Lecture 5 Heap Sort Priority queue and Heapsort
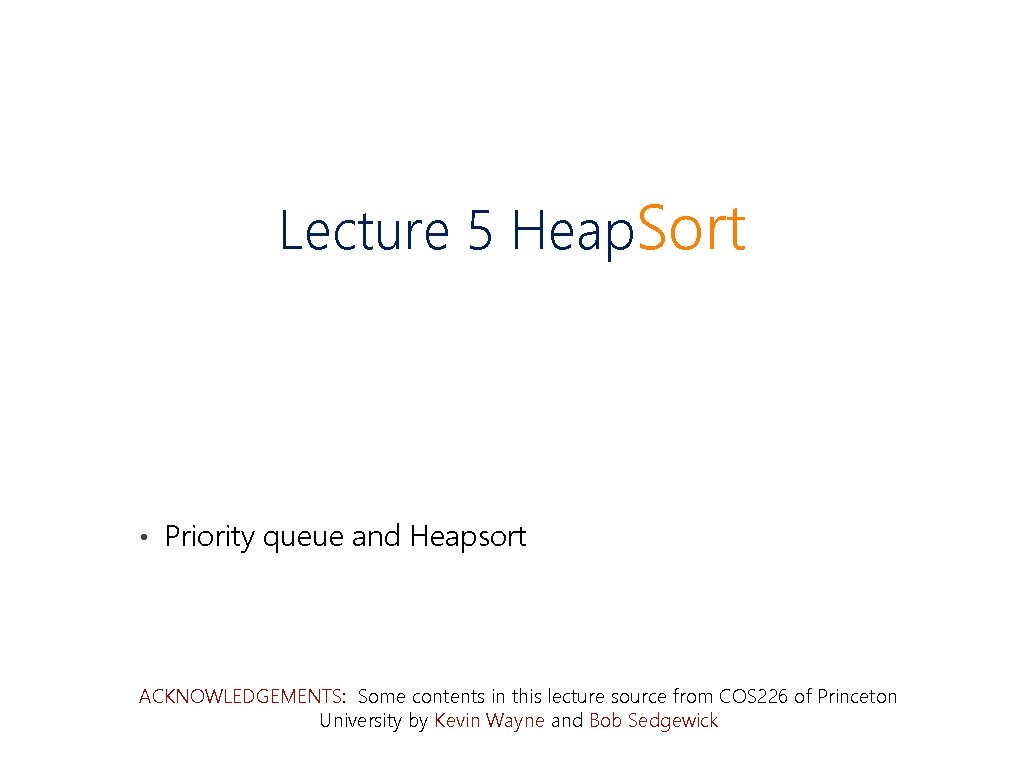
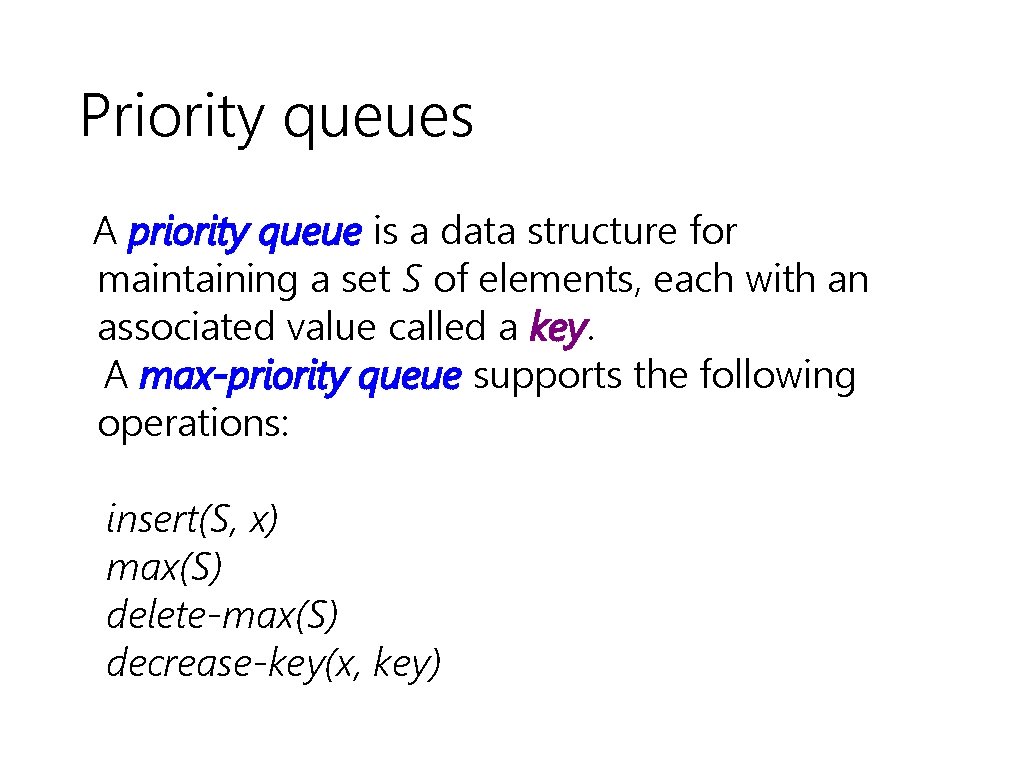
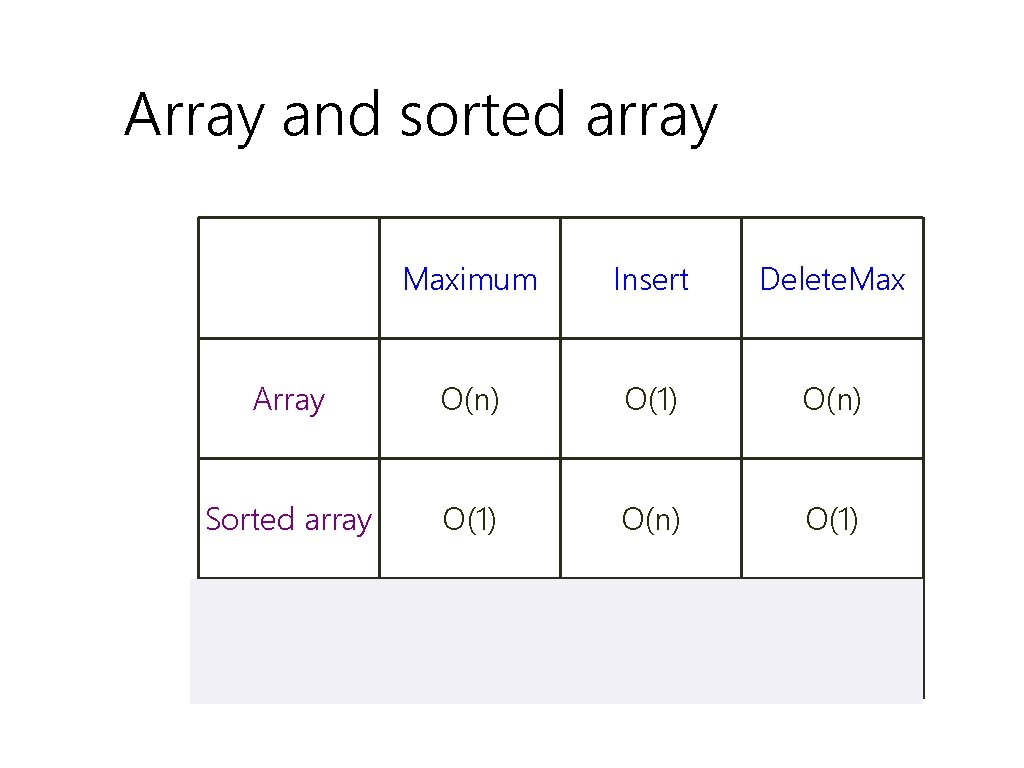
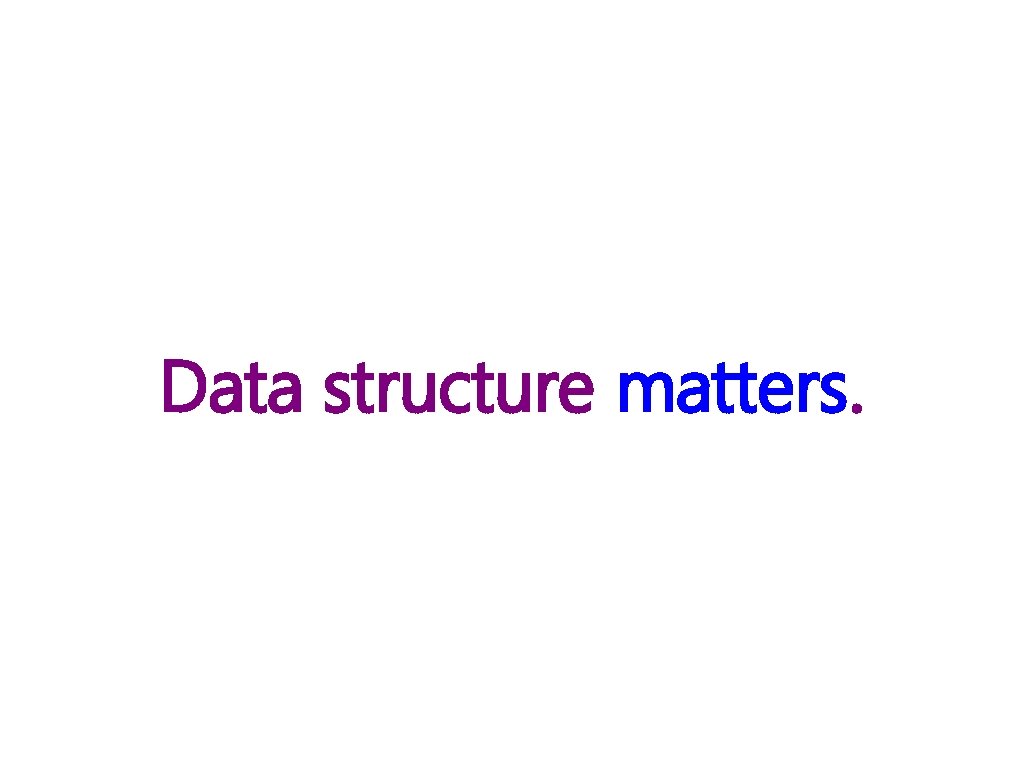
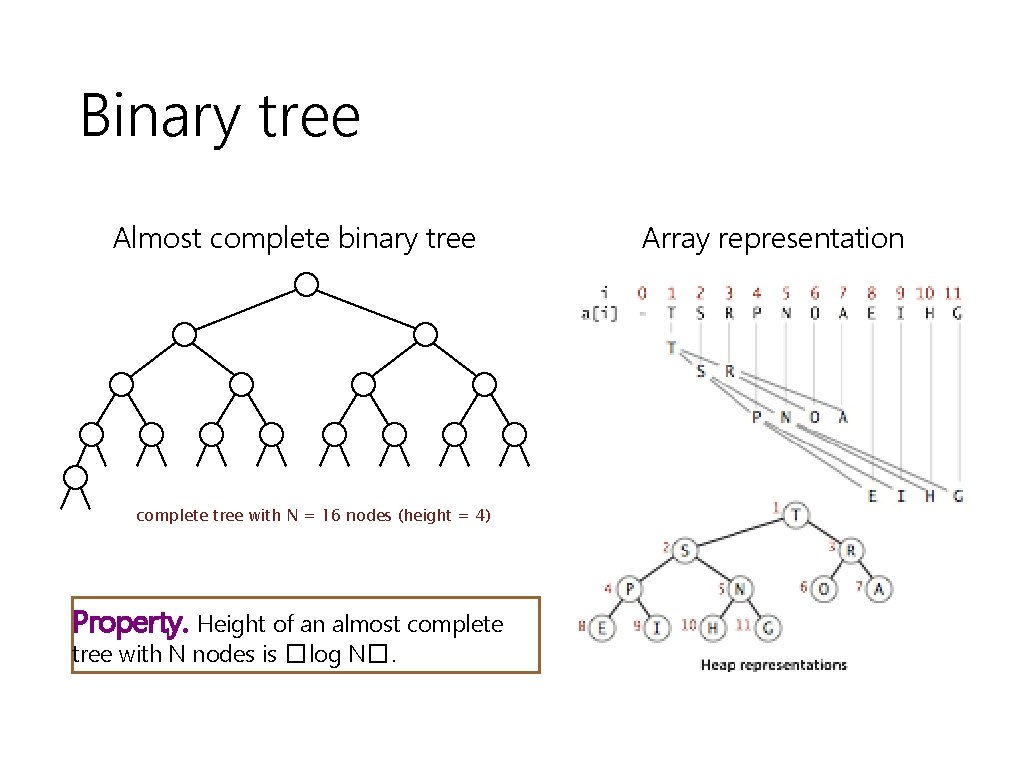
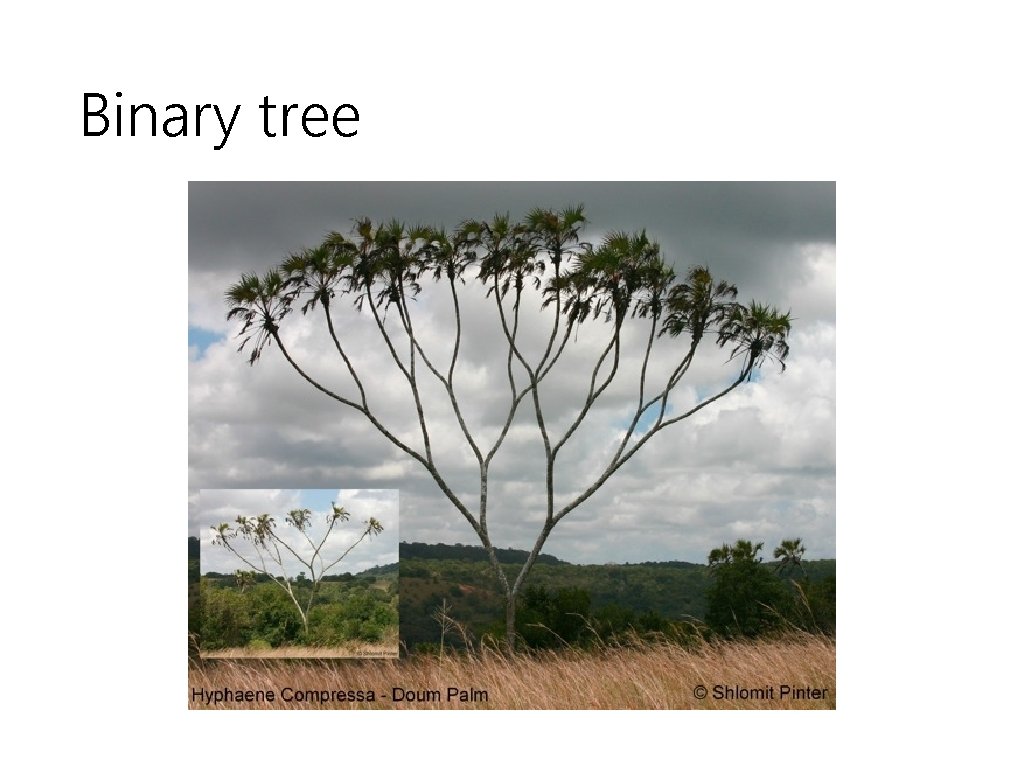
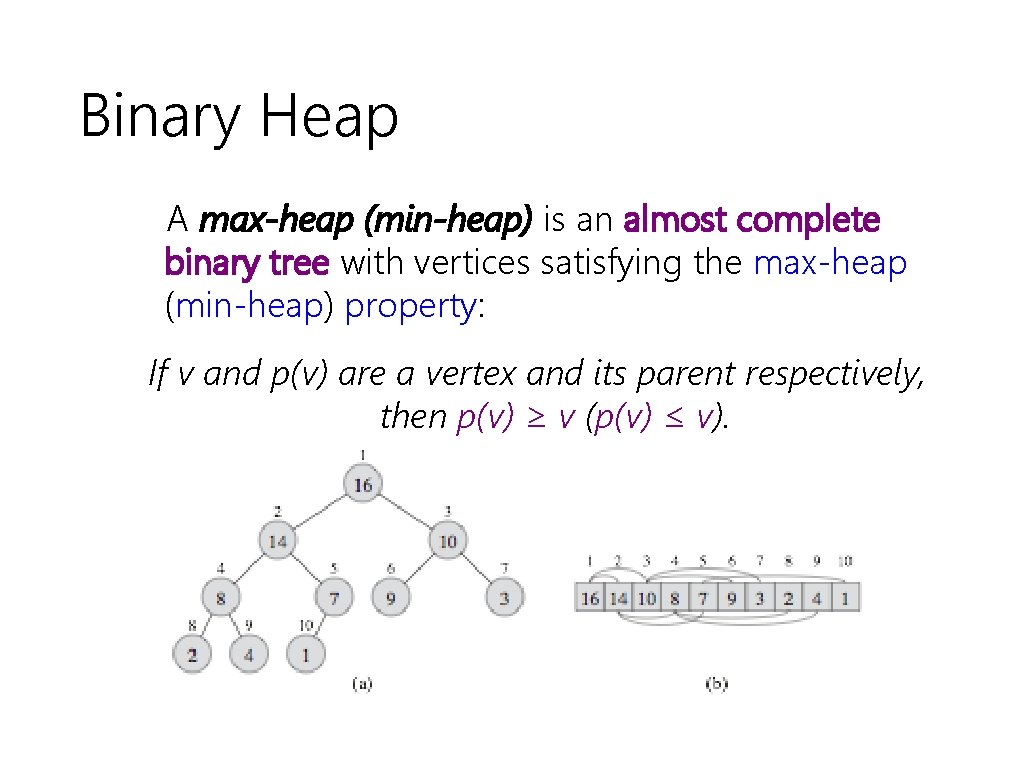
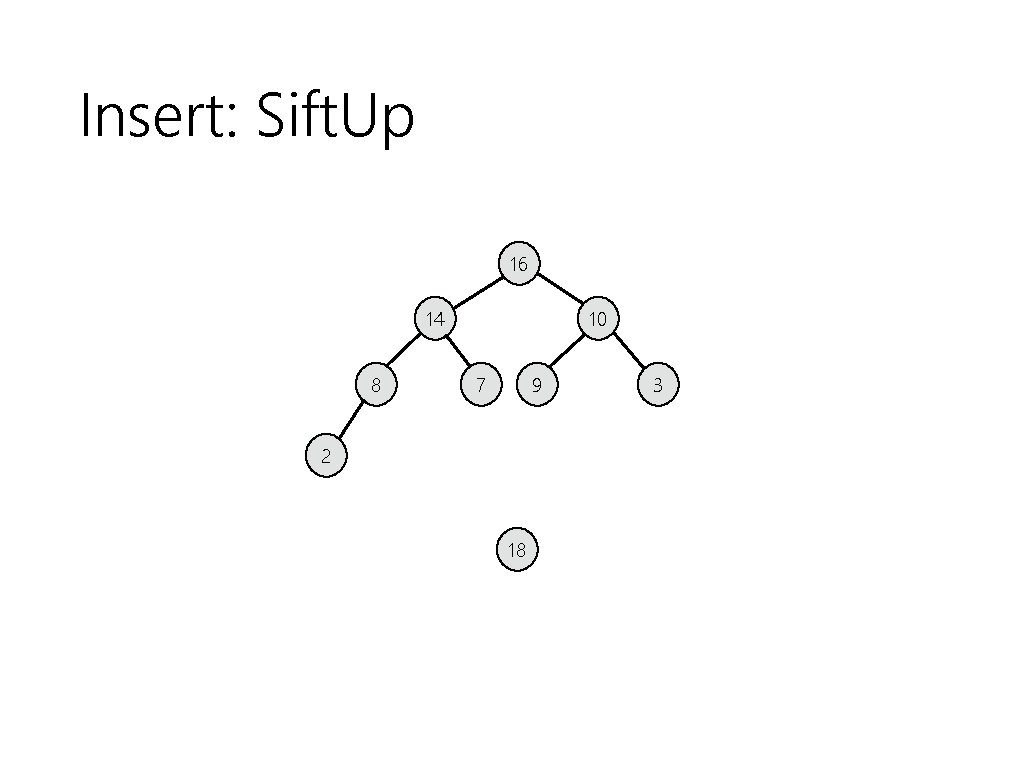
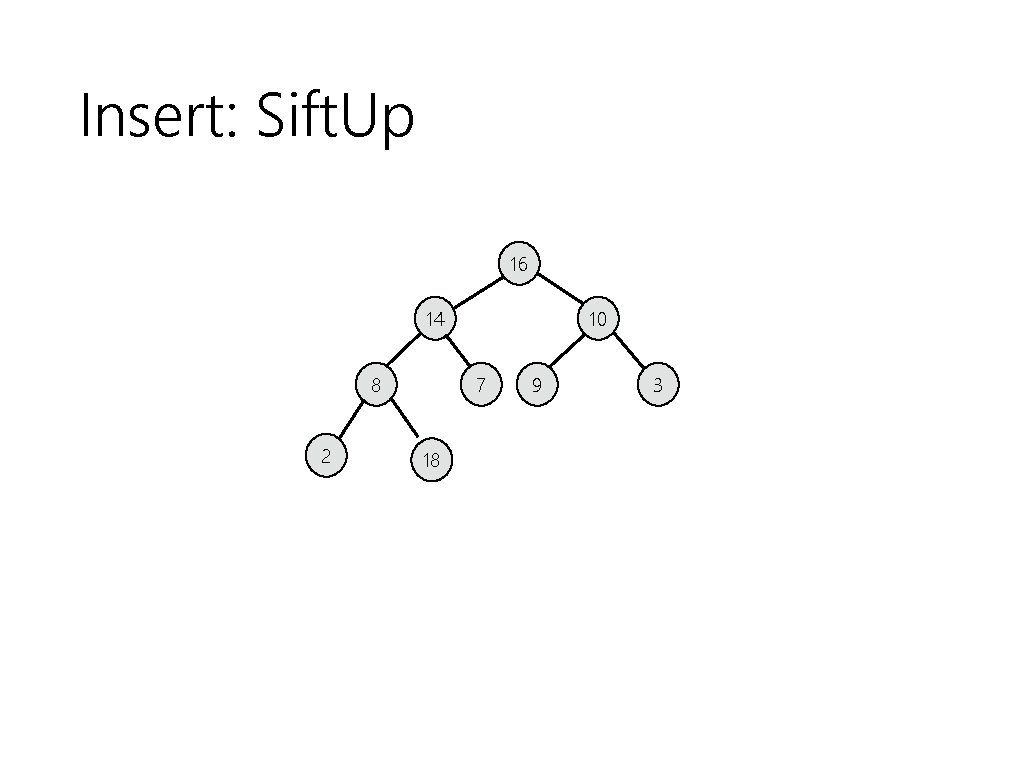
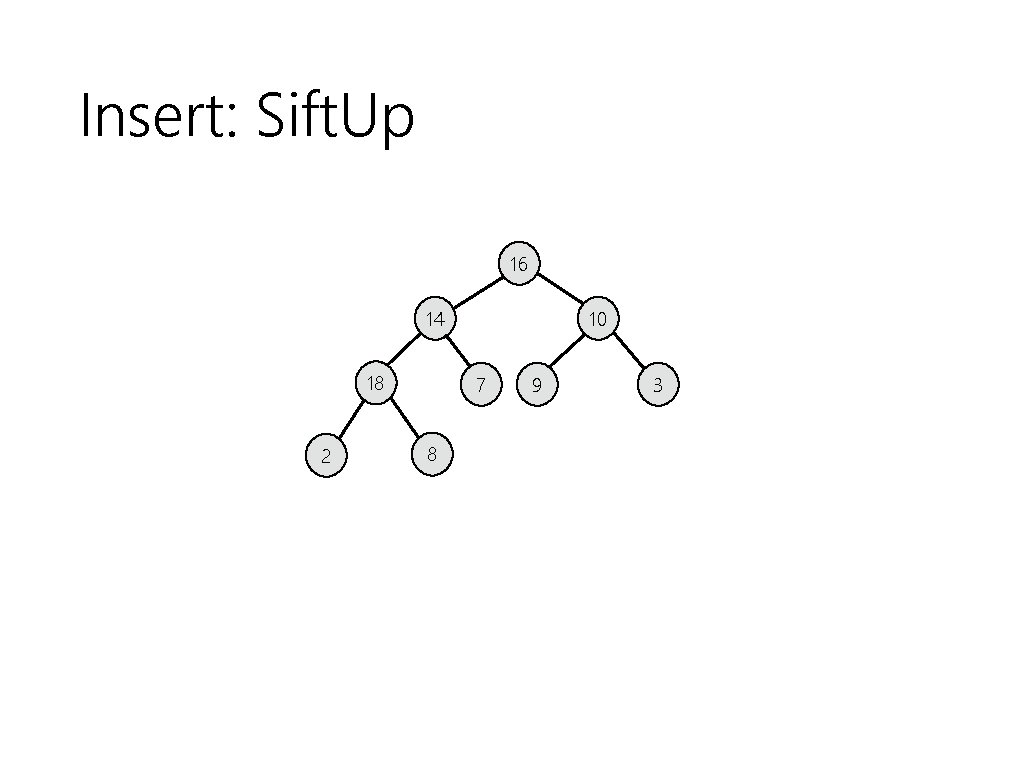
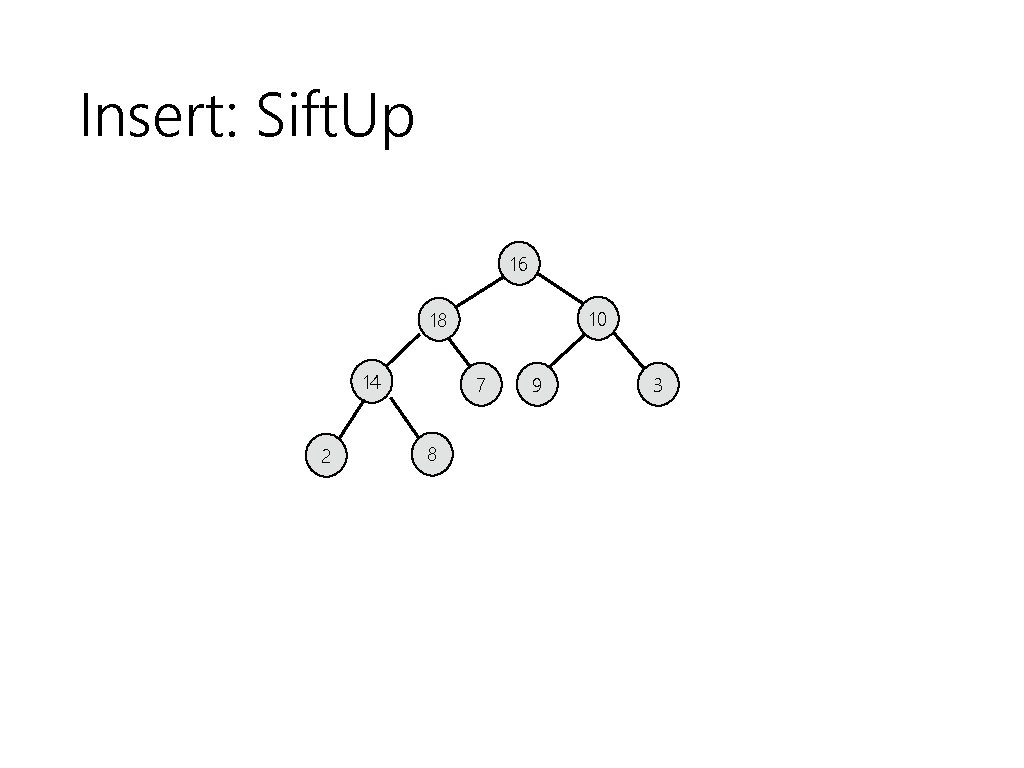
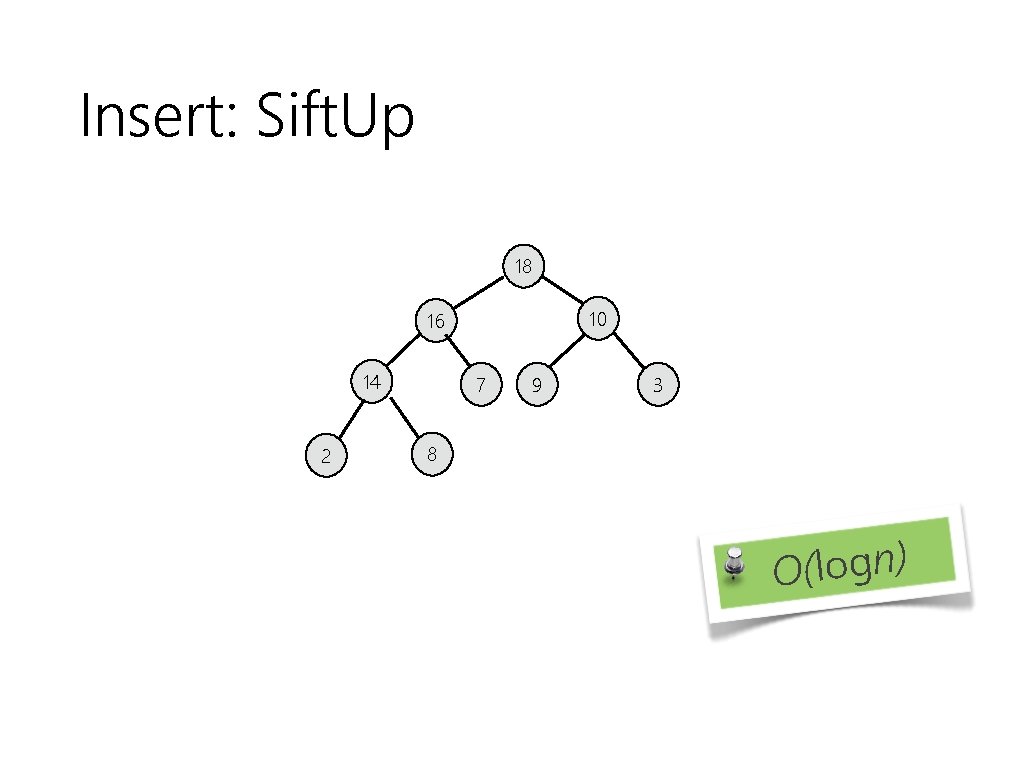
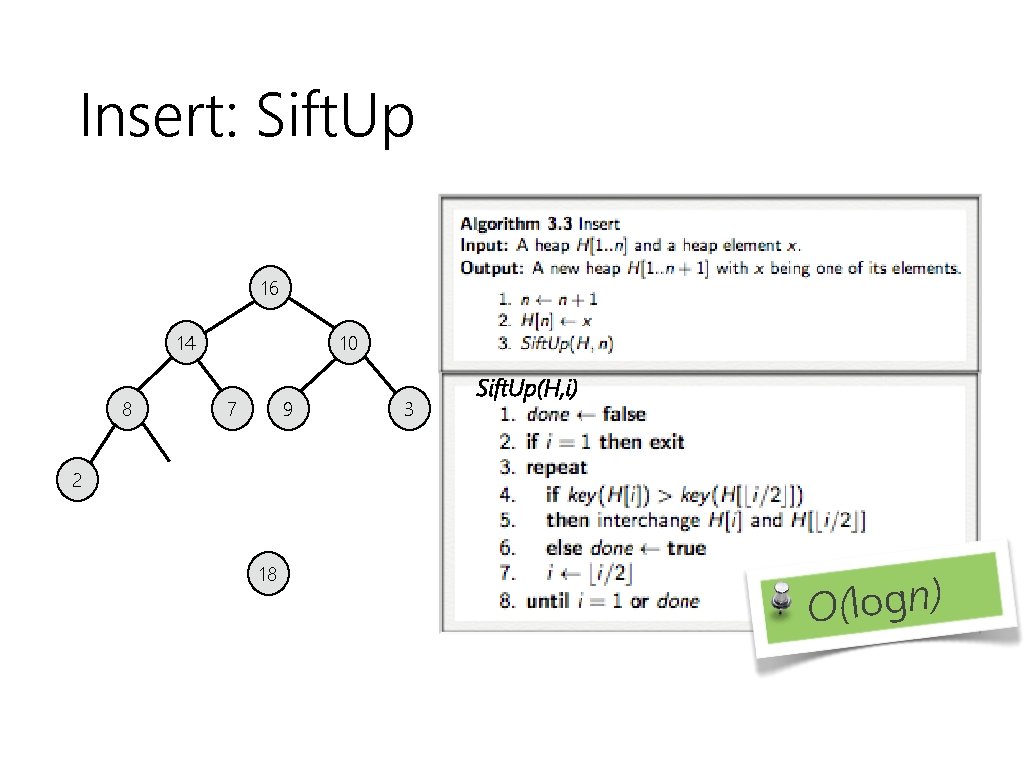
