CSCICMPE 4341 Topic Programming in Python Review Final
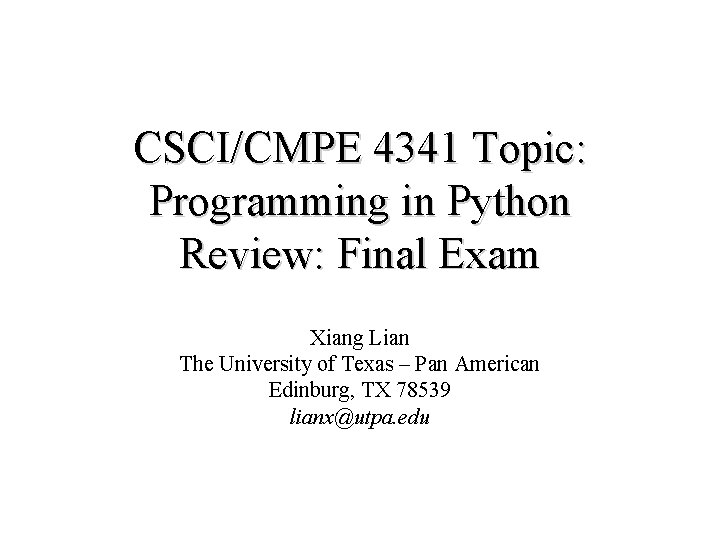
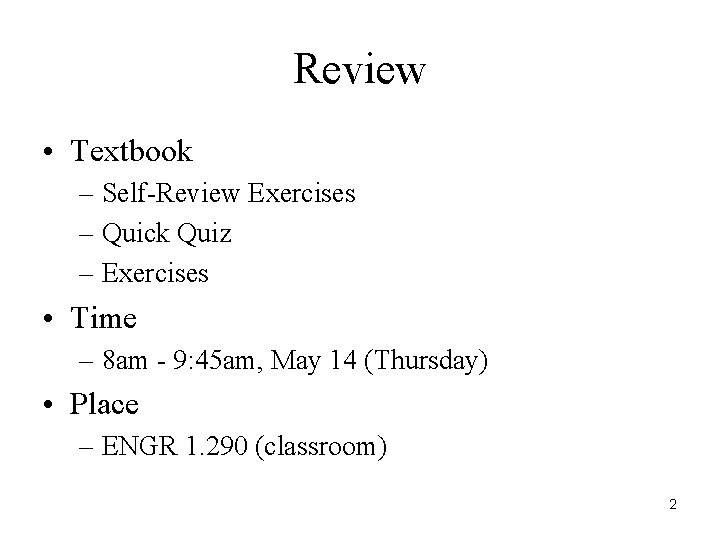
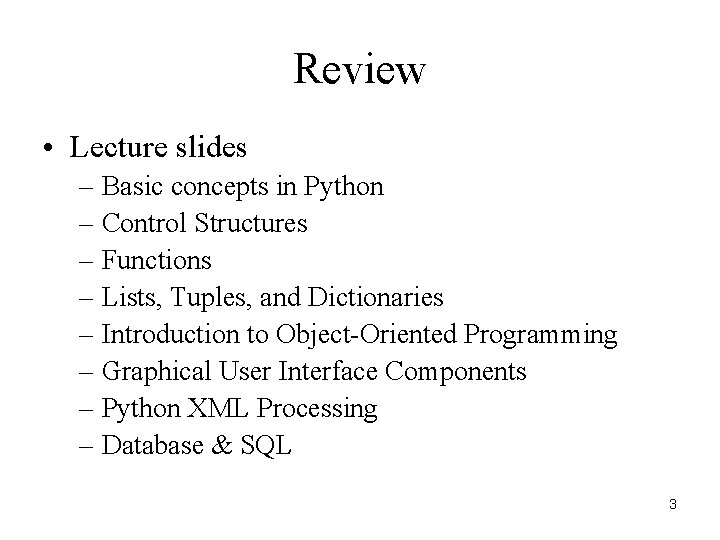
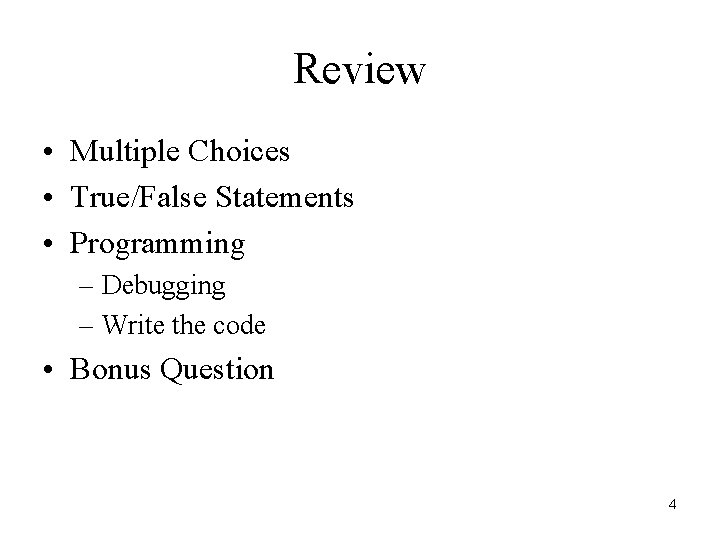
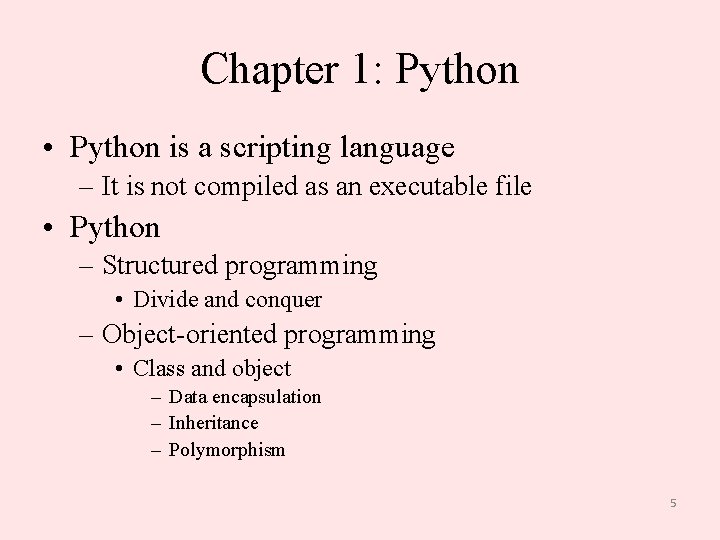
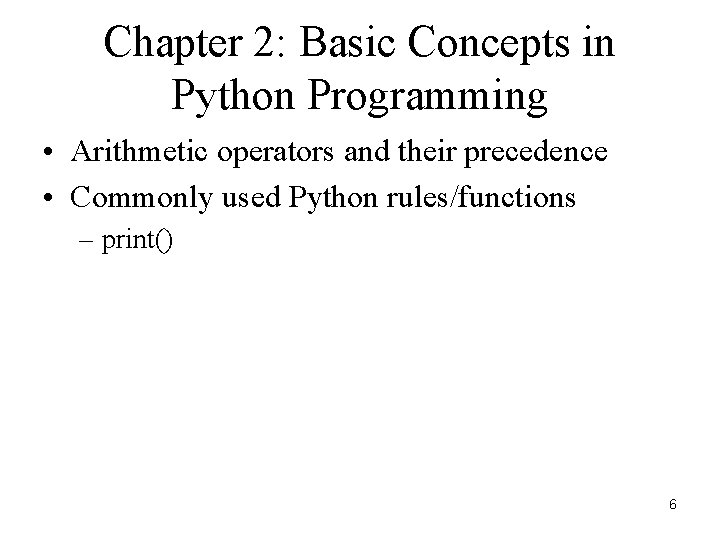
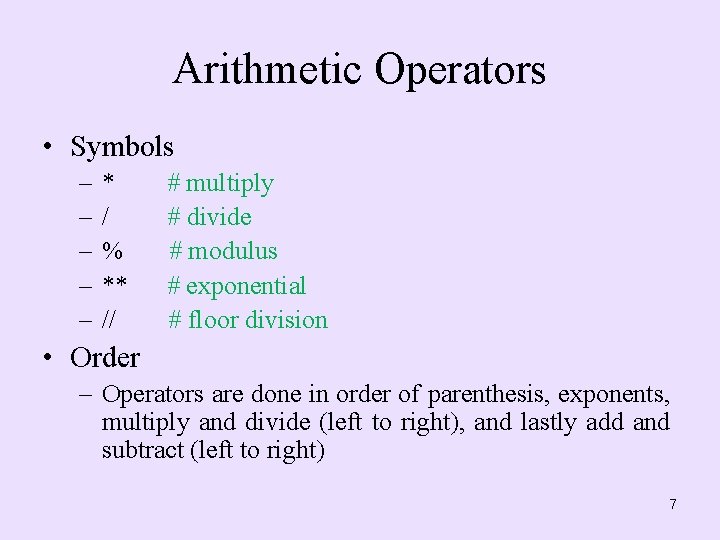
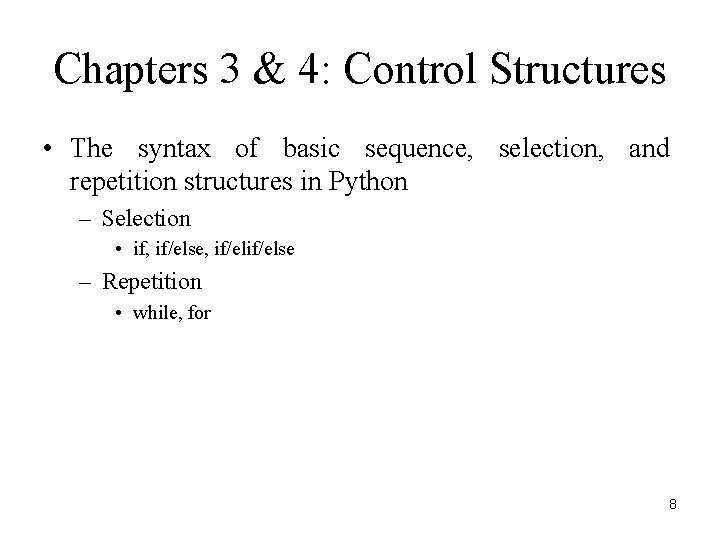
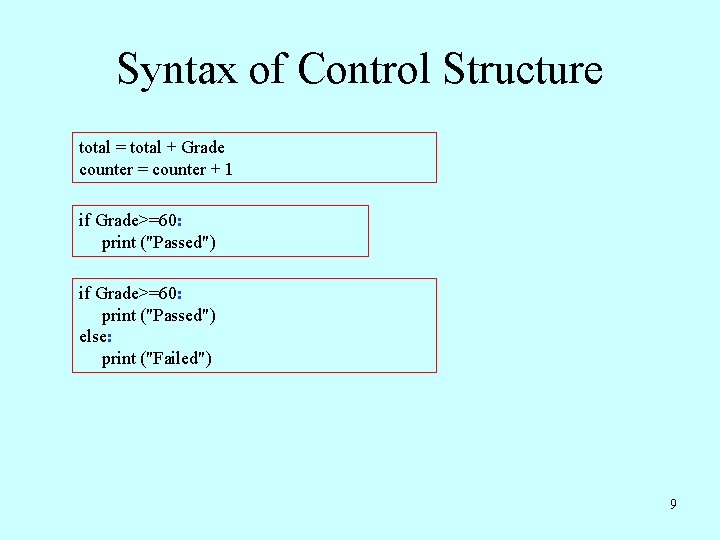
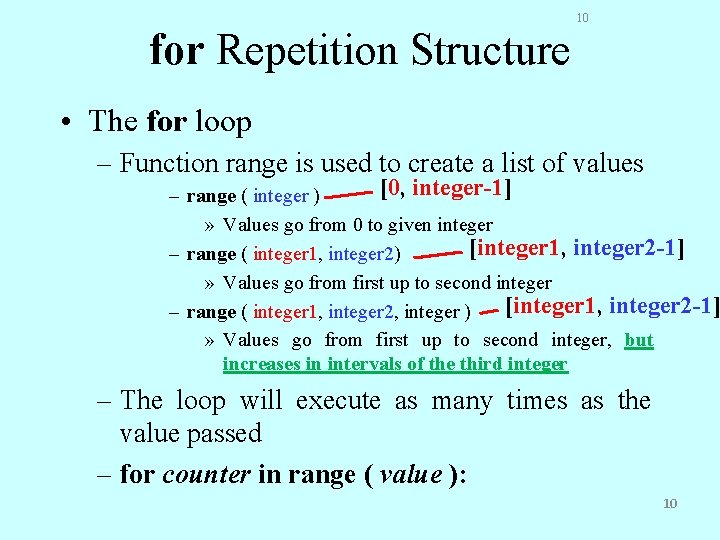
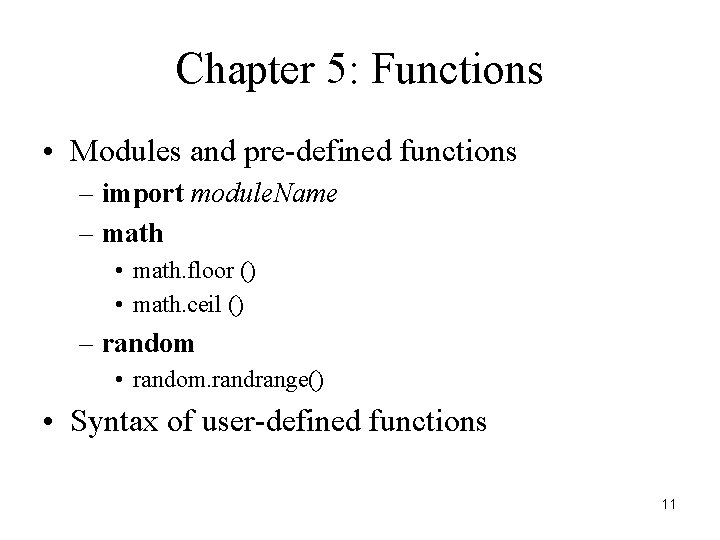
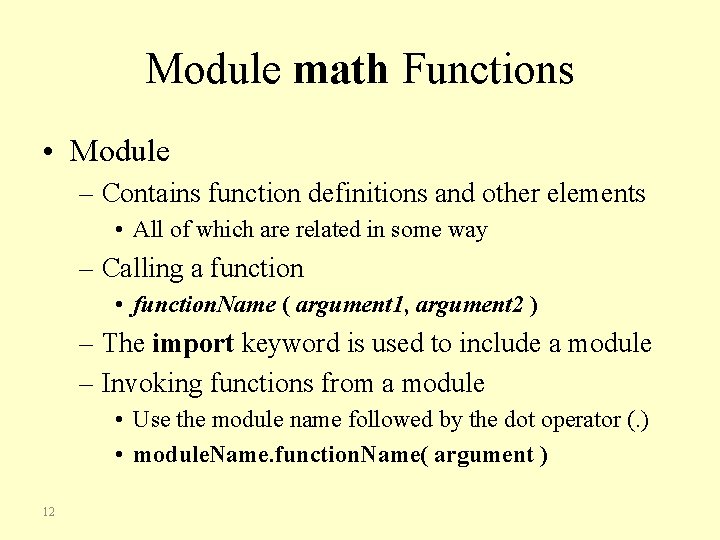
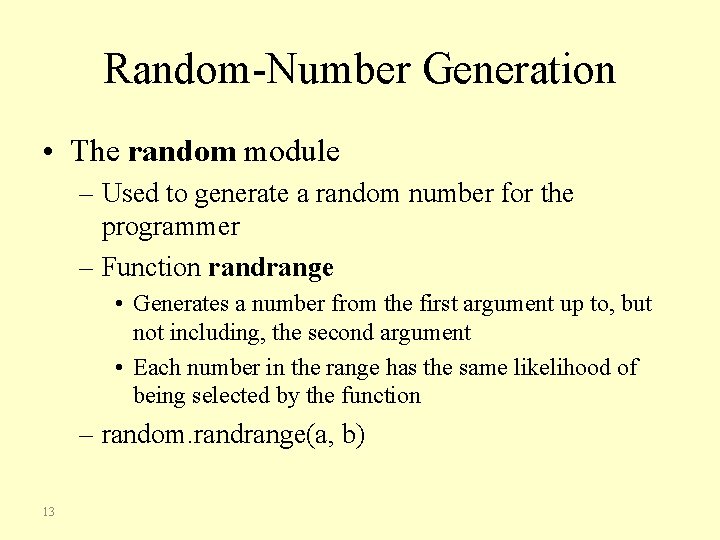
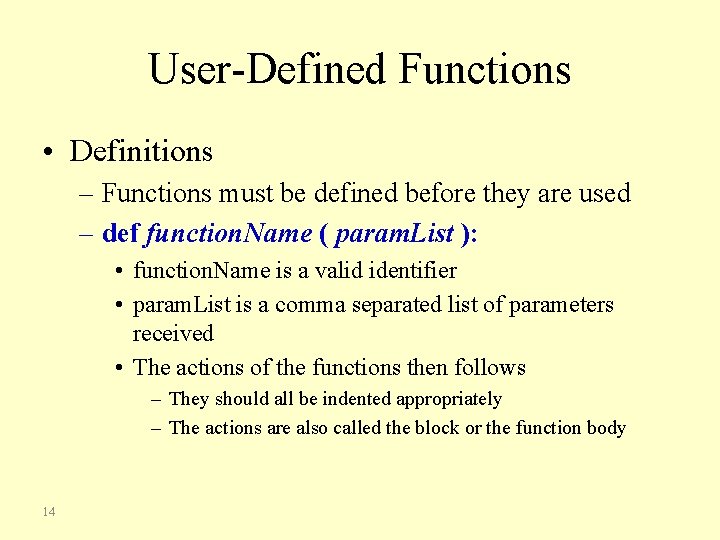
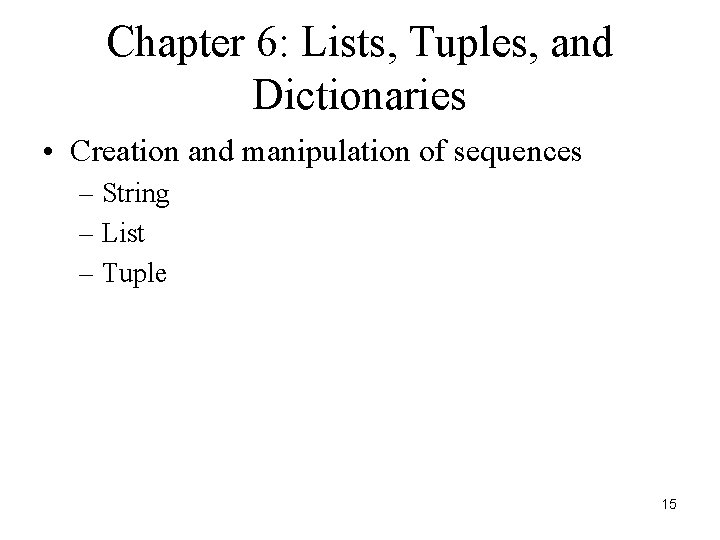
![Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0 Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0](https://slidetodoc.com/presentation_image_h2/cdceccb5945645edb11f94d7da8007f5/image-16.jpg)
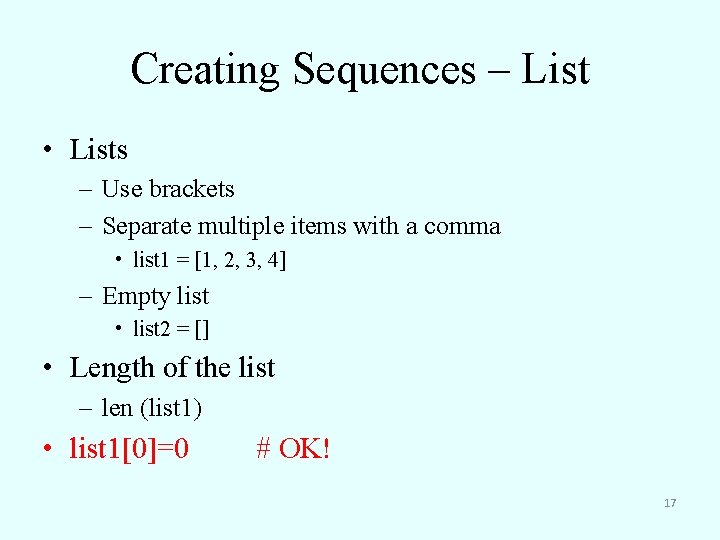
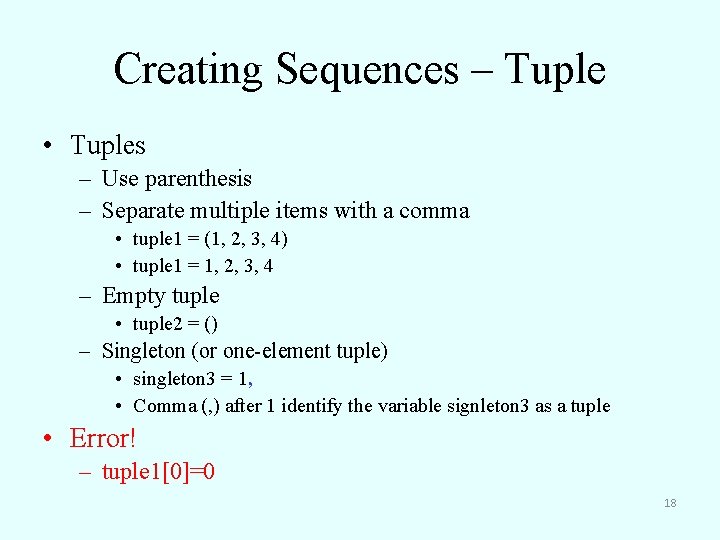
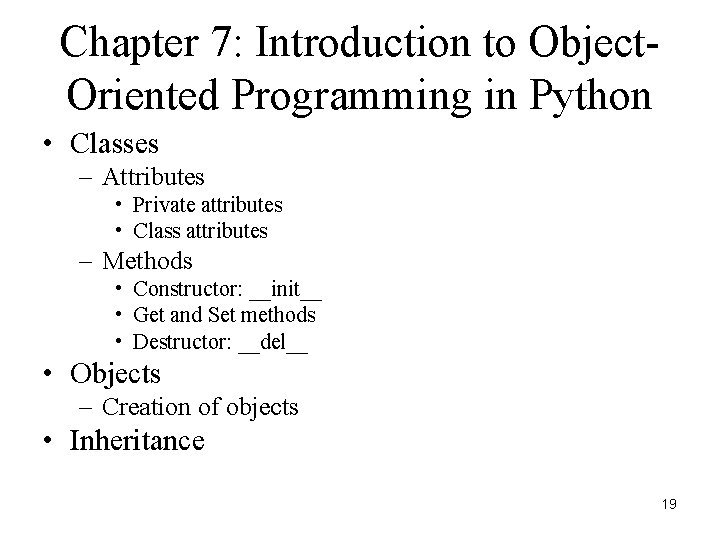
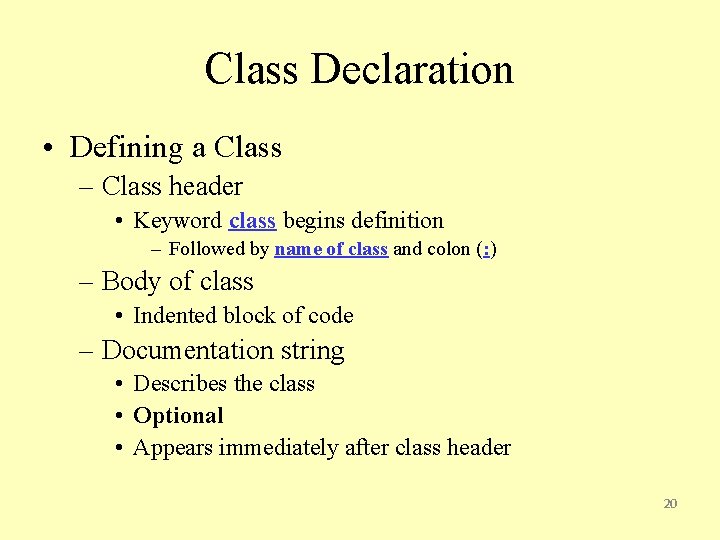
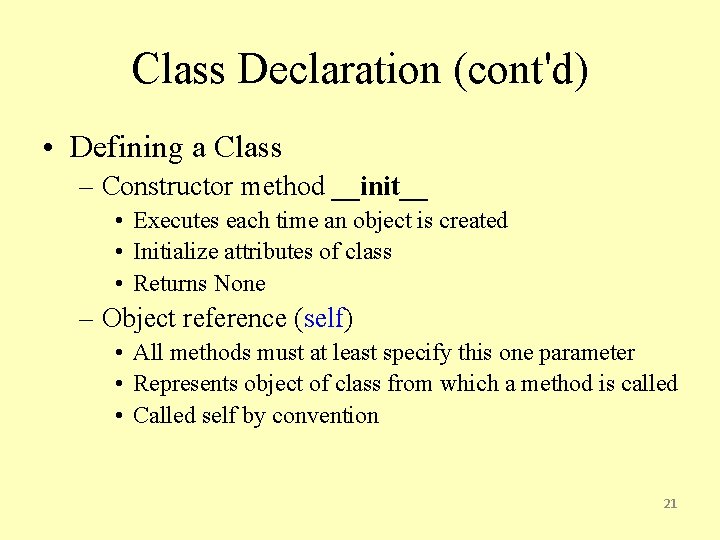
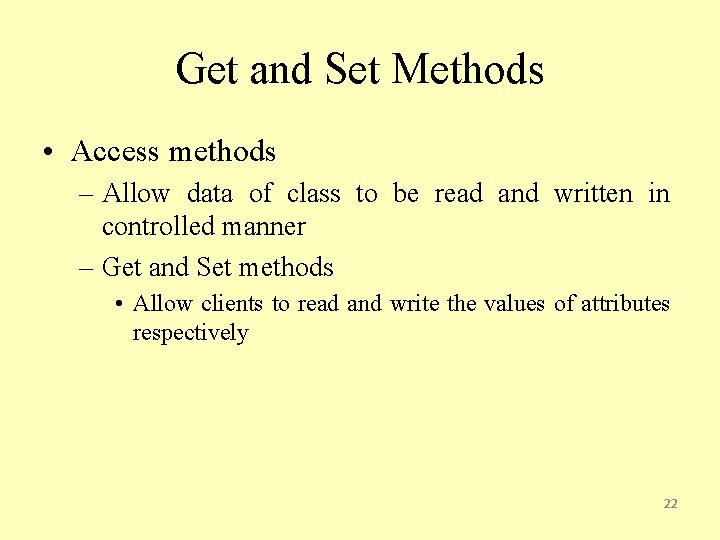
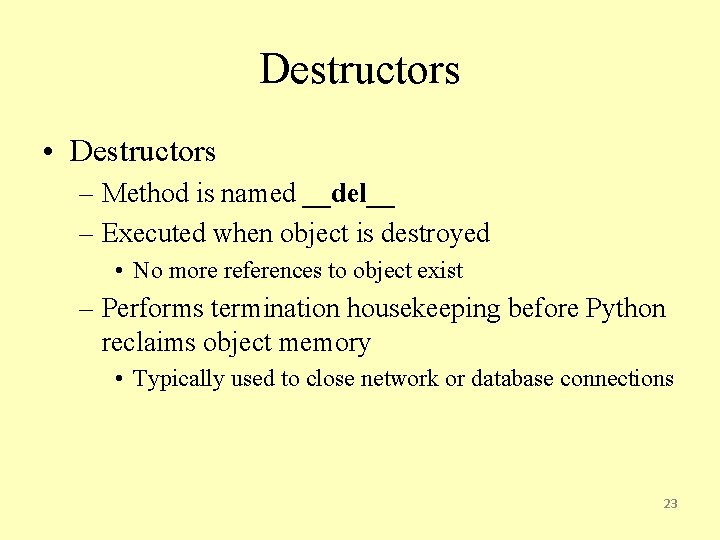
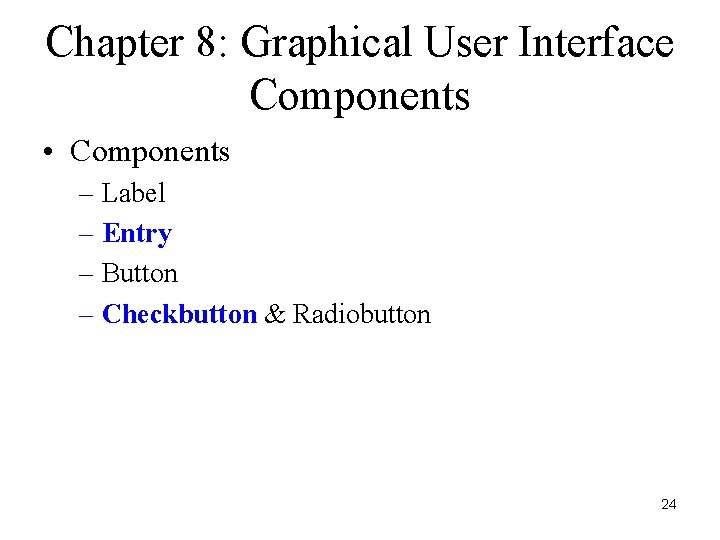
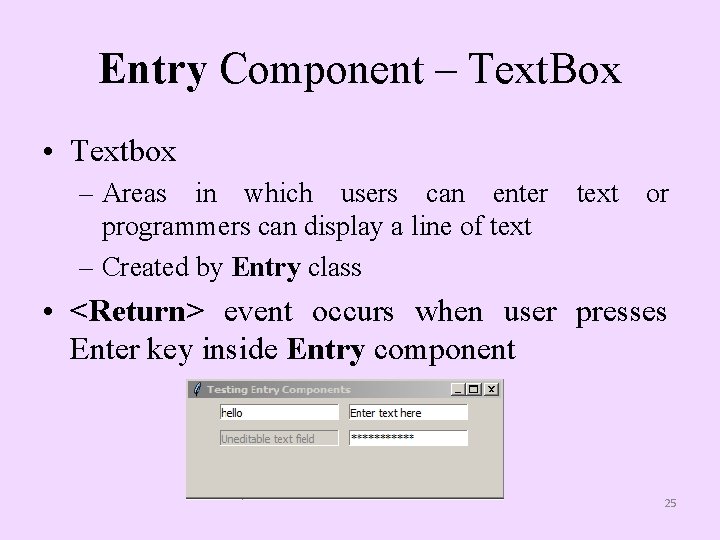
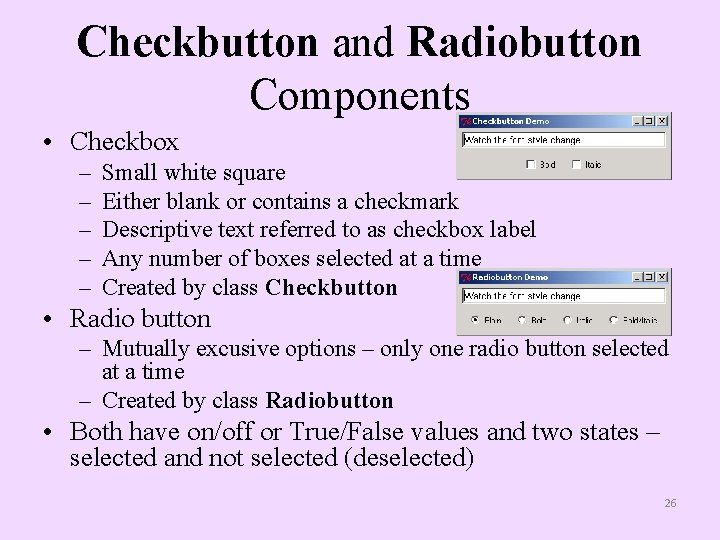
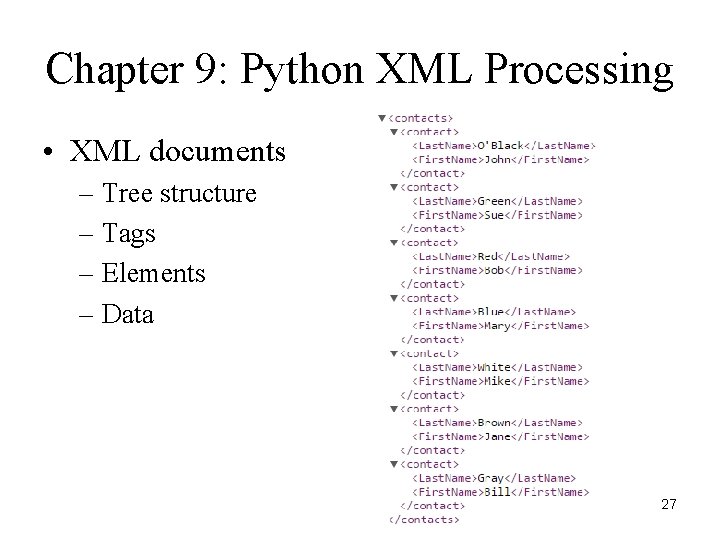
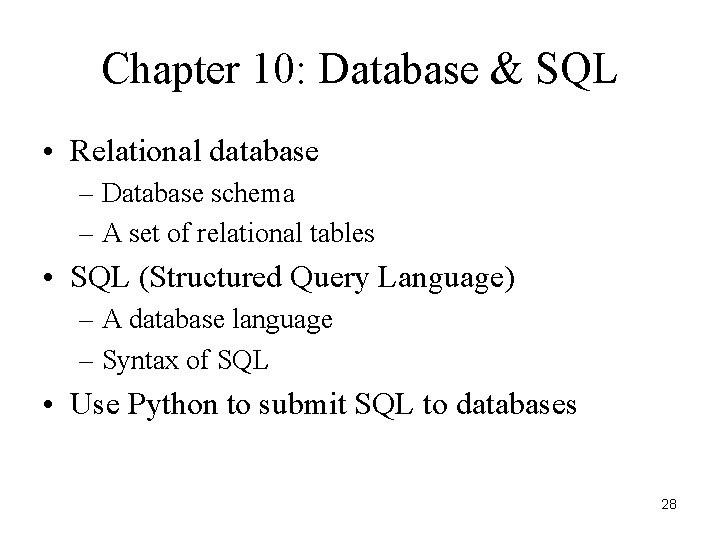
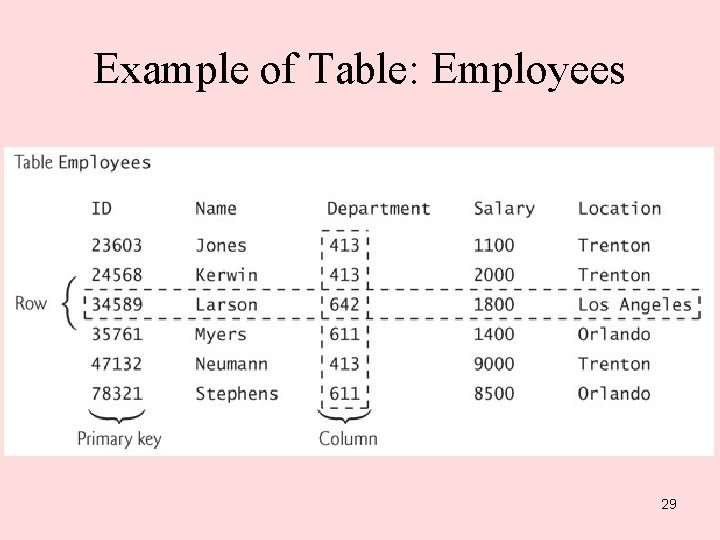
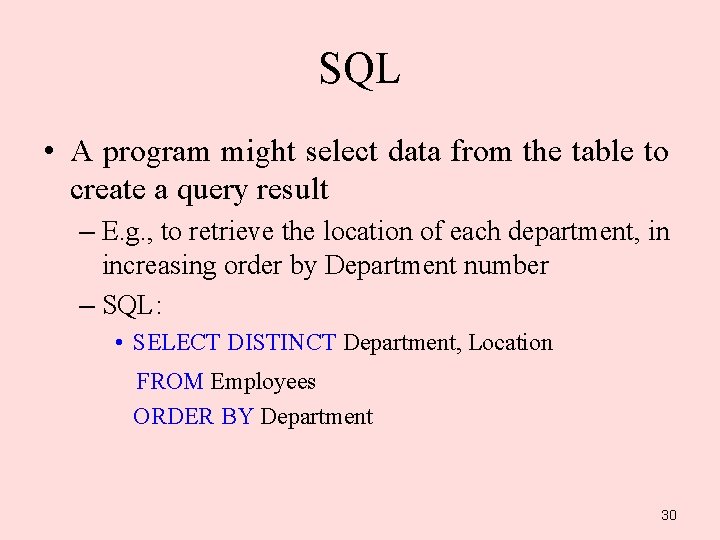
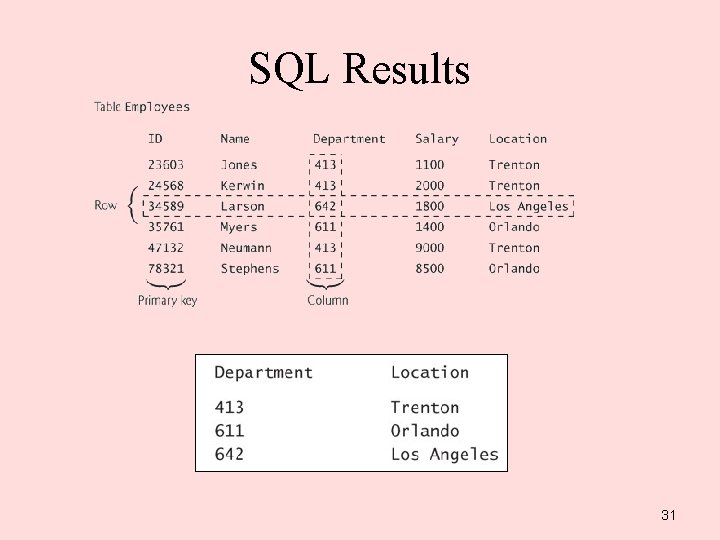
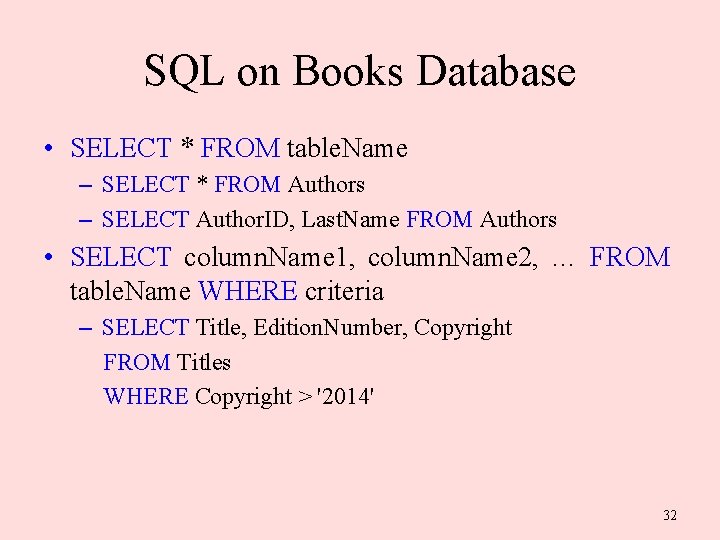
