Lecture 18 Continue Evaluator 1 Representing procedures eval
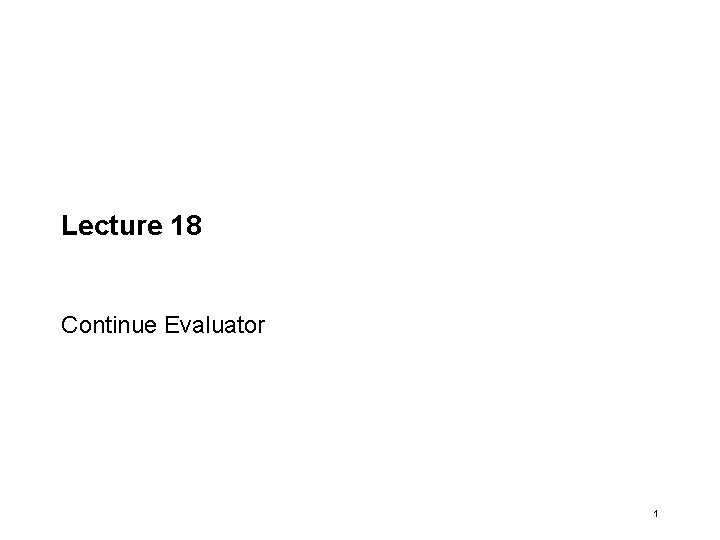
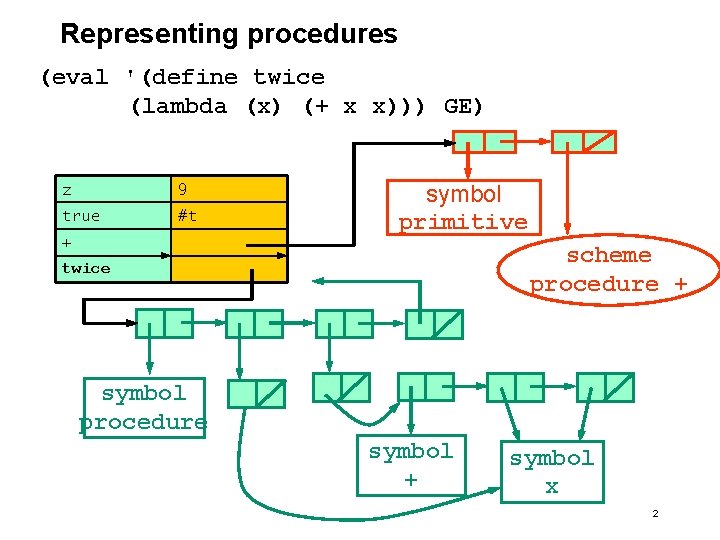
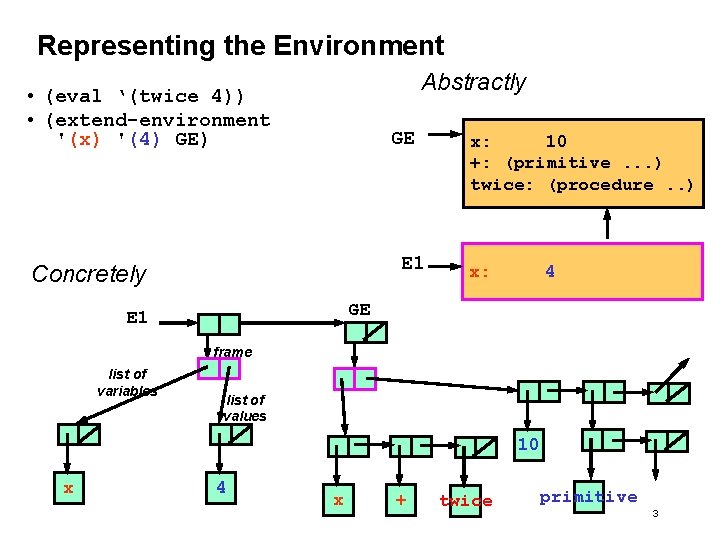
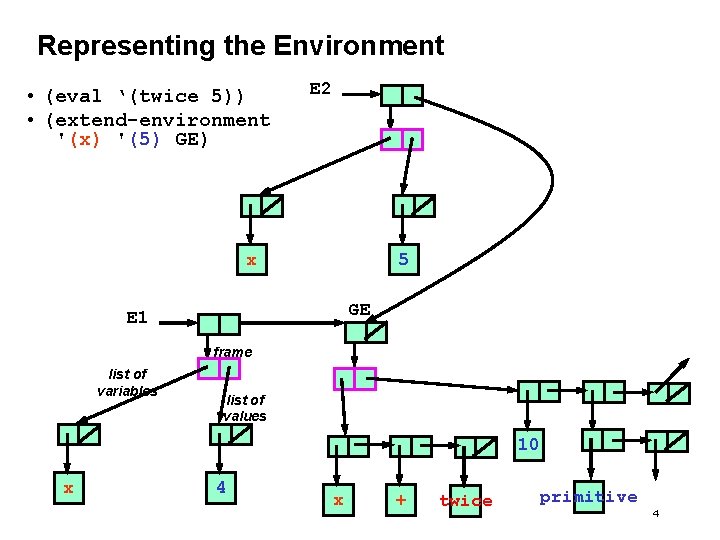
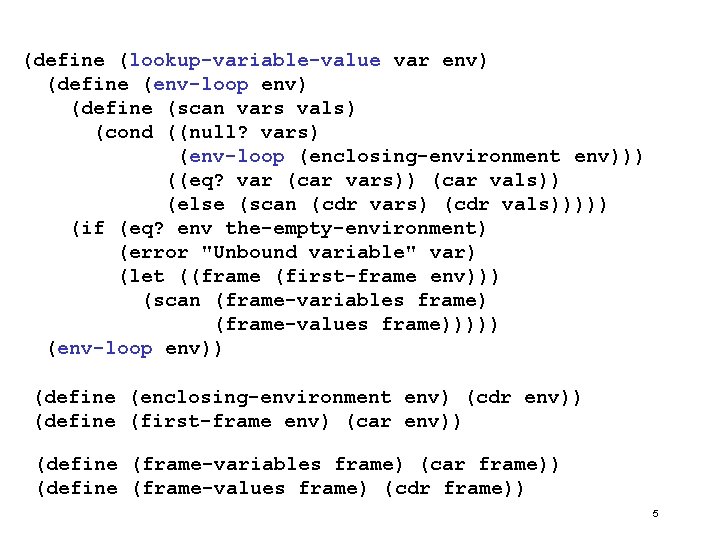
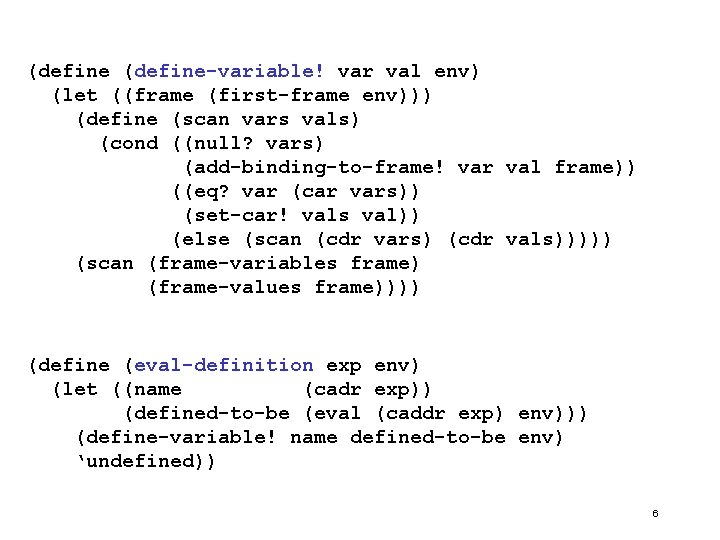
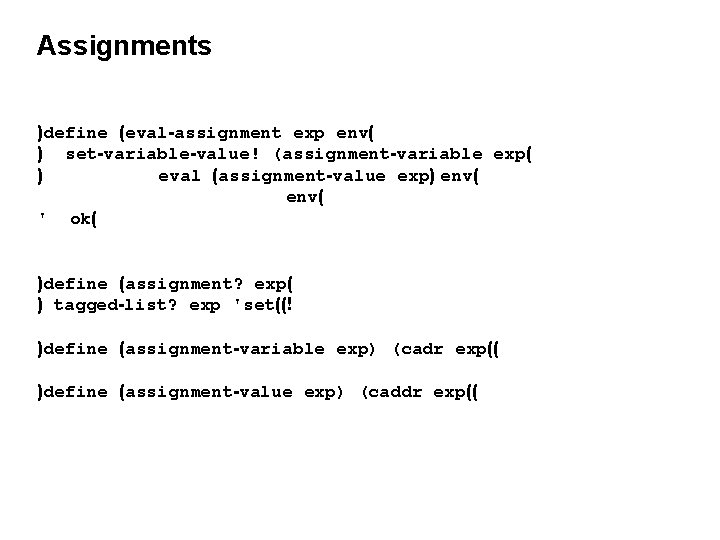
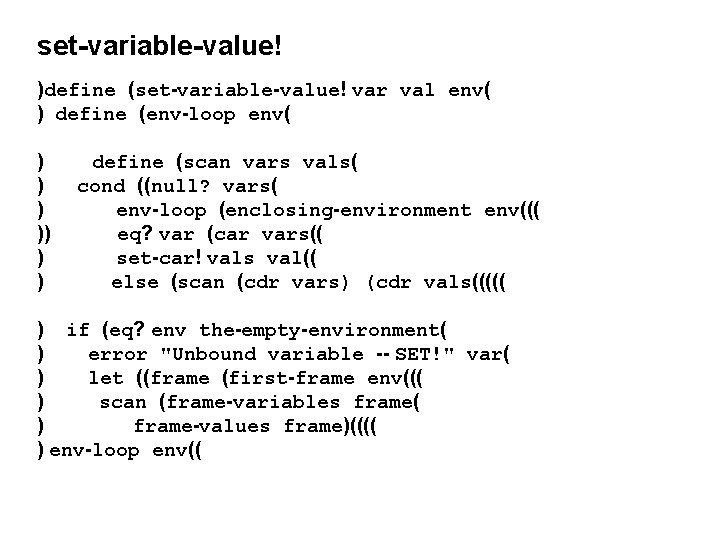
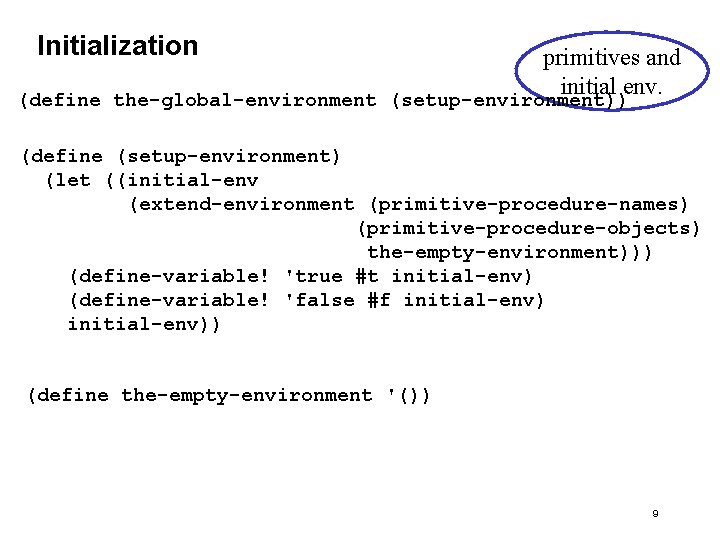
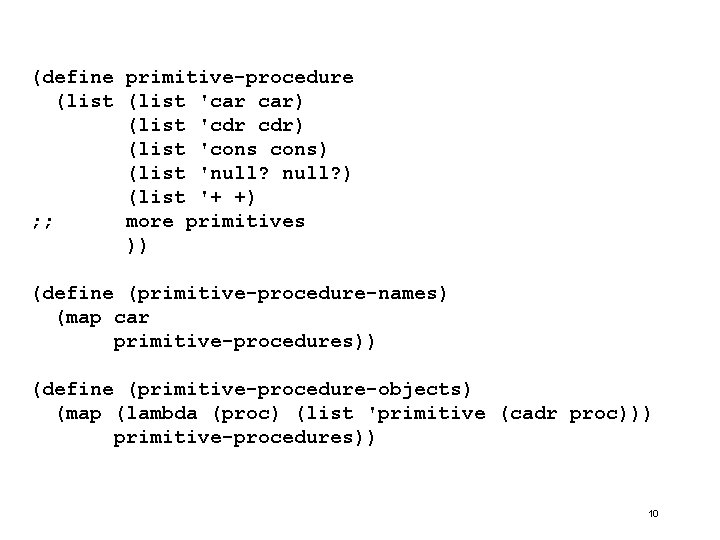
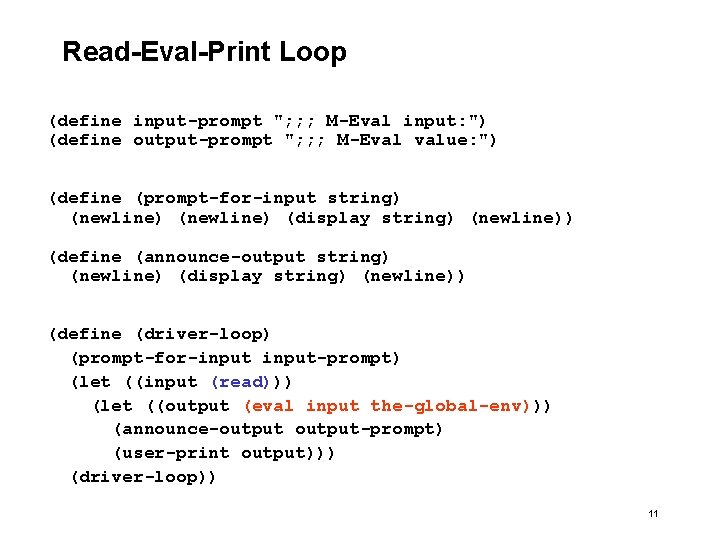
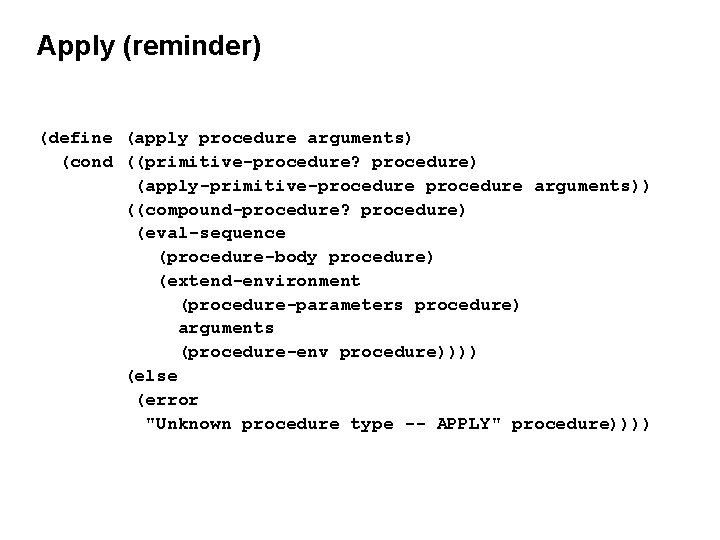
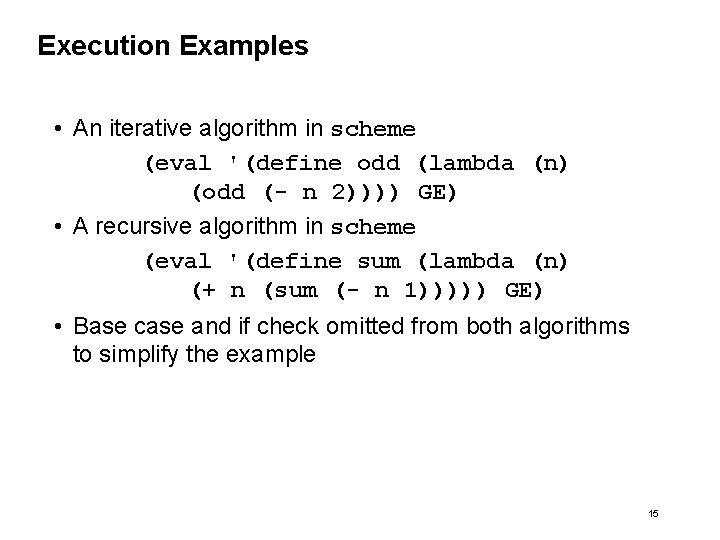
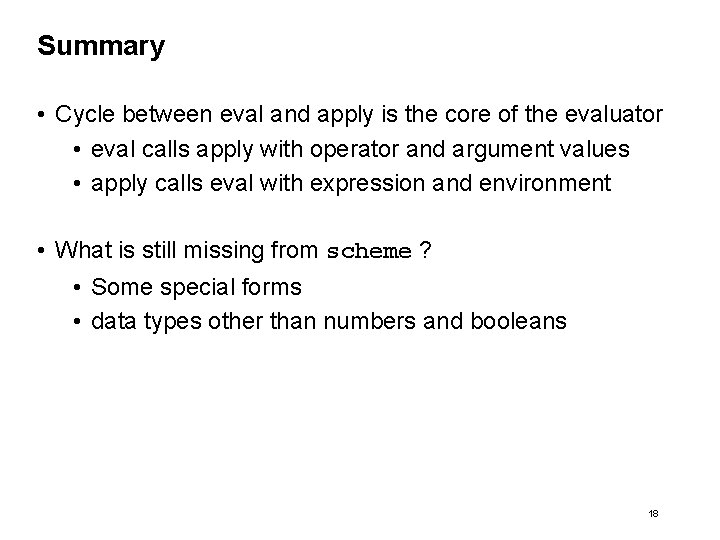
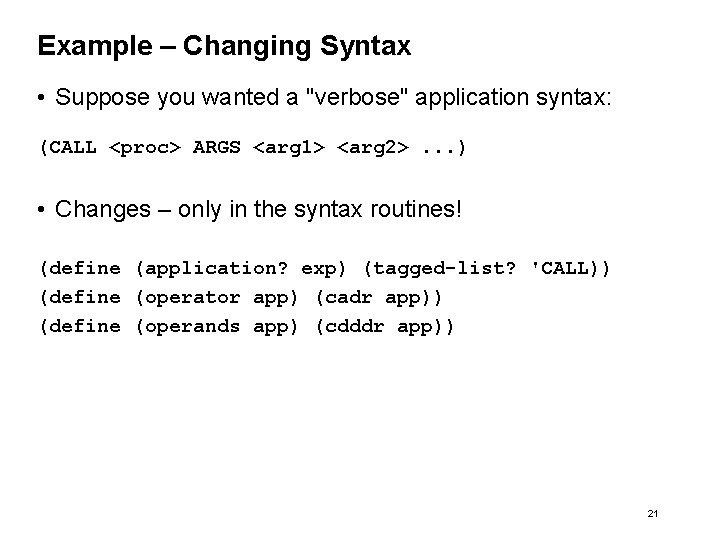
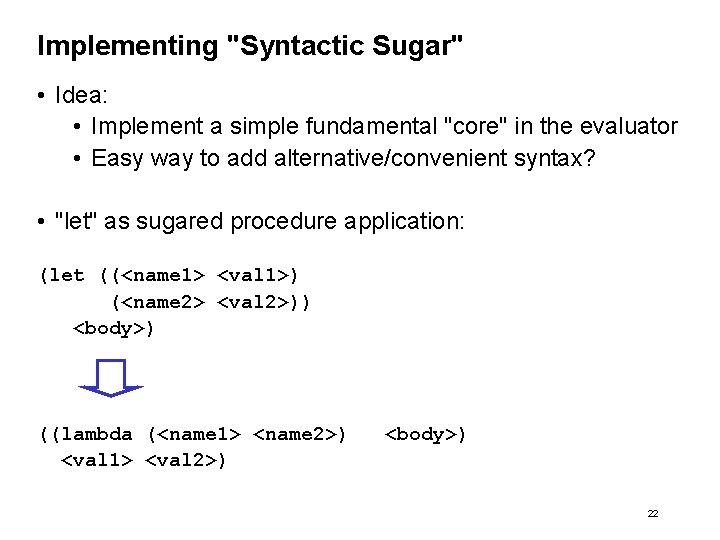
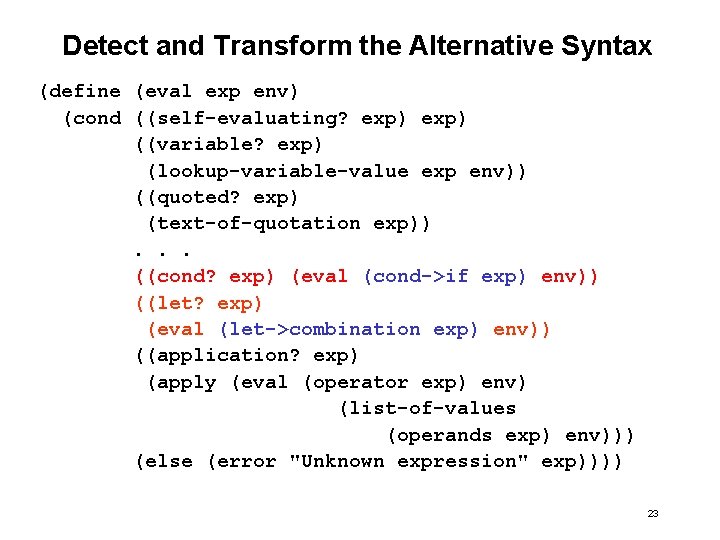
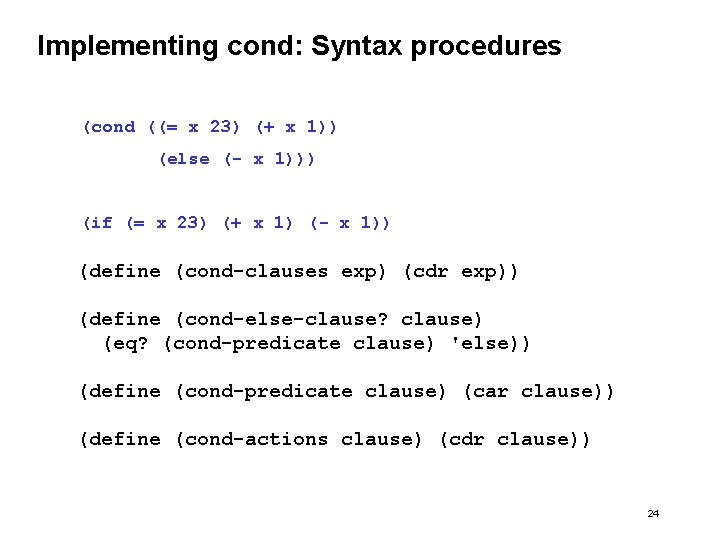
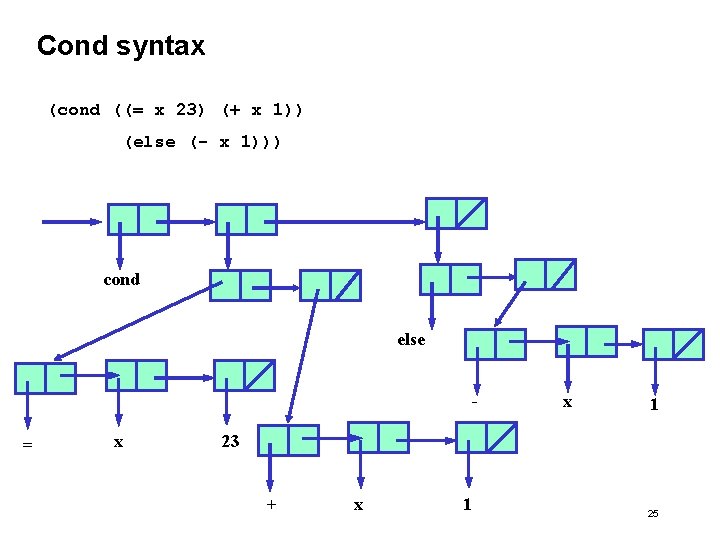
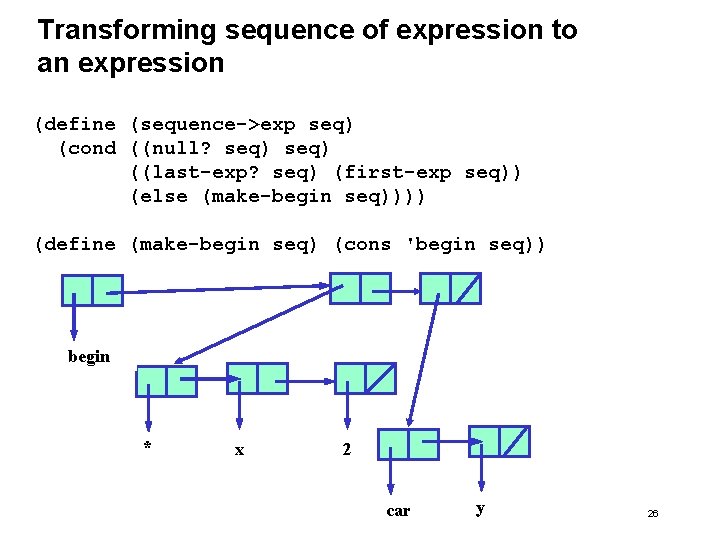
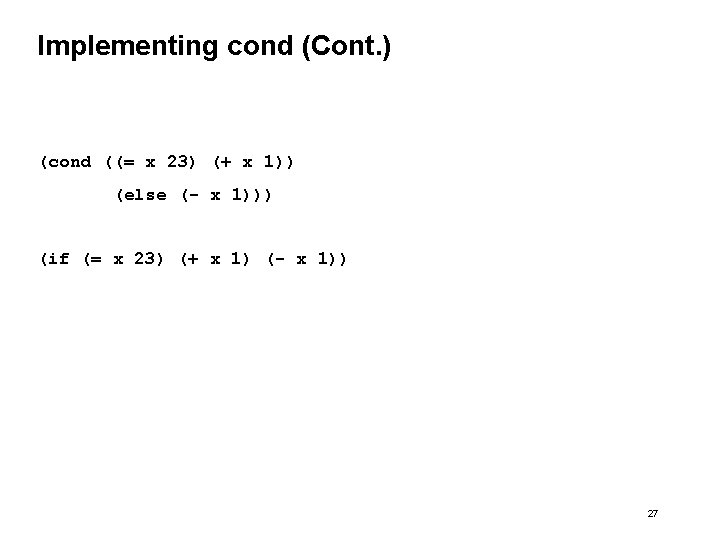
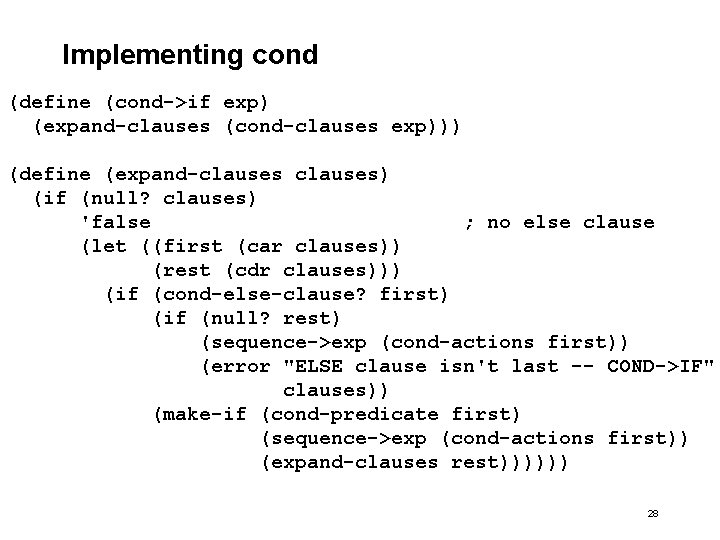
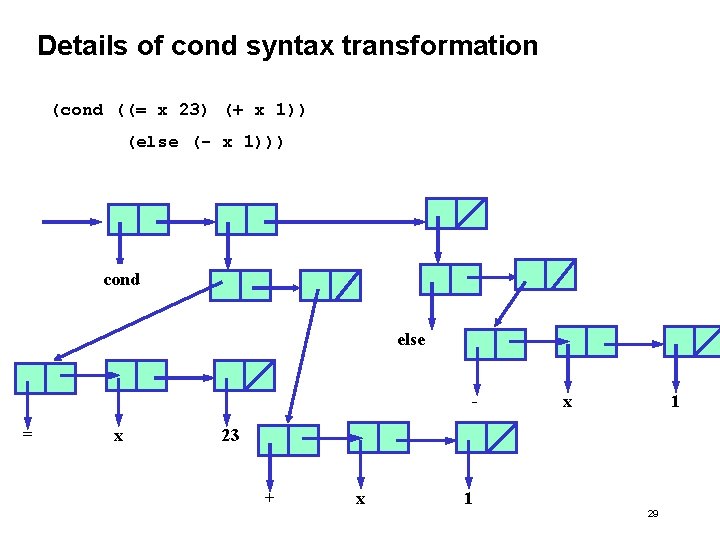
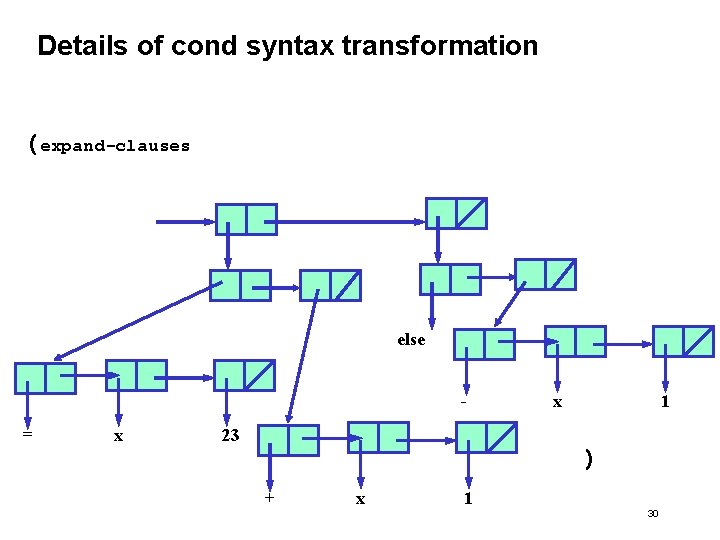
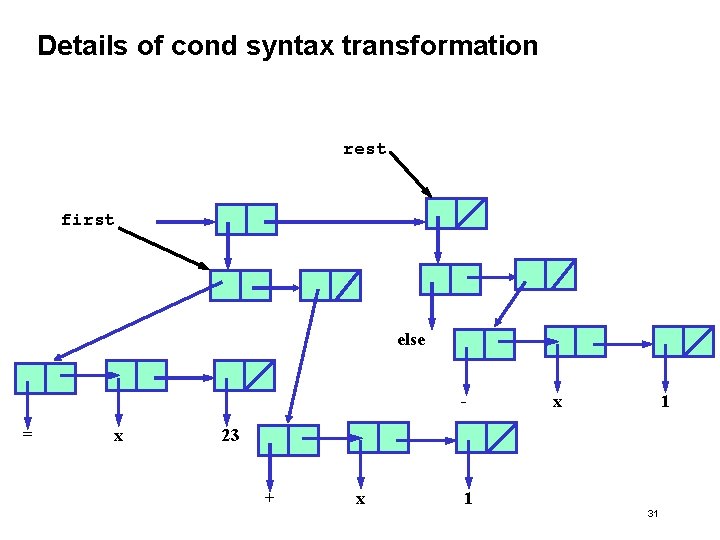
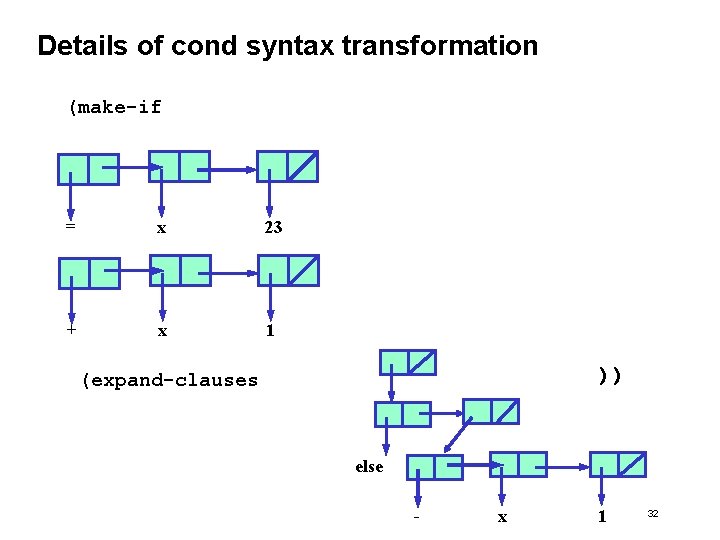
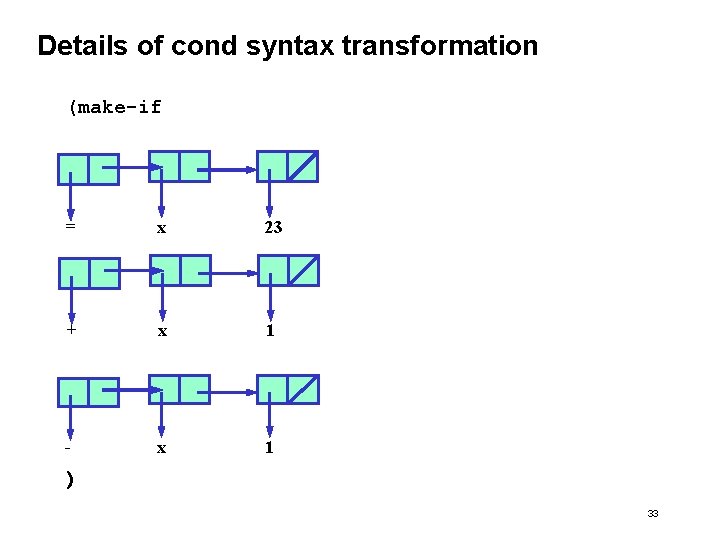
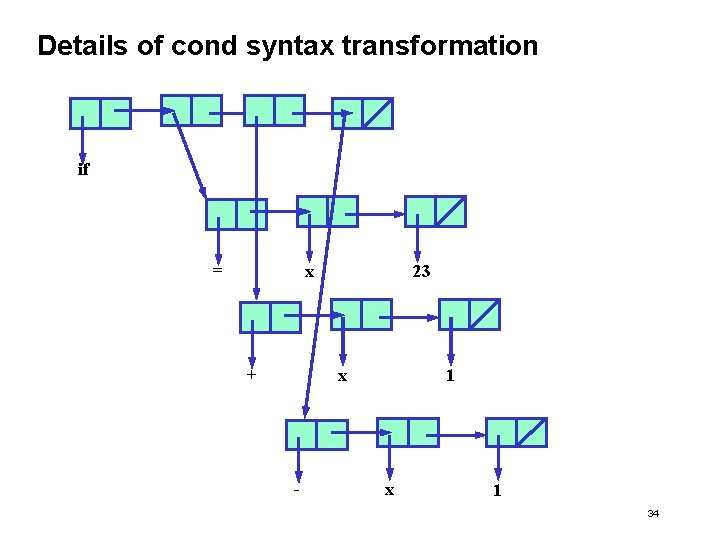
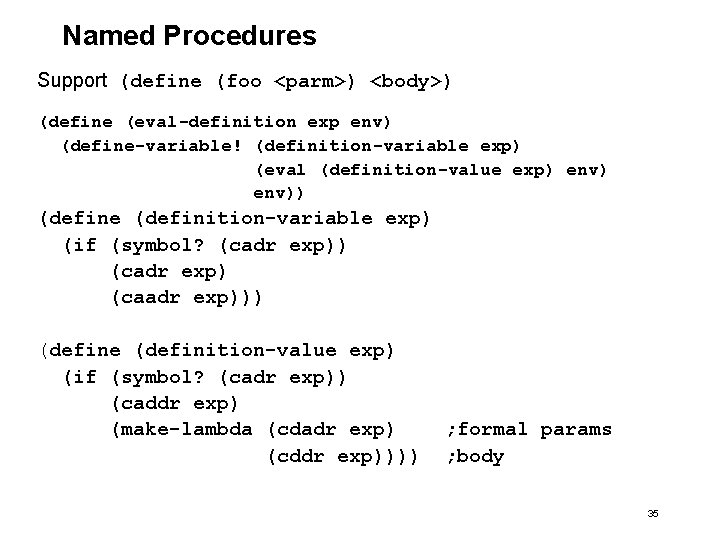
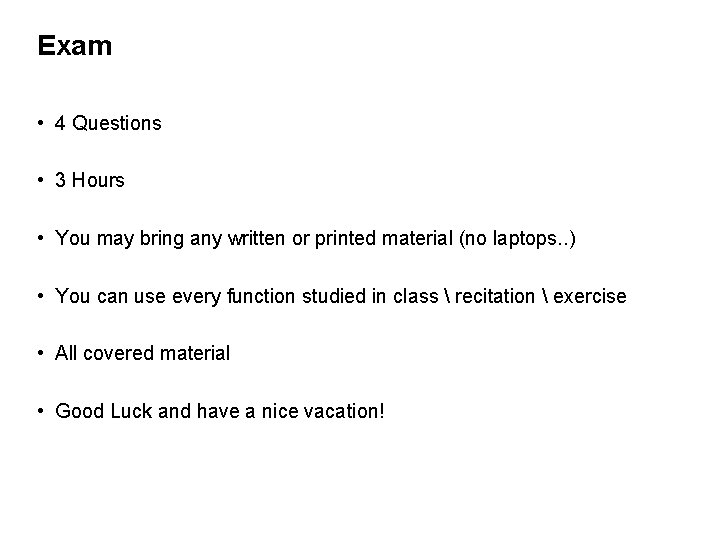
- Slides: 30
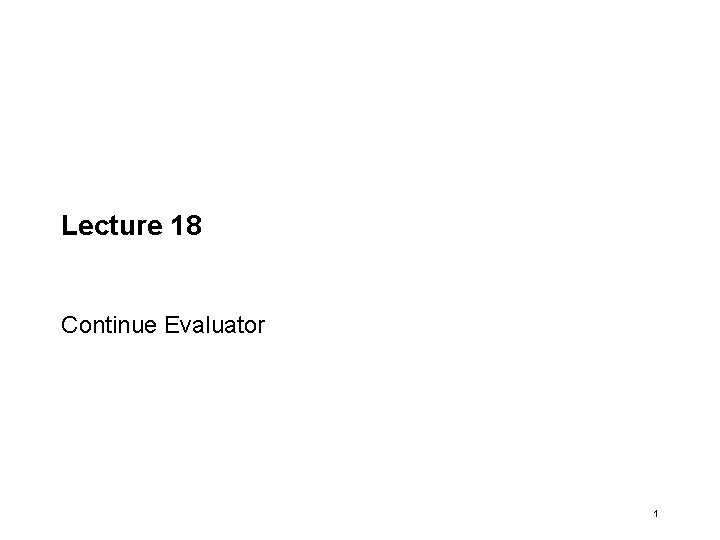