![Maximum 16 14 8 2 10 7 9 3 4 return H[1]; Θ(1) Maximum 16 14 8 2 10 7 9 3 4 return H[1]; Θ(1)](https://slidetodoc.com/presentation_image_h/183eebe2d038f216738a8e95d090d3bd/image-14.jpg)
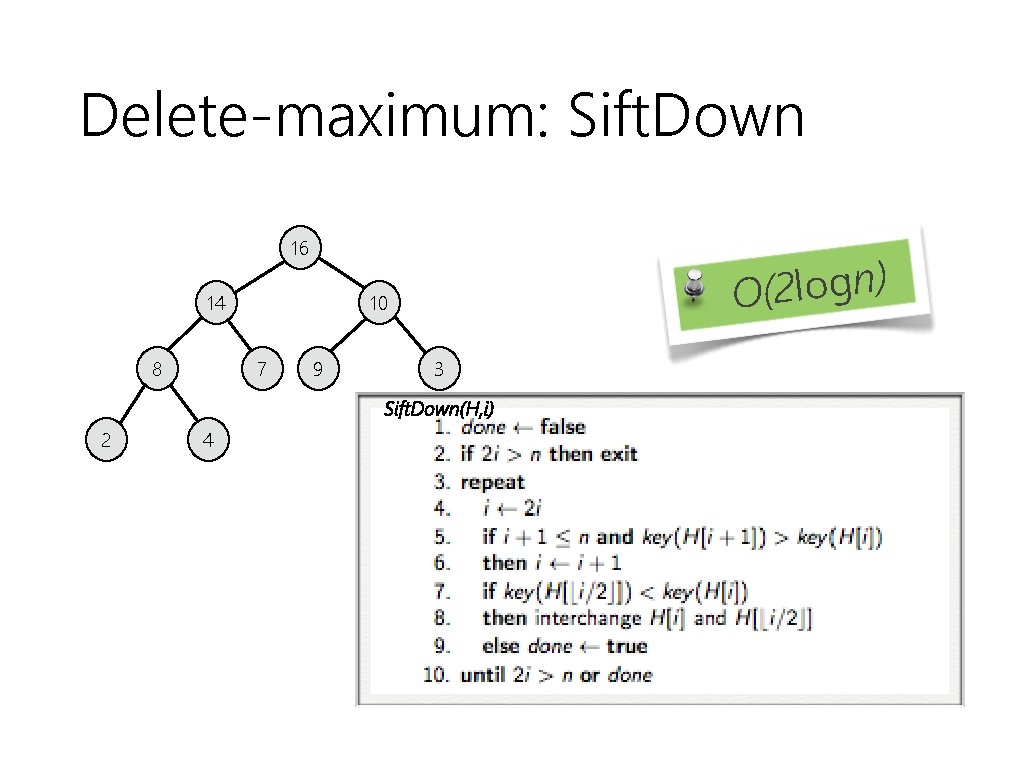
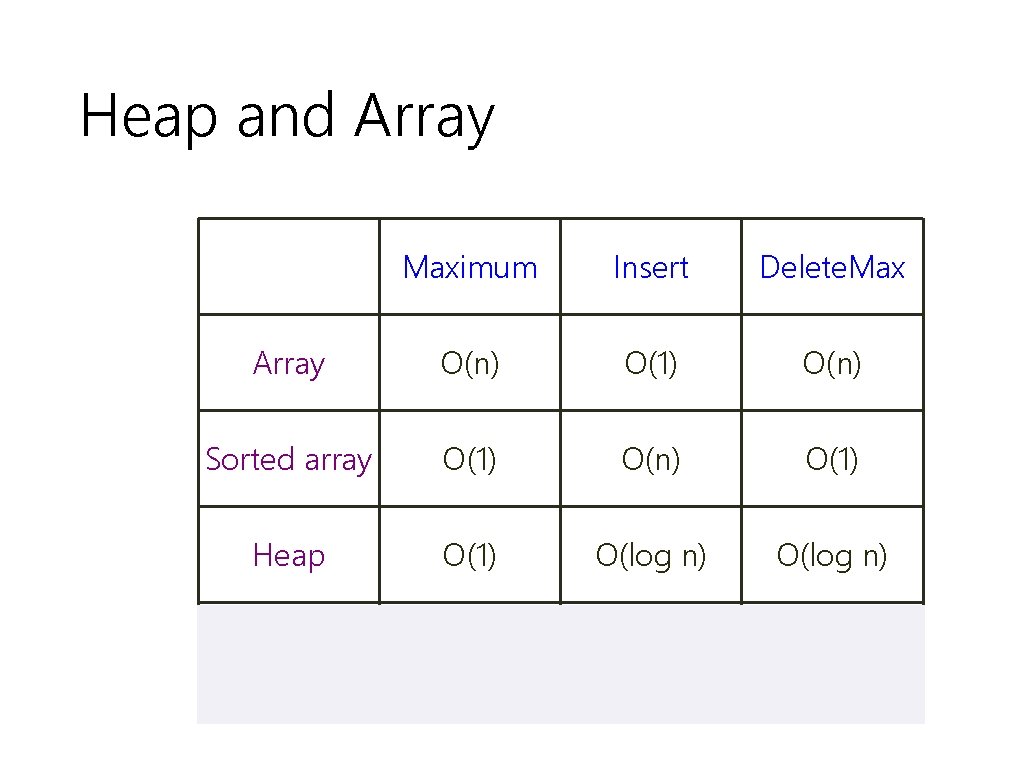
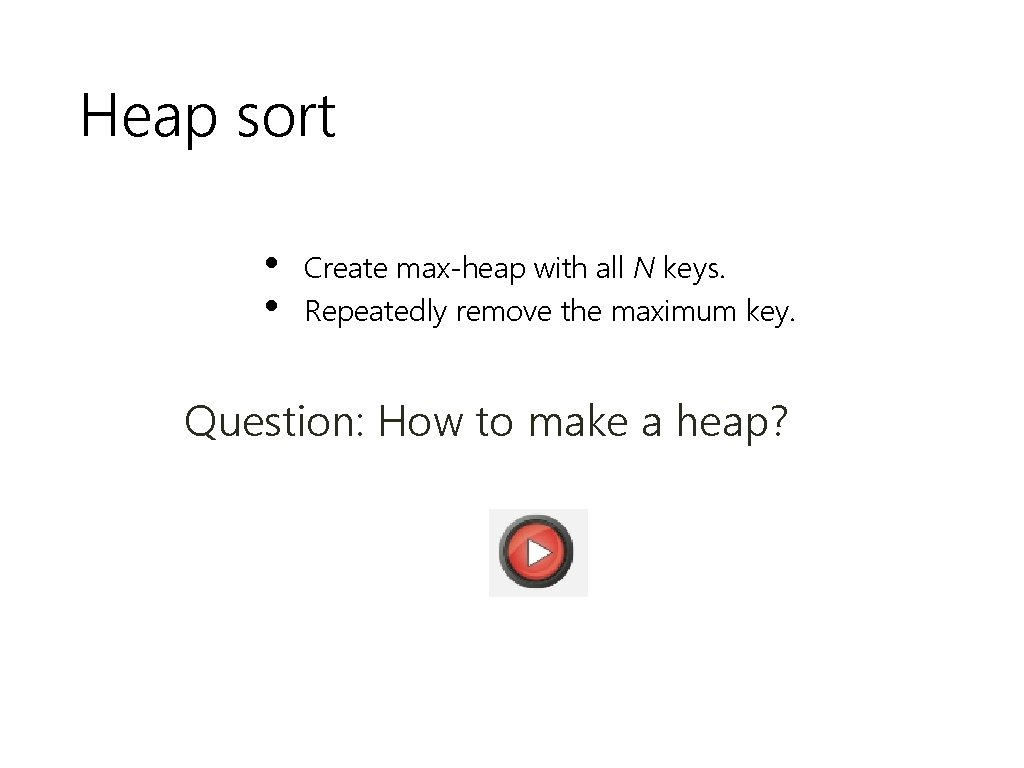
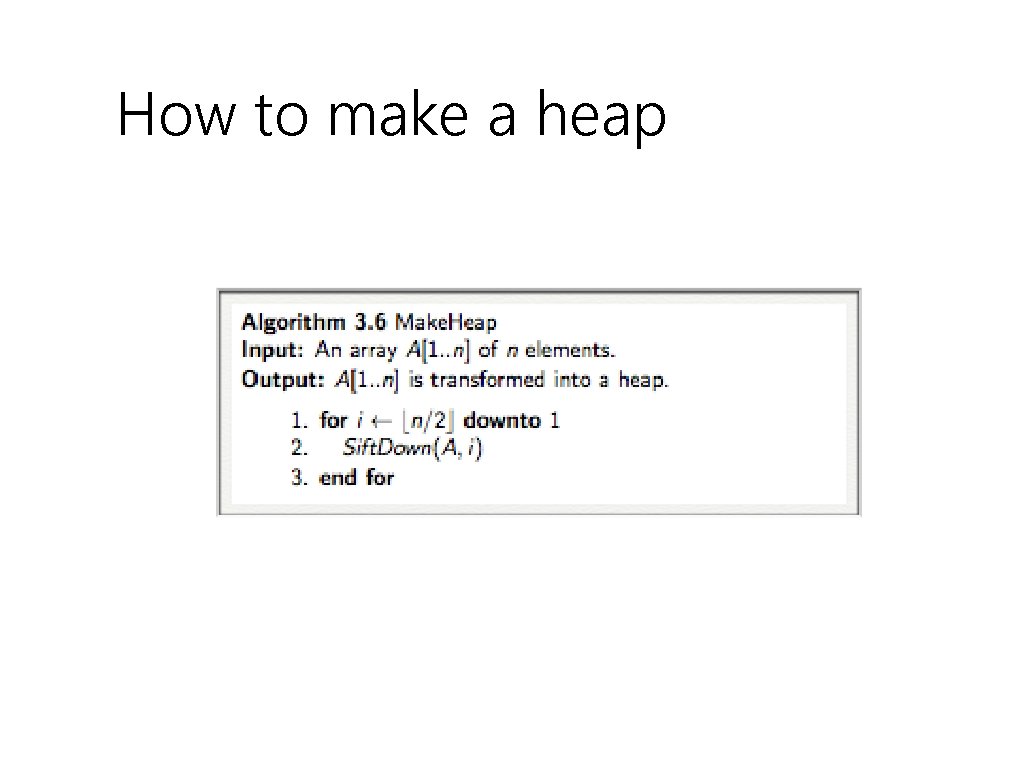
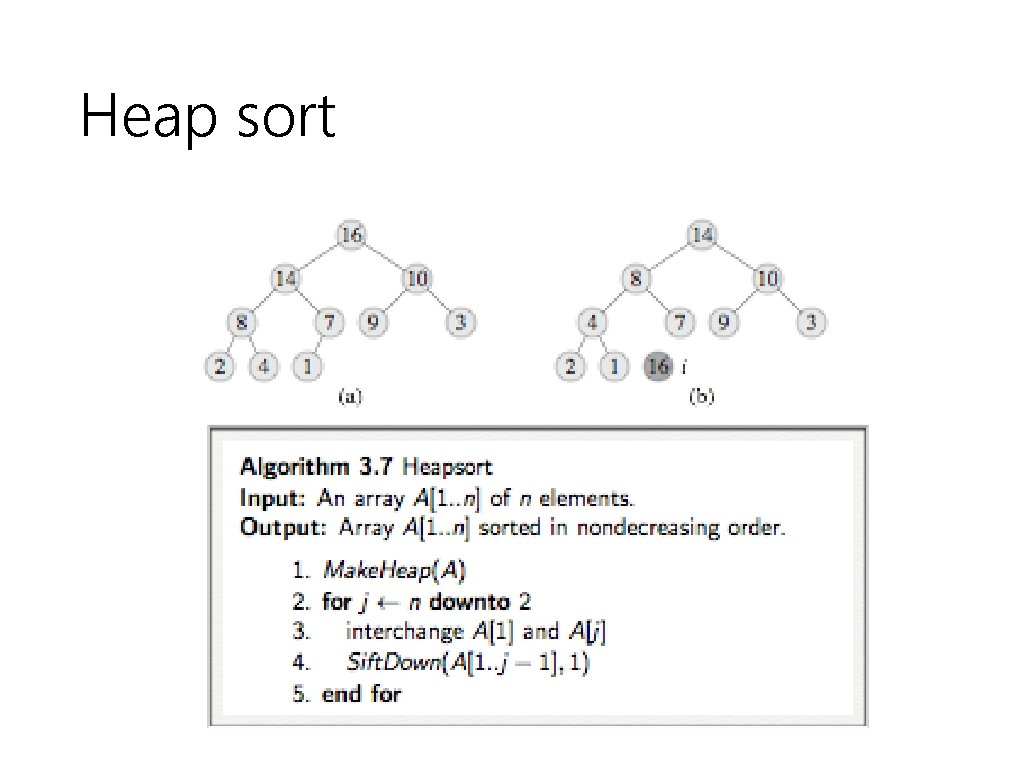
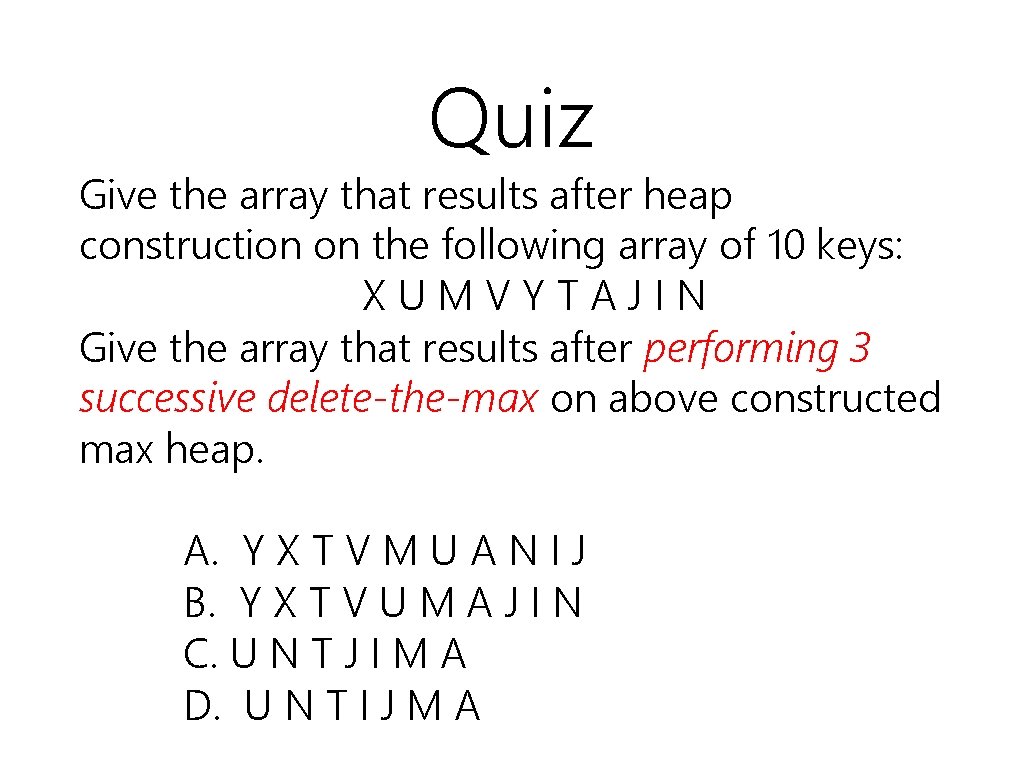
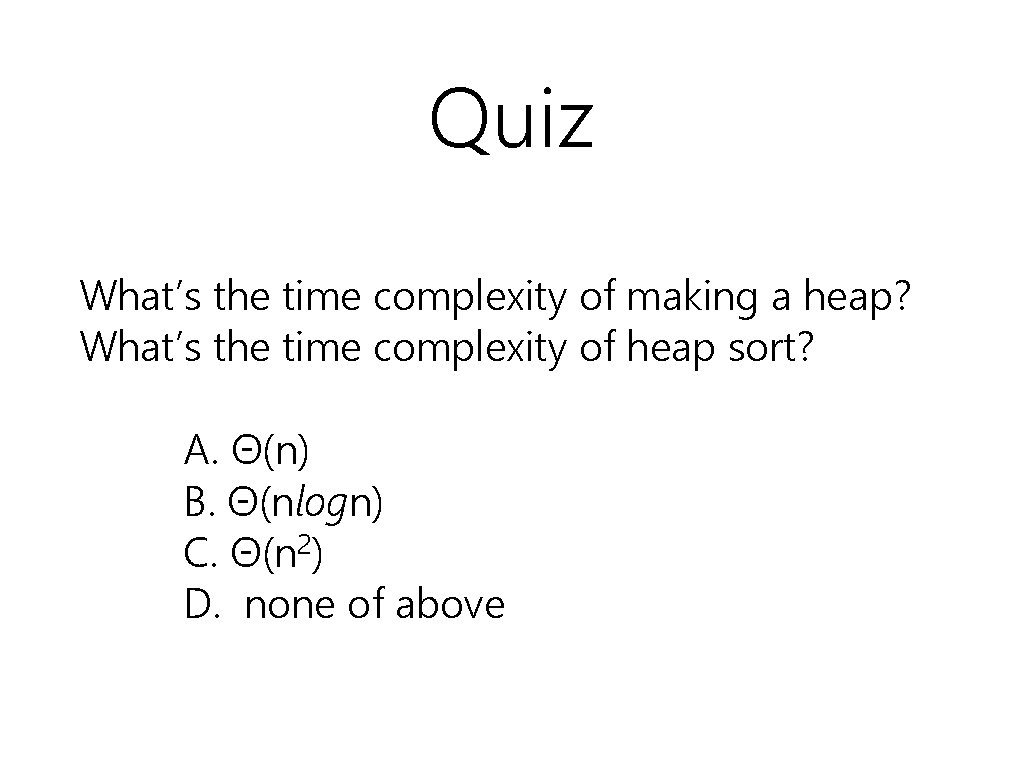
![Applications • Event-driven simulation. [customers in a line] • Data compression. [Huffman codes] • Applications • Event-driven simulation. [customers