CSCICMPE 4341 Topic Programming in Python Review Exam
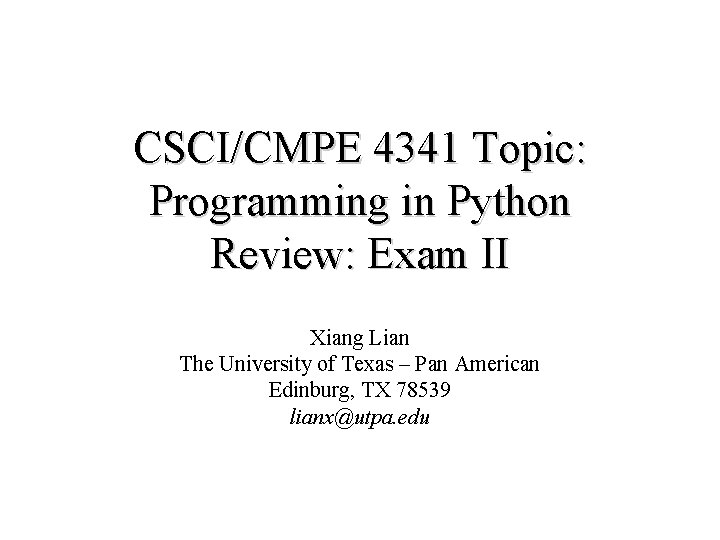
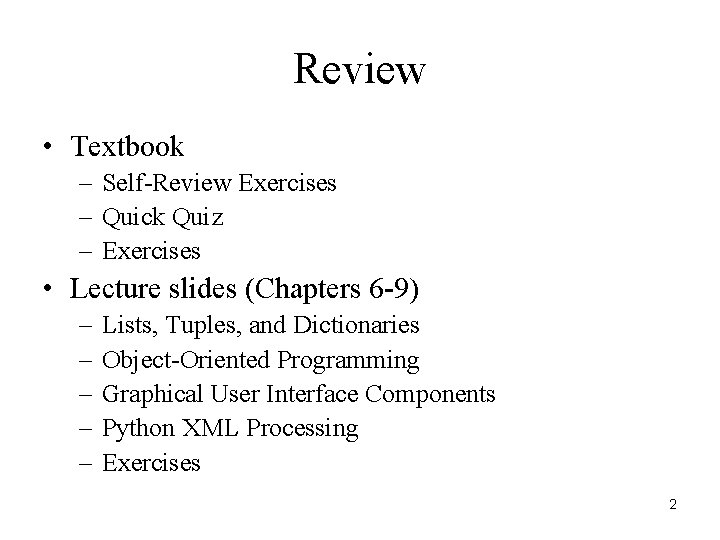
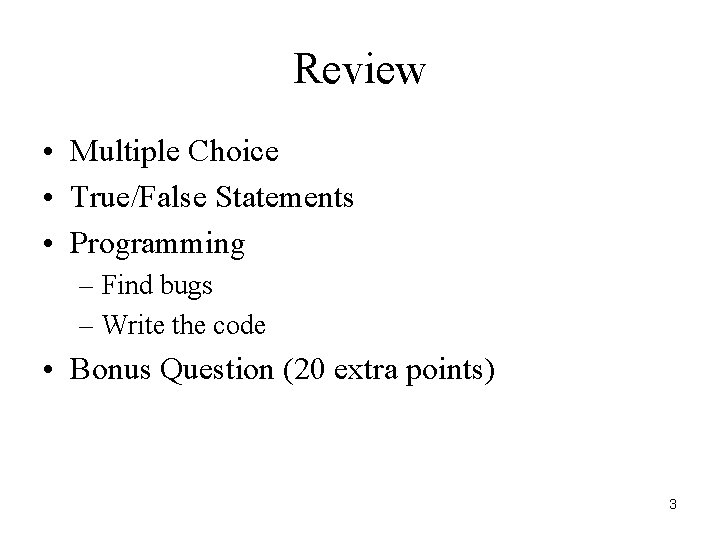
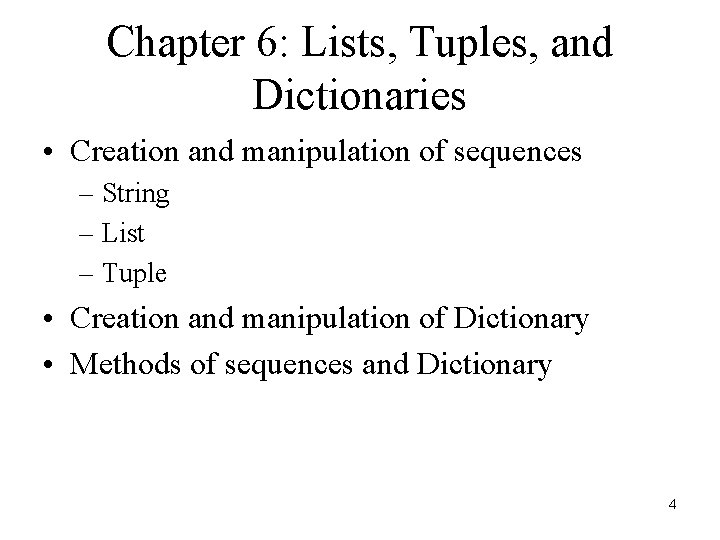
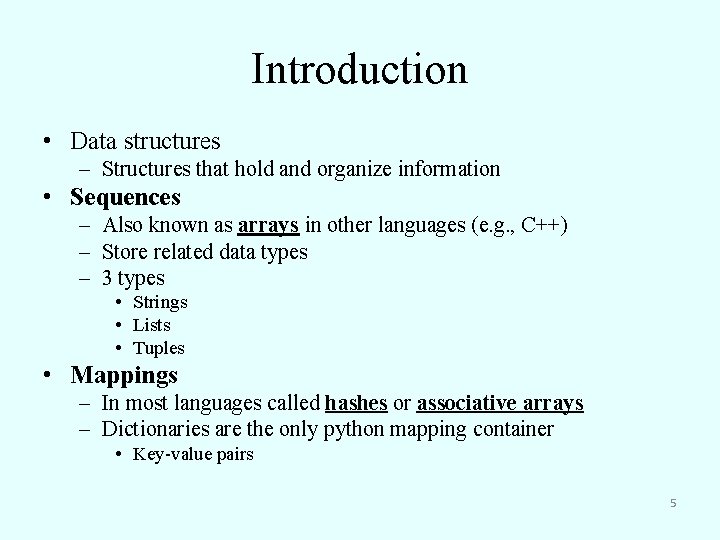
![Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0 Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0](https://slidetodoc.com/presentation_image_h/3284ce6250626bbcc9b71bad7d8606bb/image-6.jpg)
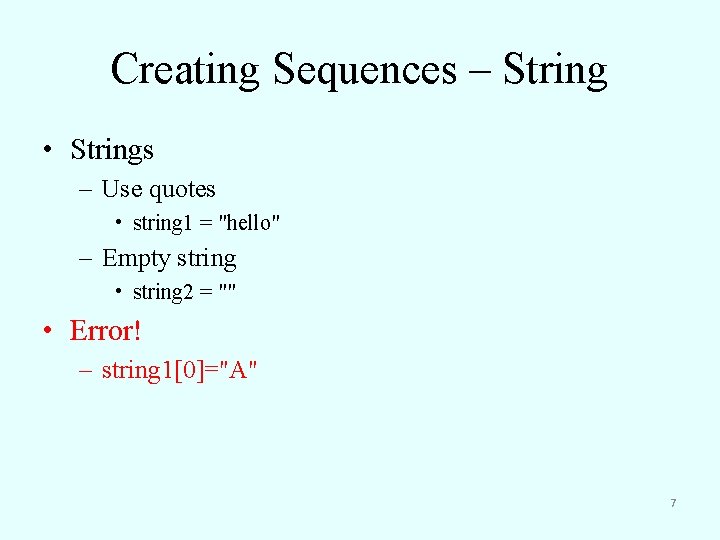
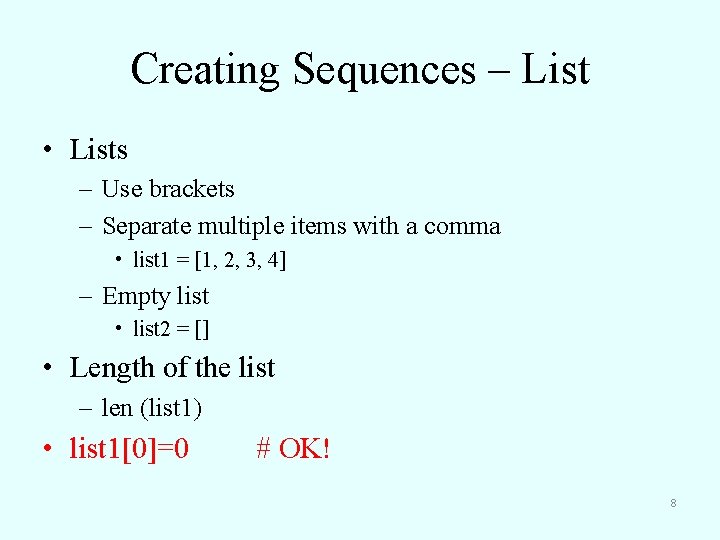
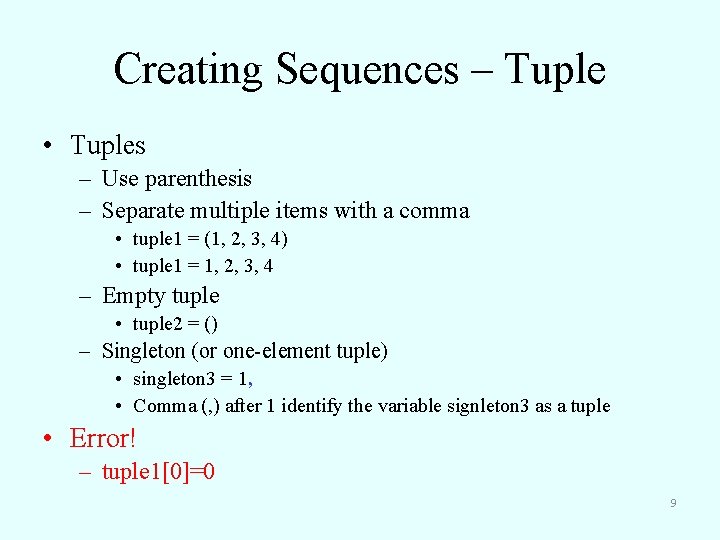
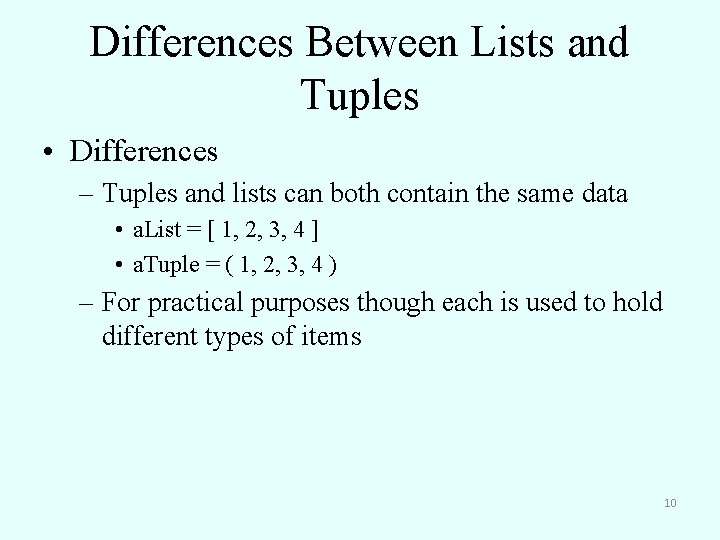
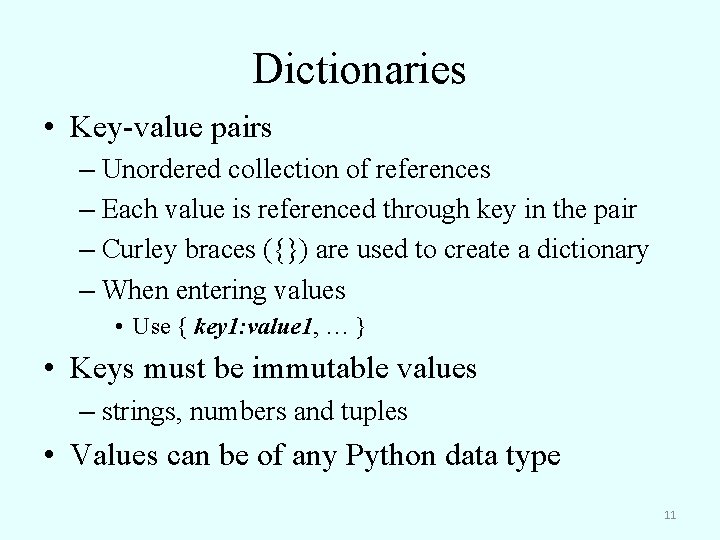
![Operations in Dictionaries • Access dictionary element – dictionary. Name [key] = value # Operations in Dictionaries • Access dictionary element – dictionary. Name [key] = value #](https://slidetodoc.com/presentation_image_h/3284ce6250626bbcc9b71bad7d8606bb/image-12.jpg)
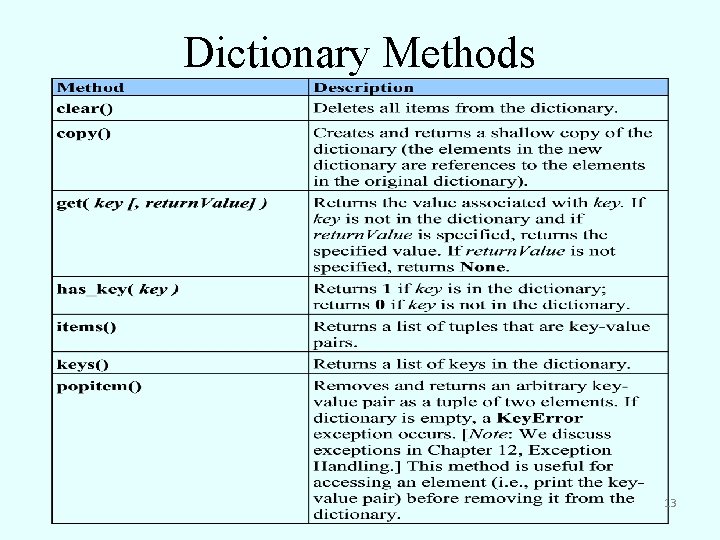
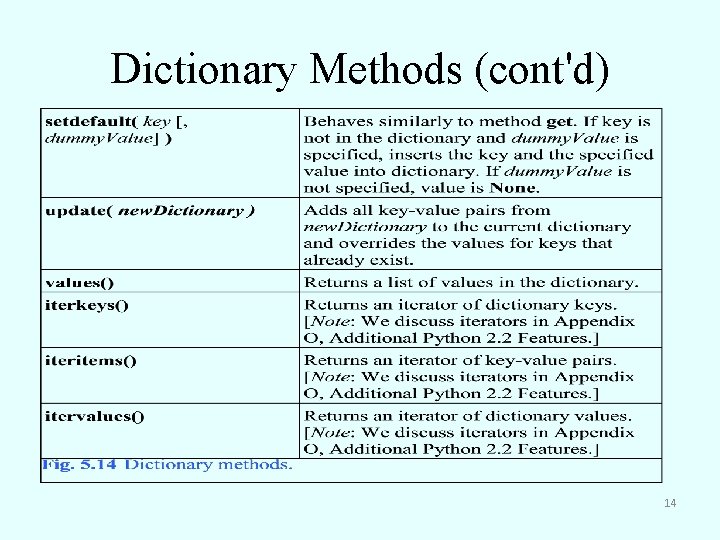
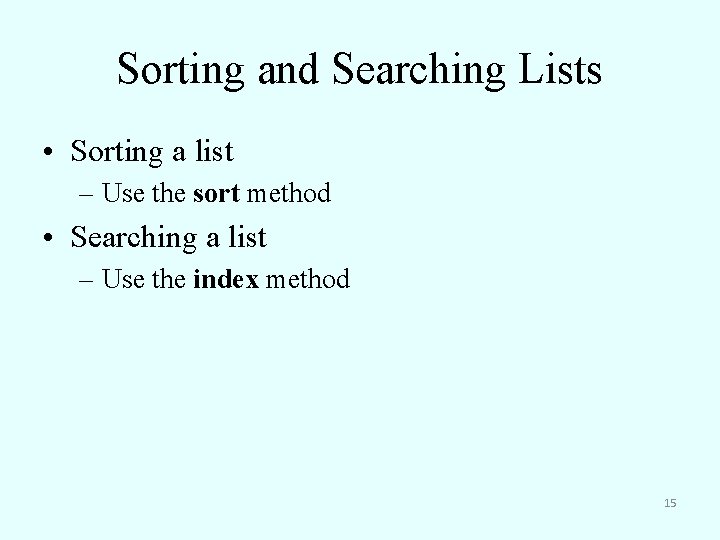
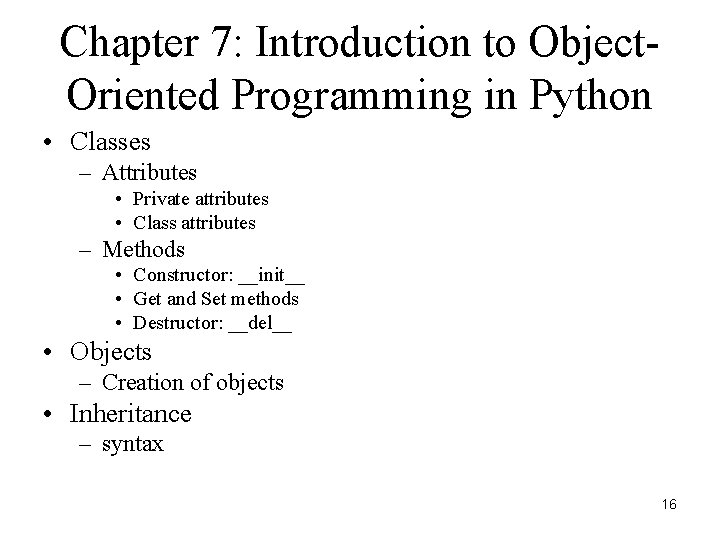
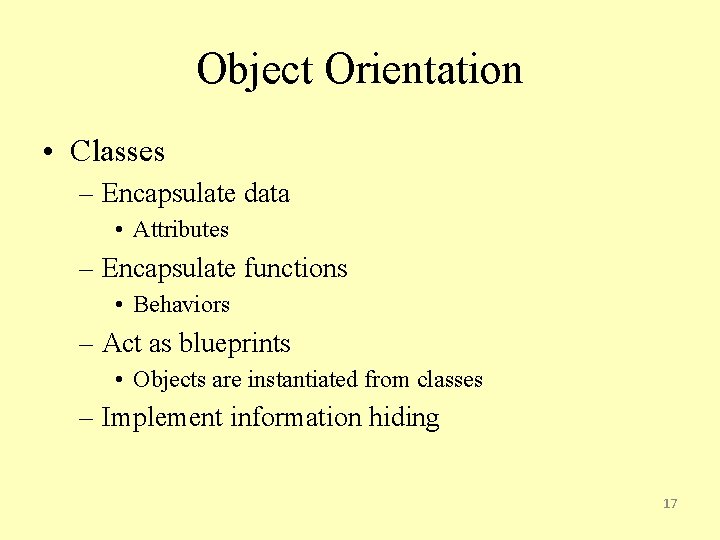
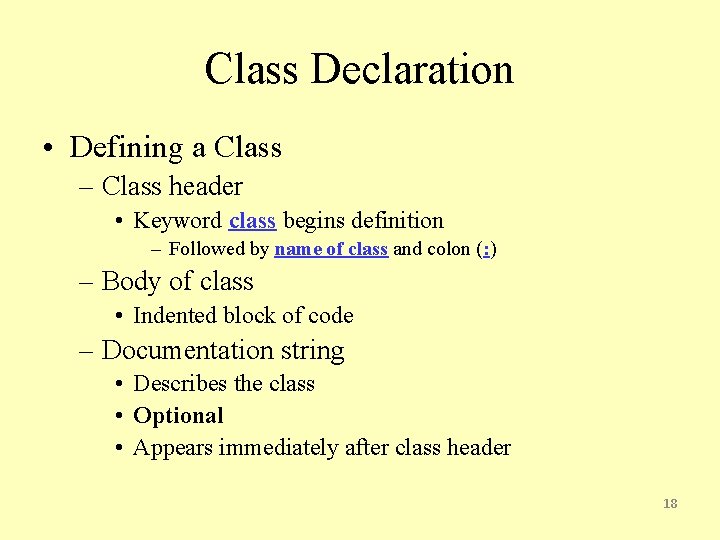
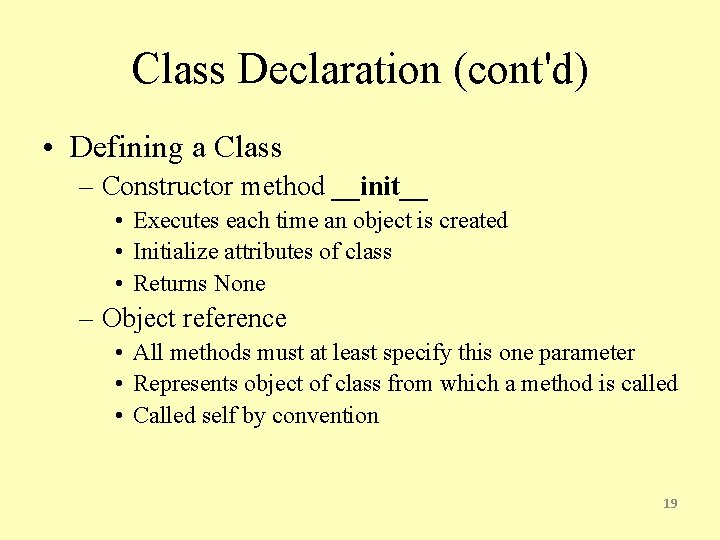
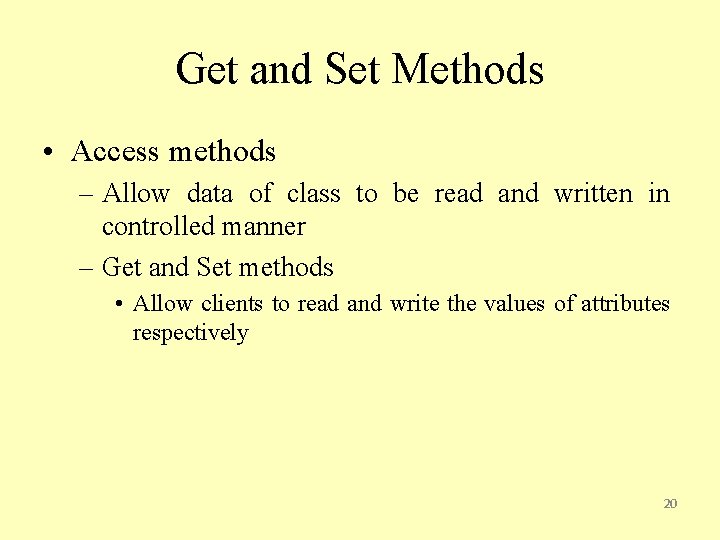
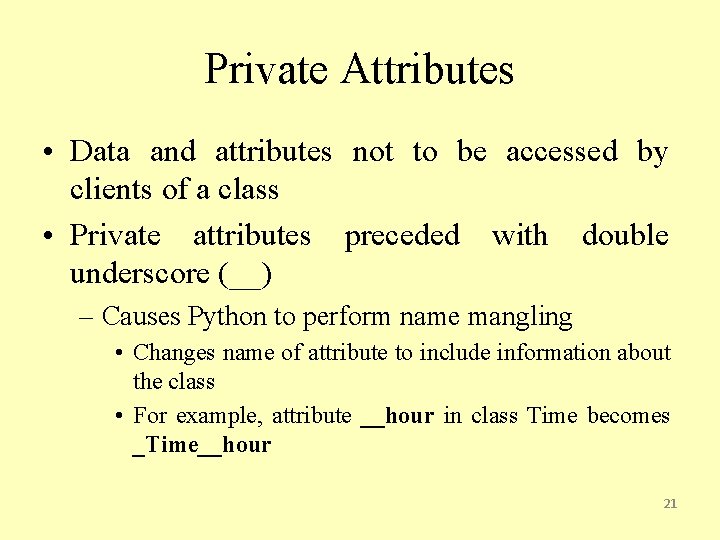
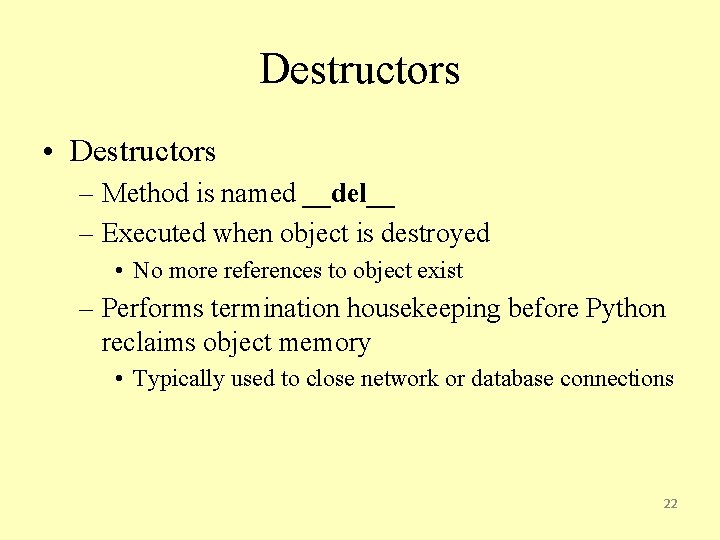
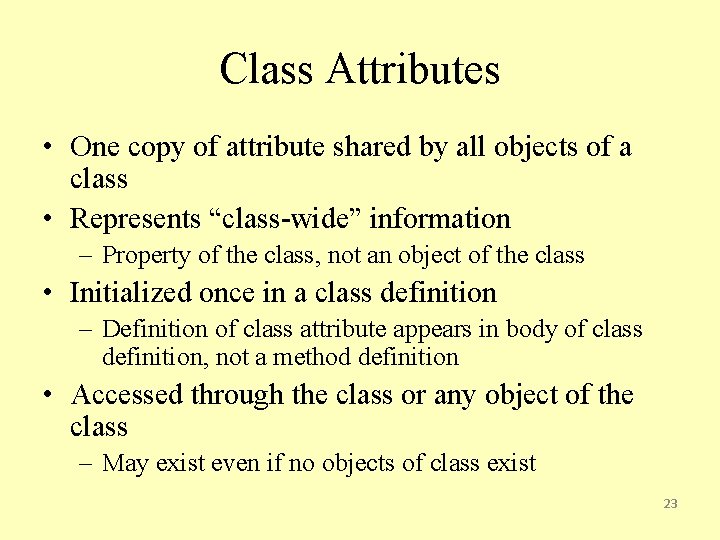
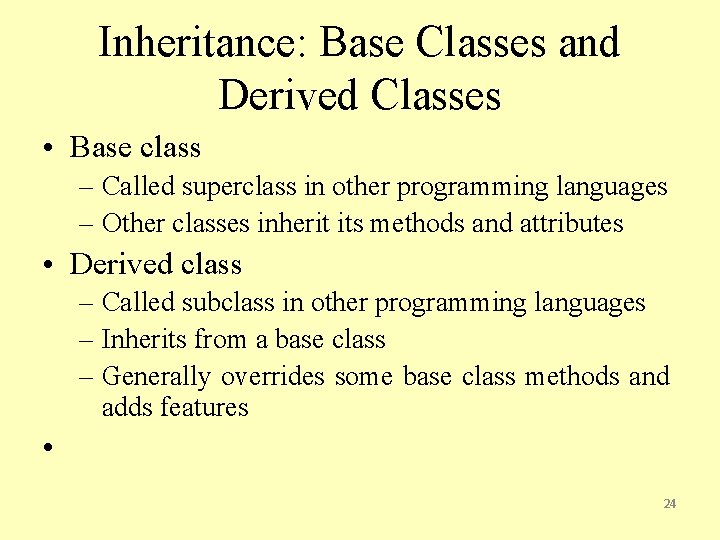
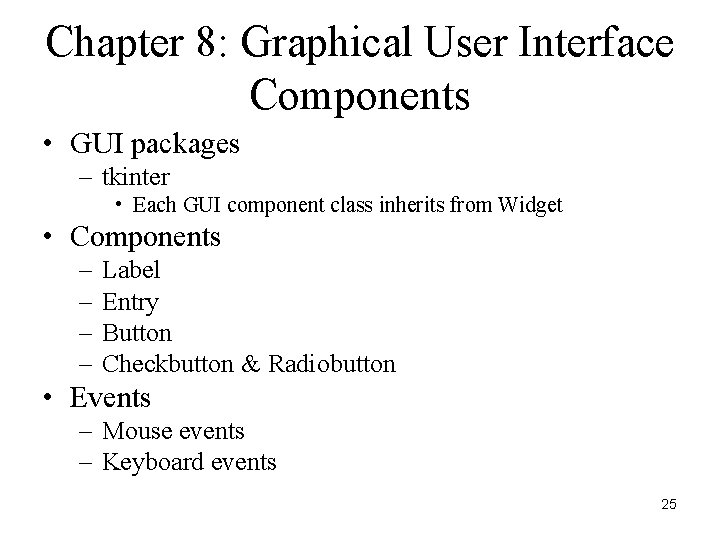
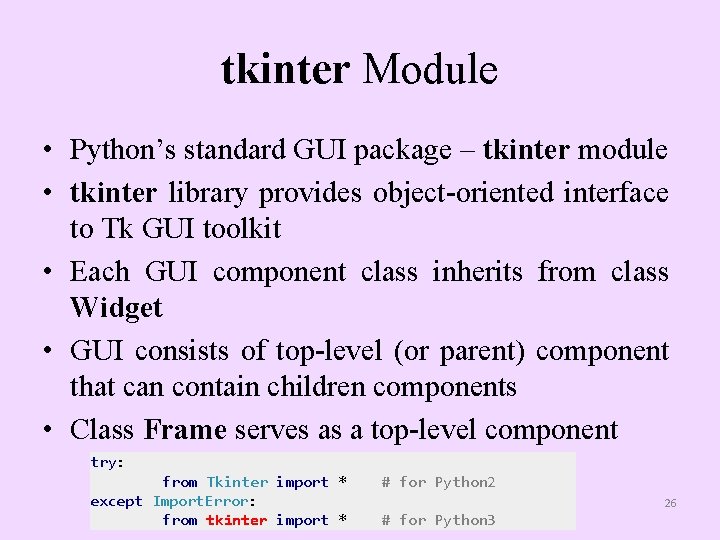
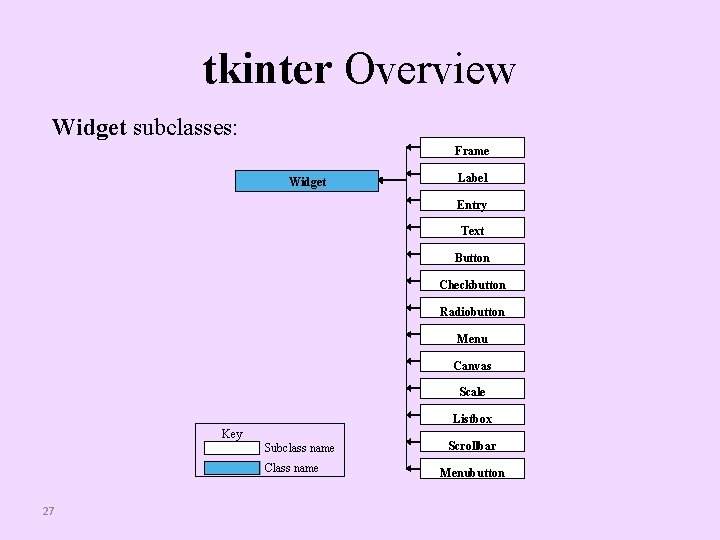
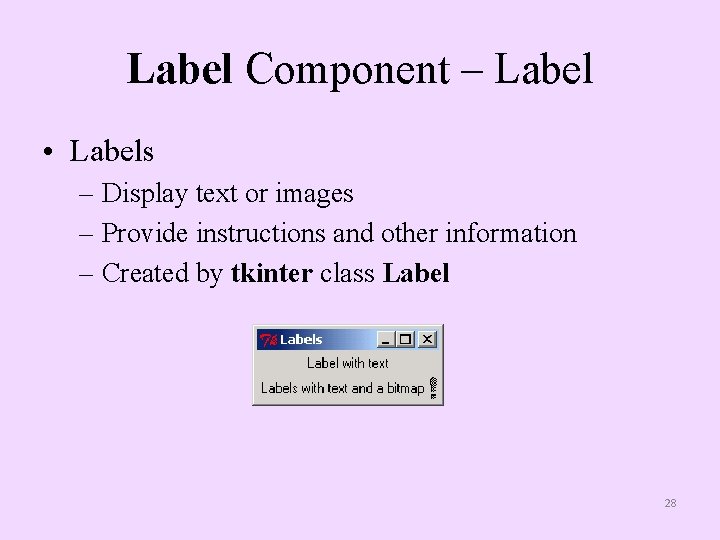
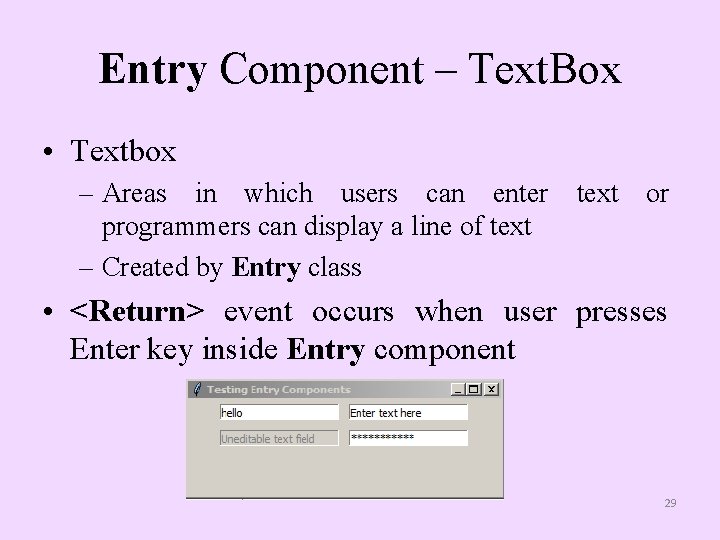
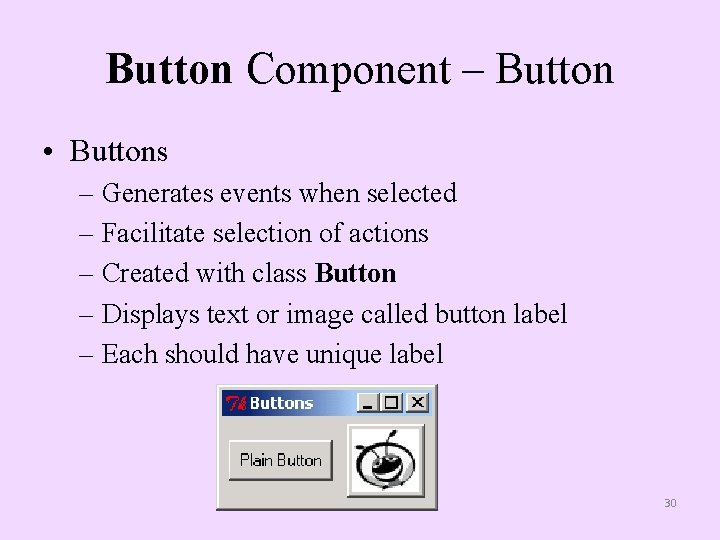
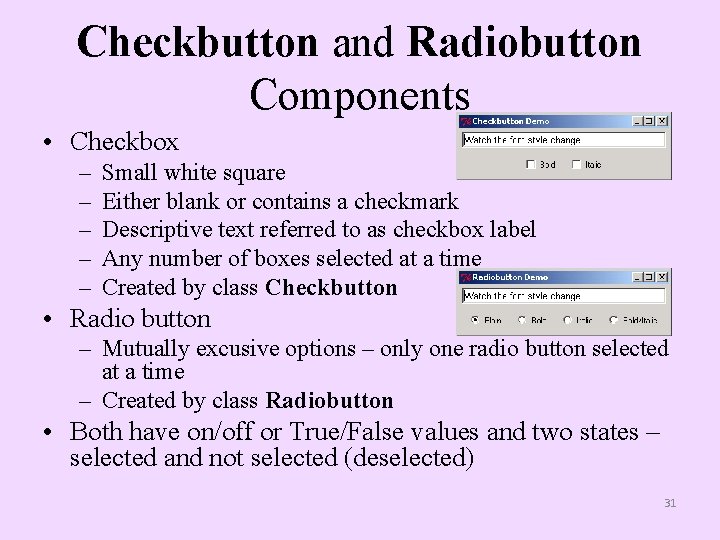
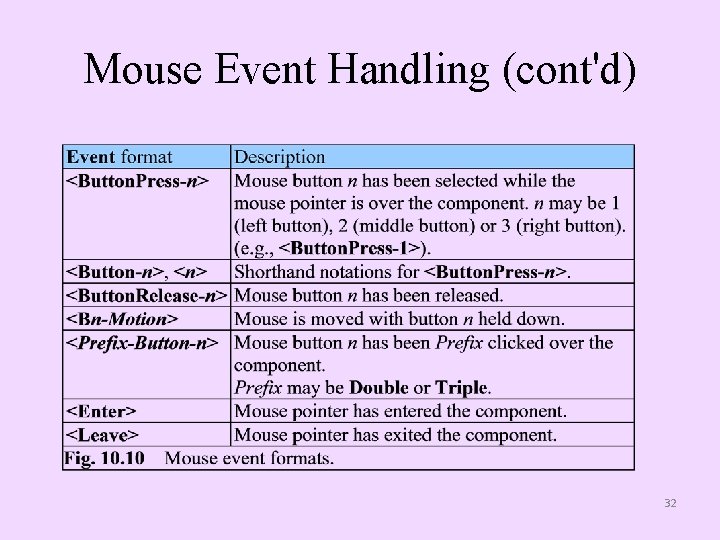
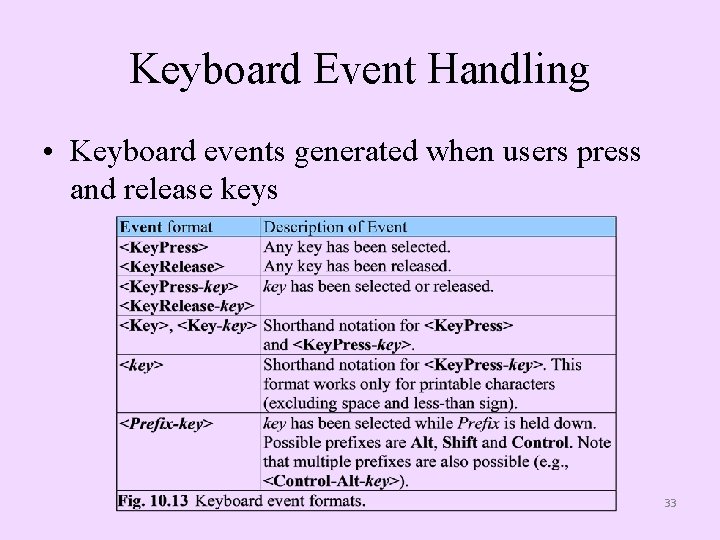
