Lecture 8 POSIX Threads 1 Review Threads Why
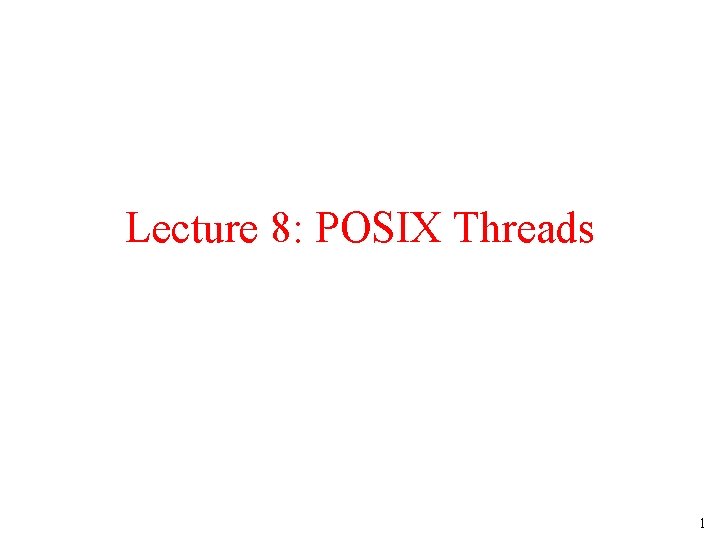
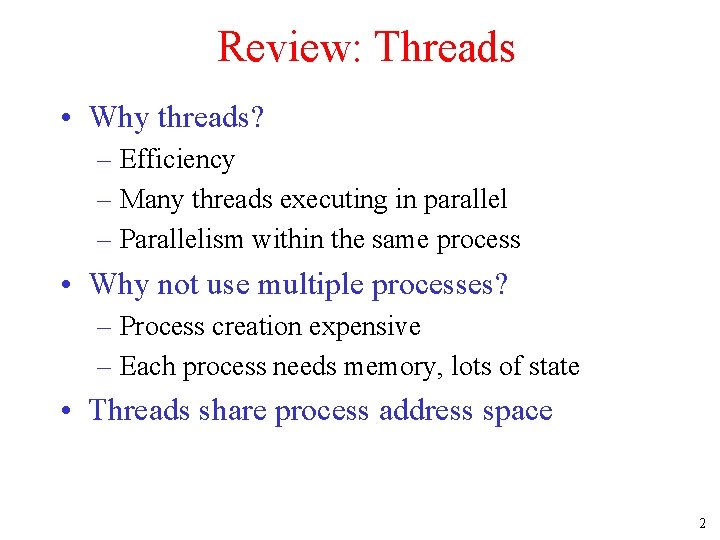
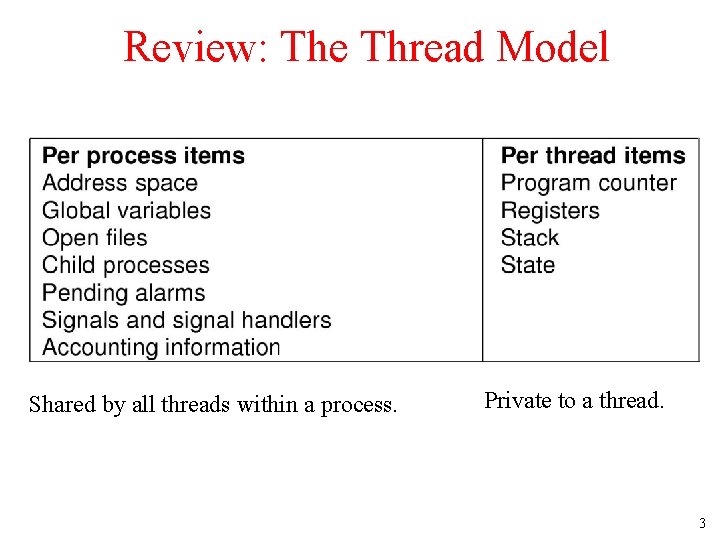
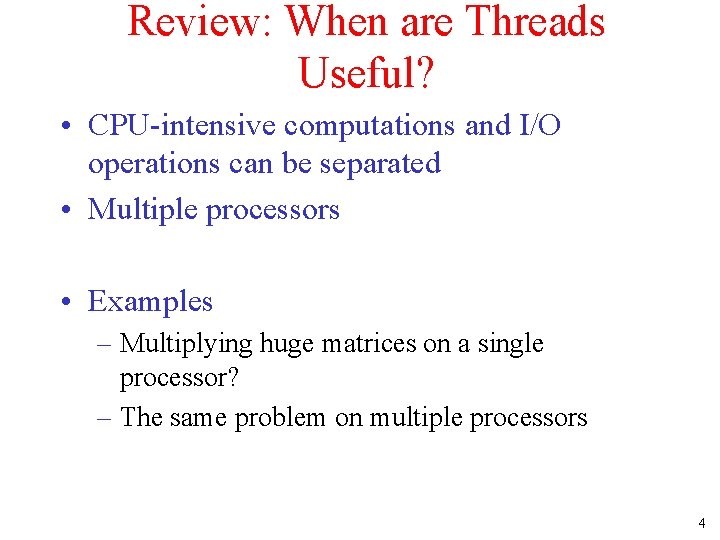
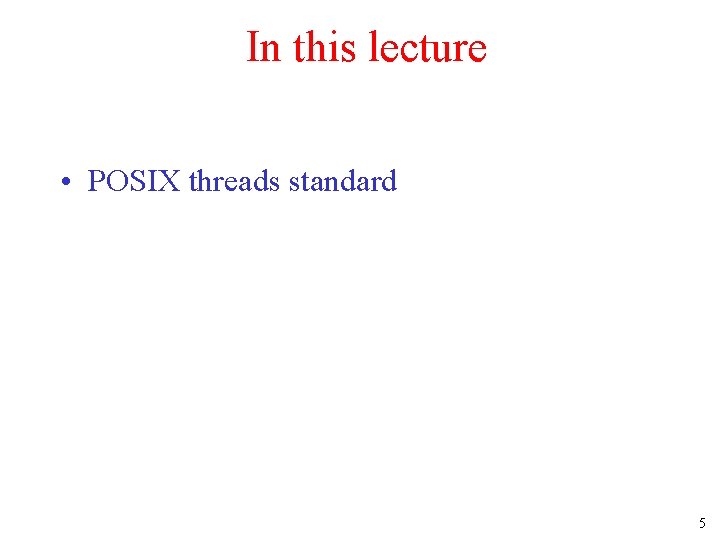
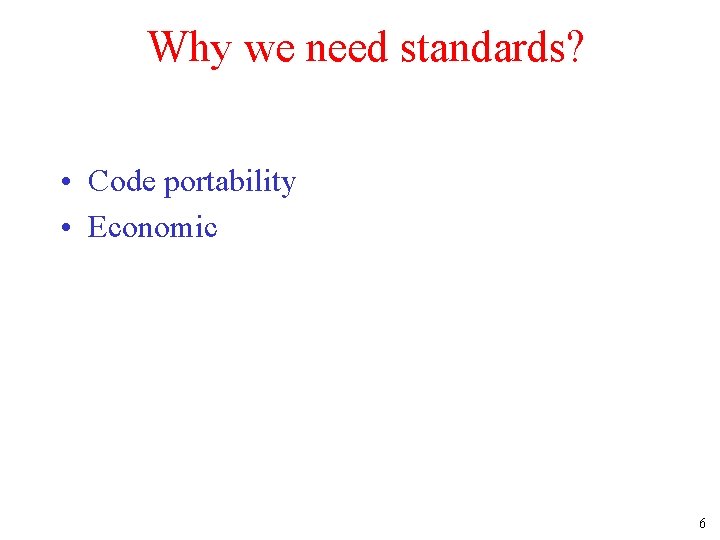
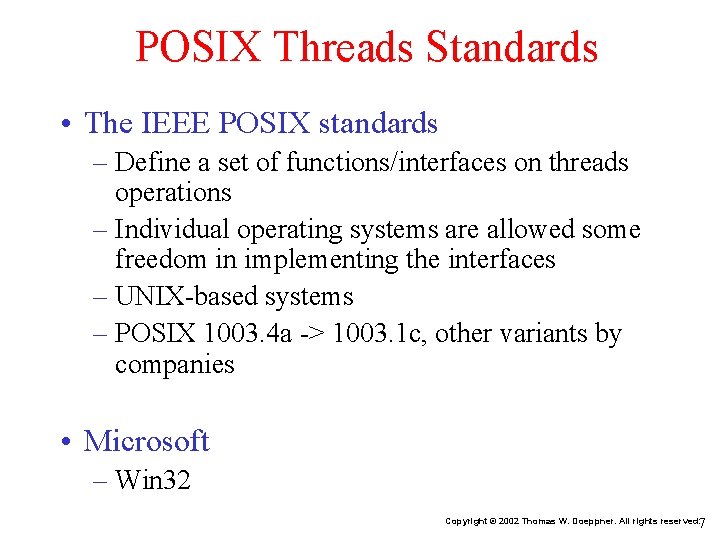
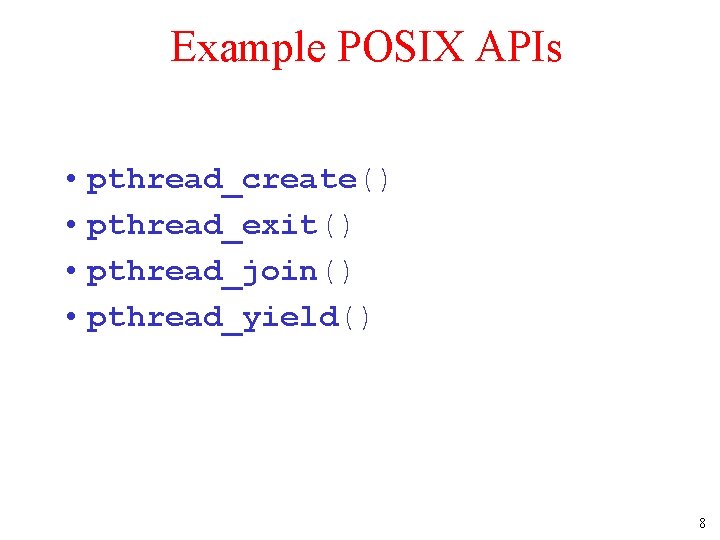
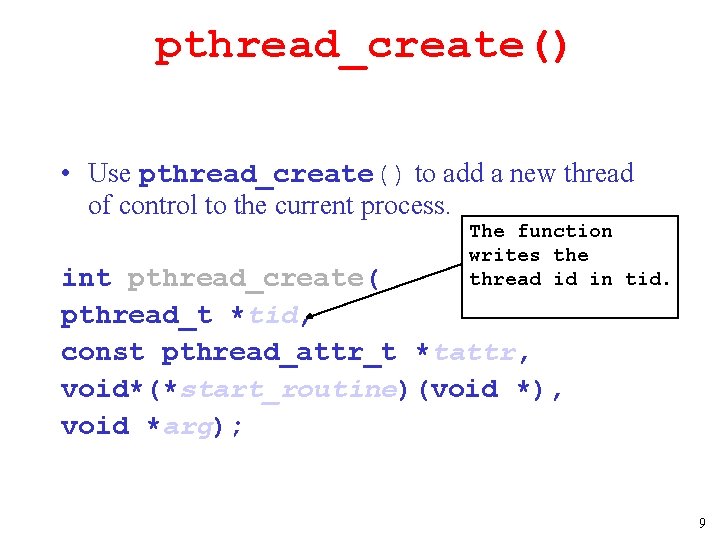
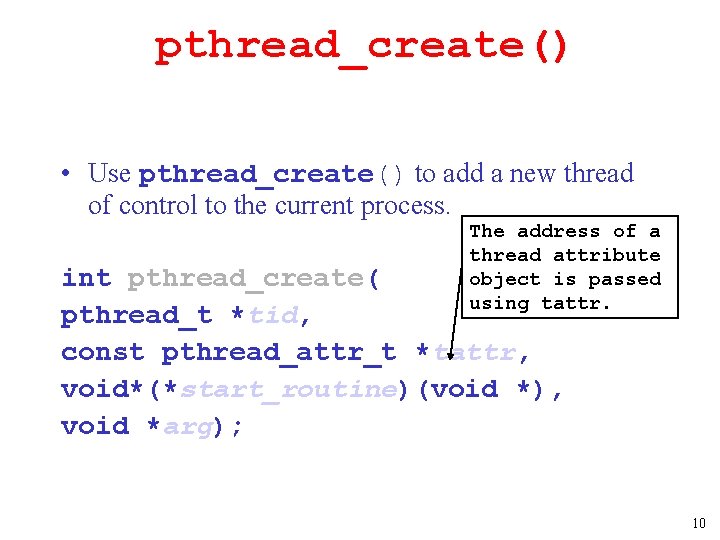
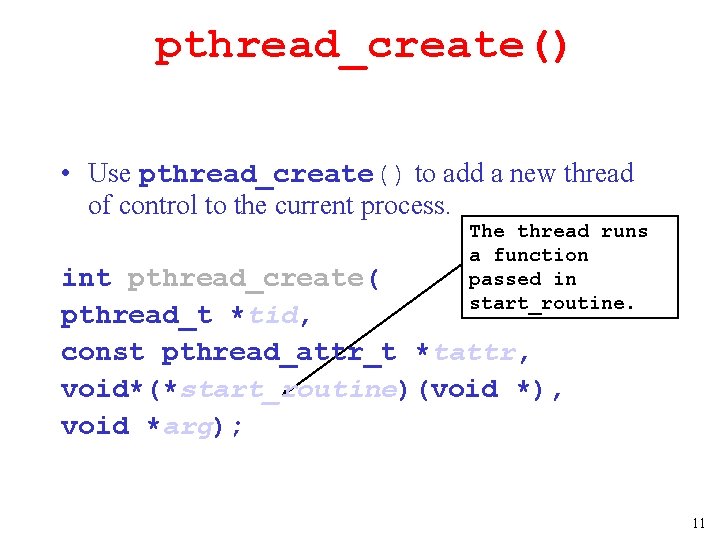
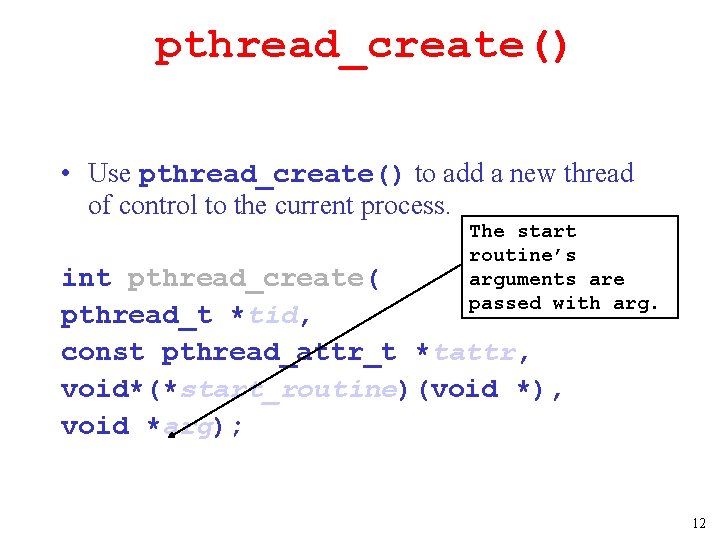
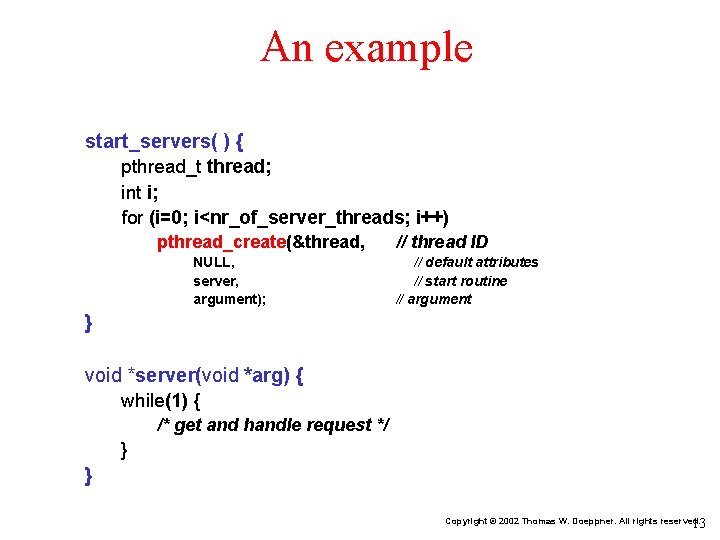
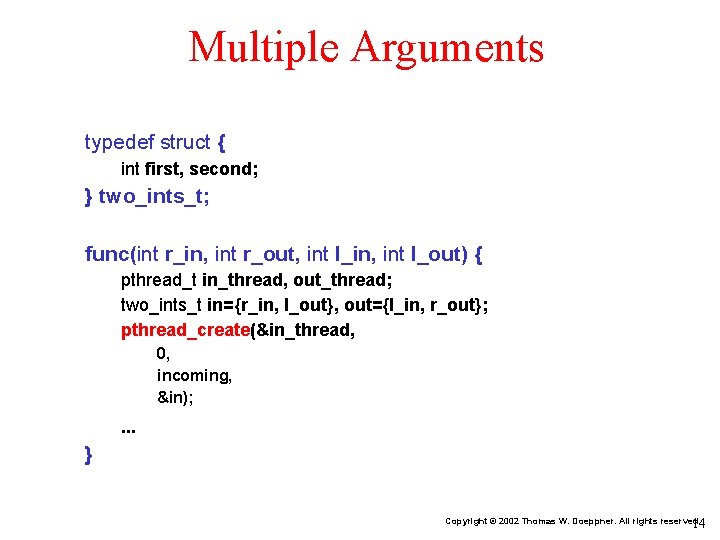
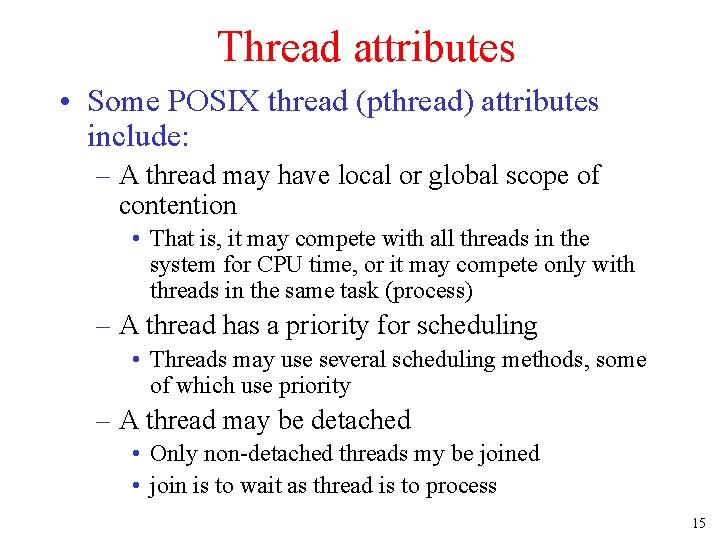
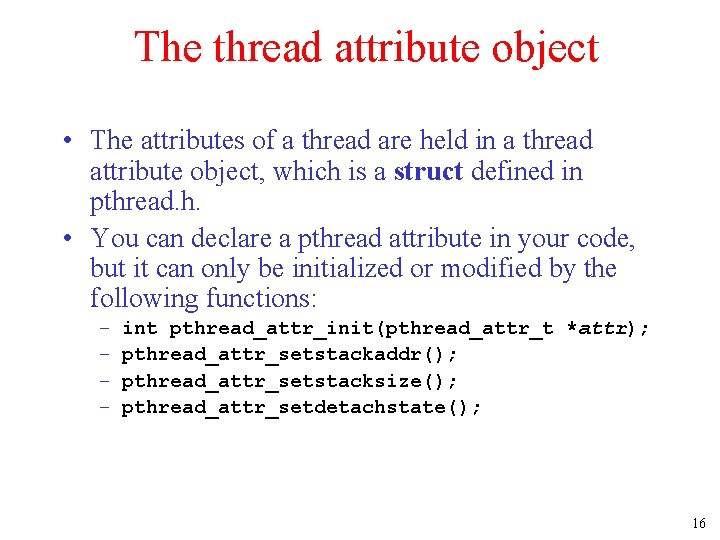
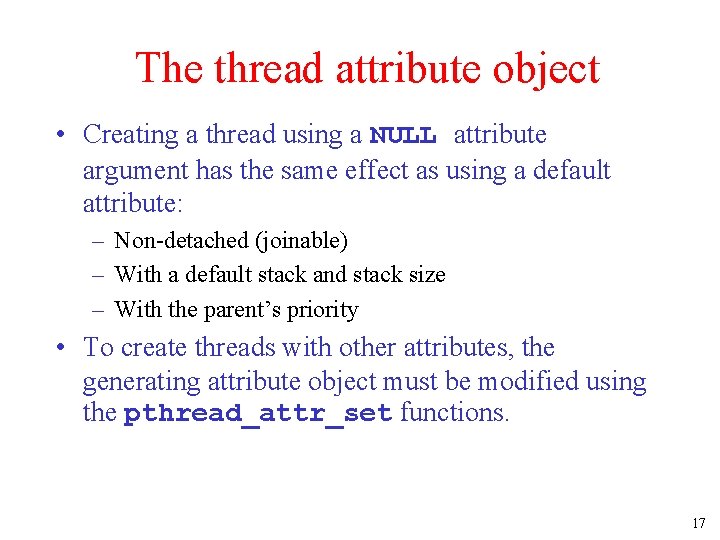
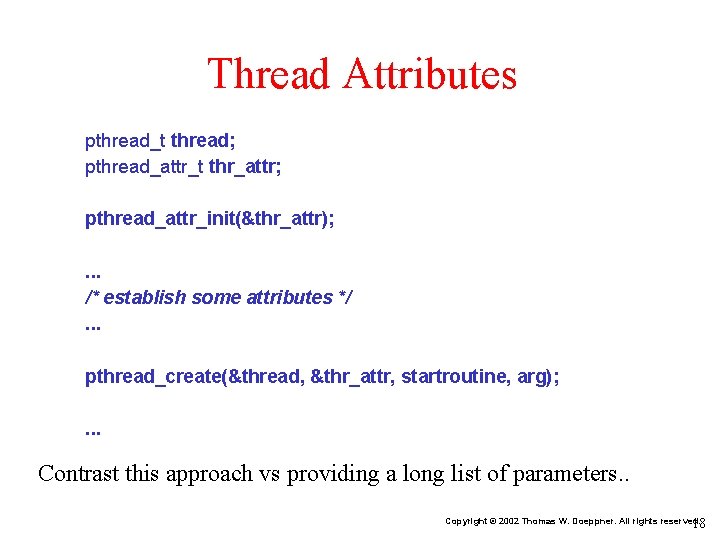
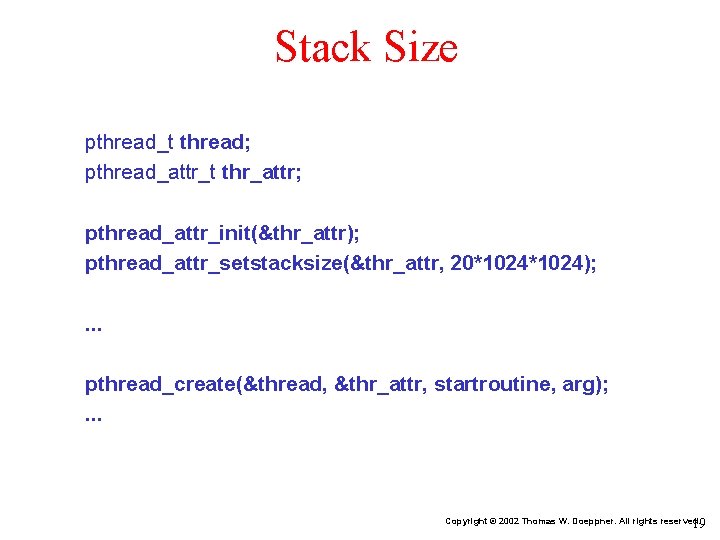
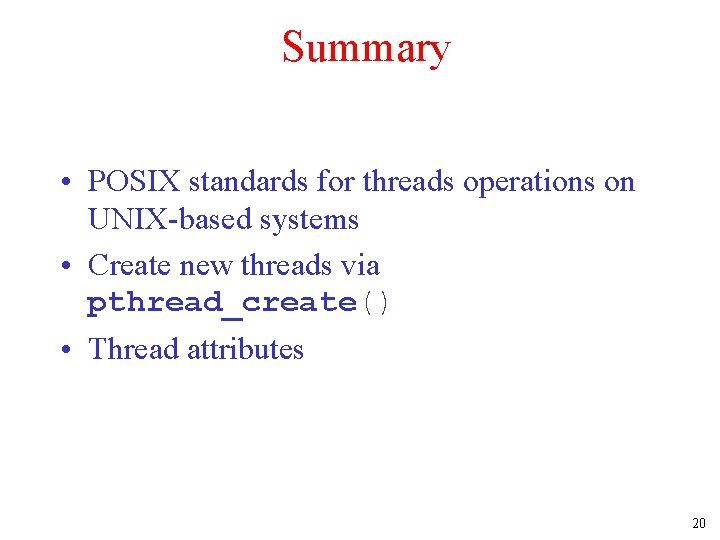
- Slides: 20