in a line] • Data compression. [Huffman codes] •](https://slidetodoc.com/presentation_image_h/183eebe2d038f216738a8e95d090d3bd/image-22.jpg)
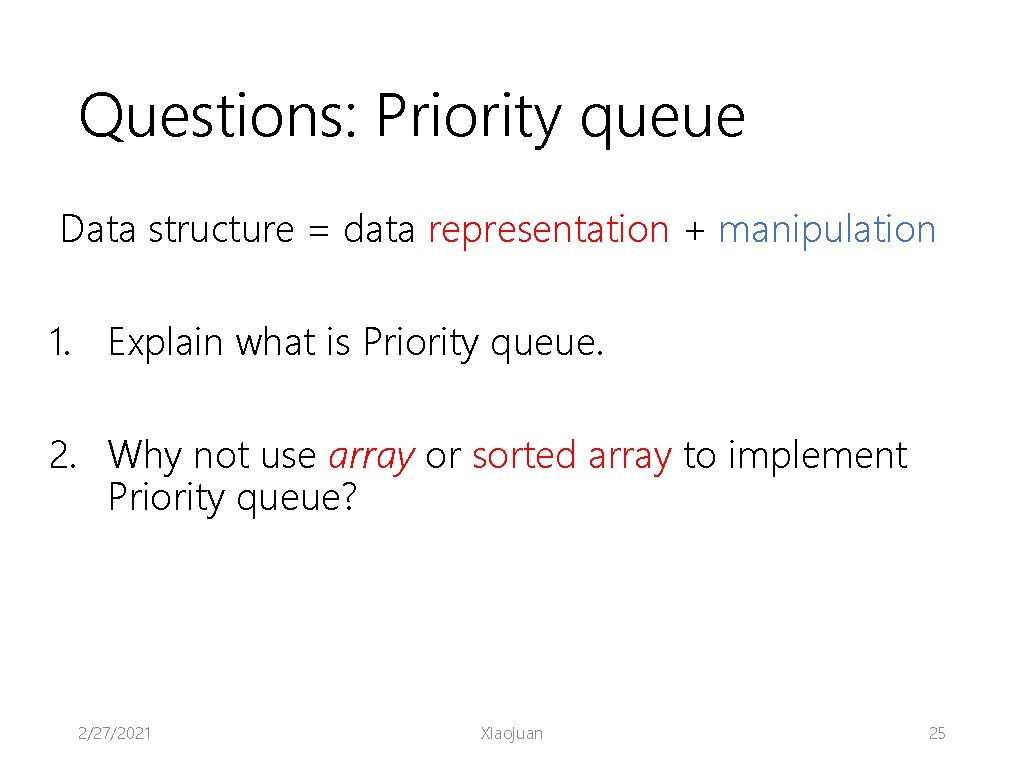
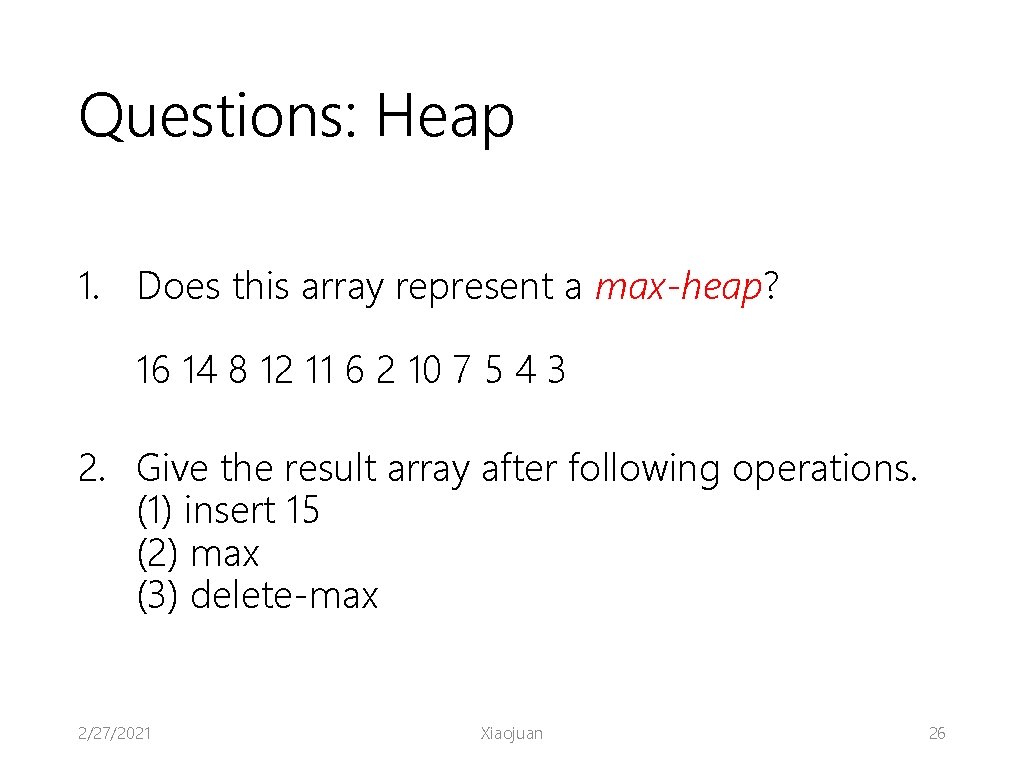
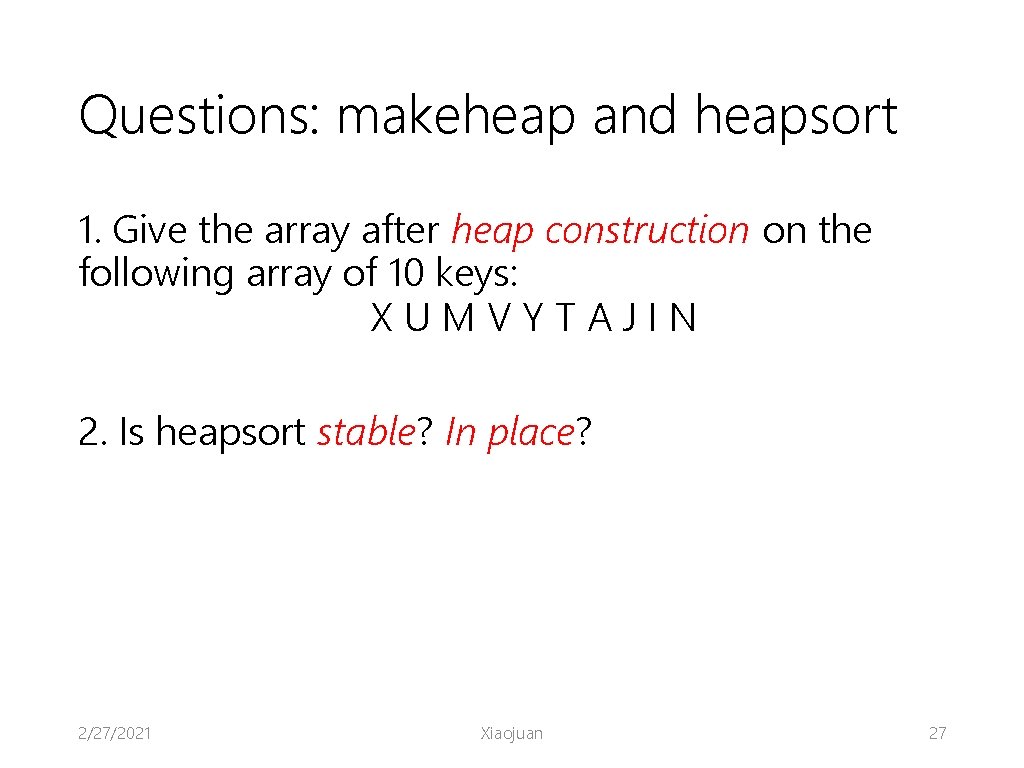
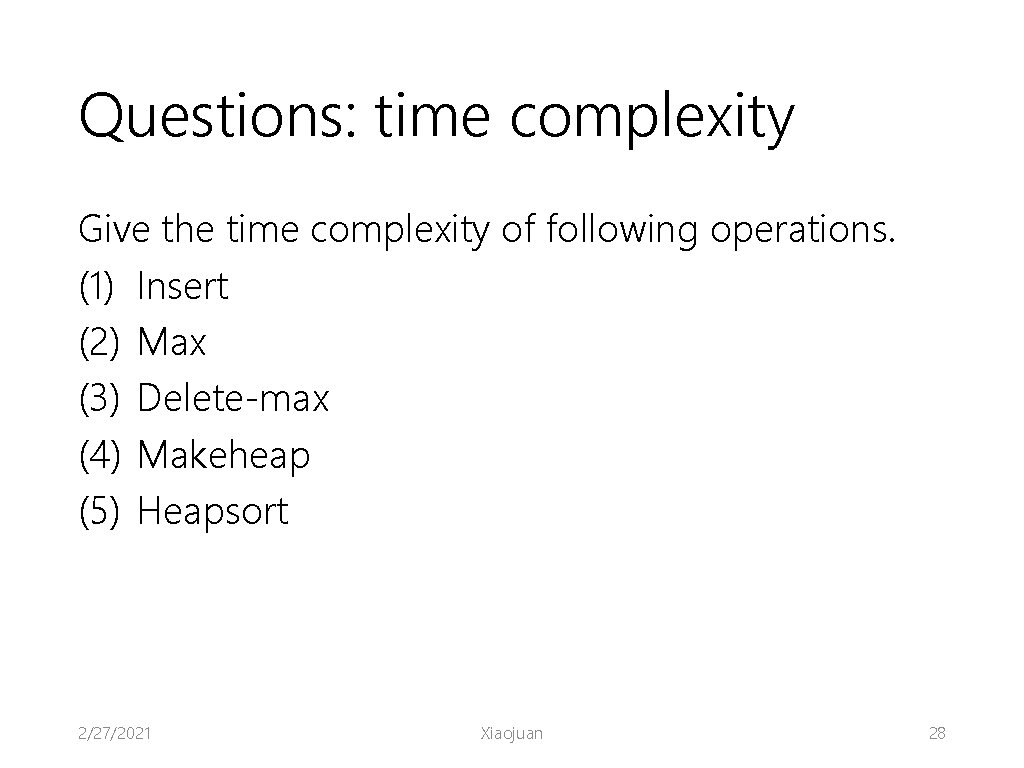
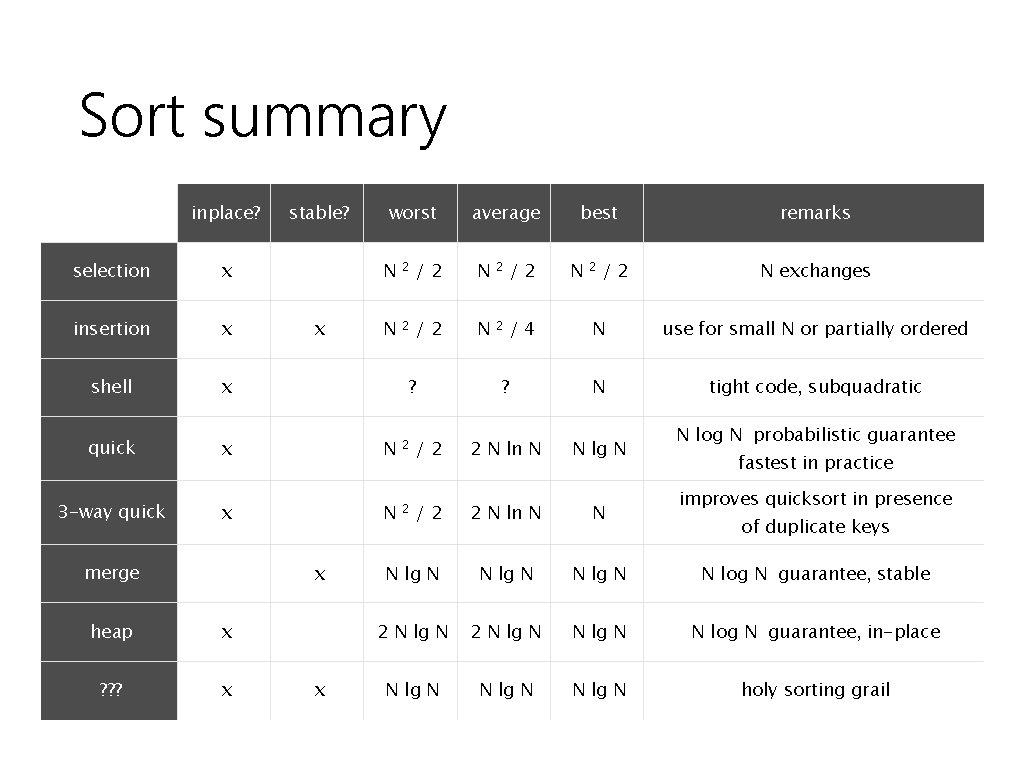
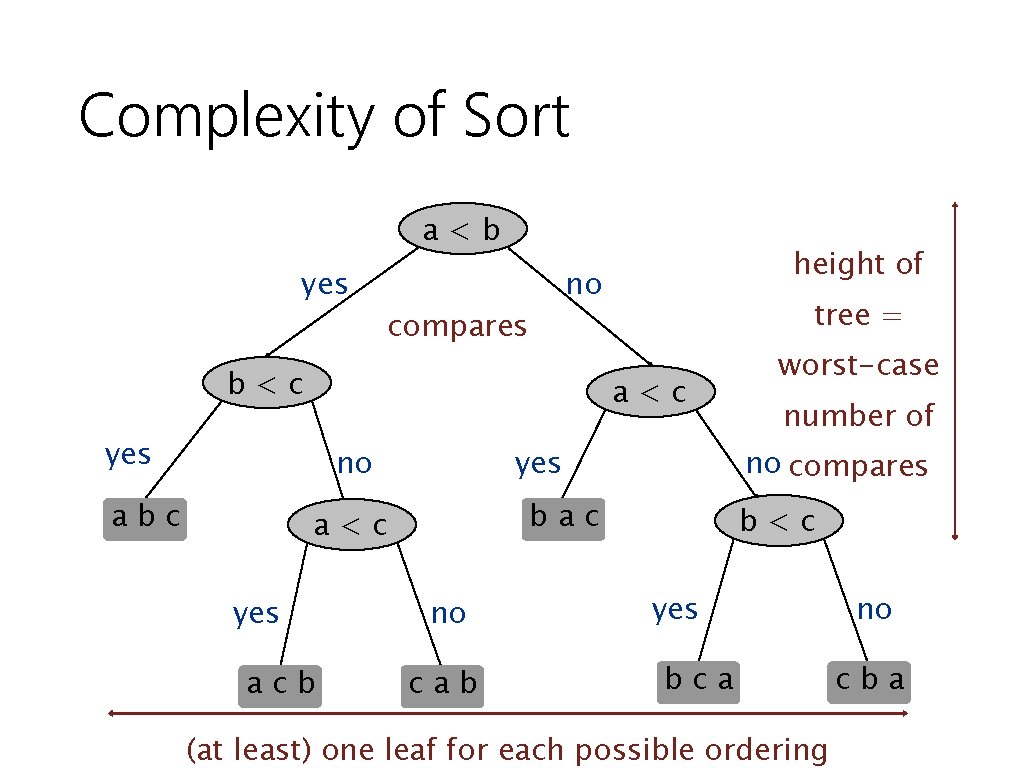
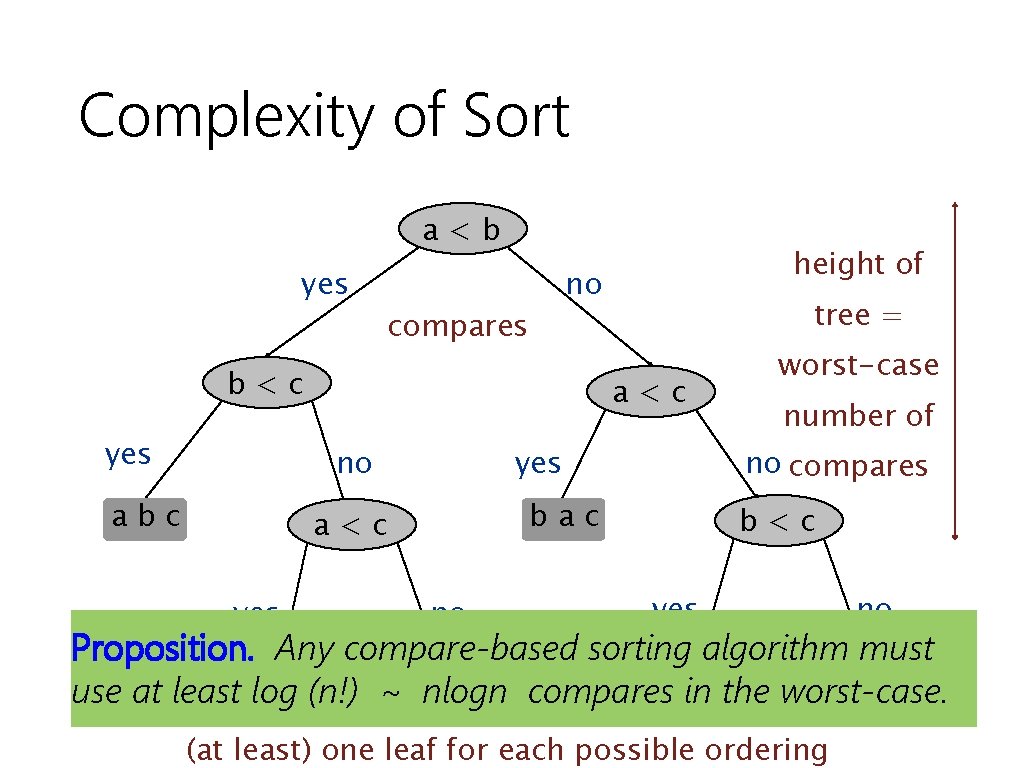
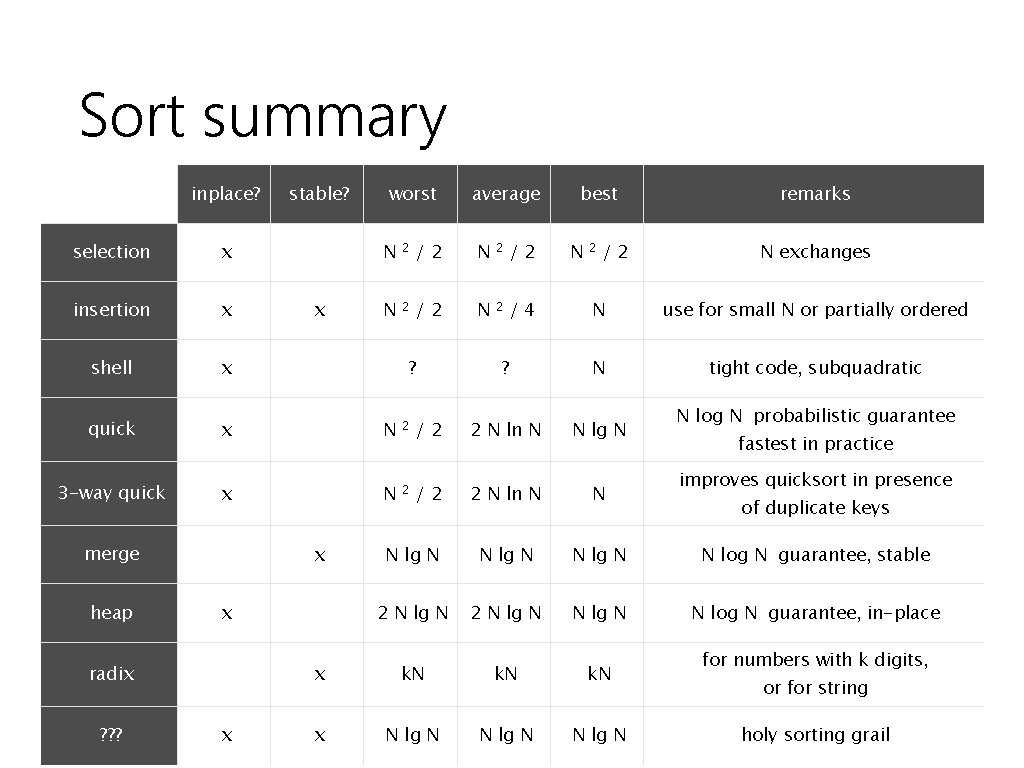
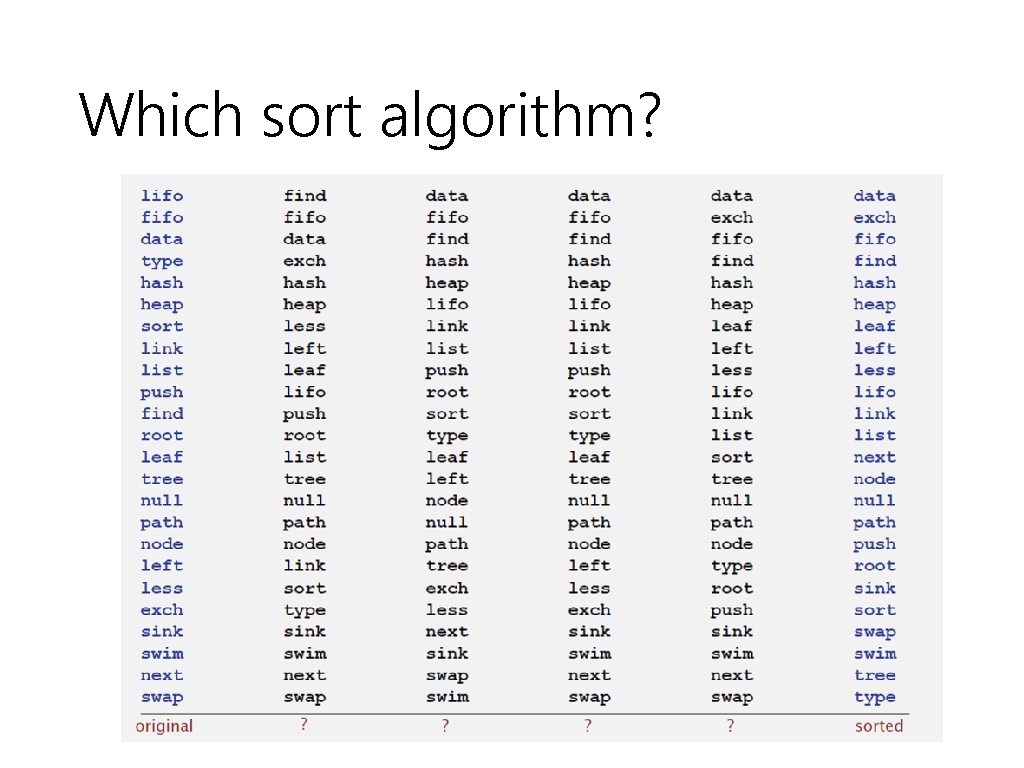
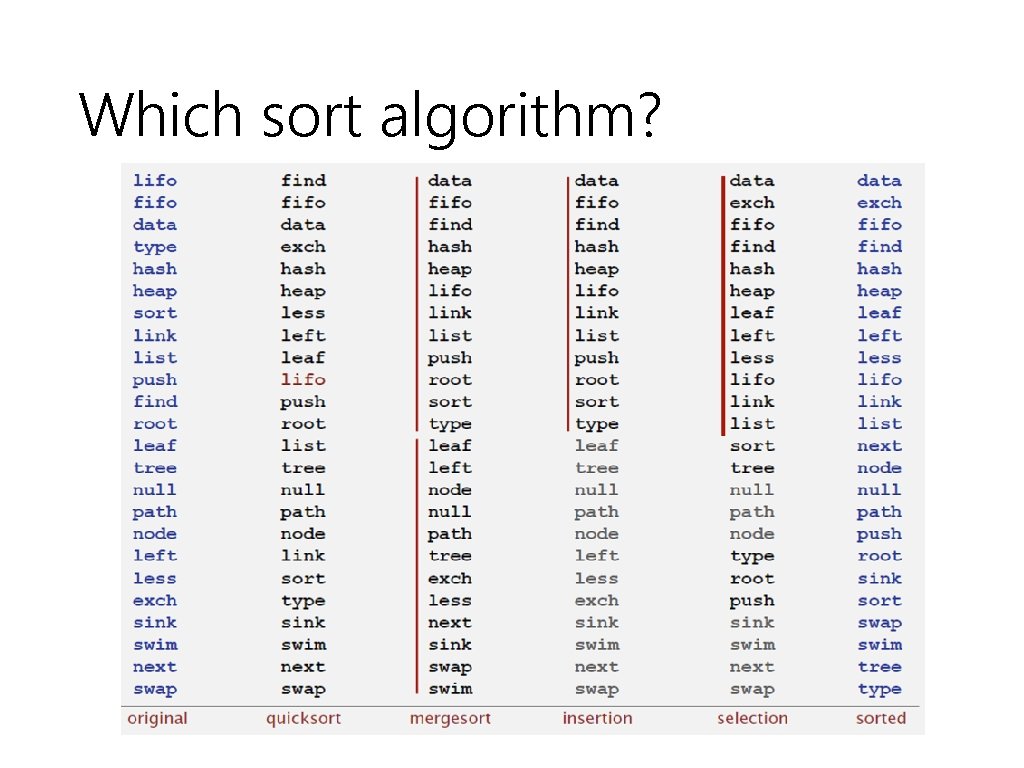
- Slides: 32
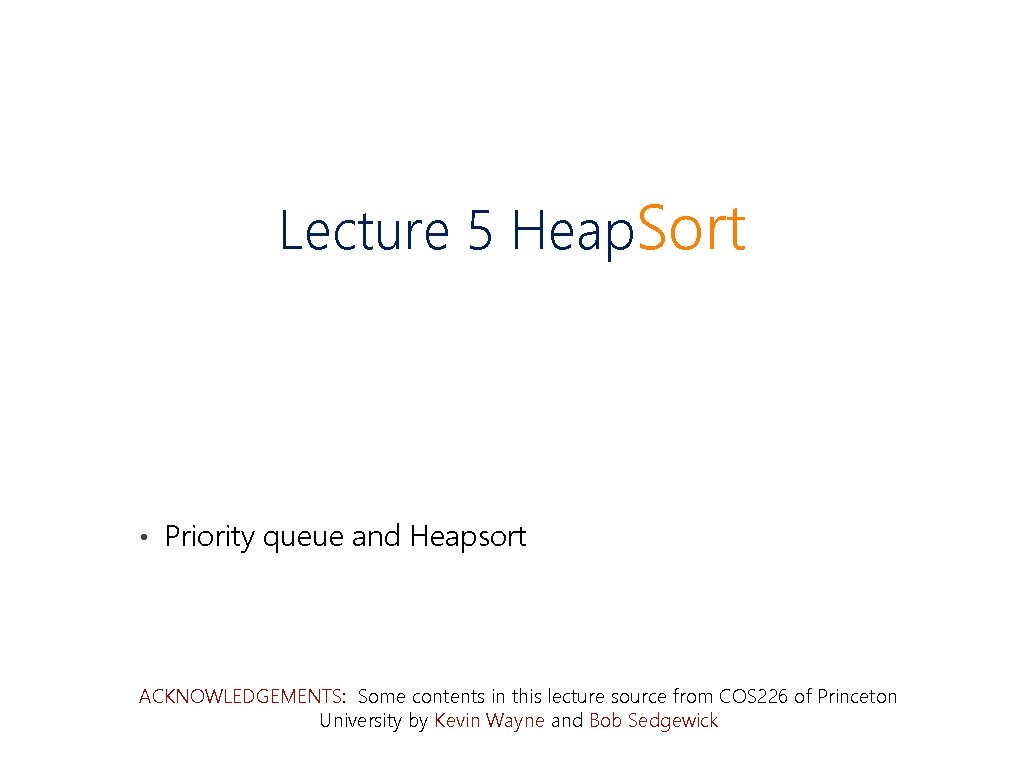
