Java Socket Programming Java Sockets Programming q The
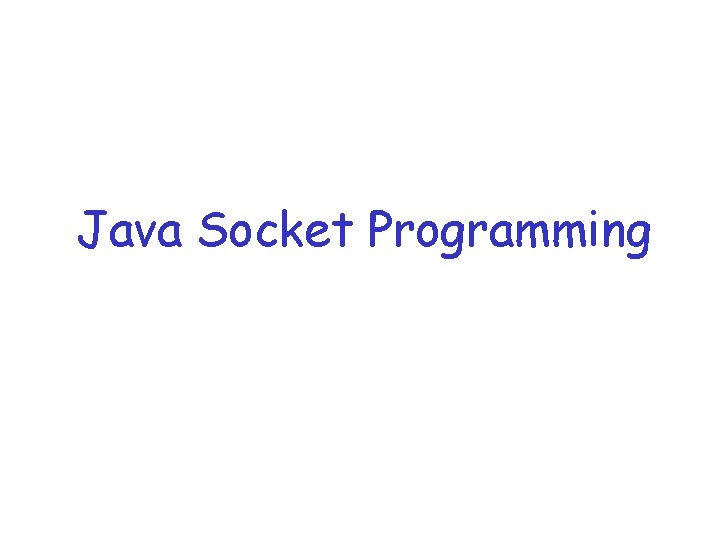
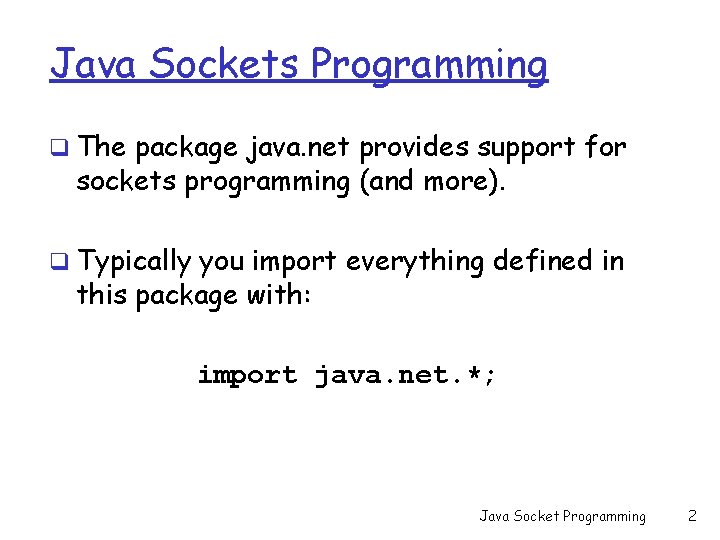
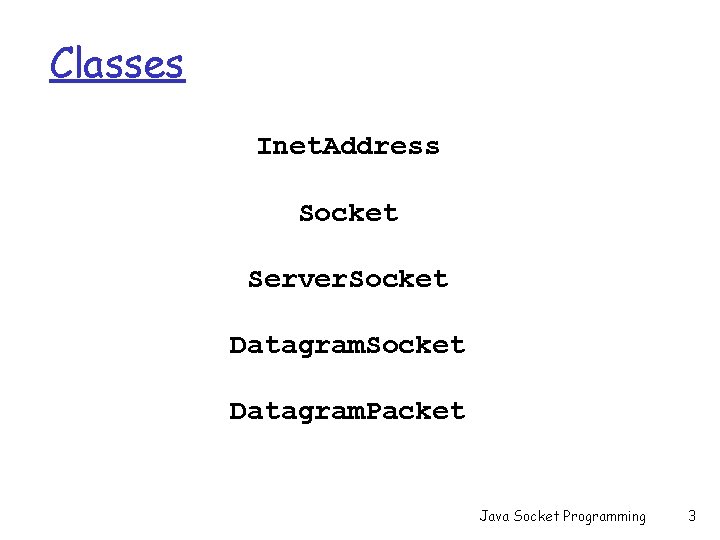
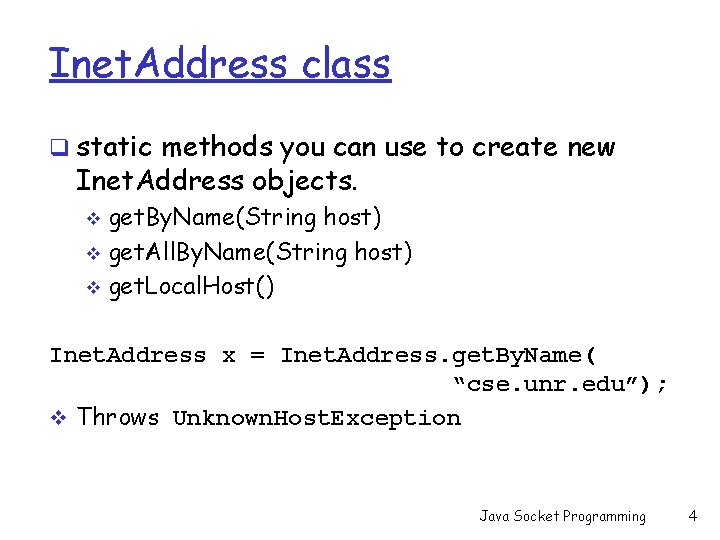
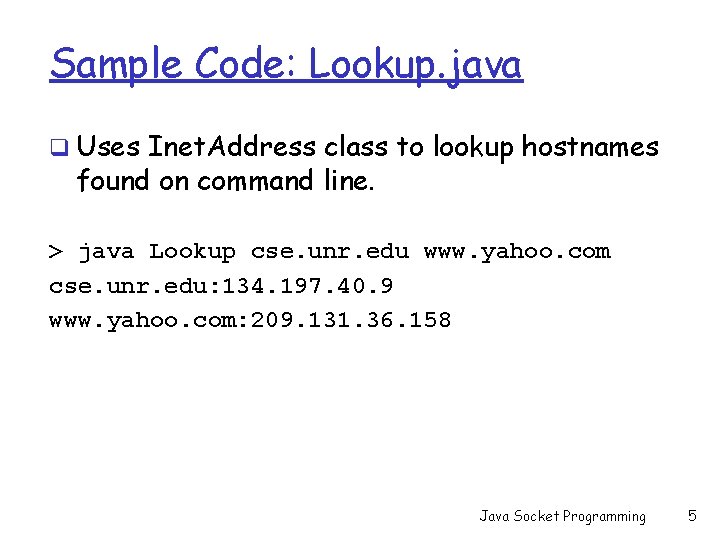
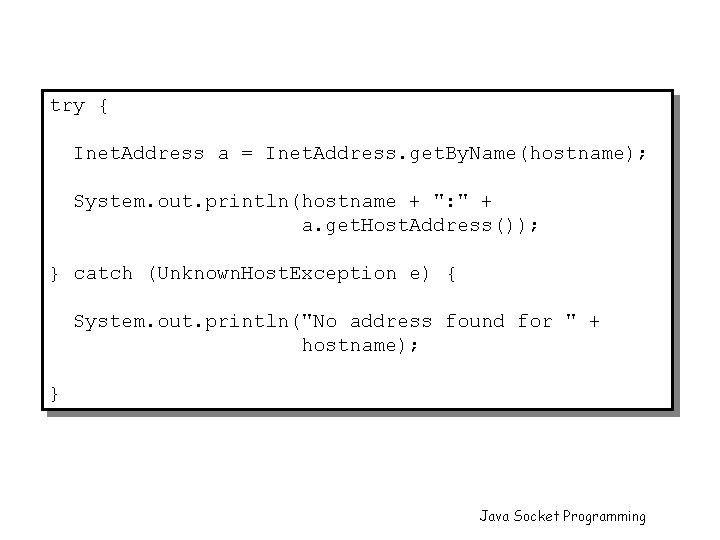
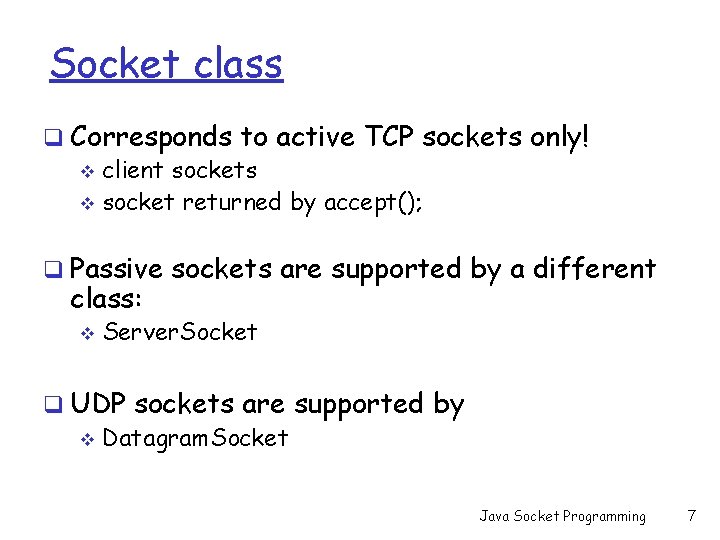
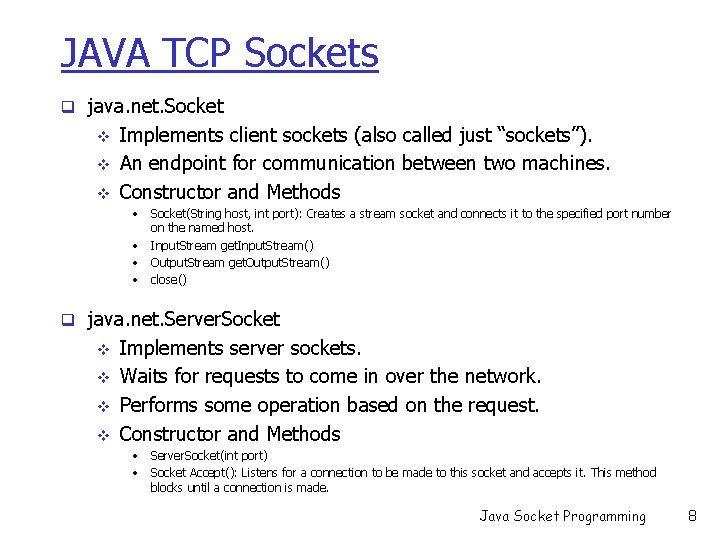
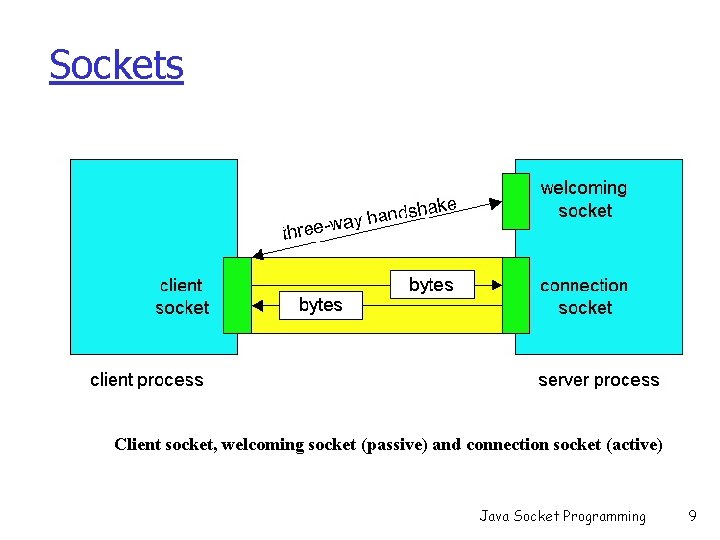
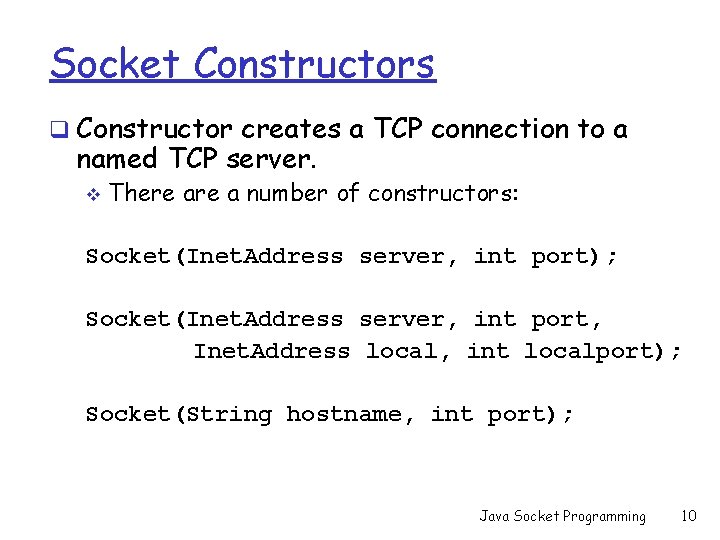
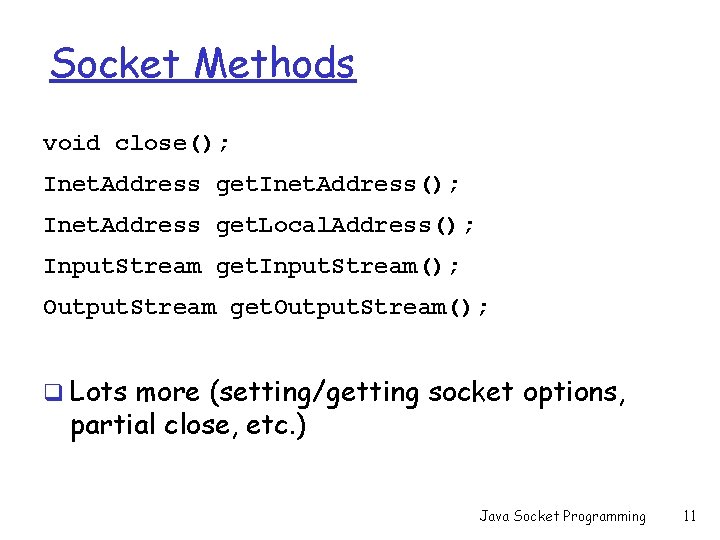
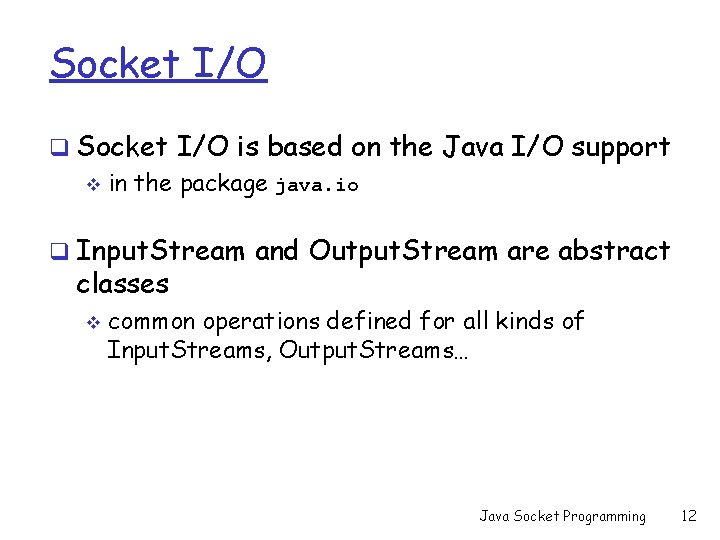
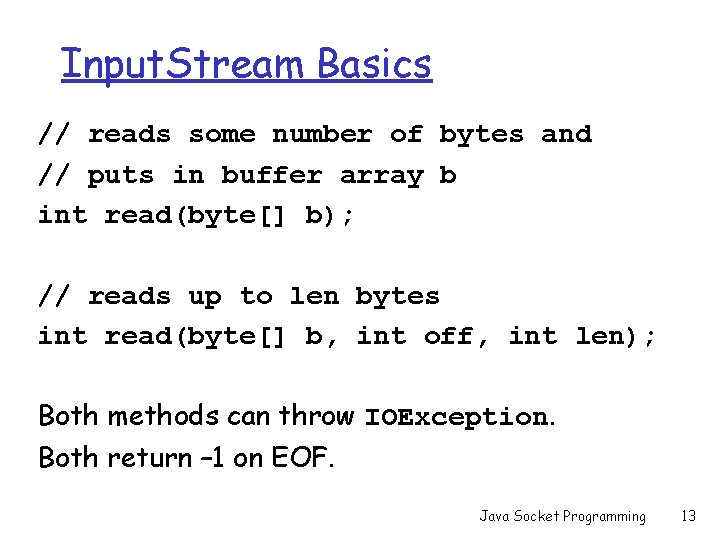
![Output. Stream Basics // writes b. length bytes void write(byte[] b); // writes len Output. Stream Basics // writes b. length bytes void write(byte[] b); // writes len](https://slidetodoc.com/presentation_image_h2/78d1503d20759a59fd7bf7b6bcb70766/image-14.jpg)
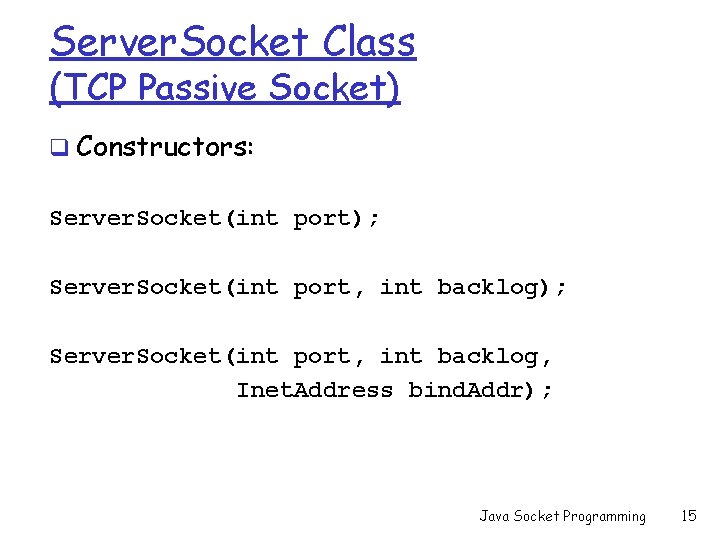
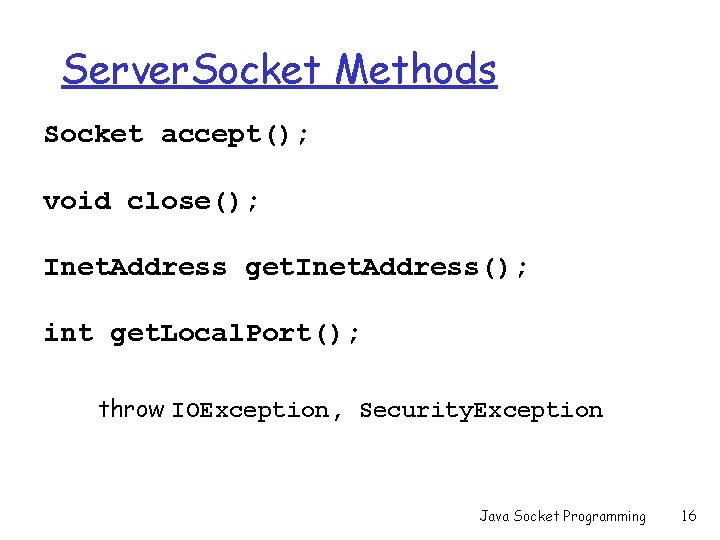
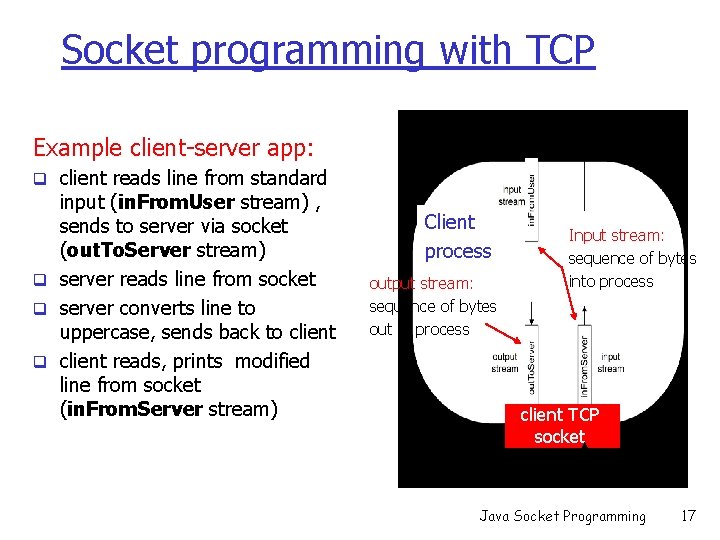
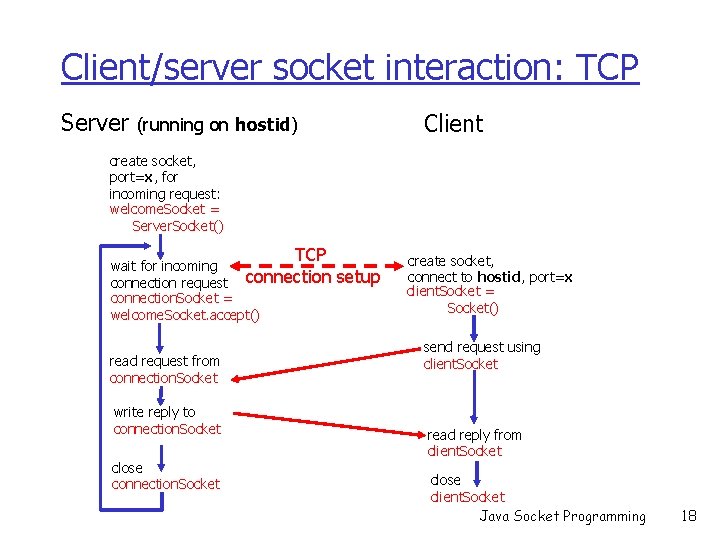
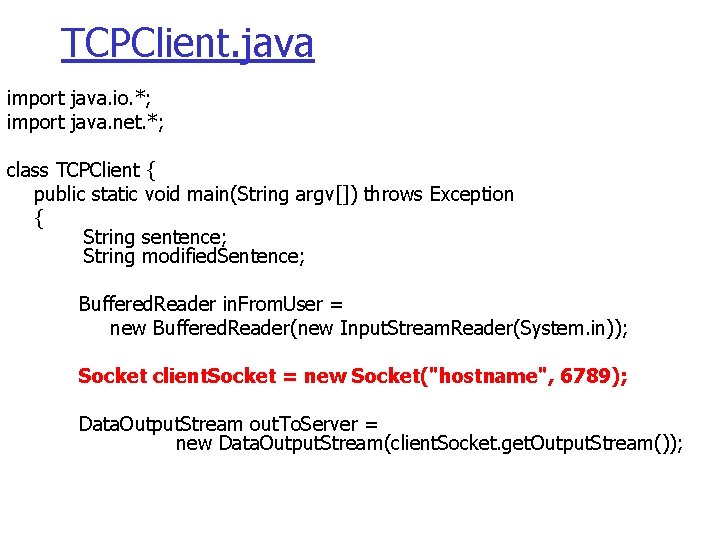
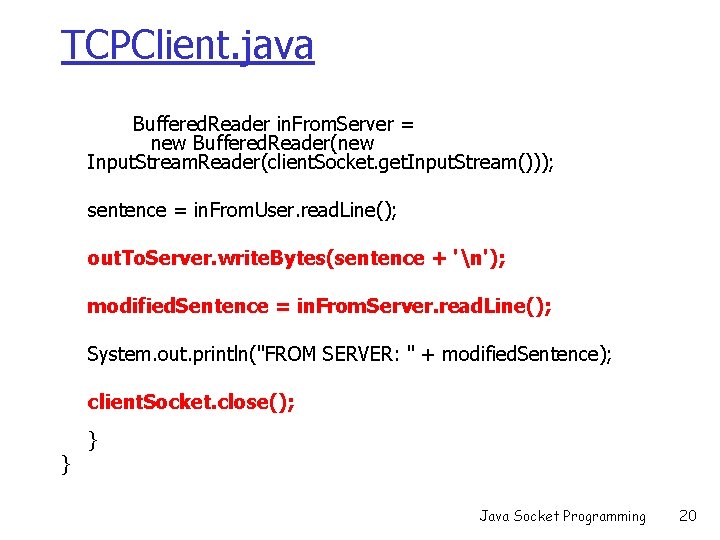
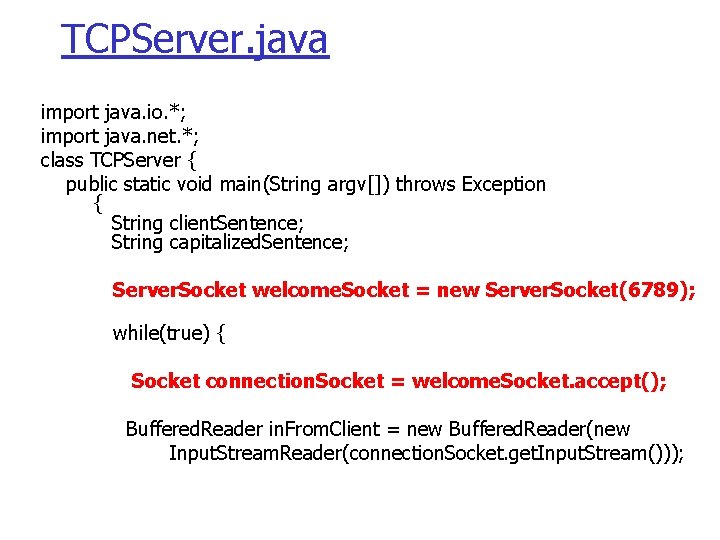
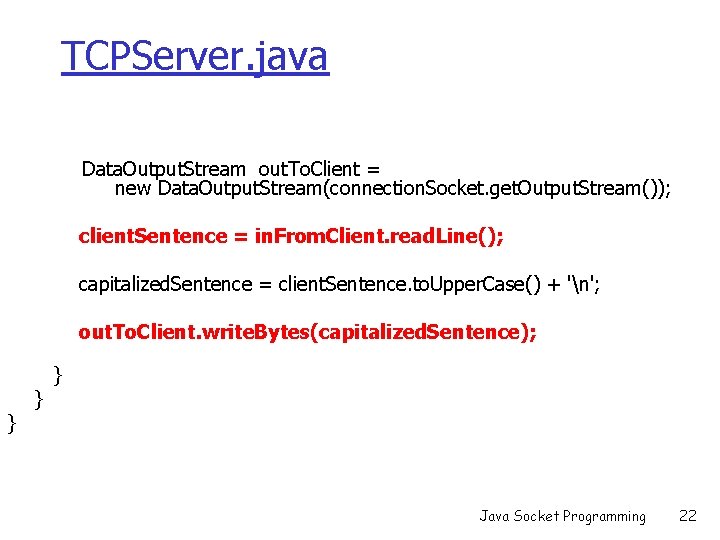
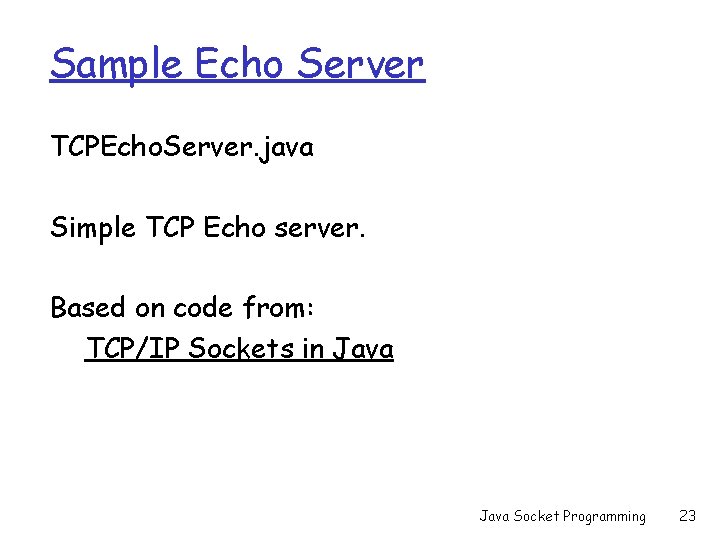
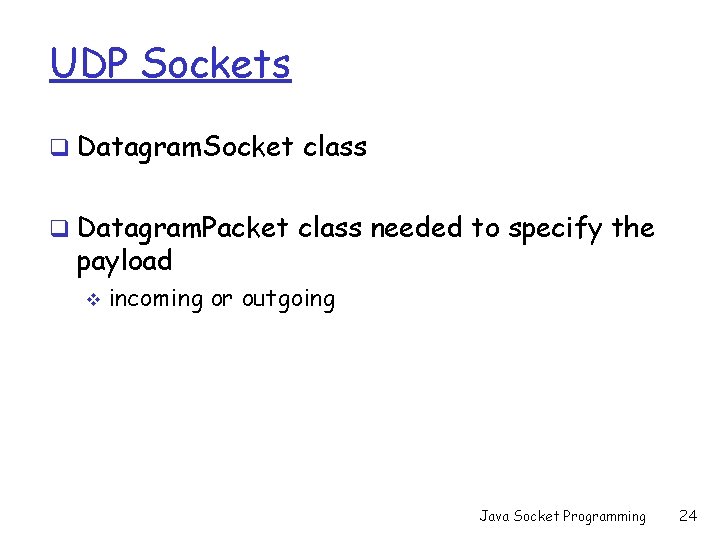
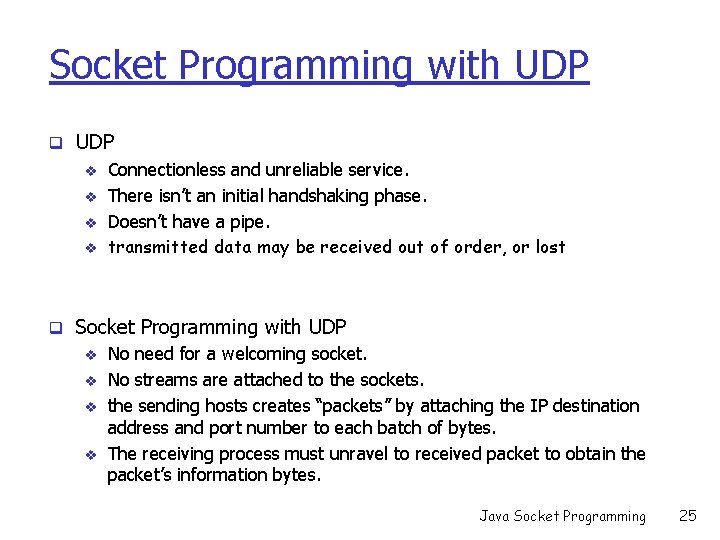
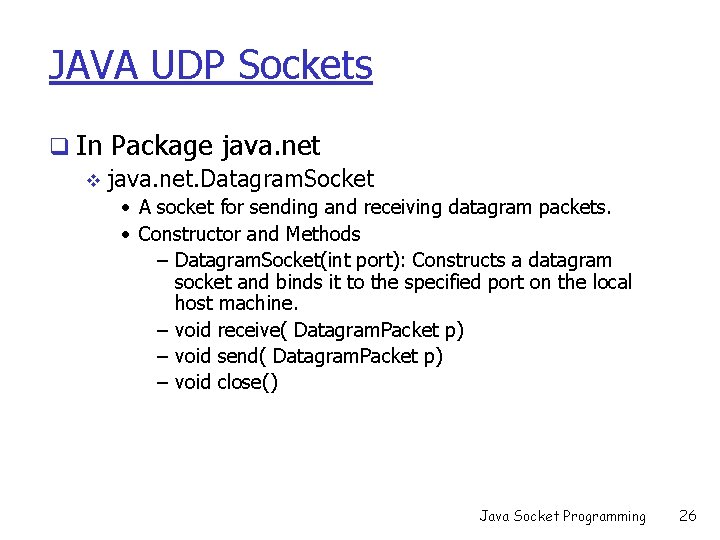
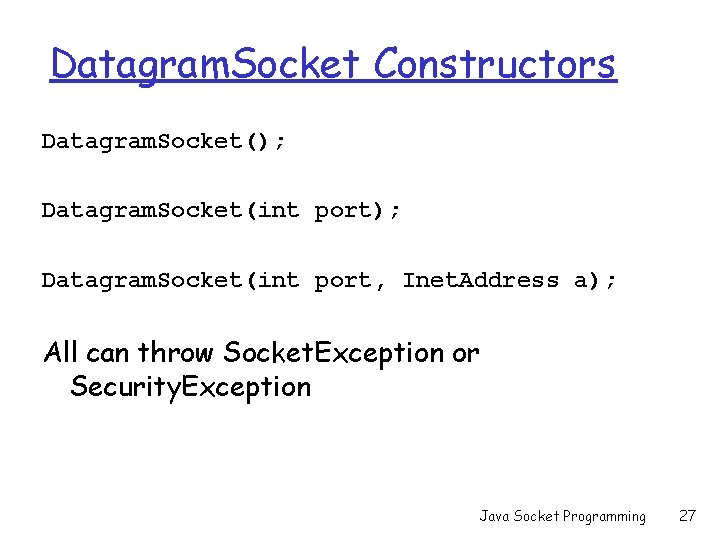
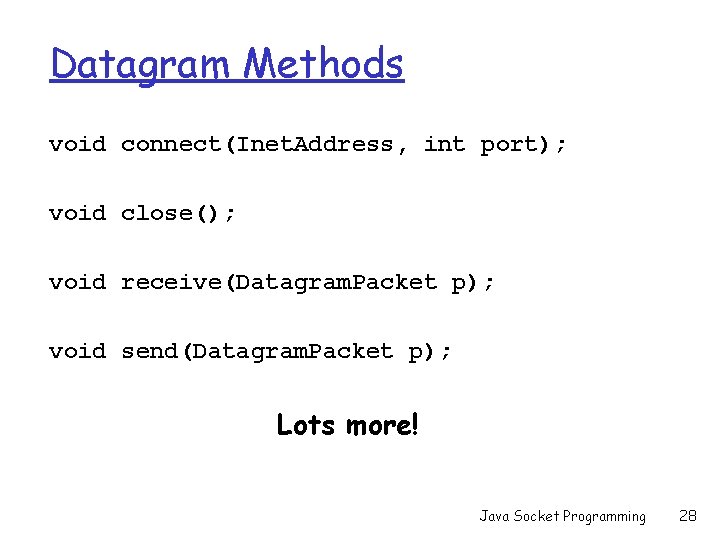
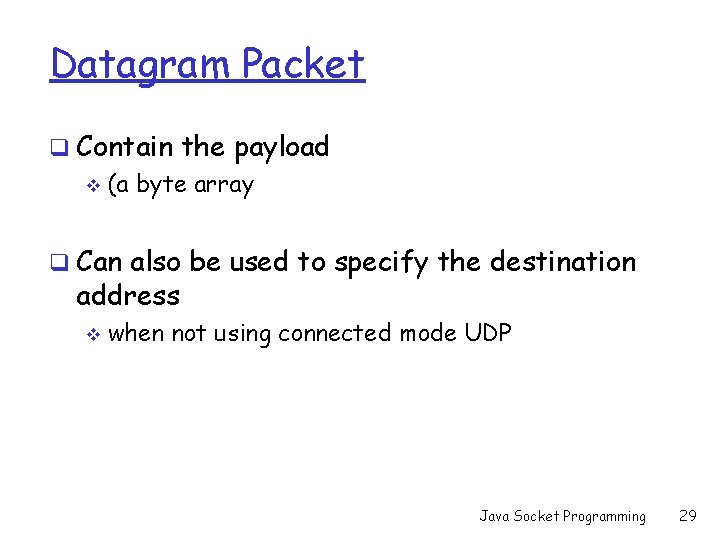
![Datagram. Packet Constructors For receiving: Datagram. Packet( byte[] buf, int len); For sending: Datagram. Datagram. Packet Constructors For receiving: Datagram. Packet( byte[] buf, int len); For sending: Datagram.](https://slidetodoc.com/presentation_image_h2/78d1503d20759a59fd7bf7b6bcb70766/image-30.jpg)
![Datagram. Packet methods byte[] get. Data(); void set. Data(byte[] buf); void set. Address(Inet. Address Datagram. Packet methods byte[] get. Data(); void set. Data(byte[] buf); void set. Address(Inet. Address](https://slidetodoc.com/presentation_image_h2/78d1503d20759a59fd7bf7b6bcb70766/image-31.jpg)
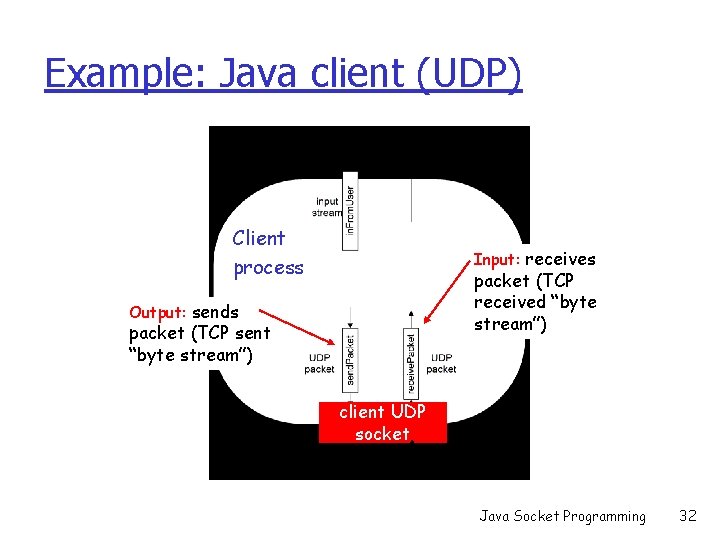
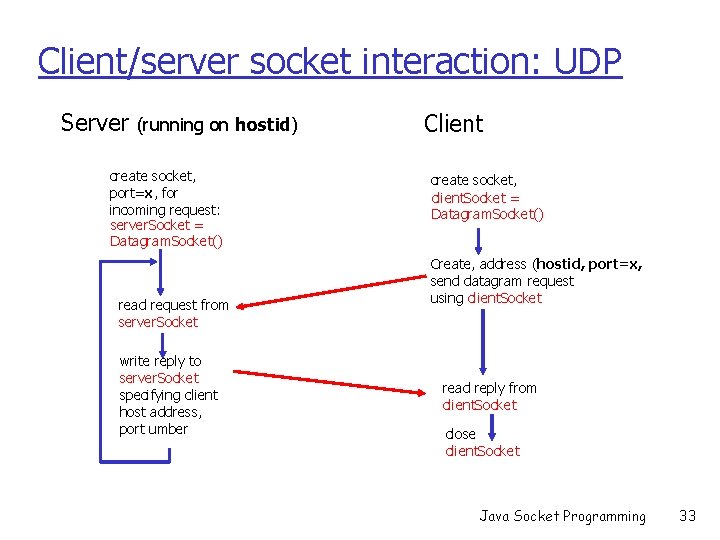
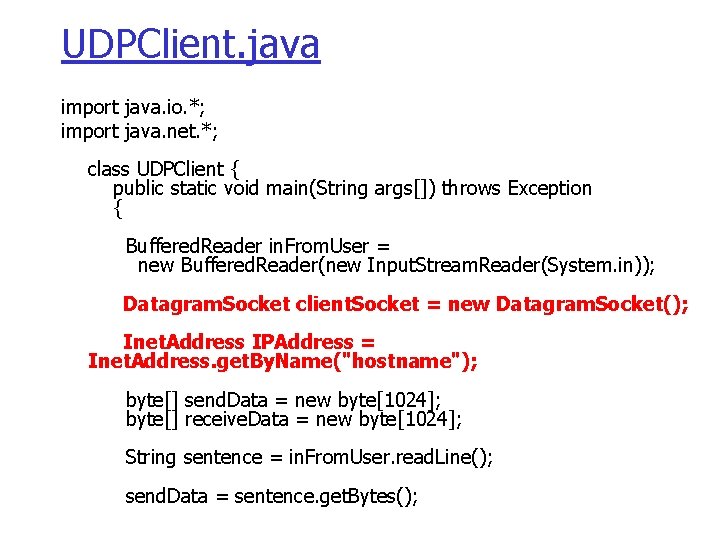
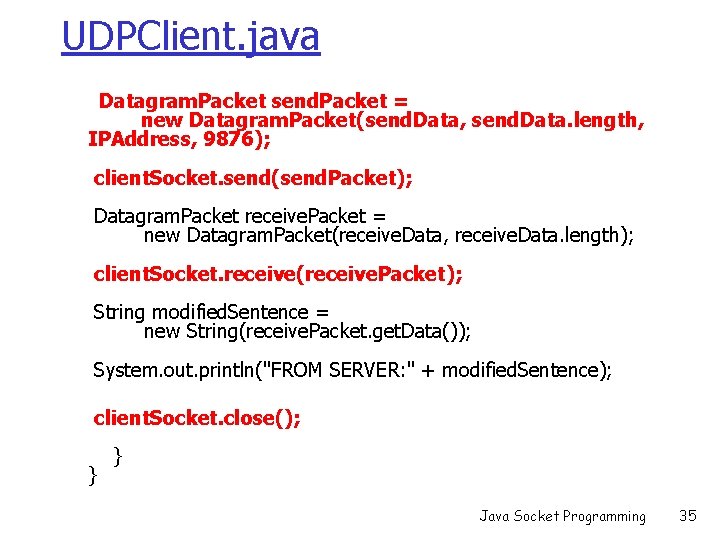
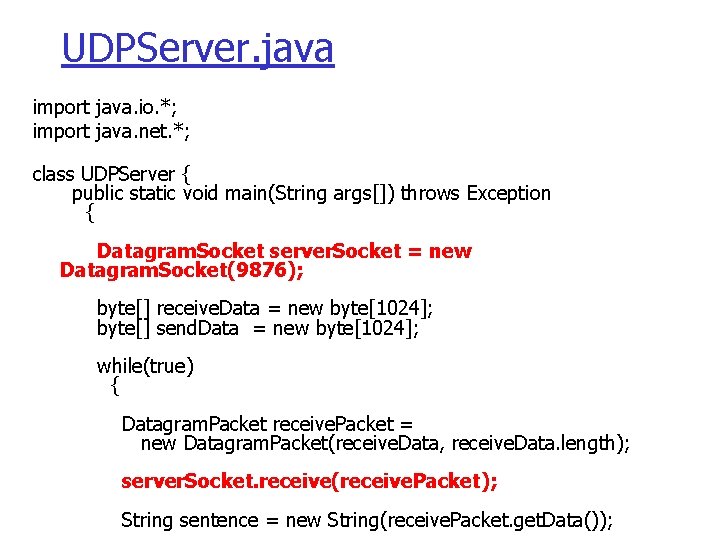
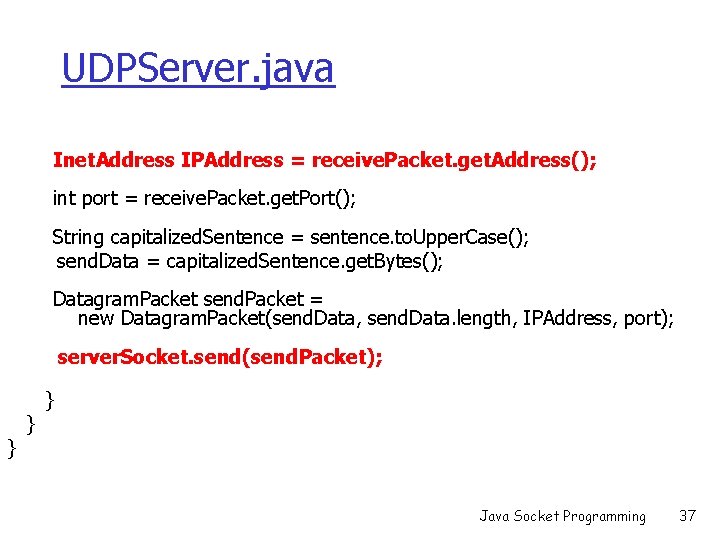
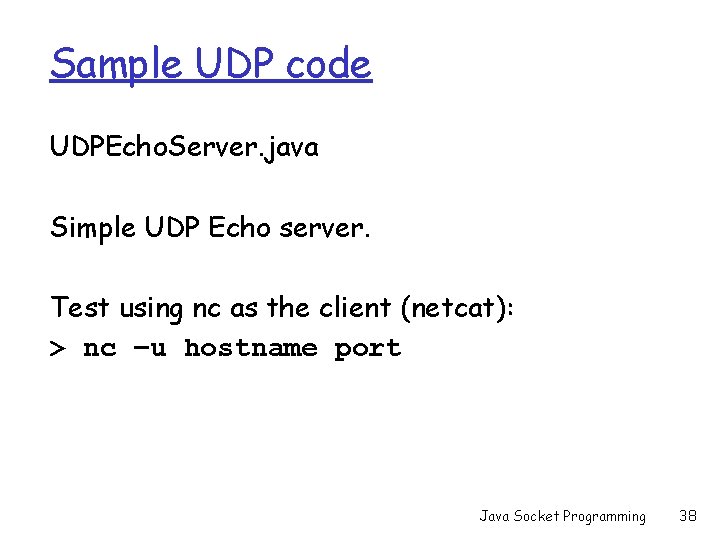
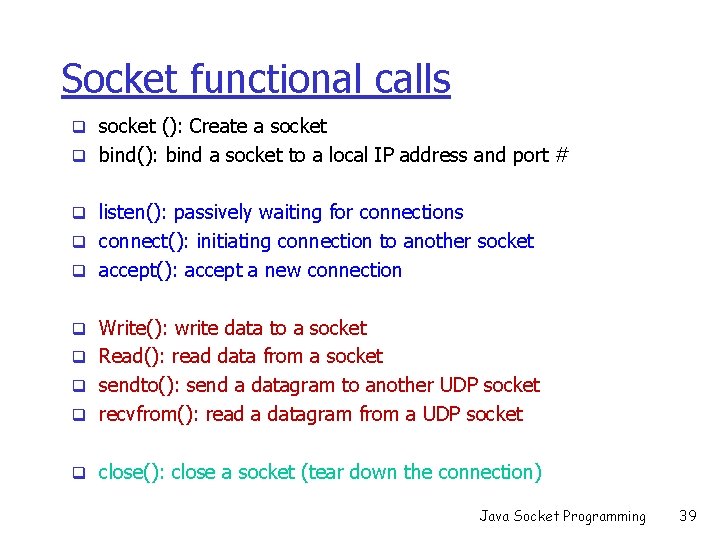
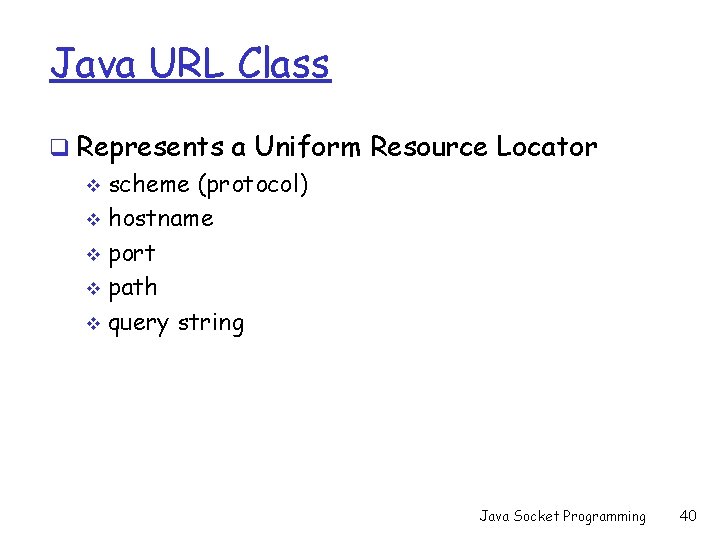