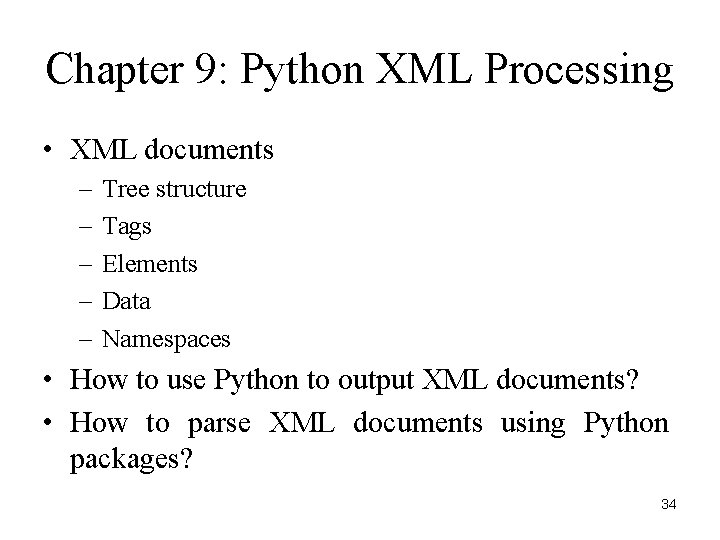
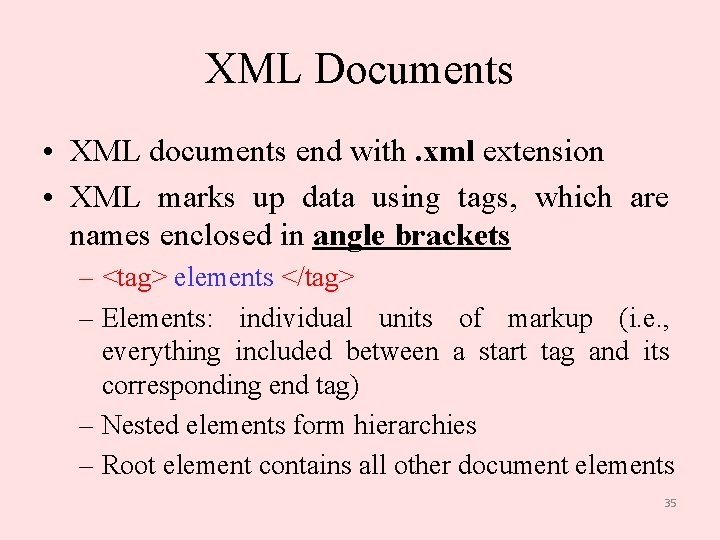
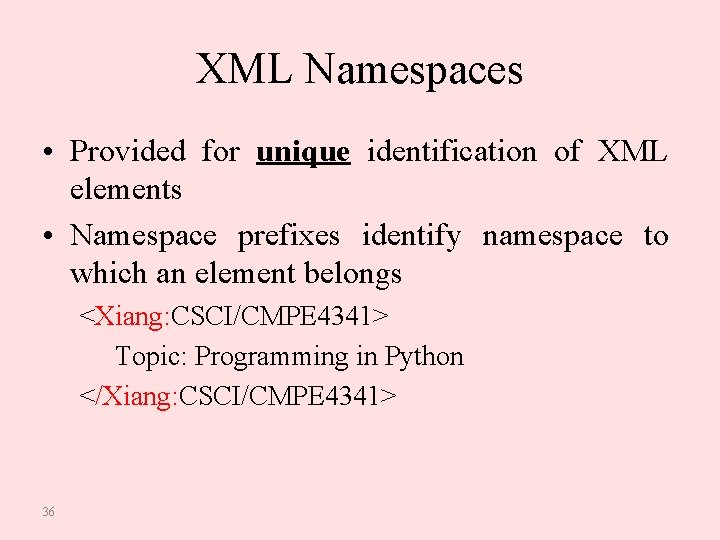
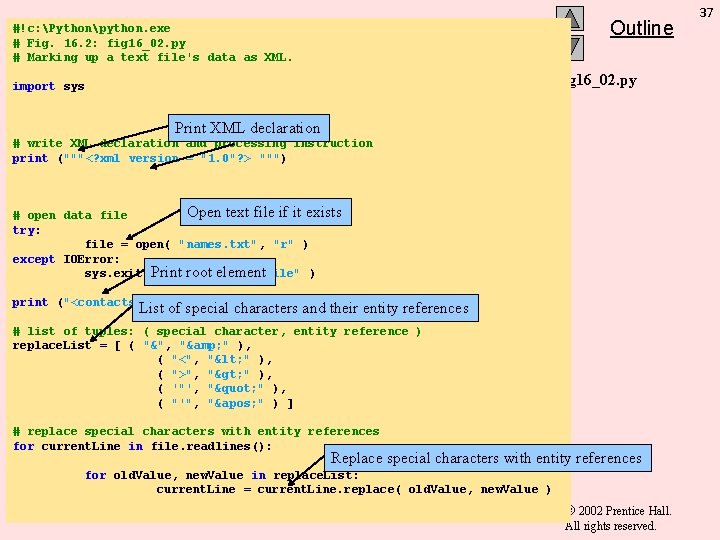
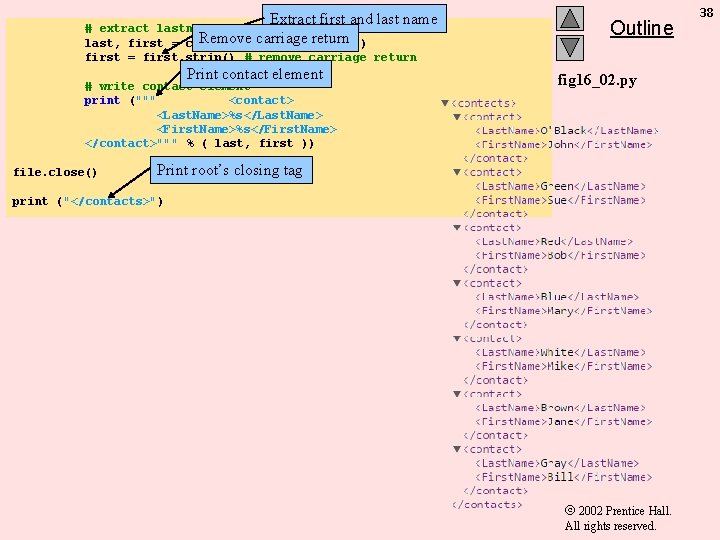
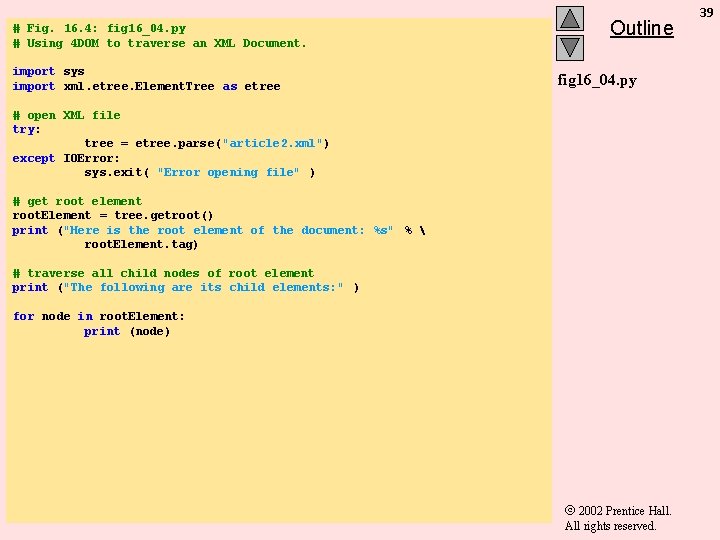
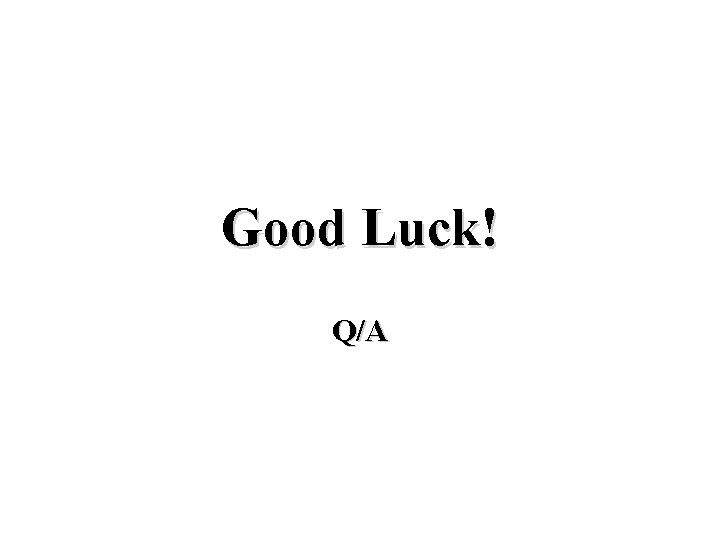
- Slides: 40
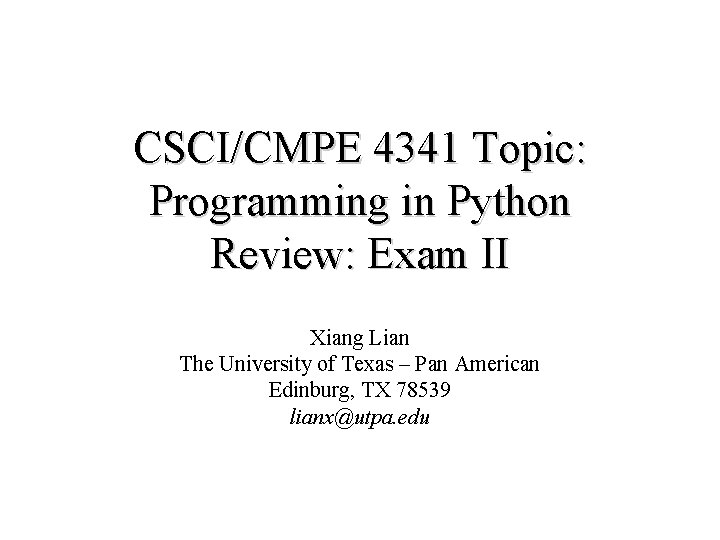
CSCI/CMPE 4341 Topic: Programming in Python Review: Exam II Xiang Lian The University of Texas – Pan American Edinburg, TX 78539 lianx@utpa. edu
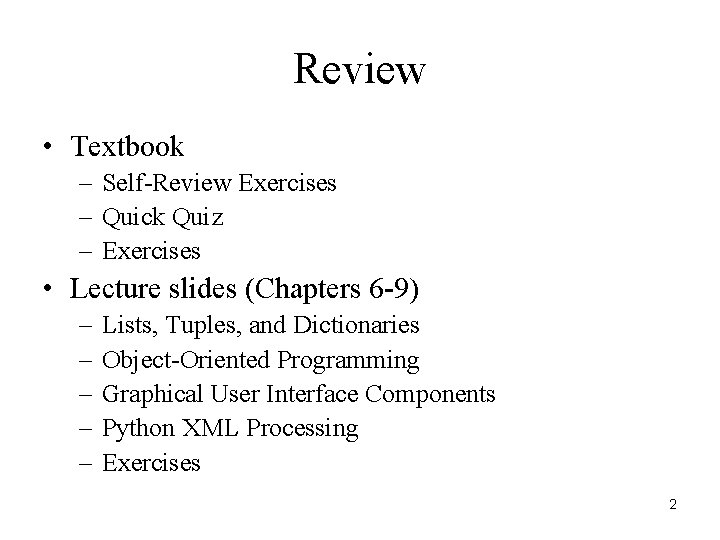
Review • Textbook – Self-Review Exercises – Quick Quiz – Exercises • Lecture slides (Chapters 6 -9) – – – Lists, Tuples, and Dictionaries Object-Oriented Programming Graphical User Interface Components Python XML Processing Exercises 2
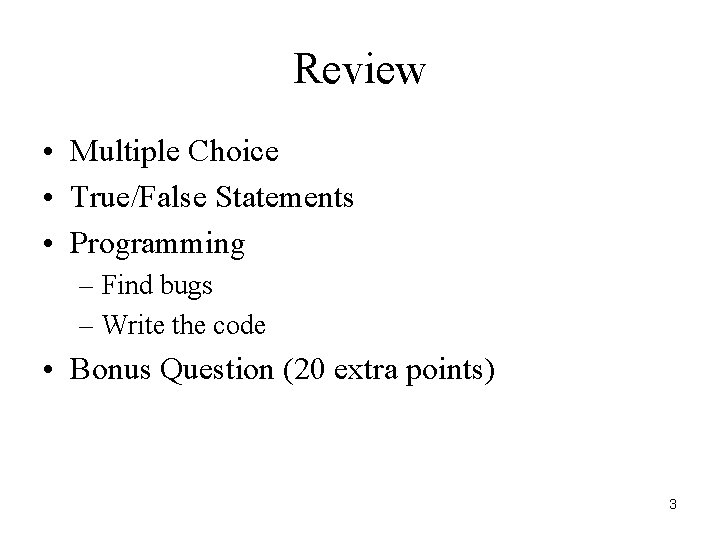
Review • Multiple Choice • True/False Statements • Programming – Find bugs – Write the code • Bonus Question (20 extra points) 3
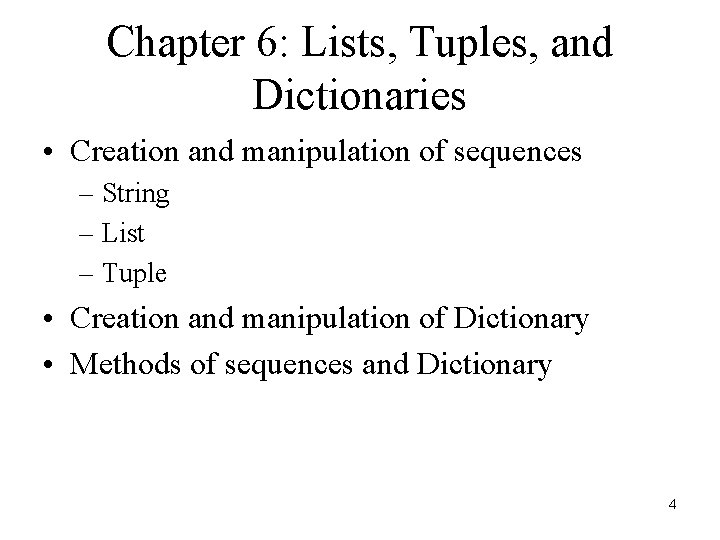
Chapter 6: Lists, Tuples, and Dictionaries • Creation and manipulation of sequences – String – List – Tuple • Creation and manipulation of Dictionary • Methods of sequences and Dictionary 4
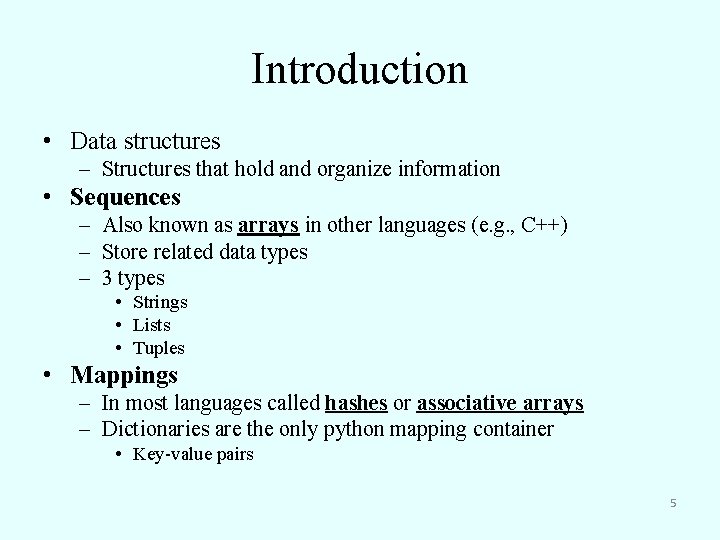
Introduction • Data structures – Structures that hold and organize information • Sequences – Also known as arrays in other languages (e. g. , C++) – Store related data types – 3 types • Strings • Lists • Tuples • Mappings – In most languages called hashes or associative arrays – Dictionaries are the only python mapping container • Key-value pairs 5
![Example of Sequences Name sequence C C0 45 C12 C1 6 C11 C2 0 Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0](https://slidetodoc.com/presentation_image_h/3284ce6250626bbcc9b71bad7d8606bb/image-6.jpg)
