Week Four Event Handling Inner classes Event Handling
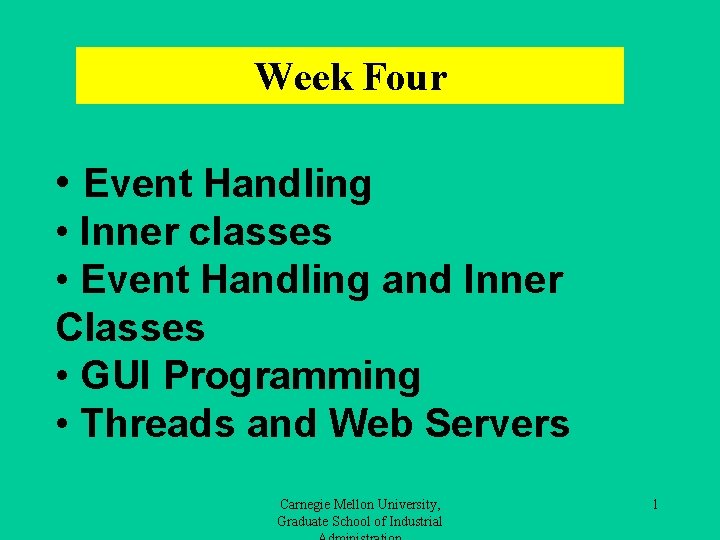
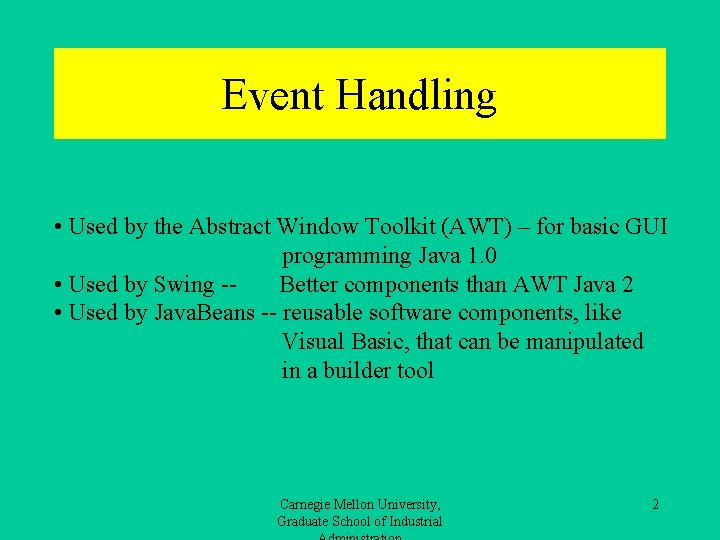
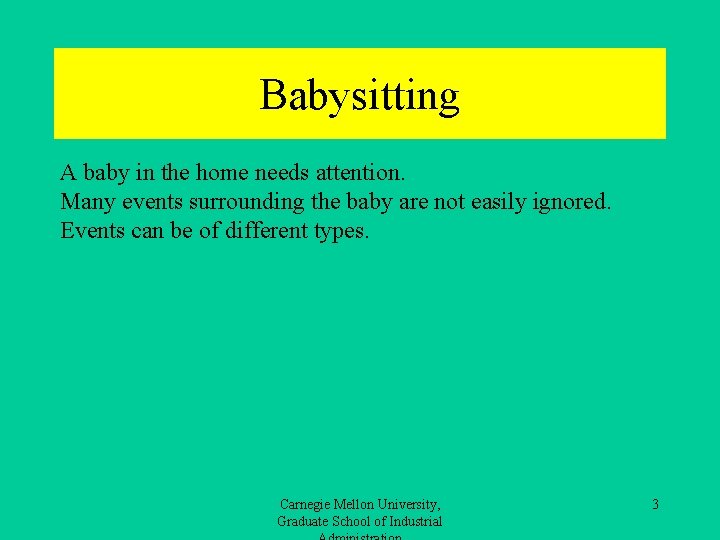
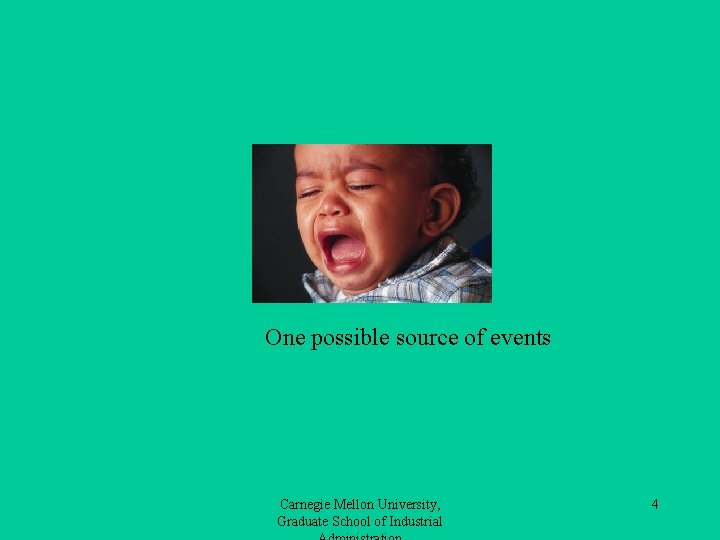
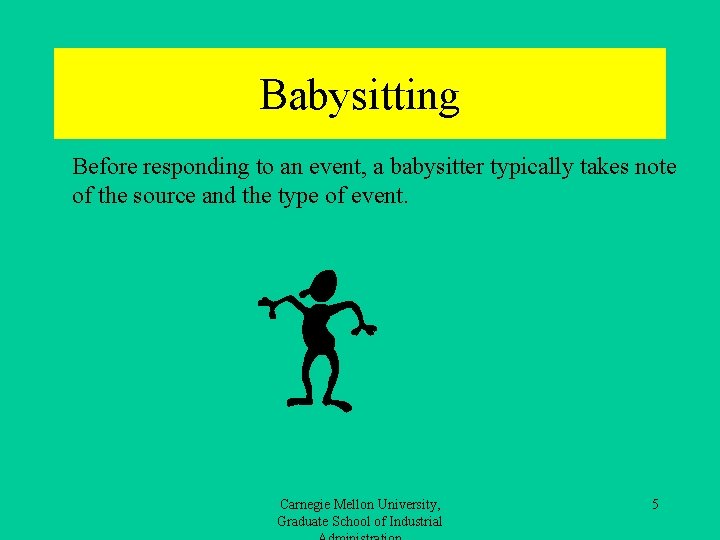
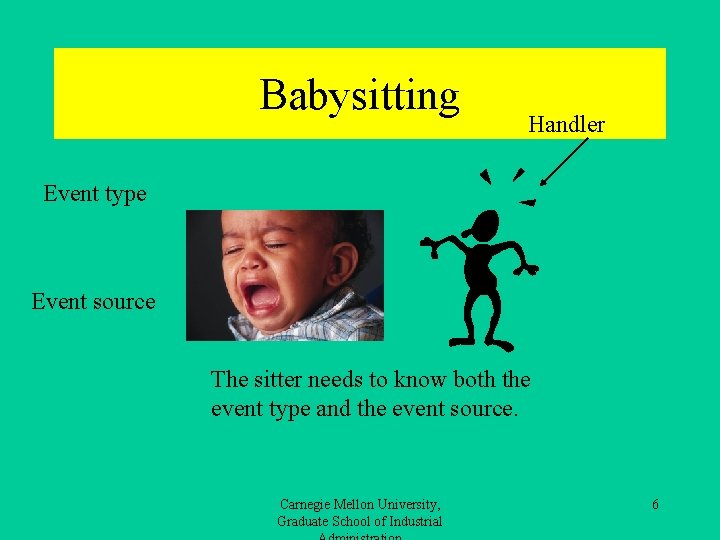
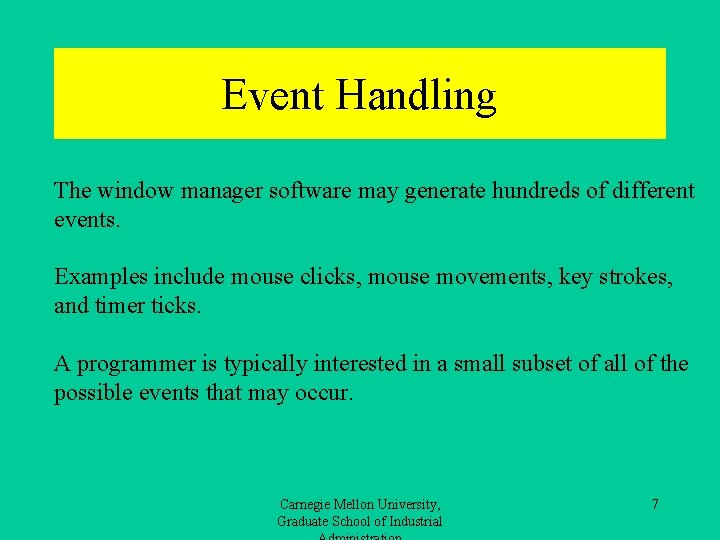
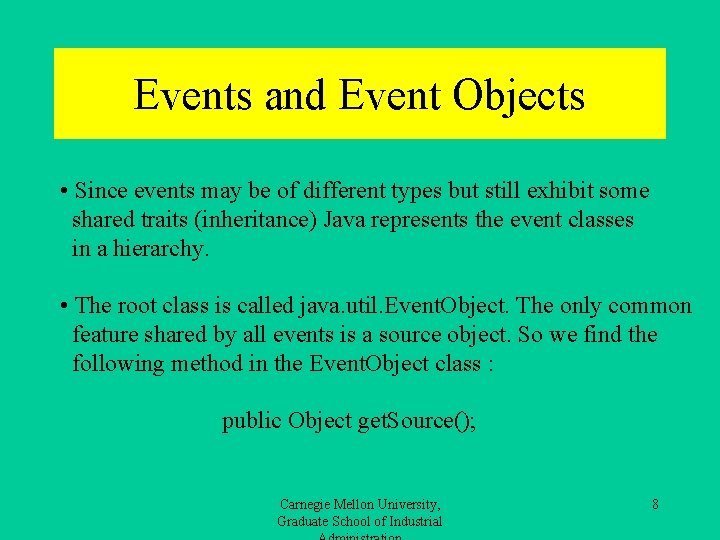
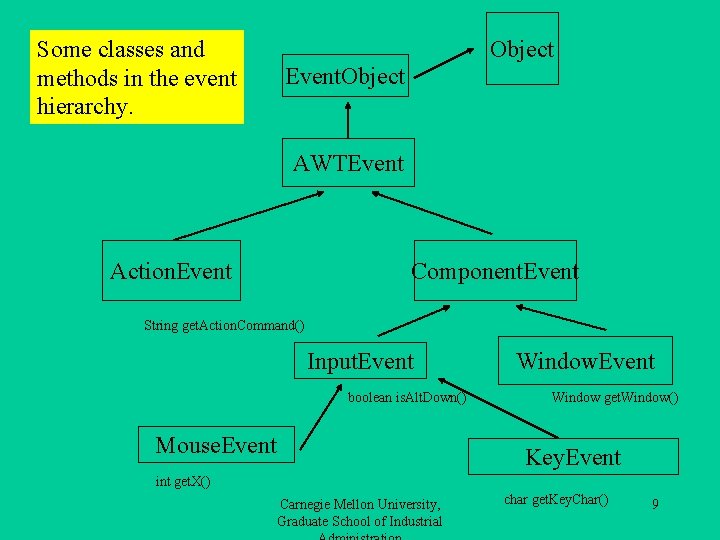
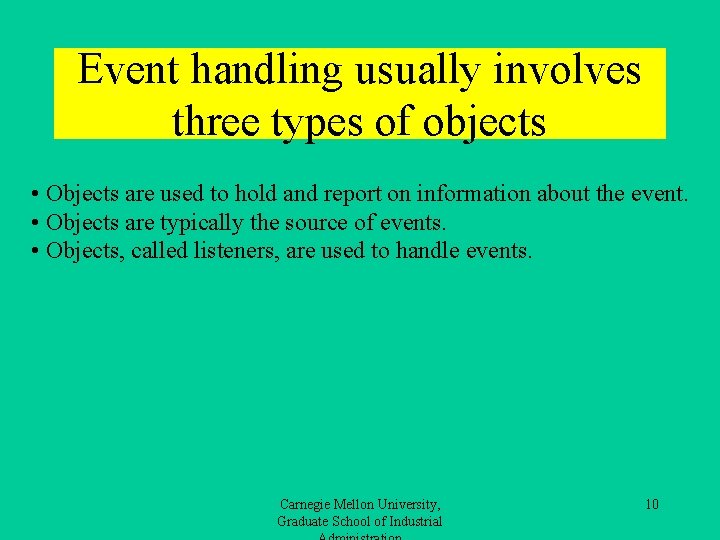
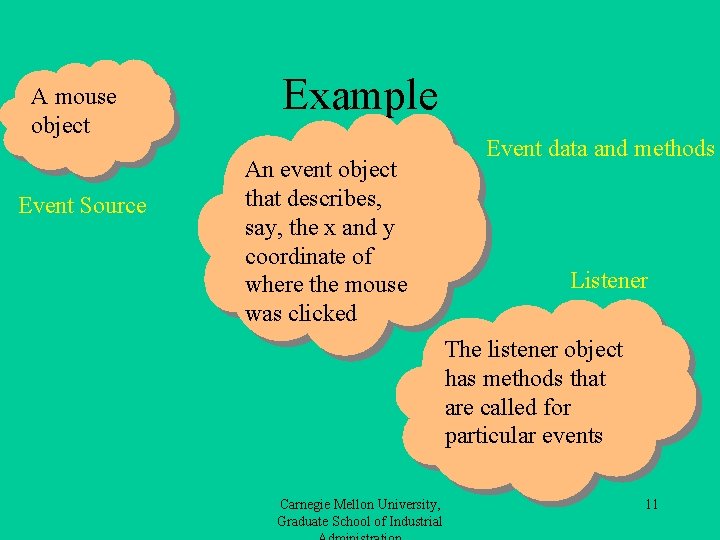
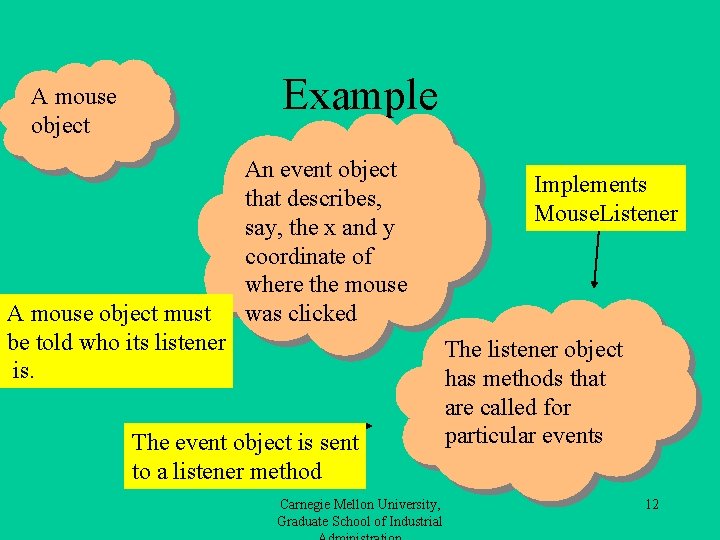
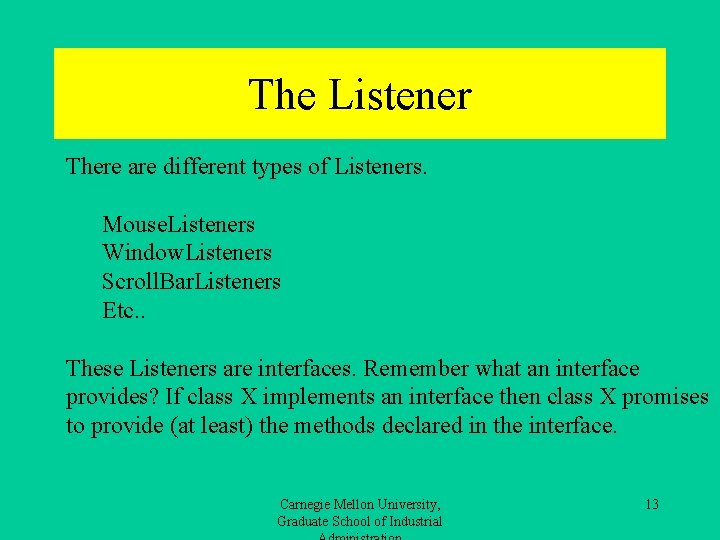
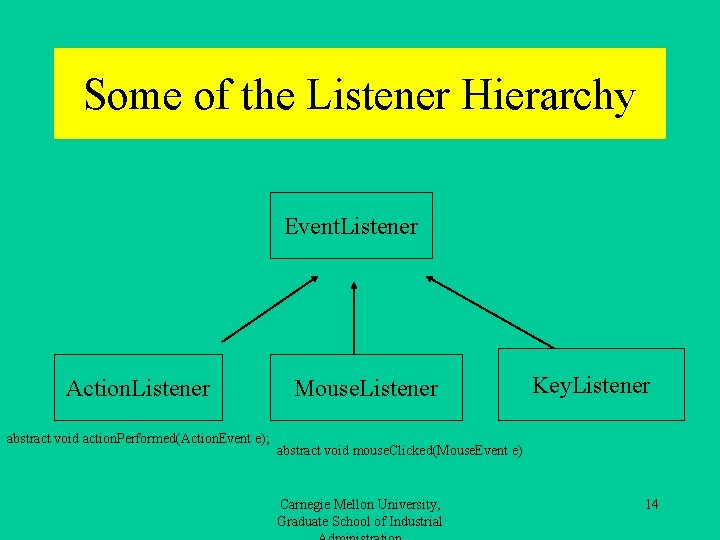
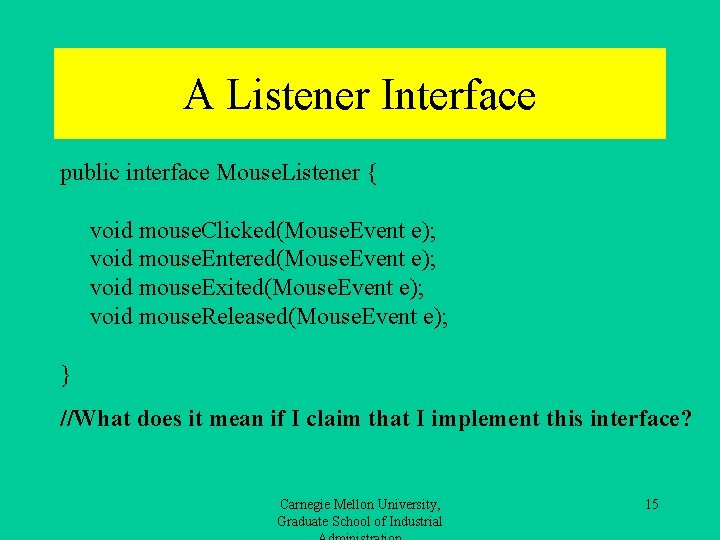
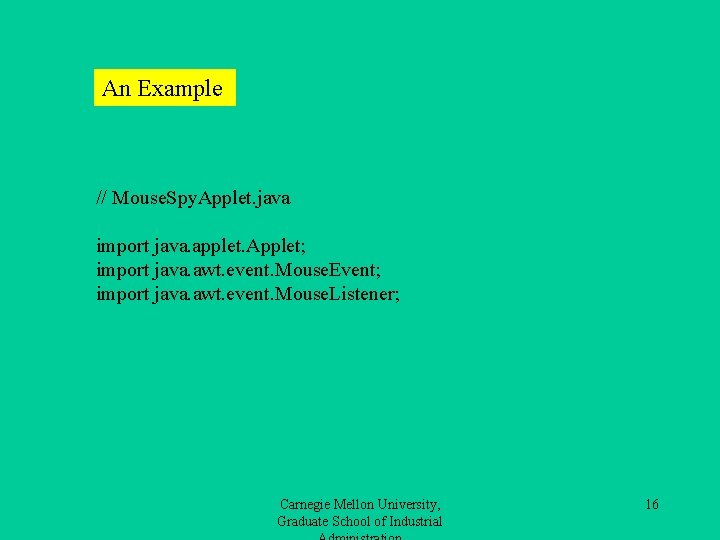
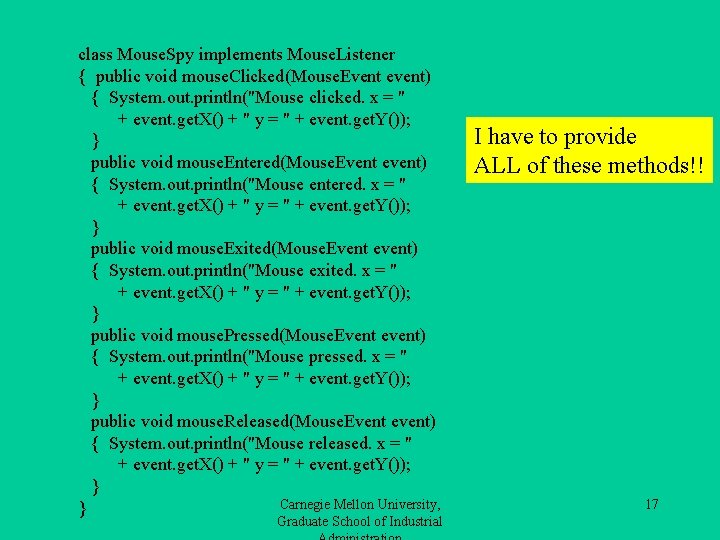
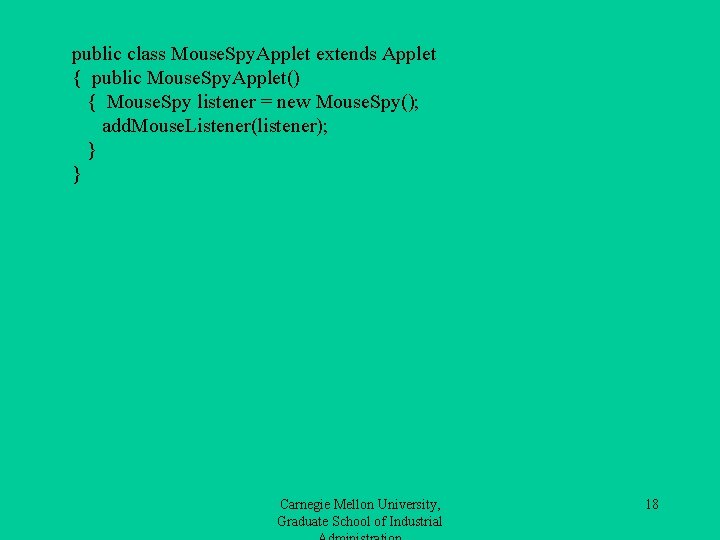
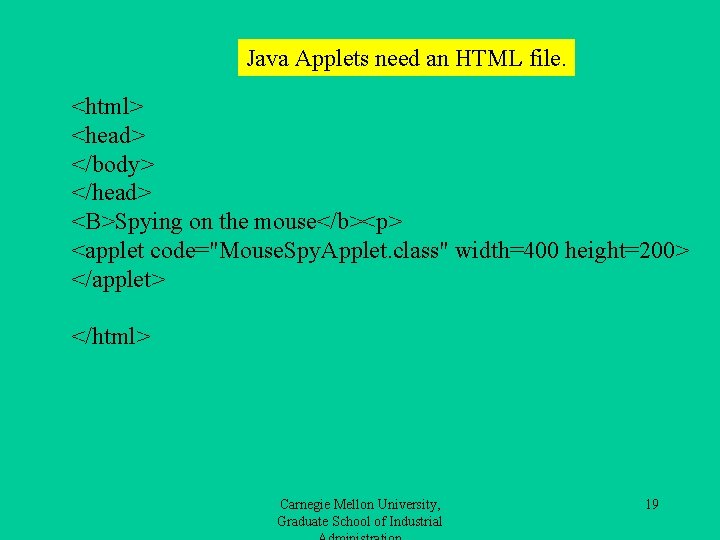
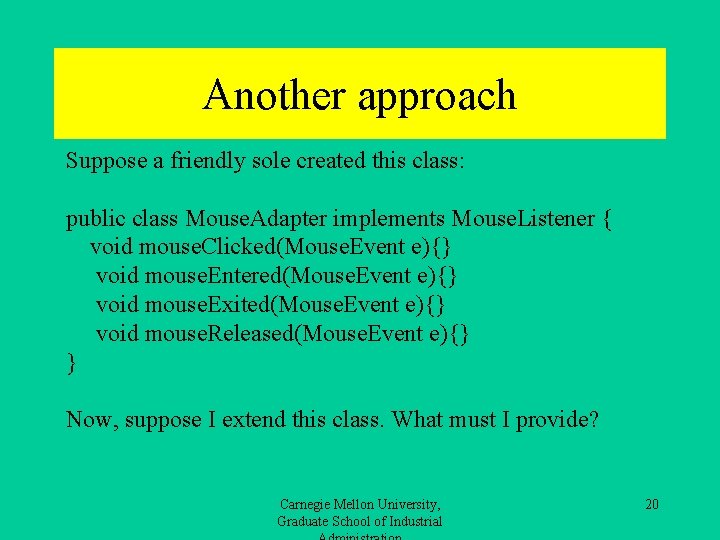
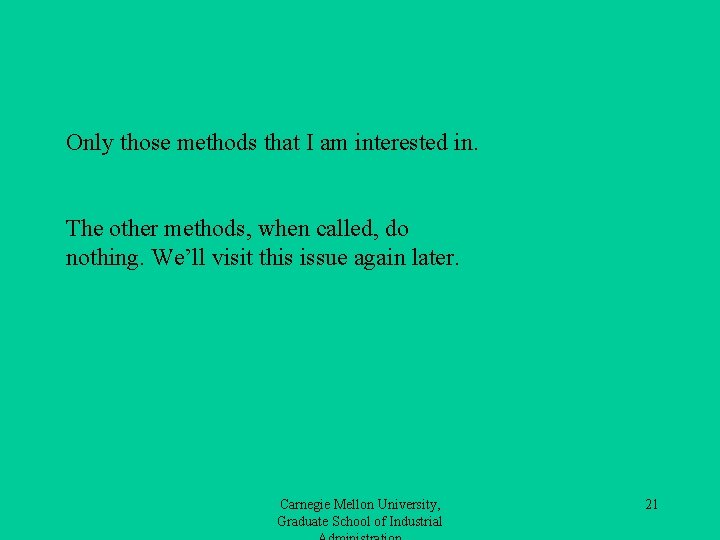
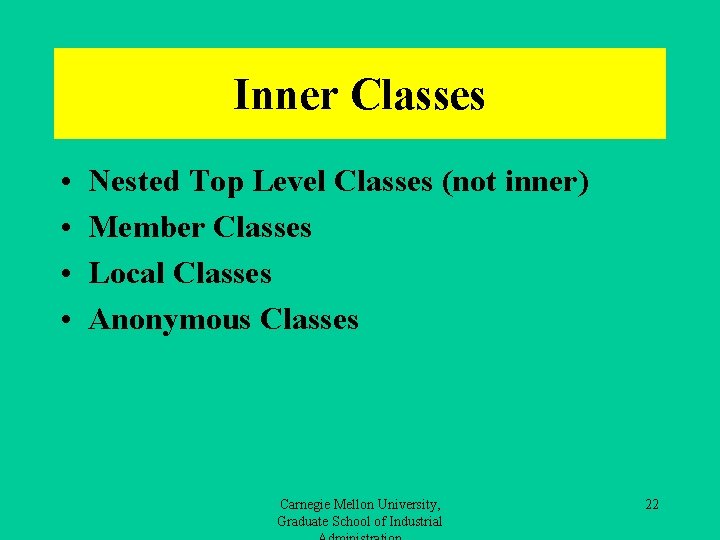
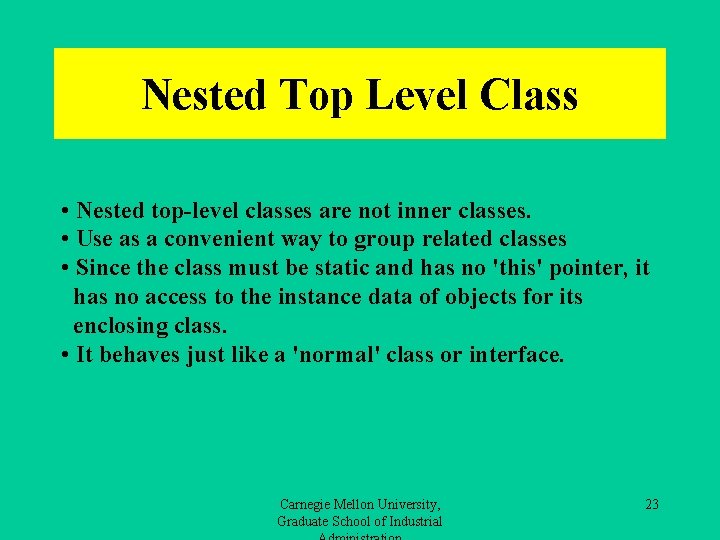
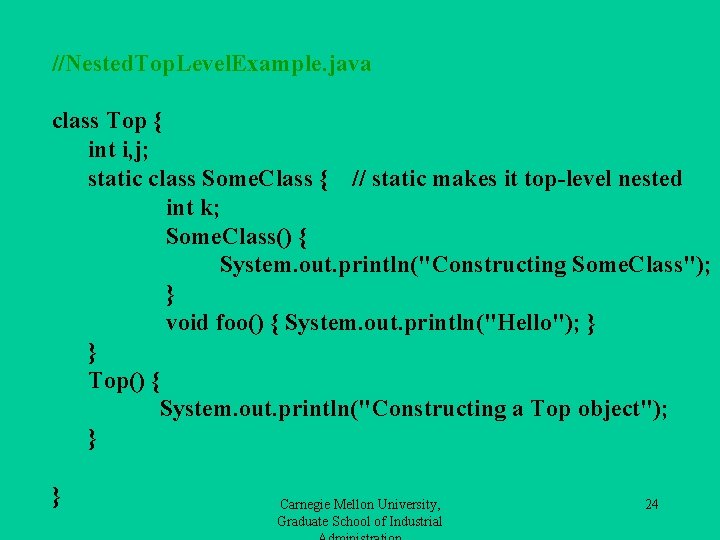
![public class Nested. Top. Level. Example { public static void main(String args[]) { Top public class Nested. Top. Level. Example { public static void main(String args[]) { Top](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-25.jpg)
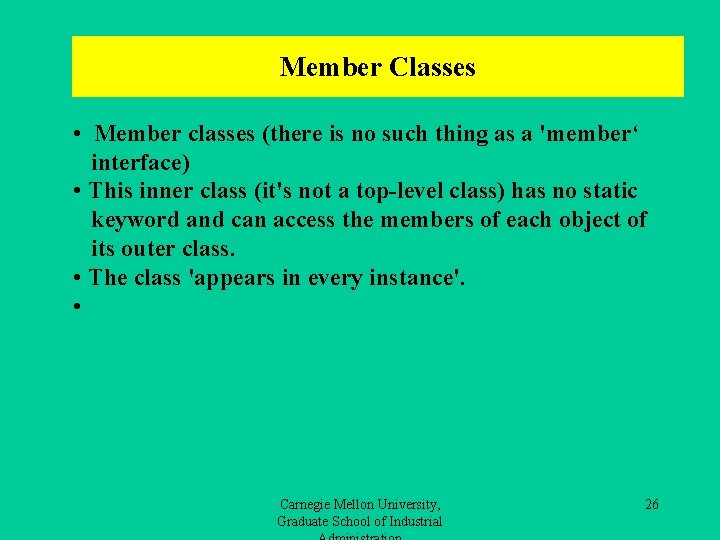
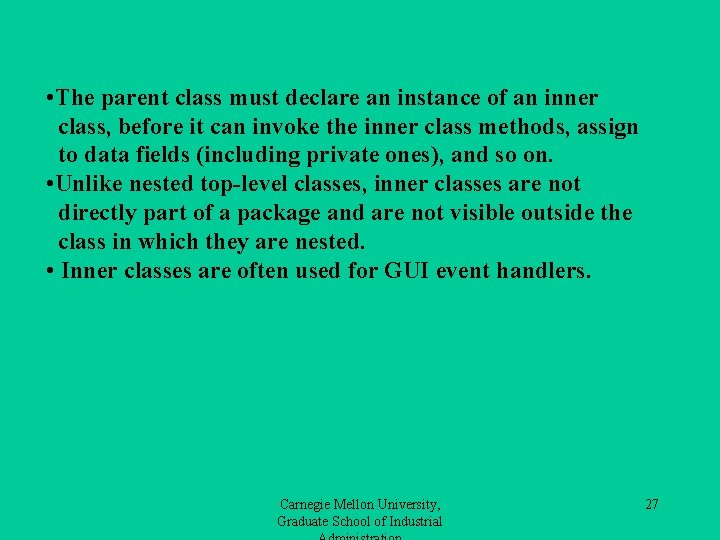
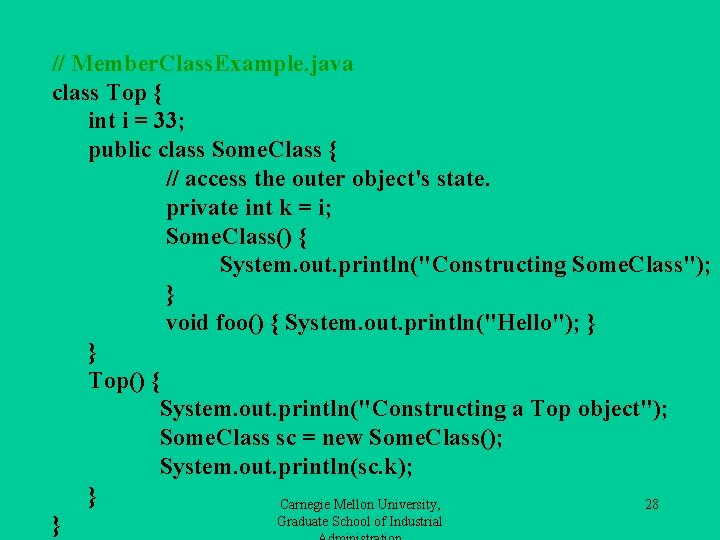
![public class Member. Class. Example { public static void main(String args[]) { Top my. public class Member. Class. Example { public static void main(String args[]) { Top my.](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-29.jpg)
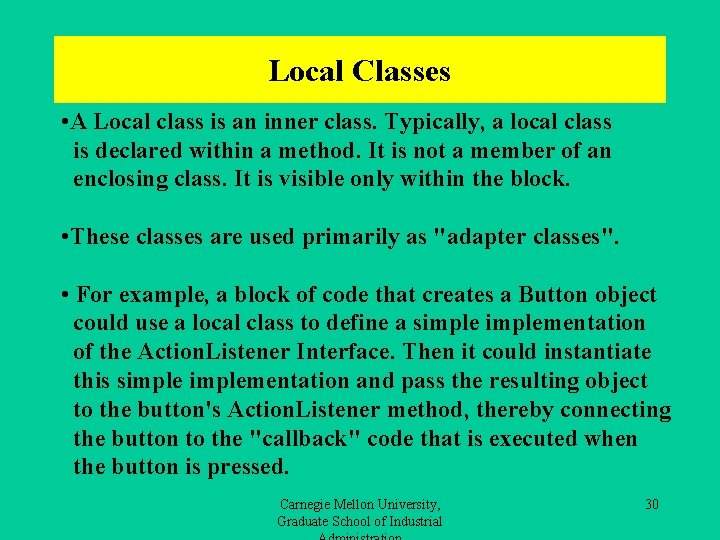
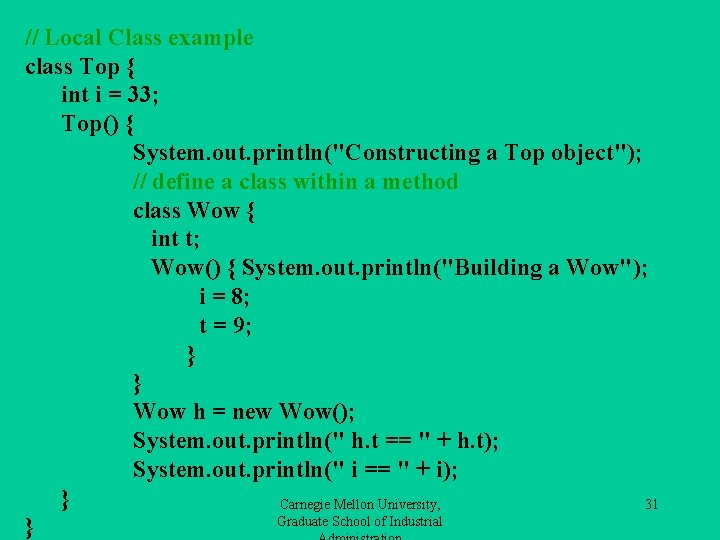
![public class Local. Example { public static void main(String args[]) { Top my. Object public class Local. Example { public static void main(String args[]) { Top my. Object](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-32.jpg)
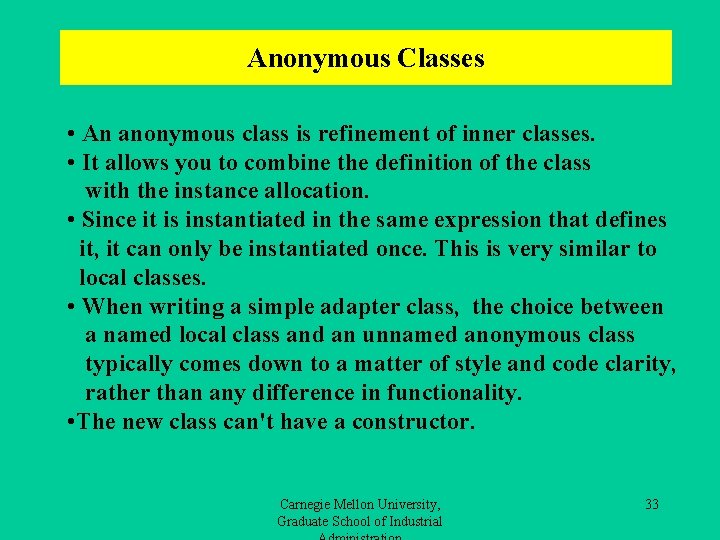
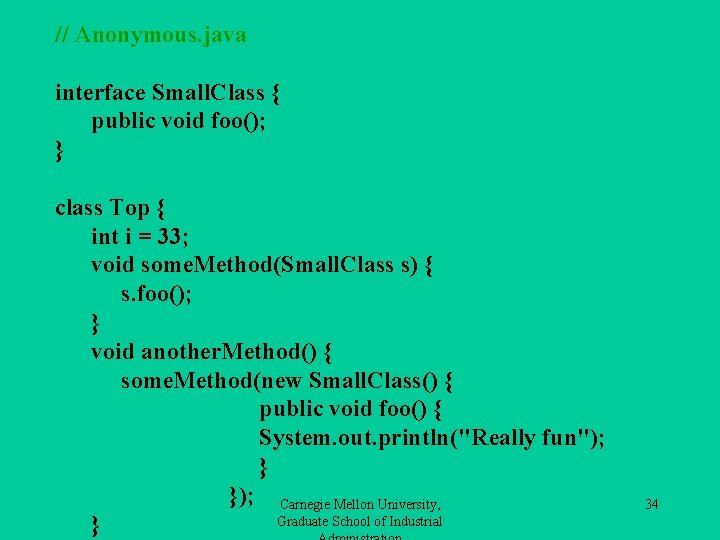
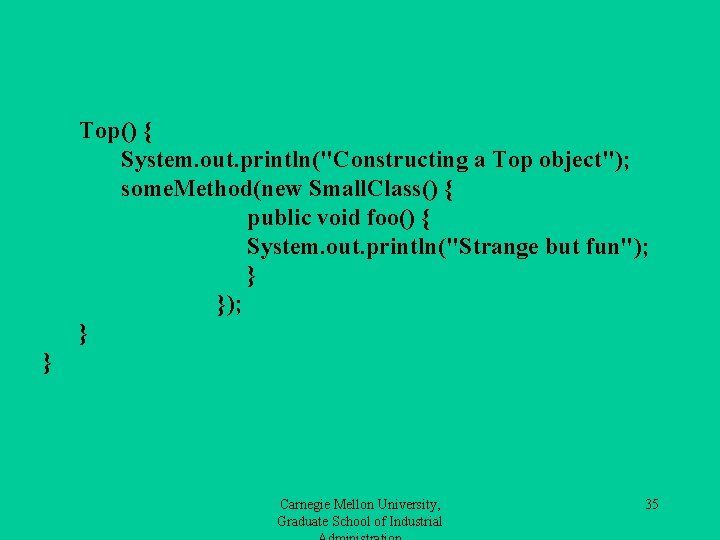
![public class Anonymous { public static void main(String args[]) { // We can't create public class Anonymous { public static void main(String args[]) { // We can't create](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-36.jpg)
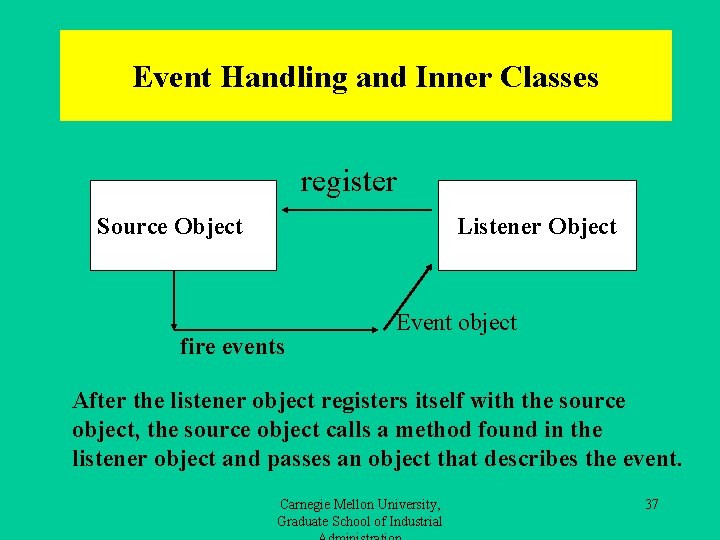
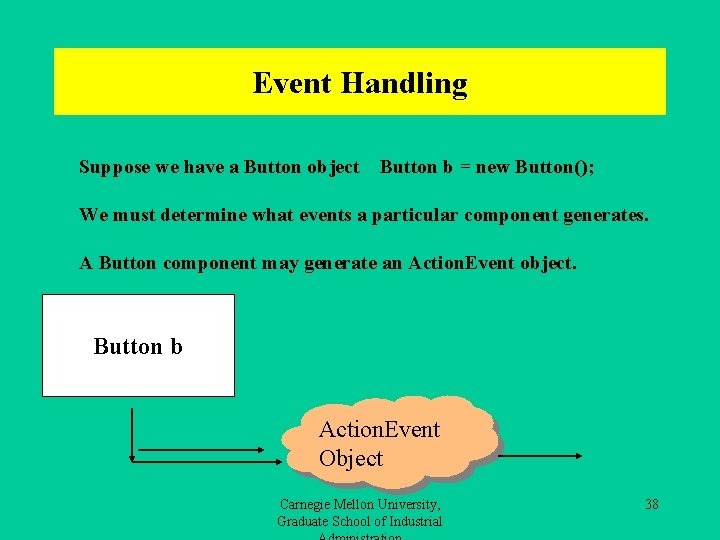
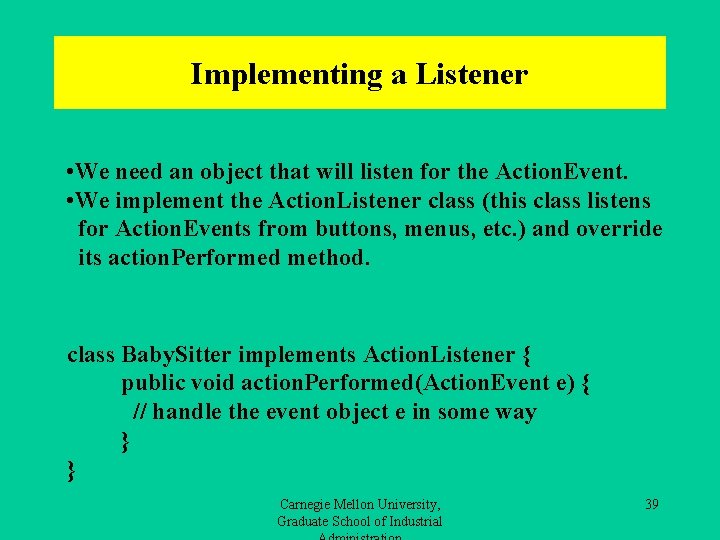
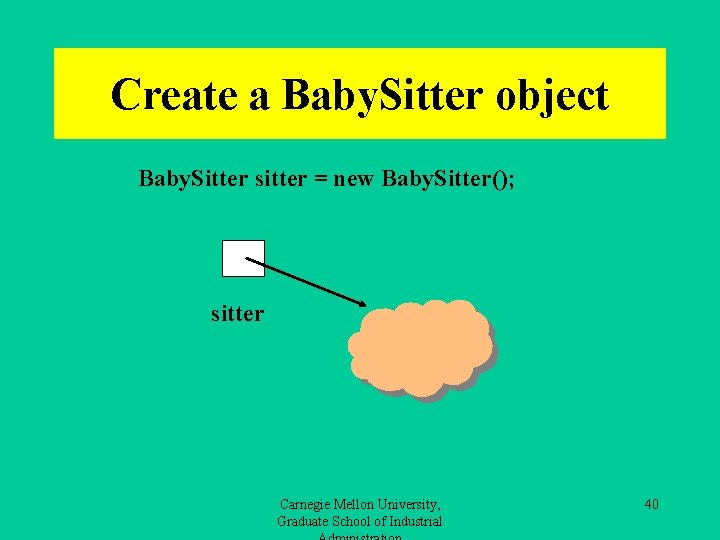
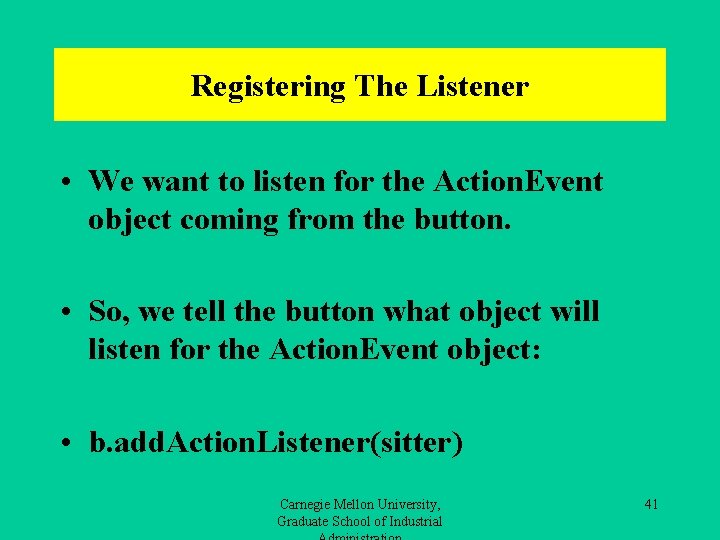
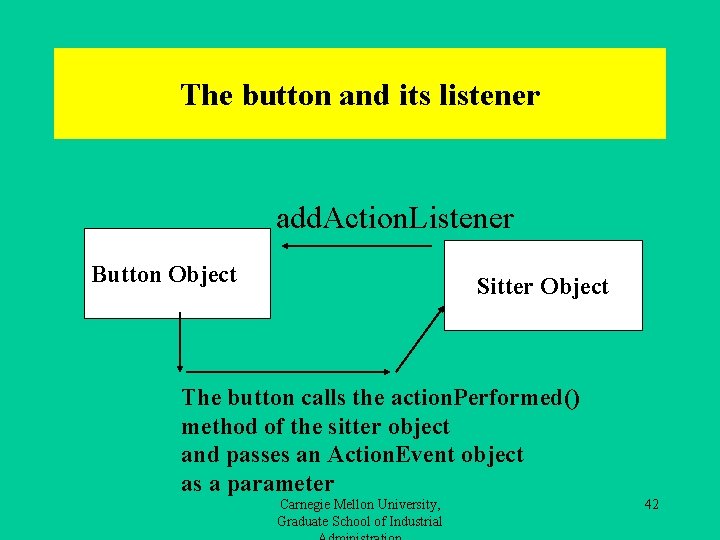
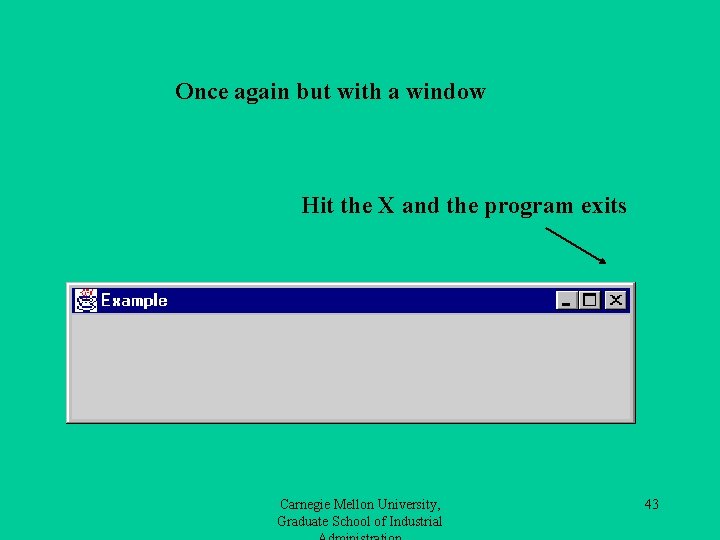
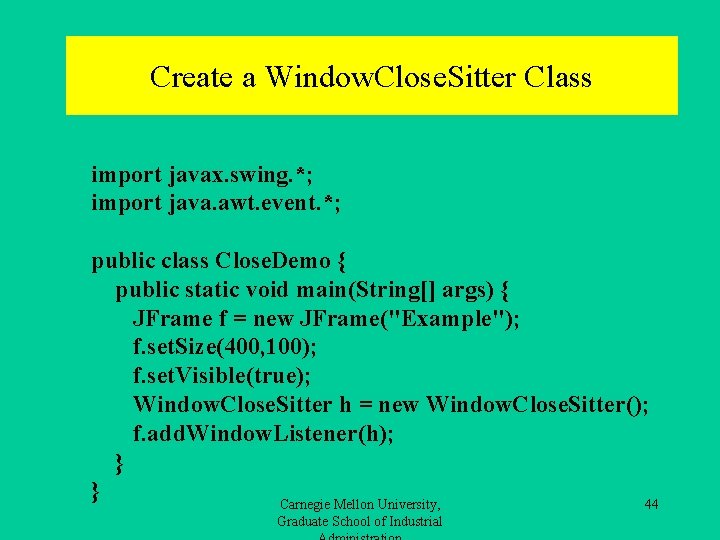
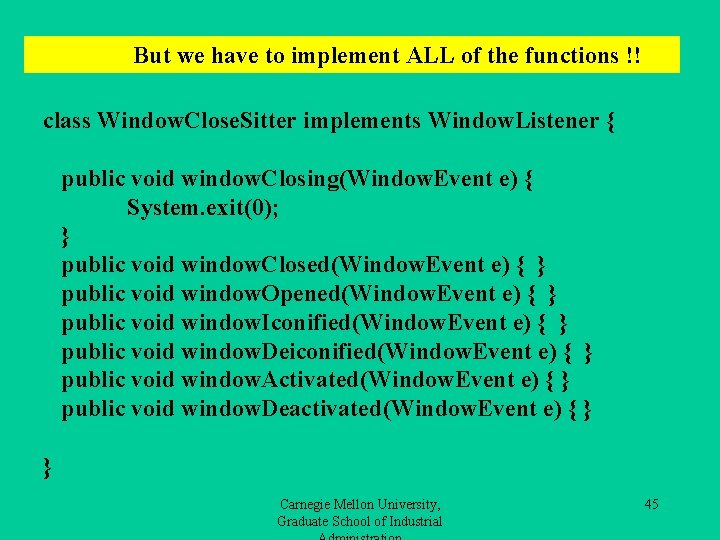
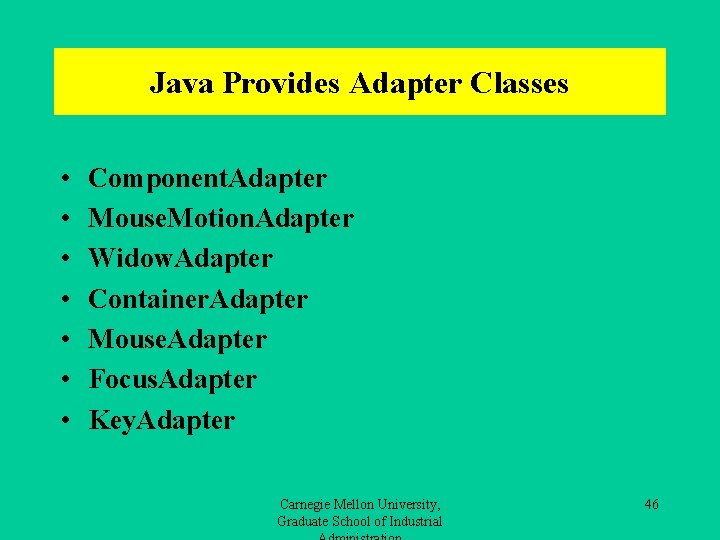
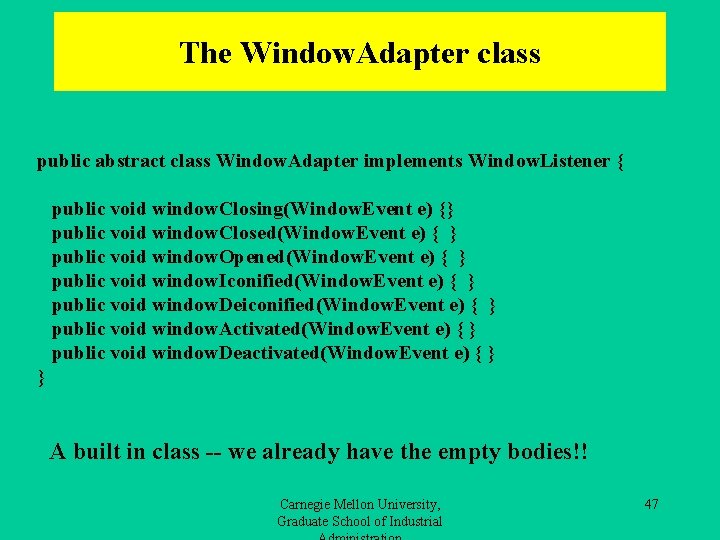
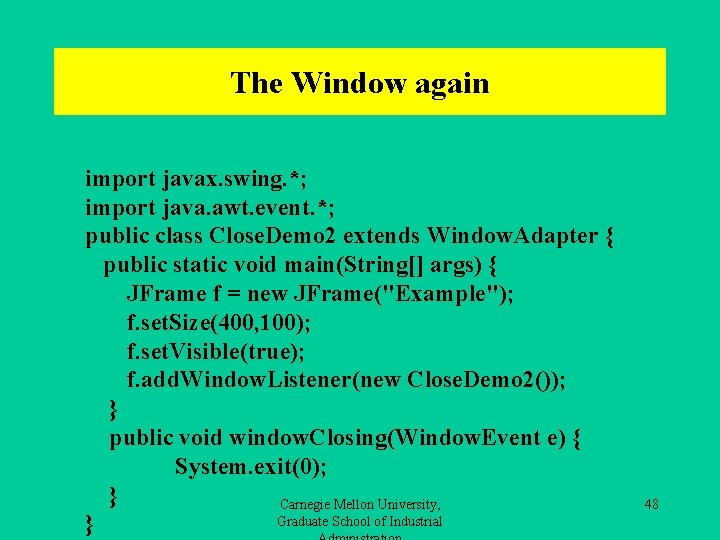
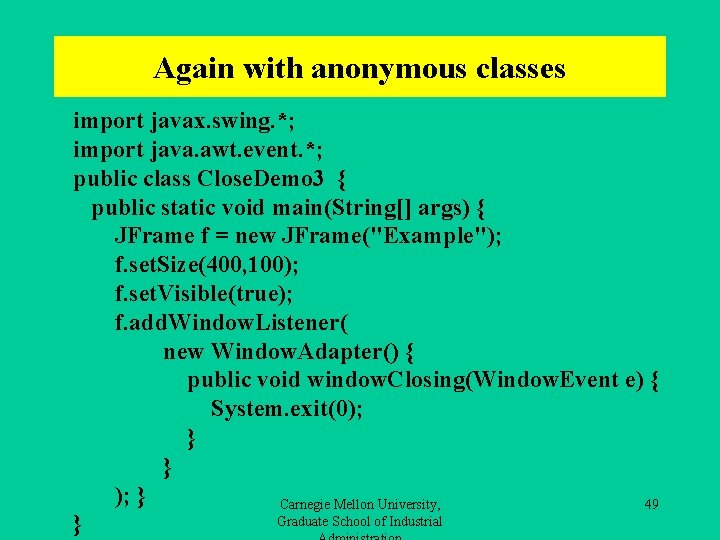
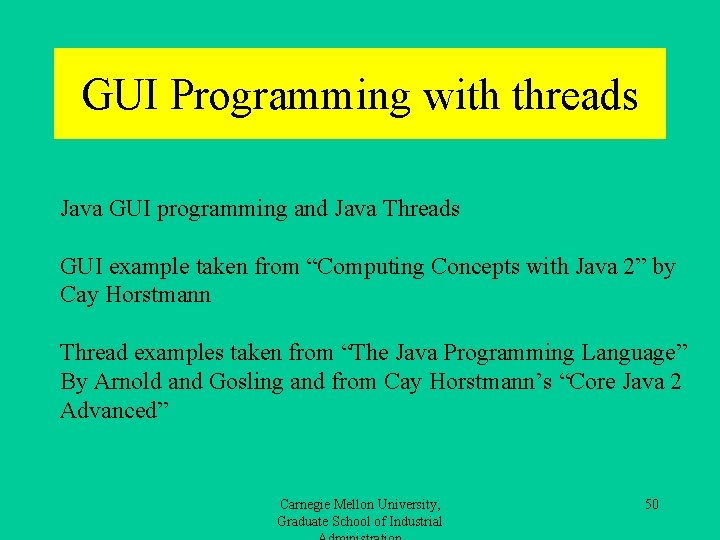
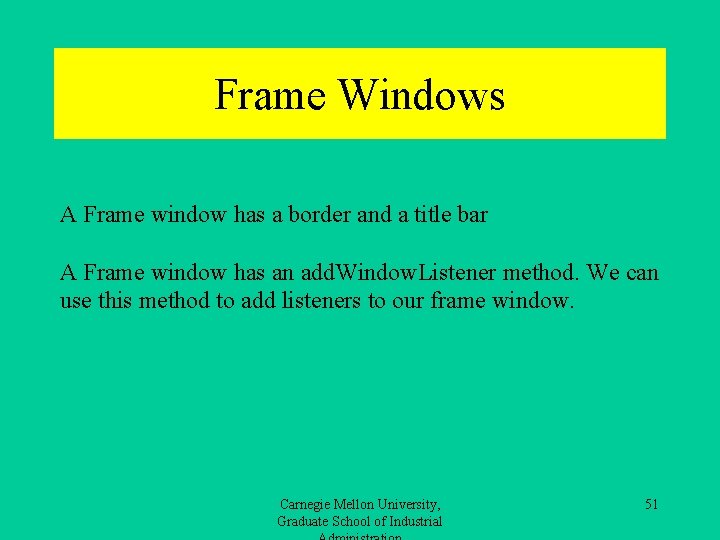
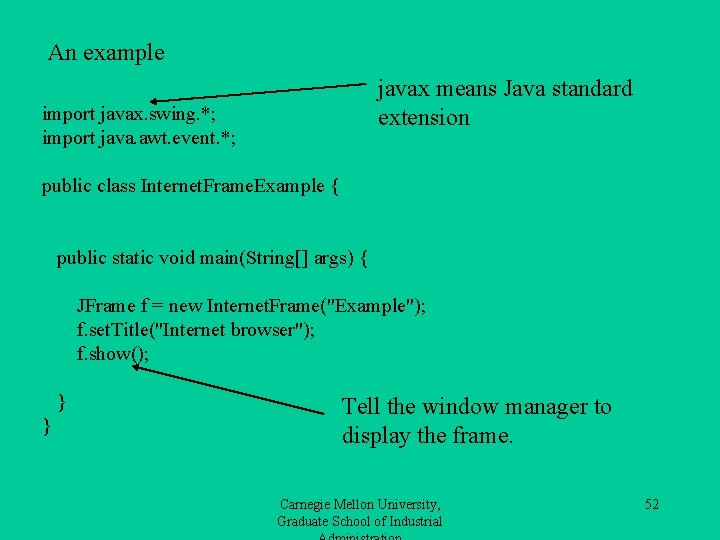
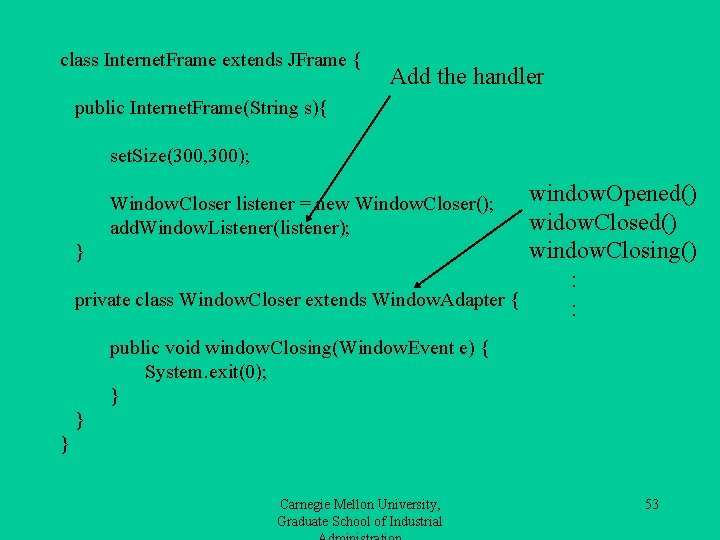
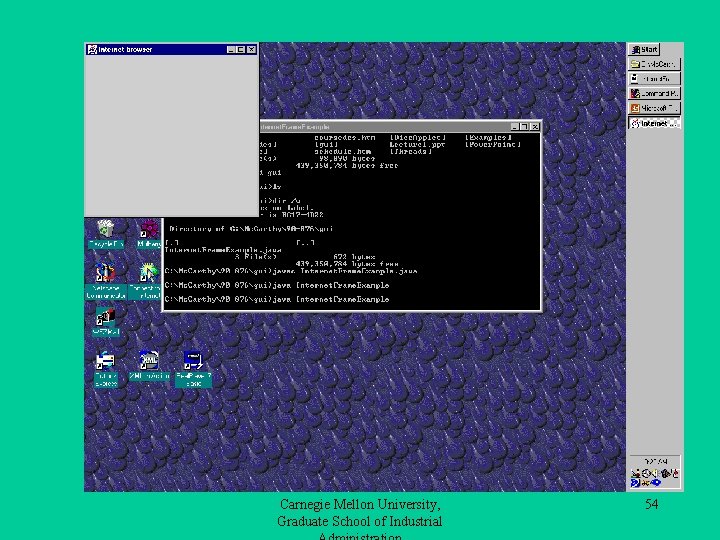
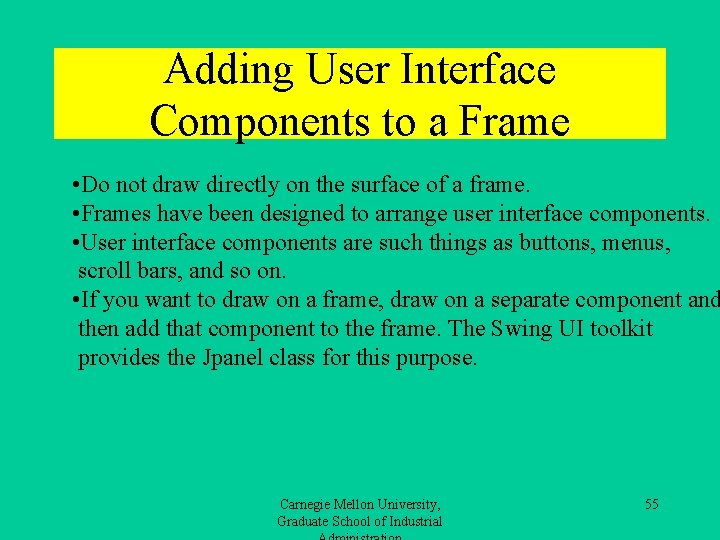
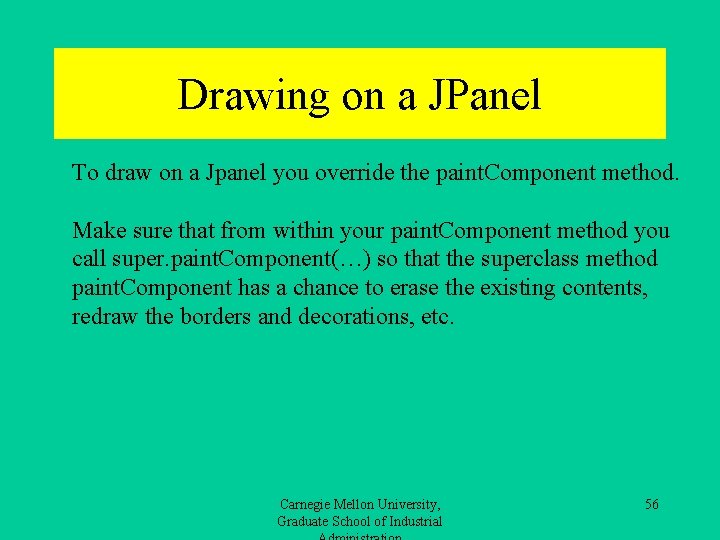
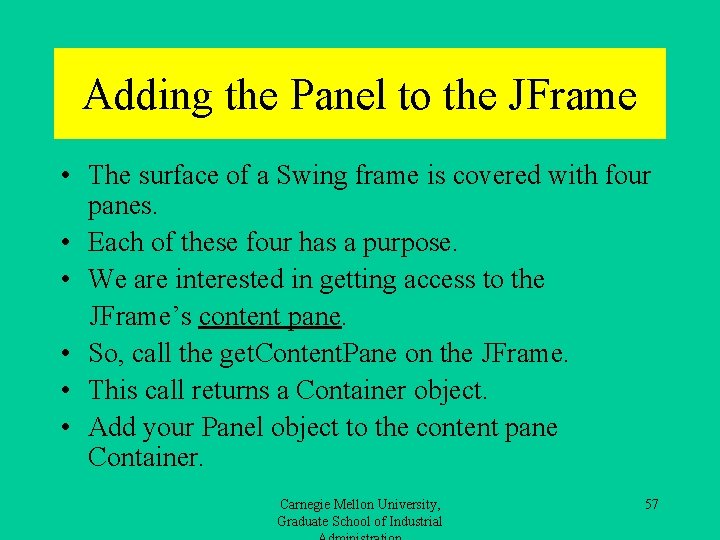
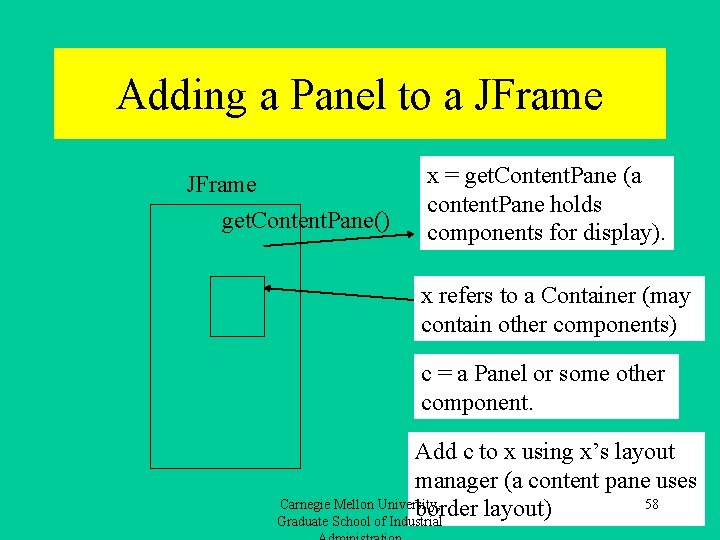
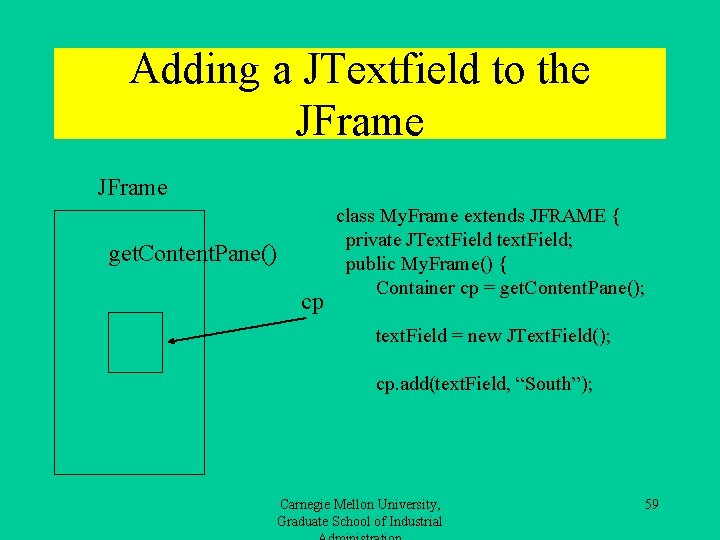
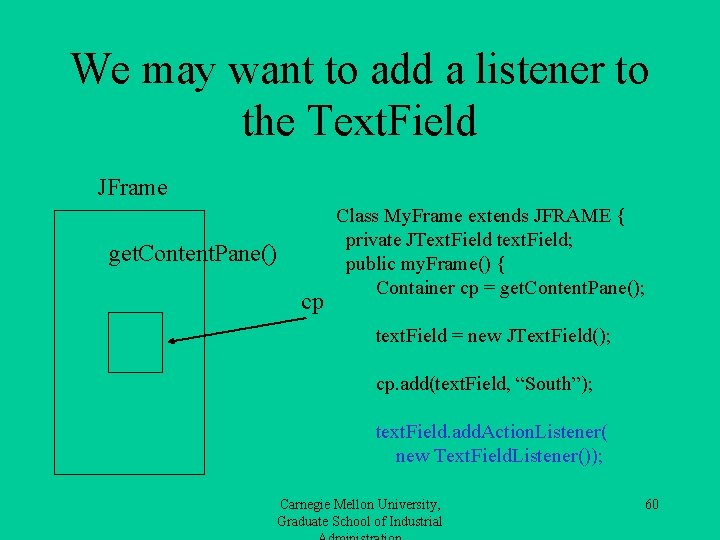
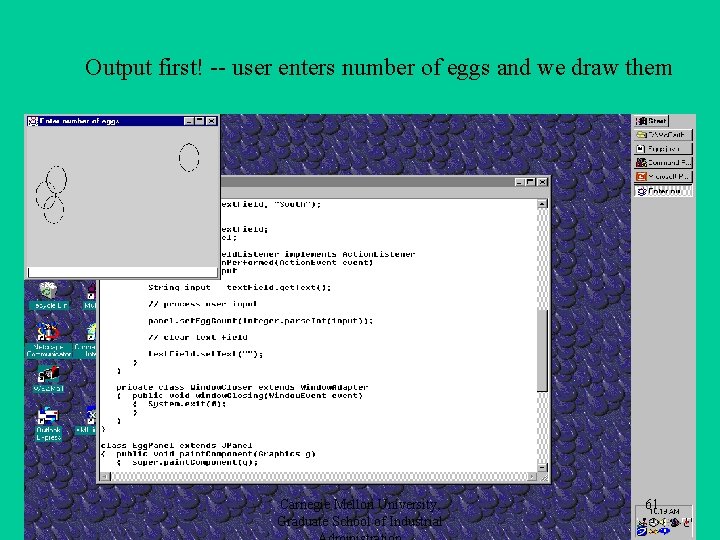
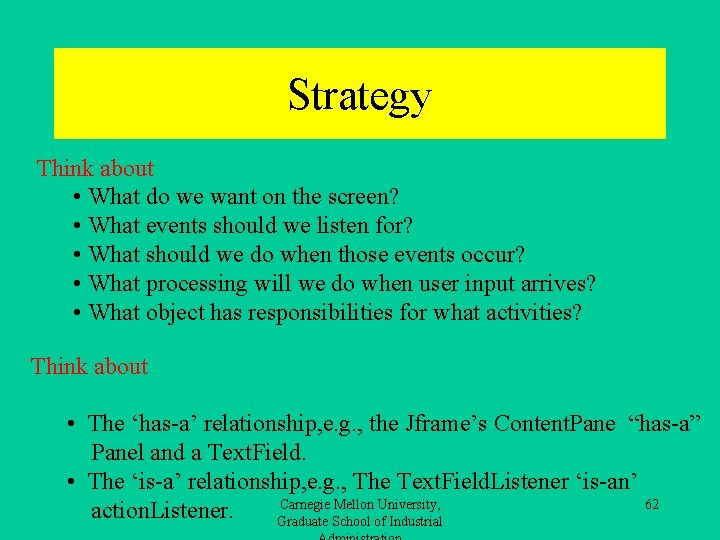
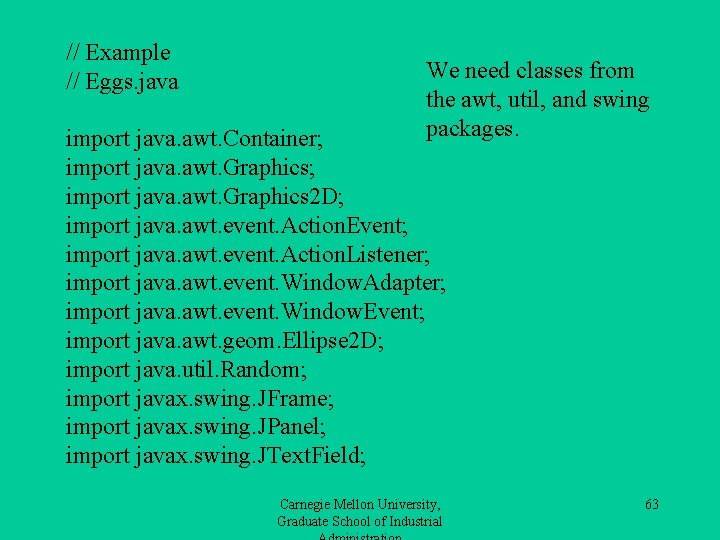
![public class Eggs { public static void main(String[] args) { Egg. Frame frame = public class Eggs { public static void main(String[] args) { Egg. Frame frame =](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-64.jpg)
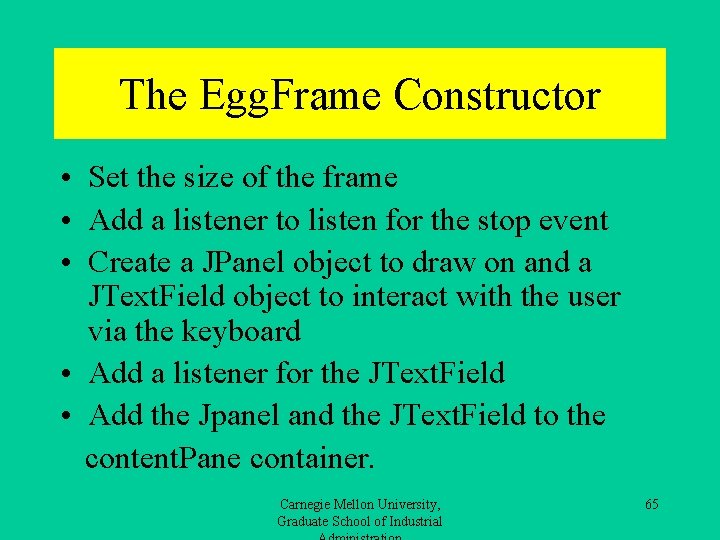
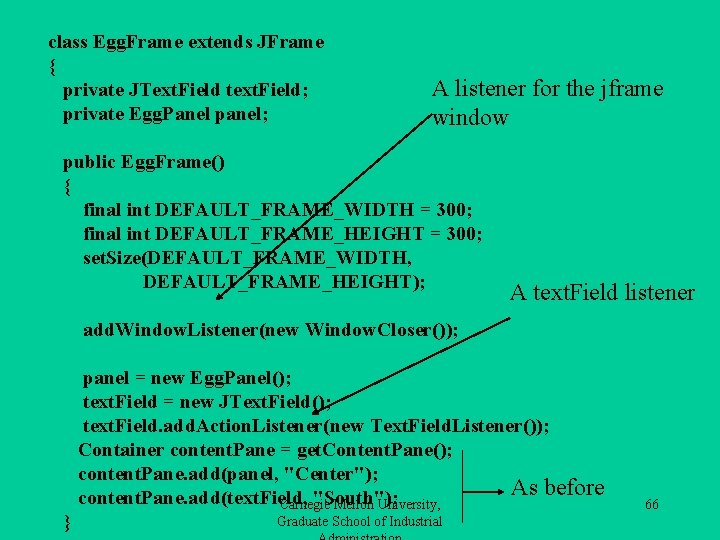
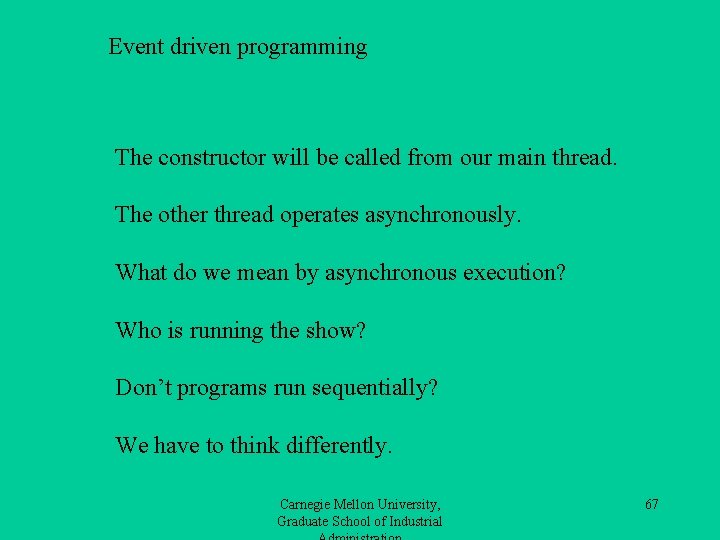
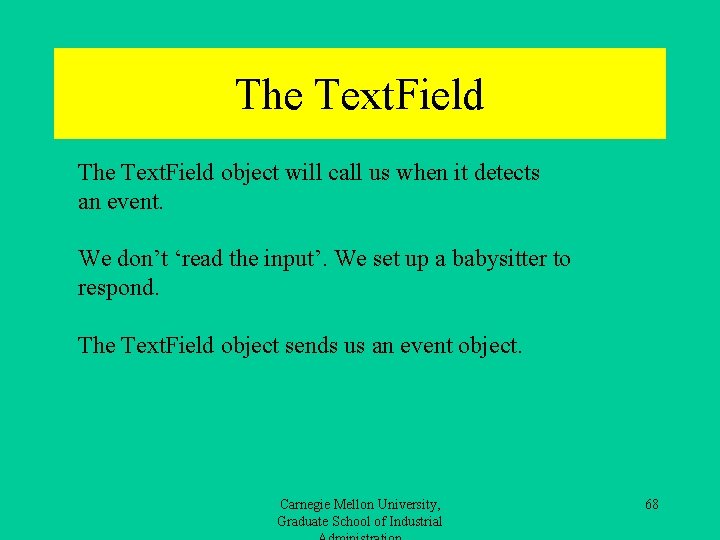
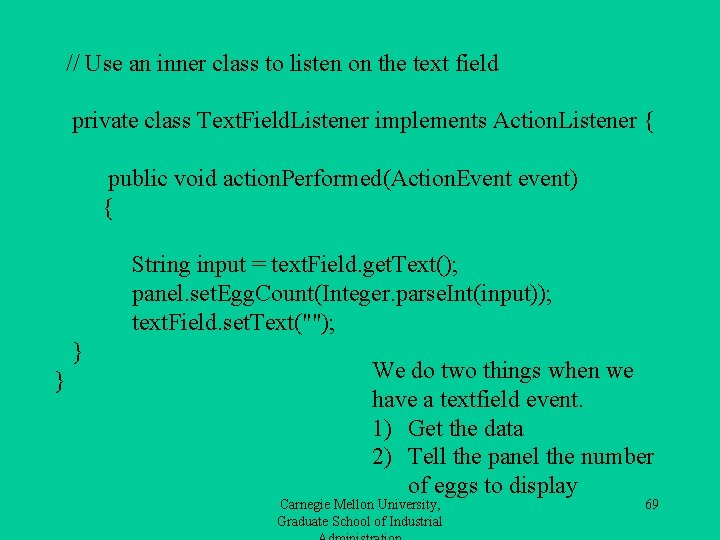
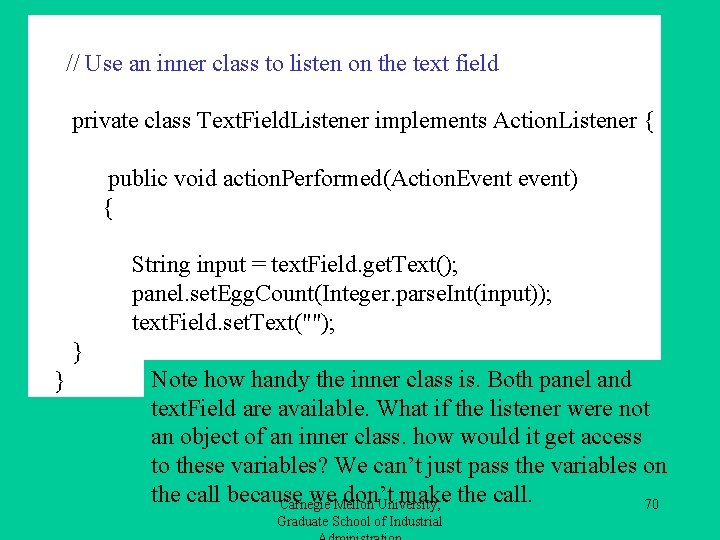
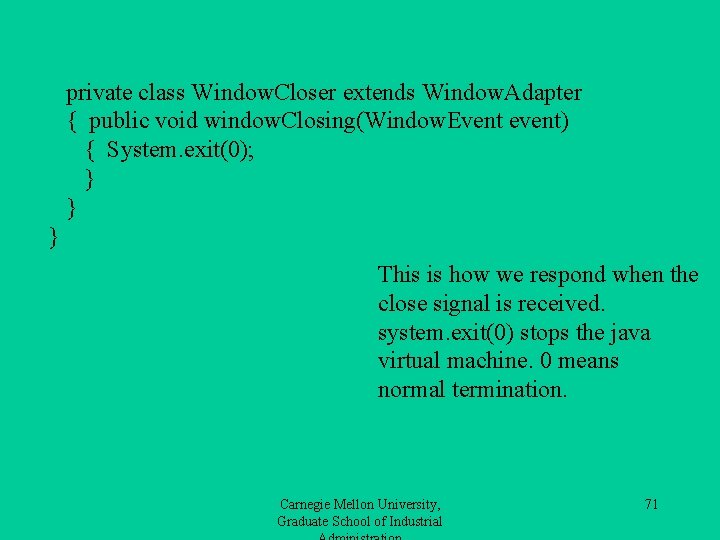
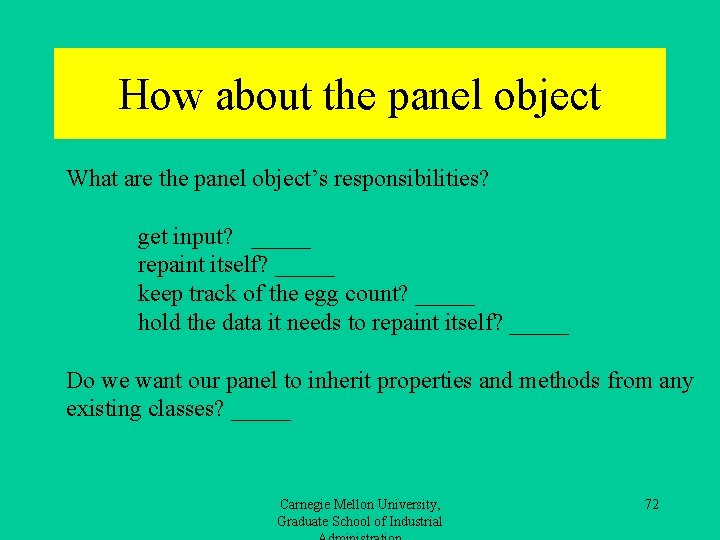
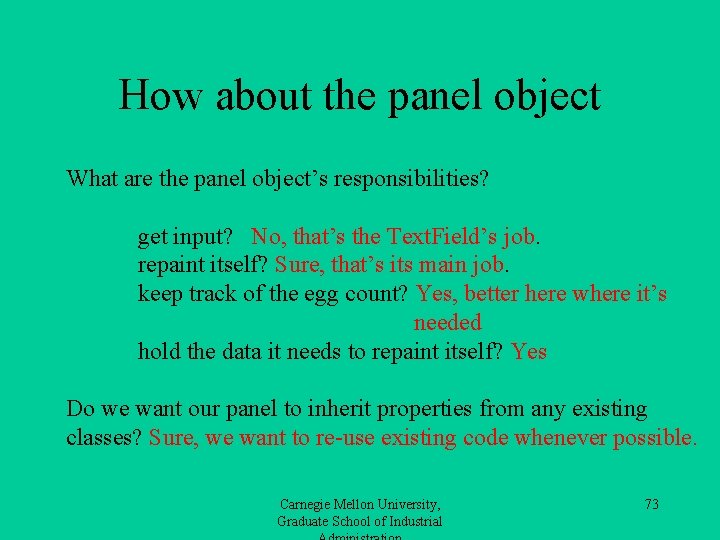
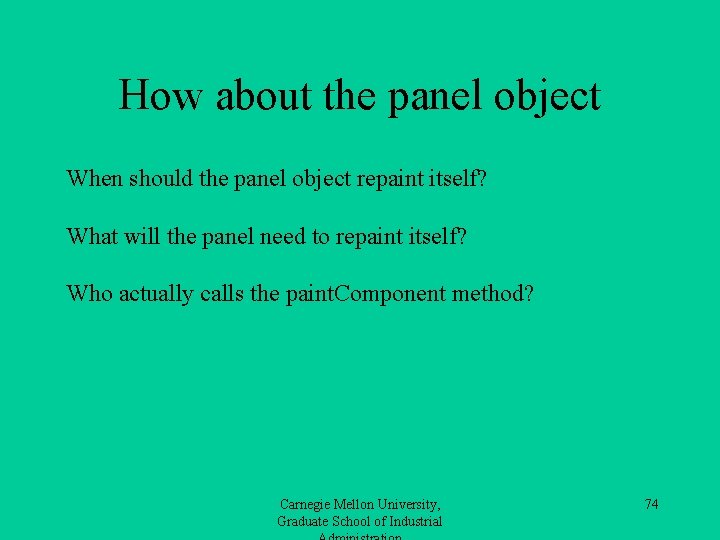
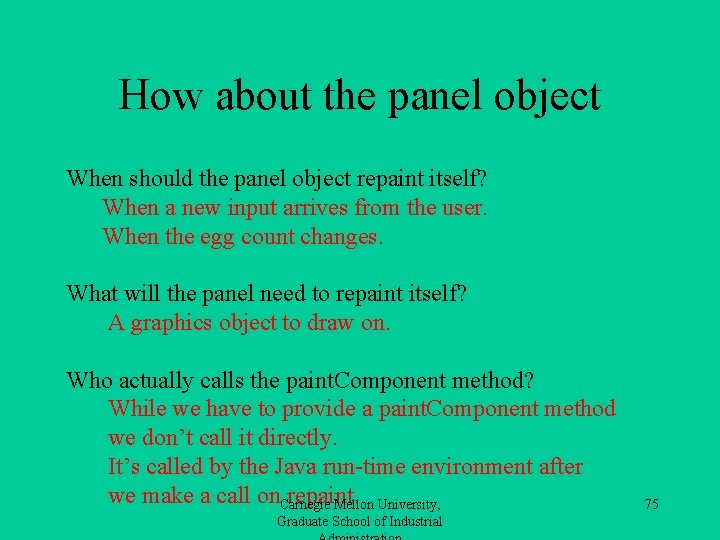
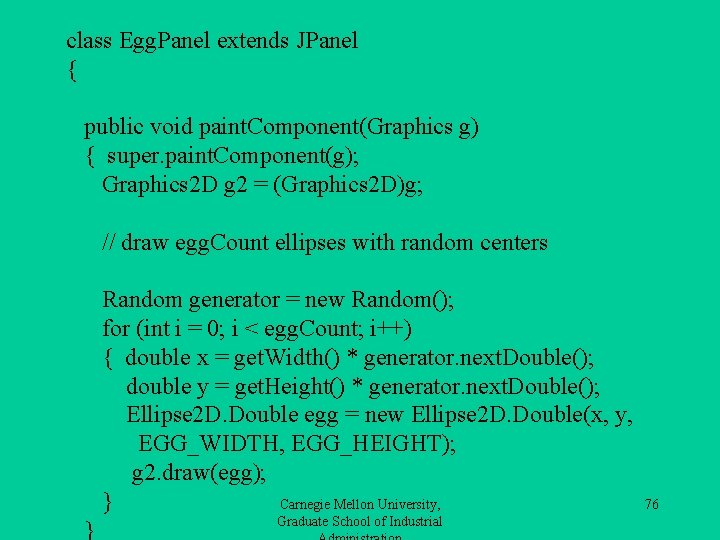
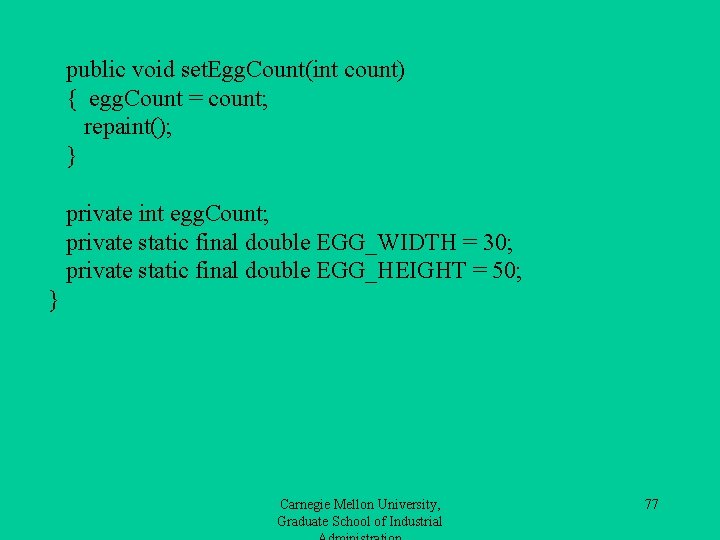
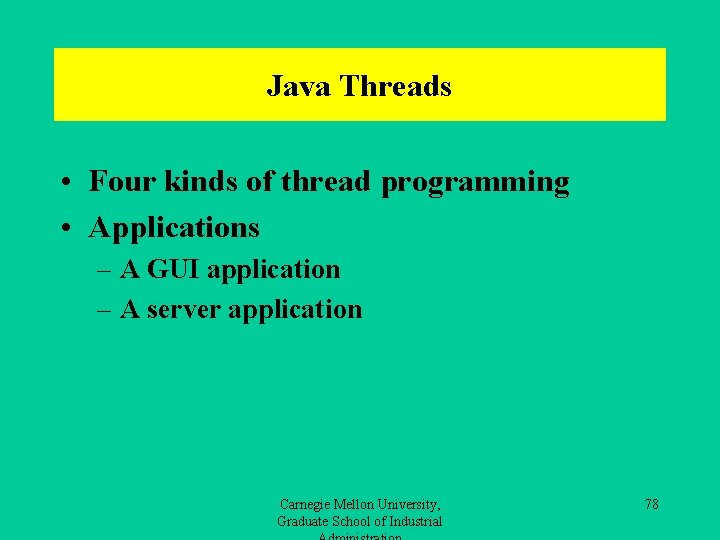
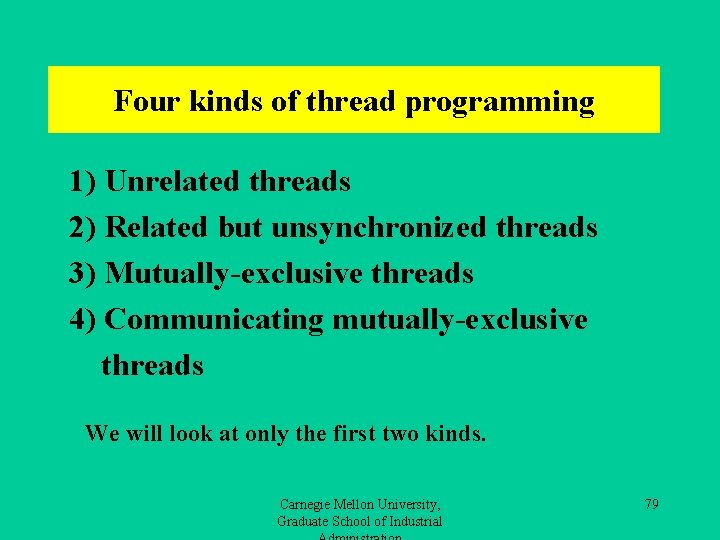
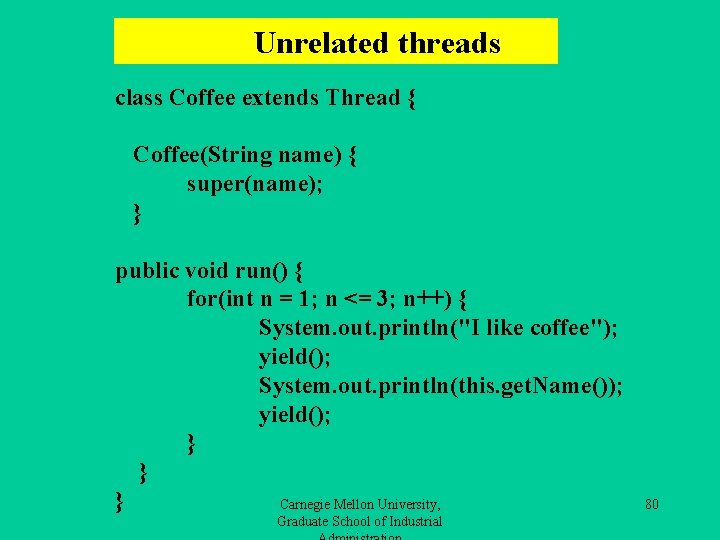
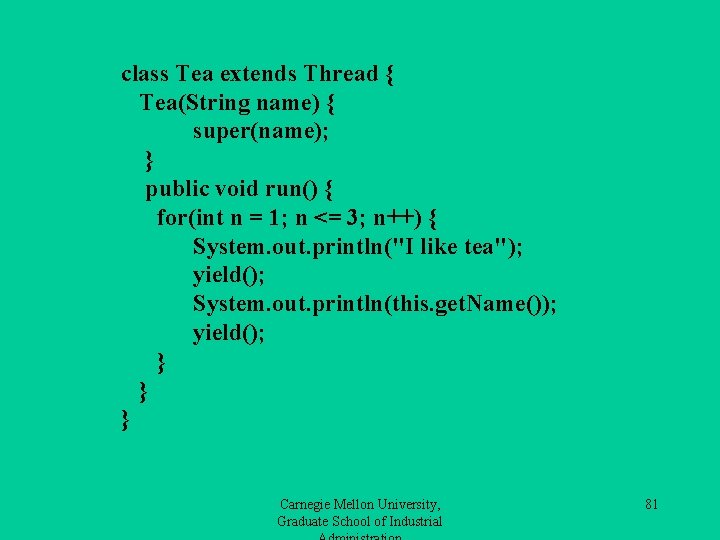
![public class Drinks { public static void main(String args[]) { System. out. println("I am public class Drinks { public static void main(String args[]) { System. out. println("I am](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-82.jpg)
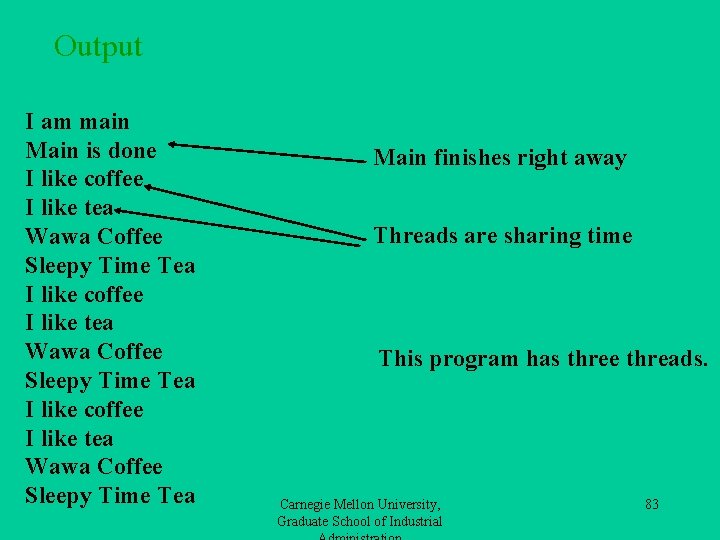
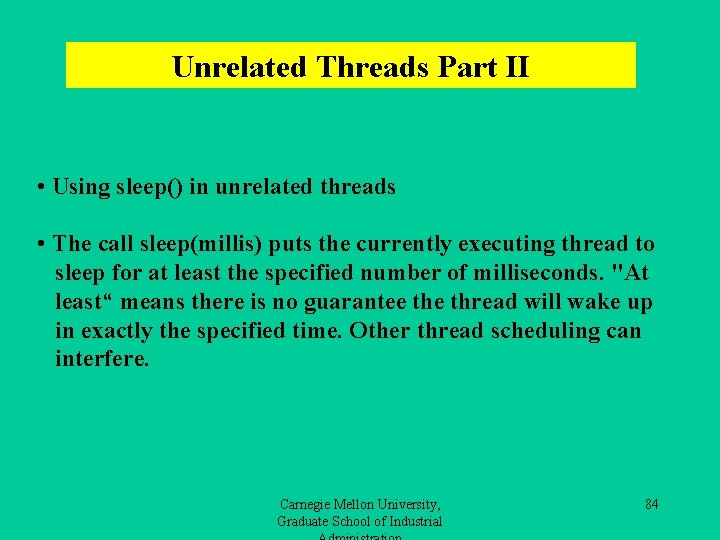
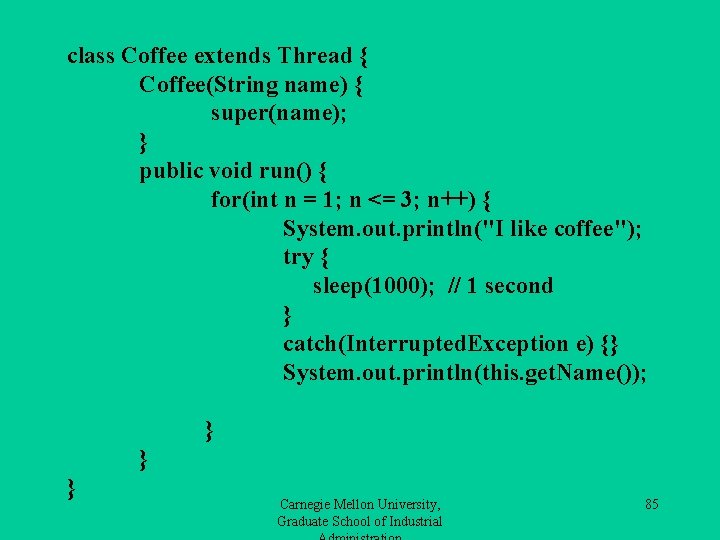
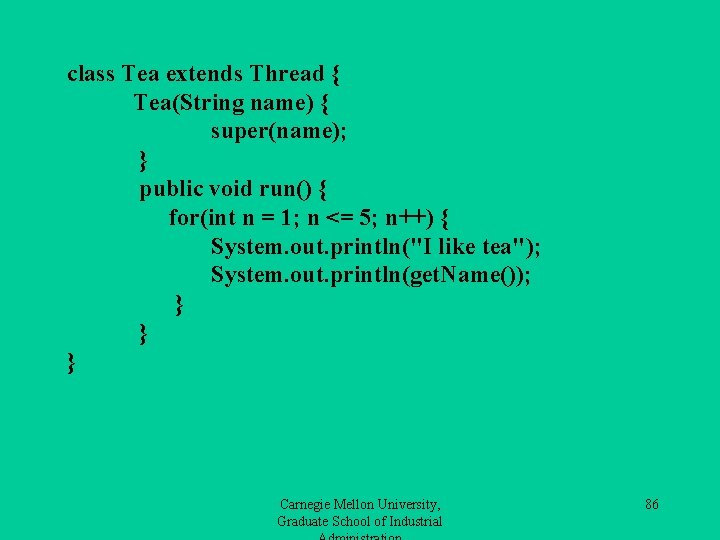
![public class Drinks 2 { public static void main(String args[]) { System. out. println("I public class Drinks 2 { public static void main(String args[]) { System. out. println("I](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-87.jpg)
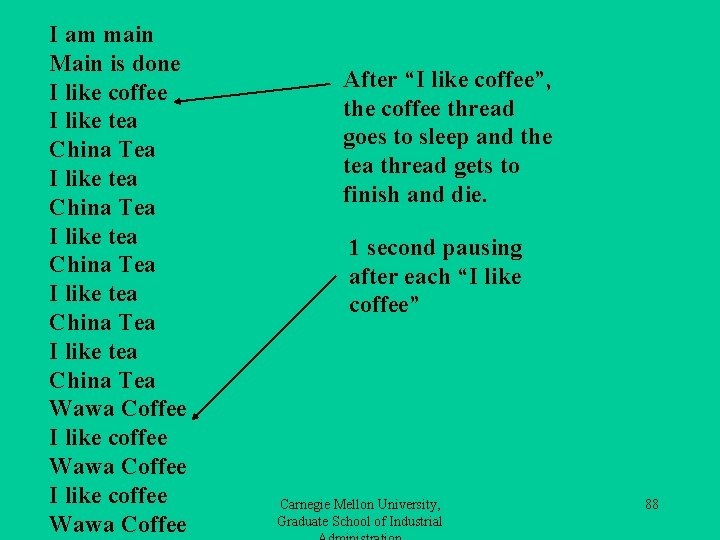
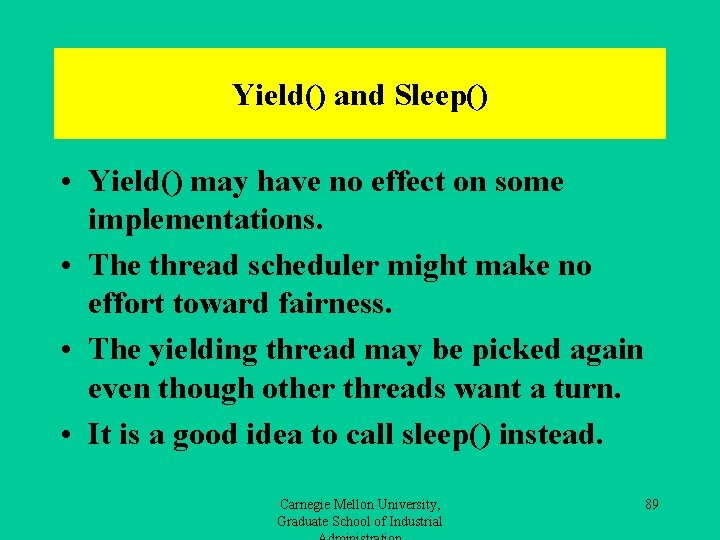
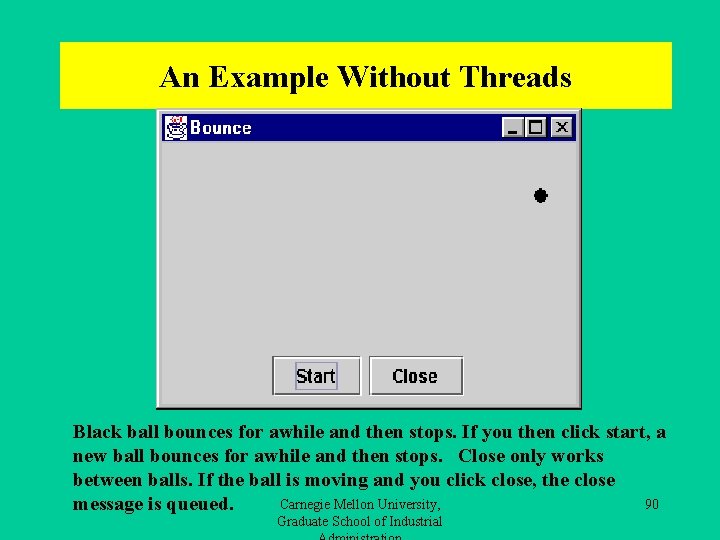
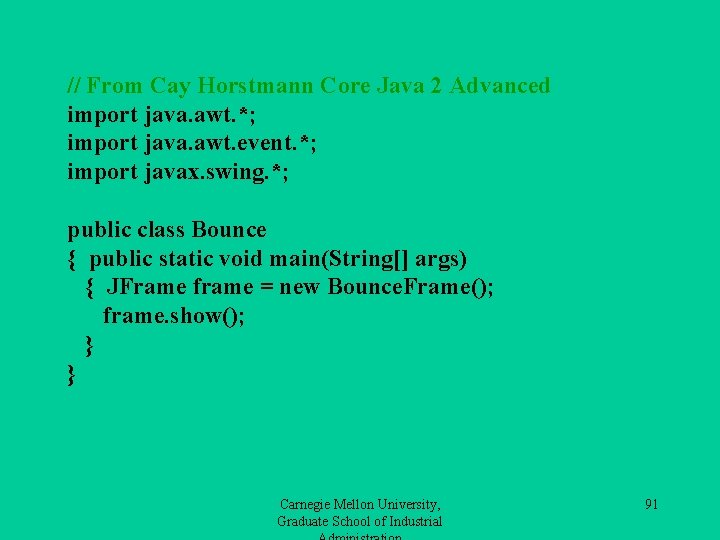
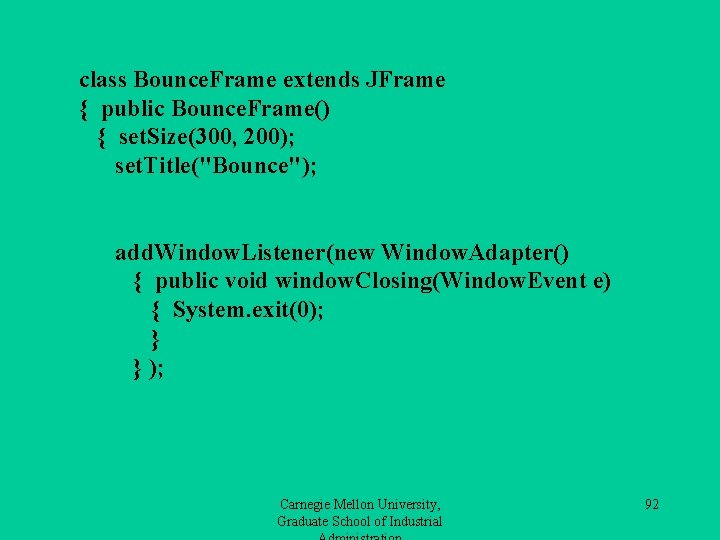
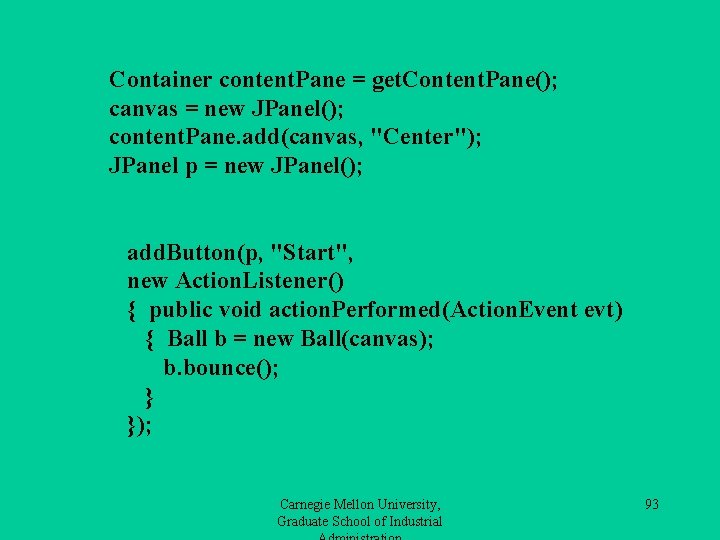
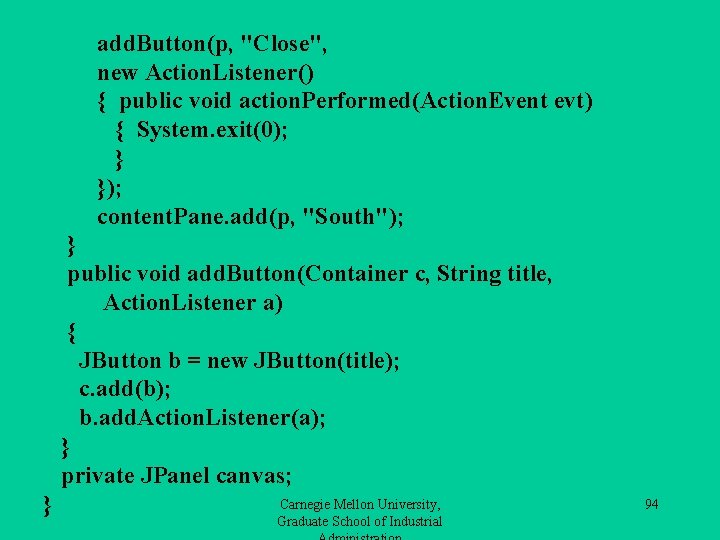
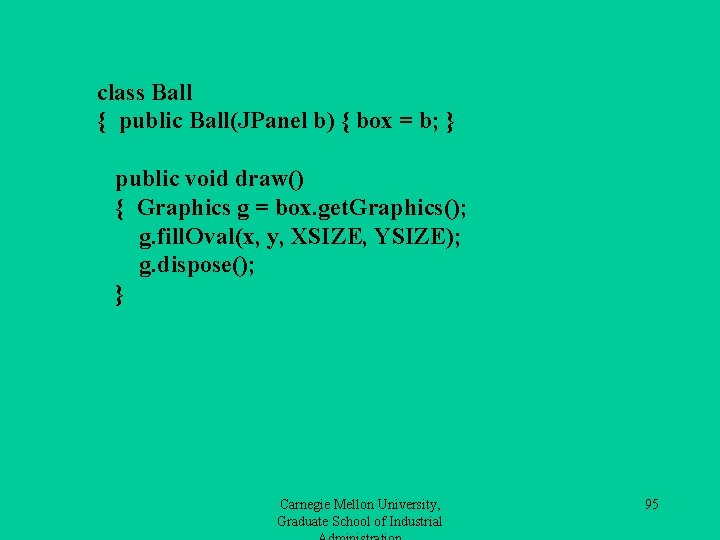
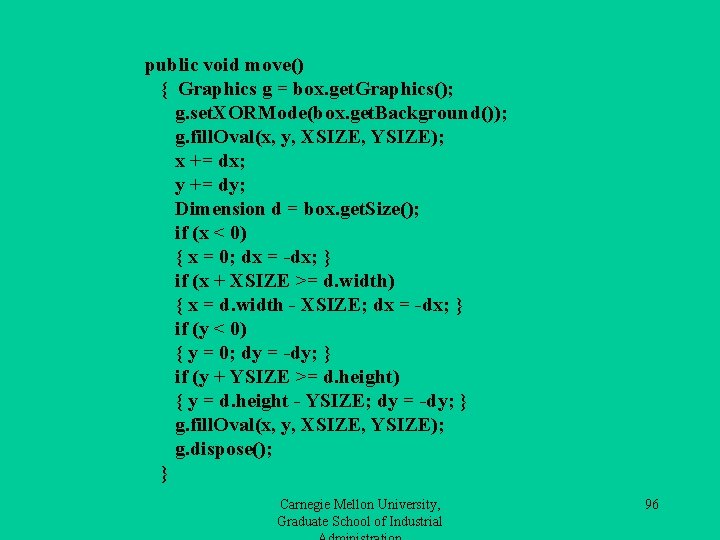
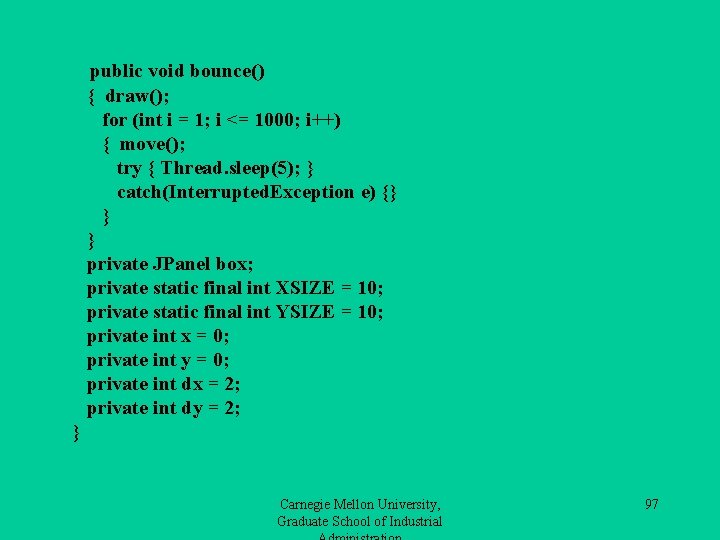
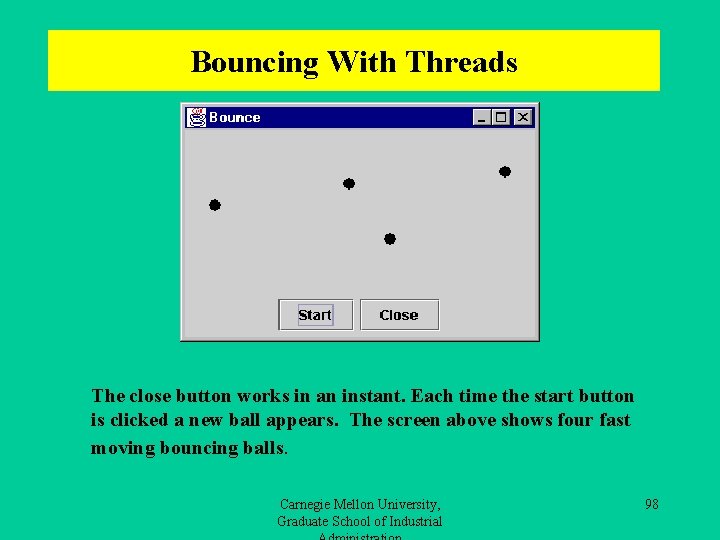
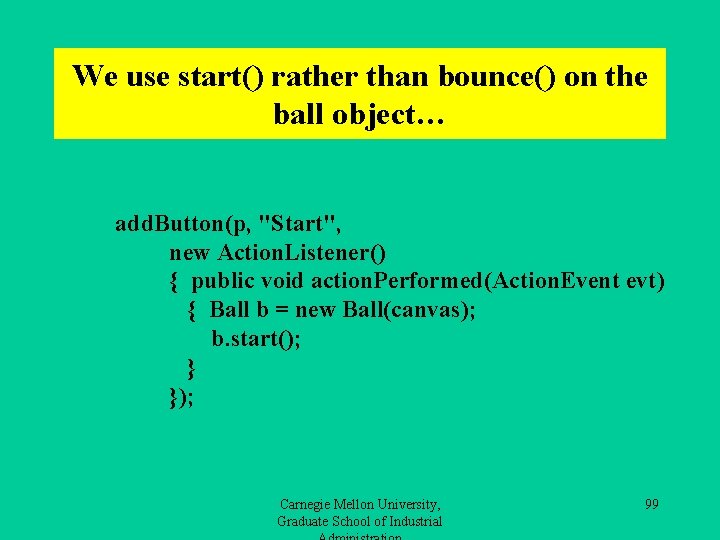
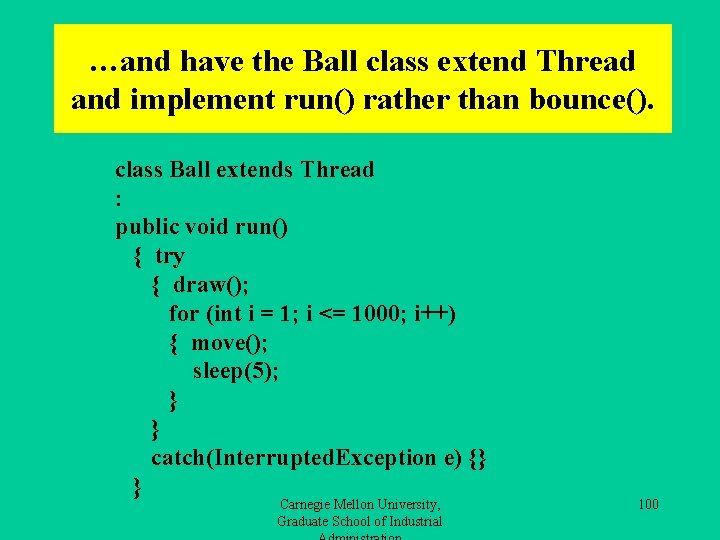
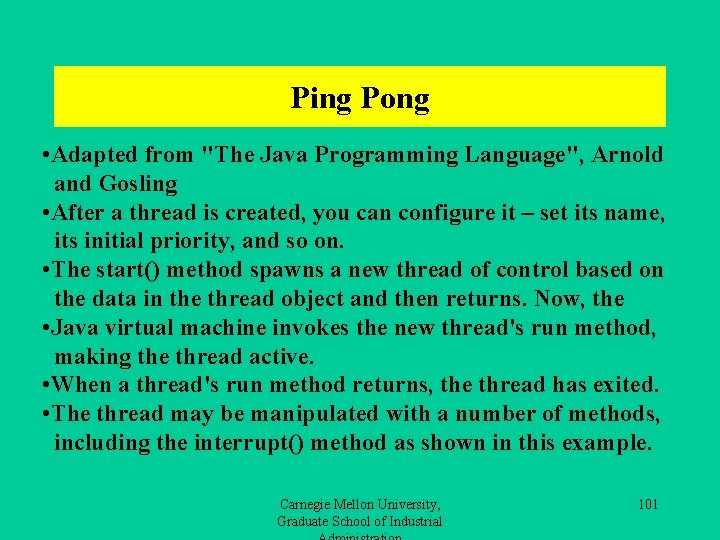
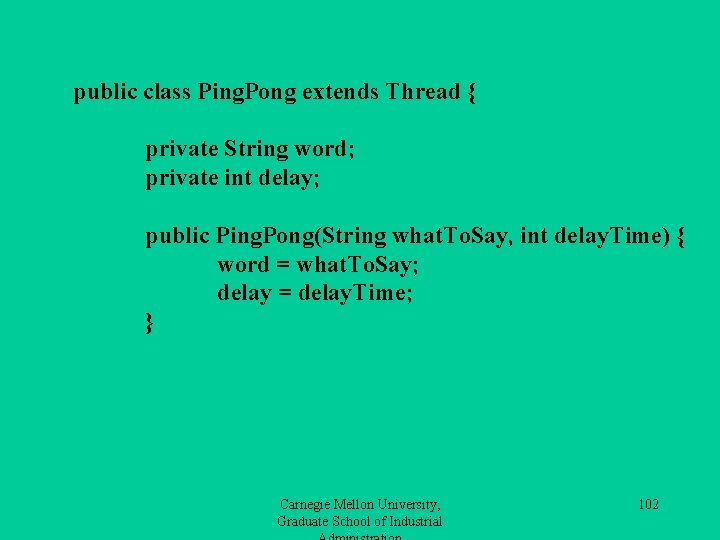
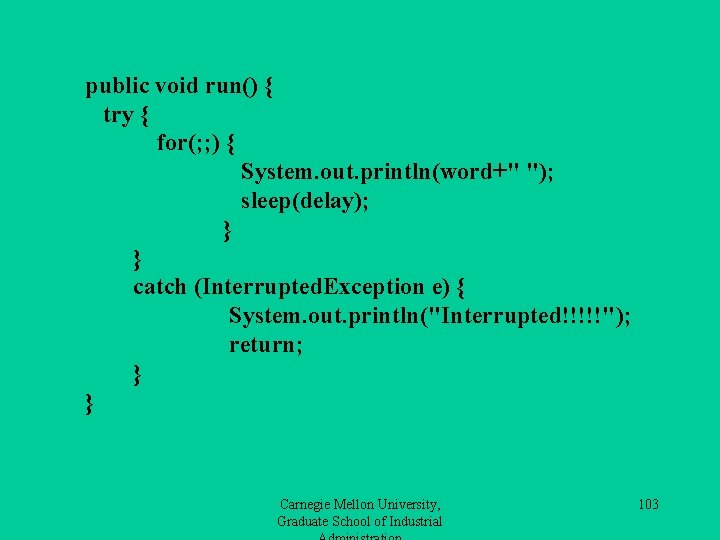
![public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping", public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping",](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-104.jpg)
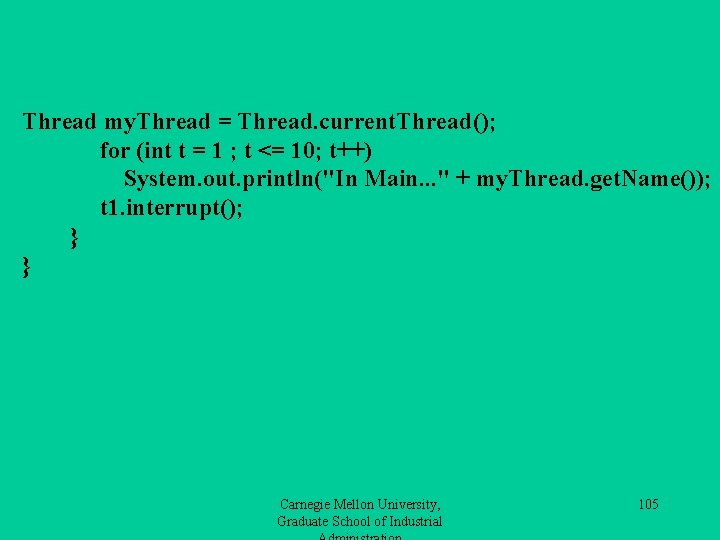
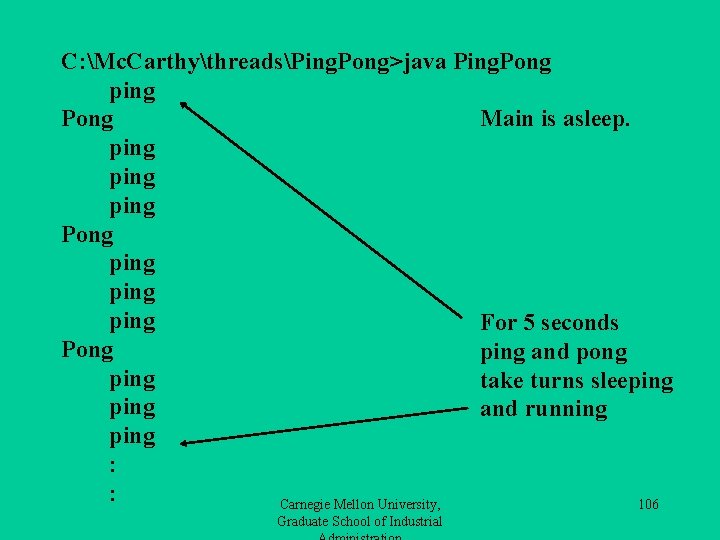
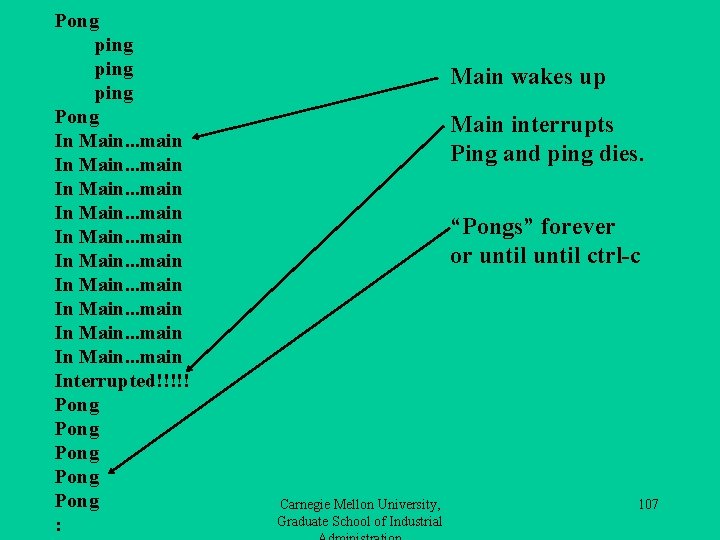
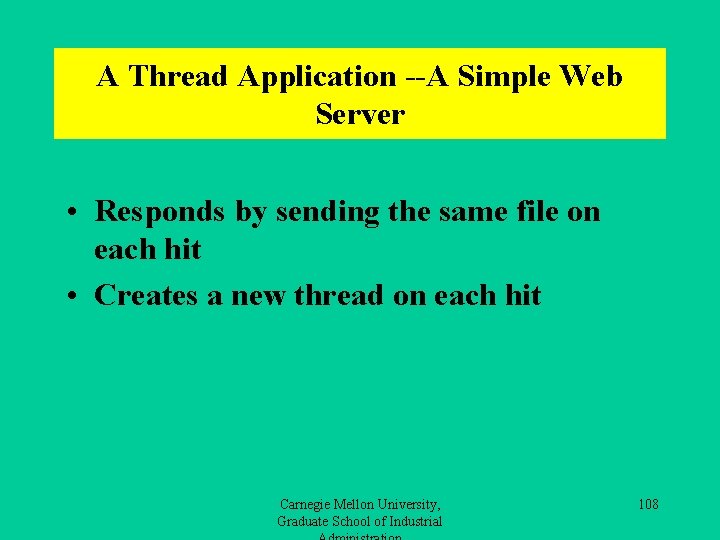
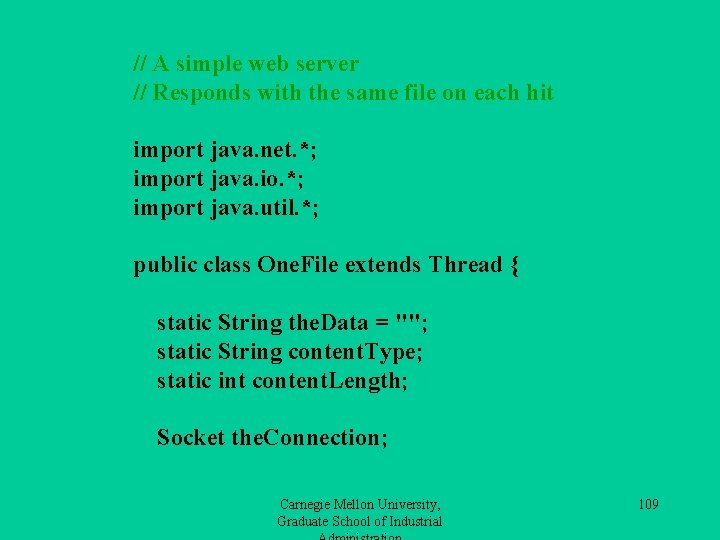
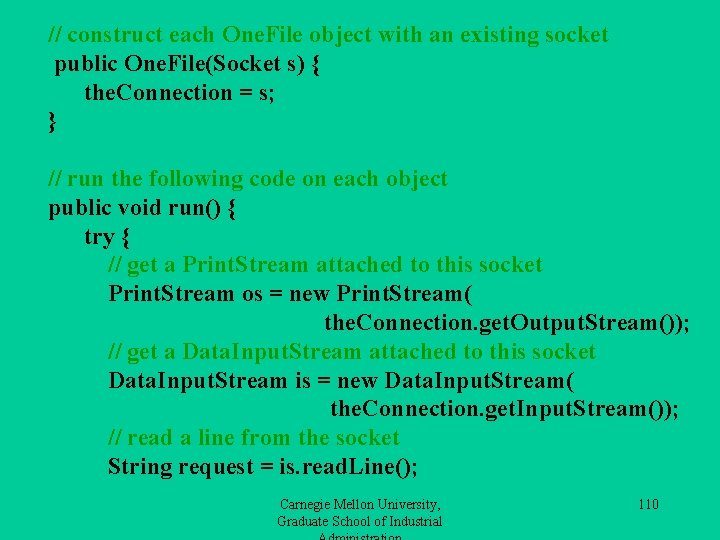
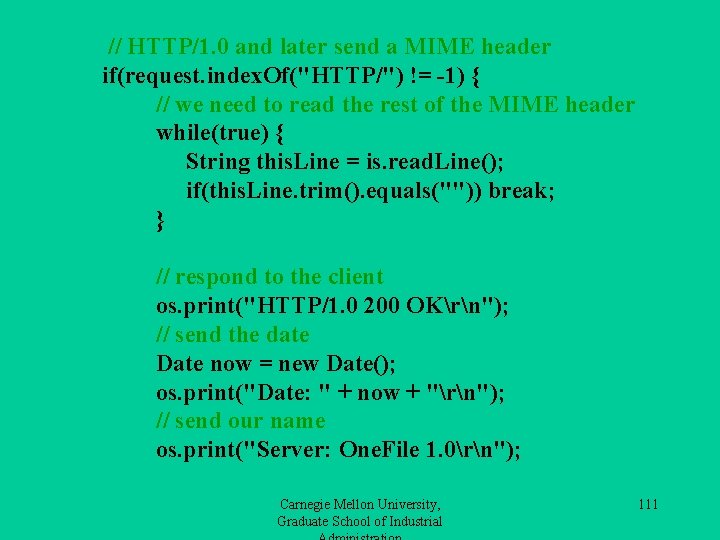
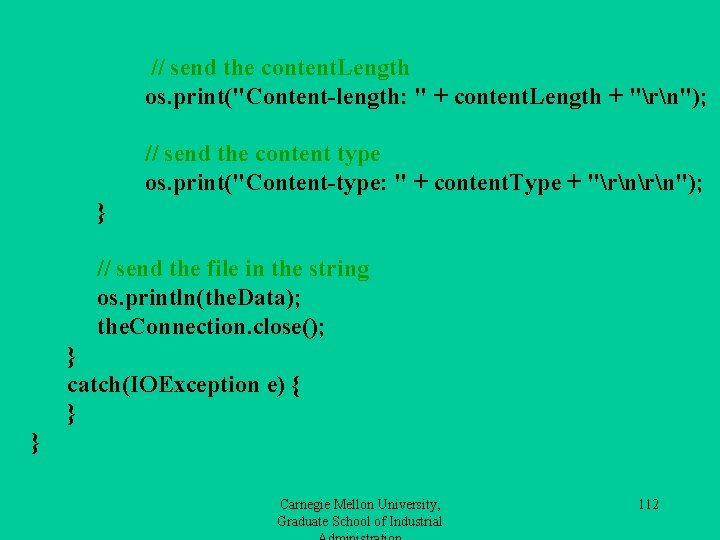
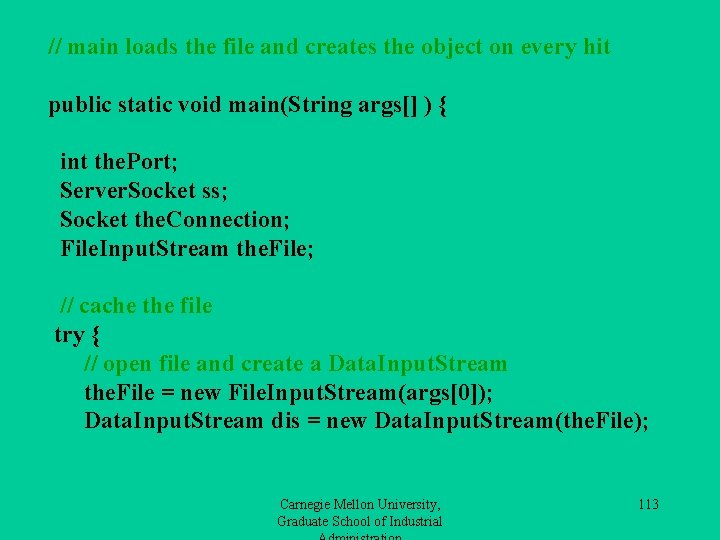
![} // determine the content type of this file if(args[0]. ends. With(". html") || } // determine the content type of this file if(args[0]. ends. With(". html") ||](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-114.jpg)
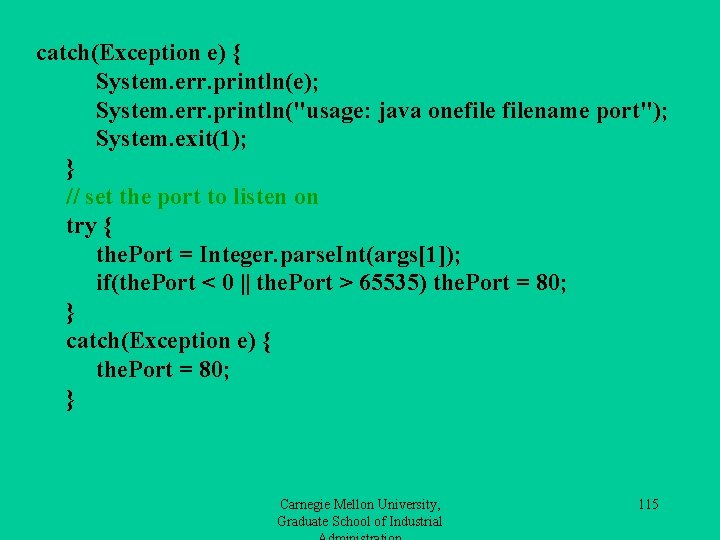
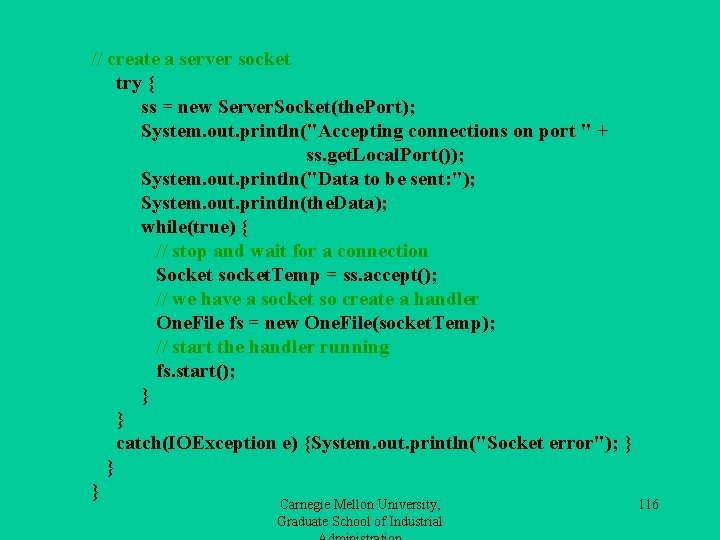