Lecture 5 Heap. Sort • Priority queue and Heapsort ACKNOWLEDGEMENTS: Some contents in this lecture source from COS 226 of Princeton University by Kevin Wayne and Bob Sedgewick
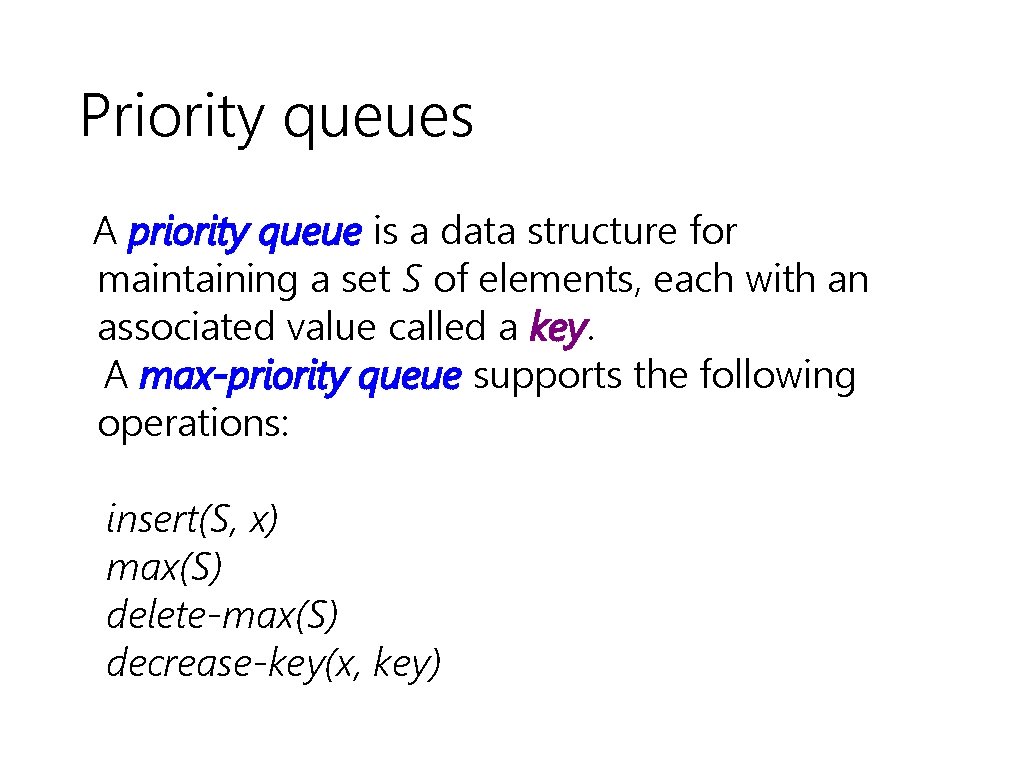
Priority queues A priority queue is a data structure for maintaining a set S of elements, each with an associated value called a key. A max-priority queue supports the following operations: insert(S, x) max(S) delete-max(S) decrease-key(x, key)
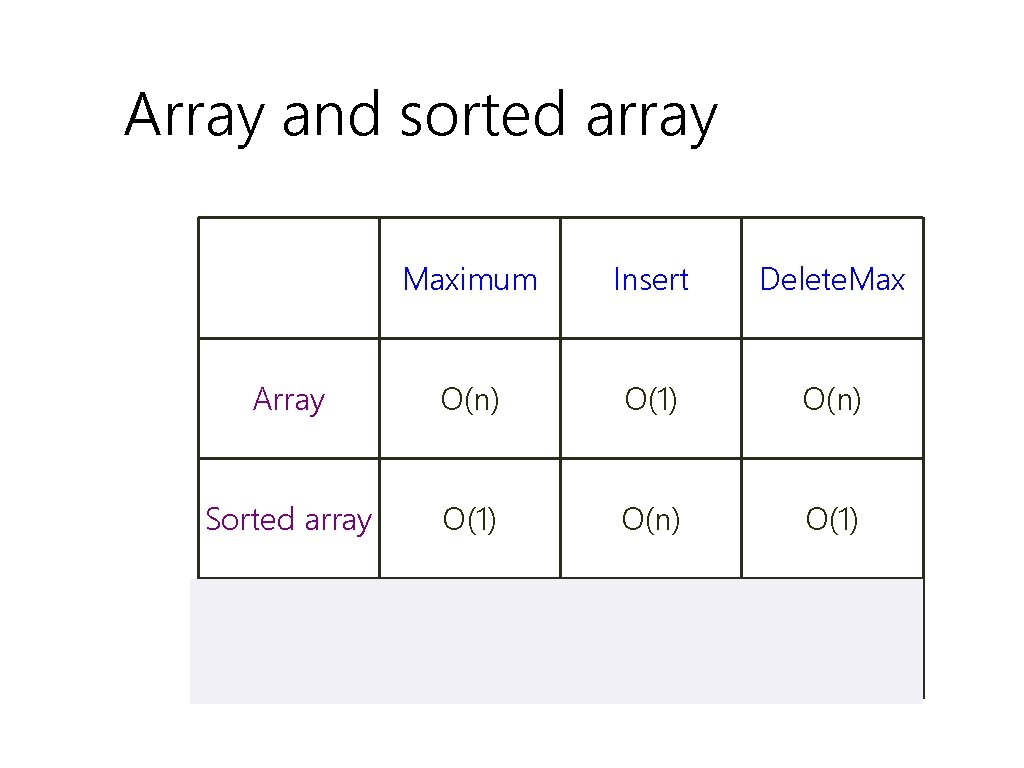
Array and sorted array Maximum Insert Delete. Max Array O(n) O(1) O(n) Sorted array O(1) O(n) O(1) Heap O(1) O(log n)
