precisely known as proper lists. n A proper list is either nil, or a cons whose cdr is a proper list. n However, conses are not just for building lists. n Whenever you need a structure with two fields you can use a cons. n Mitthögskolan 10/24/2020 31
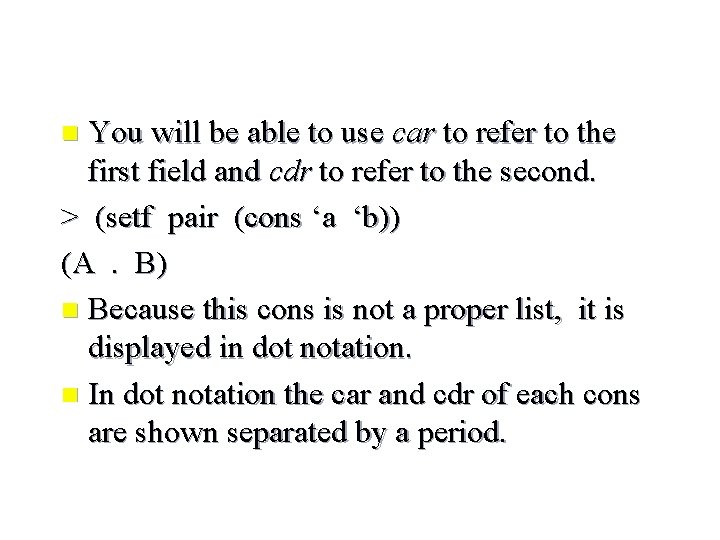
You will be able to use car to refer to the first field and cdr to refer to the second. > (setf pair (cons ‘a ‘b)) (A. B) n Because this cons is not a proper list, it is displayed in dot notation. n In dot notation the car and cdr of each cons are shown separated by a period. n Mitthögskolan 10/24/2020 32
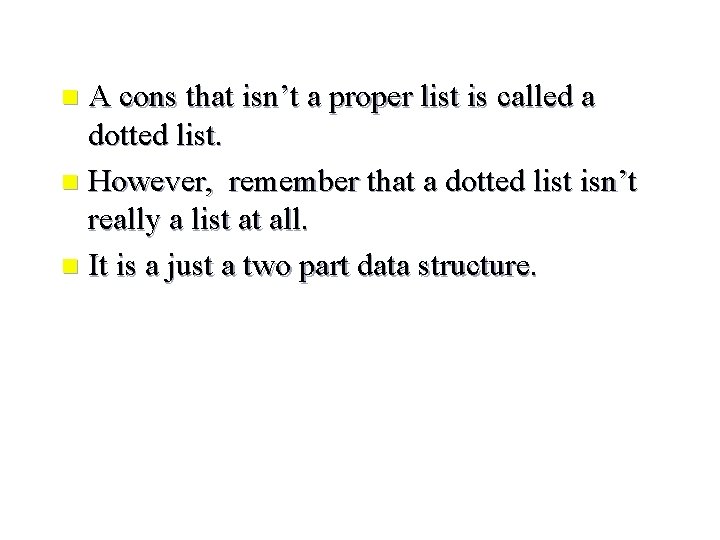
A cons that isn’t a proper list is called a dotted list. n However, remember that a dotted list isn’t really a list at all. n It is a just a two part data structure. n a b (A. B) Mitthögskolan 10/24/2020 33
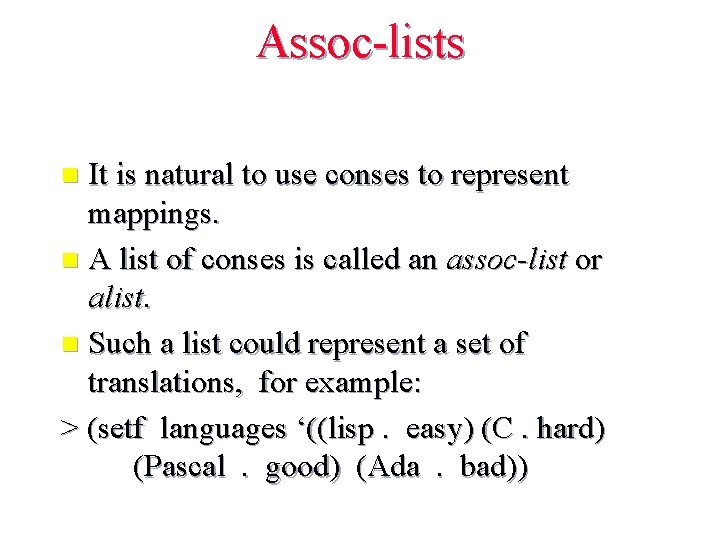
Assoc-lists It is natural to use conses to represent mappings. n A list of conses is called an assoc-list or alist. n Such a list could represent a set of translations, for example: > (setf languages ‘((lisp. easy) (C. hard) (Pascal. good) (Ada. bad)) n Mitthögskolan 10/24/2020 34
