Decision Structures The IfElse If Statement Nested If
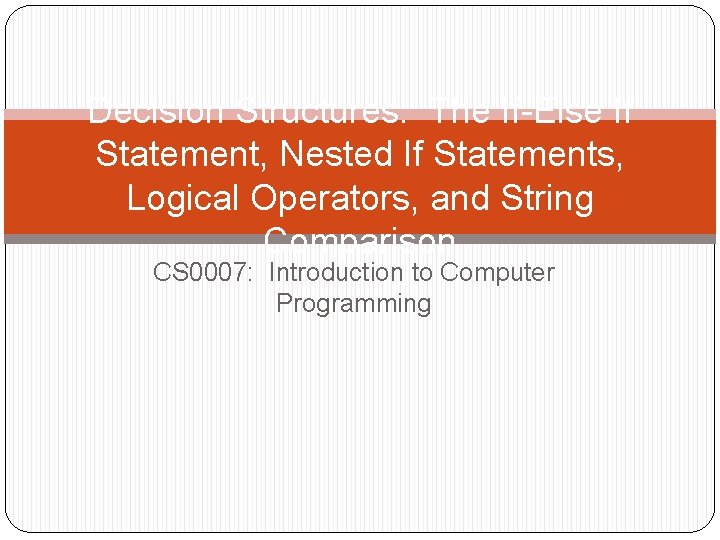
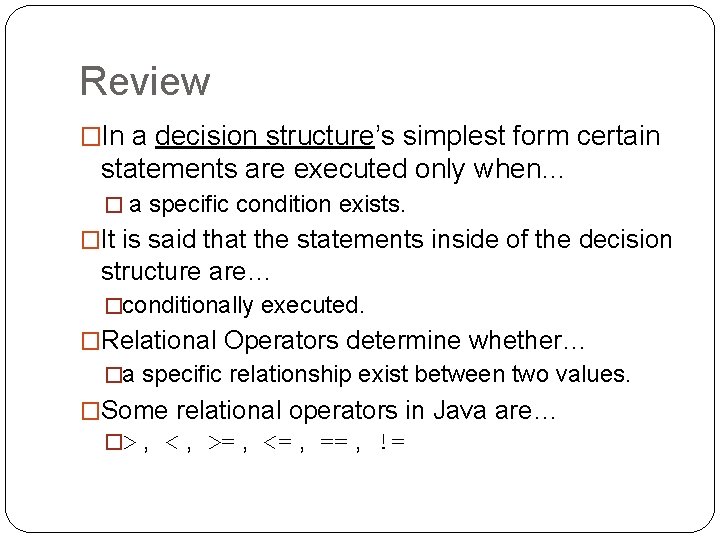
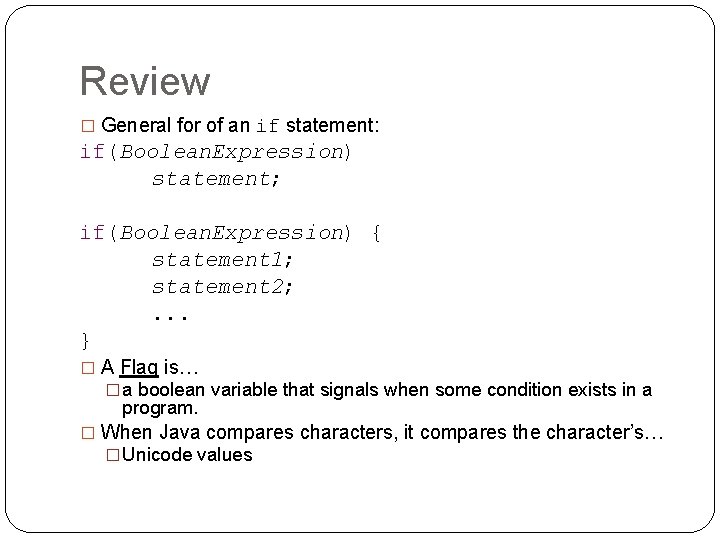
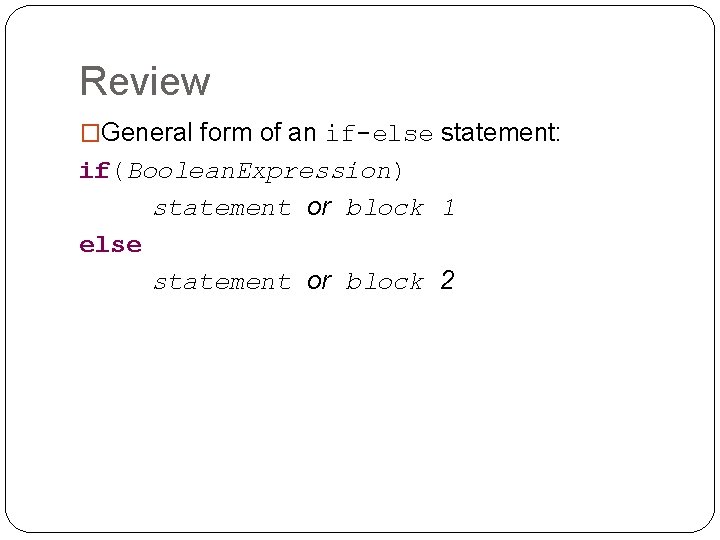
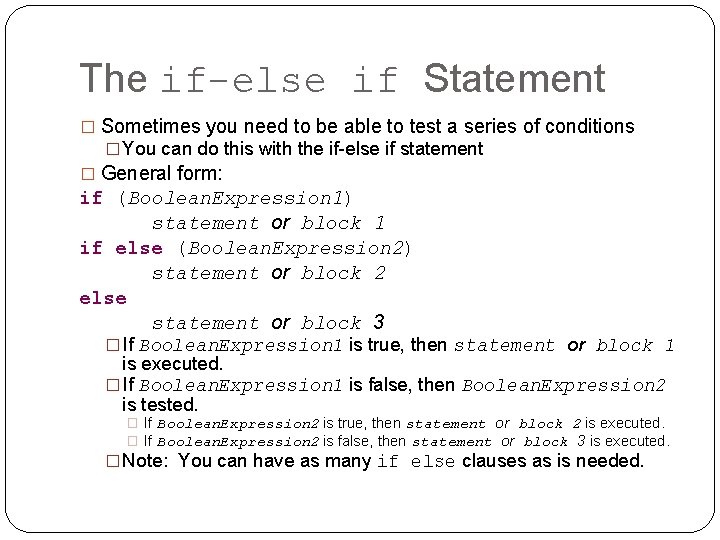
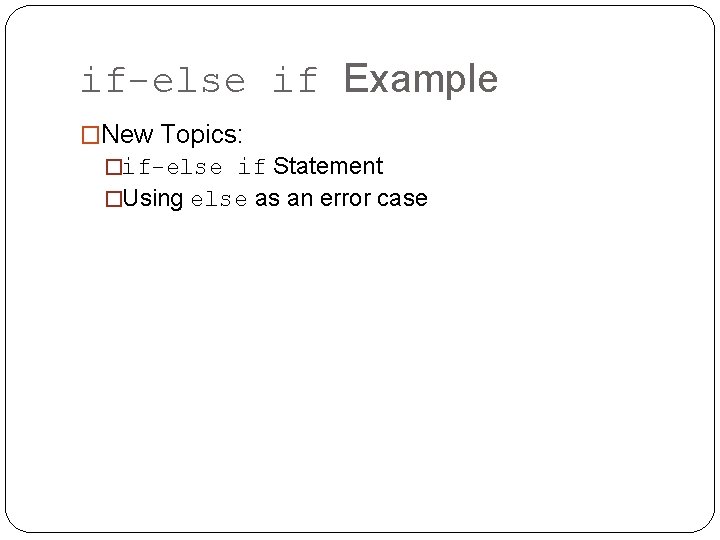
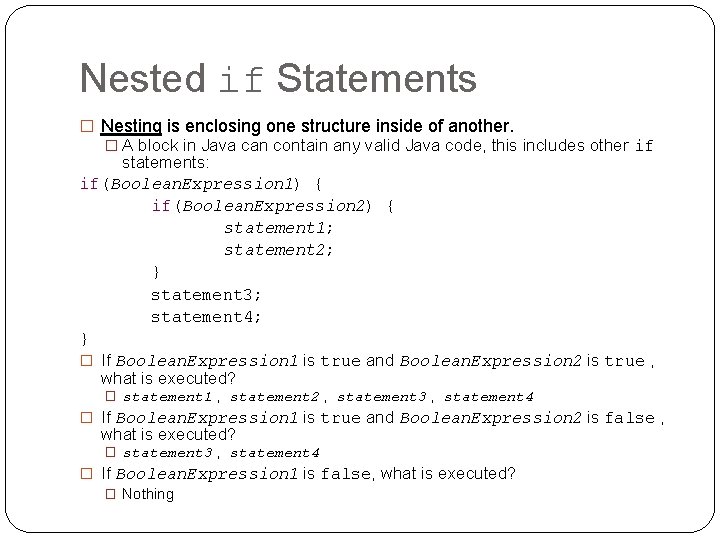
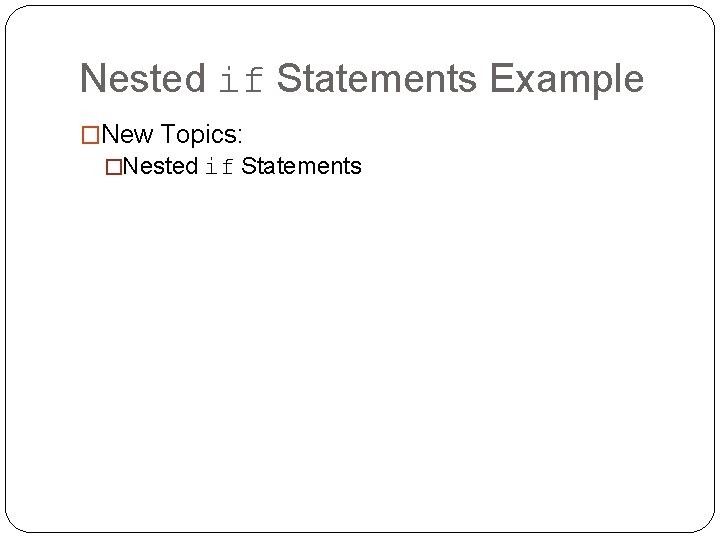
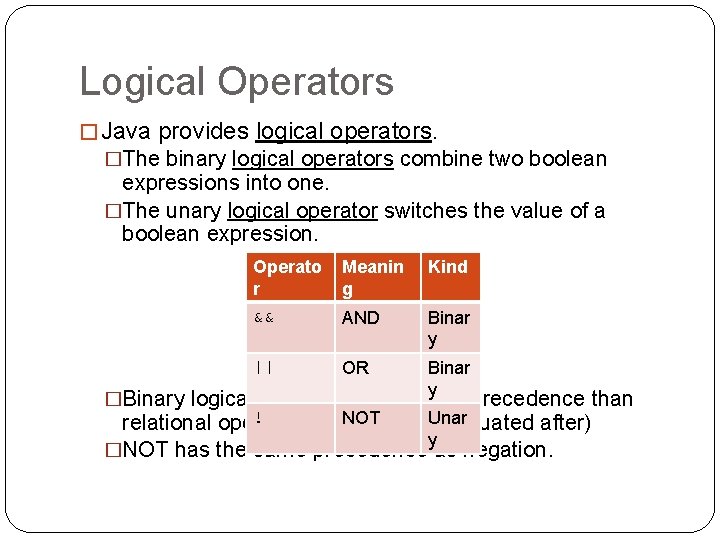
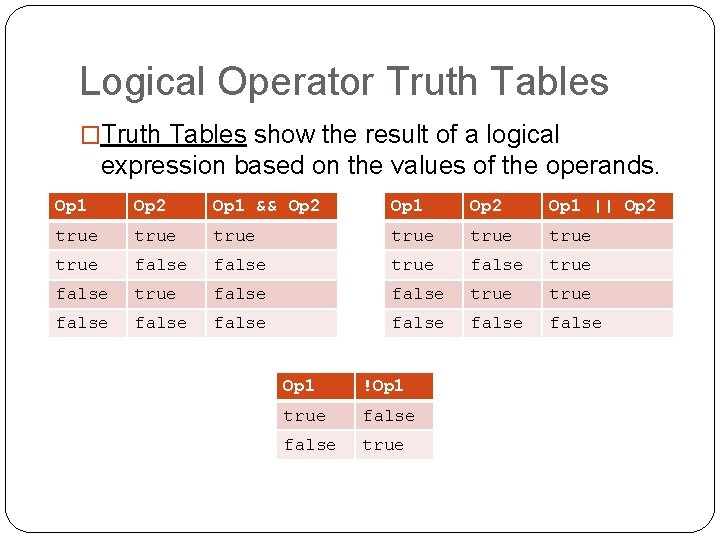
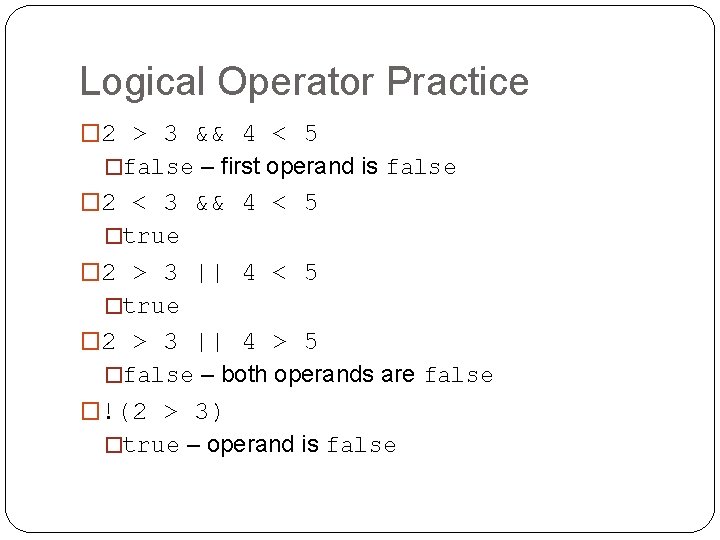
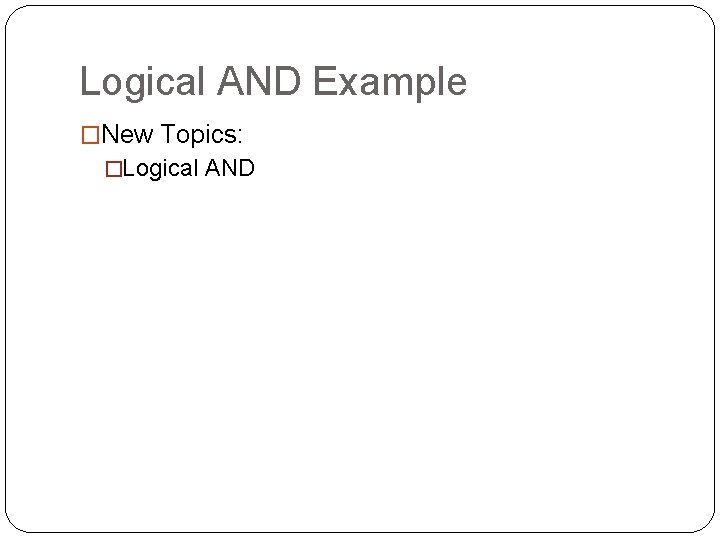
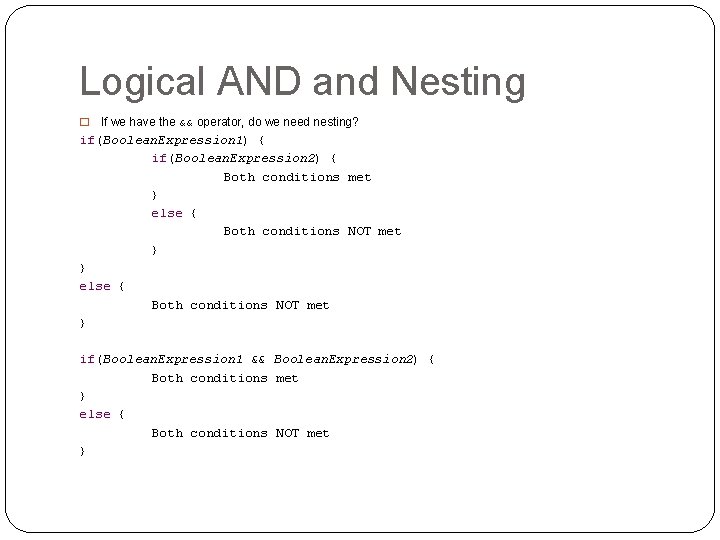
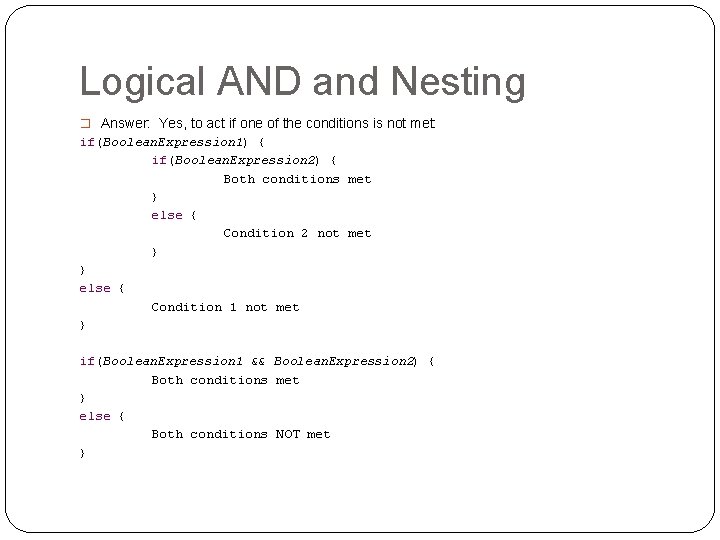
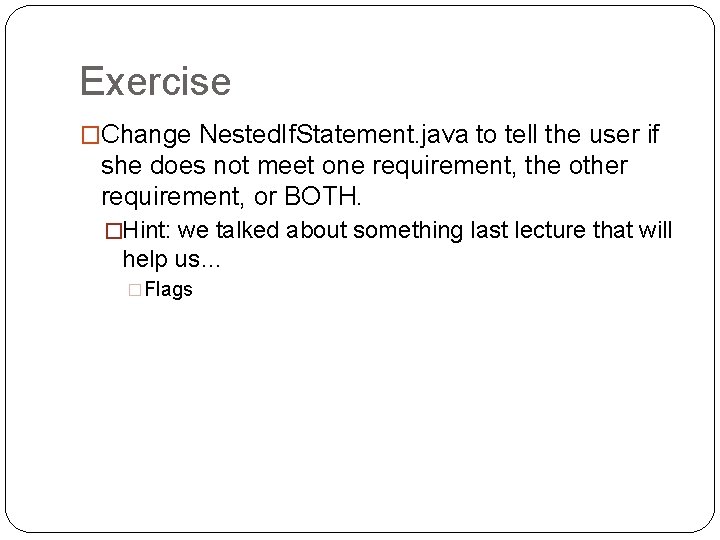
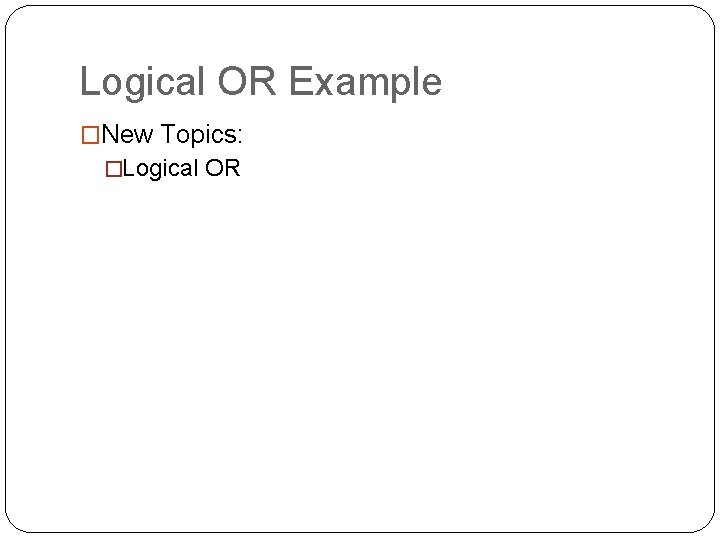
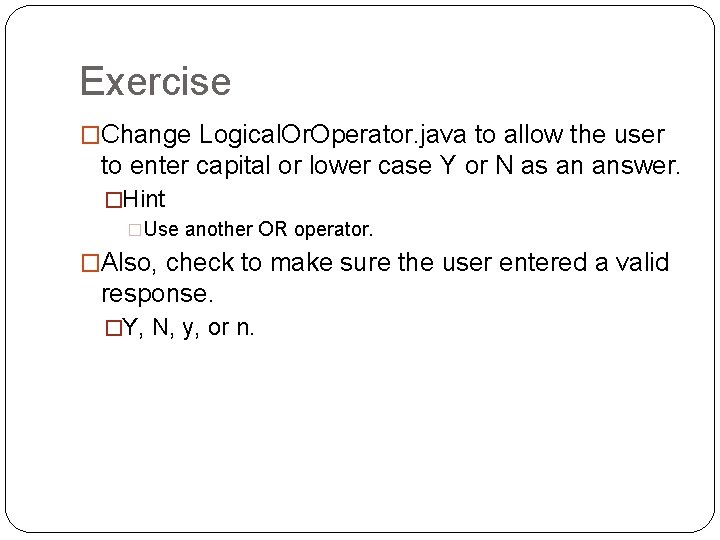
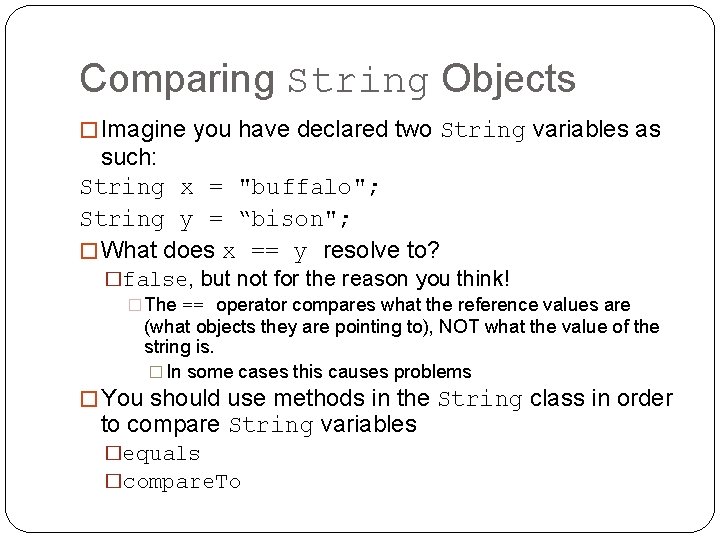
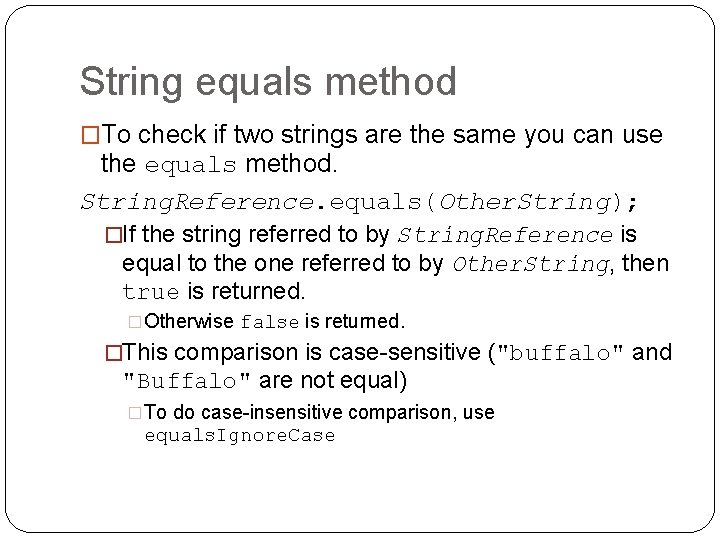
