Threads ICW Lecture 10 Tom Chothia Last Time
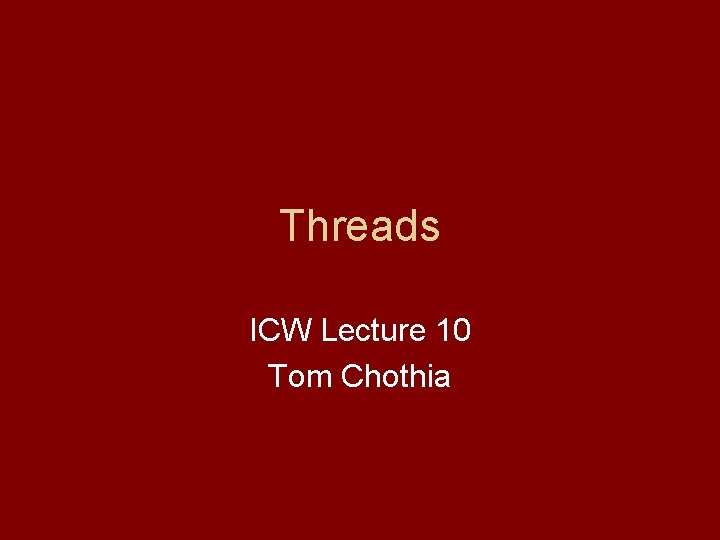
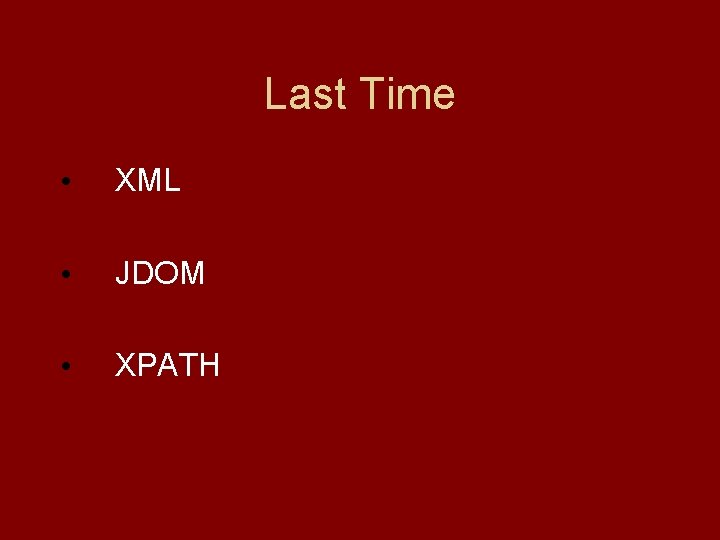
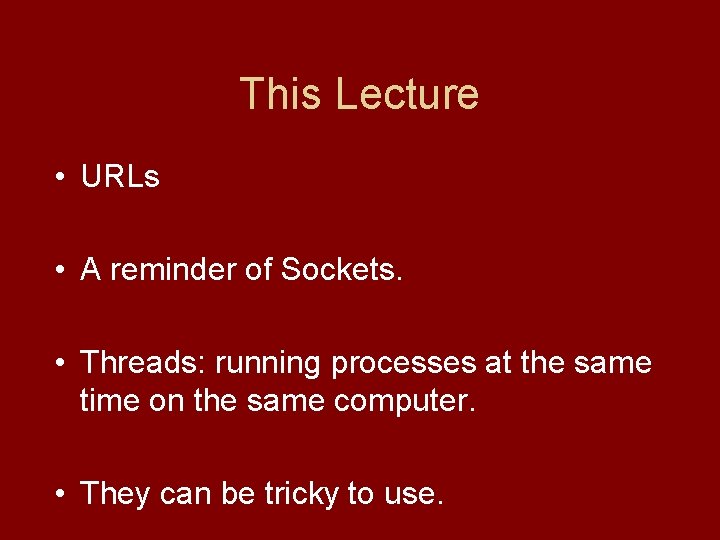
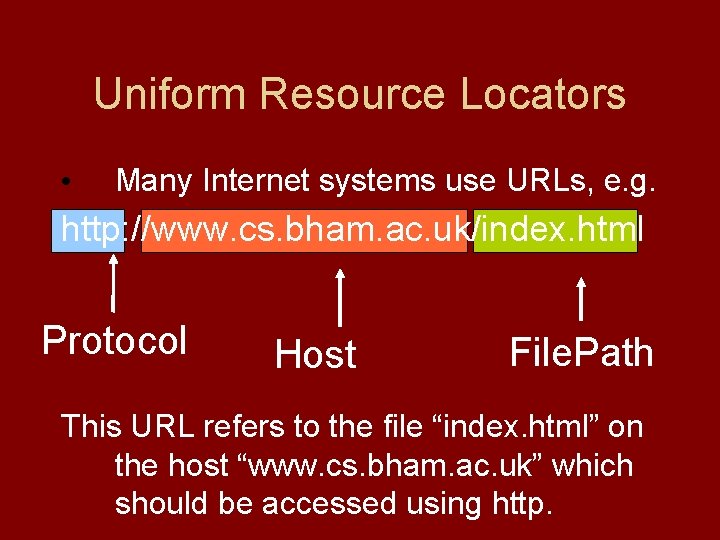
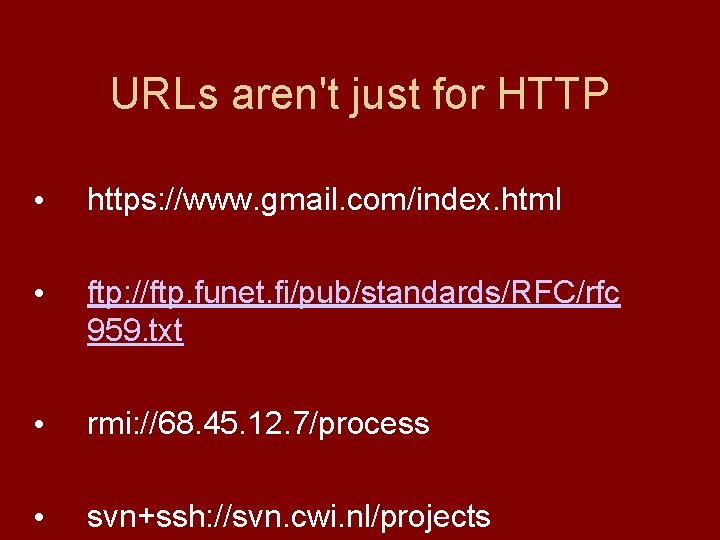
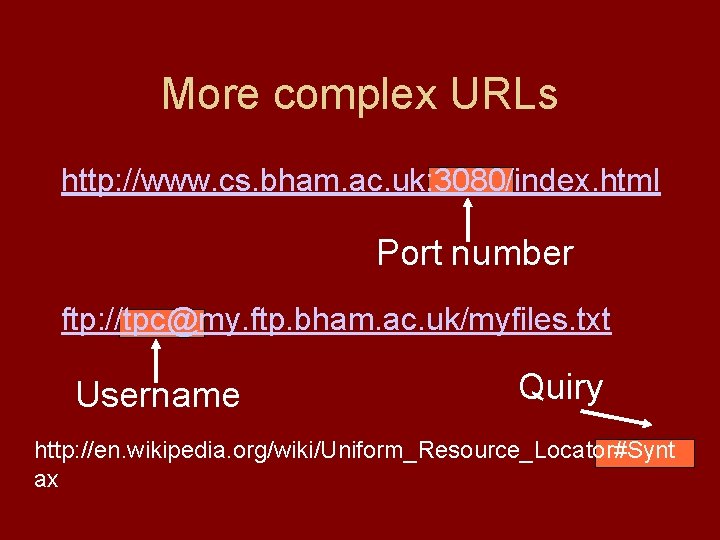
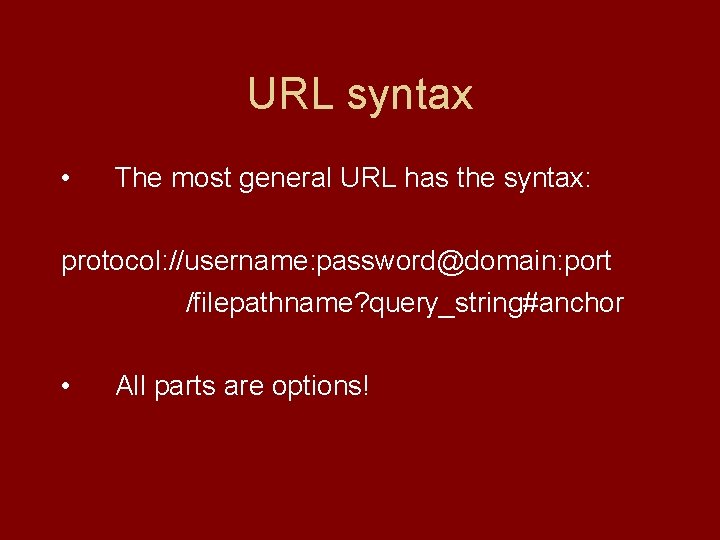
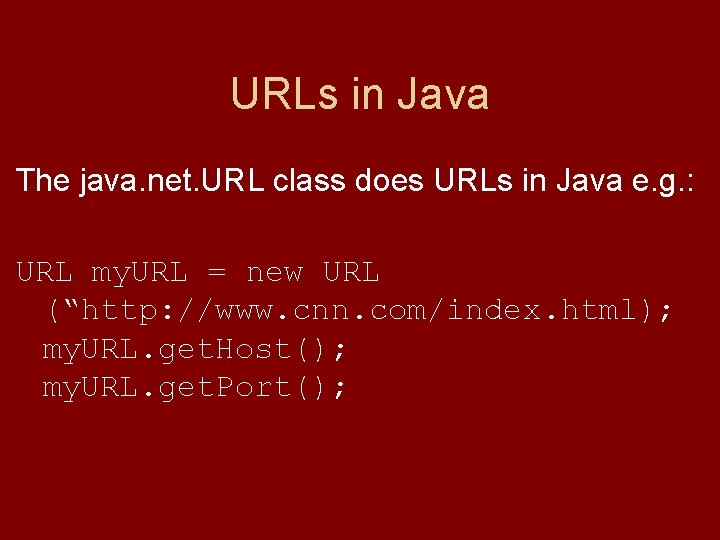
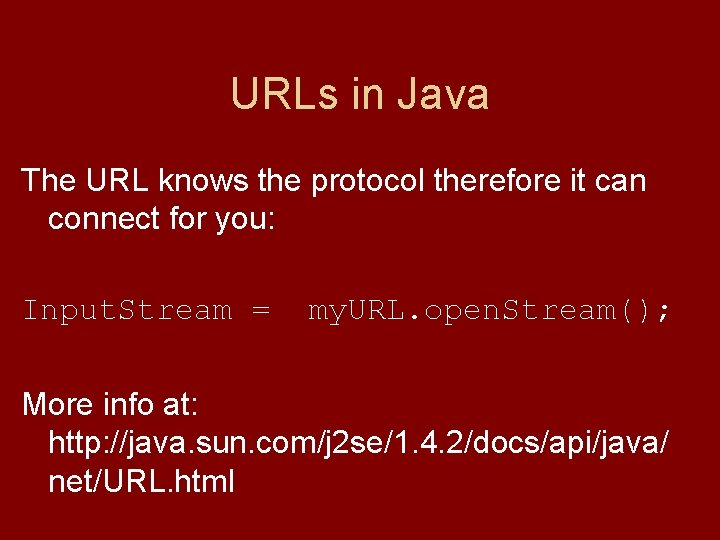