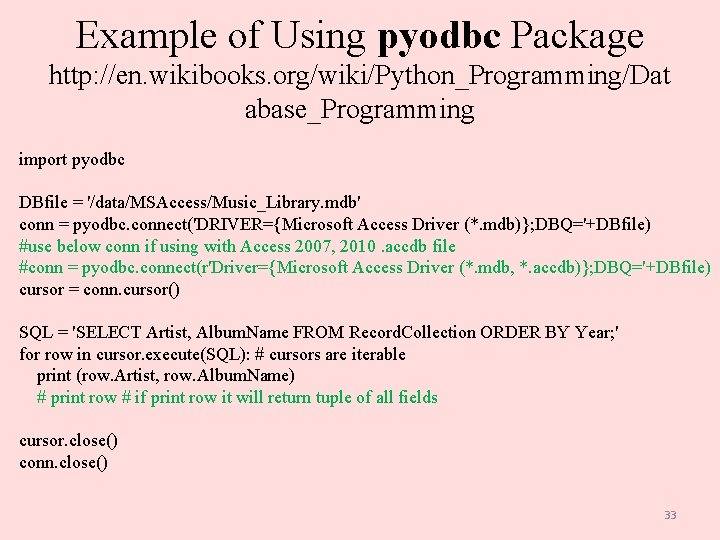
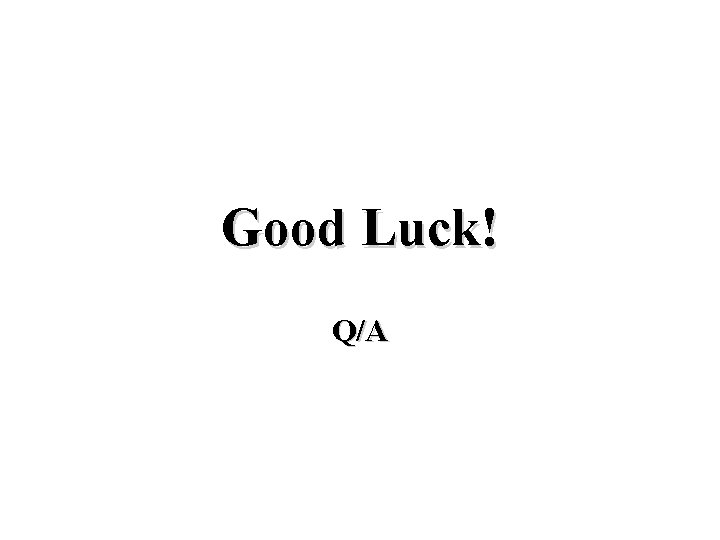
- Slides: 34
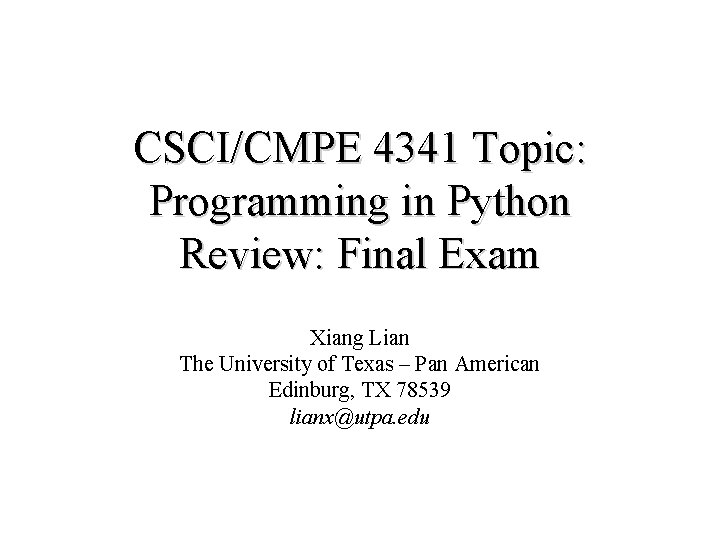
CSCI/CMPE 4341 Topic: Programming in Python Review: Final Exam Xiang Lian The University of Texas – Pan American Edinburg, TX 78539 lianx@utpa. edu
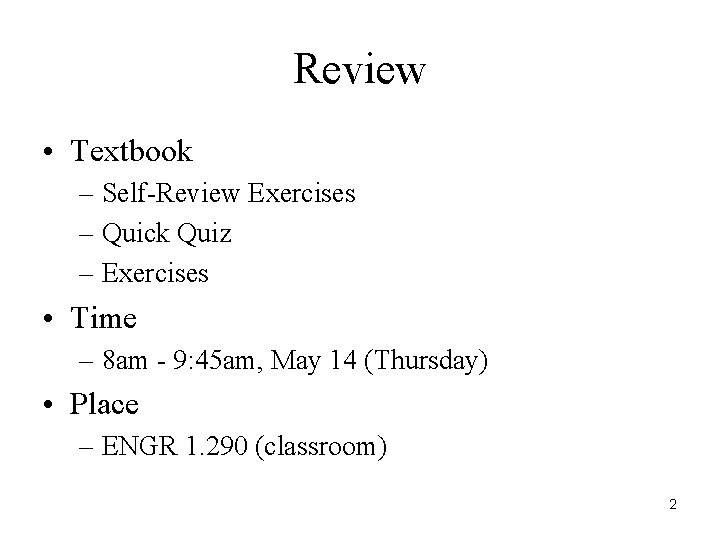
Review • Textbook – Self-Review Exercises – Quick Quiz – Exercises • Time – 8 am - 9: 45 am, May 14 (Thursday) • Place – ENGR 1. 290 (classroom) 2
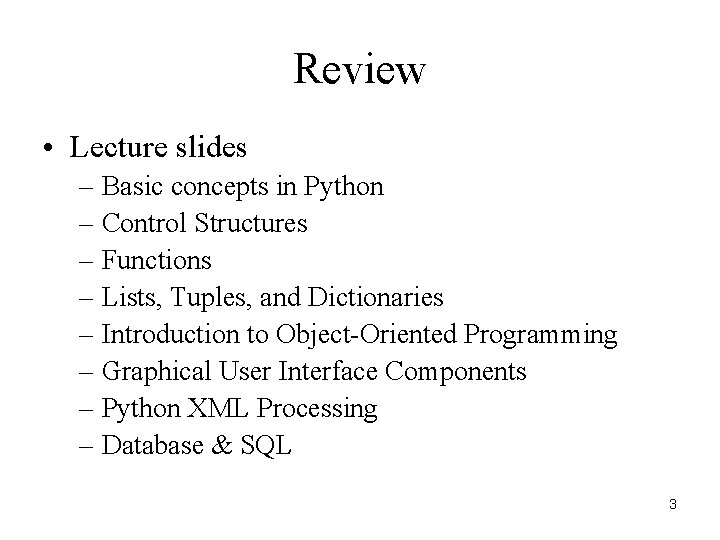
Review • Lecture slides – Basic concepts in Python – Control Structures – Functions – Lists, Tuples, and Dictionaries – Introduction to Object-Oriented Programming – Graphical User Interface Components – Python XML Processing – Database & SQL 3
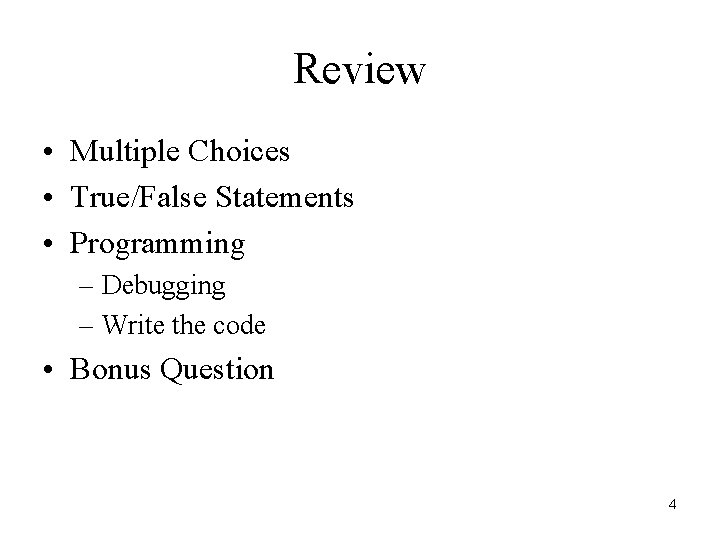
Review • Multiple Choices • True/False Statements • Programming – Debugging – Write the code • Bonus Question 4
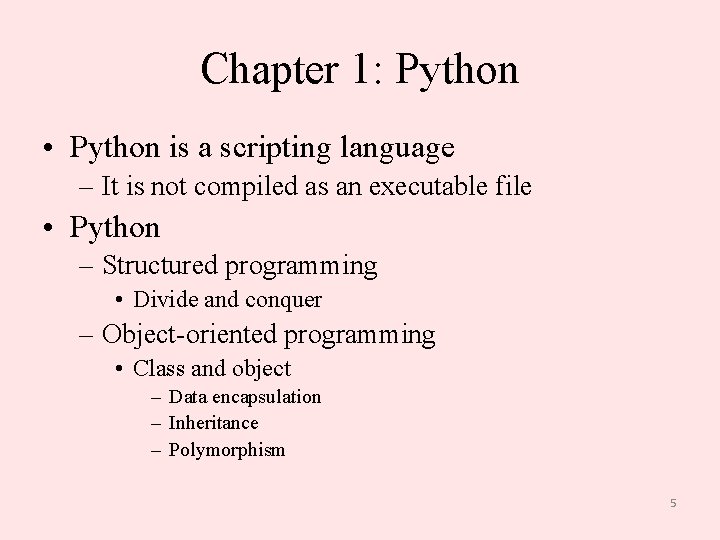
Chapter 1: Python • Python is a scripting language – It is not compiled as an executable file • Python – Structured programming • Divide and conquer – Object-oriented programming • Class and object – Data encapsulation – Inheritance – Polymorphism 5
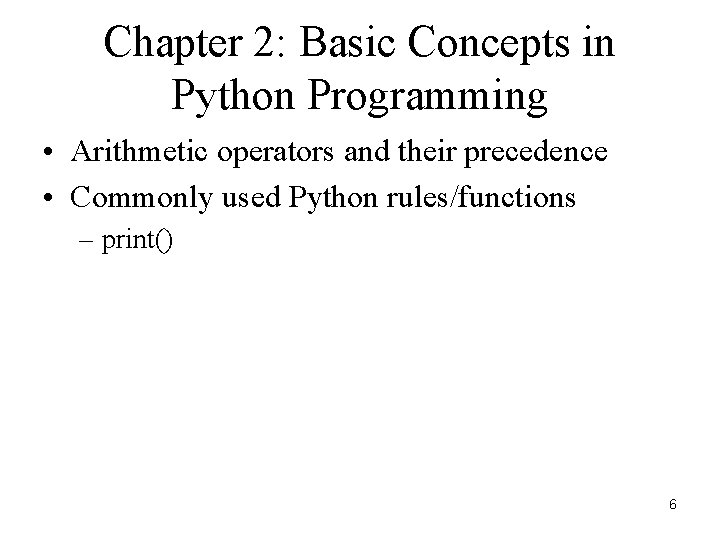
Chapter 2: Basic Concepts in Python Programming • Arithmetic operators and their precedence • Commonly used Python rules/functions – print() 6
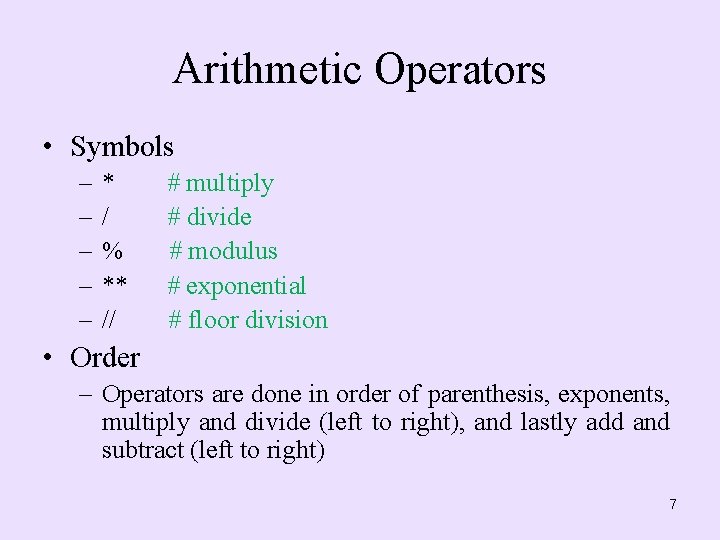
