Lecture 16 Quicker Sorting CS 200 Computer Science
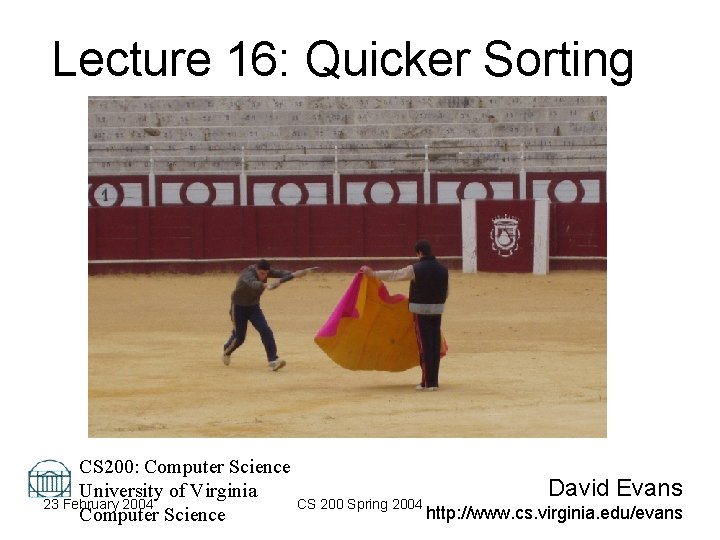
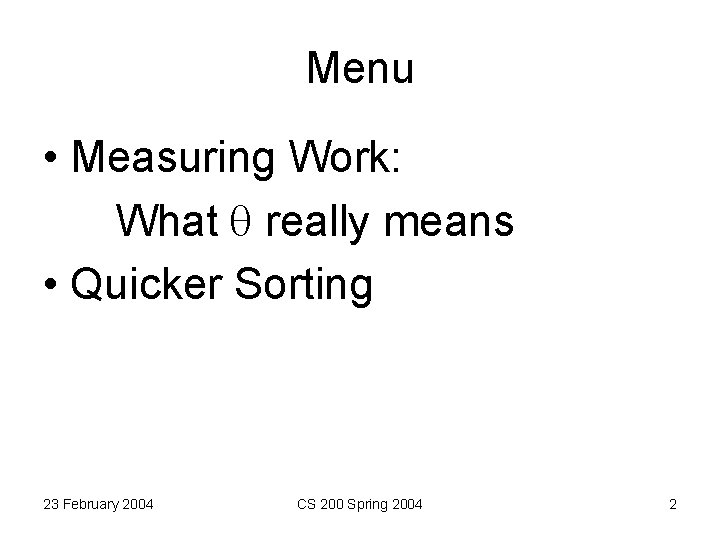
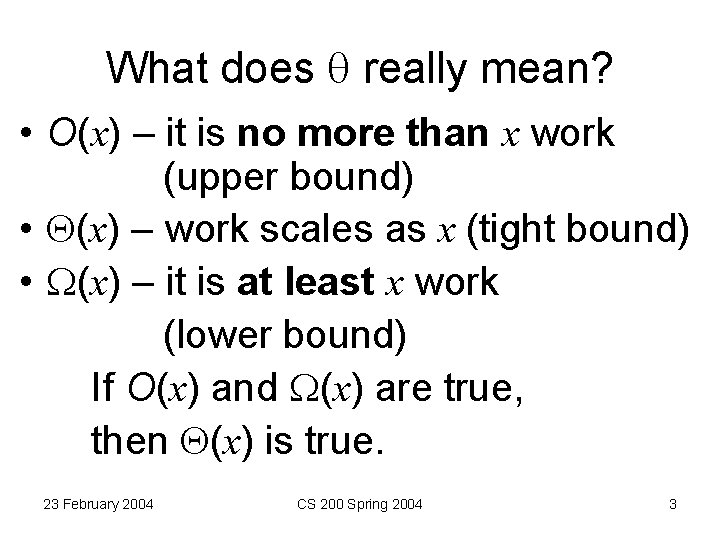
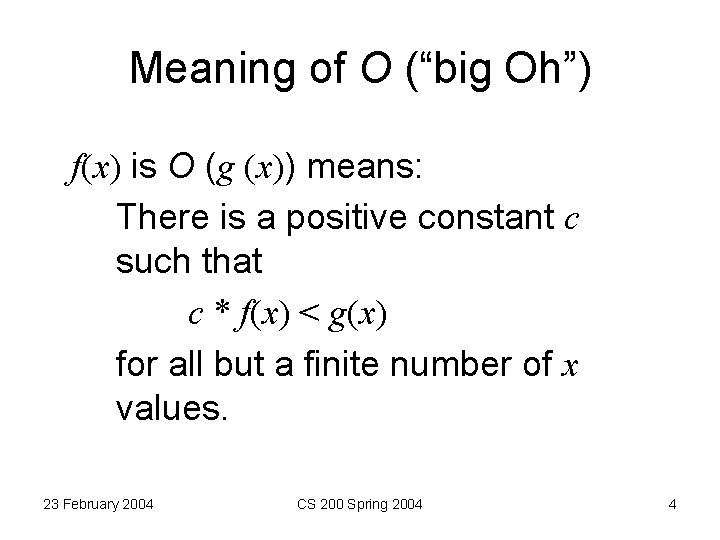
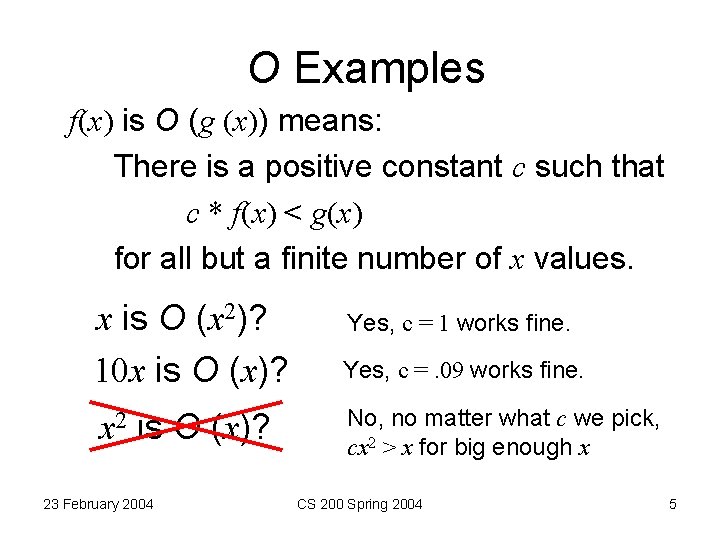
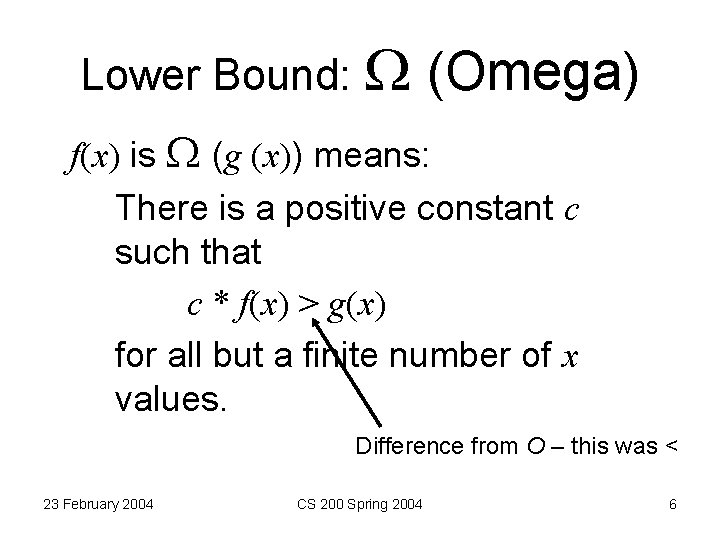
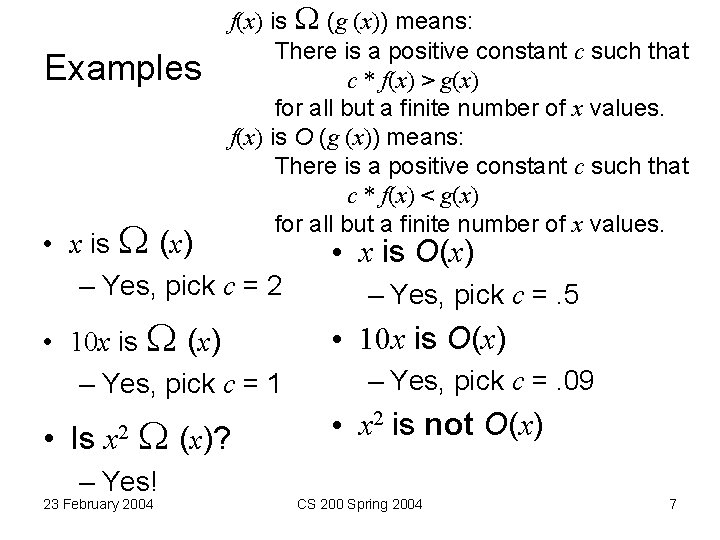
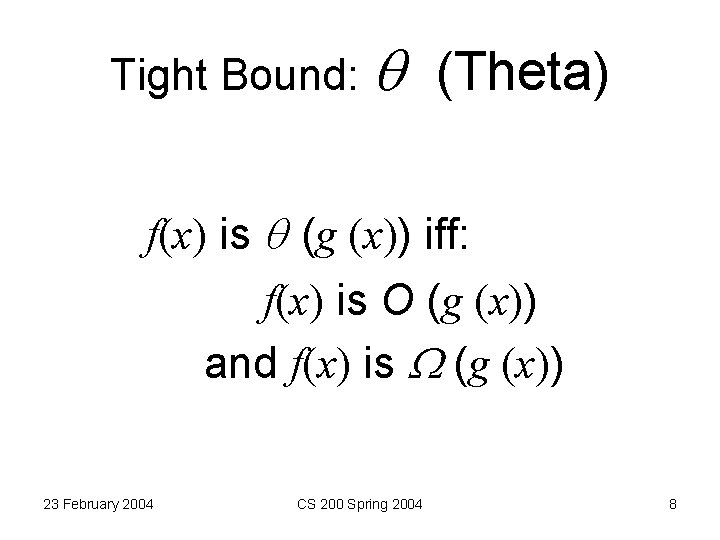
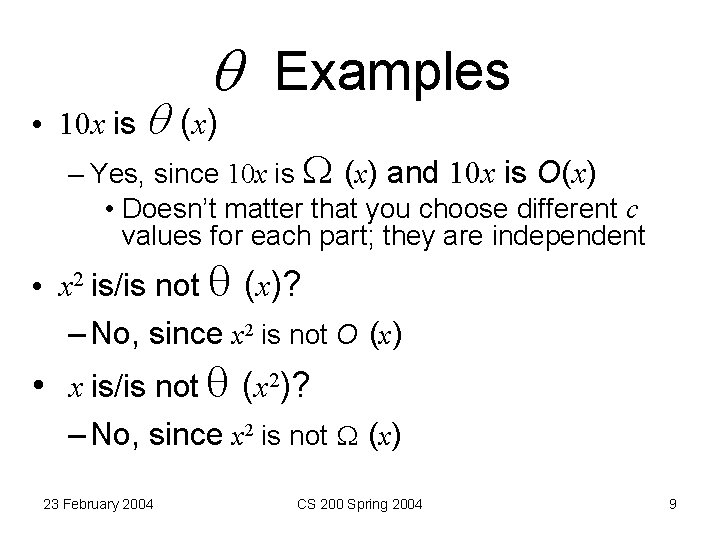
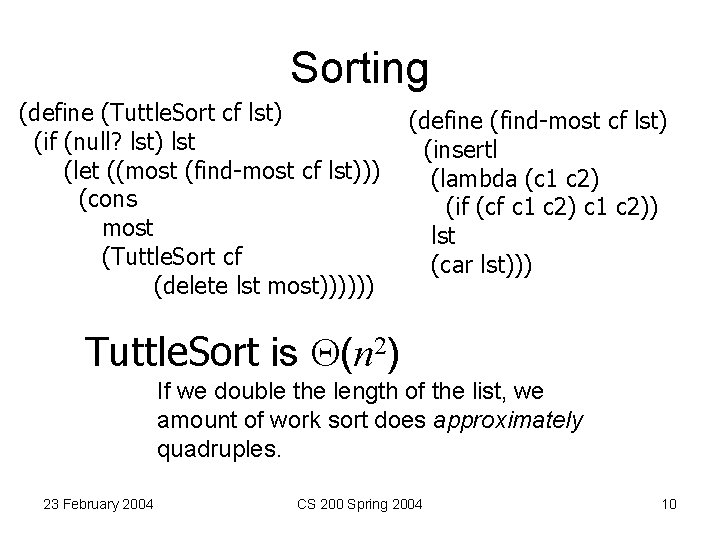
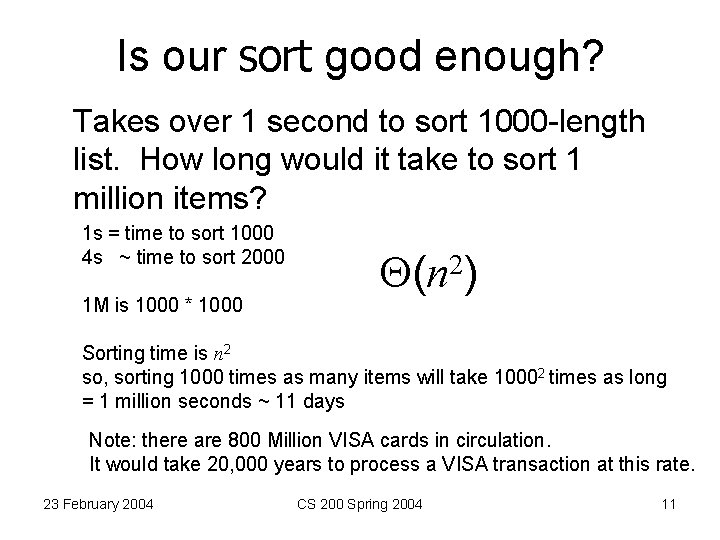
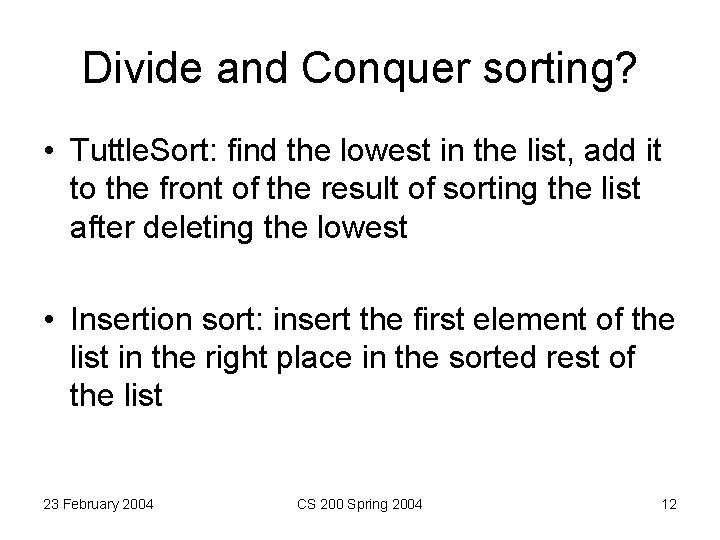
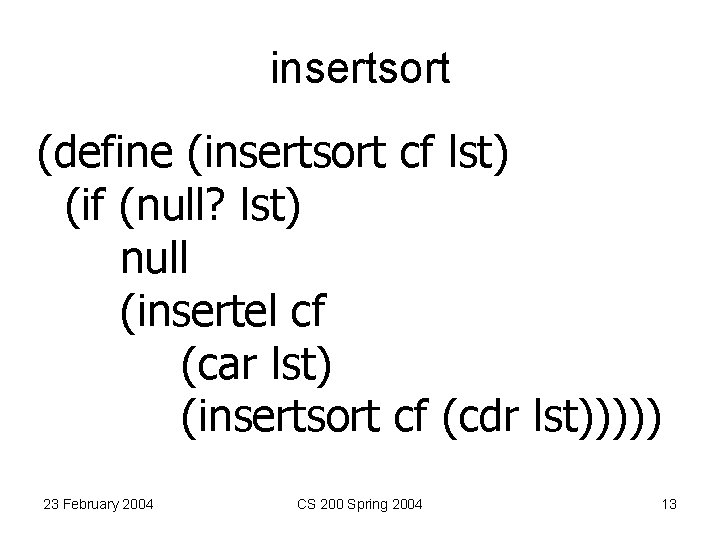
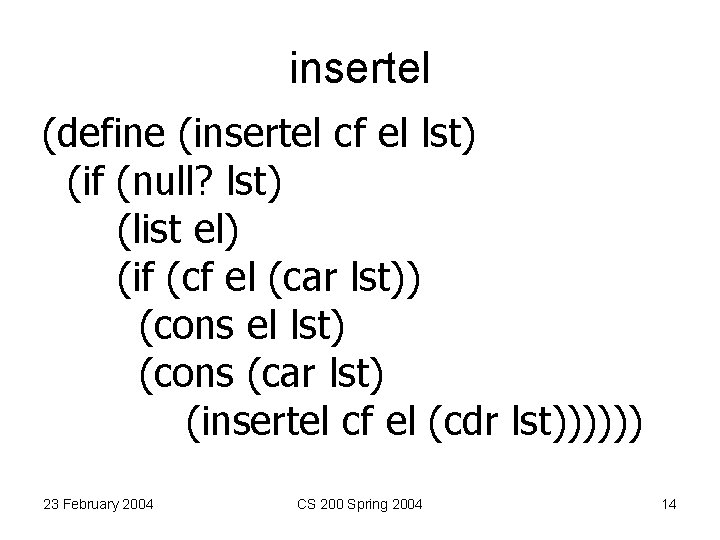
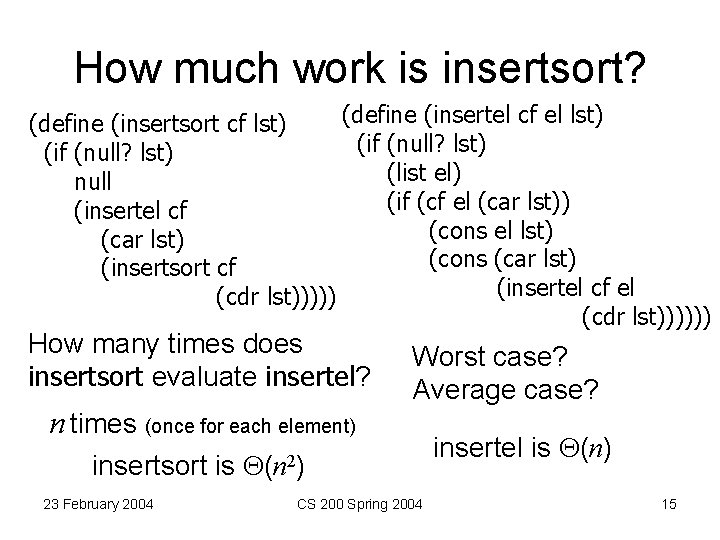
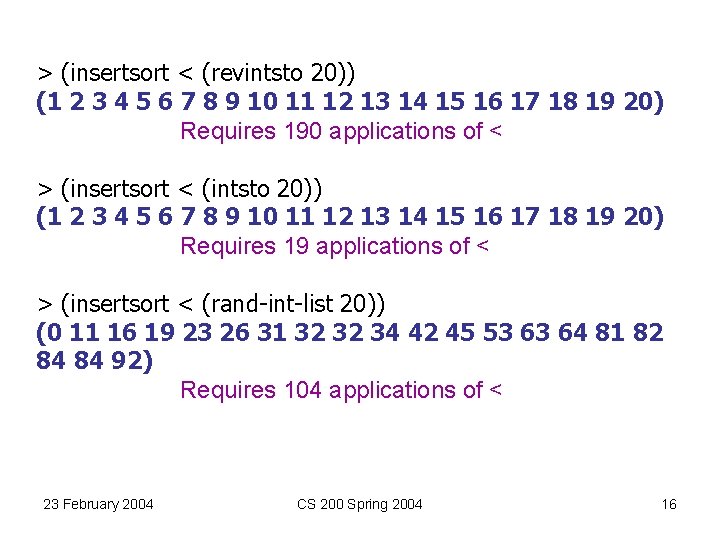
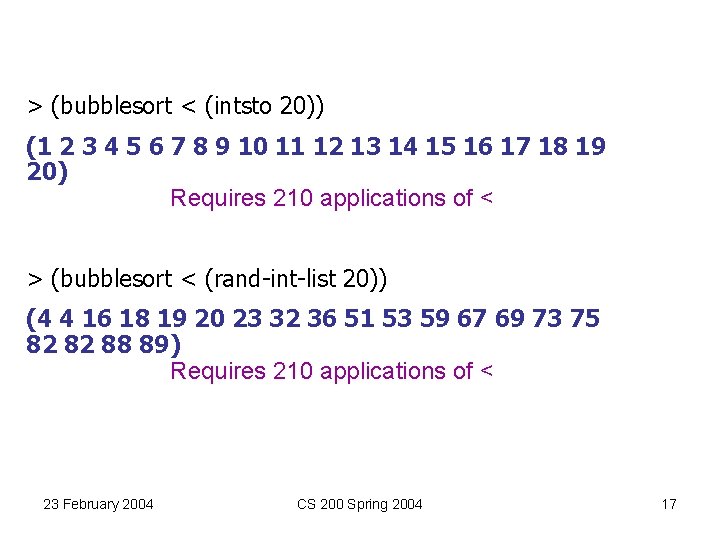
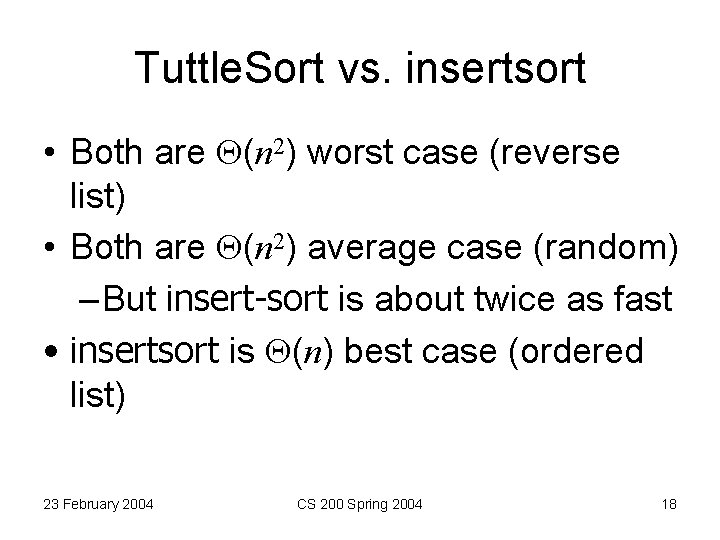
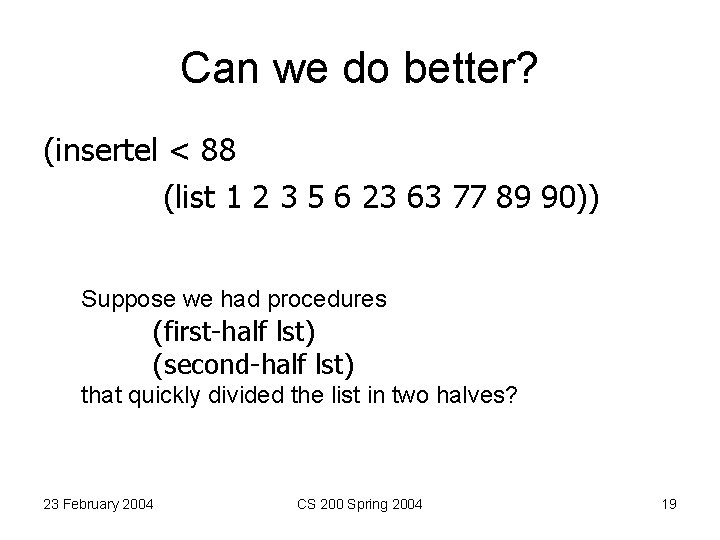
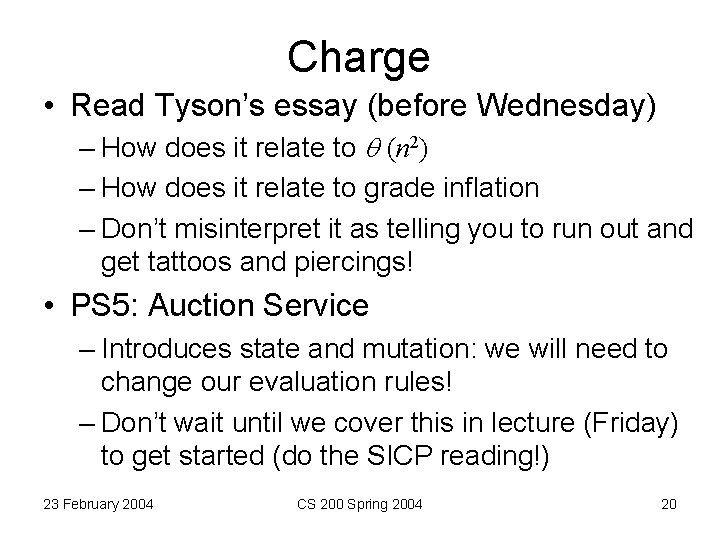
- Slides: 20
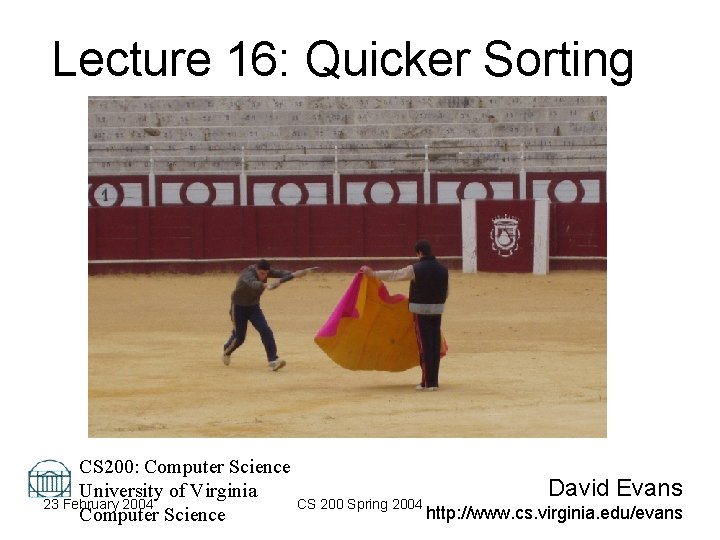
