Relational Expression equal to not equal to less
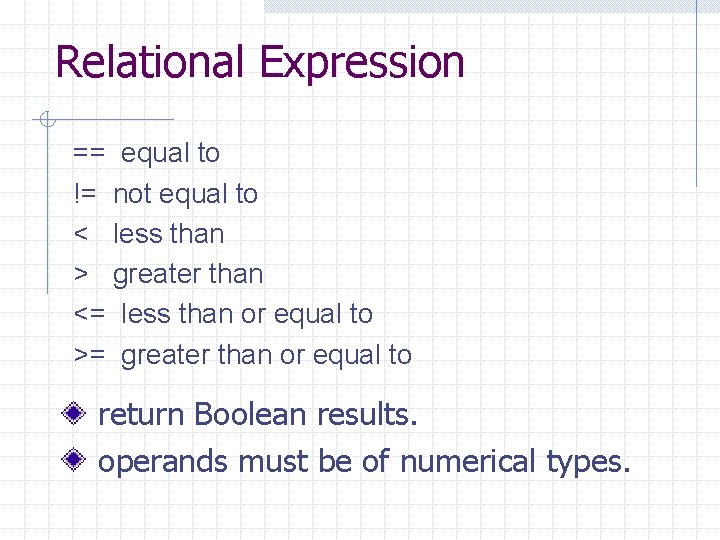
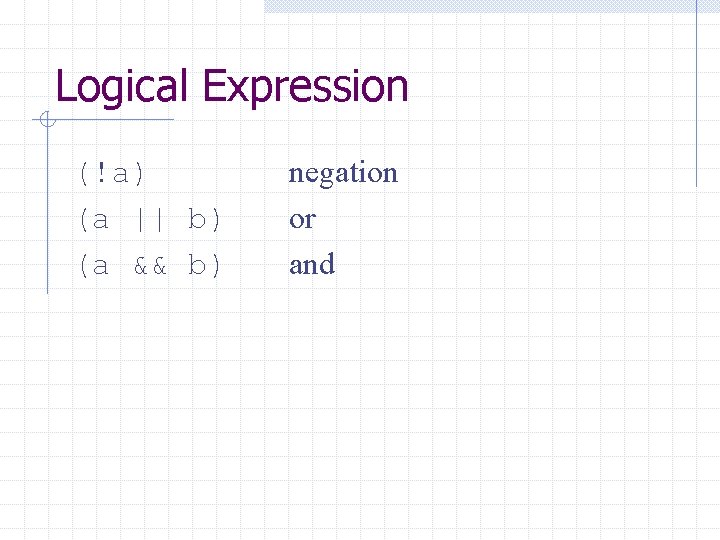
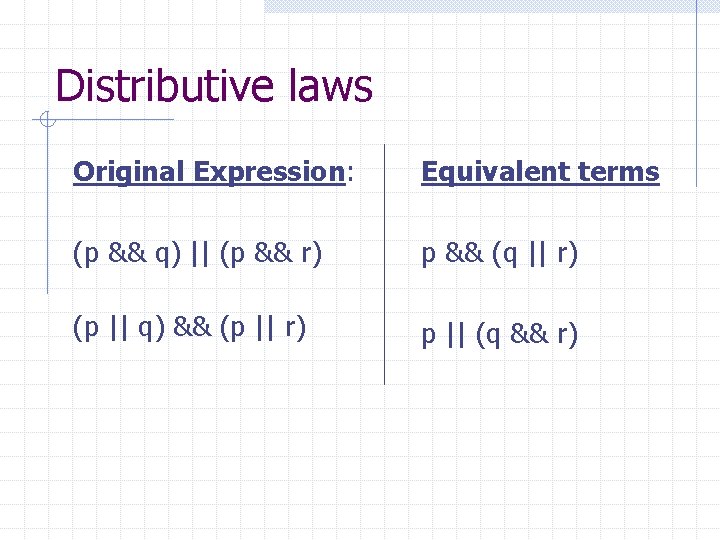
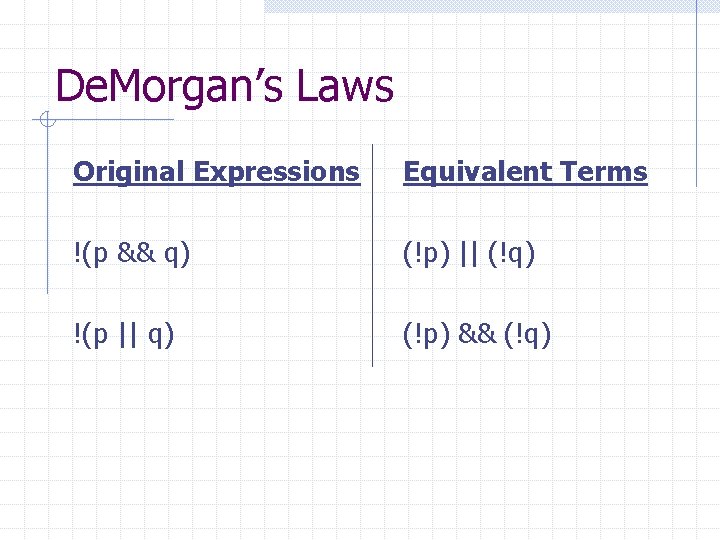
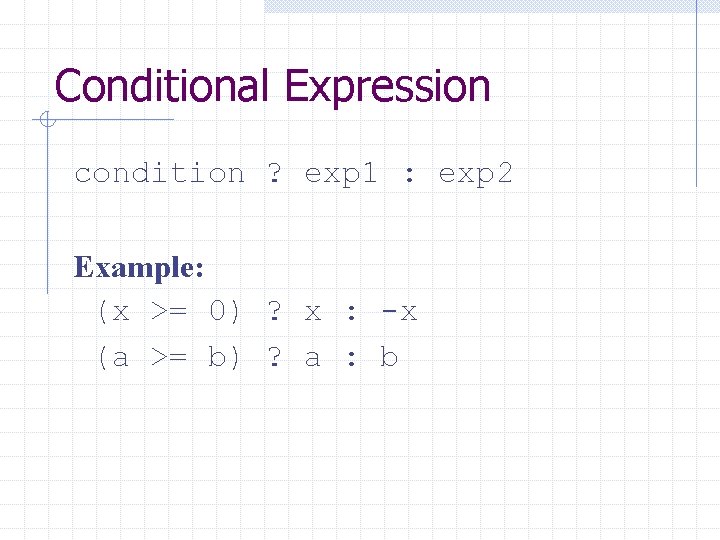
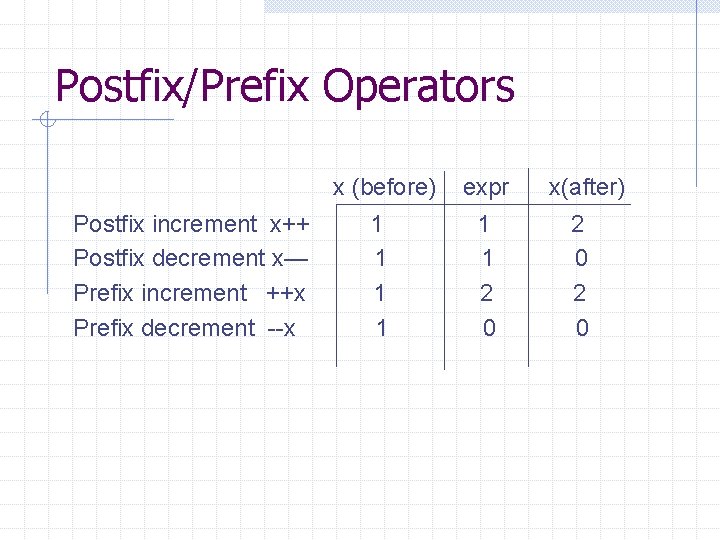
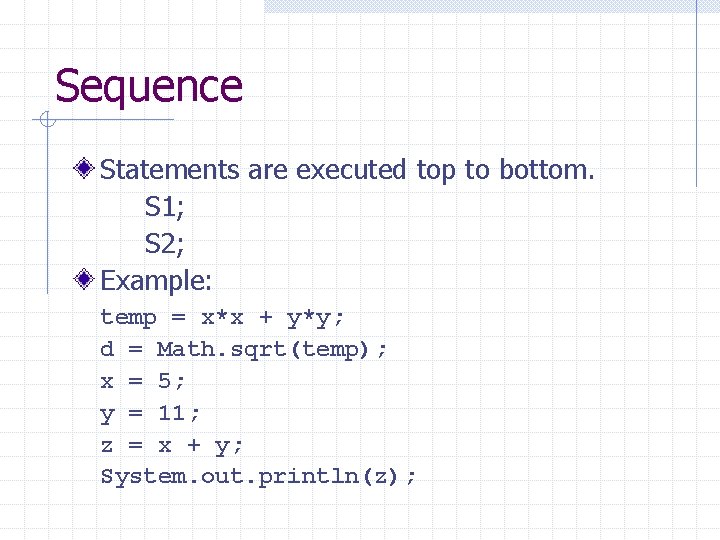
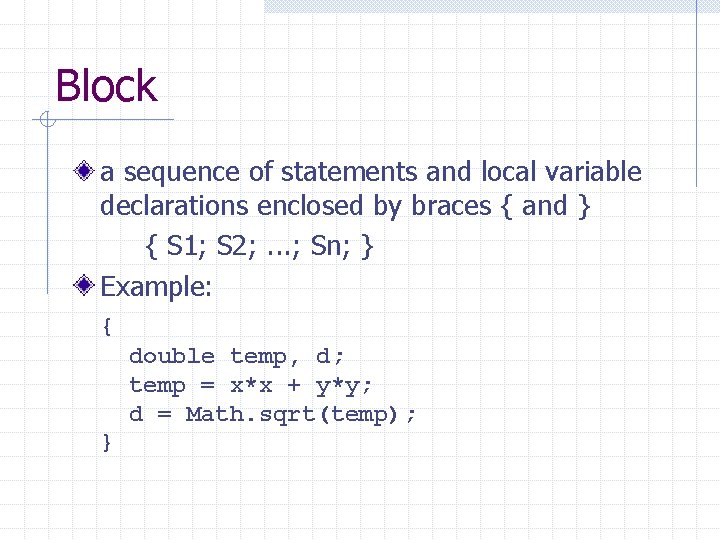
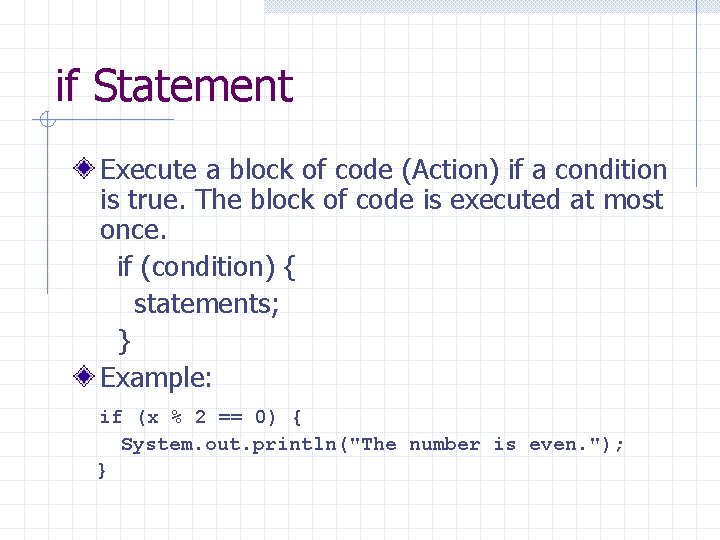
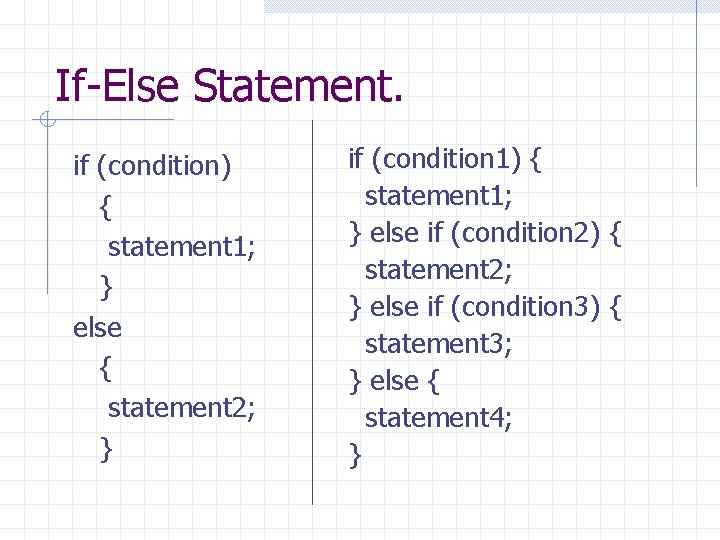