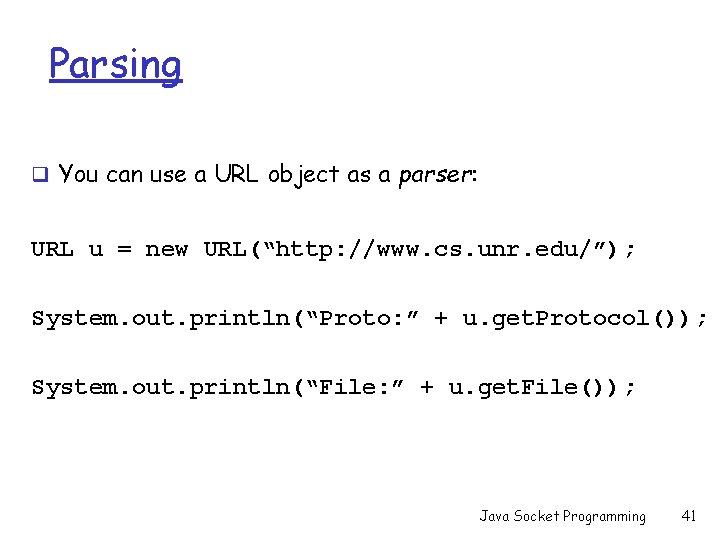
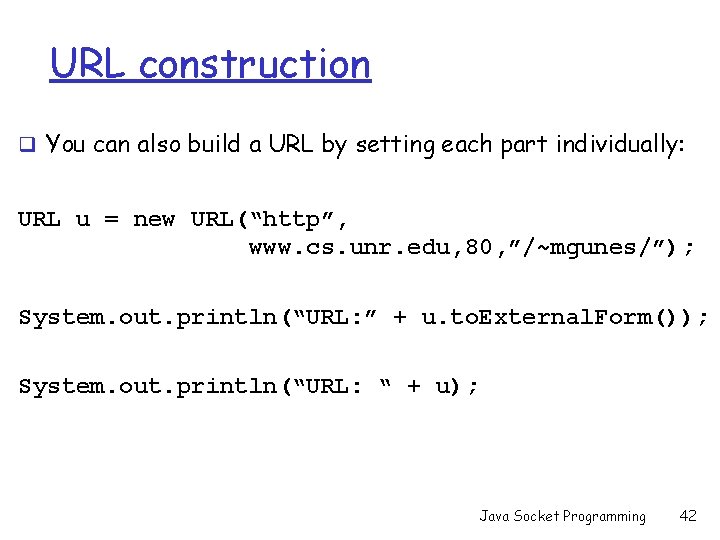
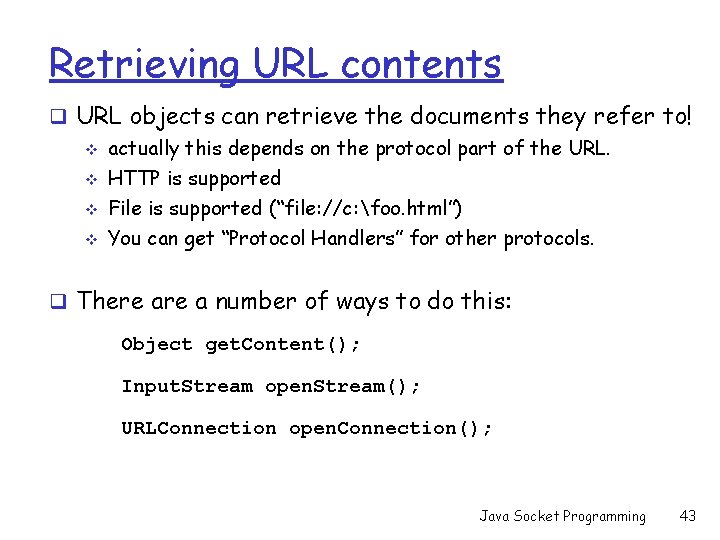
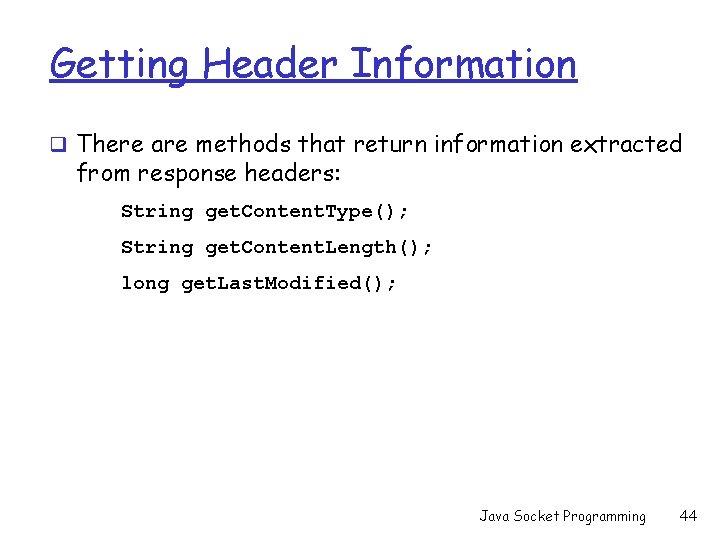
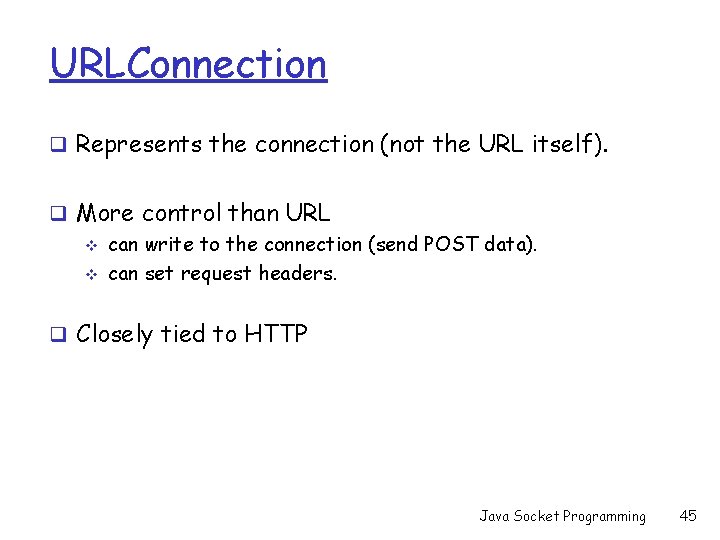
- Slides: 45
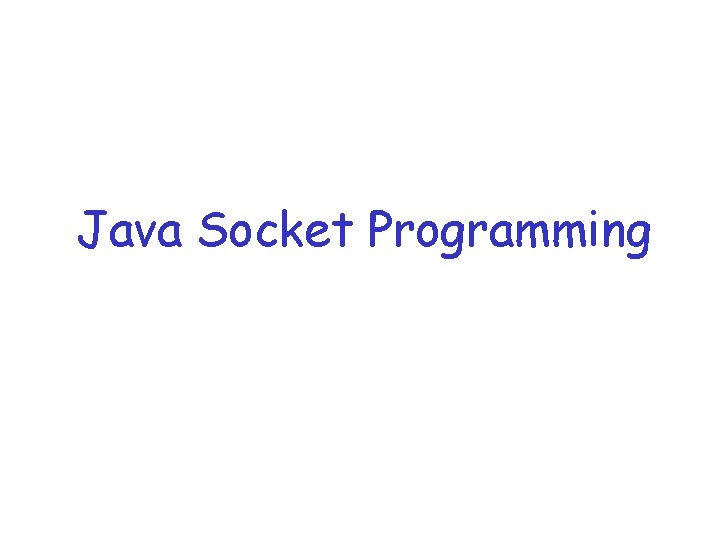
Java Socket Programming
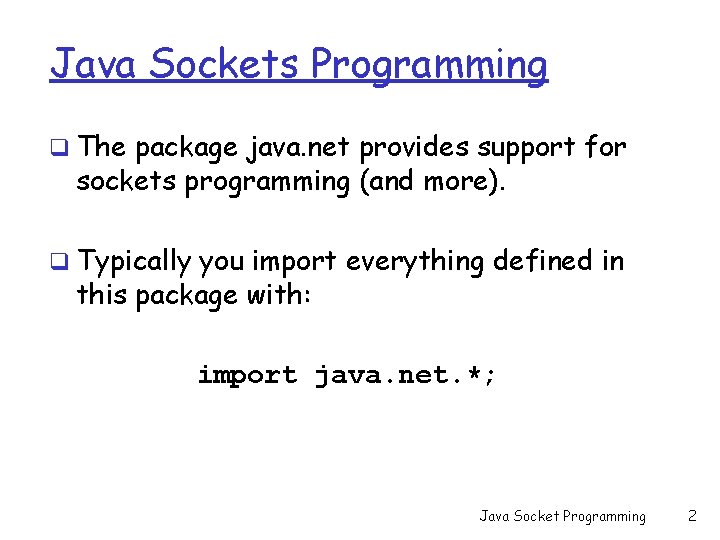
Java Sockets Programming q The package java. net provides support for sockets programming (and more). q Typically you import everything defined in this package with: import java. net. *; Java Socket Programming 2
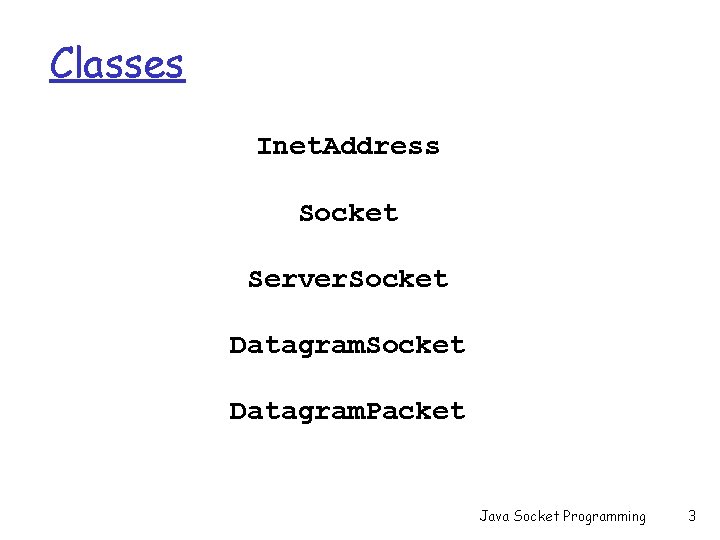
Classes Inet. Address Socket Server. Socket Datagram. Packet Java Socket Programming 3
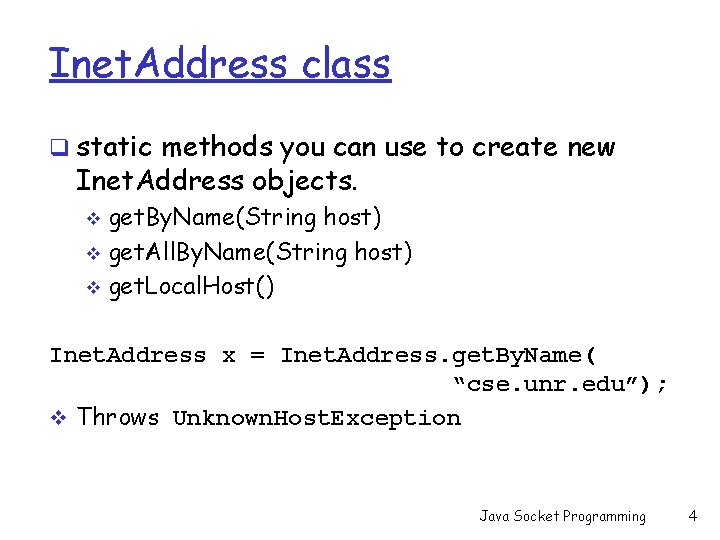
Inet. Address class q static methods you can use to create new Inet. Address objects. get. By. Name(String host) v get. All. By. Name(String host) v get. Local. Host() v Inet. Address x = Inet. Address. get. By. Name( “cse. unr. edu”); v Throws Unknown. Host. Exception Java Socket Programming 4
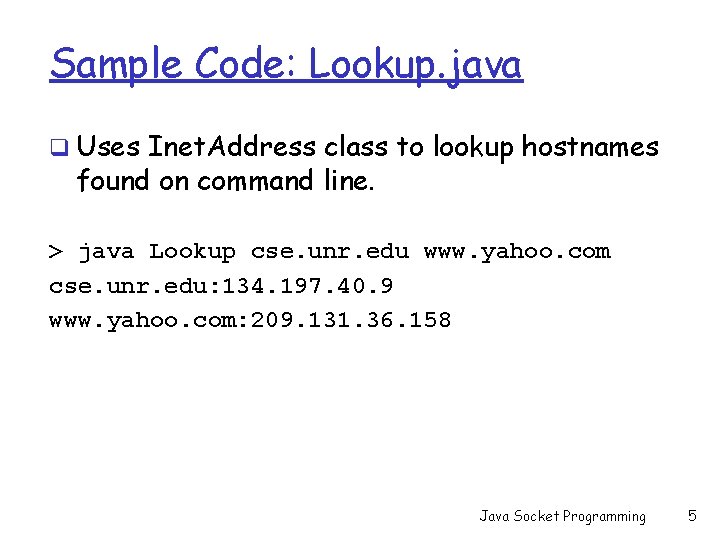
Sample Code: Lookup. java q Uses Inet. Address class to lookup hostnames found on command line. > java Lookup cse. unr. edu www. yahoo. com cse. unr. edu: 134. 197. 40. 9 www. yahoo. com: 209. 131. 36. 158 Java Socket Programming 5
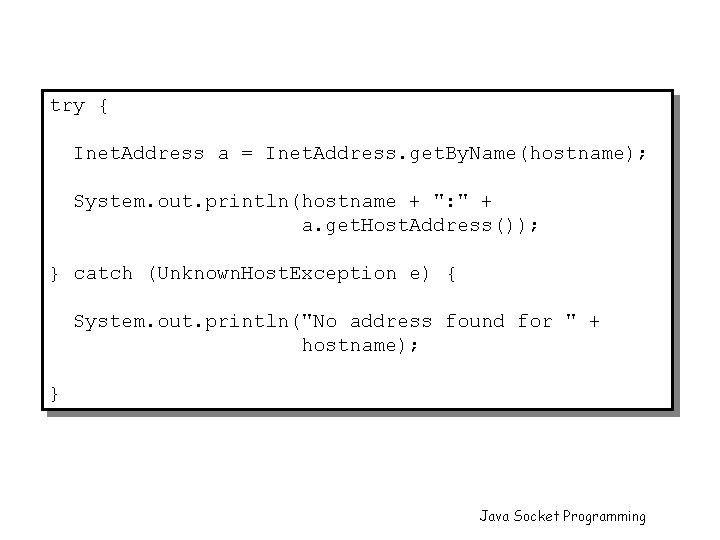
try { Inet. Address a = Inet. Address. get. By. Name(hostname); System. out. println(hostname + ": " + a. get. Host. Address()); } catch (Unknown. Host. Exception e) { System. out. println("No address found for " + hostname); } Java Socket Programming
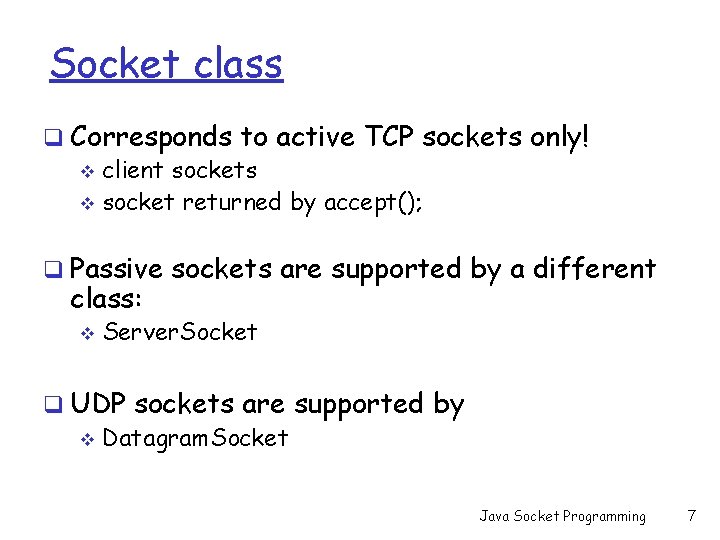
Socket class q Corresponds to active TCP sockets only! v client sockets v socket returned by accept(); q Passive sockets are supported by a different class: v Server. Socket q UDP sockets are supported by v Datagram. Socket Java Socket Programming 7
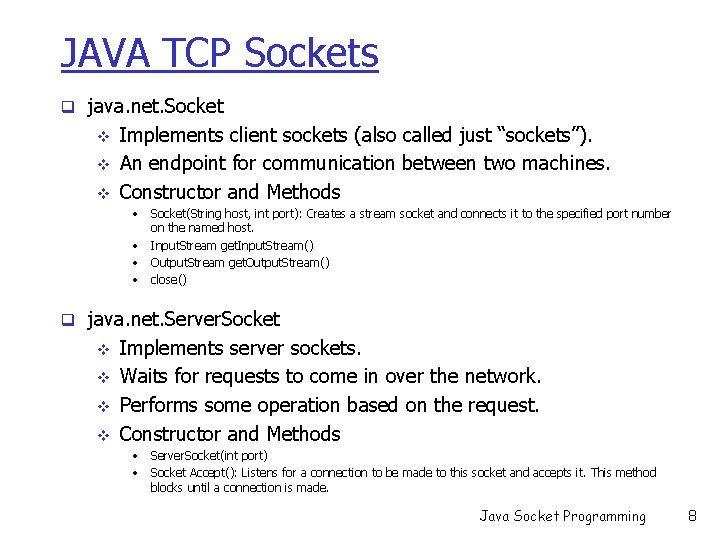
JAVA TCP Sockets q java. net. Socket v v v Implements client sockets (also called just “sockets”). An endpoint for communication between two machines. Constructor and Methods • Socket(String host, int port): Creates a stream socket and connects it to the specified port number on the named host. • Input. Stream get. Input. Stream() • Output. Stream get. Output. Stream() • close() q java. net. Server. Socket v v Implements server sockets. Waits for requests to come in over the network. Performs some operation based on the request. Constructor and Methods • Server. Socket(int port) • Socket Accept(): Listens for a connection to be made to this socket and accepts it. This method blocks until a connection is made. Java Socket Programming 8
