Memory Management Memory Organization u During run time
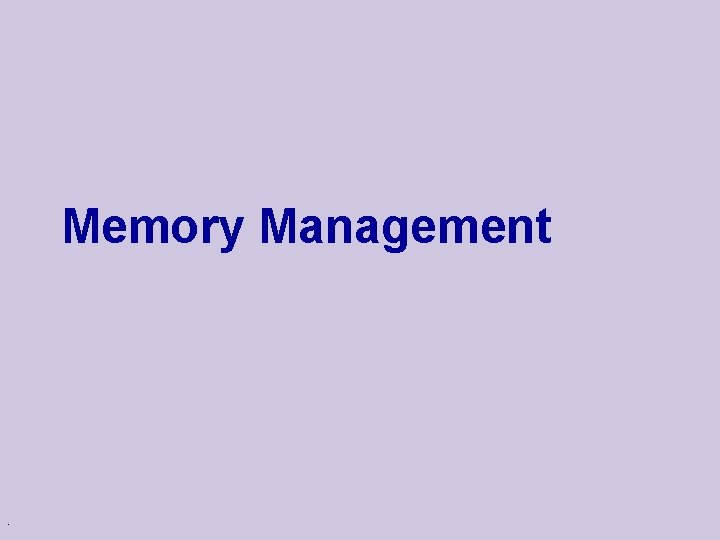
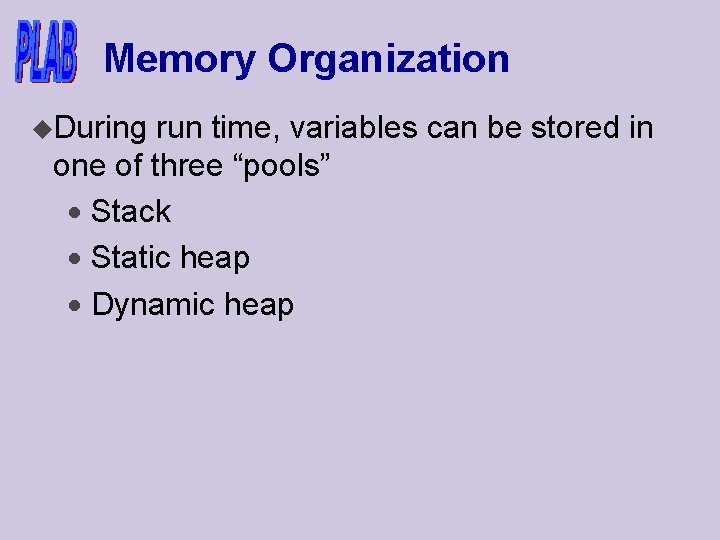
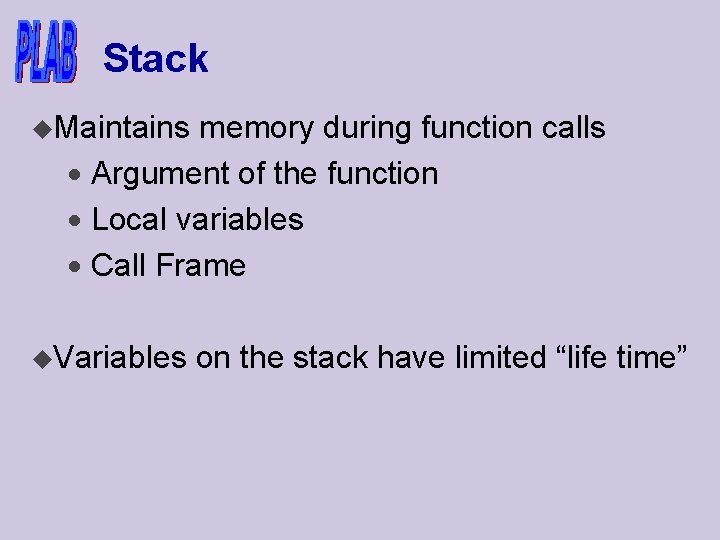
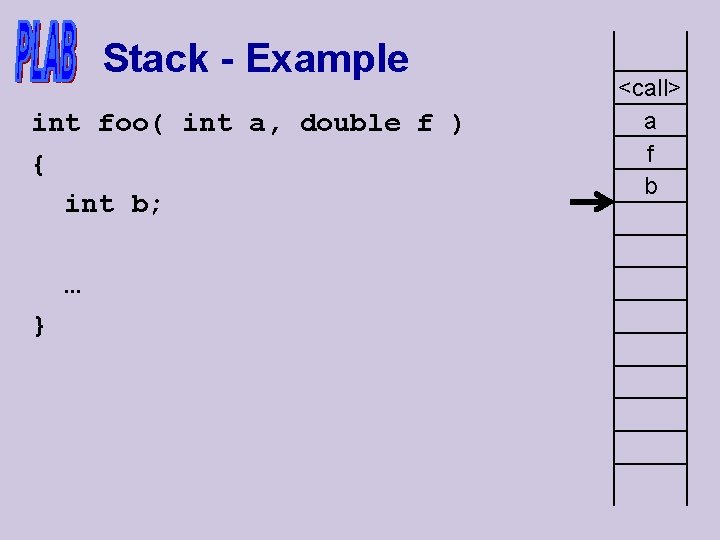
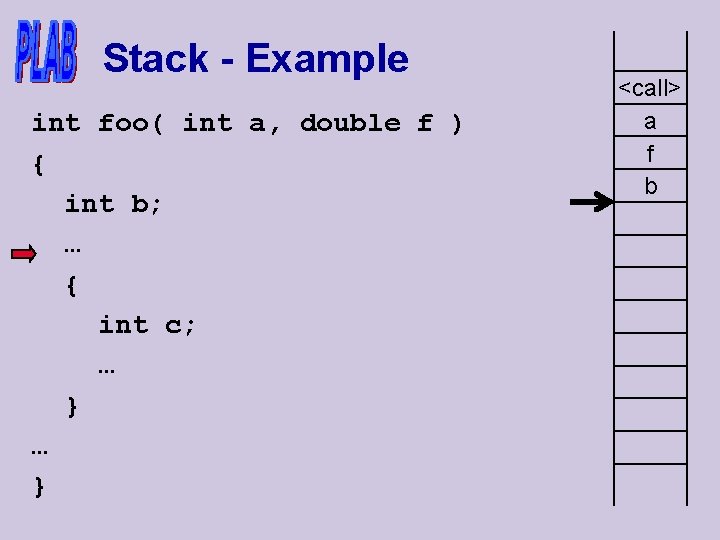
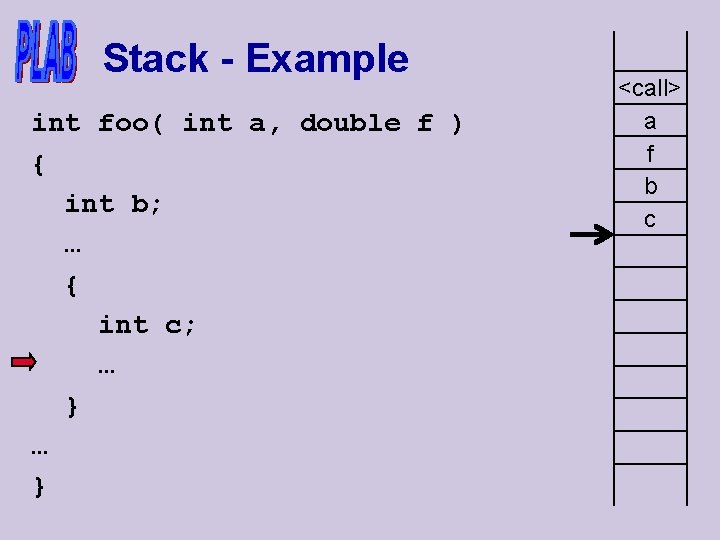
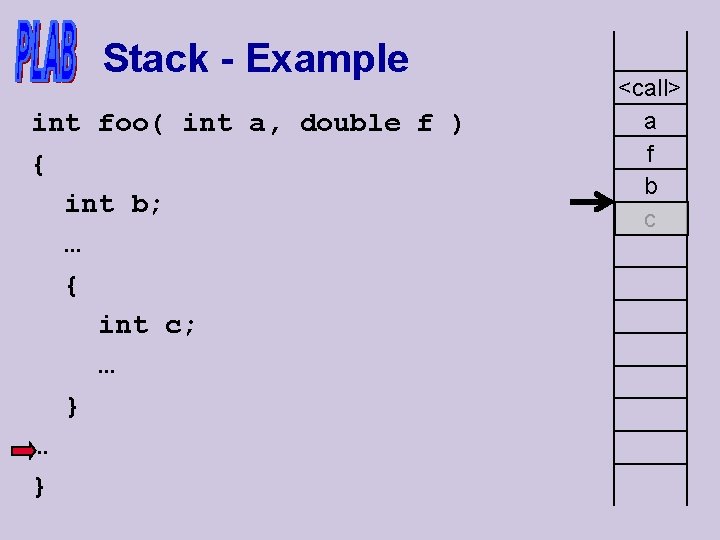
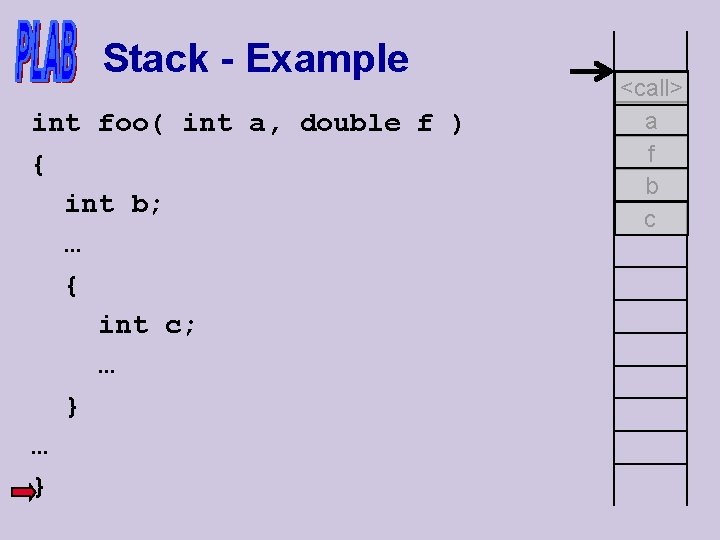