Lecture 18 Continue Evaluator 1
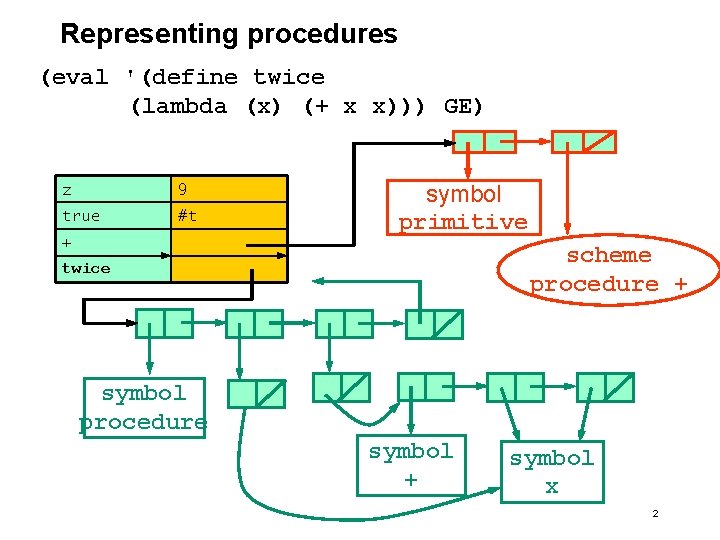
Representing procedures (eval '(define twice (lambda (x) (+ x x))) GE) z 9 true #t + symbol primitive scheme procedure + twice symbol procedure symbol + symbol x 2
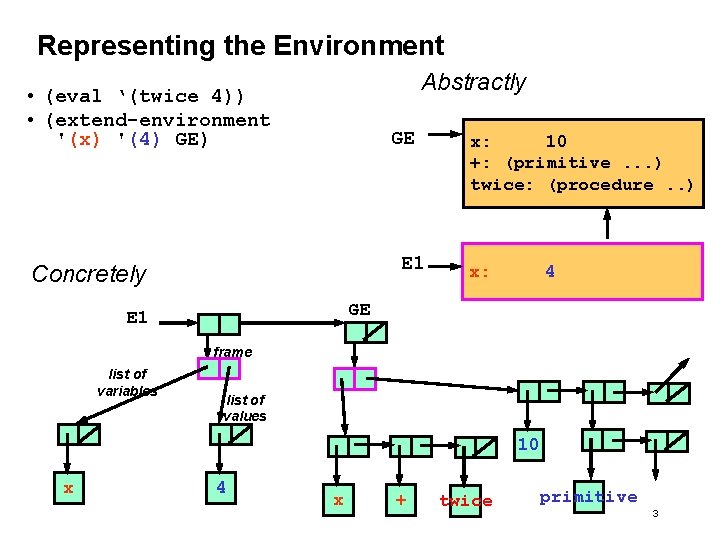
Representing the Environment Abstractly • (eval ‘(twice 4)) • (extend-environment '(x) '(4) GE E 1 Concretely x: 10 +: (primitive. . . ) twice: (procedure. . ) x: 4 GE E 1 frame list of variables list of values 10 x 4 x + twice primitive 3
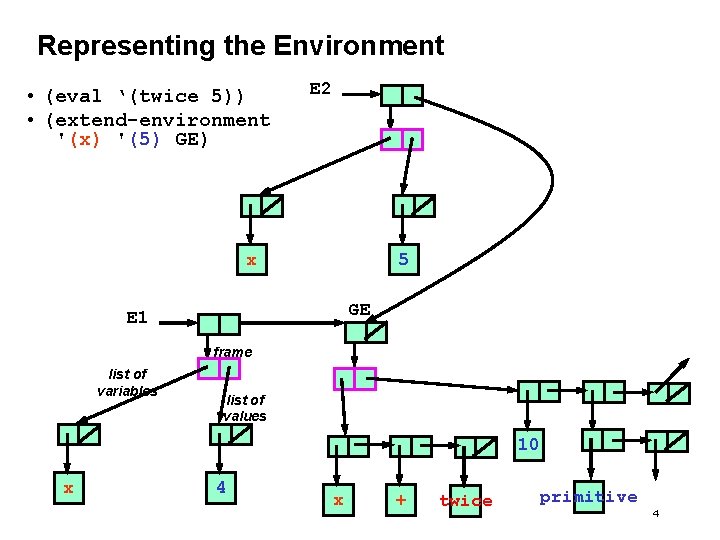
Representing the Environment • (eval ‘(twice 5)) • (extend-environment '(x) '(5) GE) E 2 x 5 GE E 1 frame list of variables list of values 10 x 4 x + twice primitive 4
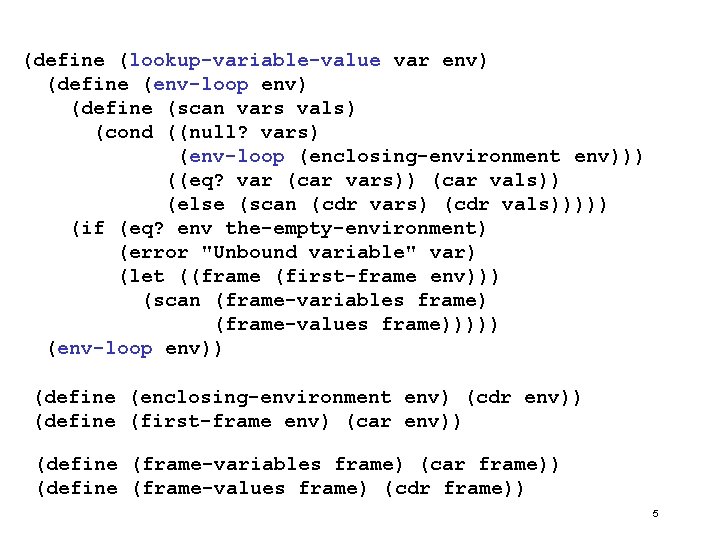
(define (lookup-variable-value var env) (define (env-loop env) (define (scan vars vals) (cond ((null? vars) (env-loop (enclosing-environment env))) ((eq? var (car vars)) (car vals)) (else (scan (cdr vars) (cdr vals))))) (if (eq? env the-empty-environment) (error "Unbound variable" var) (let ((frame (first-frame env))) (scan (frame-variables frame) (frame-values frame))))) (env-loop env)) (define (enclosing-environment env) (cdr env)) (define (first-frame env) (car env)) (define (frame-variables frame) (car frame)) (define (frame-values frame) (cdr frame)) 5