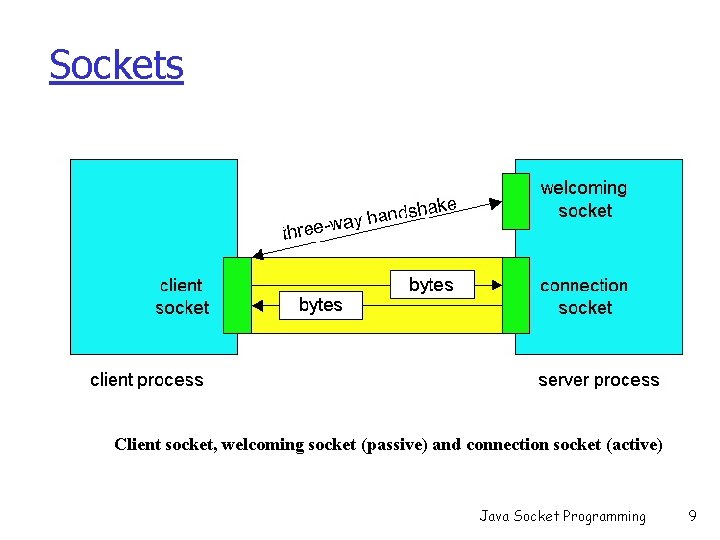
Sockets Client socket, welcoming socket (passive) and connection socket (active) Java Socket Programming 9
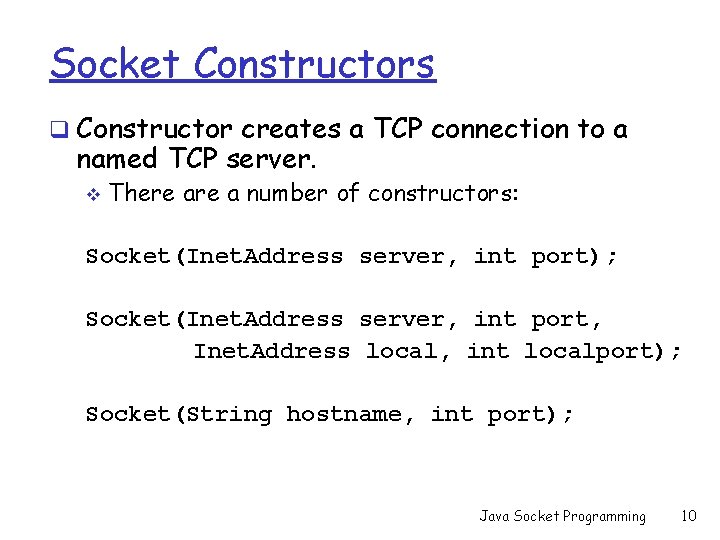
Socket Constructors q Constructor creates a TCP connection to a named TCP server. v There a number of constructors: Socket(Inet. Address server, int port); Socket(Inet. Address server, int port, Inet. Address local, int localport); Socket(String hostname, int port); Java Socket Programming 10
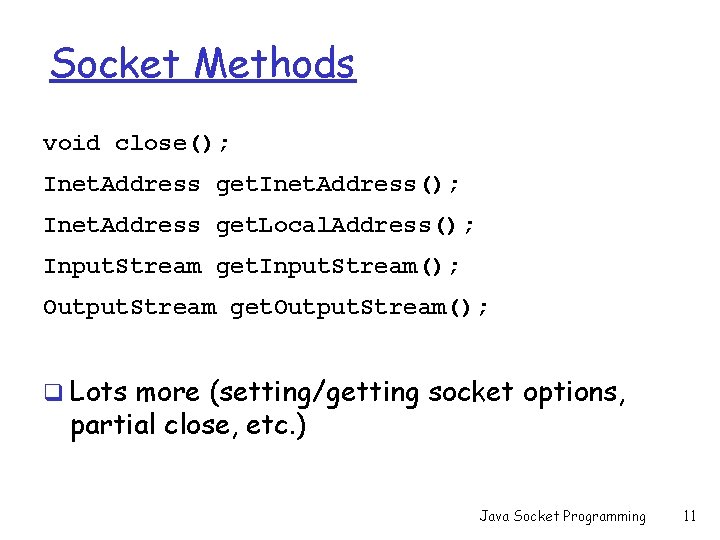
Socket Methods void close(); Inet. Address get. Inet. Address(); Inet. Address get. Local. Address(); Input. Stream get. Input. Stream(); Output. Stream get. Output. Stream(); q Lots more (setting/getting socket options, partial close, etc. ) Java Socket Programming 11
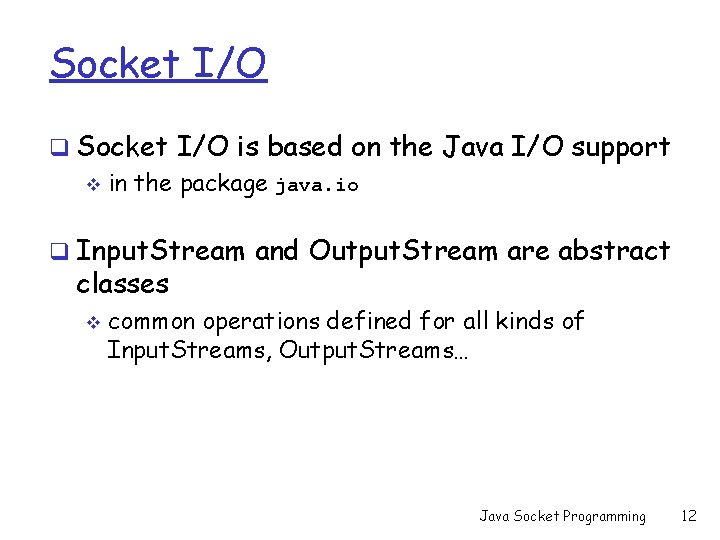
Socket I/O q Socket I/O is based on the Java I/O support v in the package java. io q Input. Stream and Output. Stream are abstract classes v common operations defined for all kinds of Input. Streams, Output. Streams… Java Socket Programming 12
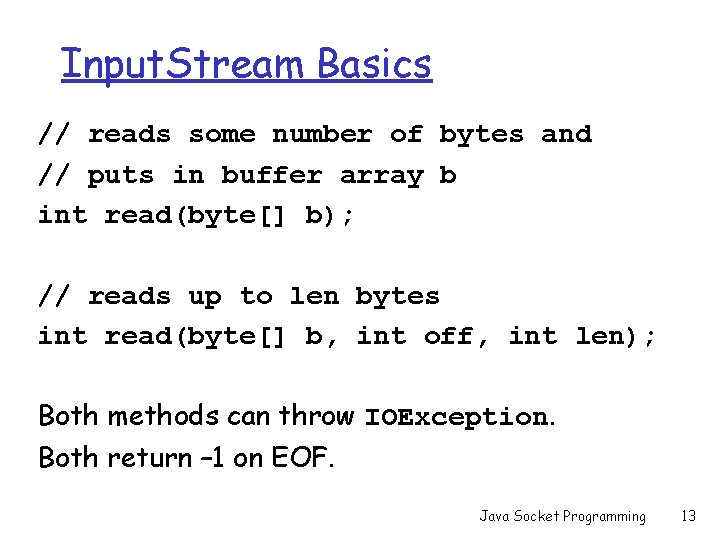
Input. Stream Basics // reads some number of bytes and // puts in buffer array b int read(byte[] b); // reads up to len bytes int read(byte[] b, int off, int len); Both methods can throw IOException. Both return – 1 on EOF. Java Socket Programming 13
![Output Stream Basics writes b length bytes void writebyte b writes len Output. Stream Basics // writes b. length bytes void write(byte[] b); // writes len](https://slidetodoc.com/presentation_image_h2/78d1503d20759a59fd7bf7b6bcb70766/image-14.jpg)
Output. Stream Basics // writes b. length bytes void write(byte[] b); // writes len bytes starting // at offset off void write(byte[] b, int off, int len); Both methods can throw IOException. Java Socket Programming 14
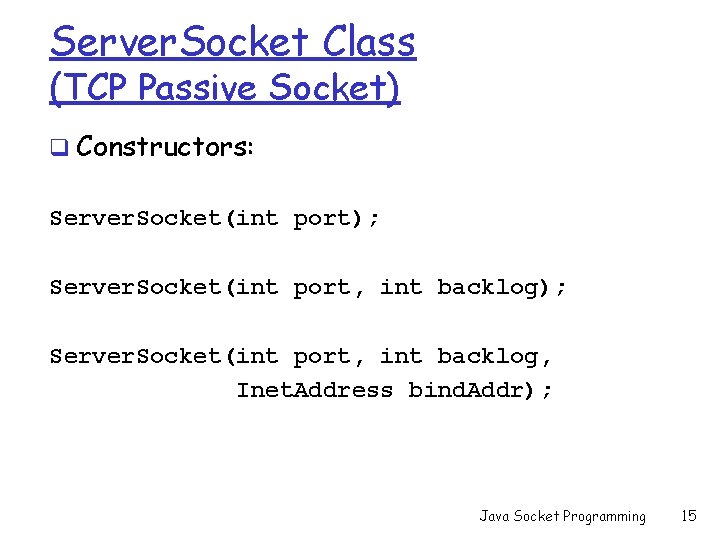
Server. Socket Class (TCP Passive Socket) q Constructors: Server. Socket(int port); Server. Socket(int port, int backlog, Inet. Address bind. Addr); Java Socket Programming 15
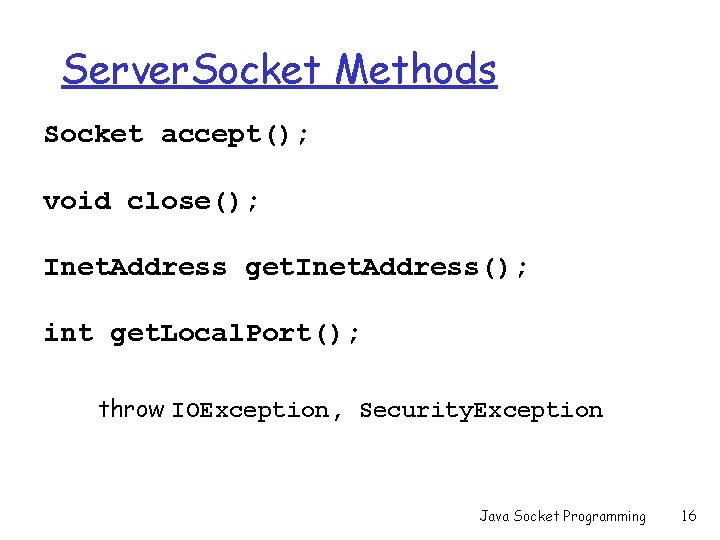
Server. Socket Methods Socket accept(); void close(); Inet. Address get. Inet. Address(); int get. Local. Port(); throw IOException, Security. Exception Java Socket Programming 16