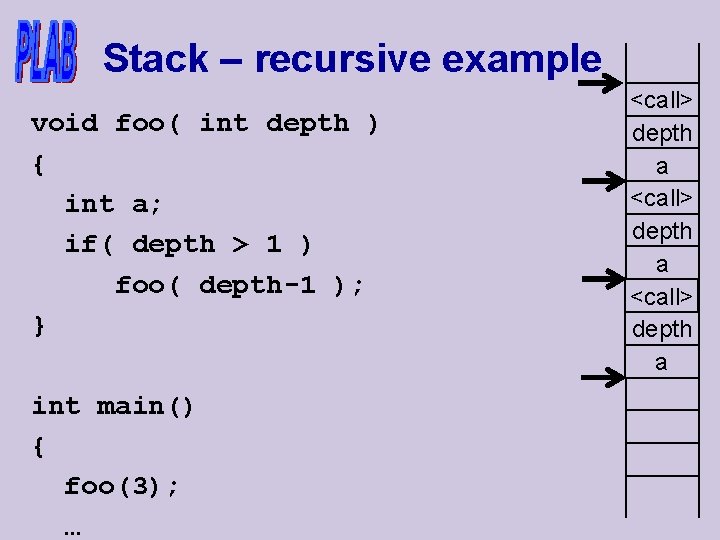
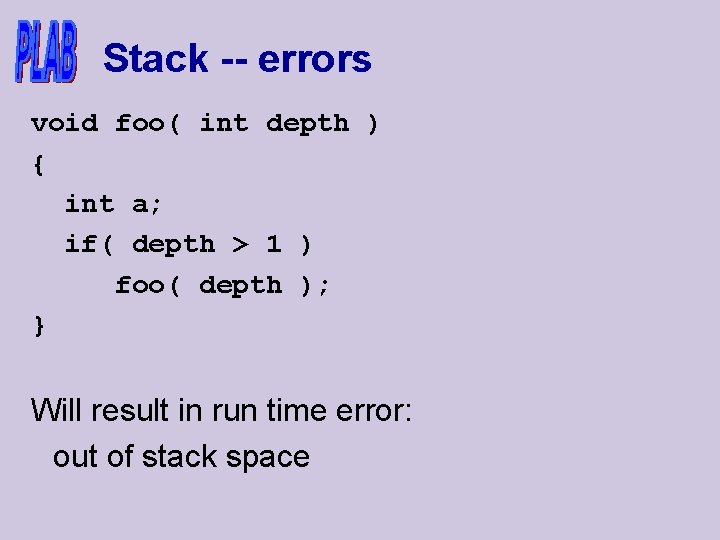
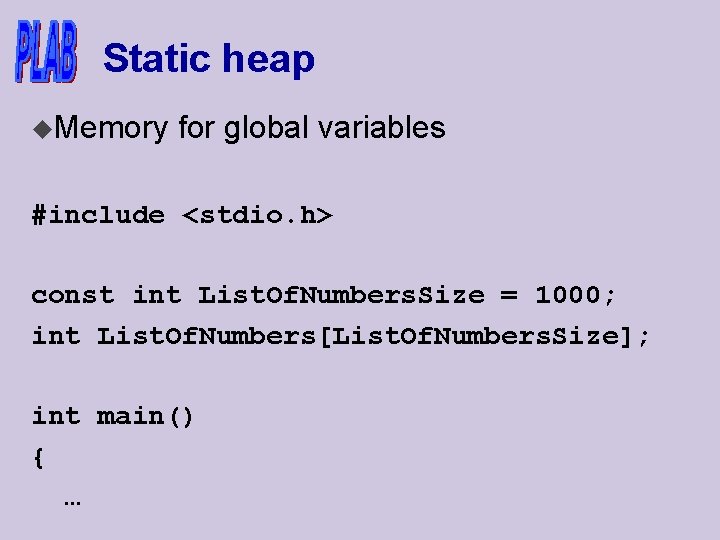
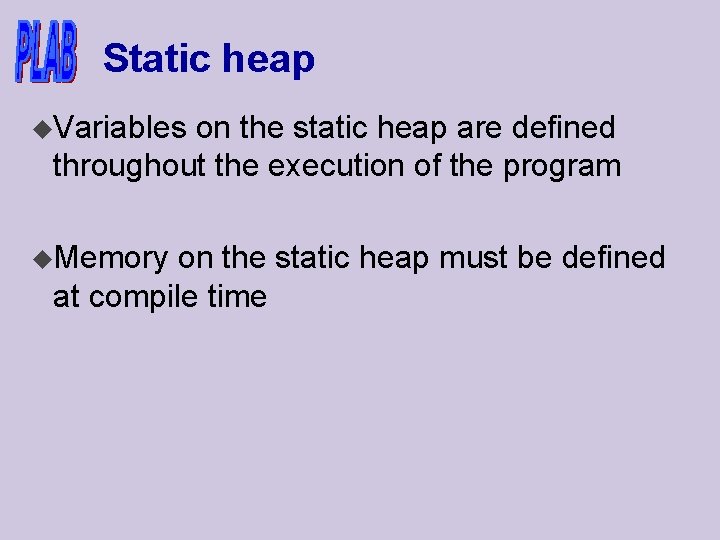
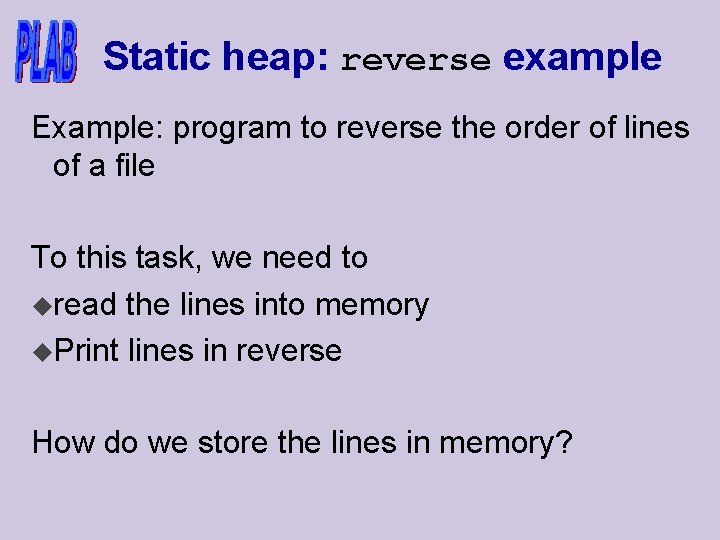
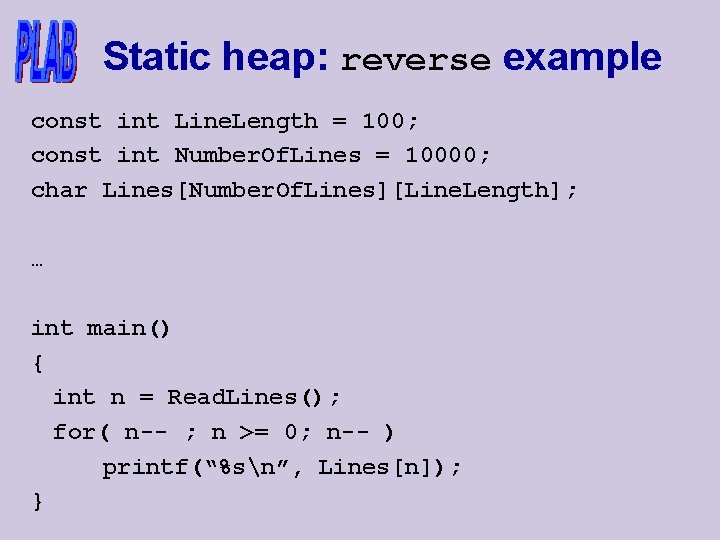
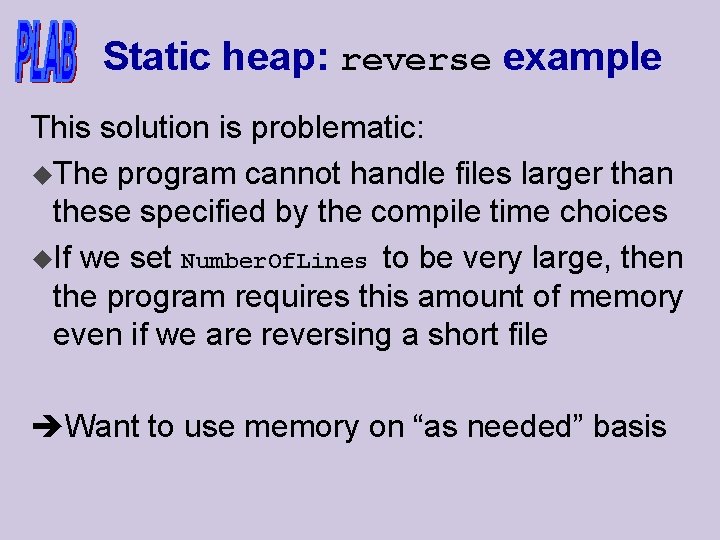
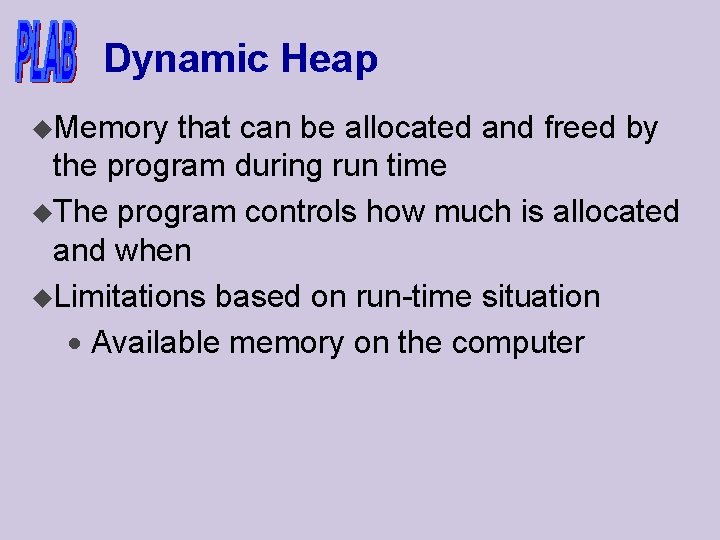
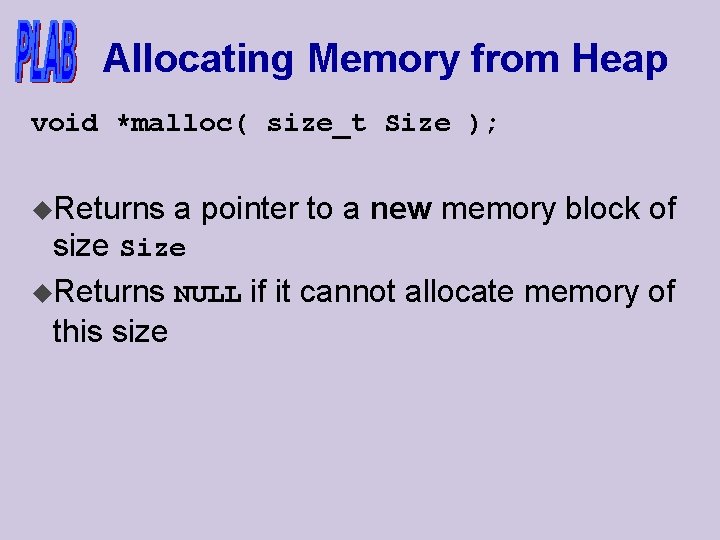
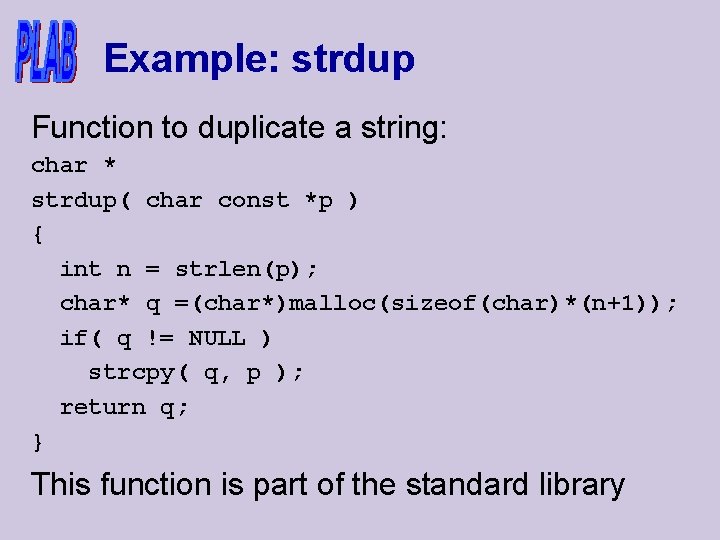