- Slides: 116
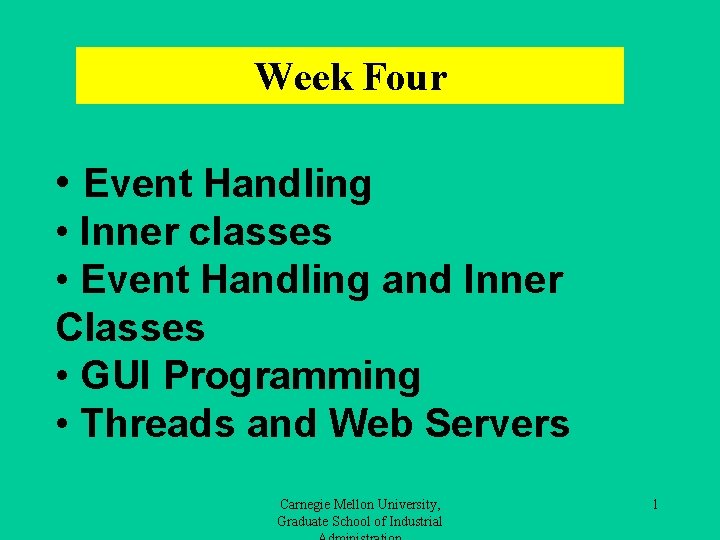
Week Four • Event Handling • Inner classes • Event Handling and Inner Classes • GUI Programming • Threads and Web Servers Carnegie Mellon University, Graduate School of Industrial 1
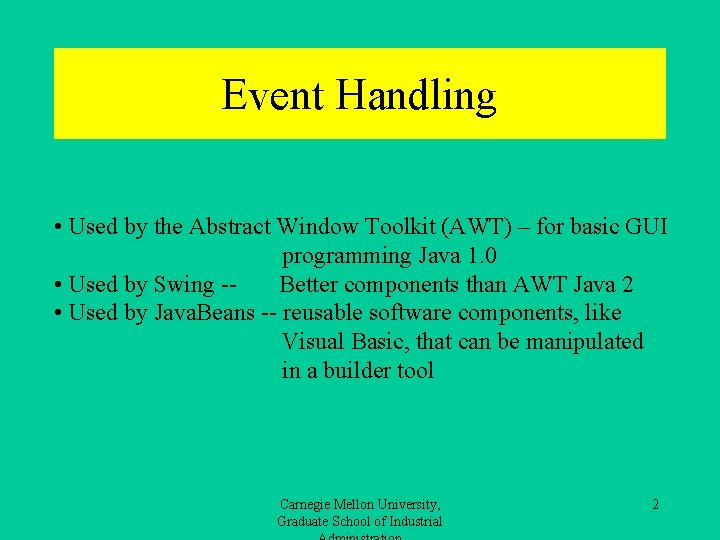
Event Handling • Used by the Abstract Window Toolkit (AWT) – for basic GUI programming Java 1. 0 • Used by Swing -Better components than AWT Java 2 • Used by Java. Beans -- reusable software components, like Visual Basic, that can be manipulated in a builder tool Carnegie Mellon University, Graduate School of Industrial 2
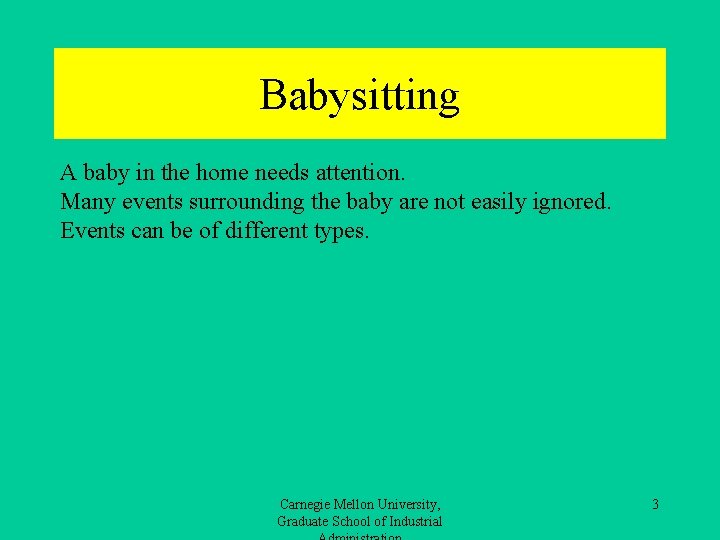
Babysitting A baby in the home needs attention. Many events surrounding the baby are not easily ignored. Events can be of different types. Carnegie Mellon University, Graduate School of Industrial 3
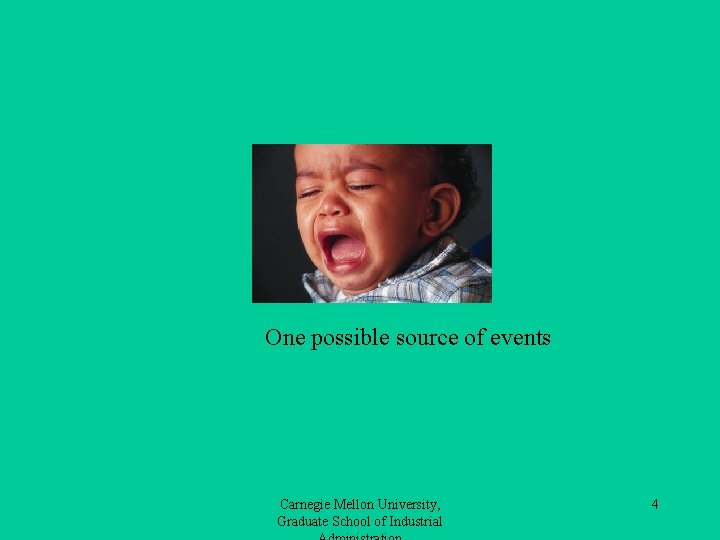
One possible source of events Carnegie Mellon University, Graduate School of Industrial 4
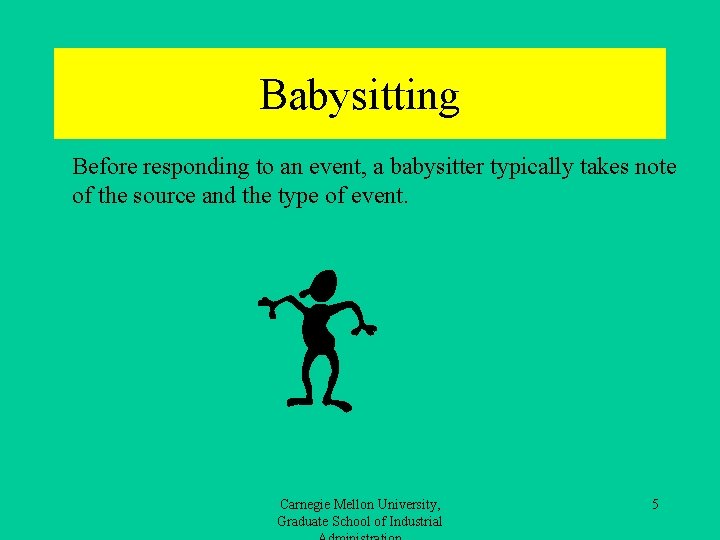
Babysitting Before responding to an event, a babysitter typically takes note of the source and the type of event. Carnegie Mellon University, Graduate School of Industrial 5
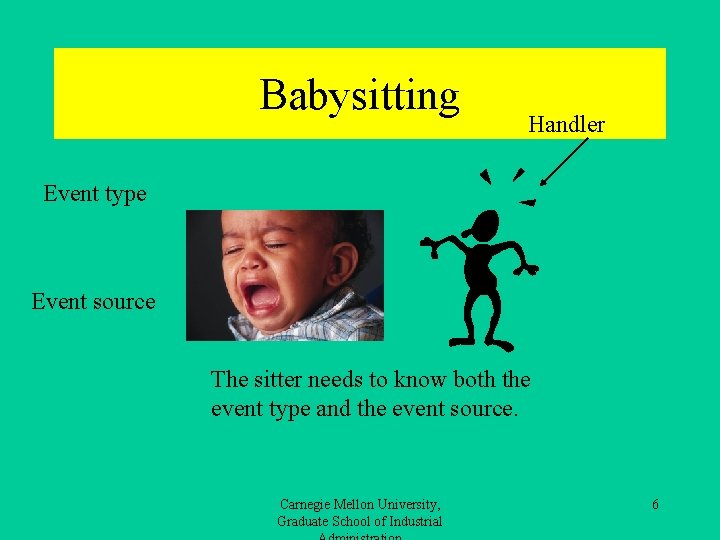
Babysitting Handler Event type Event source The sitter needs to know both the event type and the event source. Carnegie Mellon University, Graduate School of Industrial 6
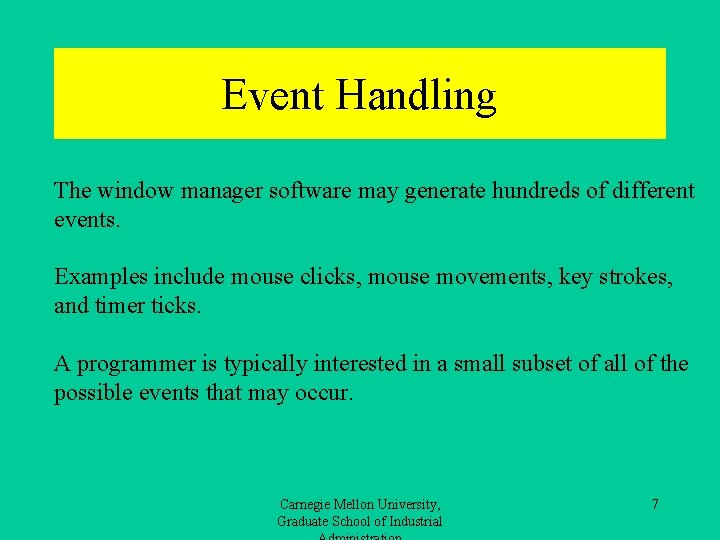
Event Handling The window manager software may generate hundreds of different events. Examples include mouse clicks, mouse movements, key strokes, and timer ticks. A programmer is typically interested in a small subset of all of the possible events that may occur. Carnegie Mellon University, Graduate School of Industrial 7
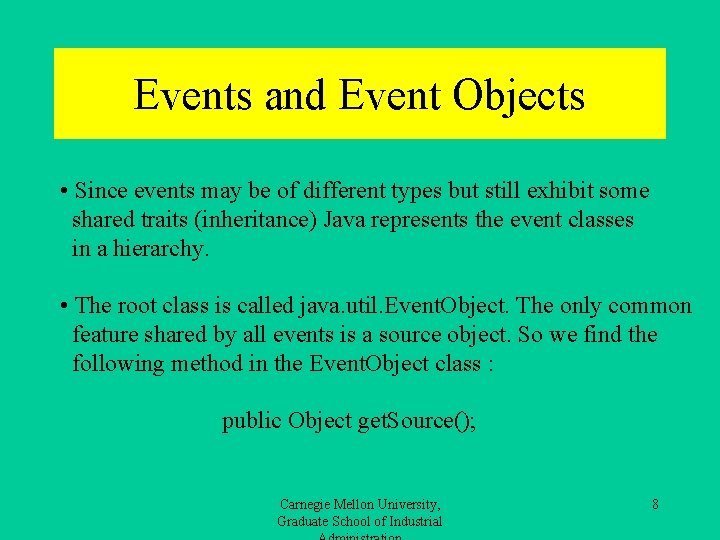
Events and Event Objects • Since events may be of different types but still exhibit some shared traits (inheritance) Java represents the event classes in a hierarchy. • The root class is called java. util. Event. Object. The only common feature shared by all events is a source object. So we find the following method in the Event. Object class : public Object get. Source(); Carnegie Mellon University, Graduate School of Industrial 8
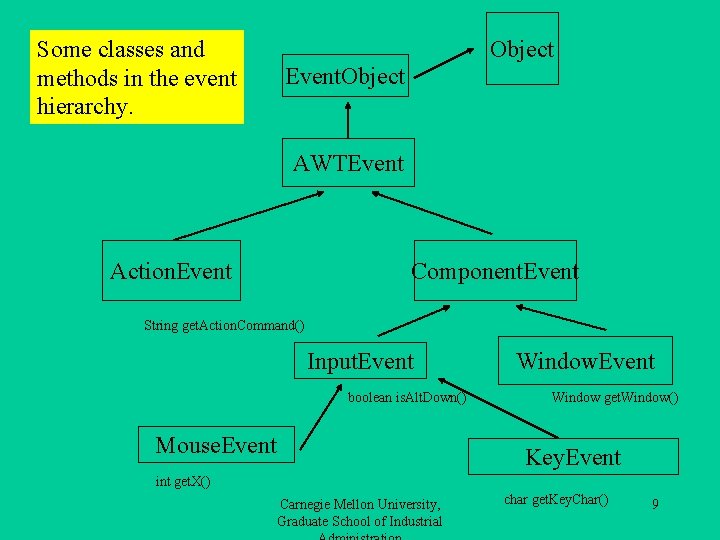
Some classes and methods in the event hierarchy. Object Event. Object AWTEvent Action. Event Component. Event String get. Action. Command() Input. Event boolean is. Alt. Down() Mouse. Event Window get. Window() Key. Event int get. X() Carnegie Mellon University, Graduate School of Industrial char get. Key. Char() 9
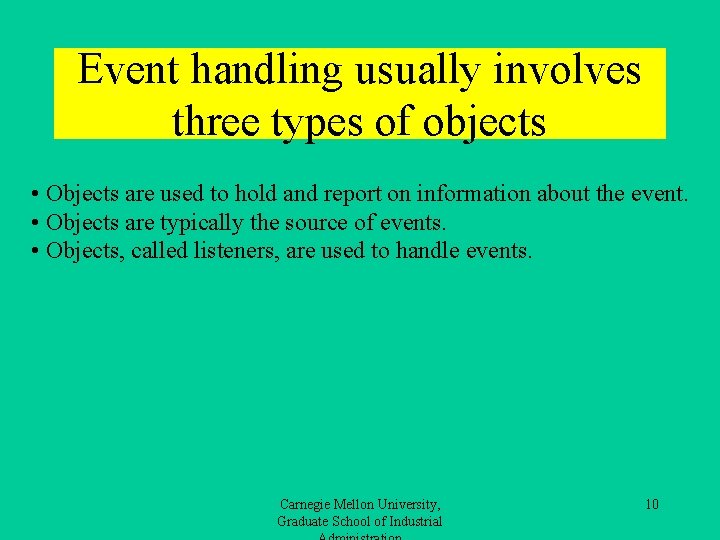
Event handling usually involves three types of objects • Objects are used to hold and report on information about the event. • Objects are typically the source of events. • Objects, called listeners, are used to handle events. Carnegie Mellon University, Graduate School of Industrial 10
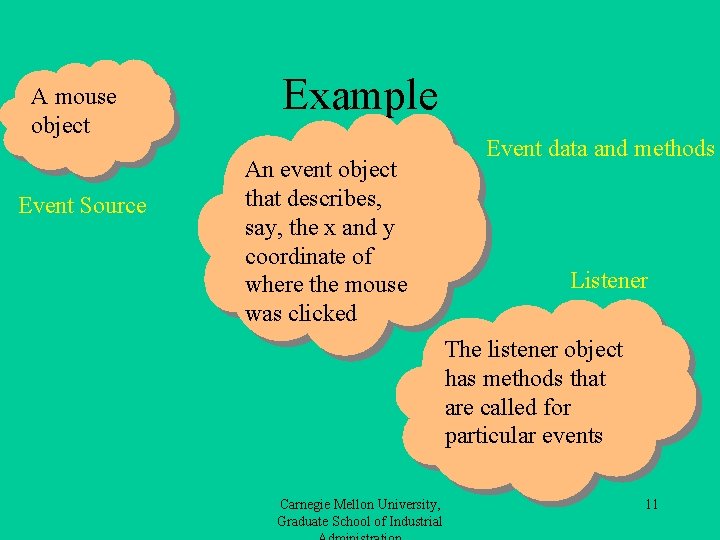
A mouse object Event Source Example An event object that describes, say, the x and y coordinate of where the mouse was clicked Event data and methods Listener The listener object has methods that are called for particular events Carnegie Mellon University, Graduate School of Industrial 11
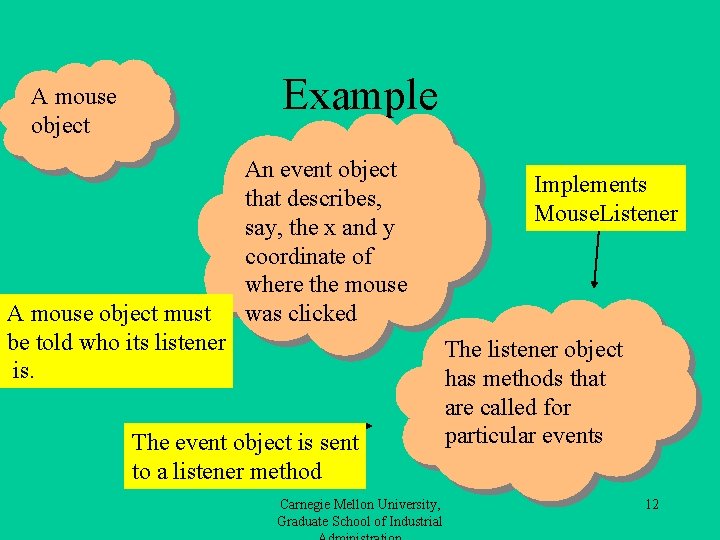
Example A mouse object must be told who its listener is. An event object that describes, say, the x and y coordinate of where the mouse was clicked The event object is sent to a listener method Carnegie Mellon University, Graduate School of Industrial Implements Mouse. Listener The listener object has methods that are called for particular events 12
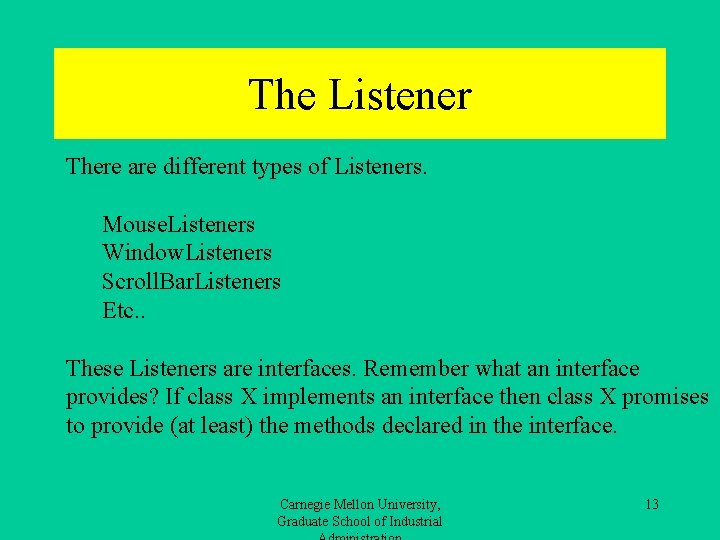
The Listener There are different types of Listeners. Mouse. Listeners Window. Listeners Scroll. Bar. Listeners Etc. . These Listeners are interfaces. Remember what an interface provides? If class X implements an interface then class X promises to provide (at least) the methods declared in the interface. Carnegie Mellon University, Graduate School of Industrial 13
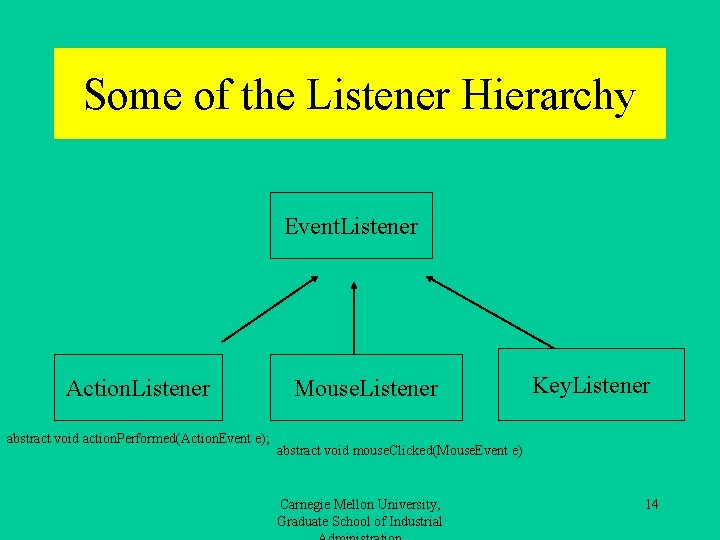
Some of the Listener Hierarchy Event. Listener Action. Listener abstract void action. Performed(Action. Event e); Mouse. Listener Key. Listener abstract void mouse. Clicked(Mouse. Event e) Carnegie Mellon University, Graduate School of Industrial 14
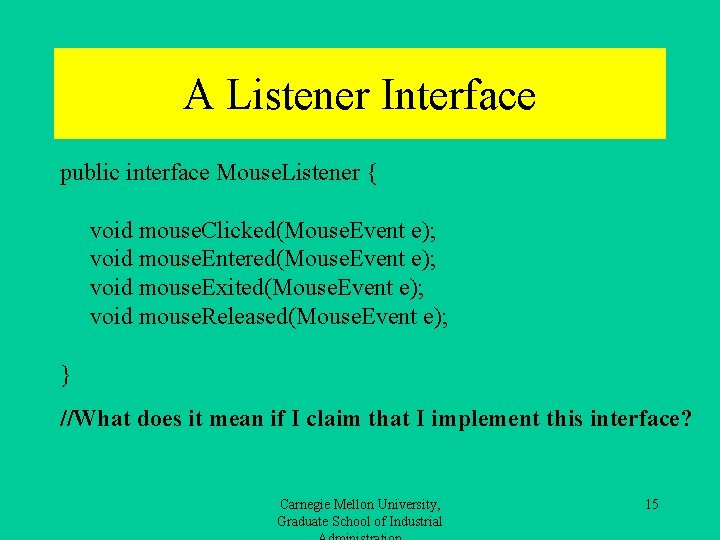
A Listener Interface public interface Mouse. Listener { void mouse. Clicked(Mouse. Event e); void mouse. Entered(Mouse. Event e); void mouse. Exited(Mouse. Event e); void mouse. Released(Mouse. Event e); } //What does it mean if I claim that I implement this interface? Carnegie Mellon University, Graduate School of Industrial 15
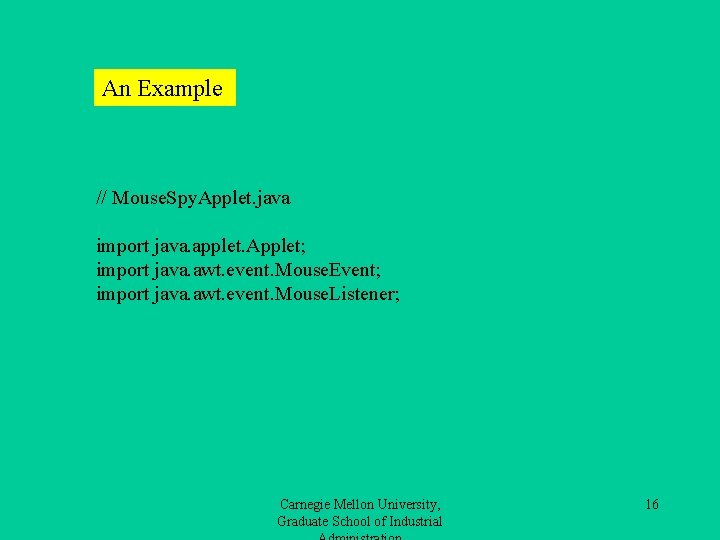
An Example // Mouse. Spy. Applet. java import java. applet. Applet; import java. awt. event. Mouse. Event; import java. awt. event. Mouse. Listener; Carnegie Mellon University, Graduate School of Industrial 16
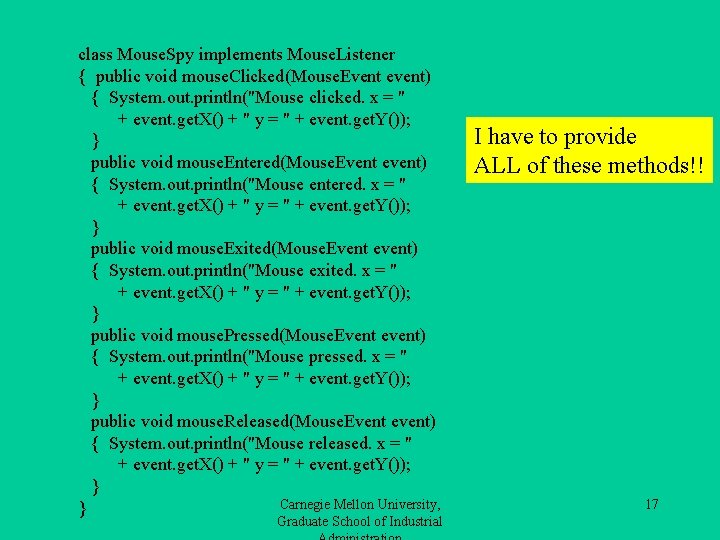
class Mouse. Spy implements Mouse. Listener { public void mouse. Clicked(Mouse. Event event) { System. out. println("Mouse clicked. x = " + event. get. X() + " y = " + event. get. Y()); } public void mouse. Entered(Mouse. Event event) { System. out. println("Mouse entered. x = " + event. get. X() + " y = " + event. get. Y()); } public void mouse. Exited(Mouse. Event event) { System. out. println("Mouse exited. x = " + event. get. X() + " y = " + event. get. Y()); } public void mouse. Pressed(Mouse. Event event) { System. out. println("Mouse pressed. x = " + event. get. X() + " y = " + event. get. Y()); } public void mouse. Released(Mouse. Event event) { System. out. println("Mouse released. x = " + event. get. X() + " y = " + event. get. Y()); } Carnegie Mellon University, } Graduate School of Industrial I have to provide ALL of these methods!! 17
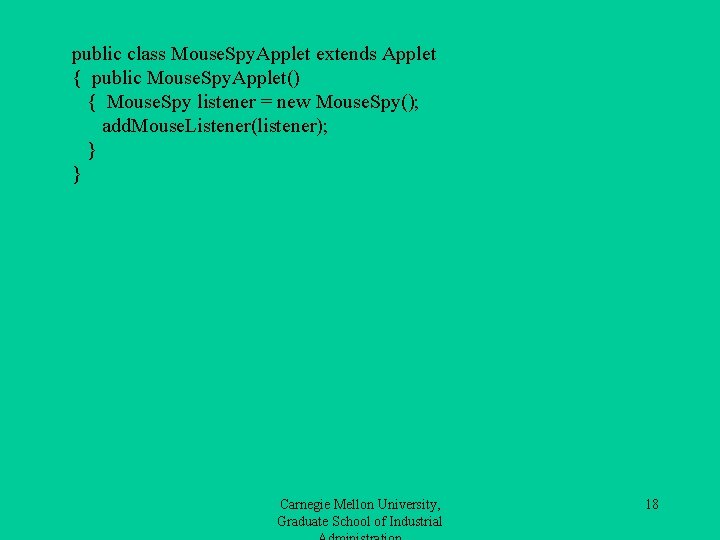
public class Mouse. Spy. Applet extends Applet { public Mouse. Spy. Applet() { Mouse. Spy listener = new Mouse. Spy(); add. Mouse. Listener(listener); } } Carnegie Mellon University, Graduate School of Industrial 18
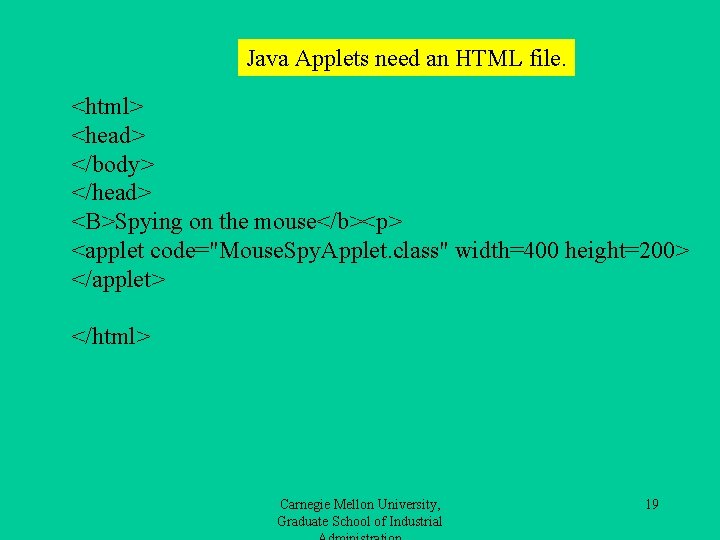
Java Applets need an HTML file. <html> <head> </body> </head> <B>Spying on the mouse</b><p> <applet code="Mouse. Spy. Applet. class" width=400 height=200> </applet> </html> Carnegie Mellon University, Graduate School of Industrial 19
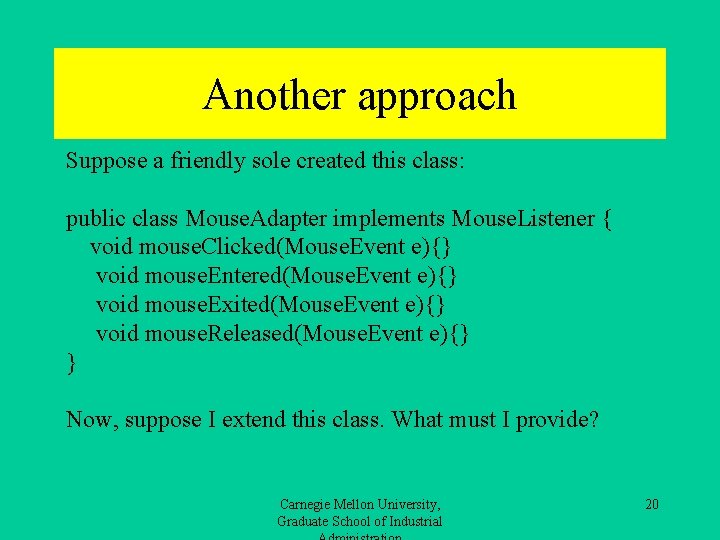