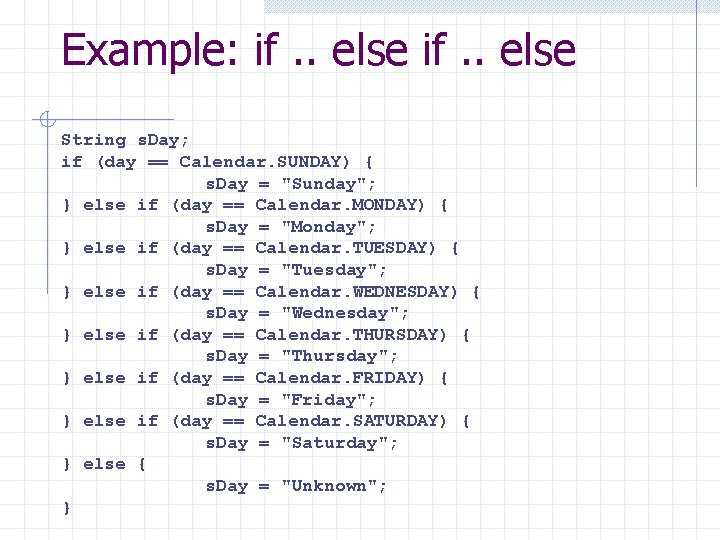
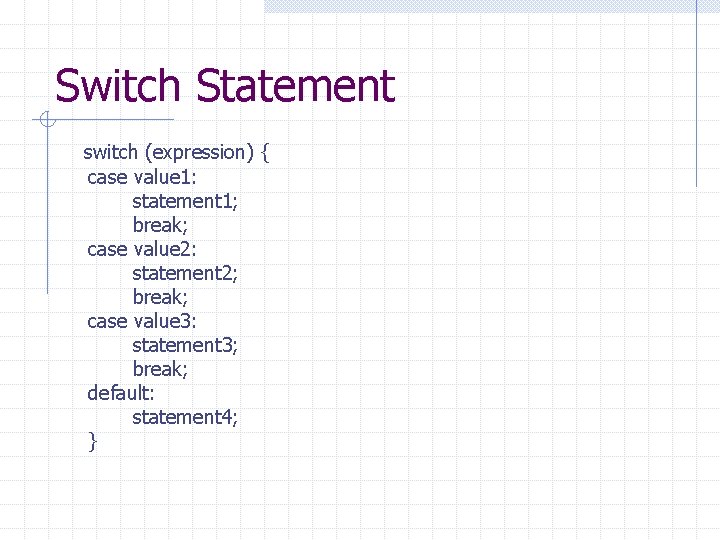
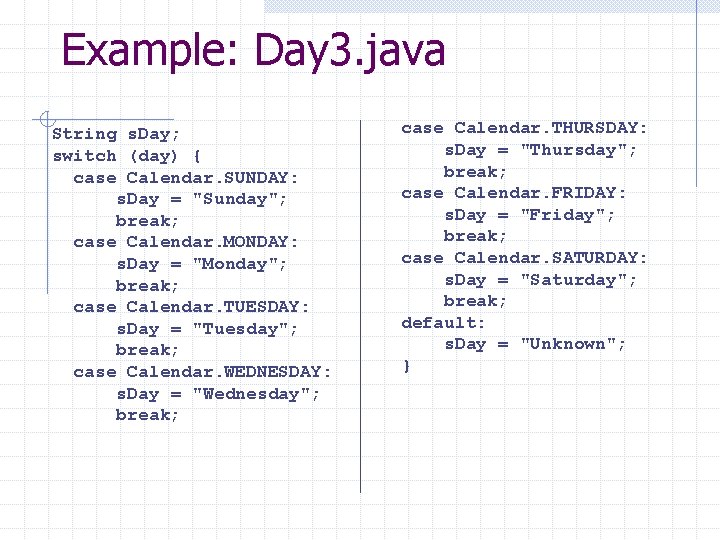
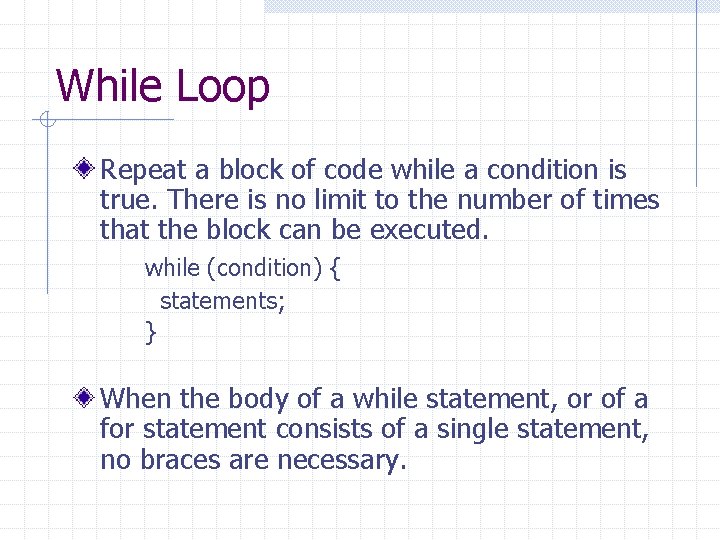
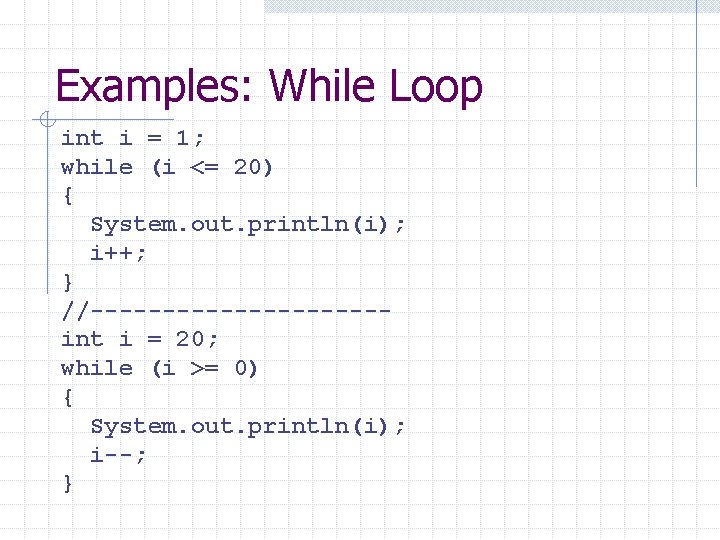
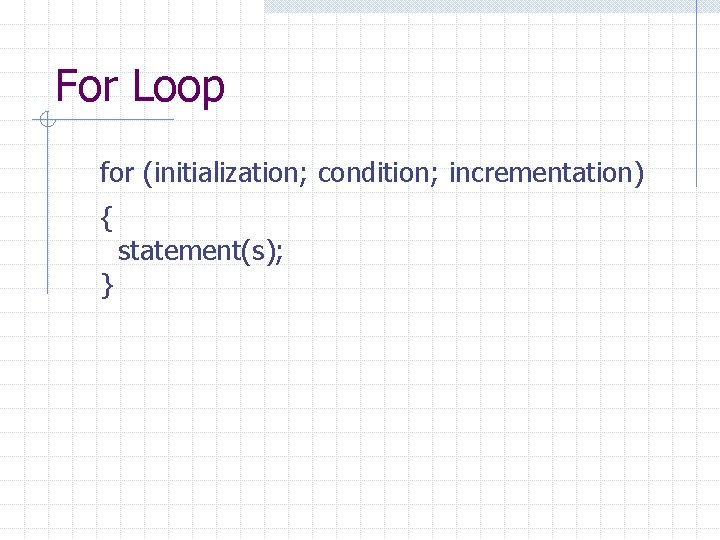
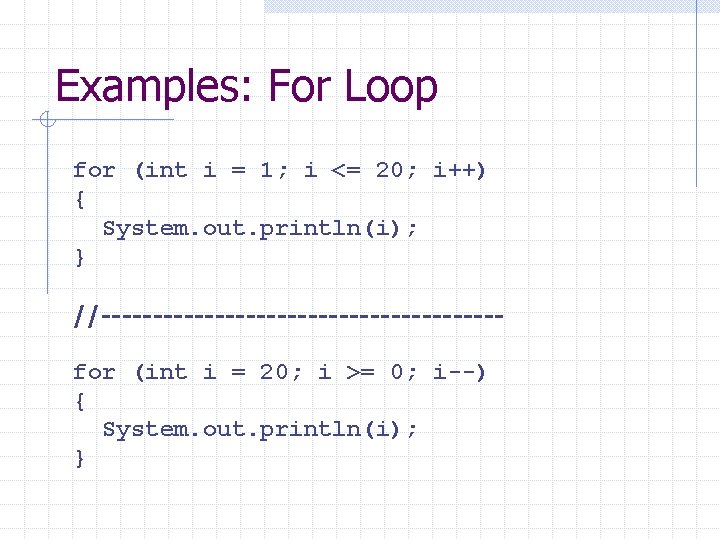
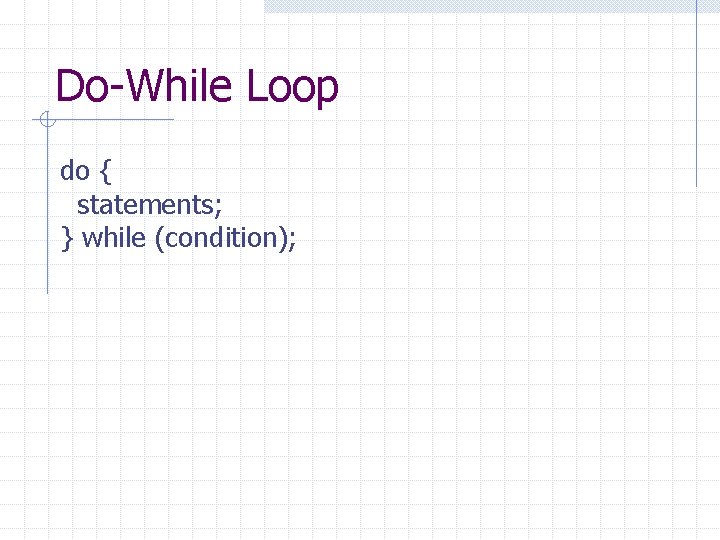
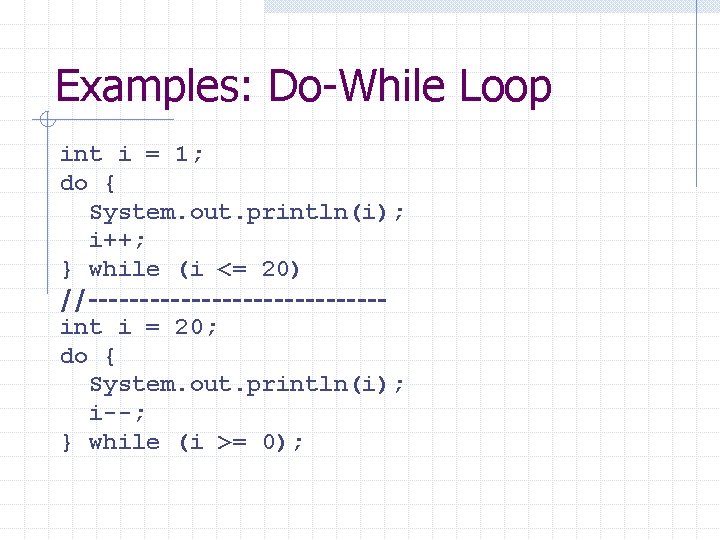
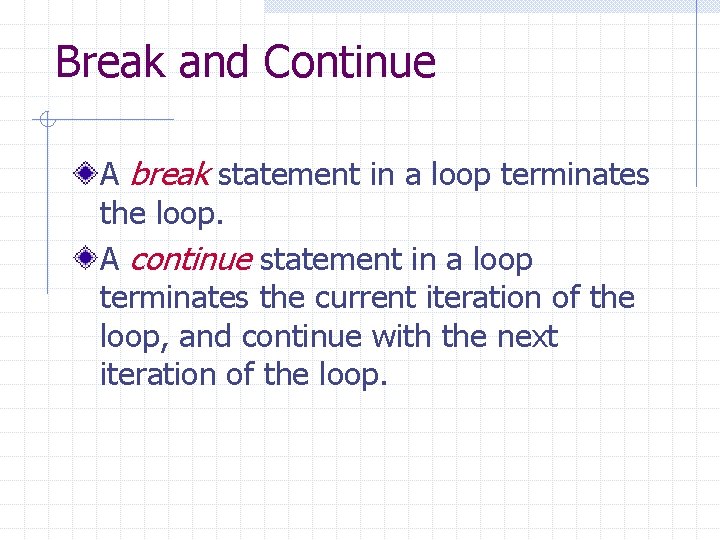
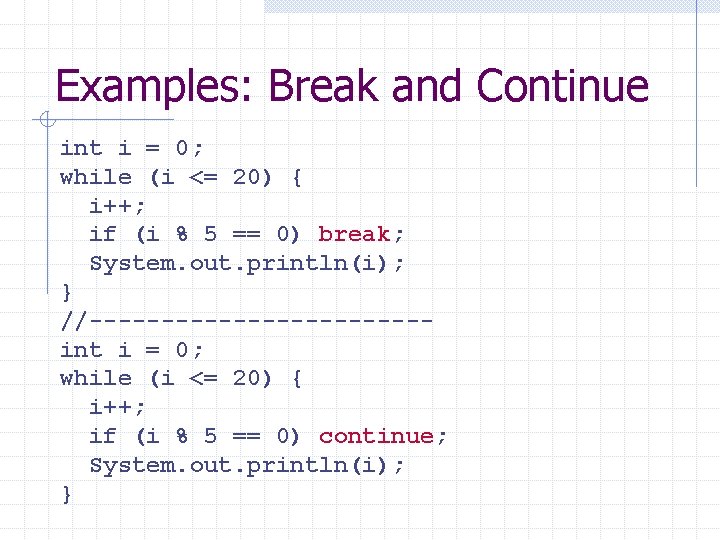
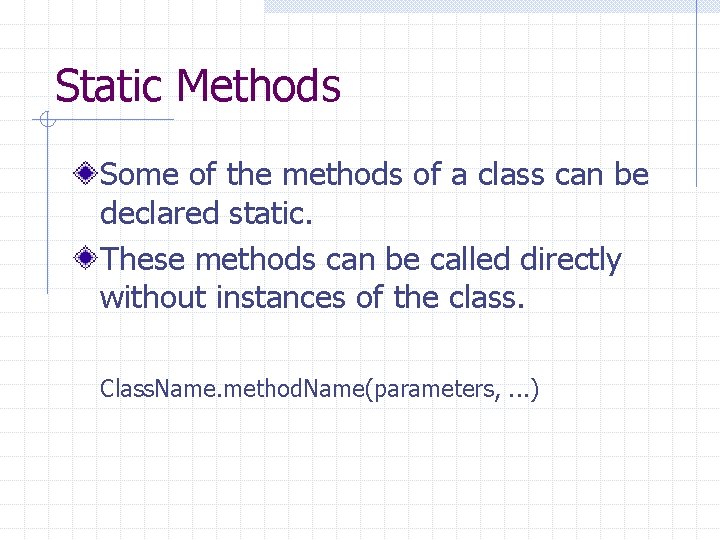
