Swing and Event handling L Grewe Swing Differences
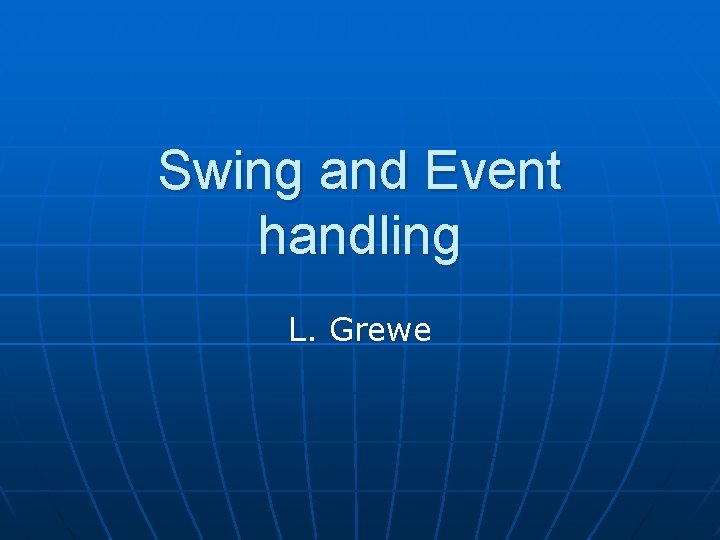
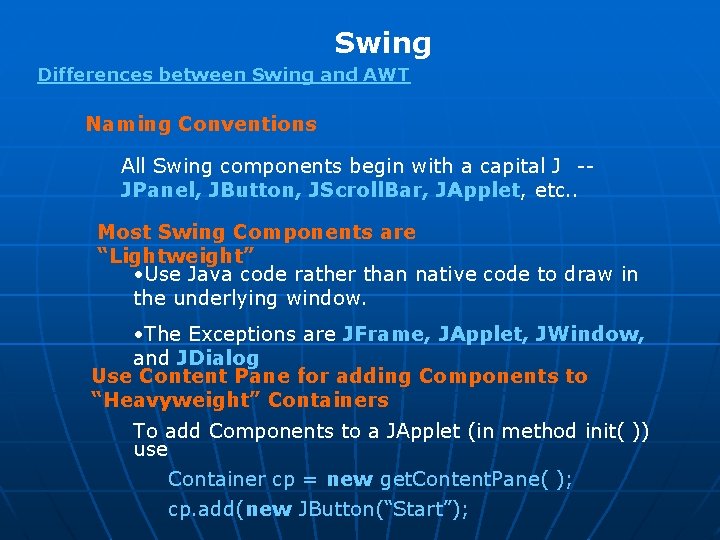
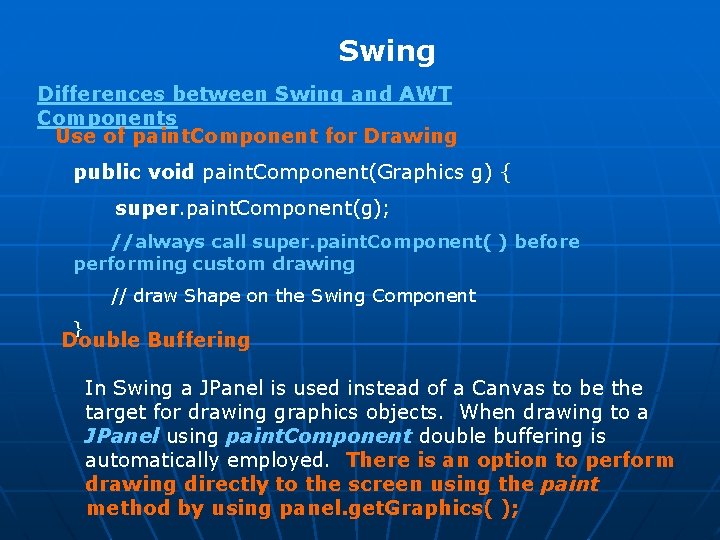
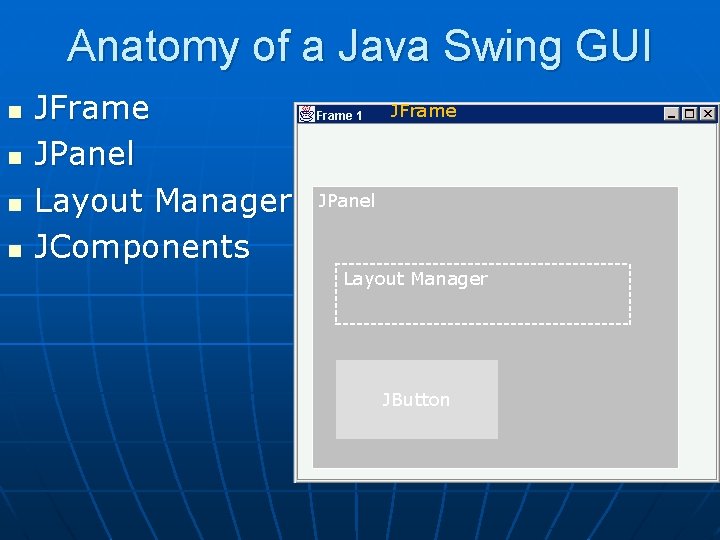
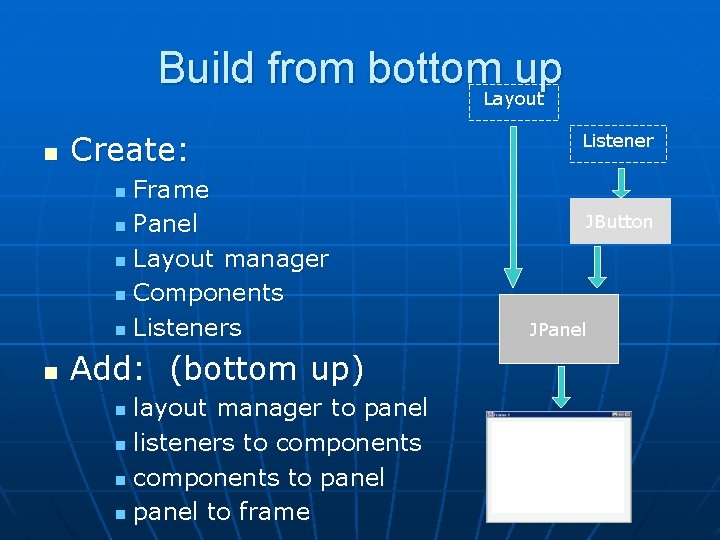
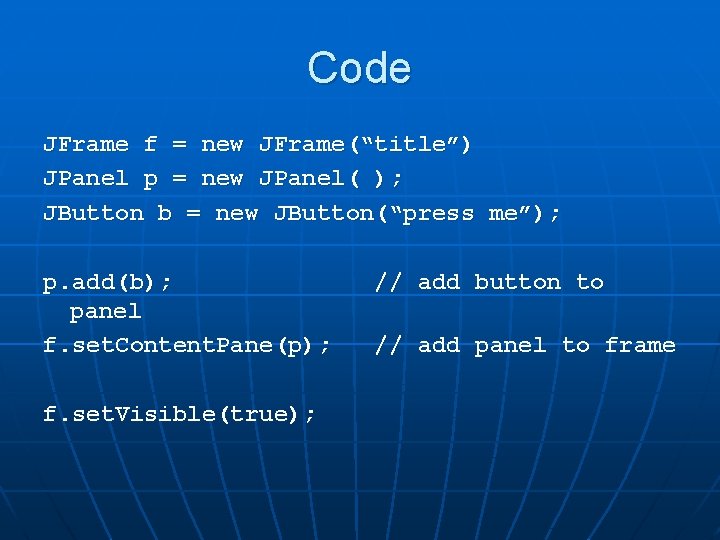
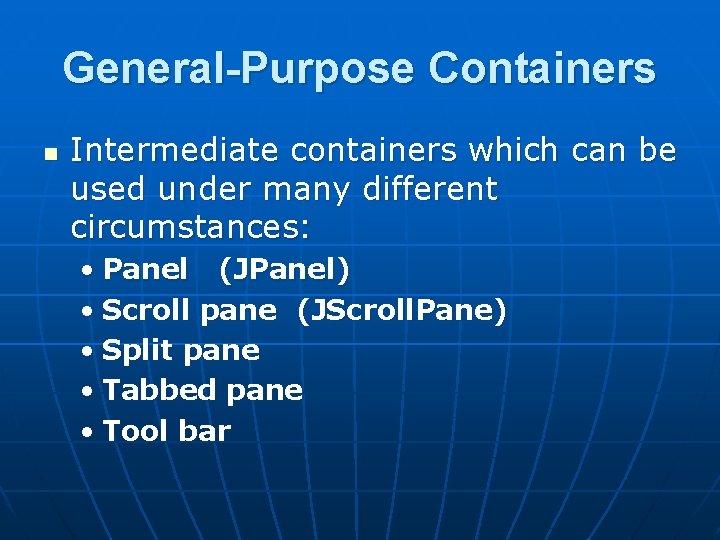
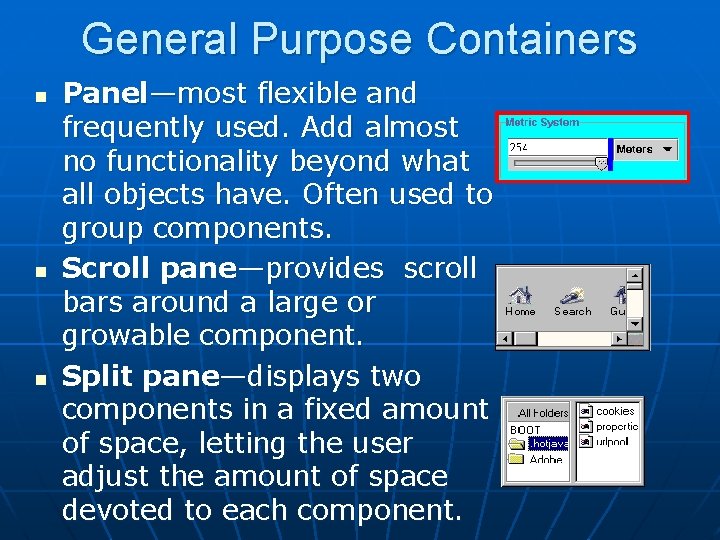
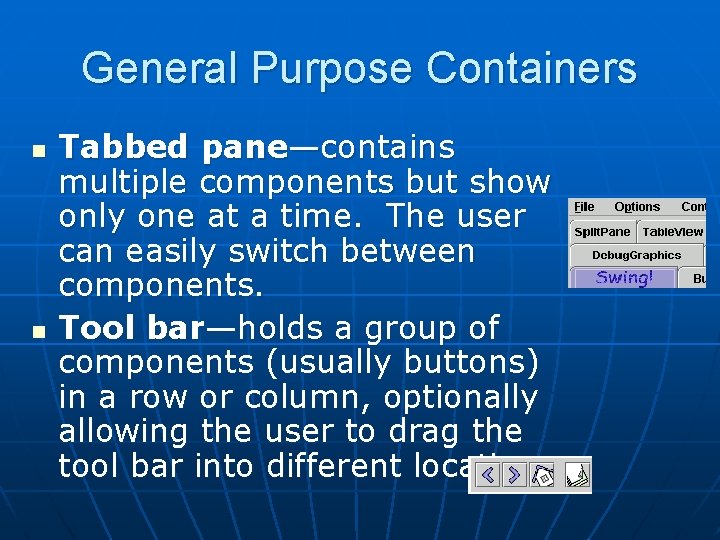
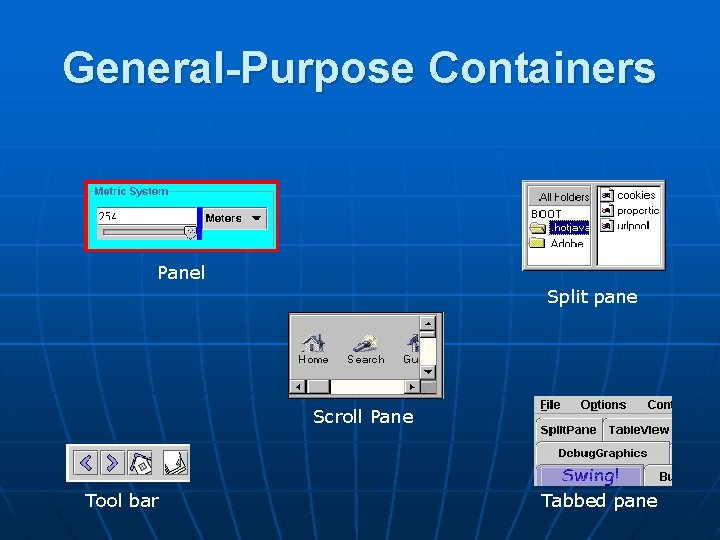
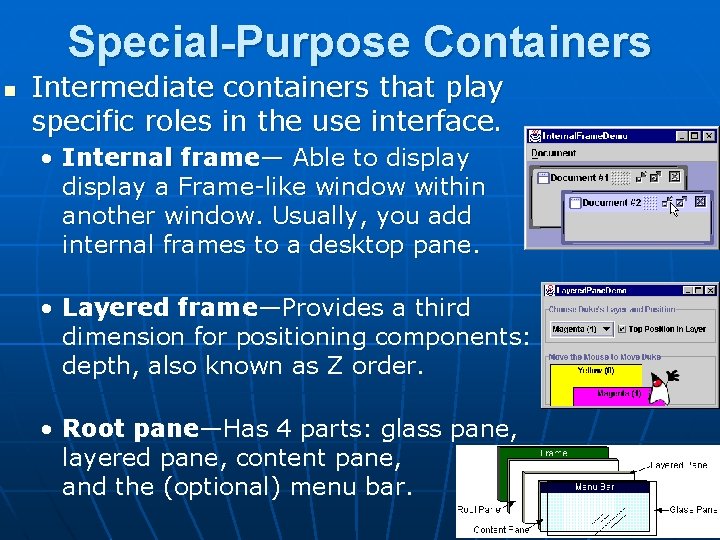
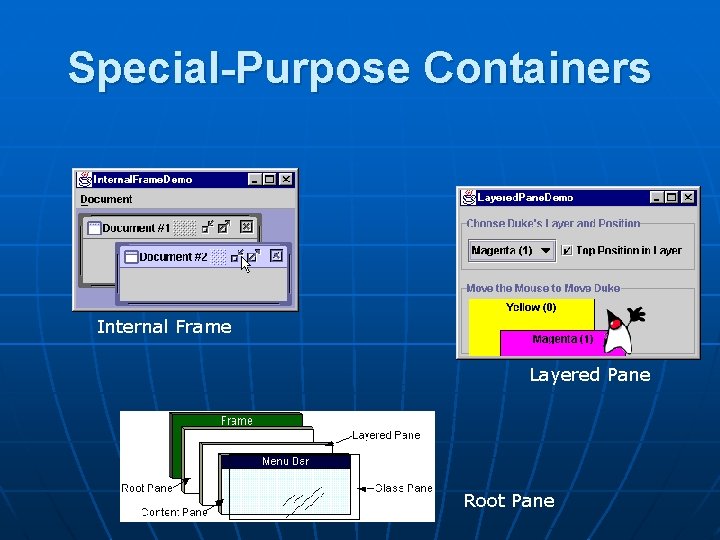
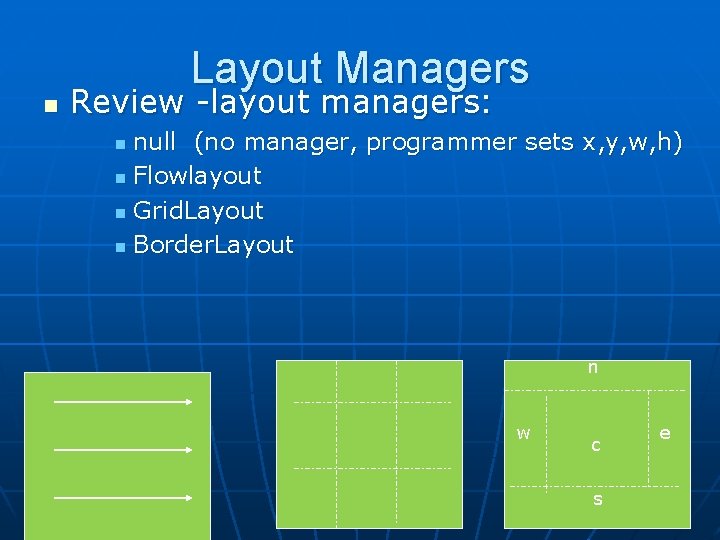
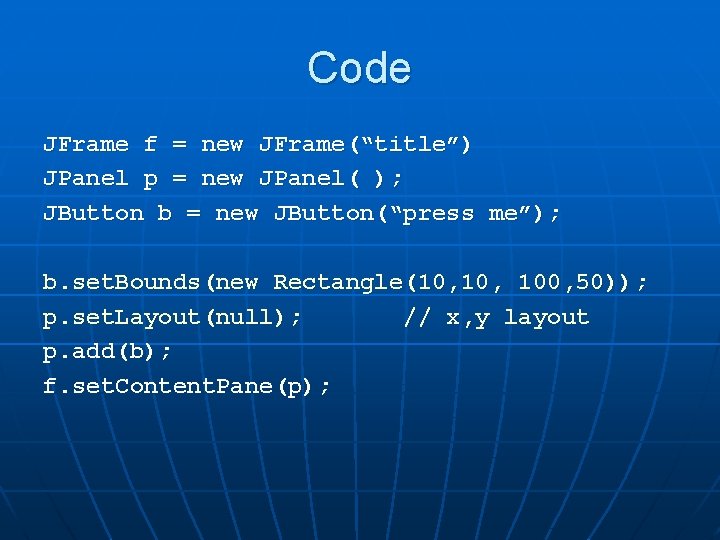
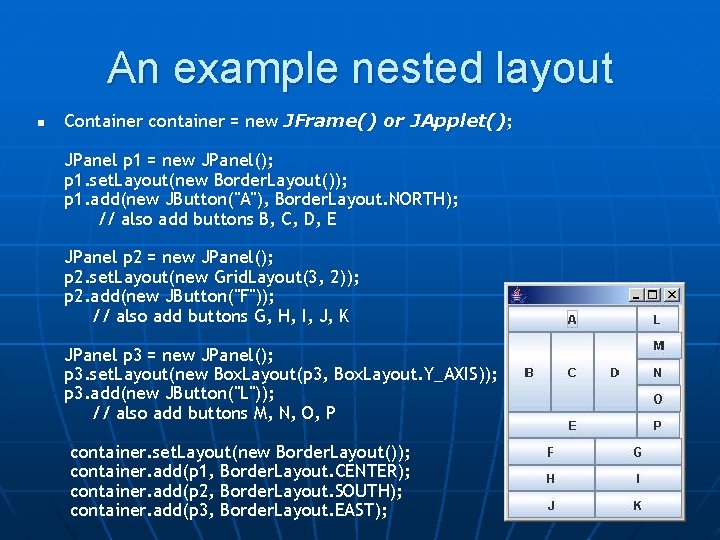
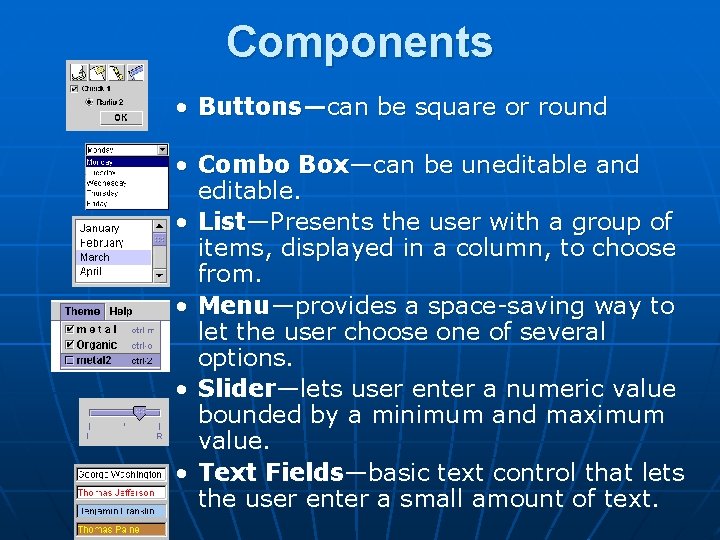
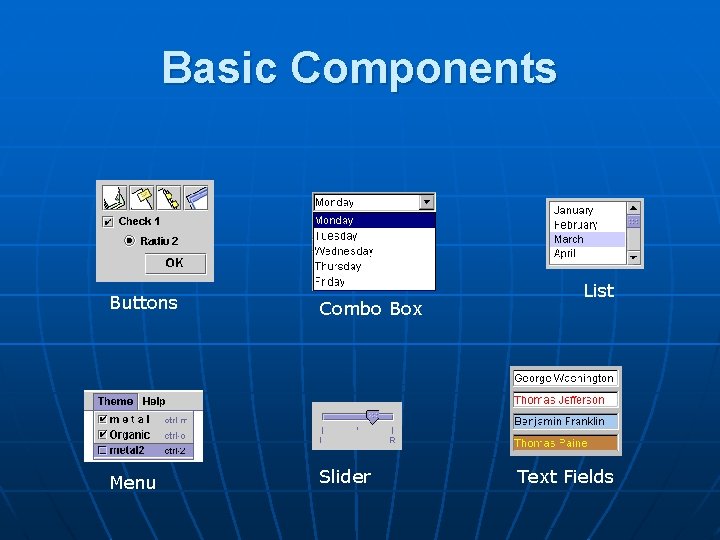
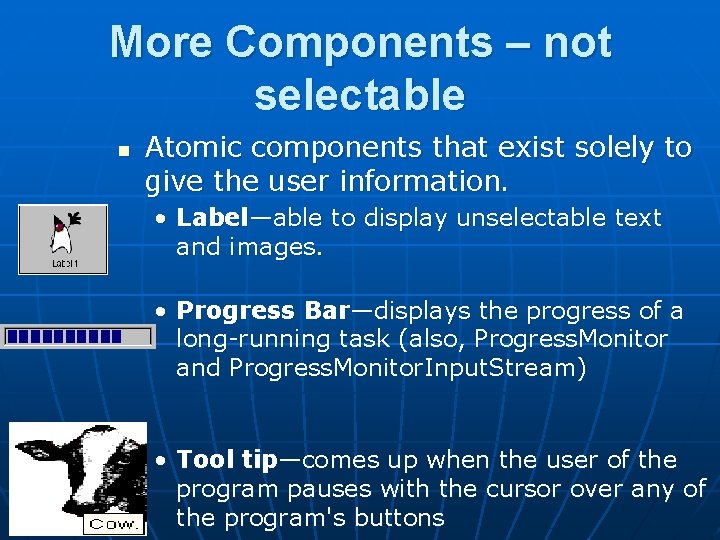
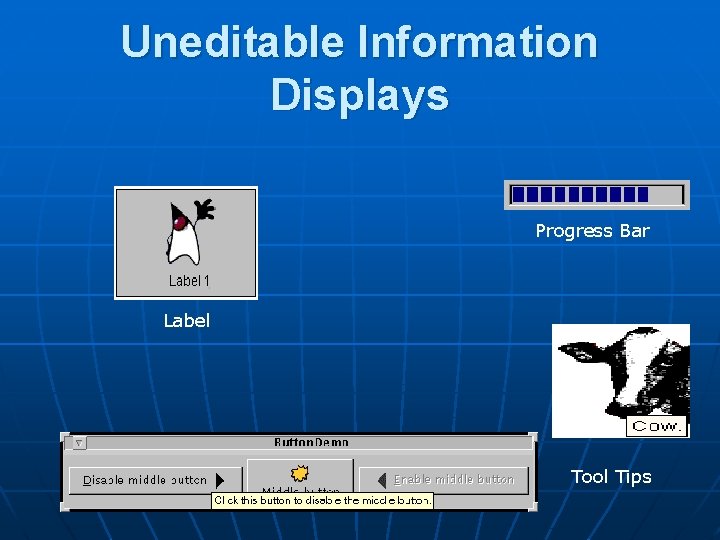
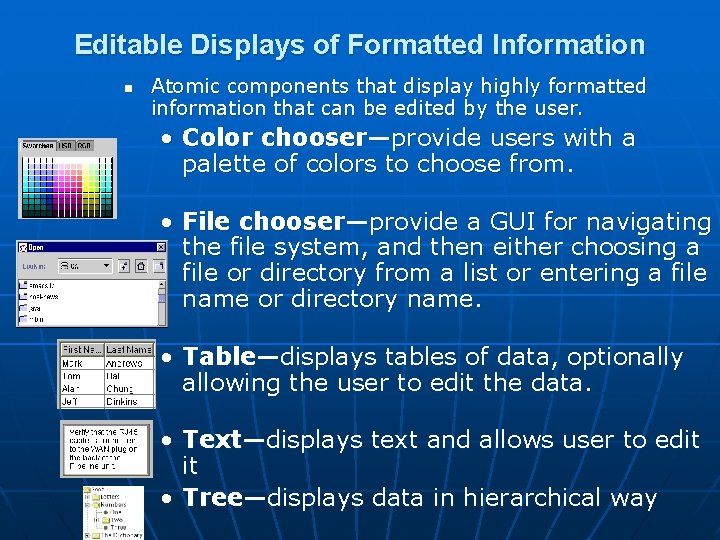
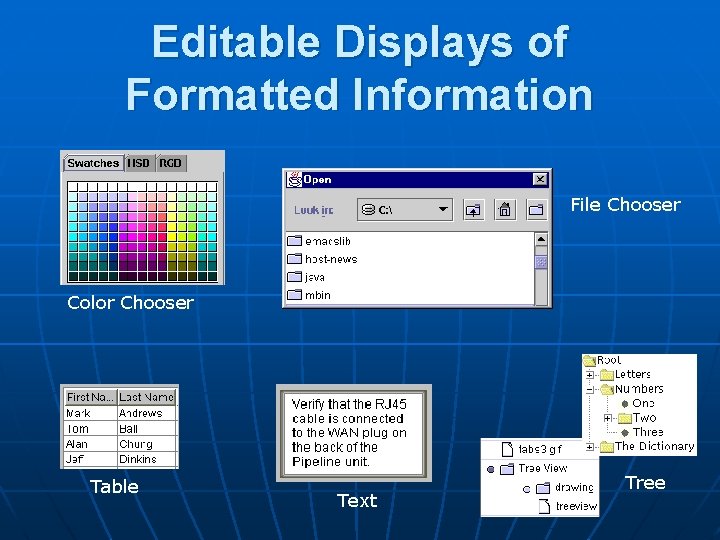
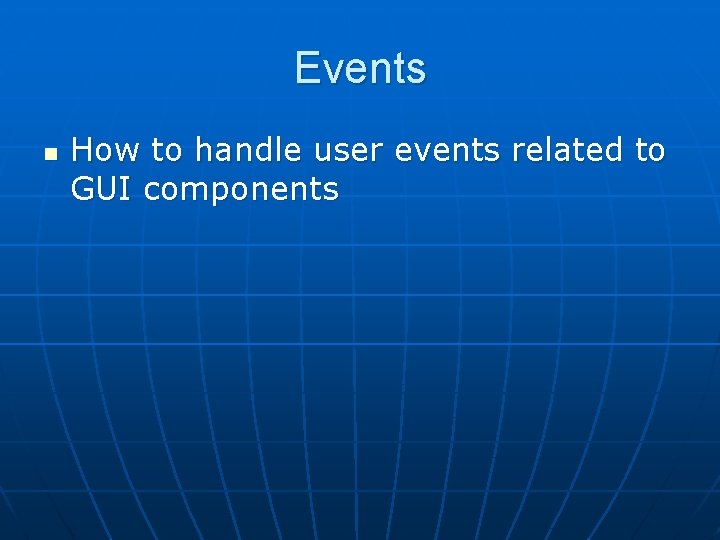
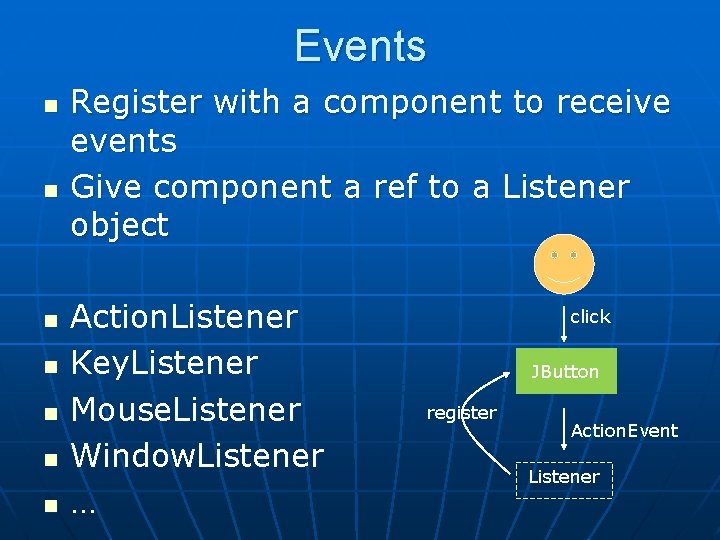
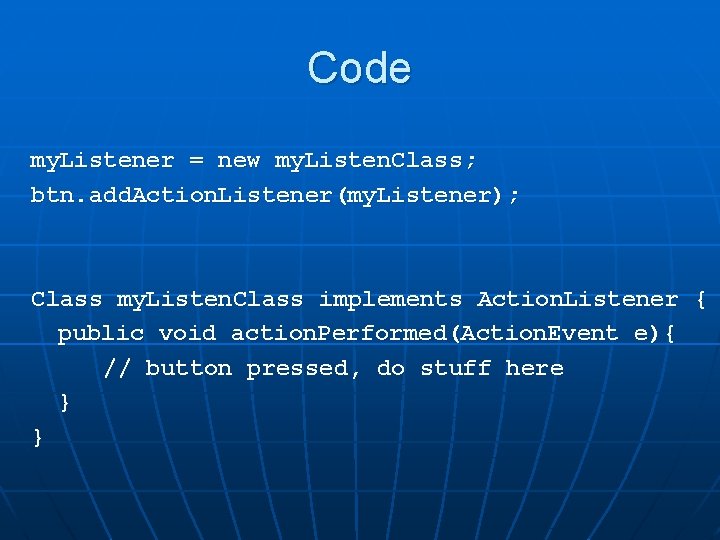
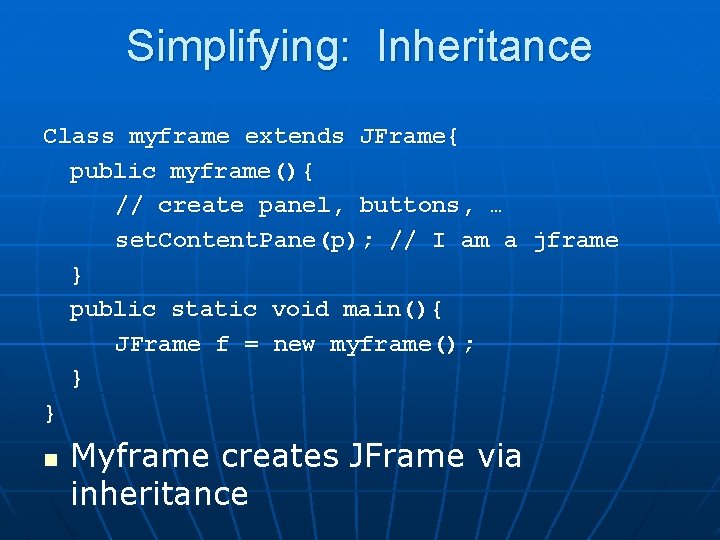
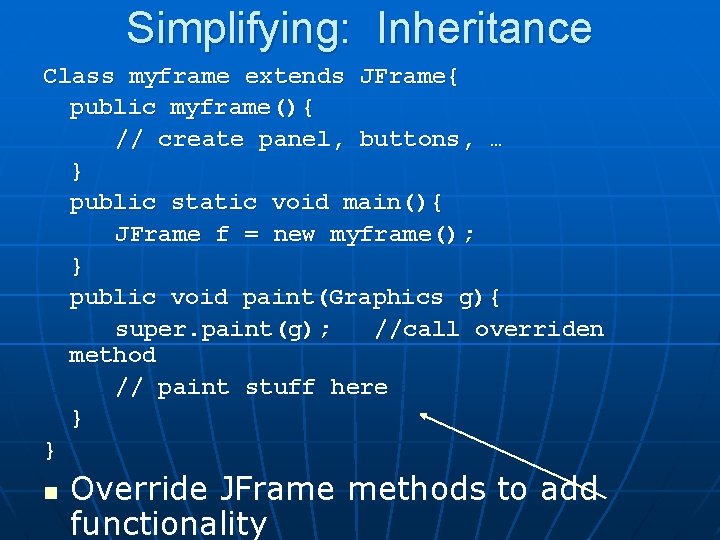
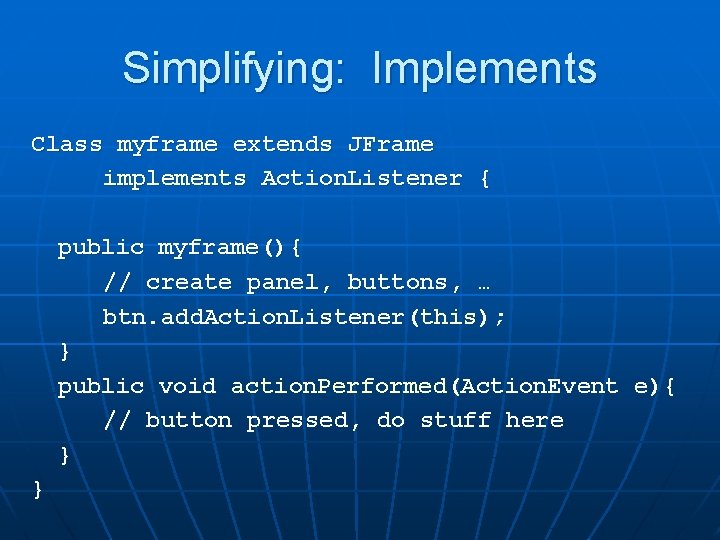
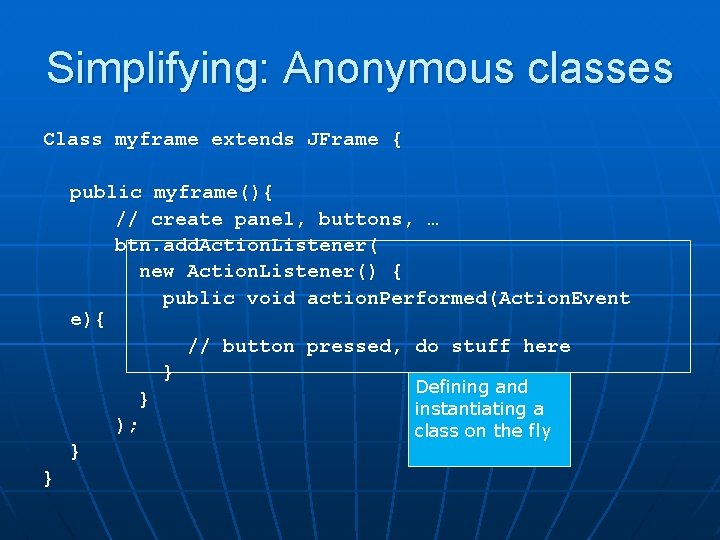
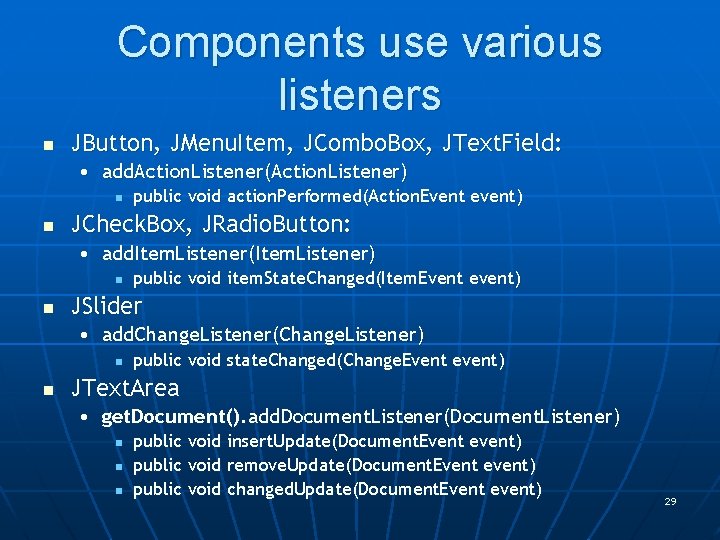