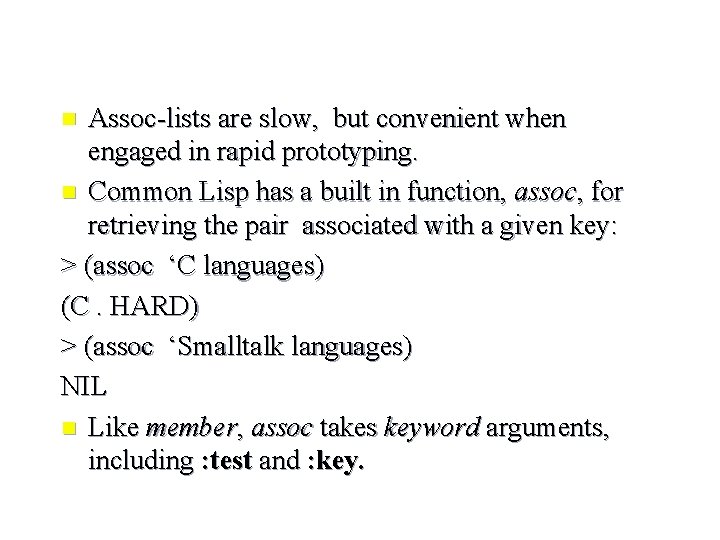
Assoc-lists are slow, but convenient when engaged in rapid prototyping. n Common Lisp has a built in function, assoc, for retrieving the pair associated with a given key: > (assoc ‘C languages) (C. HARD) > (assoc ‘Smalltalk languages) NIL n Like member, assoc takes keyword arguments, including : test and : key. n Mitthögskolan 10/24/2020 35
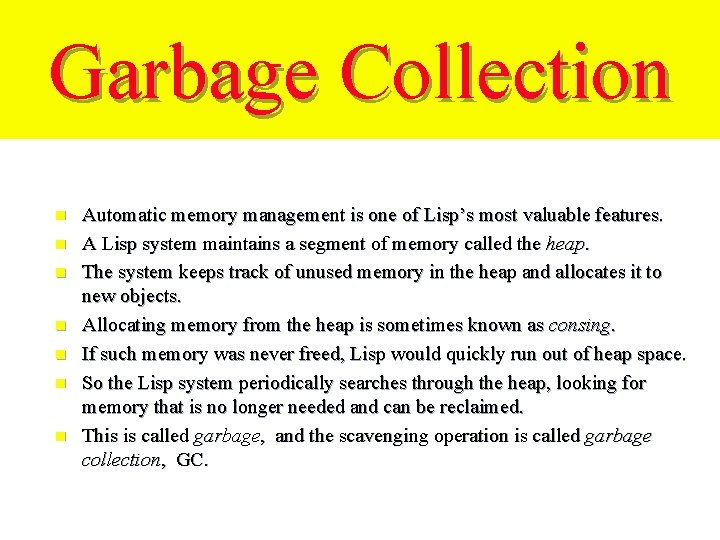
Garbage Collection n n n Automatic memory management is one of Lisp’s most valuable features. A Lisp system maintains a segment of memory called the heap. The system keeps track of unused memory in the heap and allocates it to new objects. Allocating memory from the heap is sometimes known as consing. If such memory was never freed, Lisp would quickly run out of heap space. So the Lisp system periodically searches through the heap, looking for memory that is no longer needed and can be reclaimed. This is called garbage, and the scavenging operation is called garbage collection, GC. Mitthögskolan 10/24/2020 36
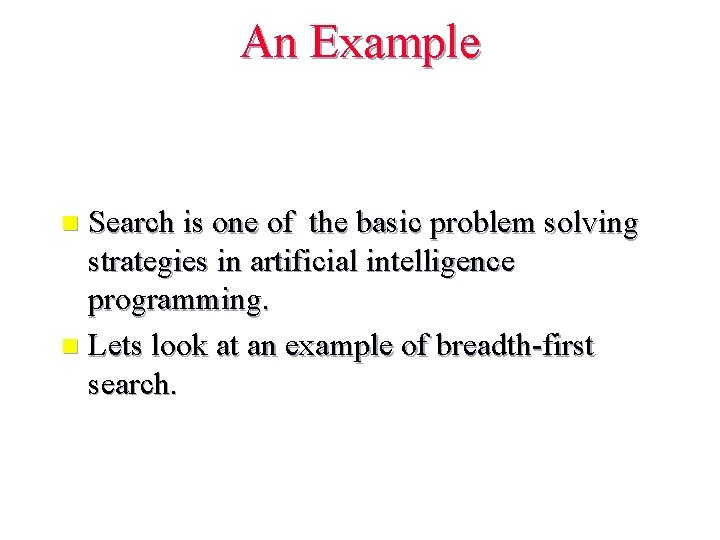
