CS 5103 Software Engineering Lecture 13 Software Refactoring
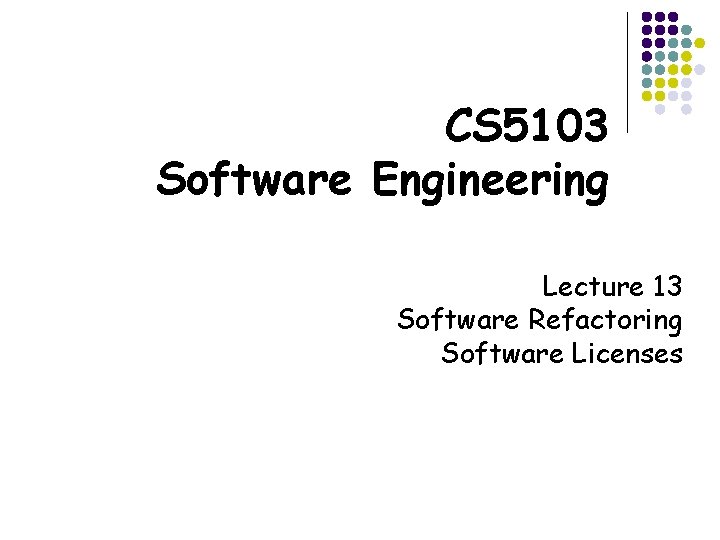
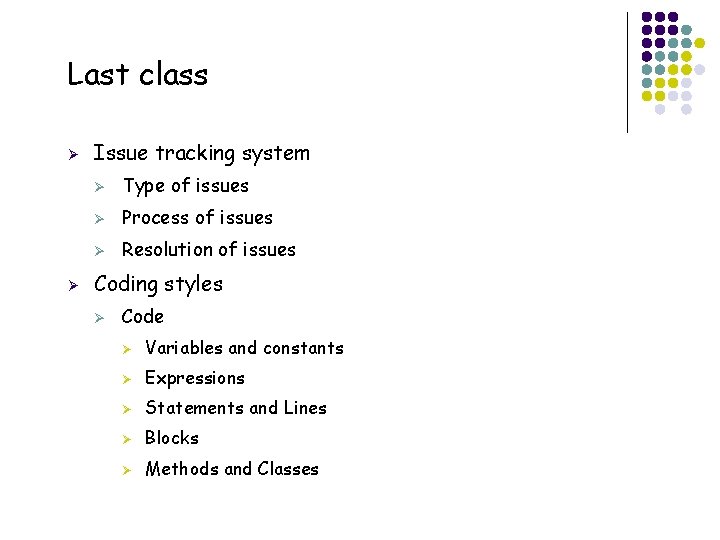