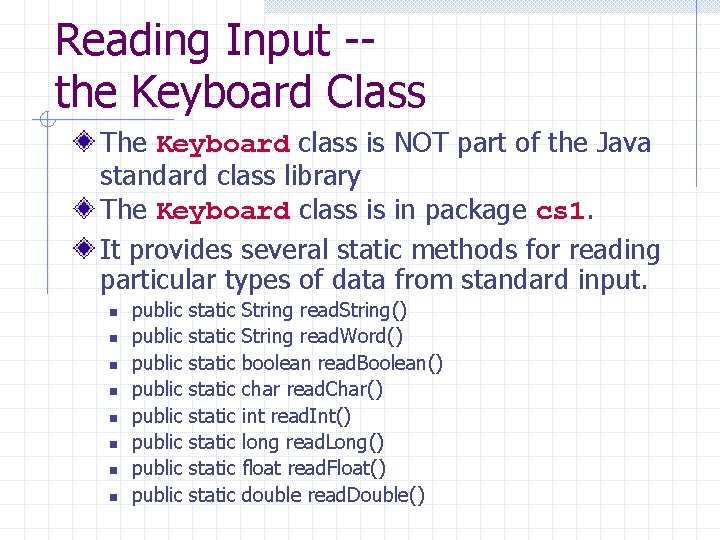
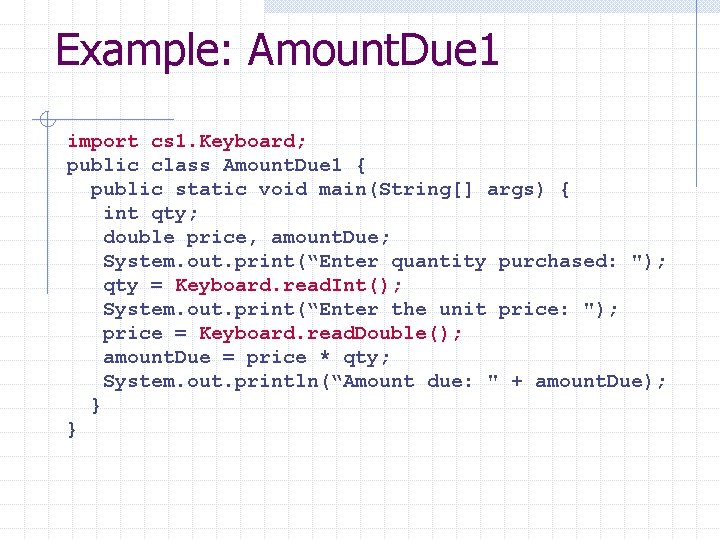
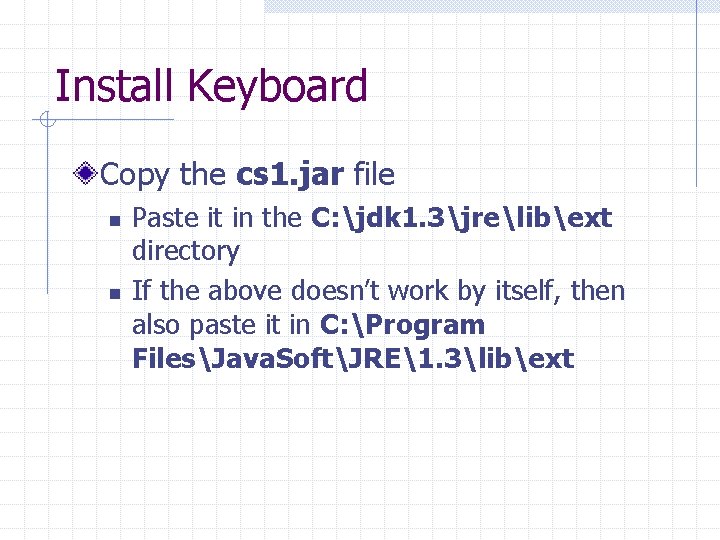
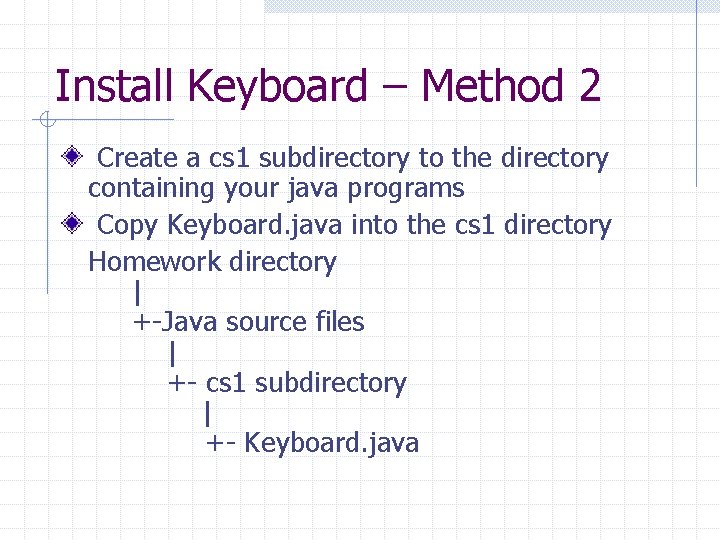
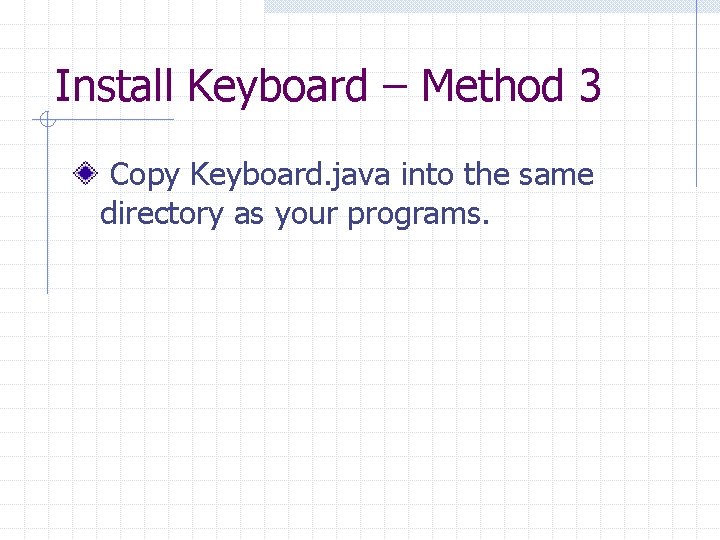
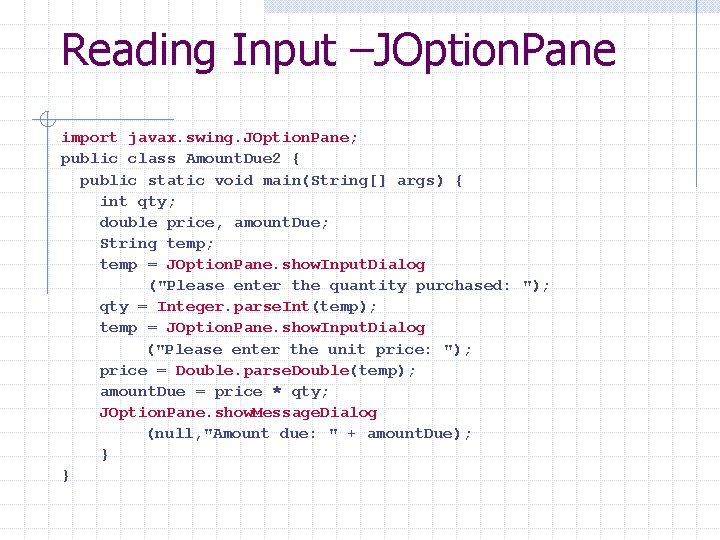
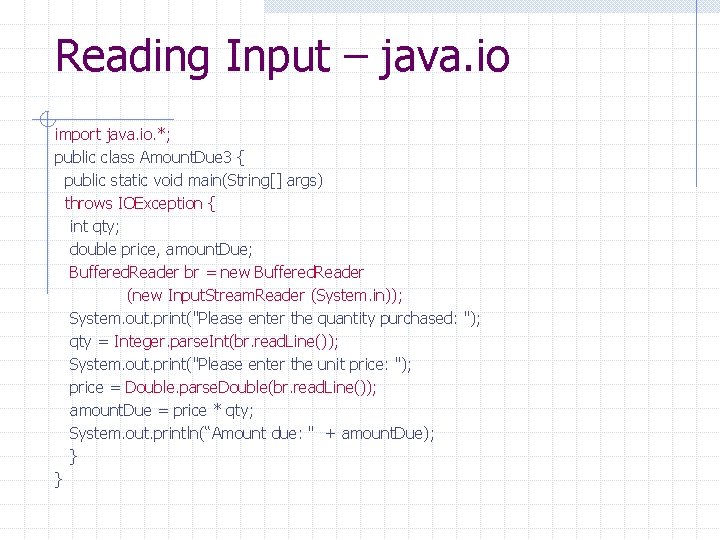
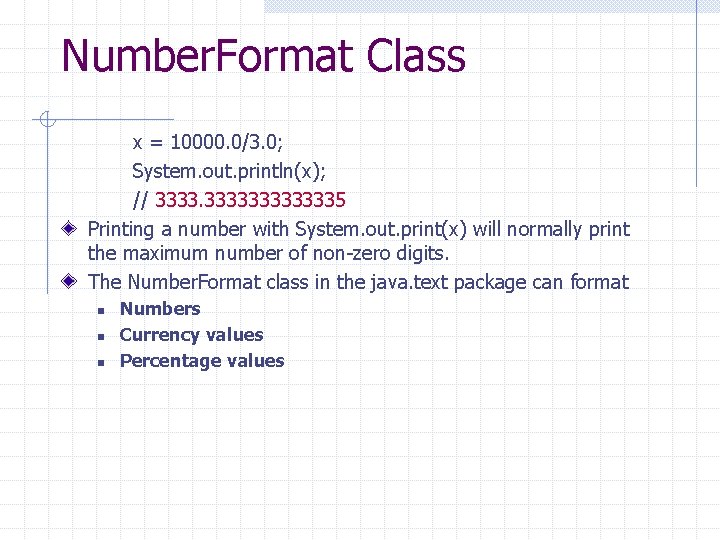
![Number Format import java. text. *; public class Formatted. Output{ public static void main(String[] Number Format