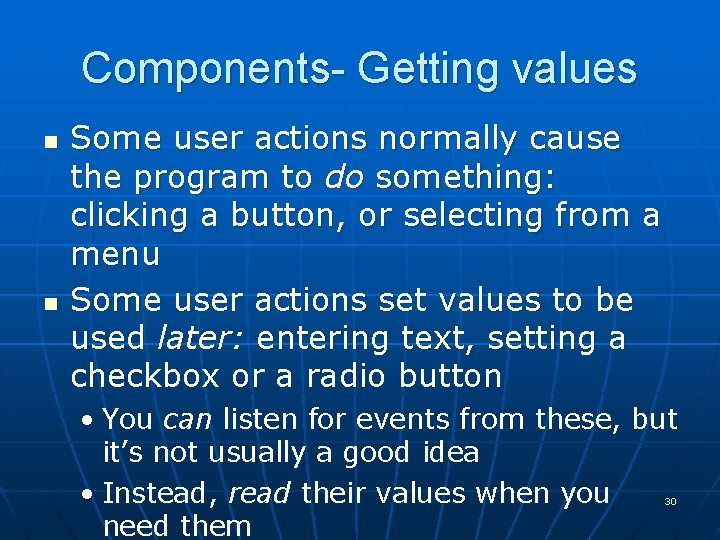
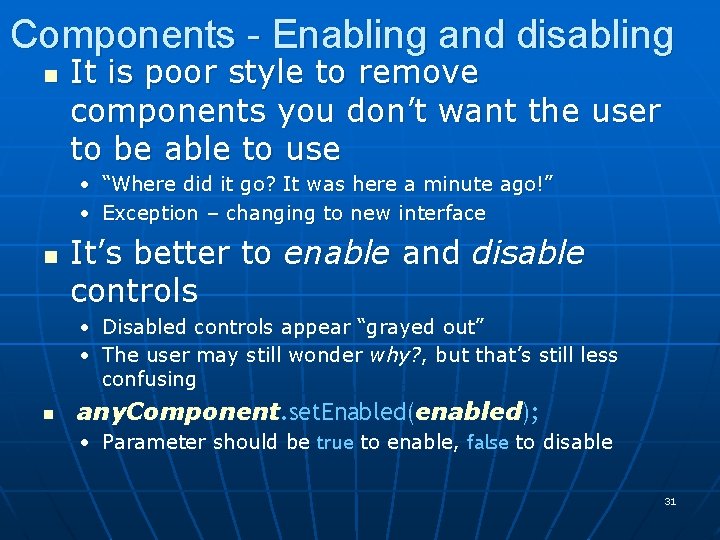
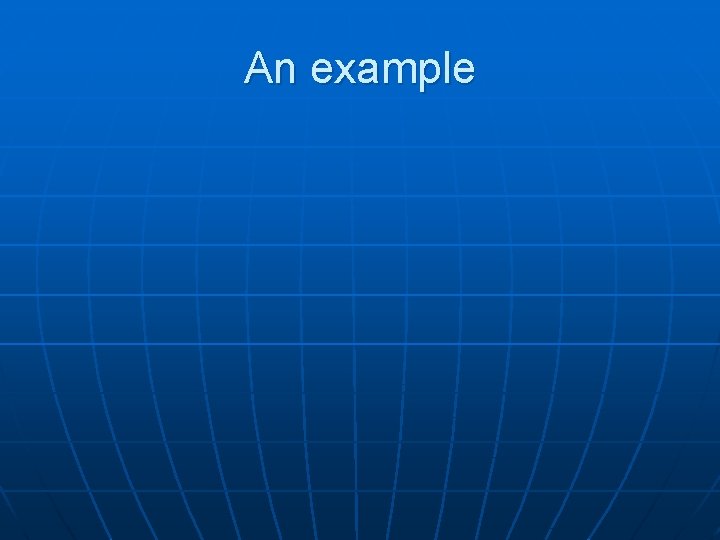
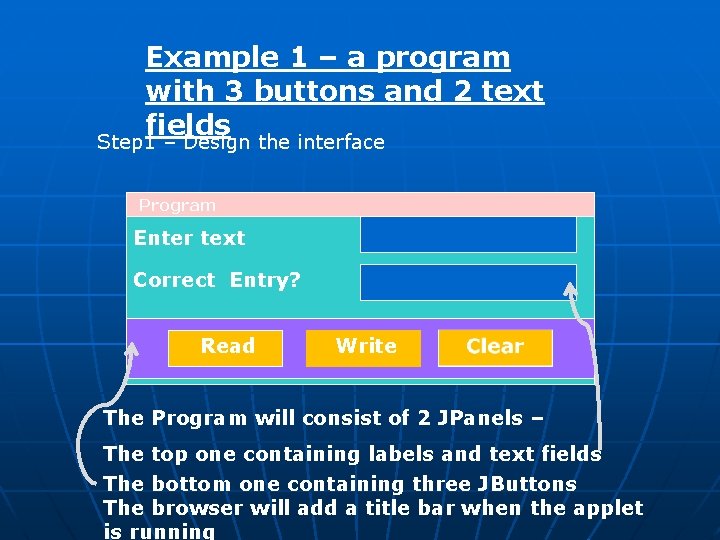
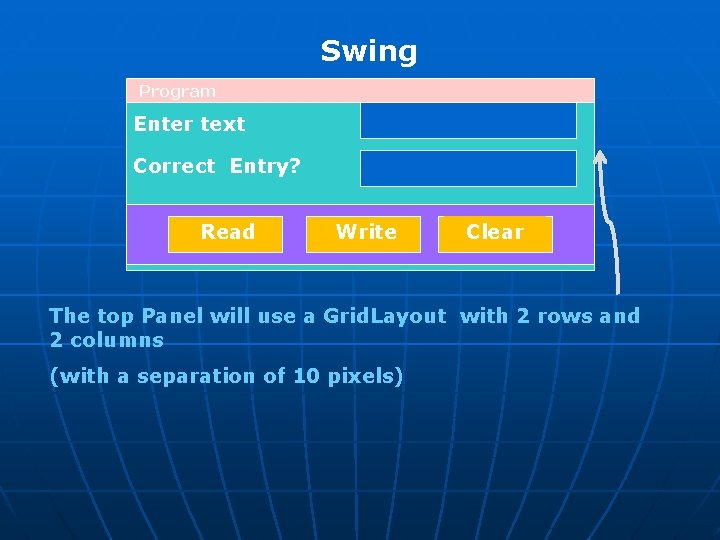
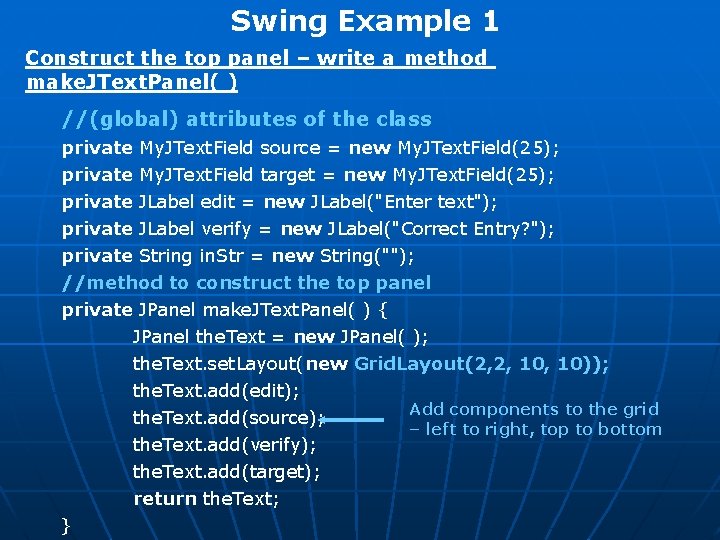
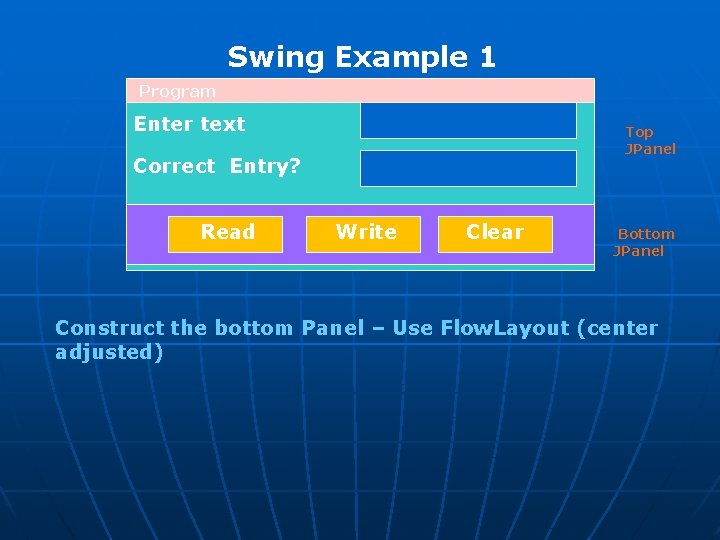
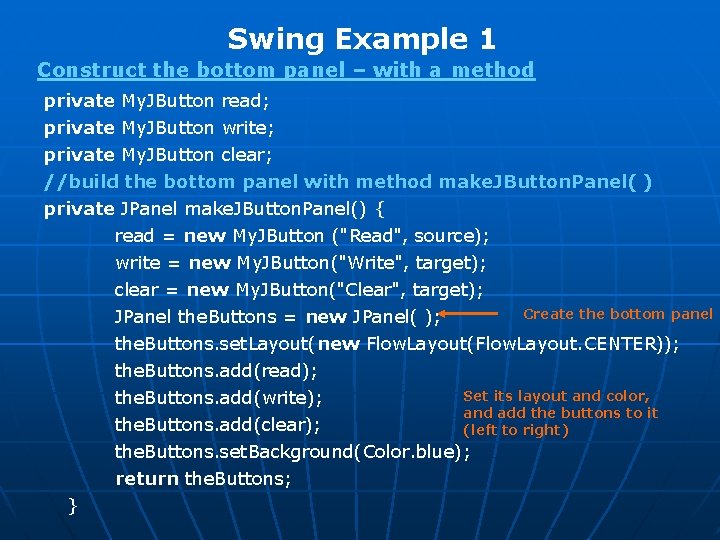
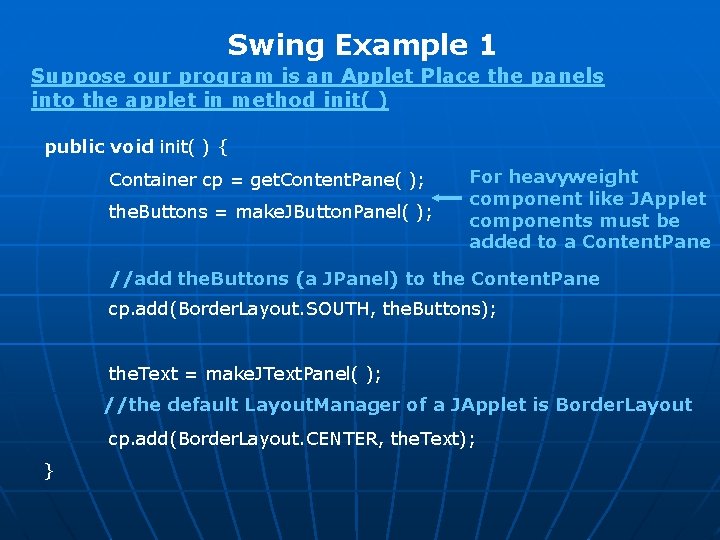
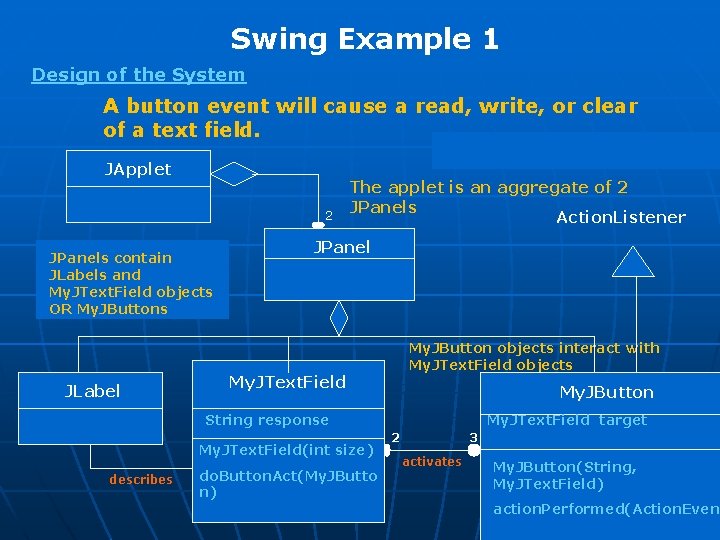
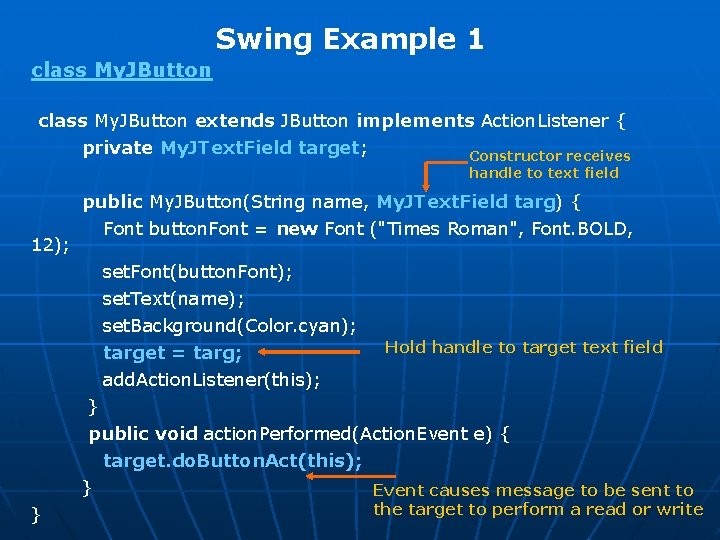
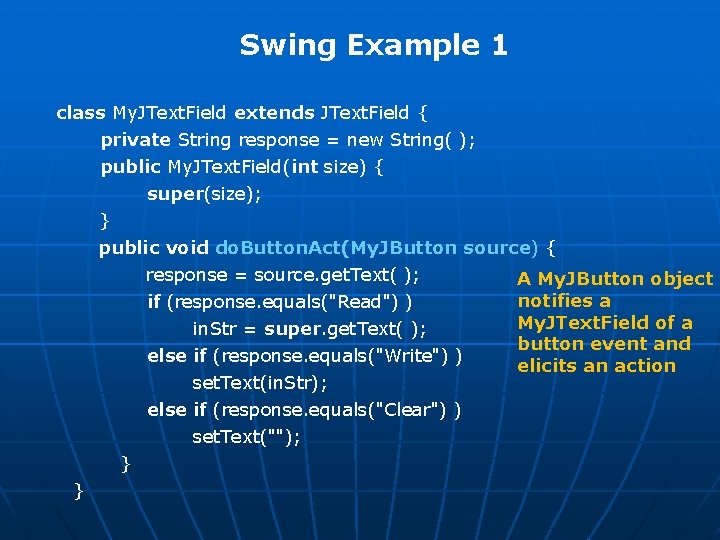
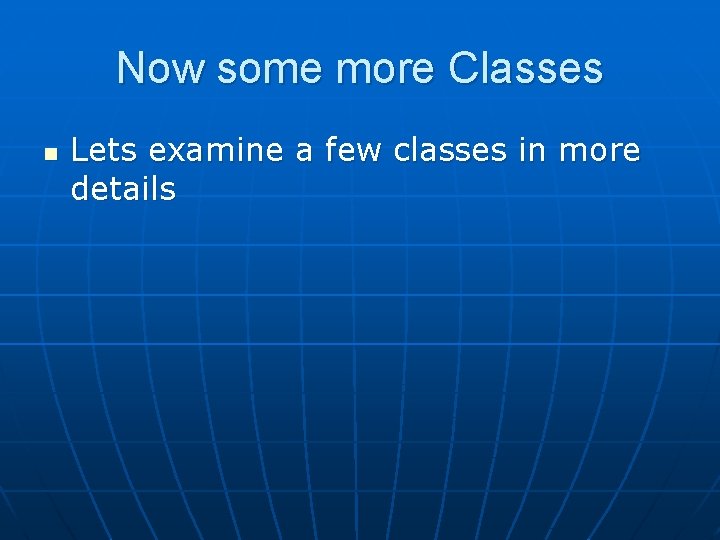
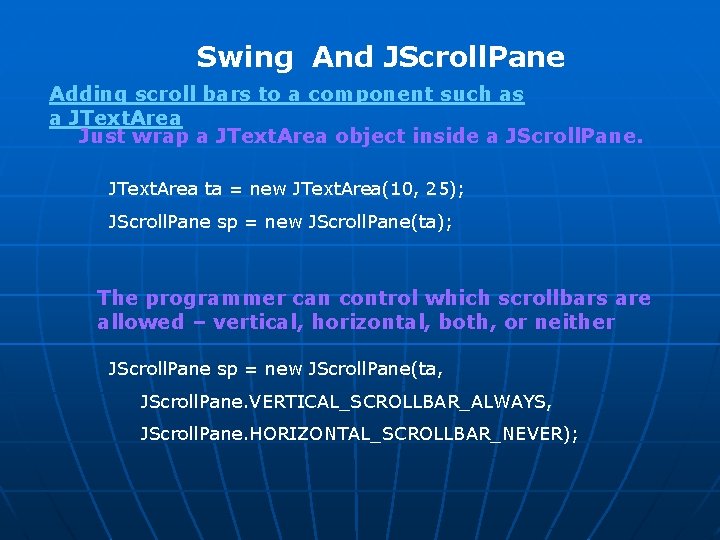
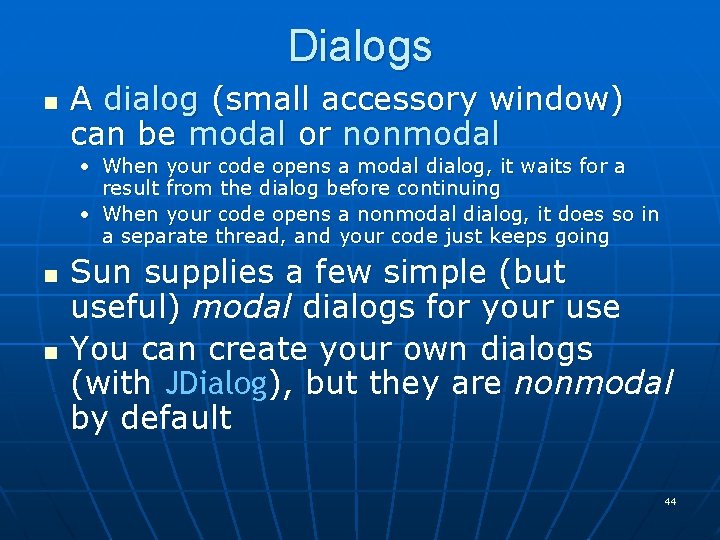
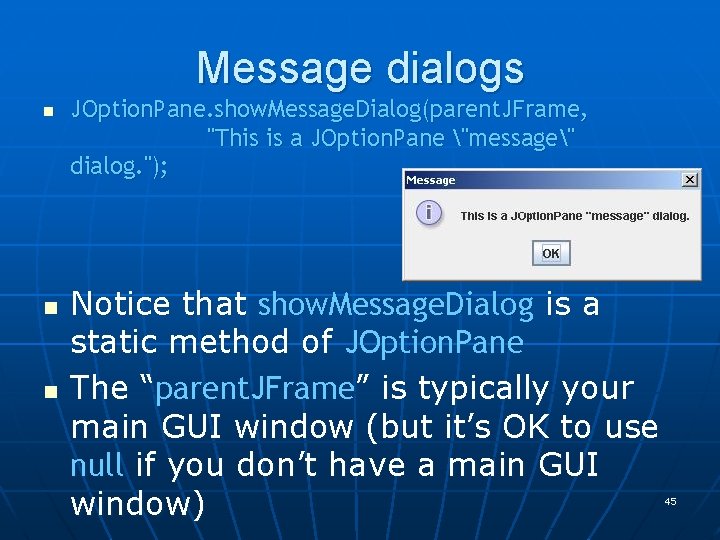
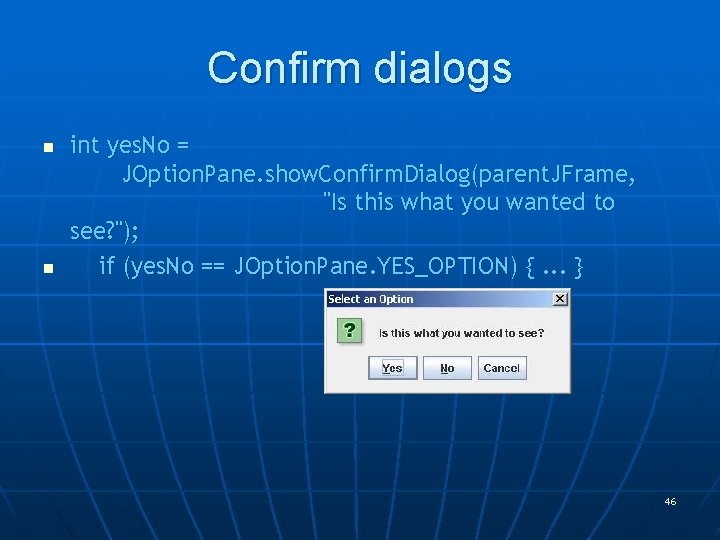
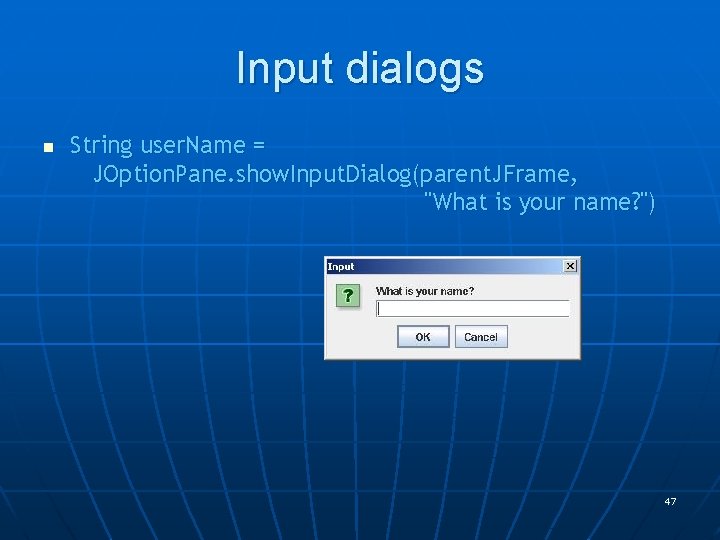
![Option dialogs n n Object[] options = new String[] {"English", "Chinese", "French", "German" }; Option dialogs n n Object[] options = new String[] {"English", "Chinese", "French", "German" };](https://slidetodoc.com/presentation_image_h2/384449579051cfddef07e896ba449a47/image-48.jpg)
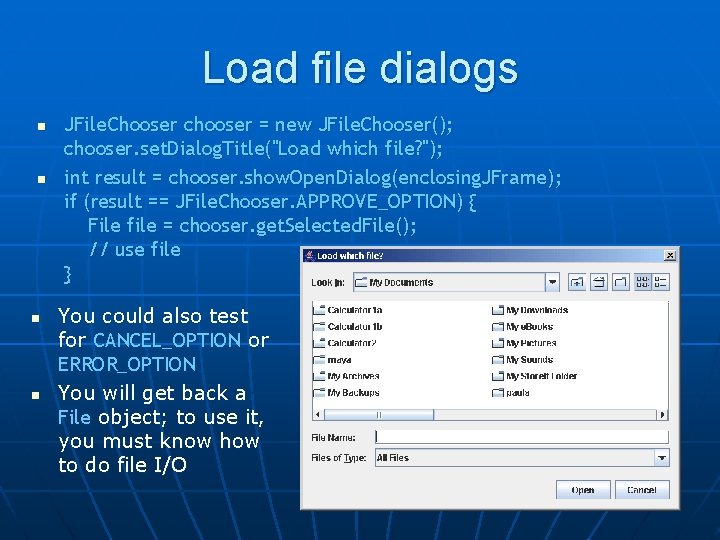
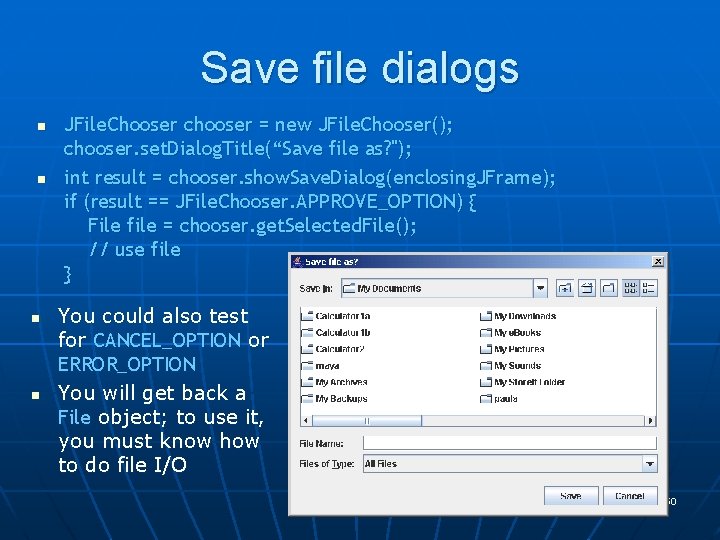
- Slides: 50
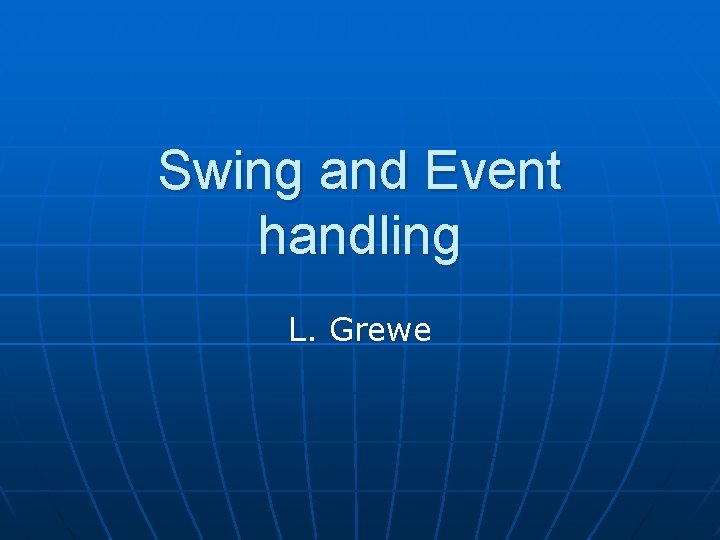
Swing and Event handling L. Grewe
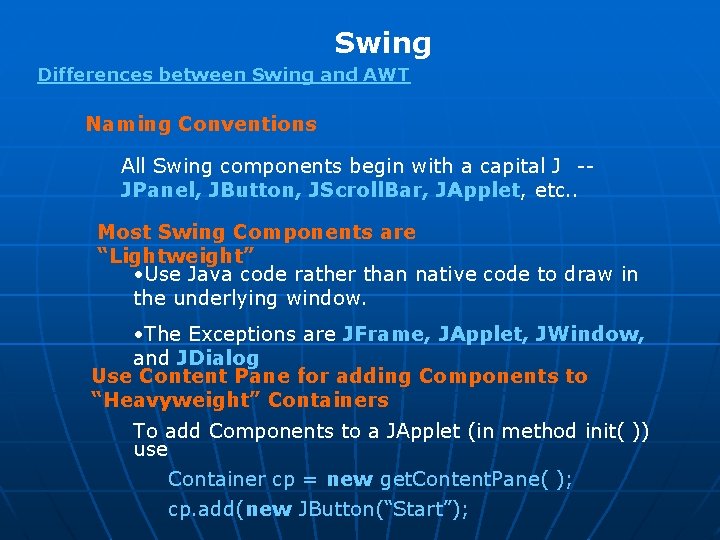