Lecture 16: Quicker Sorting CS 200: Computer Science David Evans University of Virginia 23 February 2004 CS 200 Spring 2004 http: //www. cs. virginia. edu/evans Computer Science
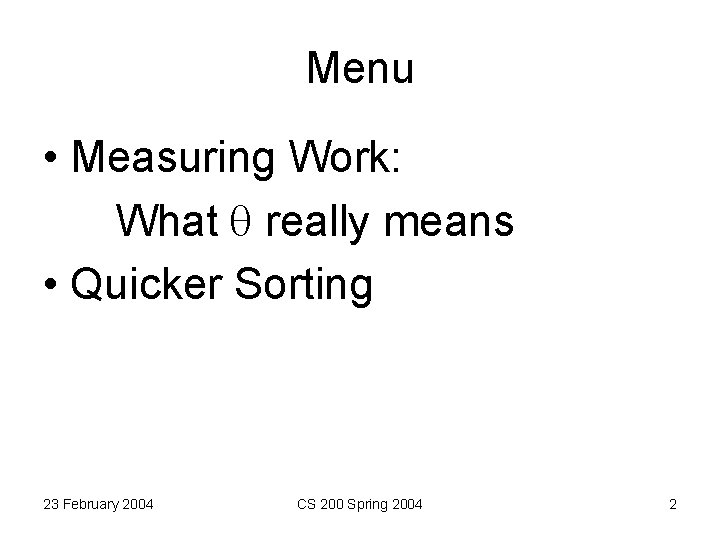
Menu • Measuring Work: What really means • Quicker Sorting 23 February 2004 CS 200 Spring 2004 2
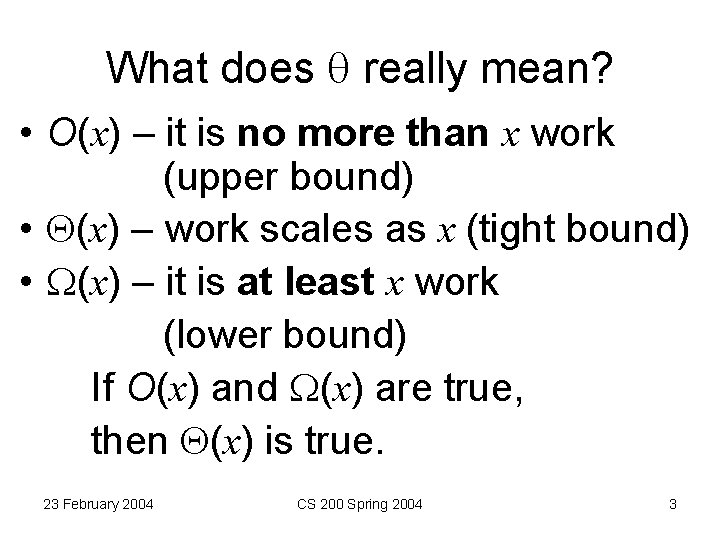
What does really mean? • O(x) – it is no more than x work (upper bound) • (x) – work scales as x (tight bound) • (x) – it is at least x work (lower bound) If O(x) and (x) are true, then (x) is true. 23 February 2004 CS 200 Spring 2004 3
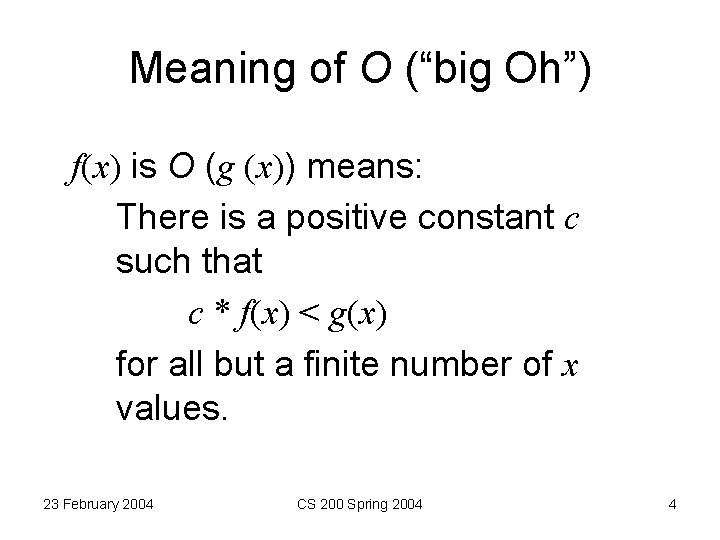
Meaning of O (“big Oh”) f(x) is O (g (x)) means: There is a positive constant c such that c * f(x) < g(x) for all but a finite number of x values. 23 February 2004 CS 200 Spring 2004 4
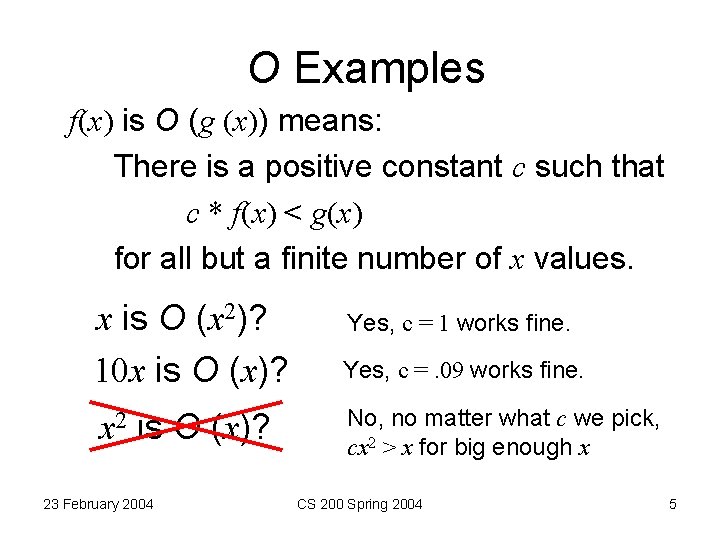
O Examples f(x) is O (g (x)) means: There is a positive constant c such that c * f(x) < g(x) for all but a finite number of x values. x is O (x 2)? 10 x is O (x)? x 2 is O (x)? 23 February 2004 Yes, c = 1 works fine. Yes, c =. 09 works fine. No, no matter what c we pick, cx 2 > x for big enough x CS 200 Spring 2004 5
