Programao Funcional Estilo de Programao Figuras etc em
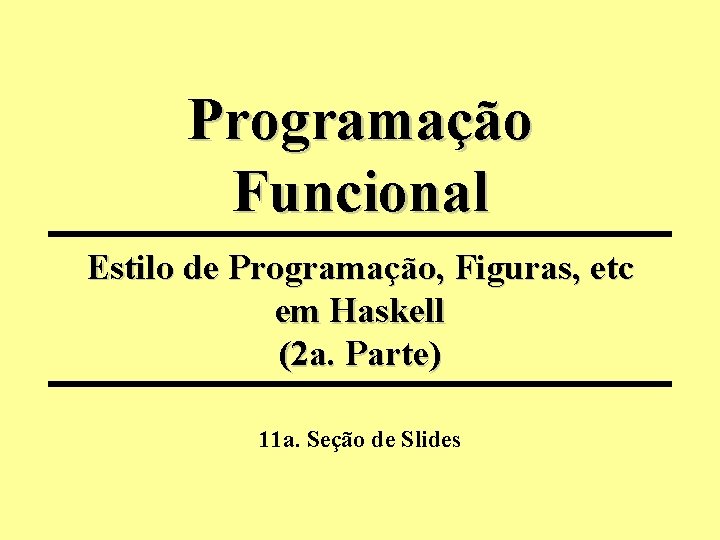
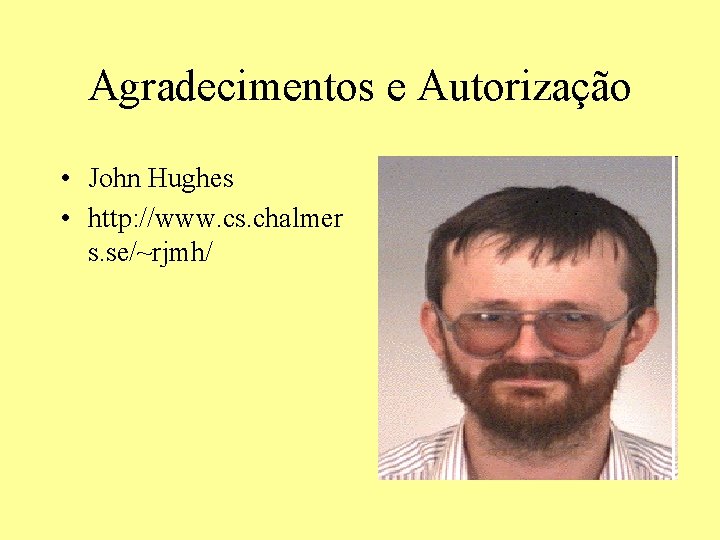
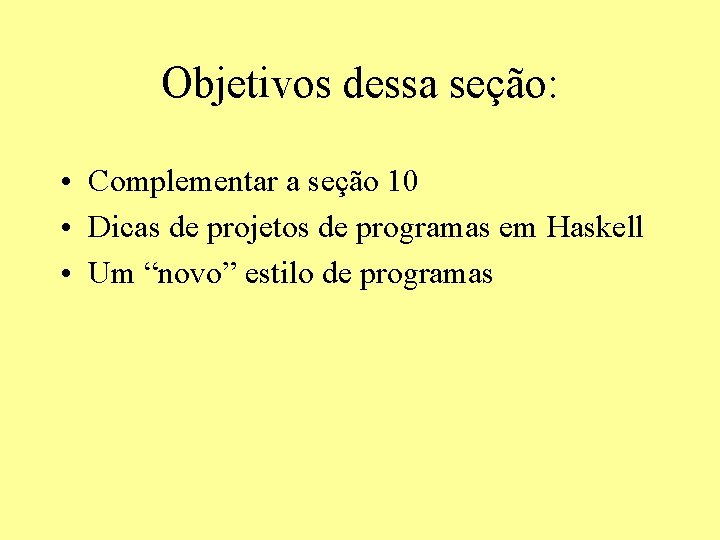
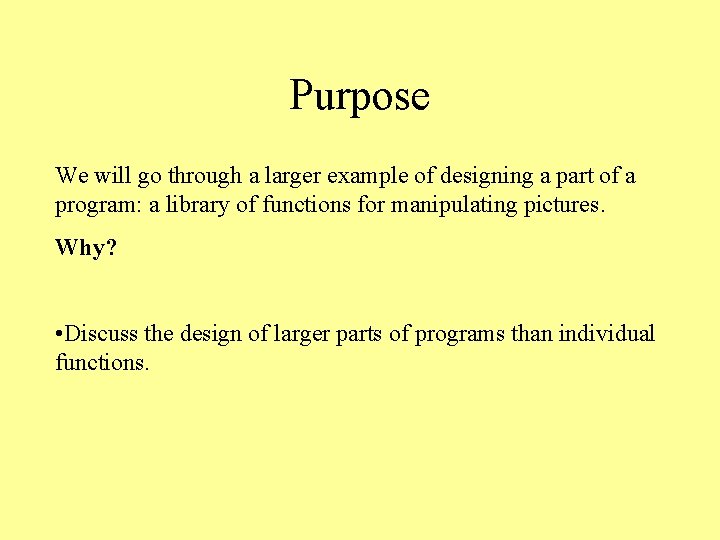
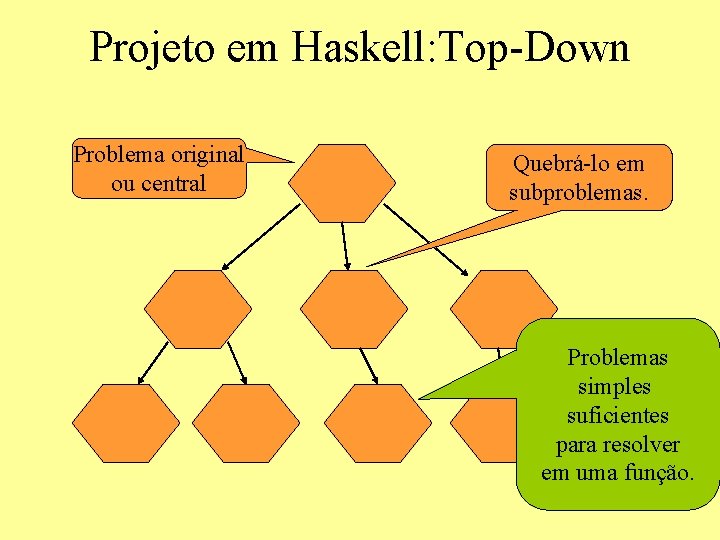
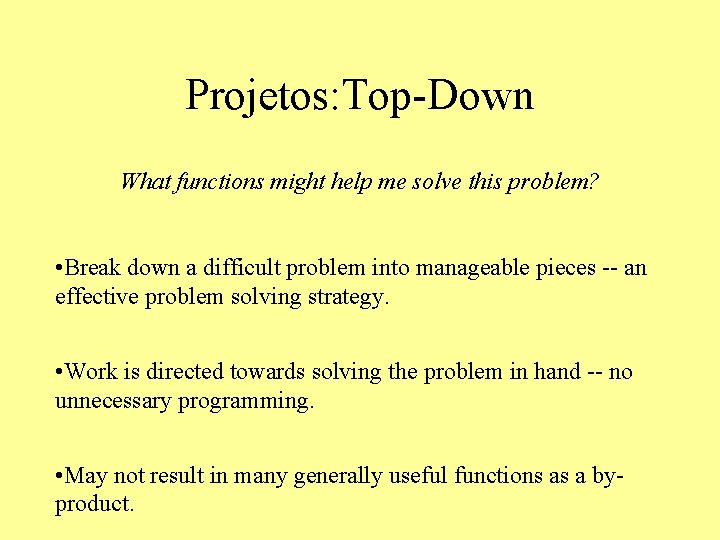
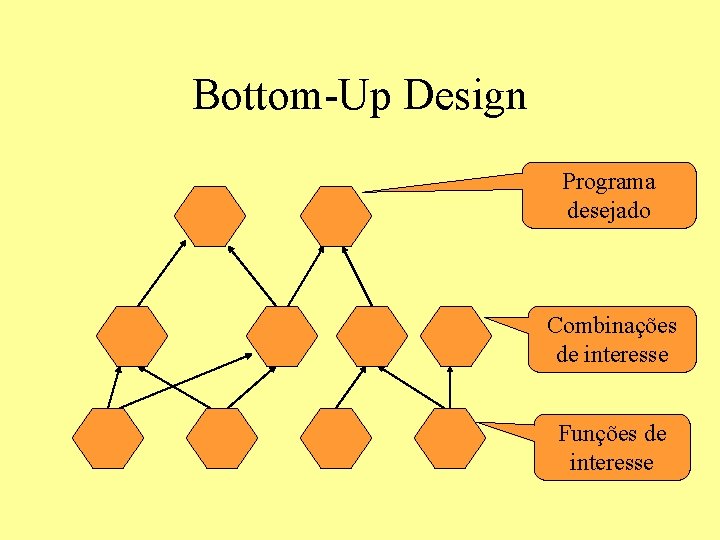
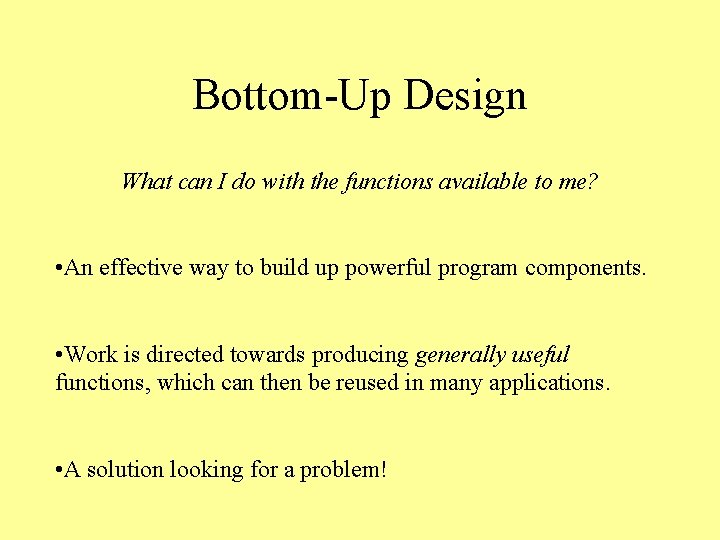
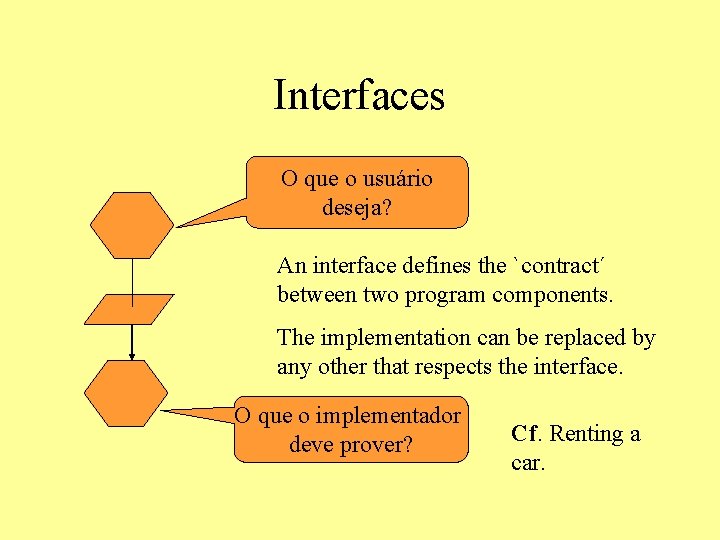
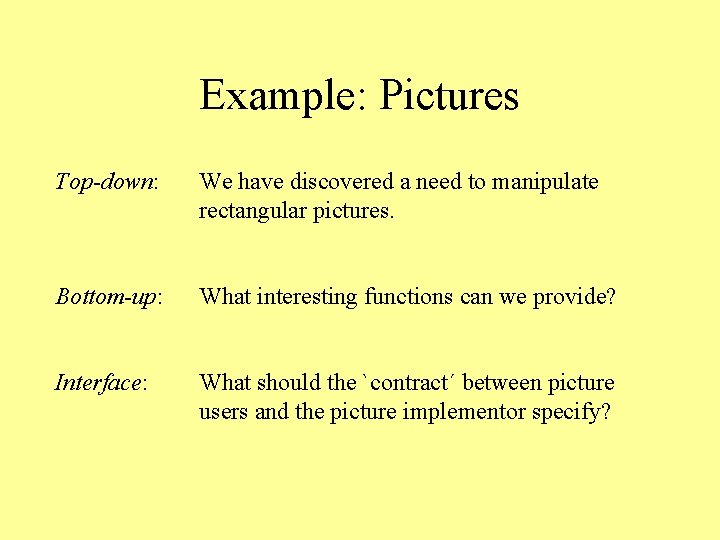
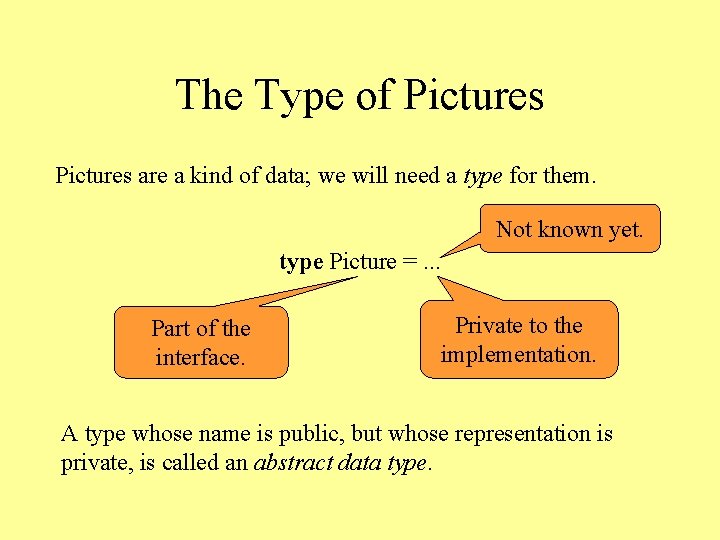
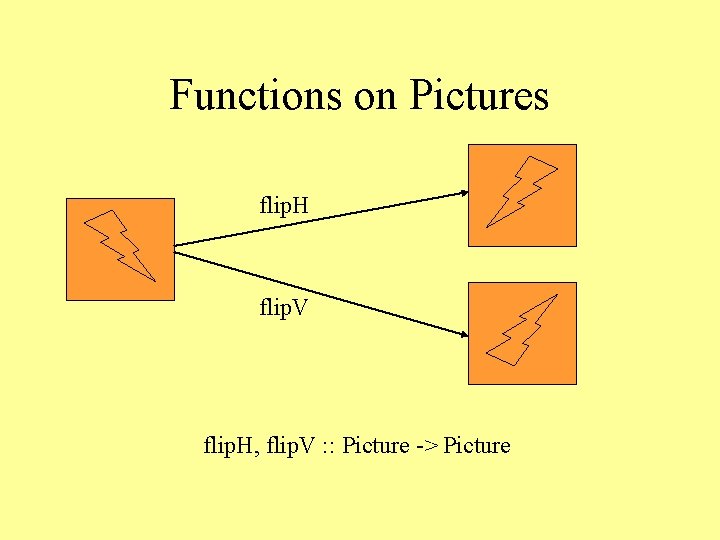
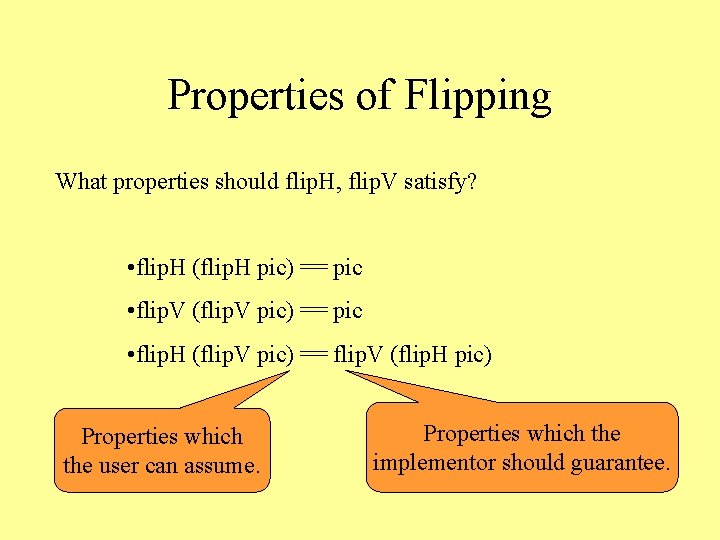
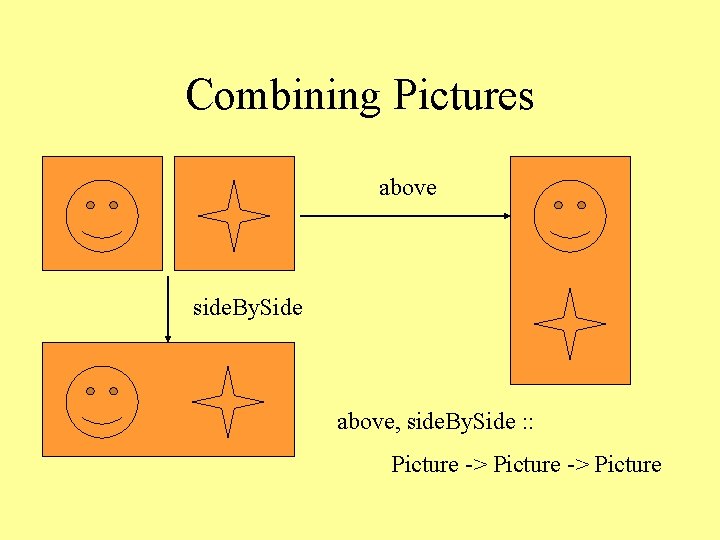
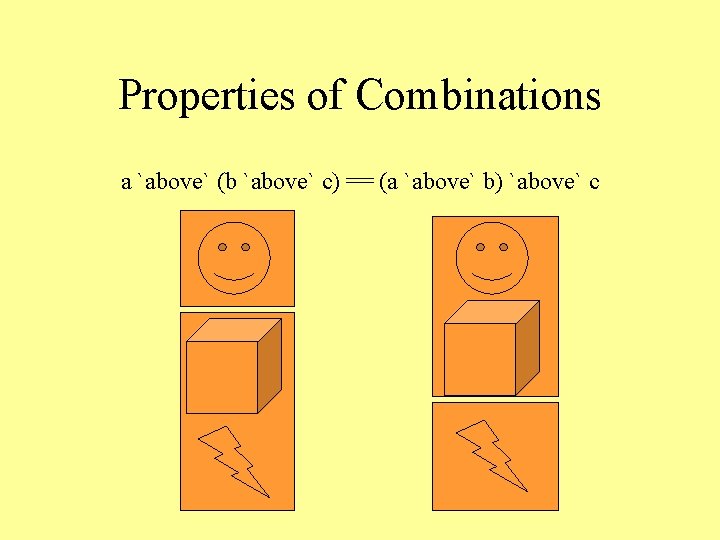
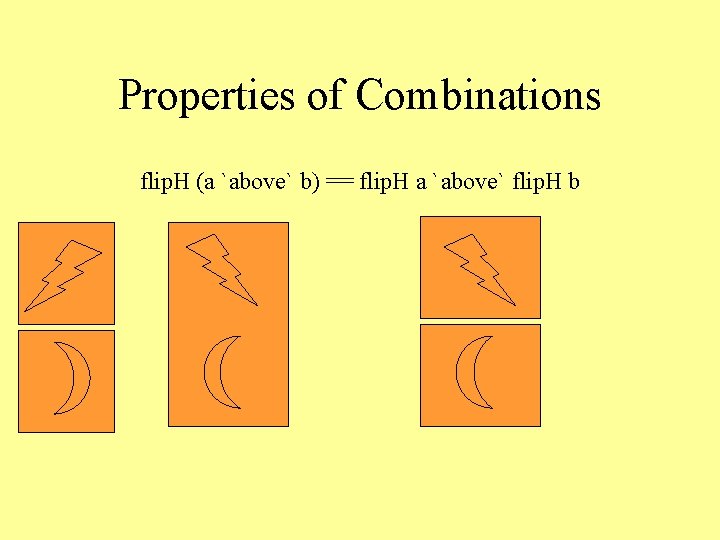
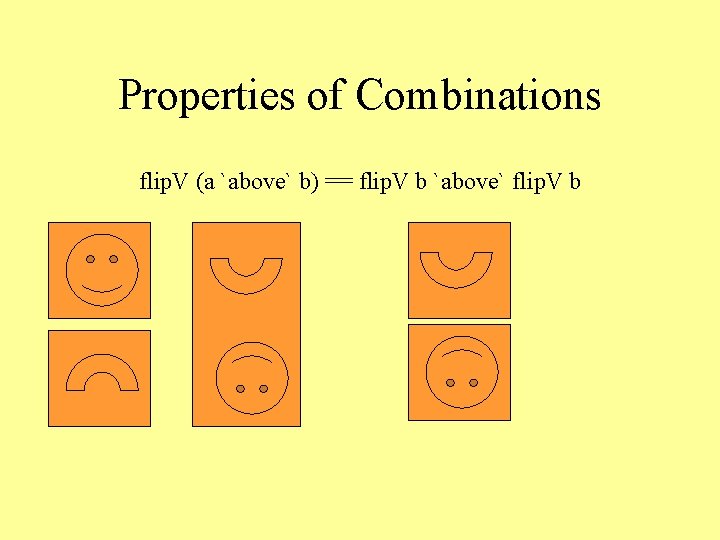
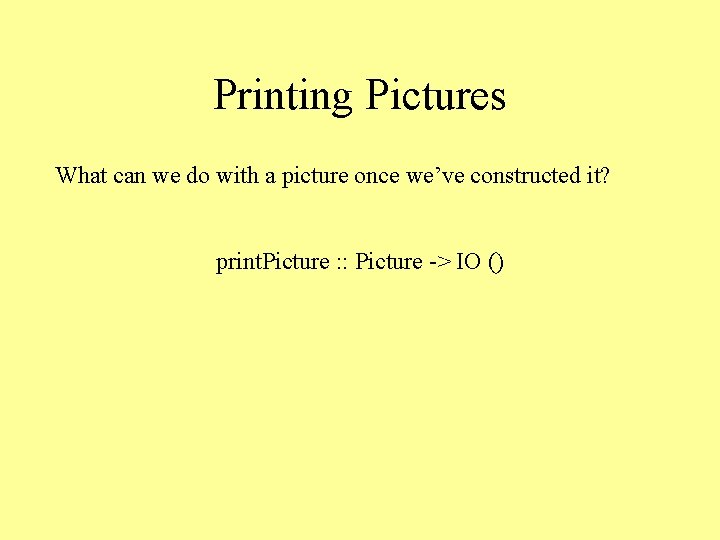
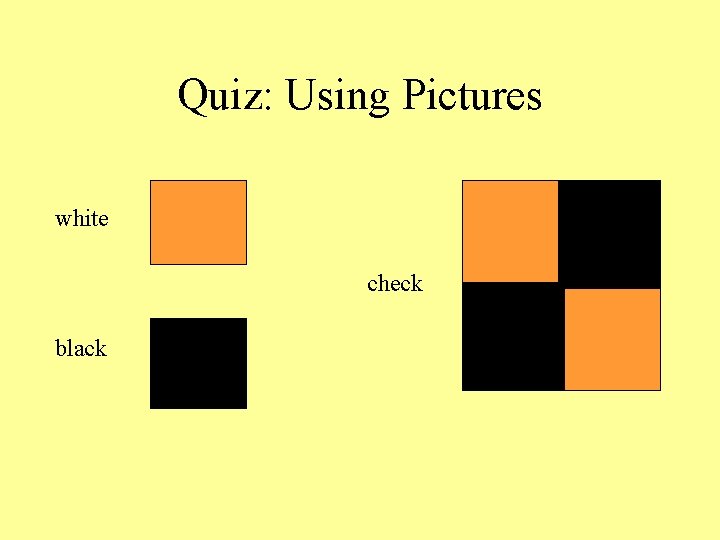
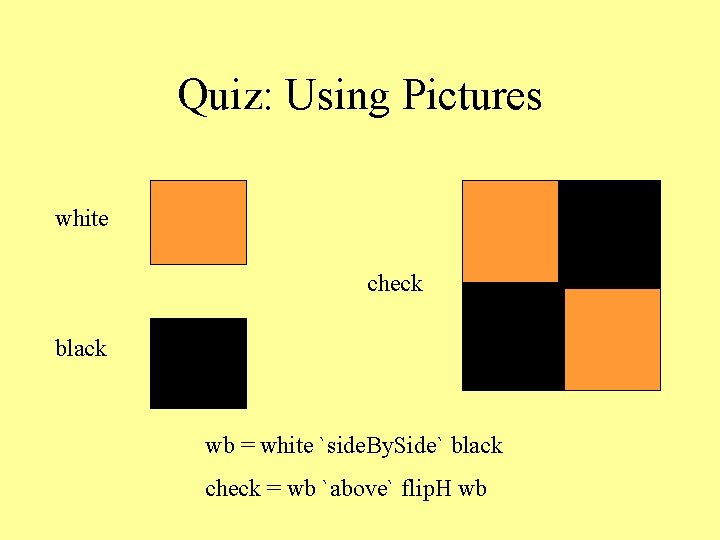