Swing Differences between Swing and AWT Naming Conventions All Swing components begin with a capital J -JPanel, JButton, JScroll. Bar, JApplet, etc. . Most Swing Components are “Lightweight” • Use Java code rather than native code to draw in the underlying window. • The Exceptions are JFrame, JApplet, JWindow, and JDialog Use Content Pane for adding Components to “Heavyweight” Containers To add Components to a JApplet (in method init( )) use Container cp = new get. Content. Pane( ); cp. add(new JButton(“Start”);
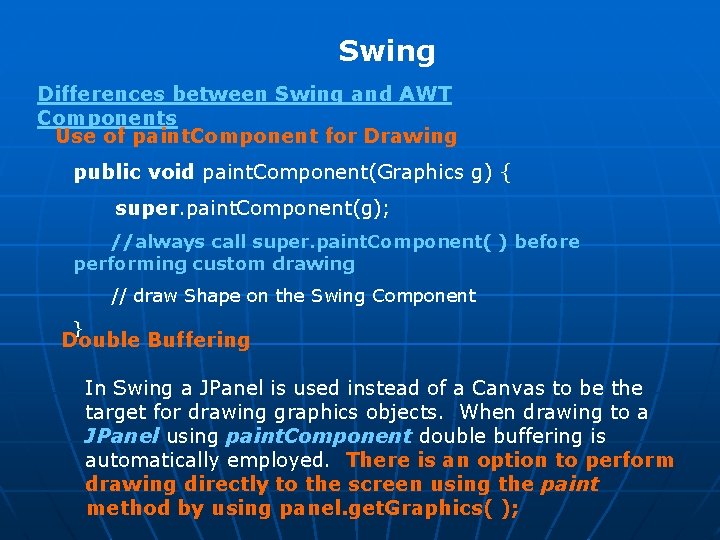
Swing Differences between Swing and AWT Components Use of paint. Component for Drawing public void paint. Component(Graphics g) { super. paint. Component(g); //always call super. paint. Component( ) before performing custom drawing // draw Shape on the Swing Component } Double Buffering In Swing a JPanel is used instead of a Canvas to be the target for drawing graphics objects. When drawing to a JPanel using paint. Component double buffering is automatically employed. There is an option to perform drawing directly to the screen using the paint method by using panel. get. Graphics( );
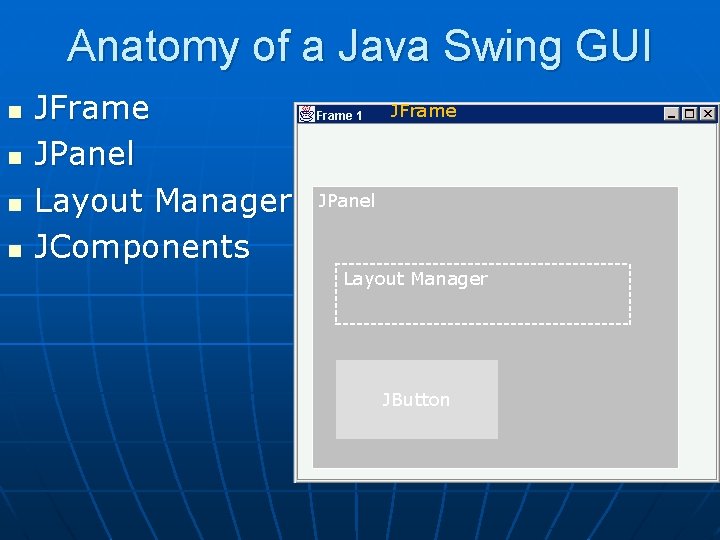