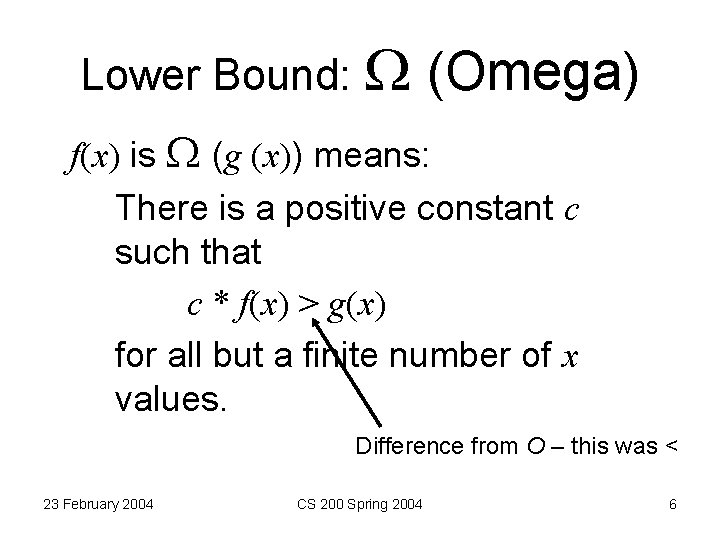
Lower Bound: (Omega) f(x) is (g (x)) means: There is a positive constant c such that c * f(x) > g(x) for all but a finite number of x values. Difference from O – this was < 23 February 2004 CS 200 Spring 2004 6
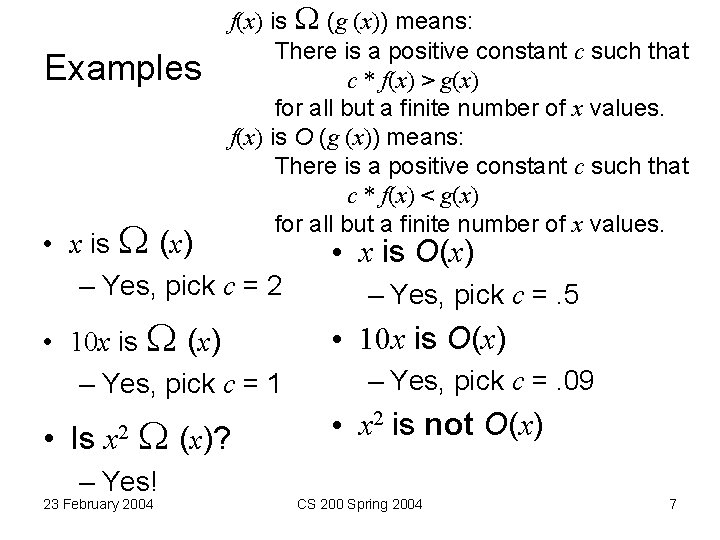
Examples f(x) is (g (x)) means: There is a positive constant c such that c * f(x) > g(x) for all but a finite number of x values. f(x) is O (g (x)) means: There is a positive constant c such that c * f(x) < g(x) for all but a finite number of x values. • x is (x) – Yes, pick c = 2 • 10 x is (x) – Yes, pick c = 1 • Is x 2 (x)? – Yes! 23 February 2004 • x is O(x) – Yes, pick c =. 5 • 10 x is O(x) – Yes, pick c =. 09 • x 2 is not O(x) CS 200 Spring 2004 7
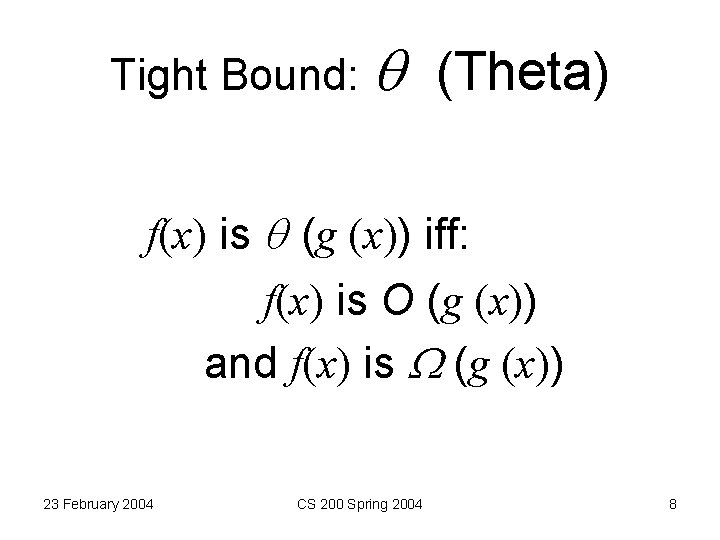