Example of Sequences Name sequence (C) C[0] -45 C[-12] C[1] 6 C[-11] C[2] 0 C[-10] C[3] 72 C[-9] C[4] 34 C[-8] C[5] 39 C[-7] C[6] 98 C[-6] C[7] -1345 C[-5] C[8] 939 C[-4] 10 C[-3] 40 C[-2] 33 C[-1] Position number of C[9] the element within C[10] sequence C C[11] 6
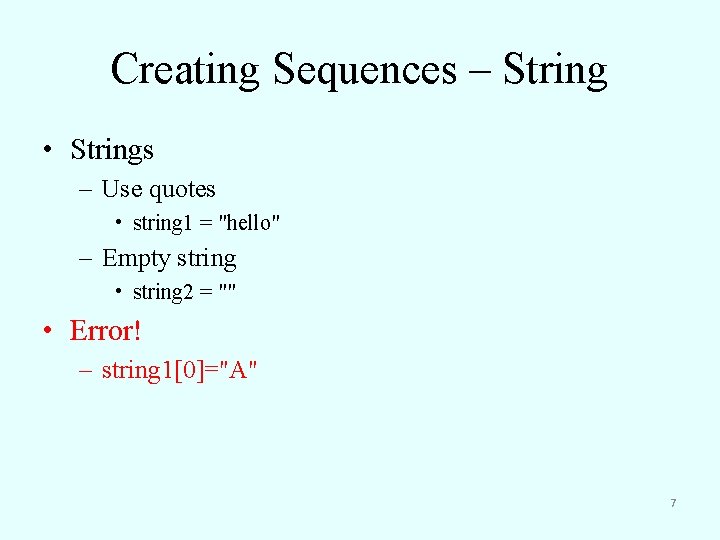
Creating Sequences – String • Strings – Use quotes • string 1 = "hello" – Empty string • string 2 = "" • Error! – string 1[0]="A" 7
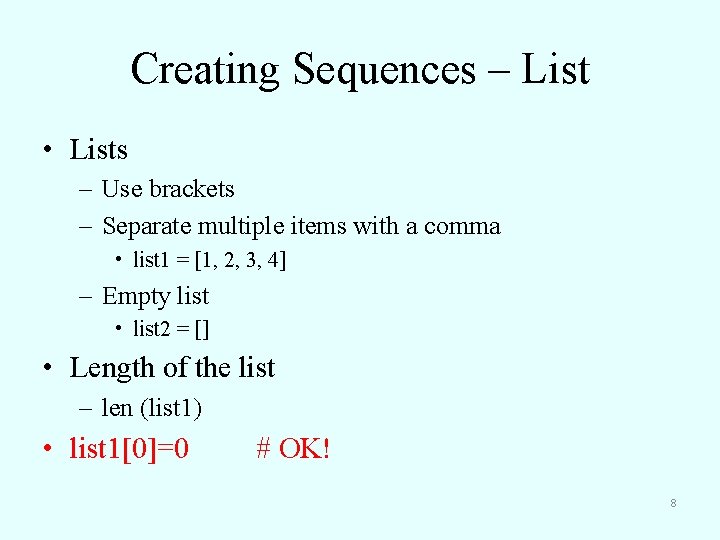
Creating Sequences – List • Lists – Use brackets – Separate multiple items with a comma • list 1 = [1, 2, 3, 4] – Empty list • list 2 = [] • Length of the list – len (list 1) • list 1[0]=0 # OK! 8
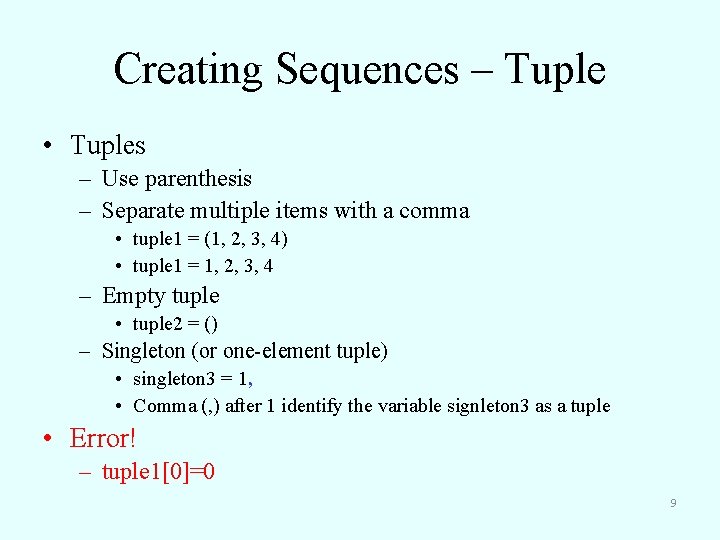
Creating Sequences – Tuple • Tuples – Use parenthesis – Separate multiple items with a comma • tuple 1 = (1, 2, 3, 4) • tuple 1 = 1, 2, 3, 4 – Empty tuple • tuple 2 = () – Singleton (or one-element tuple) • singleton 3 = 1, • Comma (, ) after 1 identify the variable signleton 3 as a tuple • Error! – tuple 1[0]=0 9
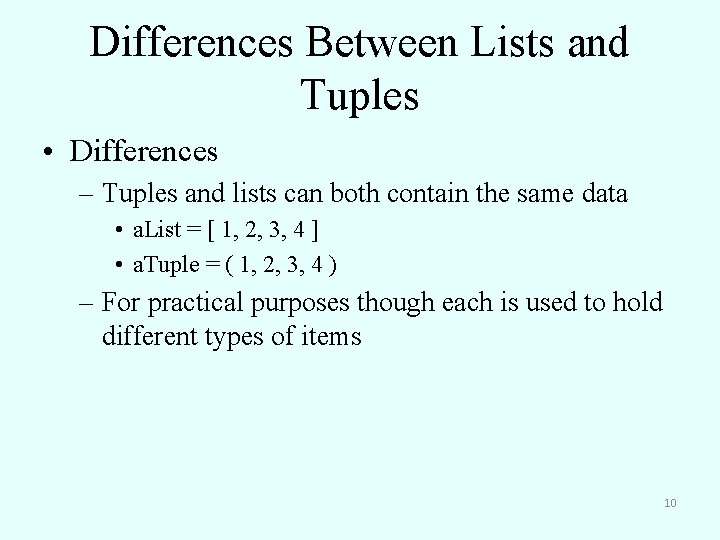
Differences Between Lists and Tuples • Differences – Tuples and lists can both contain the same data • a. List = [ 1, 2, 3, 4 ] • a. Tuple = ( 1, 2, 3, 4 ) – For practical purposes though each is used to hold different types of items 10
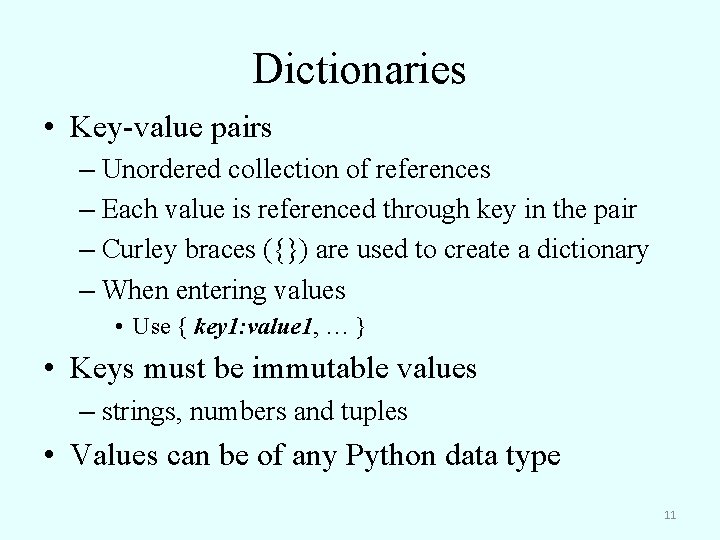
Dictionaries • Key-value pairs – Unordered collection of references – Each value is referenced through key in the pair – Curley braces ({}) are used to create a dictionary – When entering values • Use { key 1: value 1, … } • Keys must be immutable values – strings, numbers and tuples • Values can be of any Python data type 11