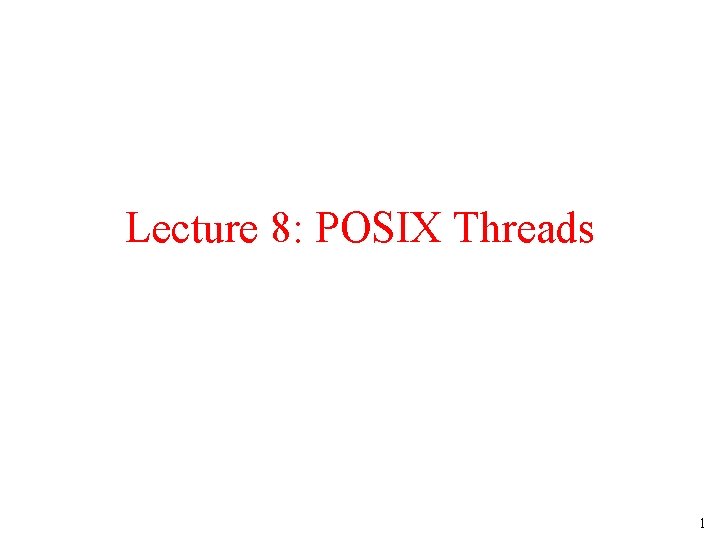
Lecture 8: POSIX Threads 1
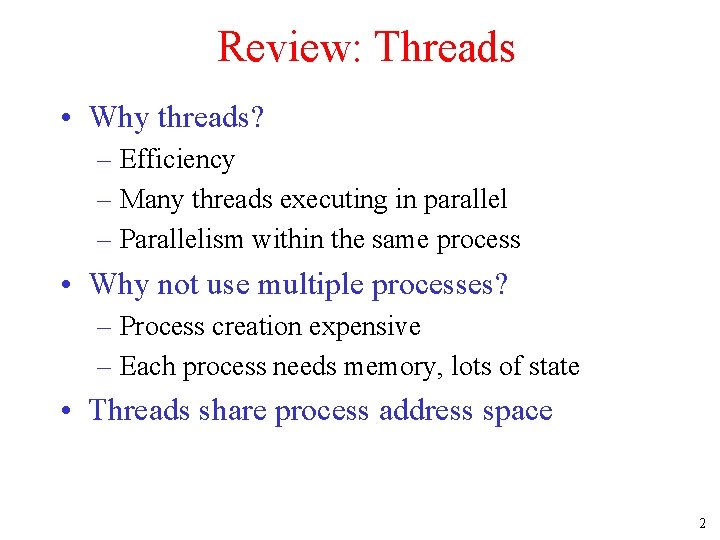
Review: Threads • Why threads? – Efficiency – Many threads executing in parallel – Parallelism within the same process • Why not use multiple processes? – Process creation expensive – Each process needs memory, lots of state • Threads share process address space 2
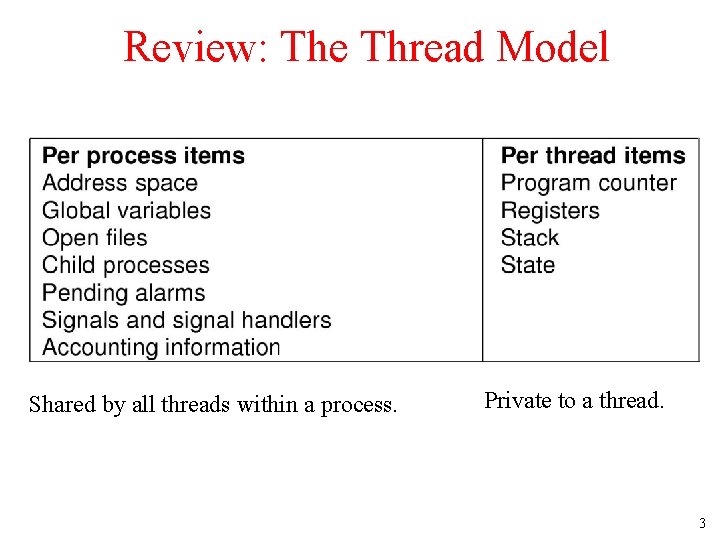
Review: The Thread Model Shared by all threads within a process. Private to a thread. 3
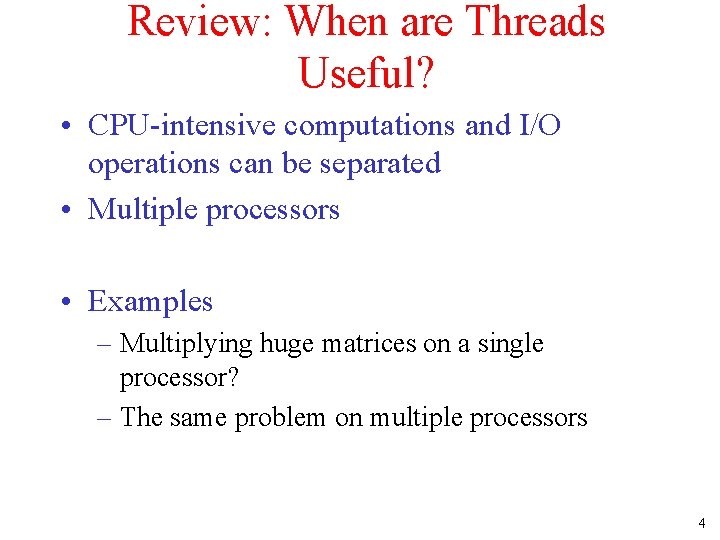
Review: When are Threads Useful? • CPU-intensive computations and I/O operations can be separated • Multiple processors • Examples – Multiplying huge matrices on a single processor? – The same problem on multiple processors 4
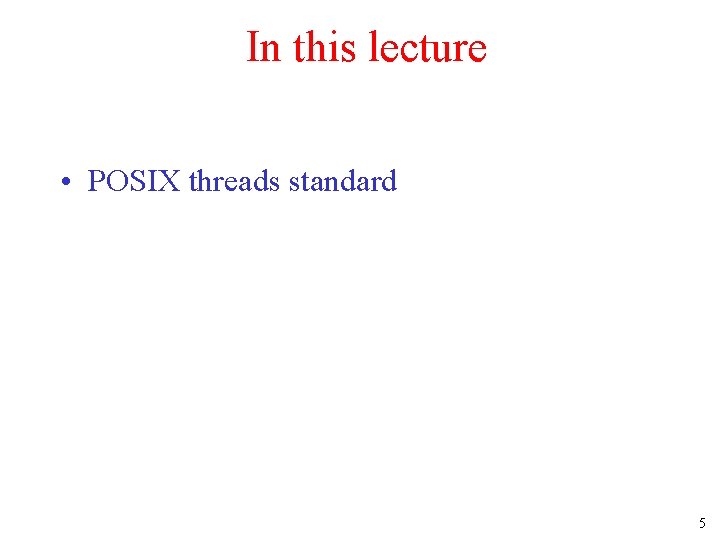
In this lecture • POSIX threads standard 5
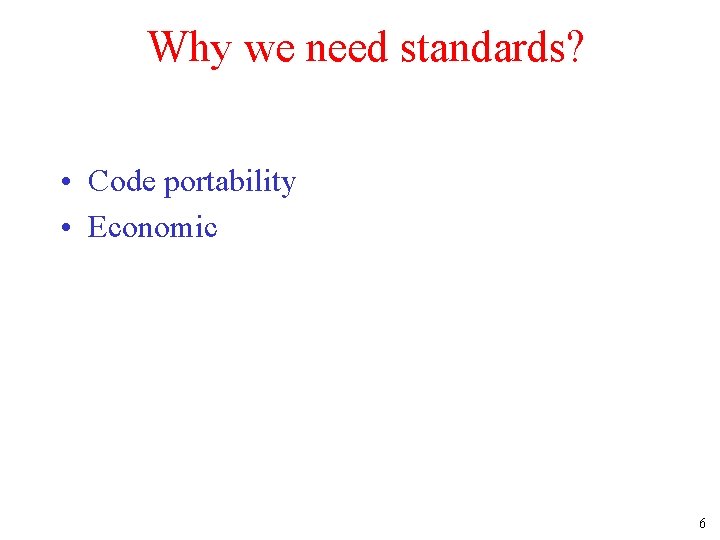