Anatomy of a Java Swing GUI n n JFrame JPanel Layout Manager JComponents JFrame JPanel Layout Manager JButton
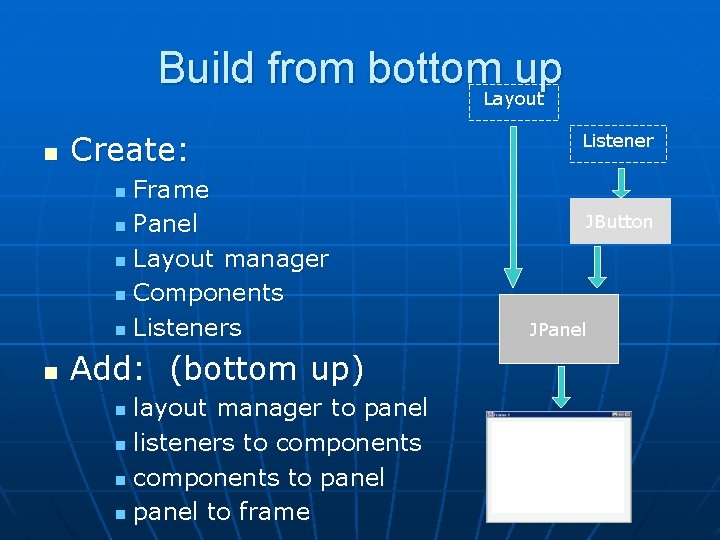
Build from bottom up Layout n Create: Frame n Panel n Layout manager n Components n Listeners Listener n n JButton JPanel Add: (bottom up) layout manager to panel n listeners to components n components to panel n panel to frame n JFrame
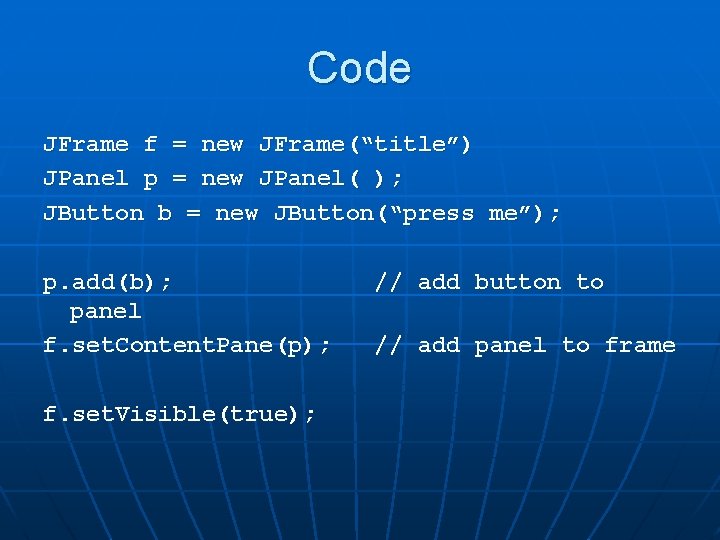
Code JFrame f = new JFrame(“title”) JPanel p = new JPanel( ); JButton b = new JButton(“press me”); p. add(b); panel f. set. Content. Pane(p); f. set. Visible(true); // add button to // add panel to frame
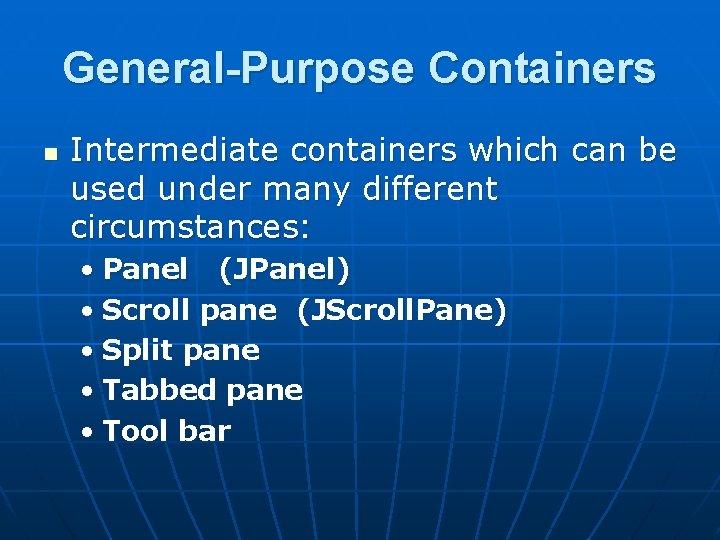
General-Purpose Containers n Intermediate containers which can be used under many different circumstances: • Panel (JPanel) • Scroll pane (JScroll. Pane) • Split pane • Tabbed pane • Tool bar
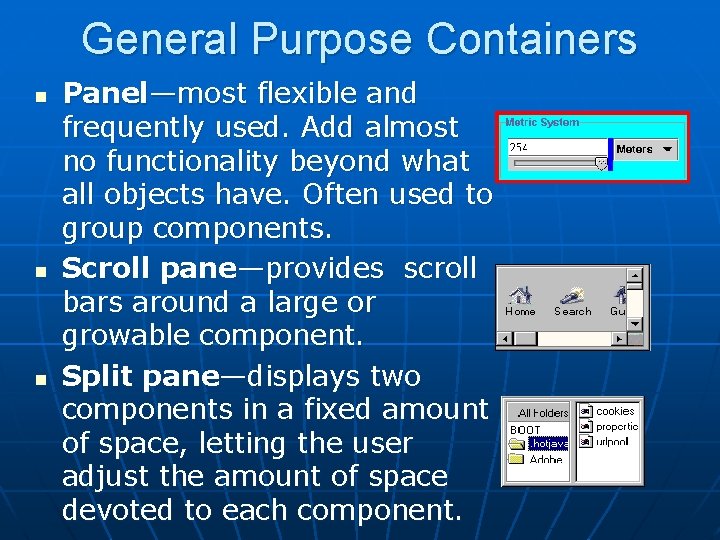
General Purpose Containers n n n Panel—most flexible and frequently used. Add almost no functionality beyond what all objects have. Often used to group components. Scroll pane—provides scroll bars around a large or growable component. Split pane—displays two components in a fixed amount of space, letting the user adjust the amount of space devoted to each component.