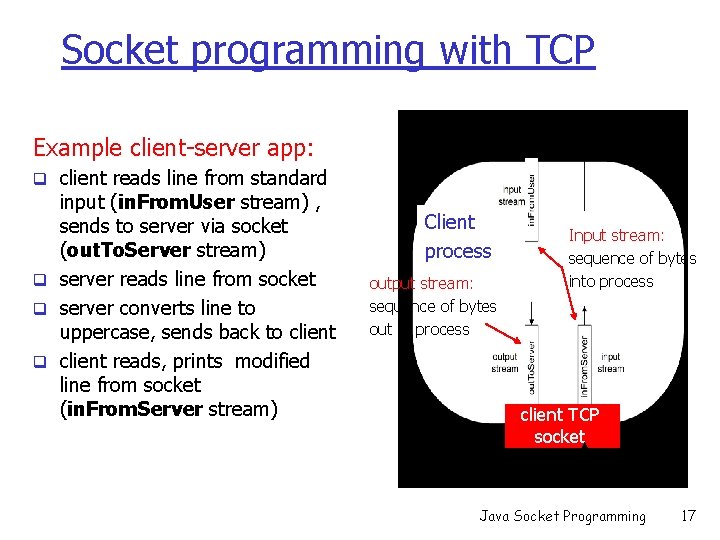
Socket programming with TCP Example client-server app: q client reads line from standard input (in. From. User stream) , sends to server via socket (out. To. Server stream) q server reads line from socket q server converts line to uppercase, sends back to client q client reads, prints modified line from socket (in. From. Server stream) Client process output stream: sequence of bytes out of process Input stream: sequence of bytes into process client TCP socket Java Socket Programming 17
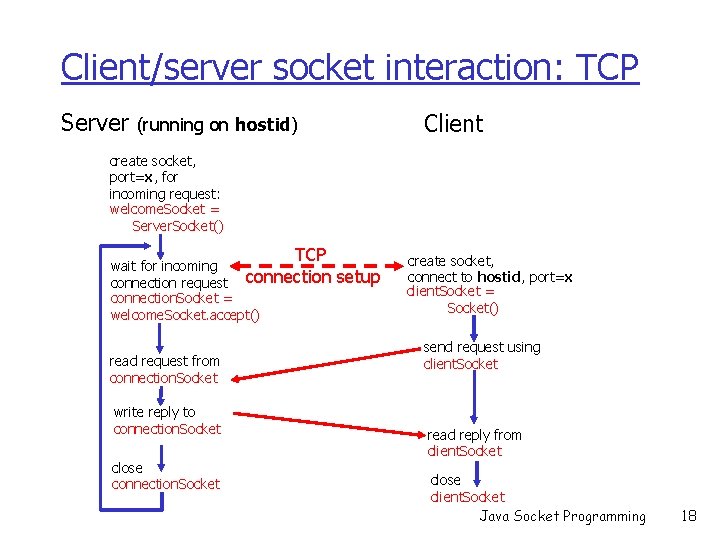
Client/server socket interaction: TCP Server Client (running on hostid) create socket, port=x, for incoming request: welcome. Socket = Server. Socket() TCP wait for incoming connection request connection. Socket = welcome. Socket. accept() read request from connection. Socket write reply to connection. Socket close connection. Socket setup create socket, connect to hostid, port=x client. Socket = Socket() send request using client. Socket read reply from client. Socket close client. Socket Java Socket Programming 18
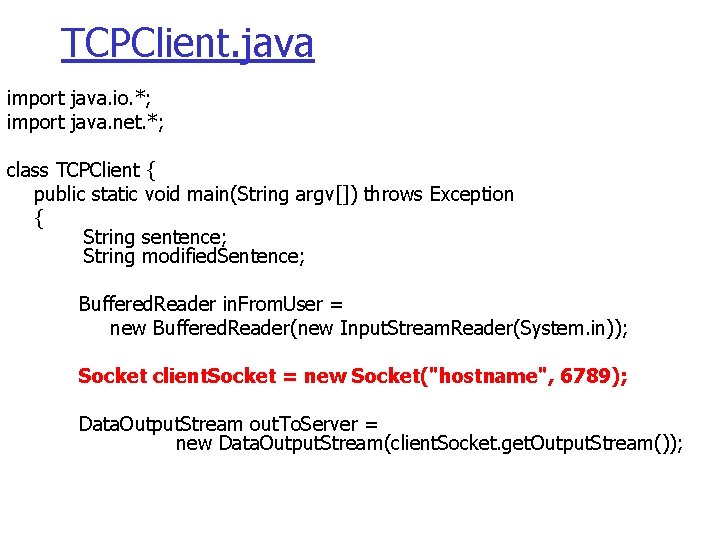
TCPClient. java import java. io. *; import java. net. *; class TCPClient { public static void main(String argv[]) throws Exception { String sentence; String modified. Sentence; Buffered. Reader in. From. User = new Buffered. Reader(new Input. Stream. Reader(System. in)); Socket client. Socket = new Socket("hostname", 6789); Data. Output. Stream out. To. Server = new Data. Output. Stream(client. Socket. get. Output. Stream());
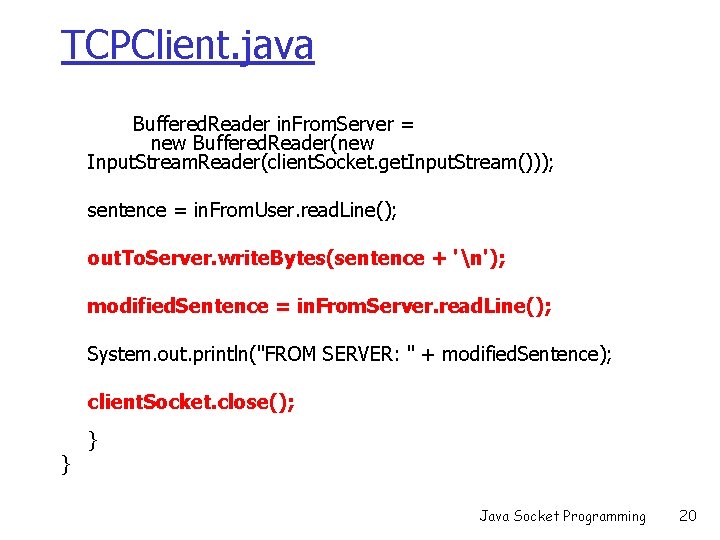
TCPClient. java Buffered. Reader in. From. Server = new Buffered. Reader(new Input. Stream. Reader(client. Socket. get. Input. Stream())); sentence = in. From. User. read. Line(); out. To. Server. write. Bytes(sentence + 'n'); modified. Sentence = in. From. Server. read. Line(); System. out. println("FROM SERVER: " + modified. Sentence); client. Socket. close(); } } Java Socket Programming 20
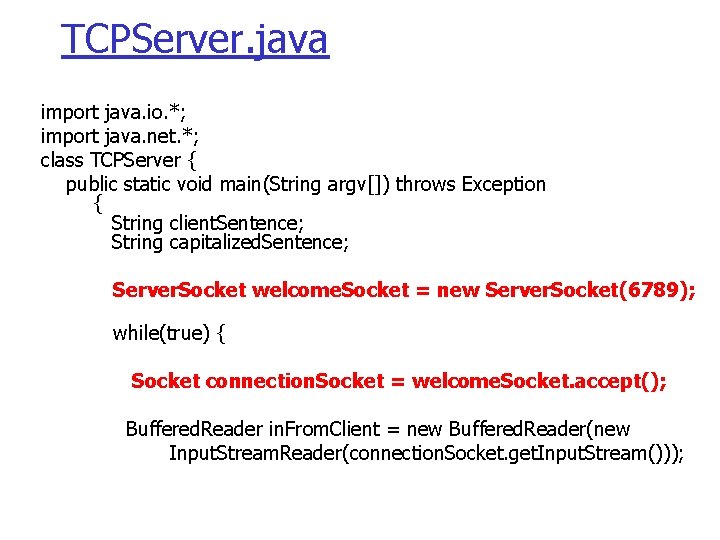
TCPServer. java import java. io. *; import java. net. *; class TCPServer { public static void main(String argv[]) throws Exception { String client. Sentence; String capitalized. Sentence; Server. Socket welcome. Socket = new Server. Socket(6789); while(true) { Socket connection. Socket = welcome. Socket. accept(); Buffered. Reader in. From. Client = new Buffered. Reader(new Input. Stream. Reader(connection. Socket. get. Input. Stream()));