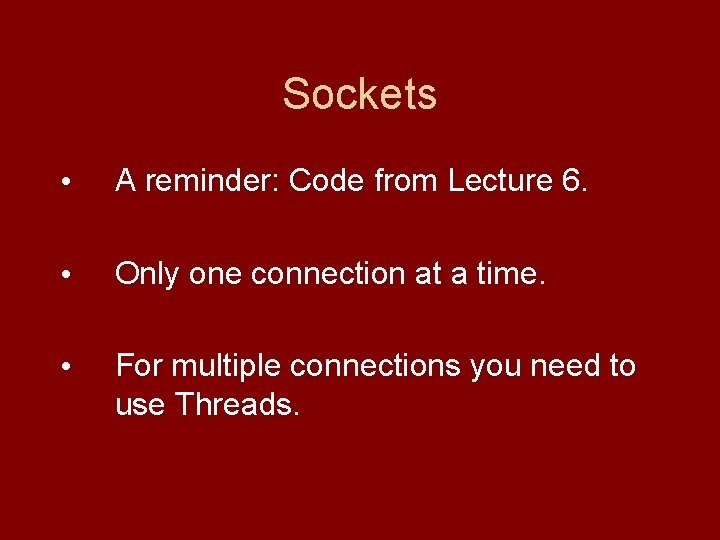
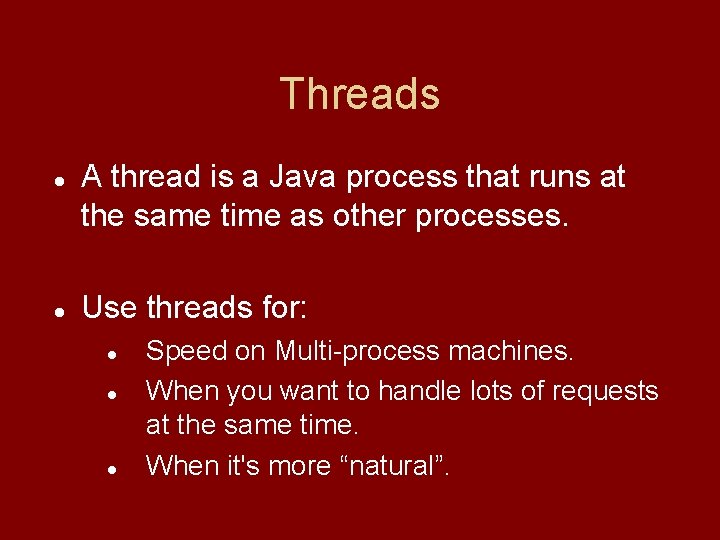
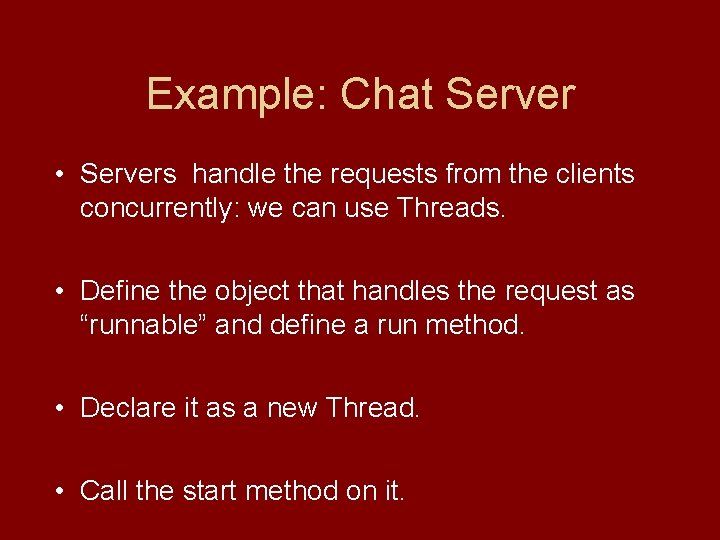
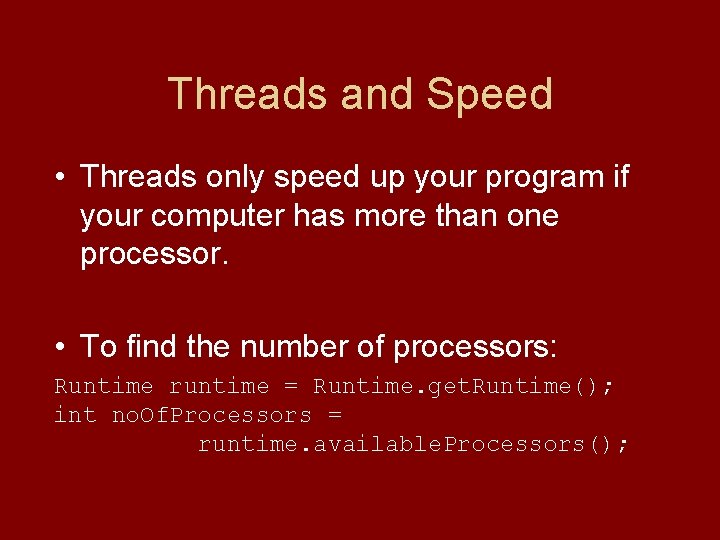
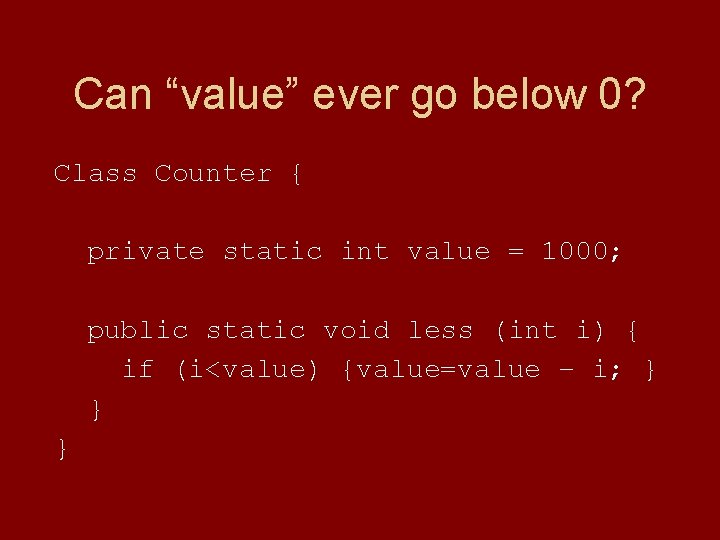
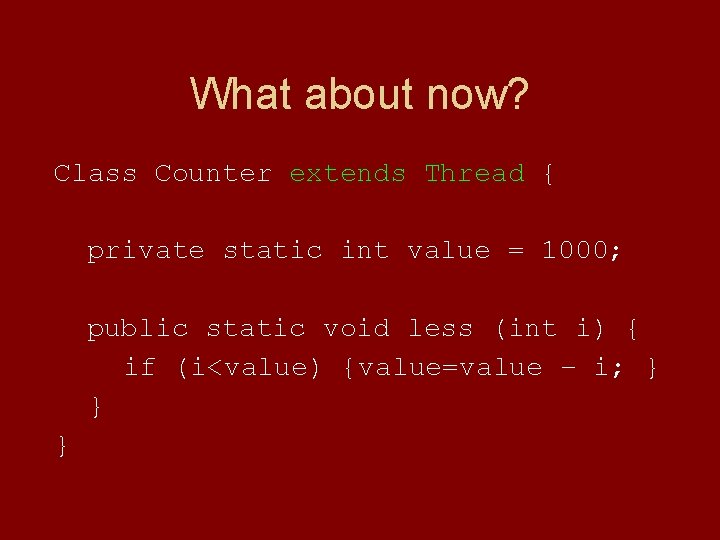
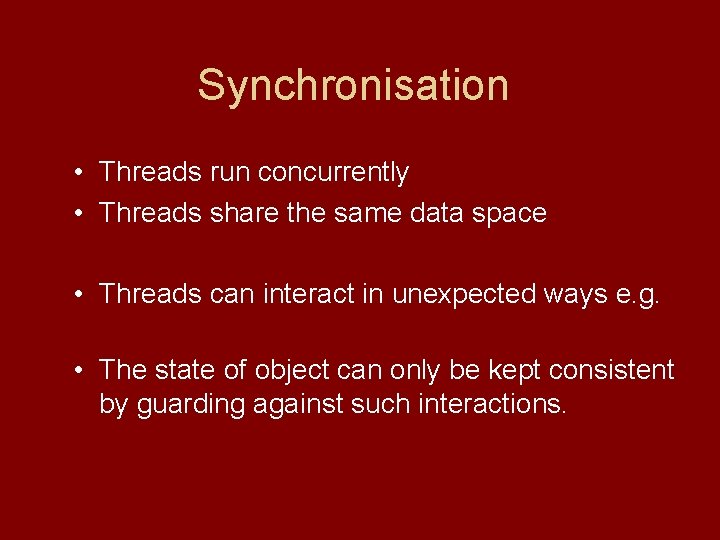
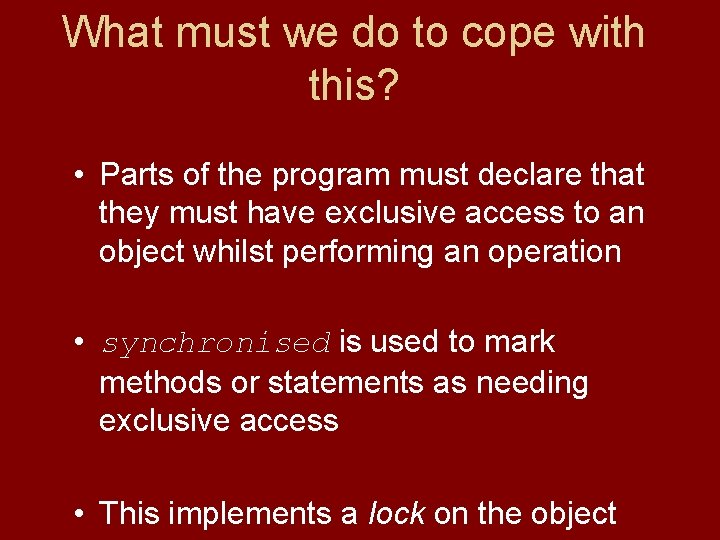
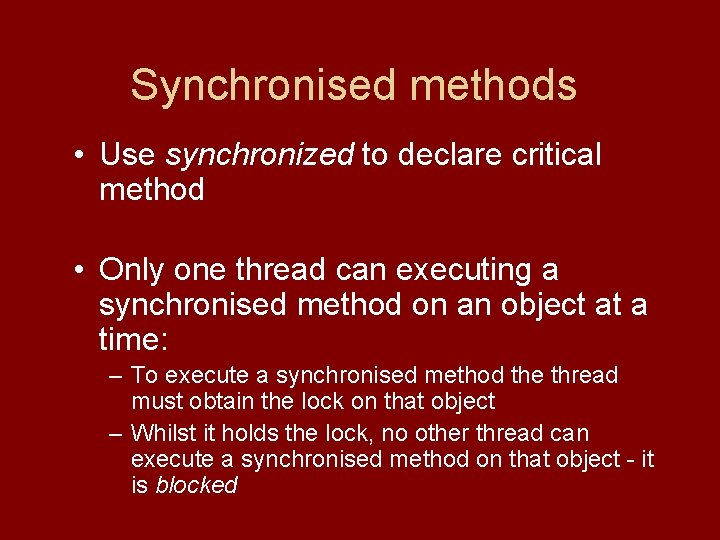
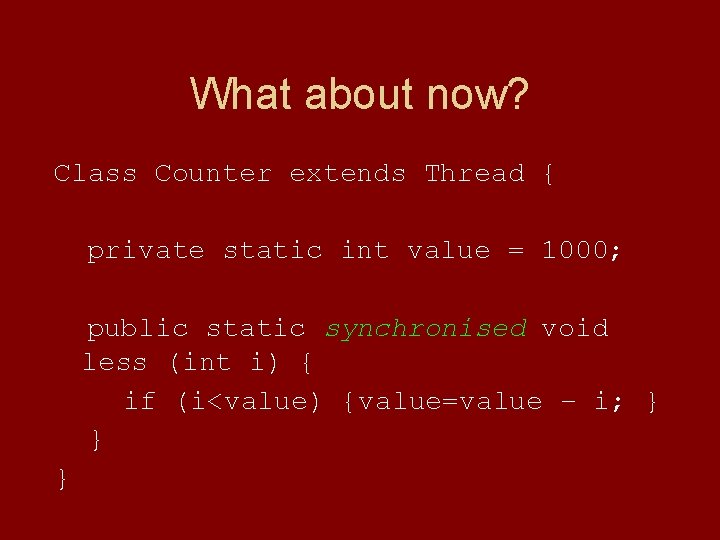
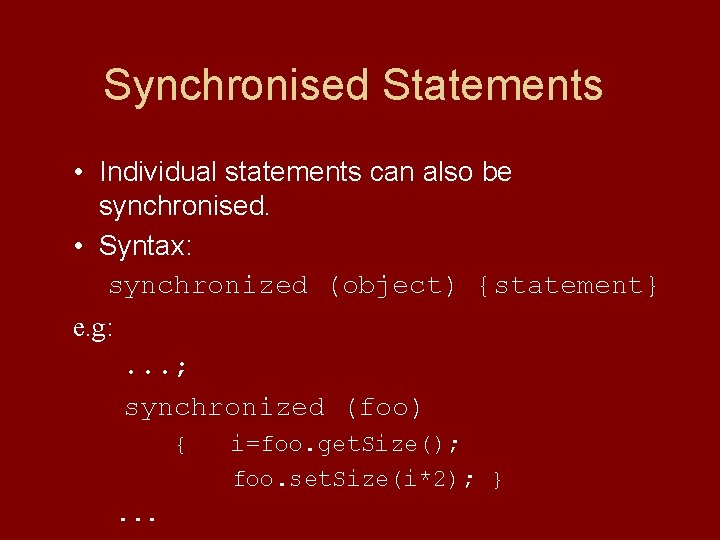
