Writing LISP functions COND Rule 1 Unless the
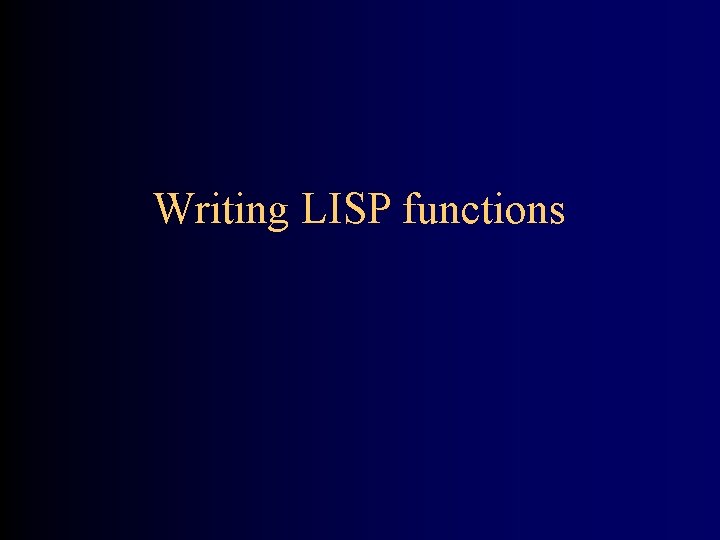
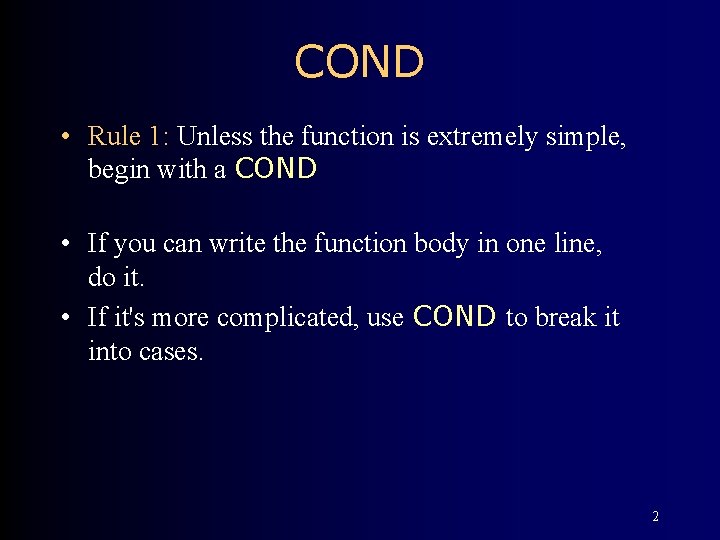
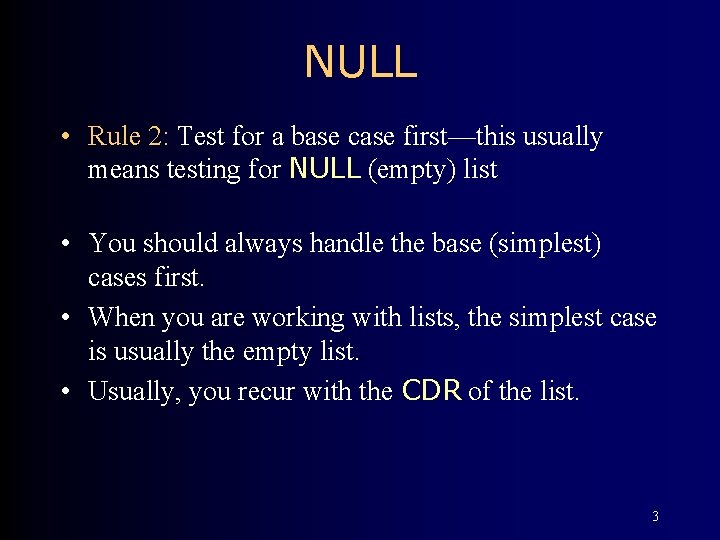
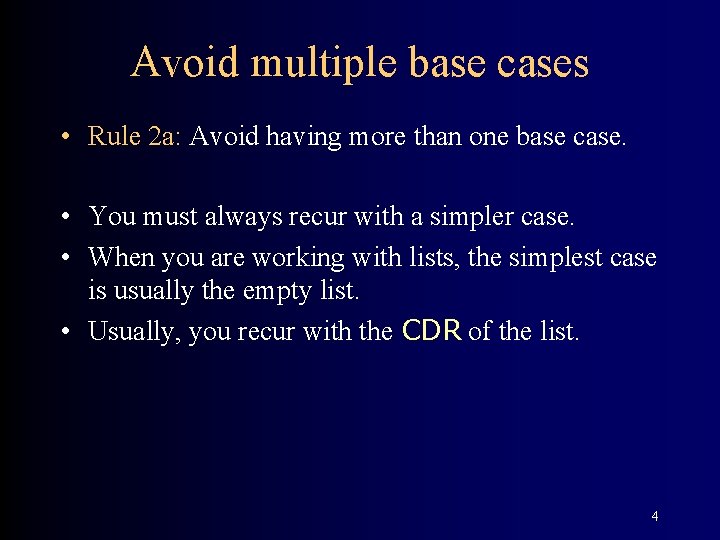
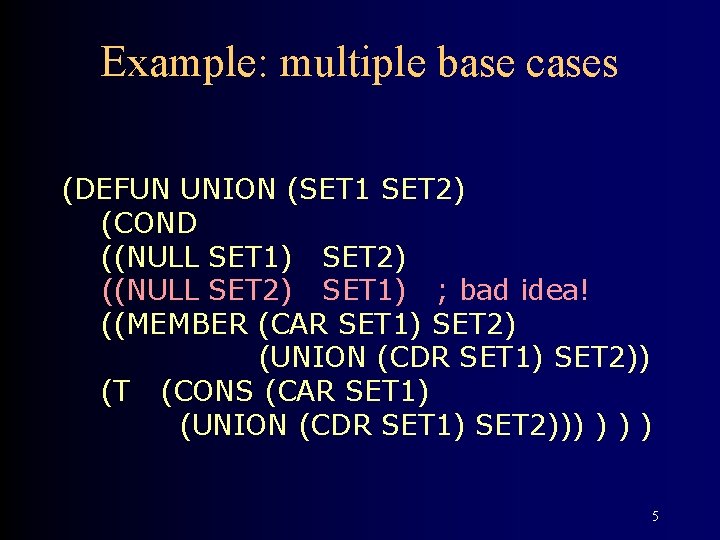
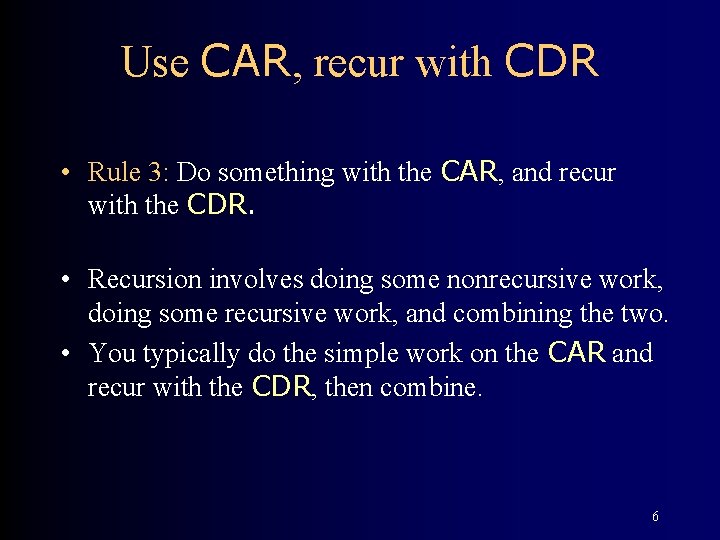
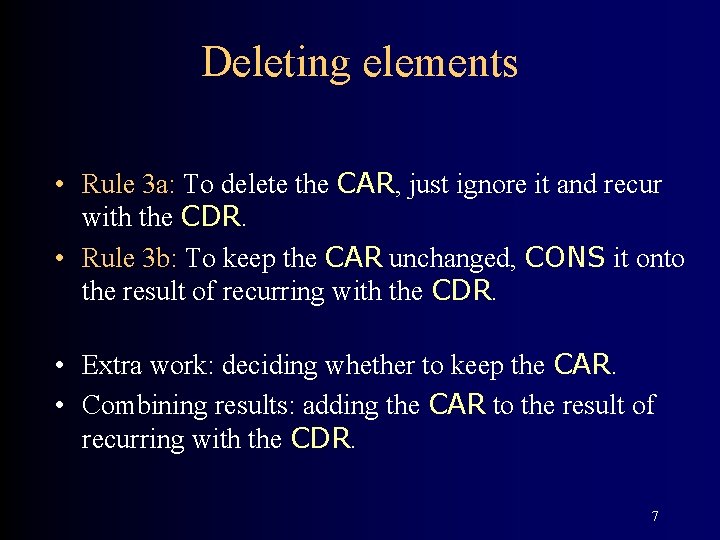
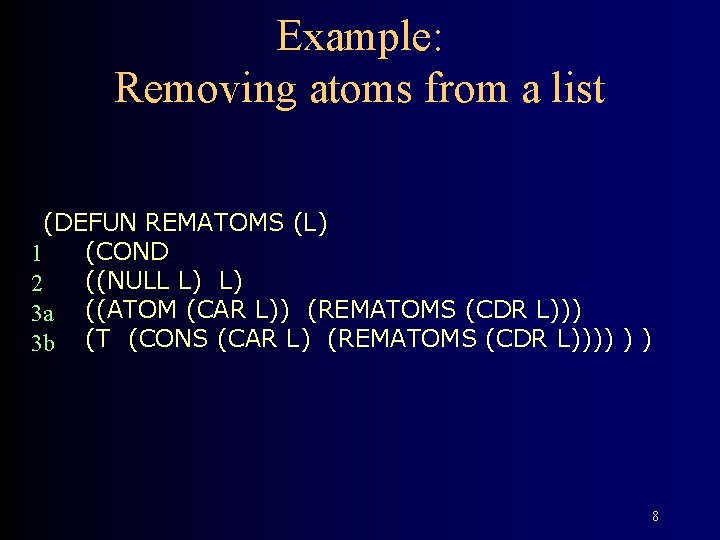
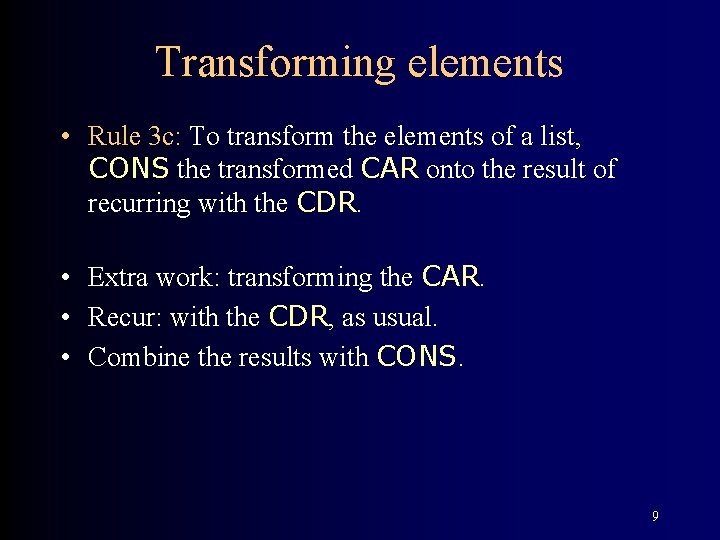
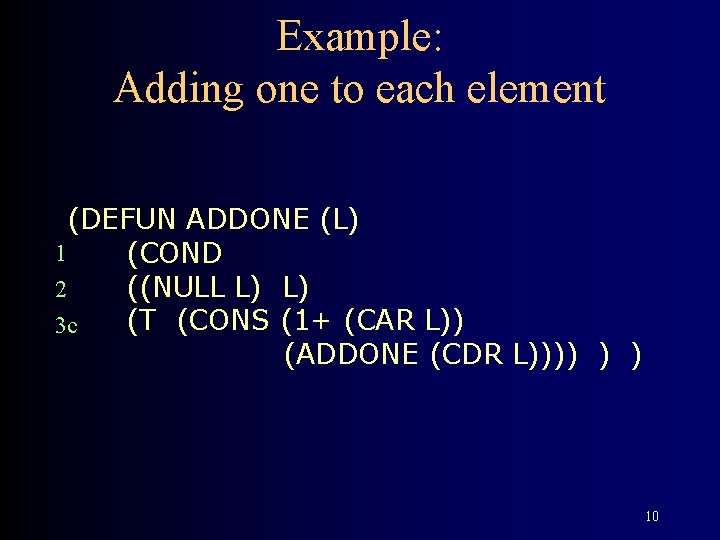
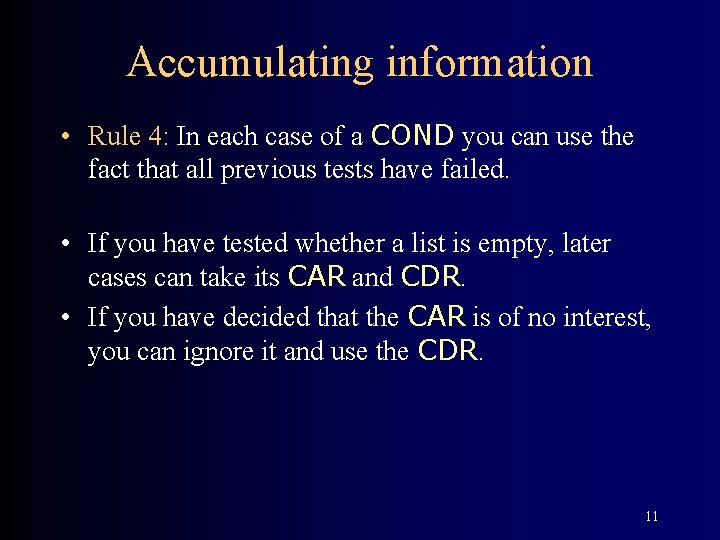
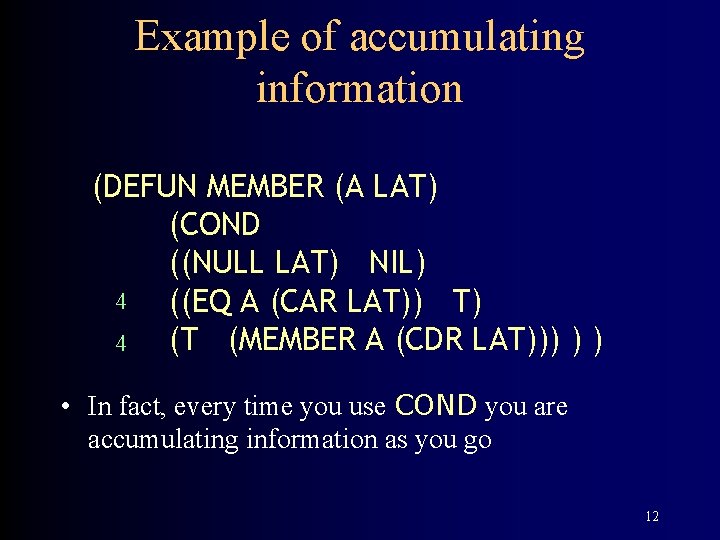
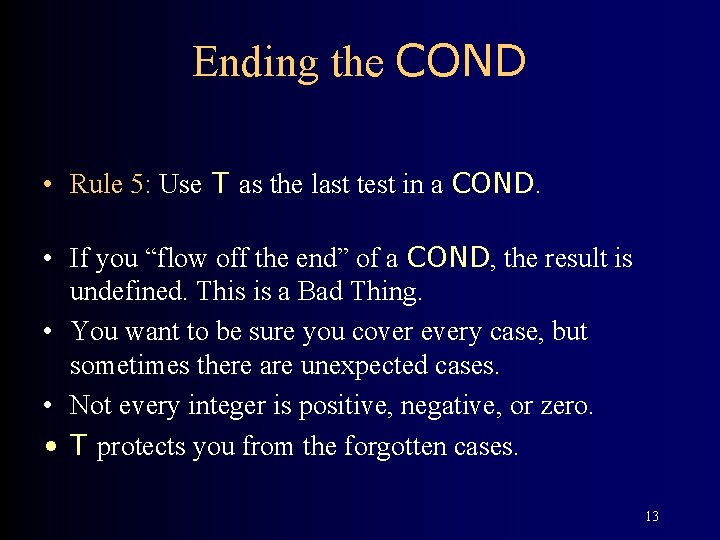
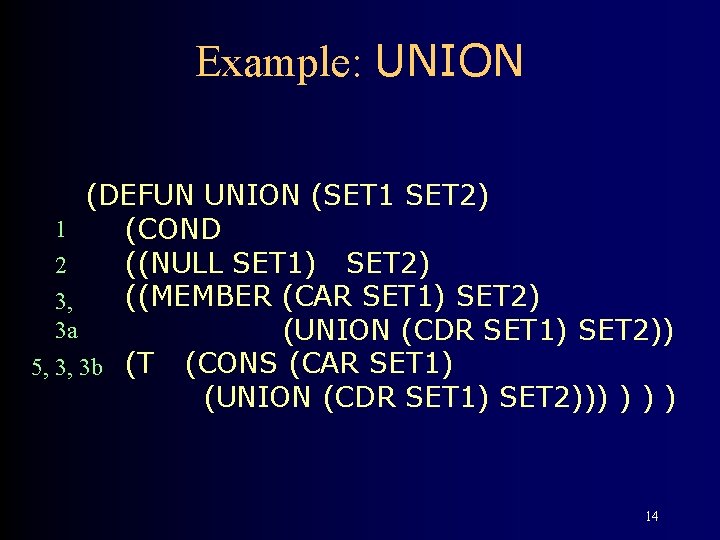