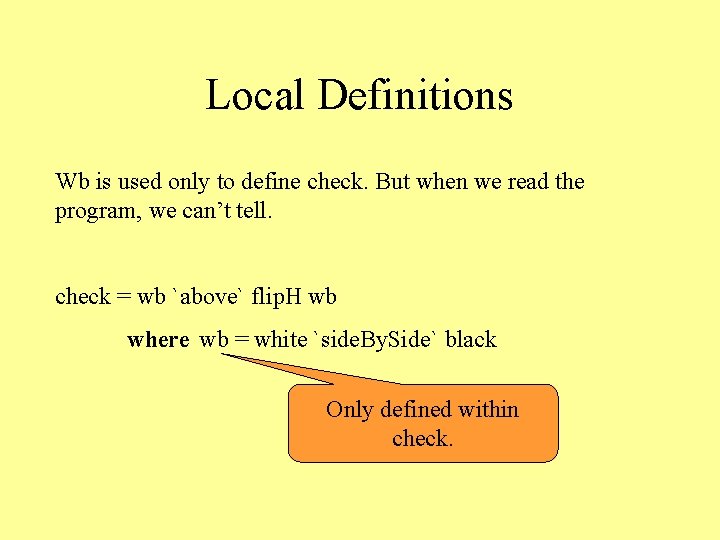
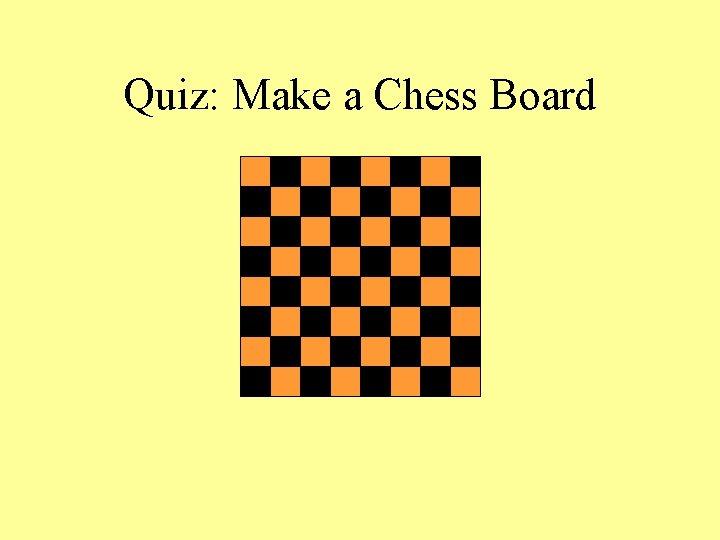
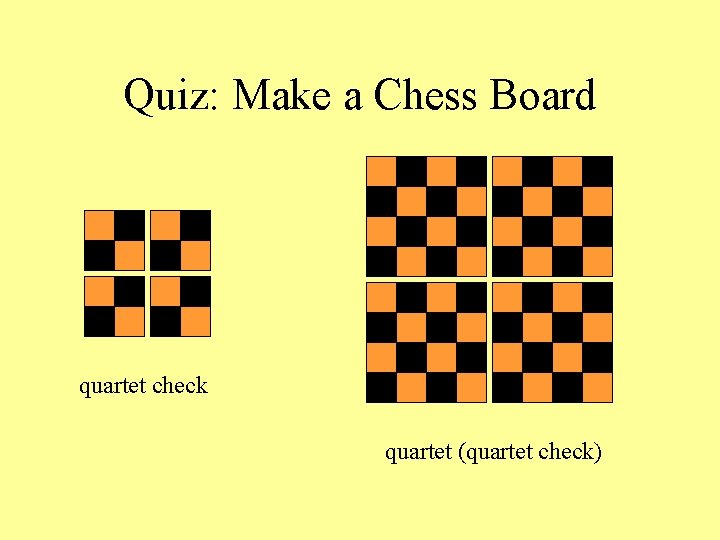
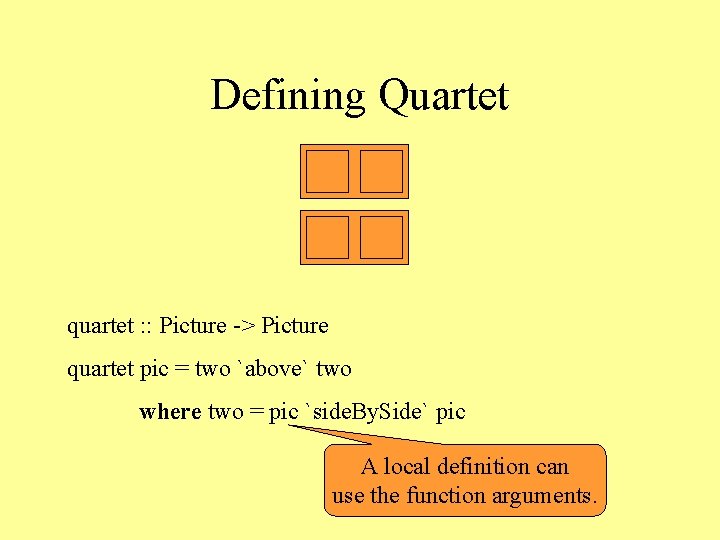
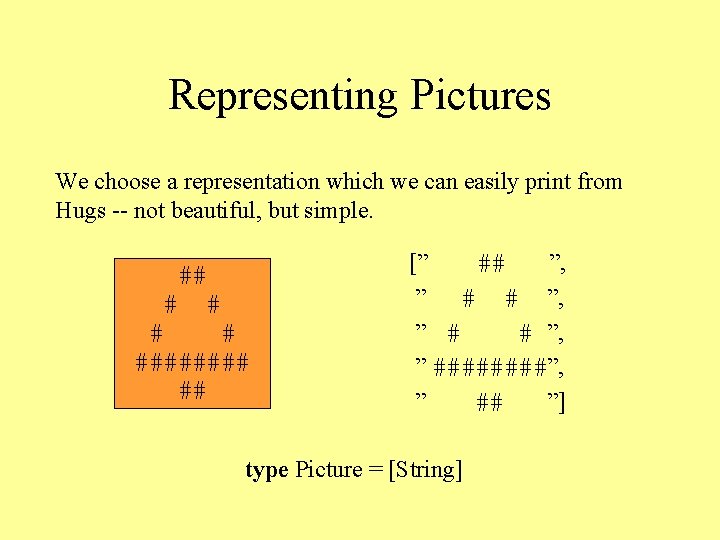
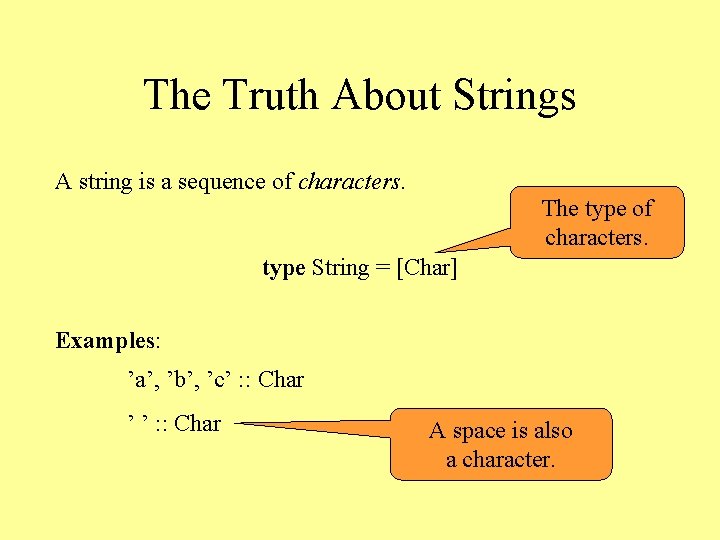
![Flipping Vertically flip. V [” ## ”, ” ####”, ” ## ”] flip. V Flipping Vertically flip. V [” ## ”, ” ####”, ” ## ”] flip. V](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-27.jpg)
![Quiz: Flipping Horizontally flip. H Recall: [” # ”, ”######”, ” # ”] Picture Quiz: Flipping Horizontally flip. H Recall: [” # ”, ”######”, ” # ”] Picture](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-28.jpg)
![Quiz: Flipping Horizontally flip. H [” # ”, ”######”, ” # ”] flip. H Quiz: Flipping Horizontally flip. H [” # ”, ”######”, ” # ”] flip. H](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-29.jpg)