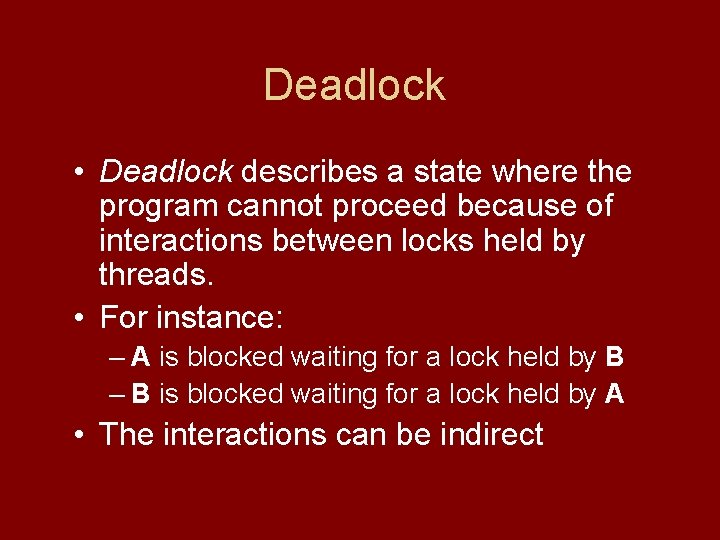
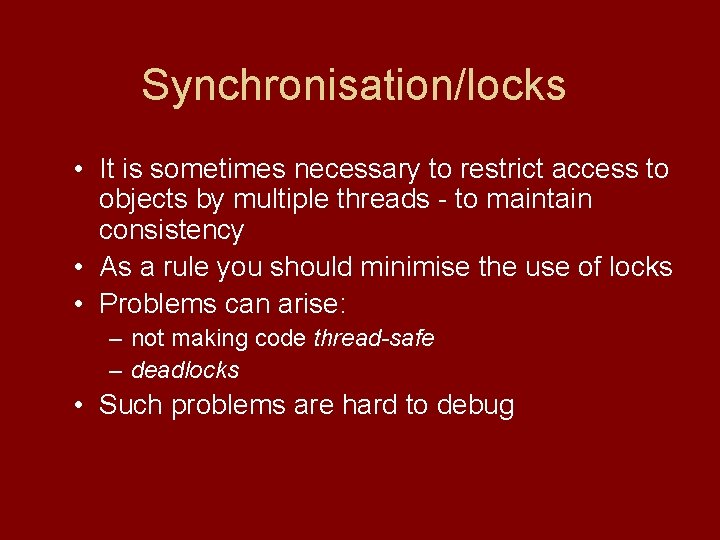
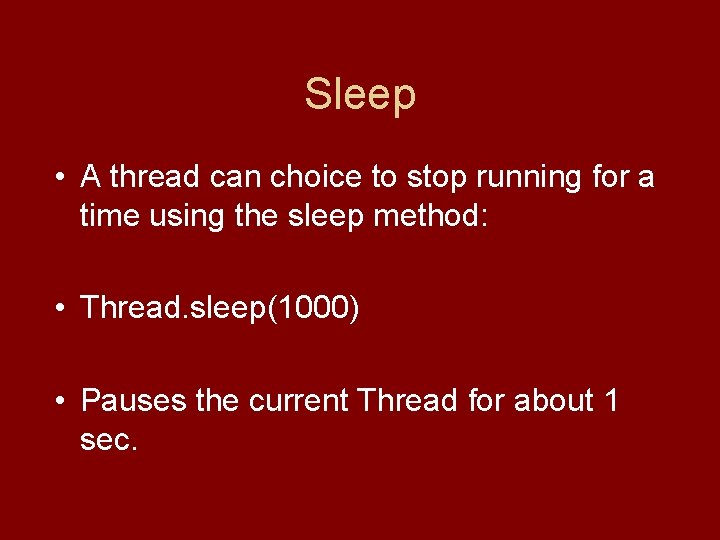
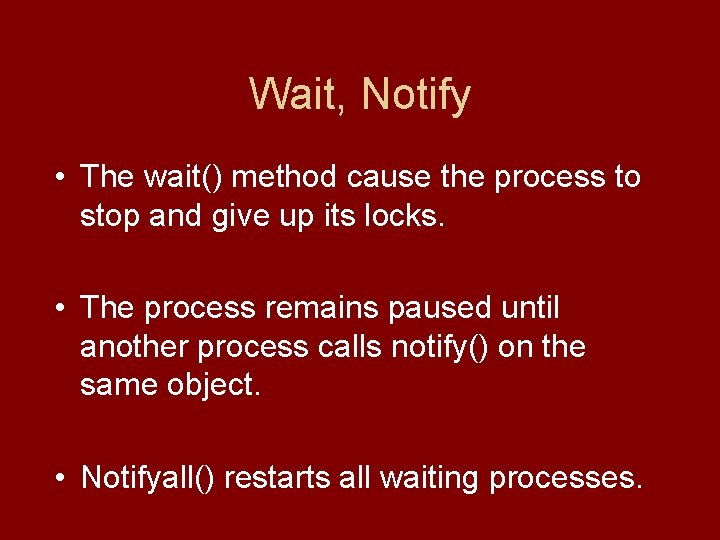
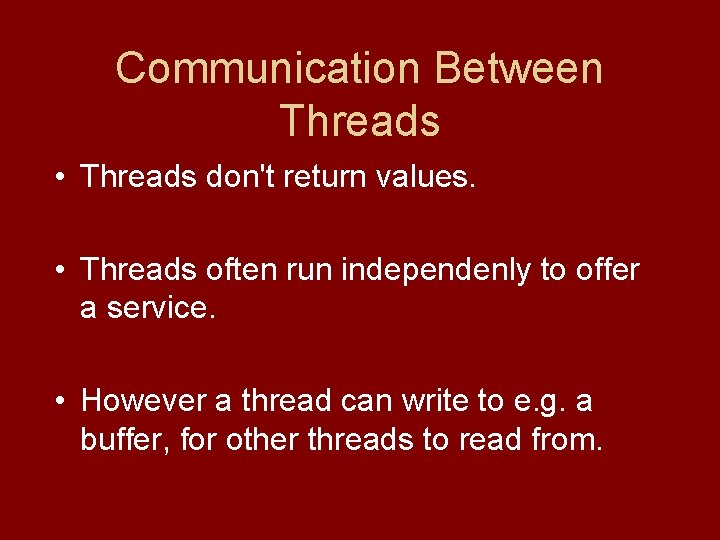
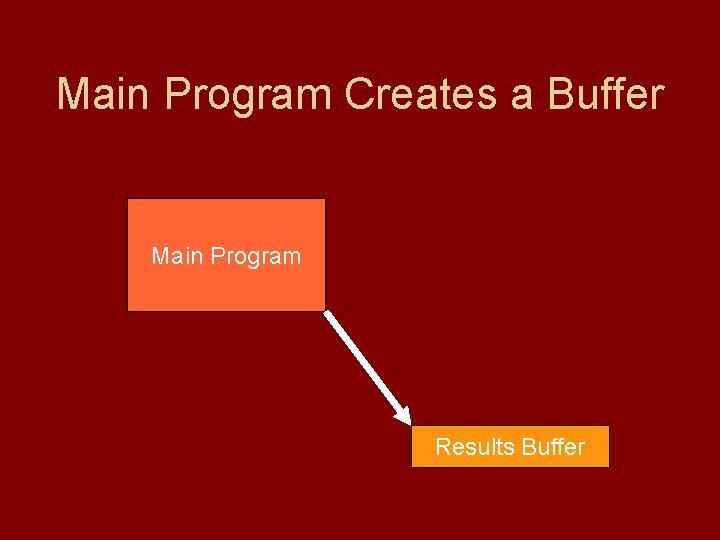
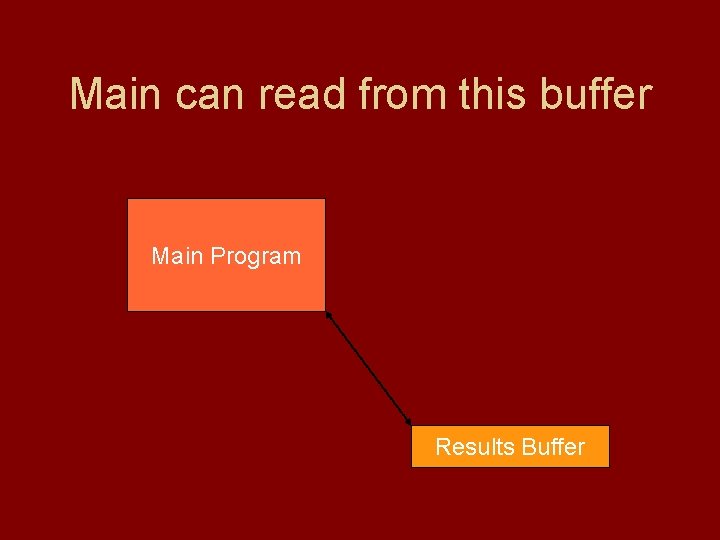
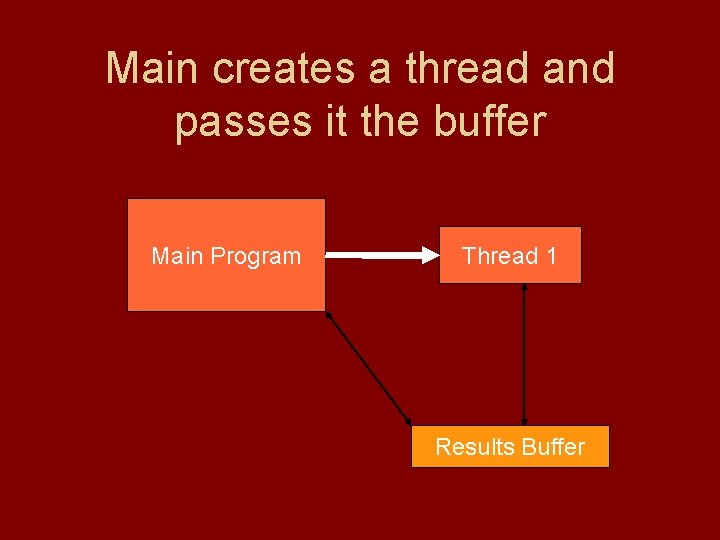
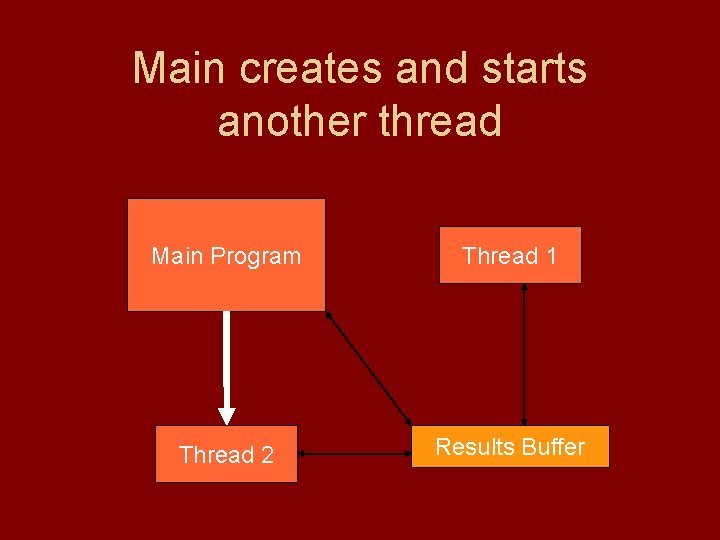
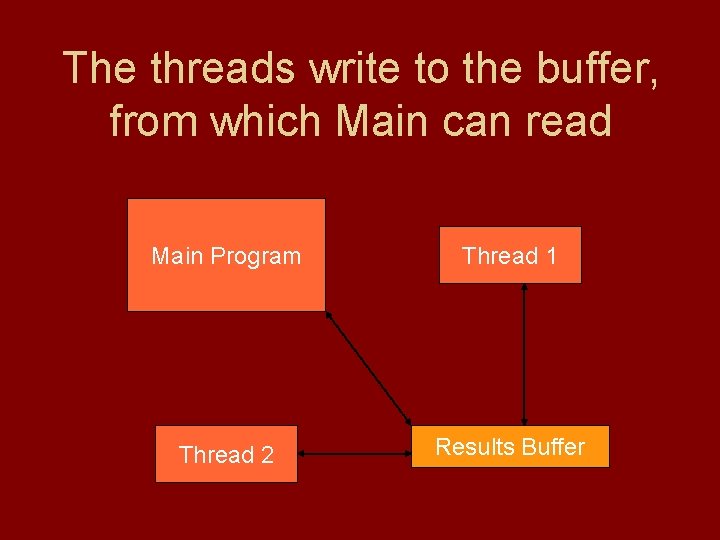