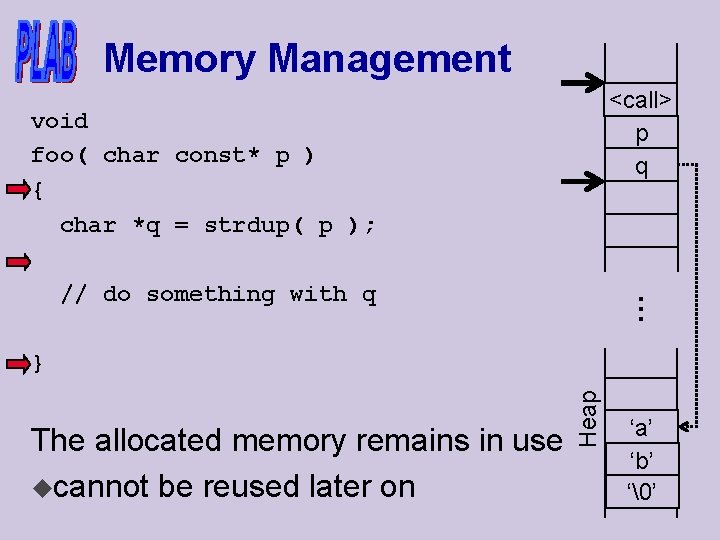
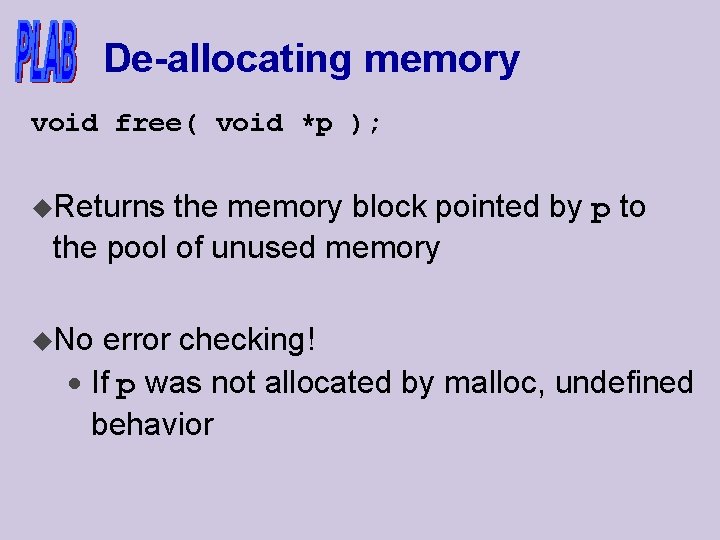
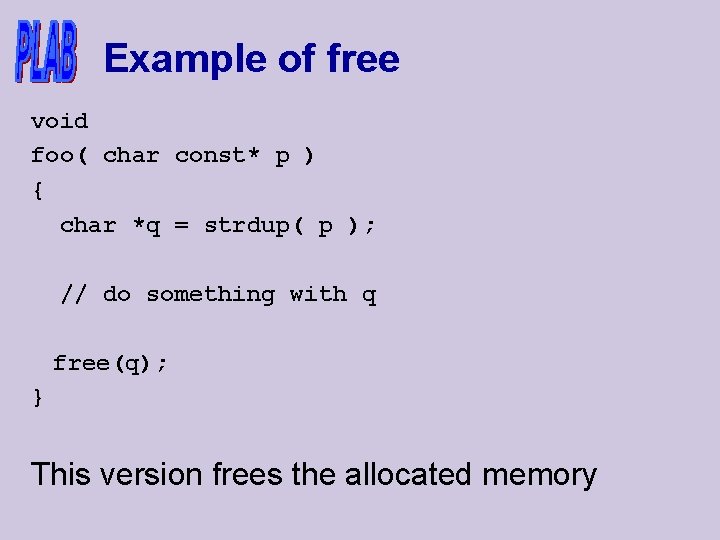
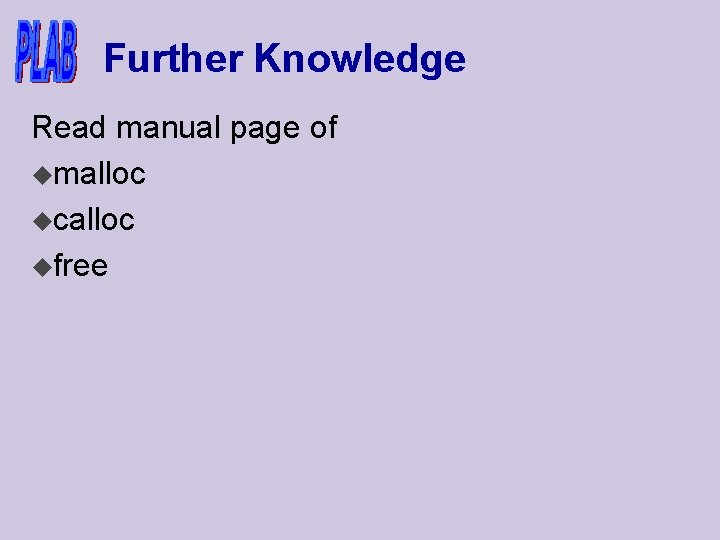
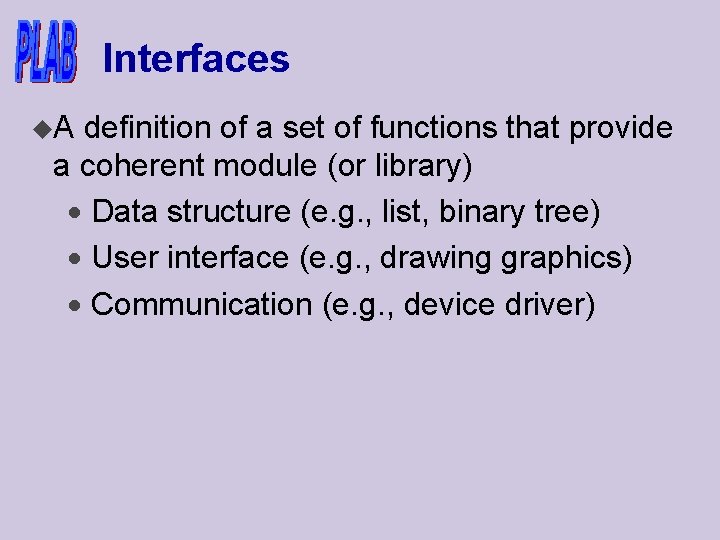
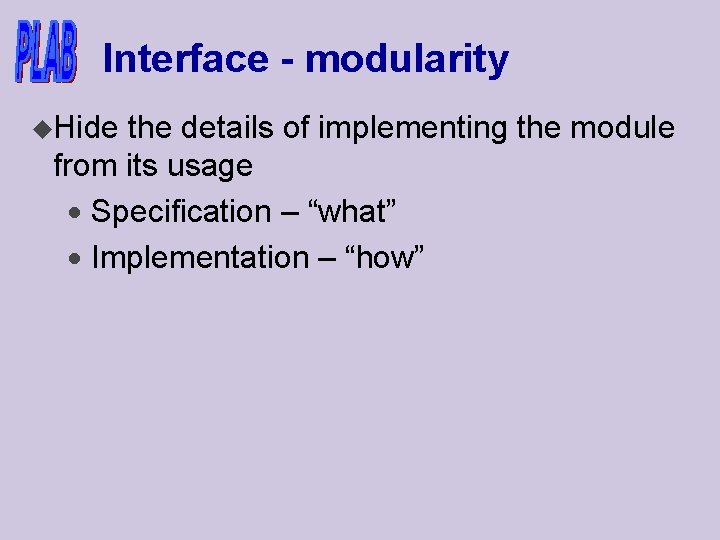
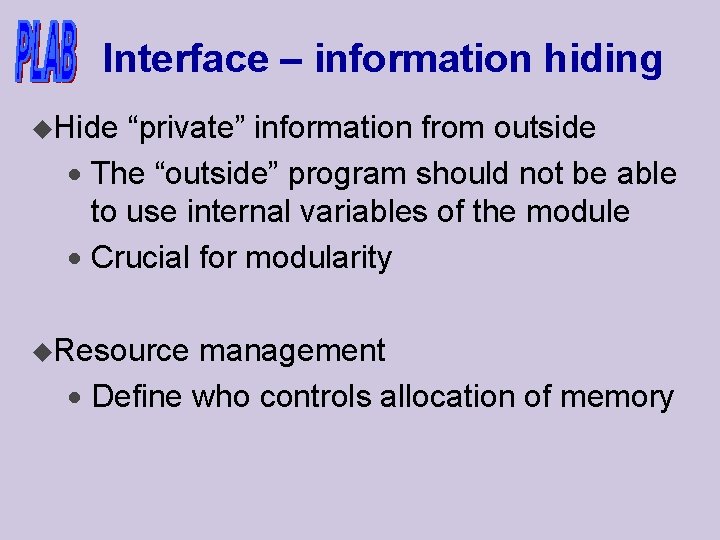
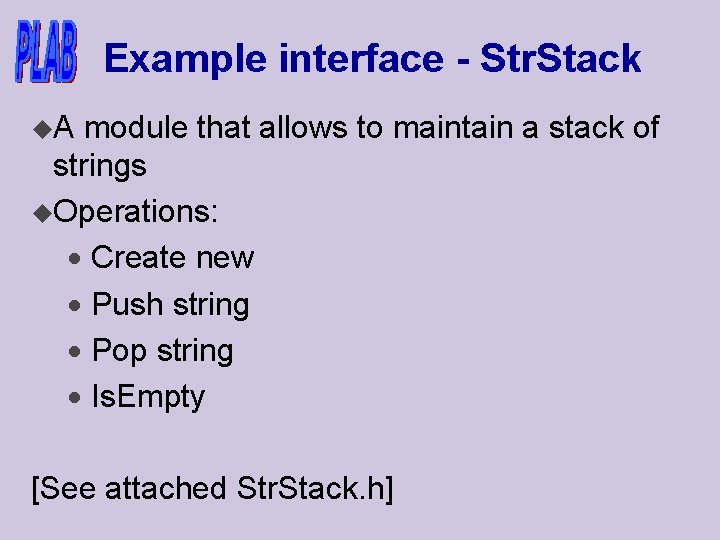
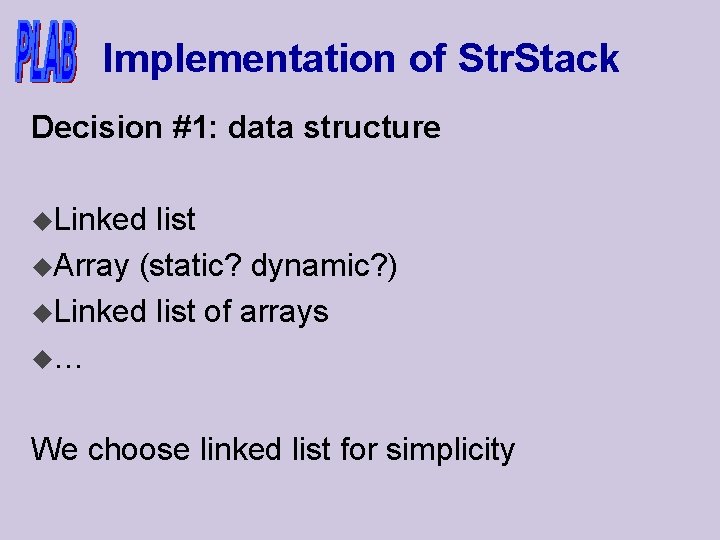
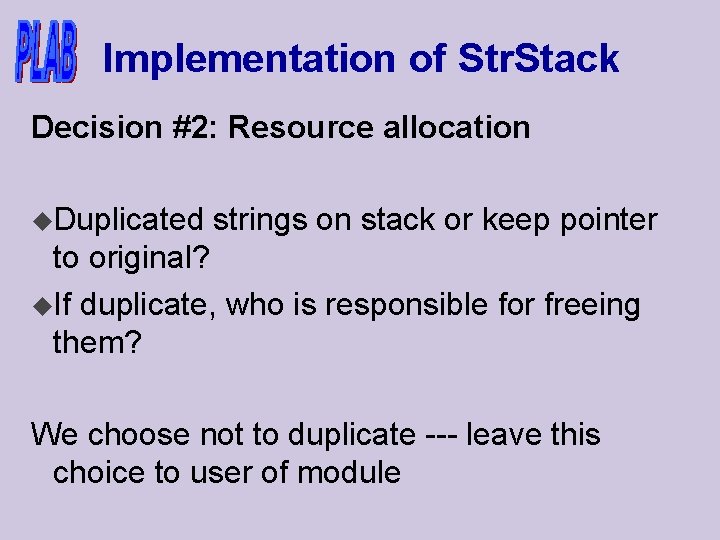