Why we need standards? • Code portability • Economic 6
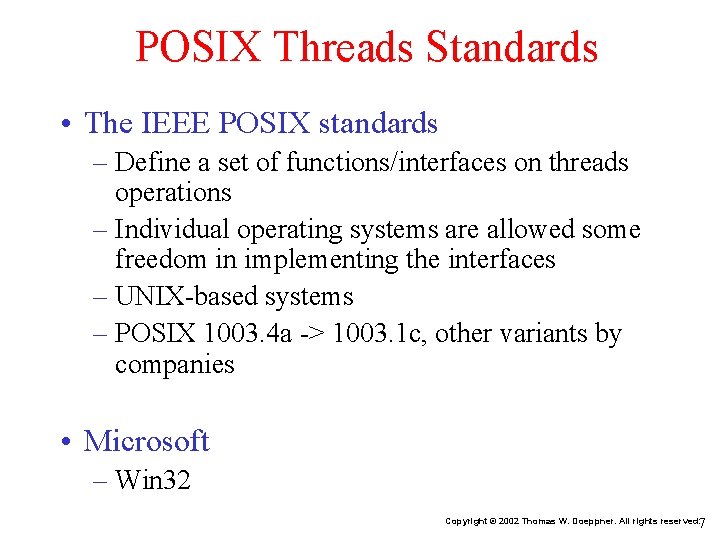
POSIX Threads Standards • The IEEE POSIX standards – Define a set of functions/interfaces on threads operations – Individual operating systems are allowed some freedom in implementing the interfaces – UNIX-based systems – POSIX 1003. 4 a -> 1003. 1 c, other variants by companies • Microsoft – Win 32 Copyright © 2002 Thomas W. Doeppner. All rights reserved. 7
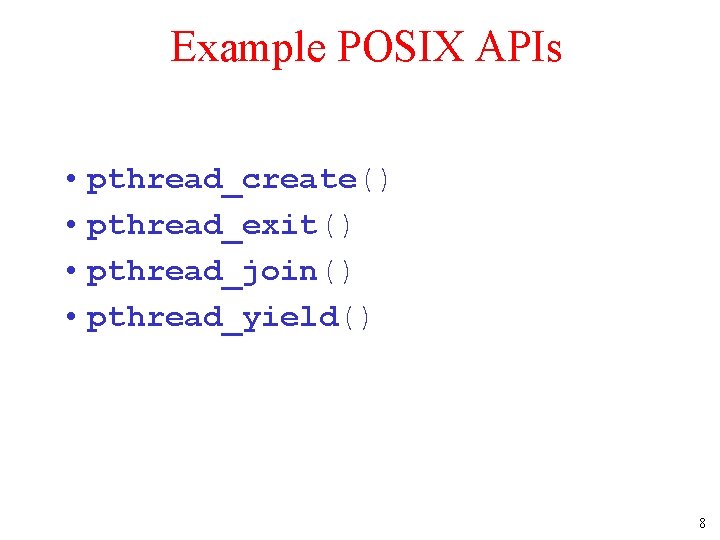
Example POSIX APIs • pthread_create() • pthread_exit() • pthread_join() • pthread_yield() 8
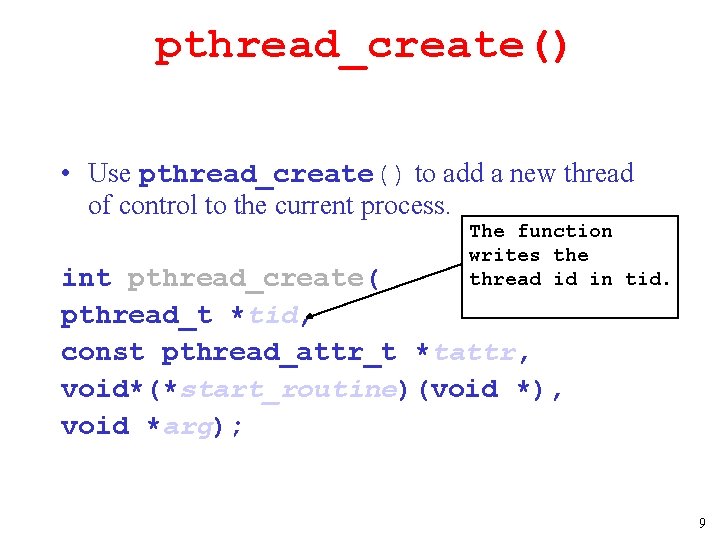
pthread_create() • Use pthread_create() to add a new thread of control to the current process. The function writes the thread id in tid. int pthread_create( pthread_t *tid, const pthread_attr_t *tattr, void*(*start_routine)(void *), void *arg); 9