Another approach Suppose a friendly sole created this class: public class Mouse. Adapter implements Mouse. Listener { void mouse. Clicked(Mouse. Event e){} void mouse. Entered(Mouse. Event e){} void mouse. Exited(Mouse. Event e){} void mouse. Released(Mouse. Event e){} } Now, suppose I extend this class. What must I provide? Carnegie Mellon University, Graduate School of Industrial 20
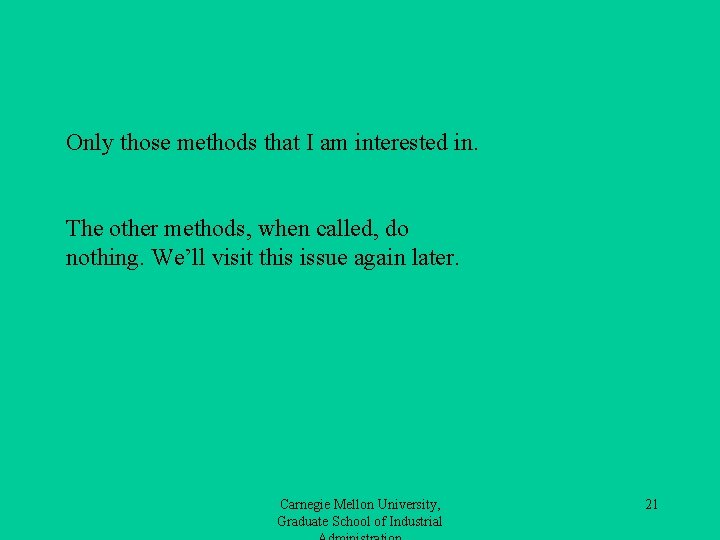
Only those methods that I am interested in. The other methods, when called, do nothing. We’ll visit this issue again later. Carnegie Mellon University, Graduate School of Industrial 21
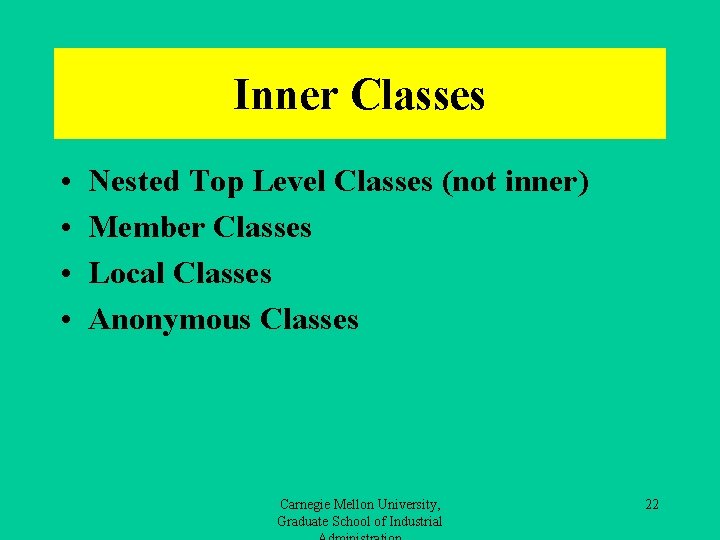
Inner Classes • • Nested Top Level Classes (not inner) Member Classes Local Classes Anonymous Classes Carnegie Mellon University, Graduate School of Industrial 22
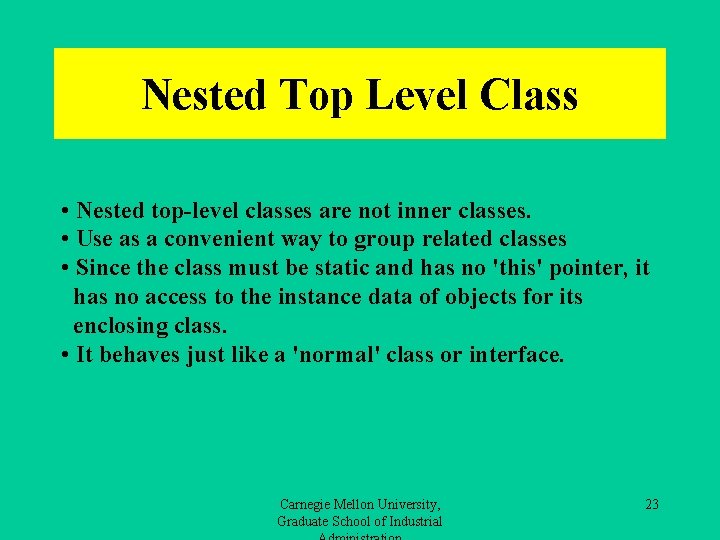
Nested Top Level Class • Nested top-level classes are not inner classes. • Use as a convenient way to group related classes • Since the class must be static and has no 'this' pointer, it has no access to the instance data of objects for its enclosing class. • It behaves just like a 'normal' class or interface. Carnegie Mellon University, Graduate School of Industrial 23
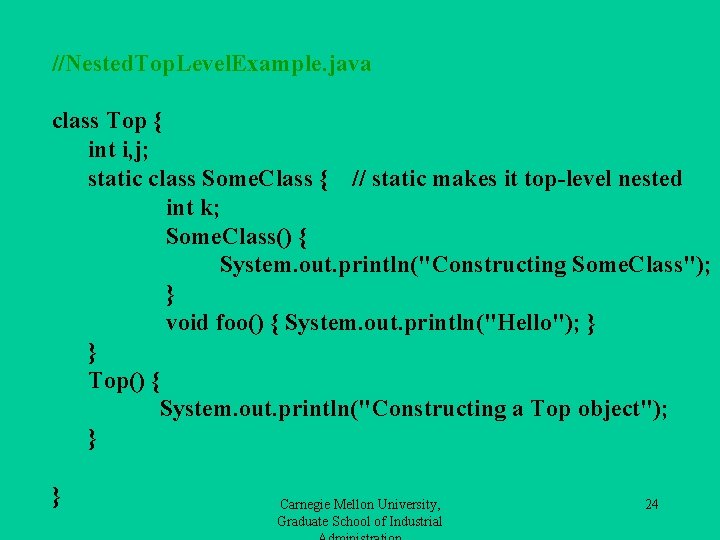
//Nested. Top. Level. Example. java class Top { int i, j; static class Some. Class { // static makes it top-level nested int k; Some. Class() { System. out. println("Constructing Some. Class"); } void foo() { System. out. println("Hello"); } } Top() { System. out. println("Constructing a Top object"); } } Carnegie Mellon University, Graduate School of Industrial 24
![public class Nested Top Level Example public static void mainString args Top public class Nested. Top. Level. Example { public static void main(String args[]) { Top](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-25.jpg)
public class Nested. Top. Level. Example { public static void main(String args[]) { Top my. Top = new Top(); Top. Some. Class my. Object = new Top. Some. Class(); my. Object. foo(); } } Output Constructing a Top object Constructing Some. Class Hello Carnegie Mellon University, Graduate School of Industrial 25
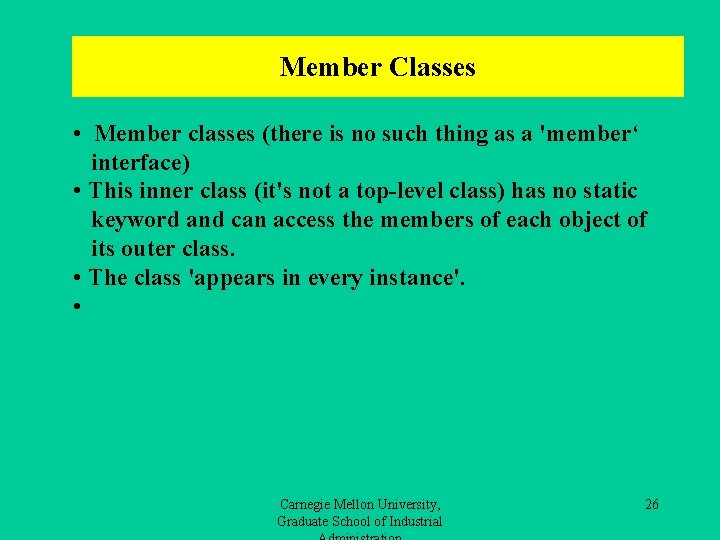
Member Classes • Member classes (there is no such thing as a 'member‘ interface) • This inner class (it's not a top-level class) has no static keyword and can access the members of each object of its outer class. • The class 'appears in every instance'. • Carnegie Mellon University, Graduate School of Industrial 26
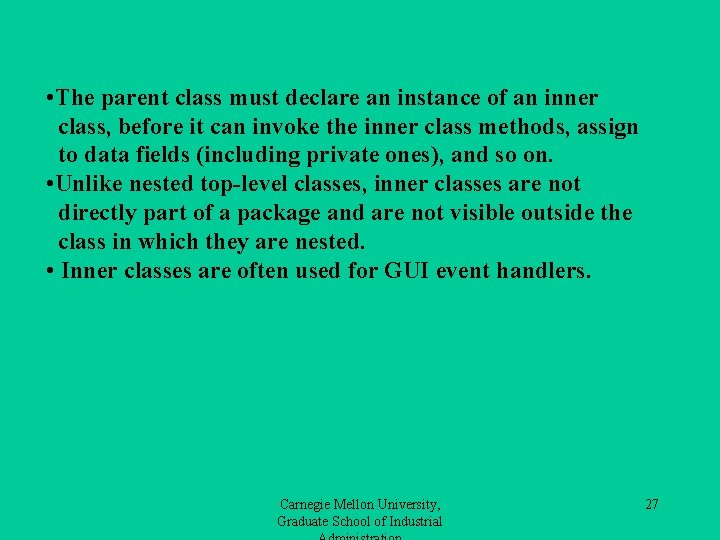
• The parent class must declare an instance of an inner class, before it can invoke the inner class methods, assign to data fields (including private ones), and so on. • Unlike nested top-level classes, inner classes are not directly part of a package and are not visible outside the class in which they are nested. • Inner classes are often used for GUI event handlers. Carnegie Mellon University, Graduate School of Industrial 27
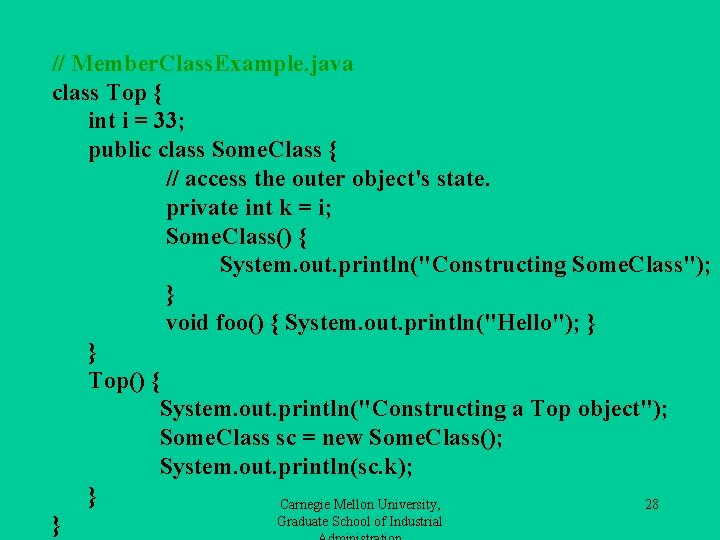
// Member. Class. Example. java class Top { int i = 33; public class Some. Class { // access the outer object's state. private int k = i; Some. Class() { System. out. println("Constructing Some. Class"); } void foo() { System. out. println("Hello"); } } Top() { System. out. println("Constructing a Top object"); Some. Class sc = new Some. Class(); System. out. println(sc. k); } Carnegie Mellon University, 28 Graduate School of Industrial }
![public class Member Class Example public static void mainString args Top my public class Member. Class. Example { public static void main(String args[]) { Top my.](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-29.jpg)
public class Member. Class. Example { public static void main(String args[]) { Top my. Object = new Top(); } } // OUTPUT Constructing a Top object Constructing Some. Class 33 Carnegie Mellon University, Graduate School of Industrial 29
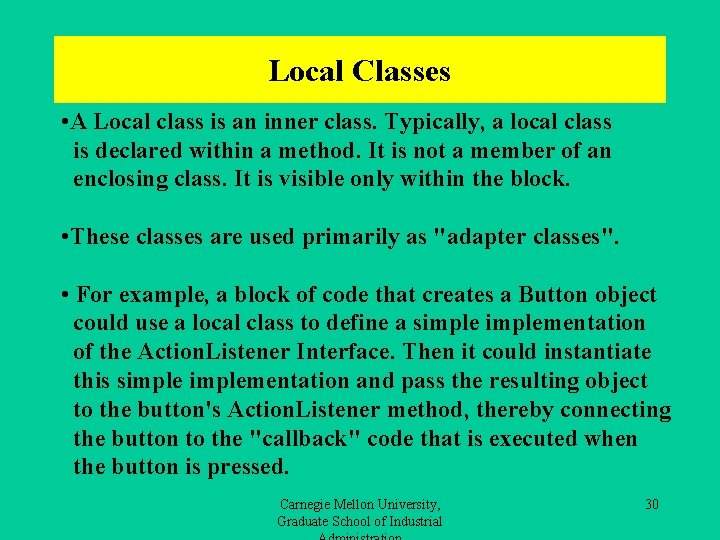
Local Classes • A Local class is an inner class. Typically, a local class is declared within a method. It is not a member of an enclosing class. It is visible only within the block. • These classes are used primarily as "adapter classes". • For example, a block of code that creates a Button object could use a local class to define a simplementation of the Action. Listener Interface. Then it could instantiate this simplementation and pass the resulting object to the button's Action. Listener method, thereby connecting the button to the "callback" code that is executed when the button is pressed. Carnegie Mellon University, Graduate School of Industrial 30
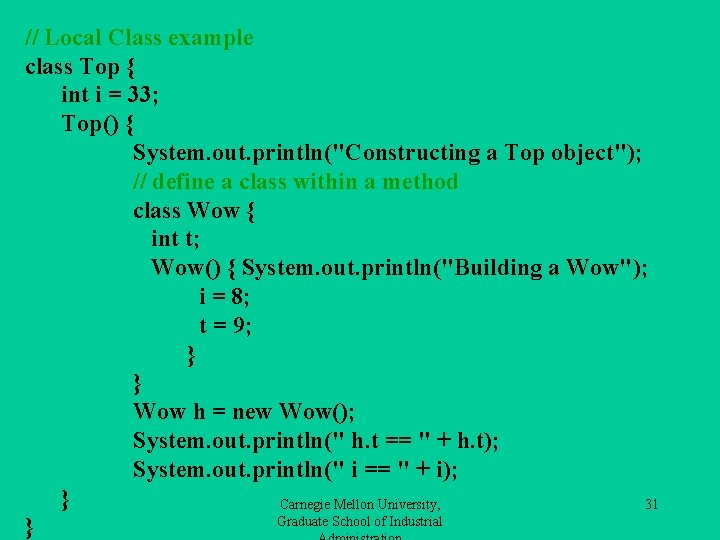
// Local Class example class Top { int i = 33; Top() { System. out. println("Constructing a Top object"); // define a class within a method class Wow { int t; Wow() { System. out. println("Building a Wow"); i = 8; t = 9; } } Wow h = new Wow(); System. out. println(" h. t == " + h. t); System. out. println(" i == " + i); } Carnegie Mellon University, 31 Graduate School of Industrial }
![public class Local Example public static void mainString args Top my Object public class Local. Example { public static void main(String args[]) { Top my. Object](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-32.jpg)
public class Local. Example { public static void main(String args[]) { Top my. Object = new Top(); } } // OUTPUT Constructing a Top object Building a Wow h. t == 9 i == 8 Carnegie Mellon University, Graduate School of Industrial 32
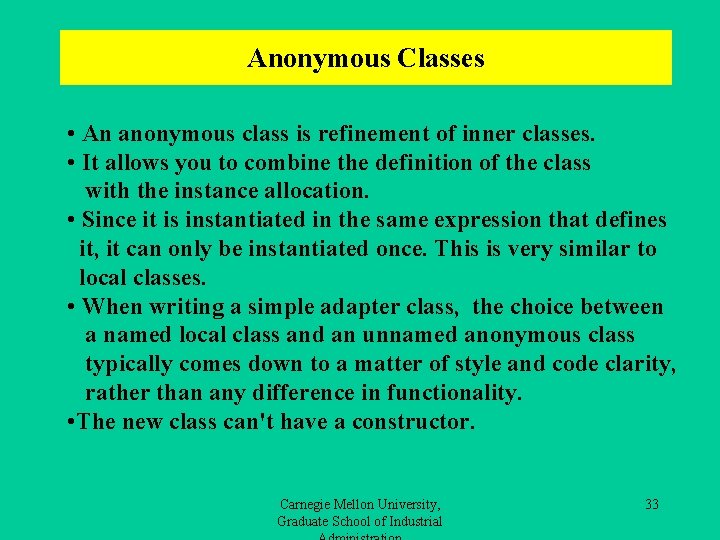
Anonymous Classes • An anonymous class is refinement of inner classes. • It allows you to combine the definition of the class with the instance allocation. • Since it is instantiated in the same expression that defines it, it can only be instantiated once. This is very similar to local classes. • When writing a simple adapter class, the choice between a named local class and an unnamed anonymous class typically comes down to a matter of style and code clarity, rather than any difference in functionality. • The new class can't have a constructor. Carnegie Mellon University, Graduate School of Industrial 33
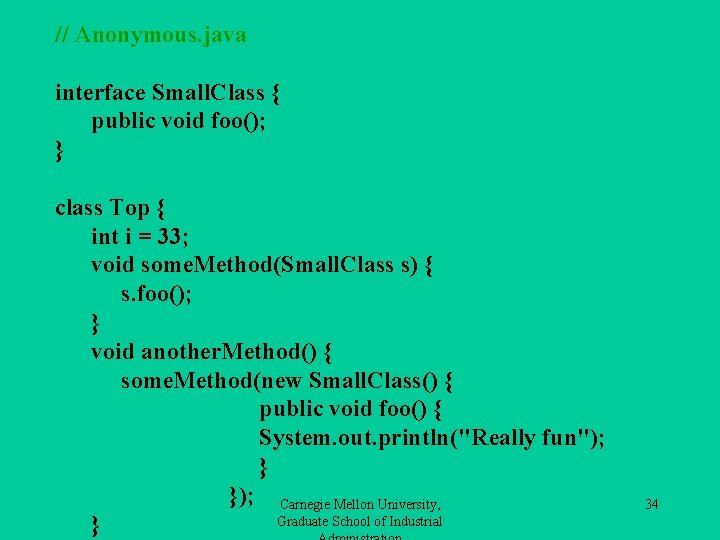
// Anonymous. java interface Small. Class { public void foo(); } class Top { int i = 33; void some. Method(Small. Class s) { s. foo(); } void another. Method() { some. Method(new Small. Class() { public void foo() { System. out. println("Really fun"); } }); Carnegie Mellon University, Graduate School of Industrial } 34
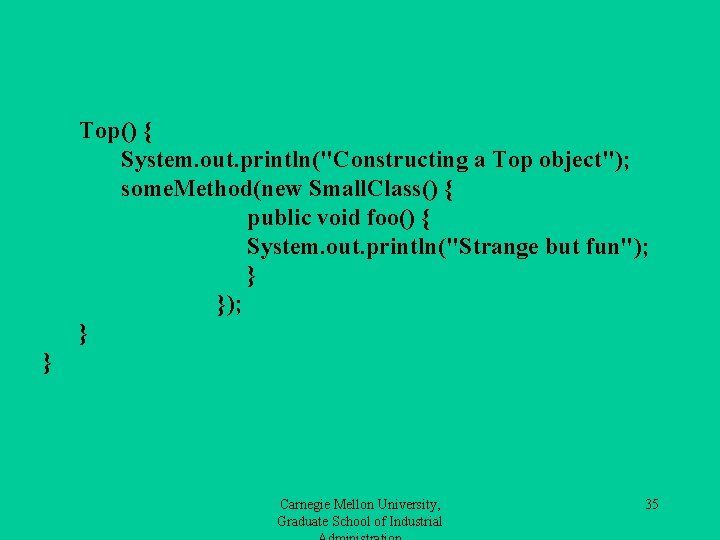