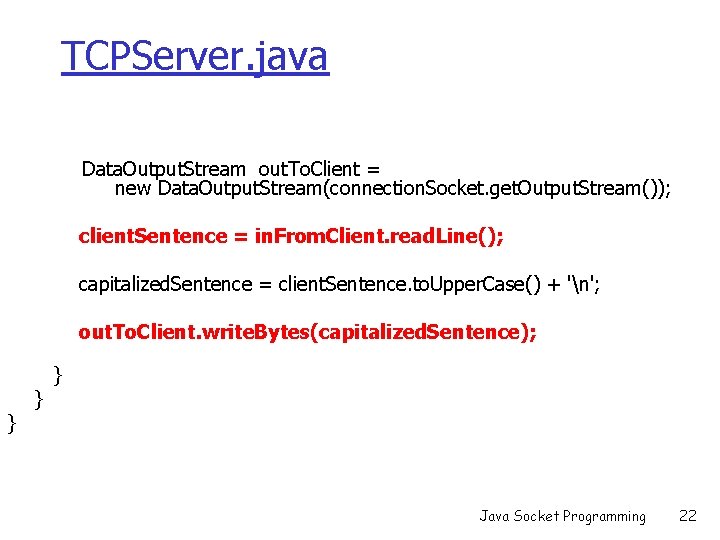
TCPServer. java Data. Output. Stream out. To. Client = new Data. Output. Stream(connection. Socket. get. Output. Stream()); client. Sentence = in. From. Client. read. Line(); capitalized. Sentence = client. Sentence. to. Upper. Case() + 'n'; out. To. Client. write. Bytes(capitalized. Sentence); } } } Java Socket Programming 22
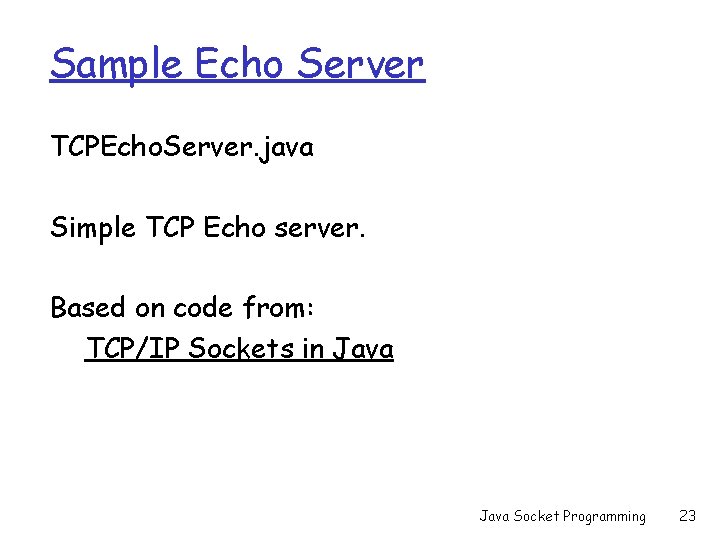
Sample Echo Server TCPEcho. Server. java Simple TCP Echo server. Based on code from: TCP/IP Sockets in Java Socket Programming 23
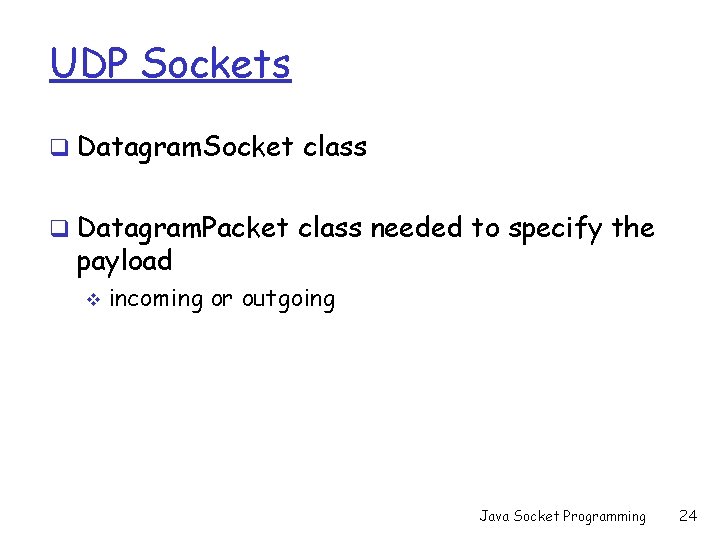
UDP Sockets q Datagram. Socket class q Datagram. Packet class needed to specify the payload v incoming or outgoing Java Socket Programming 24
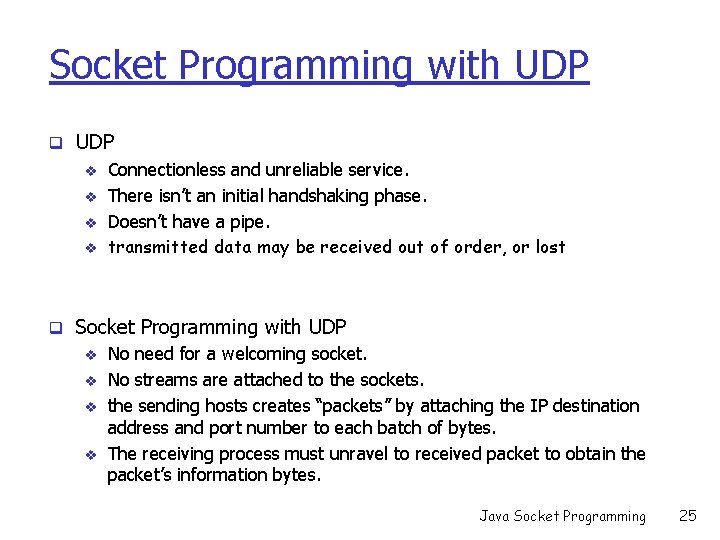
Socket Programming with UDP q UDP v v Connectionless and unreliable service. There isn’t an initial handshaking phase. Doesn’t have a pipe. transmitted data may be received out of order, or lost q Socket Programming with UDP v v No need for a welcoming socket. No streams are attached to the sockets. the sending hosts creates “packets” by attaching the IP destination address and port number to each batch of bytes. The receiving process must unravel to received packet to obtain the packet’s information bytes. Java Socket Programming 25
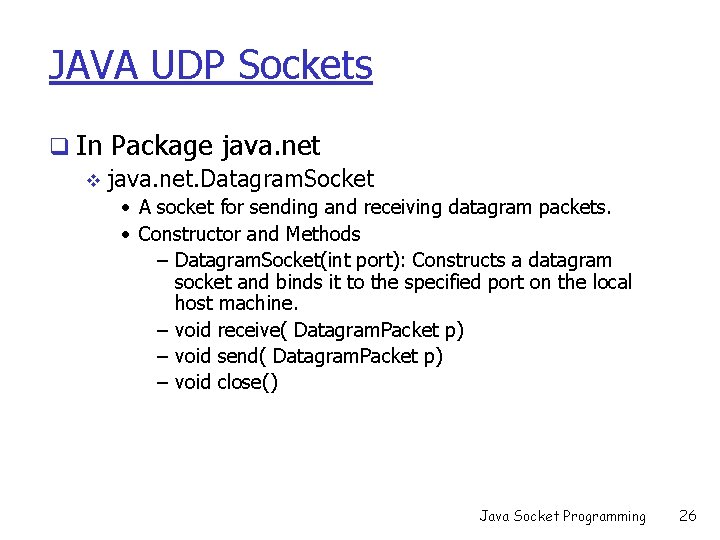
JAVA UDP Sockets q In Package java. net v java. net. Datagram. Socket • A socket for sending and receiving datagram packets. • Constructor and Methods – Datagram. Socket(int port): Constructs a datagram socket and binds it to the specified port on the local host machine. – void receive( Datagram. Packet p) – void send( Datagram. Packet p) – void close() Java Socket Programming 26
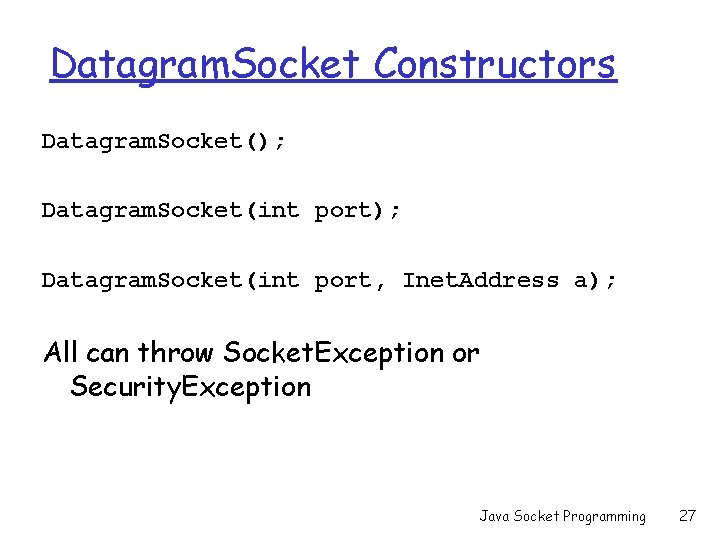
Datagram. Socket Constructors Datagram. Socket(); Datagram. Socket(int port, Inet. Address a); All can throw Socket. Exception or Security. Exception Java Socket Programming 27
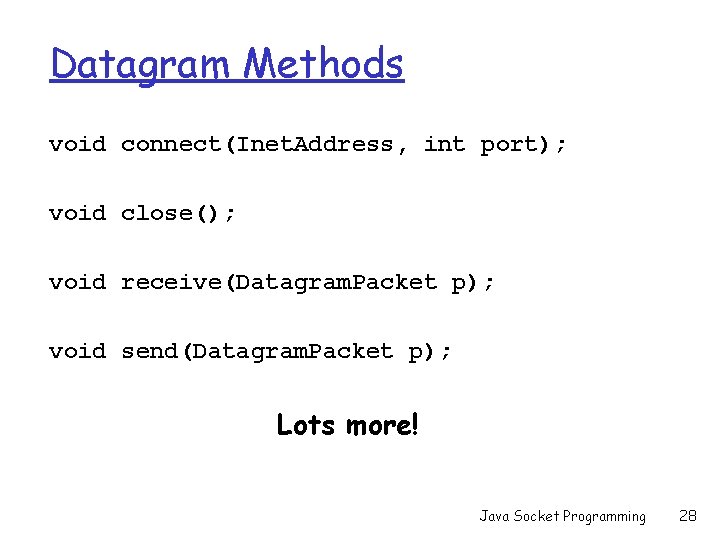
Datagram Methods void connect(Inet. Address, int port); void close(); void receive(Datagram. Packet p); void send(Datagram. Packet p); Lots more! Java Socket Programming 28
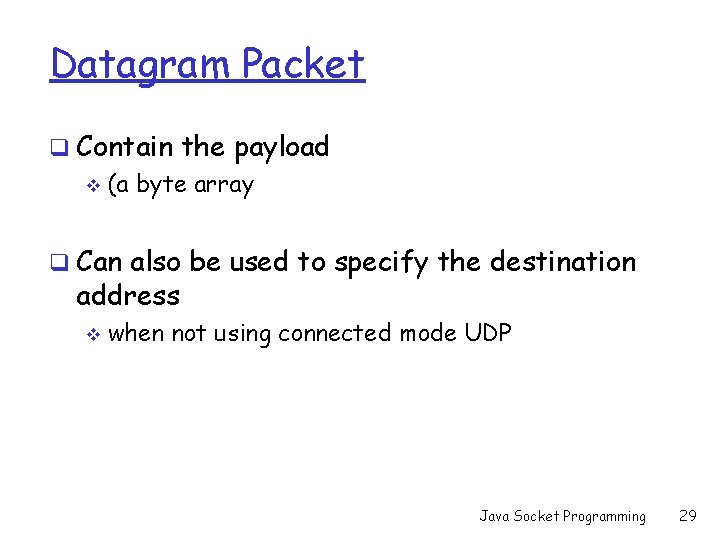
Datagram Packet q Contain the payload v (a byte array q Can also be used to specify the destination address v when not using connected mode UDP Java Socket Programming 29