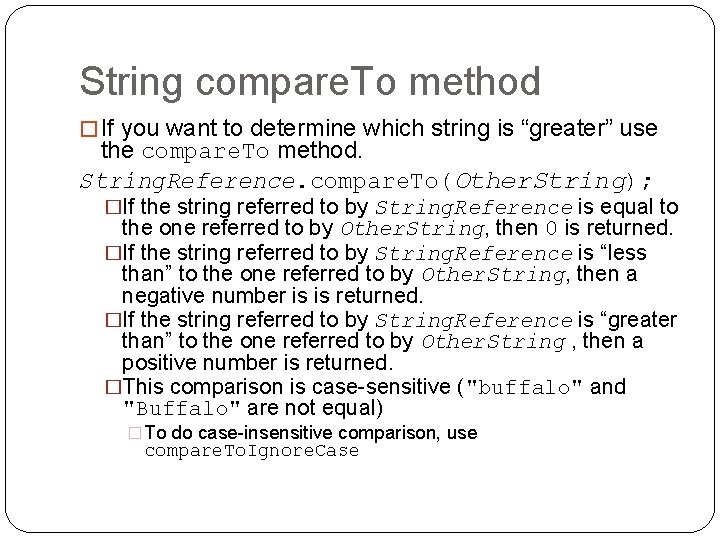
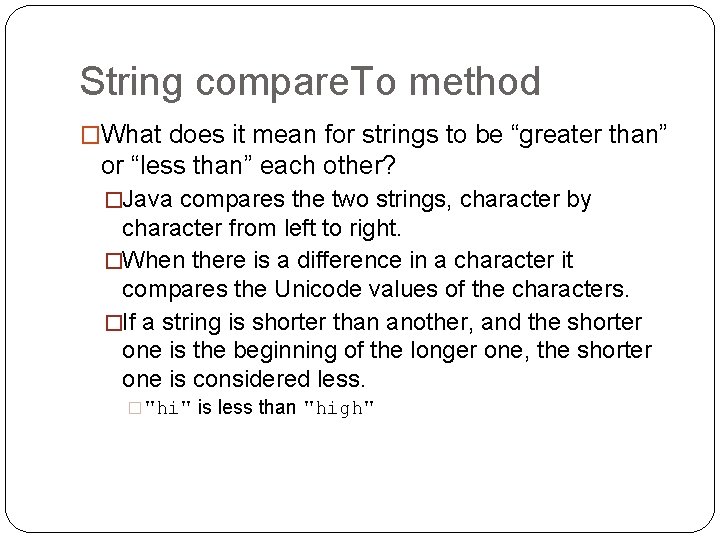
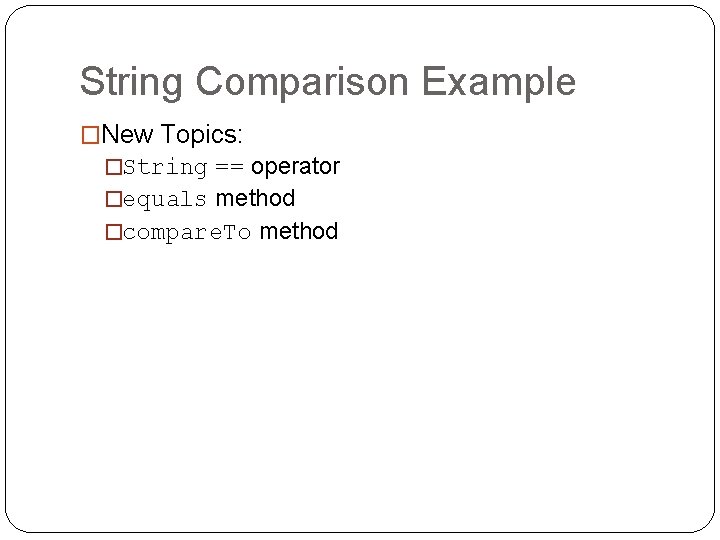
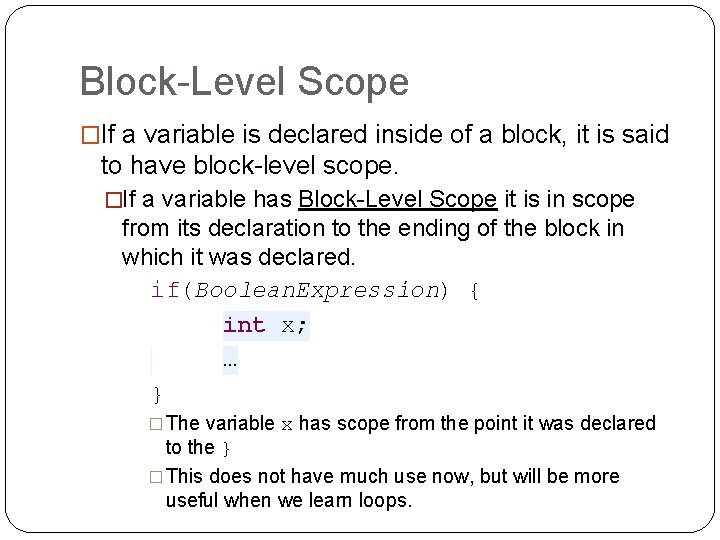
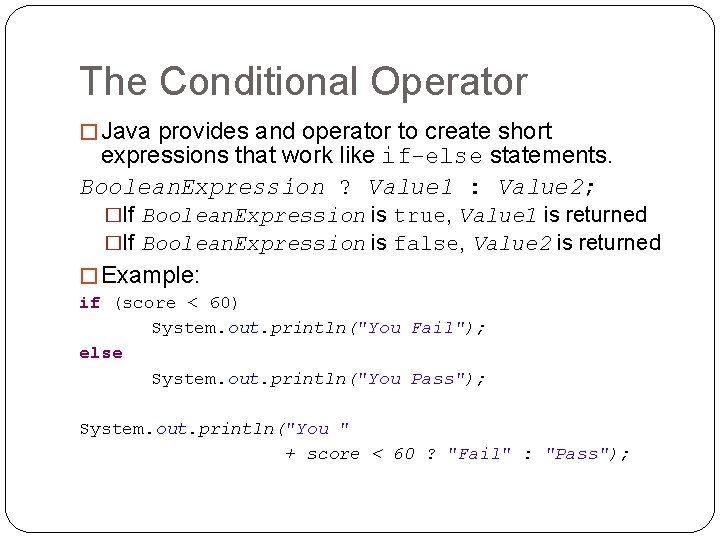
- Slides: 24
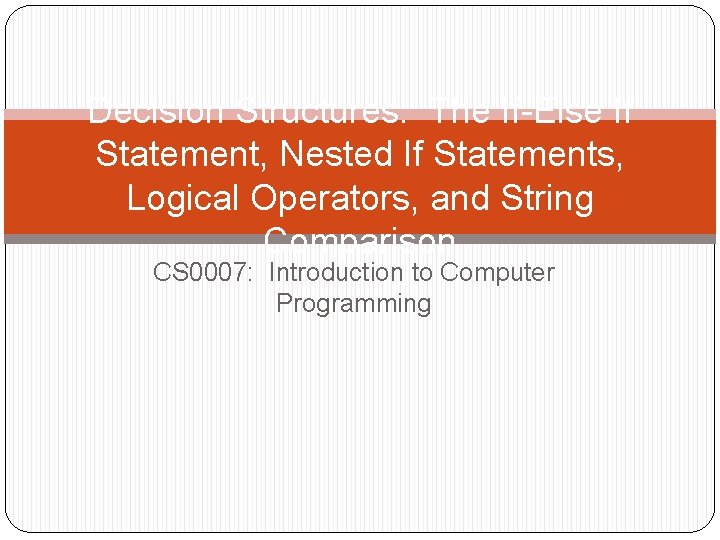
Decision Structures: The If-Else If Statement, Nested If Statements, Logical Operators, and String Comparison CS 0007: Introduction to Computer Programming
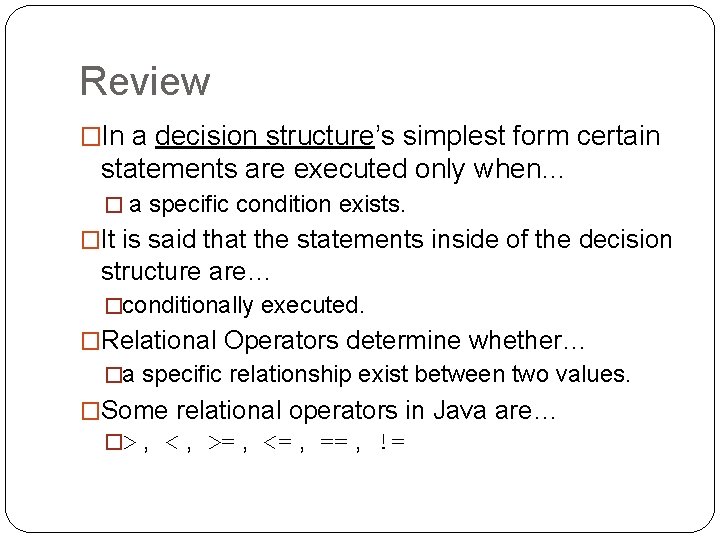
Review �In a decision structure’s simplest form certain statements are executed only when… � a specific condition exists. �It is said that the statements inside of the decision structure are… �conditionally executed. �Relational Operators determine whether… �a specific relationship exist between two values. �Some relational operators in Java are… �> , < , >= , <= , == , !=