Tight Bound: (Theta) f(x) is (g (x)) iff: f(x) is O (g (x)) and f(x) is (g (x)) 23 February 2004 CS 200 Spring 2004 8
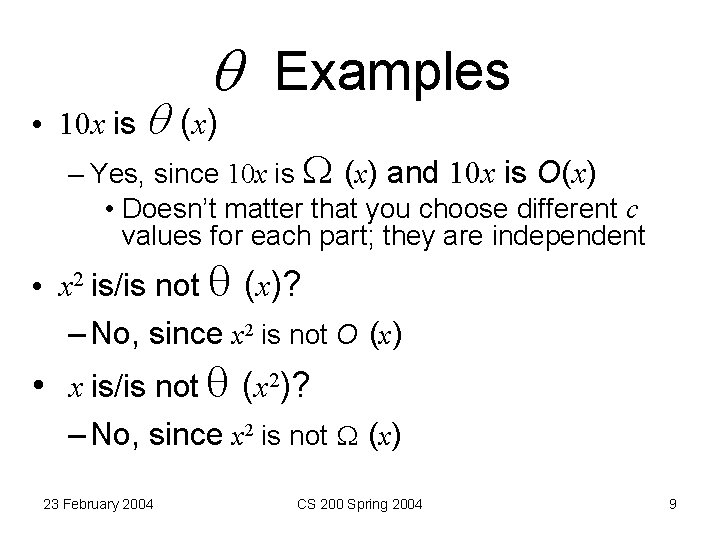
Examples • 10 x is (x) – Yes, since 10 x is (x) and 10 x is O(x) • Doesn’t matter that you choose different c values for each part; they are independent • x 2 is/is not (x)? – No, since x 2 is not O (x) • x is/is not (x 2)? – No, since x 2 is not (x) 23 February 2004 CS 200 Spring 2004 9
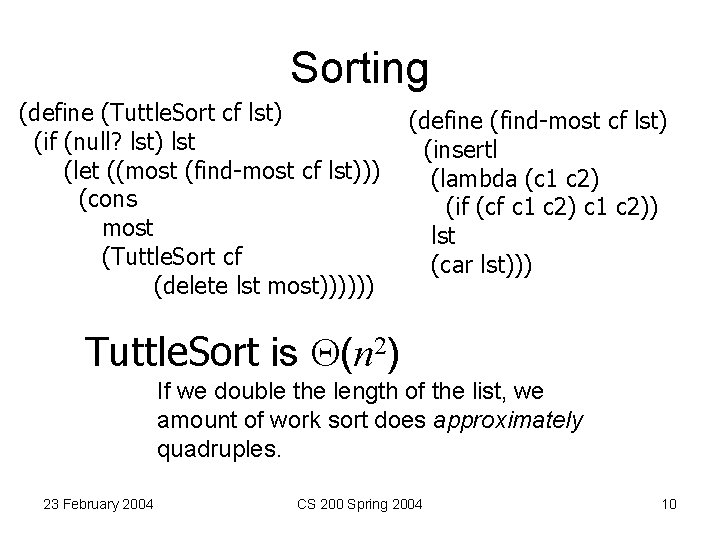
Sorting (define (Tuttle. Sort cf lst) (if (null? lst) lst (let ((most (find-most cf lst))) (cons most (Tuttle. Sort cf (delete lst most)))))) (define (find-most cf lst) (insertl (lambda (c 1 c 2) (if (cf c 1 c 2)) lst (car lst))) Tuttle. Sort is (n 2) If we double the length of the list, we amount of work sort does approximately quadruples. 23 February 2004 CS 200 Spring 2004 10
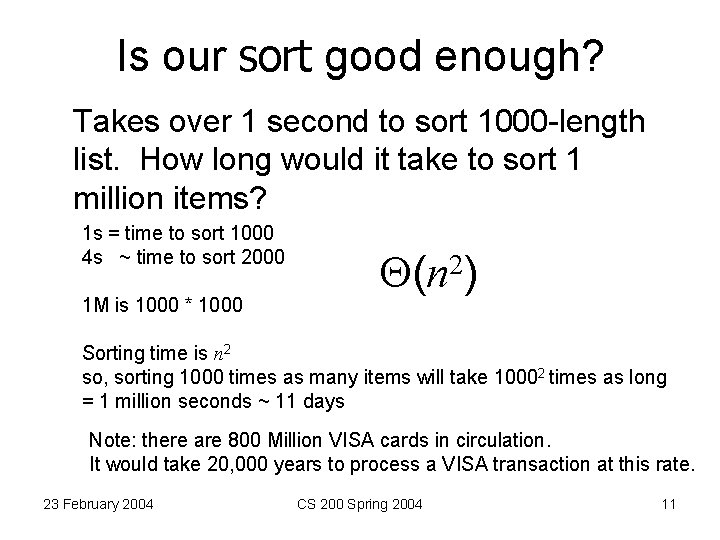