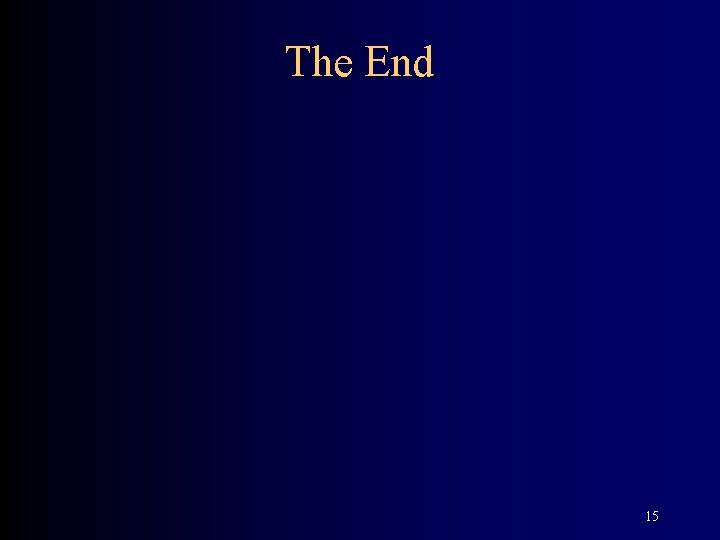
- Slides: 15
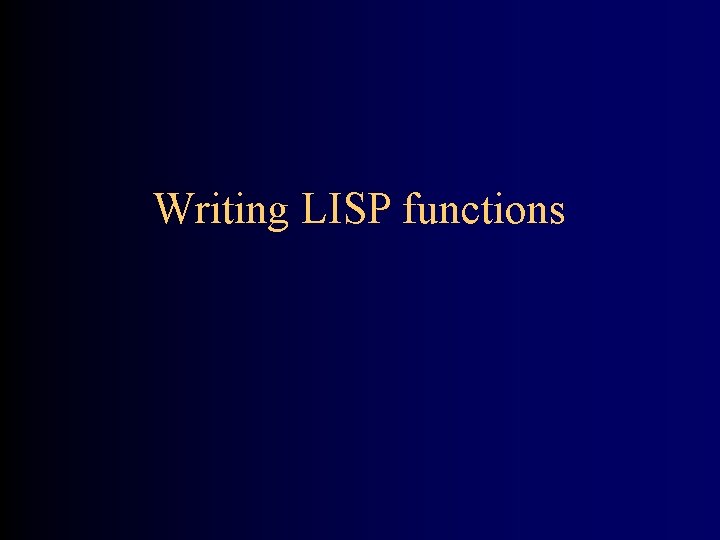
Writing LISP functions
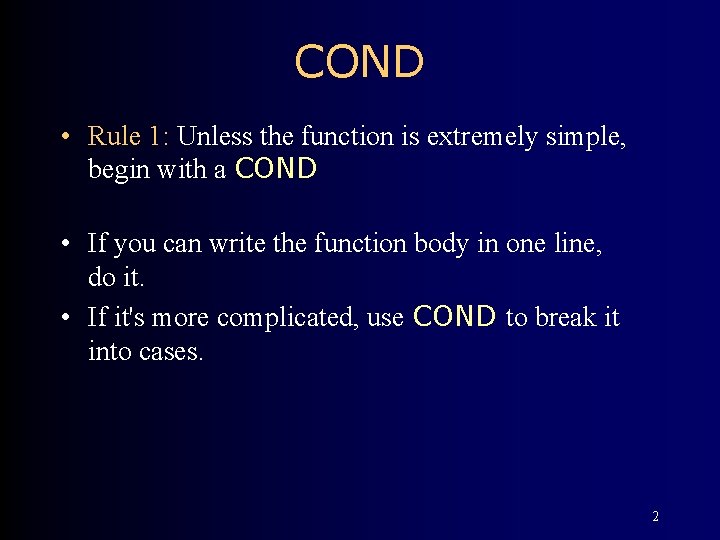
COND • Rule 1: Unless the function is extremely simple, begin with a COND • If you can write the function body in one line, do it. • If it's more complicated, use COND to break it into cases. 2
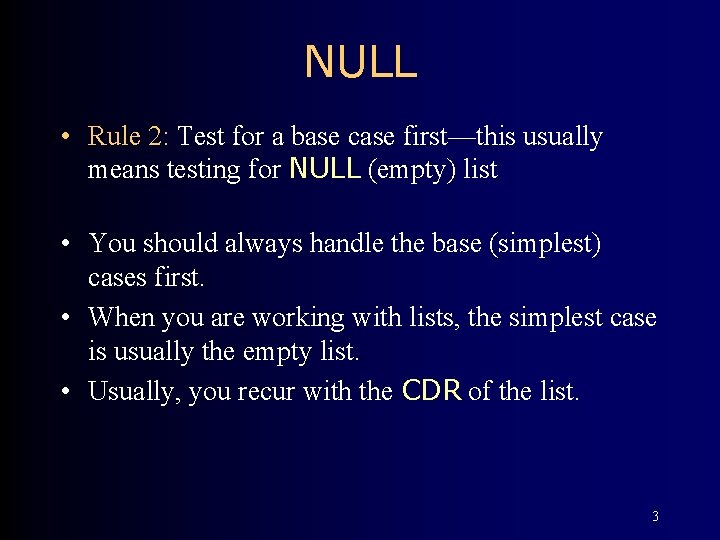
NULL • Rule 2: Test for a base case first—this usually means testing for NULL (empty) list • You should always handle the base (simplest) cases first. • When you are working with lists, the simplest case is usually the empty list. • Usually, you recur with the CDR of the list. 3