import java. text. *; public class Formatted. Output{ public static void main(String[]](https://slidetodoc.com/presentation_image_h2/af18d6e3bfe53baf24f33d0018393672/image-31.jpg)
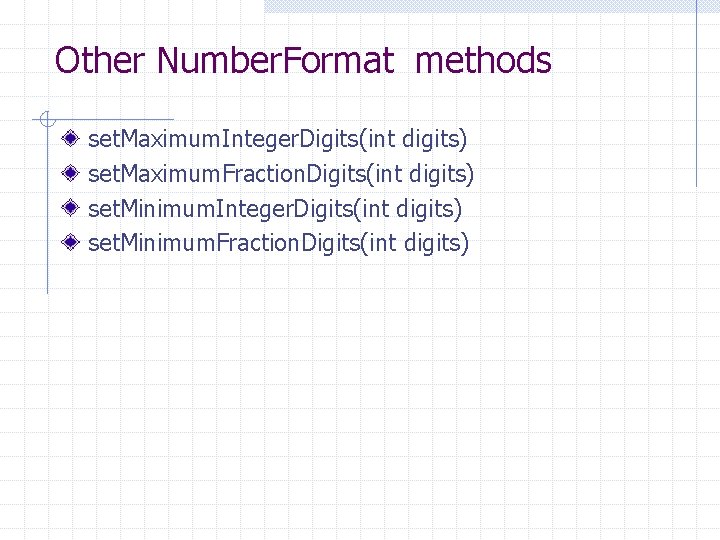
- Slides: 32
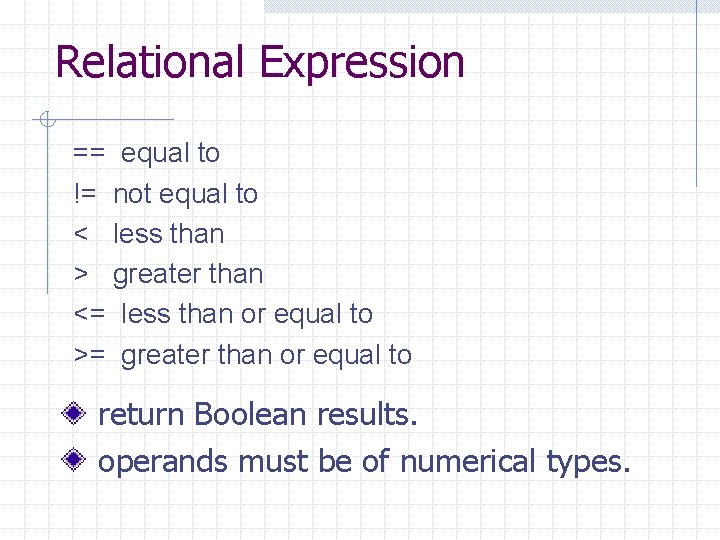
Relational Expression == equal to != not equal to < less than > greater than <= less than or equal to >= greater than or equal to return Boolean results. operands must be of numerical types.