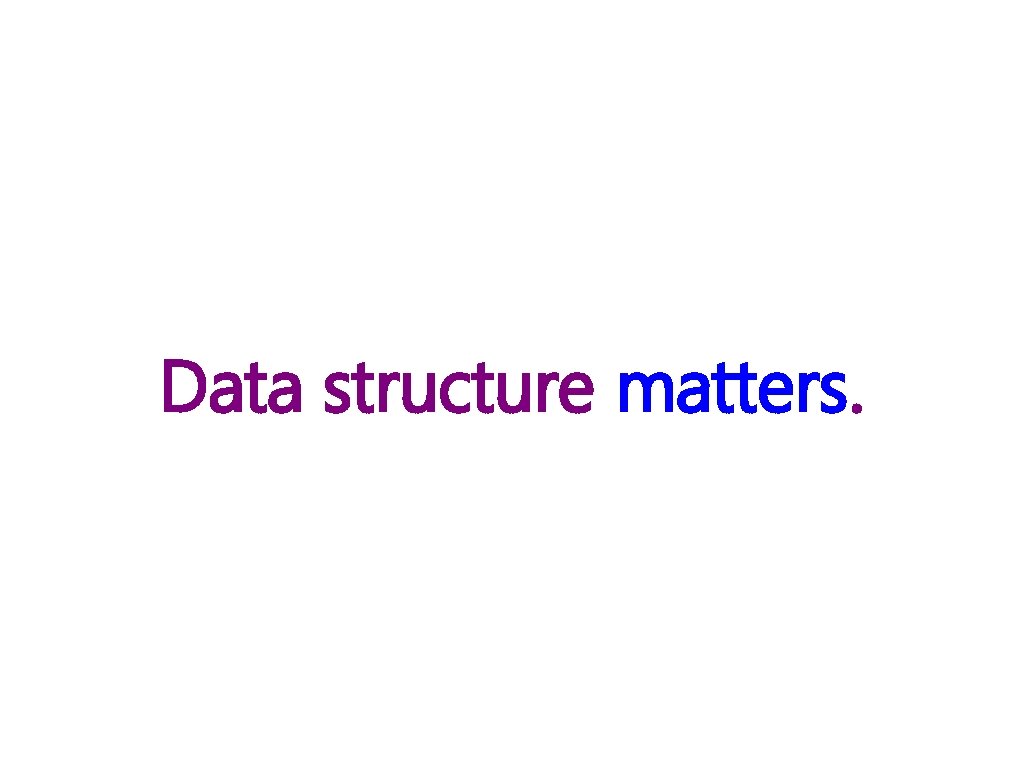
Data structure matters.
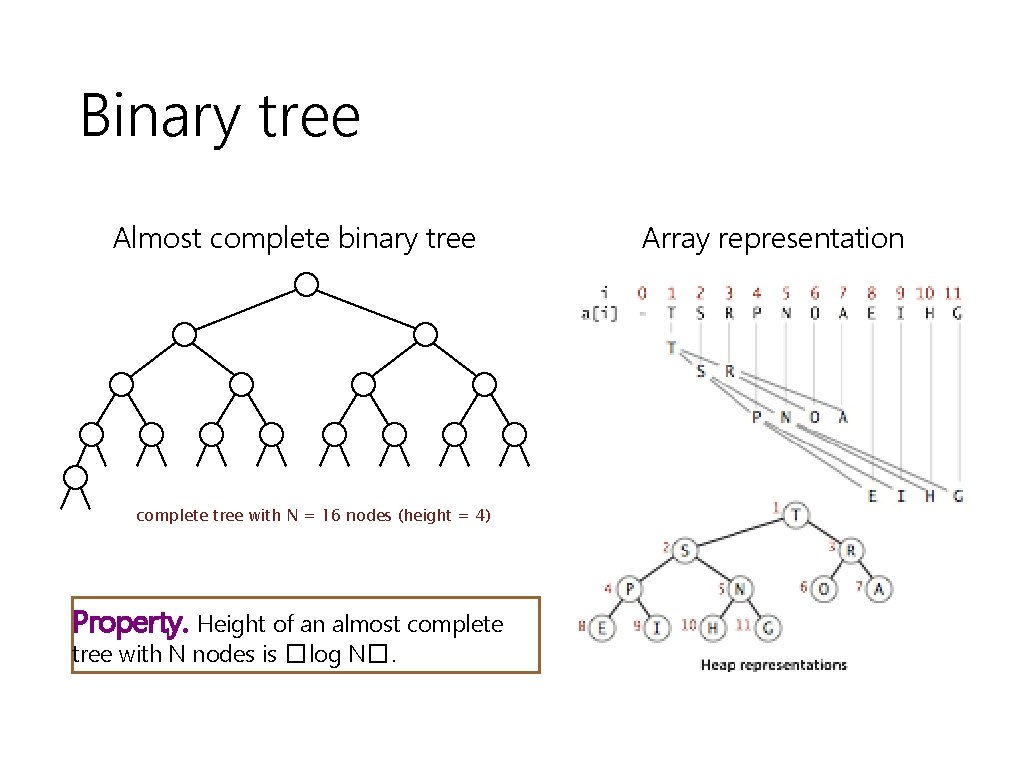
Binary tree Almost complete binary tree complete tree with N = 16 nodes (height = 4) Property. Height of an almost complete tree with N nodes is �log N�. Array representation
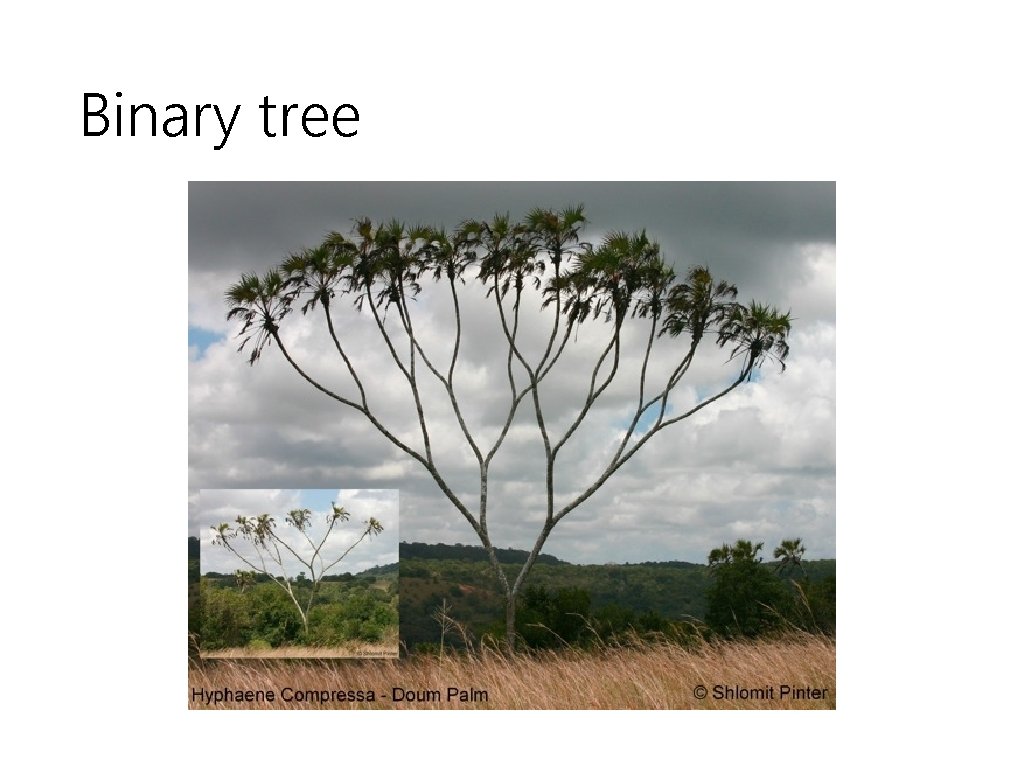
Binary tree
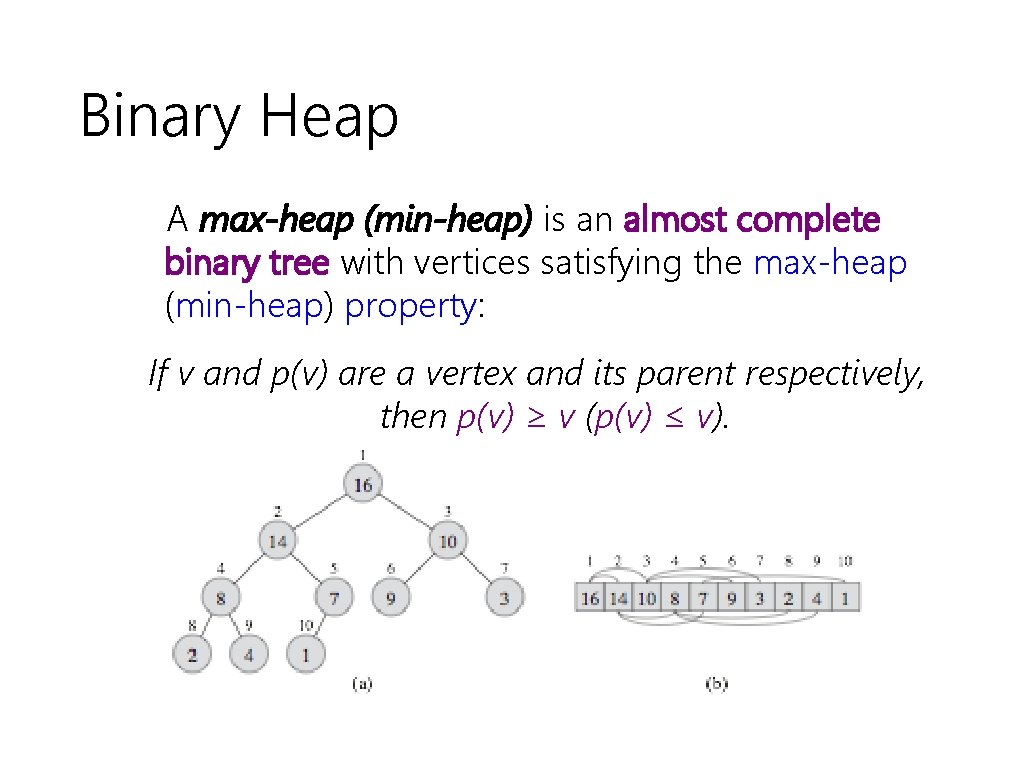
Binary Heap A max-heap (min-heap) is an almost complete binary tree with vertices satisfying the max-heap (min-heap) property: If v and p(v) are a vertex and its parent respectively, then p(v) ≥ v (p(v) ≤ v).
