UserDefined Functions contd Reference Parameters CSCE 106 Outline
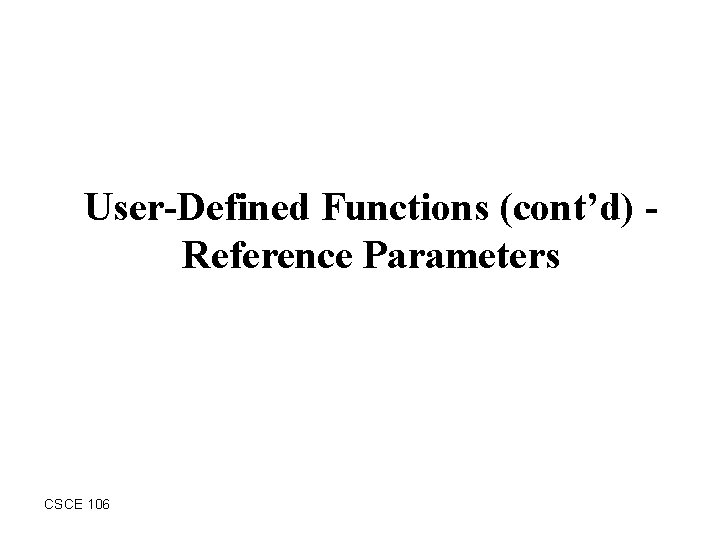
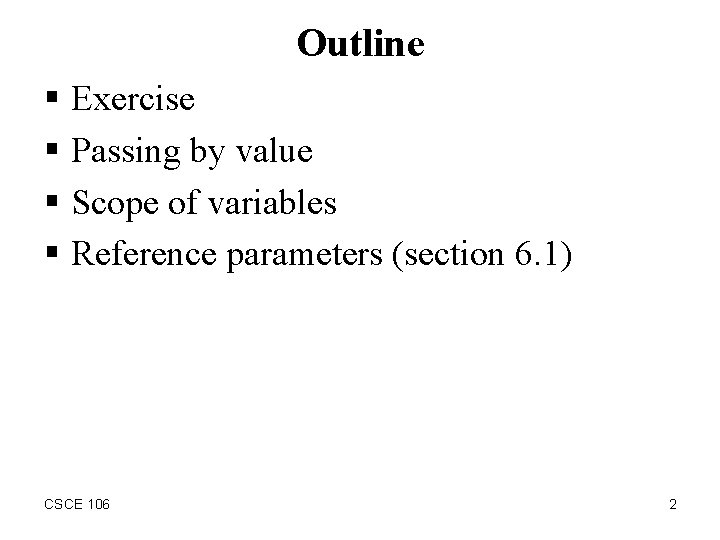
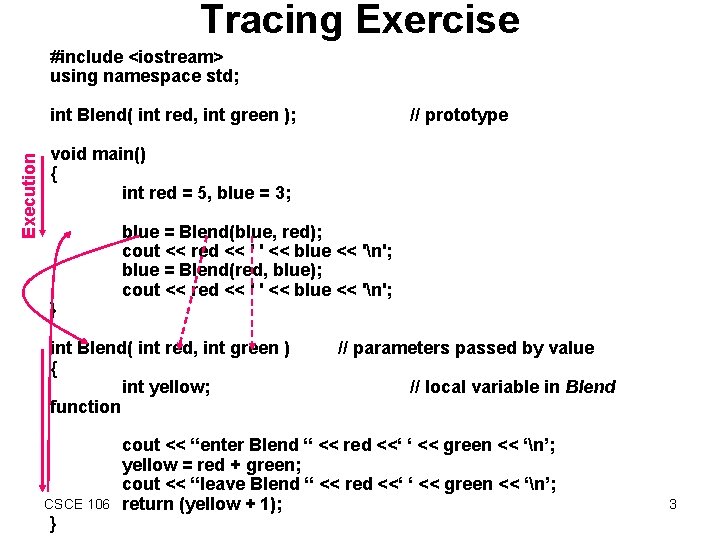
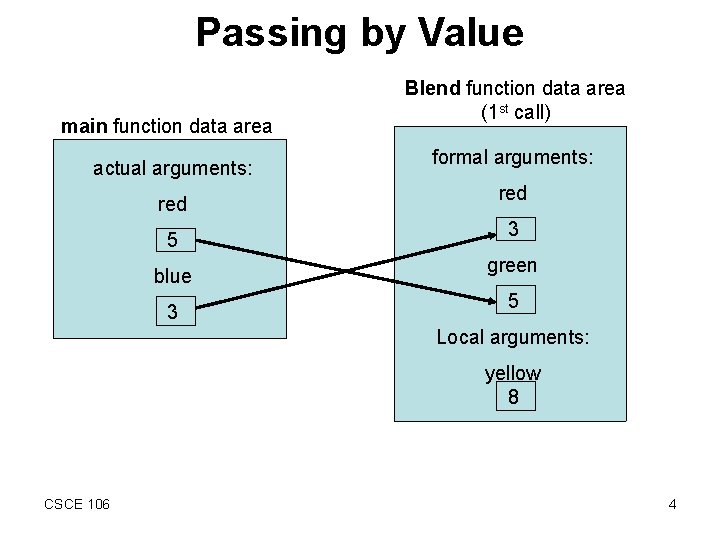
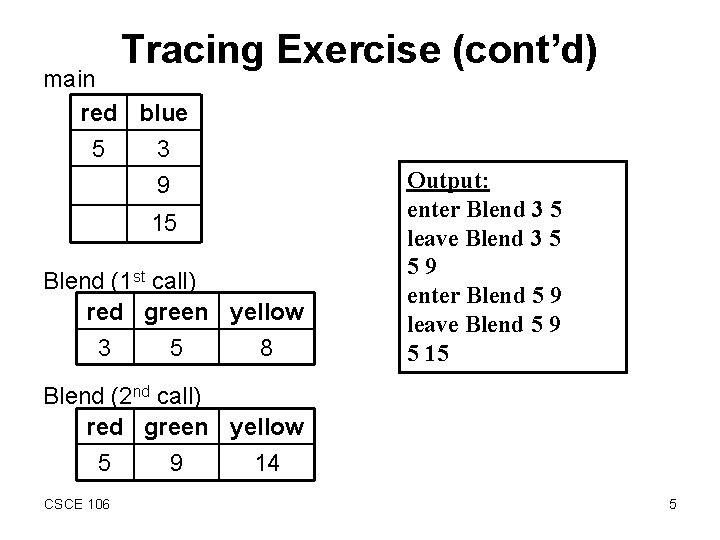
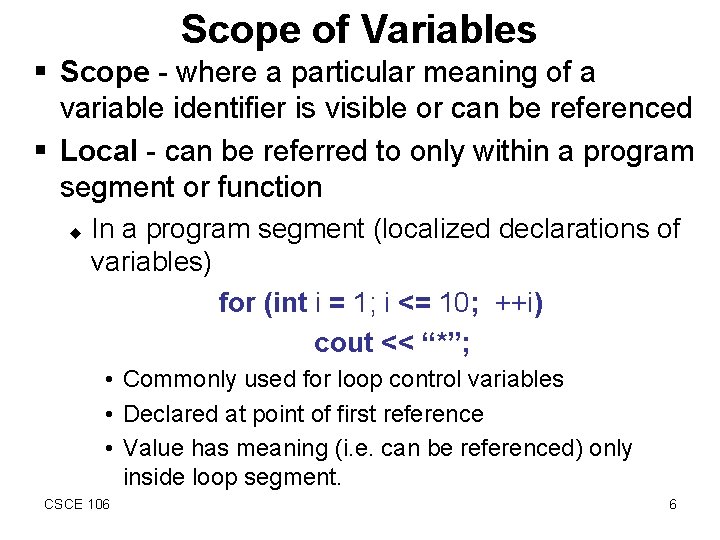
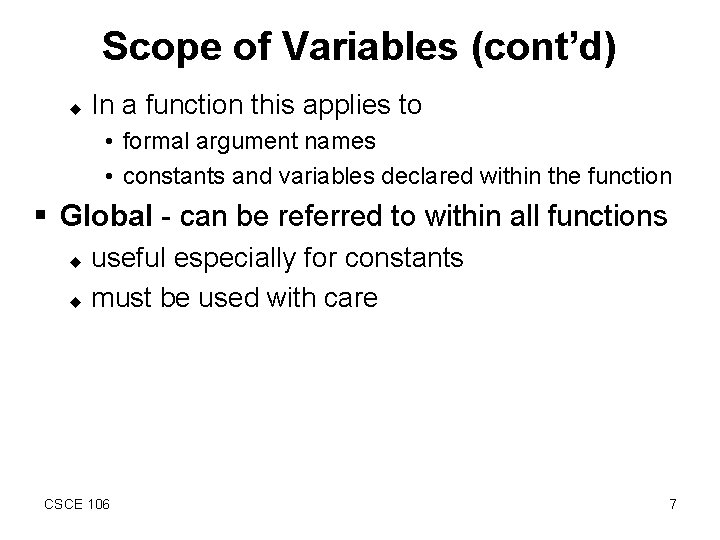
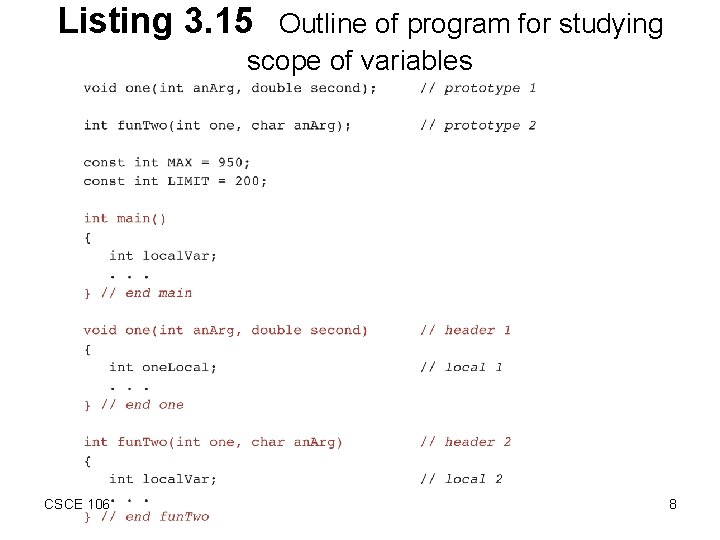
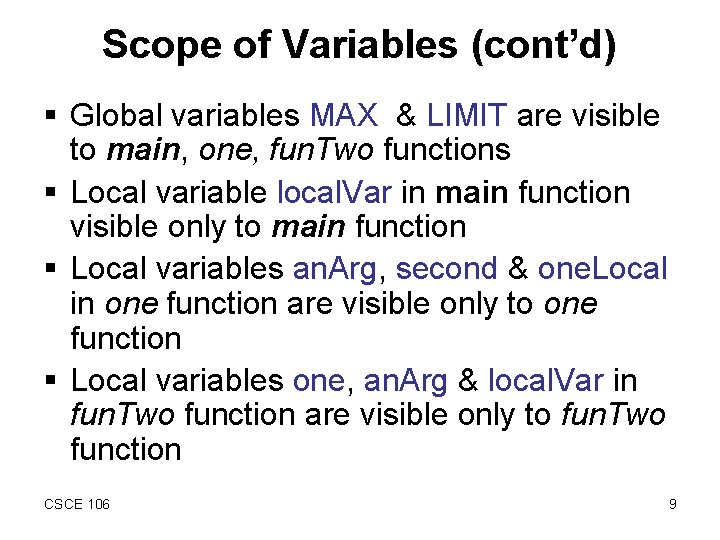
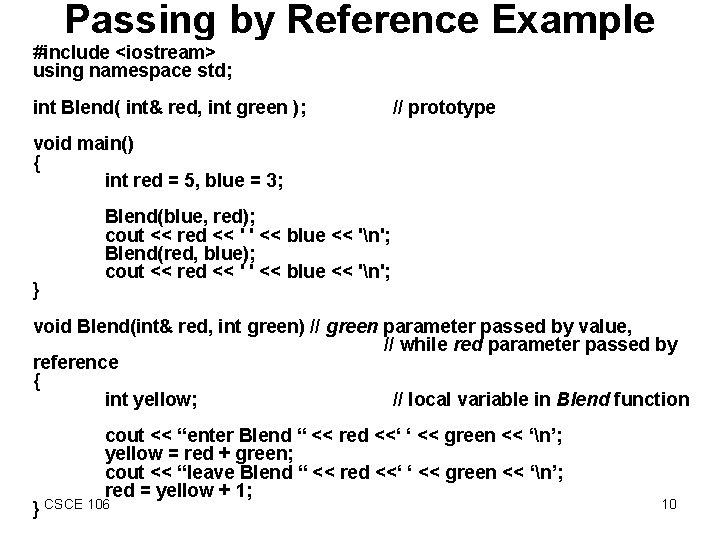
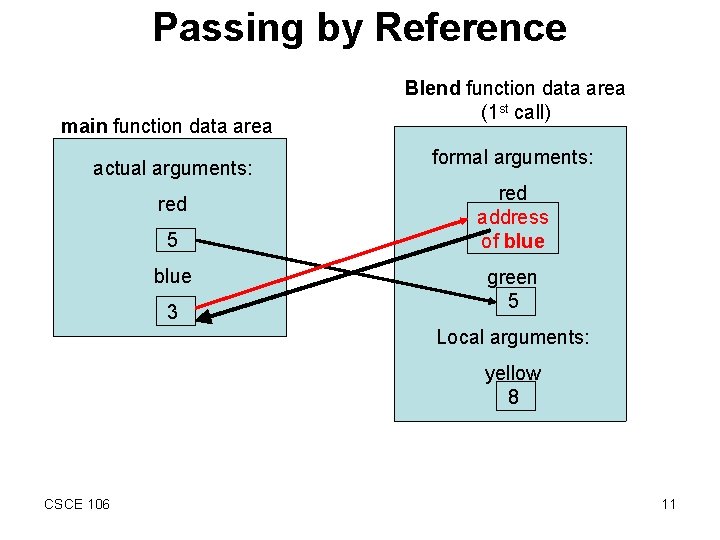
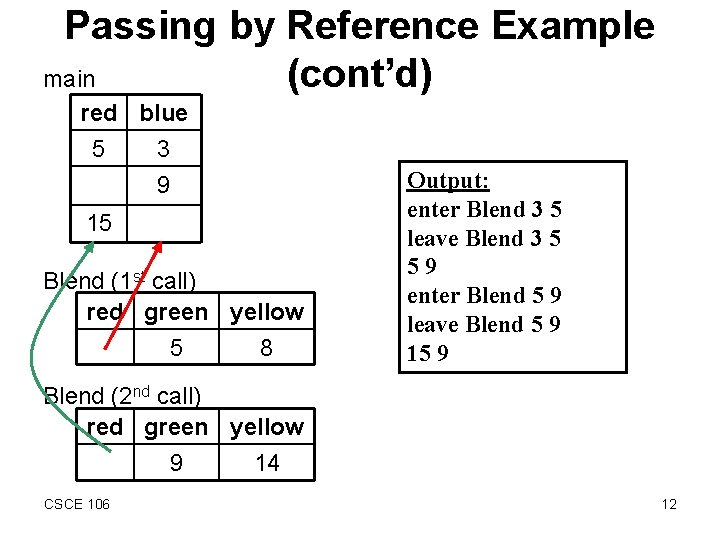
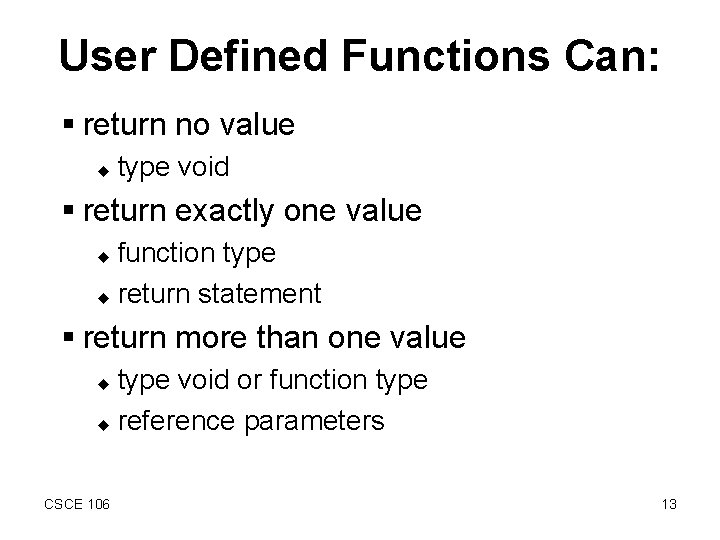
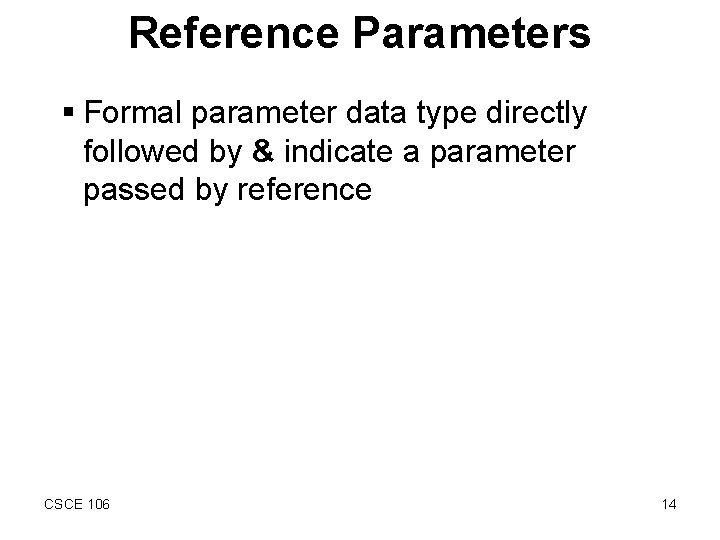
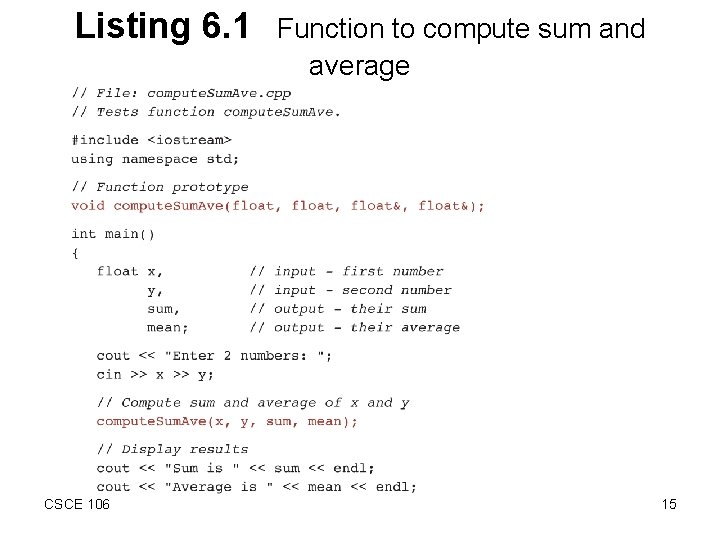
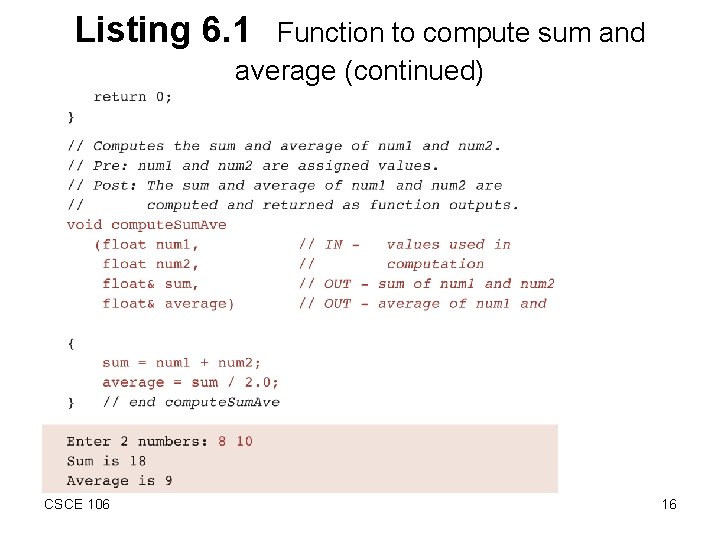
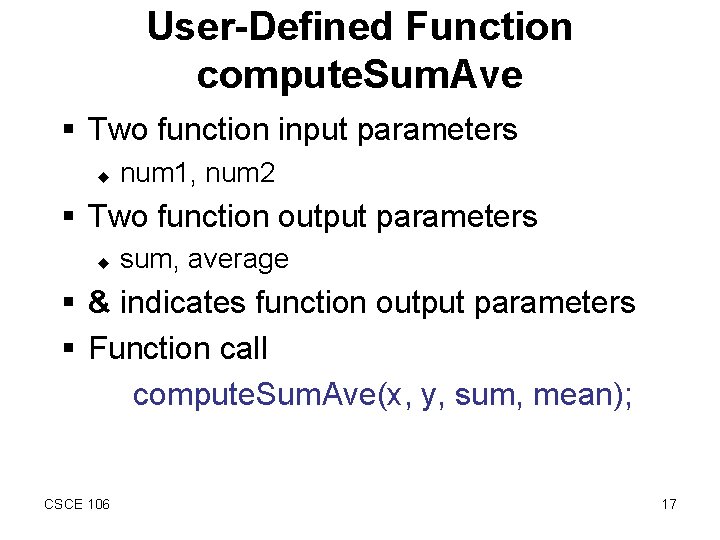
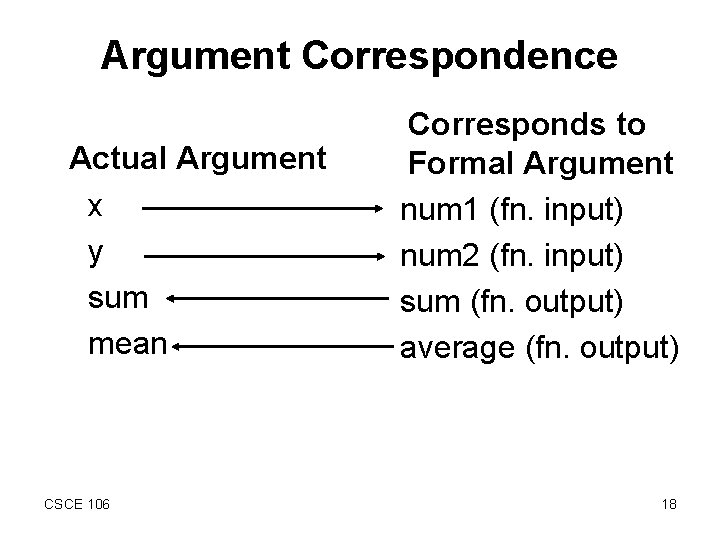
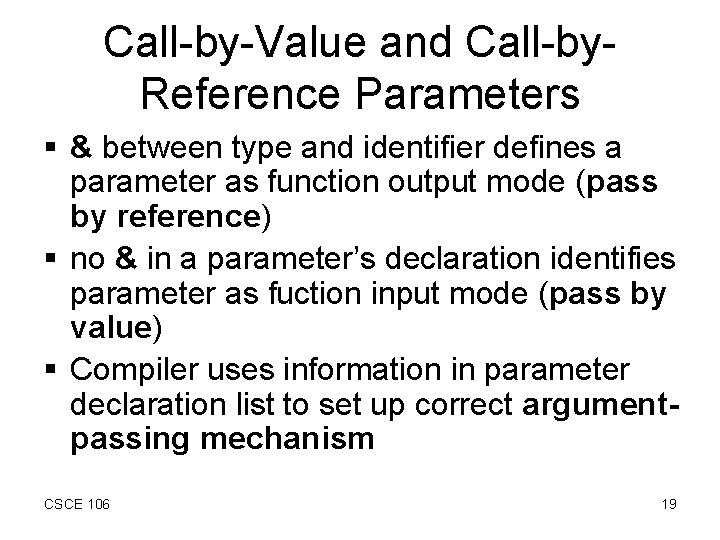
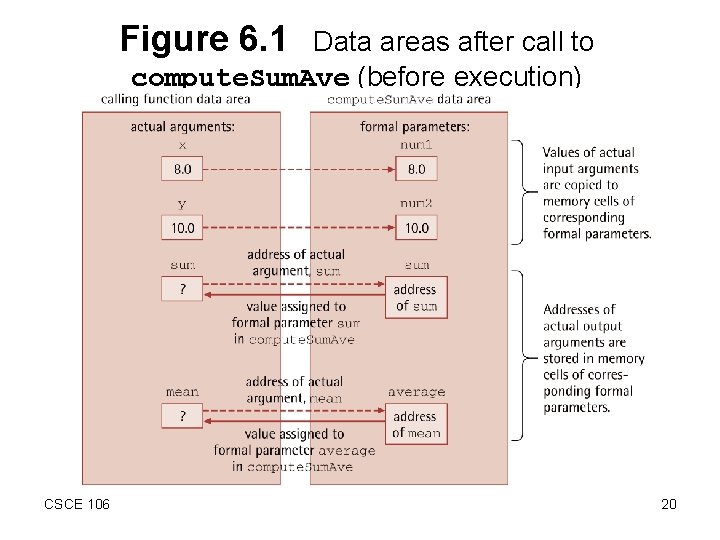
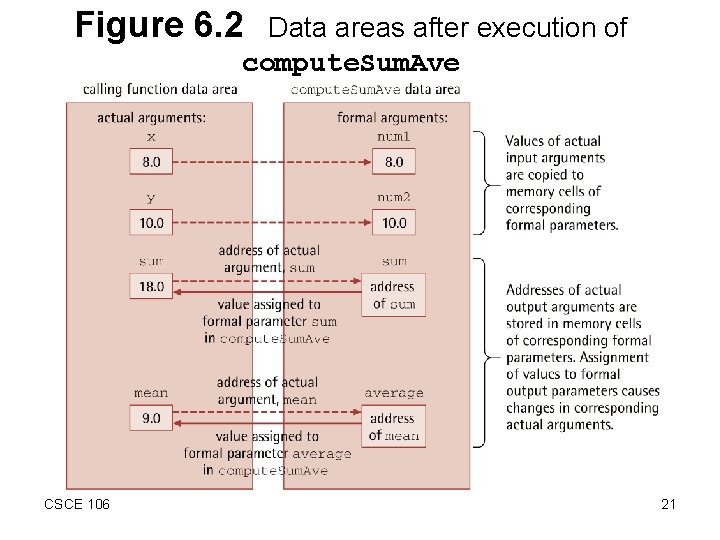
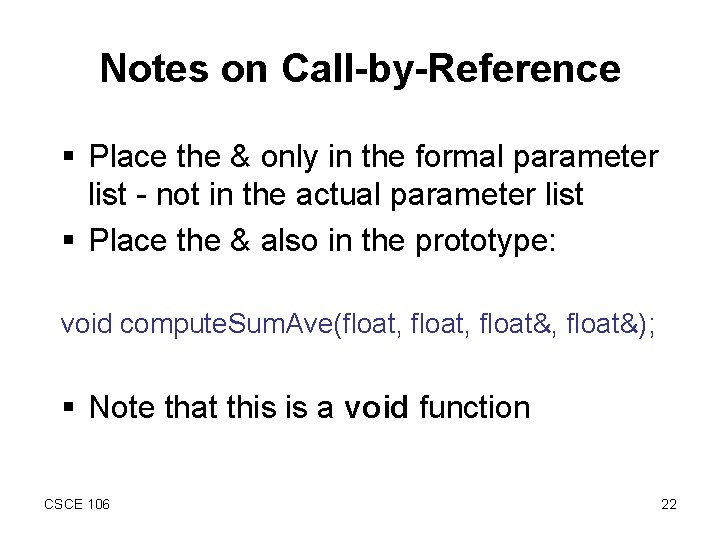
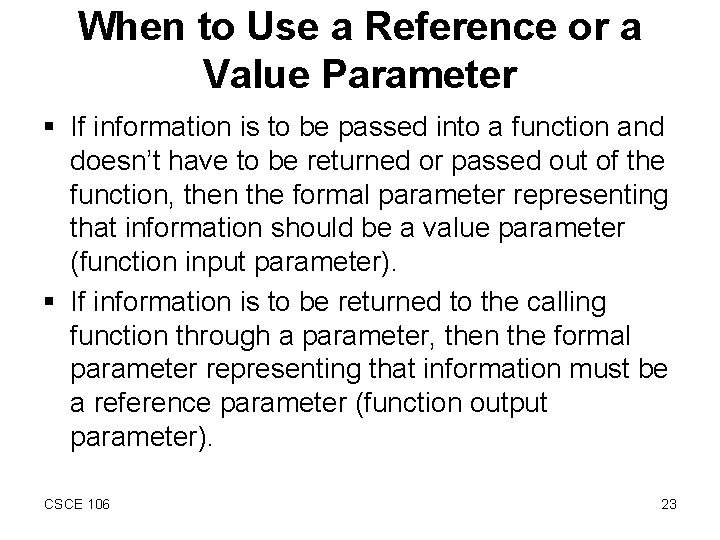
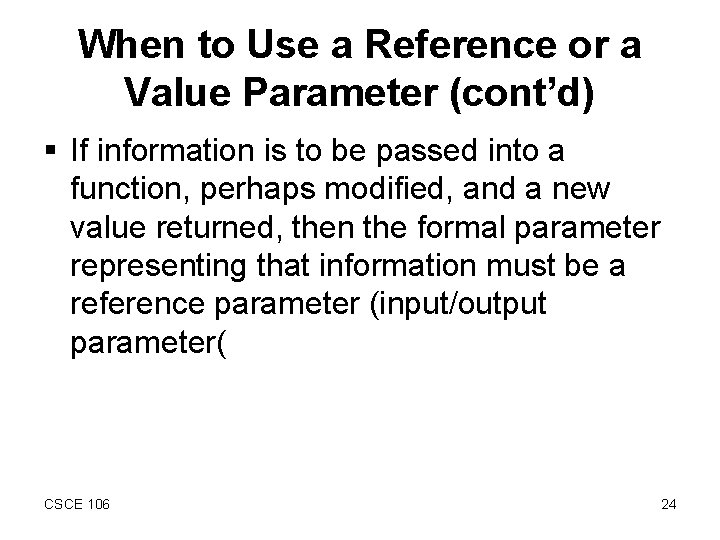