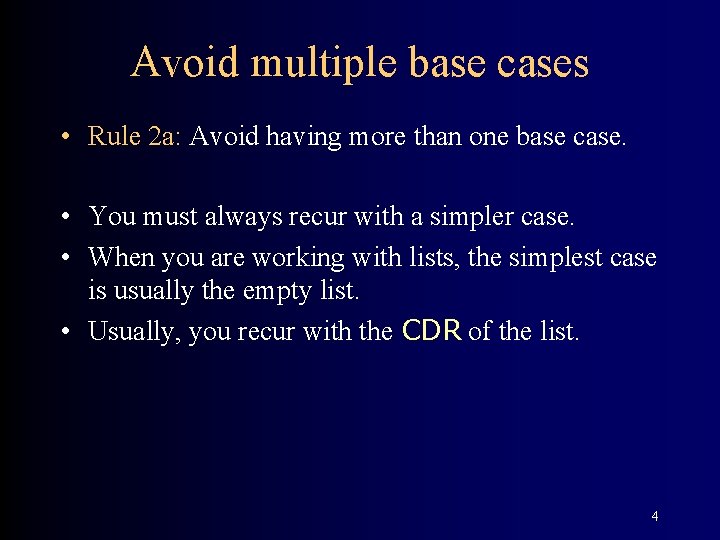
Avoid multiple base cases • Rule 2 a: Avoid having more than one base case. • You must always recur with a simpler case. • When you are working with lists, the simplest case is usually the empty list. • Usually, you recur with the CDR of the list. 4
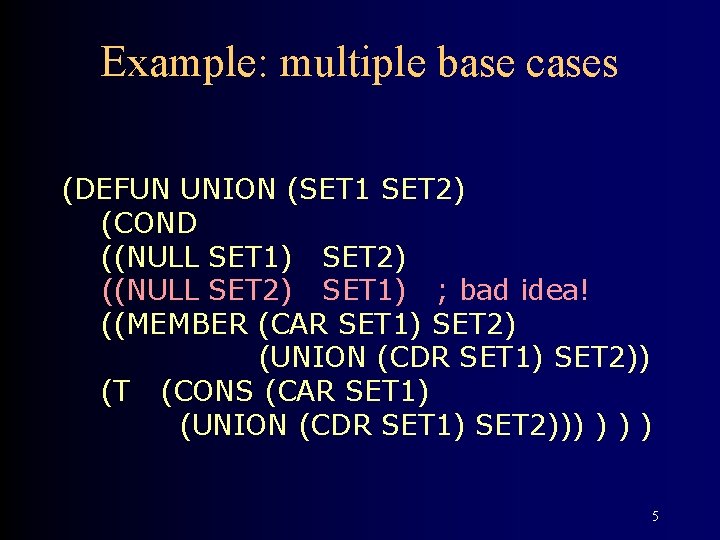
Example: multiple base cases (DEFUN UNION (SET 1 SET 2) (COND ((NULL SET 1) SET 2) ((NULL SET 2) SET 1) ; bad idea! ((MEMBER (CAR SET 1) SET 2) (UNION (CDR SET 1) SET 2)) (T (CONS (CAR SET 1) (UNION (CDR SET 1) SET 2))) ) 5