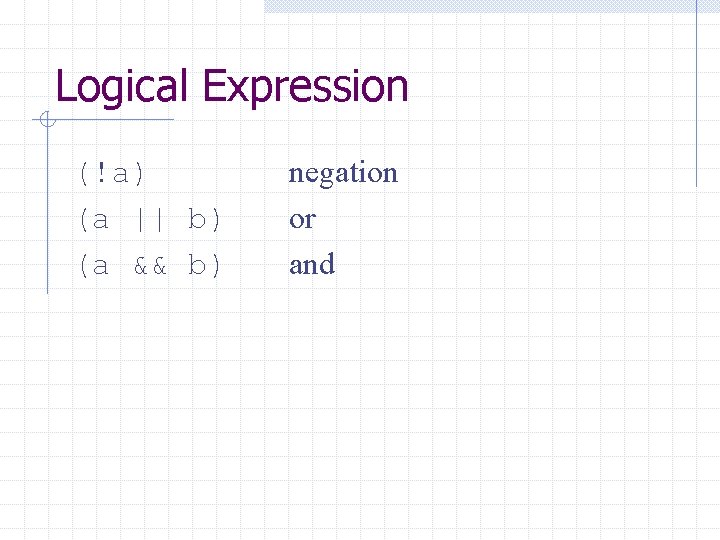
Logical Expression (!a) (a || b) (a && b) negation or and
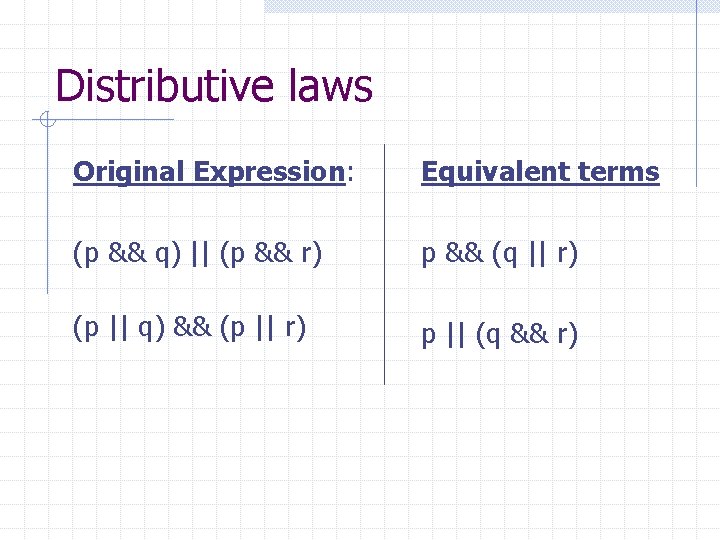
Distributive laws Original Expression: Equivalent terms (p && q) || (p && r) p && (q || r) (p || q) && (p || r) p || (q && r)
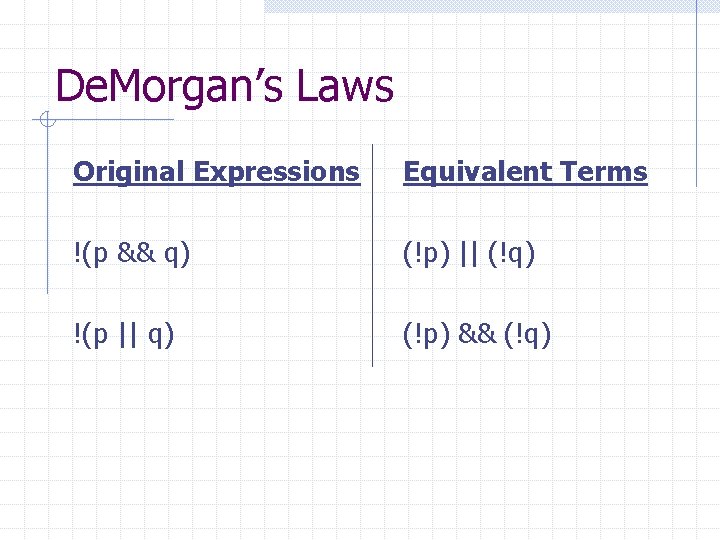
De. Morgan’s Laws Original Expressions Equivalent Terms !(p && q) (!p) || (!q) !(p || q) (!p) && (!q)
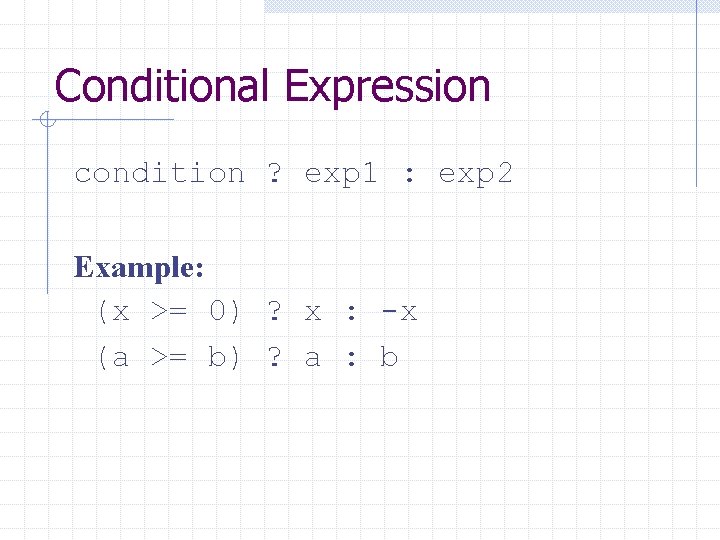
Conditional Expression condition ? exp 1 : exp 2 Example: (x >= 0) ? x : -x (a >= b) ? a : b
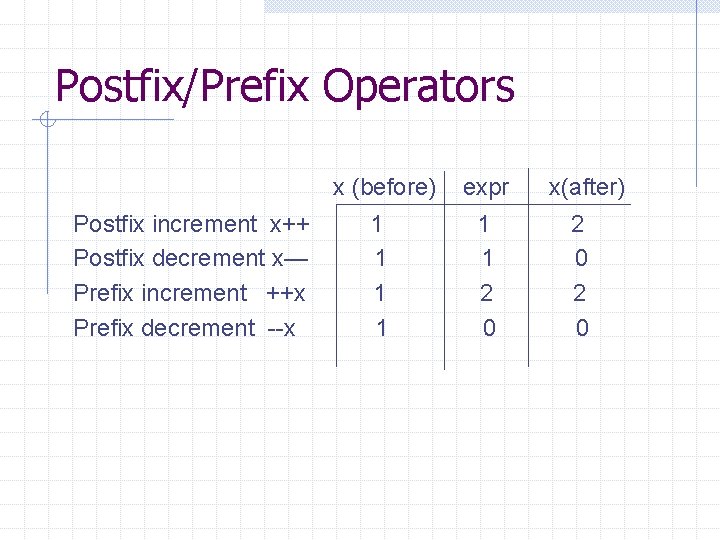
Postfix/Prefix Operators Postfix increment x++ Postfix decrement x— Prefix increment ++x Prefix decrement --x x (before) expr 1 1 1 2 0 x(after) 2 0
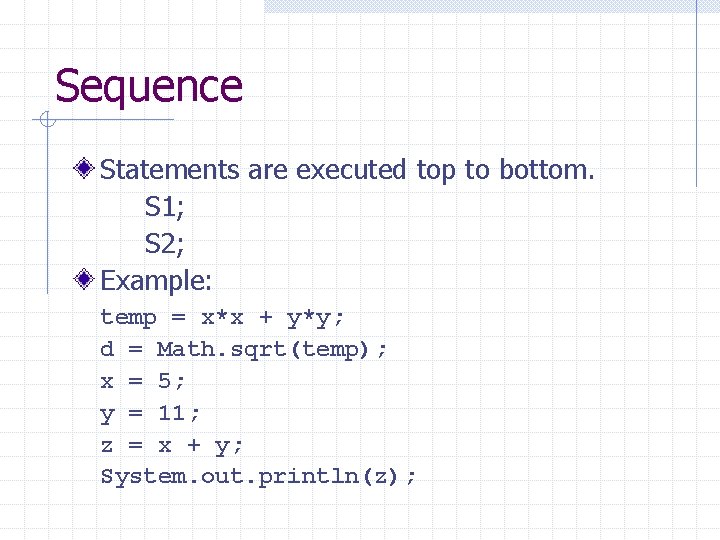
Sequence Statements are executed top to bottom. S 1; S 2; Example: temp = x*x + y*y; d = Math. sqrt(temp); x = 5; y = 11; z = x + y; System. out. println(z);
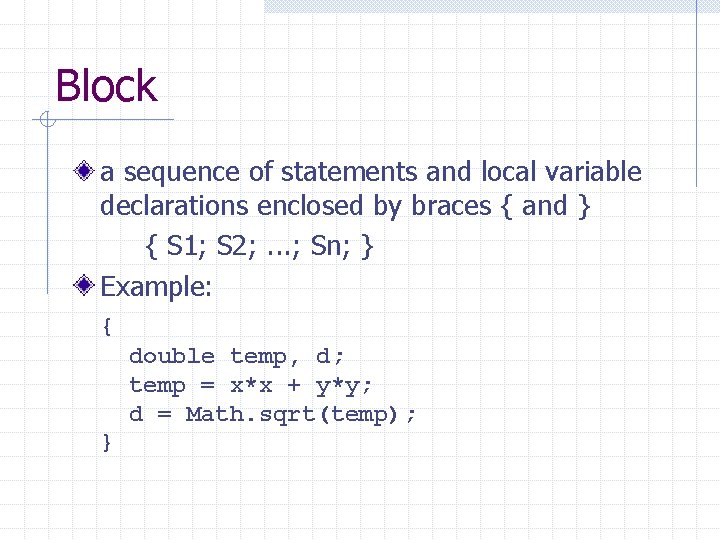
Block a sequence of statements and local variable declarations enclosed by braces { and } { S 1; S 2; . . . ; Sn; } Example: { double temp, d; temp = x*x + y*y; d = Math. sqrt(temp); }
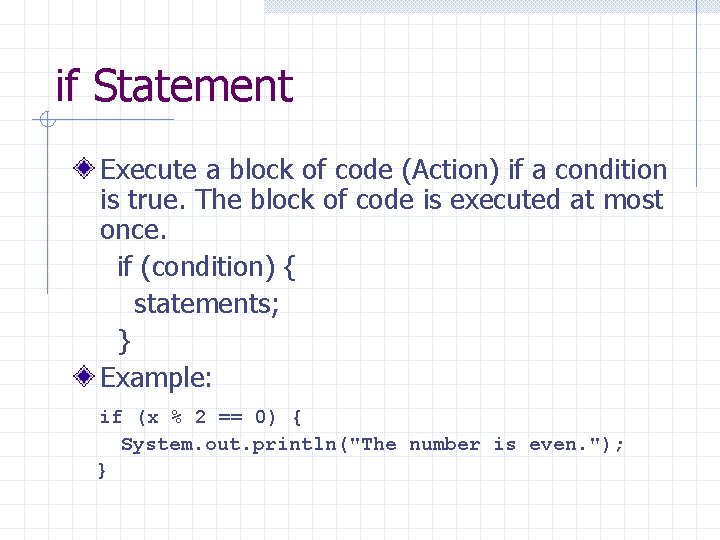
if Statement Execute a block of code (Action) if a condition is true. The block of code is executed at most once. if (condition) { statements; } Example: if (x % 2 == 0) { System. out. println("The number is even. "); }
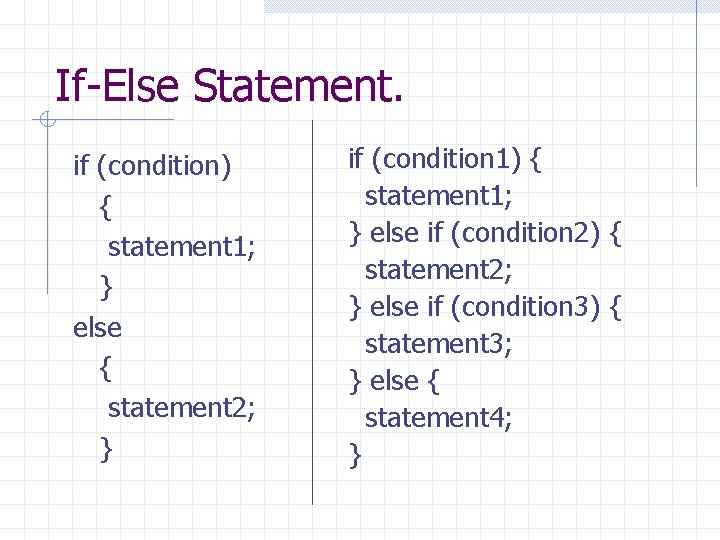
If-Else Statement. if (condition) { statement 1; } else { statement 2; } if (condition 1) { statement 1; } else if (condition 2) { statement 2; } else if (condition 3) { statement 3; } else { statement 4; }
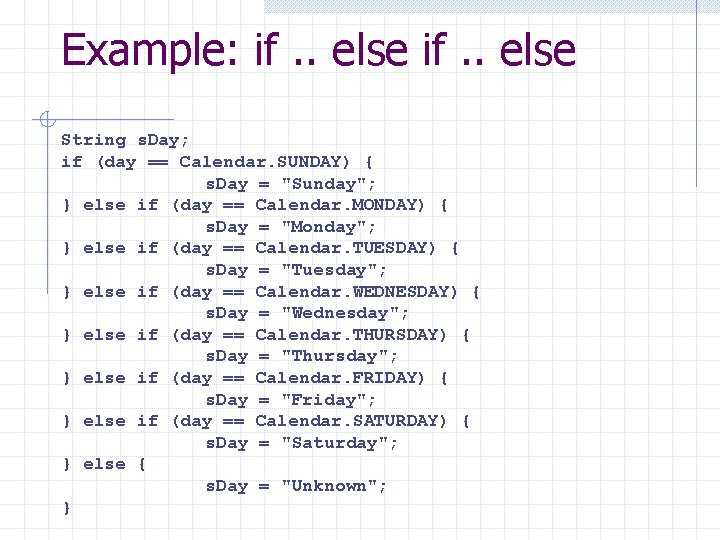
Example: if. . else String s. Day; if (day == Calendar. SUNDAY) { s. Day = "Sunday"; } else if (day == Calendar. MONDAY) { s. Day = "Monday"; } else if (day == Calendar. TUESDAY) { s. Day = "Tuesday"; } else if (day == Calendar. WEDNESDAY) { s. Day = "Wednesday"; } else if (day == Calendar. THURSDAY) { s. Day = "Thursday"; } else if (day == Calendar. FRIDAY) { s. Day = "Friday"; } else if (day == Calendar. SATURDAY) { s. Day = "Saturday"; } else { s. Day = "Unknown"; }