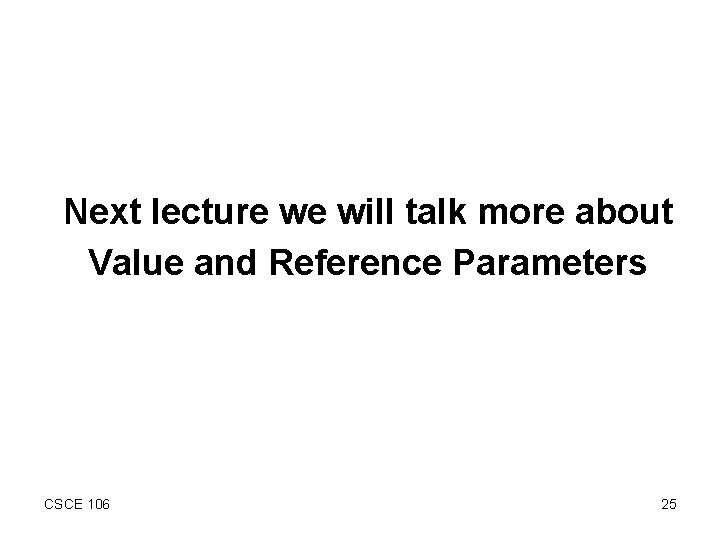
- Slides: 25
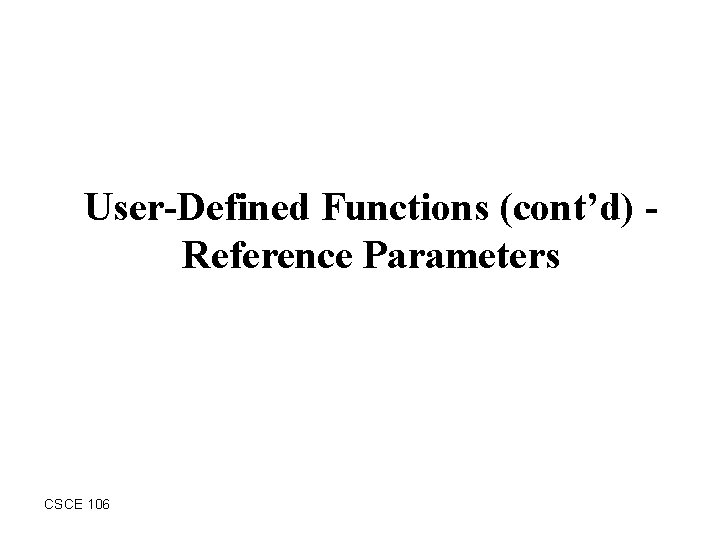
User-Defined Functions (cont’d) Reference Parameters CSCE 106
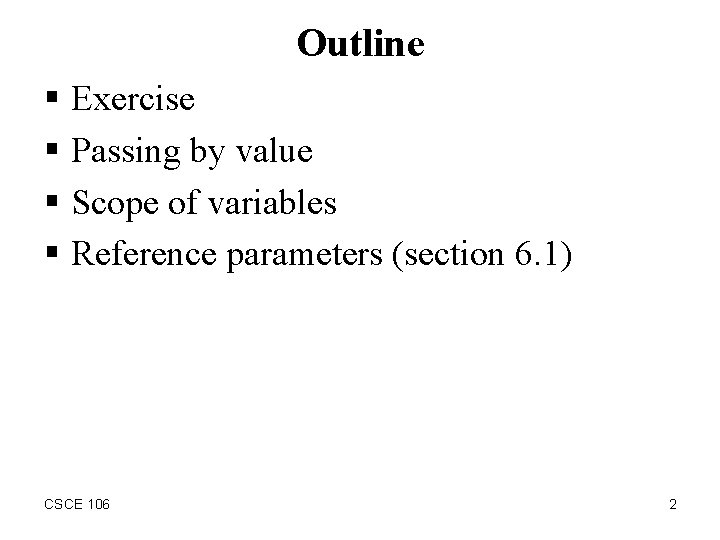
Outline § Exercise § Passing by value § Scope of variables § Reference parameters (section 6. 1) CSCE 106 2
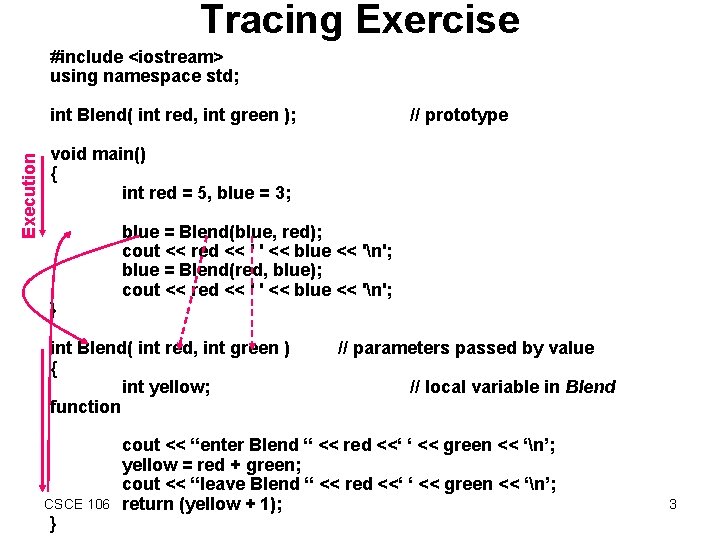
Tracing Exercise #include <iostream> using namespace std; Execution int Blend( int red, int green ); // prototype void main() { int red = 5, blue = 3; } blue = Blend(blue, red); cout << red << ' ' << blue << 'n'; blue = Blend(red, blue); cout << red << ' ' << blue << 'n'; int Blend( int red, int green ) { int yellow; function CSCE 106 } // parameters passed by value // local variable in Blend cout << “enter Blend “ << red <<‘ ‘ << green << ‘n’; yellow = red + green; cout << “leave Blend “ << red <<‘ ‘ << green << ‘n’; return (yellow + 1); 3
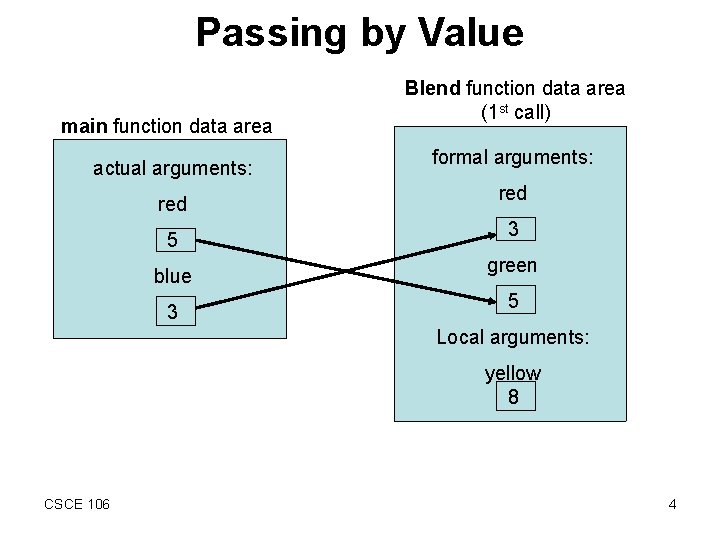
Passing by Value main function data area actual arguments: red 5 blue 3 Blend function data area (1 st call) formal arguments: red 3 green 5 Local arguments: yellow 8 CSCE 106 4