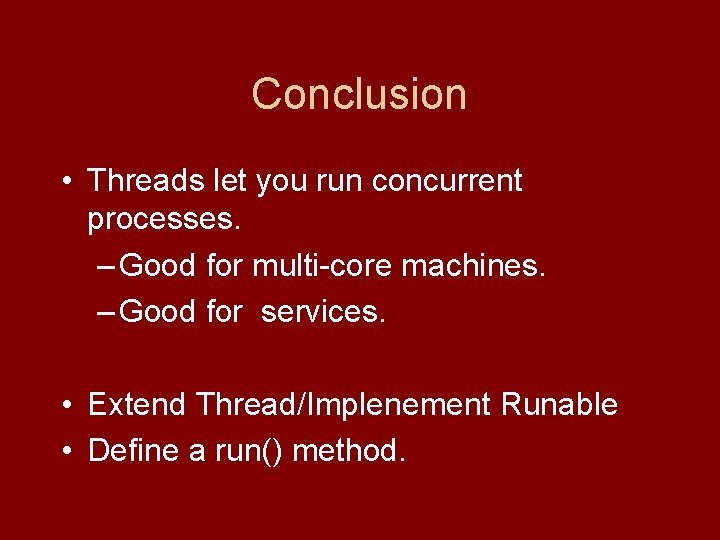
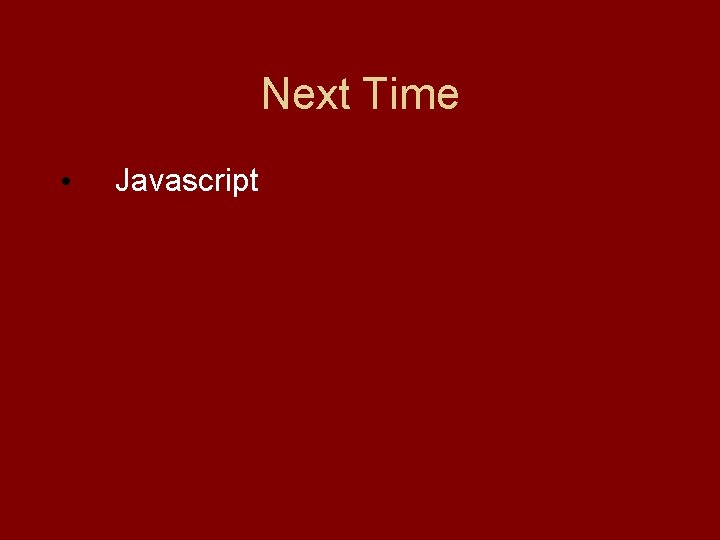
- Slides: 32
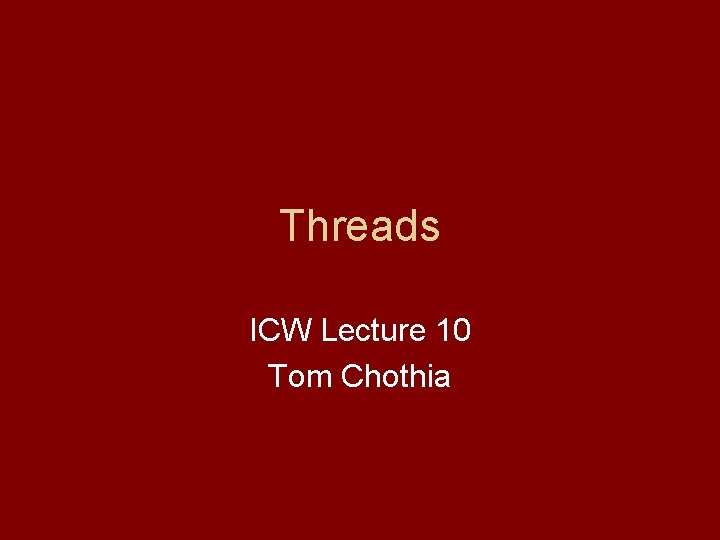
Threads ICW Lecture 10 Tom Chothia
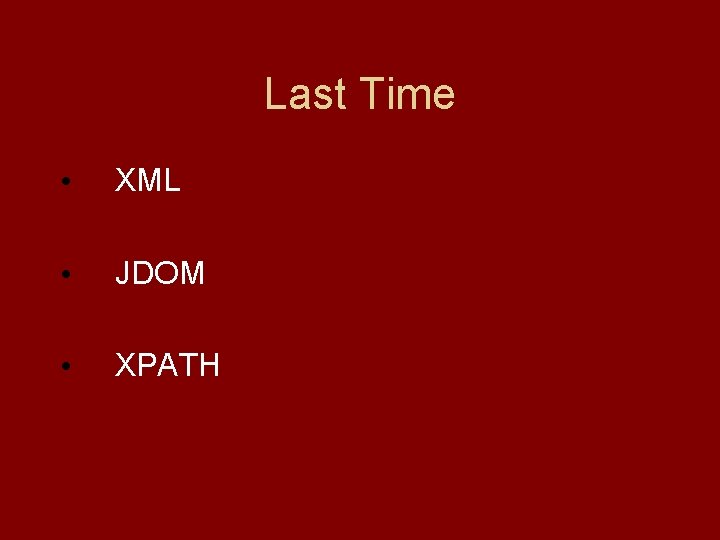
Last Time • XML • JDOM • XPATH
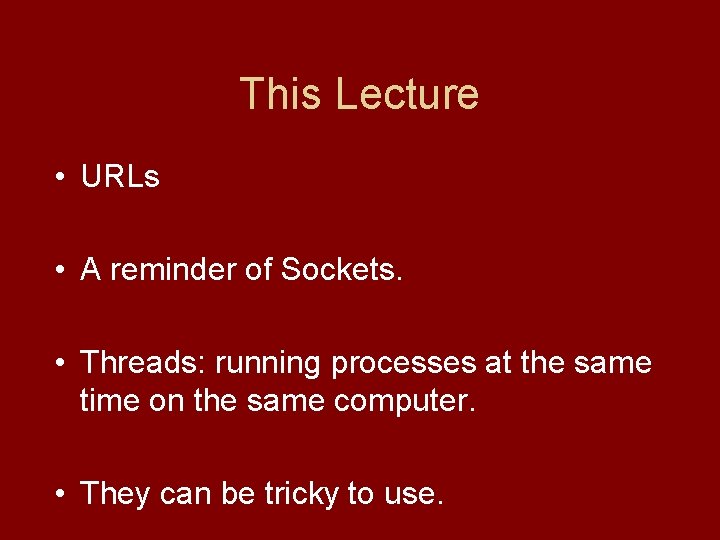
This Lecture • URLs • A reminder of Sockets. • Threads: running processes at the same time on the same computer. • They can be tricky to use.