Arithmetic Operators • Symbols – – – * / % ** // # multiply # divide # modulus # exponential # floor division • Order – Operators are done in order of parenthesis, exponents, multiply and divide (left to right), and lastly add and subtract (left to right) 7
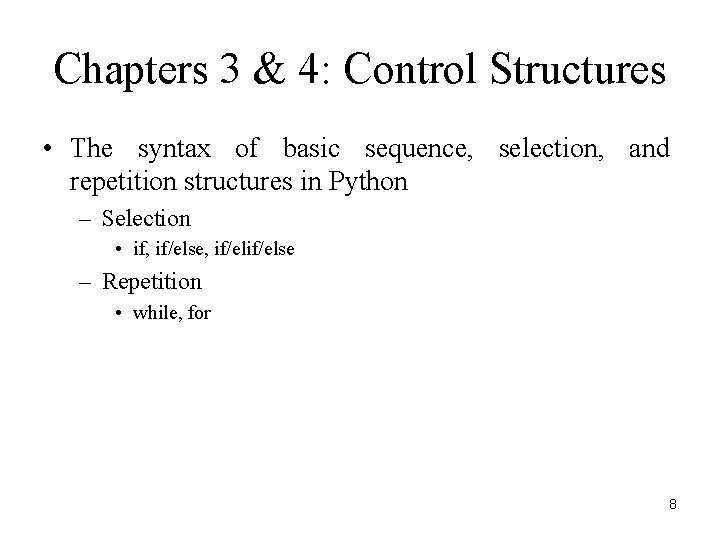
Chapters 3 & 4: Control Structures • The syntax of basic sequence, selection, and repetition structures in Python – Selection • if, if/else, if/else – Repetition • while, for 8
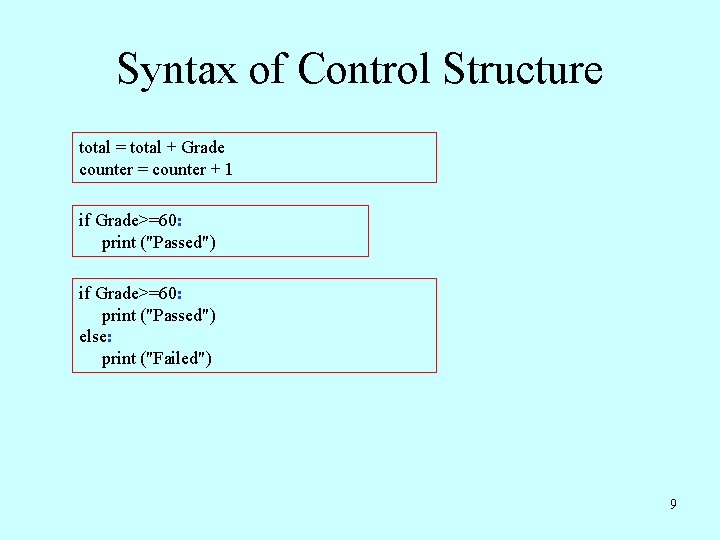
Syntax of Control Structure total = total + Grade counter = counter + 1 if Grade>=60: print ("Passed") else: print ("Failed") 9
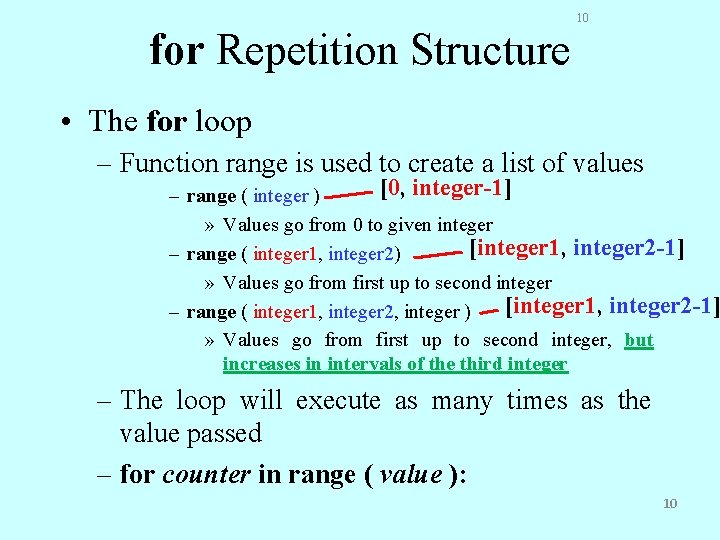
for Repetition Structure 10 • The for loop – Function range is used to create a list of values [0, integer-1] – range ( integer ) » Values go from 0 to given integer [integer 1, integer 2 -1] – range ( integer 1, integer 2) » Values go from first up to second integer [integer 1, integer 2 -1] – range ( integer 1, integer 2, integer ) » Values go from first up to second integer, but increases in intervals of the third integer – The loop will execute as many times as the value passed – for counter in range ( value ): 10
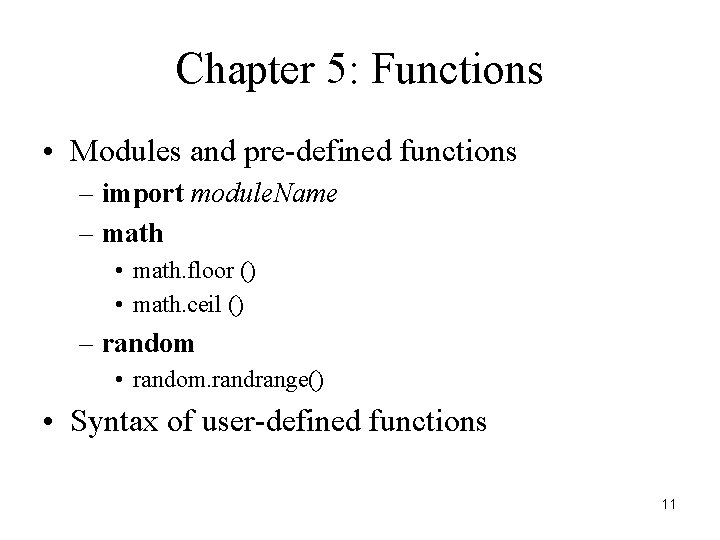
Chapter 5: Functions • Modules and pre-defined functions – import module. Name – math • math. floor () • math. ceil () – random • random. randrange() • Syntax of user-defined functions 11
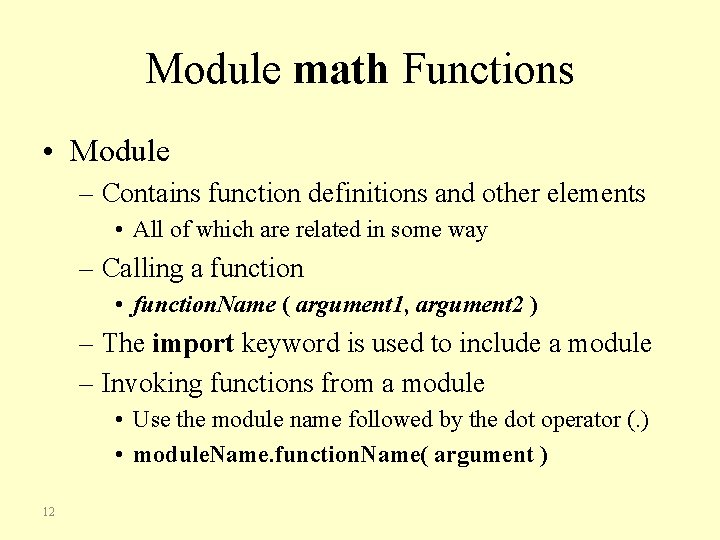