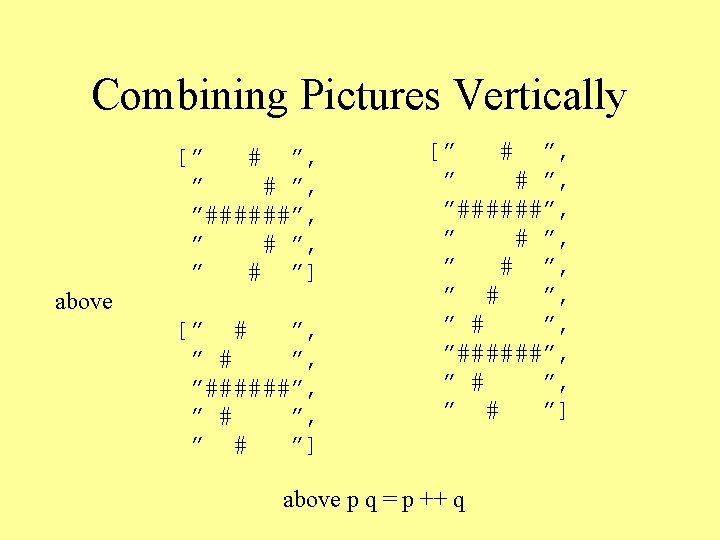
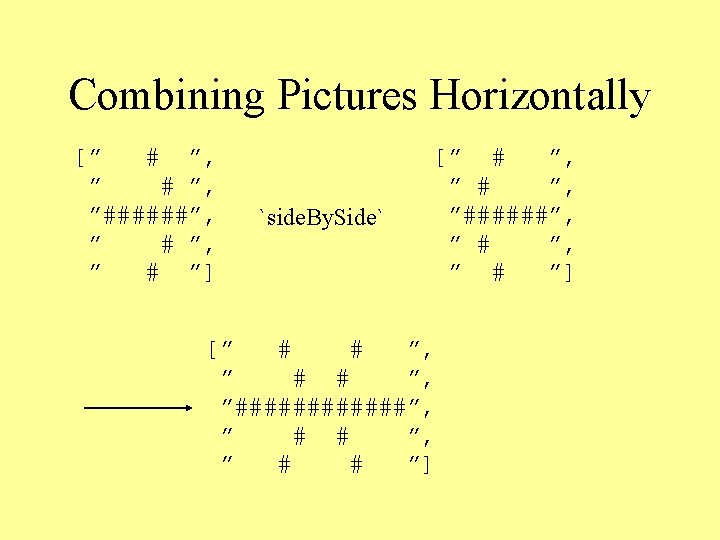
![Combining Pictures Horizontally [” # ”, ”######”, ” # ”] `side. By. Side` [” Combining Pictures Horizontally [” # ”, ”######”, ” # ”] `side. By. Side` [”](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-32.jpg)
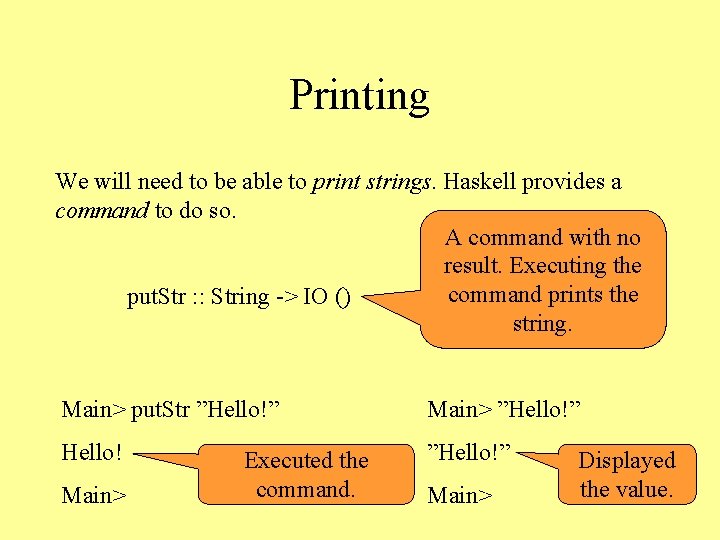
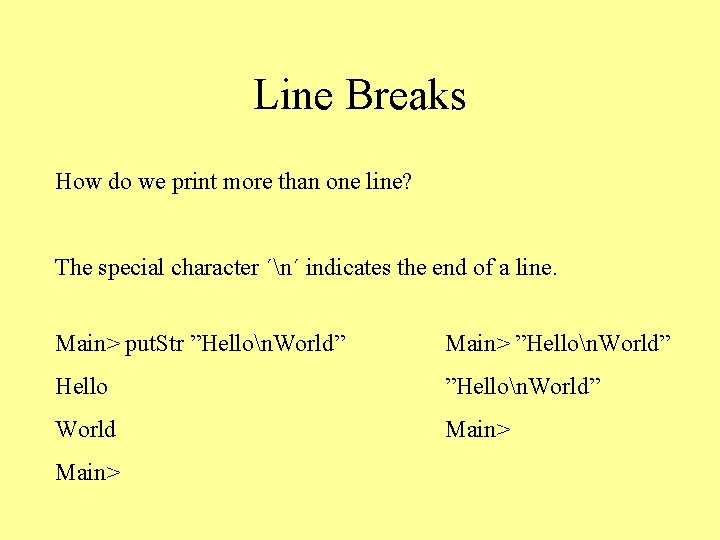
![Printing a Picture Print [”###”, ”###”] as ”###n###n” A common operation, so there is Printing a Picture Print [”###”, ”###”] as ”###n###n” A common operation, so there is](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-35.jpg)
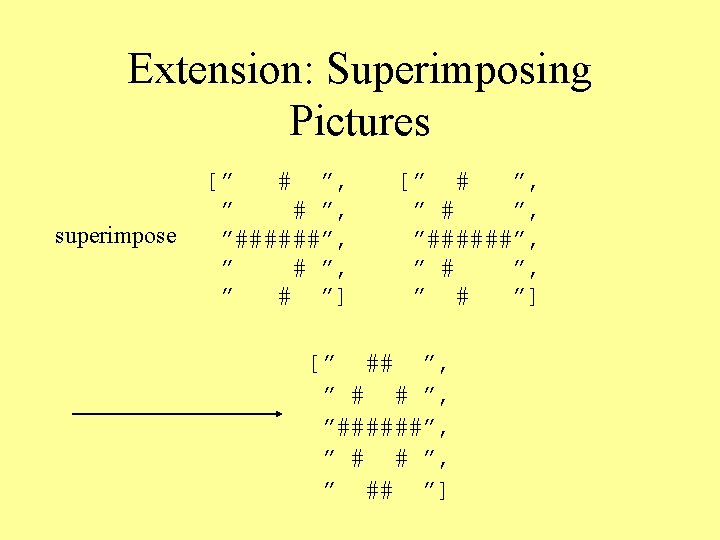
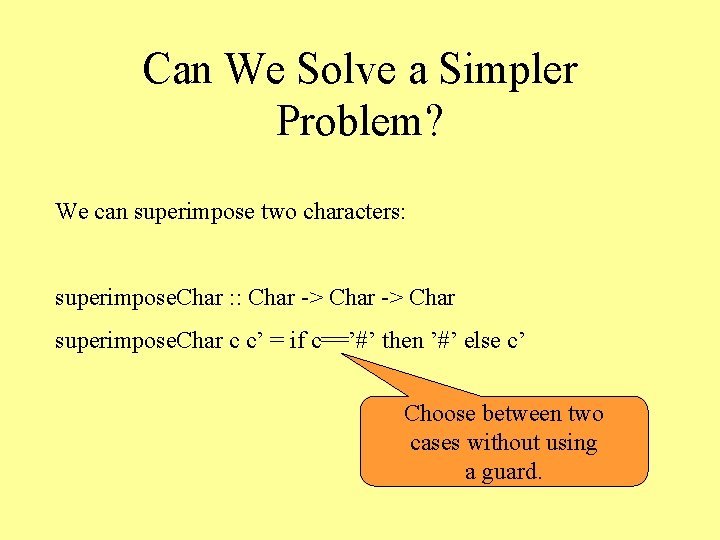
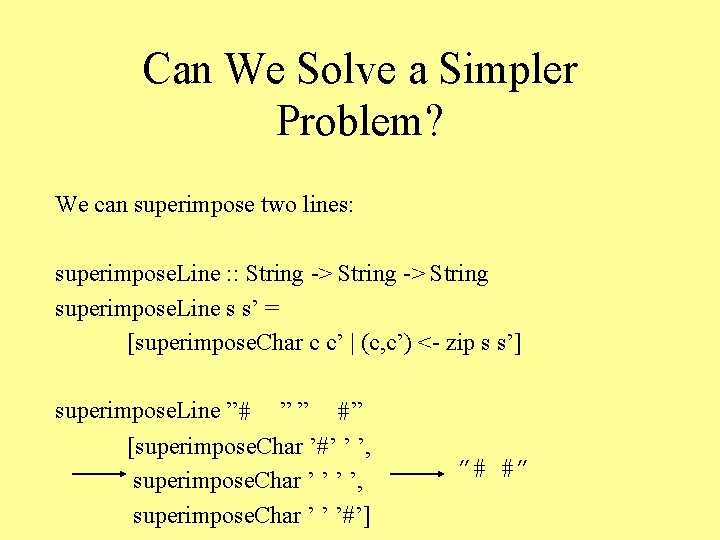
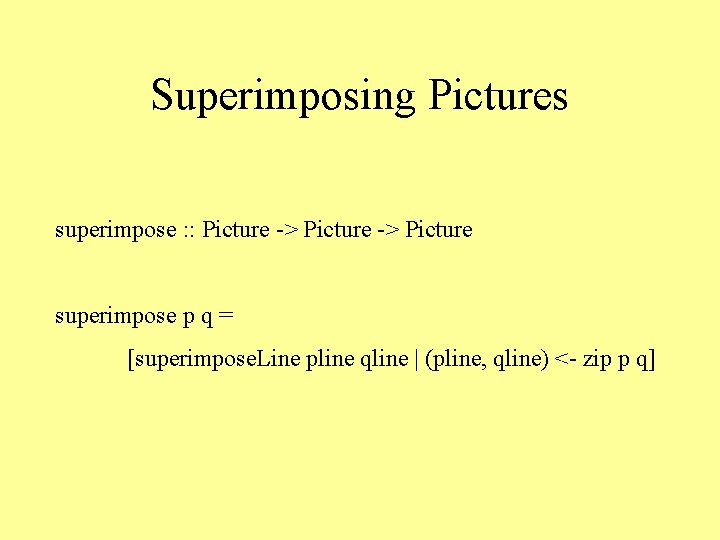
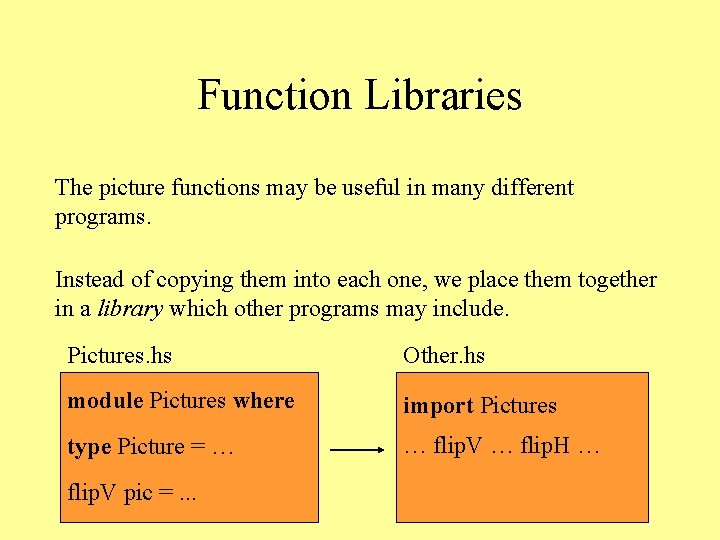