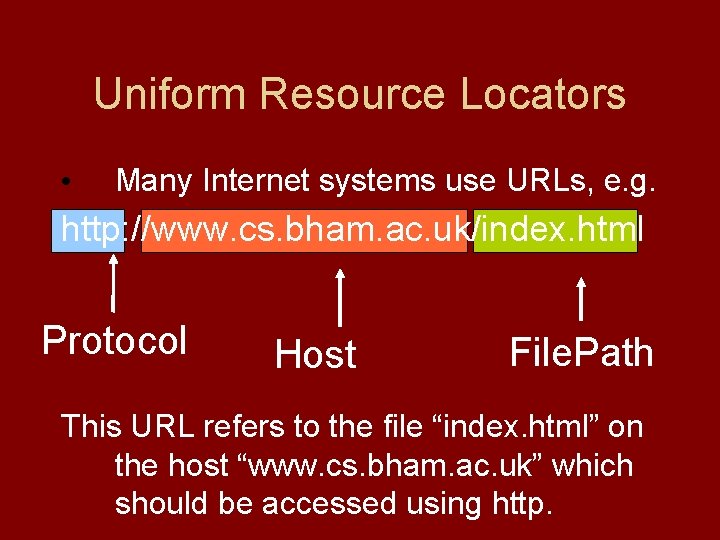
Uniform Resource Locators • Many Internet systems use URLs, e. g. http: //www. cs. bham. ac. uk/index. html Protocol Host File. Path This URL refers to the file “index. html” on the host “www. cs. bham. ac. uk” which should be accessed using http.
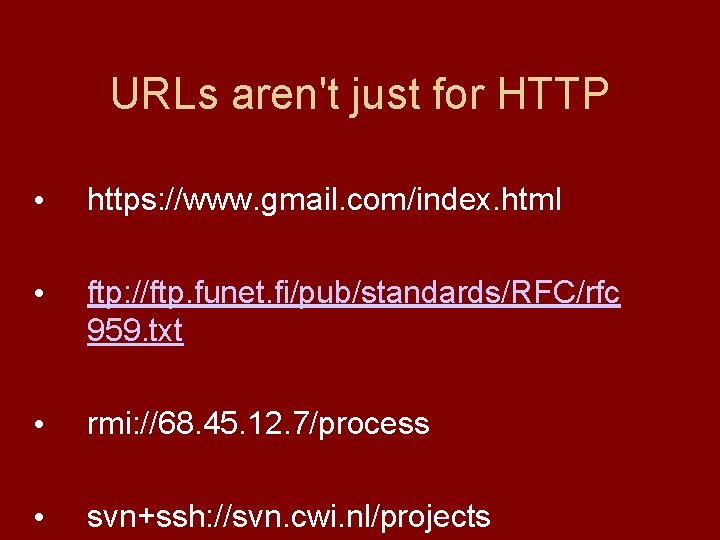
URLs aren't just for HTTP • https: //www. gmail. com/index. html • ftp: //ftp. funet. fi/pub/standards/RFC/rfc 959. txt • rmi: //68. 45. 12. 7/process • svn+ssh: //svn. cwi. nl/projects
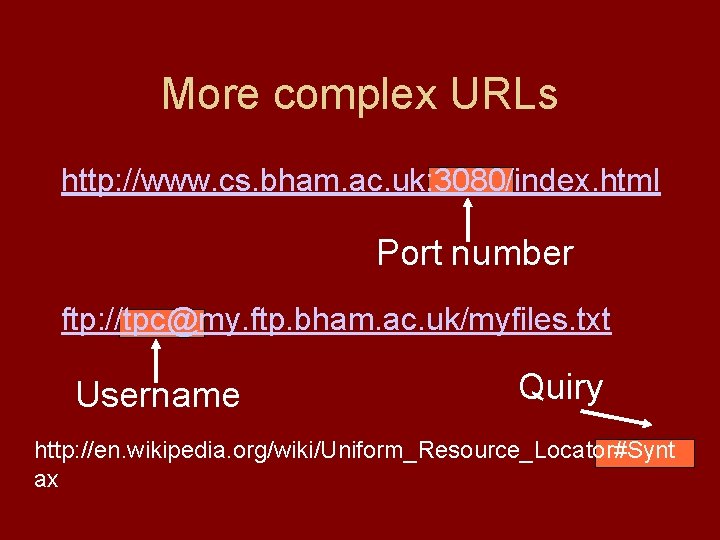
More complex URLs http: //www. cs. bham. ac. uk: 3080/index. html Port number ftp: //tpc@my. ftp. bham. ac. uk/myfiles. txt Username Quiry http: //en. wikipedia. org/wiki/Uniform_Resource_Locator#Synt ax
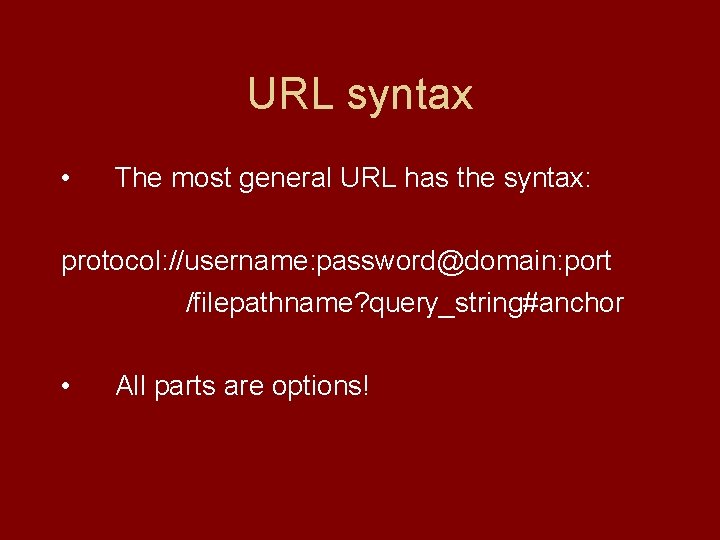
URL syntax • The most general URL has the syntax: protocol: //username: password@domain: port /filepathname? query_string#anchor • All parts are options!
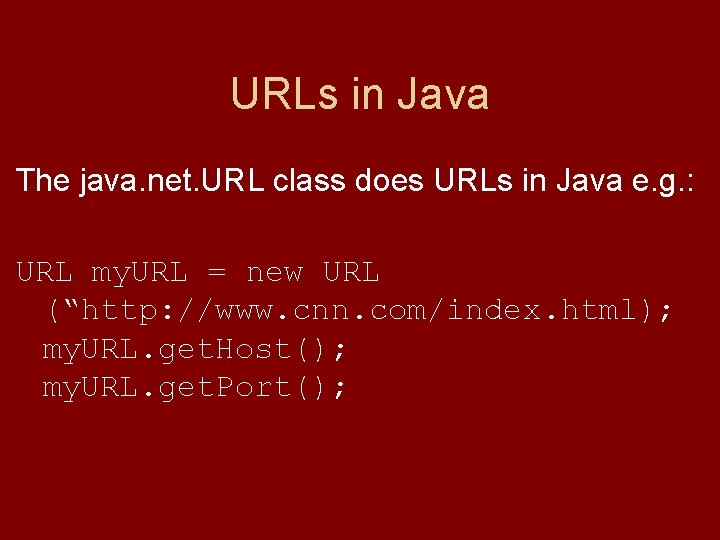
URLs in Java The java. net. URL class does URLs in Java e. g. : URL my. URL = new URL (“http: //www. cnn. com/index. html); my. URL. get. Host(); my. URL. get. Port();
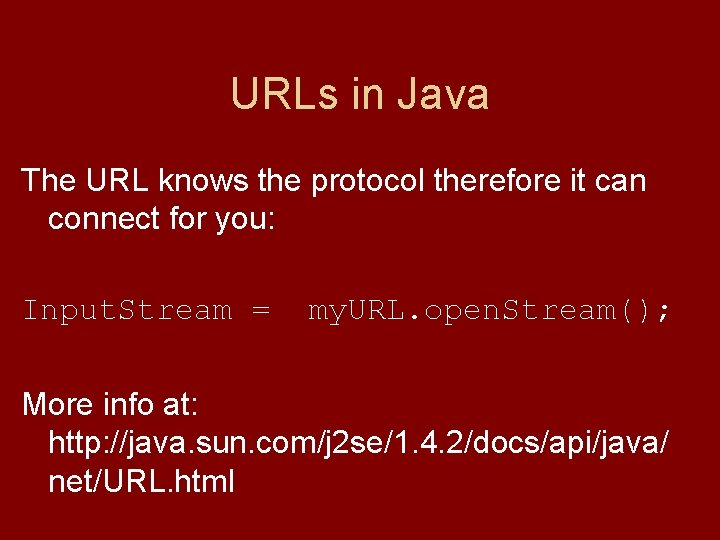
URLs in Java The URL knows the protocol therefore it can connect for you: Input. Stream = my. URL. open. Stream(); More info at: http: //java. sun. com/j 2 se/1. 4. 2/docs/api/java/ net/URL. html
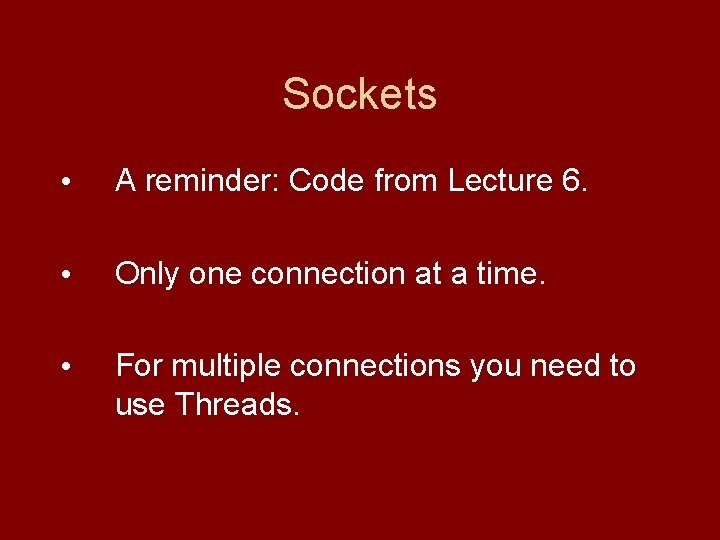
Sockets • A reminder: Code from Lecture 6. • Only one connection at a time. • For multiple connections you need to use Threads.