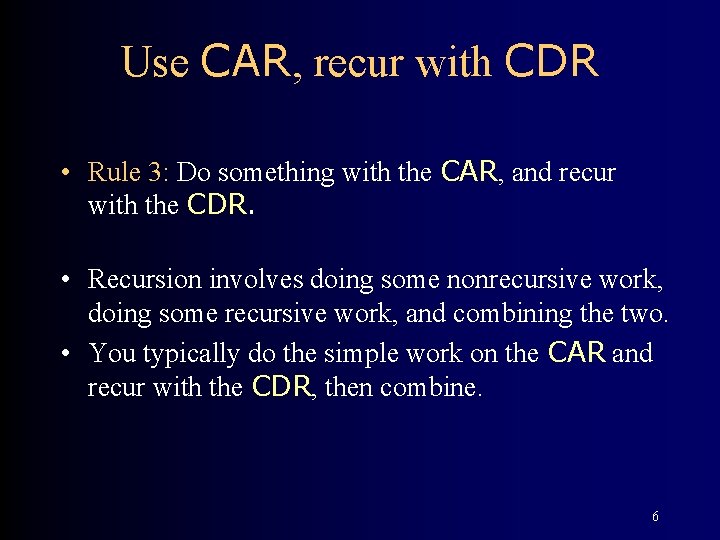
Use CAR, recur with CDR • Rule 3: Do something with the CAR, and recur with the CDR. • Recursion involves doing some nonrecursive work, doing some recursive work, and combining the two. • You typically do the simple work on the CAR and recur with the CDR, then combine. 6
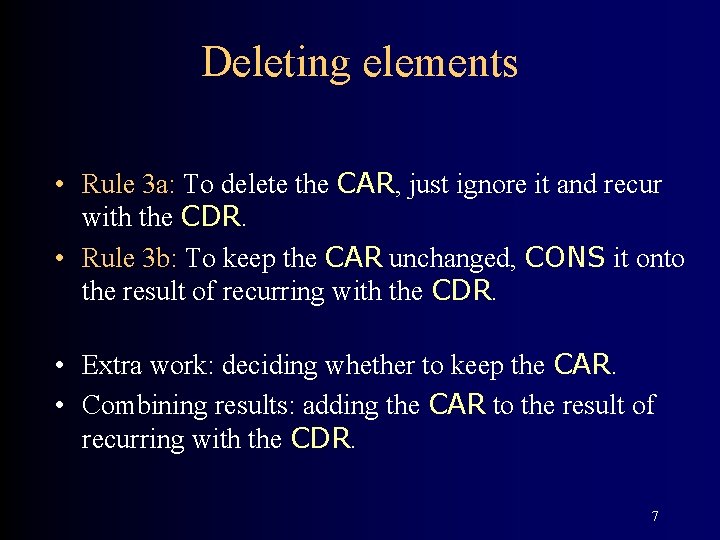
Deleting elements • Rule 3 a: To delete the CAR, just ignore it and recur with the CDR. • Rule 3 b: To keep the CAR unchanged, CONS it onto the result of recurring with the CDR. • Extra work: deciding whether to keep the CAR. • Combining results: adding the CAR to the result of recurring with the CDR. 7