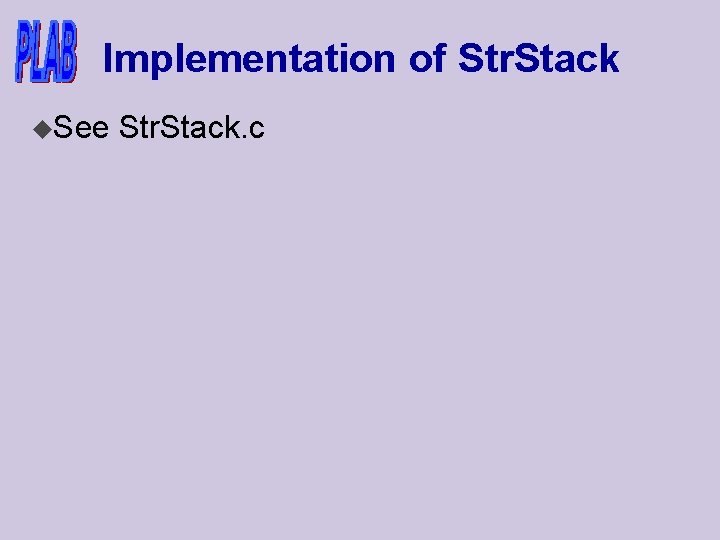
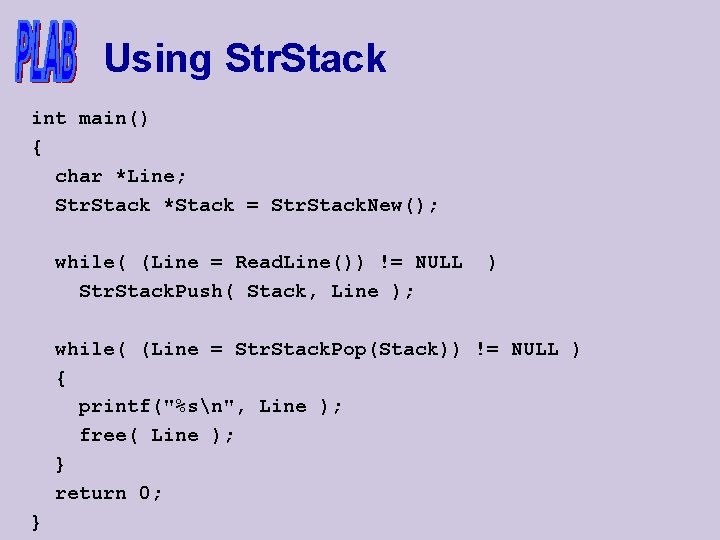
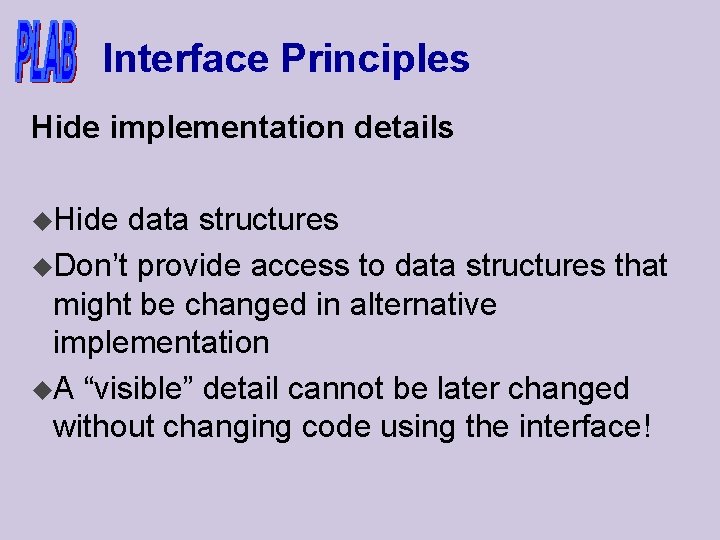
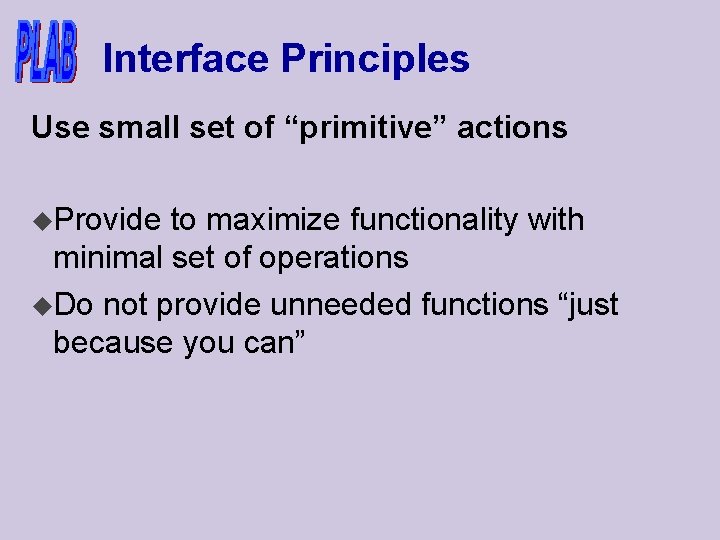
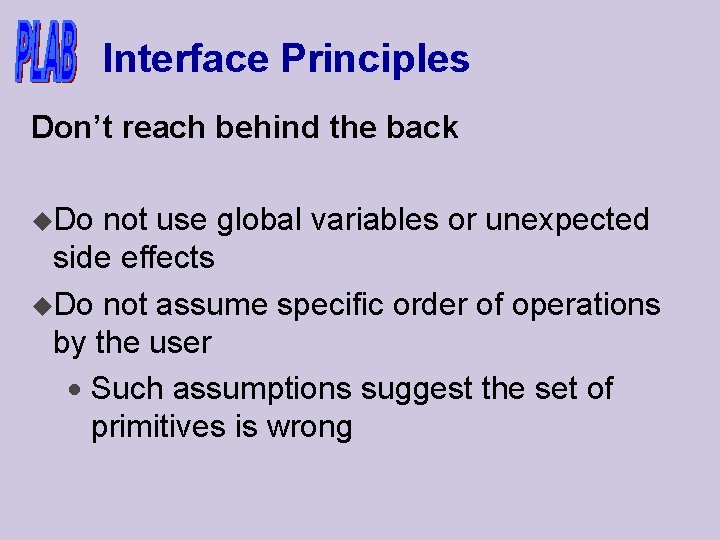
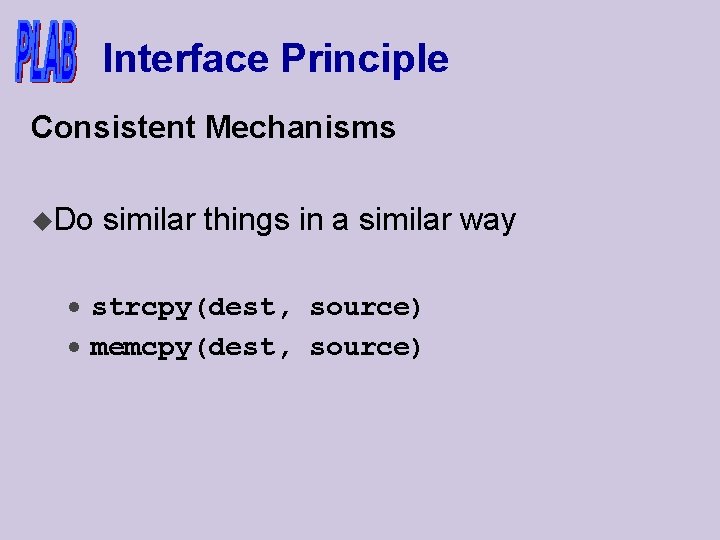
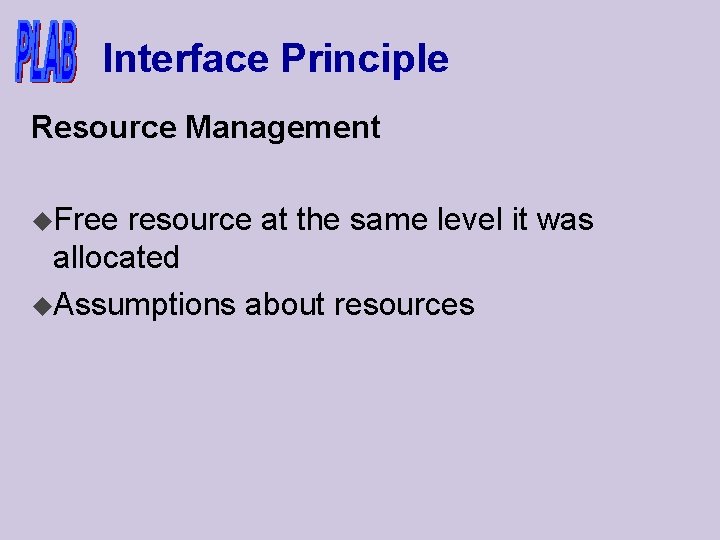
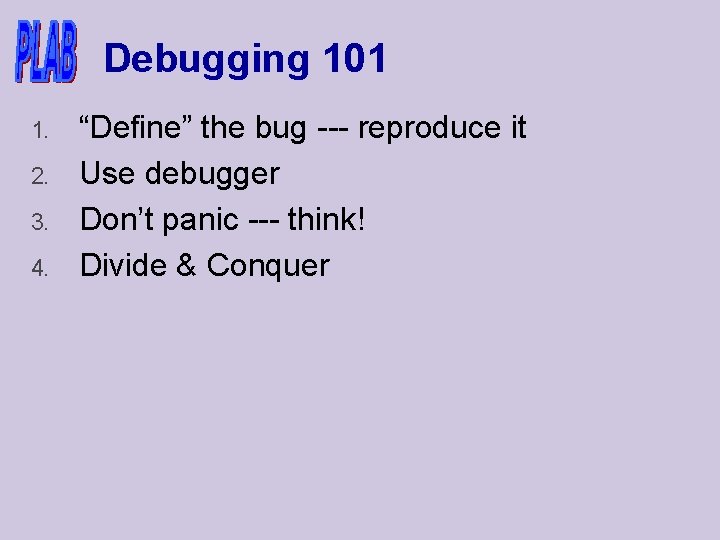
- Slides: 36
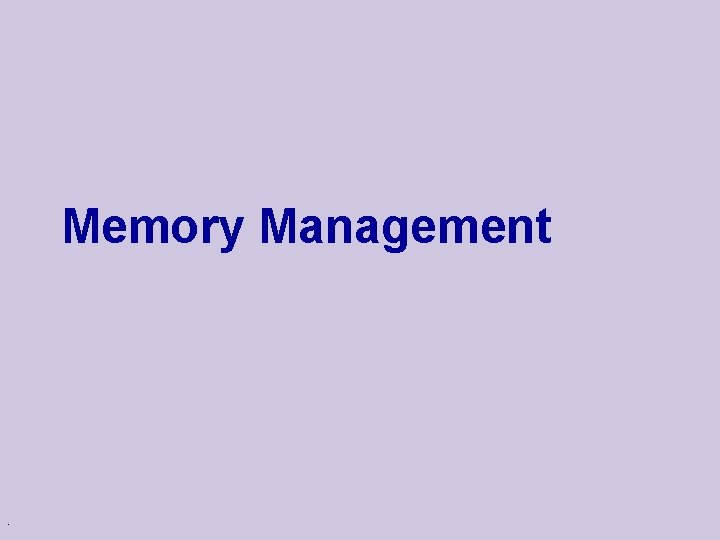
Memory Management .