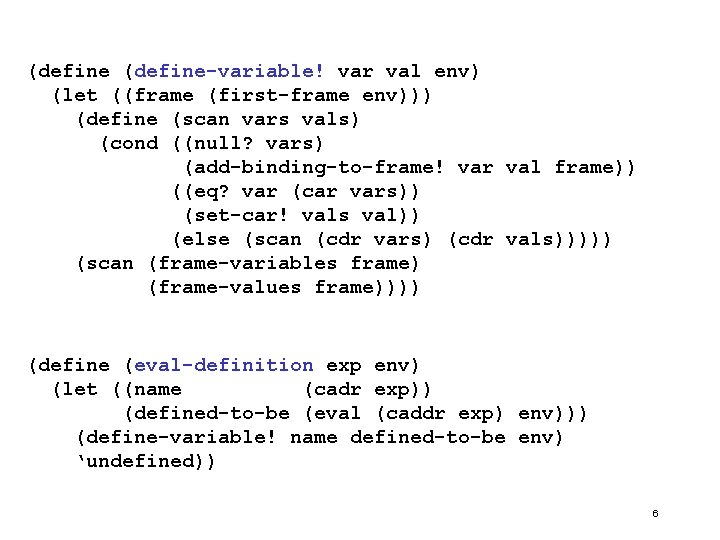
(define-variable! var val env) (let ((frame (first-frame env))) (define (scan vars vals) (cond ((null? vars) (add-binding-to-frame! var val frame)) ((eq? var (car vars)) (set-car! vals val)) (else (scan (cdr vars) (cdr vals))))) (scan (frame-variables frame) (frame-values frame)))) (define (eval-definition exp env) (let ((name (cadr exp)) (defined-to-be (eval (caddr exp) env))) (define-variable! name defined-to-be env) ‘undefined)) 6
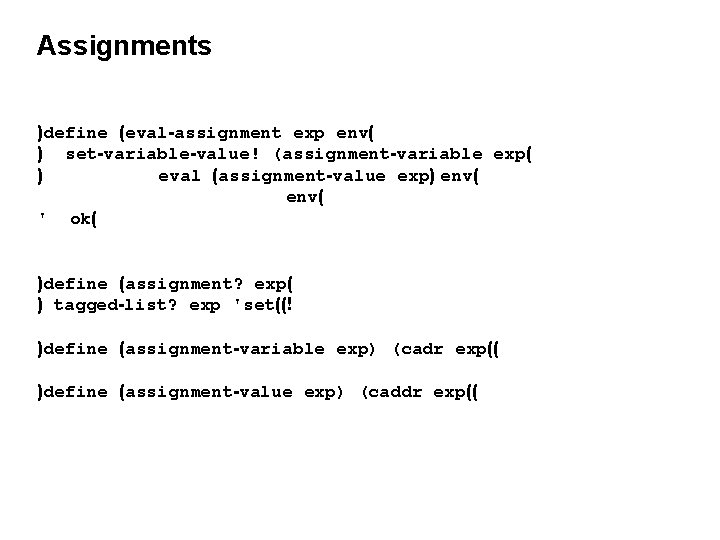
Assignments )define (eval-assignment exp env( ) set-variable-value! (assignment-variable exp( ) eval (assignment-value exp) env( ' ok( )define (assignment? exp( ) tagged-list? exp 'set((! )define (assignment-variable exp) (cadr exp(( )define (assignment-value exp) (caddr exp((
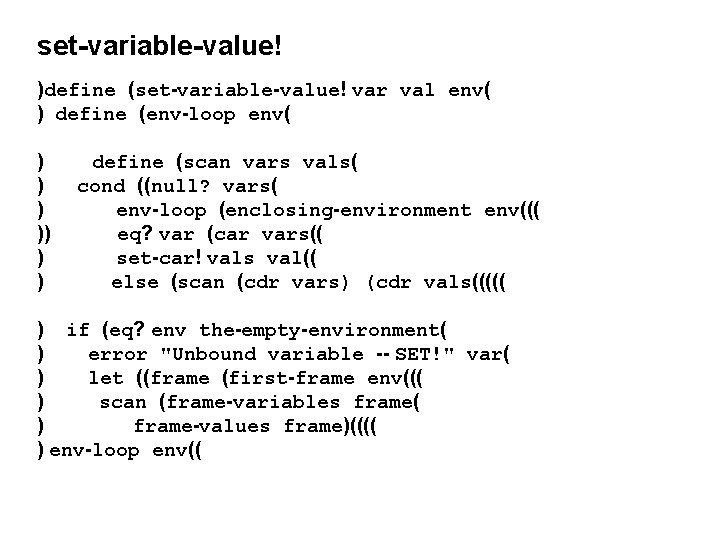
set-variable-value! )define (set-variable-value! var val env( ) define (env-loop env( ) )) ) ) define (scan vars vals( cond ((null? vars( env-loop (enclosing-environment env((( eq? var (car vars(( set-car! vals val(( else (scan (cdr vars) (cdr vals((((( ) if (eq? env the-empty-environment( ) error "Unbound variable -- SET!" var( ) let ((frame (first-frame env((( ) scan (frame-variables frame( ) frame-values frame)(((( ) env-loop env((
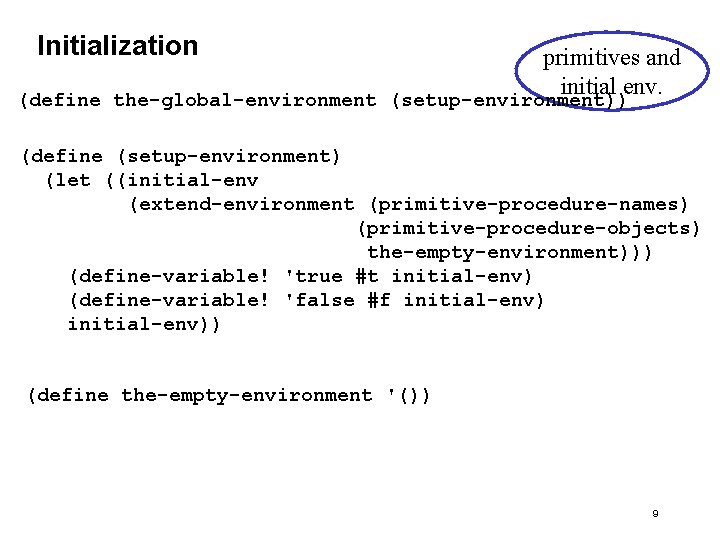