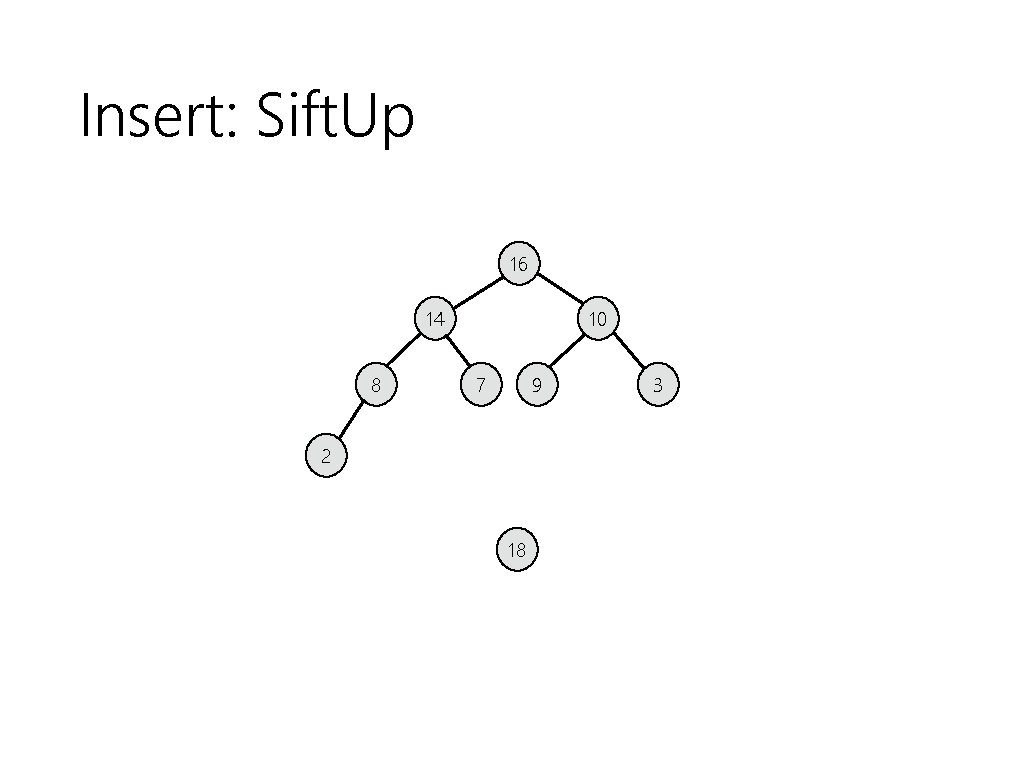
Insert: Sift. Up 16 14 8 10 7 9 2 18 3
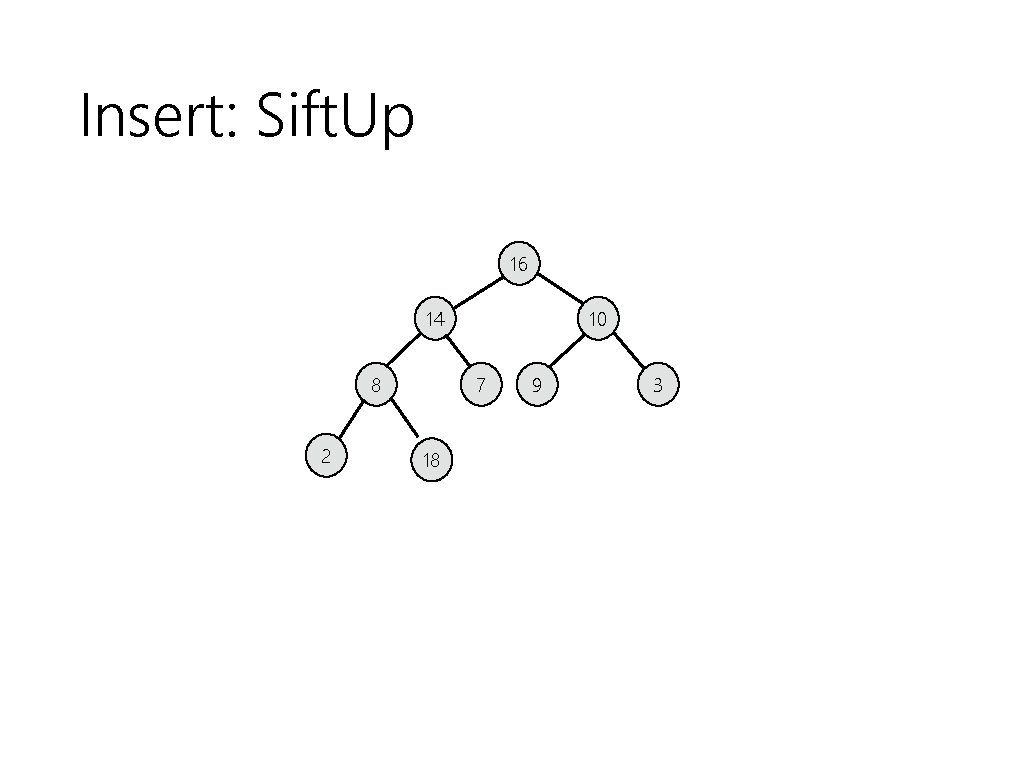
Insert: Sift. Up 16 14 8 2 10 7 18 9 3
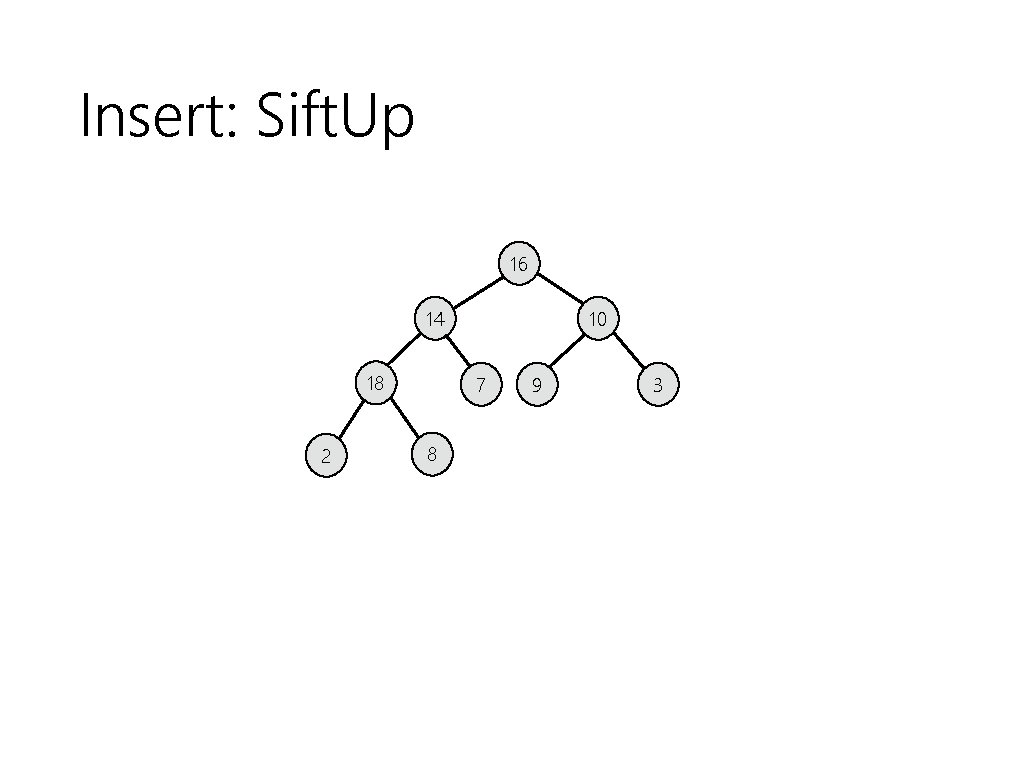
Insert: Sift. Up 16 14 18 2 10 7 8 9 3
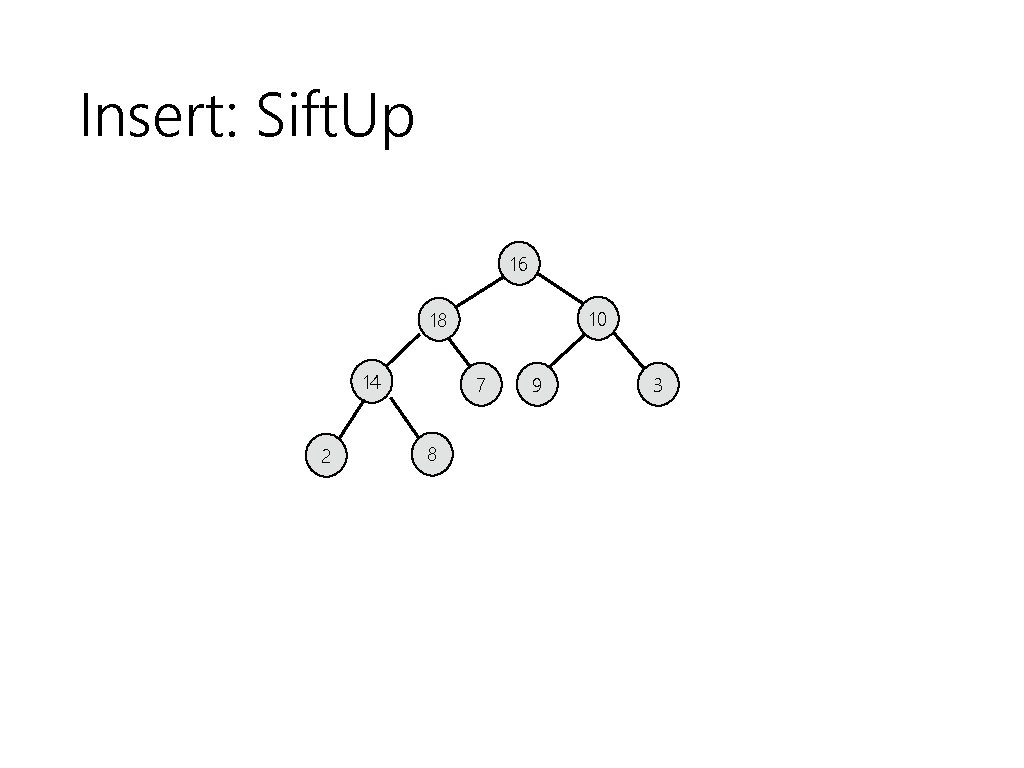
Insert: Sift. Up 16 10 18 14 2 7 8 9 3
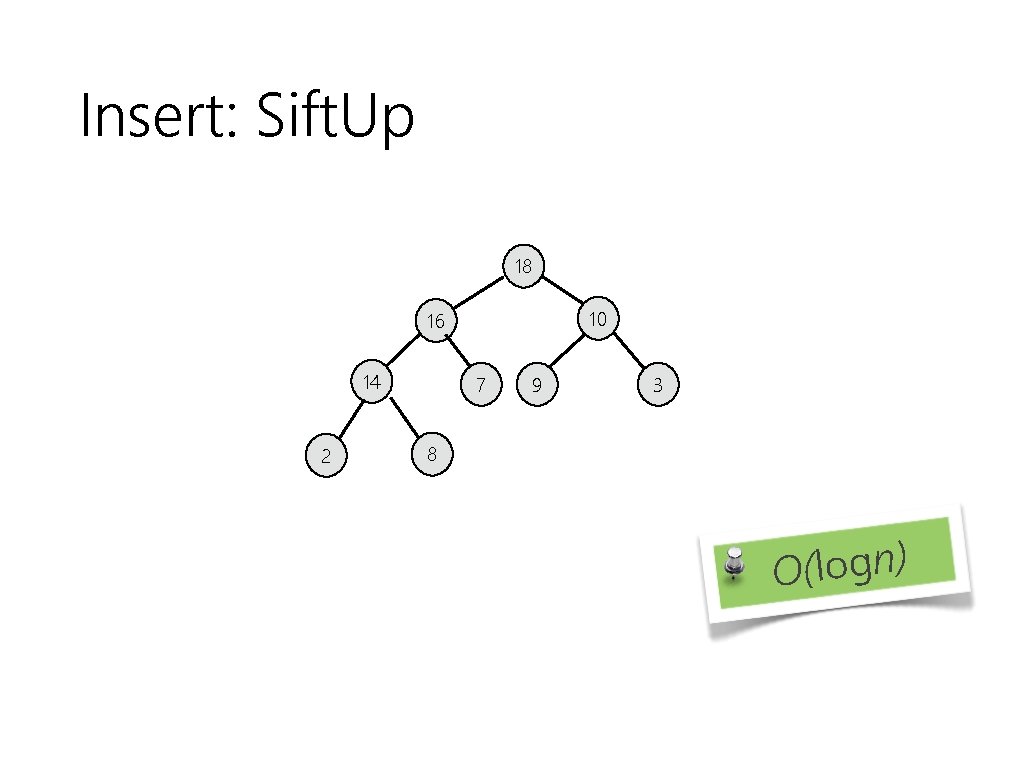
Insert: Sift. Up 18 10 16 14 2 7 9 3 8 ) n g o l ( O
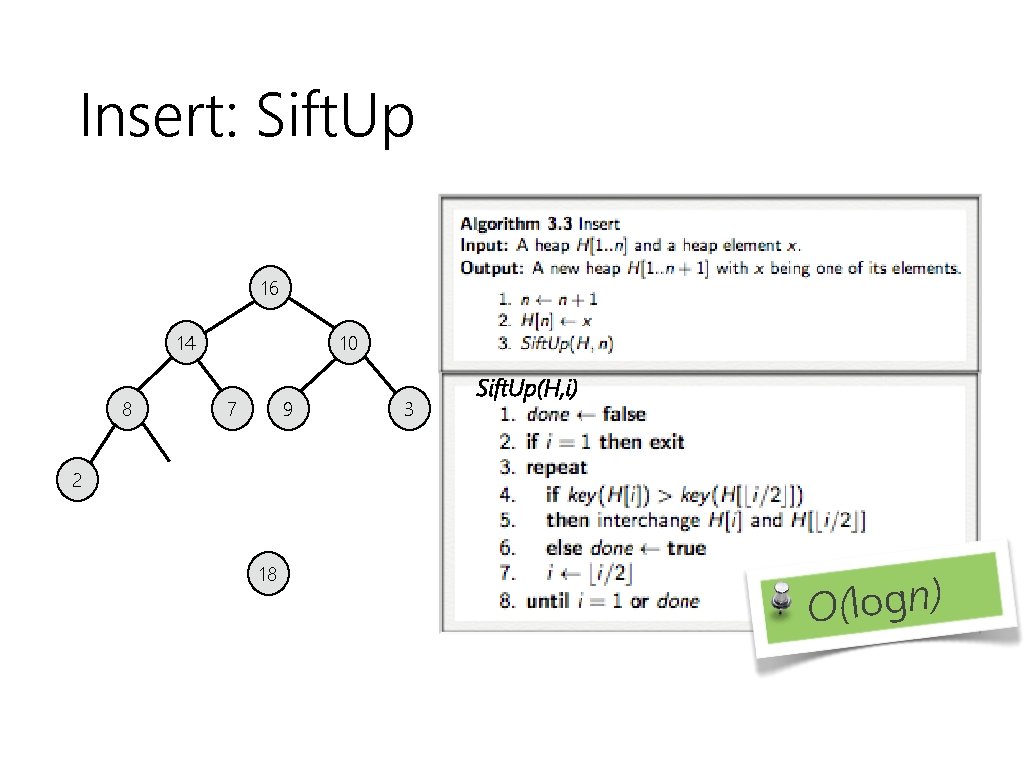
Insert: Sift. Up 16 14 8 10 7 9 3 Sift. Up(H, i) 2 18 ) n g o l ( O
![Maximum 16 14 8 2 10 7 9 3 4 return H1 Θ1 Maximum 16 14 8 2 10 7 9 3 4 return H[1]; Θ(1)](https://slidetodoc.com/presentation_image_h/183eebe2d038f216738a8e95d090d3bd/image-14.jpg)
Maximum 16 14 8 2 10 7 9 3 4 return H[1]; Θ(1)
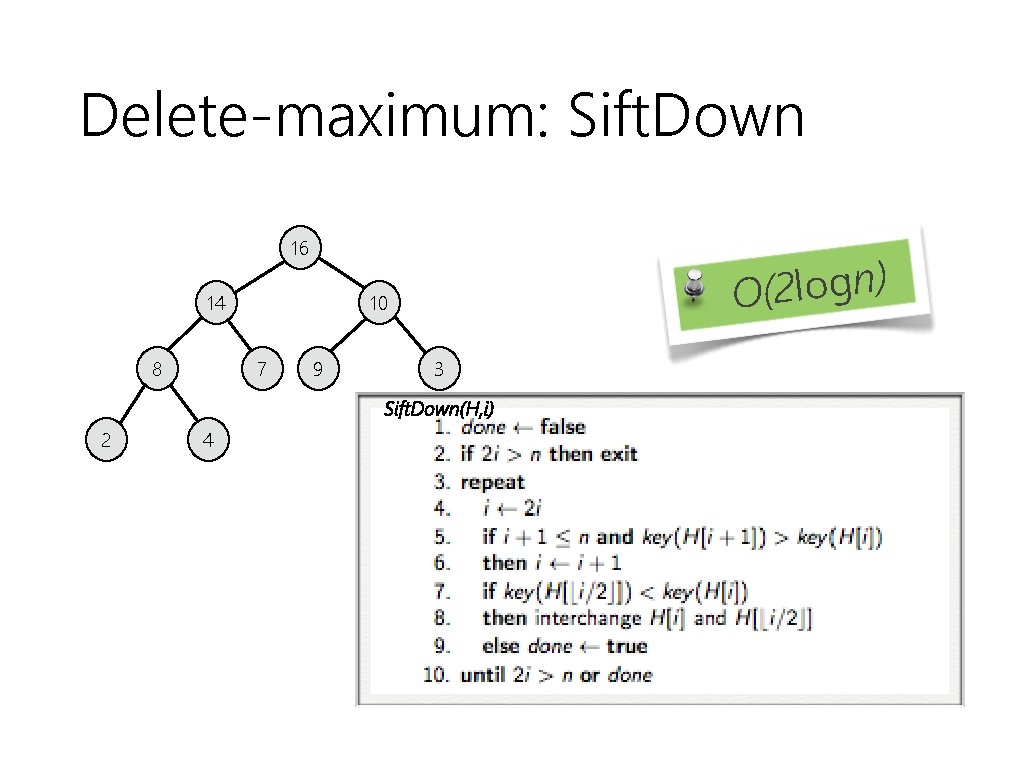
Delete-maximum: Sift. Down 16 14 8 ) n g o l 2 O( 10 7 9 3 Sift. Down(H, i) 2 4
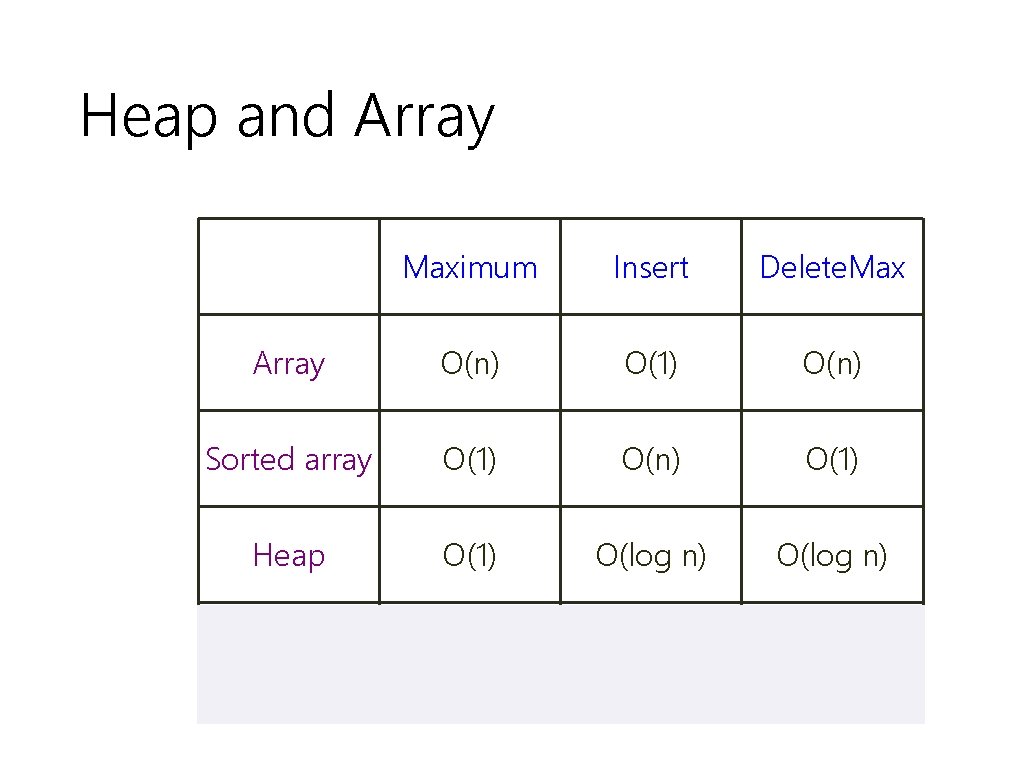
Heap and Array Maximum Insert Delete. Max Array O(n) O(1) O(n) Sorted array O(1) O(n) O(1) Heap O(1) O(log n) d-heap O(1) O(logd n) O(dlogd n)
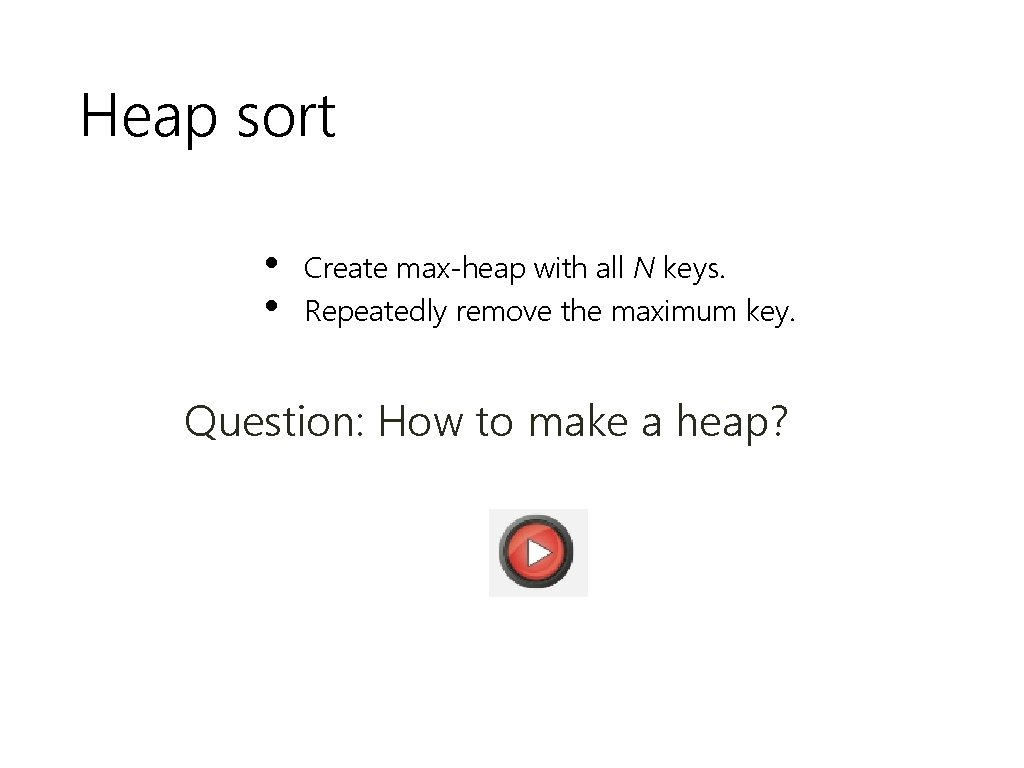
Heap sort • • Create max-heap with all N keys. Repeatedly remove the maximum key. Question: How to make a heap?