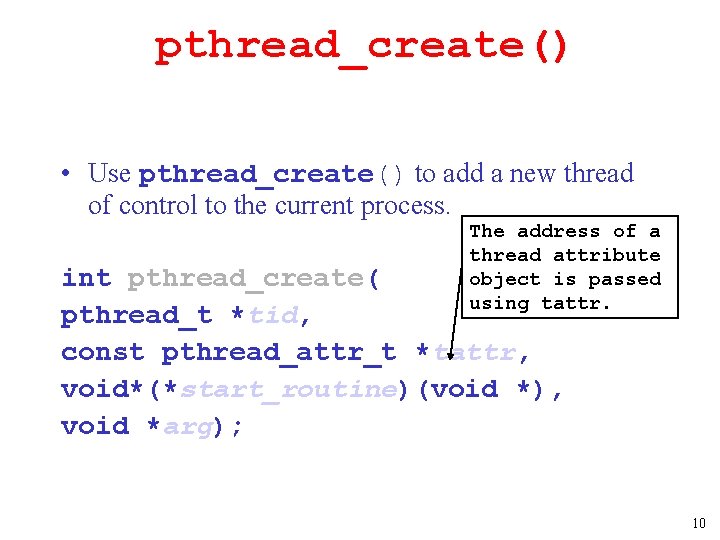
pthread_create() • Use pthread_create() to add a new thread of control to the current process. The address of a thread attribute object is passed using tattr. int pthread_create( pthread_t *tid, const pthread_attr_t *tattr, void*(*start_routine)(void *), void *arg); 10
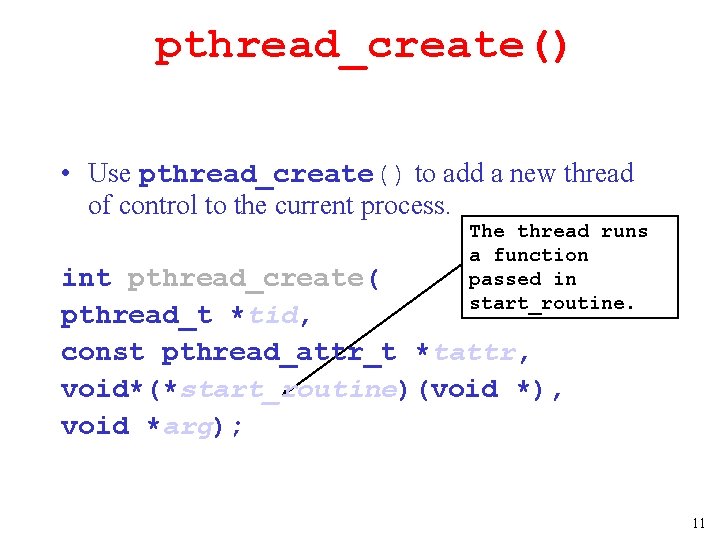
pthread_create() • Use pthread_create() to add a new thread of control to the current process. The thread runs a function passed in start_routine. int pthread_create( pthread_t *tid, const pthread_attr_t *tattr, void*(*start_routine)(void *), void *arg); 11
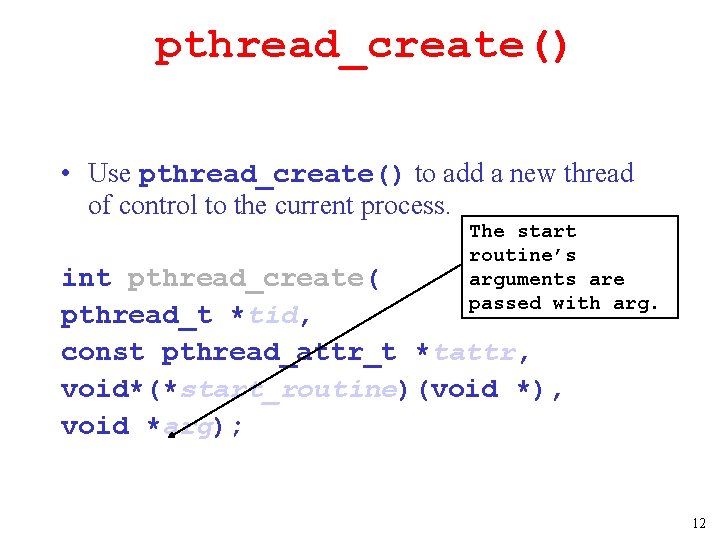
pthread_create() • Use pthread_create() to add a new thread of control to the current process. The start routine’s arguments are passed with arg. int pthread_create( pthread_t *tid, const pthread_attr_t *tattr, void*(*start_routine)(void *), void *arg); 12