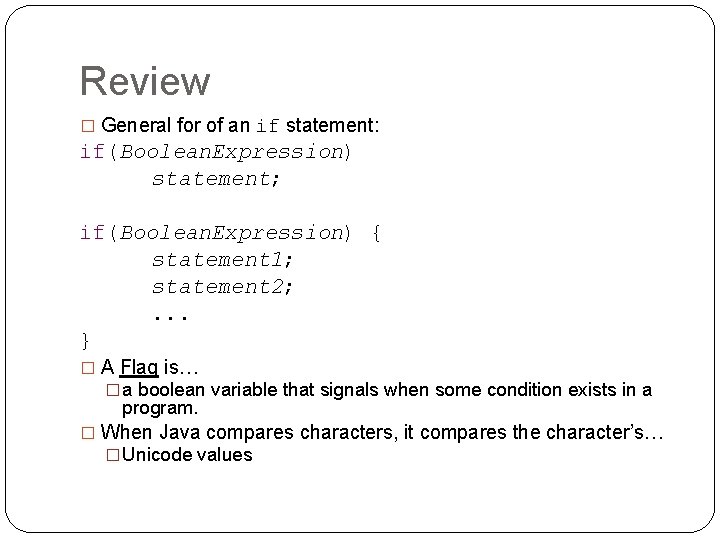
Review � General for of an if statement: if(Boolean. Expression) statement; if(Boolean. Expression) { statement 1; statement 2; . . . } � A Flag is… � a boolean variable that signals when some condition exists in a program. � When Java compares characters, it compares the character’s… � Unicode values
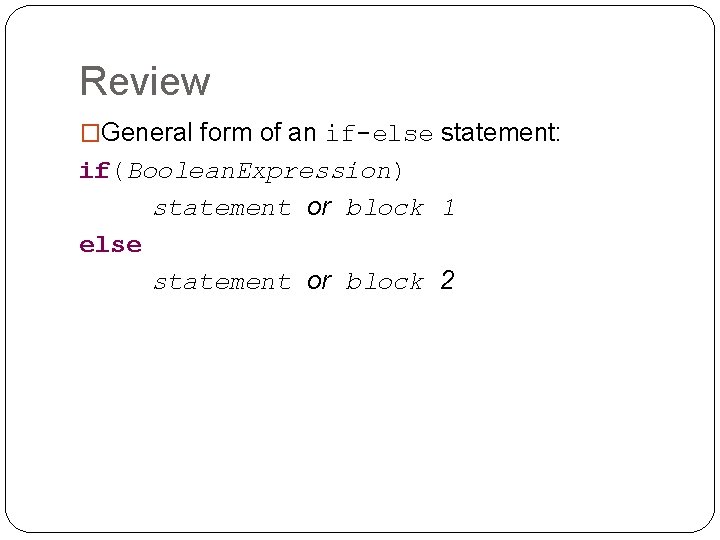
Review �General form of an if-else statement: if(Boolean. Expression) statement or block 1 else statement or block 2
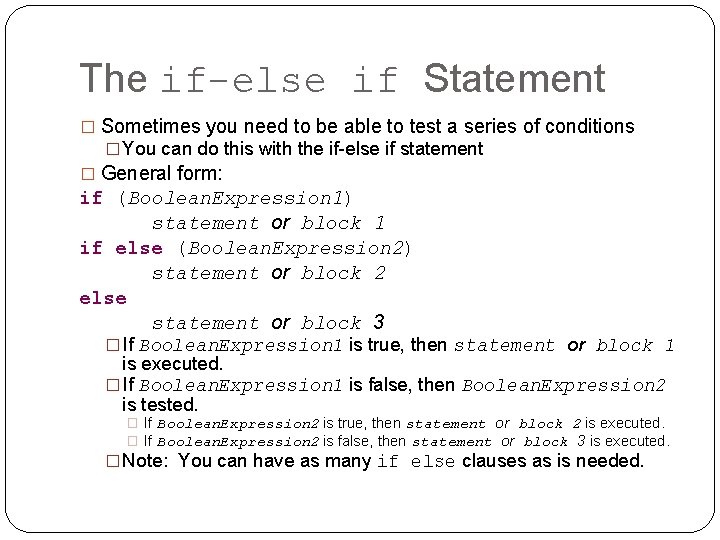
The if-else if Statement � Sometimes you need to be able to test a series of conditions � You can do this with the if-else if statement � General form: if (Boolean. Expression 1) statement or block 1 if else (Boolean. Expression 2) statement or block 2 else statement or block 3 � If Boolean. Expression 