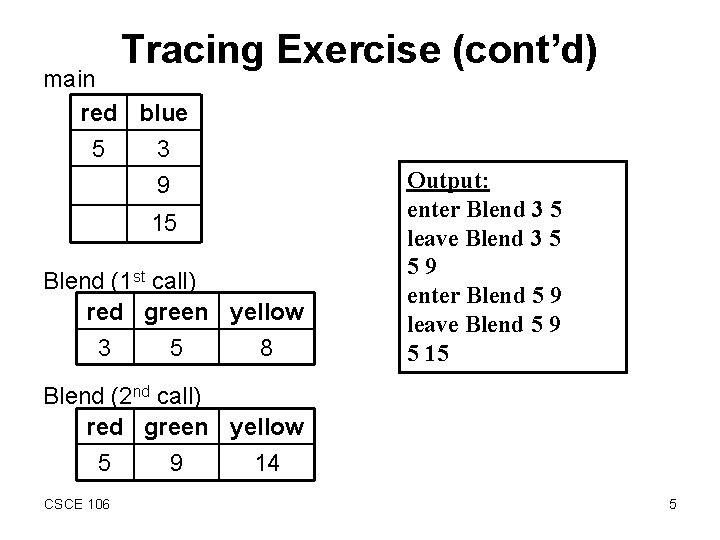
Tracing Exercise (cont’d) main red blue 5 3 9 15 Blend (1 st call) red green yellow 3 5 8 Output: enter Blend 3 5 leave Blend 3 5 59 enter Blend 5 9 leave Blend 5 9 5 15 Blend (2 nd call) red green yellow 5 9 14 CSCE 106 5
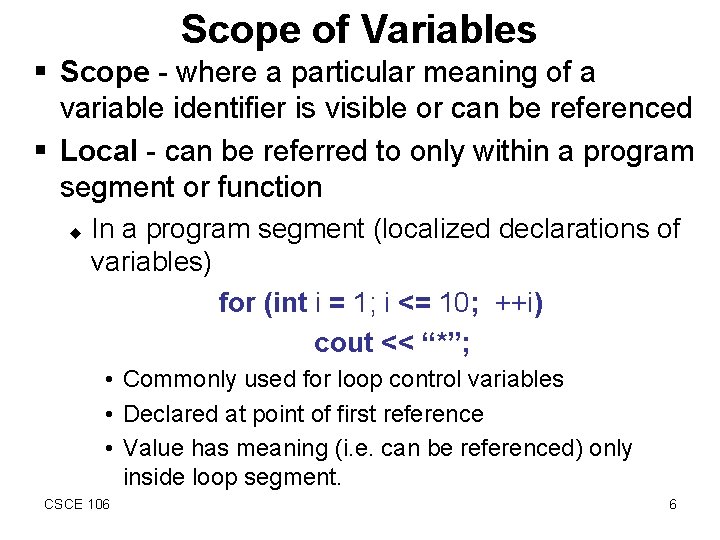
Scope of Variables § Scope - where a particular meaning of a variable identifier is visible or can be referenced § Local - can be referred to only within a program segment or function u In a program segment (localized declarations of variables) for (int i = 1; i <= 10; ++i) cout << “*”; • Commonly used for loop control variables • Declared at point of first reference • Value has meaning (i. e. can be referenced) only inside loop segment. CSCE 106 6
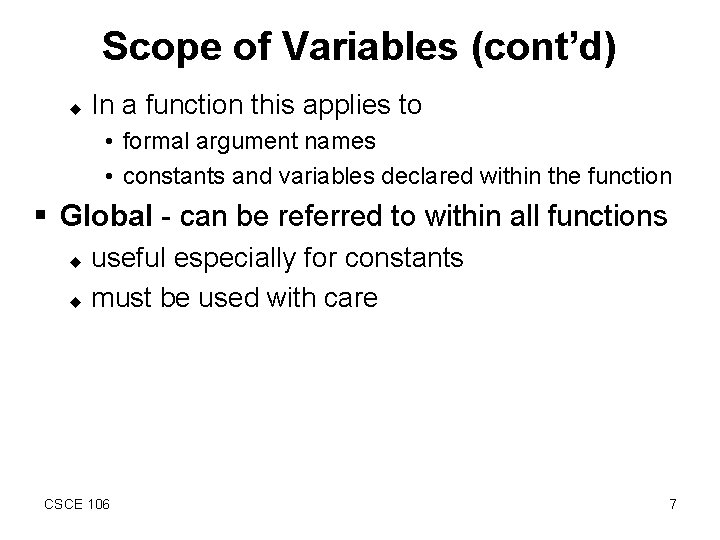
Scope of Variables (cont’d) u In a function this applies to • formal argument names • constants and variables declared within the function § Global - can be referred to within all functions useful especially for constants u must be used with care u CSCE 106 7