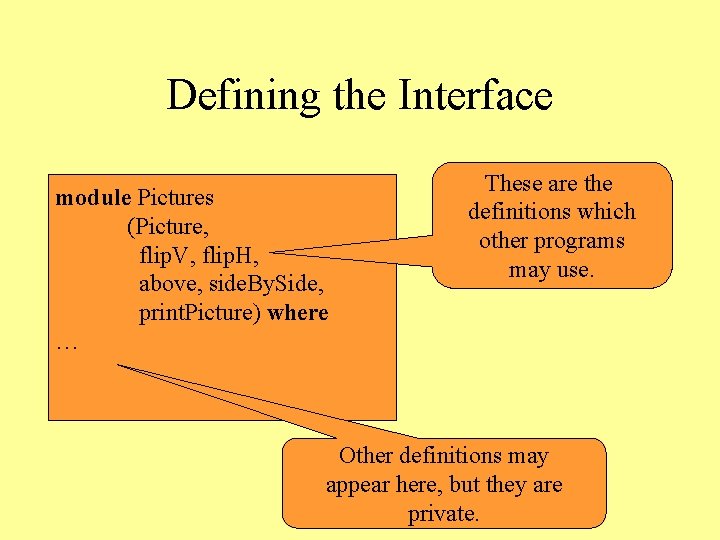
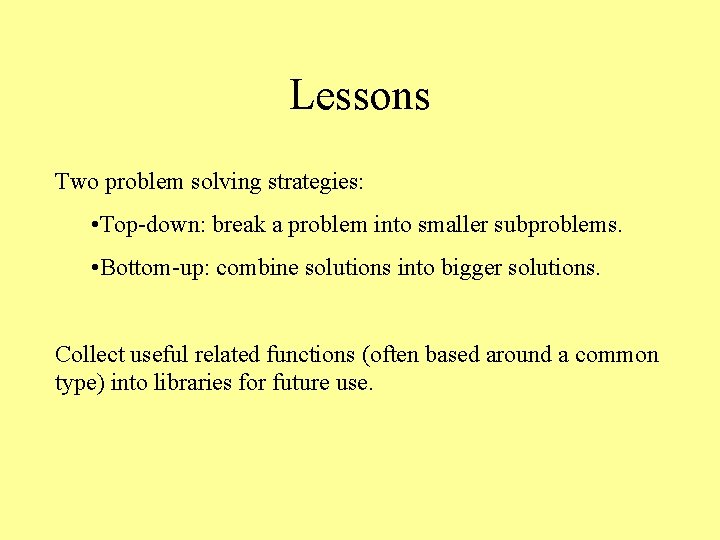
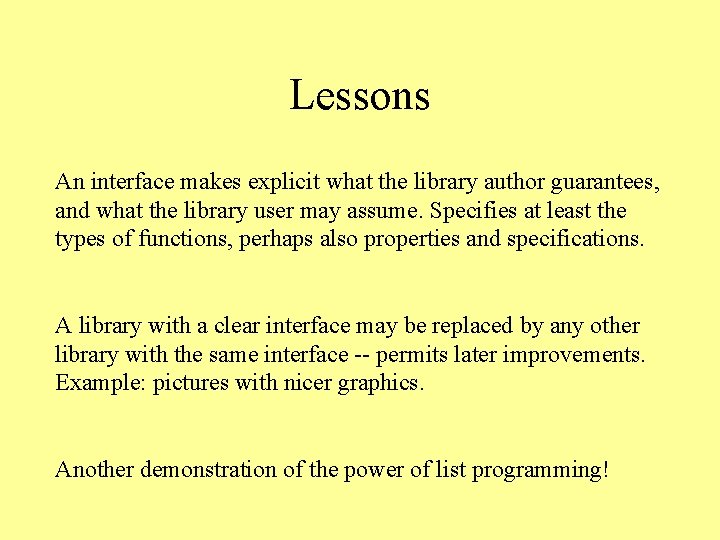
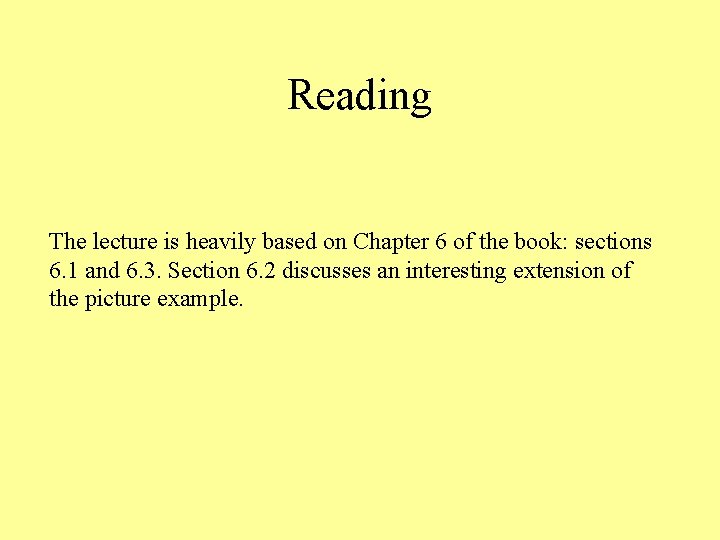
- Slides: 44
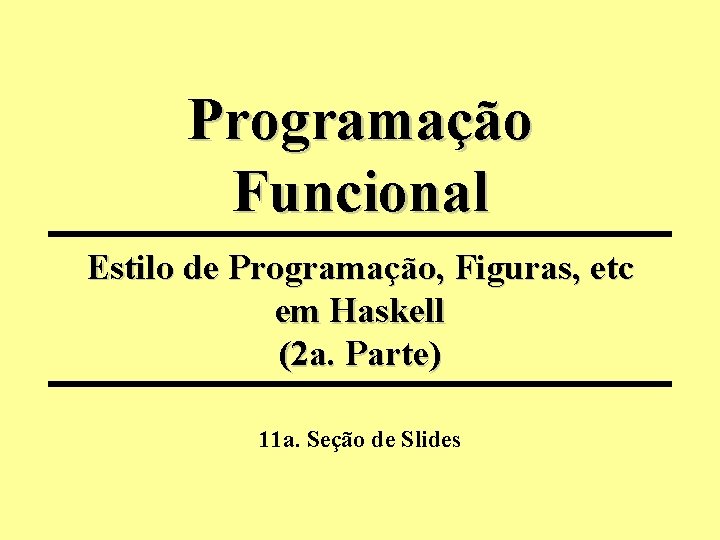
Programação Funcional Estilo de Programação, Figuras, etc em Haskell (2 a. Parte) 11 a. Seção de Slides
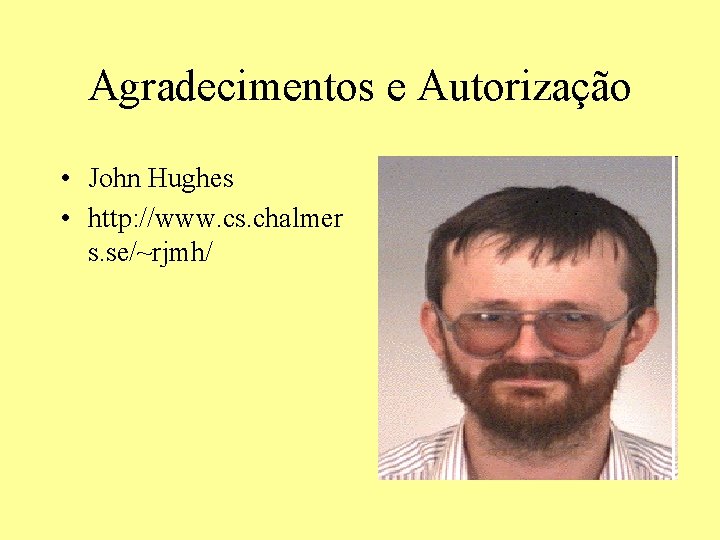
Agradecimentos e Autorização • John Hughes • http: //www. cs. chalmer s. se/~rjmh/
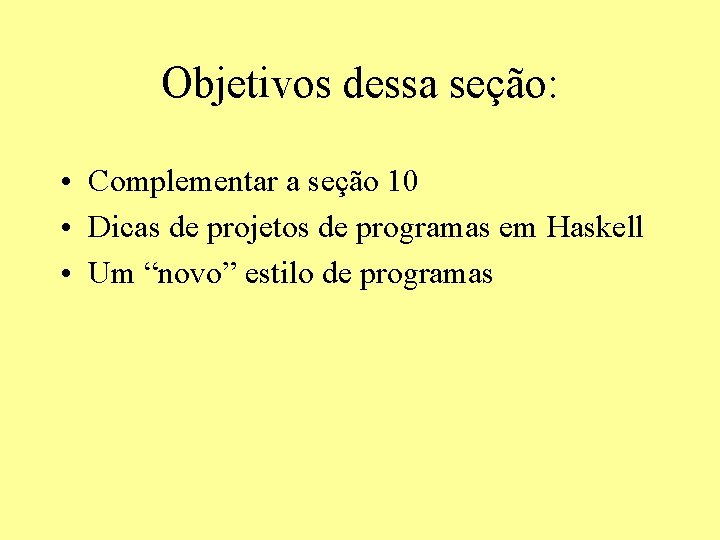
Objetivos dessa seção: • Complementar a seção 10 • Dicas de projetos de programas em Haskell • Um “novo” estilo de programas
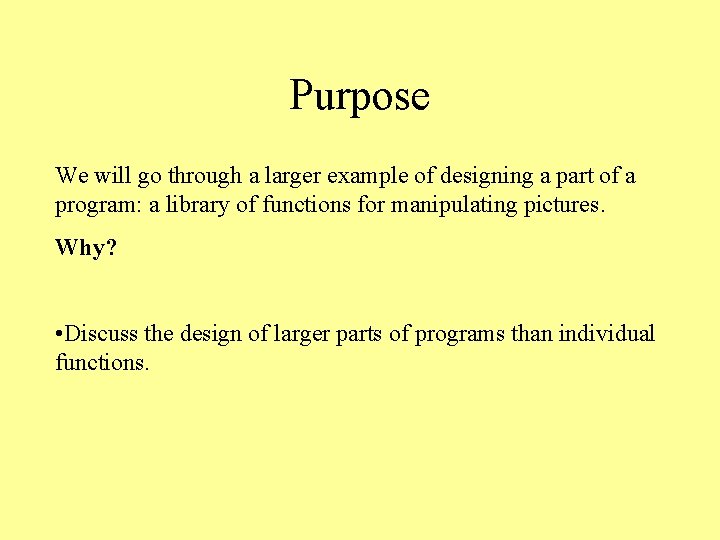
Purpose We will go through a larger example of designing a part of a program: a library of functions for manipulating pictures. Why? • Discuss the design of larger parts of programs than individual functions.