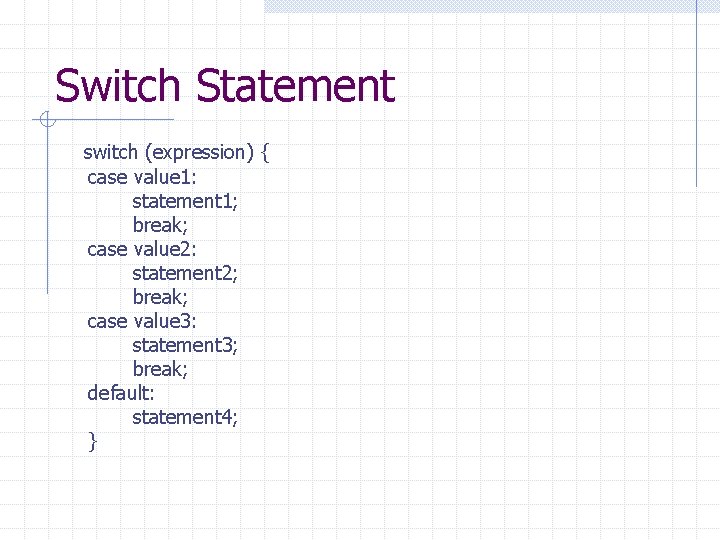
Switch Statement switch (expression) { case value 1: statement 1; break; case value 2: statement 2; break; case value 3: statement 3; break; default: statement 4; }
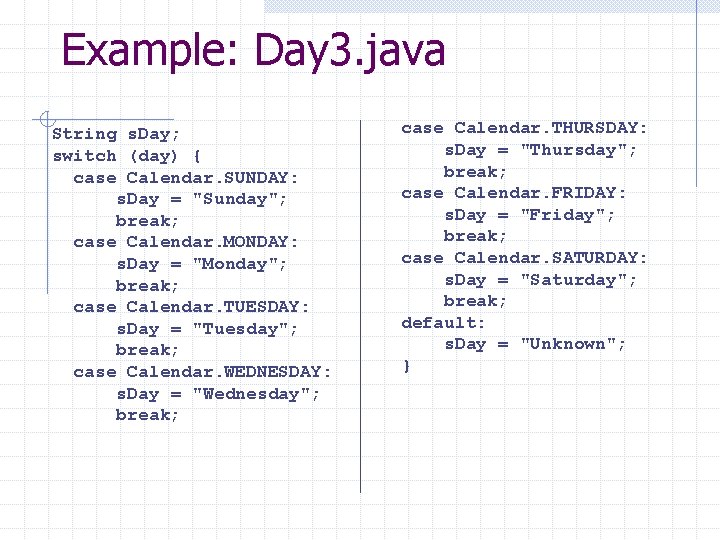
Example: Day 3. java String s. Day; switch (day) { case Calendar. SUNDAY: s. Day = "Sunday"; break; case Calendar. MONDAY: s. Day = "Monday"; break; case Calendar. TUESDAY: s. Day = "Tuesday"; break; case Calendar. WEDNESDAY: s. Day = "Wednesday"; break; case Calendar. THURSDAY: s. Day = "Thursday"; break; case Calendar. FRIDAY: s. Day = "Friday"; break; case Calendar. SATURDAY: s. Day = "Saturday"; break; default: s. Day = "Unknown"; }
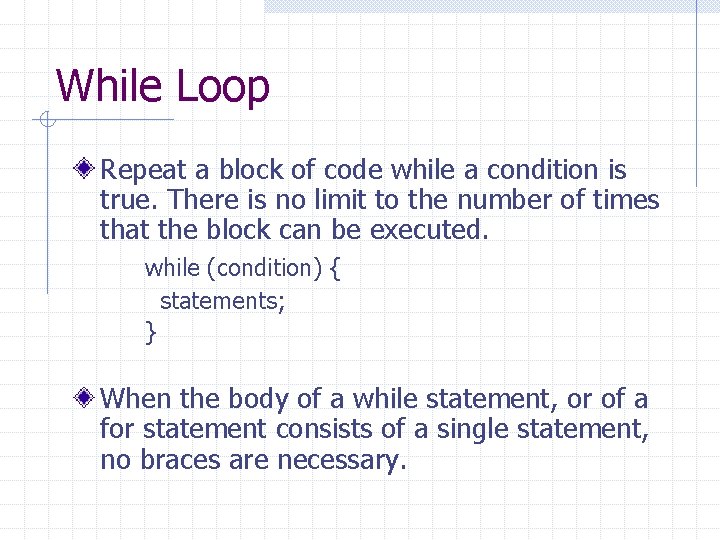
While Loop Repeat a block of code while a condition is true. There is no limit to the number of times that the block can be executed. while (condition) { statements; } When the body of a while statement, or of a for statement consists of a single statement, no braces are necessary.
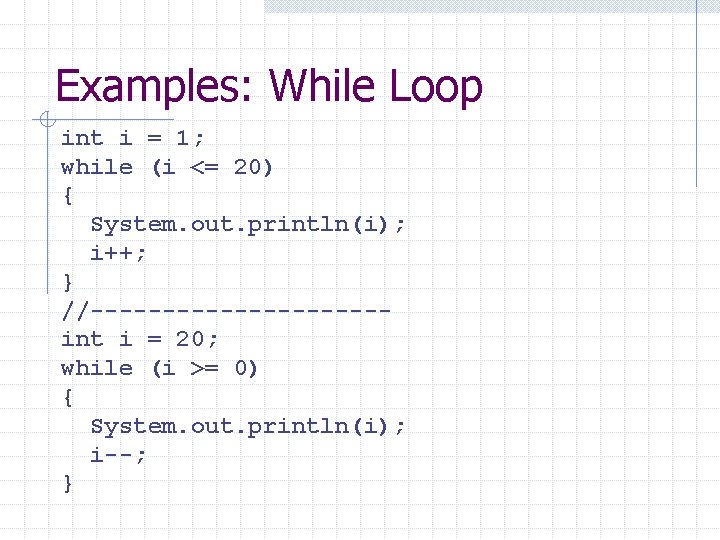
Examples: While Loop int i = 1; while (i <= 20) { System. out. println(i); i++; } //----------int i = 20; while (i >= 0) { System. out. println(i); i--; }
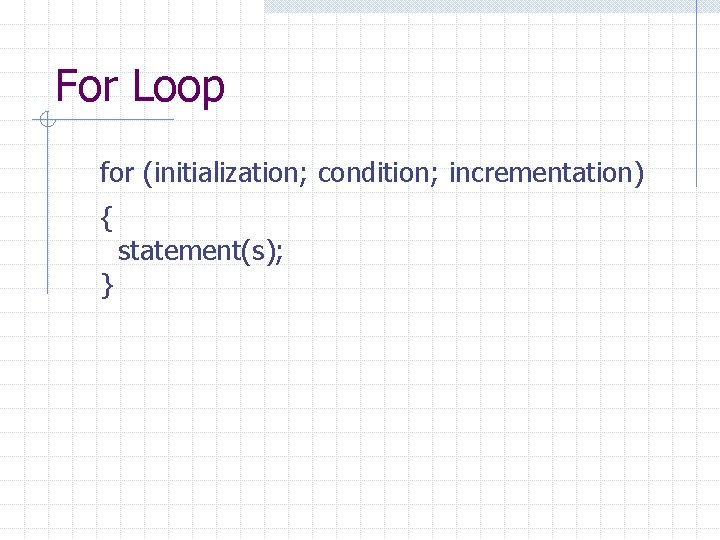
For Loop for (initialization; condition; incrementation) { } statement(s);
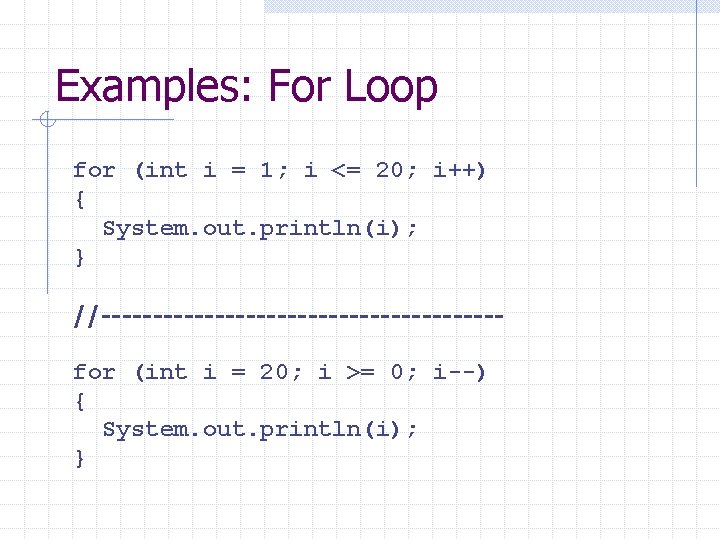
Examples: For Loop for (int i = 1; i <= 20; i++) { System. out. println(i); } //-------------------for (int i = 20; i >= 0; i--) { System. out. println(i); }
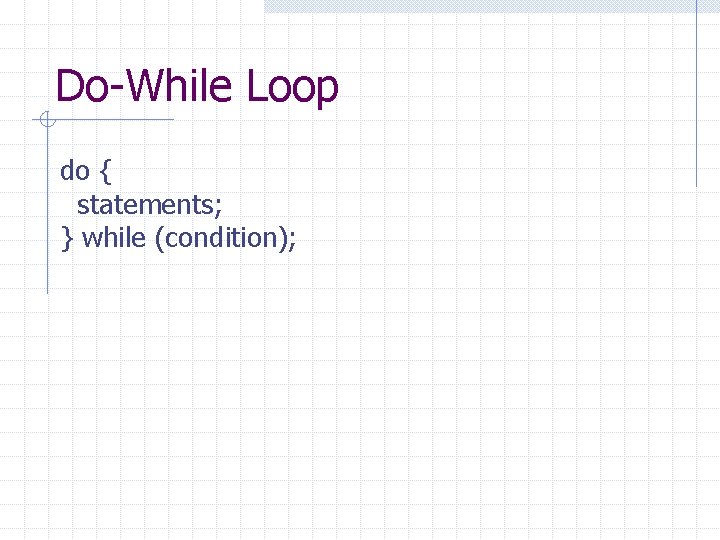
Do-While Loop do { statements; } while (condition);
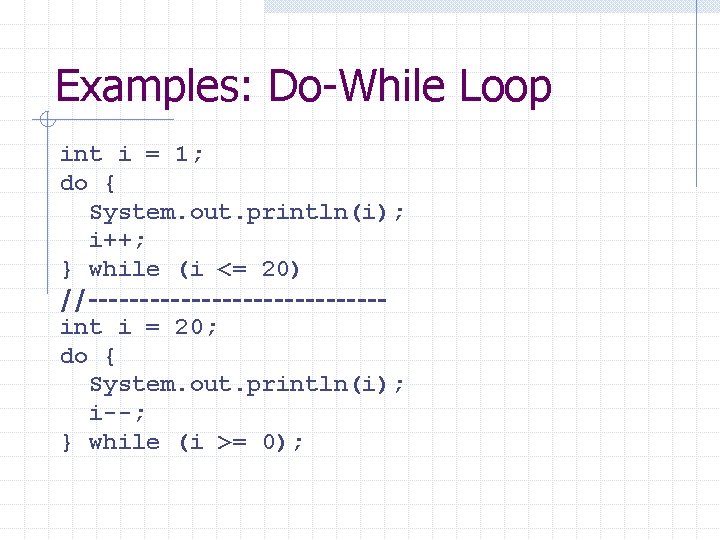
Examples: Do-While Loop int i = 1; do { System. out. println(i); i++; } while (i <= 20) //--------------int i = 20; do { System. out. println(i); i--; } while (i >= 0);
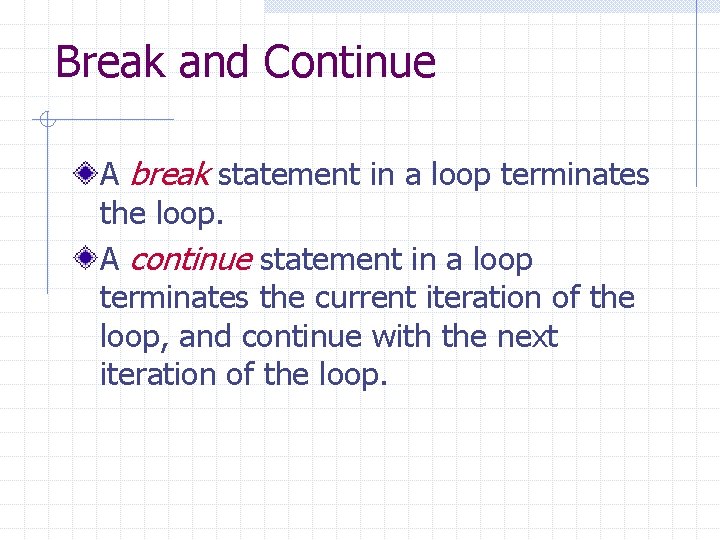
Break and Continue A break statement in a loop terminates the loop. A continue statement in a loop terminates the current iteration of the loop, and continue with the next iteration of the loop.