Top() { System. out. println("Constructing a Top object"); some. Method(new Small. Class() { public void foo() { System. out. println("Strange but fun"); } } Carnegie Mellon University, Graduate School of Industrial 35
![public class Anonymous public static void mainString args We cant create public class Anonymous { public static void main(String args[]) { // We can't create](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-36.jpg)
public class Anonymous { public static void main(String args[]) { // We can't create interface objects // error: Small. Class s = new Small. Class(); Top my. Object = new Top(); my. Object. another. Method(); } } // OUTPUT Constructing a Top object Strange but fun Really fun Carnegie Mellon University, Graduate School of Industrial 36
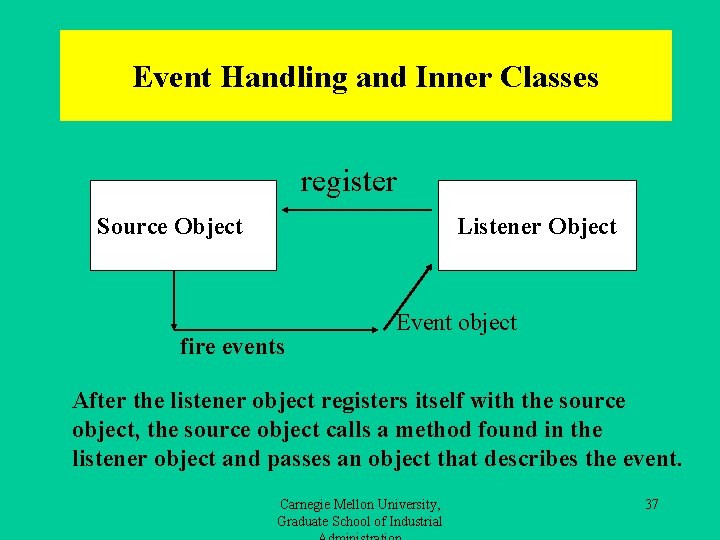
Event Handling and Inner Classes register Source Object Listener Object fire events Event object After the listener object registers itself with the source object, the source object calls a method found in the listener object and passes an object that describes the event. Carnegie Mellon University, Graduate School of Industrial 37
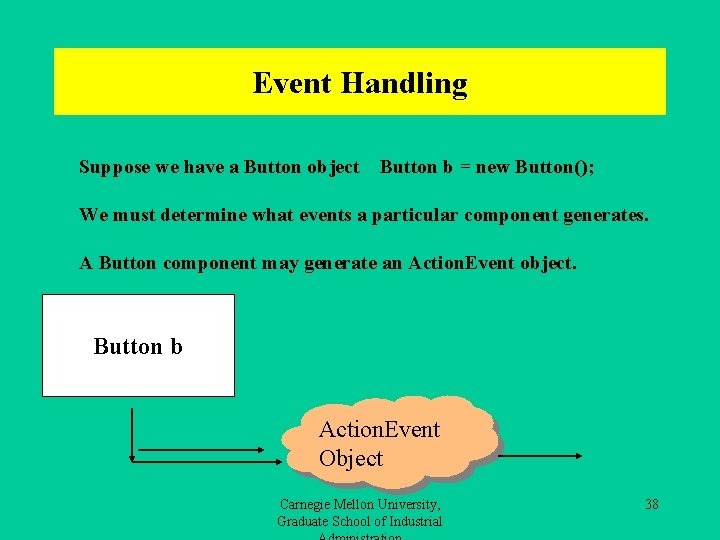
Event Handling Suppose we have a Button object Button b = new Button(); We must determine what events a particular component generates. A Button component may generate an Action. Event object. Button b Action. Event Object Carnegie Mellon University, Graduate School of Industrial 38
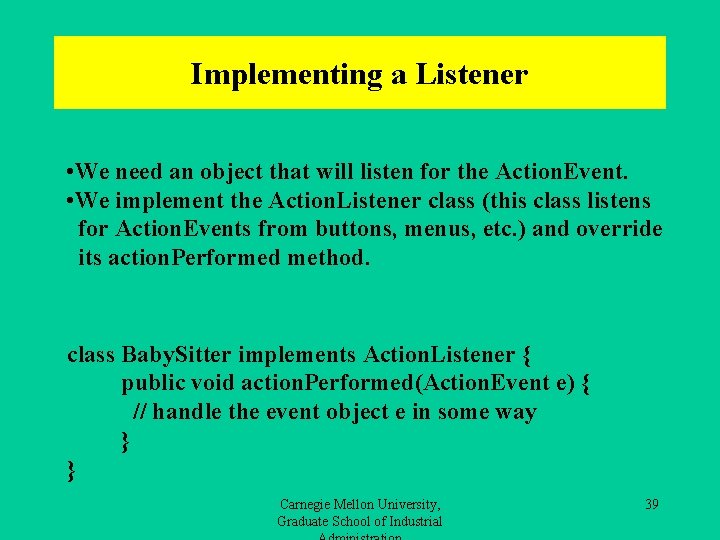
Implementing a Listener • We need an object that will listen for the Action. Event. • We implement the Action. Listener class (this class listens for Action. Events from buttons, menus, etc. ) and override its action. Performed method. class Baby. Sitter implements Action. Listener { public void action. Performed(Action. Event e) { // handle the event object e in some way } } Carnegie Mellon University, Graduate School of Industrial 39
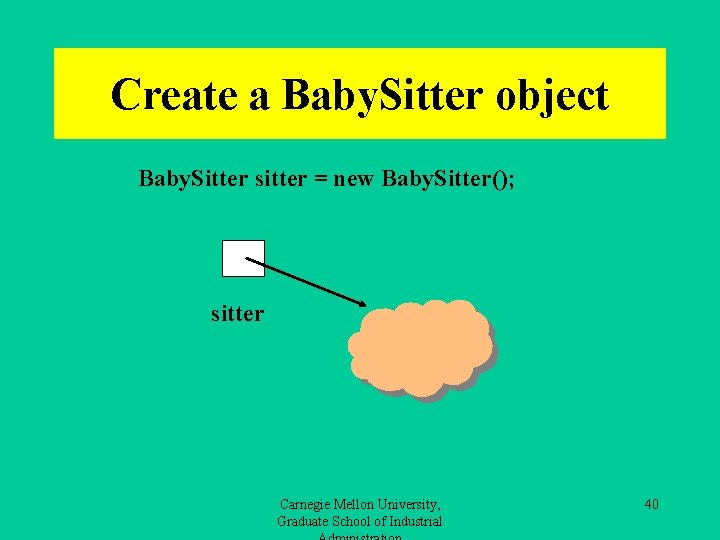
Create a Baby. Sitter object Baby. Sitter sitter = new Baby. Sitter(); sitter Carnegie Mellon University, Graduate School of Industrial 40
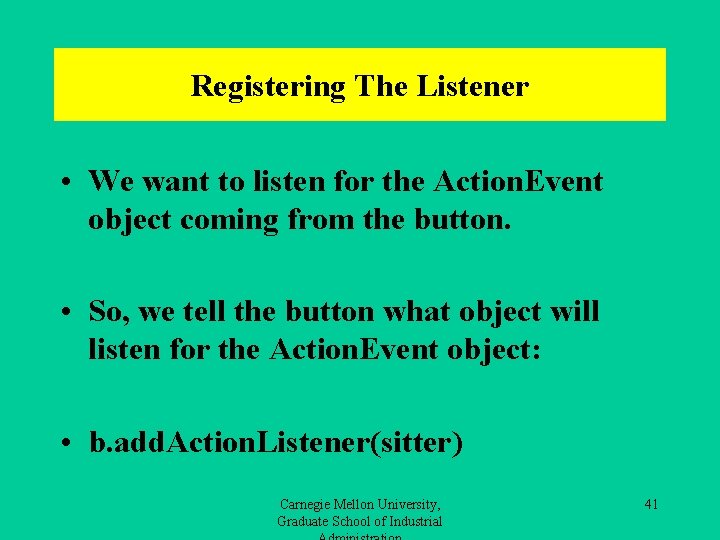
Registering The Listener • We want to listen for the Action. Event object coming from the button. • So, we tell the button what object will listen for the Action. Event object: • b. add. Action. Listener(sitter) Carnegie Mellon University, Graduate School of Industrial 41
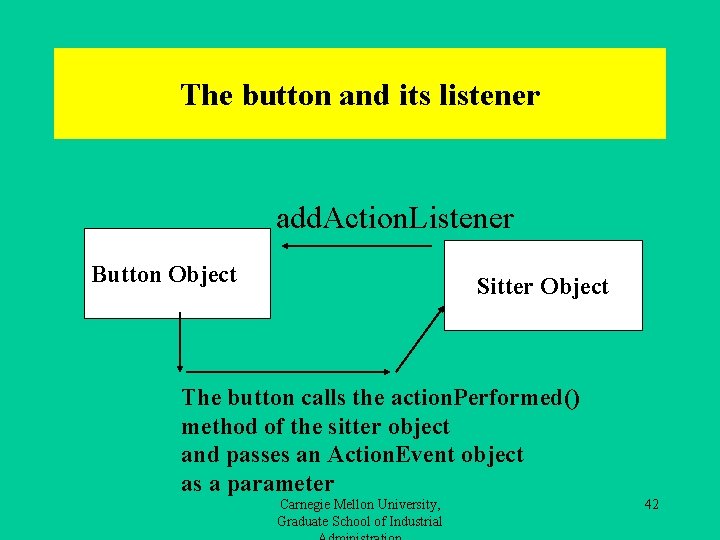
The button and its listener add. Action. Listener Button Object Sitter Object The button calls the action. Performed() method of the sitter object and passes an Action. Event object as a parameter Carnegie Mellon University, Graduate School of Industrial 42
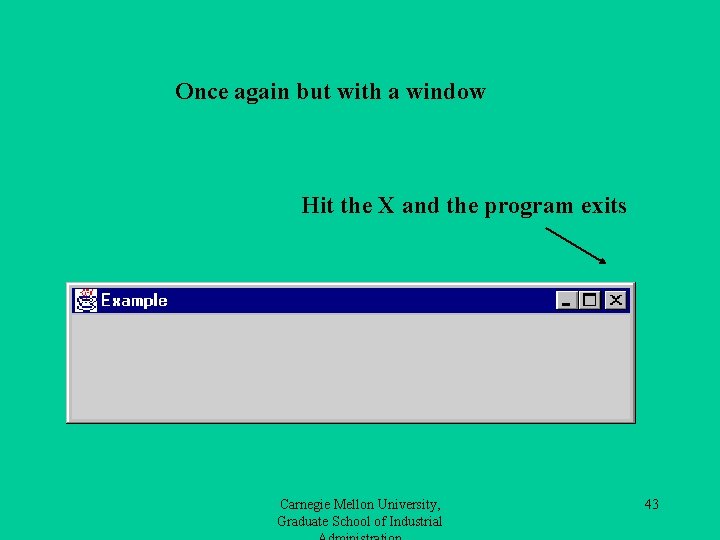
Once again but with a window Hit the X and the program exits Carnegie Mellon University, Graduate School of Industrial 43
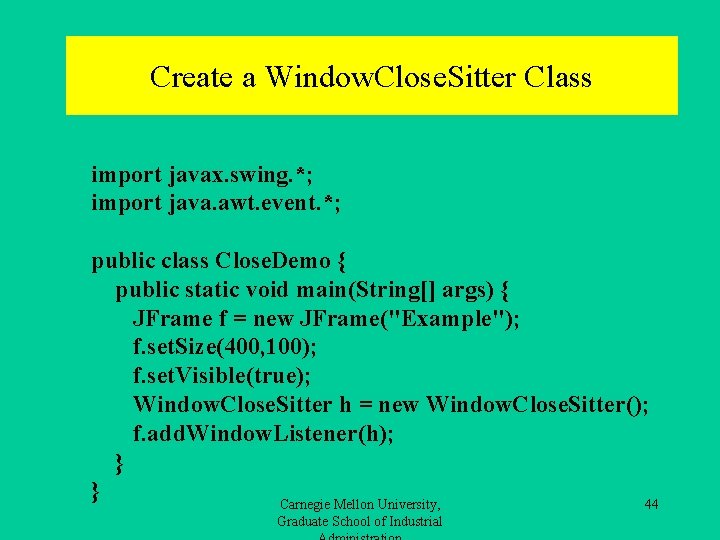
Create a Window. Close. Sitter Class import javax. swing. *; import java. awt. event. *; public class Close. Demo { public static void main(String[] args) { JFrame f = new JFrame("Example"); f. set. Size(400, 100); f. set. Visible(true); Window. Close. Sitter h = new Window. Close. Sitter(); f. add. Window. Listener(h); } } Carnegie Mellon University, 44 Graduate School of Industrial
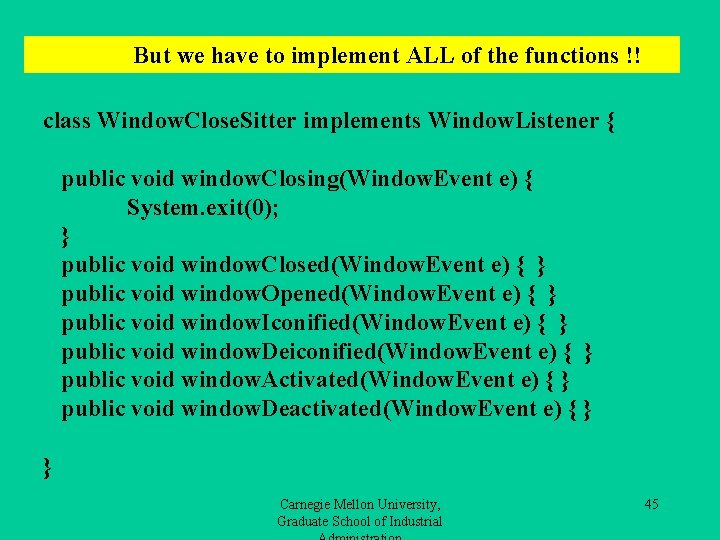
But we have to implement ALL of the functions !! class Window. Close. Sitter implements Window. Listener { public void window. Closing(Window. Event e) { System. exit(0); } public void window. Closed(Window. Event e) { } public void window. Opened(Window. Event e) { } public void window. Iconified(Window. Event e) { } public void window. Deiconified(Window. Event e) { } public void window. Activated(Window. Event e) { } public void window. Deactivated(Window. Event e) { } } Carnegie Mellon University, Graduate School of Industrial 45
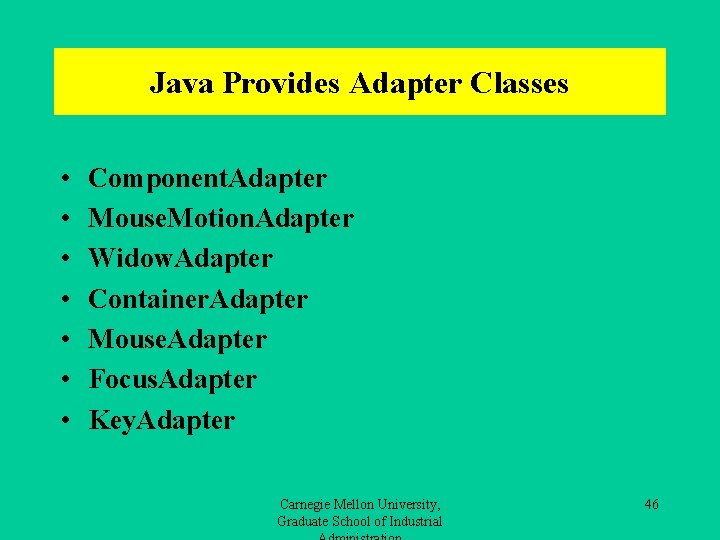
Java Provides Adapter Classes • • Component. Adapter Mouse. Motion. Adapter Widow. Adapter Container. Adapter Mouse. Adapter Focus. Adapter Key. Adapter Carnegie Mellon University, Graduate School of Industrial 46
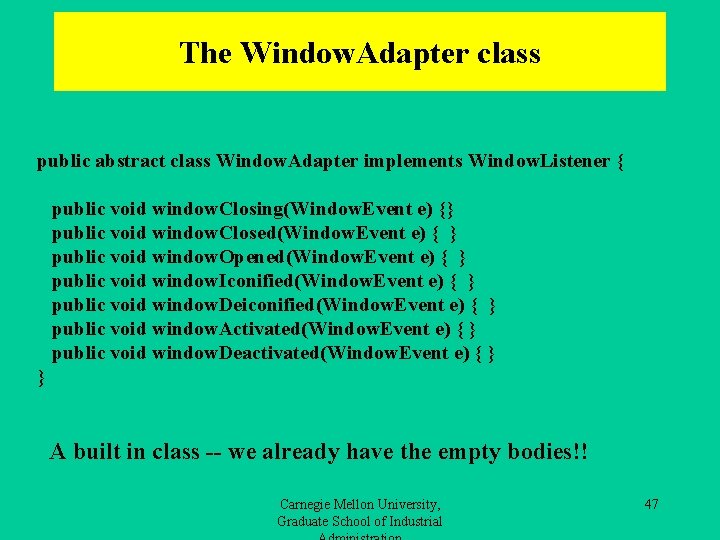
The Window. Adapter class public abstract class Window. Adapter implements Window. Listener { public void window. Closing(Window. Event e) {} public void window. Closed(Window. Event e) { } public void window. Opened(Window. Event e) { } public void window. Iconified(Window. Event e) { } public void window. Deiconified(Window. Event e) { } public void window. Activated(Window. Event e) { } public void window. Deactivated(Window. Event e) { } } A built in class -- we already have the empty bodies!! Carnegie Mellon University, Graduate School of Industrial 47
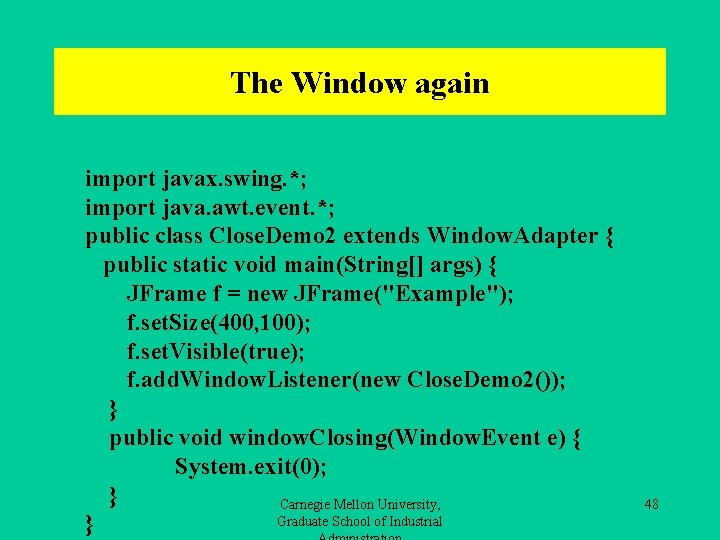
The Window again import javax. swing. *; import java. awt. event. *; public class Close. Demo 2 extends Window. Adapter { public static void main(String[] args) { JFrame f = new JFrame("Example"); f. set. Size(400, 100); f. set. Visible(true); f. add. Window. Listener(new Close. Demo 2()); } public void window. Closing(Window. Event e) { System. exit(0); } Carnegie Mellon University, Graduate School of Industrial } 48
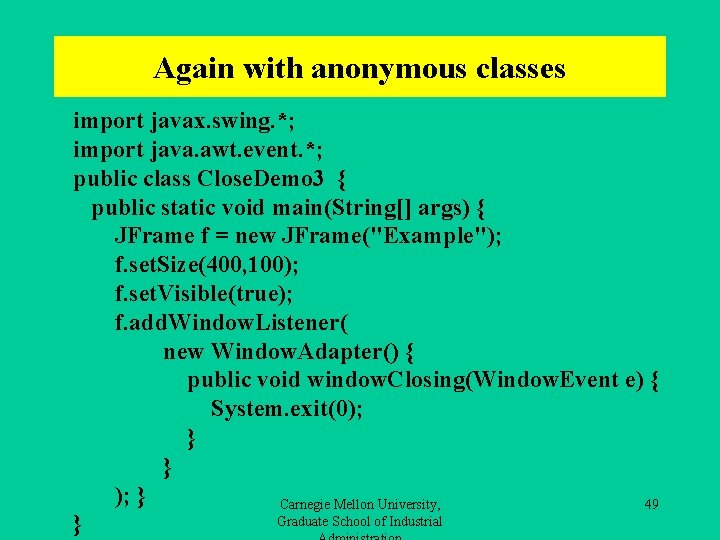
Again with anonymous classes import javax. swing. *; import java. awt. event. *; public class Close. Demo 3 { public static void main(String[] args) { JFrame f = new JFrame("Example"); f. set. Size(400, 100); f. set. Visible(true); f. add. Window. Listener( new Window. Adapter() { public void window. Closing(Window. Event e) { System. exit(0); } } ); } Carnegie Mellon University, 49 Graduate School of Industrial }
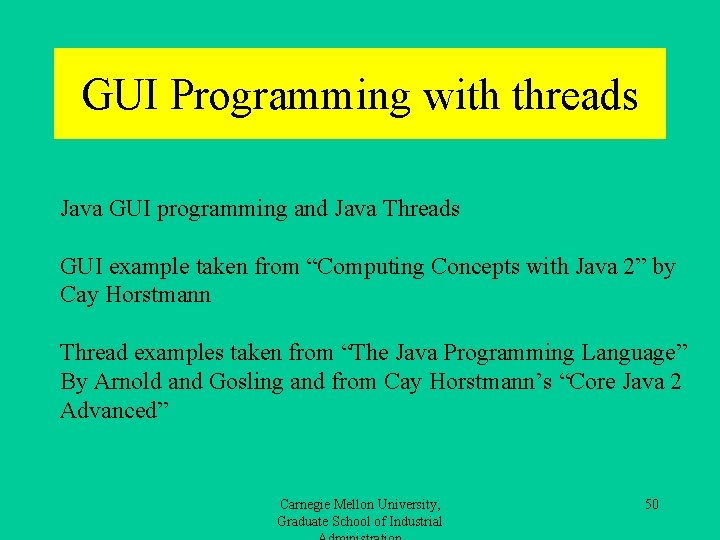
GUI Programming with threads Java GUI programming and Java Threads GUI example taken from “Computing Concepts with Java 2” by Cay Horstmann Thread examples taken from “The Java Programming Language” By Arnold and Gosling and from Cay Horstmann’s “Core Java 2 Advanced” Carnegie Mellon University, Graduate School of Industrial 50
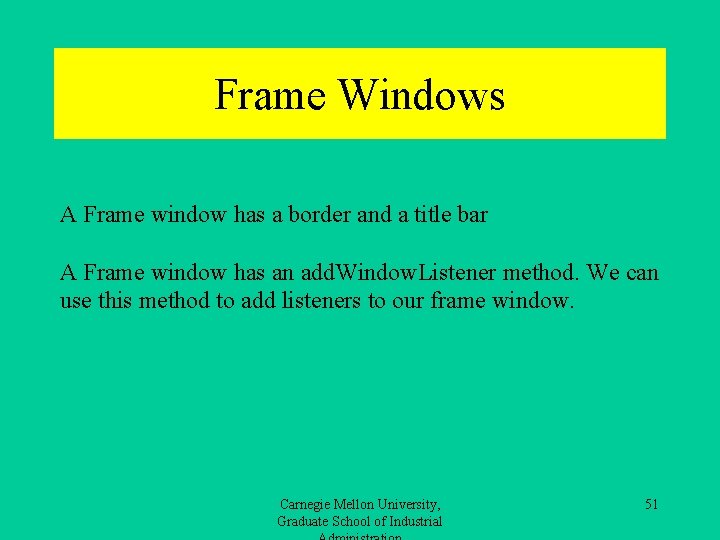
Frame Windows A Frame window has a border and a title bar A Frame window has an add. Window. Listener method. We can use this method to add listeners to our frame window. Carnegie Mellon University, Graduate School of Industrial 51
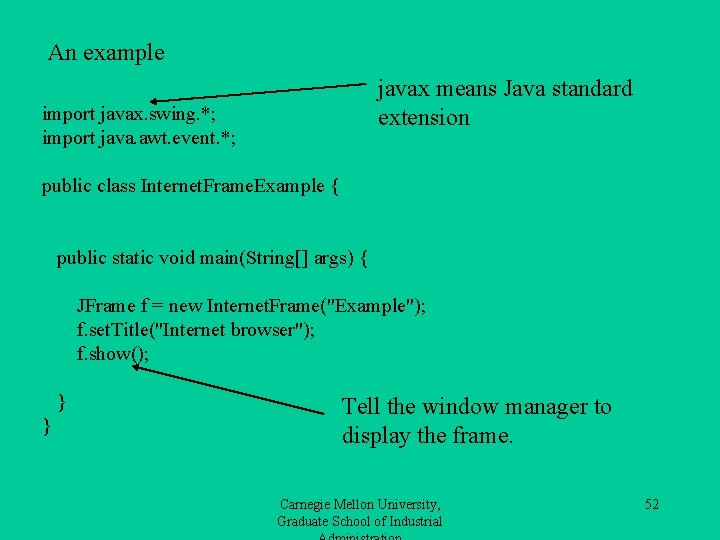