Is our sort good enough? Takes over 1 second to sort 1000 -length list. How long would it take to sort 1 million items? 1 s = time to sort 1000 4 s ~ time to sort 2000 1 M is 1000 * 1000 (n 2) Sorting time is n 2 so, sorting 1000 times as many items will take 10002 times as long = 1 million seconds ~ 11 days Note: there are 800 Million VISA cards in circulation. It would take 20, 000 years to process a VISA transaction at this rate. 23 February 2004 CS 200 Spring 2004 11
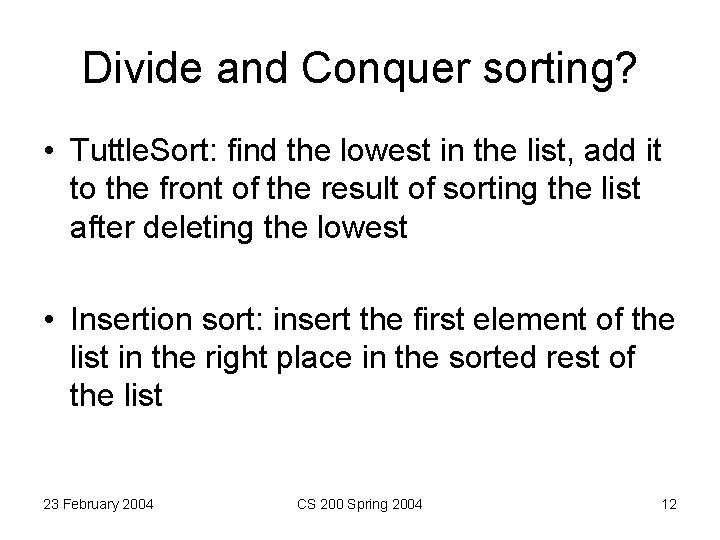
Divide and Conquer sorting? • Tuttle. Sort: find the lowest in the list, add it to the front of the result of sorting the list after deleting the lowest • Insertion sort: insert the first element of the list in the right place in the sorted rest of the list 23 February 2004 CS 200 Spring 2004 12
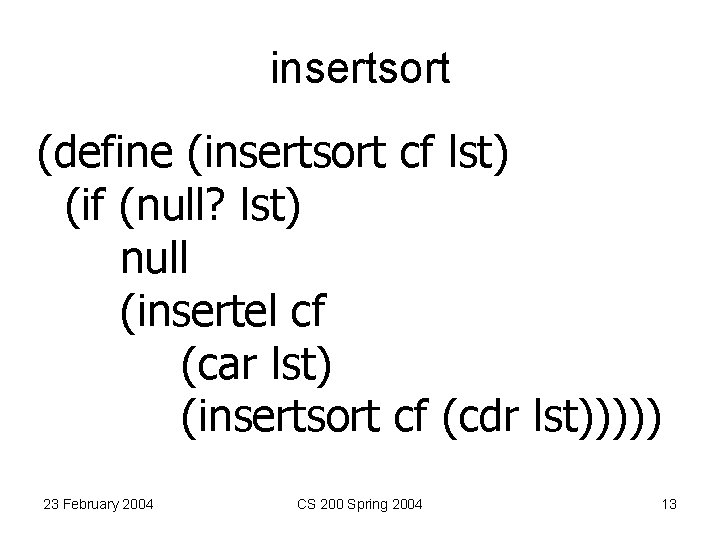
insertsort (define (insertsort cf lst) (if (null? lst) null (insertel cf (car lst) (insertsort cf (cdr lst))))) 23 February 2004 CS 200 Spring 2004 13
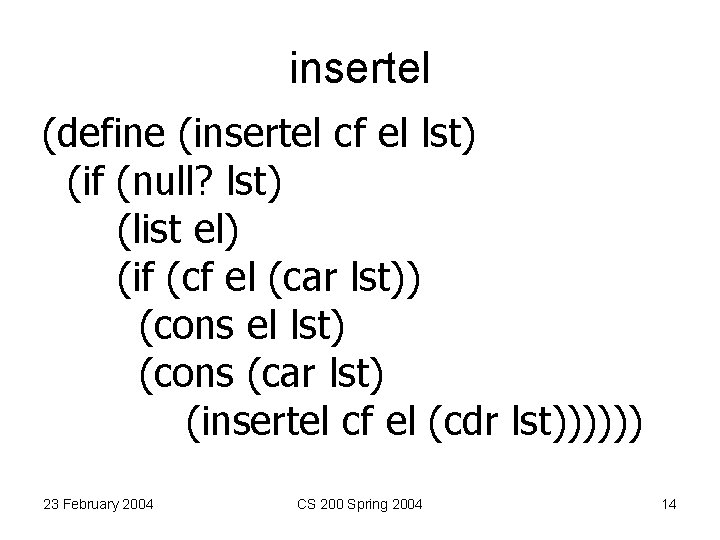