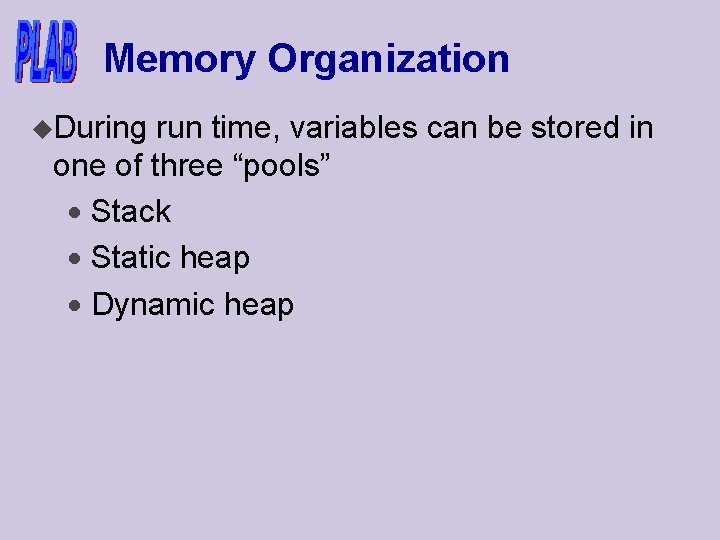
Memory Organization u. During run time, variables can be stored in one of three “pools” · Stack · Static heap · Dynamic heap
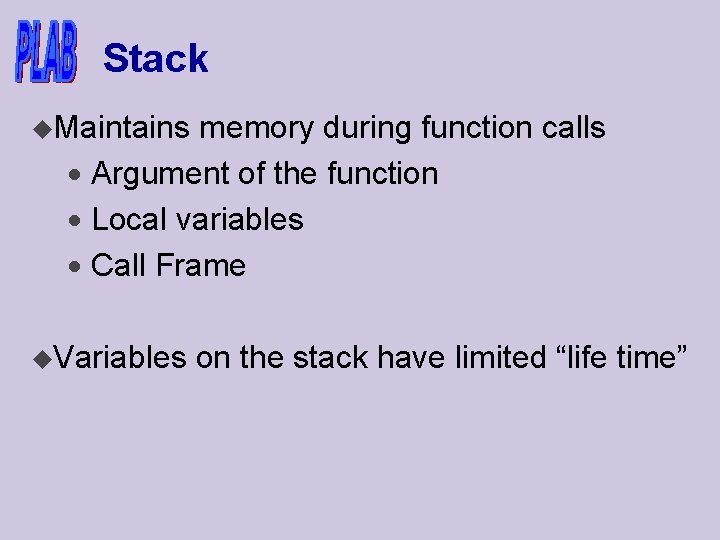
Stack u. Maintains memory during function calls · Argument of the function · Local variables · Call Frame u. Variables on the stack have limited “life time”
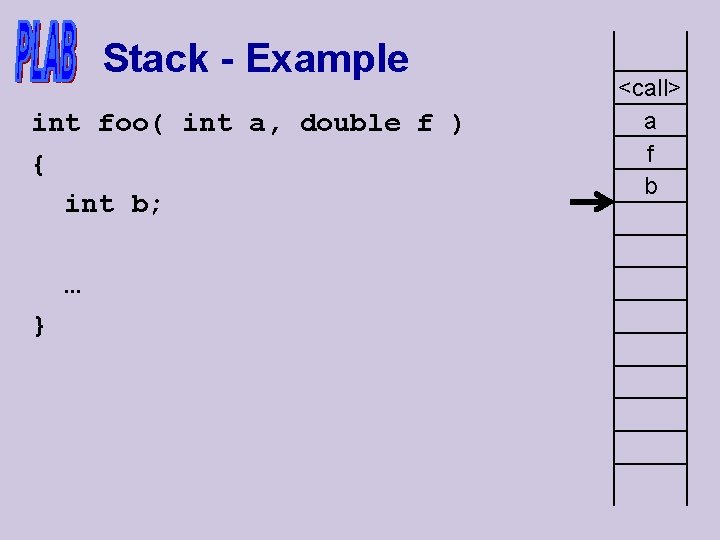
Stack - Example int foo( int a, double f ) { int b; … } <call> a f b
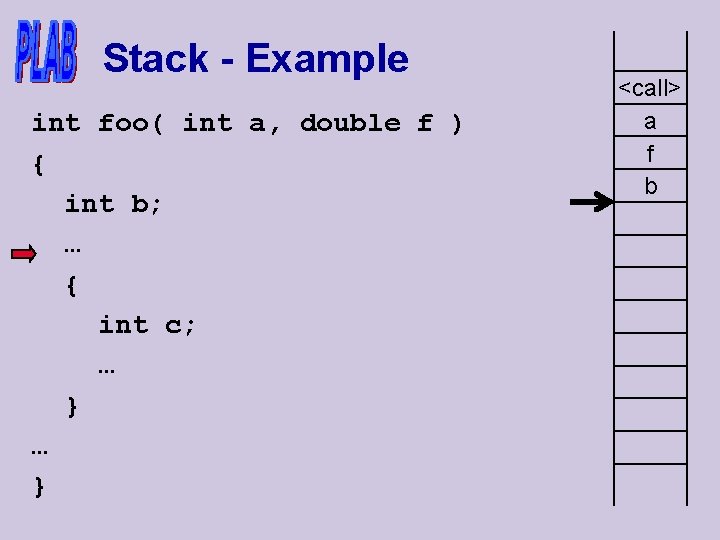
Stack - Example int foo( int a, double f ) { int b; … { int c; … } <call> a f b
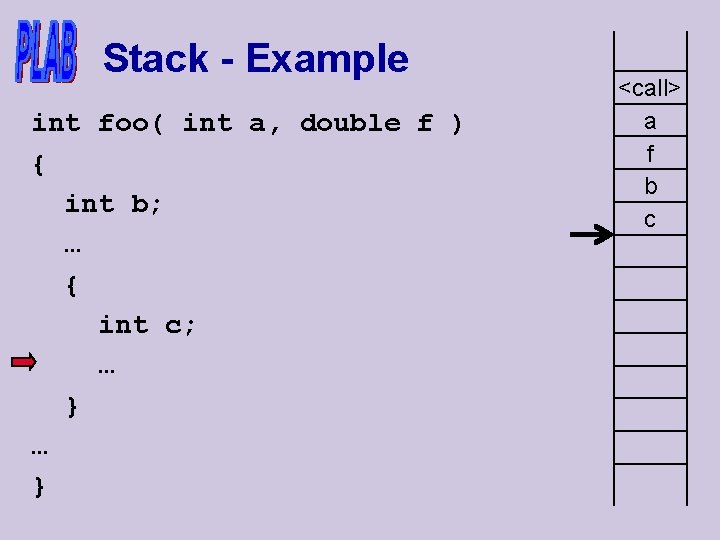
Stack - Example int foo( int a, double f ) { int b; … { int c; … } <call> a f b c
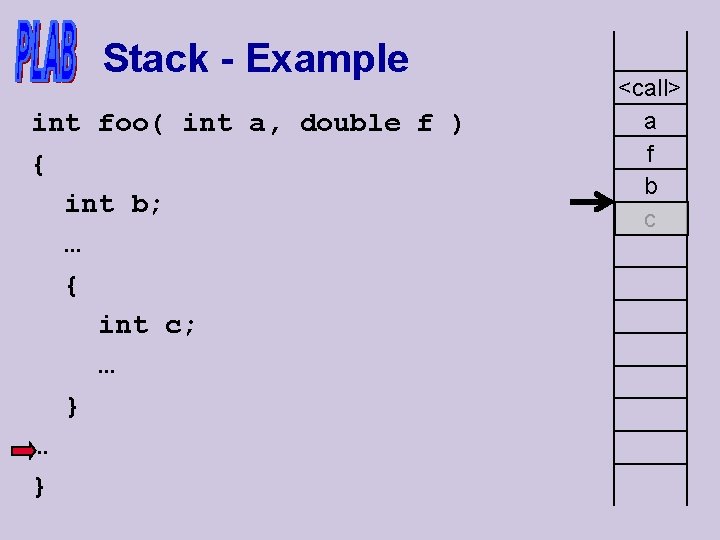
Stack - Example int foo( int a, double f ) { int b; … { int c; … } <call> a f b c
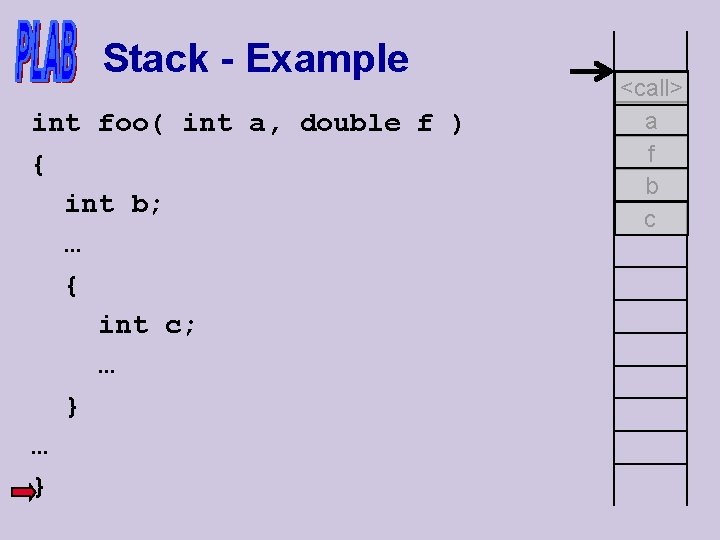
Stack - Example int foo( int a, double f ) { int b; … { int c; … } <call> a f b c
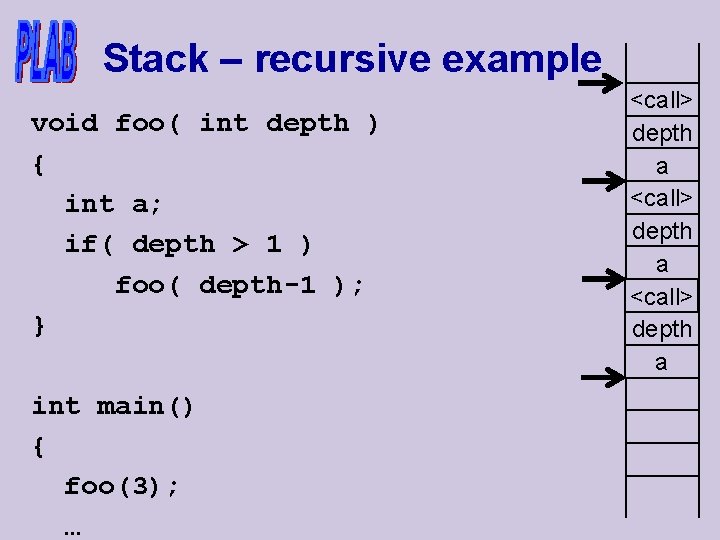
Stack – recursive example void foo( int depth ) { int a; if( depth > 1 ) foo( depth-1 ); } int main() { foo(3); … <call> depth a
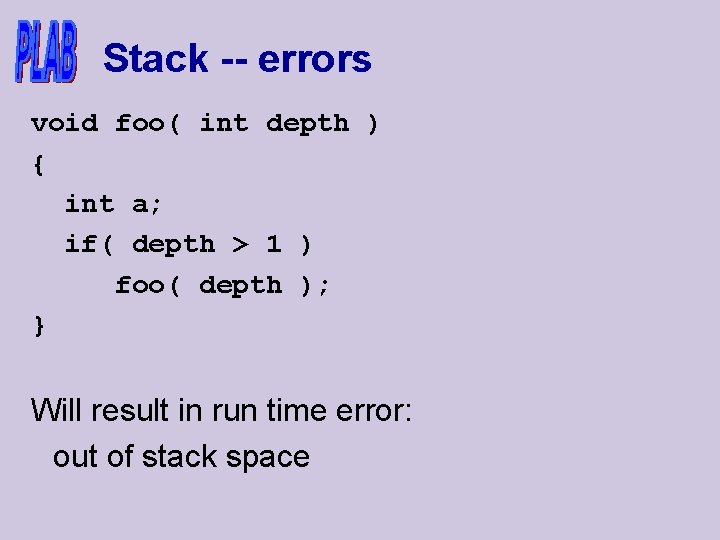
Stack -- errors void foo( int depth ) { int a; if( depth > 1 ) foo( depth ); } Will result in run time error: out of stack space
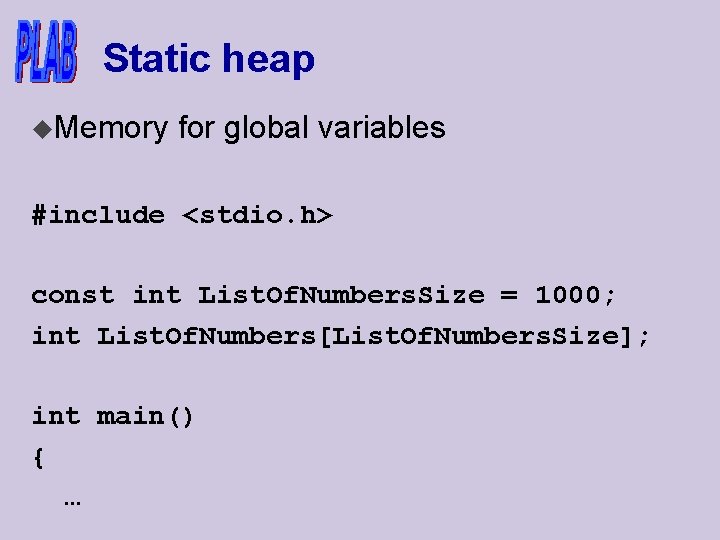
Static heap u. Memory for global variables #include <stdio. h> const int List. Of. Numbers. Size = 1000; int List. Of. Numbers[List. Of. Numbers. Size]; int main() { …
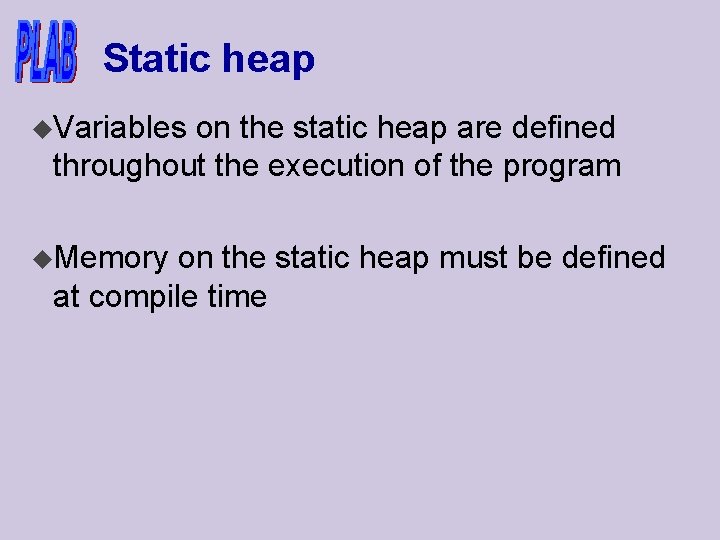
Static heap u. Variables on the static heap are defined throughout the execution of the program u. Memory on the static heap must be defined at compile time
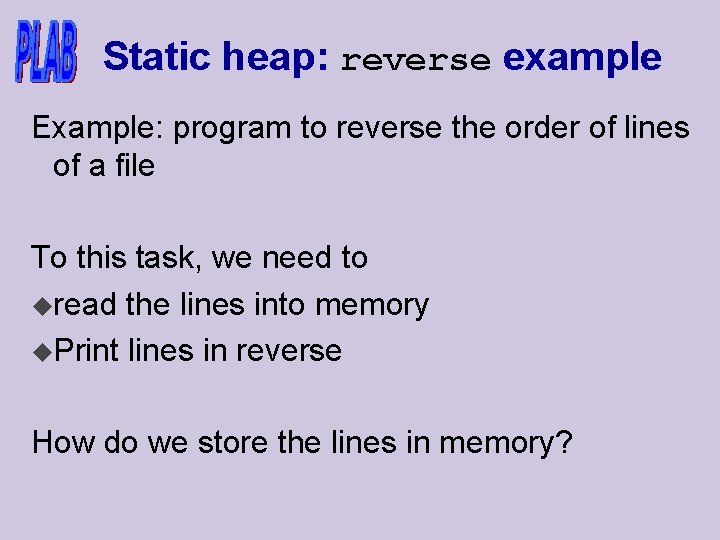
Static heap: reverse example Example: program to reverse the order of lines of a file To this task, we need to uread the lines into memory u. Print lines in reverse How do we store the lines in memory?