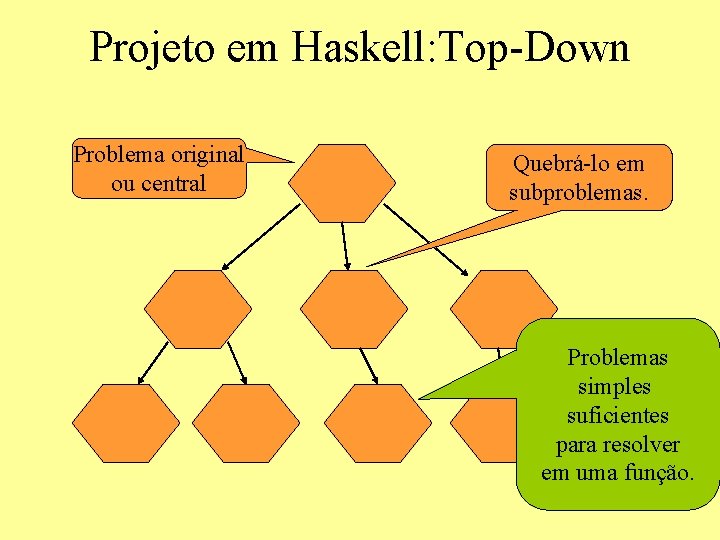
Projeto em Haskell: Top-Down Problema original ou central Quebrá-lo em subproblemas. Problemas simples suficientes para resolver em uma função.
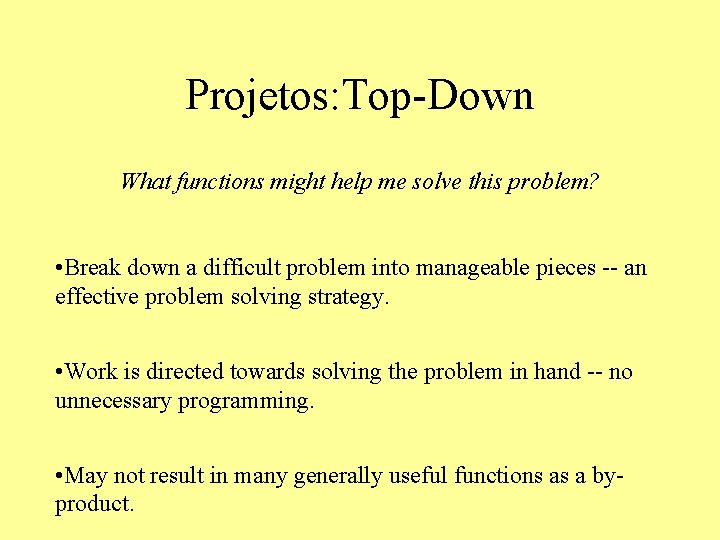
Projetos: Top-Down What functions might help me solve this problem? • Break down a difficult problem into manageable pieces -- an effective problem solving strategy. • Work is directed towards solving the problem in hand -- no unnecessary programming. • May not result in many generally useful functions as a byproduct.
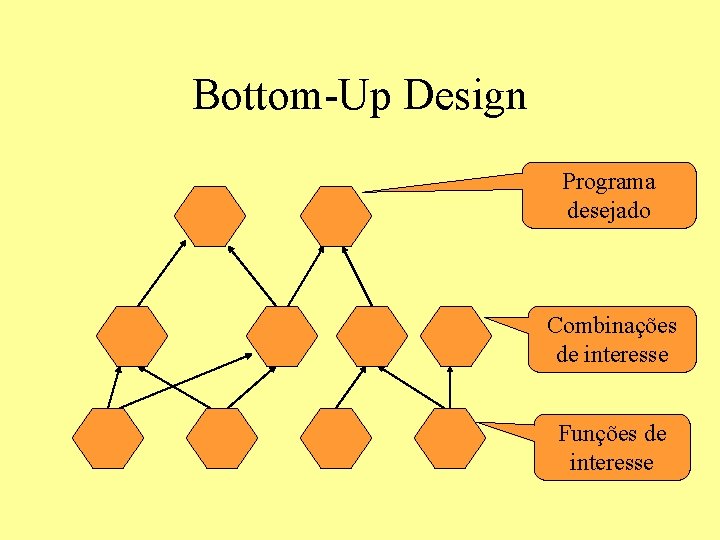
Bottom-Up Design Programa desejado Combinações de interesse Funções de interesse
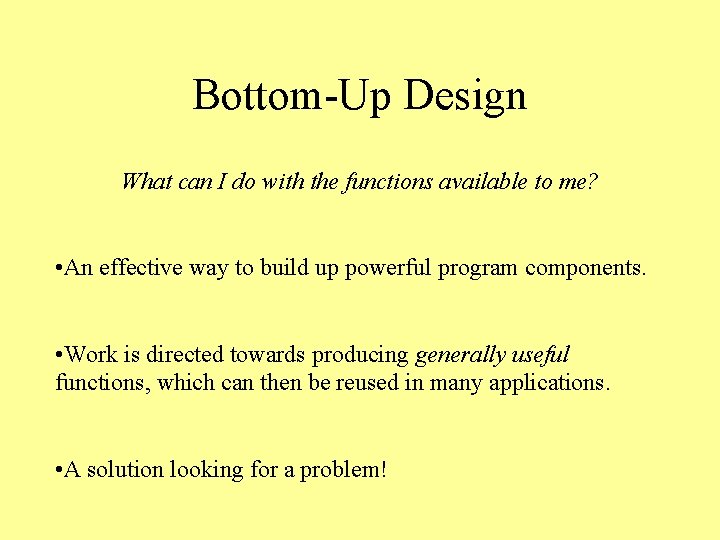
Bottom-Up Design What can I do with the functions available to me? • An effective way to build up powerful program components. • Work is directed towards producing generally useful functions, which can then be reused in many applications. • A solution looking for a problem!
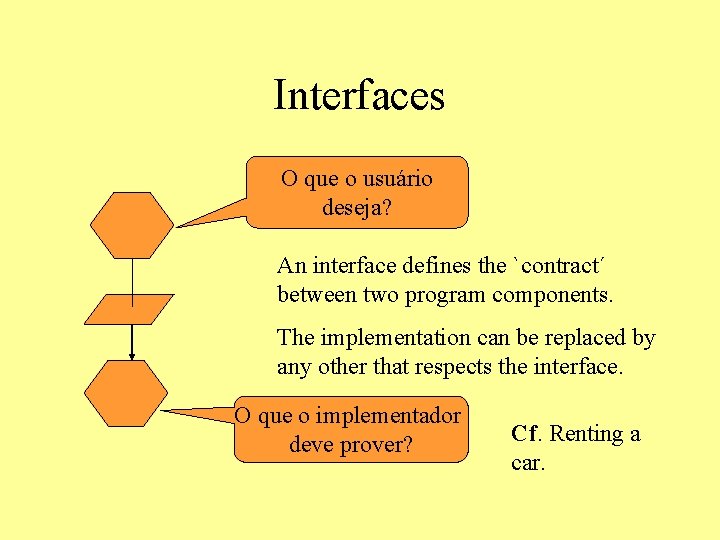
Interfaces O que o usuário deseja? An interface defines the `contract´ between two program components. The implementation can be replaced by any other that respects the interface. O que o implementador deve prover? Cf. Renting a car.
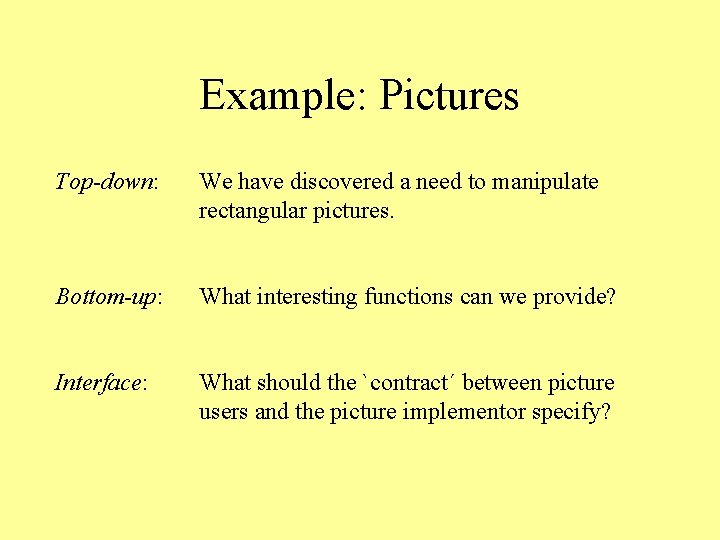
Example: Pictures Top-down: We have discovered a need to manipulate rectangular pictures. Bottom-up: What interesting functions can we provide? Interface: What should the `contract´ between picture users and the picture implementor specify?
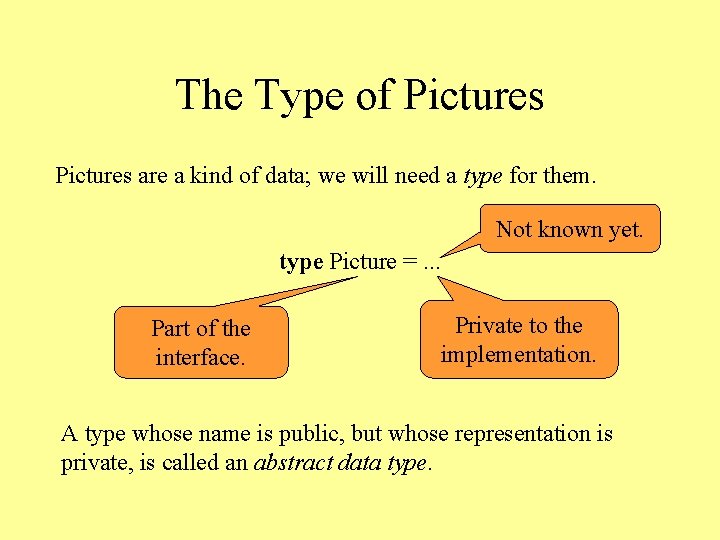
The Type of Pictures are a kind of data; we will need a type for them. Not known yet. type Picture =. . . Part of the interface. Private to the implementation. A type whose name is public, but whose representation is private, is called an abstract data type.
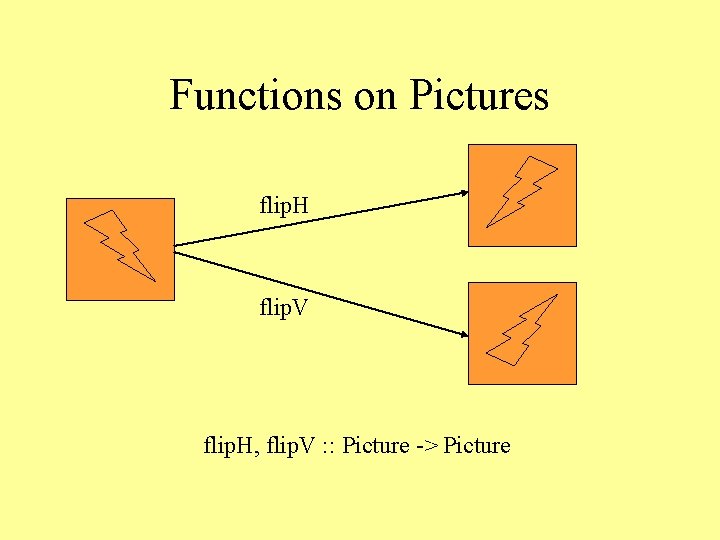
Functions on Pictures flip. H flip. V flip. H, flip. V : : Picture -> Picture
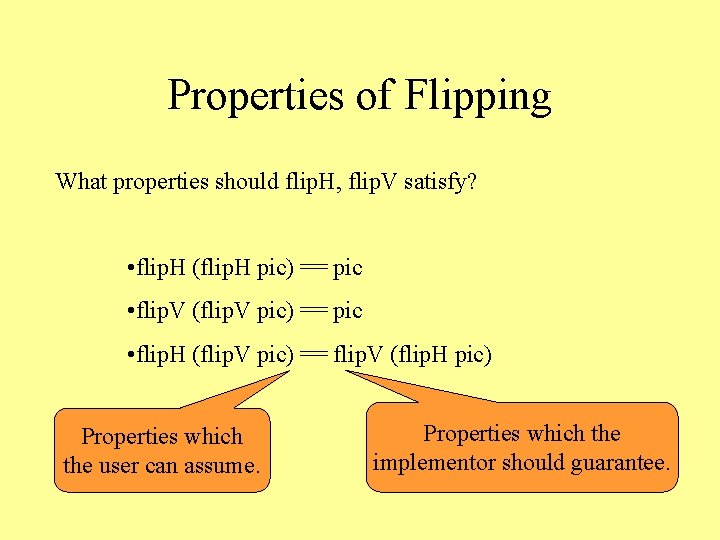
Properties of Flipping What properties should flip. H, flip. V satisfy? • flip. H (flip. H pic) == pic • flip. V (flip. V pic) == pic • flip. H (flip. V pic) == flip. V (flip. H pic) Properties which the user can assume. Properties which the implementor should guarantee.