Initialization primitives and initial env. (define the-global-environment (setup-environment)) (define (setup-environment) (let ((initial-env (extend-environment (primitive-procedure-names) (primitive-procedure-objects) the-empty-environment))) (define-variable! 'true #t initial-env) (define-variable! 'false #f initial-env)) (define the-empty-environment '()) 9
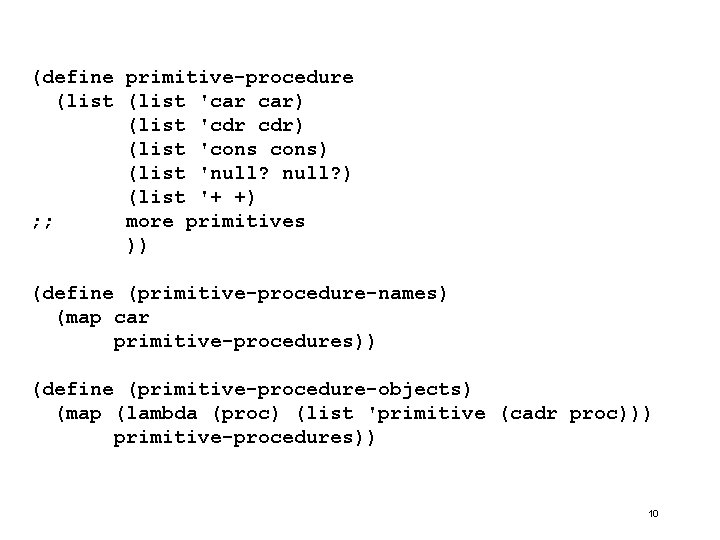
(define primitive-procedure (list 'car car) (list 'cdr cdr) (list 'cons) (list 'null? ) (list '+ +) ; ; more primitives )) (define (primitive-procedure-names) (map car primitive-procedures)) (define (primitive-procedure-objects) (map (lambda (proc) (list 'primitive (cadr proc))) primitive-procedures)) 10
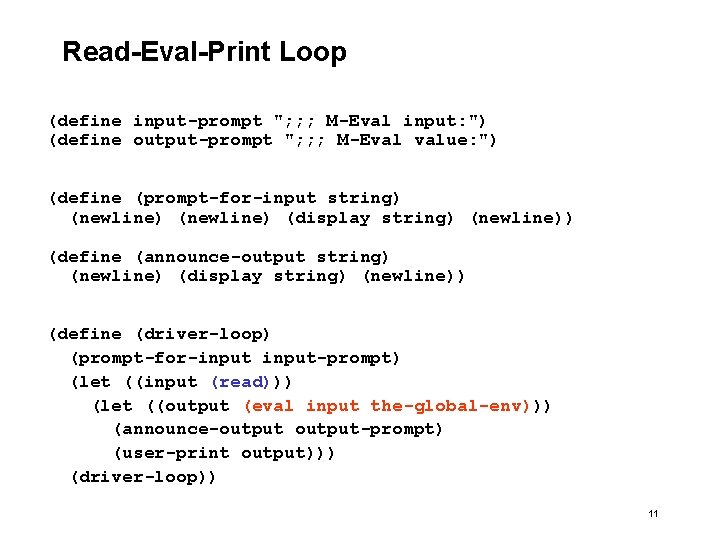
Read-Eval-Print Loop (define input-prompt "; ; ; M-Eval input: ") (define output-prompt "; ; ; M-Eval value: ") (define (prompt-for-input string) (newline) (display string) (newline)) (define (announce-output string) (newline) (display string) (newline)) (define (driver-loop) (prompt-for-input-prompt) (let ((input (read))) (let ((output (eval input the-global-env))) (announce-output-prompt) (user-print output))) (driver-loop)) 11
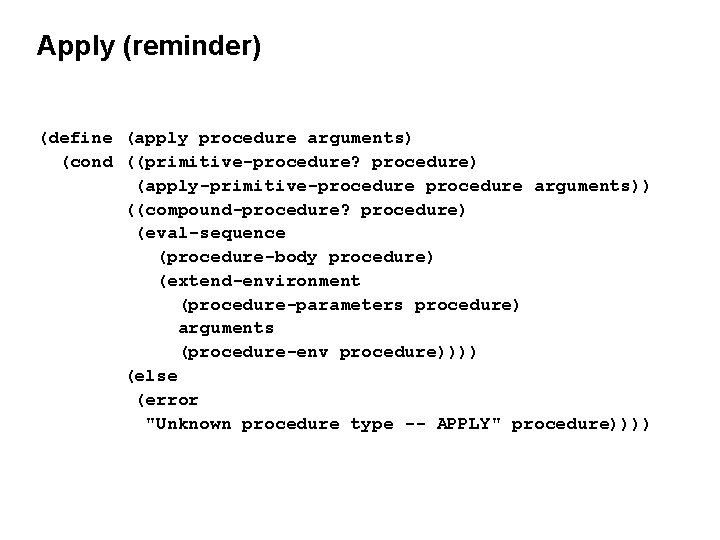