1 is true, then statement or block 1 is executed. � If Boolean. Expression 1 is false, then Boolean. Expression 2 is tested. � If Boolean. Expression 2 is true, then statement or block 2 is executed. � If Boolean. Expression 2 is false, then statement or block 3 is executed. � Note: You can have as many if else clauses as is needed.
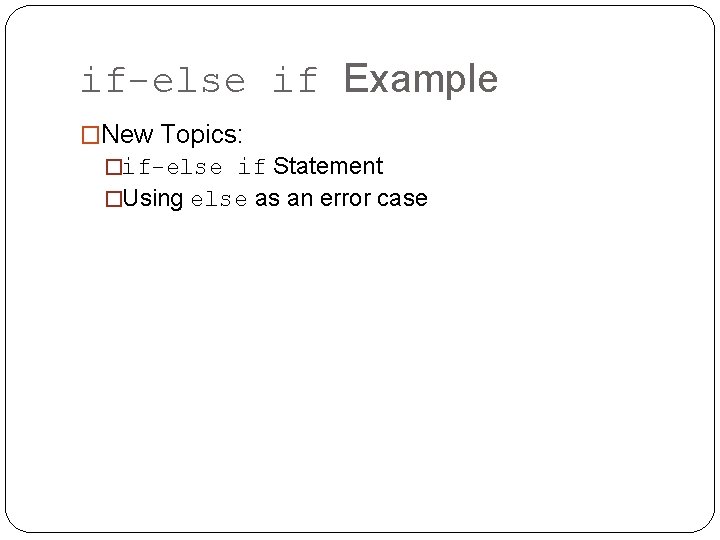
if-else if Example �New Topics: �if-else if Statement �Using else as an error case
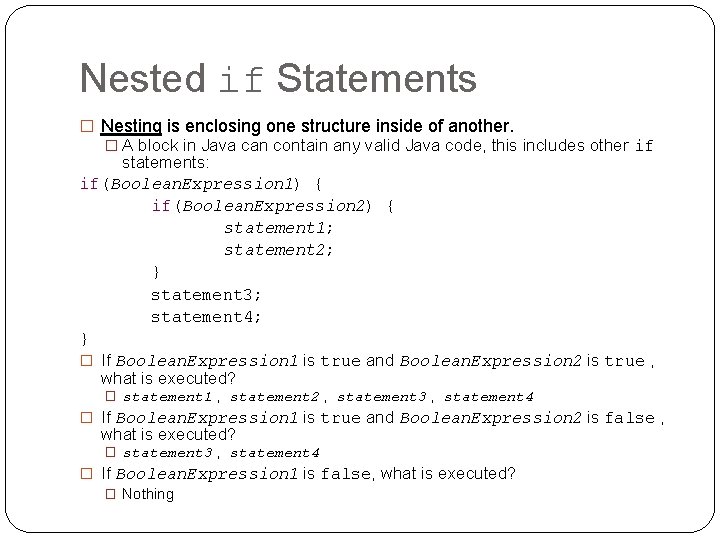
Nested if Statements � Nesting is enclosing one structure inside of another. � A block in Java can contain any valid Java code, this includes other if statements: if(Boolean. Expression 1) { if(Boolean. Expression 2) { statement 1; statement 2; } statement 3; statement 4; } � If Boolean. Expression 1 is true and Boolean. Expression 