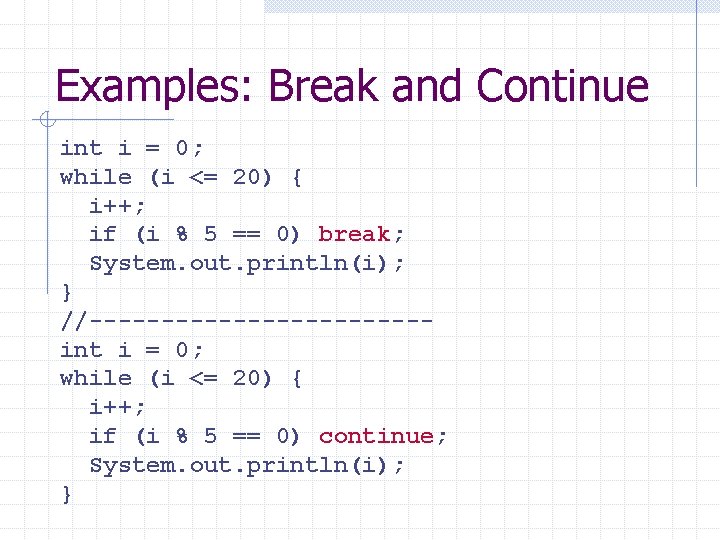
Examples: Break and Continue int i = 0; while (i <= 20) { i++; if (i % 5 == 0) break; System. out. println(i); } //------------int i = 0; while (i <= 20) { i++; if (i % 5 == 0) continue; System. out. println(i); }
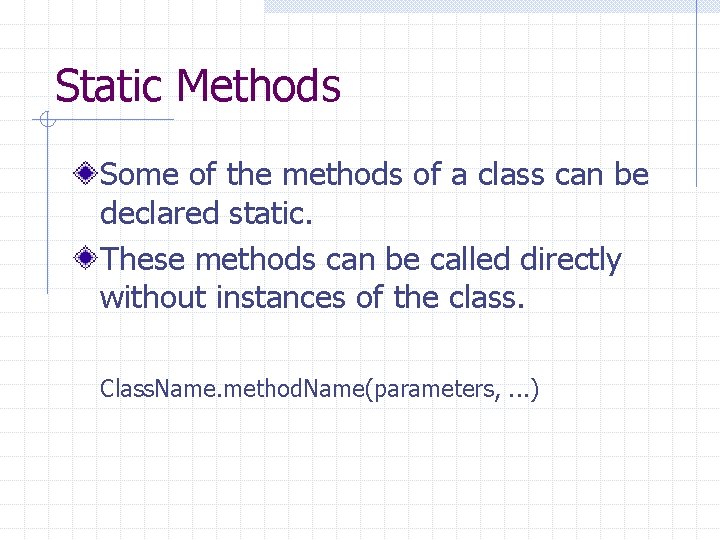
Static Methods Some of the methods of a class can be declared static. These methods can be called directly without instances of the class. Class. Name. method. Name(parameters, . . . )
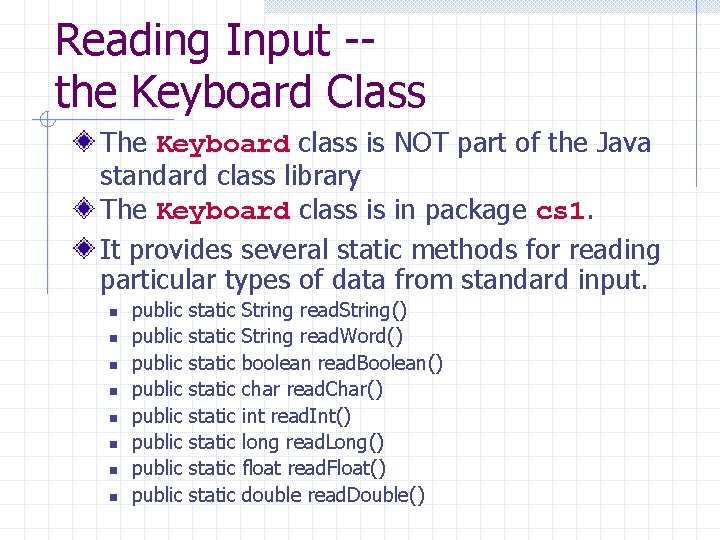
Reading Input -the Keyboard Class The Keyboard class is NOT part of the Java standard class library The Keyboard class is in package cs 1. It provides several static methods for reading particular types of data from standard input. n n n n public public static static String read. String() String read. Word() boolean read. Boolean() char read. Char() int read. Int() long read. Long() float read. Float() double read. Double()
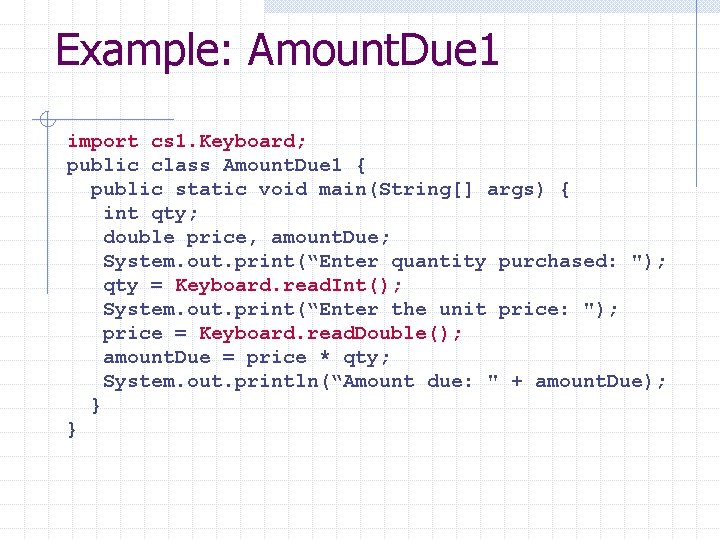
Example: Amount. Due 1 import cs 1. Keyboard; public class Amount. Due 1 { public static void main(String[] args) { int qty; double price, amount. Due; System. out. print(“Enter quantity purchased: "); qty = Keyboard. read. Int(); System. out. print(“Enter the unit price: "); price = Keyboard. read. Double(); amount. Due = price * qty; System. out. println(“Amount due: " + amount. Due); } }
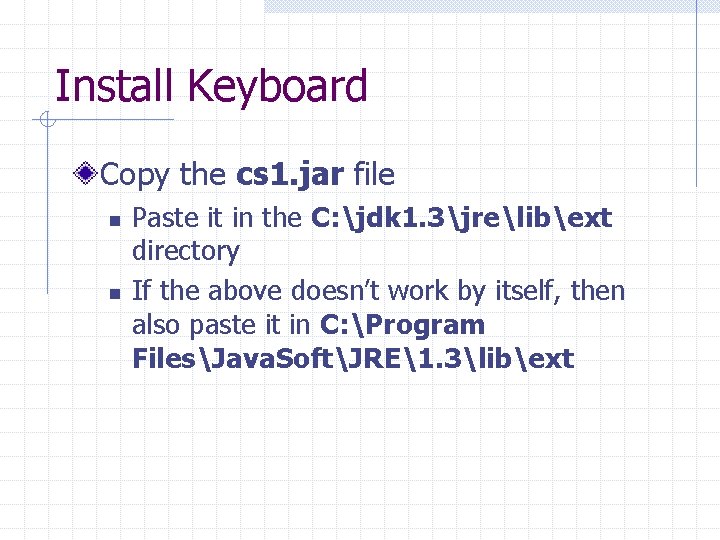
Install Keyboard Copy the cs 1. jar file n n Paste it in the C: jdk 1. 3jrelibext directory If the above doesn’t work by itself, then also paste it in C: Program FilesJava. SoftJRE1. 3libext
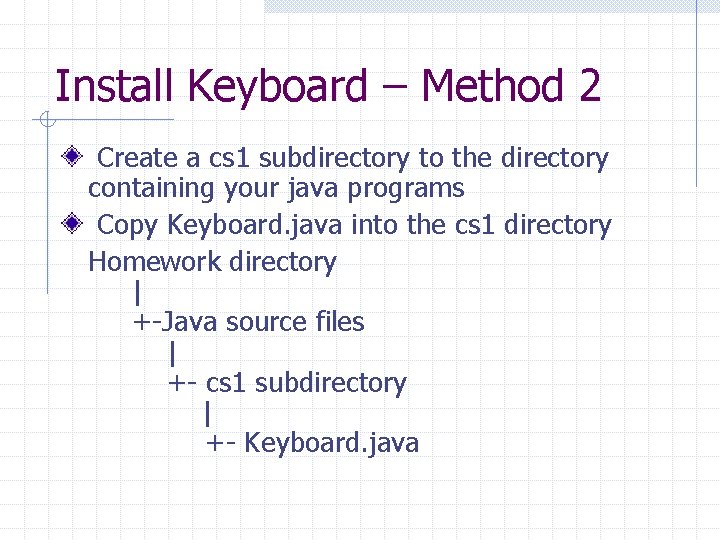
Install Keyboard – Method 2 Create a cs 1 subdirectory to the directory containing your java programs Copy Keyboard. java into the cs 1 directory Homework directory | +-Java source files | +- cs 1 subdirectory | +- Keyboard. java
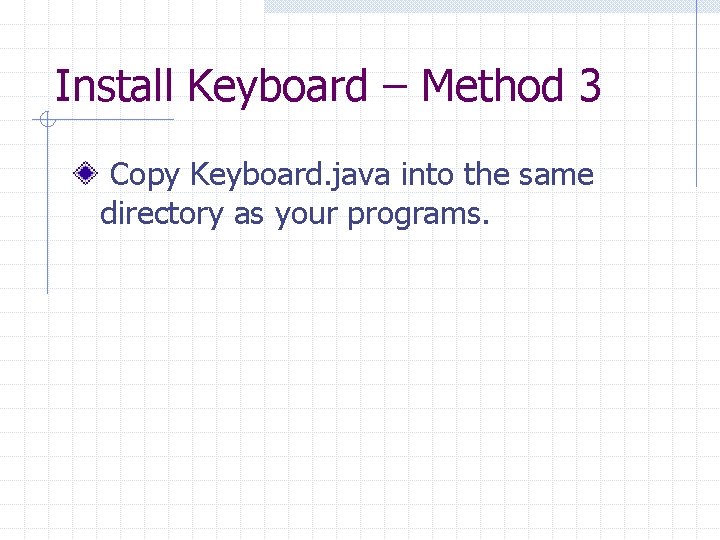
Install Keyboard – Method 3 Copy Keyboard. java into the same directory as your programs.