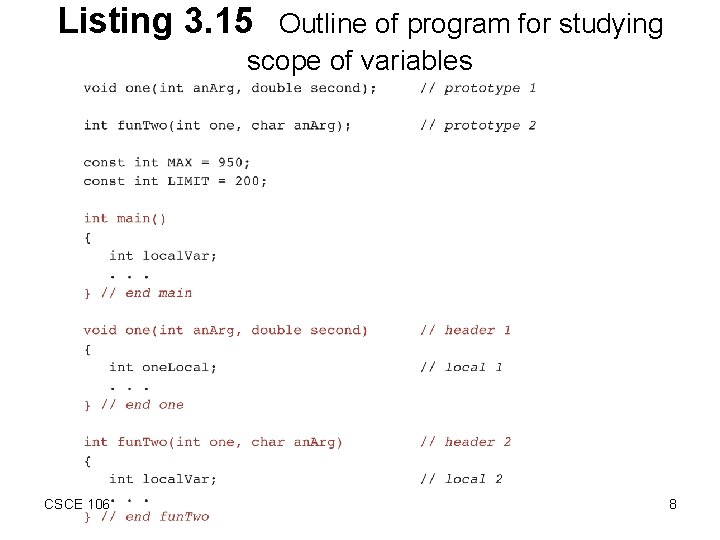
Listing 3. 15 Outline of program for studying scope of variables CSCE 106 8
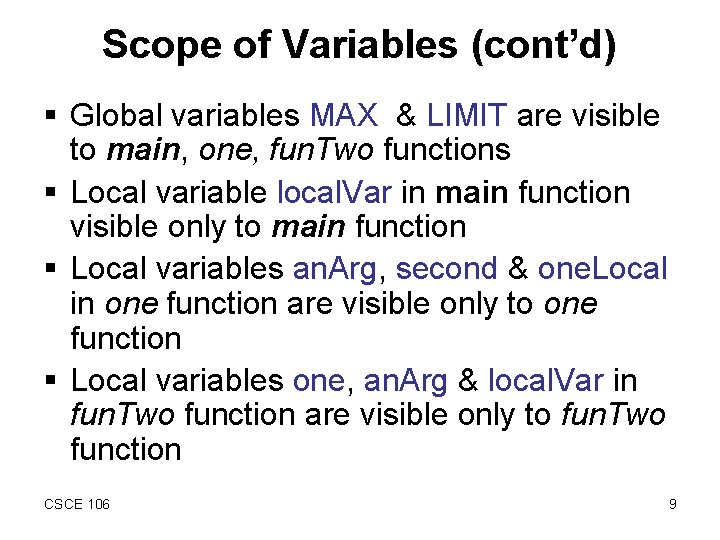
Scope of Variables (cont’d) § Global variables MAX & LIMIT are visible to main, one, fun. Two functions § Local variable local. Var in main function visible only to main function § Local variables an. Arg, second & one. Local in one function are visible only to one function § Local variables one, an. Arg & local. Var in fun. Two function are visible only to fun. Two function CSCE 106 9
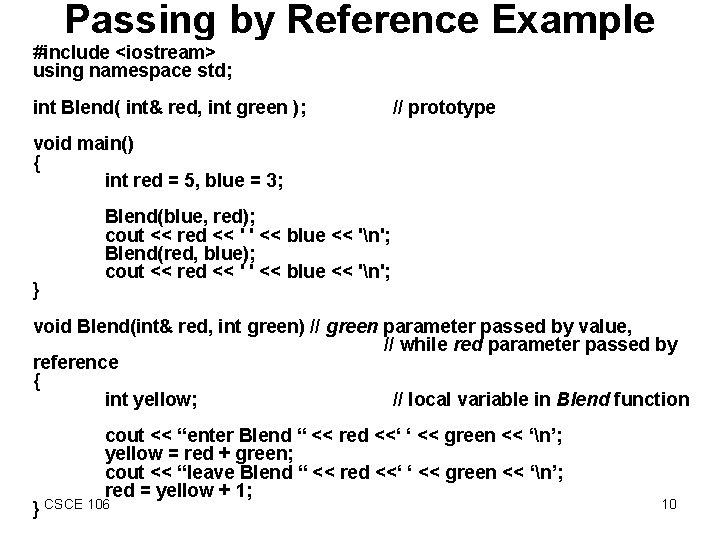
Passing by Reference Example #include <iostream> using namespace std; int Blend( int& red, int green ); // prototype void main() { int red = 5, blue = 3; } Blend(blue, red); cout << red << ' ' << blue << 'n'; Blend(red, blue); cout << red << ' ' << blue << 'n'; void Blend(int& red, int green) // green parameter passed by value, // while red parameter passed by reference { int