2 is true , what is executed? � statement 1 , statement 2 , statement 3 , statement 4 � If Boolean. Expression 1 is true and Boolean. Expression 2 is false , what is executed? � statement 3 , statement 4 � If Boolean. Expression 1 is false, what is executed? � Nothing
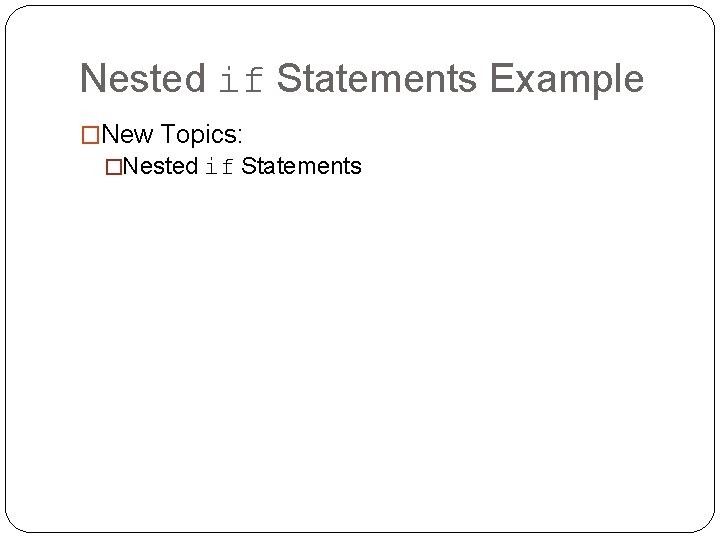
Nested if Statements Example �New Topics: �Nested if Statements
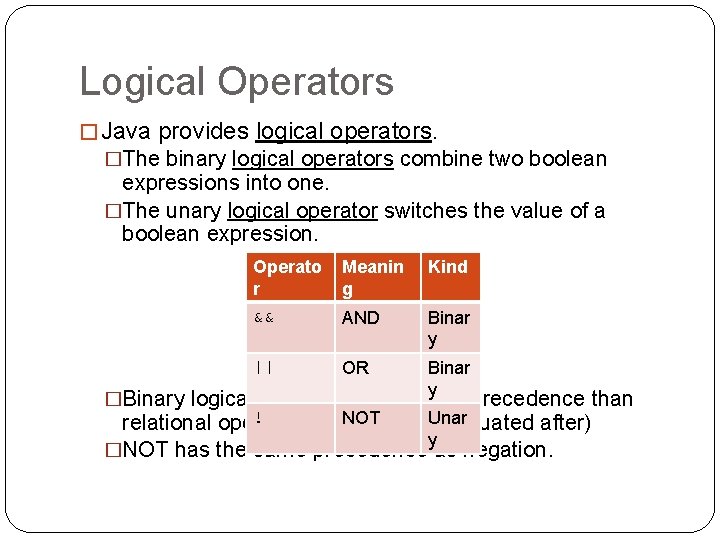
Logical Operators � Java provides logical operators. �The binary logical operators combine two boolean expressions into one. �The unary logical operator switches the value of a boolean expression. Operato r Meanin g Kind && AND Binar y || OR Binar y �Binary logical operators have lower precedence than ! NOTwill be. Unar relational operators (they evaluated after) y �NOT has the same precedence as negation.