Module math Functions • Module – Contains function definitions and other elements • All of which are related in some way – Calling a function • function. Name ( argument 1, argument 2 ) – The import keyword is used to include a module – Invoking functions from a module • Use the module name followed by the dot operator (. ) • module. Name. function. Name( argument ) 12
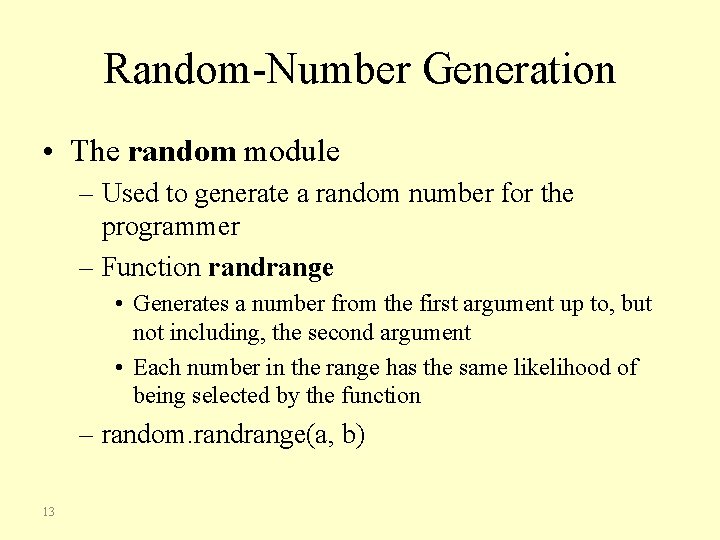
Random-Number Generation • The random module – Used to generate a random number for the programmer – Function randrange • Generates a number from the first argument up to, but not including, the second argument • Each number in the range has the same likelihood of being selected by the function – random. randrange(a, b) 13
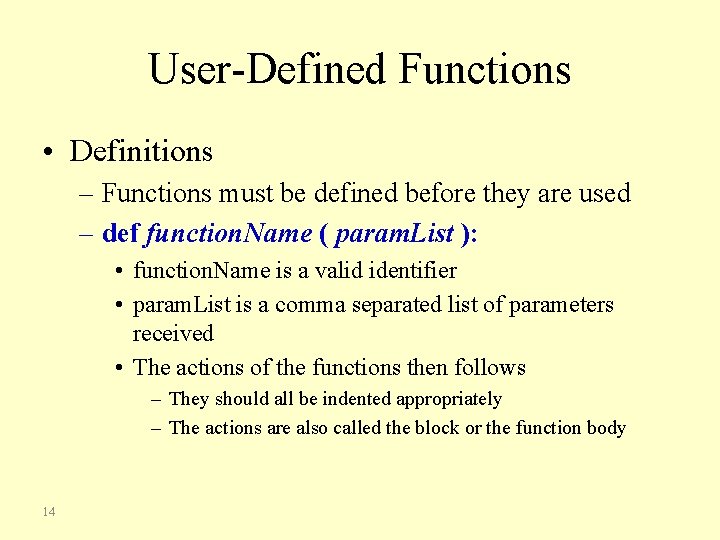
User-Defined Functions • Definitions – Functions must be defined before they are used – def function. Name ( param. List ): • function. Name is a valid identifier • param. List is a comma separated list of parameters received • The actions of the functions then follows – They should all be indented appropriately – The actions are also called the block or the function body 14
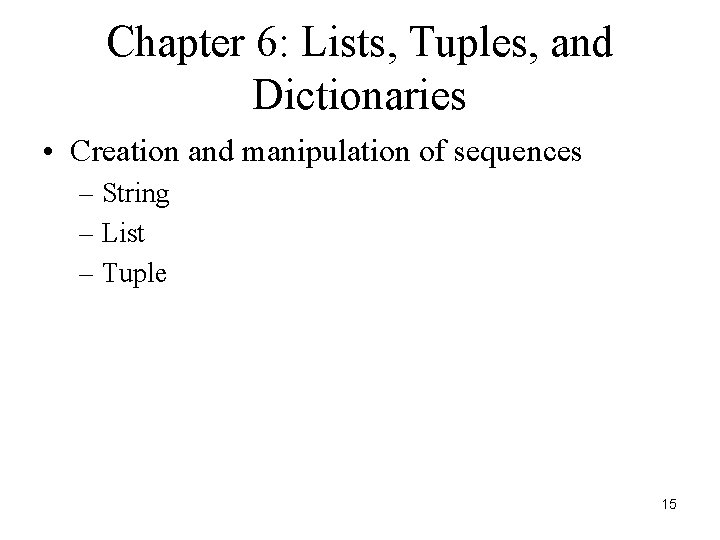
Chapter 6: Lists, Tuples, and Dictionaries • Creation and manipulation of sequences – String – List – Tuple 15
![Example of Sequences Name sequence C C0 45 C12 C1 6 C11 C2 0 Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0](https://slidetodoc.com/presentation_image_h2/cdceccb5945645edb11f94d7da8007f5/image-16.jpg)
Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0 C[-10] C[3] 72 C[-9] C[4] 34 C[-8] C[5] 39 C[-7] C[6] 98 C[-6] C[7] -1345 C[-5] C[8] 939 C[-4] 10 C[-3] 40 C[-2] 33 C[-1] Position number of C[9] the element within C[10] sequence C C[11] 16