![Datagram Packet Constructors For receiving Datagram Packet byte buf int len For sending Datagram Datagram. Packet Constructors For receiving: Datagram. Packet( byte[] buf, int len); For sending: Datagram.](https://slidetodoc.com/presentation_image_h2/78d1503d20759a59fd7bf7b6bcb70766/image-30.jpg)
Datagram. Packet Constructors For receiving: Datagram. Packet( byte[] buf, int len); For sending: Datagram. Packet( byte[] buf, int len Inet. Address a, int port); Java Socket Programming 30
![Datagram Packet methods byte get Data void set Databyte buf void set AddressInet Address Datagram. Packet methods byte[] get. Data(); void set. Data(byte[] buf); void set. Address(Inet. Address](https://slidetodoc.com/presentation_image_h2/78d1503d20759a59fd7bf7b6bcb70766/image-31.jpg)
Datagram. Packet methods byte[] get. Data(); void set. Data(byte[] buf); void set. Address(Inet. Address a); void set. Port(int port); Inet. Address get. Address(); int get. Port(); Java Socket Programming 31
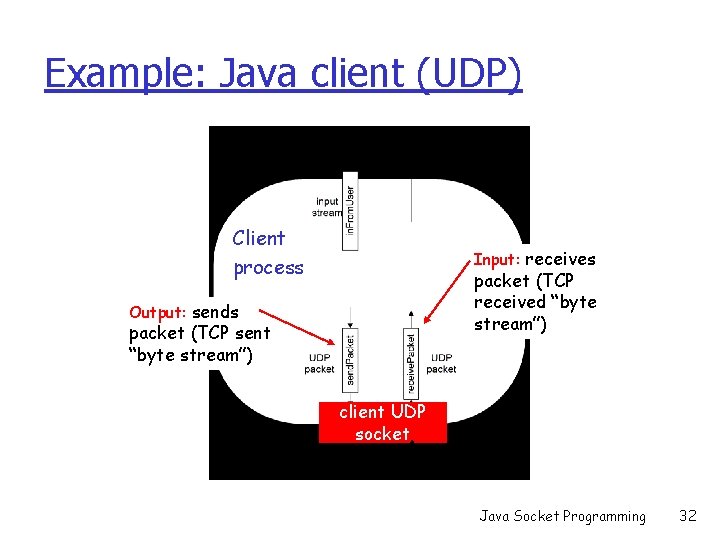
Example: Java client (UDP) Client process Input: receives packet (TCP received “byte stream”) Output: sends packet (TCP sent “byte stream”) client UDP socket Java Socket Programming 32
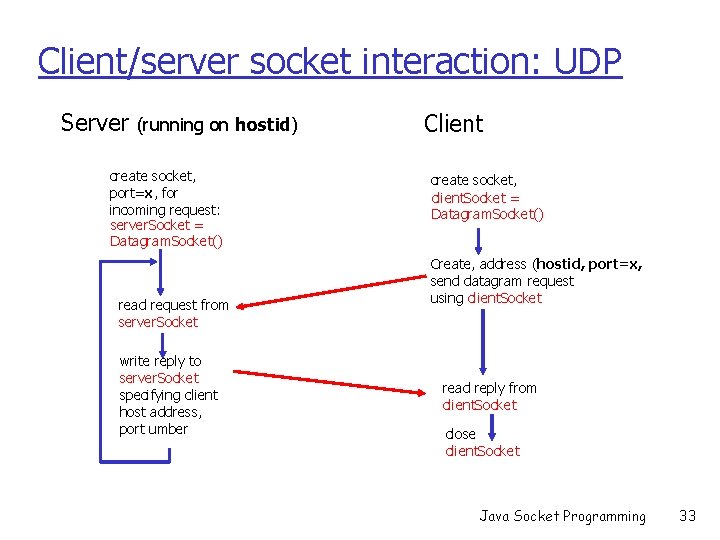
Client/server socket interaction: UDP Server (running on hostid) create socket, port=x, for incoming request: server. Socket = Datagram. Socket() read request from server. Socket write reply to server. Socket specifying client host address, port umber Client create socket, client. Socket = Datagram. Socket() Create, address (hostid, port=x, send datagram request using client. Socket read reply from client. Socket close client. Socket Java Socket Programming 33
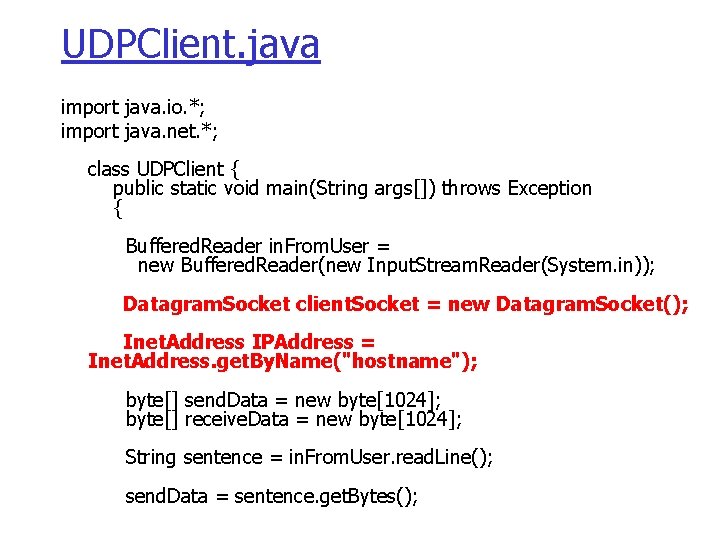
UDPClient. java import java. io. *; import java. net. *; class UDPClient { public static void main(String args[]) throws Exception { Buffered. Reader in. From. User = new Buffered. Reader(new Input. Stream. Reader(System. in)); Datagram. Socket client. Socket = new Datagram. Socket(); Inet. Address IPAddress = Inet. Address. get. By. Name("hostname"); byte[] send. Data = new byte[1024]; byte[] receive. Data = new byte[1024]; String sentence = in. From. User. read. Line(); send. Data = sentence. get. Bytes();
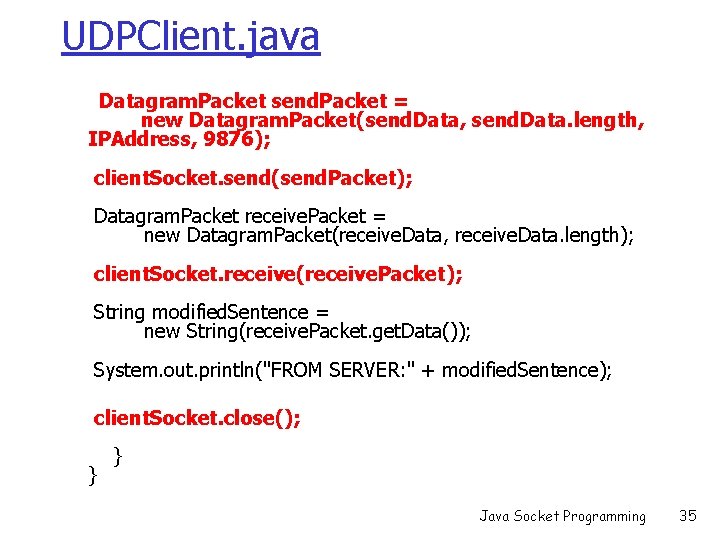