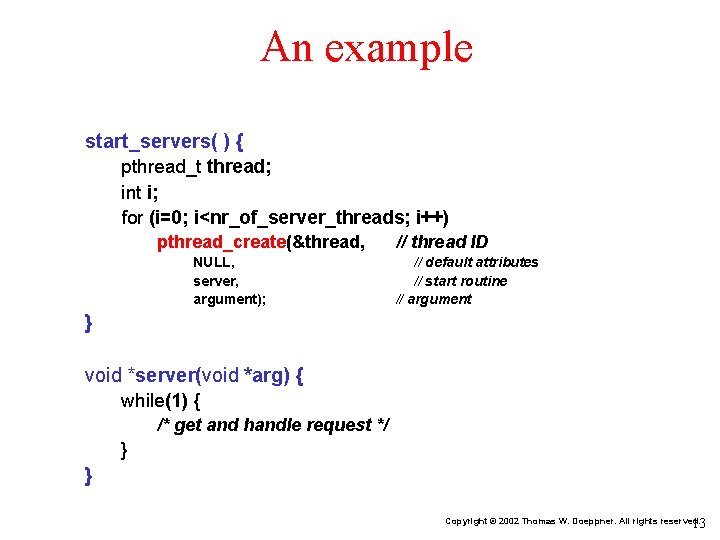
An example start_servers( ) { pthread_t thread; int i; for (i=0; i<nr_of_server_threads; i++) pthread_create(&thread, NULL, server, argument); // thread ID // default attributes // start routine // argument } void *server(void *arg) { while(1) { /* get and handle request */ } } Copyright © 2002 Thomas W. Doeppner. All rights reserved. 13
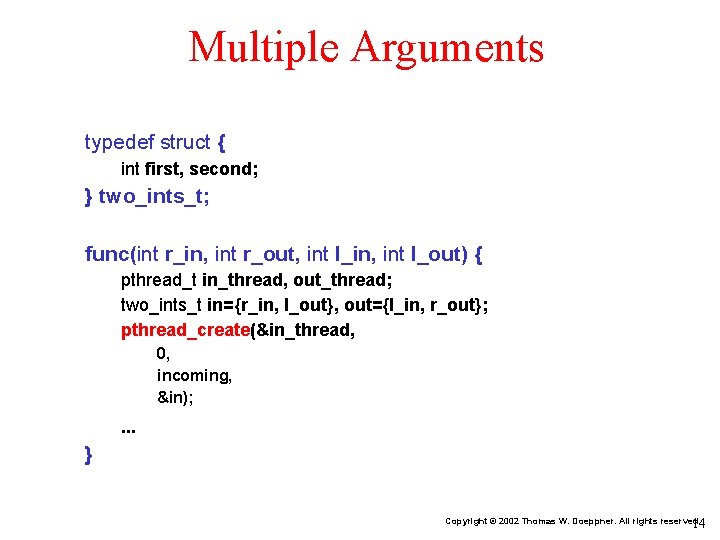
Multiple Arguments typedef struct { int first, second; } two_ints_t; func(int r_in, int r_out, int l_in, int l_out) { pthread_t in_thread, out_thread; two_ints_t in={r_in, l_out}, out={l_in, r_out}; pthread_create(&in_thread, 0, incoming, &in); . . . } Copyright © 2002 Thomas W. Doeppner. All rights reserved. 14
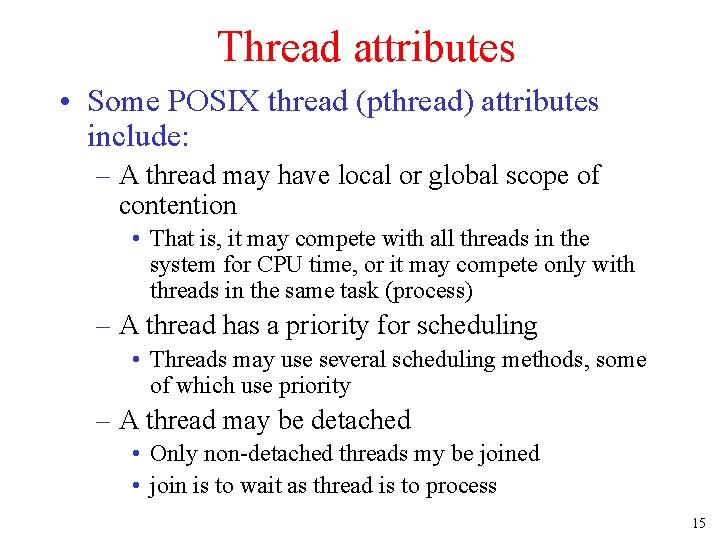