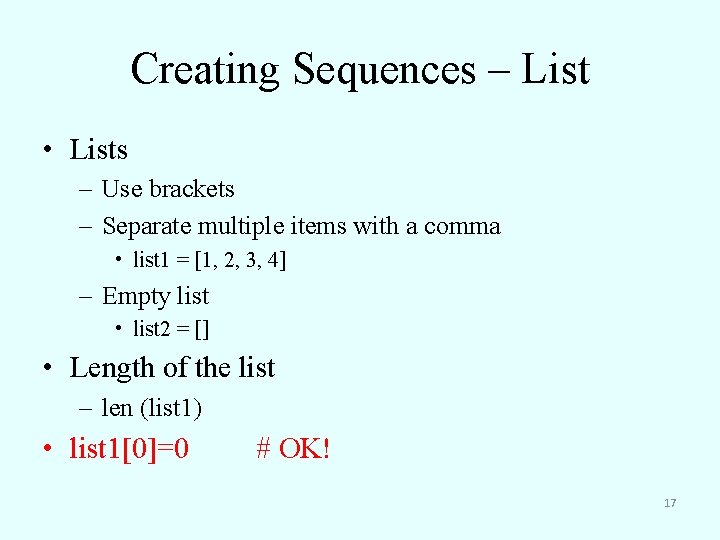
Creating Sequences – List • Lists – Use brackets – Separate multiple items with a comma • list 1 = [1, 2, 3, 4] – Empty list • list 2 = [] • Length of the list – len (list 1) • list 1[0]=0 # OK! 17
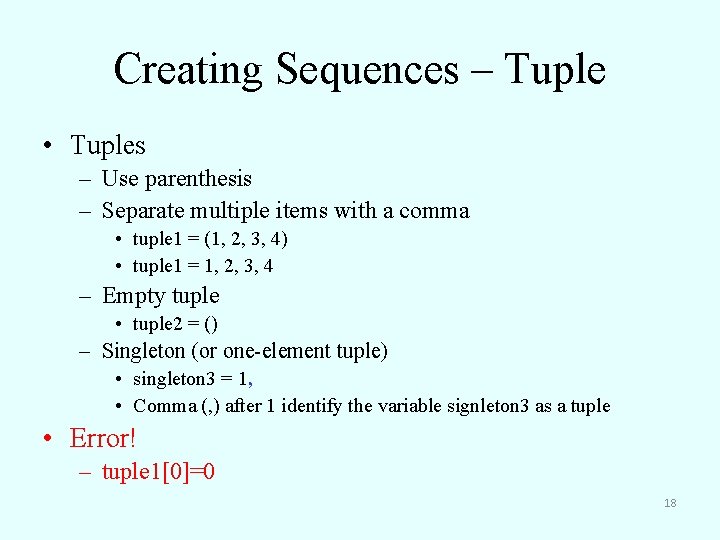
Creating Sequences – Tuple • Tuples – Use parenthesis – Separate multiple items with a comma • tuple 1 = (1, 2, 3, 4) • tuple 1 = 1, 2, 3, 4 – Empty tuple • tuple 2 = () – Singleton (or one-element tuple) • singleton 3 = 1, • Comma (, ) after 1 identify the variable signleton 3 as a tuple • Error! – tuple 1[0]=0 18
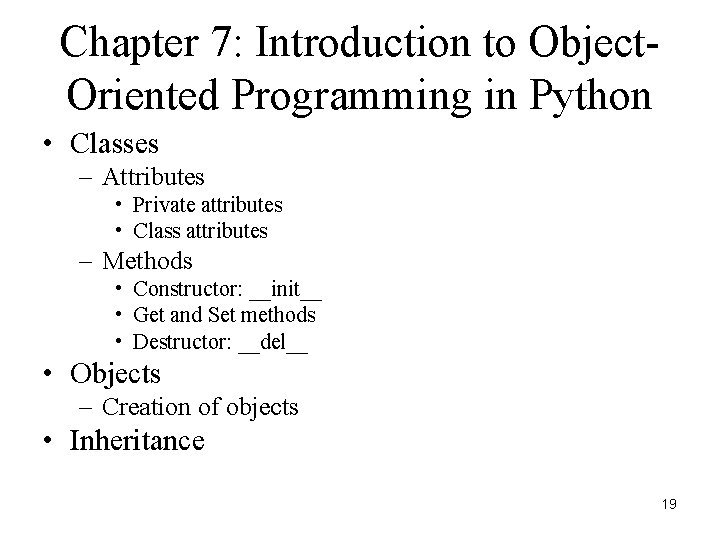
Chapter 7: Introduction to Object. Oriented Programming in Python • Classes – Attributes • Private attributes • Class attributes – Methods • Constructor: __init__ • Get and Set methods • Destructor: __del__ • Objects – Creation of objects • Inheritance 19
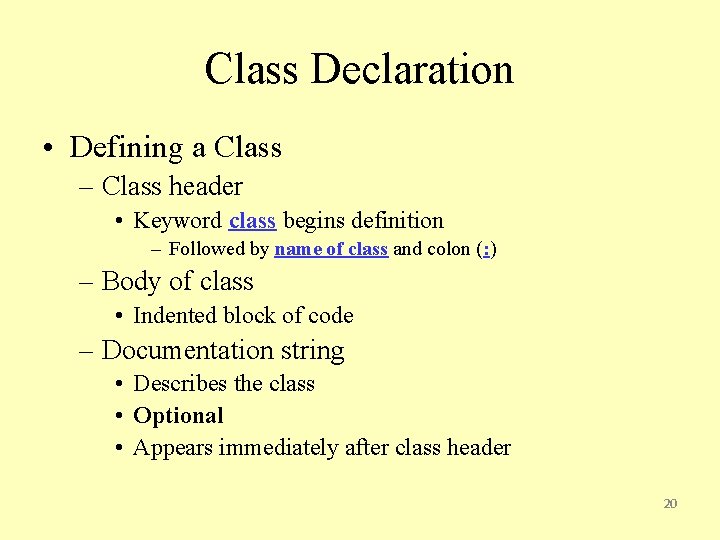
Class Declaration • Defining a Class – Class header • Keyword class begins definition – Followed by name of class and colon (: ) – Body of class • Indented block of code – Documentation string • Describes the class • Optional • Appears immediately after class header 20
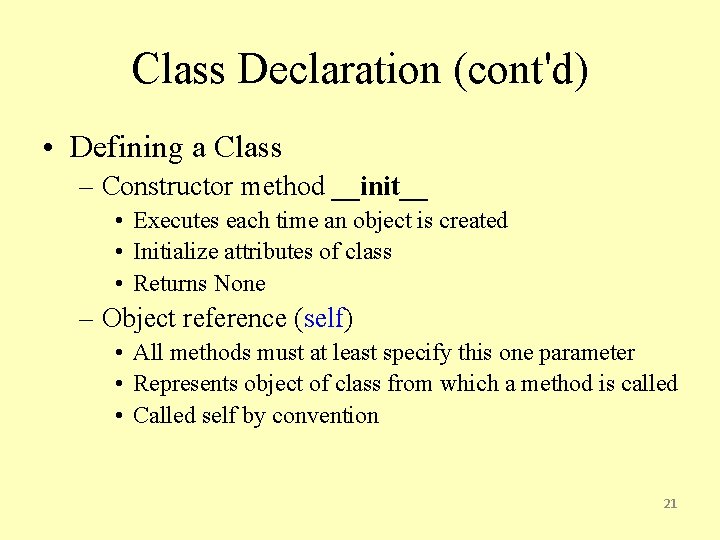