UDPClient. java Datagram. Packet send. Packet = new Datagram. Packet(send. Data, send. Data. length, IPAddress, 9876); client. Socket. send(send. Packet); Datagram. Packet receive. Packet = new Datagram. Packet(receive. Data, receive. Data. length); client. Socket. receive(receive. Packet); String modified. Sentence = new String(receive. Packet. get. Data()); System. out. println("FROM SERVER: " + modified. Sentence); client. Socket. close(); } } Java Socket Programming 35
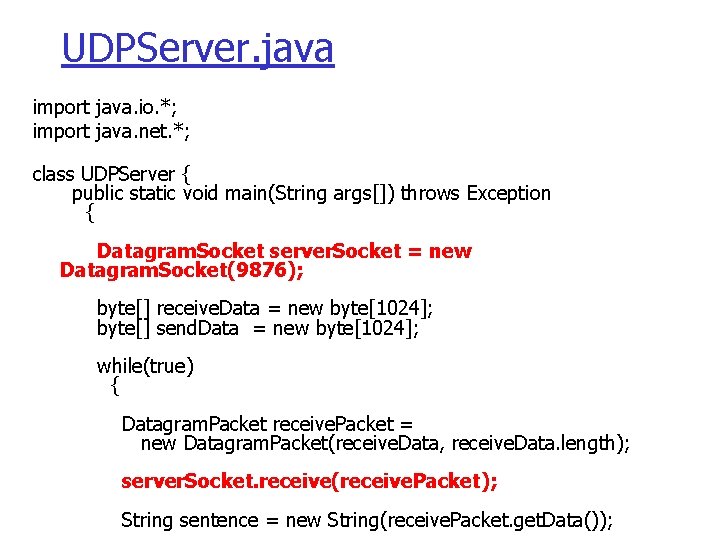
UDPServer. java import java. io. *; import java. net. *; class UDPServer { public static void main(String args[]) throws Exception { Datagram. Socket server. Socket = new Datagram. Socket(9876); byte[] receive. Data = new byte[1024]; byte[] send. Data = new byte[1024]; while(true) { Datagram. Packet receive. Packet = new Datagram. Packet(receive. Data, receive. Data. length); server. Socket. receive(receive. Packet); String sentence = new String(receive. Packet. get. Data());
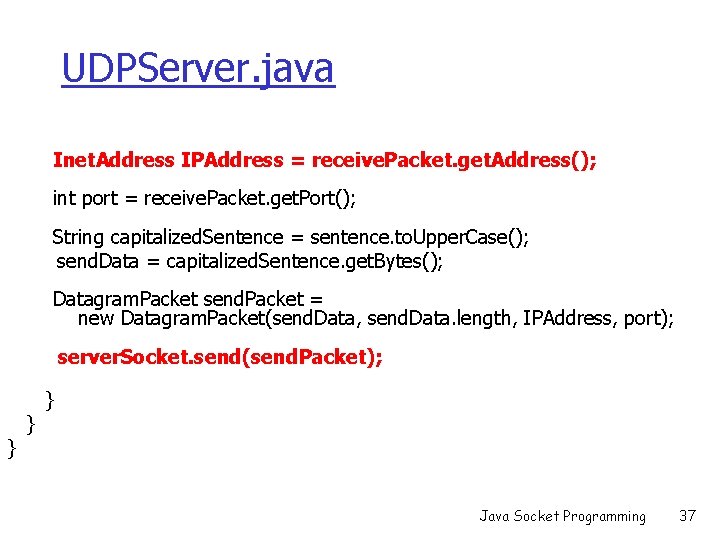
UDPServer. java Inet. Address IPAddress = receive. Packet. get. Address(); int port = receive. Packet. get. Port(); String capitalized. Sentence = sentence. to. Upper. Case(); send. Data = capitalized. Sentence. get. Bytes(); Datagram. Packet send. Packet = new Datagram. Packet(send. Data, send. Data. length, IPAddress, port); server. Socket. send(send. Packet); } } } Java Socket Programming 37
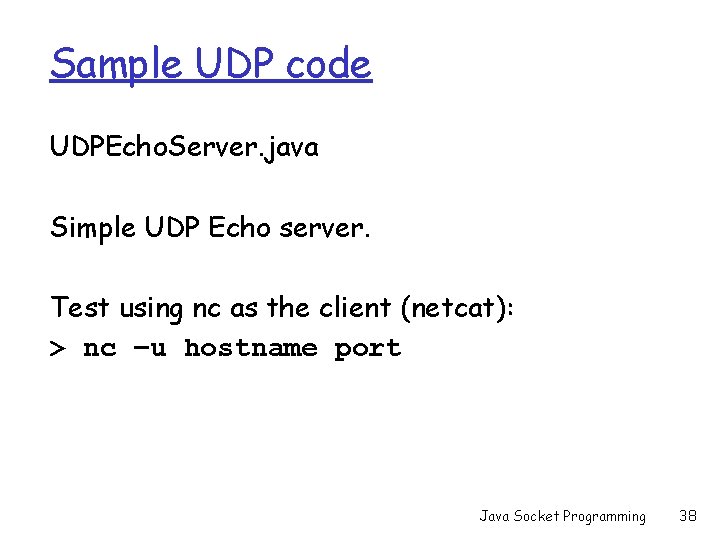
Sample UDP code UDPEcho. Server. java Simple UDP Echo server. Test using nc as the client (netcat): > nc –u hostname port Java Socket Programming 38
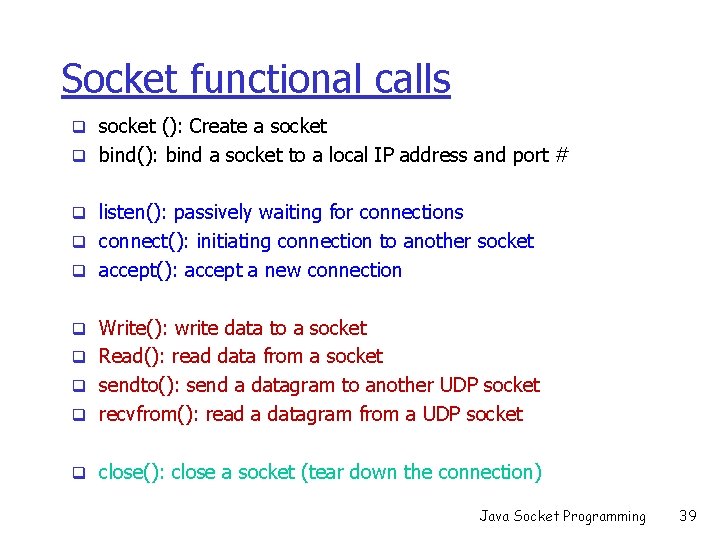
Socket functional calls q socket (): Create a socket q bind(): bind a socket to a local IP address and port # q listen(): passively waiting for connections q connect(): initiating connection to another socket q accept(): accept a new connection q Write(): write data to a socket q Read(): read data from a socket q sendto(): send a datagram to another UDP socket q recvfrom(): read a datagram from a UDP socket q close(): close a socket (tear down the connection) Java Socket Programming 39
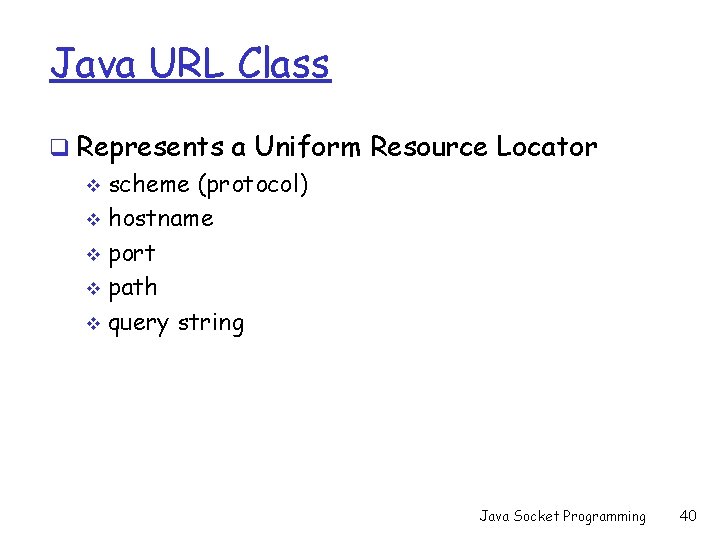
Java URL Class q Represents a Uniform Resource Locator v scheme (protocol) v hostname v port v path v query string Java Socket Programming 40
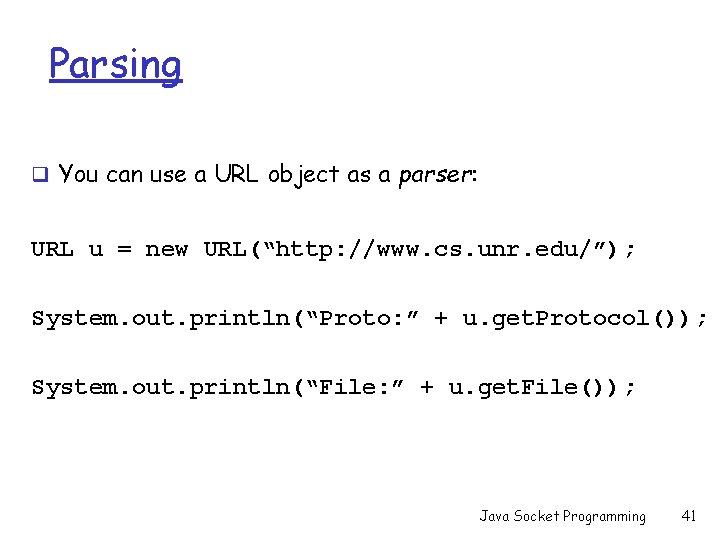
Parsing q You can use a URL object as a parser: URL u = new URL(“http: //www. cs. unr. edu/”); System. out. println(“Proto: ” + u. get. Protocol()); System. out. println(“File: ” + u. get. File()); Java Socket Programming 41