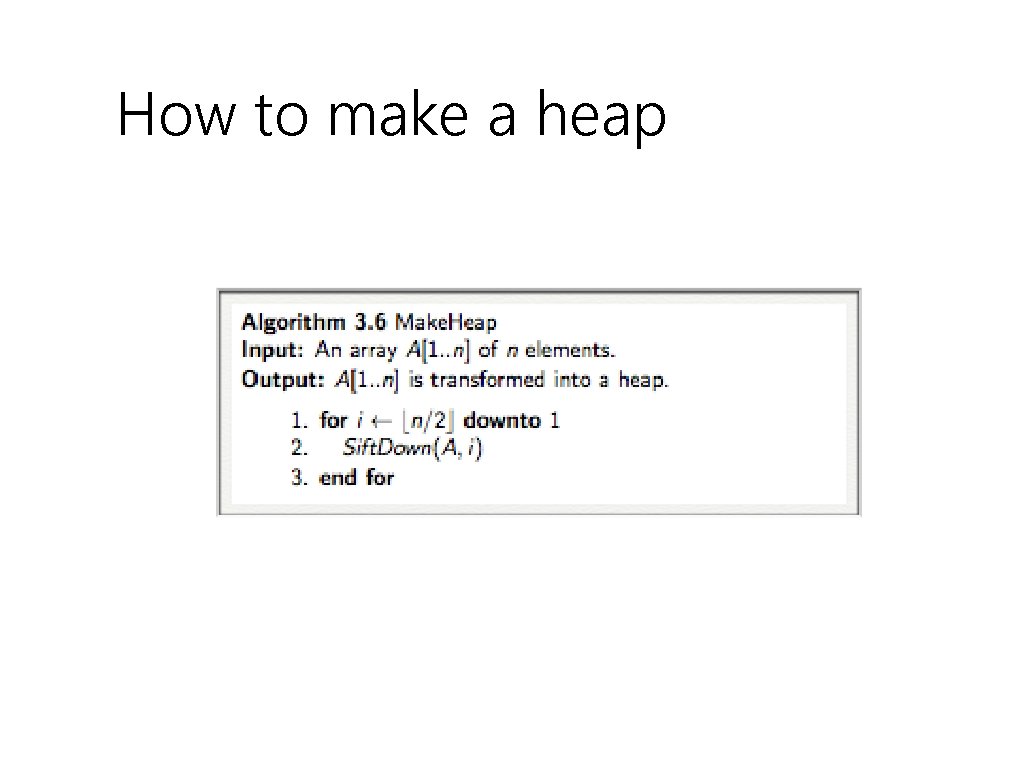
How to make a heap
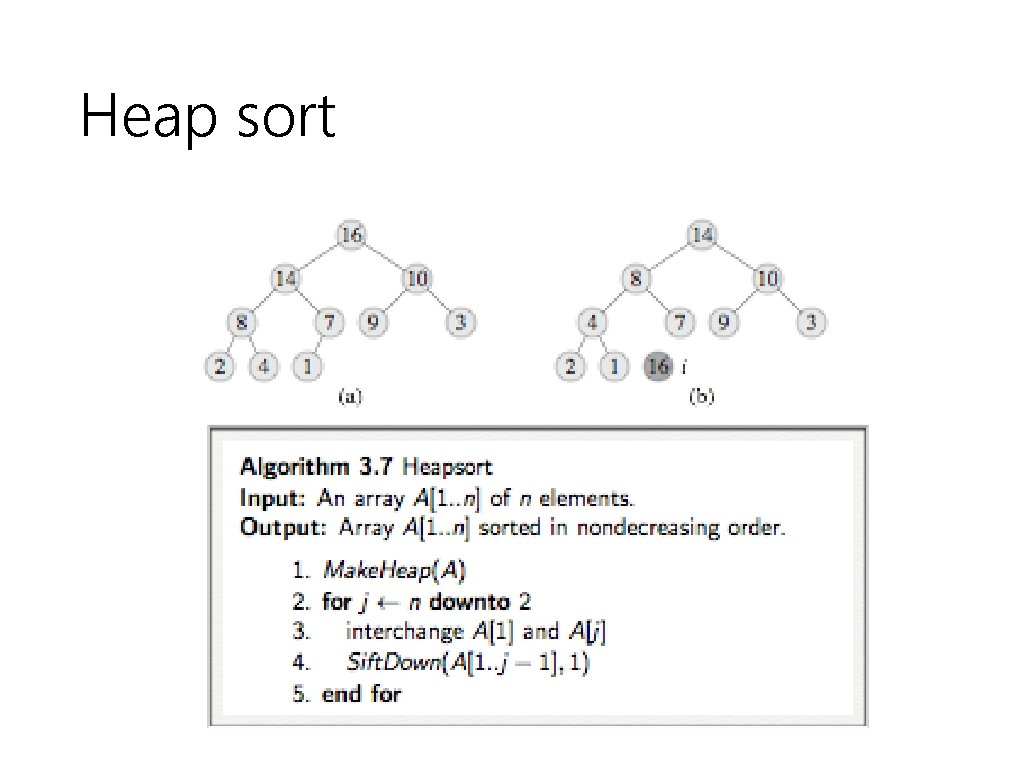
Heap sort
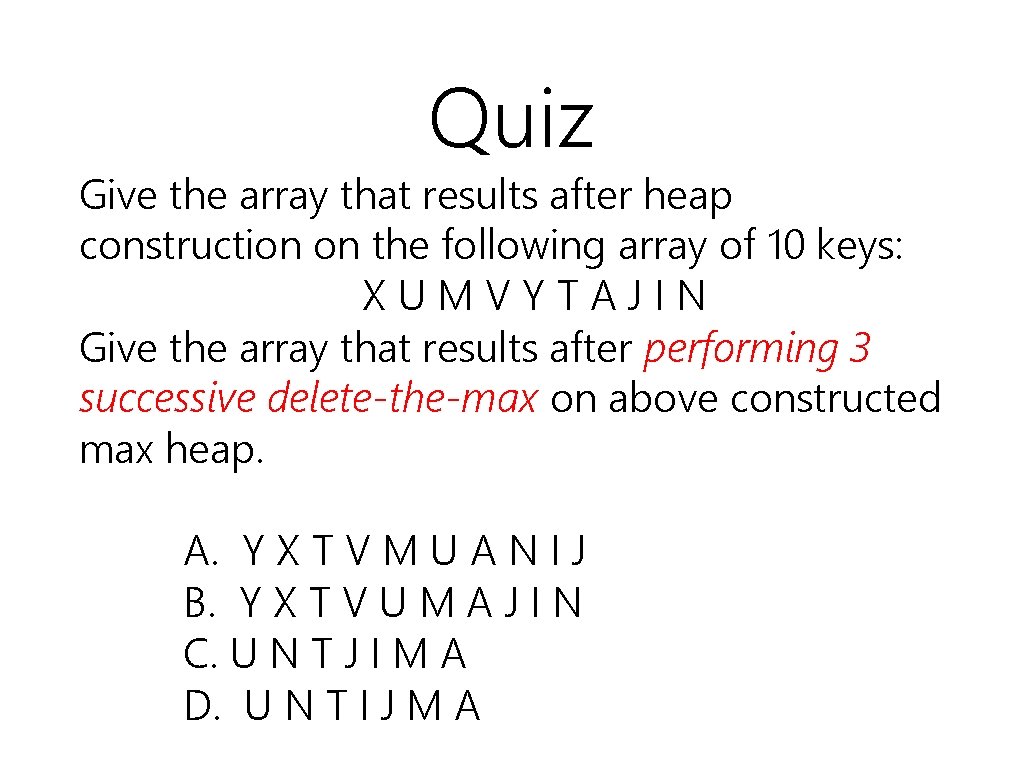
Quiz Give the array that results after heap construction on the following array of 10 keys: XUMVYTAJIN Give the array that results after performing 3 successive delete-the-max on above constructed max heap. A. Y X T V M U A N I J B. Y X T V U M A J I N C. U N T J I M A D. U N T I J M A
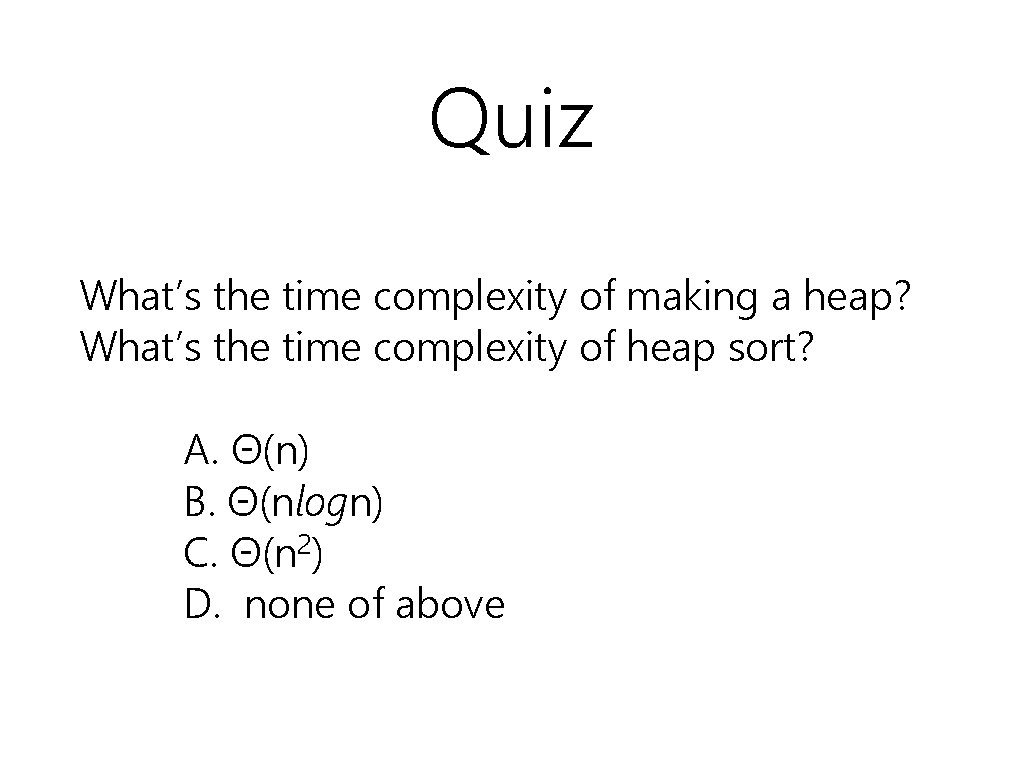
Quiz What’s the time complexity of making a heap? What’s the time complexity of heap sort? A. Θ(n) B. Θ(nlogn) C. Θ(n 2) D. none of above
![Applications Eventdriven simulation customers in a line Data compression Huffman codes Applications • Event-driven simulation. [customers in a line] • Data compression. [Huffman codes] •](https://slidetodoc.com/presentation_image_h/183eebe2d038f216738a8e95d090d3bd/image-22.jpg)
Applications • Event-driven simulation. [customers in a line] • Data compression. [Huffman codes] • Graph searching. [Dijkstra's algorithm, Prim's algorithm] • Statistics. [maintain largest M values in a sequence] • Operating systems. [load balancing, interrupt handling] • Discrete optimization. • . . . [bin packing, scheduling]
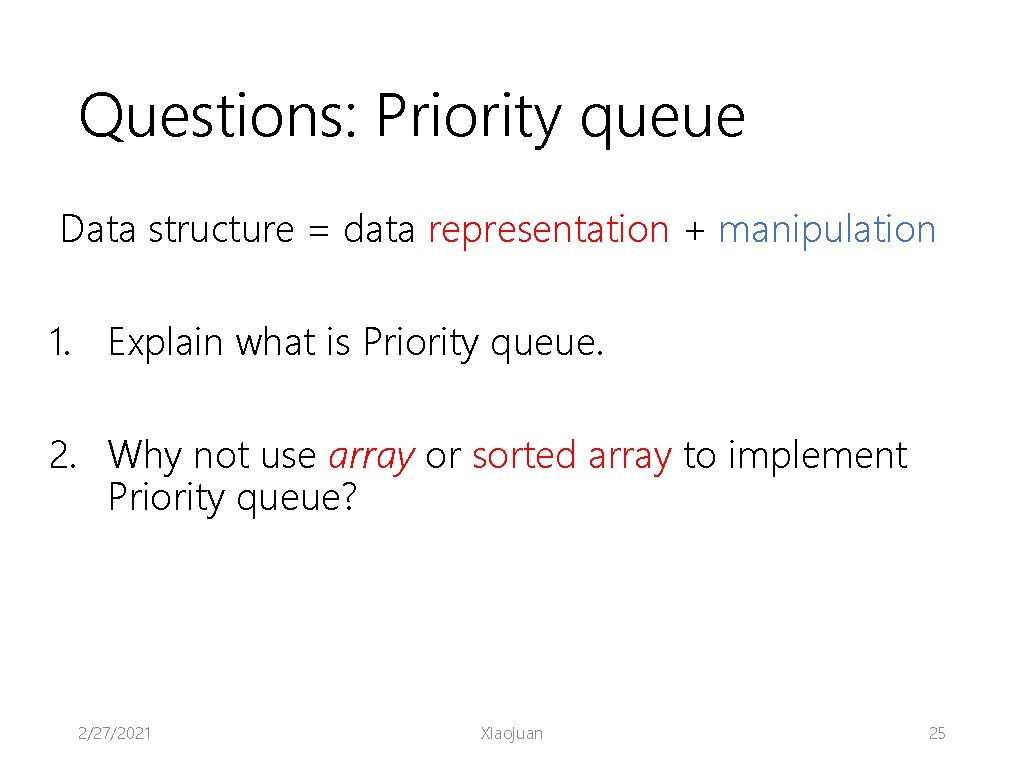
Questions: Priority queue Data structure = data representation + manipulation 1. Explain what is Priority queue. 2. Why not use array or sorted array to implement Priority queue? 2/27/2021 Xiaojuan 25
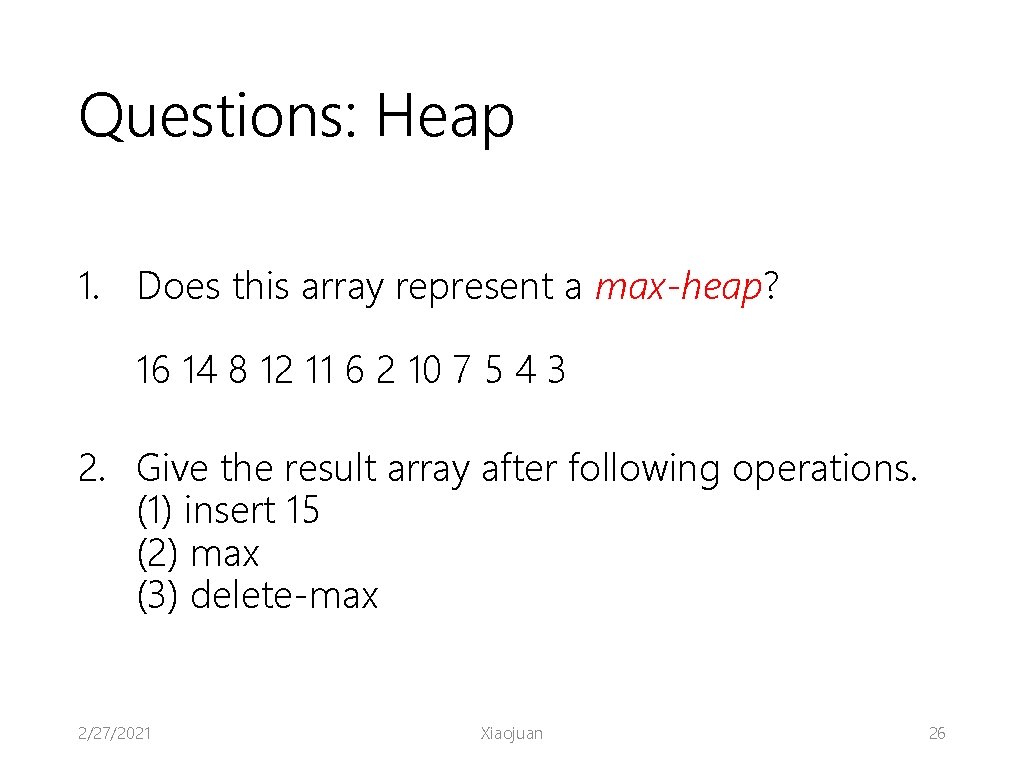
Questions: Heap 1. Does this array represent a max-heap? 16 14 8 12 11 6 2 10 7 5 4 3 2. Give the result array after following operations. (1) insert 15 (2) max (3) delete-max 2/27/2021 Xiaojuan 26
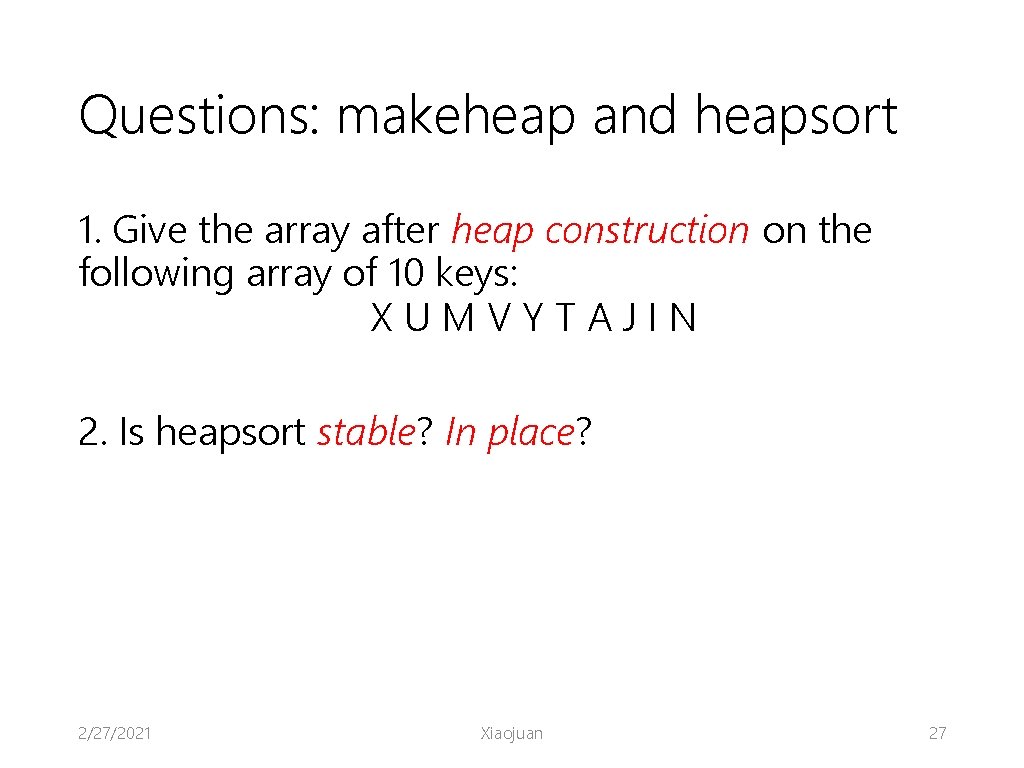
Questions: makeheap and heapsort 1. Give the array after heap construction on the following array of 10 keys: XUMVYTAJIN 2. Is heapsort stable? In place? 2/27/2021 Xiaojuan 27
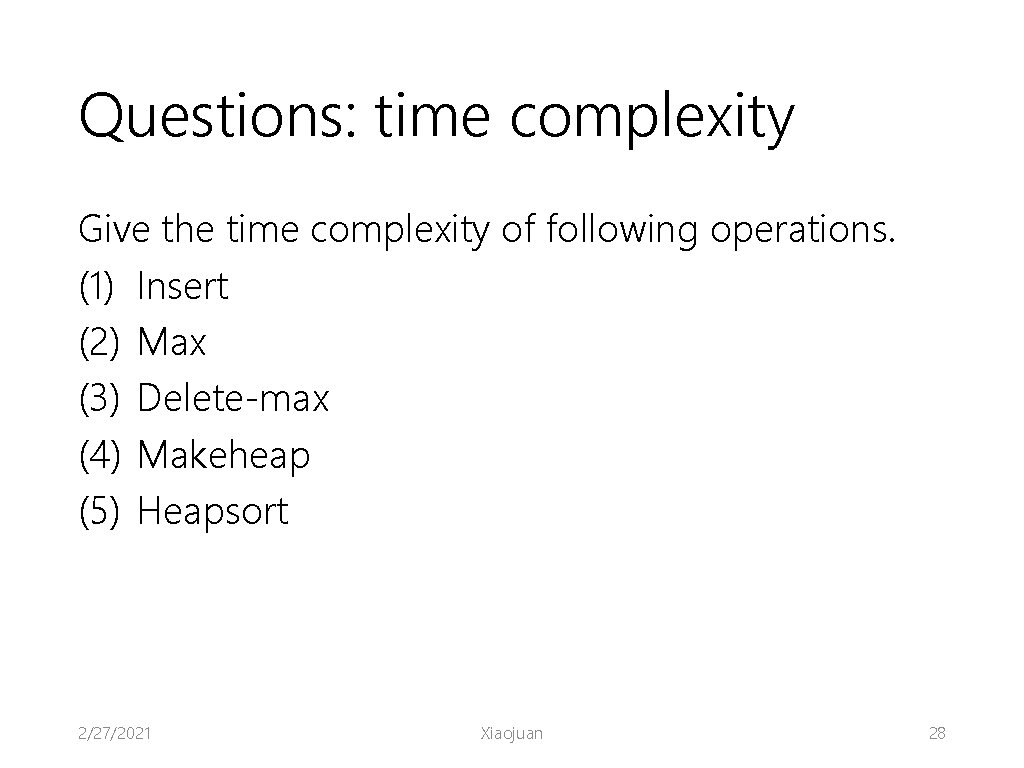
Questions: time complexity Give the time complexity of following operations. (1) Insert (2) Max (3) Delete-max (4) Makeheap (5) Heapsort 2/27/2021 Xiaojuan 28
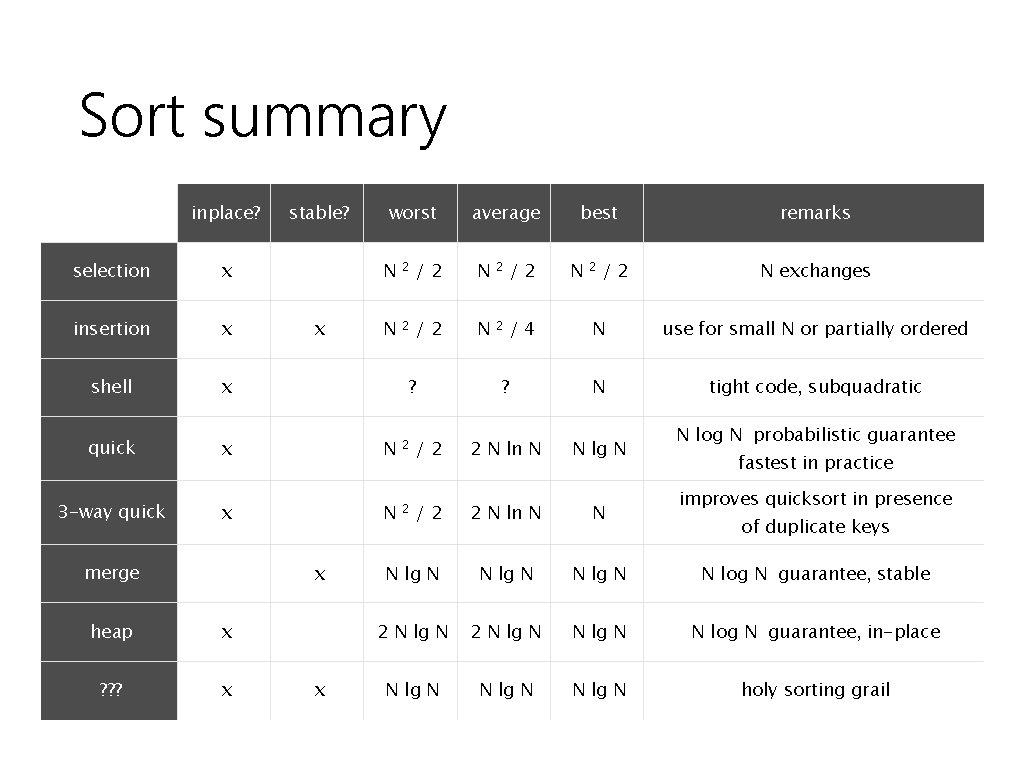