Thread attributes • Some POSIX thread (pthread) attributes include: – A thread may have local or global scope of contention • That is, it may compete with all threads in the system for CPU time, or it may compete only with threads in the same task (process) – A thread has a priority for scheduling • Threads may use several scheduling methods, some of which use priority – A thread may be detached • Only non-detached threads my be joined • join is to wait as thread is to process 15
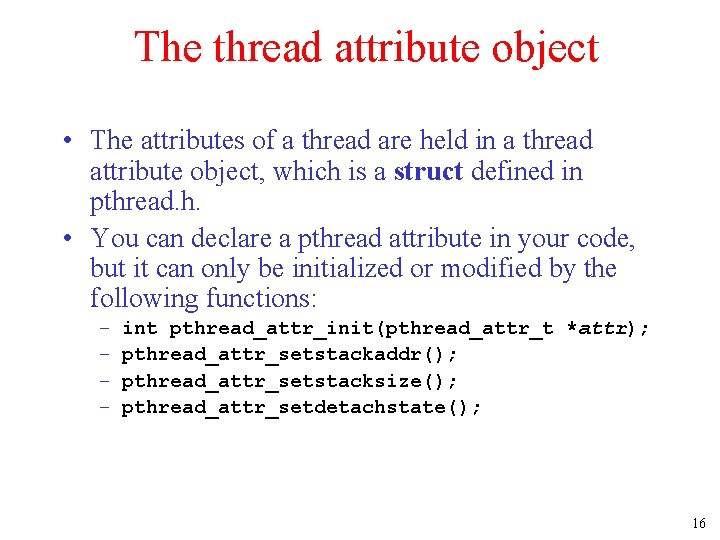
The thread attribute object • The attributes of a thread are held in a thread attribute object, which is a struct defined in pthread. h. • You can declare a pthread attribute in your code, but it can only be initialized or modified by the following functions: – – int pthread_attr_init(pthread_attr_t *attr); pthread_attr_setstackaddr(); pthread_attr_setstacksize(); pthread_attr_setdetachstate(); 16