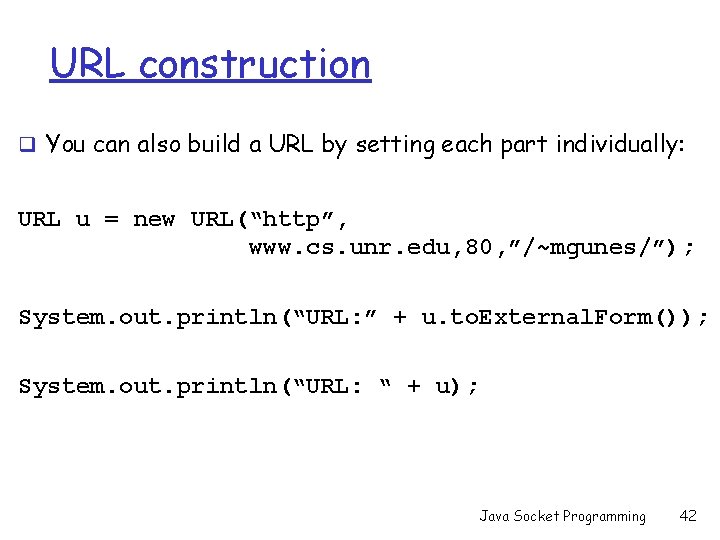
URL construction q You can also build a URL by setting each part individually: URL u = new URL(“http”, www. cs. unr. edu, 80, ”/~mgunes/”); System. out. println(“URL: ” + u. to. External. Form()); System. out. println(“URL: “ + u); Java Socket Programming 42
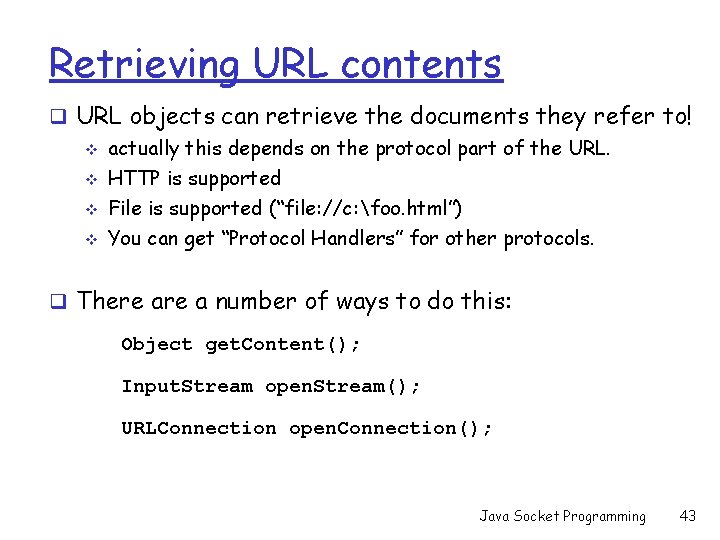
Retrieving URL contents q URL objects can retrieve the documents they refer to! v actually this depends on the protocol part of the URL. v HTTP is supported v File is supported (“file: //c: foo. html”) v You can get “Protocol Handlers” for other protocols. q There a number of ways to do this: Object get. Content(); Input. Stream open. Stream(); URLConnection open. Connection(); Java Socket Programming 43
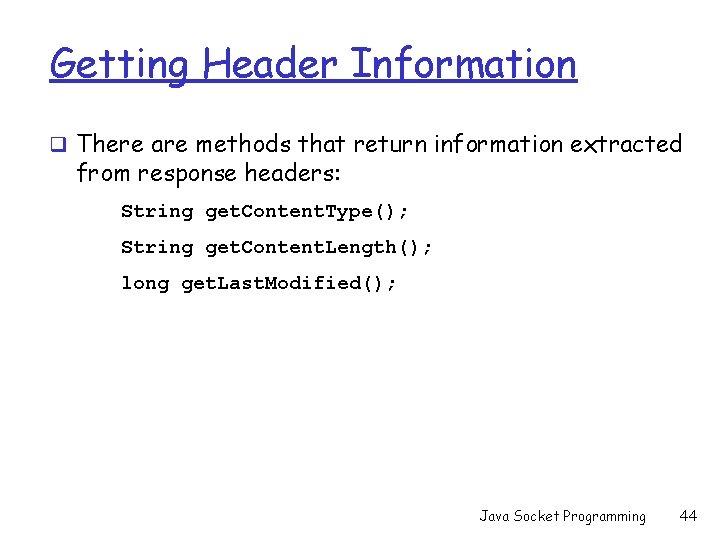
Getting Header Information q There are methods that return information extracted from response headers: String get. Content. Type(); String get. Content. Length(); long get. Last. Modified(); Java Socket Programming 44
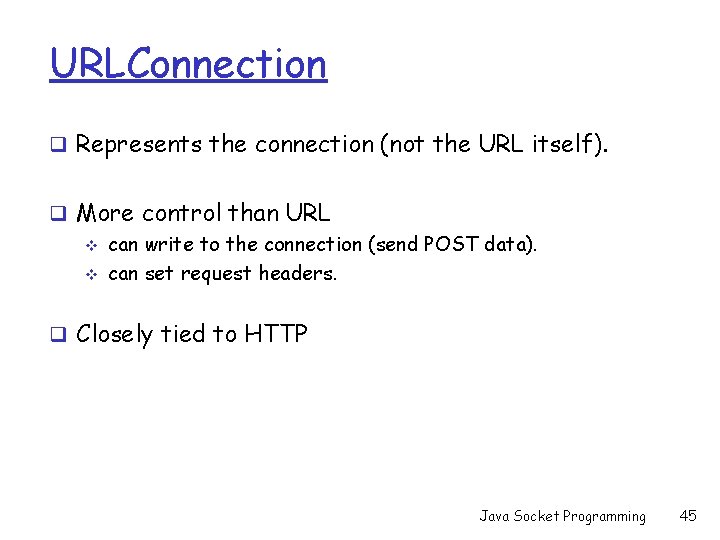
URLConnection q Represents the connection (not the URL itself). q More control than URL v can write to the connection (send POST data). v can set request headers. q Closely tied to HTTP Java Socket Programming 45
Java client server tutorial
Unix network programming stevens
Tcp/ip sockets in java: practical guide for programmers
Socket programming in c
Welcoming socket
Bind function in socket programming
Udp broadcast socket programming in c
What is socket
Bsd socket programming
R socket programming
Socket java example
Socket java example
Java socket proxy
Rmi vs socket
Applicazione distribuita
Java raw socket
Lập trình socket giao tiếp tcp client/server java
Tcp/ip sockets in c
Sockets of the tabernacle
Tcp ip sockets in c
Relay rod steering system
Infini band
Tcp socket primitives
Reliable datagram sockets
What are raw sockets
Elementary udp sockets
Sockets and threads
Cleanroom 380v sockets
Tanenbaum linux
Secure sockets
Datacenter fabric
Berkeley sockets
Hát kết hợp bộ gõ cơ thể
Ng-html
Bổ thể
Tỉ lệ cơ thể trẻ em
Voi kéo gỗ như thế nào
Glasgow thang điểm
Bài hát chúa yêu trần thế alleluia
Môn thể thao bắt đầu bằng từ chạy
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công thức tính độ biến thiên đông lượng
Trời xanh đây là của chúng ta thể thơ
Mật thư tọa độ 5x5
Phép trừ bù