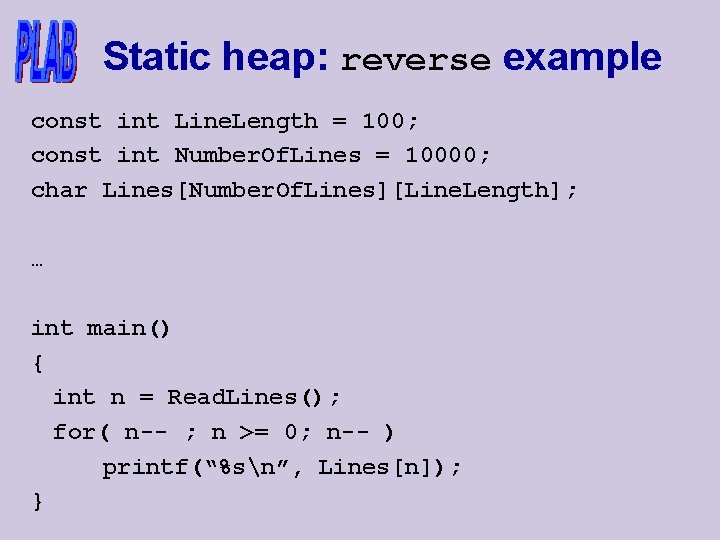
Static heap: reverse example const int Line. Length = 100; const int Number. Of. Lines = 10000; char Lines[Number. Of. Lines][Line. Length]; … int main() { int n = Read. Lines(); for( n-- ; n >= 0; n-- ) printf(“%sn”, Lines[n]); }
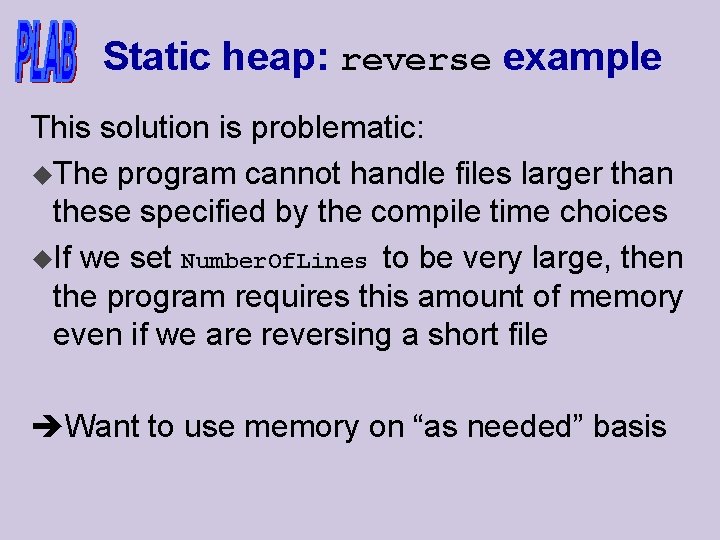
Static heap: reverse example This solution is problematic: u. The program cannot handle files larger than these specified by the compile time choices u. If we set Number. Of. Lines to be very large, then the program requires this amount of memory even if we are reversing a short file Want to use memory on “as needed” basis
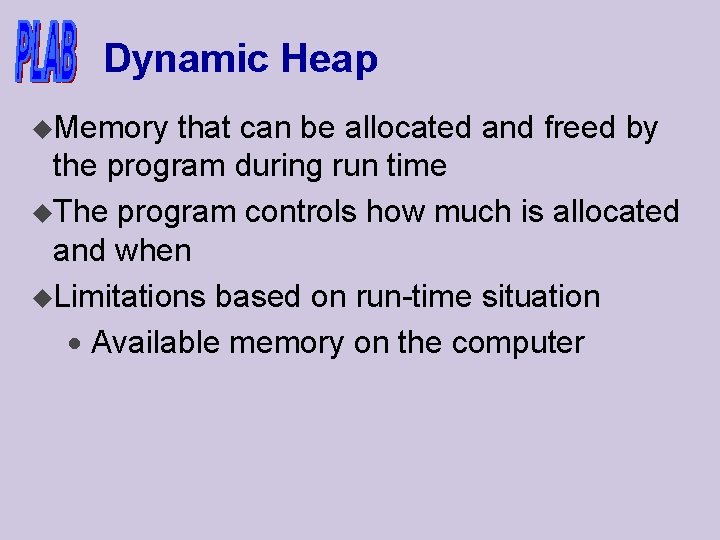
Dynamic Heap u. Memory that can be allocated and freed by the program during run time u. The program controls how much is allocated and when u. Limitations based on run-time situation · Available memory on the computer
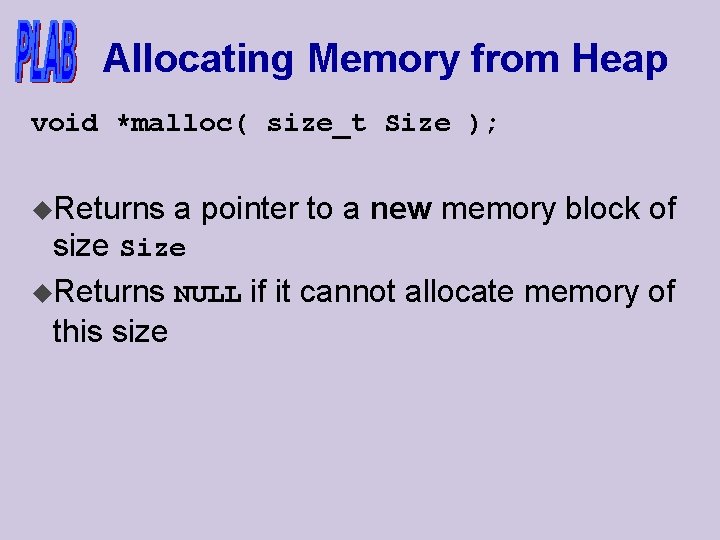
Allocating Memory from Heap void *malloc( size_t Size ); u. Returns a pointer to a new memory block of size Size u. Returns NULL if it cannot allocate memory of this size
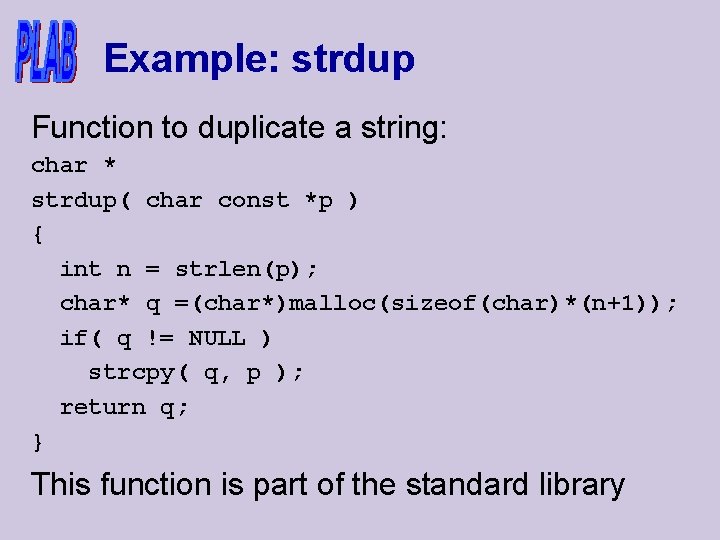
Example: strdup Function to duplicate a string: char * strdup( char const *p ) { int n = strlen(p); char* q =(char*)malloc(sizeof(char)*(n+1)); if( q != NULL ) strcpy( q, p ); return q; } This function is part of the standard library
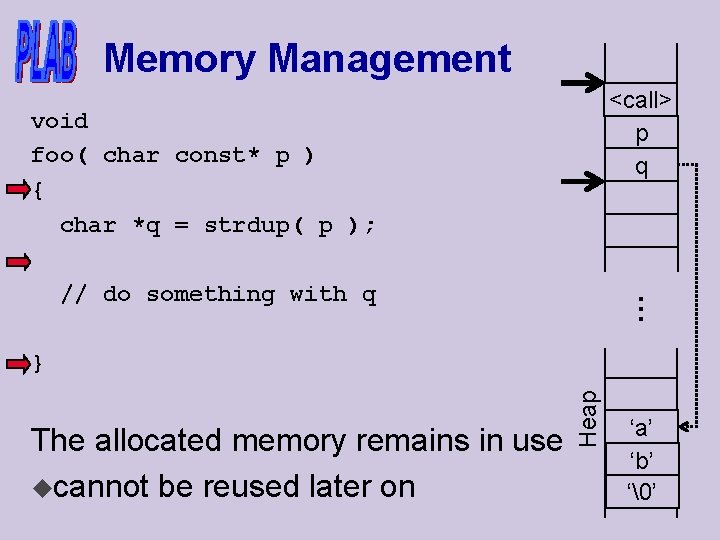
Memory Management <call> p q void foo( char const* p ) { char *q = strdup( p ); … // do something with q The allocated memory remains in use ucannot be reused later on Heap } ‘a’ ‘b’ ‘ ’
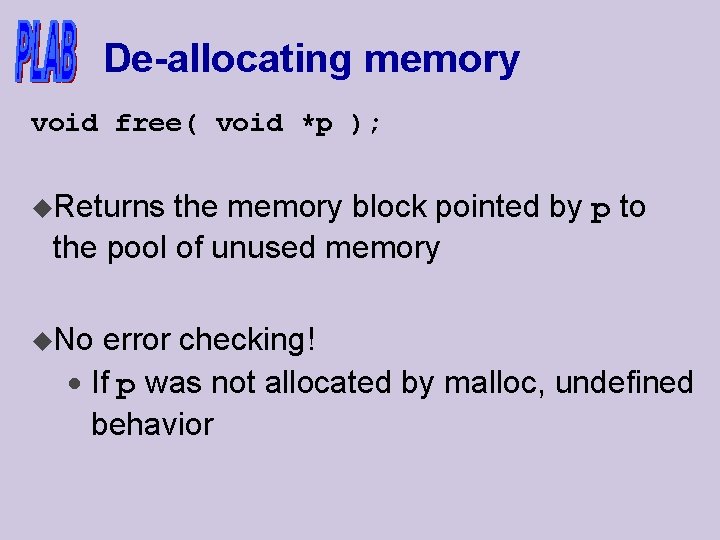
De-allocating memory void free( void *p ); u. Returns the memory block pointed by p to the pool of unused memory u. No error checking! · If p was not allocated by malloc, undefined behavior
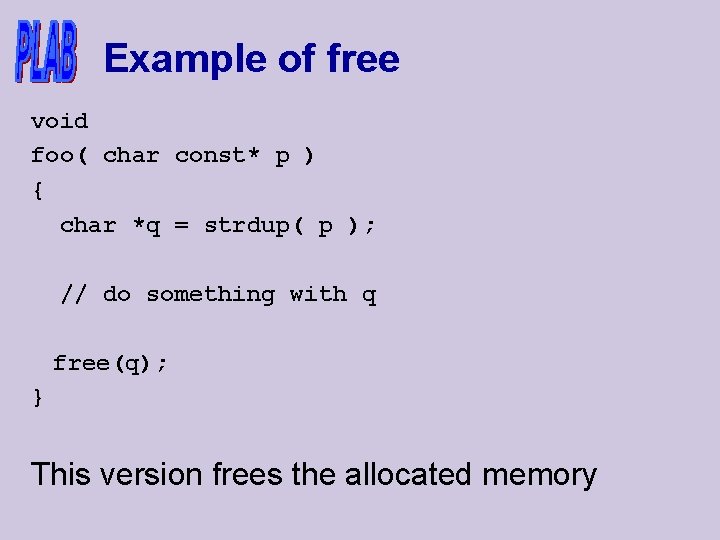
Example of free void foo( char const* p ) { char *q = strdup( p ); // do something with q free(q); } This version frees the allocated memory
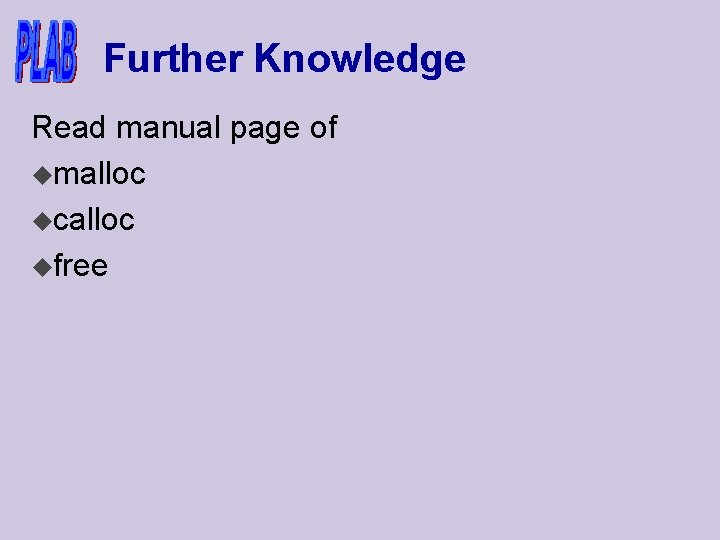
Further Knowledge Read manual page of umalloc ucalloc ufree