![Operations in Dictionaries Access dictionary element dictionary Name key value Operations in Dictionaries • Access dictionary element – dictionary. Name [key] = value #](https://slidetodoc.com/presentation_image_h/3284ce6250626bbcc9b71bad7d8606bb/image-12.jpg)
Operations in Dictionaries • Access dictionary element – dictionary. Name [key] = value # update the value of an existing key • Add key-value pair – dictionary. Name [new. Key] = new. Value • Delete key-value pair – del dictionary. Name [key] 12
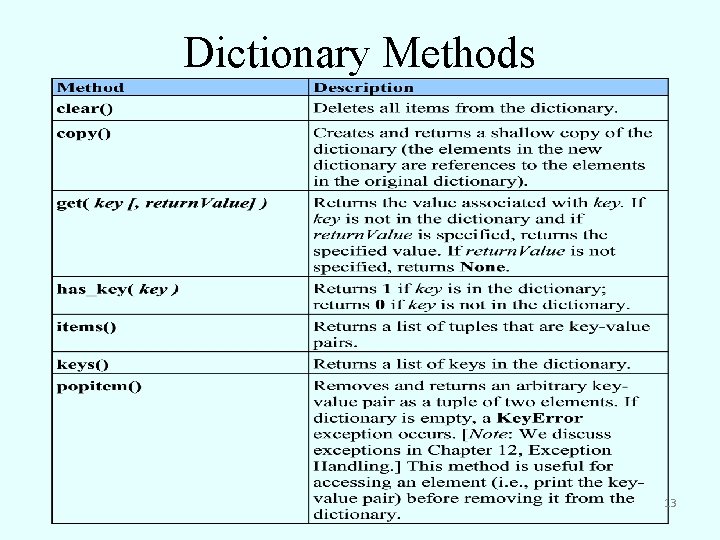
Dictionary Methods 13
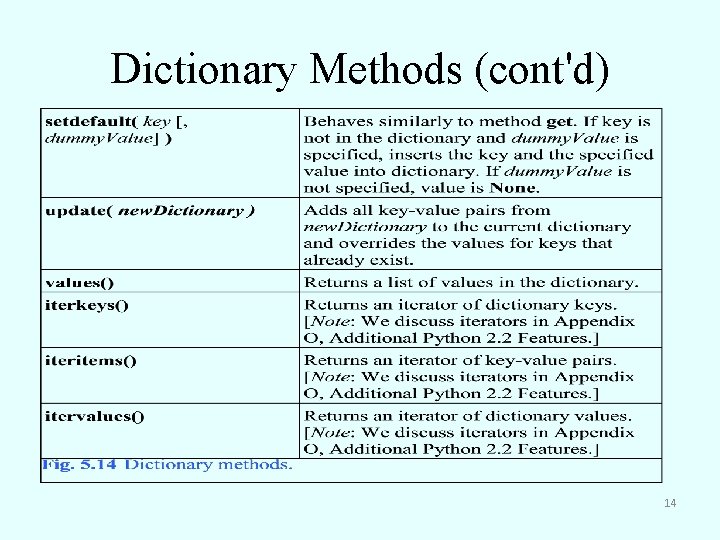
Dictionary Methods (cont'd) 14
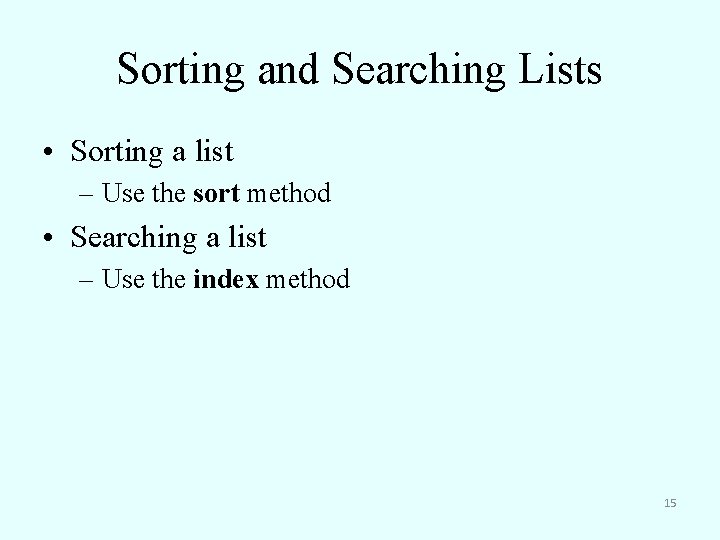
Sorting and Searching Lists • Sorting a list – Use the sort method • Searching a list – Use the index method 15
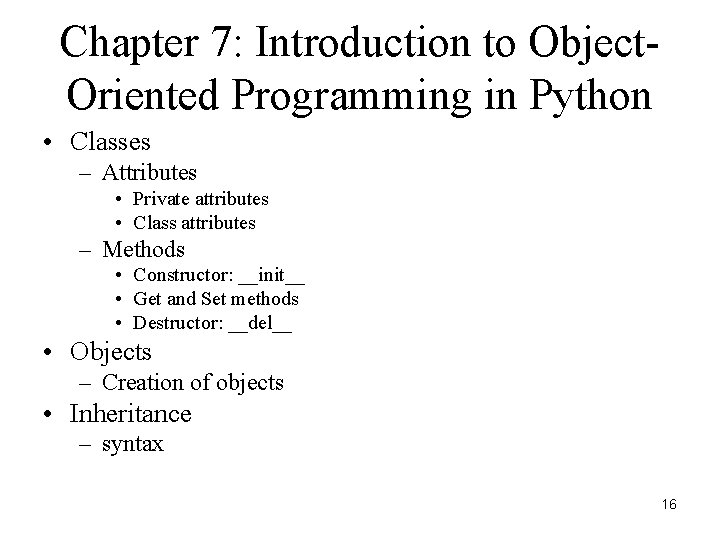
Chapter 7: Introduction to Object. Oriented Programming in Python • Classes – Attributes • Private attributes • Class attributes – Methods • Constructor: __init__ • Get and Set methods • Destructor: __del__ • Objects – Creation of objects • Inheritance – syntax 16
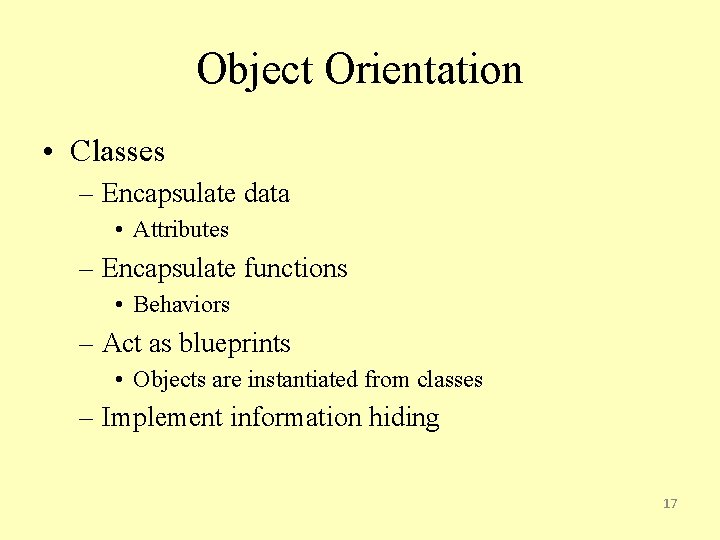
Object Orientation • Classes – Encapsulate data • Attributes – Encapsulate functions • Behaviors – Act as blueprints • Objects are instantiated from classes – Implement information hiding 17
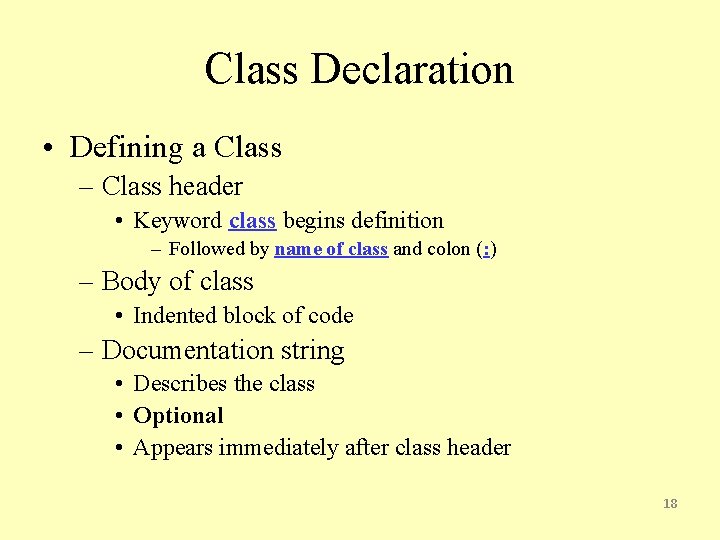
Class Declaration • Defining a Class – Class header • Keyword class begins definition – Followed by name of class and colon (: ) – Body of class • Indented block of code – Documentation string • Describes the class • Optional • Appears immediately after class header 18
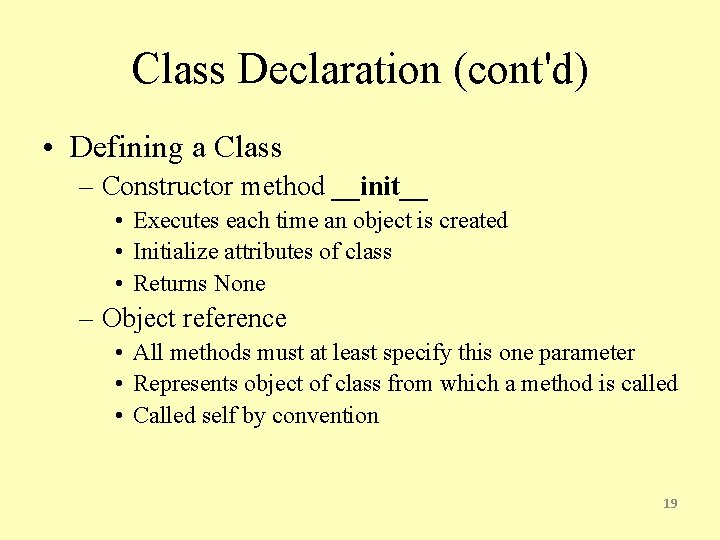
Class Declaration (cont'd) • Defining a Class – Constructor method __init__ • Executes each time an object is created • Initialize attributes of class • Returns None – Object reference • All methods must at least specify this one parameter • Represents object of class from which a method is called • Called self by convention 19