insertel (define (insertel cf el lst) (if (null? lst) (list el) (if (cf el (car lst)) (cons el lst) (cons (car lst) (insertel cf el (cdr lst)))))) 23 February 2004 CS 200 Spring 2004 14
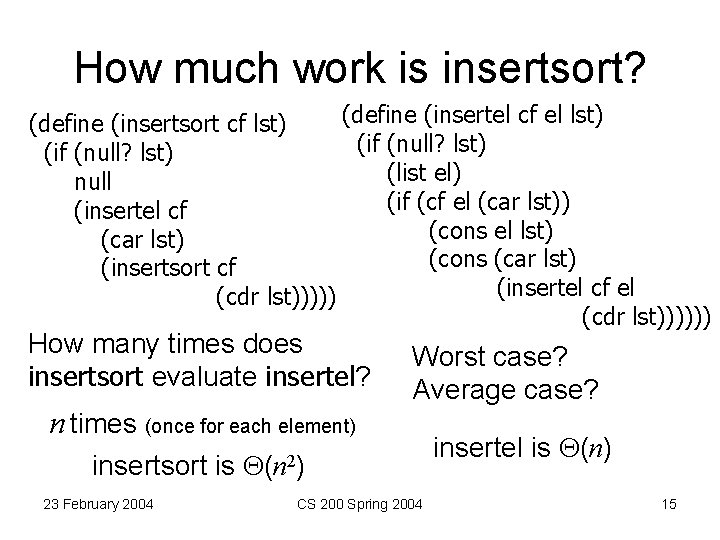
How much work is insertsort? (define (insertel cf el lst) (define (insertsort cf lst) (if (null? lst) (list el) null (if (cf el (car lst)) (insertel cf (cons el lst) (car lst) (cons (car lst) (insertsort cf (insertel cf el (cdr lst)))))) How many times does insertsort evaluate insertel? n times (once for each element) insertsort is 23 February 2004 Worst case? Average case? (n 2) CS 200 Spring 2004 insertel is (n) 15
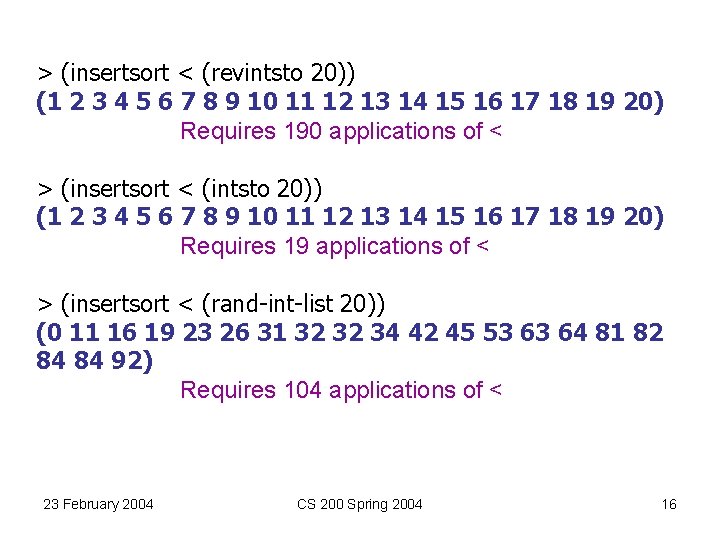
> (insertsort < (revintsto 20)) (1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20) Requires 190 applications of < > (insertsort < (intsto 20)) (1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20) Requires 19 applications of < > (insertsort < (rand-int-list 20)) (0 11 16 19 23 26 31 32 32 34 42 45 53 63 64 81 82 84 84 92) Requires 104 applications of < 23 February 2004 CS 200 Spring 2004 16