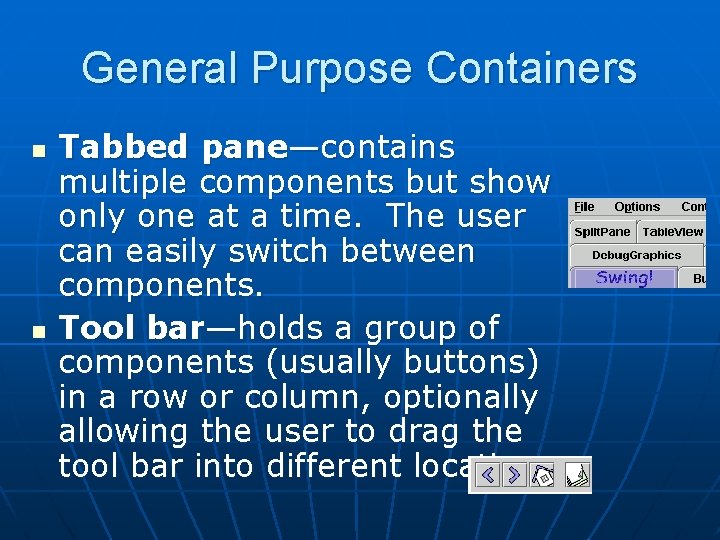
General Purpose Containers n n Tabbed pane—contains multiple components but show only one at a time. The user can easily switch between components. Tool bar—holds a group of components (usually buttons) in a row or column, optionally allowing the user to drag the tool bar into different locations.
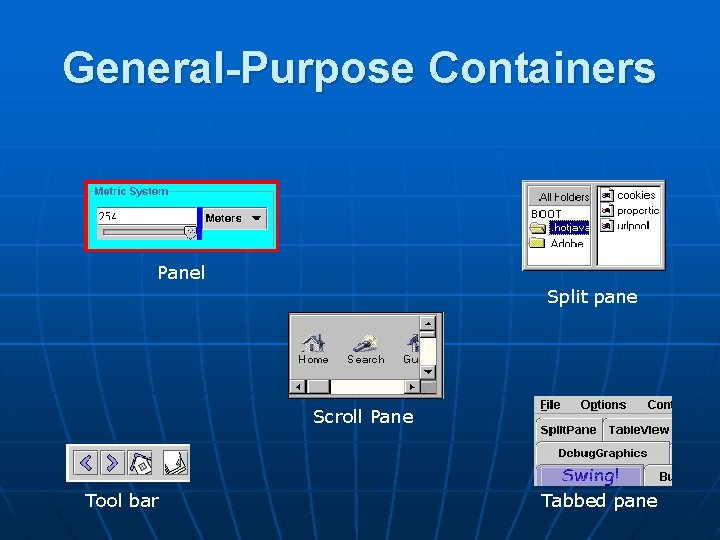
General-Purpose Containers Panel Split pane Scroll Pane Tool bar Tabbed pane
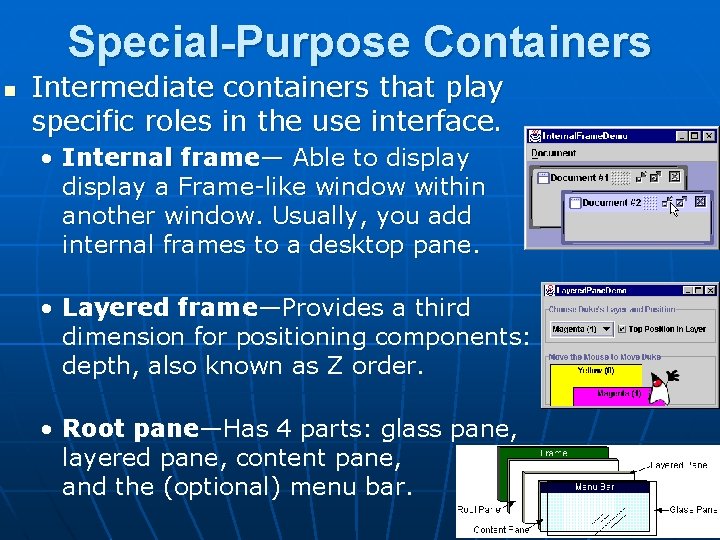
Special-Purpose Containers n Intermediate containers that play specific roles in the use interface. • Internal frame— Able to display a Frame-like window within another window. Usually, you add internal frames to a desktop pane. • Layered frame—Provides a third dimension for positioning components: depth, also known as Z order. • Root pane—Has 4 parts: glass pane, layered pane, content pane, and the (optional) menu bar.
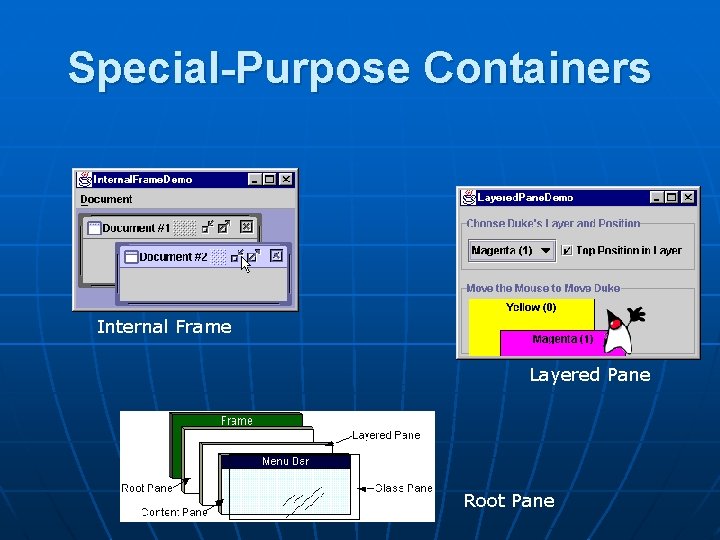
Special-Purpose Containers Internal Frame Layered Pane Root Pane
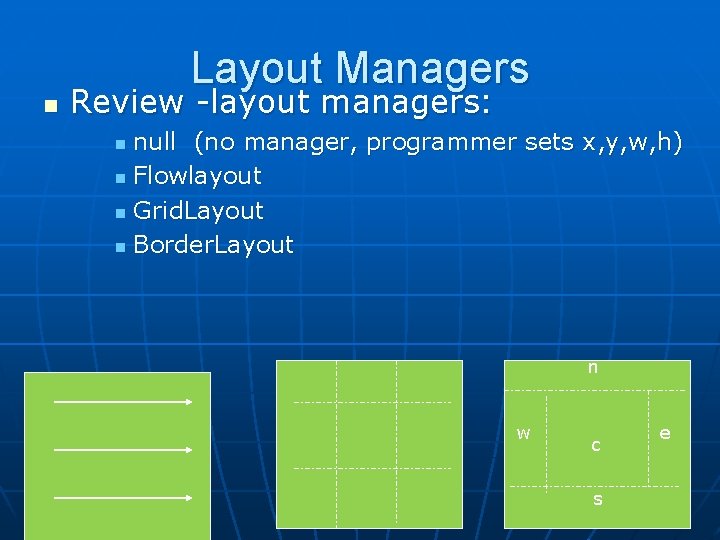
Layout Managers n Review -layout managers: null (no manager, programmer sets x, y, w, h) n Flowlayout n Grid. Layout n Border. Layout n n w c s e
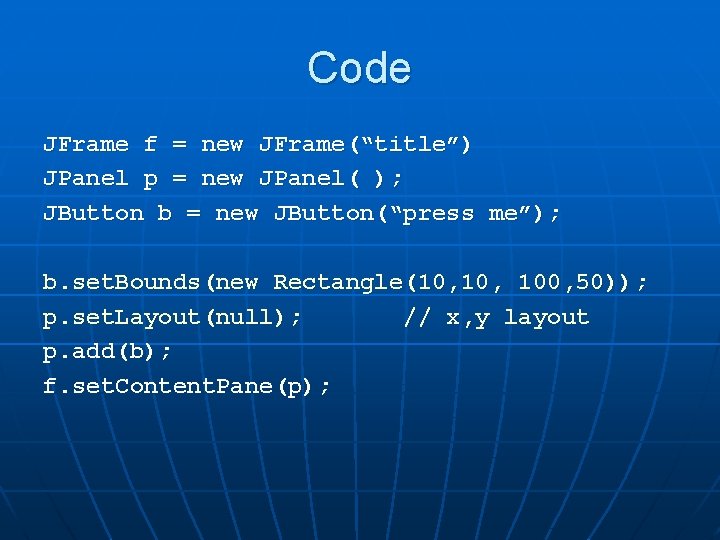
Code JFrame f = new JFrame(“title”) JPanel p = new JPanel( ); JButton b = new JButton(“press me”); b. set. Bounds(new Rectangle(10, 100, 50)); p. set. Layout(null); // x, y layout p. add(b); f. set. Content. Pane(p);
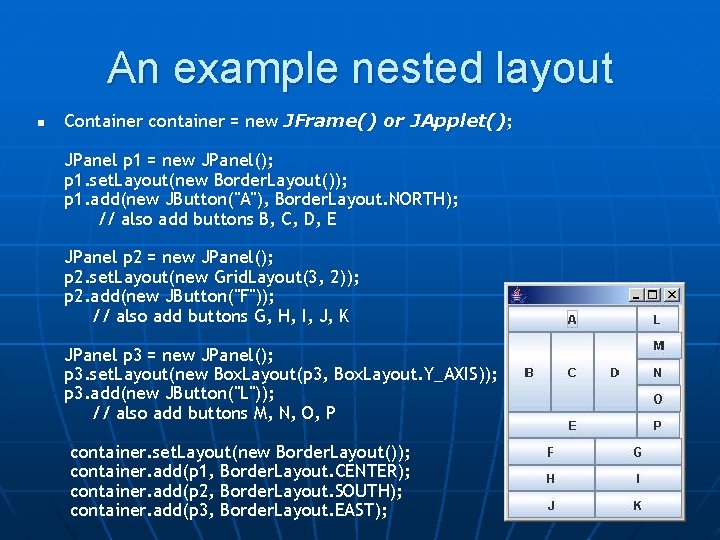
An example nested layout n Container container = new JFrame() or JApplet(); JPanel p 1 = new JPanel(); p 1. set. Layout(new Border. Layout()); p 1. add(new JButton("A"), Border. Layout. NORTH); // also add buttons B, C, D, E JPanel p 2 = new JPanel(); p 2. set. Layout(new Grid. Layout(3, 2)); p 2. add(new JButton("F")); // also add buttons G, H, I, J, K JPanel p 3 = new JPanel(); p 3. set. Layout(new Box. Layout(p 3, Box. Layout. Y_AXIS)); p 3. add(new JButton("L")); // also add buttons M, N, O, P container. set. Layout(new Border. Layout()); container. add(p 1, Border. Layout. CENTER); container. add(p 2, Border. Layout. SOUTH); container. add(p 3, Border. Layout. EAST); 15
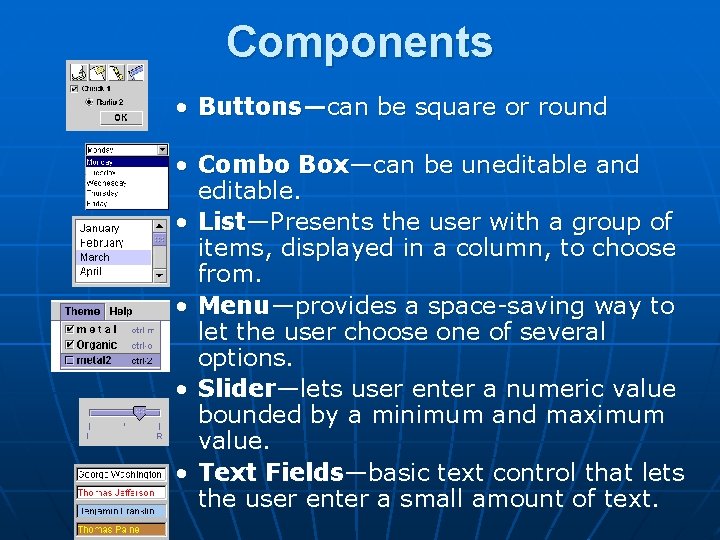
Components • Buttons—can be square or round • Combo Box—can be uneditable and editable. • List—Presents the user with a group of items, displayed in a column, to choose from. • Menu—provides a space-saving way to let the user choose one of several options. • Slider—lets user enter a numeric value bounded by a minimum and maximum value. • Text Fields—basic text control that lets the user enter a small amount of text.