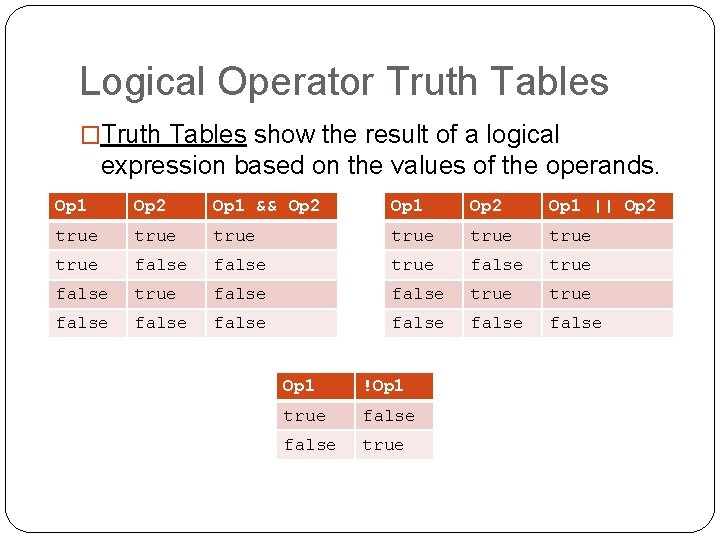
Logical Operator Truth Tables �Truth Tables show the result of a logical expression based on the values of the operands. Op 1 Op 2 Op 1 && Op 2 Op 1 || Op 2 true true false true false true false false Op 1 !Op 1 true false true
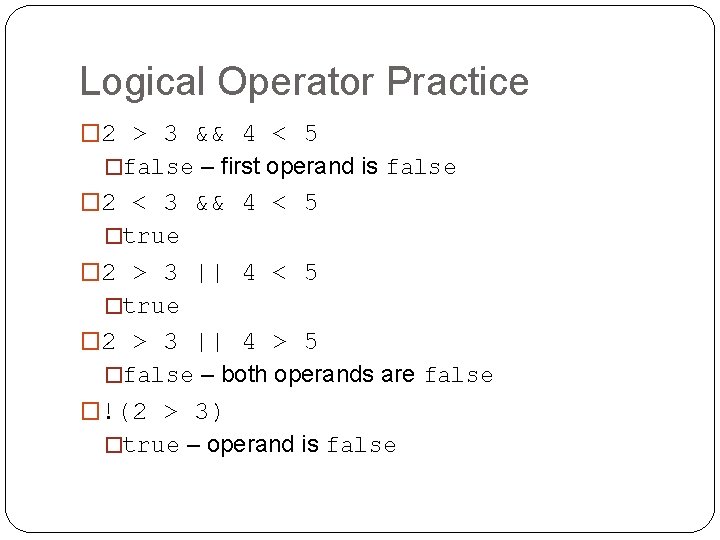
Logical Operator Practice � 2 > 3 && 4 < 5 �false – first operand is false � 2 < 3 && 4 < 5 �true � 2 > 3 || 4 > 5 �false – both operands are false �!(2 > 3) �true – operand is false
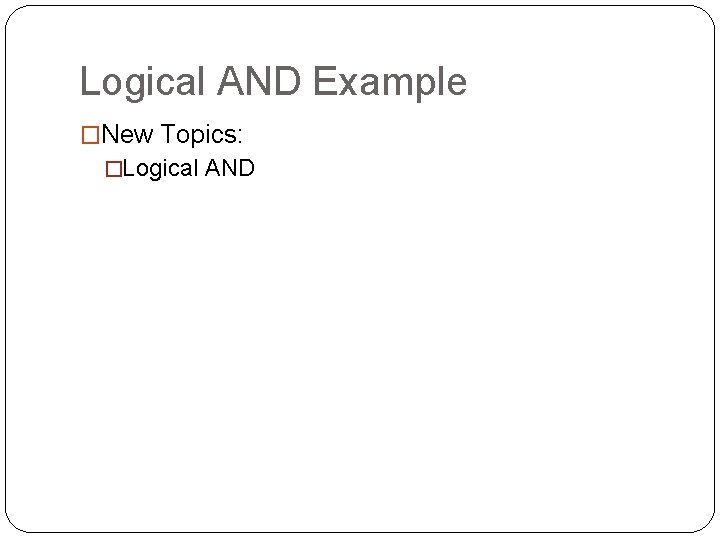
Logical AND Example �New Topics: �Logical AND
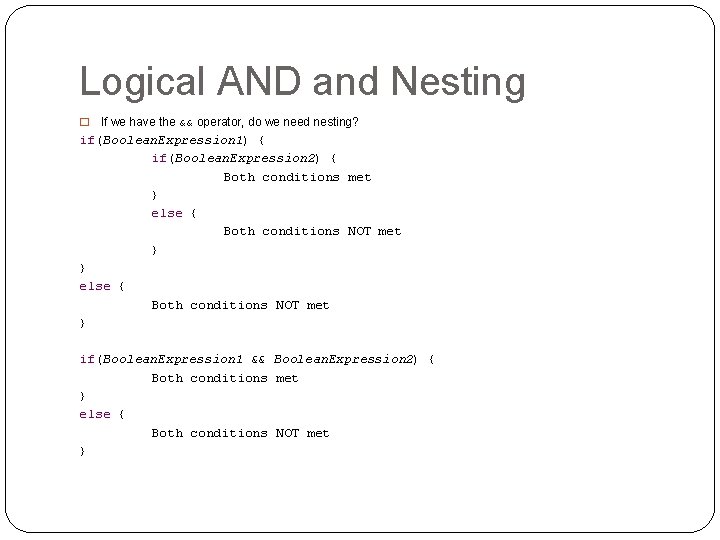
Logical AND and Nesting � If we have the && operator, do we need nesting? if(Boolean. Expression 1) { if(Boolean. Expression 2) { Both conditions met } else { Both conditions NOT met } if(Boolean. Expression 1 && Boolean. Expression 2) { Both conditions met } else { Both conditions NOT met }
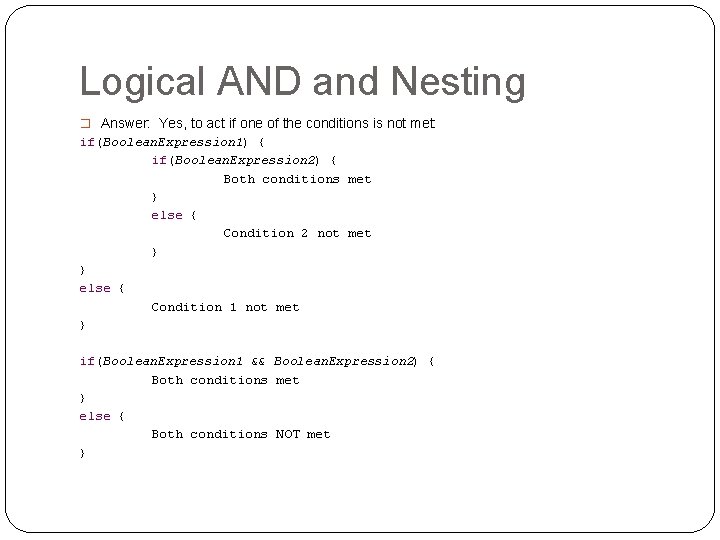
Logical AND and Nesting � Answer: Yes, to act if one of the conditions is not met: if(Boolean. Expression 1) { if(Boolean. Expression 2) { Both conditions met } else { Condition 2 not met } } else { Condition 1 not met } if(Boolean. Expression 1 && Boolean. Expression 2) { Both conditions met } else { Both conditions NOT met }
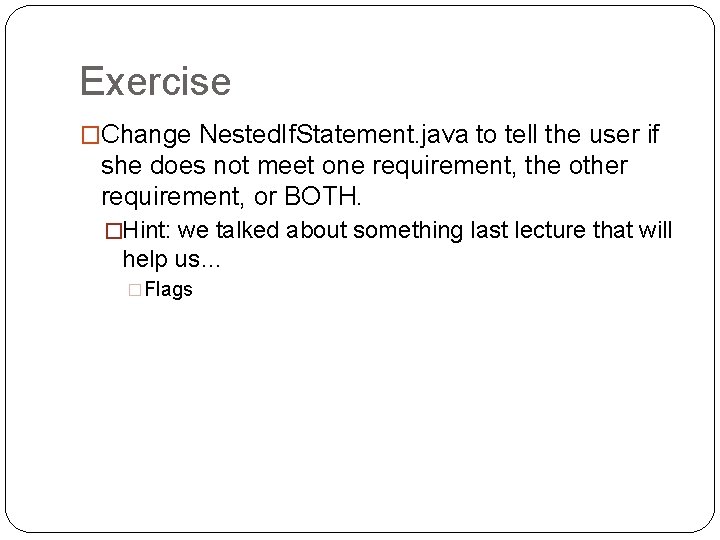
Exercise �Change Nested. If. Statement. java to tell the user if she does not meet one requirement, the other requirement, or BOTH. �Hint: we talked about something last lecture that will help us… �Flags
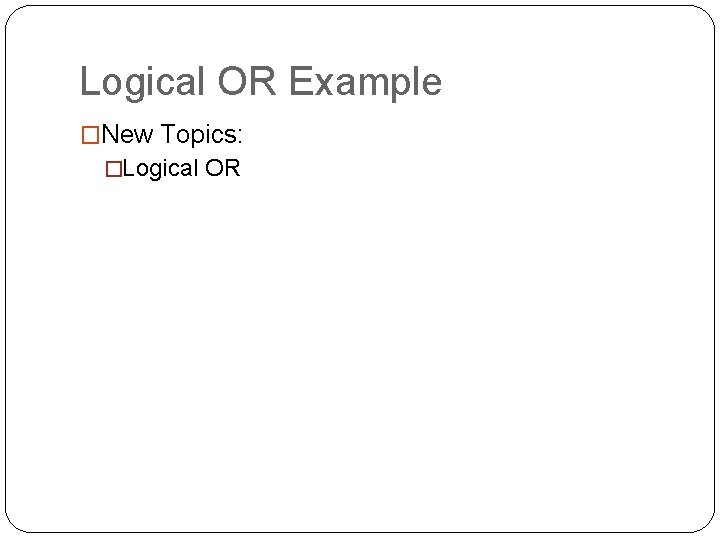
Logical OR Example �New Topics: �Logical OR
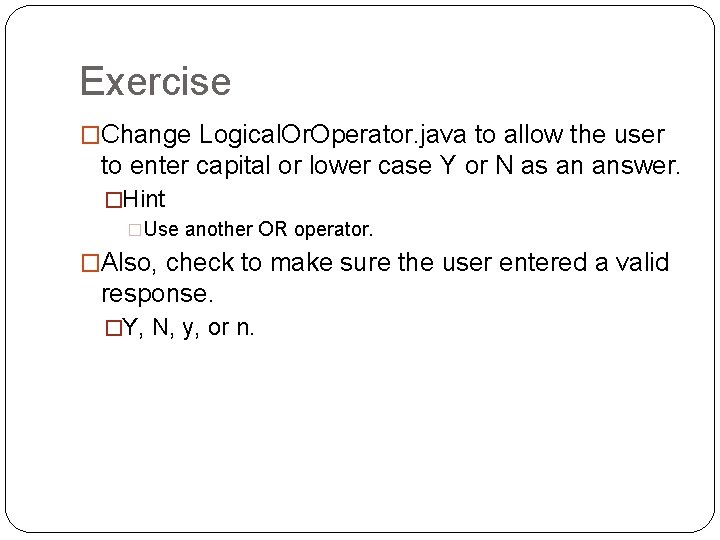
Exercise �Change Logical. Or. Operator. java to allow the user to enter capital or lower case Y or N as an answer. �Hint �Use another OR operator. �Also, check to make sure the user entered a valid response. �Y, N, y, or n.
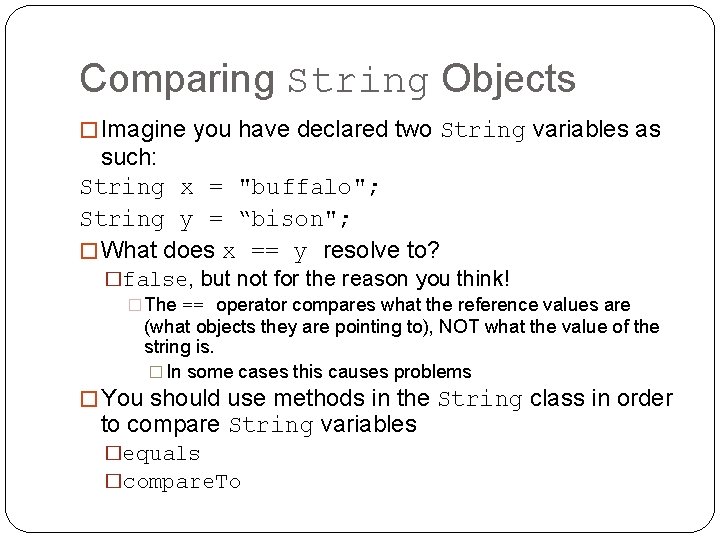
