Topological Sort Topological Sort Sorting technique over DAGs
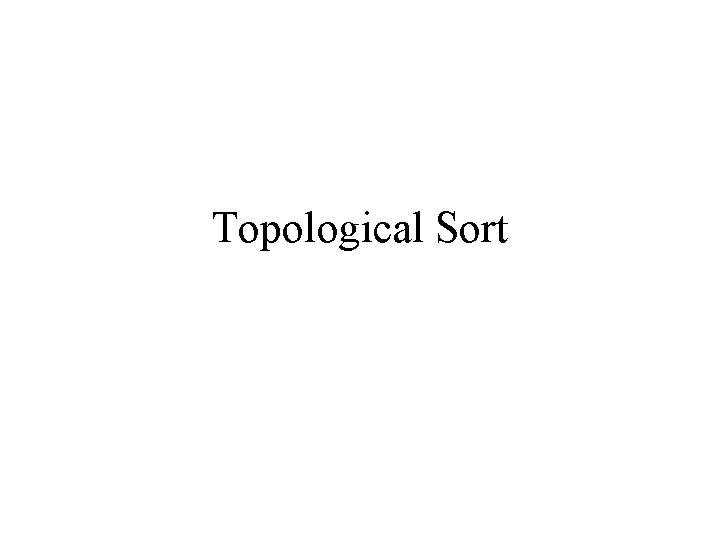
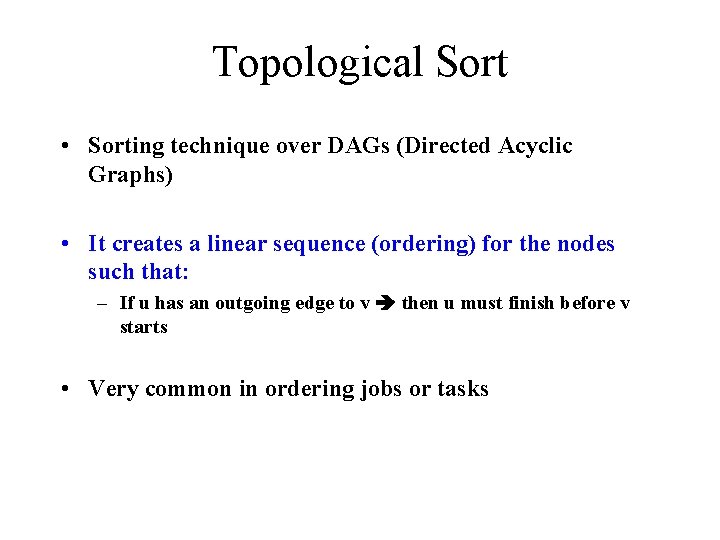
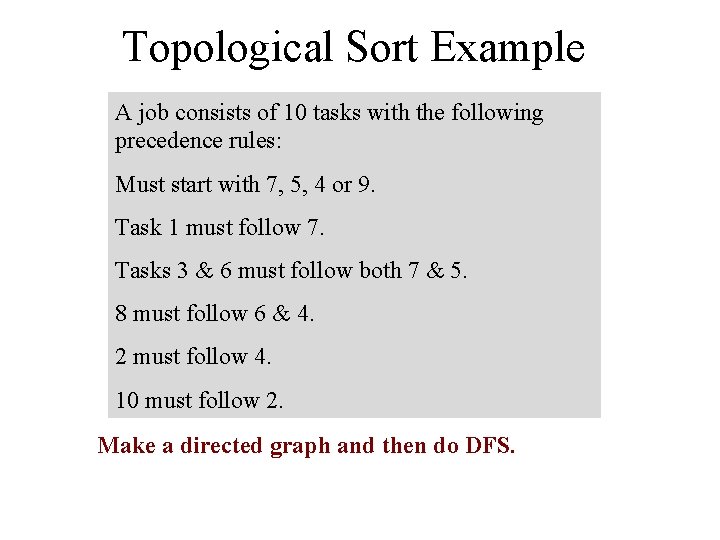
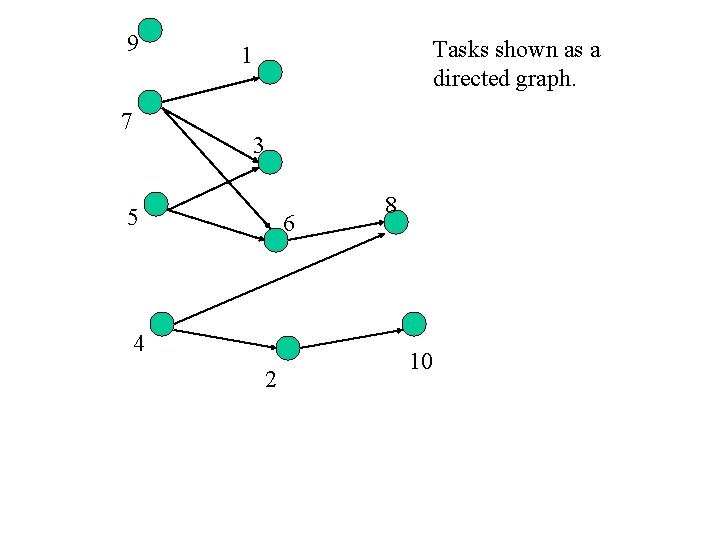
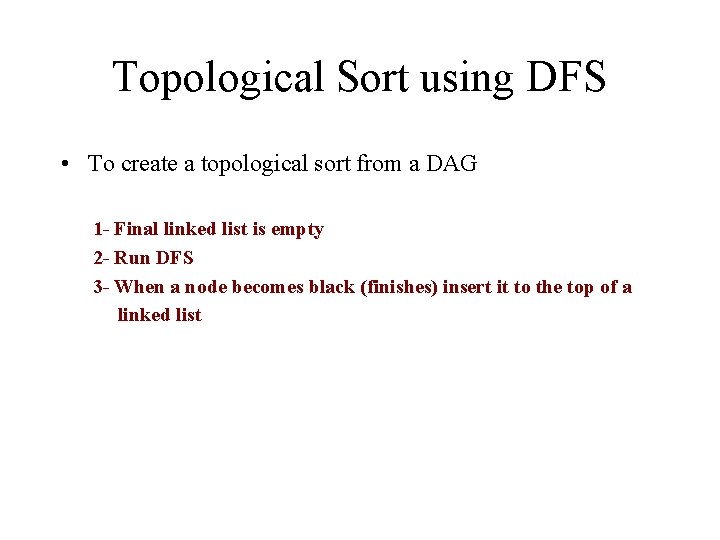
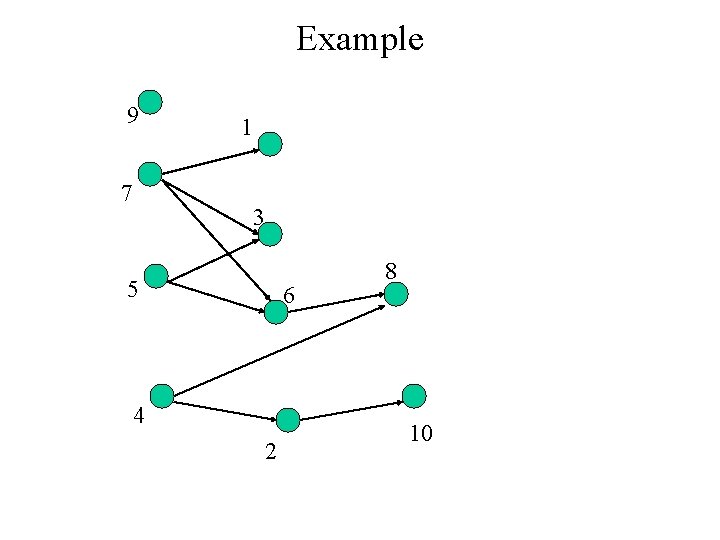
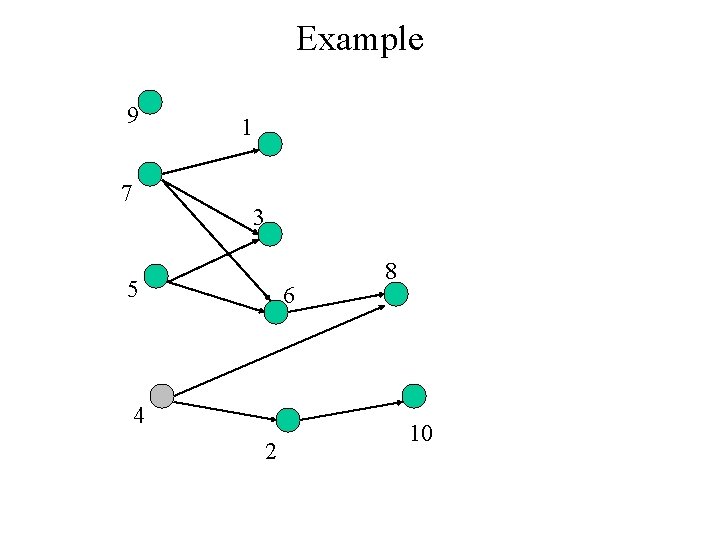
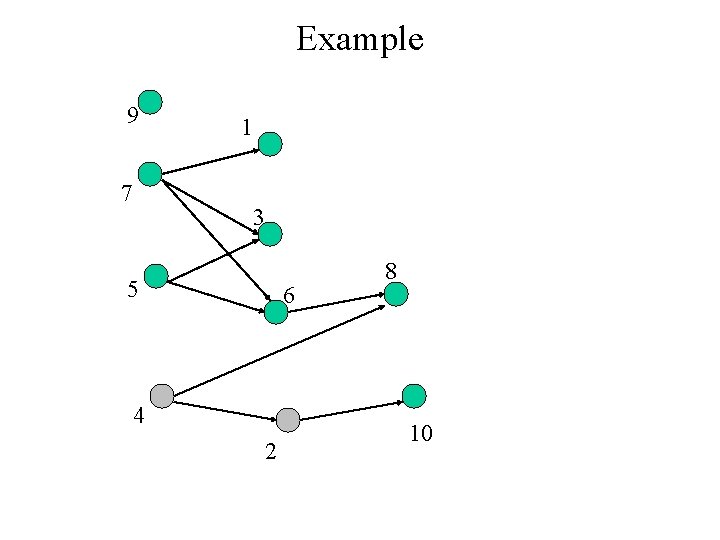
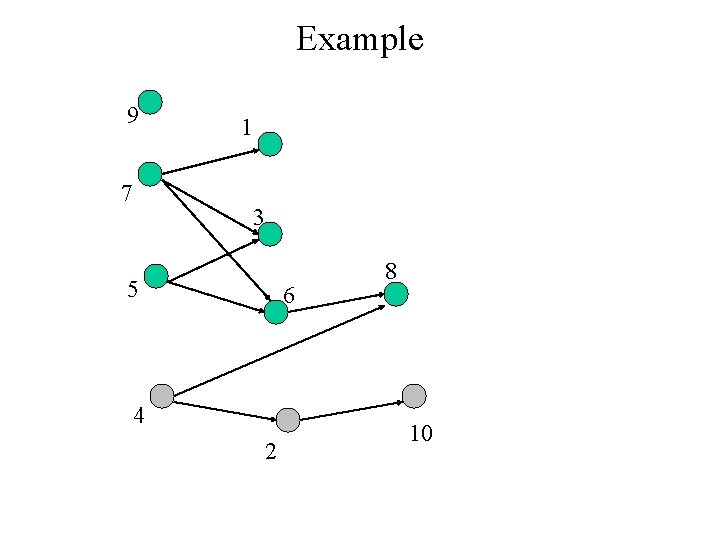
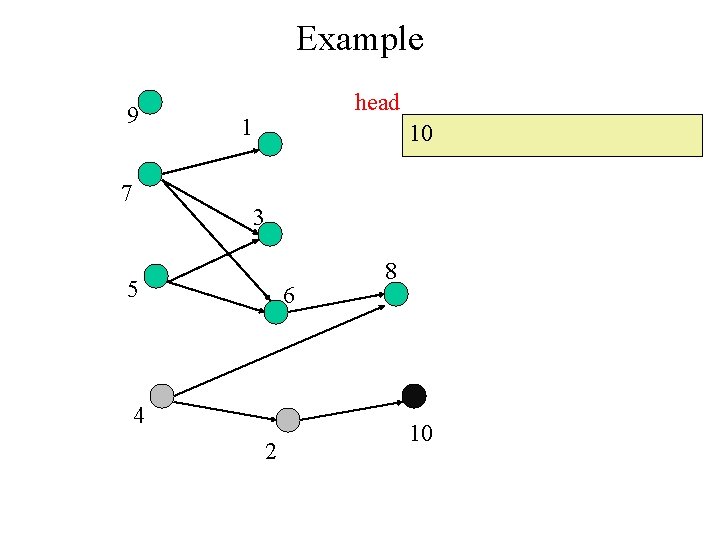
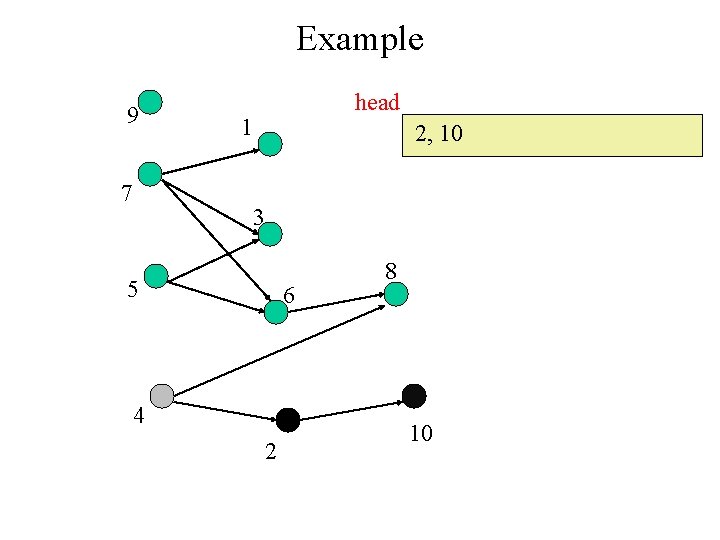
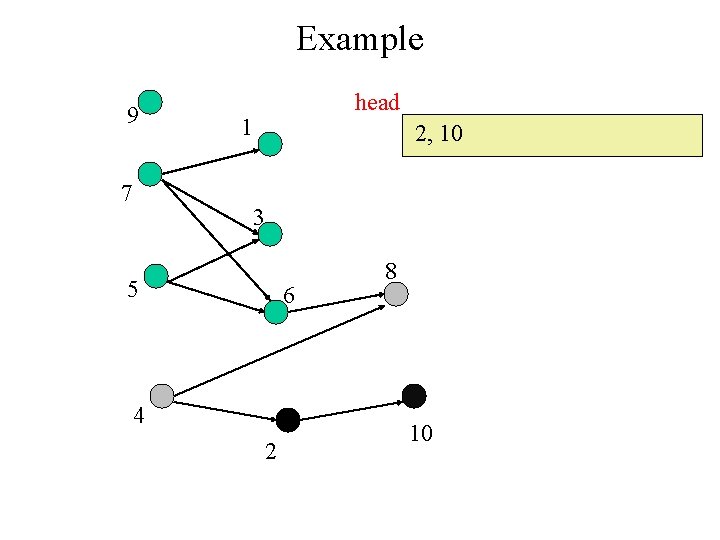
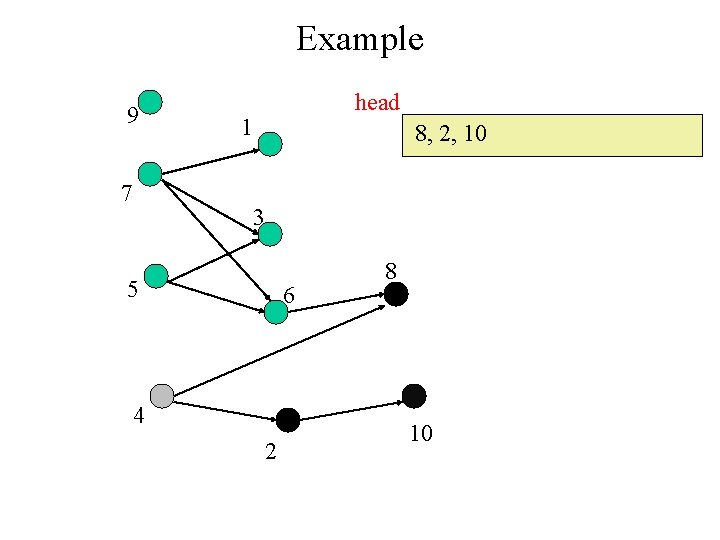
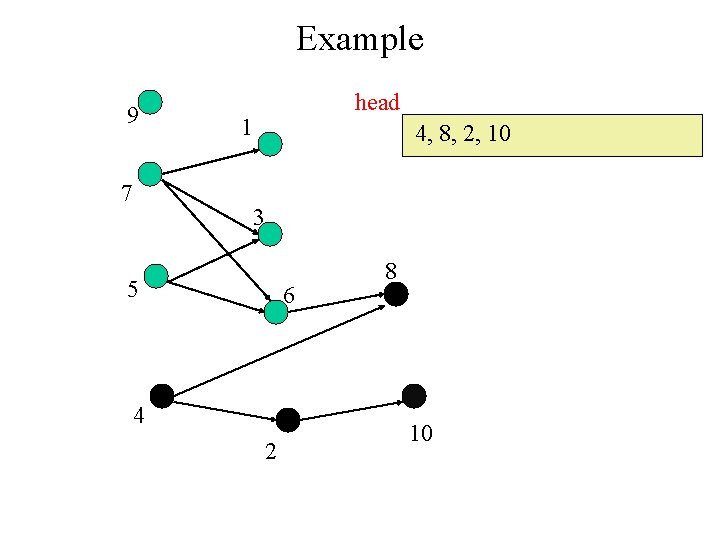