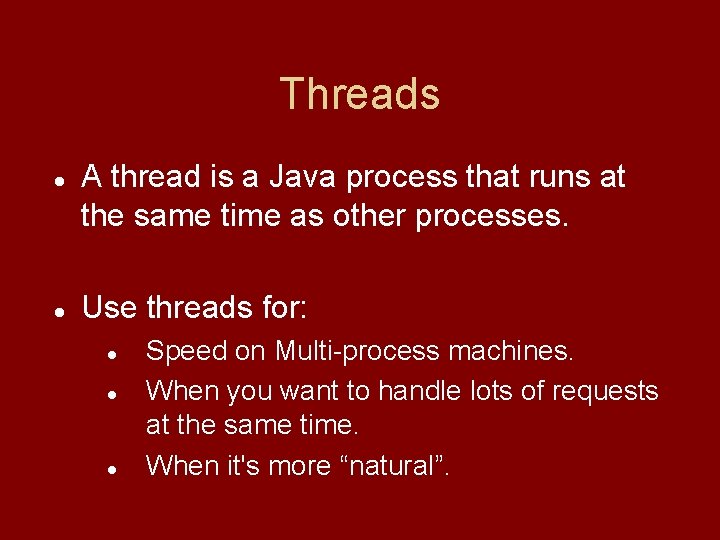
Threads A thread is a Java process that runs at the same time as other processes. Use threads for: Speed on Multi-process machines. When you want to handle lots of requests at the same time. When it's more “natural”.
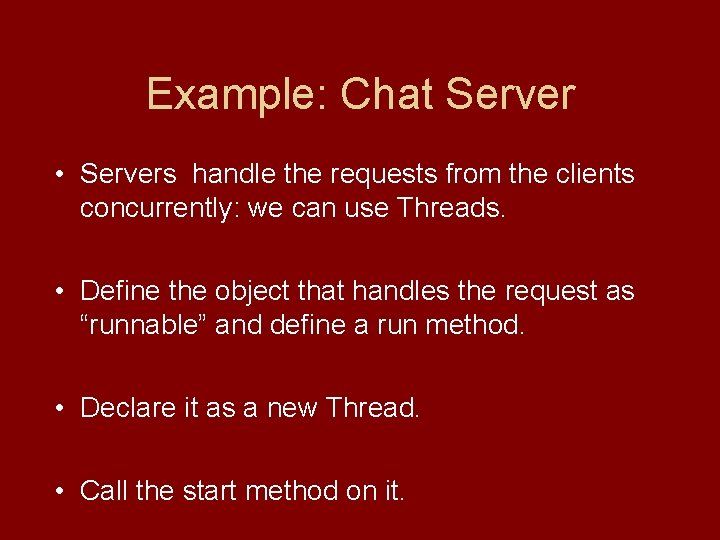
Example: Chat Server • Servers handle the requests from the clients concurrently: we can use Threads. • Define the object that handles the request as “runnable” and define a run method. • Declare it as a new Thread. • Call the start method on it.
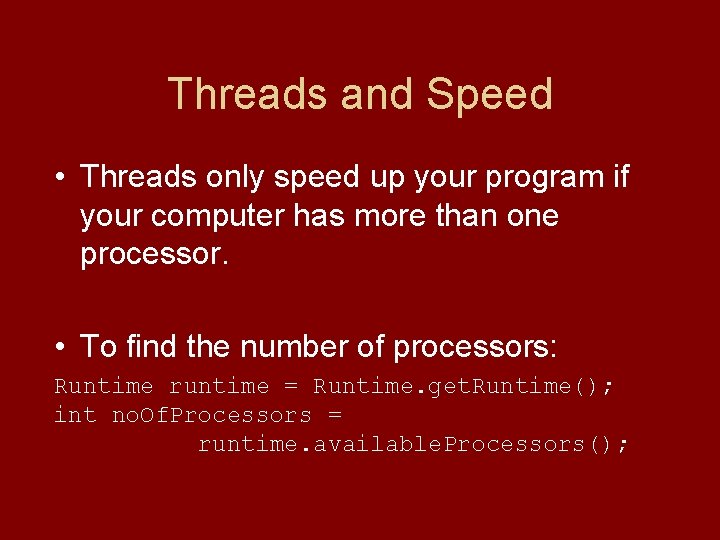
Threads and Speed • Threads only speed up your program if your computer has more than one processor. • To find the number of processors: Runtime runtime = Runtime. get. Runtime(); int no. Of. Processors = runtime. available. Processors();
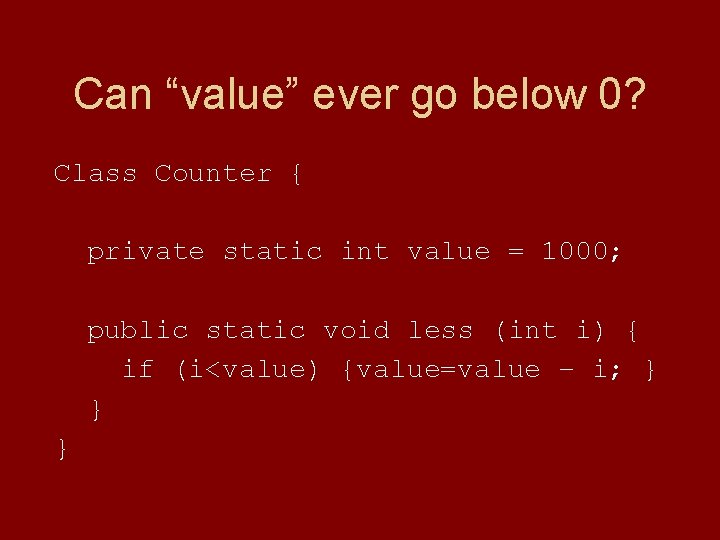
Can “value” ever go below 0? Class Counter { private static int value = 1000; public static void less (int i) { if (i<value) {value=value – i; } } }
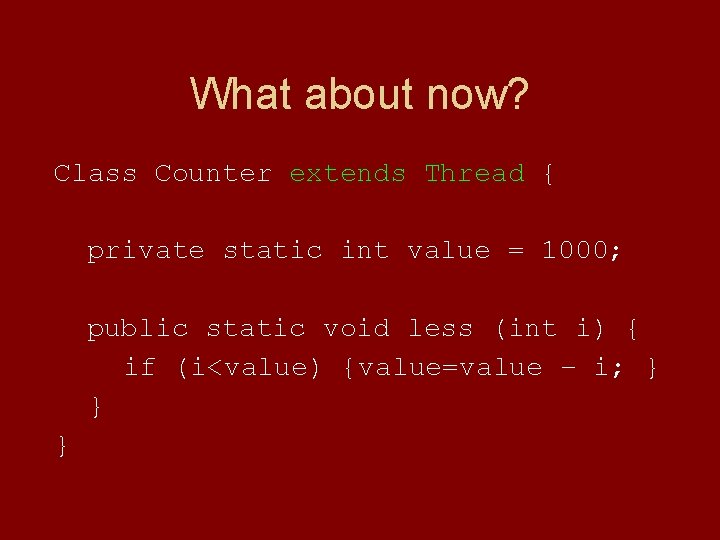
What about now? Class Counter extends Thread { private static int value = 1000; public static void less (int i) { if (i<value) {value=value – i; } } }
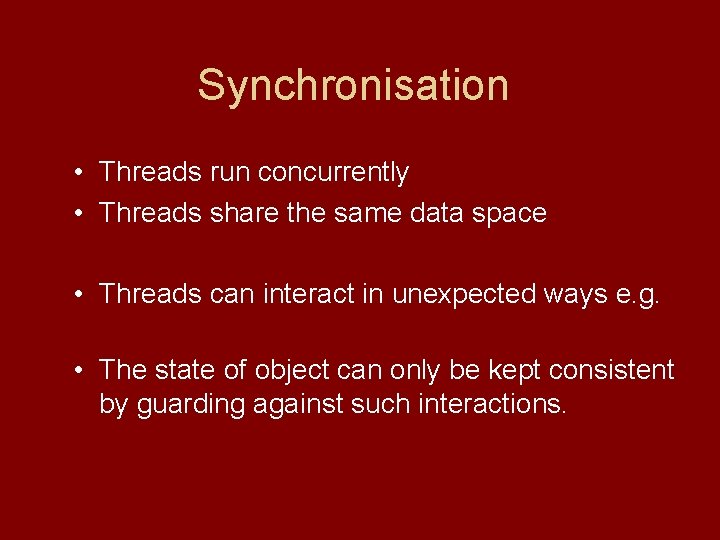
Synchronisation • Threads run concurrently • Threads share the same data space • Threads can interact in unexpected ways e. g. • The state of object can only be kept consistent by guarding against such interactions.