Apply (reminder) (define (apply procedure arguments) (cond ((primitive-procedure? procedure) (apply-primitive-procedure arguments)) ((compound-procedure? procedure) (eval-sequence (procedure-body procedure) (extend-environment (procedure-parameters procedure) arguments (procedure-env procedure)))) (else (error "Unknown procedure type -- APPLY" procedure))))
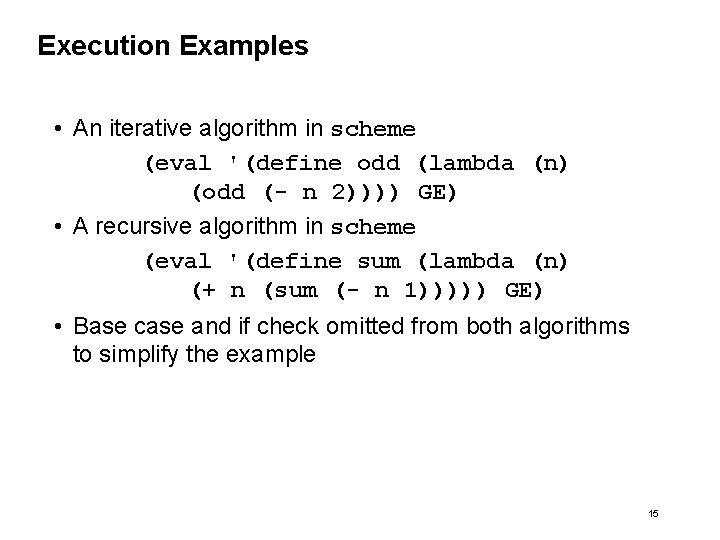
Execution Examples • An iterative algorithm in scheme (eval '(define odd (lambda (n) (odd (- n 2)))) GE) • A recursive algorithm in scheme (eval '(define sum (lambda (n) (+ n (sum (- n 1))))) GE) • Base case and if check omitted from both algorithms to simplify the example 15
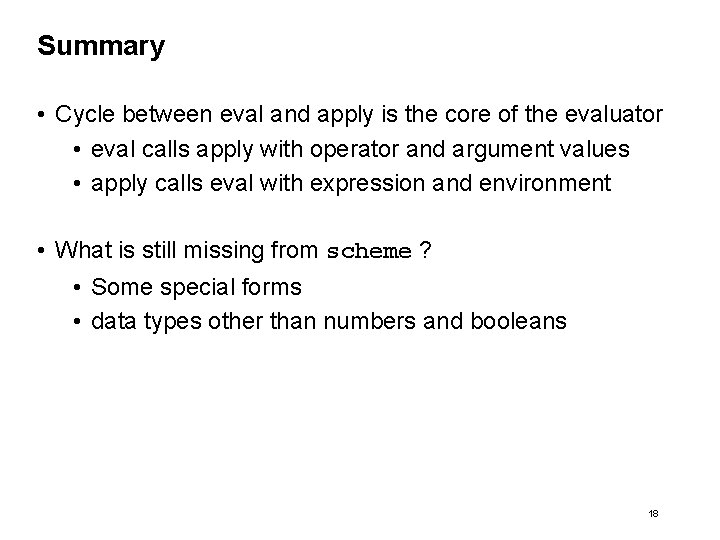
Summary • Cycle between eval and apply is the core of the evaluator • eval calls apply with operator and argument values • apply calls eval with expression and environment • What is still missing from scheme ? • Some special forms • data types other than numbers and booleans 18
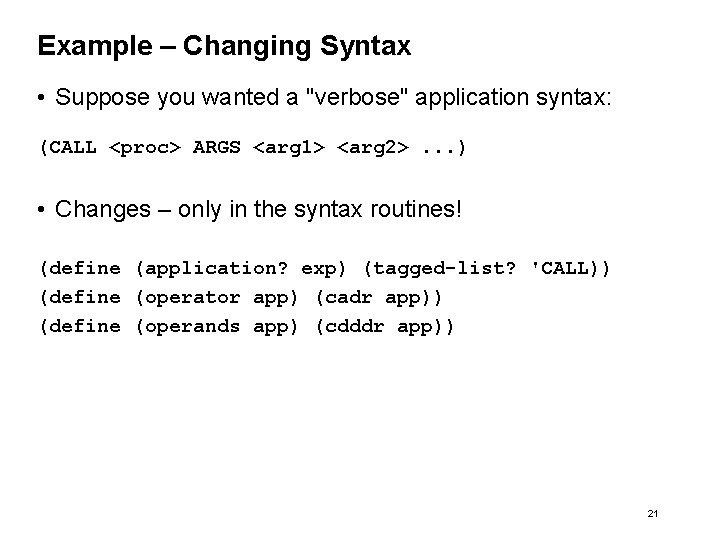
Example – Changing Syntax • Suppose you wanted a "verbose" application syntax: (CALL <proc> ARGS <arg 1> <arg 2>. . . ) • Changes – only in the syntax routines! (define (application? exp) (tagged-list? 'CALL)) (define (operator app) (cadr app)) (define (operands app) (cdddr app)) 21
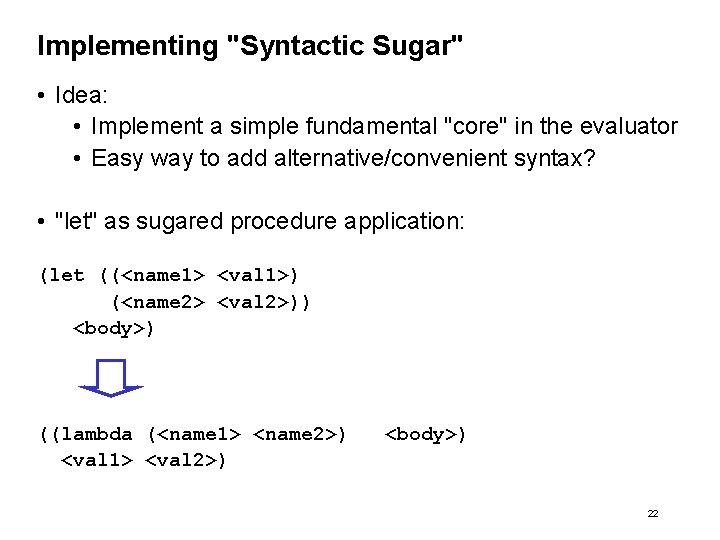