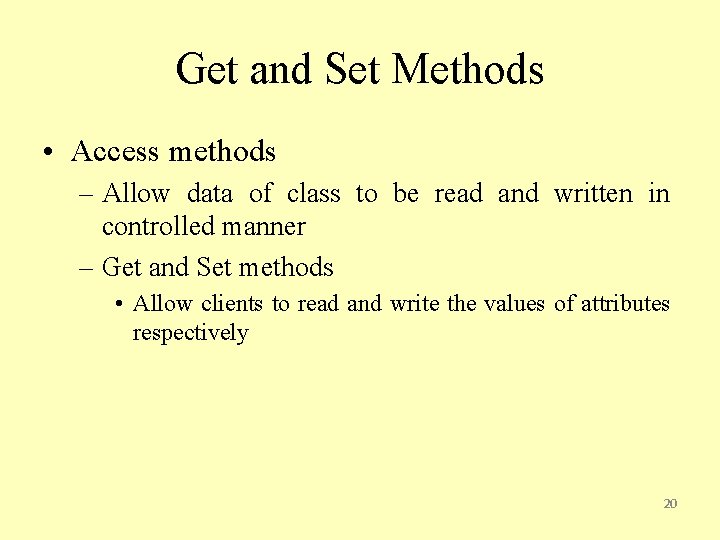
Get and Set Methods • Access methods – Allow data of class to be read and written in controlled manner – Get and Set methods • Allow clients to read and write the values of attributes respectively 20
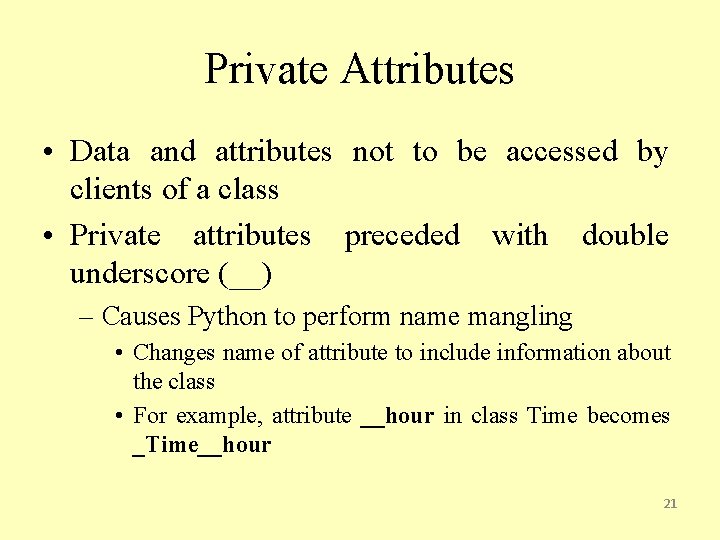
Private Attributes • Data and attributes not to be accessed by clients of a class • Private attributes preceded with double underscore (__) – Causes Python to perform name mangling • Changes name of attribute to include information about the class • For example, attribute __hour in class Time becomes _Time__hour 21
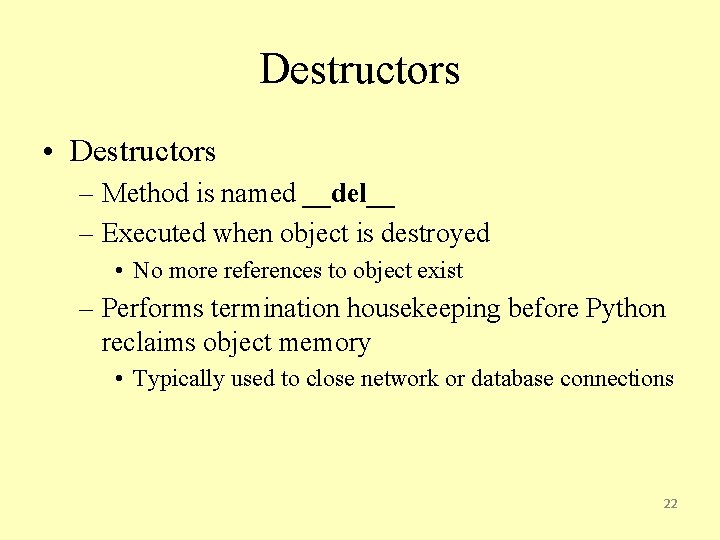
Destructors • Destructors – Method is named __del__ – Executed when object is destroyed • No more references to object exist – Performs termination housekeeping before Python reclaims object memory • Typically used to close network or database connections 22
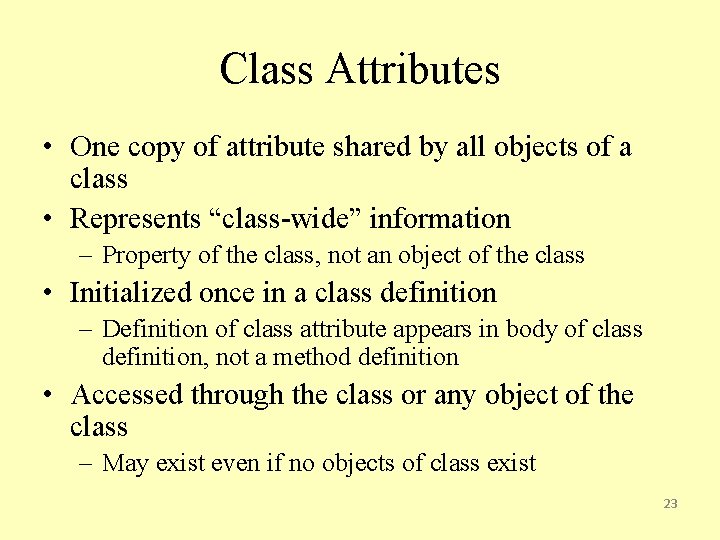
Class Attributes • One copy of attribute shared by all objects of a class • Represents “class-wide” information – Property of the class, not an object of the class • Initialized once in a class definition – Definition of class attribute appears in body of class definition, not a method definition • Accessed through the class or any object of the class – May exist even if no objects of class exist 23
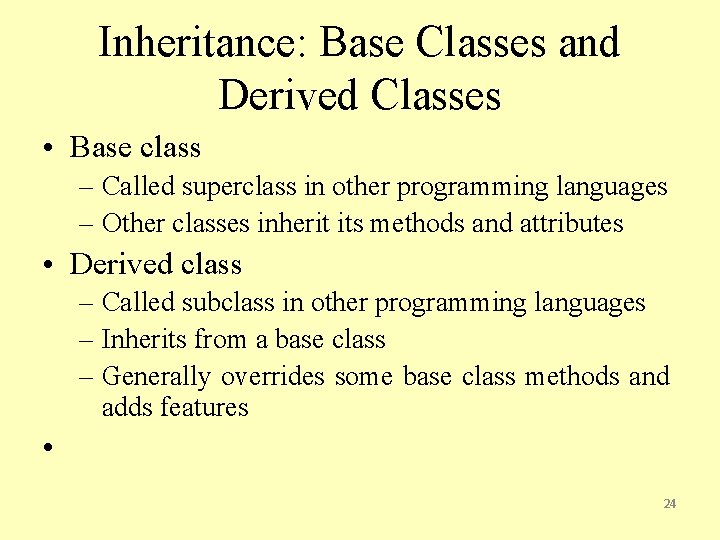
Inheritance: Base Classes and Derived Classes • Base class – Called superclass in other programming languages – Other classes inherit its methods and attributes • Derived class – Called subclass in other programming languages – Inherits from a base class – Generally overrides some base class methods and adds features • 24