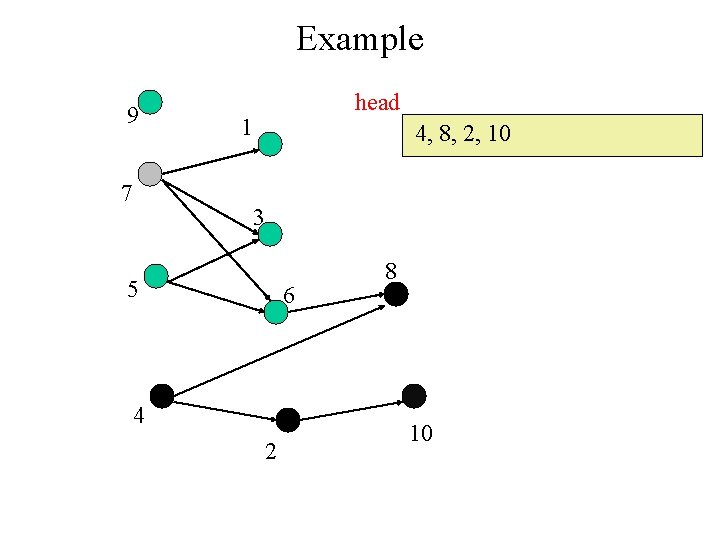
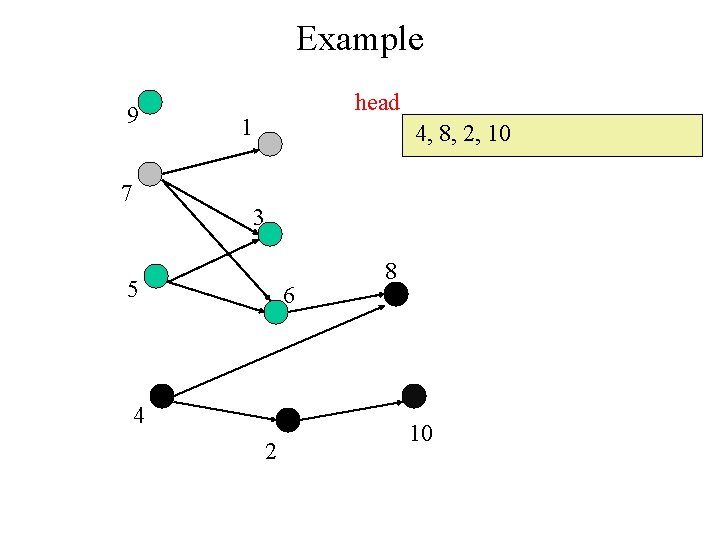
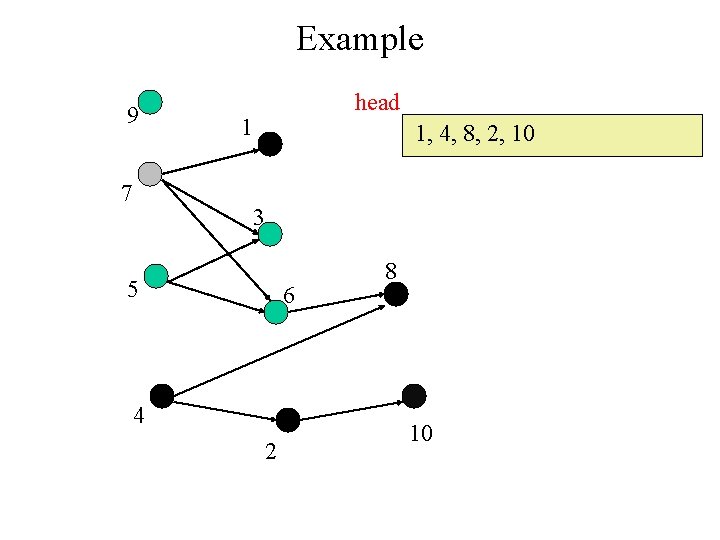
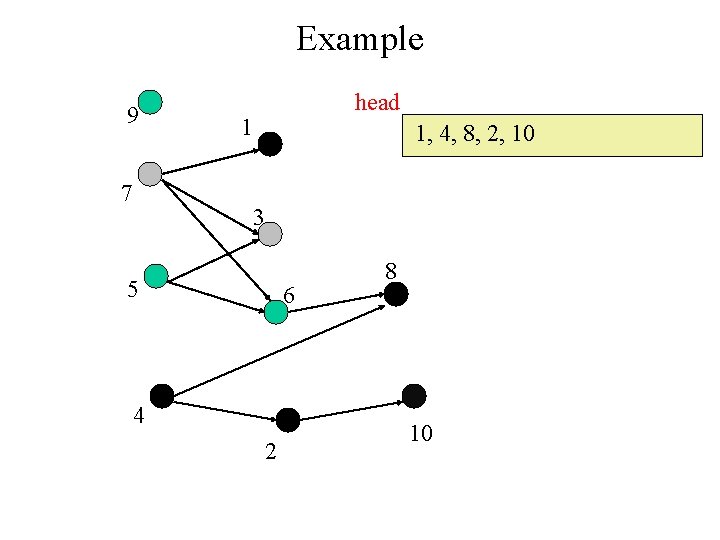
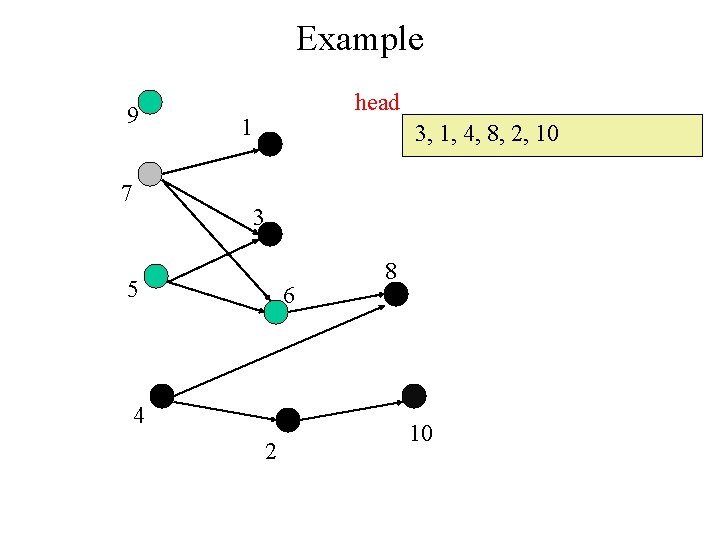
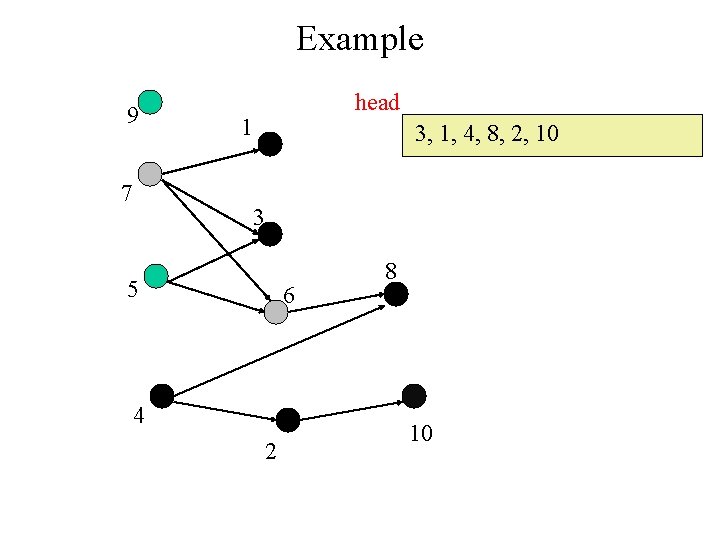
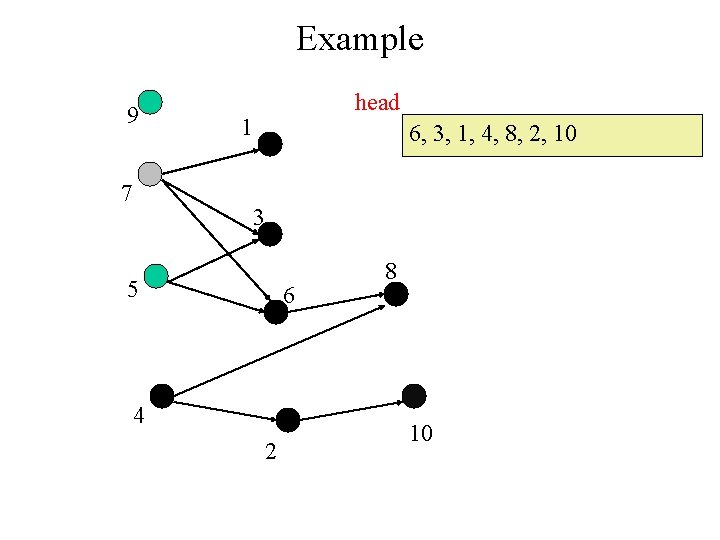
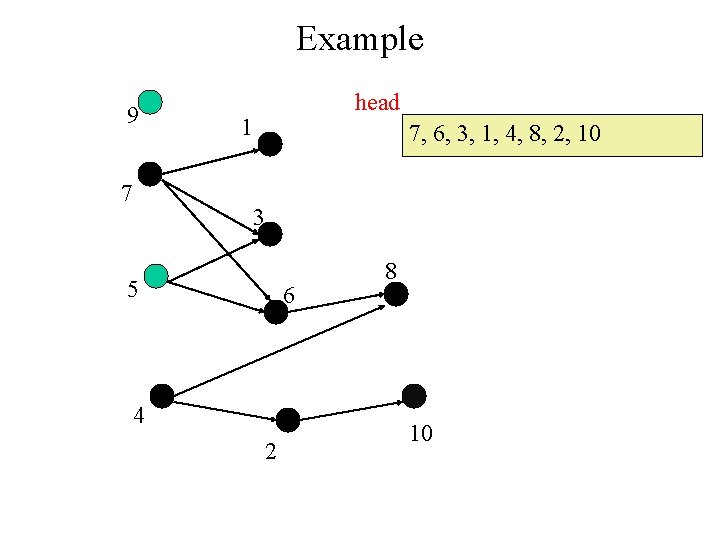
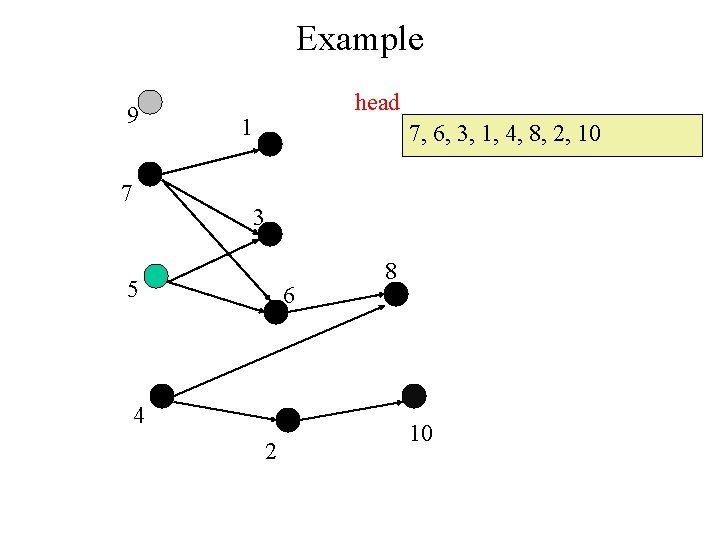
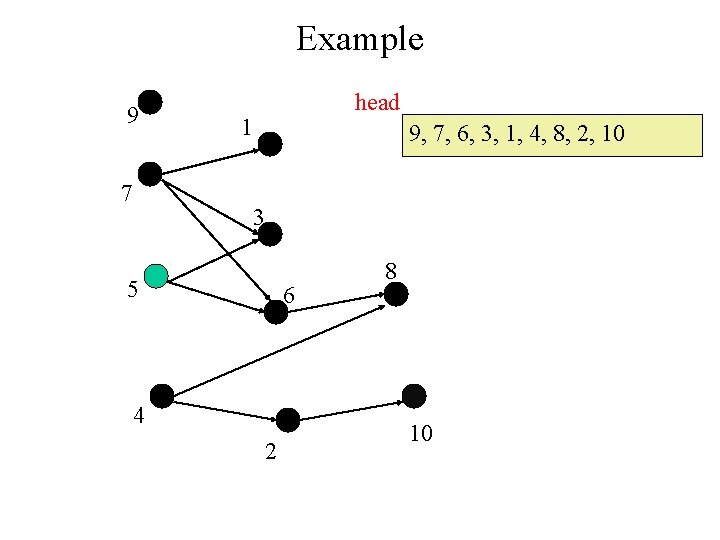
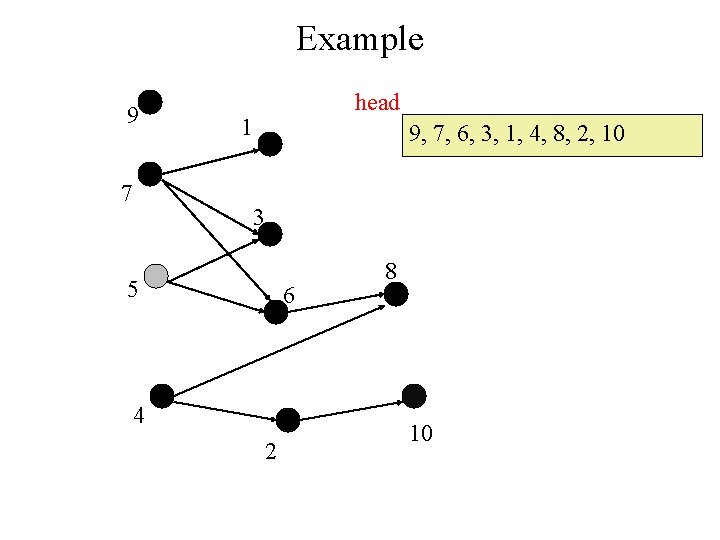
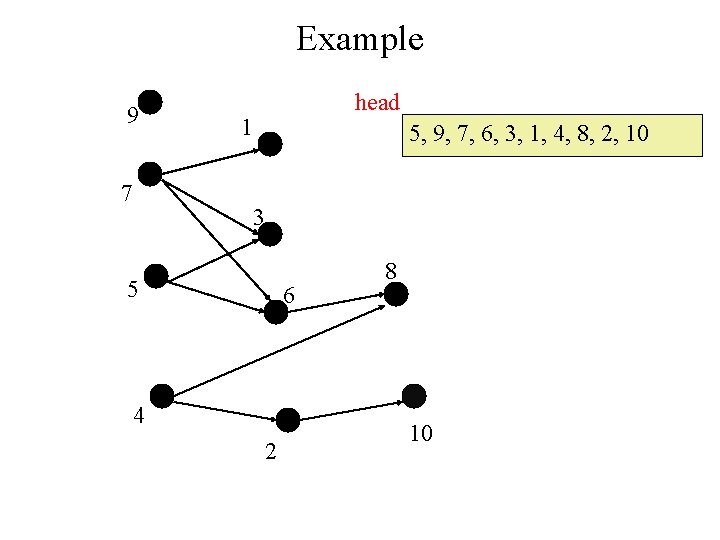
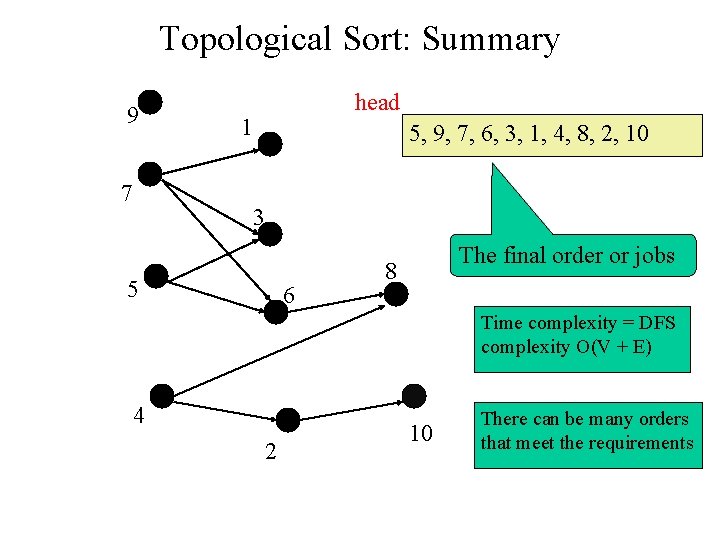
- Slides: 27
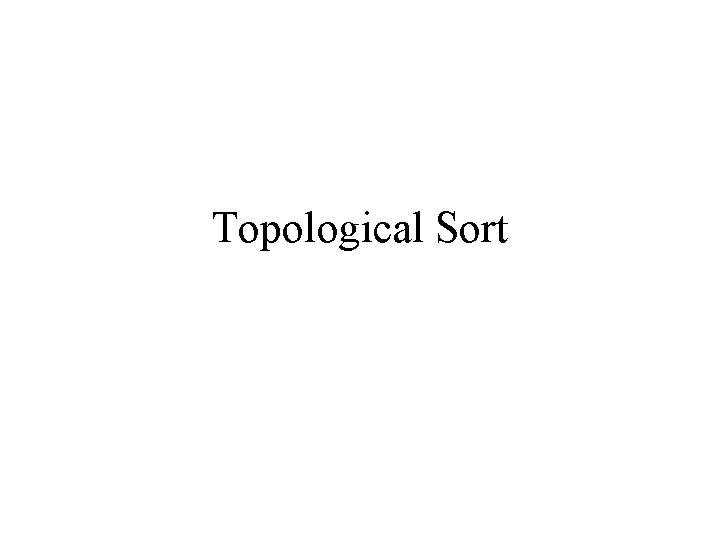
Topological Sort
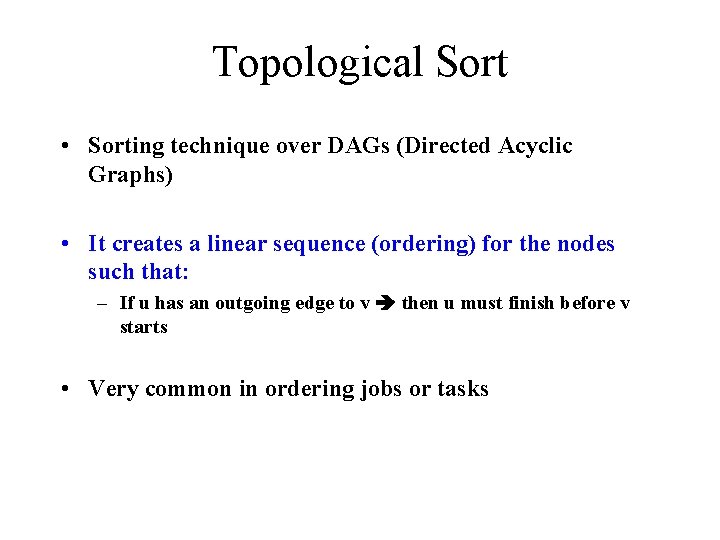