yellow; // local variable in Blend function cout << “enter Blend “ << red <<‘ ‘ << green << ‘n’; yellow = red + green; cout << “leave Blend “ << red <<‘ ‘ << green << ‘n’; red = yellow + 1; } CSCE 106 10
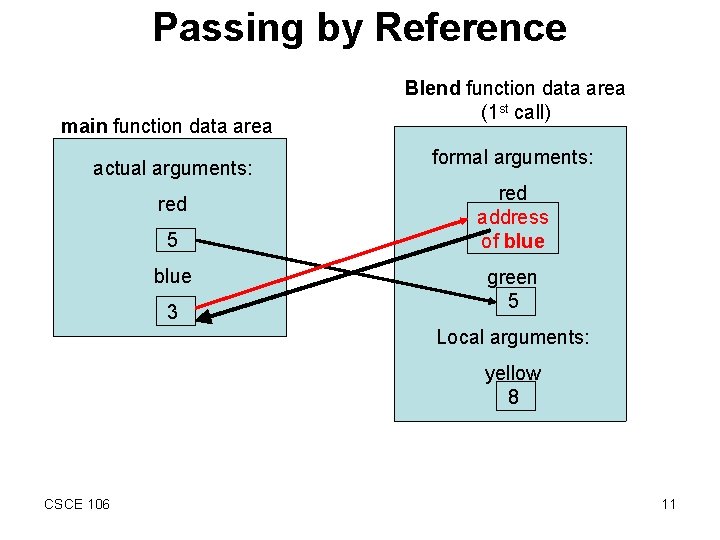
Passing by Reference main function data area actual arguments: red 5 blue 3 Blend function data area (1 st call) formal arguments: red address of blue green 5 Local arguments: yellow 8 CSCE 106 11
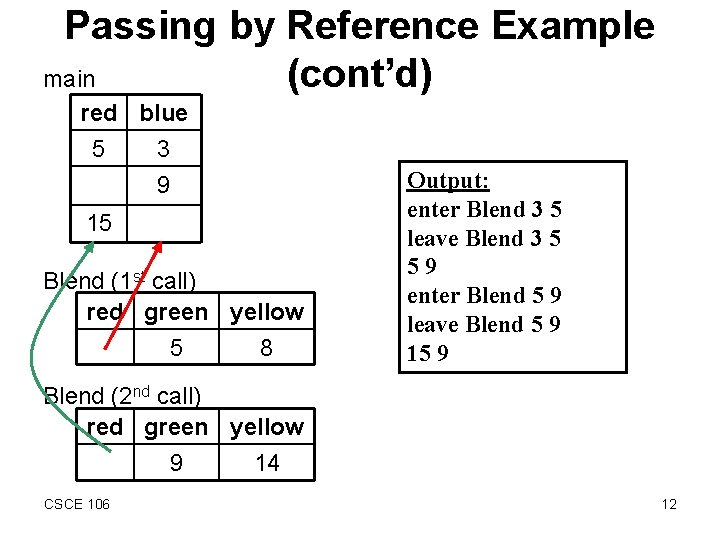
Passing by Reference Example main (cont’d) red blue 5 3 9 15 Blend (1 st call) red green yellow 5 8 Output: enter Blend 3 5 leave Blend 3 5 59 enter Blend 5 9 leave Blend 5 9 15 9 Blend (2 nd call) red green yellow 9 14 CSCE 106 12
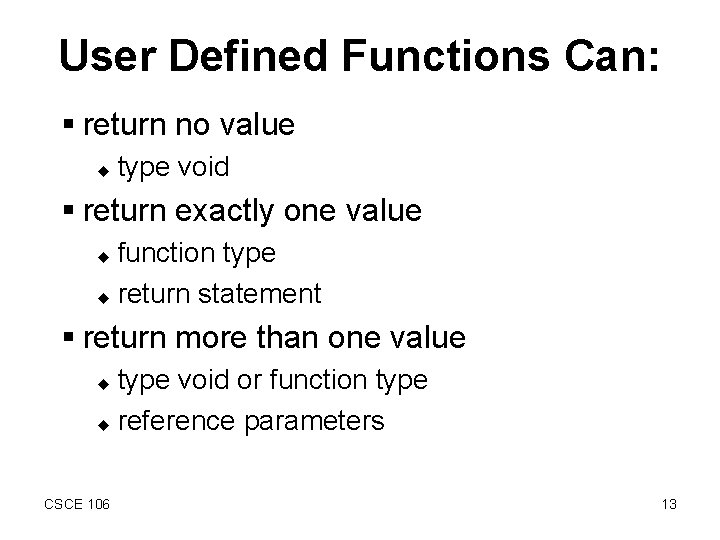
User Defined Functions Can: § return no value u type void § return exactly one value function type u return statement u § return more than one value type void or function type u reference parameters u CSCE 106 13
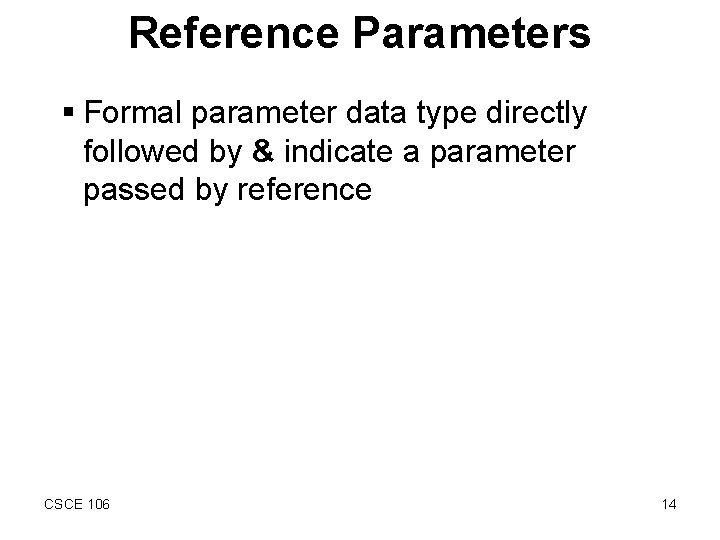