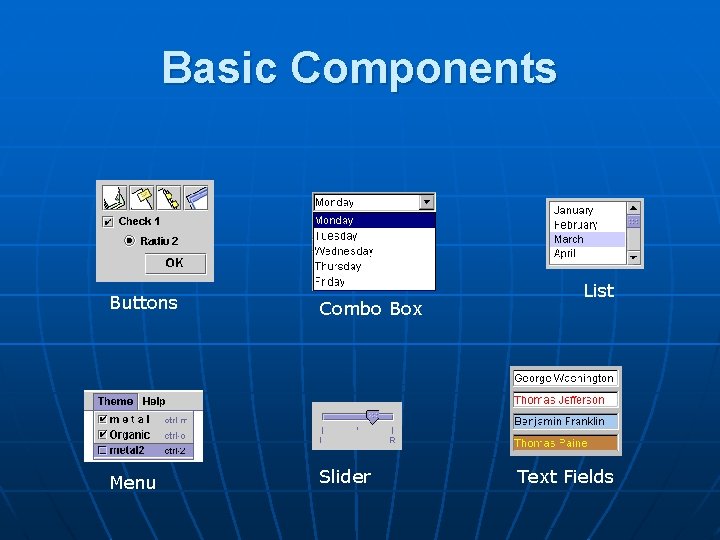
Basic Components Buttons Combo Box Menu Slider List Text Fields
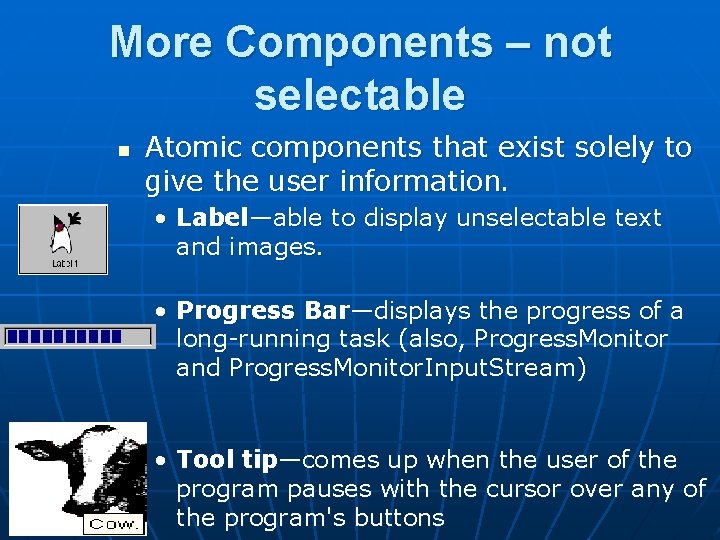
More Components – not selectable n Atomic components that exist solely to give the user information. • Label—able to display unselectable text and images. • Progress Bar—displays the progress of a long-running task (also, Progress. Monitor and Progress. Monitor. Input. Stream) • Tool tip—comes up when the user of the program pauses with the cursor over any of the program's buttons
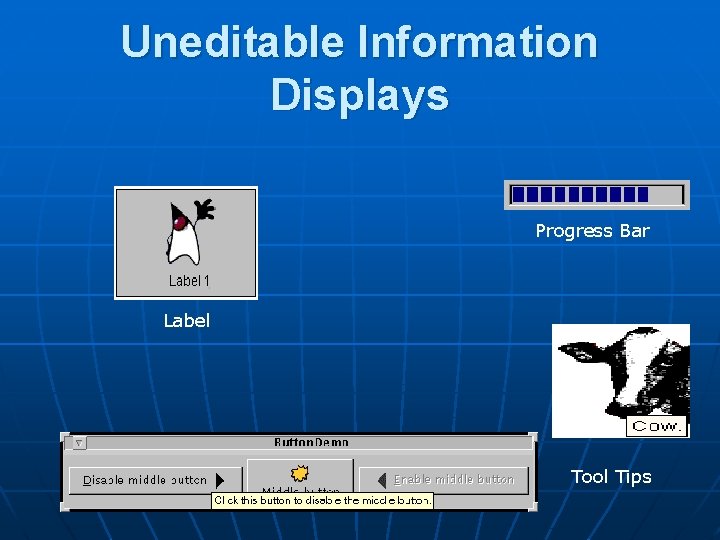
Uneditable Information Displays Progress Bar Label Tool Tips
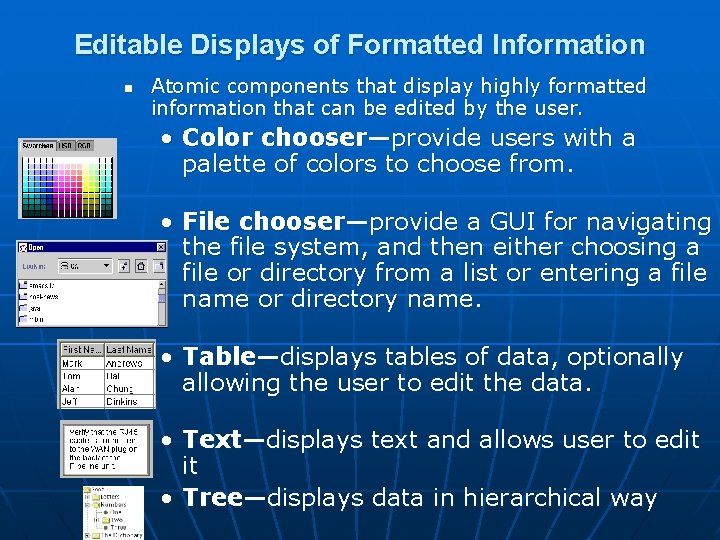
Editable Displays of Formatted Information n Atomic components that display highly formatted information that can be edited by the user. • Color chooser—provide users with a palette of colors to choose from. • File chooser—provide a GUI for navigating the file system, and then either choosing a file or directory from a list or entering a file name or directory name. • Table—displays tables of data, optionally allowing the user to edit the data. • Text—displays text and allows user to edit it • Tree—displays data in hierarchical way
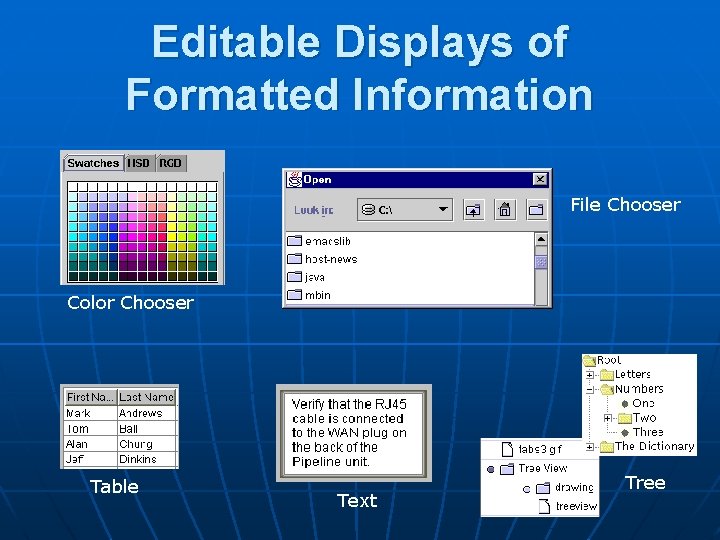
Editable Displays of Formatted Information File Chooser Color Chooser Table Text Tree
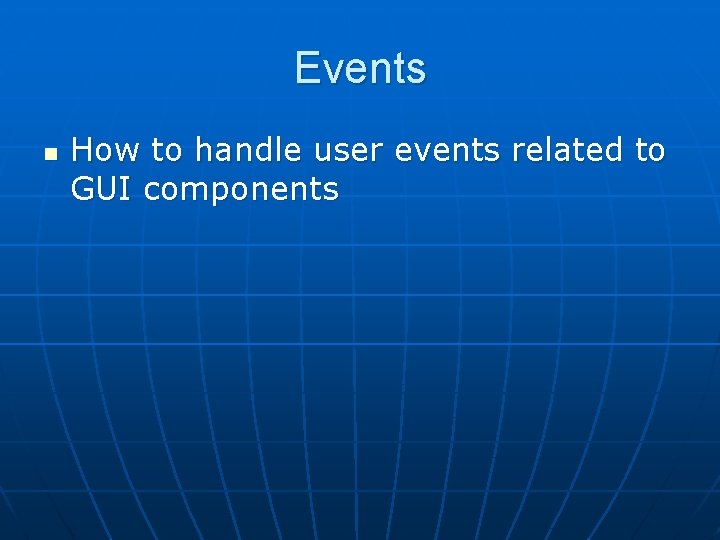
Events n How to handle user events related to GUI components
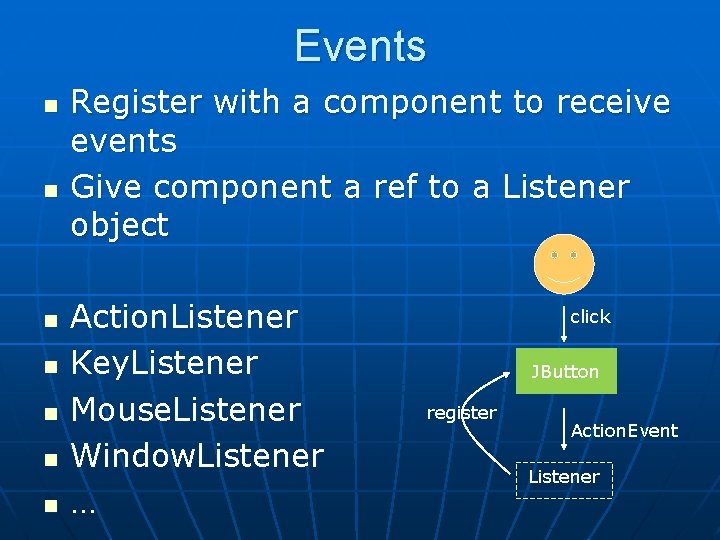
Events n n n n Register with a component to receive events Give component a ref to a Listener object Action. Listener Key. Listener Mouse. Listener Window. Listener … click JButton register Action. Event Listener
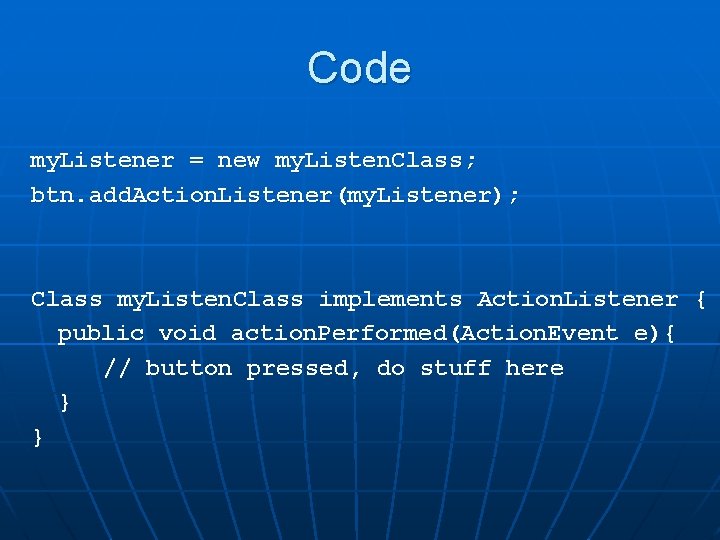
Code my. Listener = new my. Listen. Class; btn. add. Action. Listener(my. Listener); Class my. Listen. Class implements Action. Listener { public void action. Performed(Action. Event e){ // button pressed, do stuff here } }
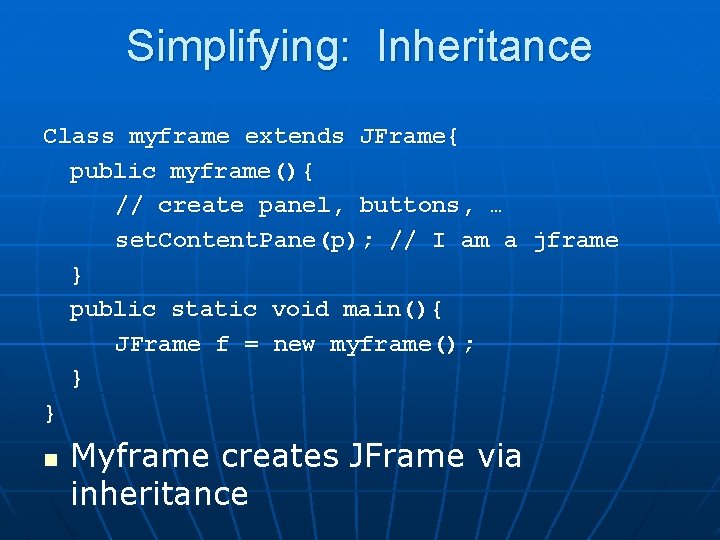
Simplifying: Inheritance Class myframe extends JFrame{ public myframe(){ // create panel, buttons, … set. Content. Pane(p); // I am a jframe } public static void main(){ JFrame f = new myframe(); } } n Myframe creates JFrame via inheritance
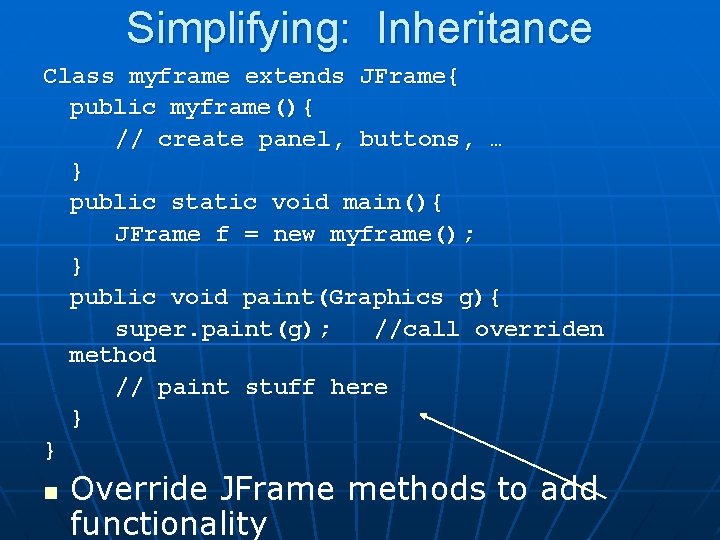
Simplifying: Inheritance Class myframe extends JFrame{ public myframe(){ // create panel, buttons, … } public static void main(){ JFrame f = new myframe(); } public void paint(Graphics g){ super. paint(g); //call overriden method // paint stuff here } } n Override JFrame methods to add functionality
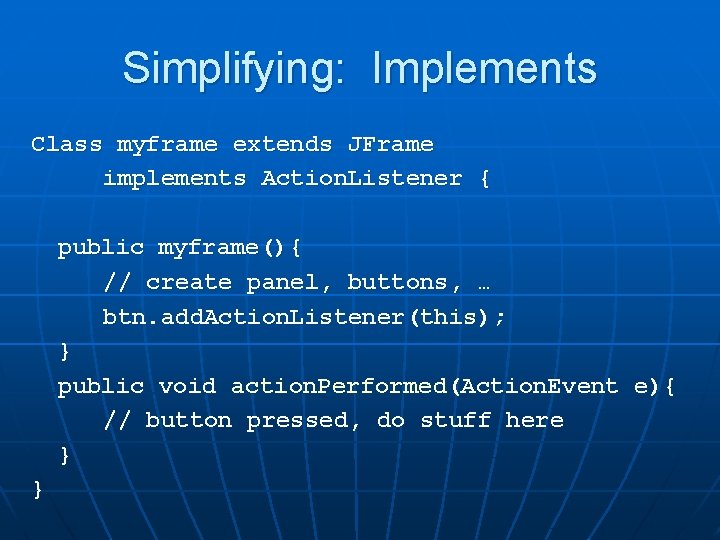
Simplifying: Implements Class myframe extends JFrame implements Action. Listener { public myframe(){ // create panel, buttons, … btn. add. Action. Listener(this); } public void action. Performed(Action. Event e){ // button pressed, do stuff here } }
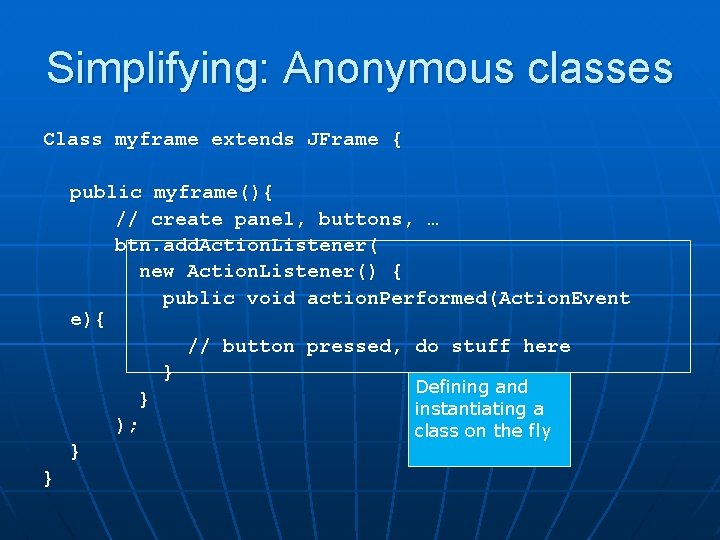
Simplifying: Anonymous classes Class myframe extends JFrame { public myframe(){ // create panel, buttons, … btn. add. Action. Listener( new Action. Listener() { public void action. Performed(Action. Event e){ // button pressed, do stuff here } Defining and } instantiating a ); class on the fly } }
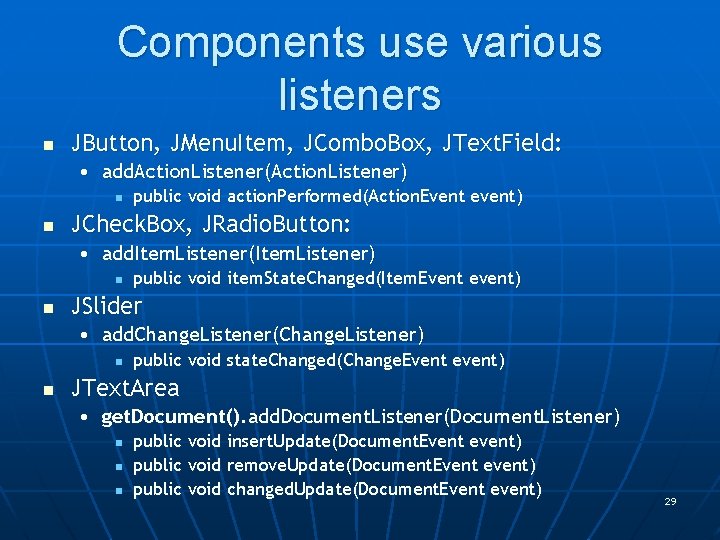
Components use various listeners n JButton, JMenu. Item, JCombo. Box, JText. Field: • add. Action. Listener(Action. Listener) n n public void action. Performed(Action. Event event) JCheck. Box, JRadio. Button: • add. Item. Listener(Item. Listener) n n public void item. State. Changed(Item. Event event) JSlider • add. Change. Listener(Change. Listener) n n public void state. Changed(Change. Event event) JText. Area • get. Document(). add. Document. Listener(Document. Listener) n n n public void insert. Update(Document. Event event) public void remove. Update(Document. Event event) public void changed. Update(Document. Event event) 29
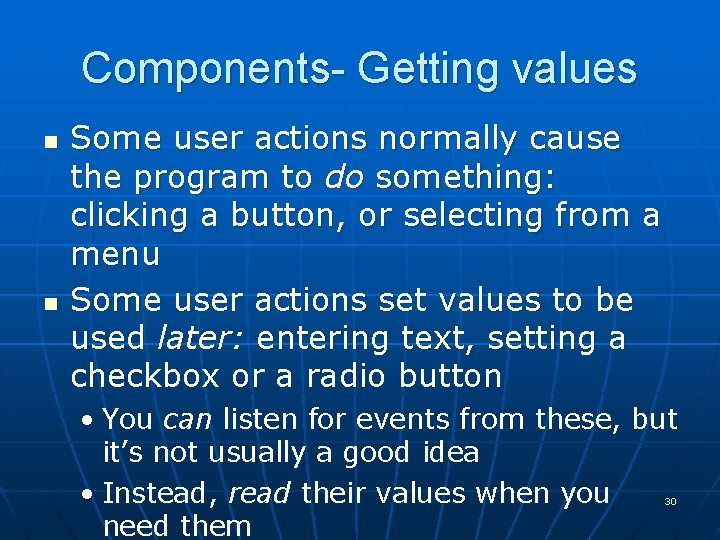
Components- Getting values n n Some user actions normally cause the program to do something: clicking a button, or selecting from a menu Some user actions set values to be used later: entering text, setting a checkbox or a radio button • You can listen for events from these, but it’s not usually a good idea • Instead, read their values when you need them 30
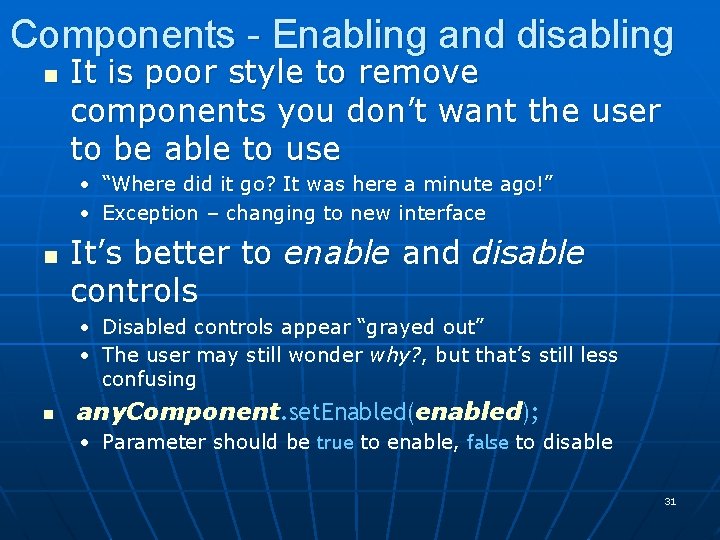
Components - Enabling and disabling n It is poor style to remove components you don’t want the user to be able to use • “Where did it go? It was here a minute ago!” • Exception – changing to new interface n It’s better to enable and disable controls • Disabled controls appear “grayed out” • The user may still wonder why? , but that’s still less confusing n any. Component. set. Enabled(enabled); • Parameter should be true to enable, false to disable 31
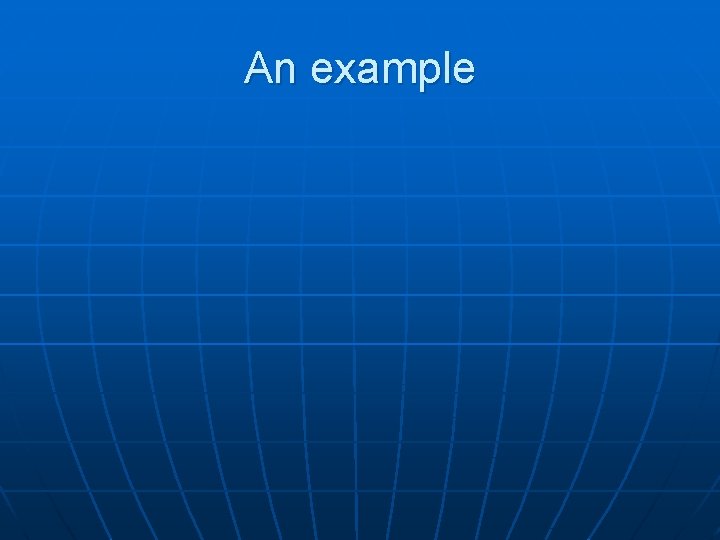
An example
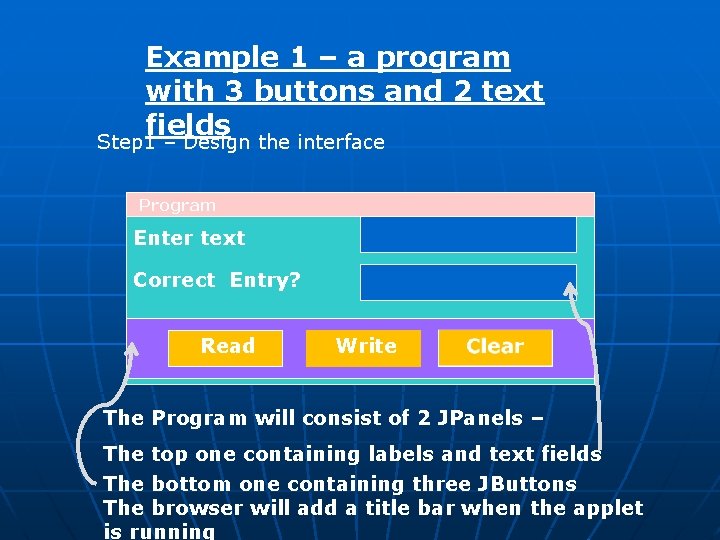