Topological Sort • Sorting technique over DAGs (Directed Acyclic Graphs) • It creates a linear sequence (ordering) for the nodes such that: – If u has an outgoing edge to v then u must finish before v starts • Very common in ordering jobs or tasks
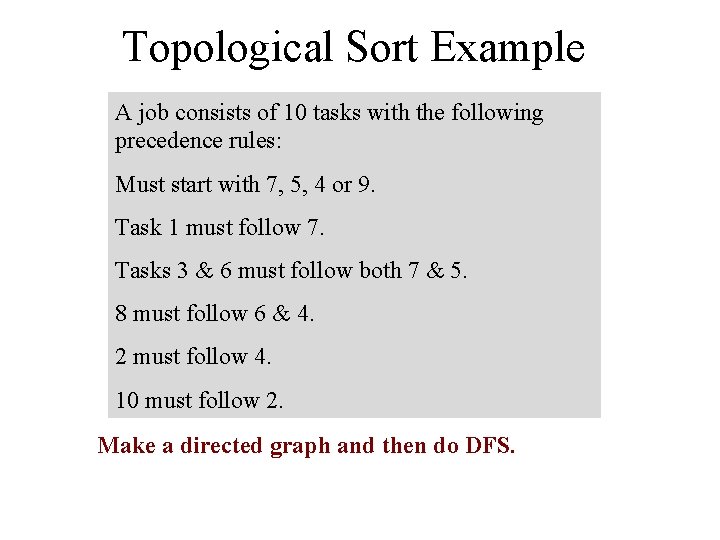
Topological Sort Example A job consists of 10 tasks with the following precedence rules: Must start with 7, 5, 4 or 9. Task 1 must follow 7. Tasks 3 & 6 must follow both 7 & 5. 8 must follow 6 & 4. 2 must follow 4. 10 must follow 2. Make a directed graph and then do DFS.
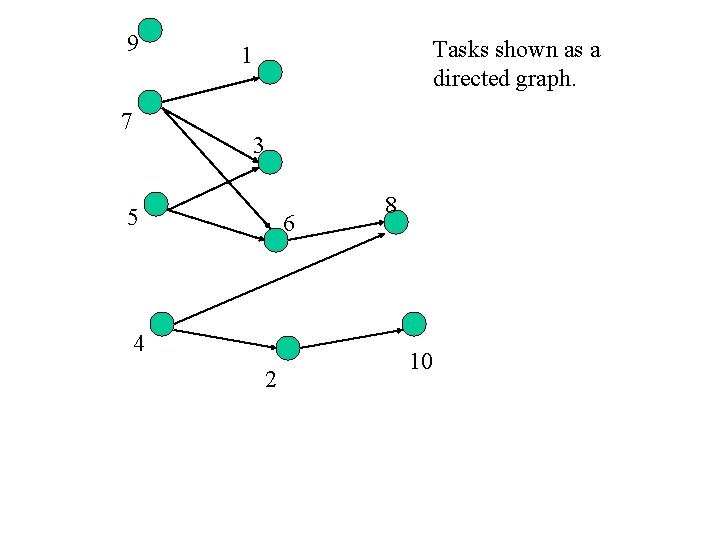