An example javax means Java standard extension import javax. swing. *; import java. awt. event. *; public class Internet. Frame. Example { public static void main(String[] args) { JFrame f = new Internet. Frame("Example"); f. set. Title("Internet browser"); f. show(); } } Tell the window manager to display the frame. Carnegie Mellon University, Graduate School of Industrial 52
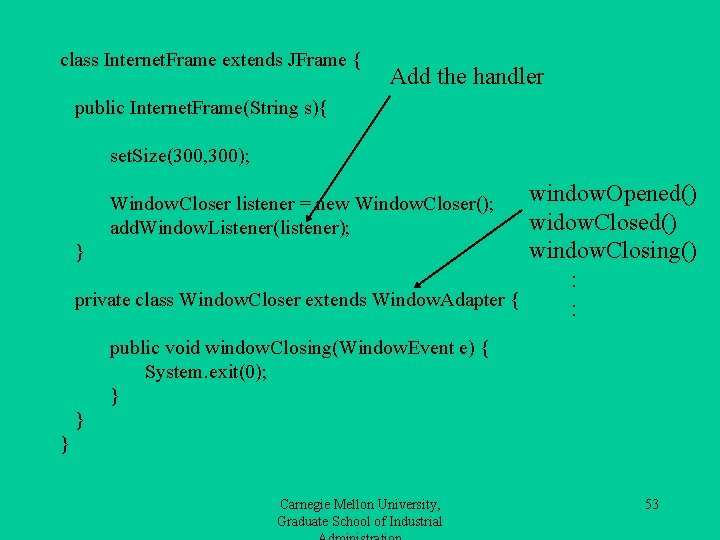
class Internet. Frame extends JFrame { Add the handler public Internet. Frame(String s){ set. Size(300, 300); window. Opened() widow. Closed() } window. Closing() : private class Window. Closer extends Window. Adapter { : Window. Closer listener = new Window. Closer(); add. Window. Listener(listener); public void window. Closing(Window. Event e) { System. exit(0); } } } Carnegie Mellon University, Graduate School of Industrial 53
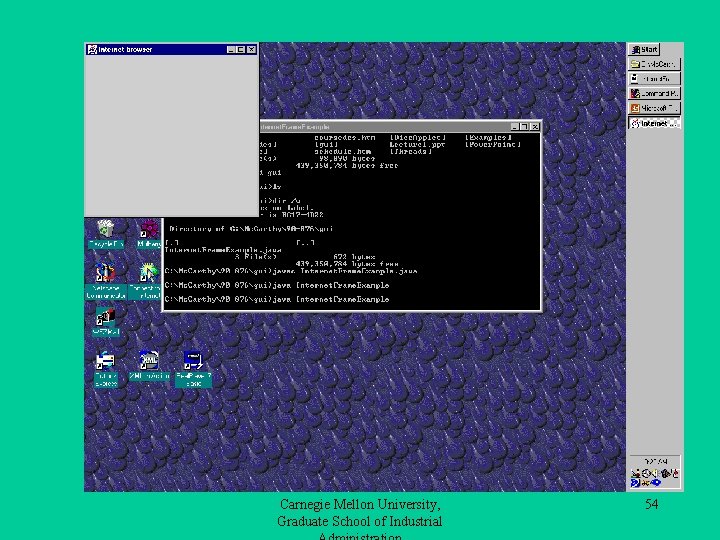
Carnegie Mellon University, Graduate School of Industrial 54
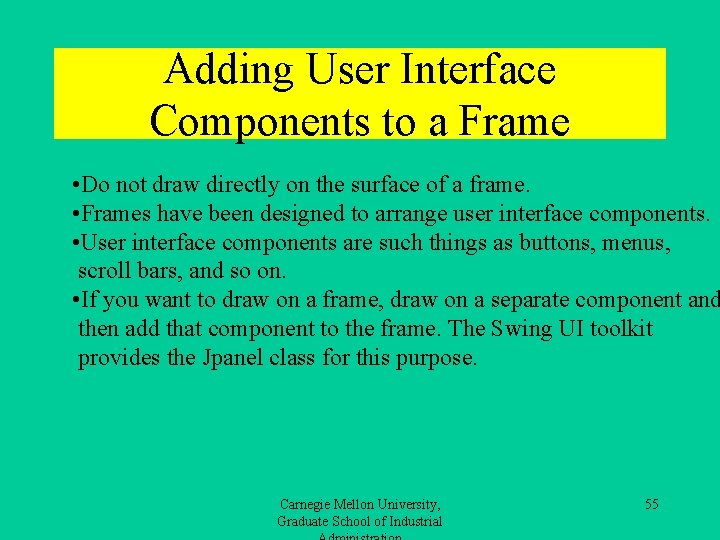
Adding User Interface Components to a Frame • Do not draw directly on the surface of a frame. • Frames have been designed to arrange user interface components. • User interface components are such things as buttons, menus, scroll bars, and so on. • If you want to draw on a frame, draw on a separate component and then add that component to the frame. The Swing UI toolkit provides the Jpanel class for this purpose. Carnegie Mellon University, Graduate School of Industrial 55
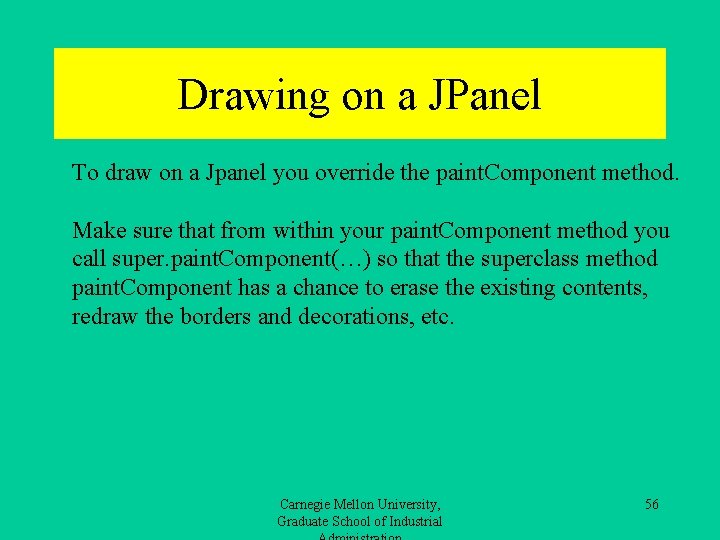
Drawing on a JPanel To draw on a Jpanel you override the paint. Component method. Make sure that from within your paint. Component method you call super. paint. Component(…) so that the superclass method paint. Component has a chance to erase the existing contents, redraw the borders and decorations, etc. Carnegie Mellon University, Graduate School of Industrial 56
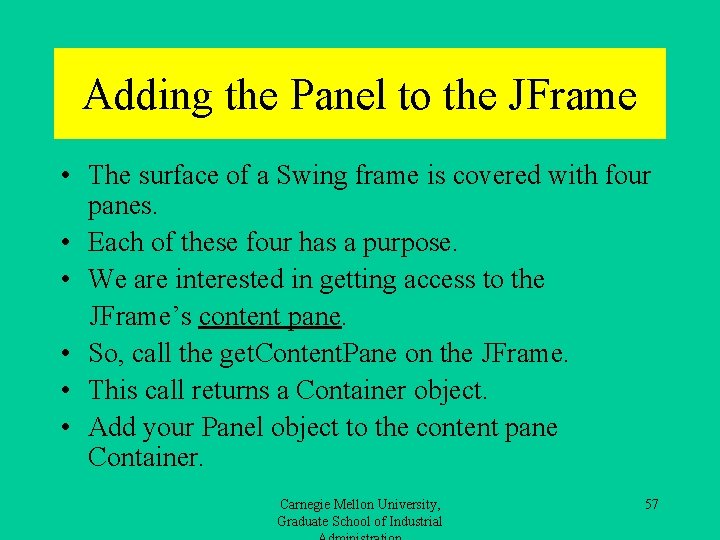
Adding the Panel to the JFrame • The surface of a Swing frame is covered with four panes. • Each of these four has a purpose. • We are interested in getting access to the JFrame’s content pane. • So, call the get. Content. Pane on the JFrame. • This call returns a Container object. • Add your Panel object to the content pane Container. Carnegie Mellon University, Graduate School of Industrial 57
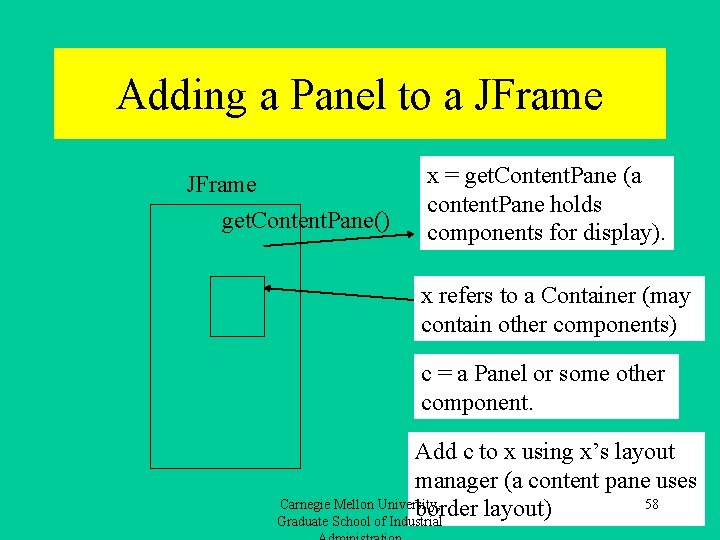
Adding a Panel to a JFrame get. Content. Pane() x = get. Content. Pane (a content. Pane holds components for display). x refers to a Container (may contain other components) c = a Panel or some other component. Add c to x using x’s layout manager (a content pane uses Carnegie Mellon University, 58 border layout) Graduate School of Industrial
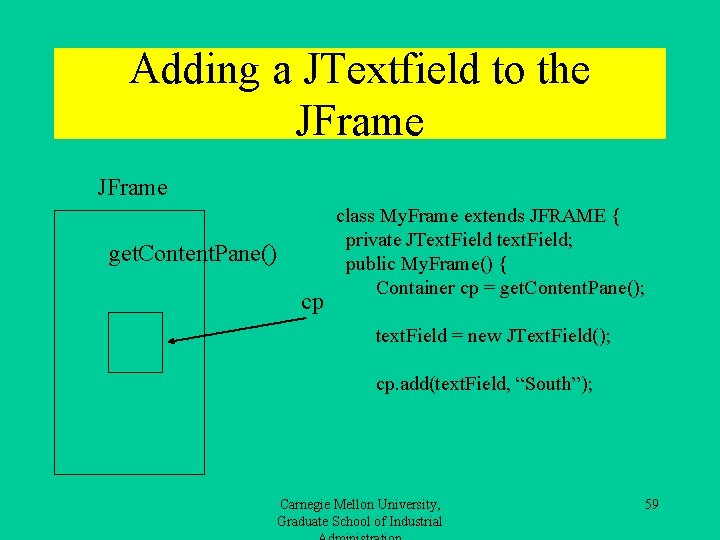
Adding a JTextfield to the JFrame get. Content. Pane() cp class My. Frame extends JFRAME { private JText. Field text. Field; public My. Frame() { Container cp = get. Content. Pane(); text. Field = new JText. Field(); cp. add(text. Field, “South”); Carnegie Mellon University, Graduate School of Industrial 59
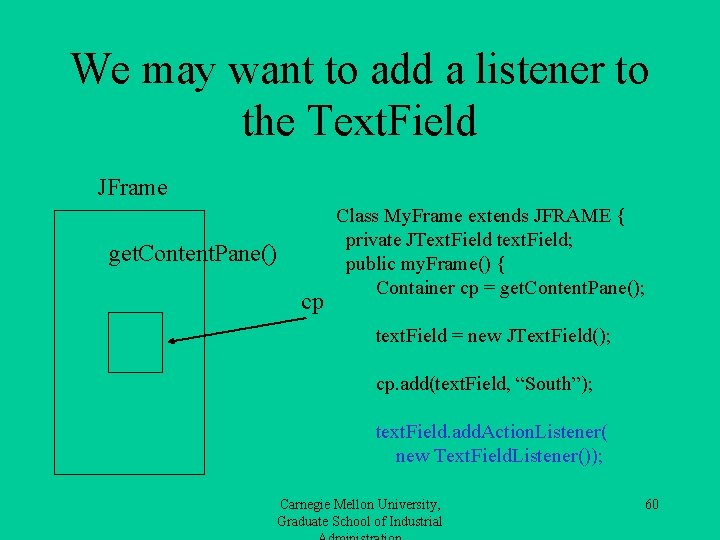
We may want to add a listener to the Text. Field JFrame get. Content. Pane() cp Class My. Frame extends JFRAME { private JText. Field text. Field; public my. Frame() { Container cp = get. Content. Pane(); text. Field = new JText. Field(); cp. add(text. Field, “South”); text. Field. add. Action. Listener( new Text. Field. Listener()); Carnegie Mellon University, Graduate School of Industrial 60
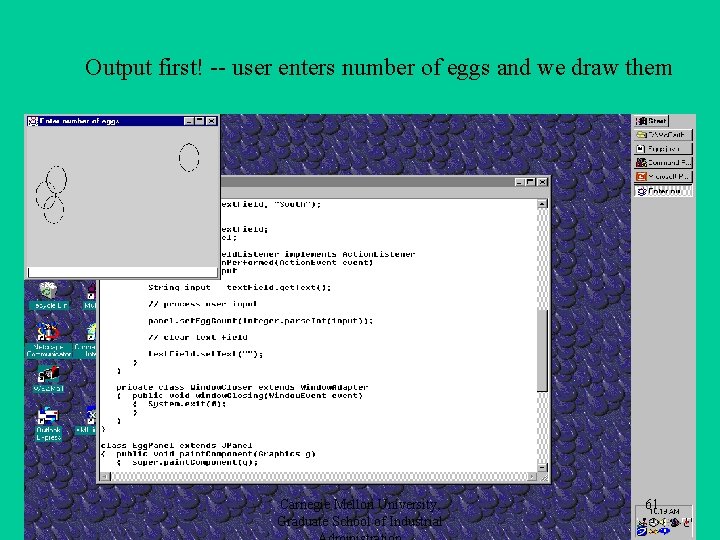
Output first! -- user enters number of eggs and we draw them Carnegie Mellon University, Graduate School of Industrial 61
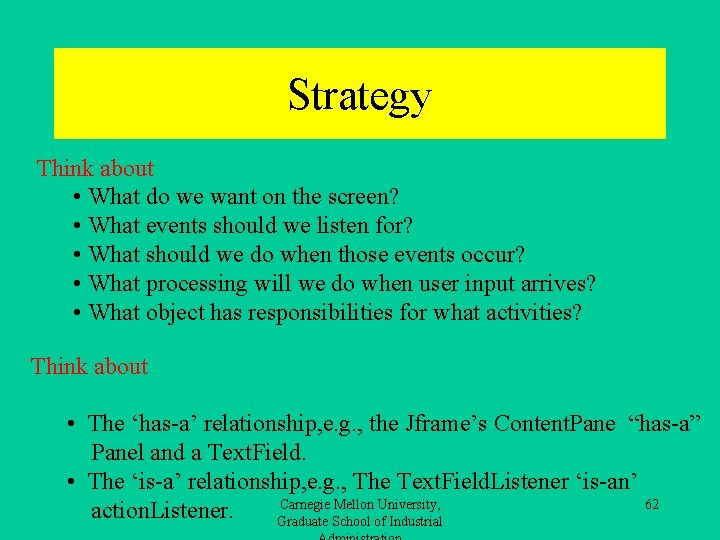
Strategy Think about • What do we want on the screen? • What events should we listen for? • What should we do when those events occur? • What processing will we do when user input arrives? • What object has responsibilities for what activities? Think about • The ‘has-a’ relationship, e. g. , the Jframe’s Content. Pane “has-a” Panel and a Text. Field. • The ‘is-a’ relationship, e. g. , The Text. Field. Listener ‘is-an’ Carnegie Mellon University, 62 action. Listener. Graduate School of Industrial
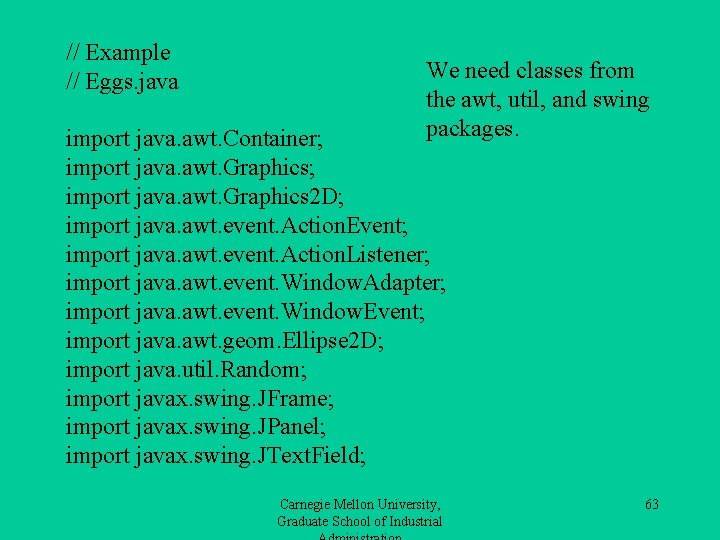
// Example // Eggs. java We need classes from the awt, util, and swing packages. import java. awt. Container; import java. awt. Graphics 2 D; import java. awt. event. Action. Event; import java. awt. event. Action. Listener; import java. awt. event. Window. Adapter; import java. awt. event. Window. Event; import java. awt. geom. Ellipse 2 D; import java. util. Random; import javax. swing. JFrame; import javax. swing. JPanel; import javax. swing. JText. Field; Carnegie Mellon University, Graduate School of Industrial 63
![public class Eggs public static void mainString args Egg Frame frame public class Eggs { public static void main(String[] args) { Egg. Frame frame =](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-64.jpg)
public class Eggs { public static void main(String[] args) { Egg. Frame frame = new Egg. Frame(); frame. set. Title("Enter number of eggs"); frame. show(); } } This thread is done after creating a frame and starting up the frame thread. A frame now exists and is running. Carnegie Mellon University, Graduate School of Industrial 64
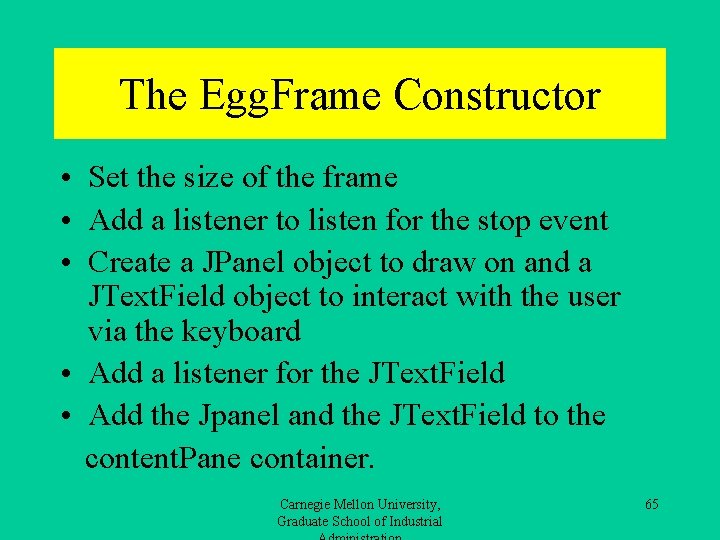
The Egg. Frame Constructor • Set the size of the frame • Add a listener to listen for the stop event • Create a JPanel object to draw on and a JText. Field object to interact with the user via the keyboard • Add a listener for the JText. Field • Add the Jpanel and the JText. Field to the content. Pane container. Carnegie Mellon University, Graduate School of Industrial 65
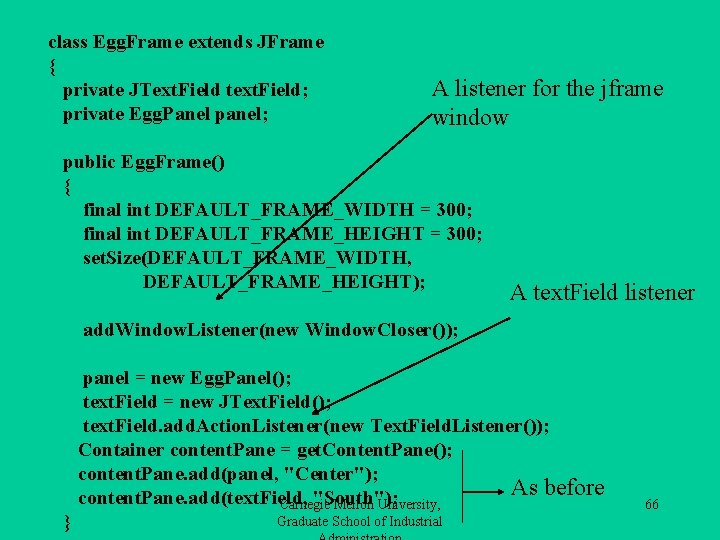
class Egg. Frame extends JFrame { private JText. Field text. Field; private Egg. Panel panel; A listener for the jframe window public Egg. Frame() { final int DEFAULT_FRAME_WIDTH = 300; final int DEFAULT_FRAME_HEIGHT = 300; set. Size(DEFAULT_FRAME_WIDTH, DEFAULT_FRAME_HEIGHT); A text. Field listener add. Window. Listener(new Window. Closer()); } panel = new Egg. Panel(); text. Field = new JText. Field(); text. Field. add. Action. Listener(new Text. Field. Listener()); Container content. Pane = get. Content. Pane(); content. Pane. add(panel, "Center"); As before content. Pane. add(text. Field, "South"); Carnegie Mellon University, Graduate School of Industrial 66
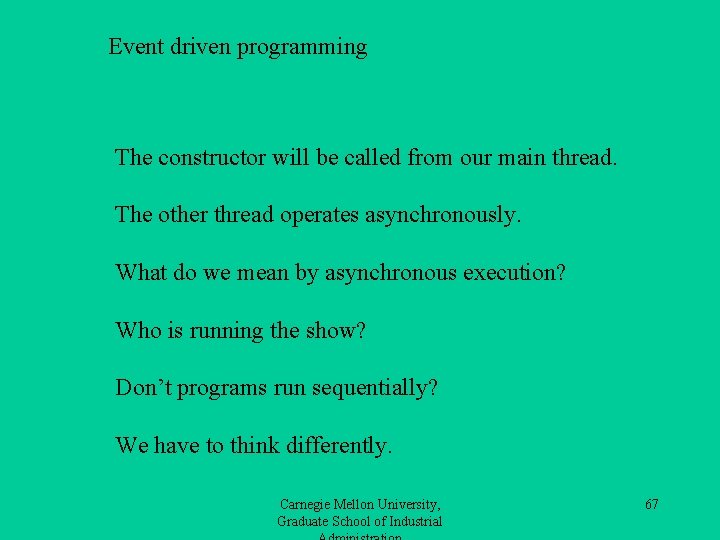
Event driven programming The constructor will be called from our main thread. The other thread operates asynchronously. What do we mean by asynchronous execution? Who is running the show? Don’t programs run sequentially? We have to think differently. Carnegie Mellon University, Graduate School of Industrial 67