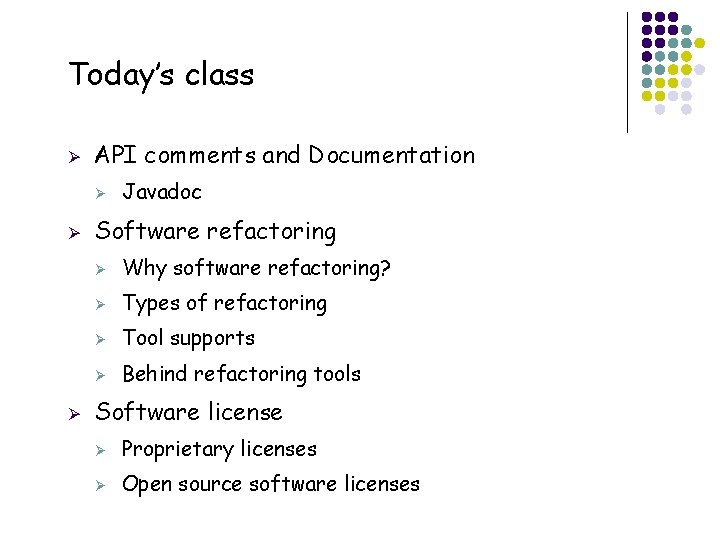
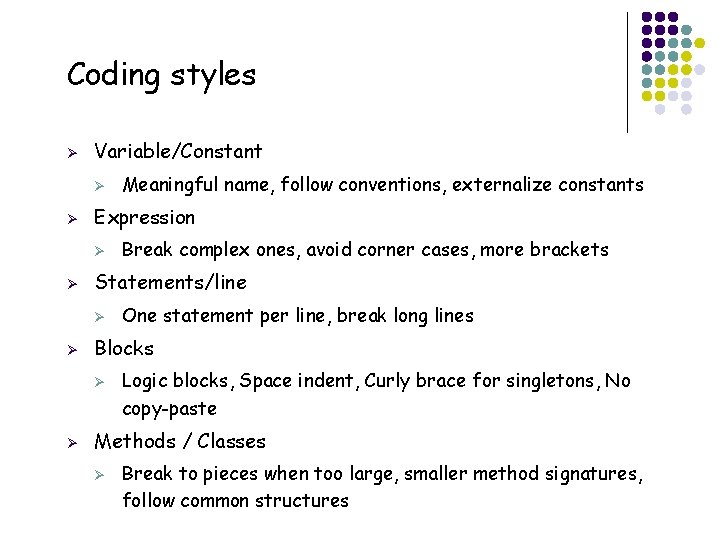
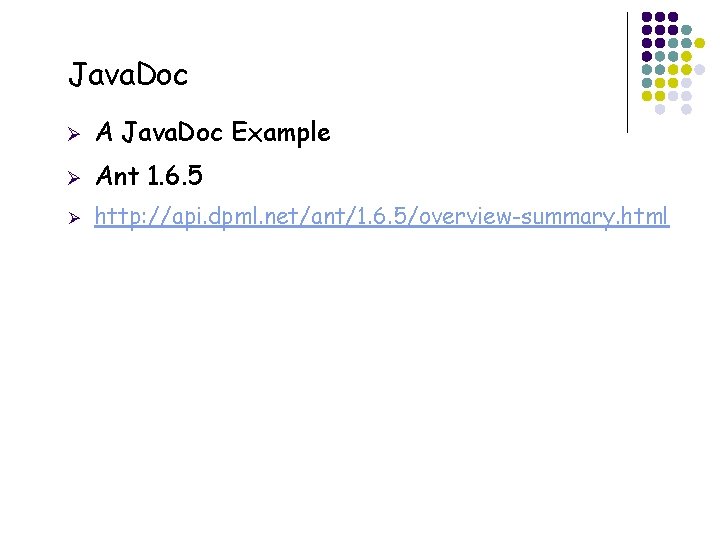
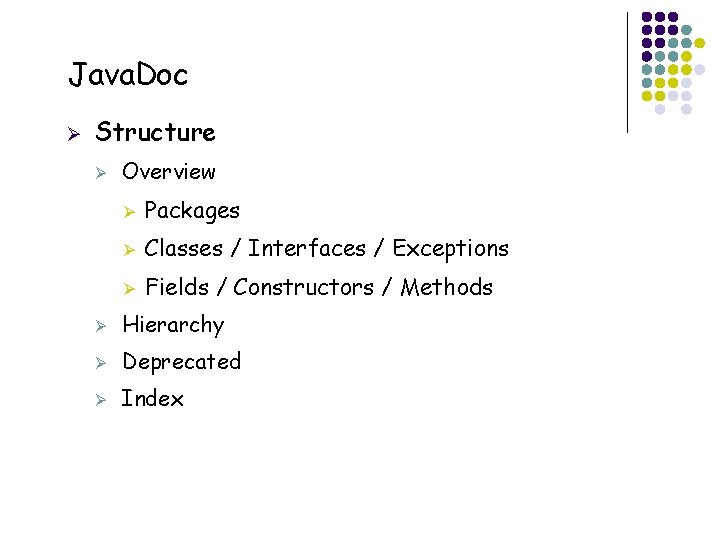