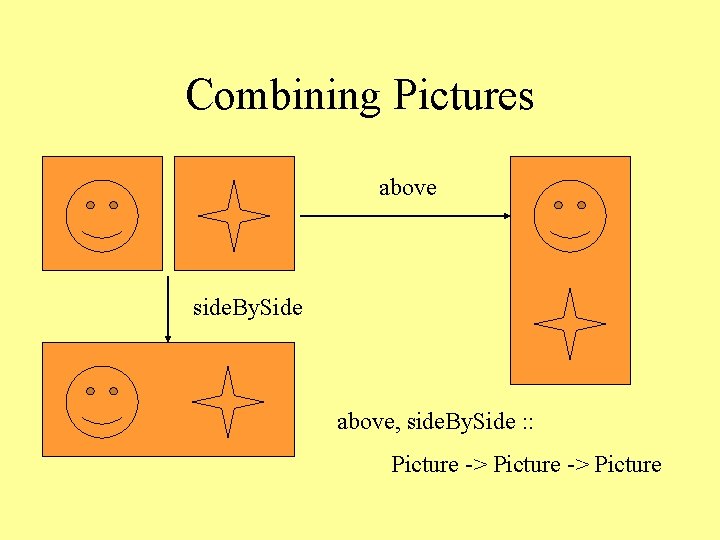
Combining Pictures above side. By. Side above, side. By. Side : : Picture -> Picture
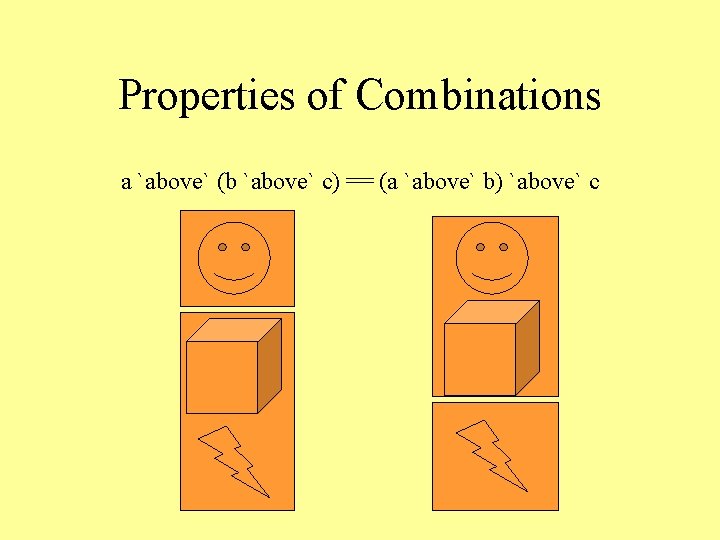
Properties of Combinations a `above` (b `above` c) == (a `above` b) `above` c
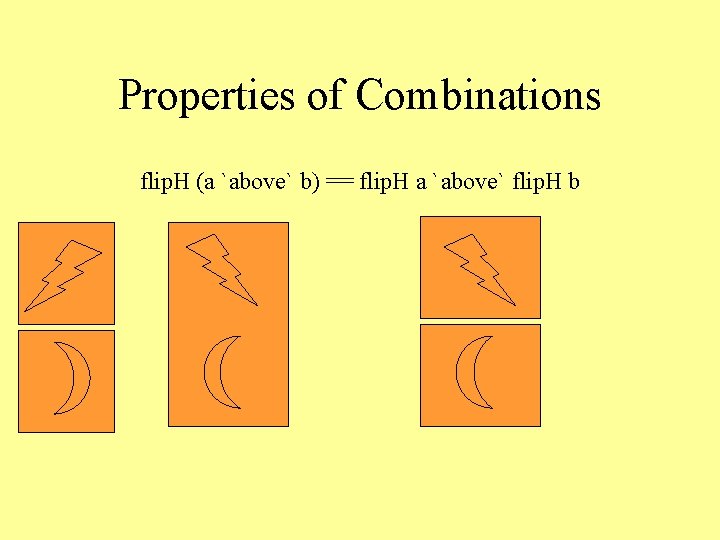
Properties of Combinations flip. H (a `above` b) == flip. H a `above` flip. H b
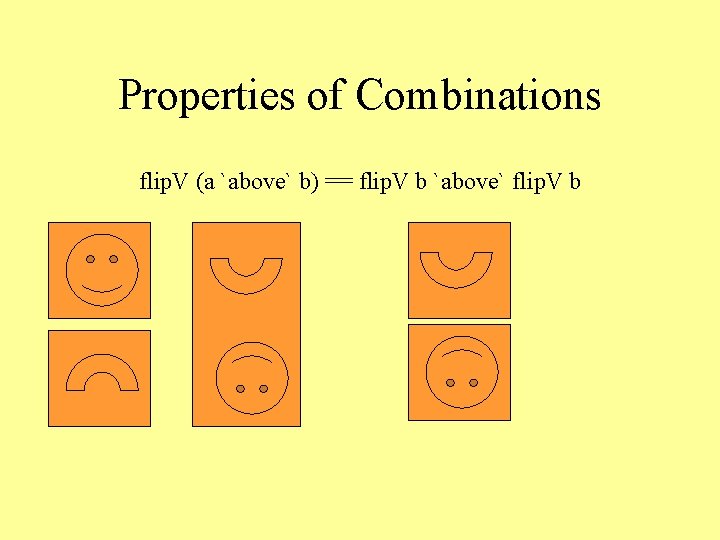
Properties of Combinations flip. V (a `above` b) == flip. V b `above` flip. V b
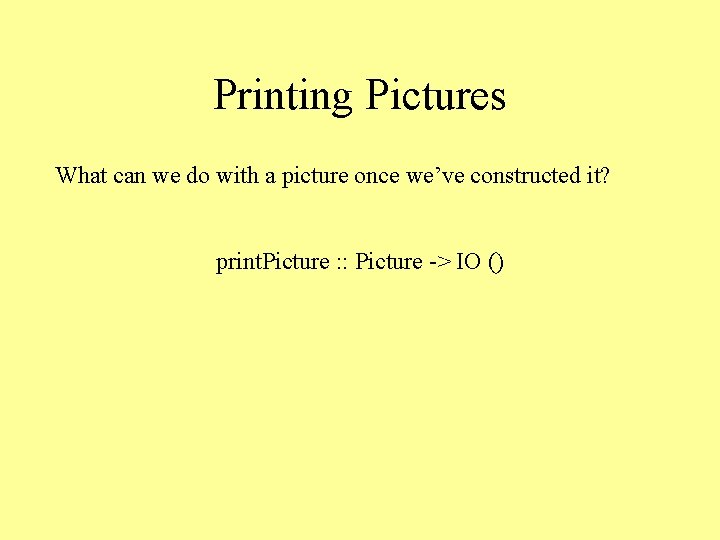
Printing Pictures What can we do with a picture once we’ve constructed it? print. Picture : : Picture -> IO ()
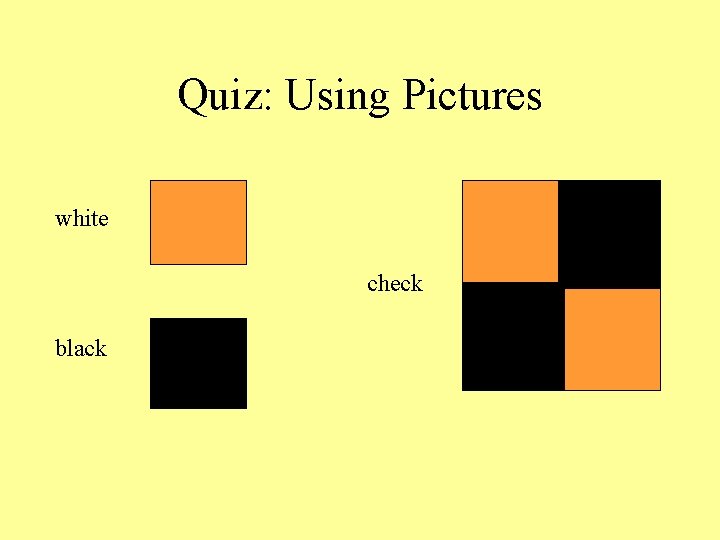
Quiz: Using Pictures white check black
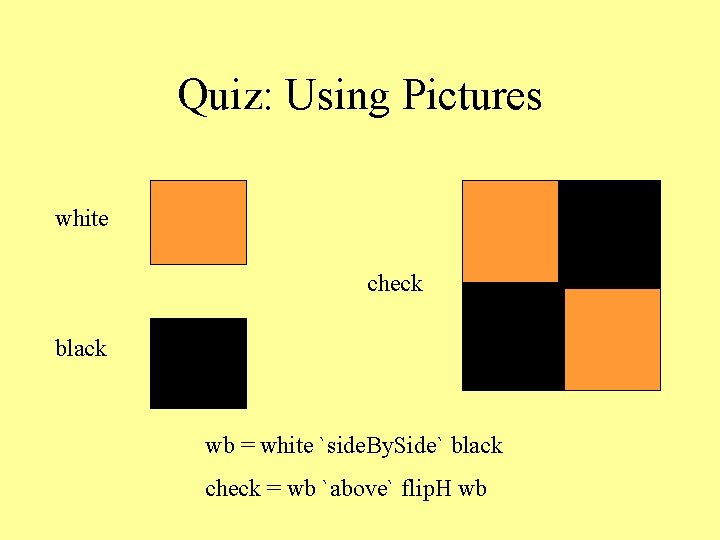
Quiz: Using Pictures white check black wb = white `side. By. Side` black check = wb `above` flip. H wb
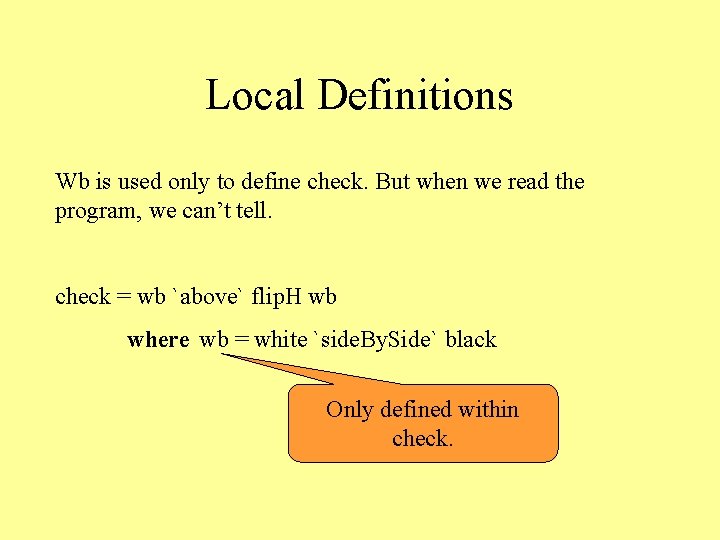
Local Definitions Wb is used only to define check. But when we read the program, we can’t tell. check = wb `above` flip. H wb where wb = white `side. By. Side` black Only defined within check.