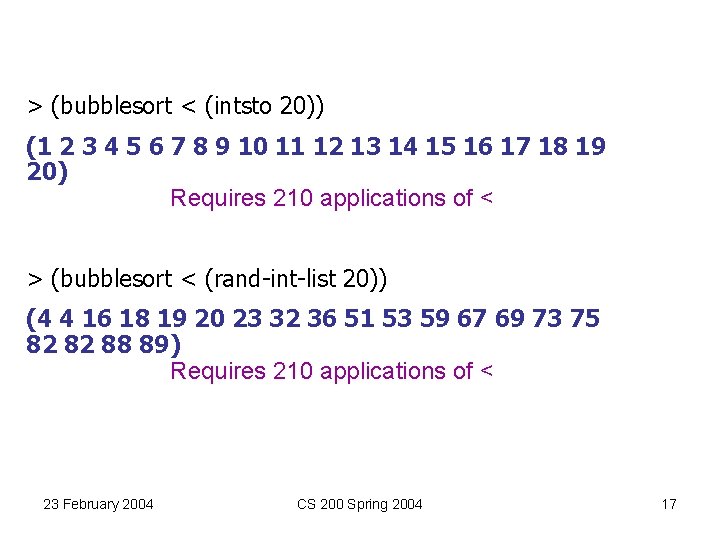
> (bubblesort < (intsto 20)) (1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20) Requires 210 applications of < > (bubblesort < (rand-int-list 20)) (4 4 16 18 19 20 23 32 36 51 53 59 67 69 73 75 82 82 88 89) Requires 210 applications of < 23 February 2004 CS 200 Spring 2004 17
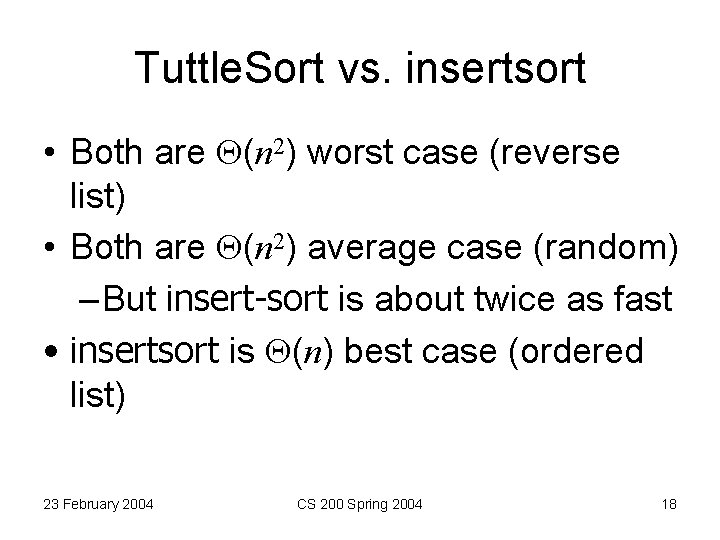
Tuttle. Sort vs. insertsort • Both are (n 2) worst case (reverse list) • Both are (n 2) average case (random) – But insert-sort is about twice as fast • insertsort is (n) best case (ordered list) 23 February 2004 CS 200 Spring 2004 18
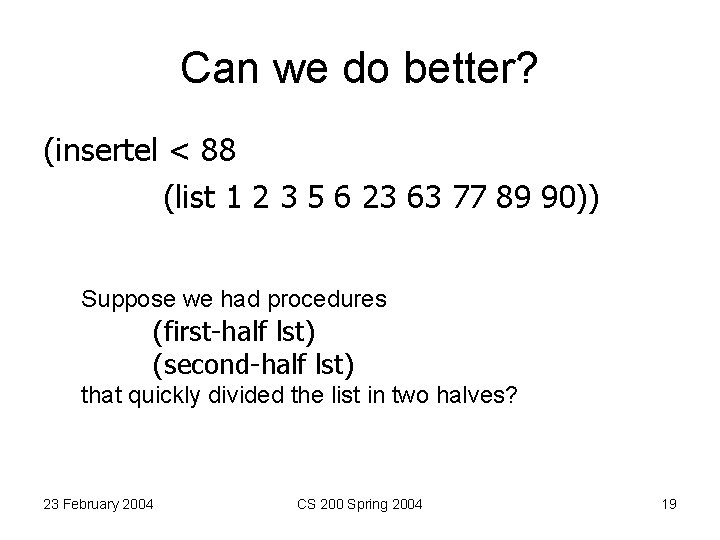
Can we do better? (insertel < 88 (list 1 2 3 5 6 23 63 77 89 90)) Suppose we had procedures (first-half lst) (second-half lst) that quickly divided the list in two halves? 23 February 2004 CS 200 Spring 2004 19
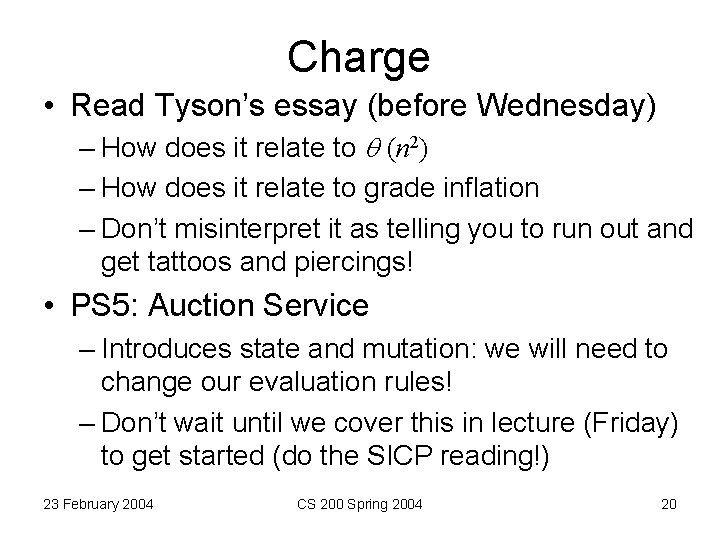
Charge • Read Tyson’s essay (before Wednesday) – How does it relate to (n 2) – How does it relate to grade inflation – Don’t misinterpret it as telling you to run out and get tattoos and piercings! • PS 5: Auction Service – Introduces state and mutation: we will need to change our evaluation rules! – Don’t wait until we cover this in lecture (Friday) to get started (do the SICP reading!) 23 February 2004 CS 200 Spring 2004 20
200+100+300
Internal vs external sorting
Image processing
Adjective comparative superlative fantastic
Quicker sort
01:640:244 lecture notes - lecture 15: plat, idah, farad
Depth sorting algorithm
Hidden surface removal in computer graphics
200+200+100+100
600+800+800
300 + 300 + 200
200 + 200 + 300
100 200 300
200+200+300+300
English is my favourite subject noun
Physical science lecture notes
Computer security 161 cryptocurrency lecture
Computer aided drug design lecture notes
Computer architecture lecture notes
Microarchitecture vs isa
Lego sorting machine instructions