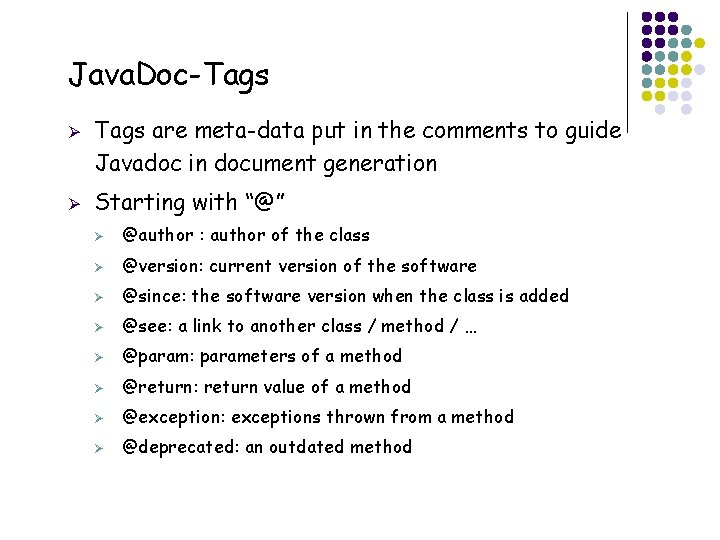
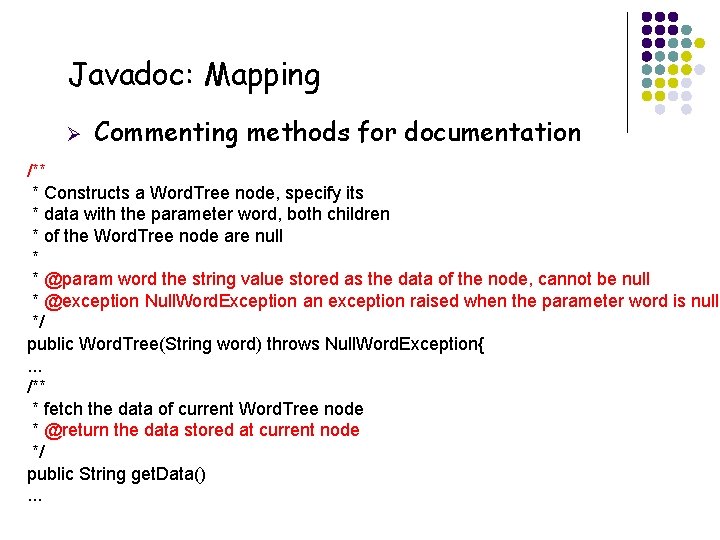
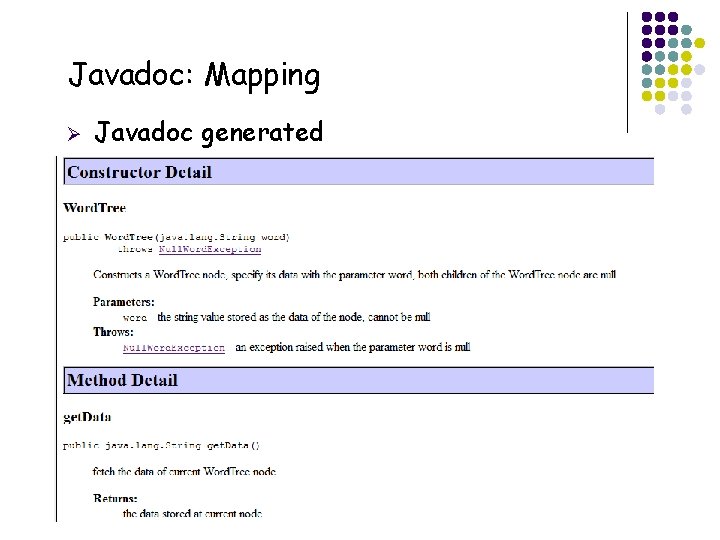