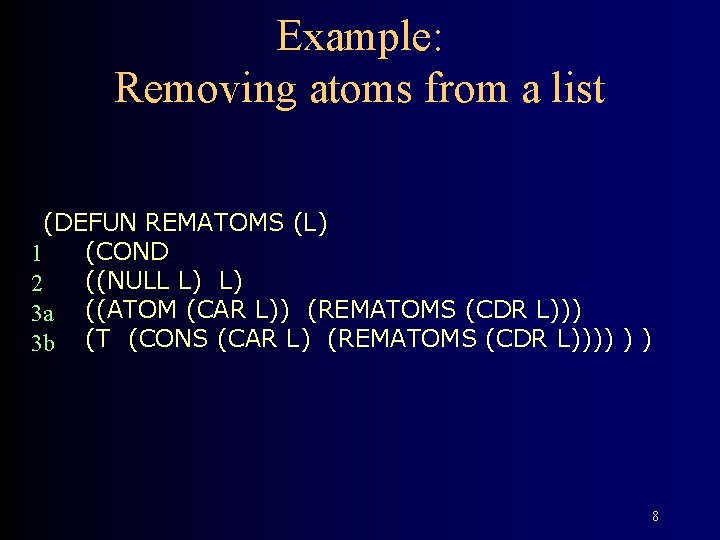
Example: Removing atoms from a list (DEFUN REMATOMS (L) (COND 1 ((NULL L) L) 2 3 a ((ATOM (CAR L)) (REMATOMS (CDR L))) 3 b (T (CONS (CAR L) (REMATOMS (CDR L)))) ) ) 8
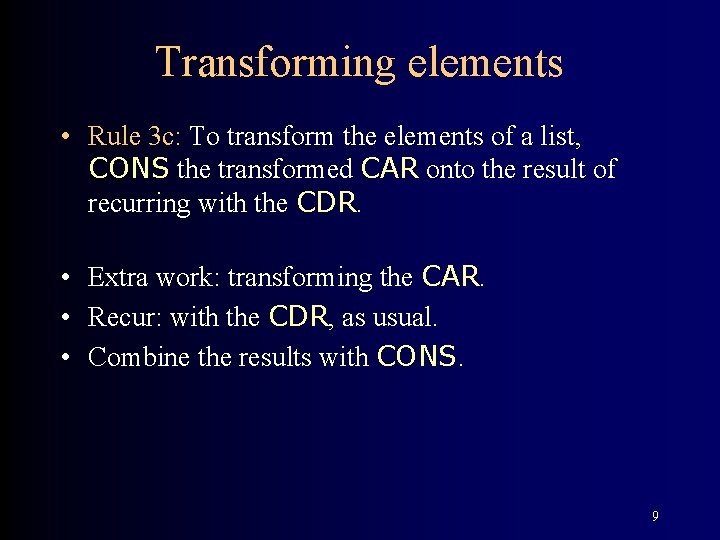
Transforming elements • Rule 3 c: To transform the elements of a list, CONS the transformed CAR onto the result of recurring with the CDR. • Extra work: transforming the CAR. • Recur: with the CDR, as usual. • Combine the results with CONS. 9
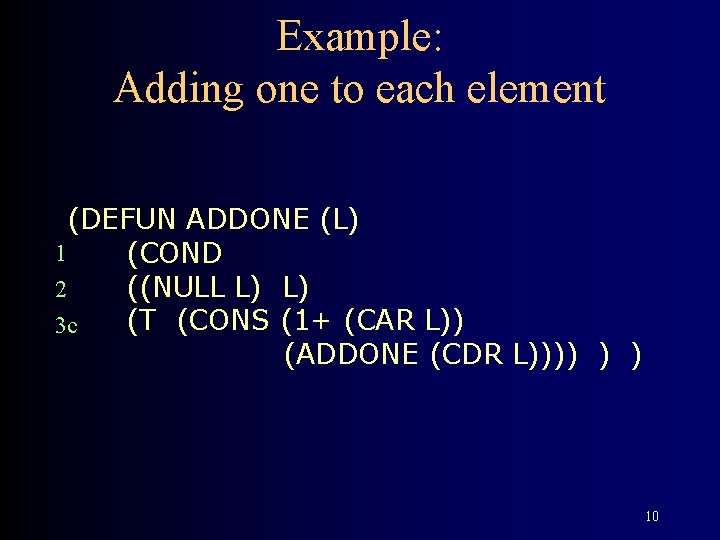