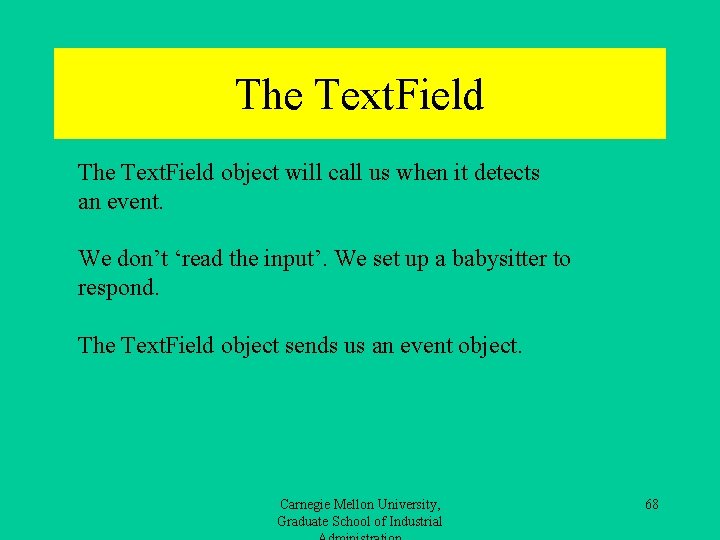
The Text. Field object will call us when it detects an event. We don’t ‘read the input’. We set up a babysitter to respond. The Text. Field object sends us an event object. Carnegie Mellon University, Graduate School of Industrial 68
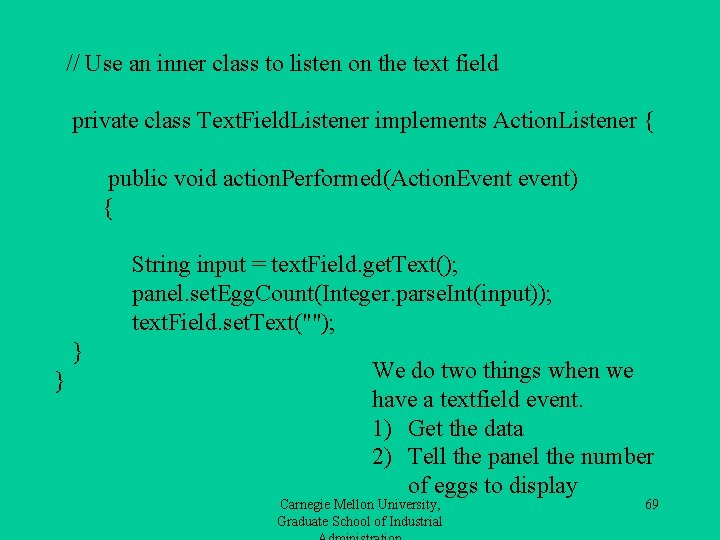
// Use an inner class to listen on the text field private class Text. Field. Listener implements Action. Listener { public void action. Performed(Action. Event event) { String input = text. Field. get. Text(); panel. set. Egg. Count(Integer. parse. Int(input)); text. Field. set. Text(""); } } We do two things when we have a textfield event. 1) Get the data 2) Tell the panel the number of eggs to display Carnegie Mellon University, Graduate School of Industrial 69
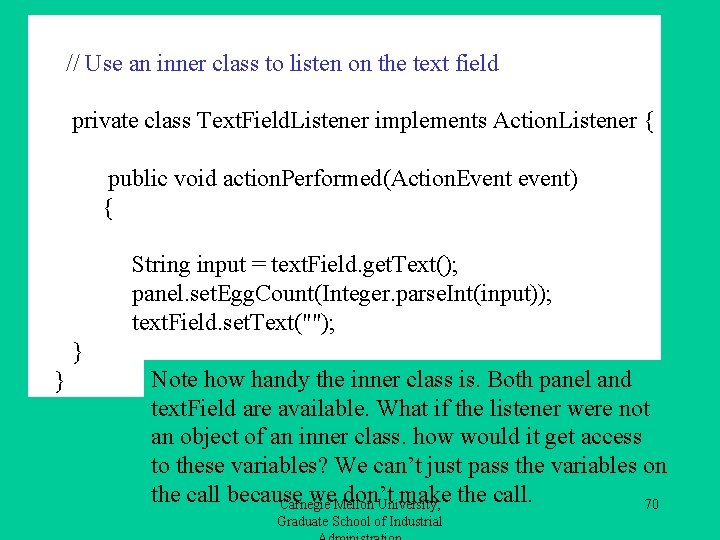
// Use an inner class to listen on the text field private class Text. Field. Listener implements Action. Listener { public void action. Performed(Action. Event event) { String input = text. Field. get. Text(); panel. set. Egg. Count(Integer. parse. Int(input)); text. Field. set. Text(""); } } Note how handy the inner class is. Both panel and text. Field are available. What if the listener were not an object of an inner class. how would it get access to these variables? We can’t just pass the variables on the call because we don’t make the call. Carnegie Mellon University, 70 Graduate School of Industrial
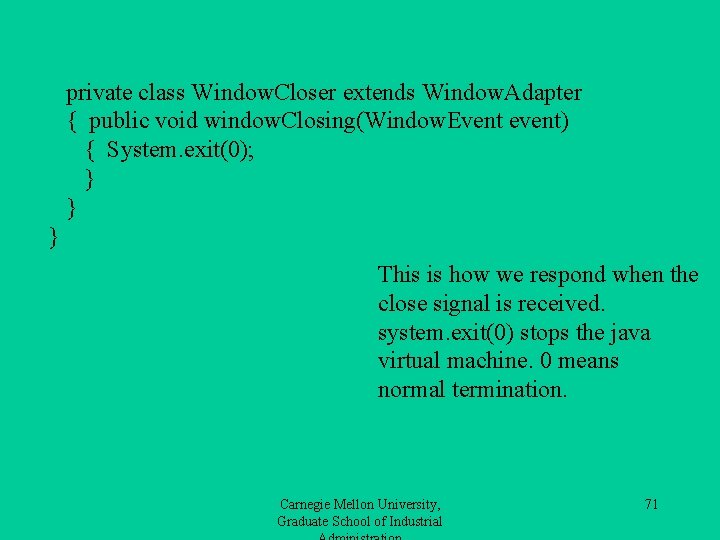
private class Window. Closer extends Window. Adapter { public void window. Closing(Window. Event event) { System. exit(0); } } } This is how we respond when the close signal is received. system. exit(0) stops the java virtual machine. 0 means normal termination. Carnegie Mellon University, Graduate School of Industrial 71
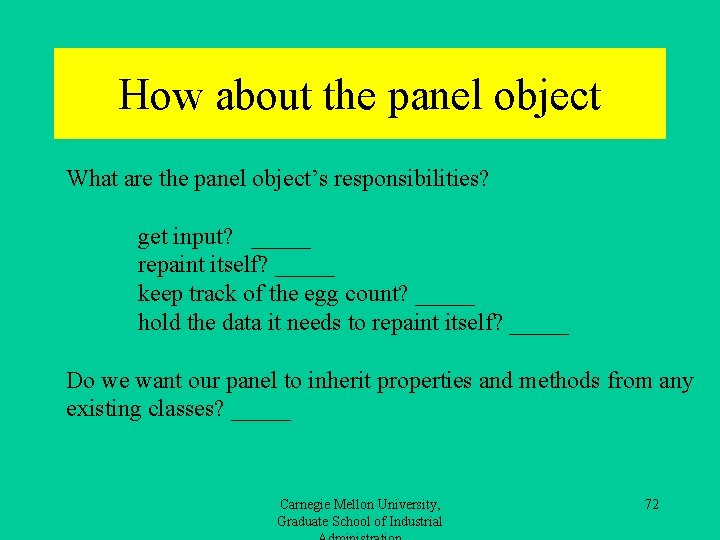
How about the panel object What are the panel object’s responsibilities? get input? _____ repaint itself? _____ keep track of the egg count? _____ hold the data it needs to repaint itself? _____ Do we want our panel to inherit properties and methods from any existing classes? _____ Carnegie Mellon University, Graduate School of Industrial 72
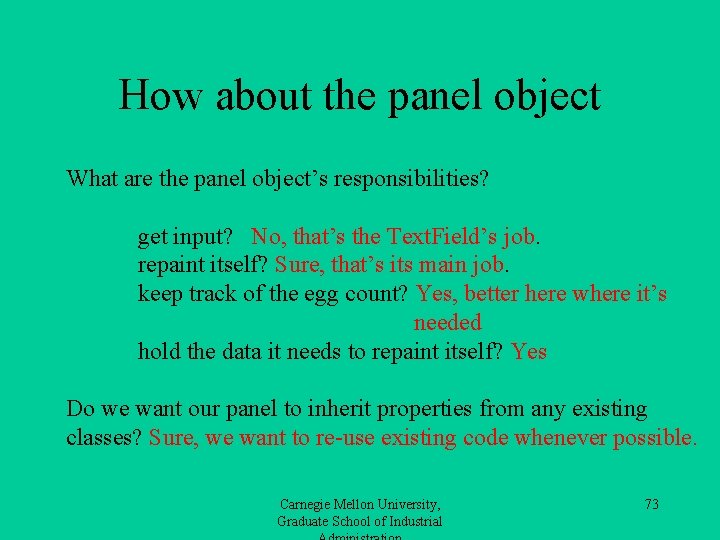
How about the panel object What are the panel object’s responsibilities? get input? No, that’s the Text. Field’s job. repaint itself? Sure, that’s its main job. keep track of the egg count? Yes, better here where it’s needed hold the data it needs to repaint itself? Yes Do we want our panel to inherit properties from any existing classes? Sure, we want to re-use existing code whenever possible. Carnegie Mellon University, Graduate School of Industrial 73
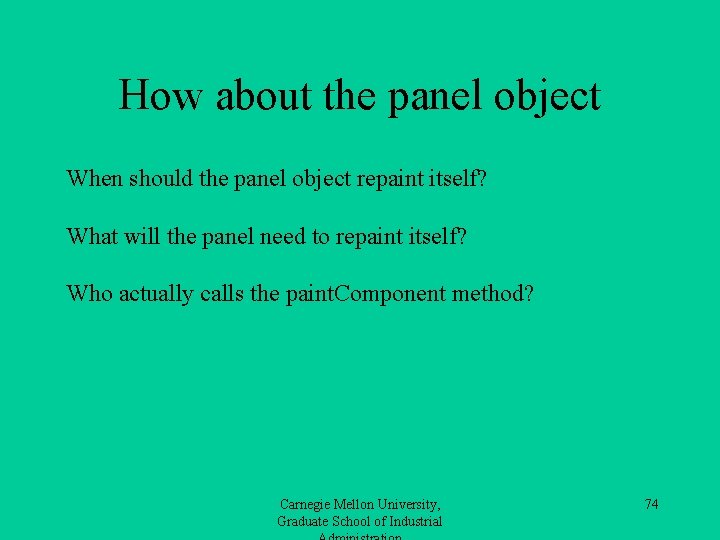
How about the panel object When should the panel object repaint itself? What will the panel need to repaint itself? Who actually calls the paint. Component method? Carnegie Mellon University, Graduate School of Industrial 74
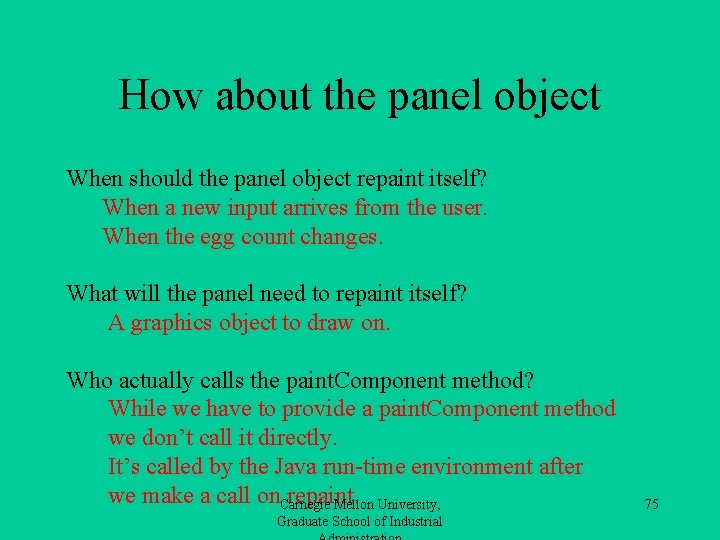
How about the panel object When should the panel object repaint itself? When a new input arrives from the user. When the egg count changes. What will the panel need to repaint itself? A graphics object to draw on. Who actually calls the paint. Component method? While we have to provide a paint. Component method we don’t call it directly. It’s called by the Java run-time environment after we make a call on. Carnegie repaint. Mellon University, Graduate School of Industrial 75
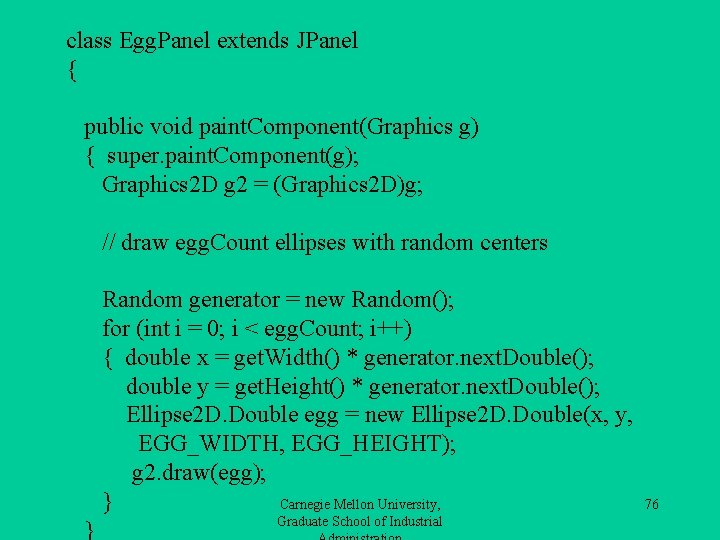
class Egg. Panel extends JPanel { public void paint. Component(Graphics g) { super. paint. Component(g); Graphics 2 D g 2 = (Graphics 2 D)g; // draw egg. Count ellipses with random centers Random generator = new Random(); for (int i = 0; i < egg. Count; i++) { double x = get. Width() * generator. next. Double(); double y = get. Height() * generator. next. Double(); Ellipse 2 D. Double egg = new Ellipse 2 D. Double(x, y, EGG_WIDTH, EGG_HEIGHT); g 2. draw(egg); } Carnegie Mellon University, } Graduate School of Industrial 76
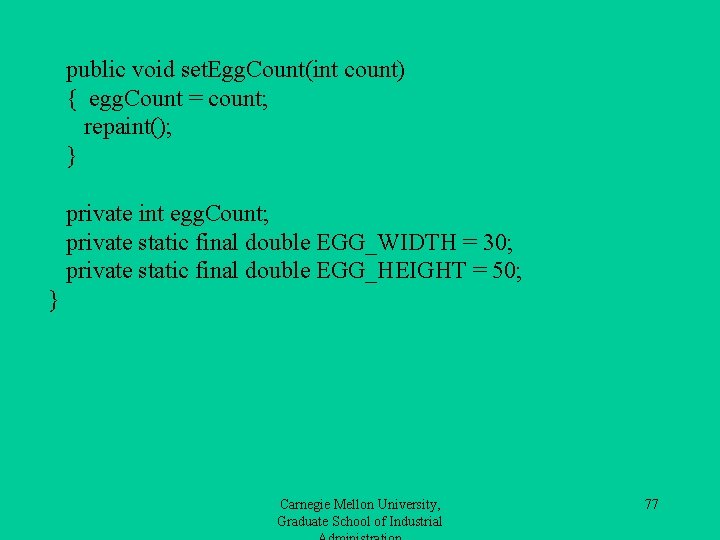
public void set. Egg. Count(int count) { egg. Count = count; repaint(); } private int egg. Count; private static final double EGG_WIDTH = 30; private static final double EGG_HEIGHT = 50; } Carnegie Mellon University, Graduate School of Industrial 77
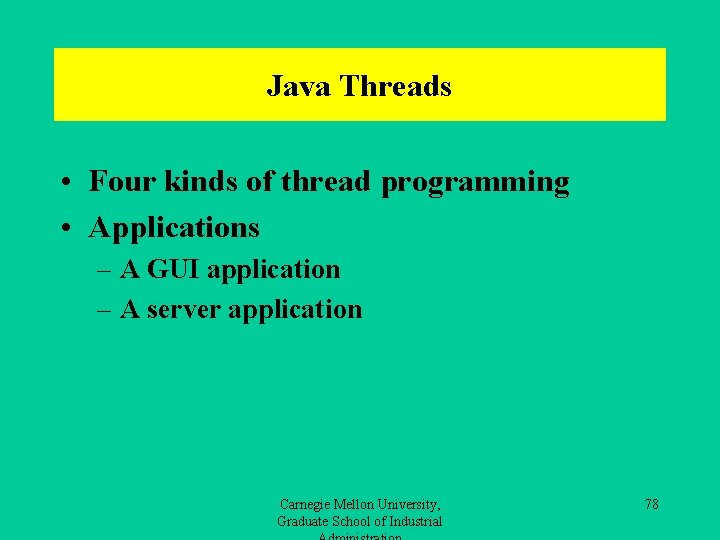
Java Threads • Four kinds of thread programming • Applications – A GUI application – A server application Carnegie Mellon University, Graduate School of Industrial 78
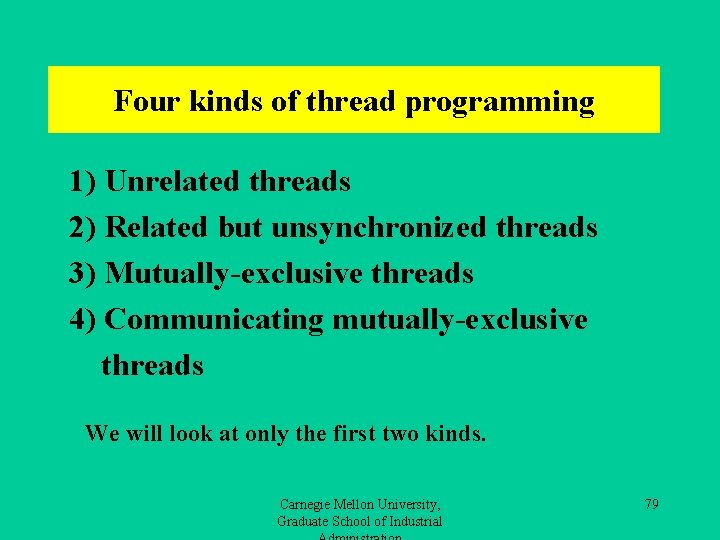
Four kinds of thread programming 1) Unrelated threads 2) Related but unsynchronized threads 3) Mutually-exclusive threads 4) Communicating mutually-exclusive threads We will look at only the first two kinds. Carnegie Mellon University, Graduate School of Industrial 79
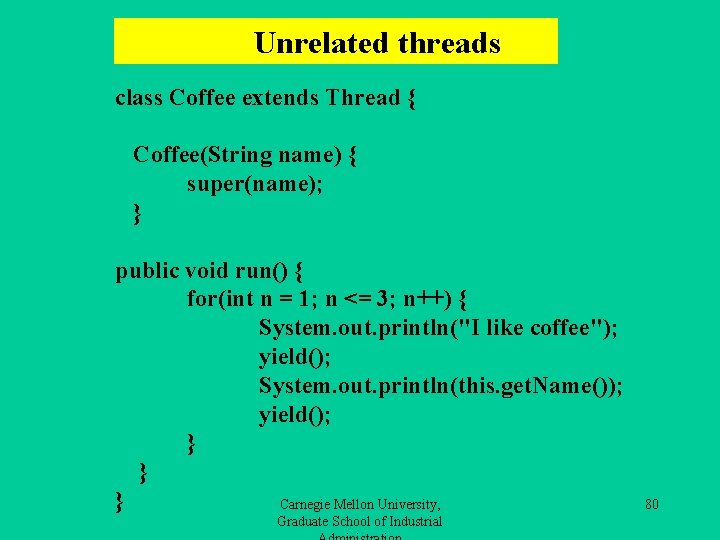
Unrelated threads class Coffee extends Thread { Coffee(String name) { super(name); } public void run() { for(int n = 1; n <= 3; n++) { System. out. println("I like coffee"); yield(); System. out. println(this. get. Name()); yield(); } } } Carnegie Mellon University, Graduate School of Industrial 80
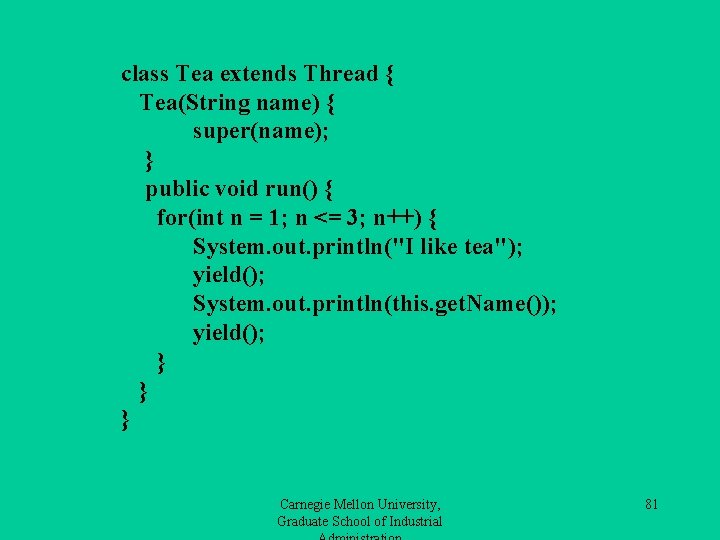
class Tea extends Thread { Tea(String name) { super(name); } public void run() { for(int n = 1; n <= 3; n++) { System. out. println("I like tea"); yield(); System. out. println(this. get. Name()); yield(); } } } Carnegie Mellon University, Graduate School of Industrial 81
![public class Drinks public static void mainString args System out printlnI am public class Drinks { public static void main(String args[]) { System. out. println("I am](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-82.jpg)
public class Drinks { public static void main(String args[]) { System. out. println("I am main"); Coffee t 1 = new Coffee("Wawa Coffee"); Tea t 2 = new Tea(“Sleepy Time Tea"); t 1. start(); t 2. start(); System. out. println("Main is done"); } } Carnegie Mellon University, Graduate School of Industrial 82
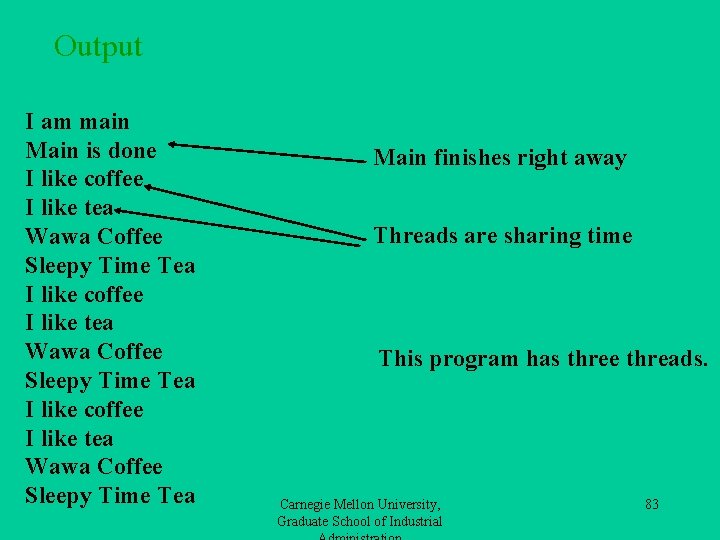
Output I am main Main is done I like coffee I like tea Wawa Coffee Sleepy Time Tea Main finishes right away Threads are sharing time This program has three threads. Carnegie Mellon University, Graduate School of Industrial 83
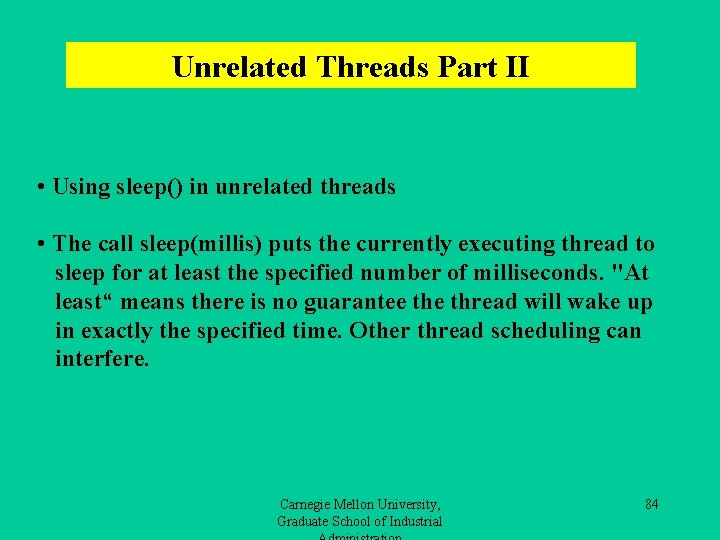