Implementing "Syntactic Sugar" • Idea: • Implement a simple fundamental "core" in the evaluator • Easy way to add alternative/convenient syntax? • "let" as sugared procedure application: (let ((<name 1> <val 1>) (<name 2> <val 2>)) <body>) ((lambda (<name 1> <name 2>) <val 1> <val 2>) <body>) 22
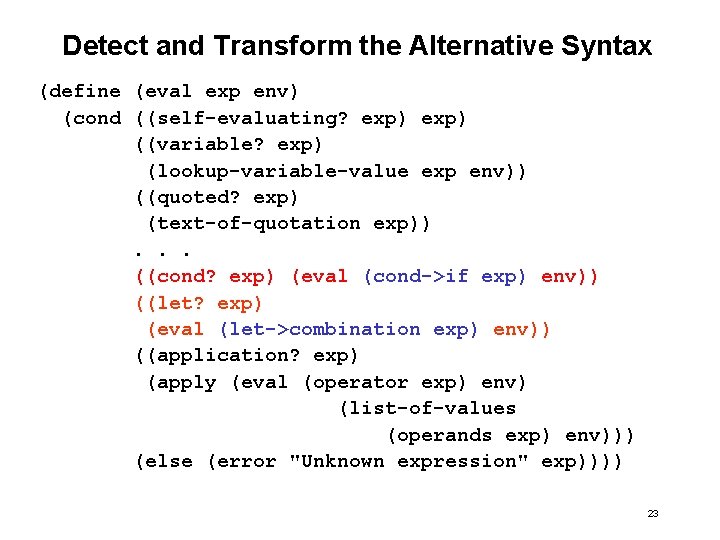
Detect and Transform the Alternative Syntax (define (eval exp env) (cond ((self-evaluating? exp) ((variable? exp) (lookup-variable-value exp env)) ((quoted? exp) (text-of-quotation exp)). . . ((cond? exp) (eval (cond->if exp) env)) ((let? exp) (eval (let->combination exp) env)) ((application? exp) (apply (eval (operator exp) env) (list-of-values (operands exp) env))) (else (error "Unknown expression" exp)))) 23
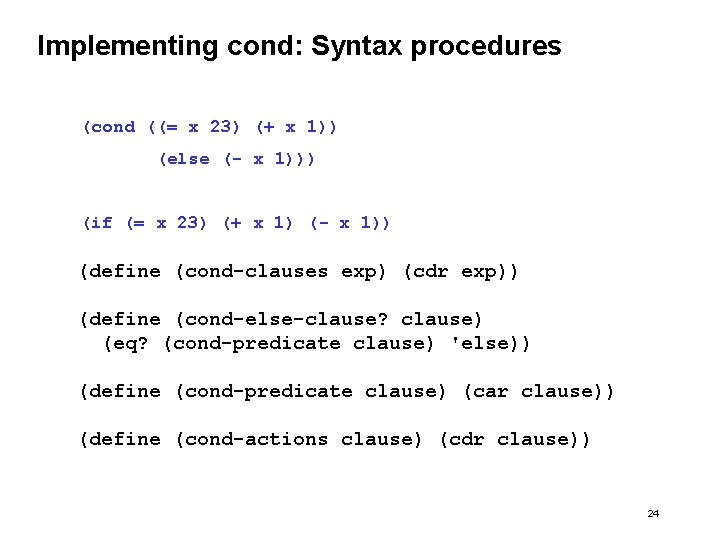
Implementing cond: Syntax procedures (cond ((= x 23) (+ x 1)) (else (- x 1))) (if (= x 23) (+ x 1) (- x 1)) (define (cond-clauses exp) (cdr exp)) (define (cond-else-clause? clause) (eq? (cond-predicate clause) 'else)) (define (cond-predicate clause) (car clause)) (define (cond-actions clause) (cdr clause)) 24
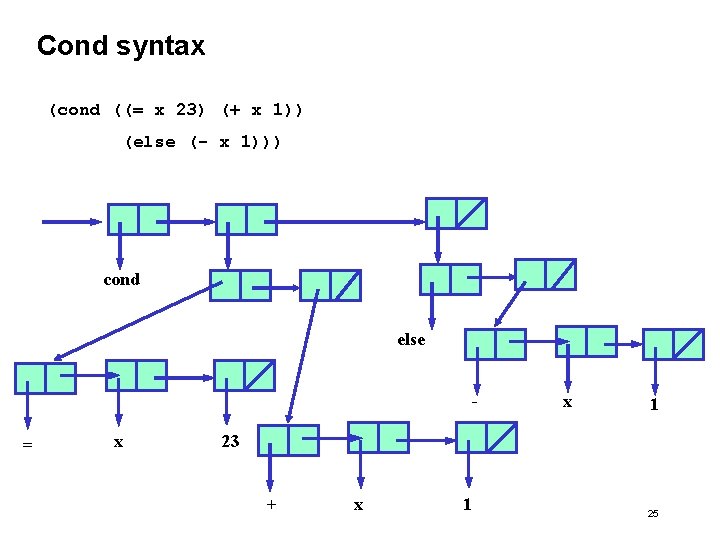
Cond syntax (cond ((= x 23) (+ x 1)) (else (- x 1))) cond else = x x 1 23 + x 1 25
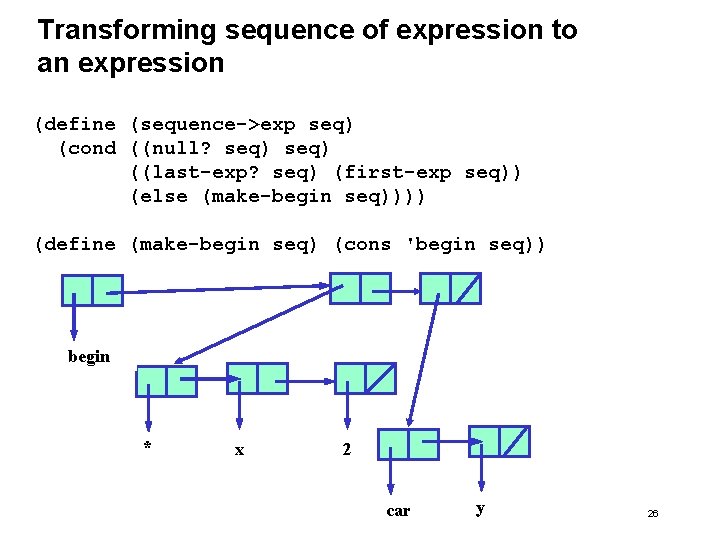
Transforming sequence of expression to an expression (define (sequence->exp seq) (cond ((null? seq) ((last-exp? seq) (first-exp seq)) (else (make-begin seq)))) (define (make-begin seq) (cons 'begin seq)) begin * x 2 car y 26