Example: Adding one to each element (DEFUN ADDONE (L) 1 (COND ((NULL L) L) 2 (T (CONS (1+ (CAR L)) 3 c (ADDONE (CDR L)))) ) ) 10
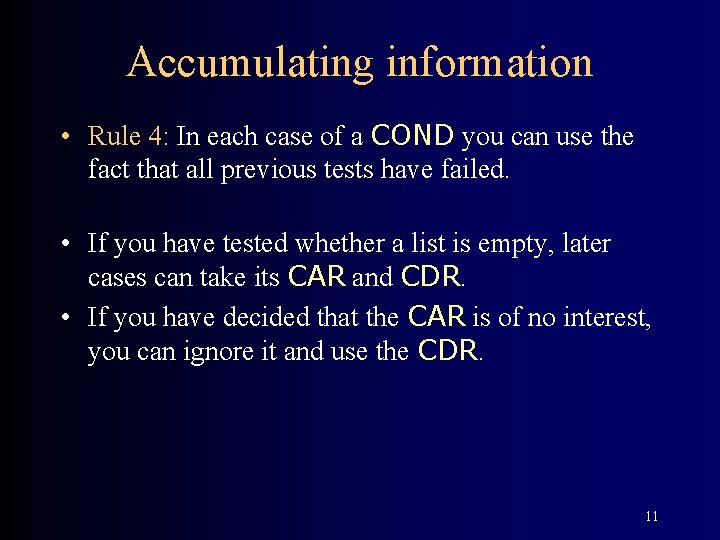
Accumulating information • Rule 4: In each case of a COND you can use the fact that all previous tests have failed. • If you have tested whether a list is empty, later cases can take its CAR and CDR. • If you have decided that the CAR is of no interest, you can ignore it and use the CDR. 11
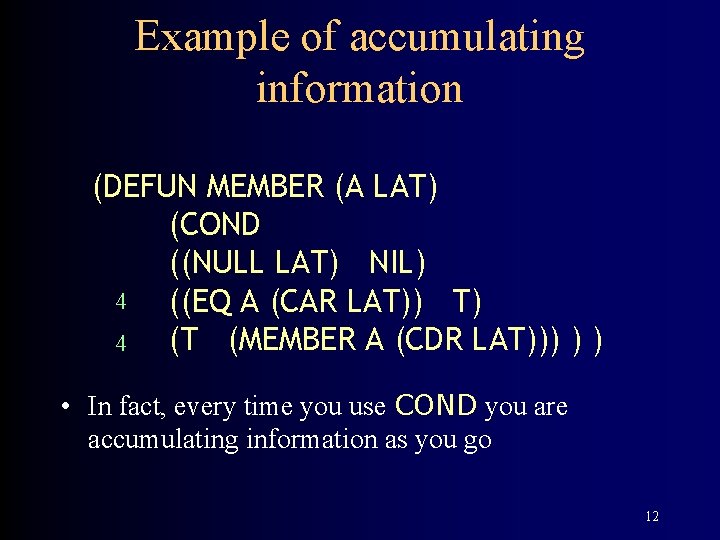
Example of accumulating information (DEFUN MEMBER (A LAT) (COND ((NULL LAT) NIL) 4 ((EQ A (CAR LAT)) T) (T (MEMBER A (CDR LAT))) ) ) 4 • In fact, every time you use COND you are accumulating information as you go 12