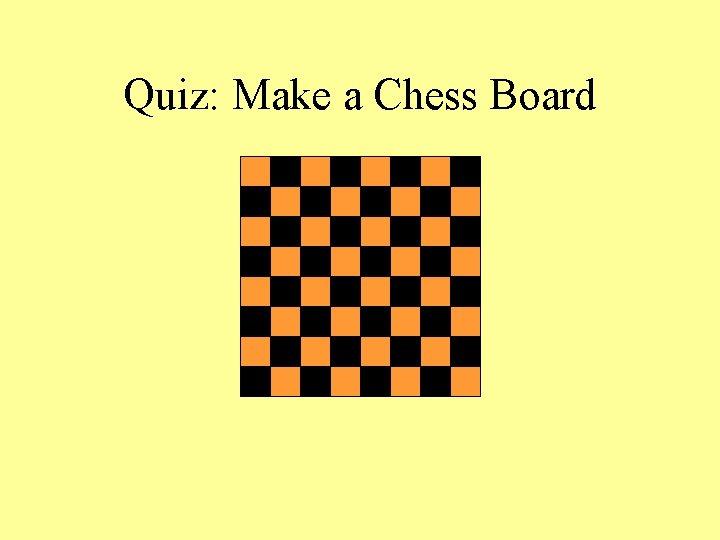
Quiz: Make a Chess Board
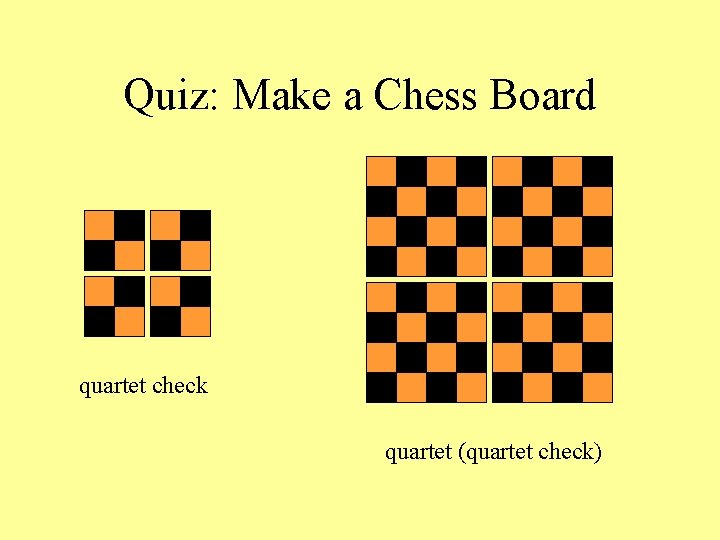
Quiz: Make a Chess Board quartet check quartet (quartet check)
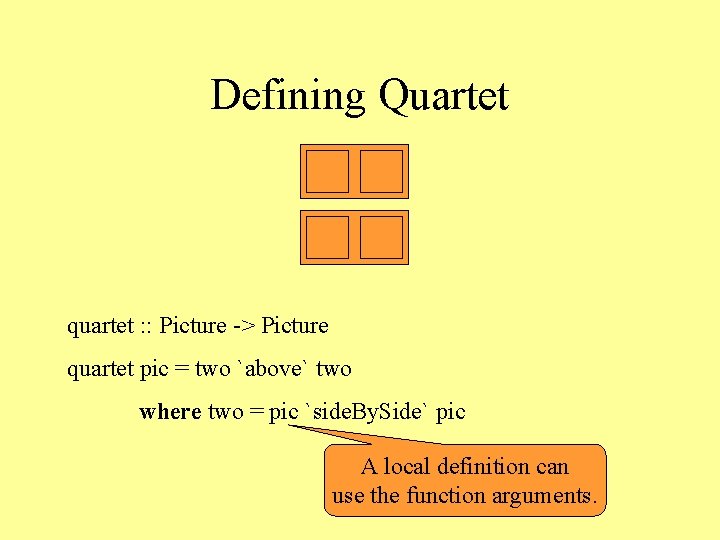
Defining Quartet quartet : : Picture -> Picture quartet pic = two `above` two where two = pic `side. By. Side` pic A local definition can use the function arguments.
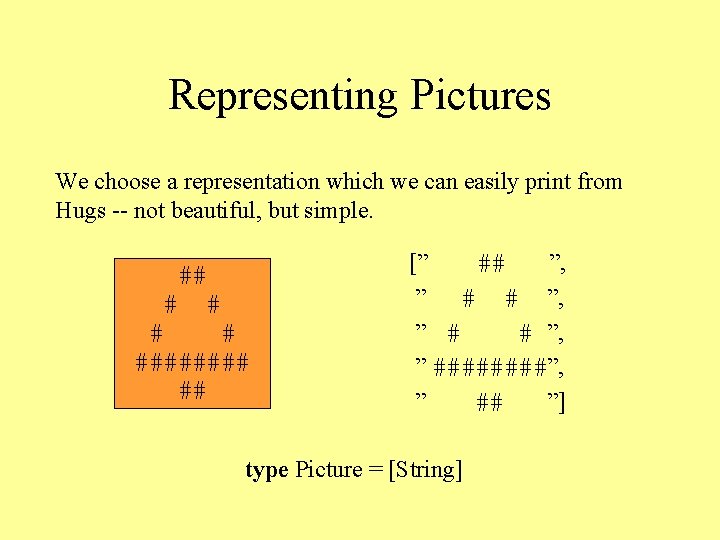
Representing Pictures We choose a representation which we can easily print from Hugs -- not beautiful, but simple. ## # # #### ## [” ## ”, ” ####”, ” ## ”] type Picture = [String]
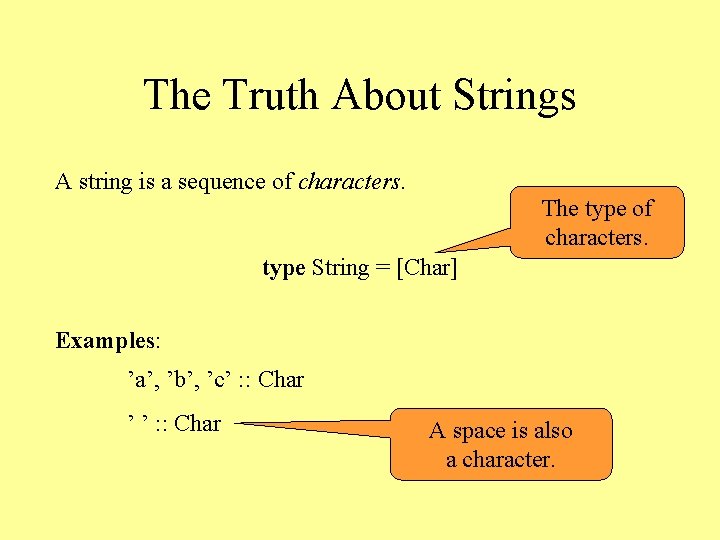
The Truth About Strings A string is a sequence of characters. The type of characters. type String = [Char] Examples: ’a’, ’b’, ’c’ : : Char ’ ’ : : Char A space is also a character.
![Flipping Vertically flip V flip V Flipping Vertically flip. V [” ## ”, ” ####”, ” ## ”] flip. V](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-27.jpg)
Flipping Vertically flip. V [” ## ”, ” ####”, ” ## ”] flip. V : : Picture -> Picture flip. V pic = reverse pic [” ## ”, ” ####”, ” # # ”, ” ## ”]
![Quiz Flipping Horizontally flip H Recall Picture Quiz: Flipping Horizontally flip. H Recall: [” # ”, ”######”, ” # ”] Picture](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-28.jpg)
Quiz: Flipping Horizontally flip. H Recall: [” # ”, ”######”, ” # ”] Picture = [[Char]] [” # ”, ”######”, ” # ”]
![Quiz Flipping Horizontally flip H flip H Quiz: Flipping Horizontally flip. H [” # ”, ”######”, ” # ”] flip. H](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-29.jpg)
Quiz: Flipping Horizontally flip. H [” # ”, ”######”, ” # ”] flip. H : : Picture -> Picture flip. H pic = [reverse line | line <- pic]
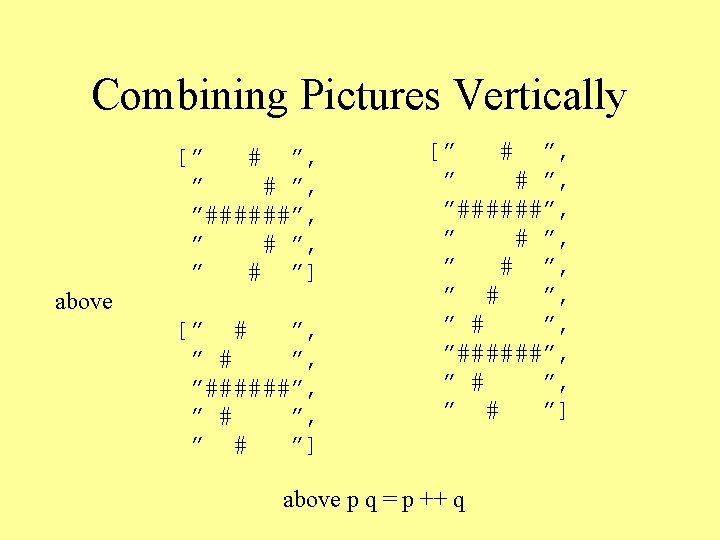
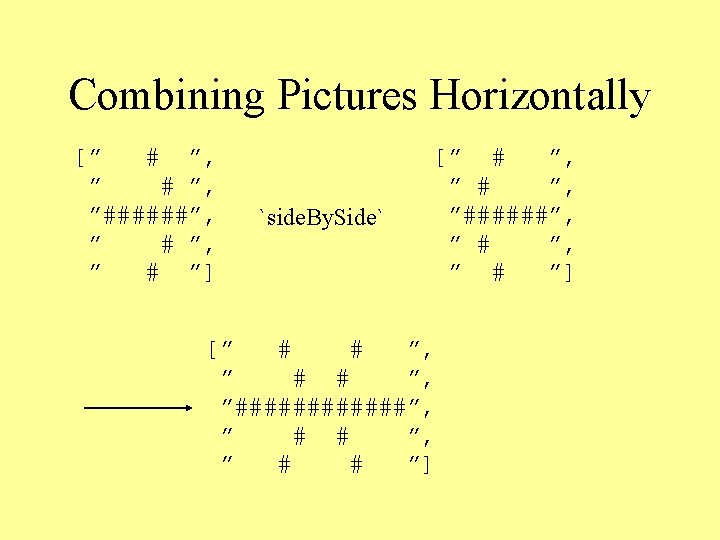
![Combining Pictures Horizontally side By Side Combining Pictures Horizontally [” # ”, ”######”, ” # ”] `side. By. Side` [”](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-32.jpg)
Combining Pictures Horizontally [” # ”, ”######”, ” # ”] `side. By. Side` [” # ”, ”######”, ” # ”] side. By. Side : : Picture -> Picture p `side. By. Side` q = [pline ++ qline | (pline, qline) <- zip p q]
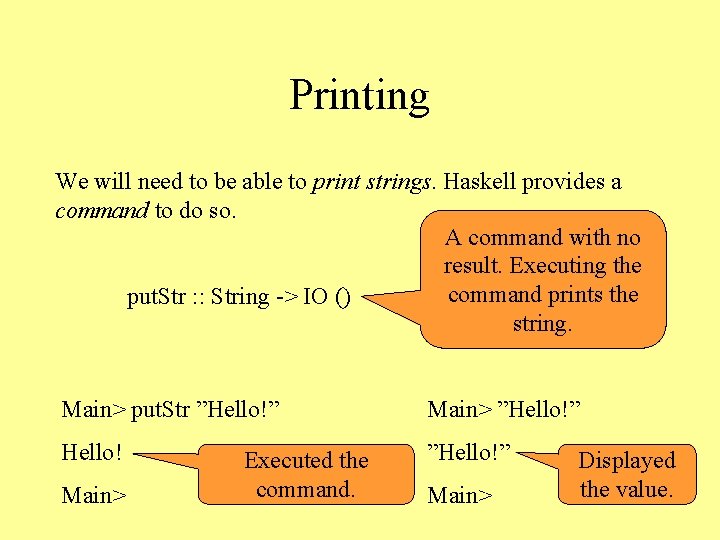
Printing We will need to be able to print strings. Haskell provides a command to do so. A command with no result. Executing the command prints the put. Str : : String -> IO () string. Main> put. Str ”Hello!” Main> ”Hello!” Hello! ”Hello!” Main> Executed the command. Main> Displayed the value.
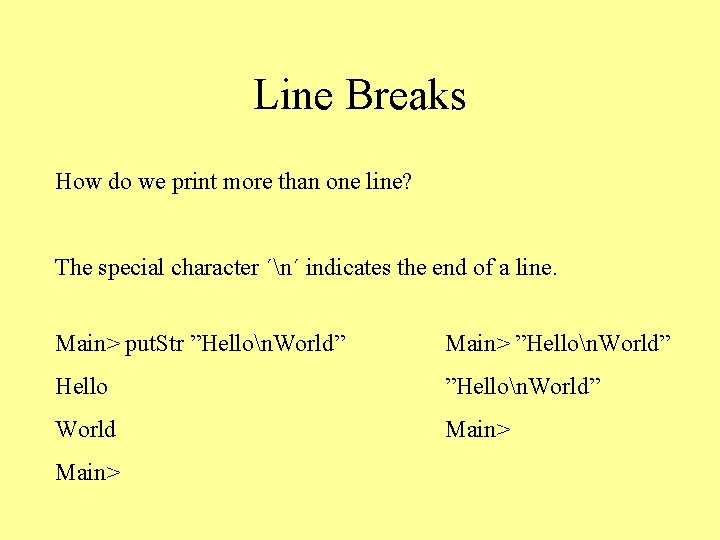
Line Breaks How do we print more than one line? The special character ´n´ indicates the end of a line. Main> put. Str ”Hellon. World” Main> ”Hellon. World” Hello ”Hellon. World” World Main>
![Printing a Picture Print as nn A common operation so there is Printing a Picture Print [”###”, ”###”] as ”###n###n” A common operation, so there is](https://slidetodoc.com/presentation_image_h2/b61bf9a10ae029d435ccf26e5b095033/image-35.jpg)
Printing a Picture Print [”###”, ”###”] as ”###n###n” A common operation, so there is a standard function unlines : : [String] -> String print. Picture : : Picture -> IO () print. Picture pic = put. Str (unlines pic)
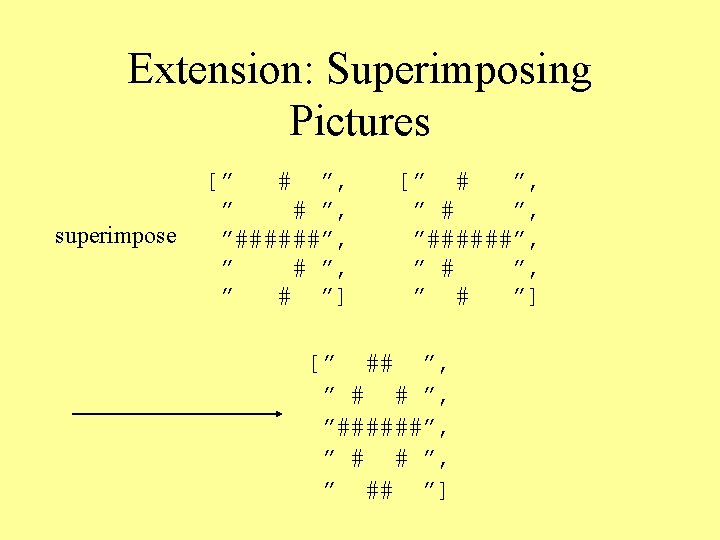
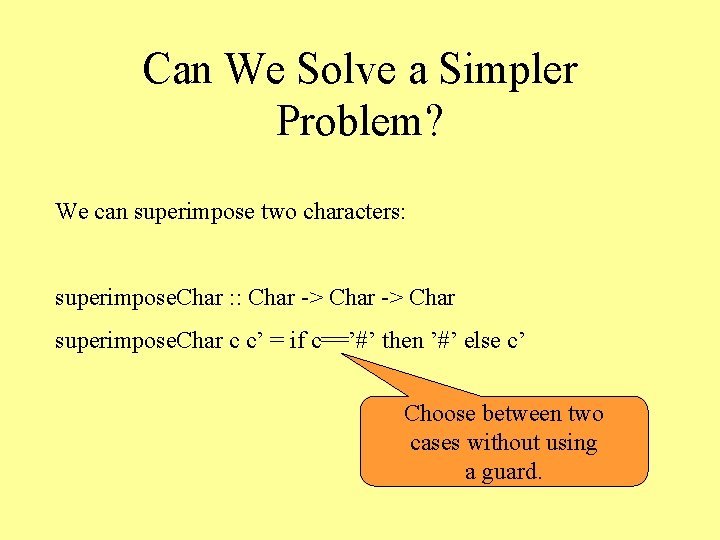
Can We Solve a Simpler Problem? We can superimpose two characters: superimpose. Char : : Char -> Char superimpose. Char c c’ = if c==’#’ then ’#’ else c’ Choose between two cases without using a guard.