Example 1 – a program with 3 buttons and 2 text fields Step 1 – Design the interface Program Enter text Correct Entry? Read Write The Program will consist of 2 JPanels – The top one containing labels and text fields The bottom one containing three JButtons The browser will add a title bar when the applet is running
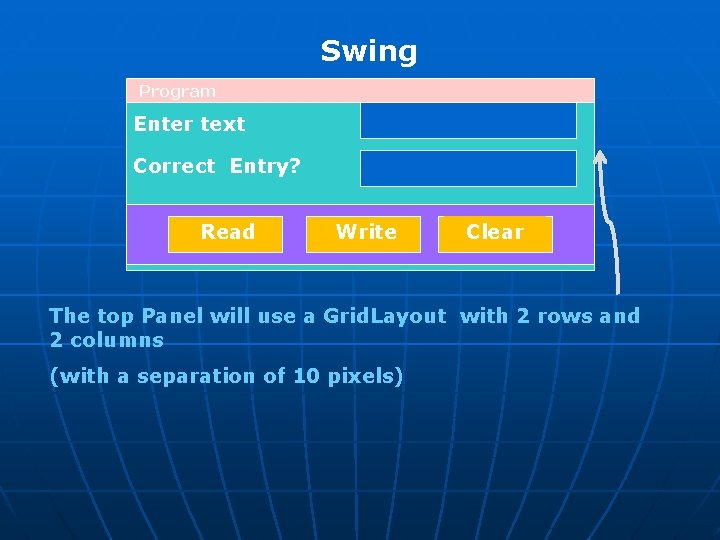
Swing Program Enter text Correct Entry? Read Write Clear The top Panel will use a Grid. Layout with 2 rows and 2 columns (with a separation of 10 pixels)
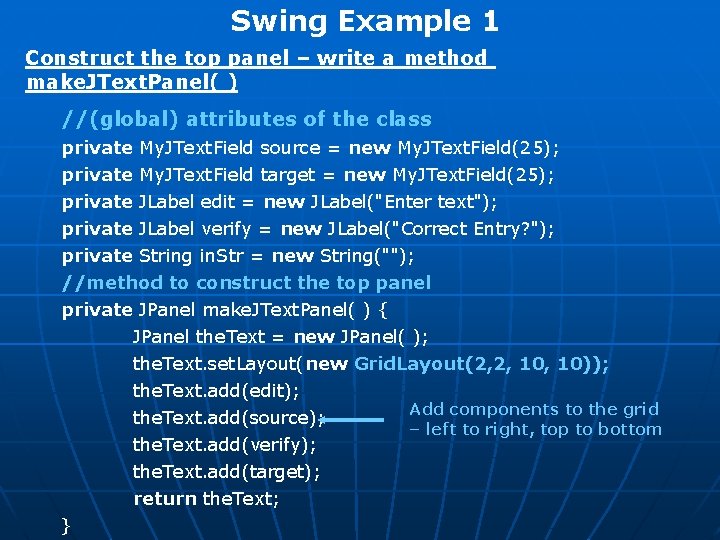
Swing Example 1 Construct the top panel – write a method make. JText. Panel( ) //(global) attributes of the class private My. JText. Field source = new My. JText. Field(25); My. JText. Field target = new My. JText. Field(25); JLabel edit = new JLabel("Enter text"); JLabel verify = new JLabel("Correct Entry? "); private String in. Str = new String(""); //method to construct the top panel private JPanel make. JText. Panel( ) { JPanel the. Text = new JPanel( ); the. Text. set. Layout(new Grid. Layout(2, 2, 10)); the. Text. add(edit); Add components to the grid the. Text. add(source); – left to right, top to bottom the. Text. add(verify); the. Text. add(target); return the. Text; }
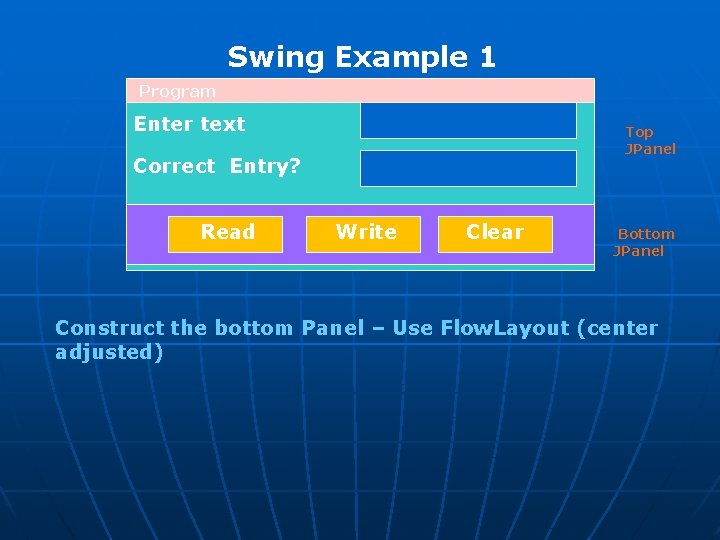
Swing Example 1 Program Enter text Top JPanel Correct Entry? Read Write Clear Bottom JPanel Construct the bottom Panel – Use Flow. Layout (center adjusted)
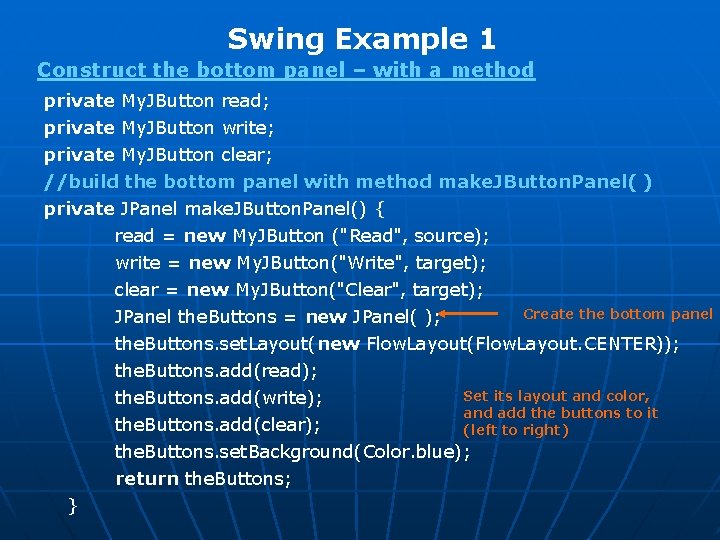
Swing Example 1 Construct the bottom panel – with a method private My. JButton read; private My. JButton write; private My. JButton clear; //build the bottom panel with method make. JButton. Panel( ) private JPanel make. JButton. Panel() { read = new My. JButton ("Read", source); write = new My. JButton("Write", target); clear = new My. JButton("Clear", target); Create the bottom panel JPanel the. Buttons = new JPanel( ); the. Buttons. set. Layout(new Flow. Layout(Flow. Layout. CENTER)); the. Buttons. add(read); Set its layout and color, the. Buttons. add(write); and add the buttons to it the. Buttons. add(clear); (left to right) the. Buttons. set. Background(Color. blue); return the. Buttons; }
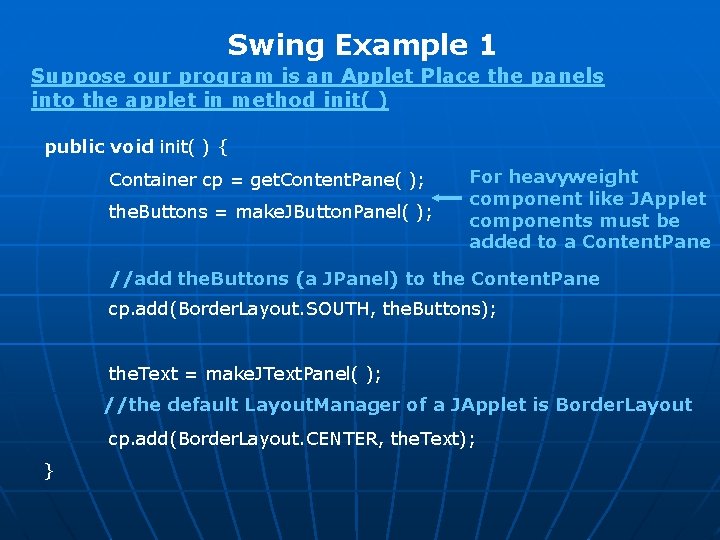
Swing Example 1 Suppose our program is an Applet Place the panels into the applet in method init( ) public void init( ) { Container cp = get. Content. Pane( ); the. Buttons = make. JButton. Panel( ); For heavyweight component like JApplet components must be added to a Content. Pane //add the. Buttons (a JPanel) to the Content. Pane cp. add(Border. Layout. SOUTH, the. Buttons); the. Text = make. JText. Panel( ); //the default Layout. Manager of a JApplet is Border. Layout cp. add(Border. Layout. CENTER, the. Text); }
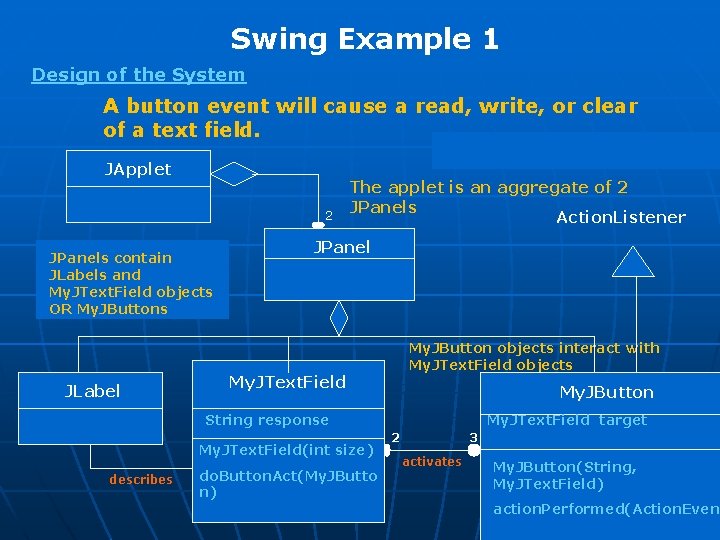
Swing Example 1 Design of the System A button event will cause a read, write, or clear of a text field. JApplet 2 JPanels contain JLabels and My. JText. Field objects OR My. JButtons JLabel The applet is an aggregate of 2 JPanels Action. Listener JPanel My. JButton objects interact with My. JText. Field objects My. JText. Field My. JButton String response My. JText. Field(int size) describes do. Button. Act(My. JButto n) My. JText. Field target 2 3 activates My. JButton(String, My. JText. Field) action. Performed(Action. Event
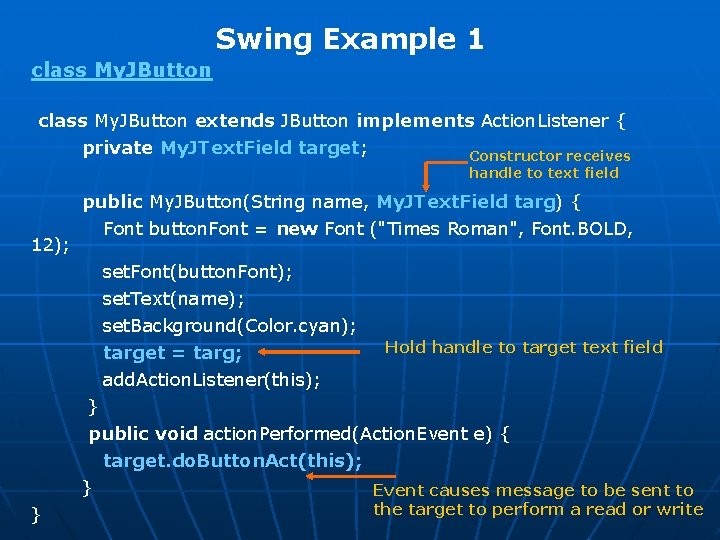
Swing Example 1 class My. JButton extends JButton implements Action. Listener { private My. JText. Field target; 12); Constructor receives handle to text field public My. JButton(String name, My. JText. Field targ) { Font button. Font = new Font ("Times Roman", Font. BOLD, set. Font(button. Font); set. Text(name); set. Background(Color. cyan); target = targ; add. Action. Listener(this); Hold handle to target text field } public void action. Performed(Action. Event e) { target. do. Button. Act(this); } Event causes message to be sent to } the target to perform a read or write
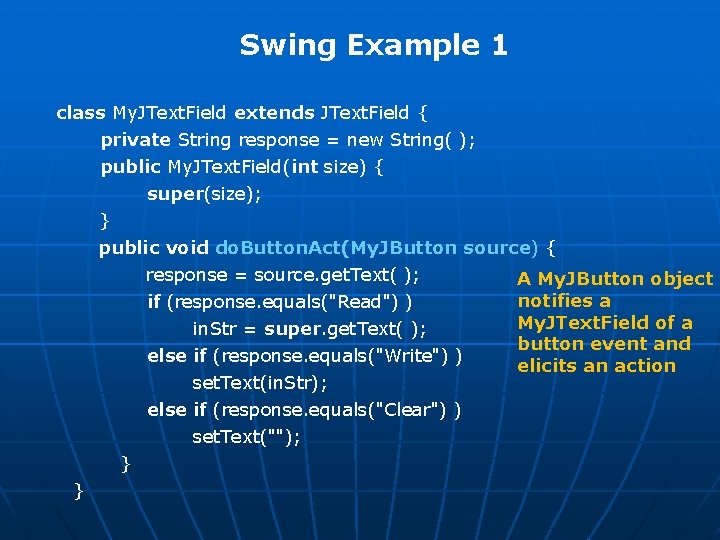
Swing Example 1 class My. JText. Field extends JText. Field { private String response = new String( ); public My. JText. Field(int size) { super(size); } public void do. Button. Act(My. JButton source) { response = source. get. Text( ); if (response. equals("Read") ) in. Str = super. get. Text( ); else if (response. equals("Write") ) set. Text(in. Str); else if (response. equals("Clear") ) set. Text(""); } } A My. JButton object notifies a My. JText. Field of a button event and elicits an action
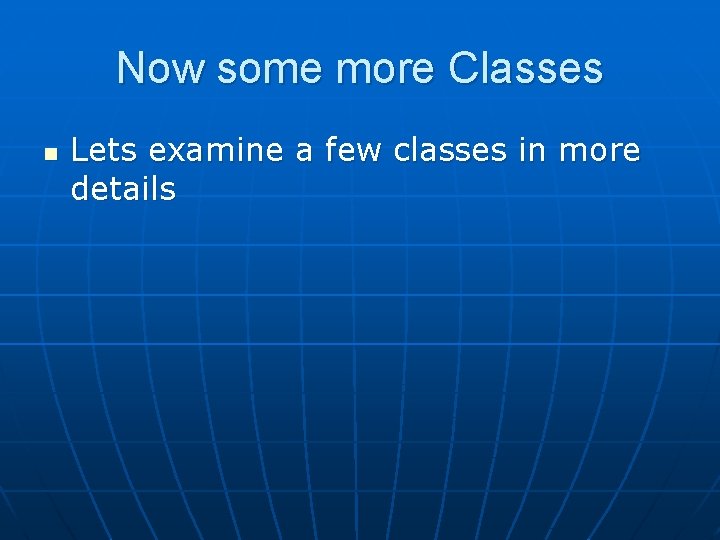
Now some more Classes n Lets examine a few classes in more details
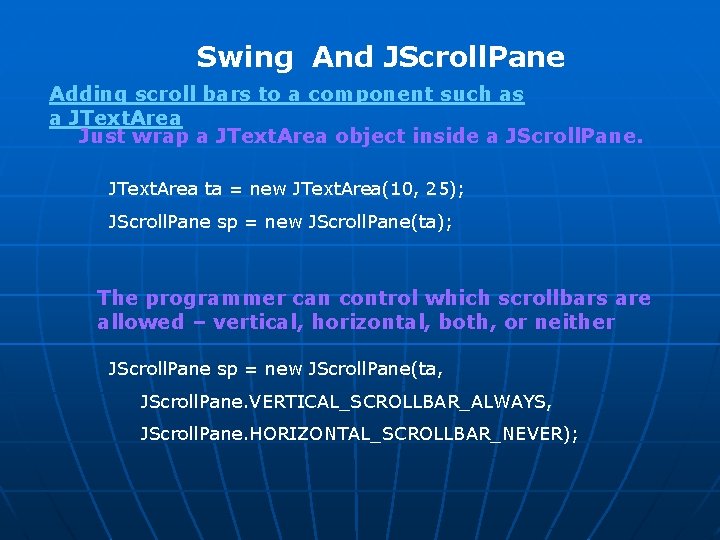
Swing And JScroll. Pane Adding scroll bars to a component such as a JText. Area Just wrap a JText. Area object inside a JScroll. Pane. JText. Area ta = new JText. Area(10, 25); JScroll. Pane sp = new JScroll. Pane(ta); The programmer can control which scrollbars are allowed – vertical, horizontal, both, or neither JScroll. Pane sp = new JScroll. Pane(ta, JScroll. Pane. VERTICAL_SCROLLBAR_ALWAYS, JScroll. Pane. HORIZONTAL_SCROLLBAR_NEVER);