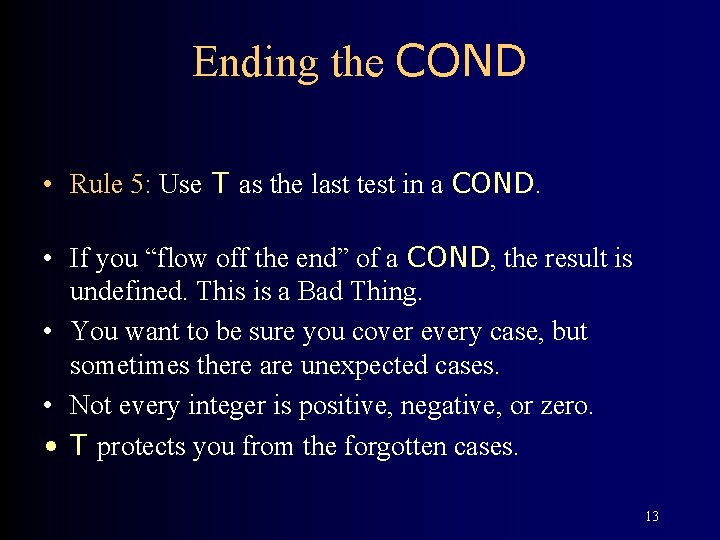
Ending the COND • Rule 5: Use T as the last test in a COND. • If you “flow off the end” of a COND, the result is undefined. This is a Bad Thing. • You want to be sure you cover every case, but sometimes there are unexpected cases. • Not every integer is positive, negative, or zero. • T protects you from the forgotten cases. 13
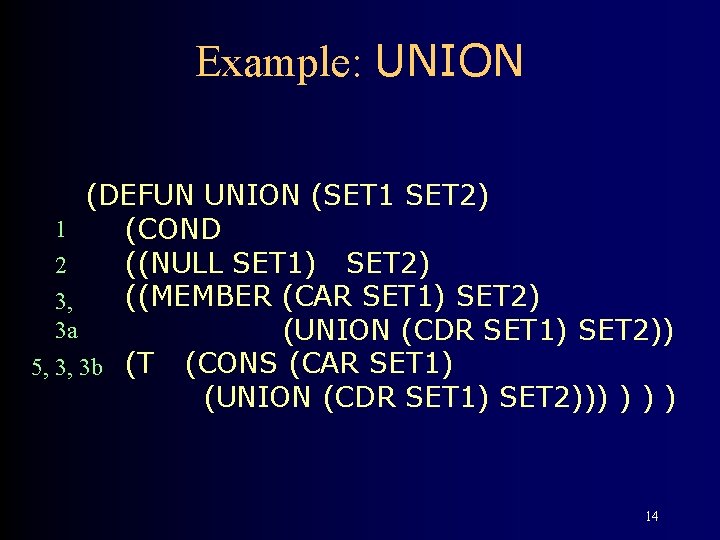
Example: UNION (DEFUN UNION (SET 1 SET 2) 1 (COND ((NULL SET 1) SET 2) 2 ((MEMBER (CAR SET 1) SET 2) 3, 3 a (UNION (CDR SET 1) SET 2)) 5, 3, 3 b (T (CONS (CAR SET 1) (UNION (CDR SET 1) SET 2))) ) 14