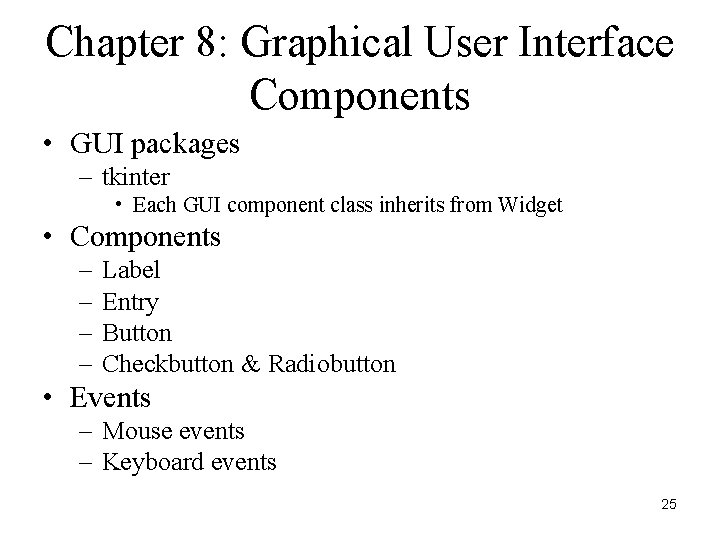
Chapter 8: Graphical User Interface Components • GUI packages – tkinter • Each GUI component class inherits from Widget • Components – – Label Entry Button Checkbutton & Radiobutton • Events – Mouse events – Keyboard events 25
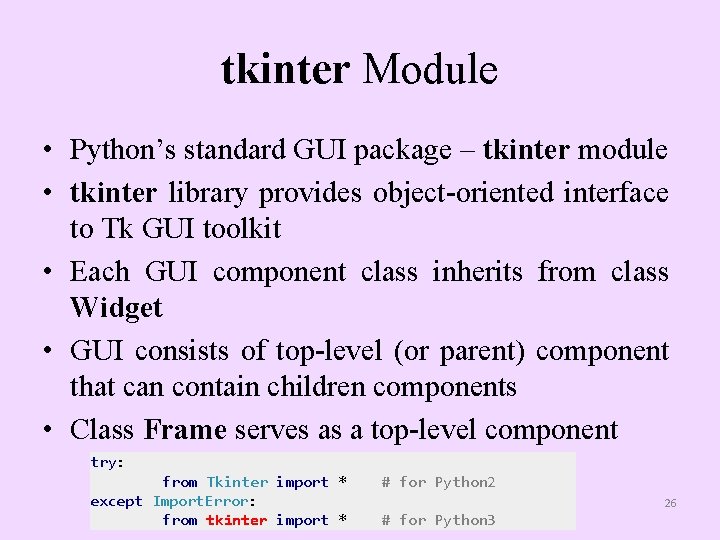
tkinter Module • Python’s standard GUI package – tkinter module • tkinter library provides object-oriented interface to Tk GUI toolkit • Each GUI component class inherits from class Widget • GUI consists of top-level (or parent) component that can contain children components • Class Frame serves as a top-level component try: from Tkinter import * except Import. Error: from tkinter import * # for Python 2 26 # for Python 3
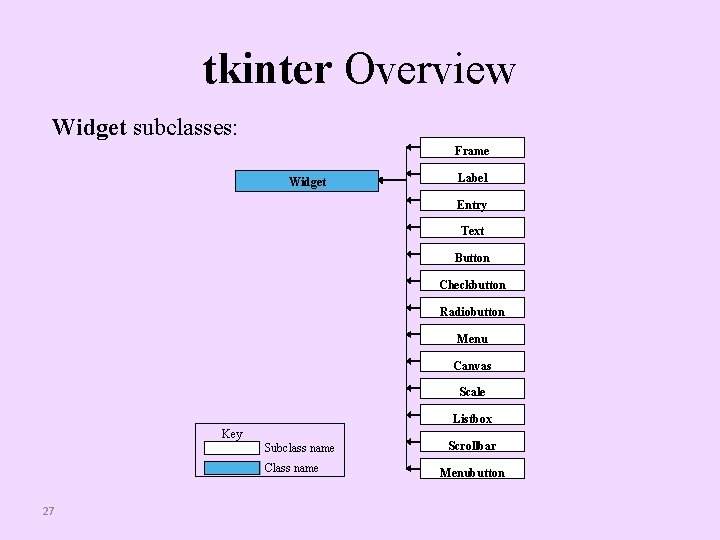
tkinter Overview Widget subclasses: Frame Widget Label Entry Text Button Checkbutton Radiobutton Menu Canvas Scale Listbox Key Subclass name Class name 27 Scrollbar Menubutton
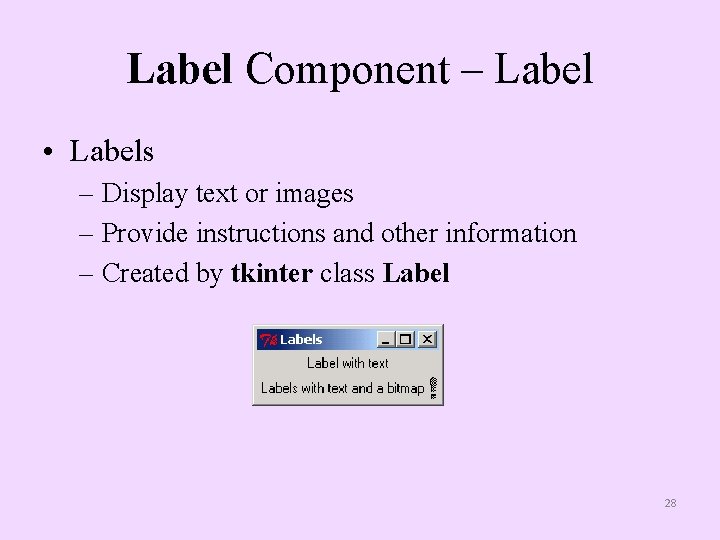
Label Component – Label • Labels – Display text or images – Provide instructions and other information – Created by tkinter class Label 28
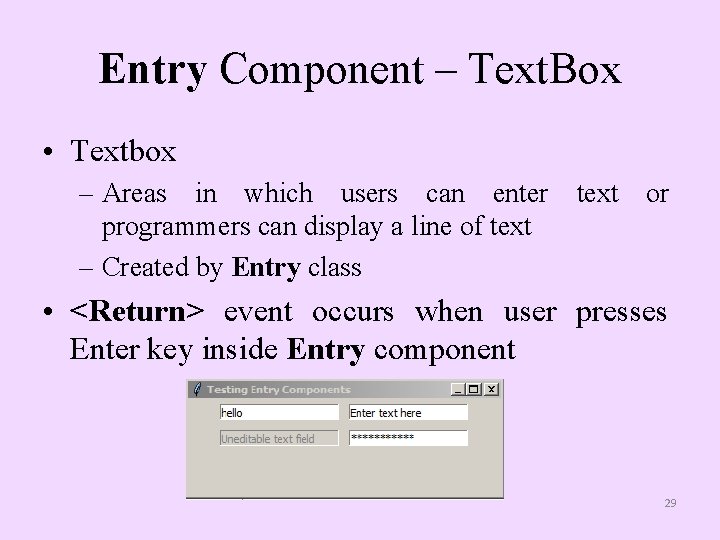
Entry Component – Text. Box • Textbox – Areas in which users can enter text or programmers can display a line of text – Created by Entry class • <Return> event occurs when user presses Enter key inside Entry component 29
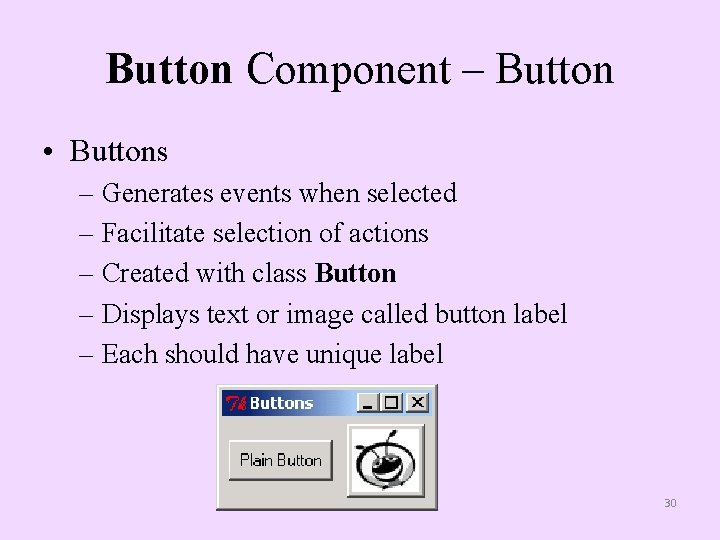
Button Component – Button • Buttons – Generates events when selected – Facilitate selection of actions – Created with class Button – Displays text or image called button label – Each should have unique label 30
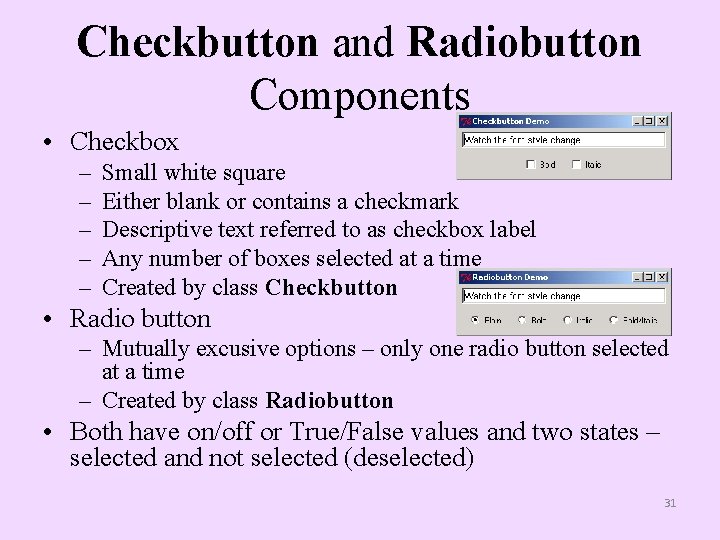