Sort summary inplace? stable? worst average N 2 /2 N 2 /4 selection x insertion x shell x quick x N 2 3 -way quick x N 2 merge x heap x ? ? ? x x N 2 /2 remarks N exchanges N use for small N or partially ordered ? N tight code, subquadratic /2 2 N ln N N lg N /2 2 N ln N N N lg N N log N guarantee, stable 2 N lg N N log N guarantee, in-place N lg N holy sorting grail ? x best N log N probabilistic guarantee fastest in practice improves quicksort in presence of duplicate keys
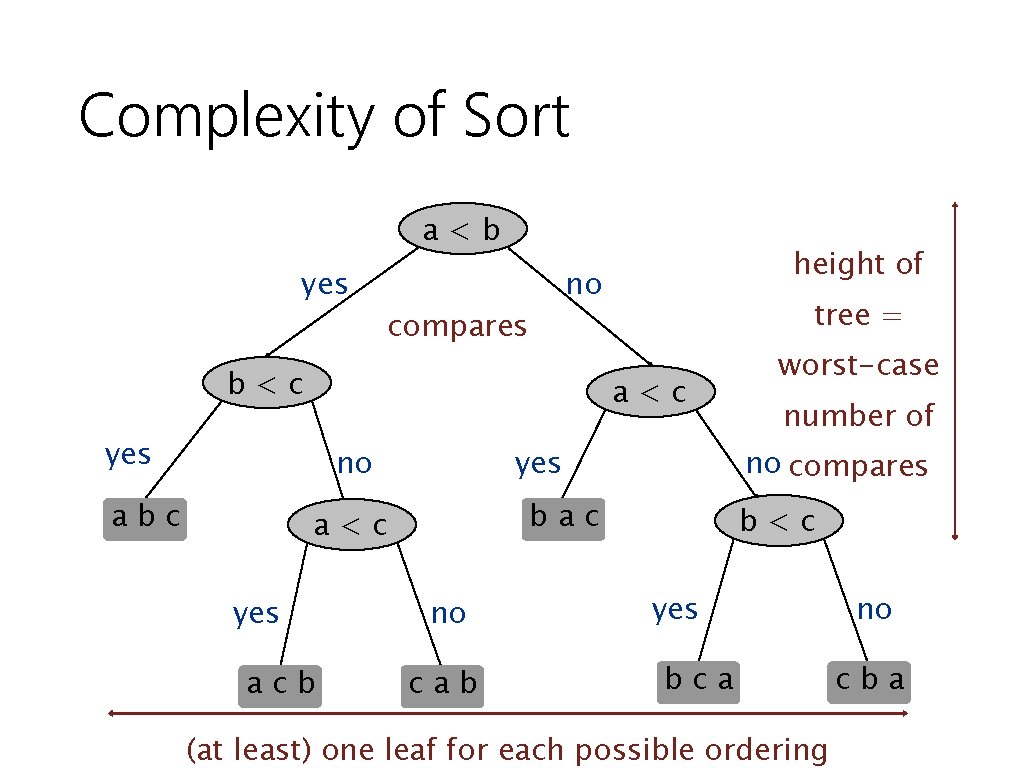
Complexity of Sort a<b yes no compares b<c tree = a<c yes no abc height of yes acb no cab number of no compares bac a<c worst-case b<c yes bca (at least) one leaf for each possible ordering no cba
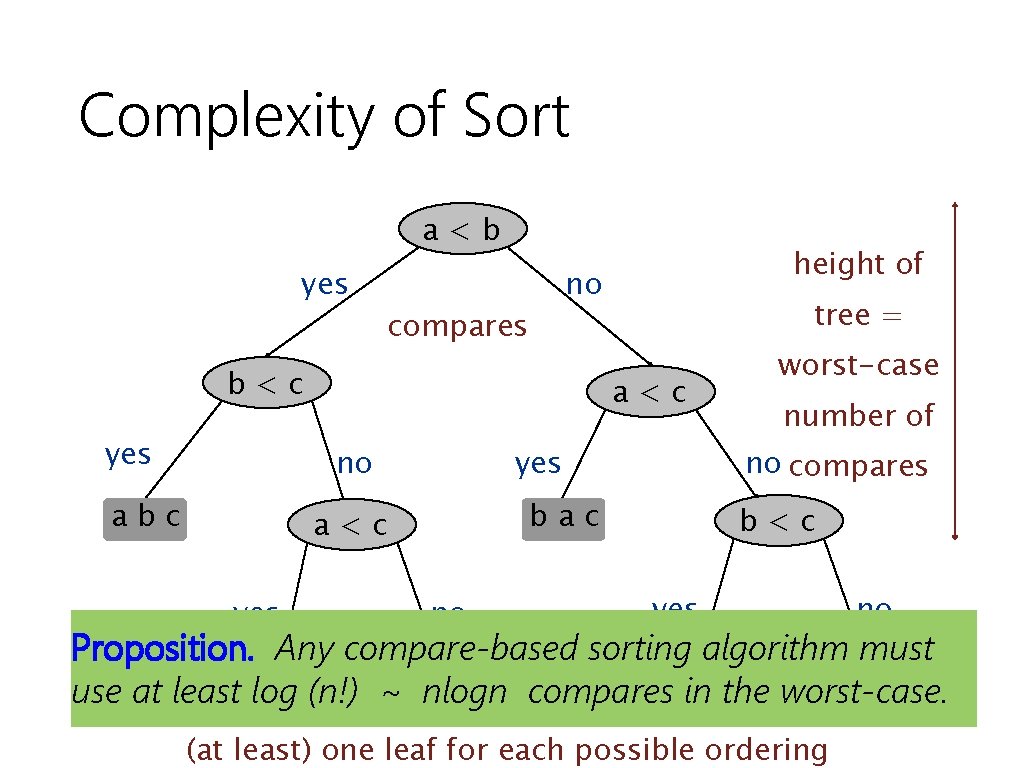
Complexity of Sort a<b yes no compares b<c yes no worst-case number of no compares bac a<c yes tree = a<c no abc height of b<c yes no Proposition. Any compare-based sorting algorithm must c athe worst-case. cba c b(n!) ~ cnlogn ab use at least alog comparesb in (at least) one leaf for each possible ordering
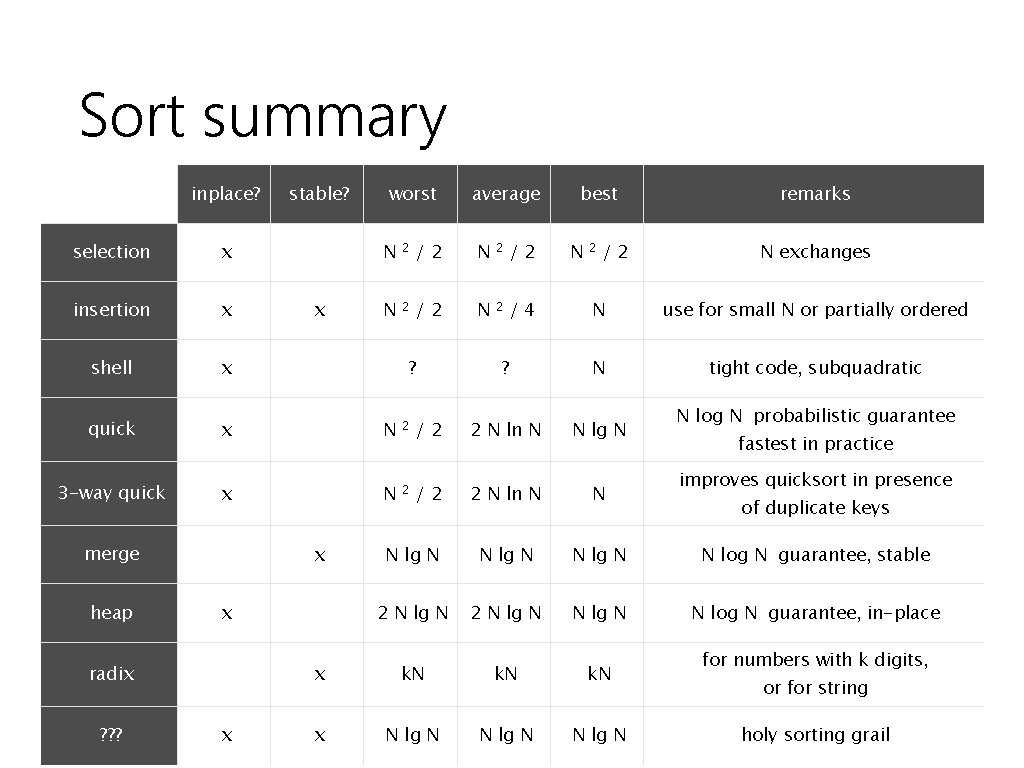
Sort summary inplace? stable? worst average N 2 /2 N 2 /4 selection x insertion x shell x quick x N 2 3 -way quick x N 2 merge heap x x N 2 /2 remarks N exchanges N use for small N or partially ordered ? N tight code, subquadratic /2 2 N ln N N lg N /2 2 N ln N N N lg N N log N guarantee, stable 2 N lg N N log N guarantee, in-place ? x radix ? ? ? x best x k. N x N lg N N log N probabilistic guarantee fastest in practice improves quicksort in presence of duplicate keys for numbers with k digits, or for string holy sorting grail
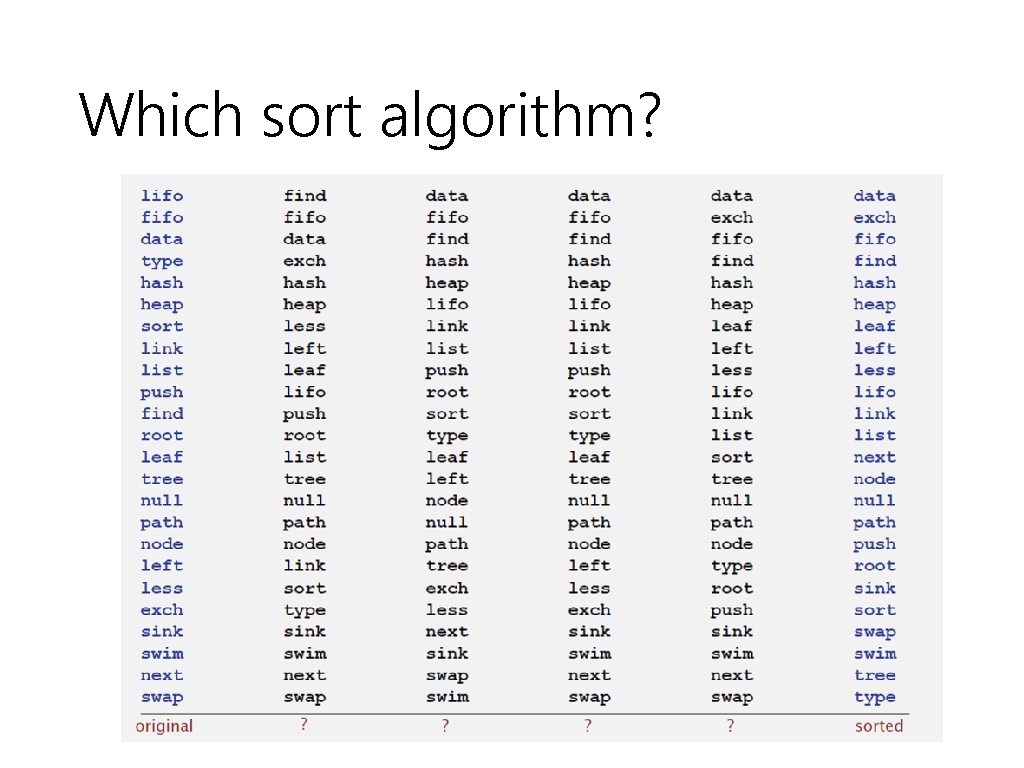
Which sort algorithm?
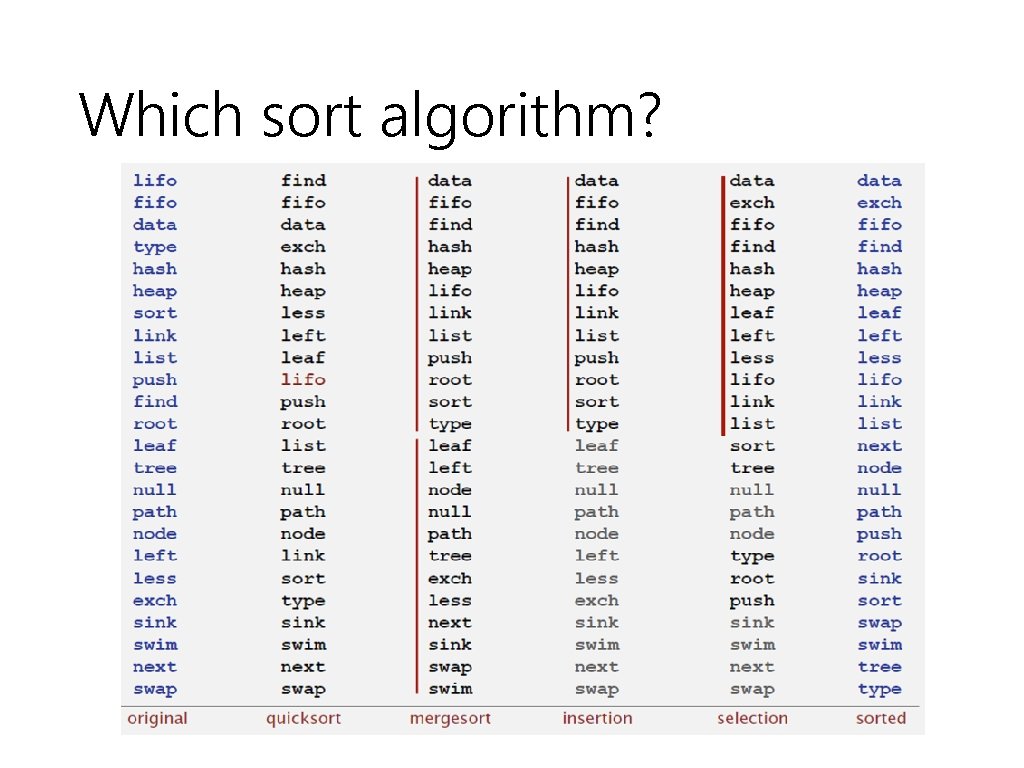
Which sort algorithm?
Priority queue using heap
Heap based priority queue
Double ended queue
Who invented selection sort
Deque and priority queue
Heap vs binary heap
Min priority queue
Priority queue abstract data type
Symmetric min-max heap
Priority queue doubly linked list
Praveen
Priority queue order
Adaptable priority queue java
Adaptable priority queue java
Priority queue lower bound
Transform and conquer technique
Operasi dalam queue
The queue summary
Priority queue animation
Burman's priority list gives priority to
Priority mail vs priority mail express
Heap sort animation
Running time of heap sort
Heap tree visualization
Heap sort worst case
Shell sort complexity
Apa itu heap
Heap sort simulation
Heap sort tutorial
Heap sort
Algoriyma
Heap sort
Algoritmo heapsort