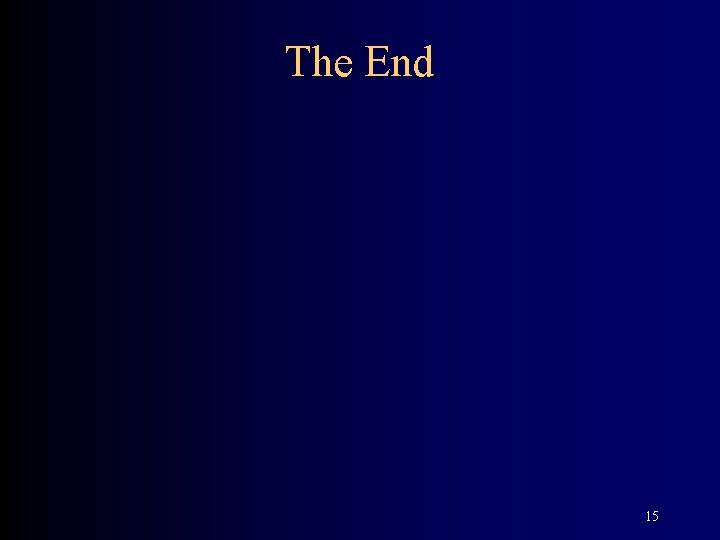
The End 15
Lisp cond
Lisp cond example
Lisp conditionals
Bacaan gas aircond r410a
The weather has been nice but it may snow again any day
The trust giant's point of view analysis
The part can never be well unless the whole is well meaning
Unless you repent you will all likewise perish
Unless noted otherwise
Unless
. . . . they do their homework last night?
Unless otherwise noted meaning
A unless b
Poetic devices of an elementary school classroom in a slum
Unless otherwise agreed
Unless what