9 7 Tasks shown as a directed graph. 1 3 5 6 4 2 8 10
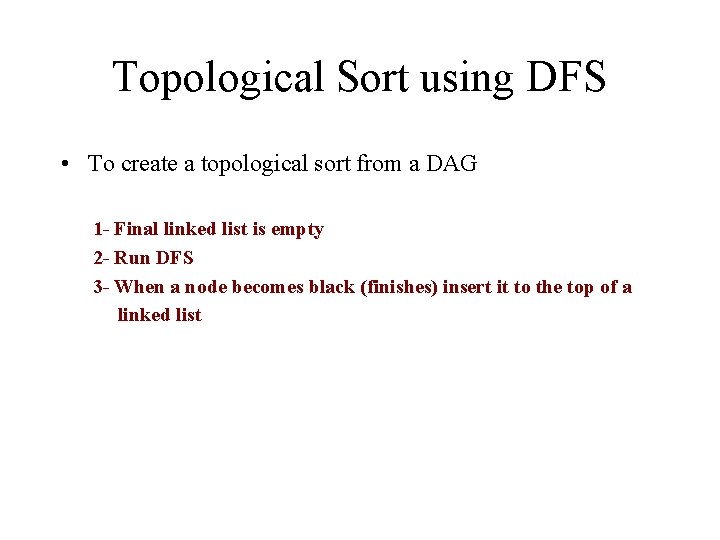
Topological Sort using DFS • To create a topological sort from a DAG 1 - Final linked list is empty 2 - Run DFS 3 - When a node becomes black (finishes) insert it to the top of a linked list
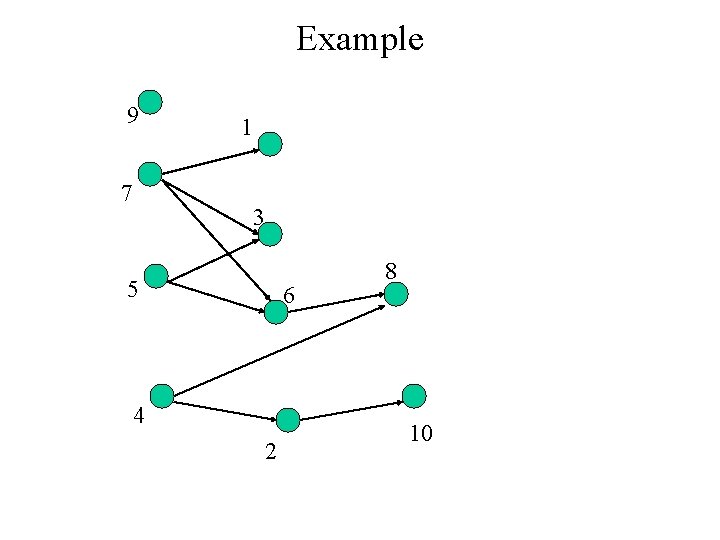
Example 9 7 1 3 5 6 4 2 8 10
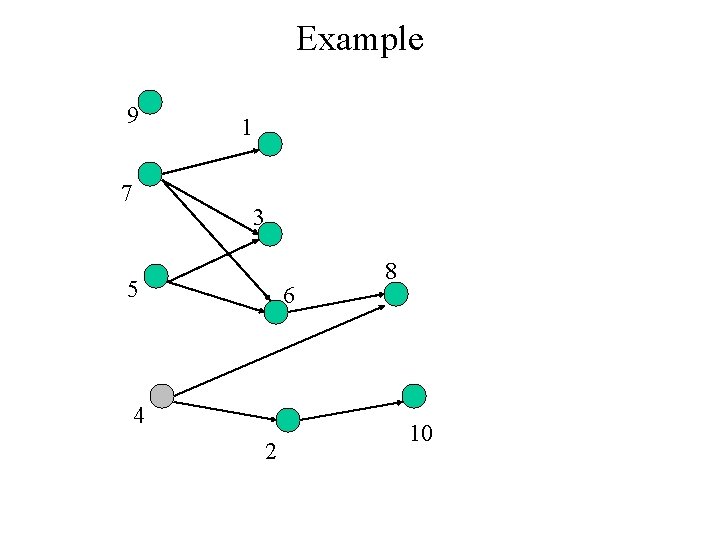
Example 9 7 1 3 5 6 4 2 8 10
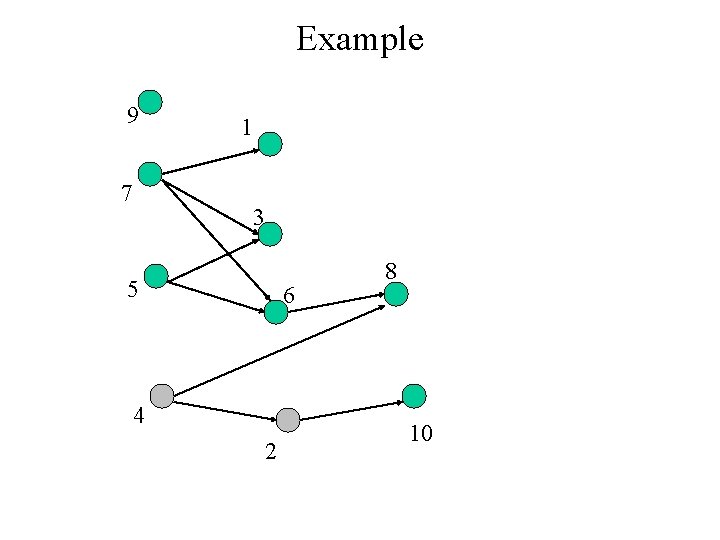
Example 9 7 1 3 5 6 4 2 8 10
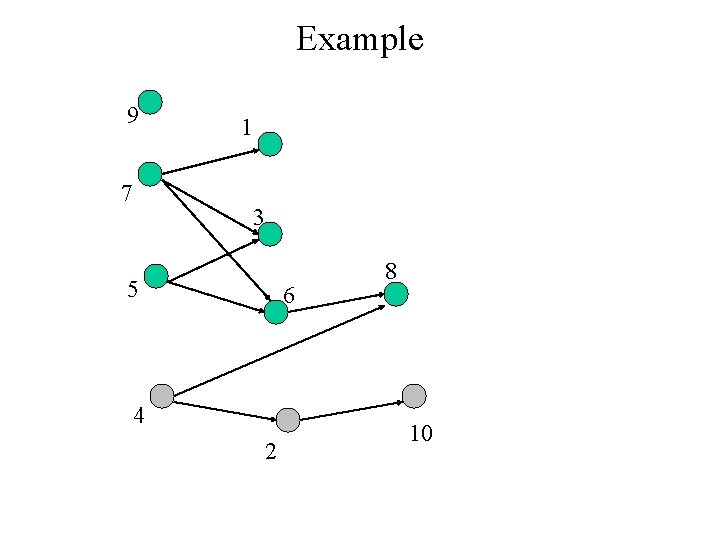
Example 9 7 1 3 5 6 4 2 8 10
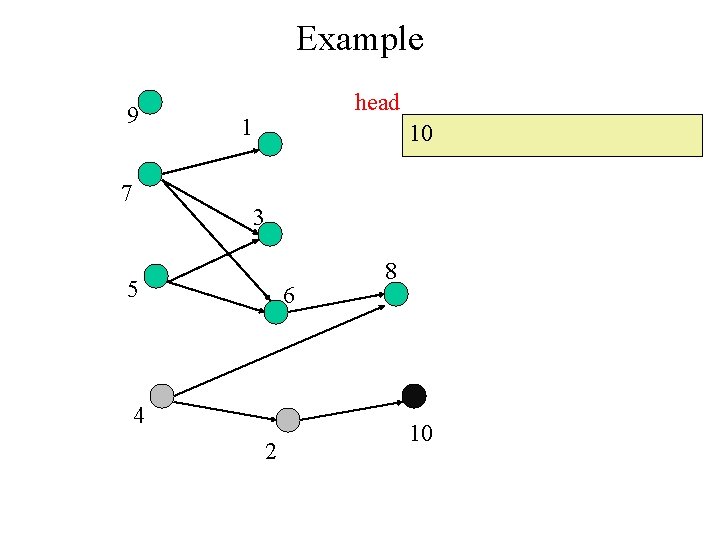
Example 9 7 head 1 10 3 5 6 4 2 8 10