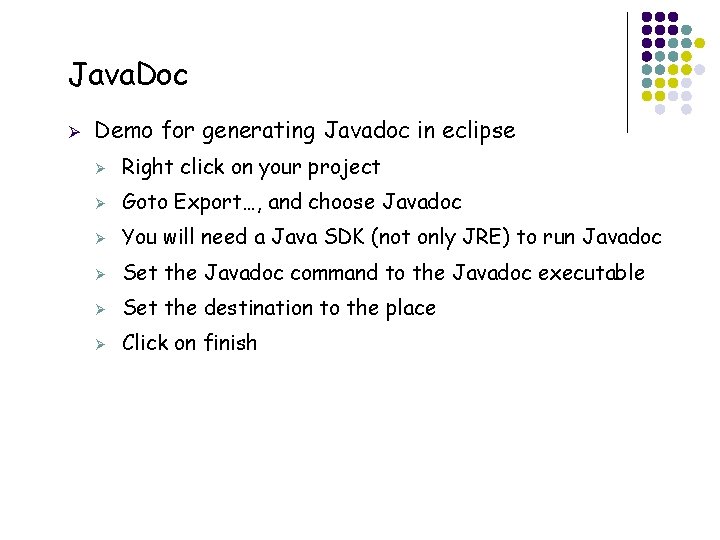
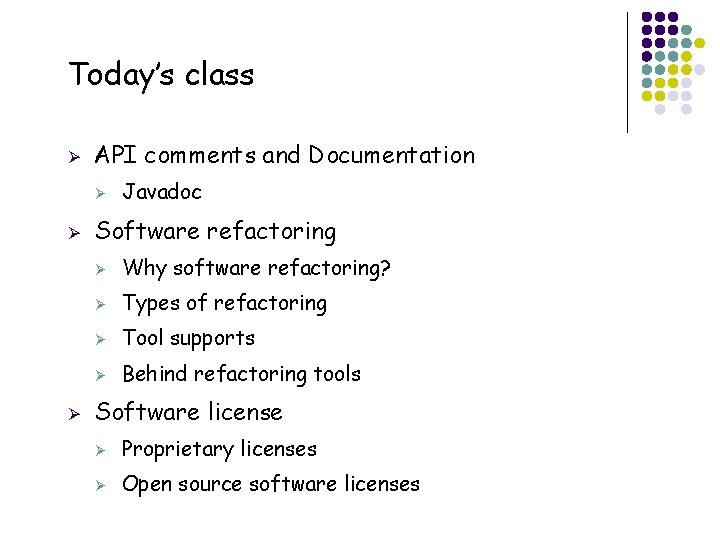
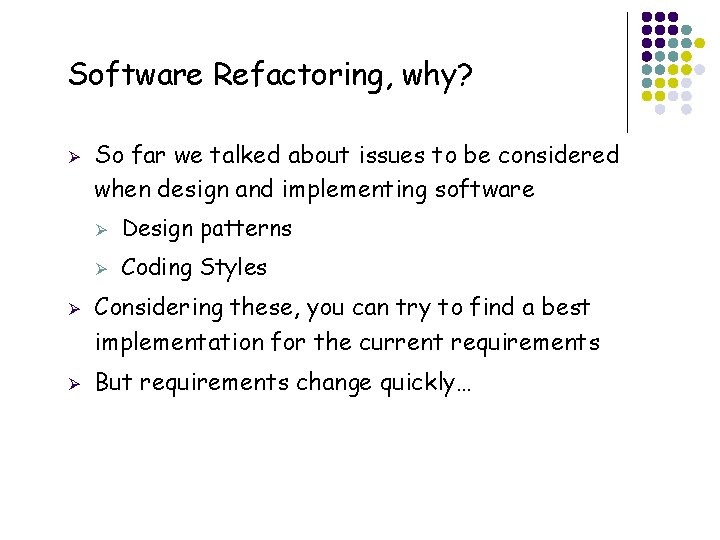
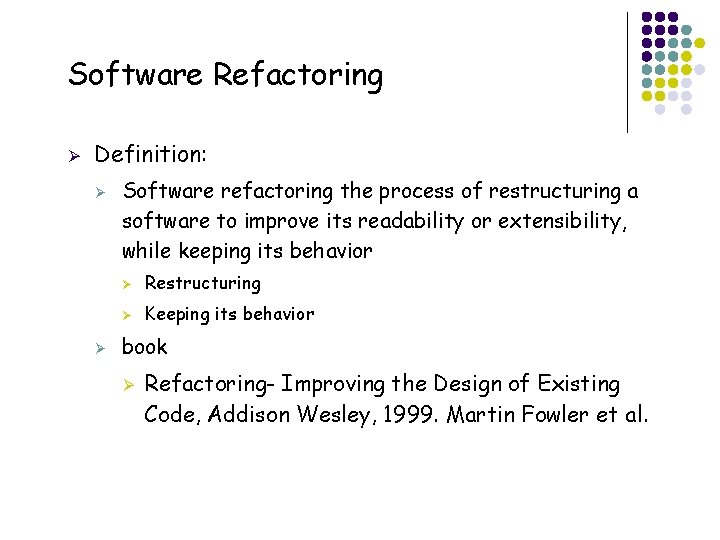