Reference Parameters § Formal parameter data type directly followed by & indicate a parameter passed by reference CSCE 106 14
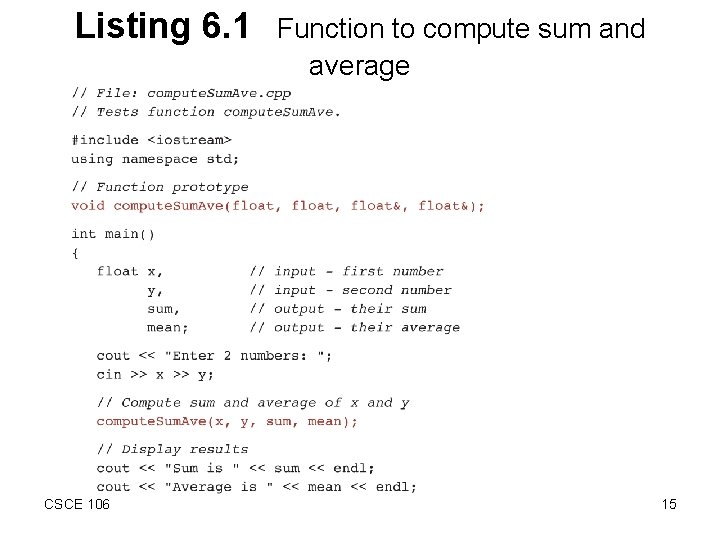
Listing 6. 1 CSCE 106 Function to compute sum and average 15
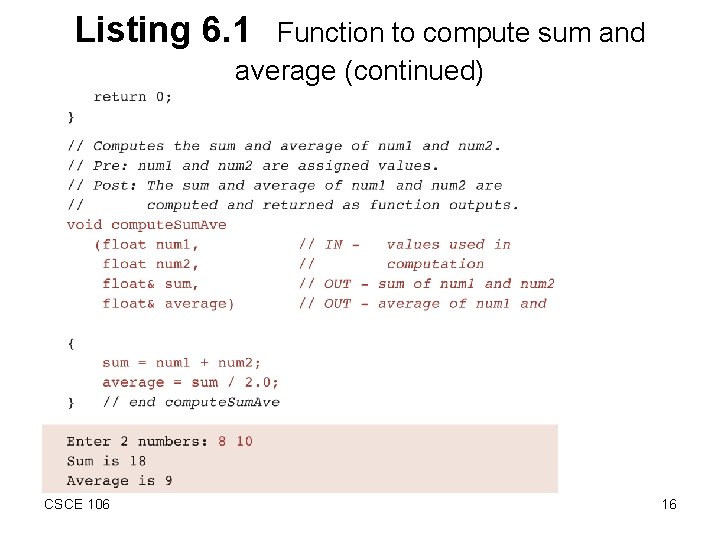
Listing 6. 1 Function to compute sum and average (continued) CSCE 106 16
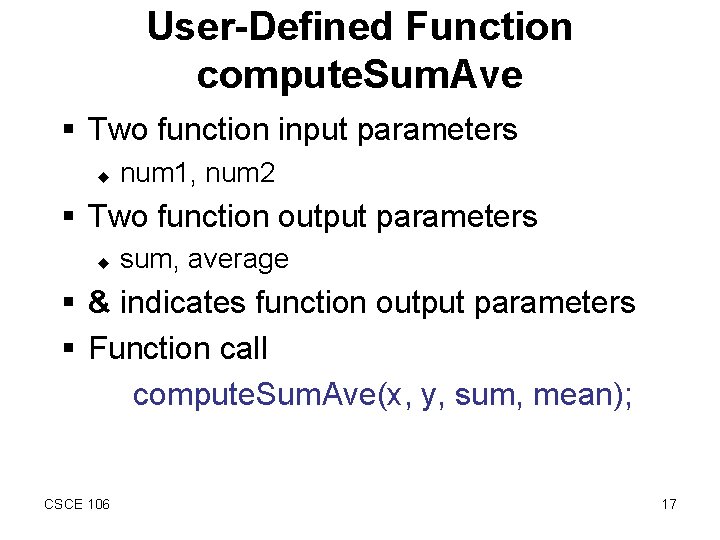
User-Defined Function compute. Sum. Ave § Two function input parameters u num 1, num 2 § Two function output parameters u sum, average § & indicates function output parameters § Function call compute. Sum. Ave(x, y, sum, mean); CSCE 106 17
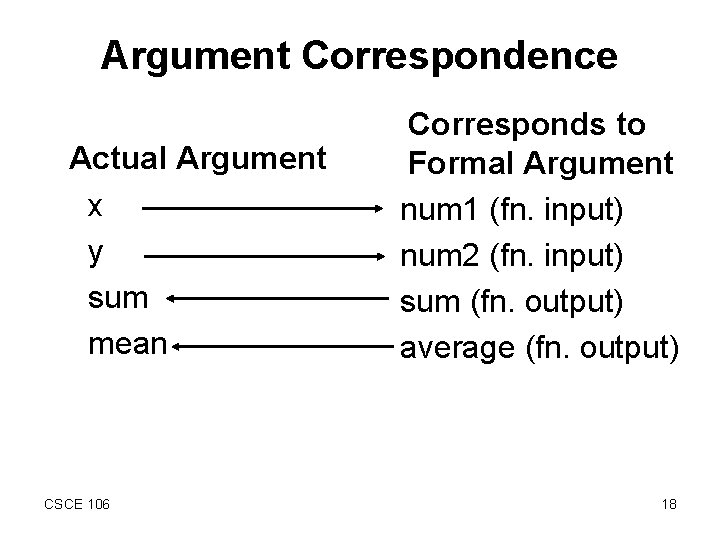
Argument Correspondence Actual Argument x y sum mean CSCE 106 Corresponds to Formal Argument num 1 (fn. input) num 2 (fn. input) sum (fn. output) average (fn. output) 18
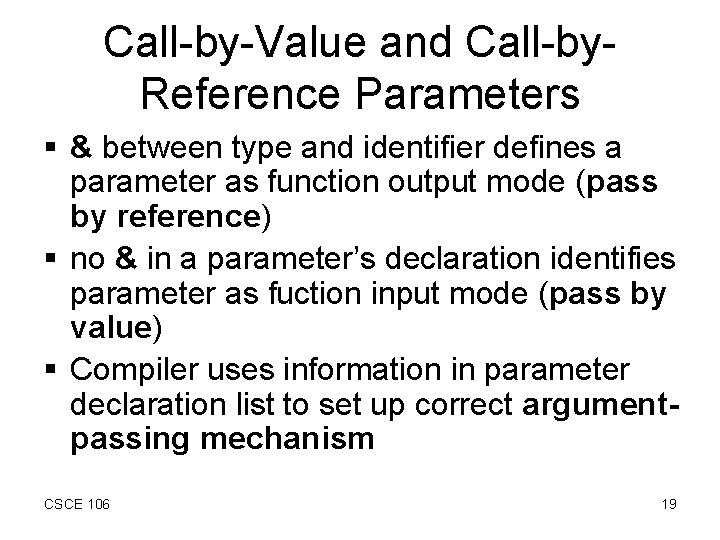
Call-by-Value and Call-by. Reference Parameters § & between type and identifier defines a parameter as function output mode (pass by reference) § no & in a parameter’s declaration identifies parameter as fuction input mode (pass by value) § Compiler uses information in parameter declaration list to set up correct argumentpassing mechanism CSCE 106 19