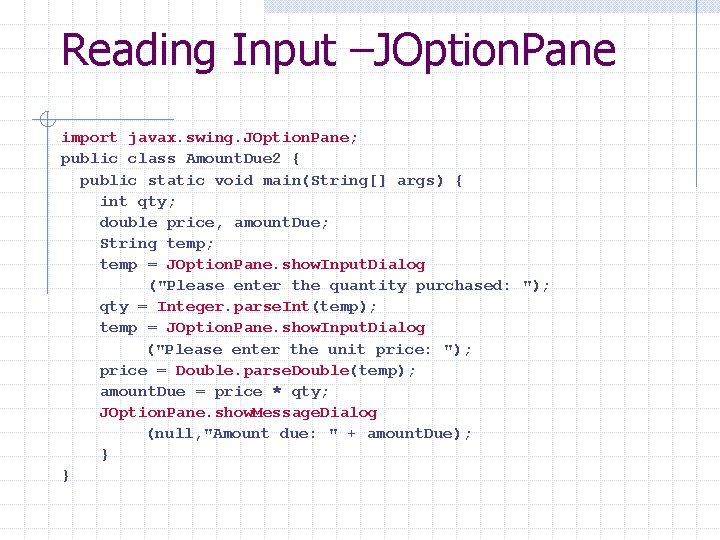
Reading Input –JOption. Pane import javax. swing. JOption. Pane; public class Amount. Due 2 { public static void main(String[] args) { int qty; double price, amount. Due; String temp; temp = JOption. Pane. show. Input. Dialog ("Please enter the quantity purchased: "); qty = Integer. parse. Int(temp); temp = JOption. Pane. show. Input. Dialog ("Please enter the unit price: "); price = Double. parse. Double(temp); amount. Due = price * qty; JOption. Pane. show. Message. Dialog (null, "Amount due: " + amount. Due); } }
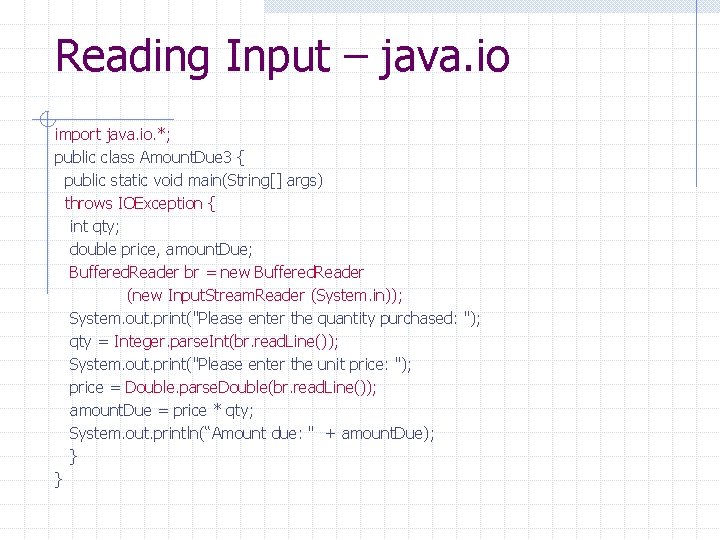
Reading Input – java. io import java. io. *; public class Amount. Due 3 { public static void main(String[] args) throws IOException { int qty; double price, amount. Due; Buffered. Reader br = new Buffered. Reader (new Input. Stream. Reader (System. in)); System. out. print("Please enter the quantity purchased: "); qty = Integer. parse. Int(br. read. Line()); System. out. print("Please enter the unit price: "); price = Double. parse. Double(br. read. Line()); amount. Due = price * qty; System. out. println(“Amount due: " + amount. Due); } }
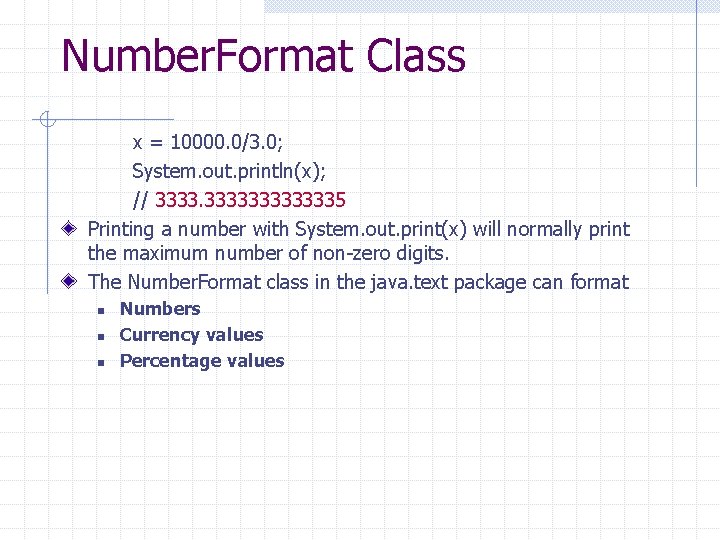
Number. Format Class x = 10000. 0/3. 0; System. out. println(x); // 3333335 Printing a number with System. out. print(x) will normally print the maximum number of non-zero digits. The Number. Format class in the java. text package can format n n n Numbers Currency values Percentage values
![Number Format import java text public class Formatted Output public static void mainString Number Format import java. text. *; public class Formatted. Output{ public static void main(String[]](https://slidetodoc.com/presentation_image_h2/af18d6e3bfe53baf24f33d0018393672/image-31.jpg)
Number Format import java. text. *; public class Formatted. Output{ public static void main(String[] args) { int qty; double price, amount. Due; double x = 10000. 0/3. 0; System. out. println("Default output is t" + x); // 3333335 Number. Format num_format = Number. Format. get. Number. Instance(); String s = num_format(x); System. out. println("Number format is t" + s); // 3, 333 Number. Format cur_format = Number. Format. get. Currency. Instance(); s = cur_format(x); System. out. println("Currency format is t" + s); //$3, 333. 33 Number. Format per_format = Number. Format. get. Percent. Instance(); s = per_format(x); System. out. println("Percent format is t" + s); // 333, 333% } }
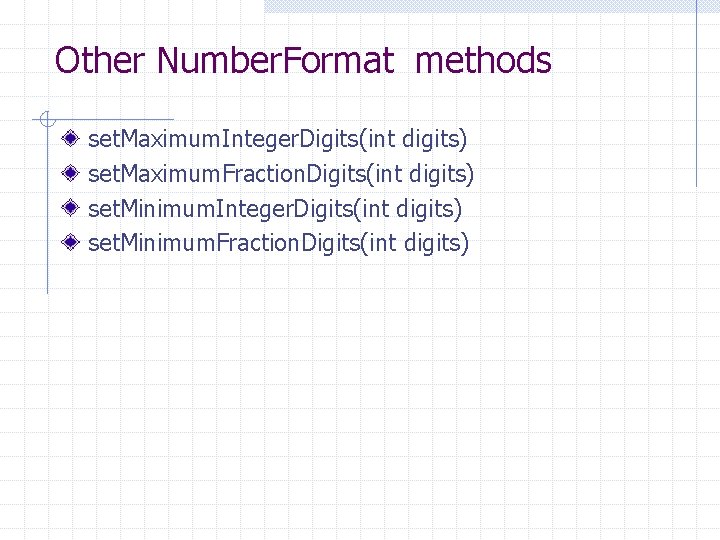
Other Number. Format methods set. Maximum. Integer. Digits(int digits) set. Maximum. Fraction. Digits(int digits) set. Minimum. Integer. Digits(int digits) set. Minimum. Fraction. Digits(int digits)
Pstat.exe
Greek and roman art similarities
Relational algebra and relational calculus
Relational calculus
Relational calculus symbols
Object relational and extended relational databases
Relational algebra is a procedural language
An expression with less strength than expected
Relational algebra expression tree
Increment operator in c
Relational expression
Relational expression
Expression tree relational algebra
Less than equal to graph
Types of royalty account
Fractions greater less than or equal to
Less than keywords
Linear term
Sudden and violent but brief; fitful; intermittent
Nonmetal period 3 mass 32
Equal height equal light
Taku komura
Equal sharing and equal grouping is known as
Opposite angles
Meridionalnet
And gate truth table
If not equal matlab
Matlab
"real system"
What is glycemic load
Perl not equal operator
The variable was not initialized flowgorithm
All multimode modems are not created equal