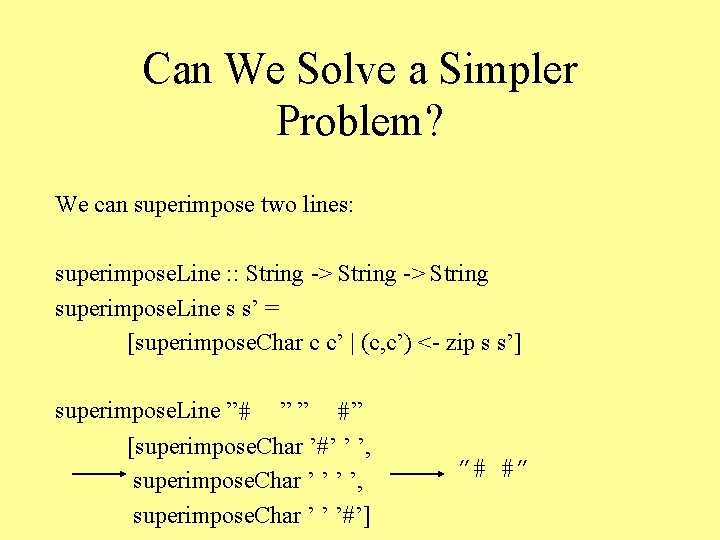
Can We Solve a Simpler Problem? We can superimpose two lines: superimpose. Line : : String -> String superimpose. Line s s’ = [superimpose. Char c c’ | (c, c’) <- zip s s’] superimpose. Line ”# ” ” #” [superimpose. Char ’#’ ’ ’, superimpose. Char ’ ’ ’#’] ”# #”
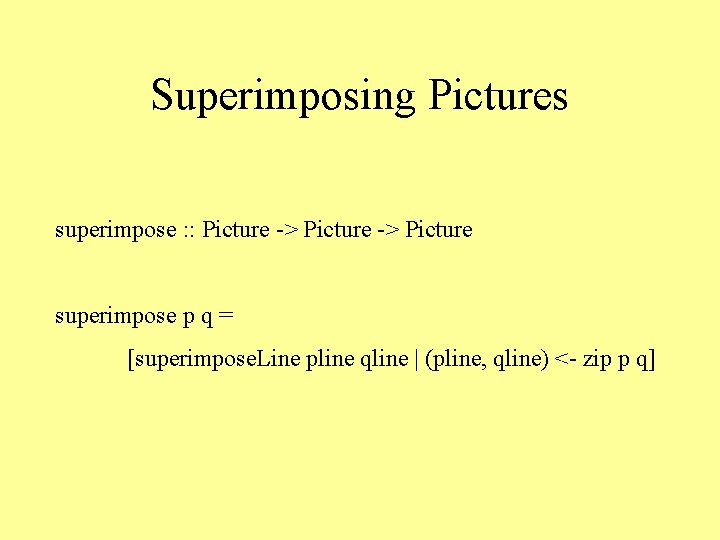
Superimposing Pictures superimpose : : Picture -> Picture superimpose p q = [superimpose. Line pline qline | (pline, qline) <- zip p q]
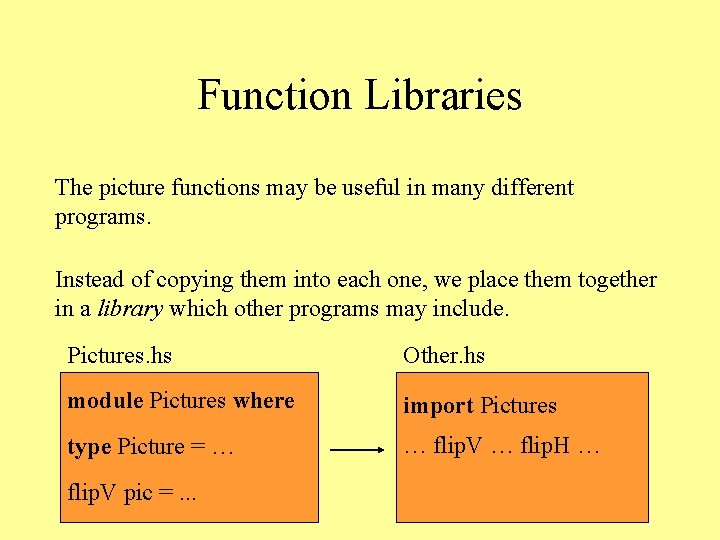
Function Libraries The picture functions may be useful in many different programs. Instead of copying them into each one, we place them together in a library which other programs may include. Pictures. hs Other. hs module Pictures where import Pictures type Picture = … … flip. V … flip. H … flip. V pic =. . .
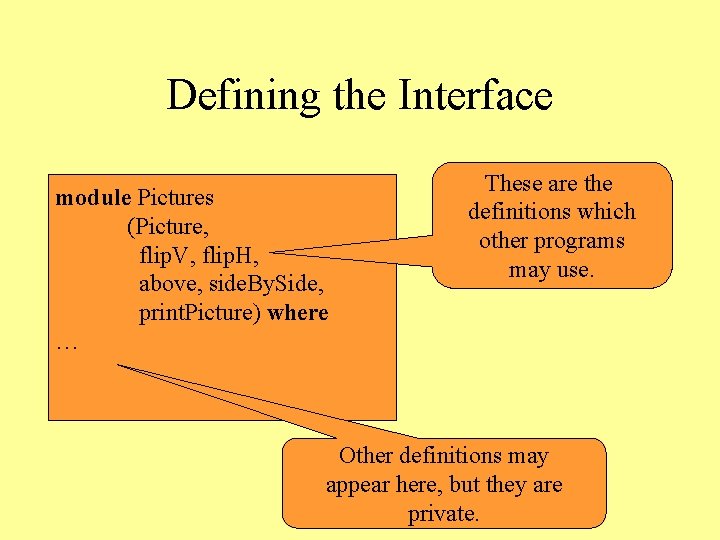
Defining the Interface module Pictures (Picture, flip. V, flip. H, above, side. By. Side, print. Picture) where … These are the definitions which other programs may use. Other definitions may appear here, but they are private.
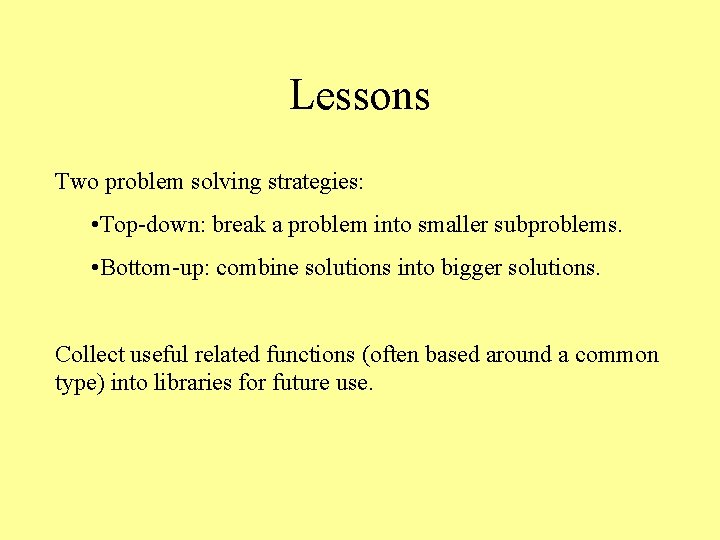
Lessons Two problem solving strategies: • Top-down: break a problem into smaller subproblems. • Bottom-up: combine solutions into bigger solutions. Collect useful related functions (often based around a common type) into libraries for future use.
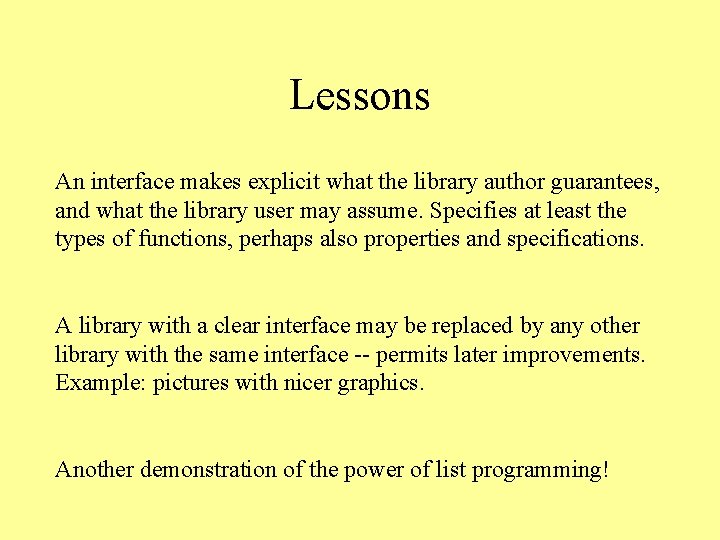
Lessons An interface makes explicit what the library author guarantees, and what the library user may assume. Specifies at least the types of functions, perhaps also properties and specifications. A library with a clear interface may be replaced by any other library with the same interface -- permits later improvements. Example: pictures with nicer graphics. Another demonstration of the power of list programming!
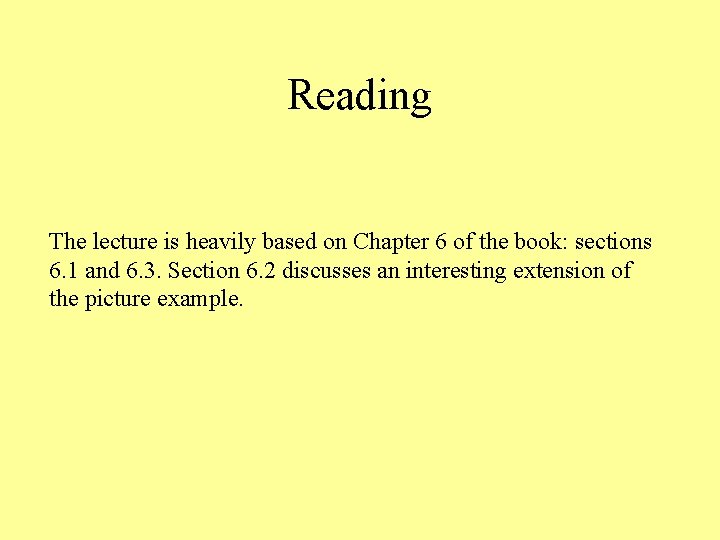
Reading The lecture is heavily based on Chapter 6 of the book: sections 6. 1 and 6. 3. Section 6. 2 discusses an interesting extension of the picture example.
Requisitos não funcionais
Requisito funcional e não funcional
Requisito funcional e não funcional
Silepse
Figura de estilo animismo
Figuras de estilo elipse
Cual es el objetivo de las figuras literarias
Figuras literaris
[email protected]
/etc/shadow linux
Springer, elsevier, taylor & francis etc. are ______. *
A passage taken from a book
Land registration act 2012
Informal writing
Exercise etc inc
Exercise etc inc
Xshooter etc
Rogues etc ..
Haswa
Exergonic cellular respiration
Etc
Opposite to photosynthesis
Violation of compulsory attendance req parent, etc
Some something any anything
Etc hong kong
Freedom from danger, risk, etc.; safety
Digbio
Cause and effect clauses
Health and safety at work act 1974 section 2
It is about service quality either tactile or visual
Etc occurs in
A feeling of visual equality in shape form value color etc
Africompost
Newton little league
Primer segundo tercero cuarto quinto
Game theory switch case
Usr local etc
Etc occurs in
2 metilpentano
Letter endings informal
Etc vs eac project management
Etc
Tweeter etc
Comparative form of polluted
Components of respiratory chain