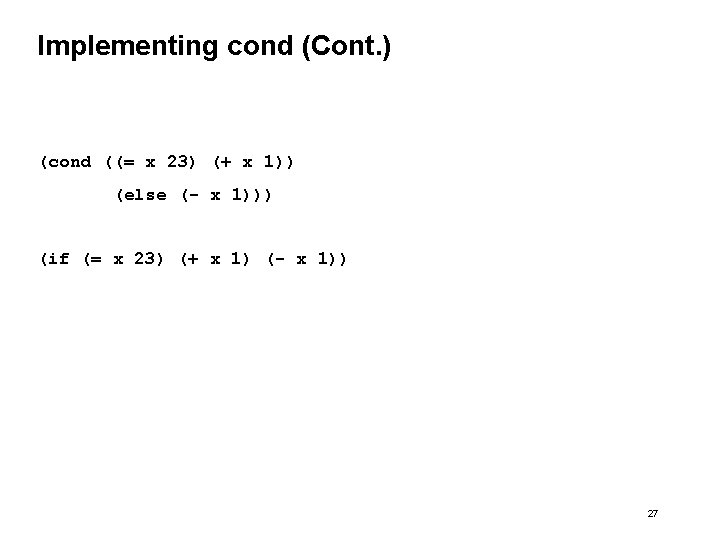
Implementing cond (Cont. ) (cond ((= x 23) (+ x 1)) (else (- x 1))) (if (= x 23) (+ x 1) (- x 1)) 27
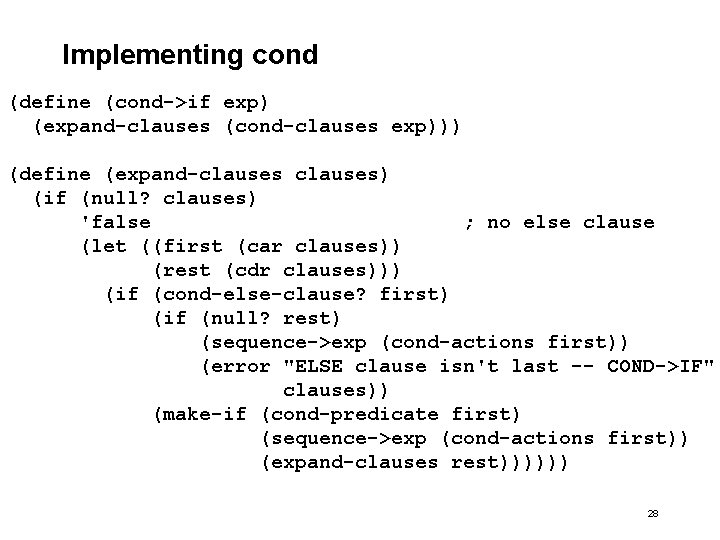
Implementing cond (define (cond->if exp) (expand-clauses (cond-clauses exp))) (define (expand-clauses) (if (null? clauses) 'false ; no else clause (let ((first (car clauses)) (rest (cdr clauses))) (if (cond-else-clause? first) (if (null? rest) (sequence->exp (cond-actions first)) (error "ELSE clause isn't last -- COND->IF" clauses)) (make-if (cond-predicate first) (sequence->exp (cond-actions first)) (expand-clauses rest)))))) 28
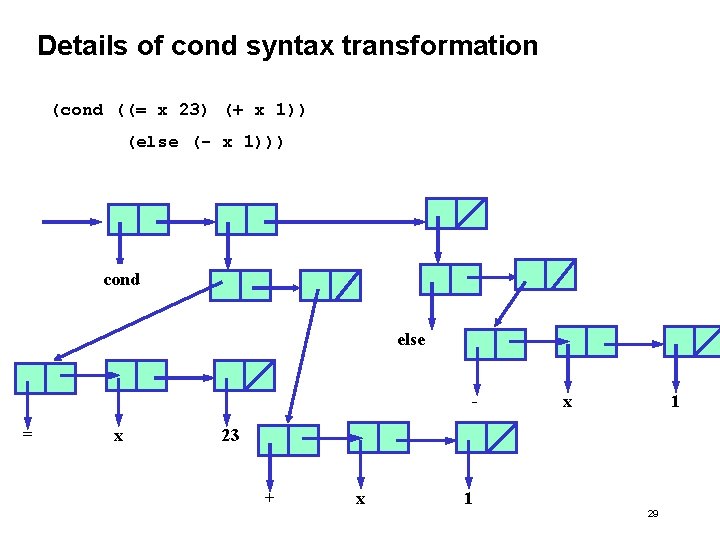
Details of cond syntax transformation (cond ((= x 23) (+ x 1)) (else (- x 1))) cond else = x x 1 23 + x 1 29
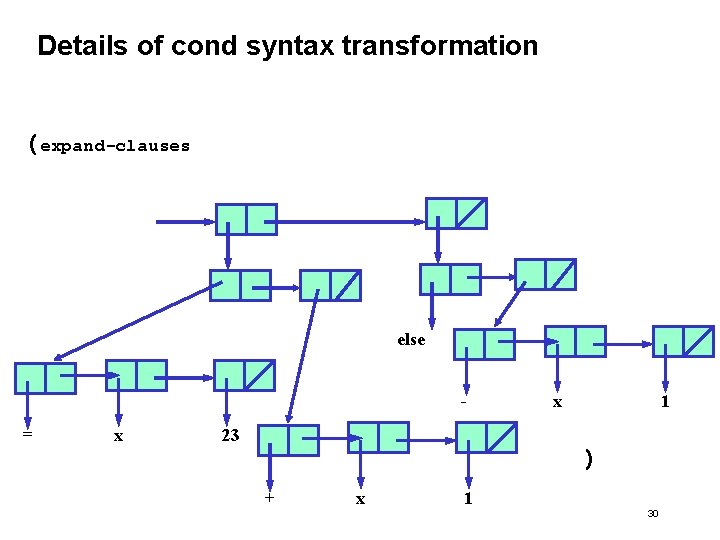
Details of cond syntax transformation (expand-clauses else = x 23 x 1 ) + x 1 30
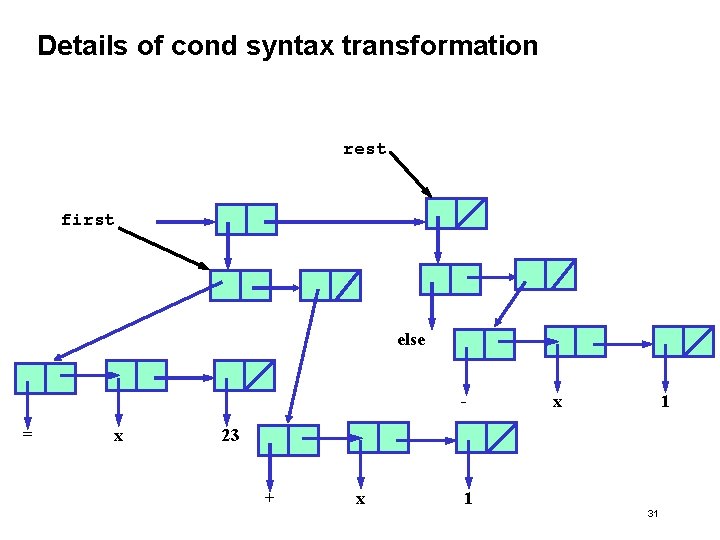
Details of cond syntax transformation rest first else = x x 1 23 + x 1 31
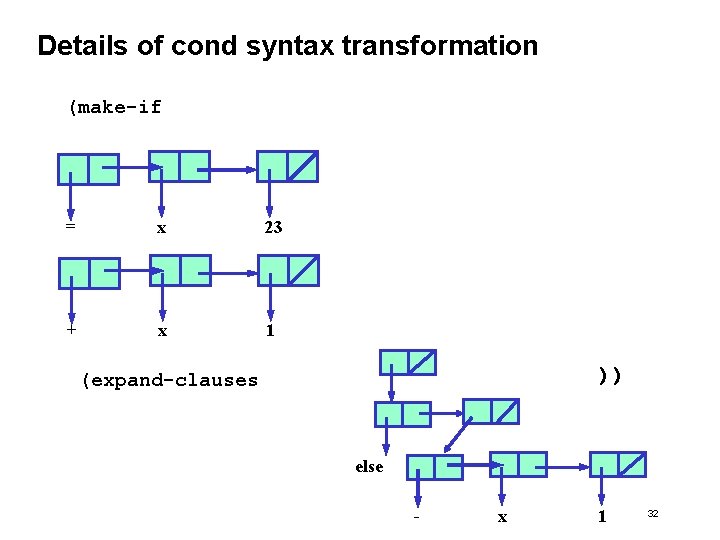
Details of cond syntax transformation (make-if = x 23 + x 1 )) (expand-clauses else - x 1 32
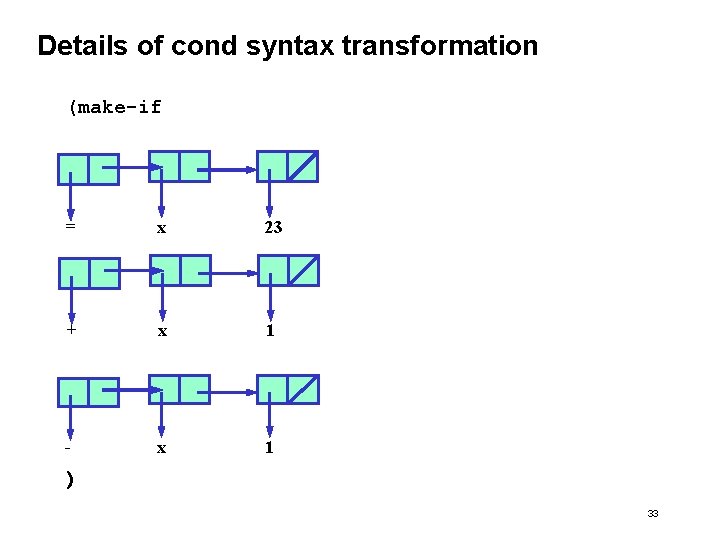
Details of cond syntax transformation (make-if = x 23 + x 1 - x 1 ) 33
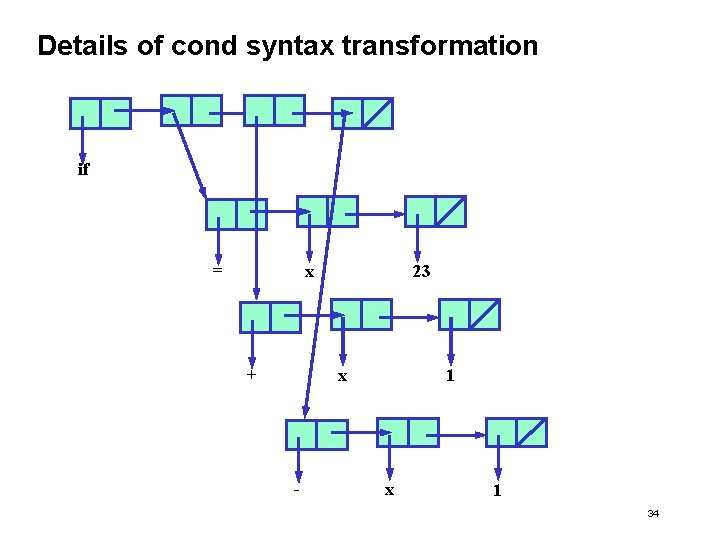
Details of cond syntax transformation if = x + 23 x - 1 x 1 34