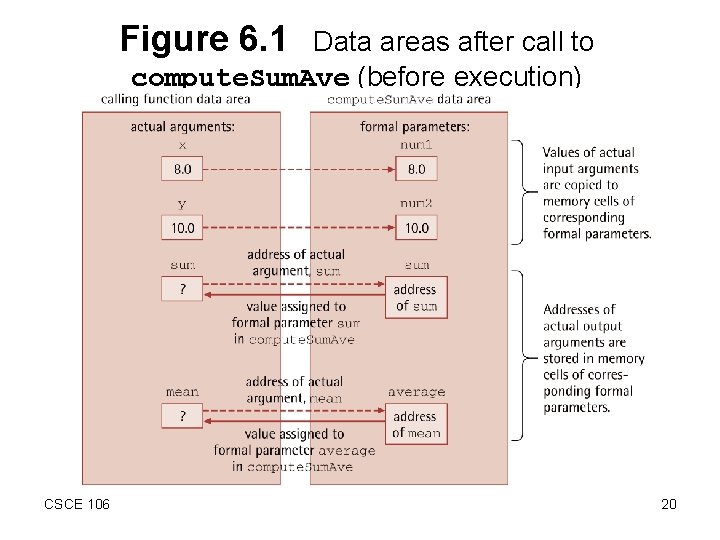
Figure 6. 1 Data areas after call to compute. Sum. Ave (before execution) CSCE 106 20
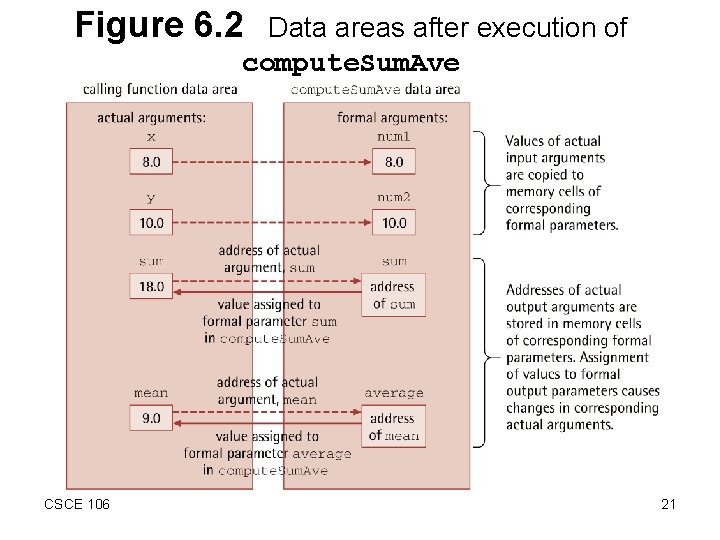
Figure 6. 2 Data areas after execution of compute. Sum. Ave CSCE 106 21
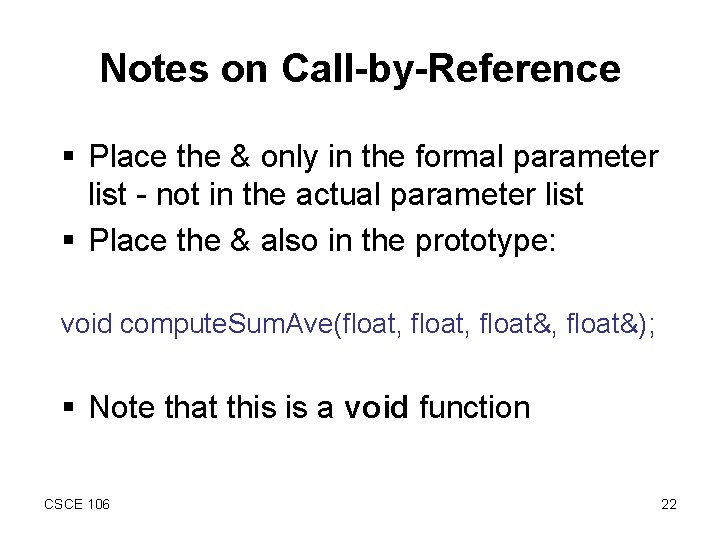
Notes on Call-by-Reference § Place the & only in the formal parameter list - not in the actual parameter list § Place the & also in the prototype: void compute. Sum. Ave(float, float&, float&); § Note that this is a void function CSCE 106 22
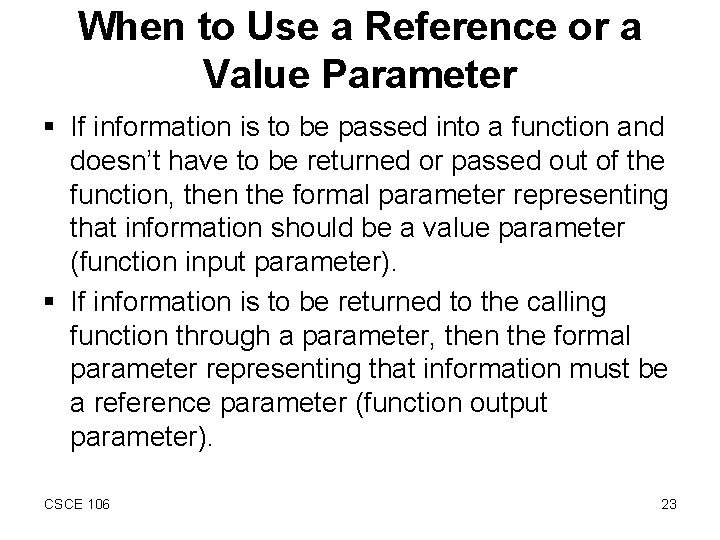
When to Use a Reference or a Value Parameter § If information is to be passed into a function and doesn’t have to be returned or passed out of the function, then the formal parameter representing that information should be a value parameter (function input parameter). § If information is to be returned to the calling function through a parameter, then the formal parameter representing that information must be a reference parameter (function output parameter). CSCE 106 23