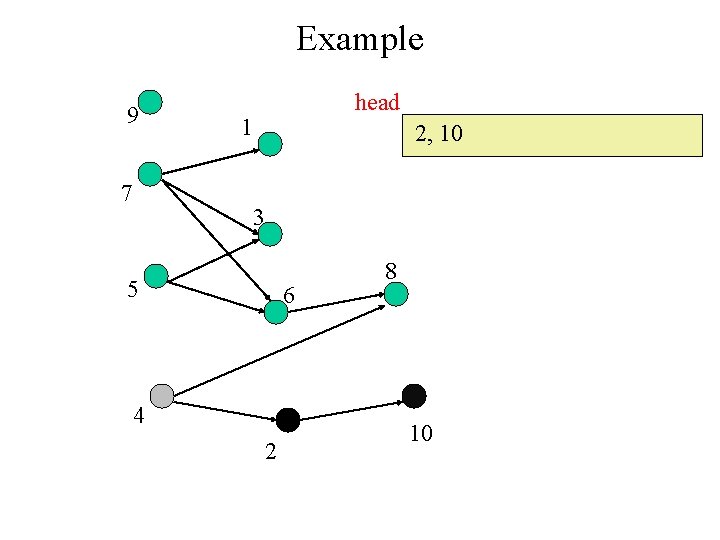
Example 9 7 head 1 2, 10 3 5 6 4 2 8 10
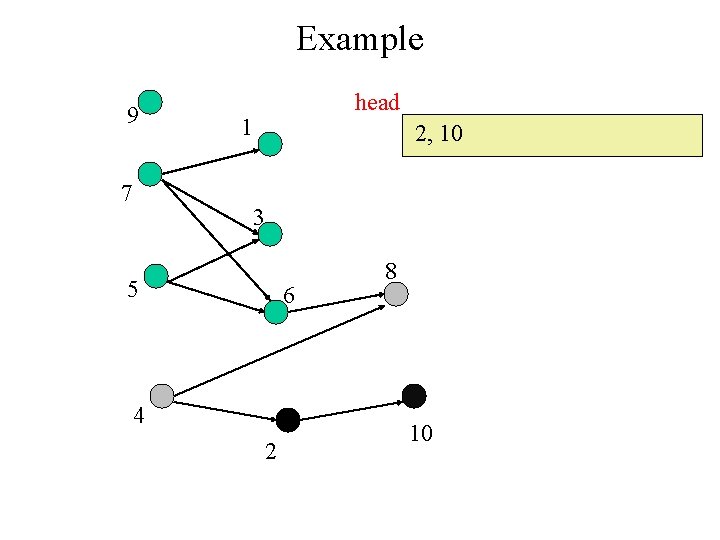
Example 9 7 head 1 2, 10 3 5 6 4 2 8 10
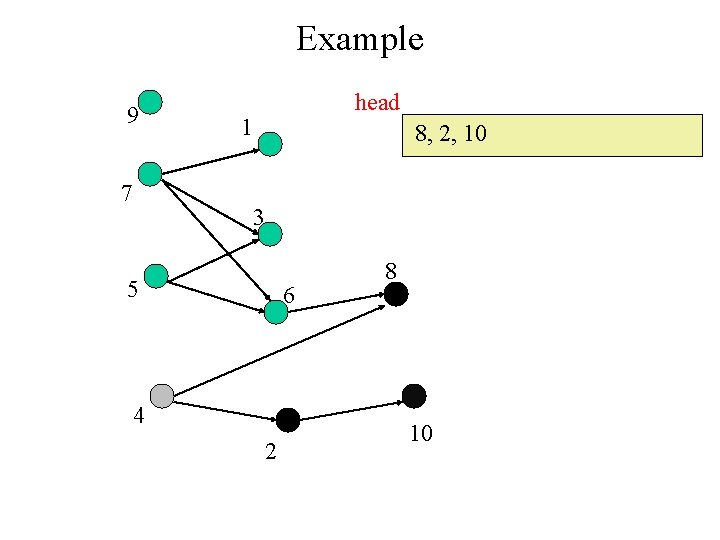
Example 9 7 head 1 8, 2, 10 3 5 6 4 2 8 10
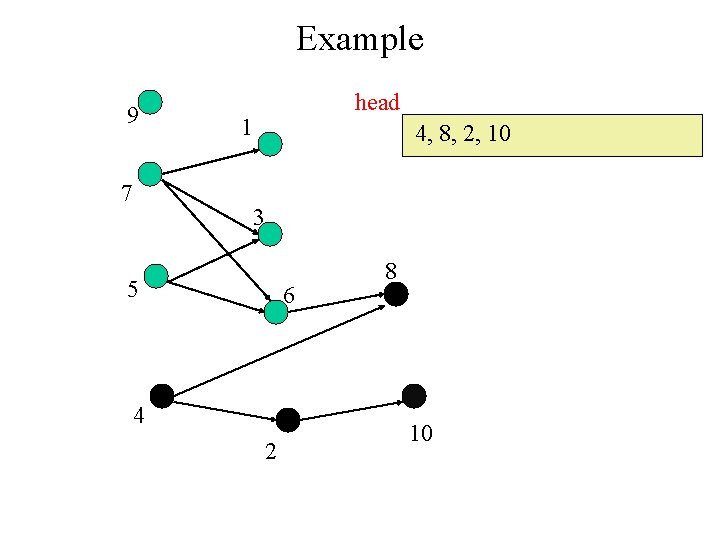
Example 9 7 head 1 4, 8, 2, 10 3 5 6 4 2 8 10
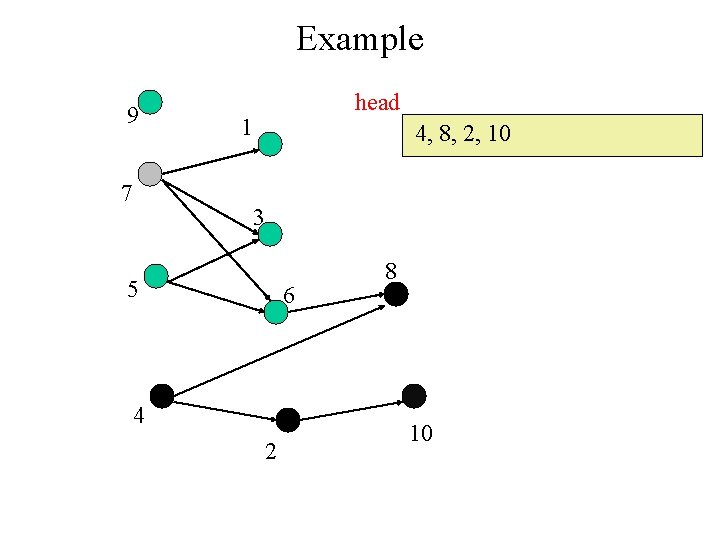
Example 9 7 head 1 4, 8, 2, 10 3 5 6 4 2 8 10
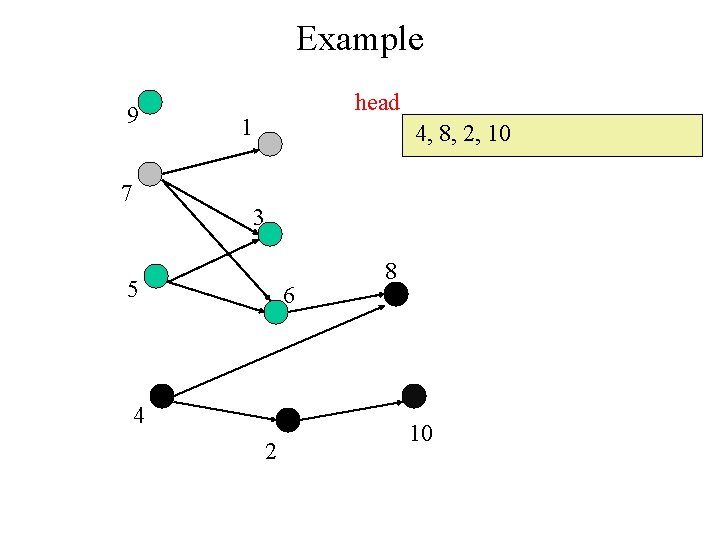
Example 9 7 head 1 4, 8, 2, 10 3 5 6 4 2 8 10
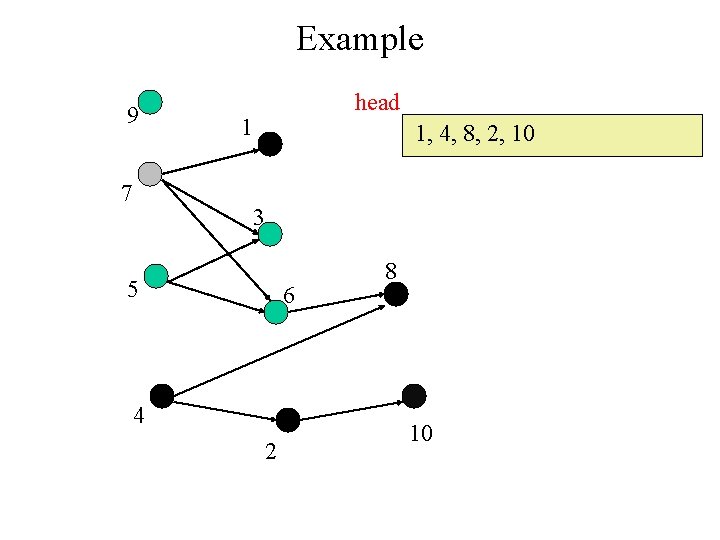
Example 9 7 head 1 1, 4, 8, 2, 10 3 5 6 4 2 8 10
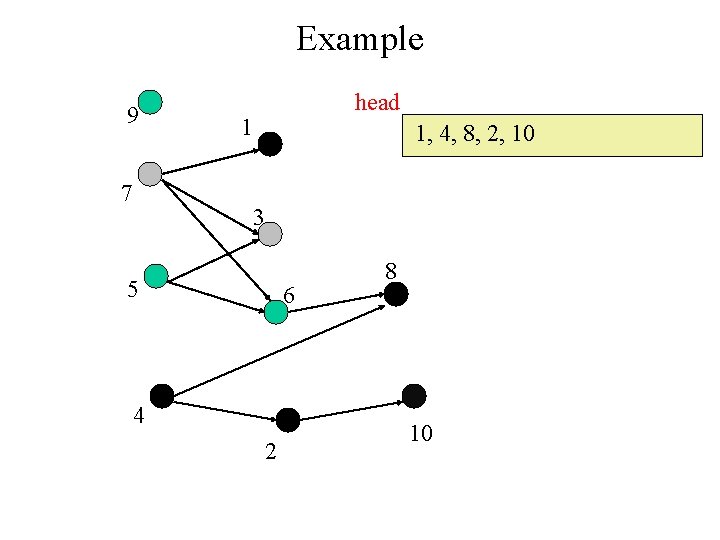