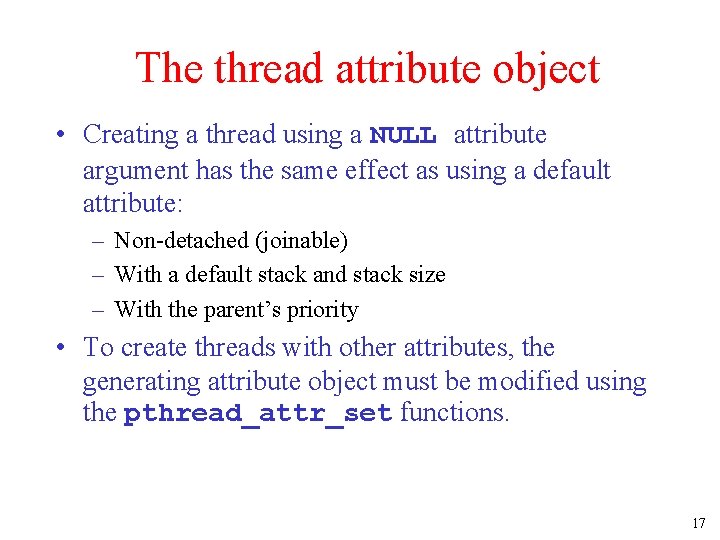
The thread attribute object • Creating a thread using a NULL attribute argument has the same effect as using a default attribute: – Non-detached (joinable) – With a default stack and stack size – With the parent’s priority • To create threads with other attributes, the generating attribute object must be modified using the pthread_attr_set functions. 17
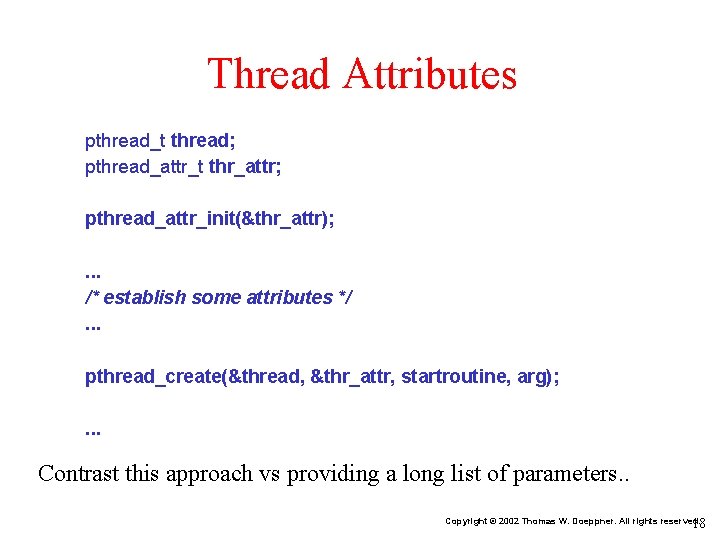
Thread Attributes pthread_t thread; pthread_attr_t thr_attr; pthread_attr_init(&thr_attr); . . . /* establish some attributes */. . . pthread_create(&thread, &thr_attr, startroutine, arg); . . . Contrast this approach vs providing a long list of parameters. . Copyright © 2002 Thomas W. Doeppner. All rights reserved. 18
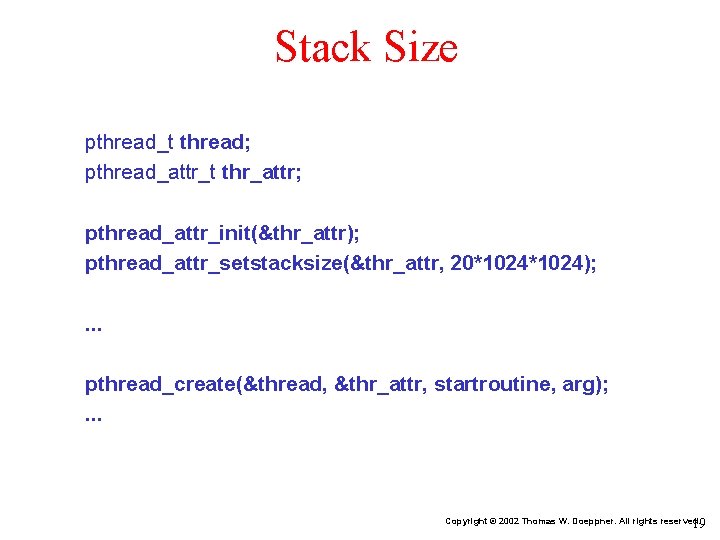