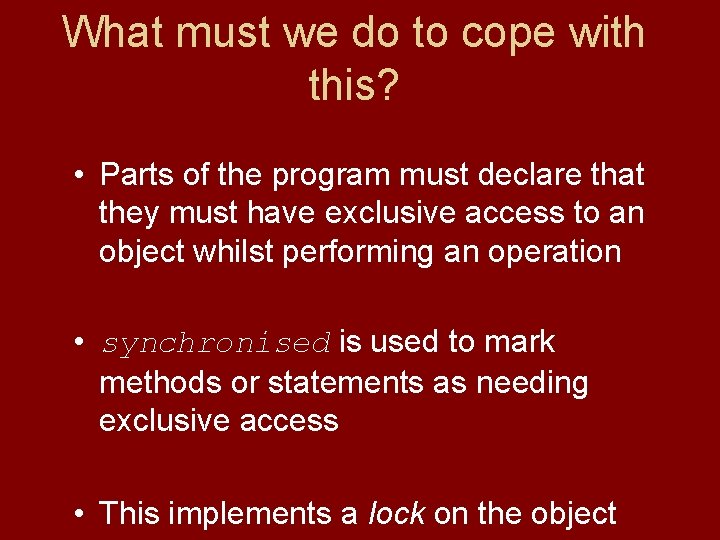
What must we do to cope with this? • Parts of the program must declare that they must have exclusive access to an object whilst performing an operation • synchronised is used to mark methods or statements as needing exclusive access • This implements a lock on the object
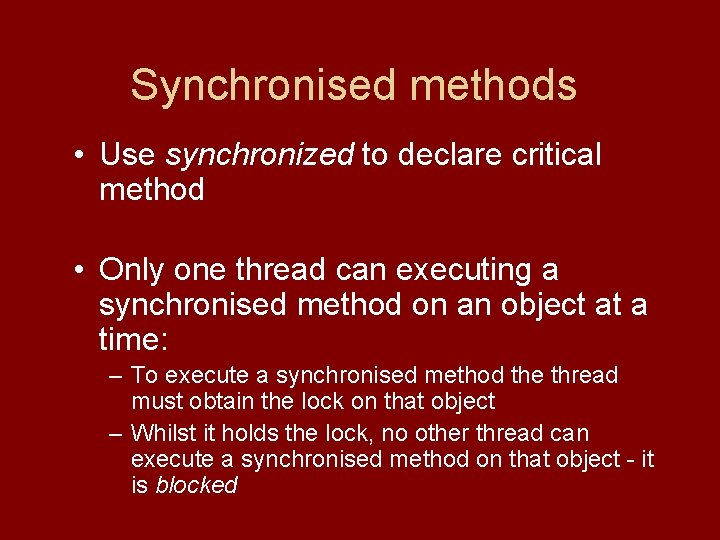
Synchronised methods • Use synchronized to declare critical method • Only one thread can executing a synchronised method on an object at a time: – To execute a synchronised method the thread must obtain the lock on that object – Whilst it holds the lock, no other thread can execute a synchronised method on that object - it is blocked
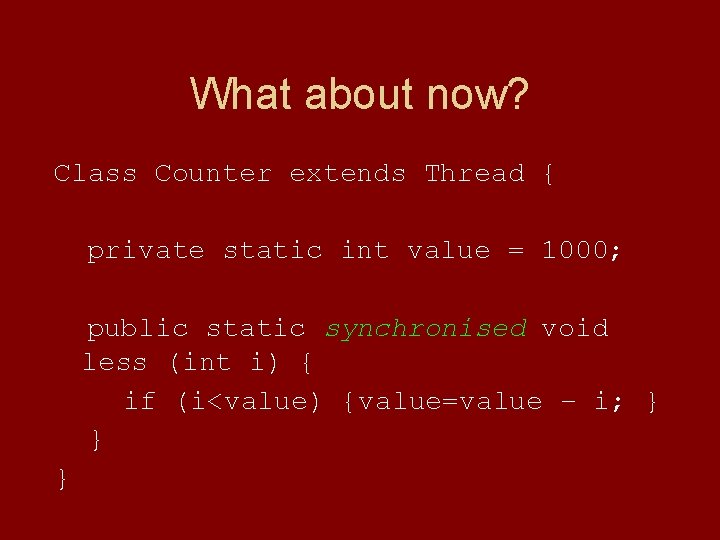
What about now? Class Counter extends Thread { private static int value = 1000; public static synchronised void less (int i) { if (i<value) {value=value – i; } } }
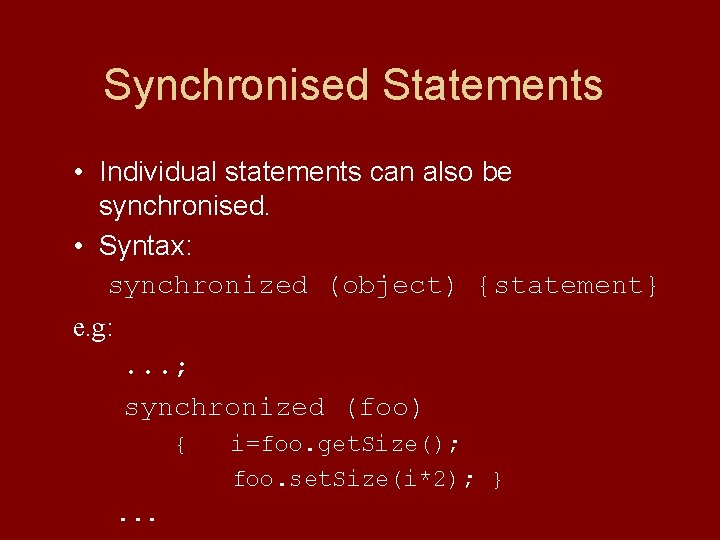
Synchronised Statements • Individual statements can also be synchronised. • Syntax: synchronized (object) {statement} e. g: . . . ; synchronized (foo) {. . . i=foo. get. Size(); foo. set. Size(i*2); }
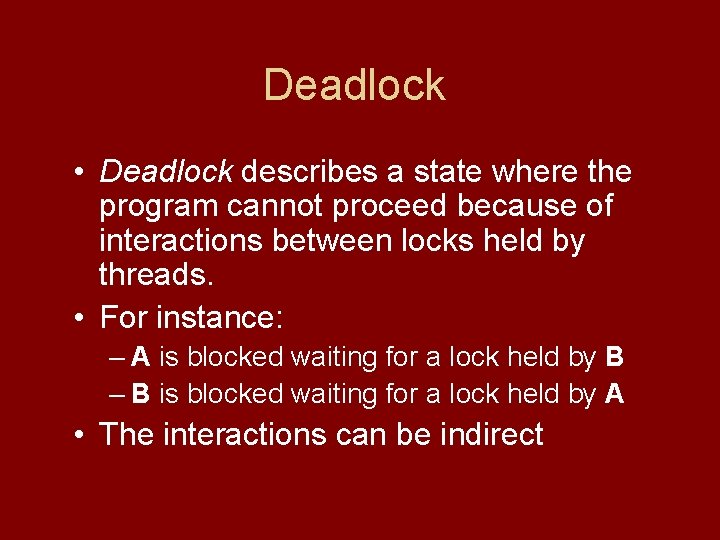
Deadlock • Deadlock describes a state where the program cannot proceed because of interactions between locks held by threads. • For instance: – A is blocked waiting for a lock held by B – B is blocked waiting for a lock held by A • The interactions can be indirect
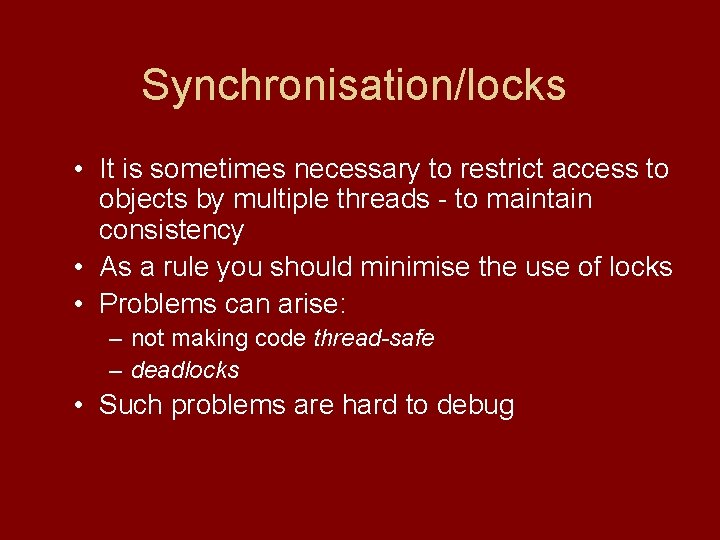
Synchronisation/locks • It is sometimes necessary to restrict access to objects by multiple threads - to maintain consistency • As a rule you should minimise the use of locks • Problems can arise: – not making code thread-safe – deadlocks • Such problems are hard to debug
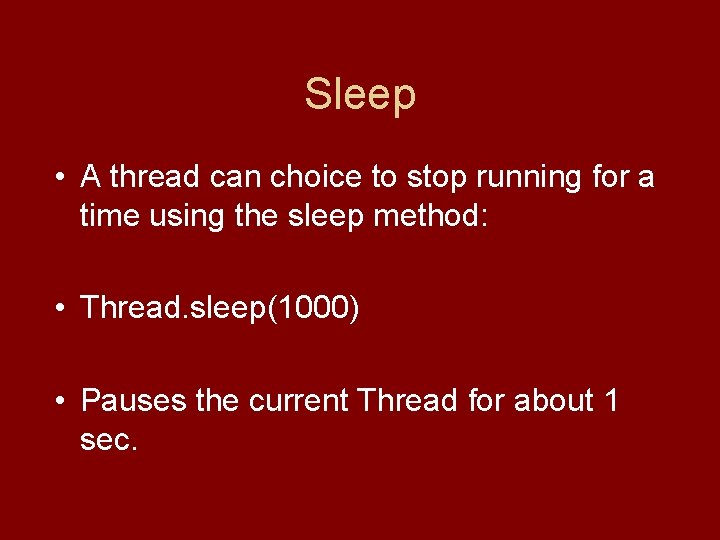
Sleep • A thread can choice to stop running for a time using the sleep method: • Thread. sleep(1000) • Pauses the current Thread for about 1 sec.
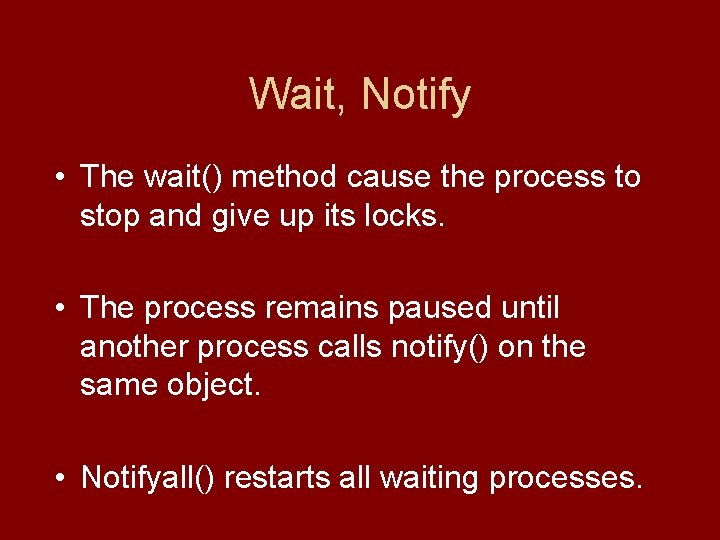
Wait, Notify • The wait() method cause the process to stop and give up its locks. • The process remains paused until another process calls notify() on the same object. • Notifyall() restarts all waiting processes.
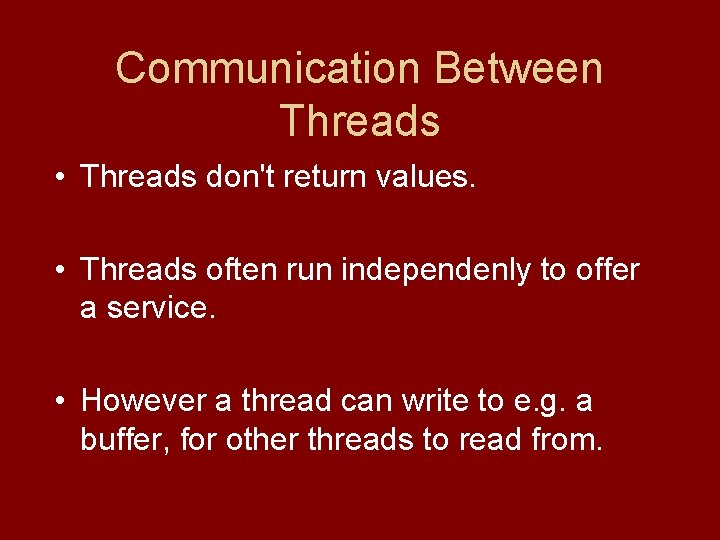
Communication Between Threads • Threads don't return values. • Threads often run independenly to offer a service. • However a thread can write to e. g. a buffer, for other threads to read from.