Example 9 7 head 1 1, 4, 8, 2, 10 3 5 6 4 2 8 10
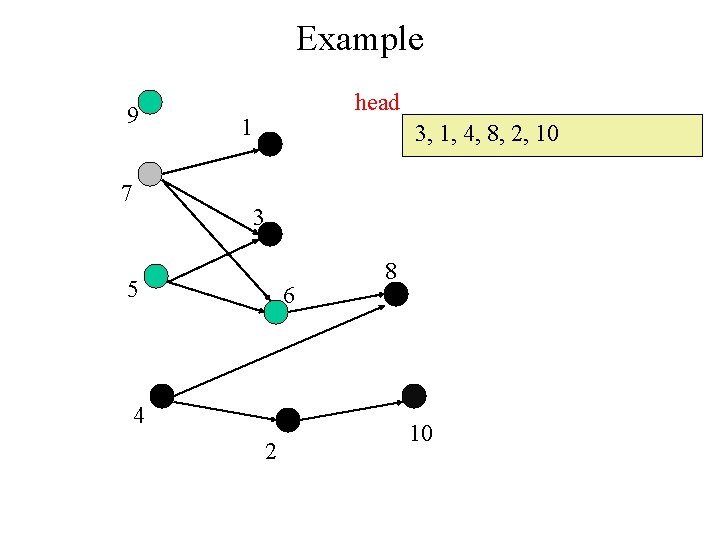
Example 9 7 head 1 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
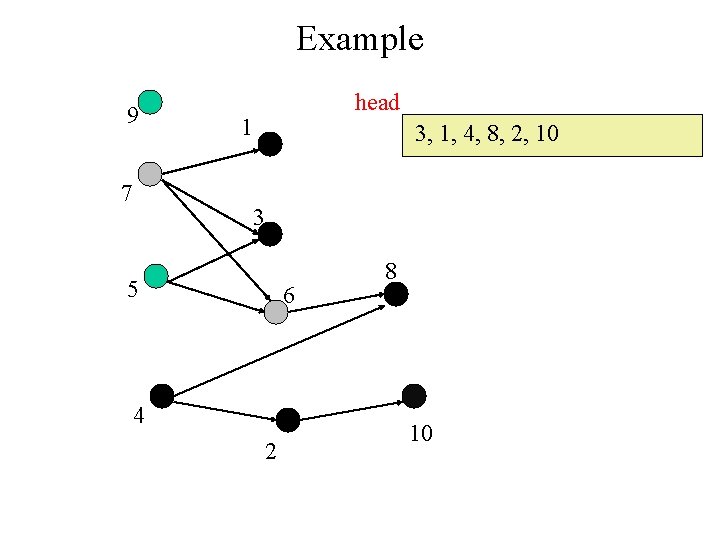
Example 9 7 head 1 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
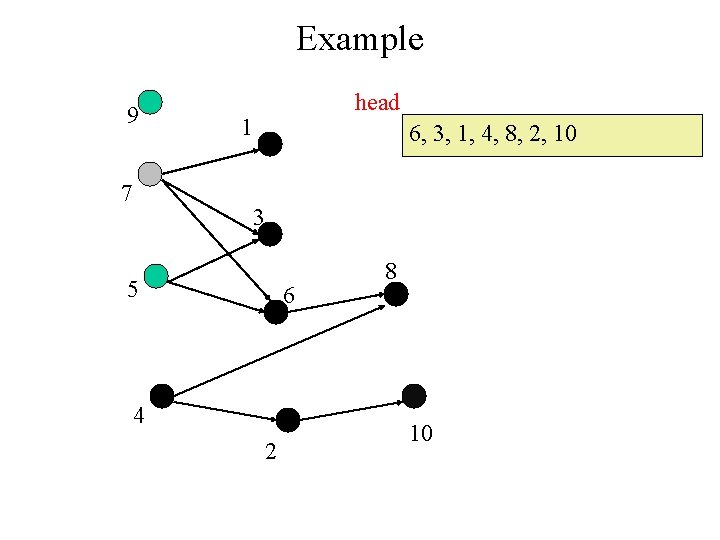
Example 9 7 head 1 6, 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
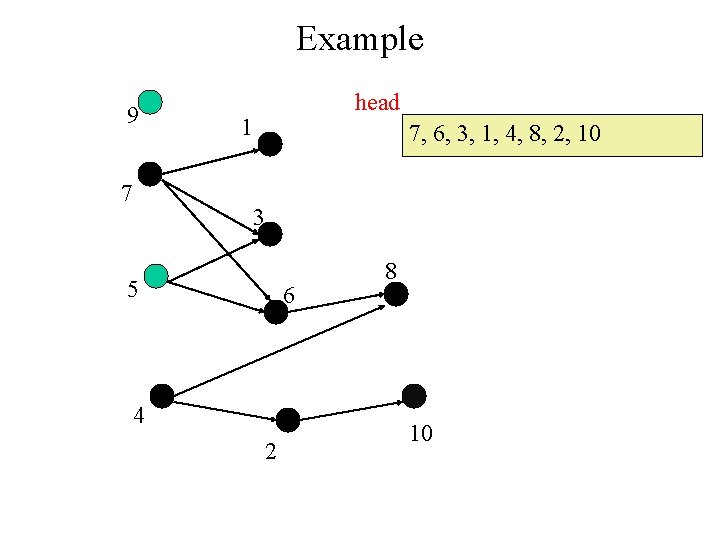
Example 9 7 head 1 7, 6, 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
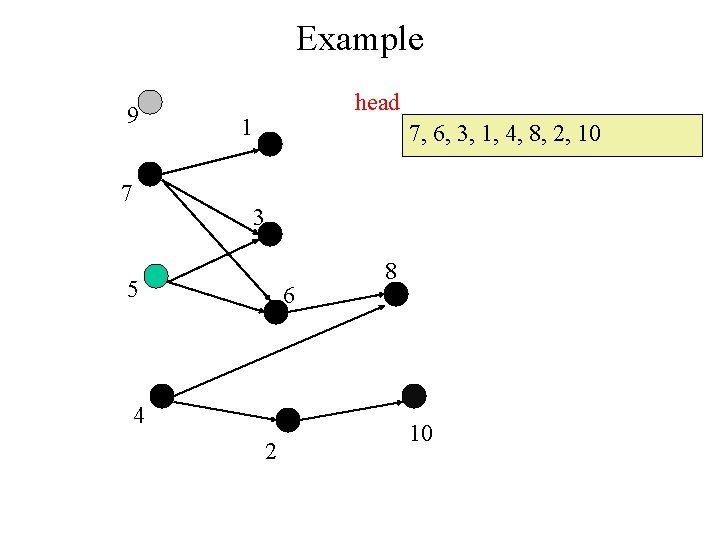
Example 9 7 head 1 7, 6, 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