Class Declaration (cont'd) • Defining a Class – Constructor method __init__ • Executes each time an object is created • Initialize attributes of class • Returns None – Object reference (self) • All methods must at least specify this one parameter • Represents object of class from which a method is called • Called self by convention 21
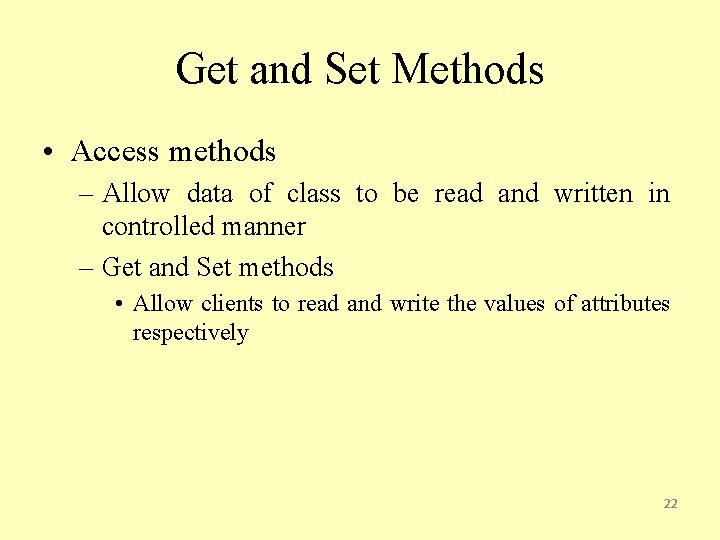
Get and Set Methods • Access methods – Allow data of class to be read and written in controlled manner – Get and Set methods • Allow clients to read and write the values of attributes respectively 22
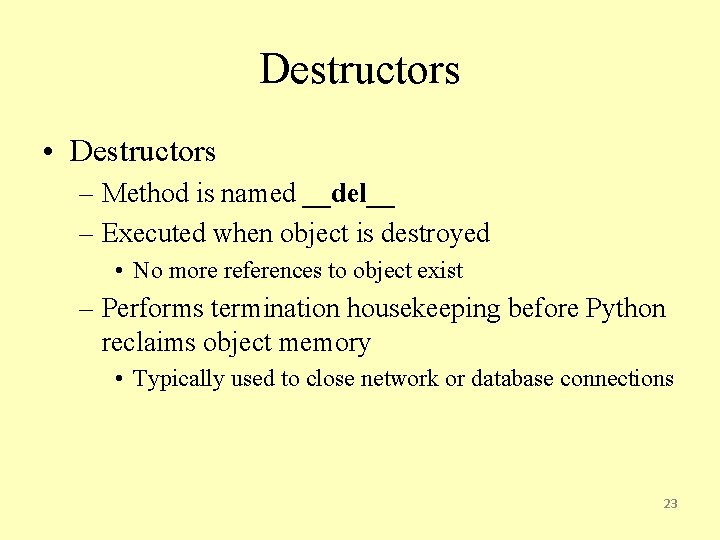
Destructors • Destructors – Method is named __del__ – Executed when object is destroyed • No more references to object exist – Performs termination housekeeping before Python reclaims object memory • Typically used to close network or database connections 23
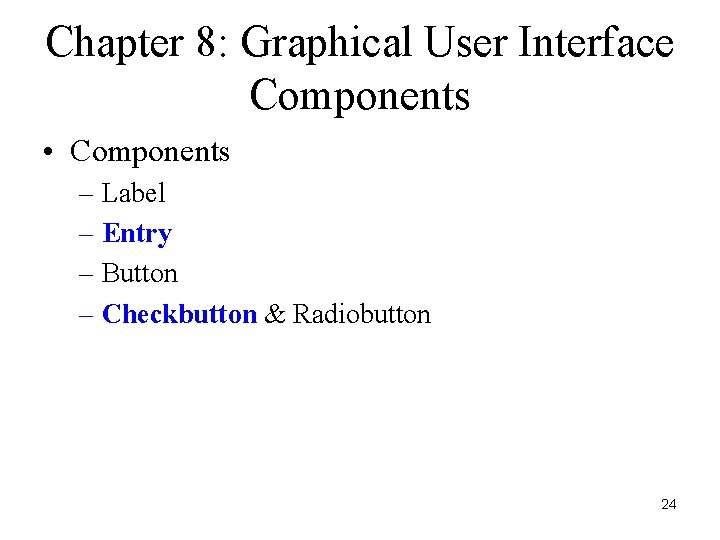
Chapter 8: Graphical User Interface Components • Components – Label – Entry – Button – Checkbutton & Radiobutton 24
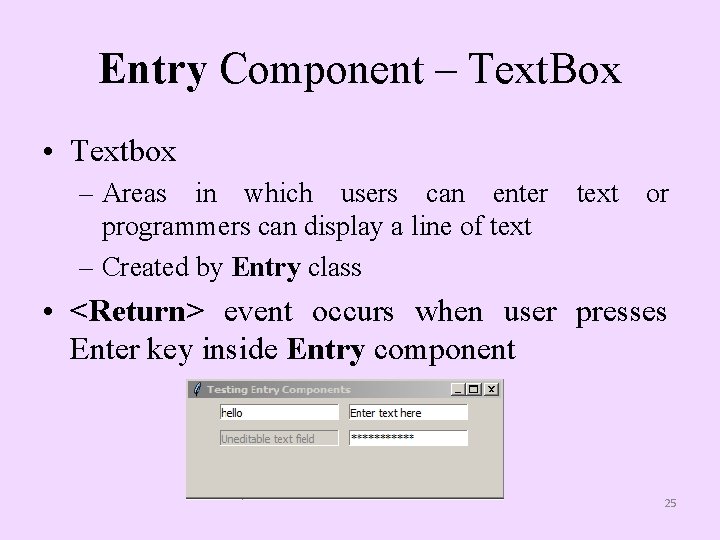
Entry Component – Text. Box • Textbox – Areas in which users can enter text or programmers can display a line of text – Created by Entry class • <Return> event occurs when user presses Enter key inside Entry component 25
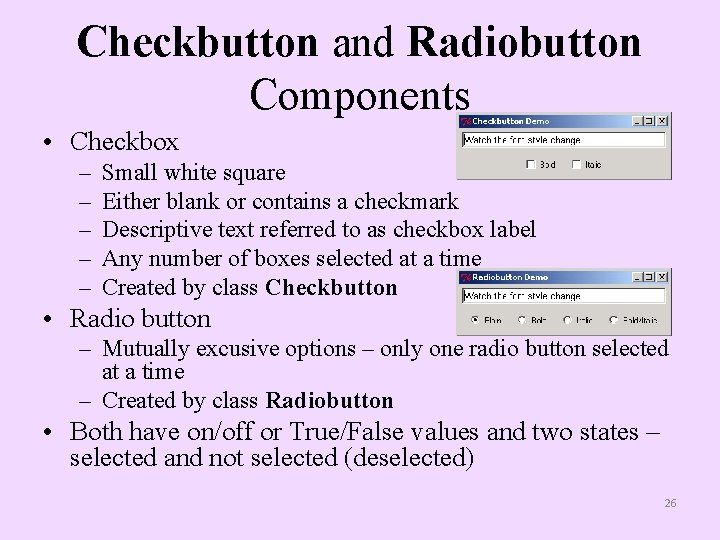
Checkbutton and Radiobutton Components • Checkbox – – – Small white square Either blank or contains a checkmark Descriptive text referred to as checkbox label Any number of boxes selected at a time Created by class Checkbutton • Radio button – Mutually excusive options – only one radio button selected at a time – Created by class Radiobutton • Both have on/off or True/False values and two states – selected and not selected (deselected) 26