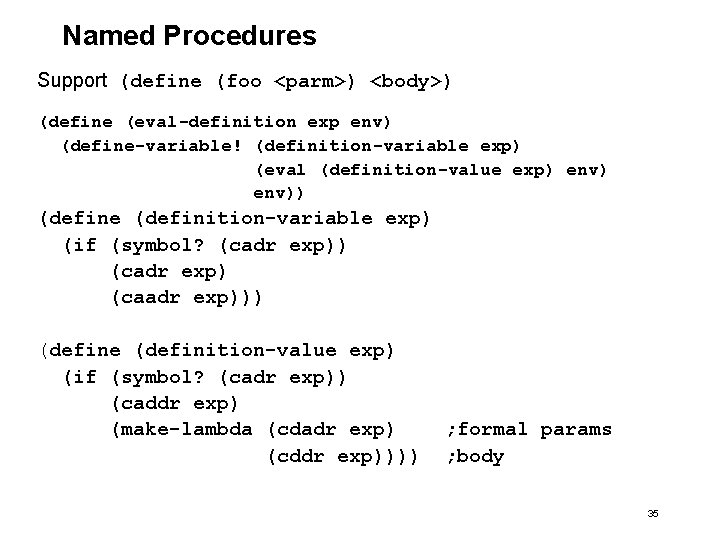
Named Procedures Support (define (foo <parm>) <body>) (define (eval-definition exp env) (define-variable! (definition-variable exp) (eval (definition-value exp) env)) (define (definition-variable exp) (if (symbol? (cadr exp)) (cadr exp) (caadr exp))) (define (definition-value exp) (if (symbol? (cadr exp)) (caddr exp) (make-lambda (cdadr exp) (cddr exp)))) ; formal params ; body 35
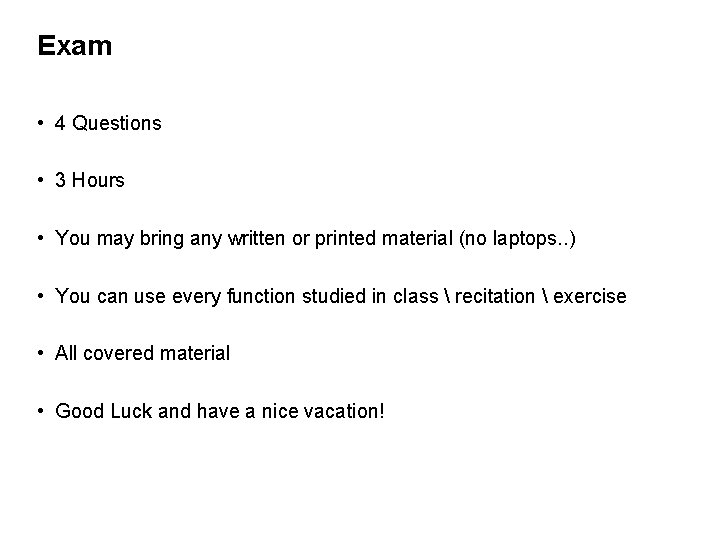
Exam • 4 Questions • 3 Hours • You may bring any written or printed material (no laptops. . ) • You can use every function studied in class recitation exercise • All covered material • Good Luck and have a nice vacation!
01:640:244 lecture notes - lecture 15: plat, idah, farad
Financial evaluator adalah
External evaluator
Excel formula evaluator
Evaluator eol
Marketing mix evaluator
Veriforce oq evaluator
Moodle emp
Evaluator competencies
Collegiate approach
Metacircular evaluator
External evaluator
Turbovisory system
Function evaluator
Wveis ed eval
Dr eval
Collateral duties eval block
Cpo eval bullets
Qualit eval
Https://eval.depp.taocloud.org/
Dr eval
Eval command
Requestmidiaccess
Bbs eval nibis
Tonya eval
Initial eval
Dr eval
How to write a fitrep
Representing sample spaces
Draw a picture representing monarchy.
Adding multiple vectors