Checkbutton and Radiobutton Components • Checkbox – – – Small white square Either blank or contains a checkmark Descriptive text referred to as checkbox label Any number of boxes selected at a time Created by class Checkbutton • Radio button – Mutually excusive options – only one radio button selected at a time – Created by class Radiobutton • Both have on/off or True/False values and two states – selected and not selected (deselected) 31
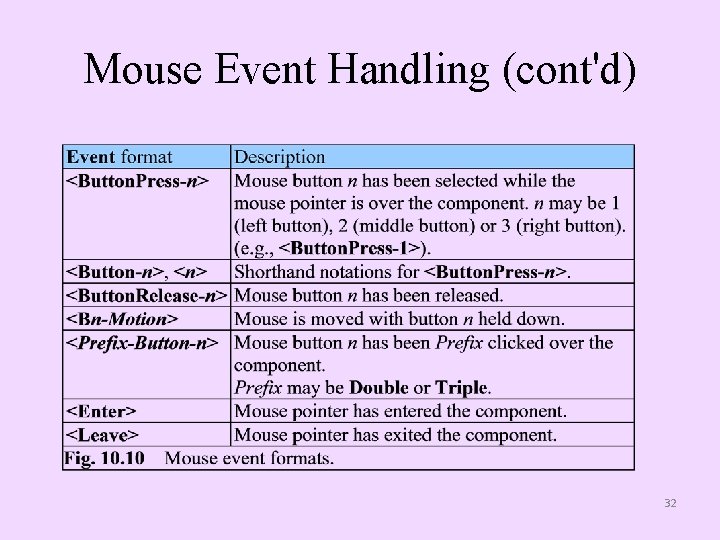
Mouse Event Handling (cont'd) 32
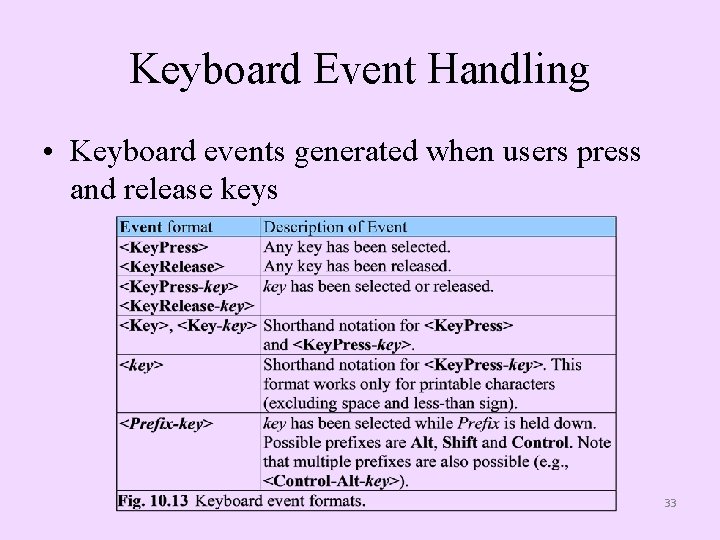
Keyboard Event Handling • Keyboard events generated when users press and release keys 33
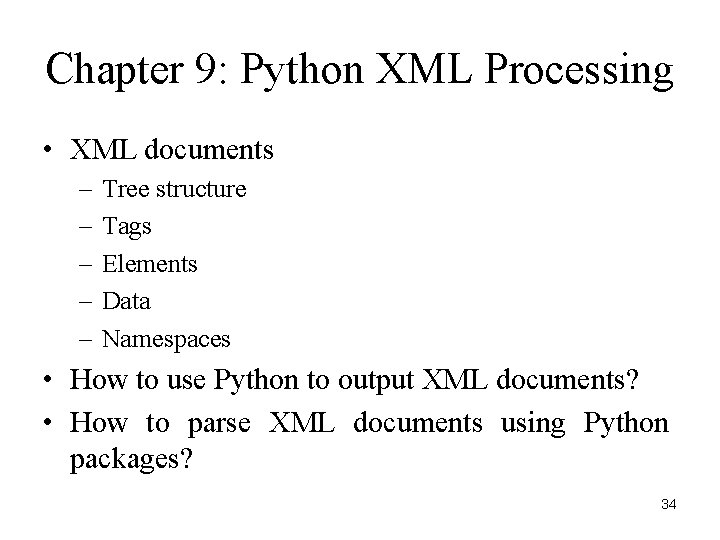
Chapter 9: Python XML Processing • XML documents – – – Tree structure Tags Elements Data Namespaces • How to use Python to output XML documents? • How to parse XML documents using Python packages? 34
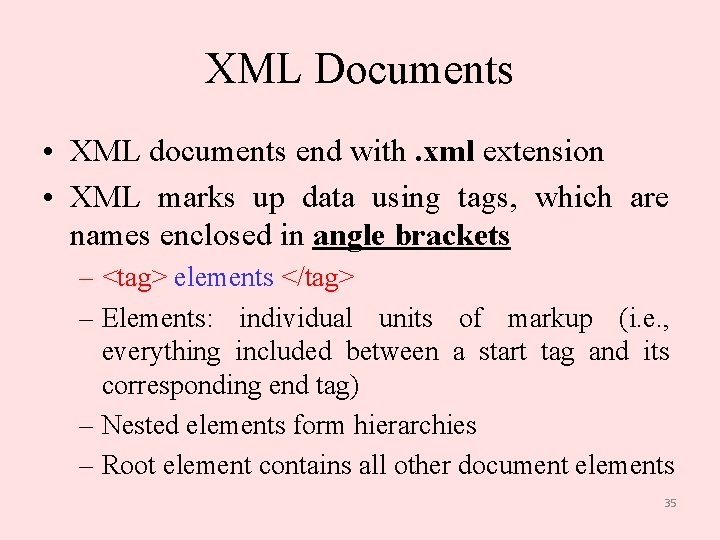
XML Documents • XML documents end with. xml extension • XML marks up data using tags, which are names enclosed in angle brackets – <tag> elements </tag> – Elements: individual units of markup (i. e. , everything included between a start tag and its corresponding end tag) – Nested elements form hierarchies – Root element contains all other document elements 35
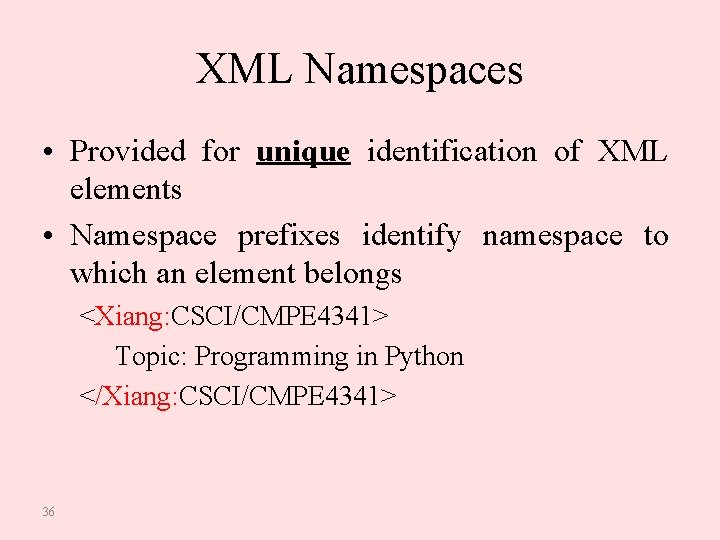
XML Namespaces • Provided for unique identification of XML elements • Namespace prefixes identify namespace to which an element belongs <Xiang: CSCI/CMPE 4341> Topic: Programming in Python </Xiang: CSCI/CMPE 4341> 36
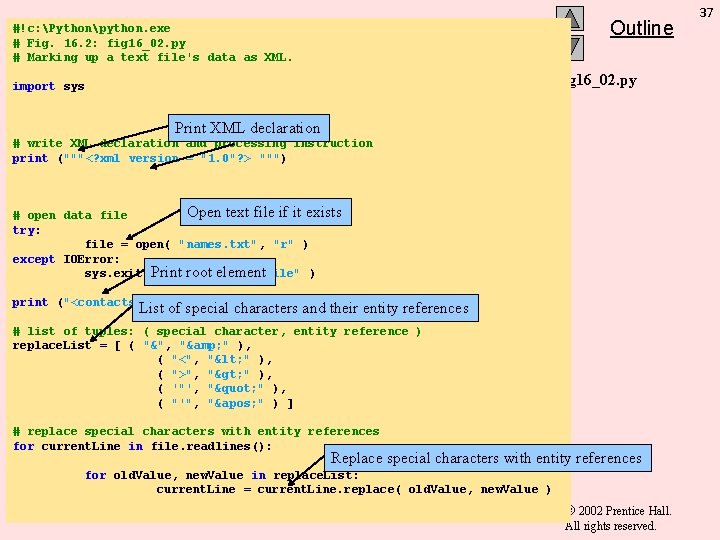
Outline #!c: Pythonpython. exe # Fig. 16. 2: fig 16_02. py # Marking up a text file's data as XML. fig 16_02. py import sys Print XML declaration # write XML declaration and processing instruction print ("""<? xml version = "1. 