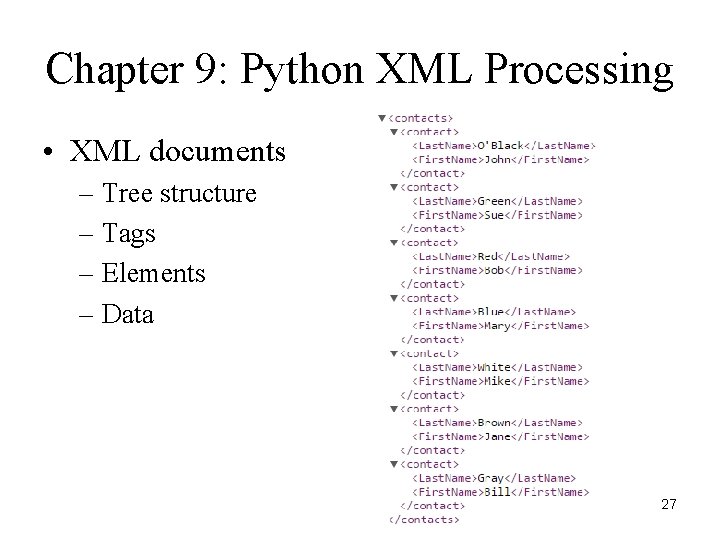
Chapter 9: Python XML Processing • XML documents – Tree structure – Tags – Elements – Data 27
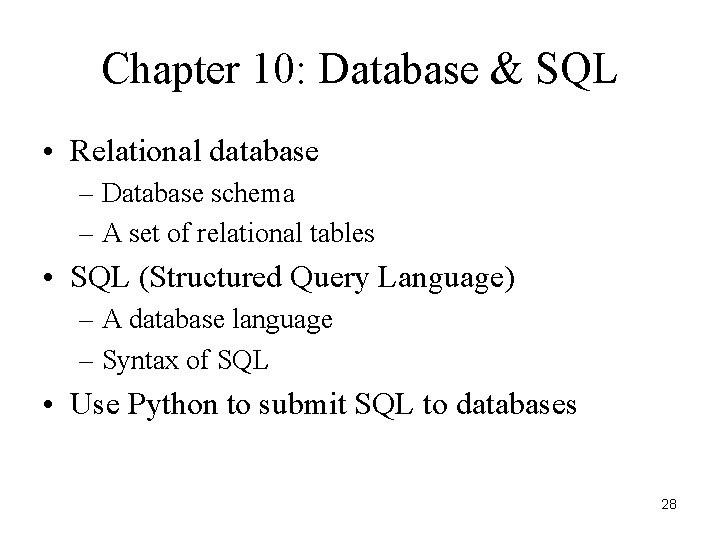
Chapter 10: Database & SQL • Relational database – Database schema – A set of relational tables • SQL (Structured Query Language) – A database language – Syntax of SQL • Use Python to submit SQL to databases 28
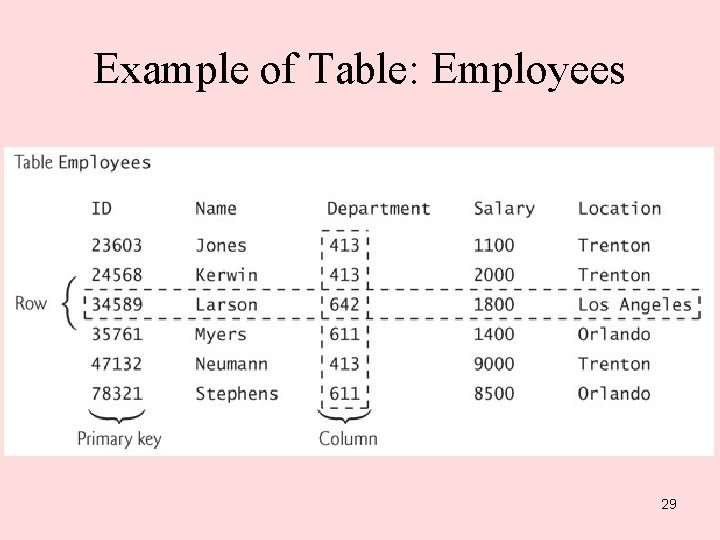
Example of Table: Employees 29
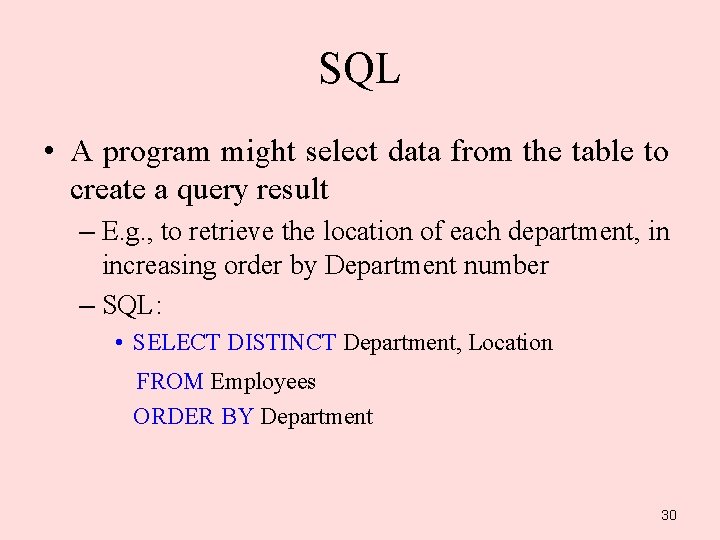
SQL • A program might select data from the table to create a query result – E. g. , to retrieve the location of each department, in increasing order by Department number – SQL: • SELECT DISTINCT Department, Location FROM Employees ORDER BY Department 30
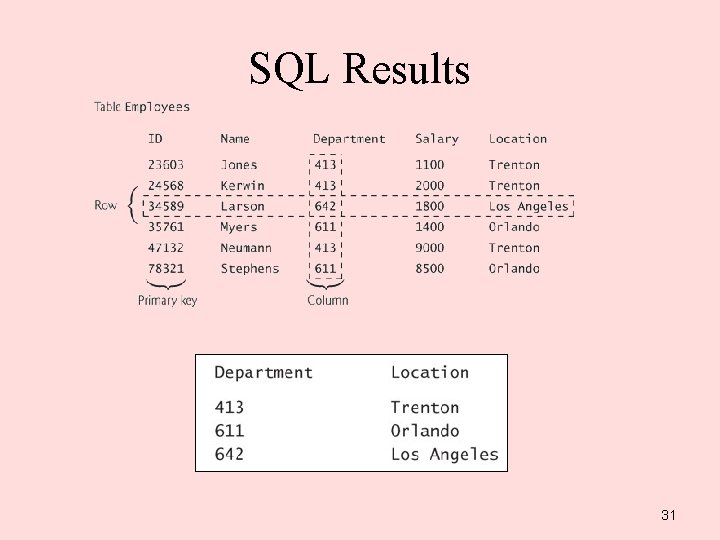
SQL Results 31
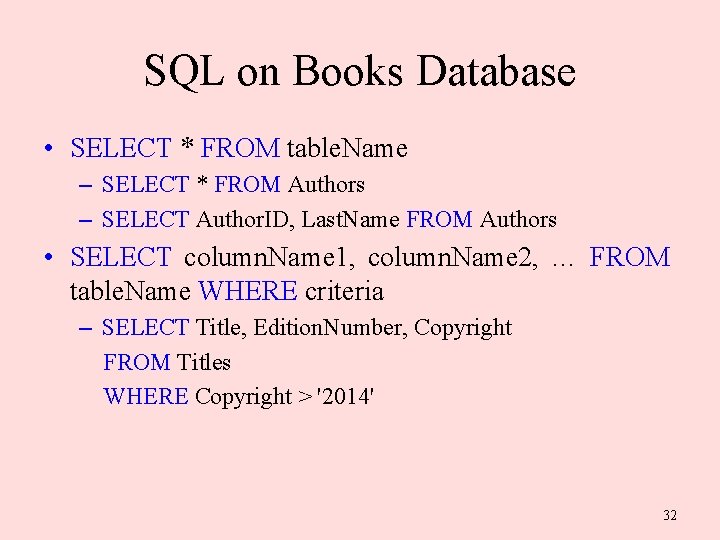
SQL on Books Database • SELECT * FROM table. Name – SELECT * FROM Authors – SELECT Author. ID, Last. Name FROM Authors • SELECT column. Name 1, column. Name 2, … FROM table. Name WHERE criteria – SELECT Title, Edition. Number, Copyright FROM Titles WHERE Copyright > '2014' 32
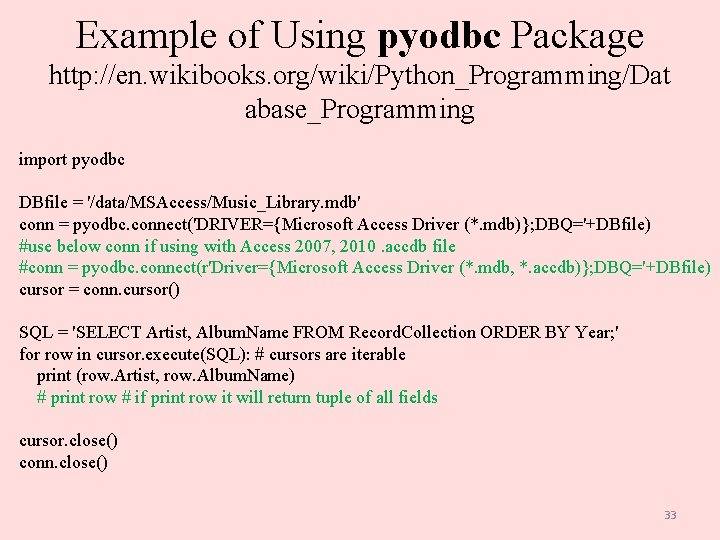
Example of Using pyodbc Package http: //en. wikibooks. org/wiki/Python_Programming/Dat abase_Programming import pyodbc DBfile = '/data/MSAccess/Music_Library. mdb' conn = pyodbc. connect('DRIVER={Microsoft Access Driver (*. mdb)}; DBQ='+DBfile) #use