Unrelated Threads Part II • Using sleep() in unrelated threads • The call sleep(millis) puts the currently executing thread to sleep for at least the specified number of milliseconds. "At least“ means there is no guarantee thread will wake up in exactly the specified time. Other thread scheduling can interfere. Carnegie Mellon University, Graduate School of Industrial 84
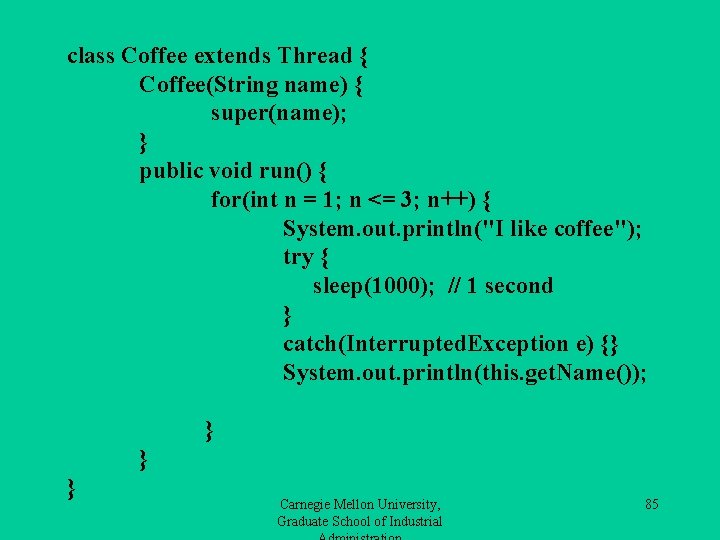
class Coffee extends Thread { Coffee(String name) { super(name); } public void run() { for(int n = 1; n <= 3; n++) { System. out. println("I like coffee"); try { sleep(1000); // 1 second } catch(Interrupted. Exception e) {} System. out. println(this. get. Name()); } } } Carnegie Mellon University, Graduate School of Industrial 85
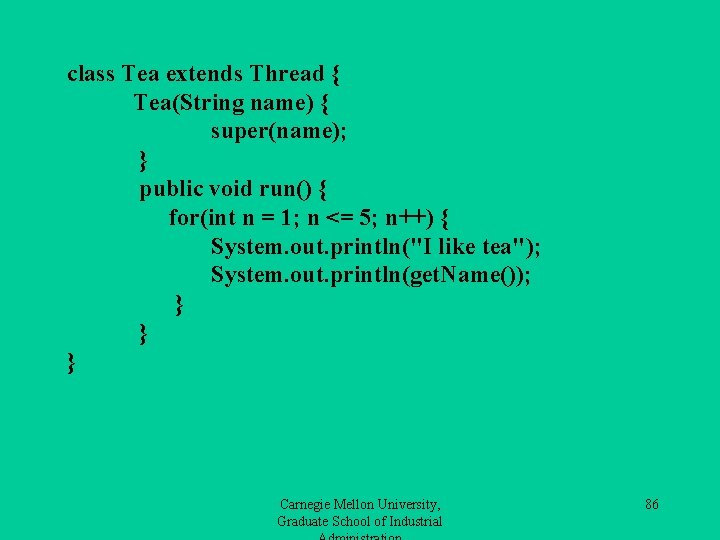
class Tea extends Thread { Tea(String name) { super(name); } public void run() { for(int n = 1; n <= 5; n++) { System. out. println("I like tea"); System. out. println(get. Name()); } } } Carnegie Mellon University, Graduate School of Industrial 86
![public class Drinks 2 public static void mainString args System out printlnI public class Drinks 2 { public static void main(String args[]) { System. out. println("I](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-87.jpg)
public class Drinks 2 { public static void main(String args[]) { System. out. println("I am main"); Coffee t 1 = new Coffee("Wawa Coffee"); Tea t 2 = new Tea("China Tea"); t 1. start(); t 2. start(); System. out. println("Main is done"); } } Carnegie Mellon University, Graduate School of Industrial 87
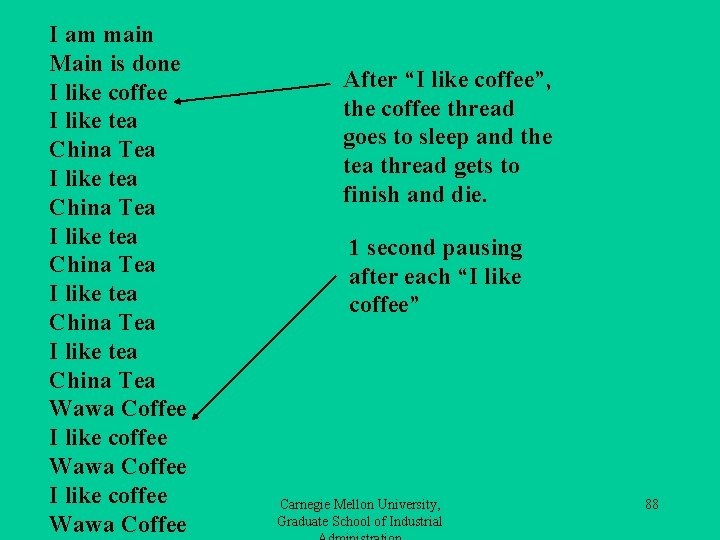
I am main Main is done I like coffee I like tea China Tea I like tea China Tea Wawa Coffee I like coffee Wawa Coffee After “I like coffee”, the coffee thread goes to sleep and the tea thread gets to finish and die. 1 second pausing after each “I like coffee” Carnegie Mellon University, Graduate School of Industrial 88
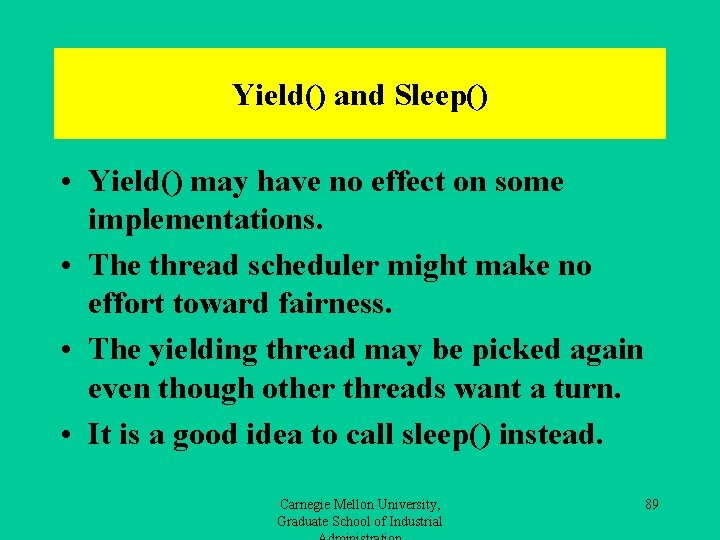
Yield() and Sleep() • Yield() may have no effect on some implementations. • The thread scheduler might make no effort toward fairness. • The yielding thread may be picked again even though other threads want a turn. • It is a good idea to call sleep() instead. Carnegie Mellon University, Graduate School of Industrial 89
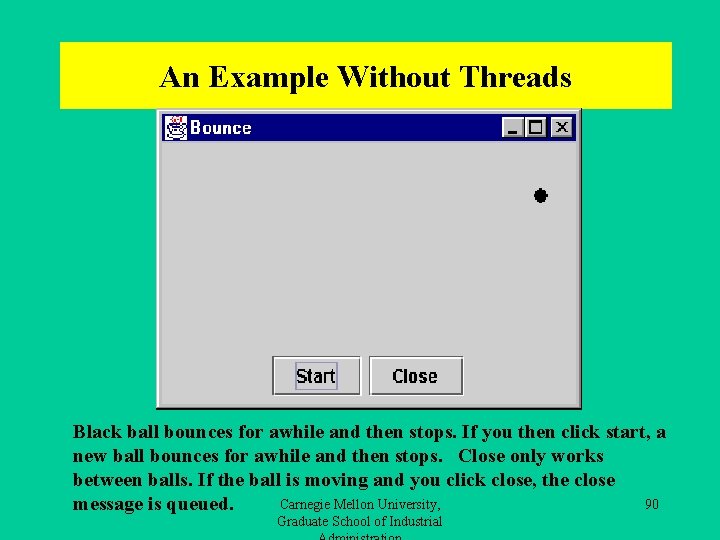
An Example Without Threads Black ball bounces for awhile and then stops. If you then click start, a new ball bounces for awhile and then stops. Close only works between balls. If the ball is moving and you click close, the close Carnegie Mellon University, 90 message is queued. Graduate School of Industrial
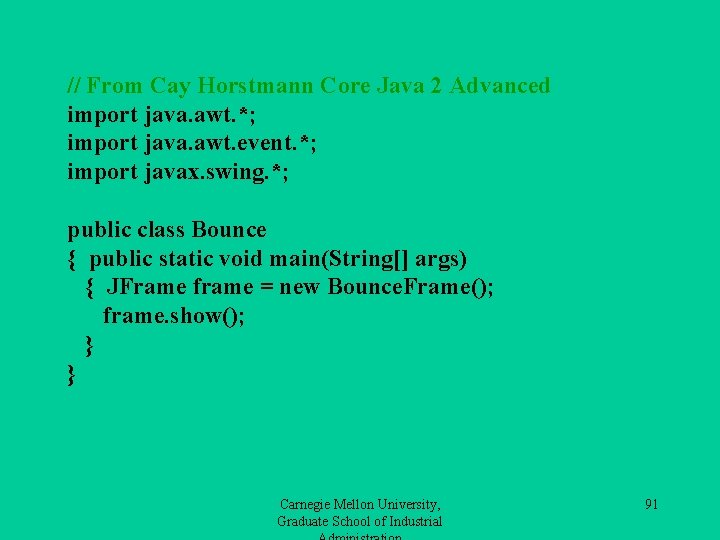
// From Cay Horstmann Core Java 2 Advanced import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Bounce { public static void main(String[] args) { JFrame frame = new Bounce. Frame(); frame. show(); } } Carnegie Mellon University, Graduate School of Industrial 91
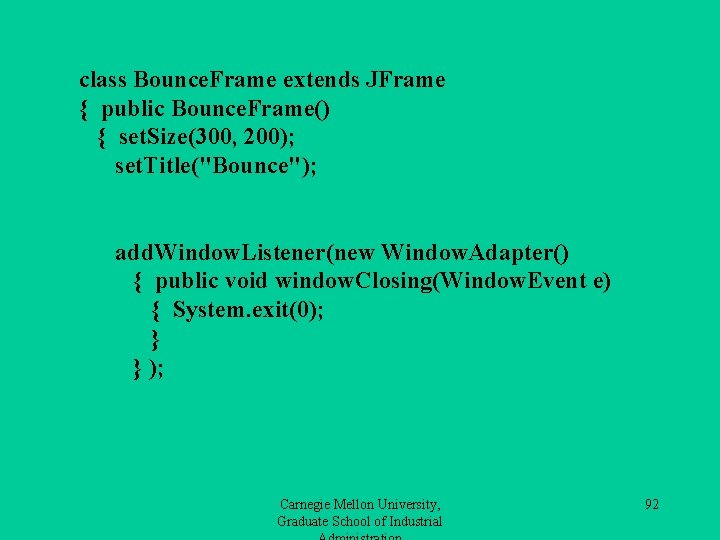
class Bounce. Frame extends JFrame { public Bounce. Frame() { set. Size(300, 200); set. Title("Bounce"); add. Window. Listener(new Window. Adapter() { public void window. Closing(Window. Event e) { System. exit(0); } } ); Carnegie Mellon University, Graduate School of Industrial 92
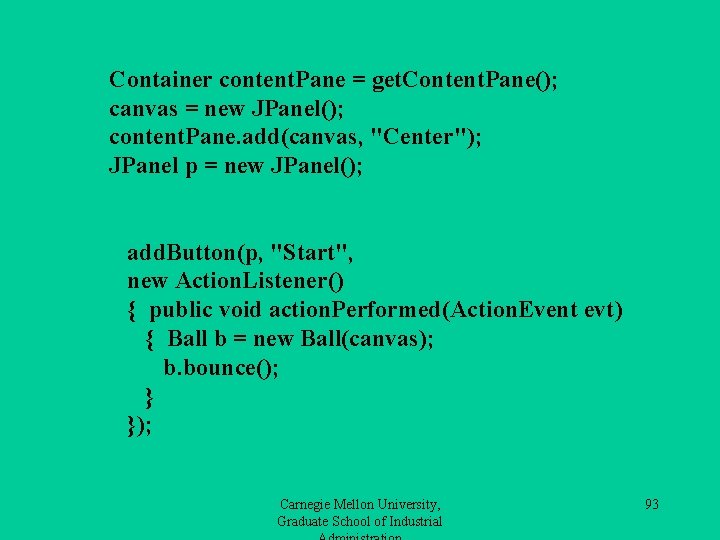
Container content. Pane = get. Content. Pane(); canvas = new JPanel(); content. Pane. add(canvas, "Center"); JPanel p = new JPanel(); add. Button(p, "Start", new Action. Listener() { public void action. Performed(Action. Event evt) { Ball b = new Ball(canvas); b. bounce(); } }); Carnegie Mellon University, Graduate School of Industrial 93
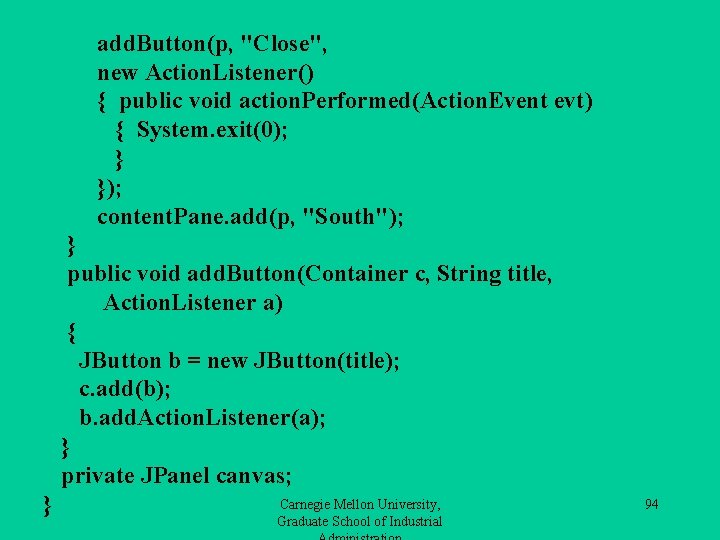
add. Button(p, "Close", new Action. Listener() { public void action. Performed(Action. Event evt) { System. exit(0); } }); content. Pane. add(p, "South"); } public void add. Button(Container c, String title, Action. Listener a) { JButton b = new JButton(title); c. add(b); b. add. Action. Listener(a); } private JPanel canvas; } Carnegie Mellon University, Graduate School of Industrial 94
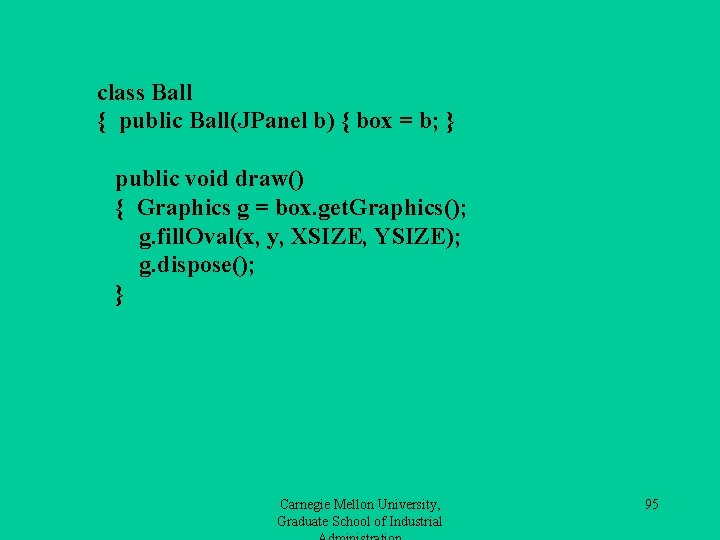
class Ball { public Ball(JPanel b) { box = b; } public void draw() { Graphics g = box. get. Graphics(); g. fill. Oval(x, y, XSIZE, YSIZE); g. dispose(); } Carnegie Mellon University, Graduate School of Industrial 95
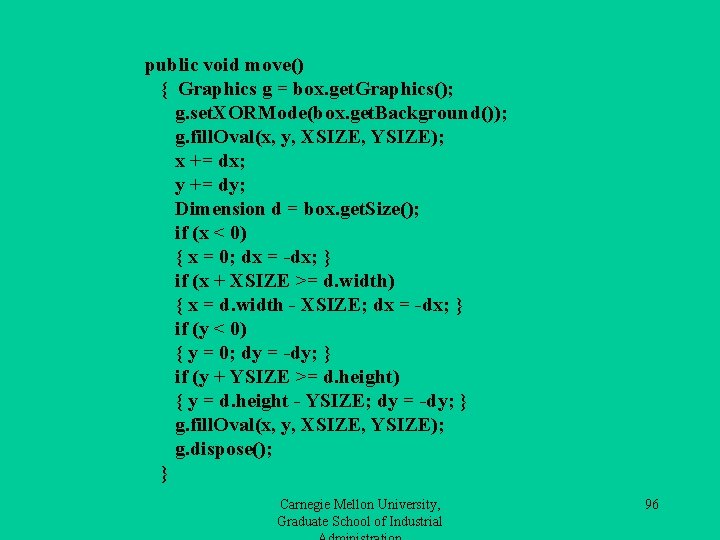
public void move() { Graphics g = box. get. Graphics(); g. set. XORMode(box. get. Background()); g. fill. Oval(x, y, XSIZE, YSIZE); x += dx; y += dy; Dimension d = box. get. Size(); if (x < 0) { x = 0; dx = -dx; } if (x + XSIZE >= d. width) { x = d. width - XSIZE; dx = -dx; } if (y < 0) { y = 0; dy = -dy; } if (y + YSIZE >= d. height) { y = d. height - YSIZE; dy = -dy; } g. fill. Oval(x, y, XSIZE, YSIZE); g. dispose(); } Carnegie Mellon University, Graduate School of Industrial 96
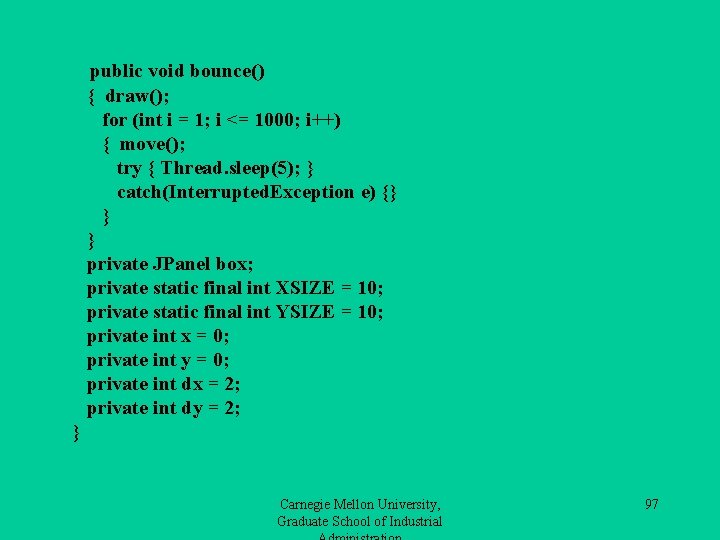
public void bounce() { draw(); for (int i = 1; i <= 1000; i++) { move(); try { Thread. sleep(5); } catch(Interrupted. Exception e) {} } } private JPanel box; private static final int XSIZE = 10; private static final int YSIZE = 10; private int x = 0; private int y = 0; private int dx = 2; private int dy = 2; } Carnegie Mellon University, Graduate School of Industrial 97
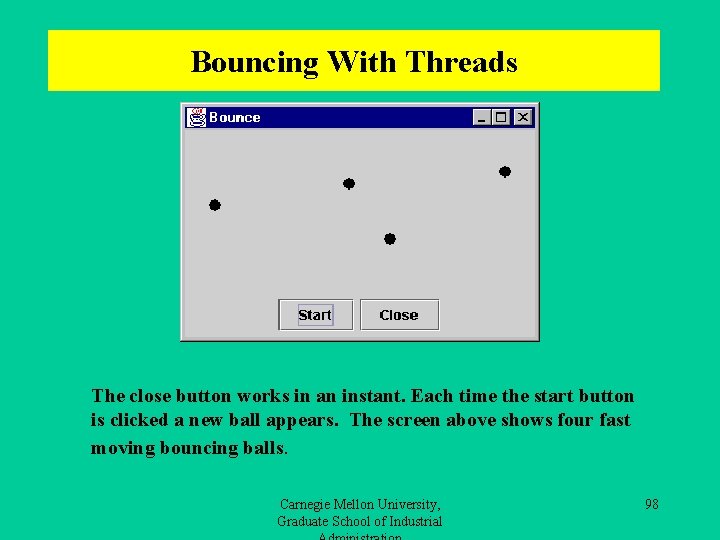
Bouncing With Threads The close button works in an instant. Each time the start button is clicked a new ball appears. The screen above shows four fast moving bouncing balls. Carnegie Mellon University, Graduate School of Industrial 98
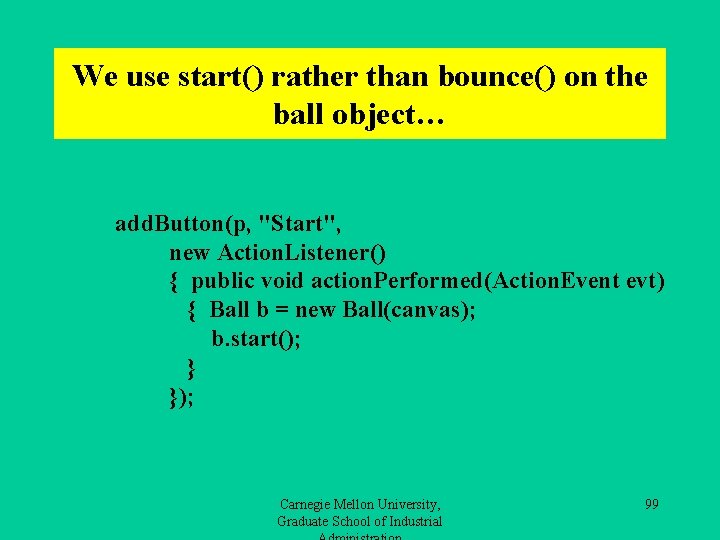
We use start() rather than bounce() on the ball object… add. Button(p, "Start", new Action. Listener() { public void action. Performed(Action. Event evt) { Ball b = new Ball(canvas); b. start(); } }); Carnegie Mellon University, Graduate School of Industrial 99
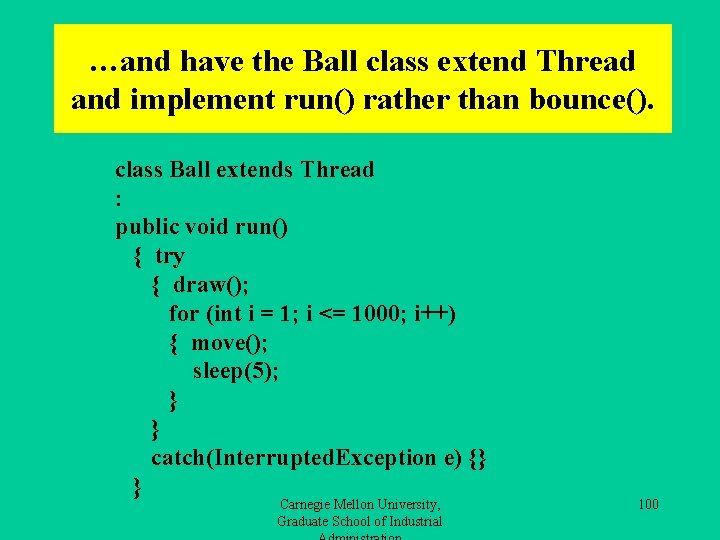
…and have the Ball class extend Thread and implement run() rather than bounce(). class Ball extends Thread : public void run() { try { draw(); for (int i = 1; i <= 1000; i++) { move(); sleep(5); } } catch(Interrupted. Exception e) {} } Carnegie Mellon University, Graduate School of Industrial 100
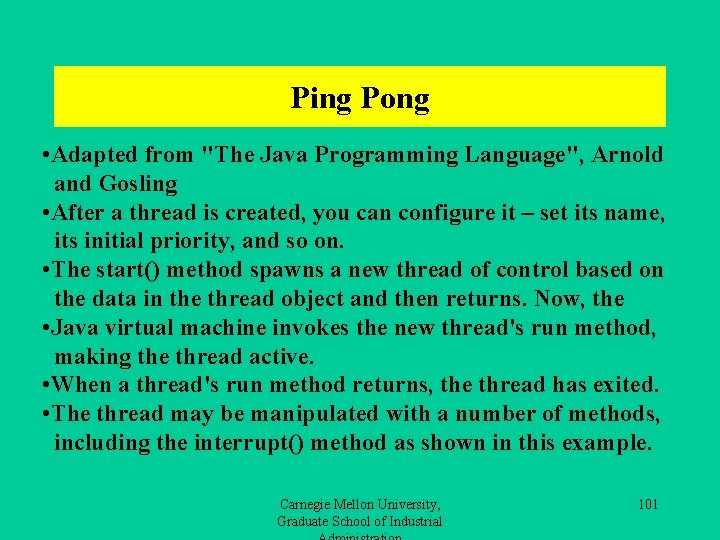