Stack Size pthread_t thread; pthread_attr_t thr_attr; pthread_attr_init(&thr_attr); pthread_attr_setstacksize(&thr_attr, 20*1024); . . . pthread_create(&thread, &thr_attr, startroutine, arg); . . . Copyright © 2002 Thomas W. Doeppner. All rights reserved. 19
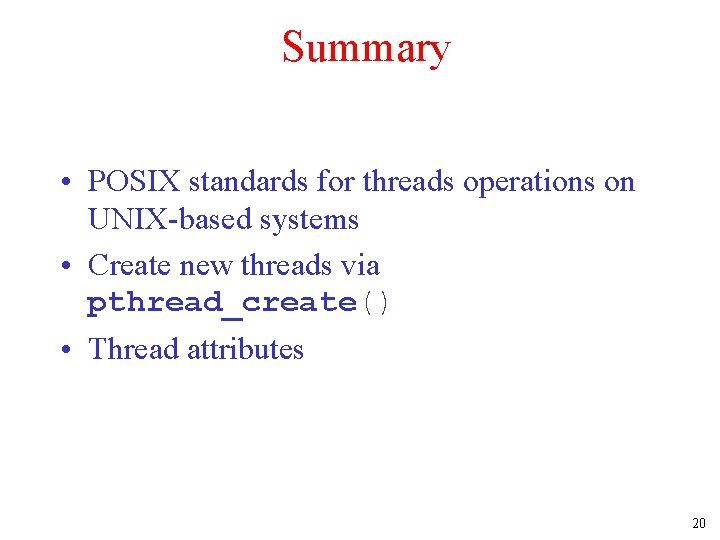
Summary • POSIX standards for threads operations on UNIX-based systems • Create new threads via pthread_create() • Thread attributes 20
Posix threads in os
Lightweight thread
Andreas carlsson bye bye bye
Standard ansi c
Posix 4
Posix basics
Shared memory in unix
Posix adalah
Procesos y comunicación sistemas operativos 2
Hilos posix
Posix filesystem
01:640:244 lecture notes - lecture 15: plat, idah, farad
Don't ask why why why
Chapter review motion part a vocabulary review answer key
Ap gov final review
Nader amin-salehi
What is inclusion and exclusion
Narrative review vs systematic review
C11 thread
Shared memory java
Conventional representation of internal thread