Comparing String Objects � Imagine you have declared two String variables as such: String x = "buffalo"; String y = “bison"; � What does x == y resolve to? �false, but not for the reason you think! � The == operator compares what the reference values are (what objects they are pointing to), NOT what the value of the string is. � In some cases this causes problems � You should use methods in the String class in order to compare String variables �equals �compare. To
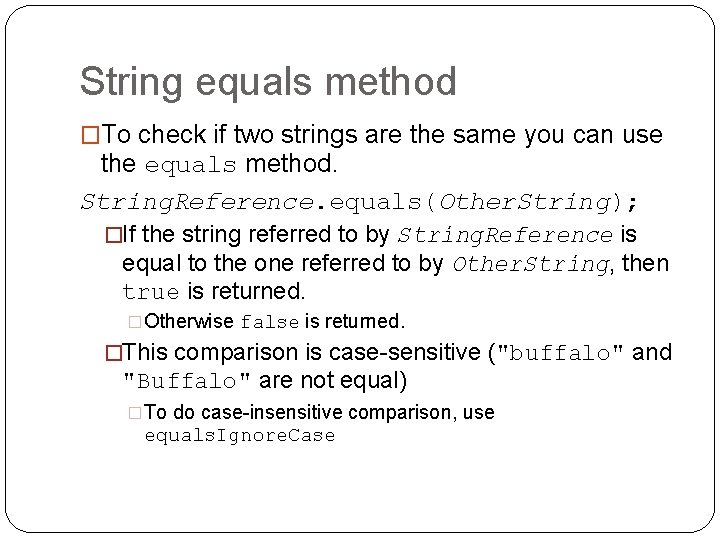
String equals method �To check if two strings are the same you can use the equals method. String. Reference. equals(Other. String); �If the string referred to by String. Reference is equal to the one referred to by Other. String, then true is returned. �Otherwise false is returned. �This comparison is case-sensitive ("buffalo" and "Buffalo" are not equal) �To do case-insensitive comparison, use equals. Ignore. Case
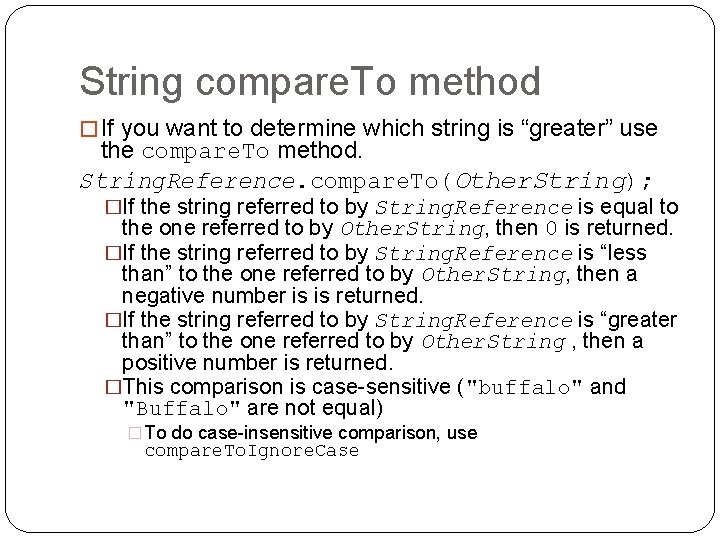
String compare. To method � If you want to determine which string is “greater” use the compare. To method. String. Reference. compare. To(Other. String); �If the string referred to by String. Reference is equal to the one referred to by Other. String, then 0 is returned. �If the string referred to by String. Reference is “less than” to the one referred to by Other. String, then a negative number is is returned. �If the string referred to by String. Reference is “greater than” to the one referred to by Other. String , then a positive number is returned. �This comparison is case-sensitive ("buffalo" and "Buffalo" are not equal) � To do case-insensitive comparison, use compare. To. Ignore. Case
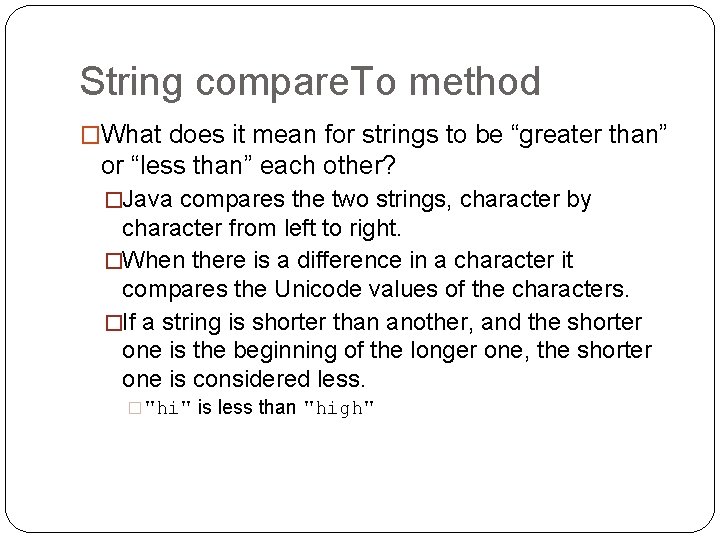
String compare. To method �What does it mean for strings to be “greater than” or “less than” each other? �Java compares the two strings, character by character from left to right. �When there is a difference in a character it compares the Unicode values of the characters. �If a string is shorter than another, and the shorter one is the beginning of the longer one, the shorter one is considered less. �"hi" is less than "high"