Ping Pong • Adapted from "The Java Programming Language", Arnold and Gosling • After a thread is created, you can configure it – set its name, its initial priority, and so on. • The start() method spawns a new thread of control based on the data in the thread object and then returns. Now, the • Java virtual machine invokes the new thread's run method, making the thread active. • When a thread's run method returns, the thread has exited. • The thread may be manipulated with a number of methods, including the interrupt() method as shown in this example. Carnegie Mellon University, Graduate School of Industrial 101
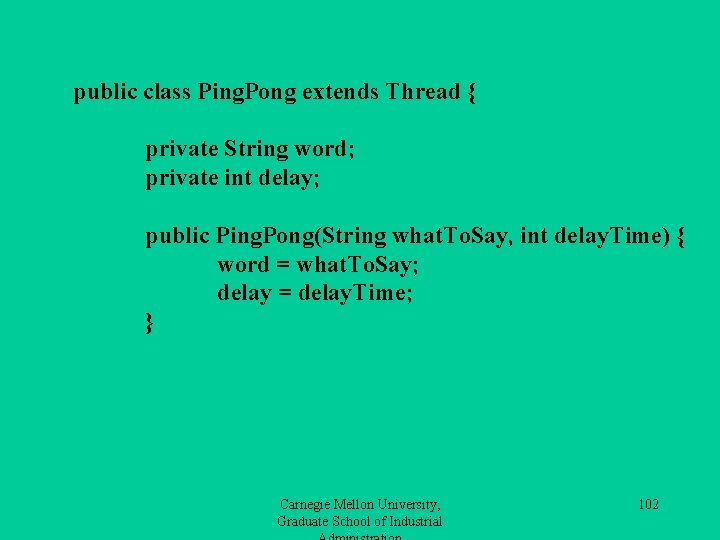
public class Ping. Pong extends Thread { private String word; private int delay; public Ping. Pong(String what. To. Say, int delay. Time) { word = what. To. Say; delay = delay. Time; } Carnegie Mellon University, Graduate School of Industrial 102
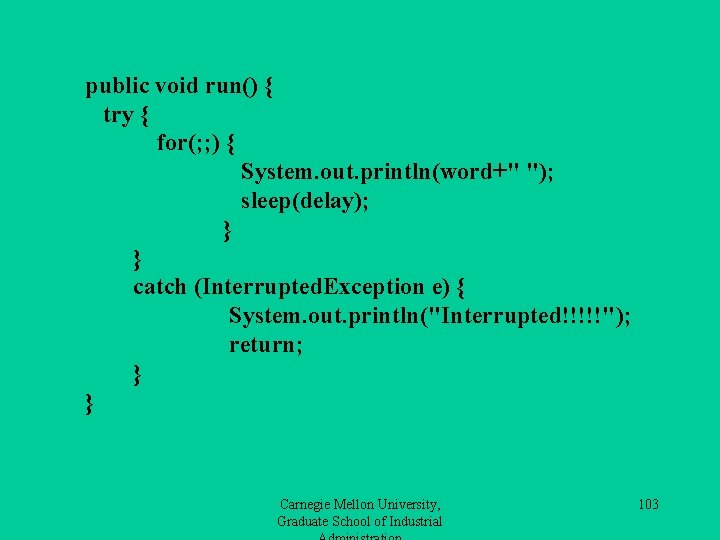
public void run() { try { for(; ; ) { System. out. println(word+" "); sleep(delay); } } catch (Interrupted. Exception e) { System. out. println("Interrupted!!!!!"); return; } } Carnegie Mellon University, Graduate School of Industrial 103
![public static void mainString args Ping Pong t 1 new Ping Pongtping public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping",](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-104.jpg)
public static void main(String args[]) { Ping. Pong t 1 = new Ping. Pong("tping", 33); t 1. start(); Ping. Pong t 2 = new Ping. Pong("Pong", 100); t 2. start(); try { Thread. sleep(5000); } catch(Interrupted. Exception e) { // will not be printed System. out. println("Good morning"); return; } Carnegie Mellon University, Graduate School of Industrial 104
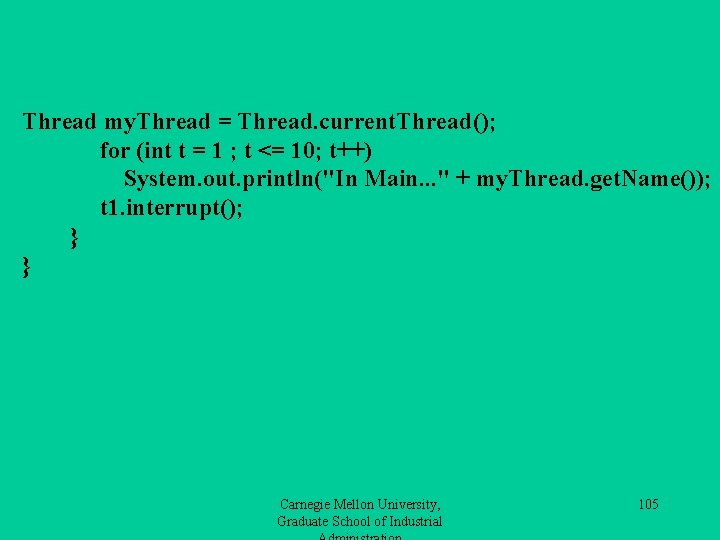
Thread my. Thread = Thread. current. Thread(); for (int t = 1 ; t <= 10; t++) System. out. println("In Main. . . " + my. Thread. get. Name()); t 1. interrupt(); } } Carnegie Mellon University, Graduate School of Industrial 105
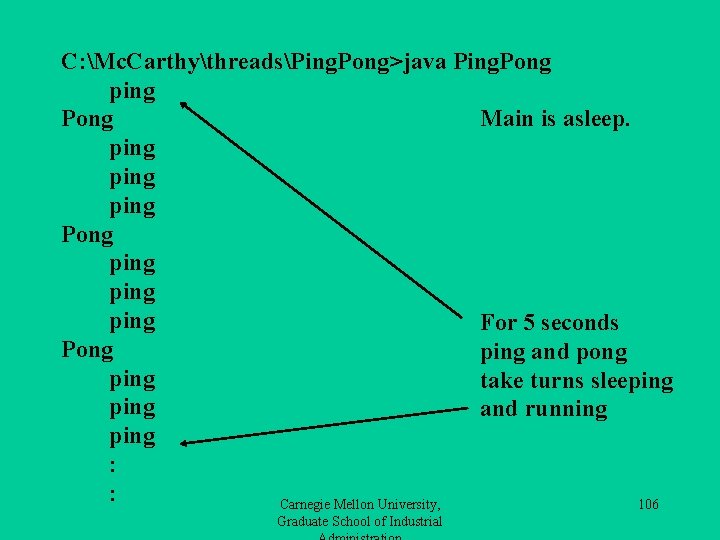
C: Mc. CarthythreadsPing. Pong>java Ping. Pong ping Main is asleep. Pong ping ping For 5 seconds Pong ping and pong ping take turns sleeping and running ping : : Carnegie Mellon University, 106 Graduate School of Industrial
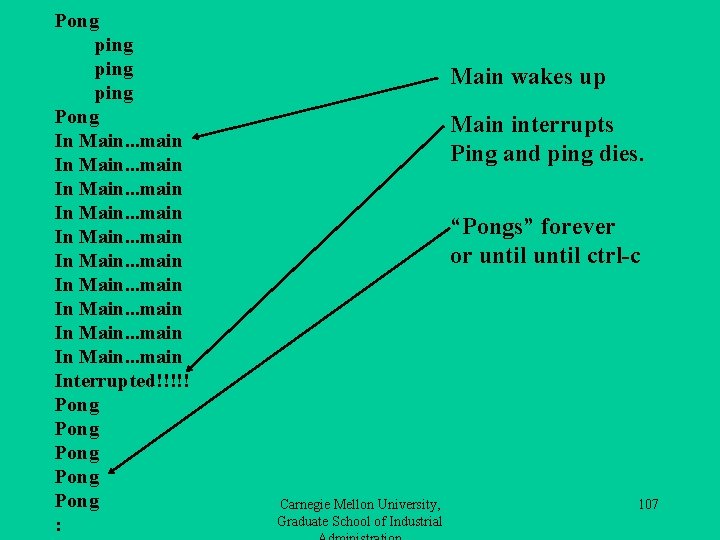
Pong ping Pong In Main. . . main In Main. . . main Interrupted!!!!! Pong Pong : Main wakes up Main interrupts Ping and ping dies. “Pongs” forever or until ctrl-c Carnegie Mellon University, Graduate School of Industrial 107
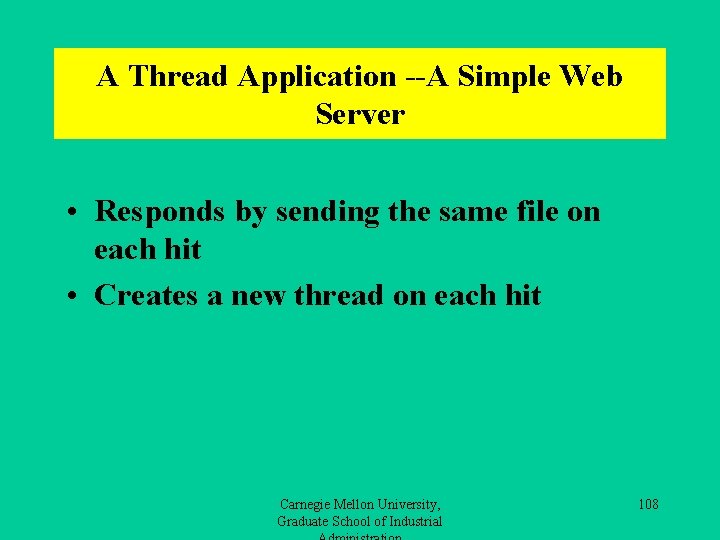
A Thread Application --A Simple Web Server • Responds by sending the same file on each hit • Creates a new thread on each hit Carnegie Mellon University, Graduate School of Industrial 108
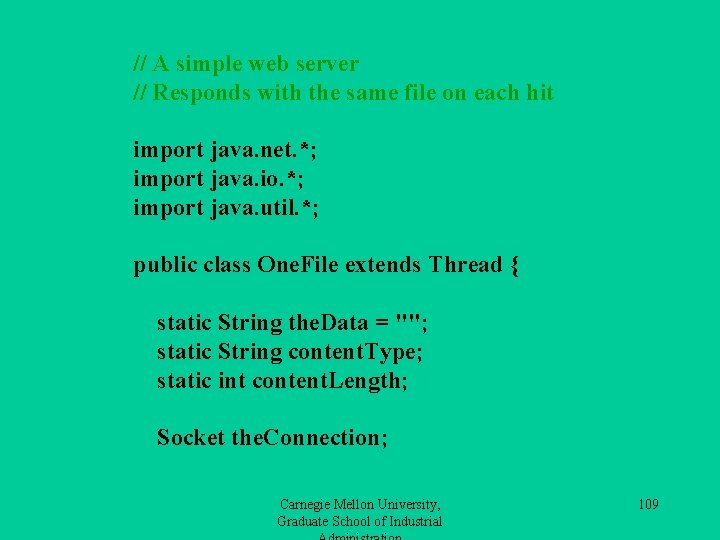
// A simple web server // Responds with the same file on each hit import java. net. *; import java. io. *; import java. util. *; public class One. File extends Thread { static String the. Data = ""; static String content. Type; static int content. Length; Socket the. Connection; Carnegie Mellon University, Graduate School of Industrial 109
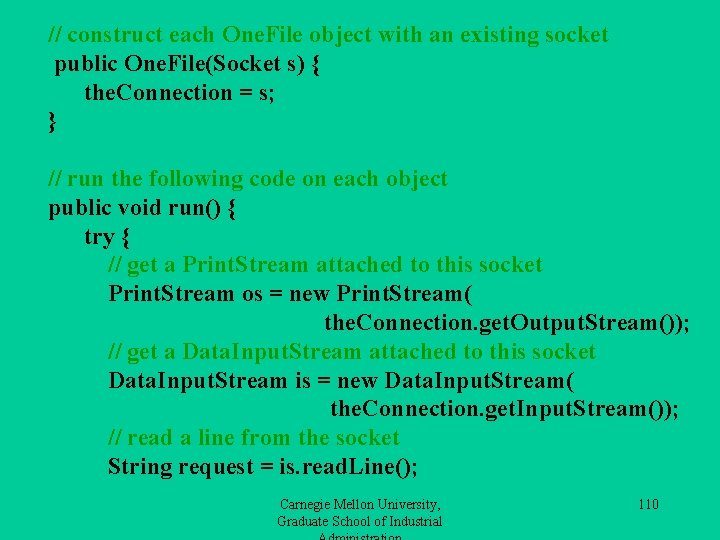
// construct each One. File object with an existing socket public One. File(Socket s) { the. Connection = s; } // run the following code on each object public void run() { try { // get a Print. Stream attached to this socket Print. Stream os = new Print. Stream( the. Connection. get. Output. Stream()); // get a Data. Input. Stream attached to this socket Data. Input. Stream is = new Data. Input. Stream( the. Connection. get. Input. Stream()); // read a line from the socket String request = is. read. Line(); Carnegie Mellon University, Graduate School of Industrial 110
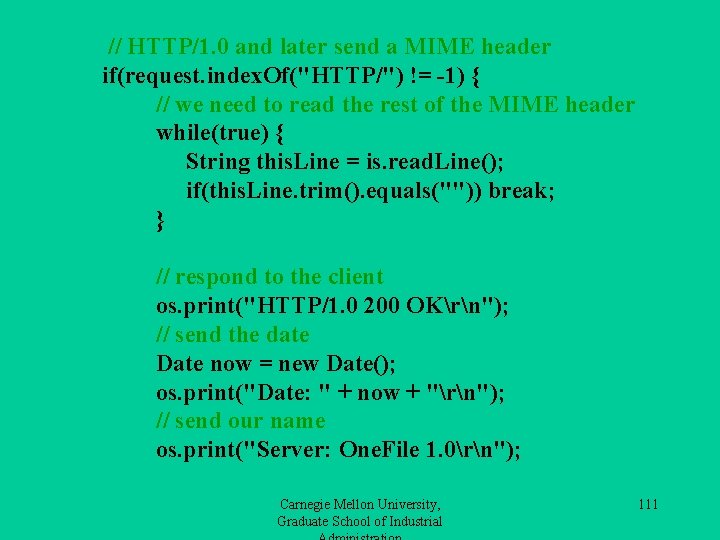
// HTTP/1. 0 and later send a MIME header if(request. index. Of("HTTP/") != -1) { // we need to read the rest of the MIME header while(true) { String this. Line = is. read. Line(); if(this. Line. trim(). equals("")) break; } // respond to the client os. print("HTTP/1. 0 200 OKrn"); // send the date Date now = new Date(); os. print("Date: " + now + "rn"); // send our name os. print("Server: One. File 1. 0rn"); Carnegie Mellon University, Graduate School of Industrial 111
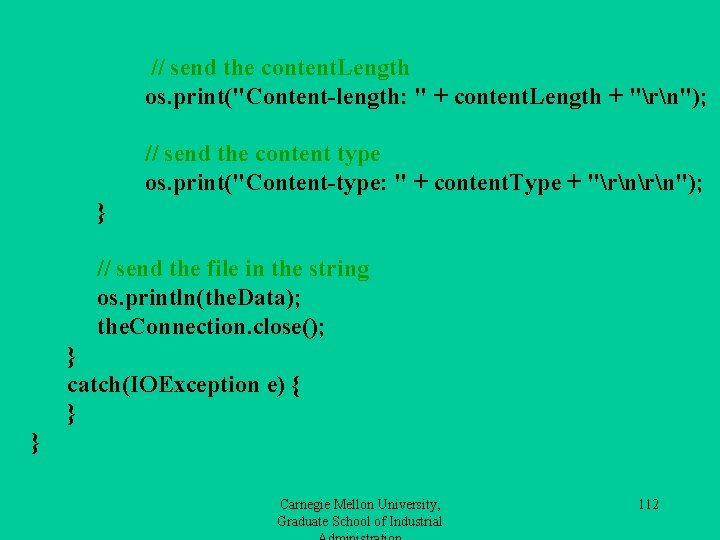
// send the content. Length os. print("Content-length: " + content. Length + "rn"); // send the content type os. print("Content-type: " + content. Type + "rn"); } // send the file in the string os. println(the. Data); the. Connection. close(); } catch(IOException e) { } } Carnegie Mellon University, Graduate School of Industrial 112
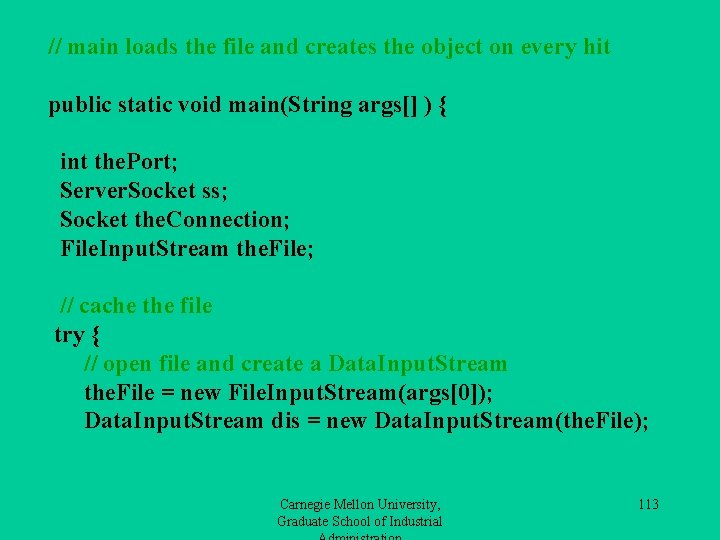
// main loads the file and creates the object on every hit public static void main(String args[] ) { int the. Port; Server. Socket ss; Socket the. Connection; File. Input. Stream the. File; // cache the file try { // open file and create a Data. Input. Stream the. File = new File. Input. Stream(args[0]); Data. Input. Stream dis = new Data. Input. Stream(the. File); Carnegie Mellon University, Graduate School of Industrial 113
![determine the content type of this file ifargs0 ends With html } // determine the content type of this file if(args[0]. ends. With(". html") ||](https://slidetodoc.com/presentation_image_h2/7df6c0bf0072c9ef2d8e790be5fb8121/image-114.jpg)
} // determine the content type of this file if(args[0]. ends. With(". html") || args[0]. ends. With(". htm") ) { content. Type = "text/html"; } else { content. Type = "text/plain"; } // read the file into the string the. Data try { String this. Line; while((this. Line = dis. read. Line()) != null) { the. Data += this. Line + "n"; } } catch(Exception e) { System. err. println("Error " + e); } Carnegie Mellon University, 114 Graduate School of Industrial
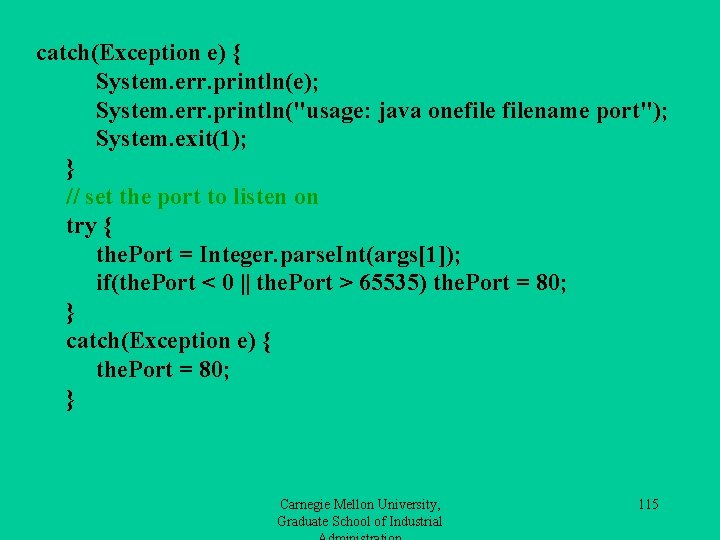
catch(Exception e) { System. err. println(e); System. err. println("usage: java onefilename port"); System. exit(1); } // set the port to listen on try { the. Port = Integer. parse. Int(args[1]); if(the. Port < 0 || the. Port > 65535) the. Port = 80; } catch(Exception e) { the. Port = 80; } Carnegie Mellon University, Graduate School of Industrial 115
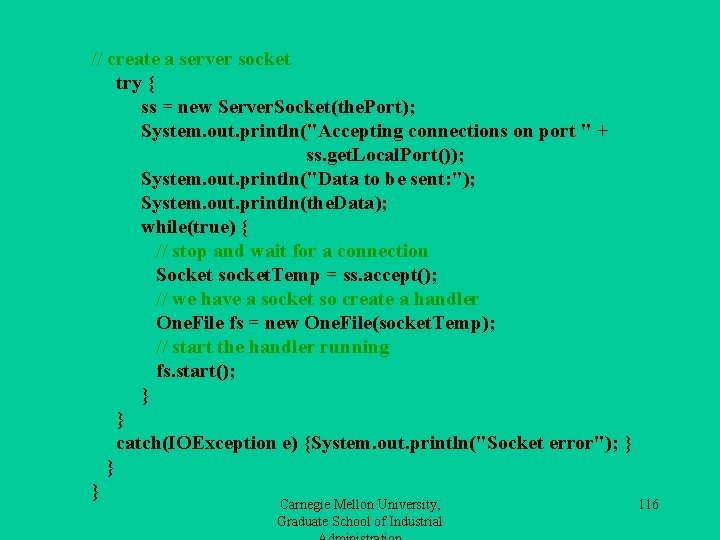
// create a server socket try { ss = new Server. Socket(the. Port); System. out. println("Accepting connections on port " + ss. get. Local. Port()); System. out. println("Data to be sent: "); System. out. println(the. Data); while(true) { // stop and wait for a connection Socket socket. Temp = ss. accept(); // we have a socket so create a handler One. File fs = new One. File(socket. Temp); // start the handler running fs. start(); } } catch(IOException e) {System. out. println("Socket error"); } } } Carnegie Mellon University, Graduate School of Industrial 116