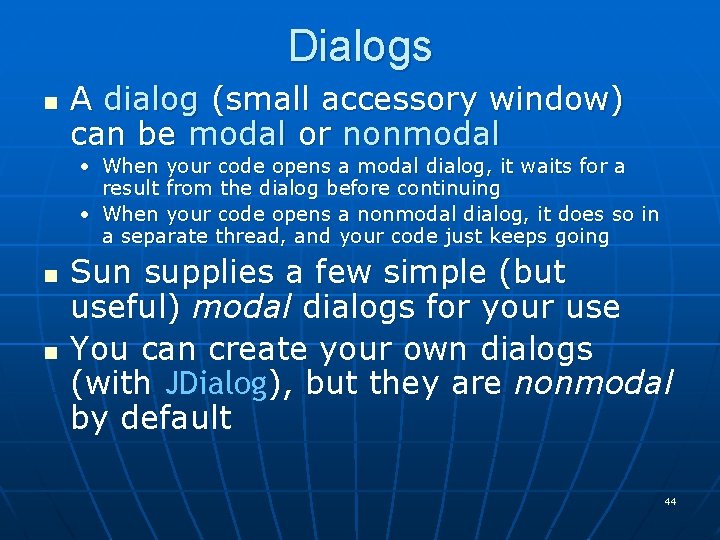
Dialogs n A dialog (small accessory window) can be modal or nonmodal • When your code opens a modal dialog, it waits for a result from the dialog before continuing • When your code opens a nonmodal dialog, it does so in a separate thread, and your code just keeps going n n Sun supplies a few simple (but useful) modal dialogs for your use You can create your own dialogs (with JDialog), but they are nonmodal by default 44
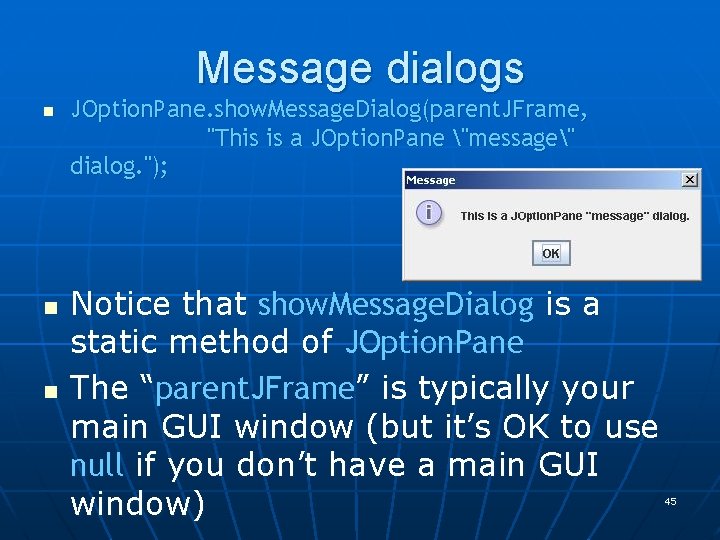
Message dialogs n n n JOption. Pane. show. Message. Dialog(parent. JFrame, "This is a JOption. Pane "message" dialog. "); Notice that show. Message. Dialog is a static method of JOption. Pane The “parent. JFrame” is typically your main GUI window (but it’s OK to use null if you don’t have a main GUI window) 45
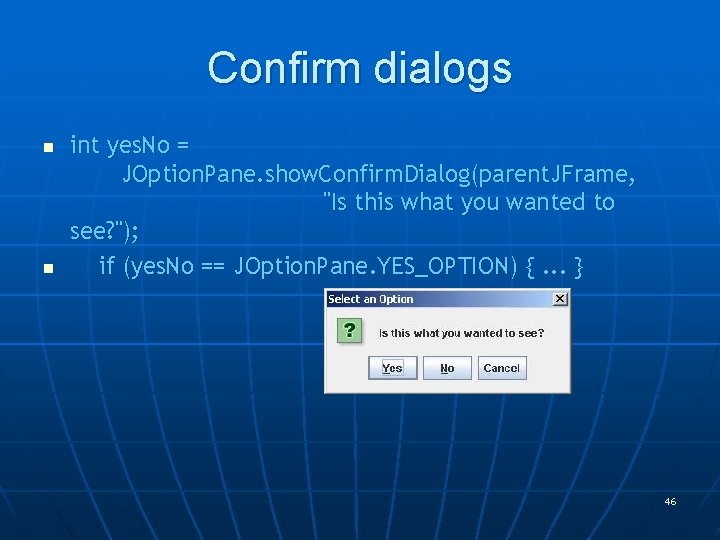
Confirm dialogs n n int yes. No = JOption. Pane. show. Confirm. Dialog(parent. JFrame, "Is this what you wanted to see? "); if (yes. No == JOption. Pane. YES_OPTION) {. . . } 46
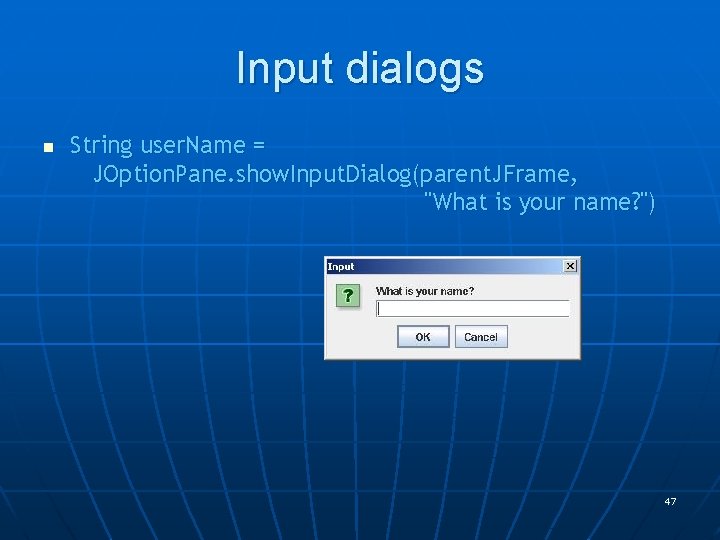
Input dialogs n String user. Name = JOption. Pane. show. Input. Dialog(parent. JFrame, "What is your name? ") 47
![Option dialogs n n Object options new String English Chinese French German Option dialogs n n Object[] options = new String[] {"English", "Chinese", "French", "German" };](https://slidetodoc.com/presentation_image_h2/384449579051cfddef07e896ba449a47/image-48.jpg)
Option dialogs n n Object[] options = new String[] {"English", "Chinese", "French", "German" }; int option = JOption. Pane. show. Option. Dialog(parent. JFrame, "Choose an option: ", "Option Dialog", JOption. Pane. YES_NO_OPTION, JOption. Pane. QUESTION_MESSAGE, null, options[0]); // use as default Fourth argument could be JOption. Pane. YES_NO_CANCEL_OPTION Fifth argument specifies which icon to use in the dialog; it could be one of ERROR_MESSAGE, INFORMATION_MESSAGE, WARNING_MESSAGE, or PLAIN_MESSAGE Sixth argument (null above) can specify a custom icon 48
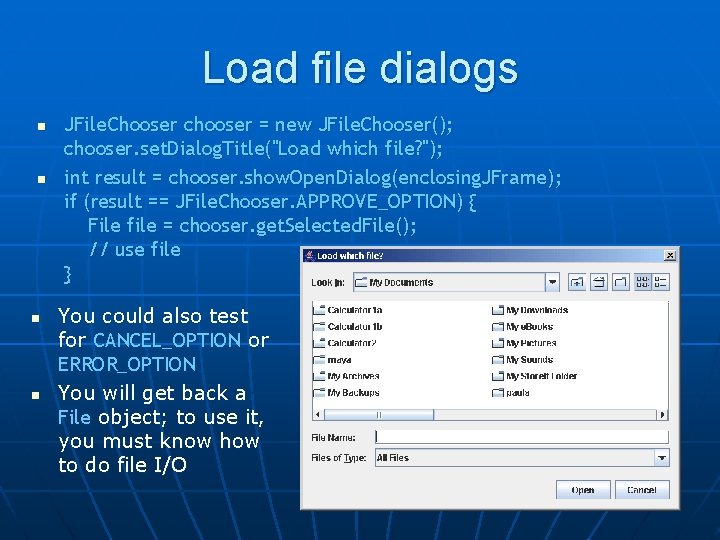
Load file dialogs n n JFile. Chooser chooser = new JFile. Chooser(); chooser. set. Dialog. Title("Load which file? "); int result = chooser. show. Open. Dialog(enclosing. JFrame); if (result == JFile. Chooser. APPROVE_OPTION) { File file = chooser. get. Selected. File(); // use file } You could also test for CANCEL_OPTION or ERROR_OPTION You will get back a File object; to use it, you must know how to do file I/O 49
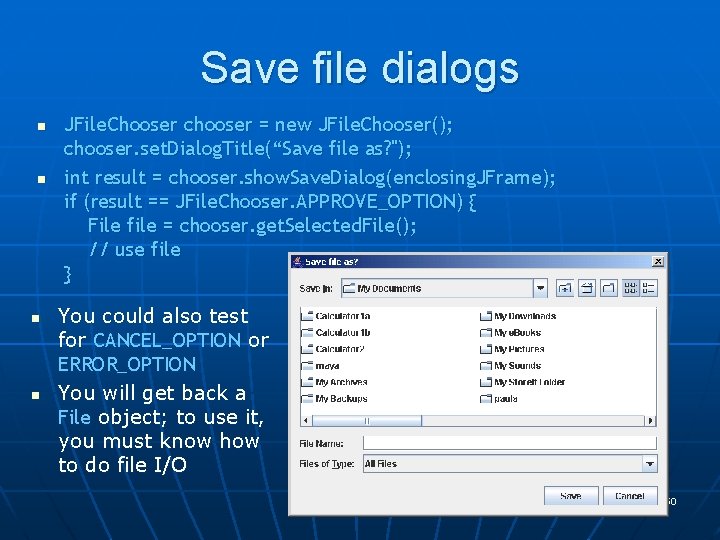
Save file dialogs n n JFile. Chooser chooser = new JFile. Chooser(); chooser. set. Dialog. Title(“Save file as? "); int result = chooser. show. Save. Dialog(enclosing. JFrame); if (result == JFile. Chooser. APPROVE_OPTION) { File file = chooser. get. Selected. File(); // use file } You could also test for CANCEL_OPTION or ERROR_OPTION You will get back a File object; to use it, you must know how to do file I/O 50
Event handling in swing
Virtuozzo docker
Differences between sequential and event-driven programming
Awteventlistener
Event handler in android
Java gui event handling
Event handling in ada
Simple and compound events
A sentinel event
Independent event vs dependent event
Independent event vs dependent event
Event management content
Event background
Newsworthy event (s) background event (s) sources
Similarities of parents
Strand for teacher
Distinctive vocal style of israel.
Venn diagram of ant and grasshopper
What are the differences between athens and sparta
Raymond's run text dependent questions and answers
Similarities of wid, wad and gad
James joyce e virginia woolf a confronto
Materials handling and storage ppt
Protective packaging and materials handling
Packing learning objectives
Wendylett sheets 1 carer
Hydrilla cell diagram
Handling and transportation of fish
Data protection act in health and social care settings
Radiographic artifacts
Chapter 20:12 measuring specific gravity
Care and handling of library materials
Informer recruitment
Chapter 2 camera handling care and support
Vaccine storage and handling sop worksheet
Manual handling competency assessment form
Facility planning
Vaccine storage and handling protocol
Tileo assessment
Post harvest handling of cut flowers and greens
Mechanical and remote handling
Barry liked playing board games
Difference between swing and applet
Components of swing
Distinguish between group and team.
What is the difference between heliocentric and geocentric?
High and low frequency waves
Difference between need and want
Diff between linux and unix
Difference between datagram and virtual circuit operation
Mechanical waves and electromagnetic waves