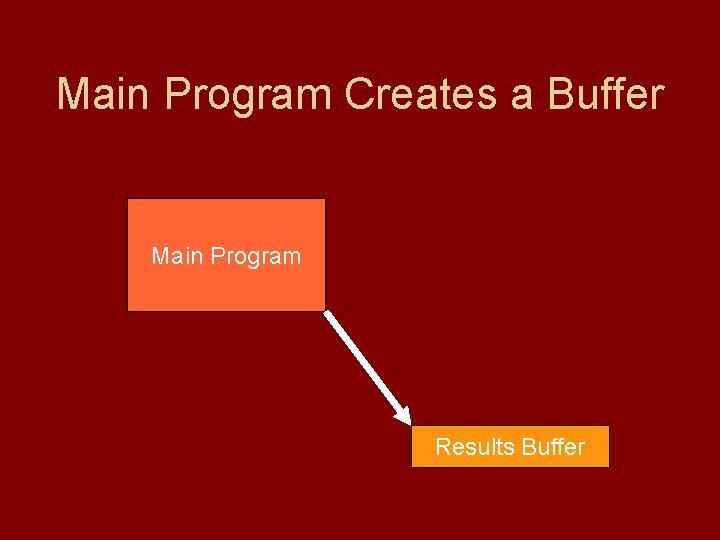
Main Program Creates a Buffer Main Program Results Buffer
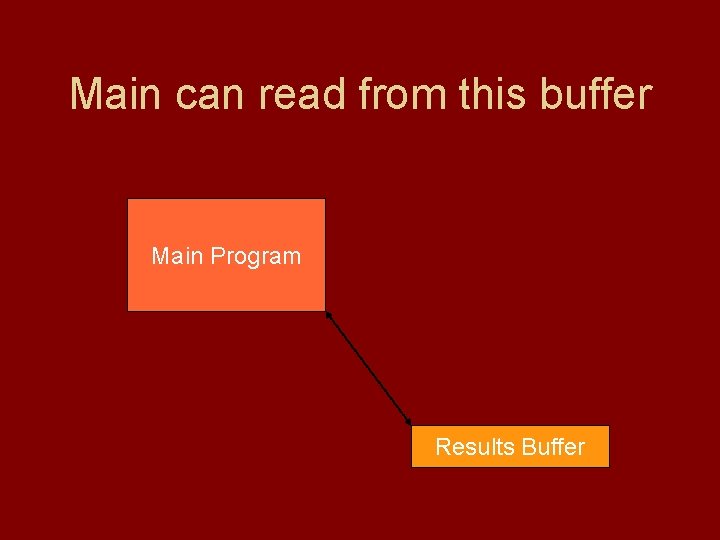
Main can read from this buffer Main Program Results Buffer
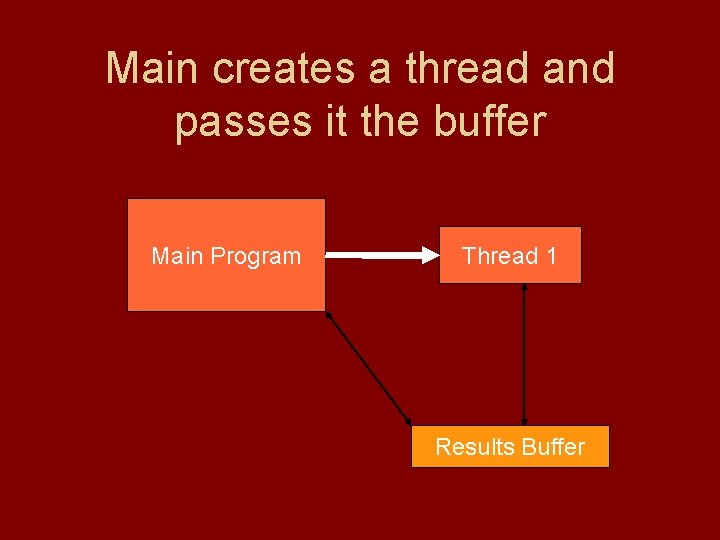
Main creates a thread and passes it the buffer Main Program Thread 1 Results Buffer
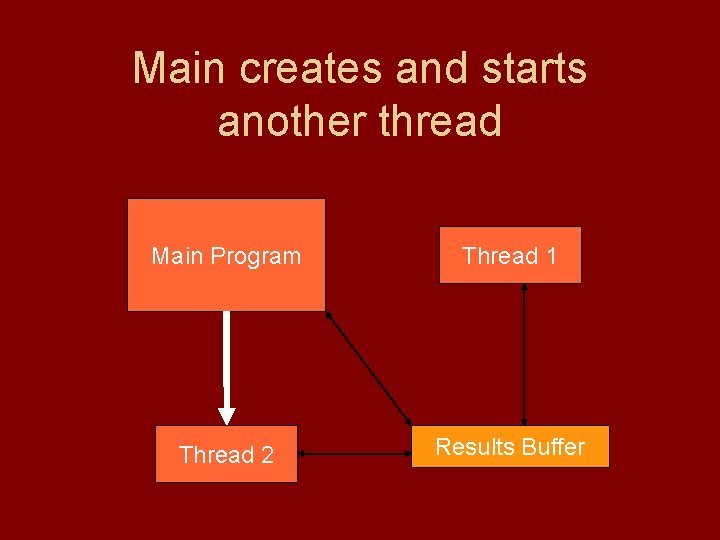
Main creates and starts another thread Main Program Thread 1 Thread 2 Results Buffer
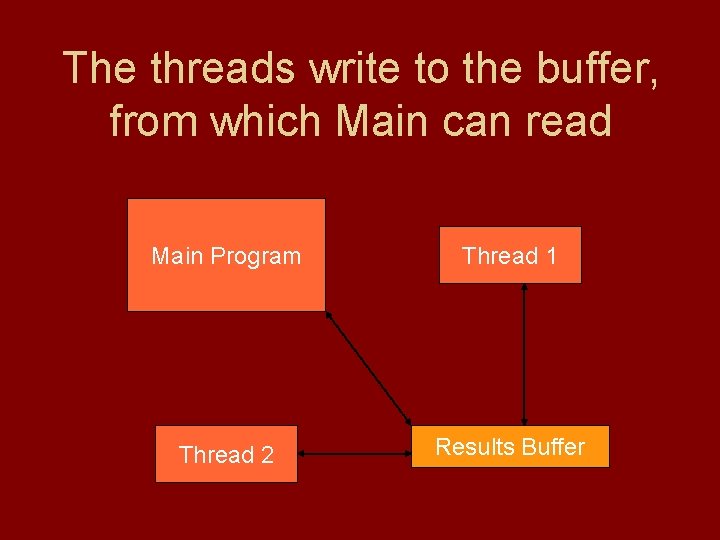
The threads write to the buffer, from which Main can read Main Program Thread 1 Thread 2 Results Buffer
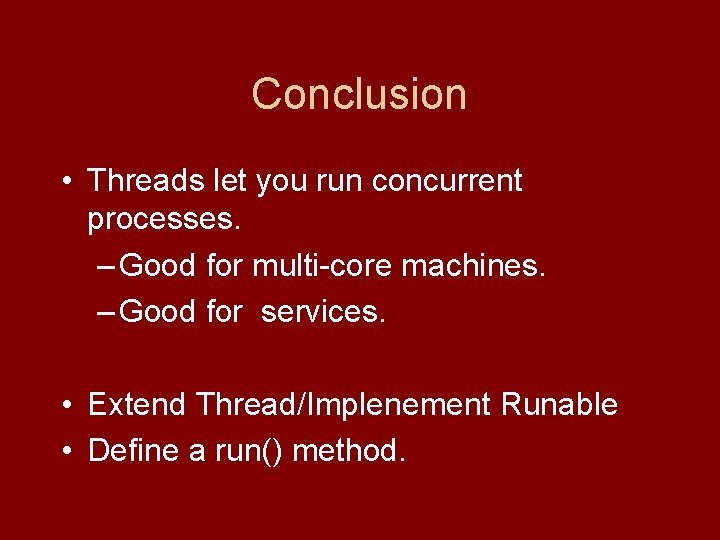
Conclusion • Threads let you run concurrent processes. – Good for multi-core machines. – Good for services. • Extend Thread/Implenement Runable • Define a run() method.
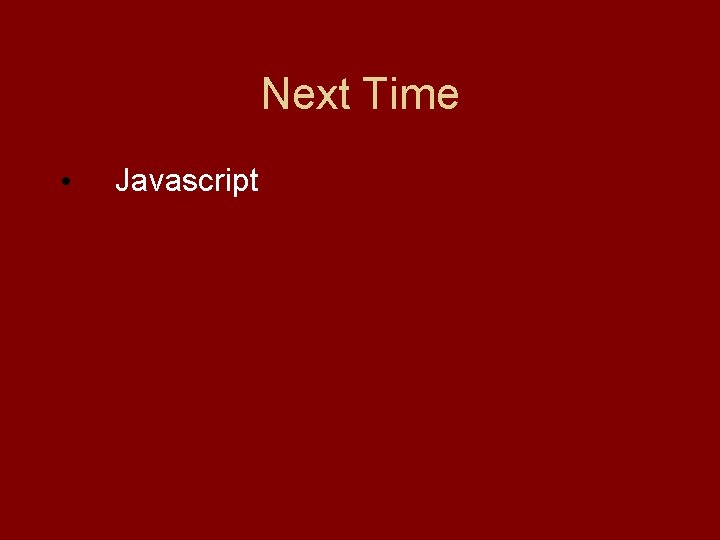
Next Time • Javascript
Tom chothia
01:640:244 lecture notes - lecture 15: plat, idah, farad
Tom tom go 910
The devil and tom walker symbols
Randy pausch last lecture summary
What will happen if tom robinson loses his last appeal?
Tom is at school
What is elapsed time
C11 thread
Java shared memory
Assembly drawing
Process and threads
A flexible flat material made by interlacing threads/fibers
Escalonamento por prioridades
Process and threads
Os threads
Basket of threads buddhism
Needle like threads of spongy bone
Waxy coated paper which transfers pattern markings
Threads performance management
Pintos priority donation
Advantages of threads in operating system
Pthreads
Threads java
Osteospermum white lightning
Forum.unity.com/threads/game-over.54735
Threads fiji
Threads consumes cpu in best possible manner
Sockets and threads
Posix threads
Forum.unity.com/threads/game-over.54735
Process vs thread
Sequence diagram threads