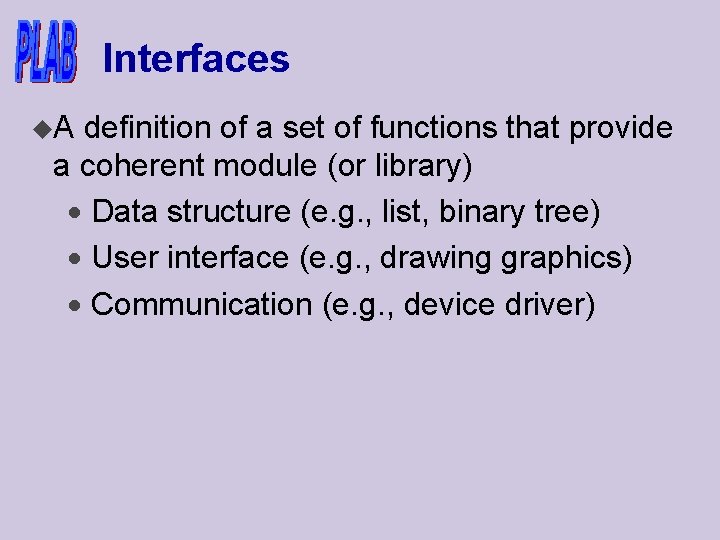
Interfaces u. A definition of a set of functions that provide a coherent module (or library) · Data structure (e. g. , list, binary tree) · User interface (e. g. , drawing graphics) · Communication (e. g. , device driver)
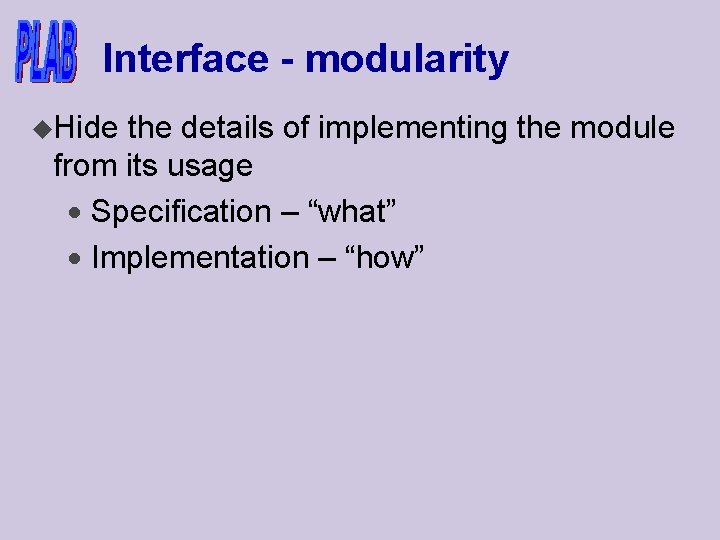
Interface - modularity u. Hide the details of implementing the module from its usage · Specification – “what” · Implementation – “how”
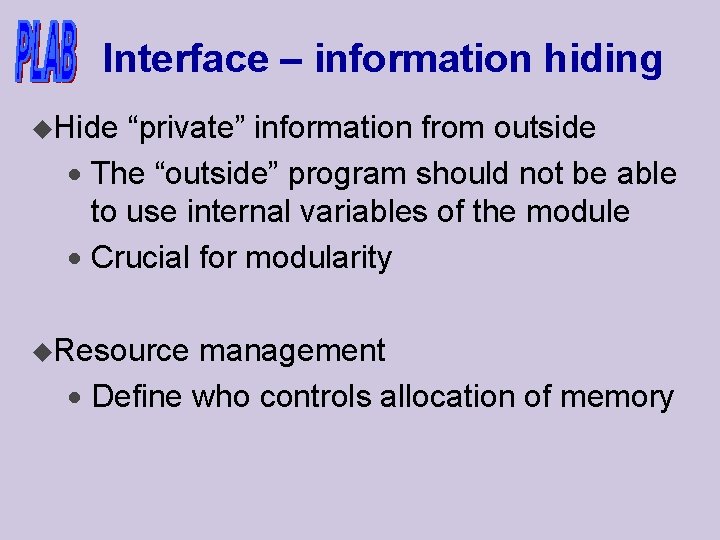
Interface – information hiding u. Hide “private” information from outside · The “outside” program should not be able to use internal variables of the module · Crucial for modularity u. Resource management · Define who controls allocation of memory
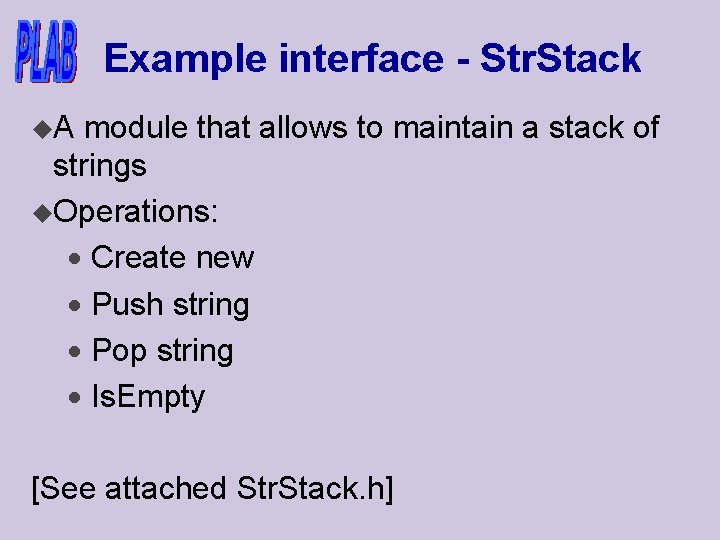
Example interface - Str. Stack u. A module that allows to maintain a stack of strings u. Operations: · Create new · Push string · Pop string · Is. Empty [See attached Str. Stack. h]
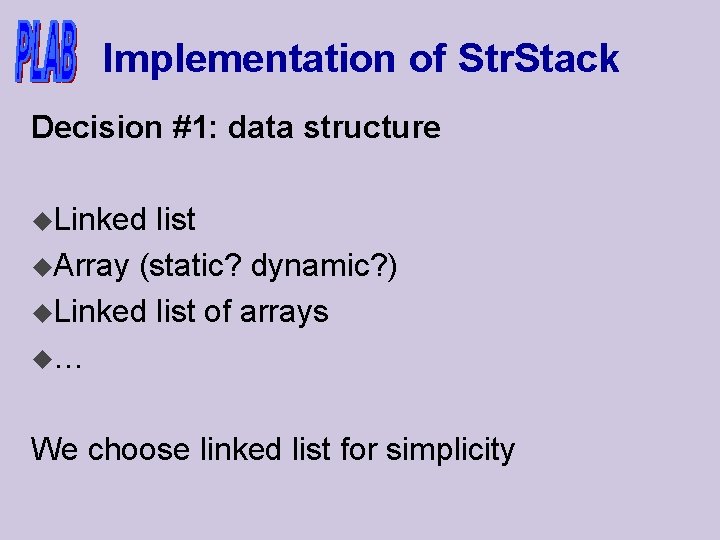
Implementation of Str. Stack Decision #1: data structure u. Linked list u. Array (static? dynamic? ) u. Linked list of arrays u… We choose linked list for simplicity
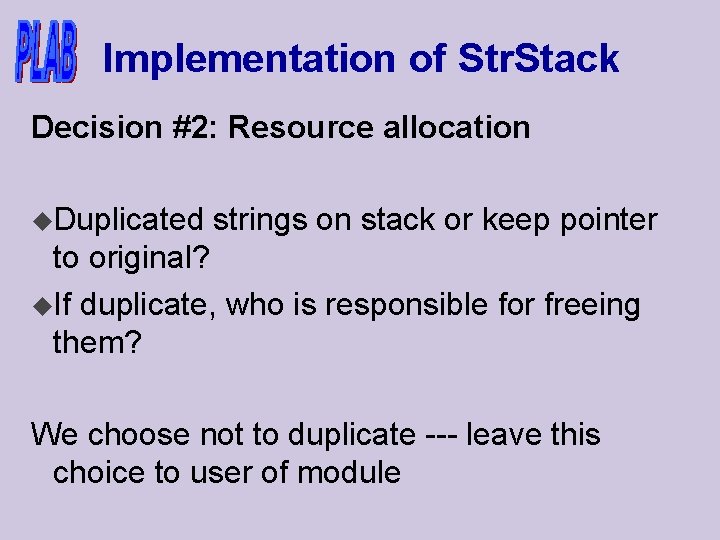
Implementation of Str. Stack Decision #2: Resource allocation u. Duplicated strings on stack or keep pointer to original? u. If duplicate, who is responsible for freeing them? We choose not to duplicate --- leave this choice to user of module
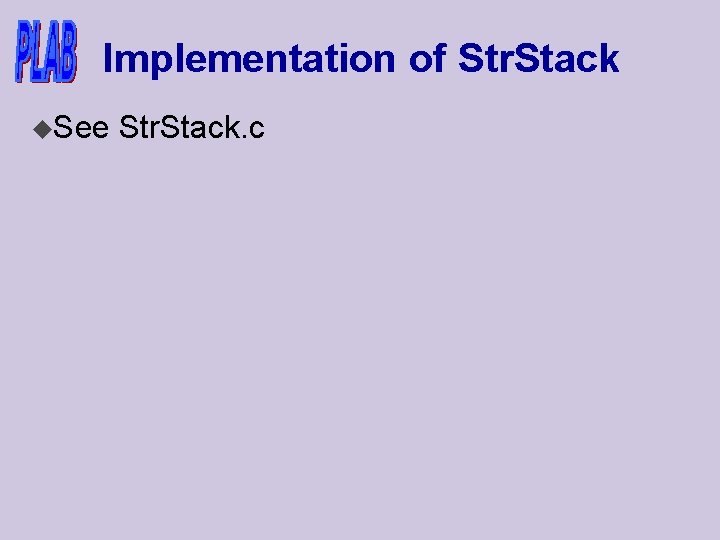
Implementation of Str. Stack u. See Str. Stack. c
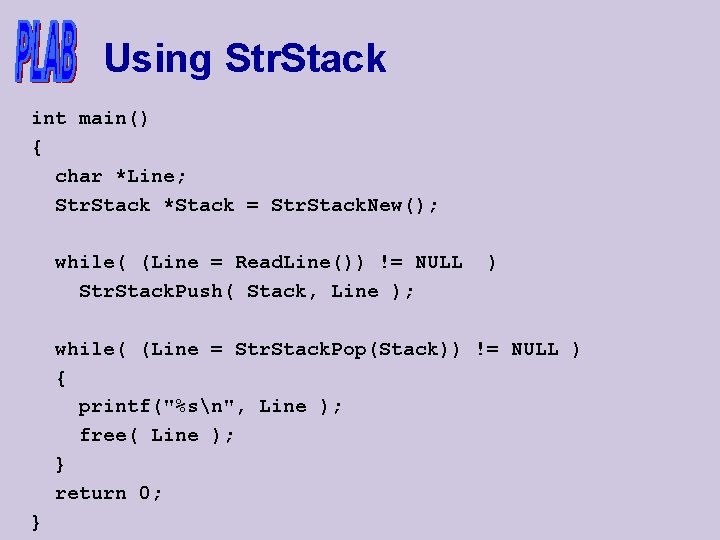
Using Str. Stack int main() { char *Line; Str. Stack *Stack = Str. Stack. New(); while( (Line = Read. Line()) != NULL Str. Stack. Push( Stack, Line ); ) while( (Line = Str. Stack. Pop(Stack)) != NULL ) { printf("%sn", Line ); free( Line ); } return 0; }
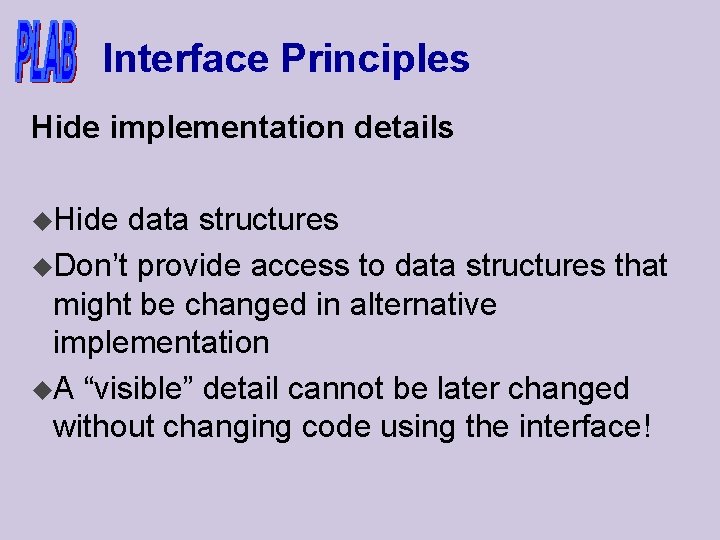
Interface Principles Hide implementation details u. Hide data structures u. Don’t provide access to data structures that might be changed in alternative implementation u. A “visible” detail cannot be later changed without changing code using the interface!