0"? > """) Open text file if it exists # open data file try: file = open( "names. txt", "r" ) except IOError: sys. exit( Print root element "Error opening file" ) print ("<contacts>") # write root element List of special characters and their entity references # list of tuples: ( special character, entity reference ) replace. List = [ ( "&", "& " ), ( "<", "< " ), ( ">", "> " ), ( '"', "" " ), ( "'", "' " ) ] # replace special characters with entity references for current. Line in file. readlines(): Replace special characters with entity references for old. Value, new. Value in replace. List: current. Line = current. Line. replace( old. Value, new. Value ) 2002 Prentice Hall. All rights reserved. 37
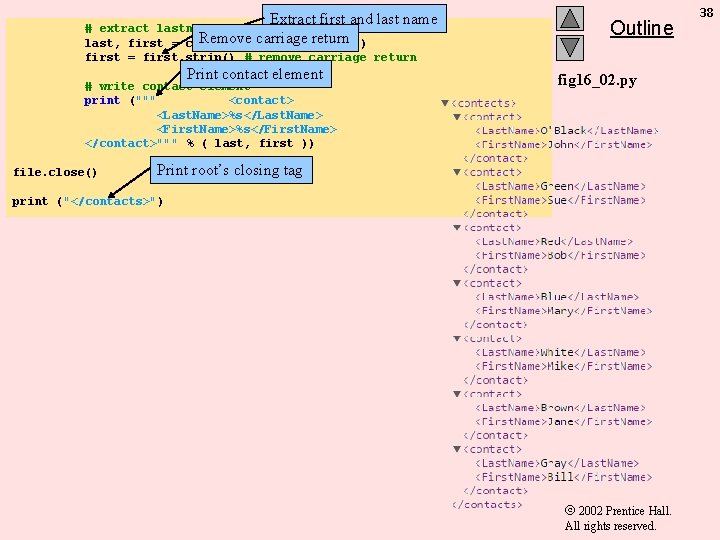
Extract first and last name # extract lastname and firstname Remove carriage return last, first = current. Line. split( ", " ) Outline first = first. strip() # remove carriage return Print contact element # write contact element print (""" <contact> <Last. Name>%s</Last. Name> <First. Name>%s</First. Name> </contact>""" % ( last, first )) file. close() fig 16_02. py Print root’s closing tag print ("</contacts>") 2002 Prentice Hall. All rights reserved. 38
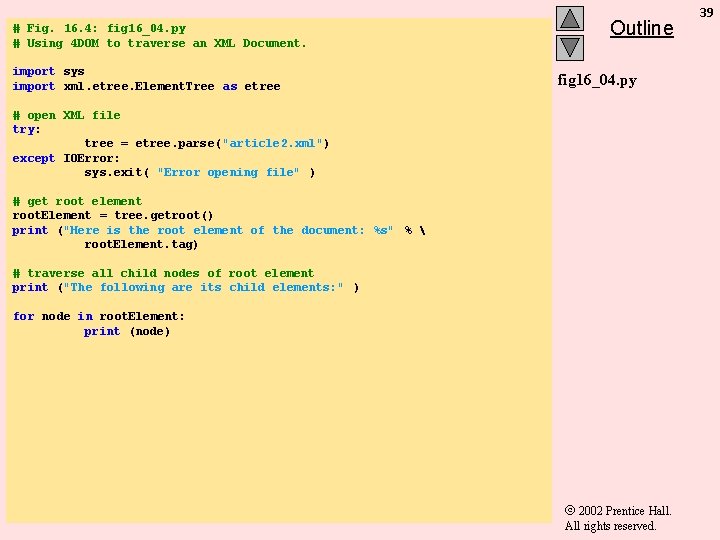
# Fig. 16. 4: fig 16_04. py # Using 4 DOM to traverse an XML Document. import sys import xml. etree. Element. Tree as etree Outline fig 16_04. py # open XML file try: tree = etree. parse("article 2. xml") except IOError: sys. exit( "Error opening file" ) # get root element root. Element = tree. getroot() print ("Here is the root element of the document: %s" % root. Element. tag) # traverse all child nodes of root element print ("The following are its child elements: " ) for node in root. Element: print (node) 2002 Prentice Hall. All rights reserved. 39
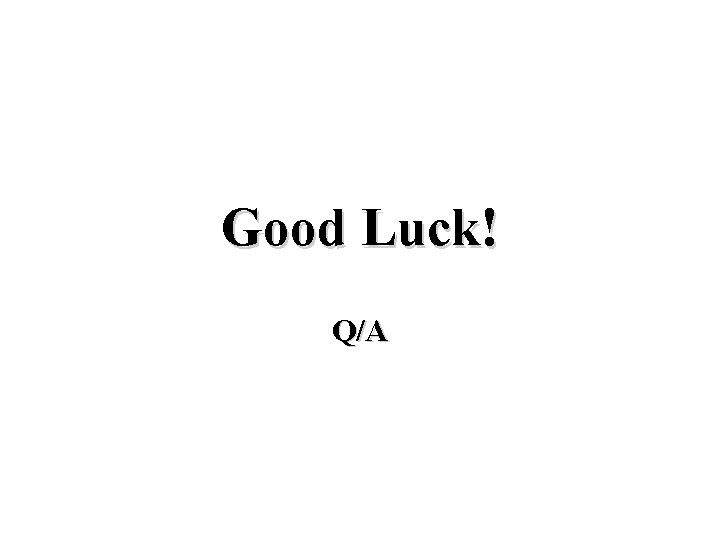
Good Luck! Q/A