below conn if using with Access 2007, 2010. accdb file #conn = pyodbc. connect(r'Driver={Microsoft Access Driver (*. mdb, *. accdb)}; DBQ='+DBfile) cursor = conn. cursor() SQL = 'SELECT Artist, Album. Name FROM Record. Collection ORDER BY Year; ' for row in cursor. execute(SQL): # cursors are iterable print (row. Artist, row. Album. Name) # print row # if print row it will return tuple of all fields cursor. close() conn. close() 33
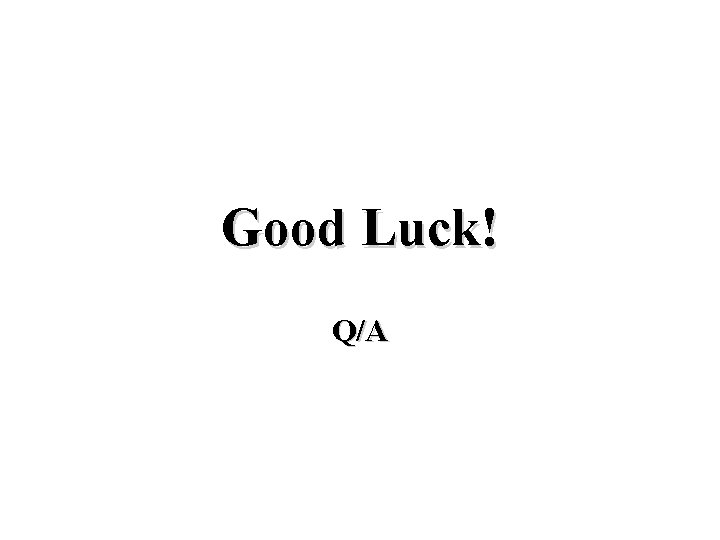
Good Luck! Q/A
Uncontrollable spending ap gov
Clincher sentence example
Tapic about internet
Python final project
Cs 1101 final
Cs-1101
Cs1101
Python procedural programming
Python chapter 5
Second order cone programming python
Python programming in context
Constraint programming python
Rapid gui programming with python and qt
Python programming in context
Cgi programming in python
Python audio programming
String python
Programming essentials in python
Python blackjack oop
Python programming an introduction to computer science
Perbedaan linear programming dan integer programming
Greedy vs dynamic
Definition of system programming
Integer programming vs linear programming
Definisi linear
Topic 5 new global connections
Transformations and congruence
Topic 3 review questions civilizations of asia answers
Topic 5 review
World history spring final exam review answers
World history final exam study guide
Spanish packet answers
Pltw human body systems final exam review
Poe practice test - materials answer key
Ied final exam review