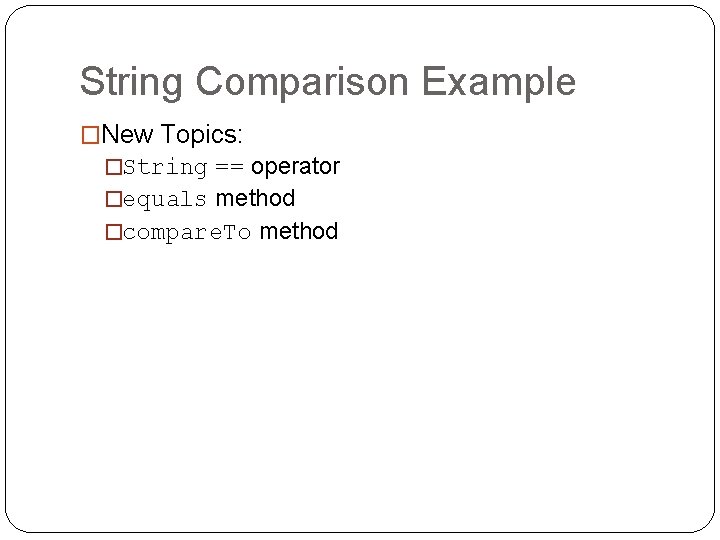
String Comparison Example �New Topics: �String == operator �equals method �compare. To method
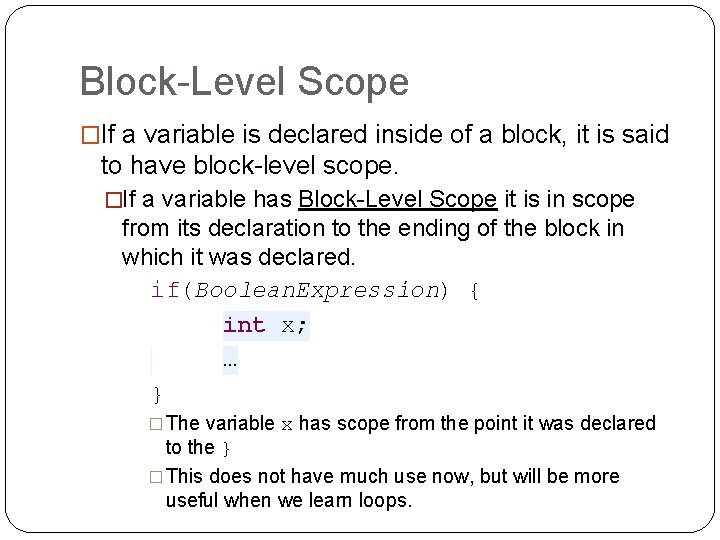
Block-Level Scope �If a variable is declared inside of a block, it is said to have block-level scope. �If a variable has Block-Level Scope it is in scope from its declaration to the ending of the block in which it was declared. if(Boolean. Expression) { int x; … } � The variable x has scope from the point it was declared to the } � This does not have much use now, but will be more useful when we learn loops.
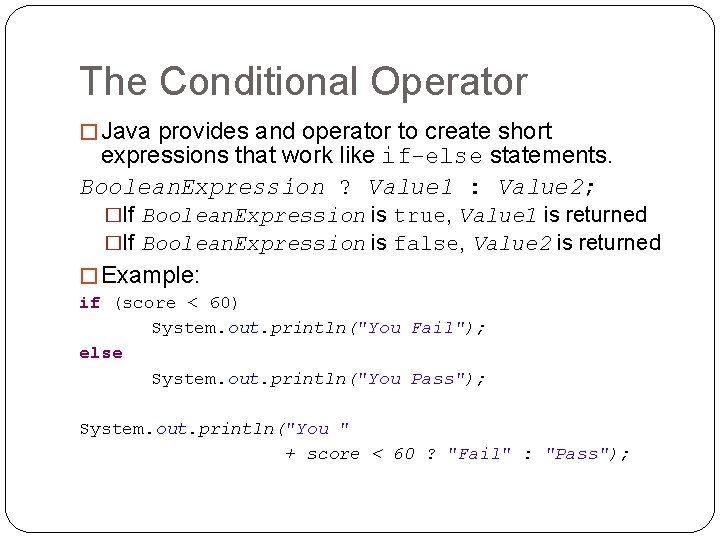
The Conditional Operator � Java provides and operator to create short expressions that work like if-else statements. Boolean. Expression ? Value 1 : Value 2; �If Boolean. Expression is true, Value 1 is returned �If Boolean. Expression is false, Value 2 is returned � Example: if (score < 60) System. out. println("You Fail"); else System. out. println("You Pass"); System. out. println("You " + score < 60 ? "Fail" : "Pass");
Python decision structures
Objectives of decision making
Financial decision
Nested decision structure
Pseudocode else if
What is nested if else statement in c
What is nested if else statement in c
Homologous structures examples
Two way decision python
Decision tree and decision table
Statement level control structures
Statement level control structures
Hát kết hợp bộ gõ cơ thể
Slidetodoc
Bổ thể
Tỉ lệ cơ thể trẻ em
Gấu đi như thế nào
Tư thế worm breton
Chúa yêu trần thế alleluia
Các môn thể thao bắt đầu bằng tiếng nhảy
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công thức tính độ biến thiên đông lượng
Trời xanh đây là của chúng ta thể thơ
Mật thư tọa độ 5x5