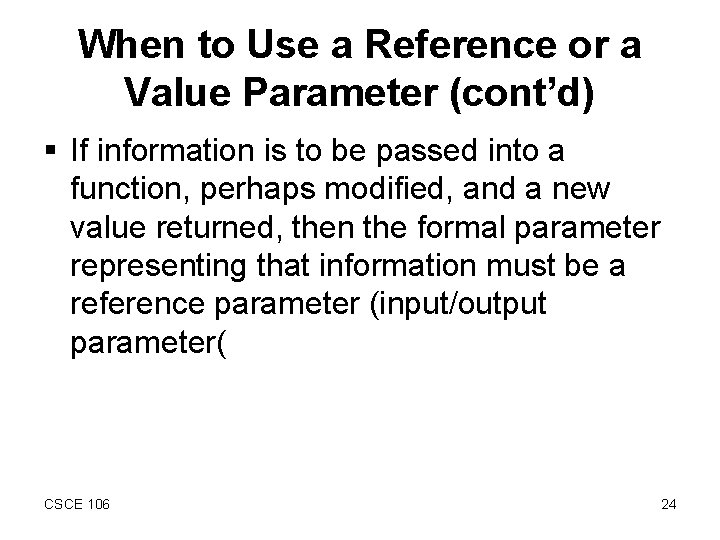
When to Use a Reference or a Value Parameter (cont’d) § If information is to be passed into a function, perhaps modified, and a new value returned, then the formal parameter representing that information must be a reference parameter (input/output parameter( CSCE 106 24
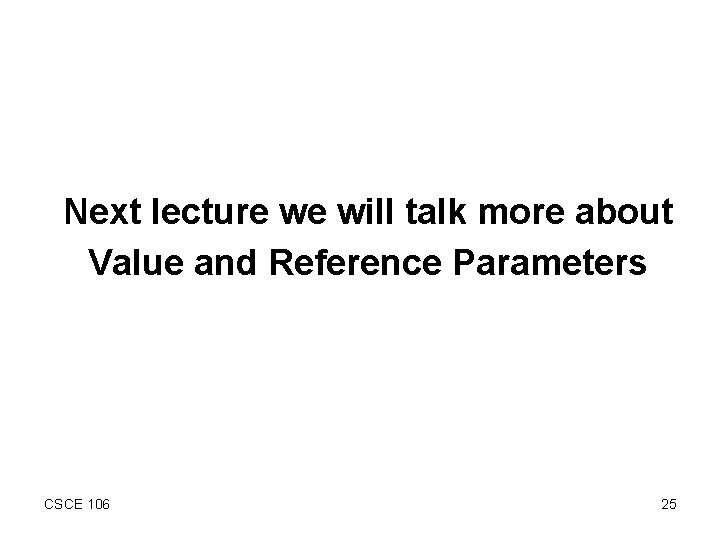
Next lecture we will talk more about Value and Reference Parameters CSCE 106 25
Alliance future internetlapowskyprotocol
Reference node and non reference node
Reference node and non reference node
What is a quote sandwich
106 spelled out
Fahrenheit 451 coloring by number answer key
Cs
Zodiac telemetry
Mae 106 uci
Plt 106
106 pal
106 signal brigade
Error n-106
Section 106 compliance
Salmo 106
Fas 106
Kj 106
Iec tc 106
Iat 106
Horizontal answers
Roberts building 106
456x42
生命聖詩106
100 101 102 103 104 105 106 107 108 109 110
Prime numbers from 101
Sqte-106