An Example Search is one of the basic problem solving strategies in artificial intelligence programming. n Lets look at an example of breadth-first search. n Mitthögskolan 10/24/2020 37
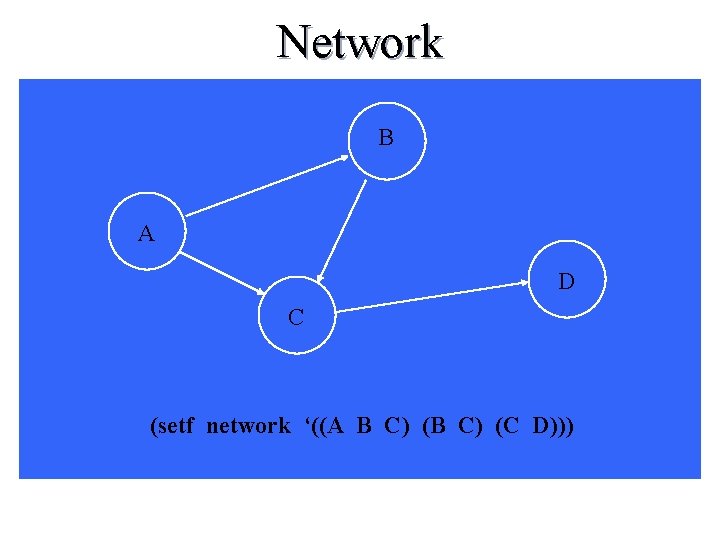
Network B A D C (setf network ‘((A B C) (C D))) Mitthögskolan 10/24/2020 38
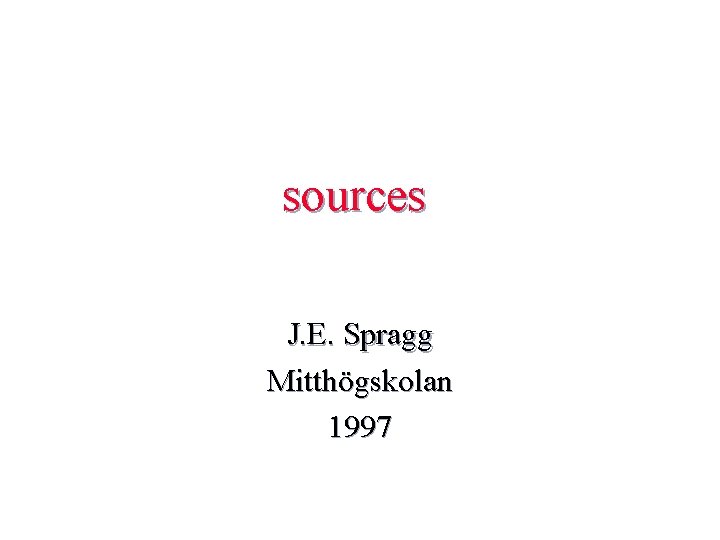
sources J. E. Spragg Mitthögskolan 1997 Mitthögskolan 10/24/2020 39
Antigentest åre
Lisp lists
Allegro common lisp
Lambda autolisp
Common lisp append
Lisp primitives
Common lisp a gentle introduction to symbolic computation
Common lisp assoc
Shen lisp
Lisp list processing
Lisp program to find factorial of a number
Lisp conditionals
Lisp functional programming
Cons in lisp
Basic cobol fortran
Cons in lisp
Lisp cisco
Lisp examples
Lisp lenguaje de programacion
Xkcd lisp
Butlast lisp
Lisp and prolog
Is lisp a functional programming language
Lisp and prolog
Positive list scheme
Lisp map reduce
Lisp stands for
Lisp unless
Stream lazy evaluation
Xkcd lisp
Lisp polish notation
Mapreduce: simplified data processing on large clusters
Introduction to lisp
Cons in lisp
Lisp functional programming
Lisp atom
Unless lisp
Racket lisp
Lisp vs clojure
Talude