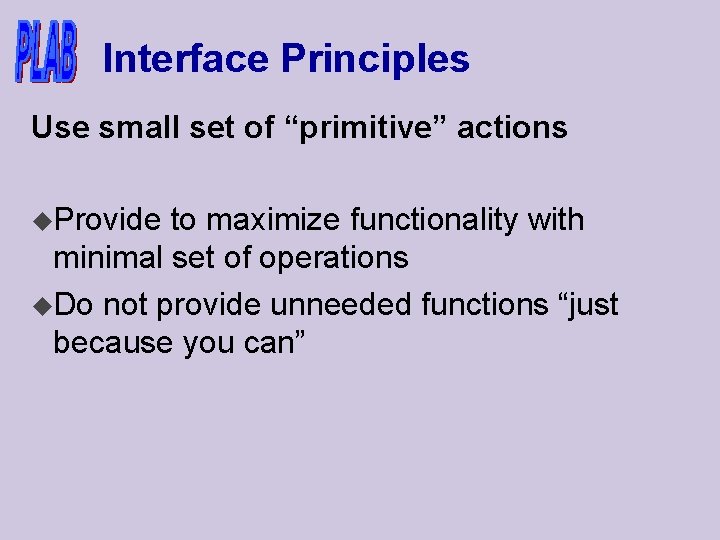
Interface Principles Use small set of “primitive” actions u. Provide to maximize functionality with minimal set of operations u. Do not provide unneeded functions “just because you can”
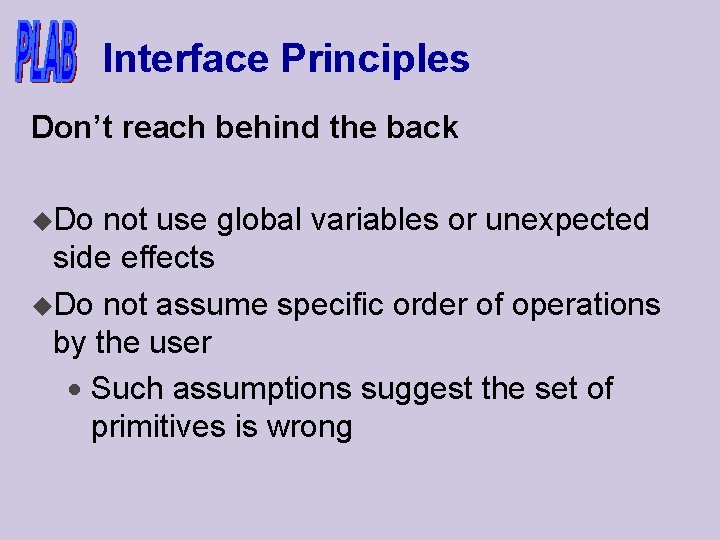
Interface Principles Don’t reach behind the back u. Do not use global variables or unexpected side effects u. Do not assume specific order of operations by the user · Such assumptions suggest the set of primitives is wrong
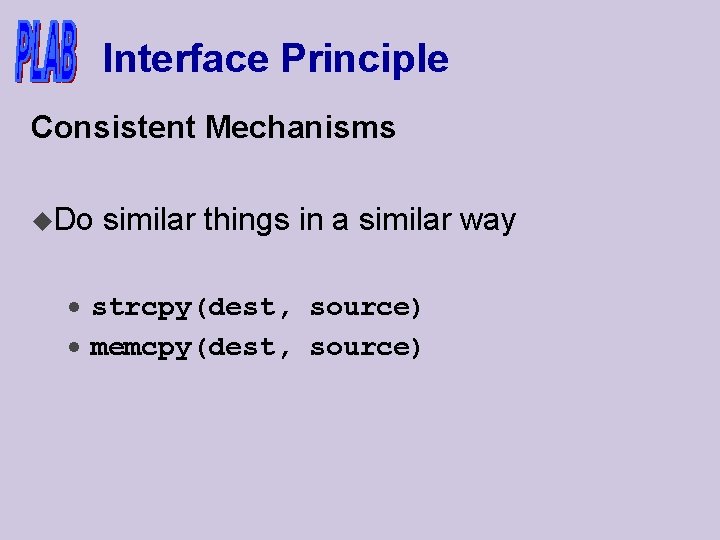
Interface Principle Consistent Mechanisms u. Do similar things in a similar way · strcpy(dest, source) · memcpy(dest, source)
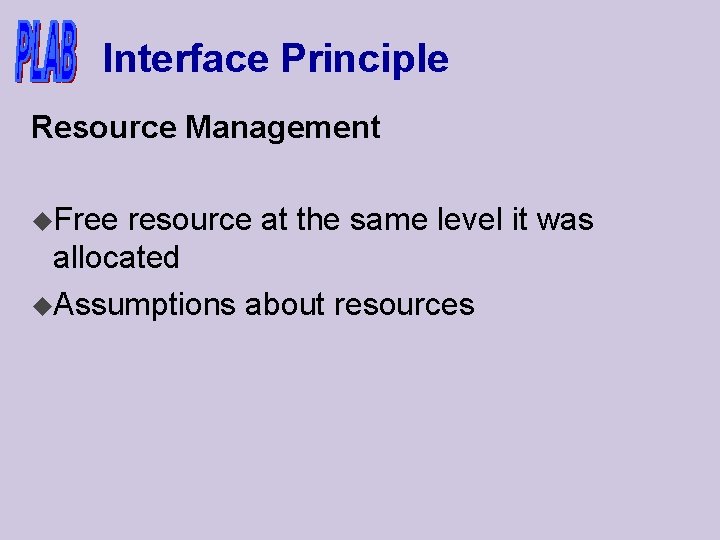
Interface Principle Resource Management u. Free resource at the same level it was allocated u. Assumptions about resources
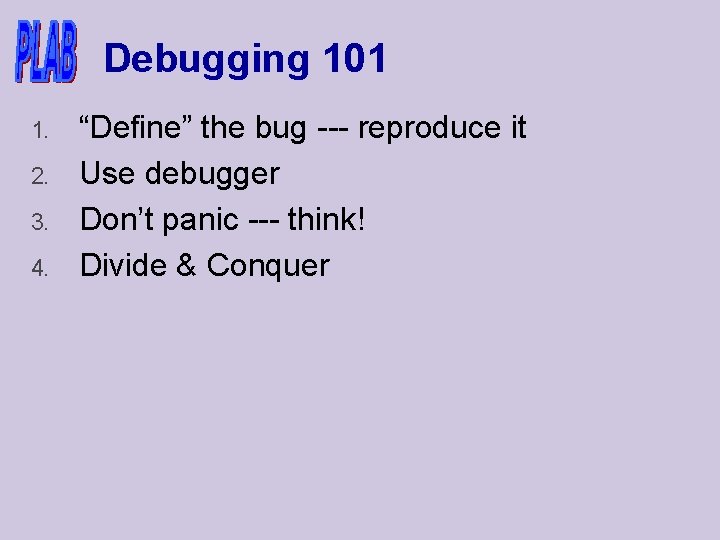
Debugging 101 1. 2. 3. 4. “Define” the bug --- reproduce it Use debugger Don’t panic --- think! Divide & Conquer
Subdivision of runtime memory in compiler design
Runtime vs compile time
Long run perfect competition equilibrium
Run lola run butterfly effect
Run lola run editing techniques
Short run vs long run economics
Multirule
Short run and long run equilibrium in perfect competition
Run lola run script
Lolas nn
Managing holistic marketing organization
For minutes. start.
Process organization in computer organization
Organization by point
Best time to run fans to aerate grain
Asymptotic cheat sheet
Logic error
Four students run up the stairs in the time shown
Vulkan run time libraries co to jest
The control which is invisible at runtime
Physical memory organisation of 8086
Internal memory organization of 8051 microcontroller
Memory cycle of plc
High order interleaving
Cache memory organization
Cache memory organization
Internal organization of memory chips
Memory organization in computer architecture
Modello von neumann
Virtual memory organization
What is memory organization
Address decoding in 8085
Prototype in semantics
Excplicit memory
Long term memory vs short term memory
Internal memory and external memory
Primary memory and secondary memory