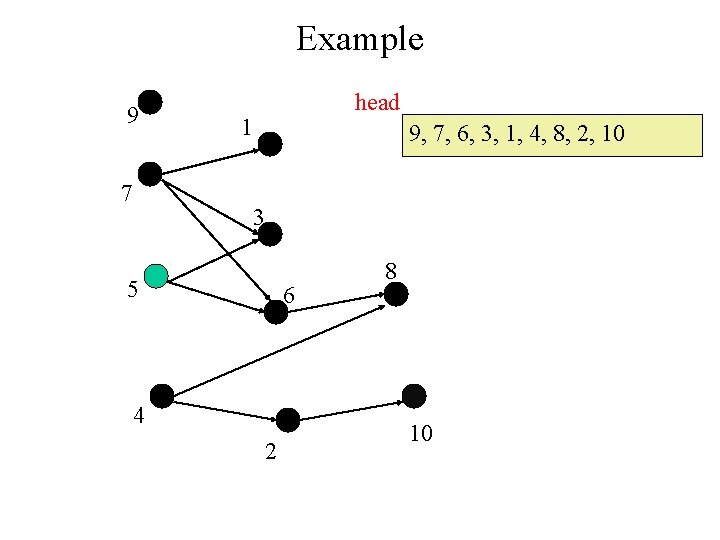
Example 9 7 head 1 9, 7, 6, 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
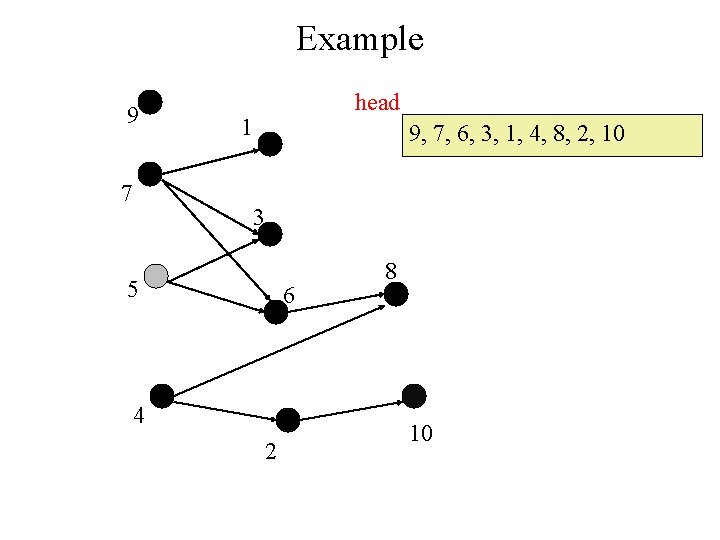
Example 9 7 head 1 9, 7, 6, 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
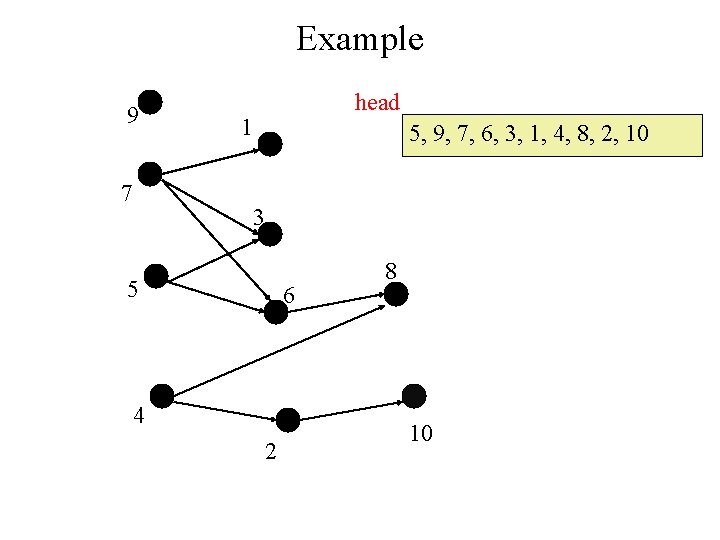
Example 9 7 head 1 5, 9, 7, 6, 3, 1, 4, 8, 2, 10 3 5 6 4 2 8 10
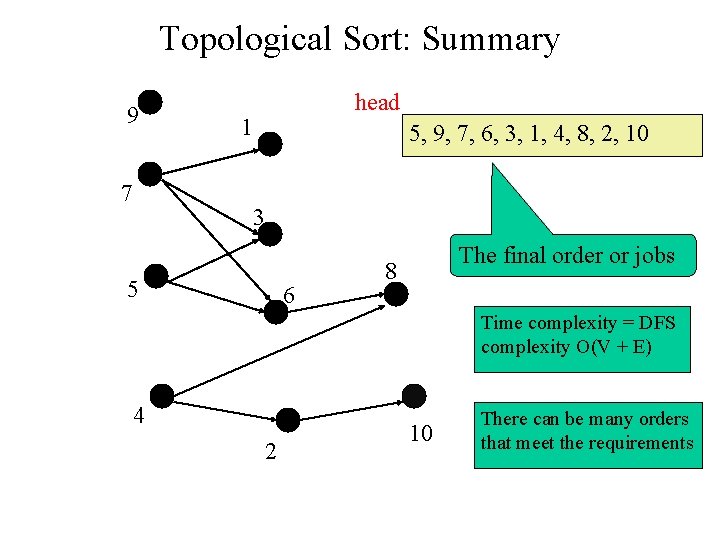
Topological Sort: Summary 9 7 head 1 5, 9, 7, 6, 3, 1, 4, 8, 2, 10 3 5 6 The final order or jobs 8 Time complexity = DFS complexity O(V + E) 4 2 10 There can be many orders that meet the requirements
Topological ordering
Topological sorting
Internal vs external sorting
Dags chen
Topological sort bfs
Topological sort calculator
Topological sort algorithm
Gscc graph
Difference between selection sort and bubble sort
Topological sort calculator
Topological sort
Search graph
Graph topological sort
Strongly connected components
Topological sort uses
Topological sort
Ioi camp
Dmca codeforces
Topological sort online
Lesson 1: analyzing a graph
A sorting technique is called stable if: *
Bubble sort 5-66
Quick sort merge sort
Bubble sort vs selection sort
Heap sort vs quick sort
Radix sort vs bucket sort
Pseudocode for bubble sort
Differentiate between bubble and quick sorting