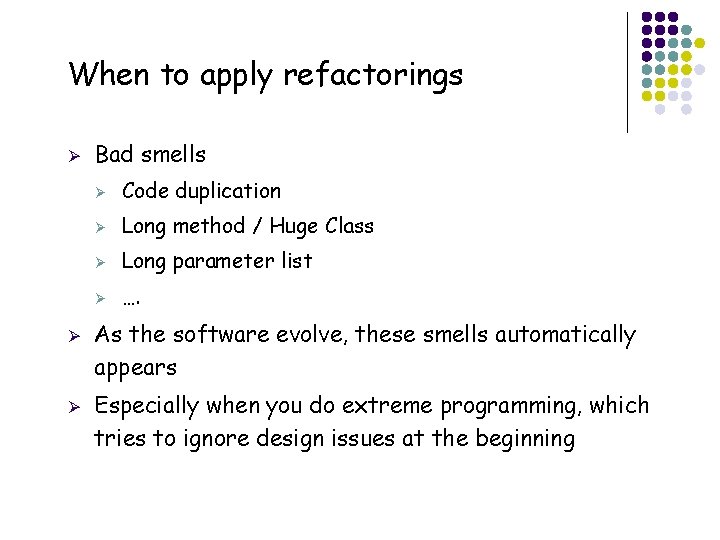
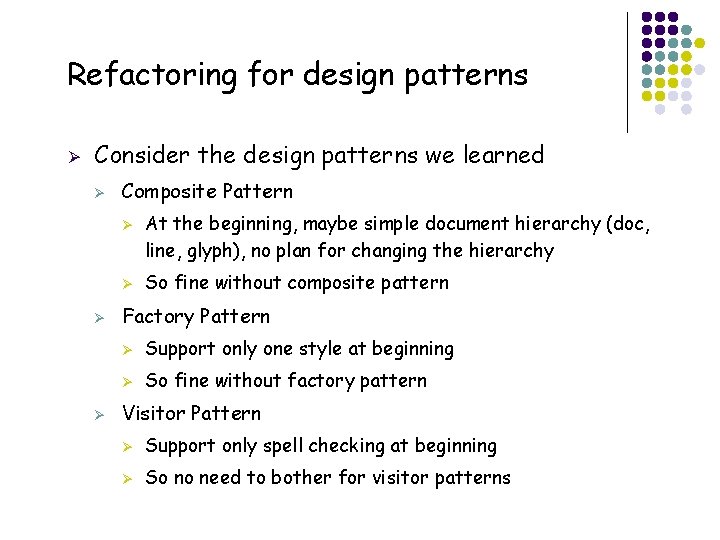
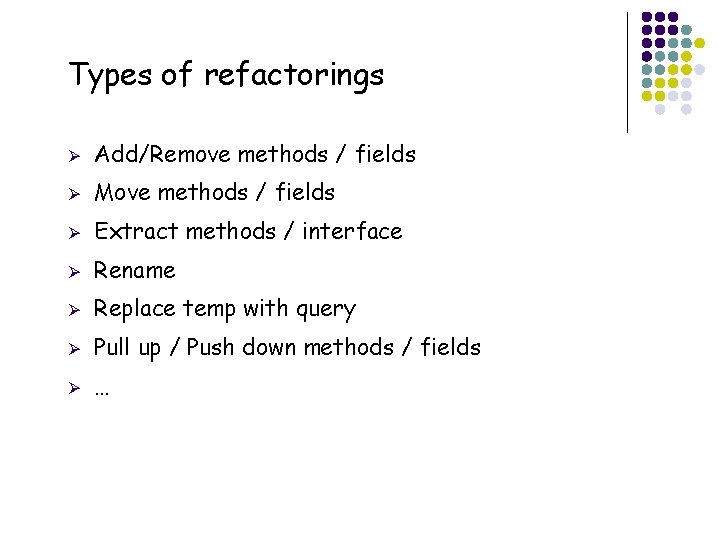
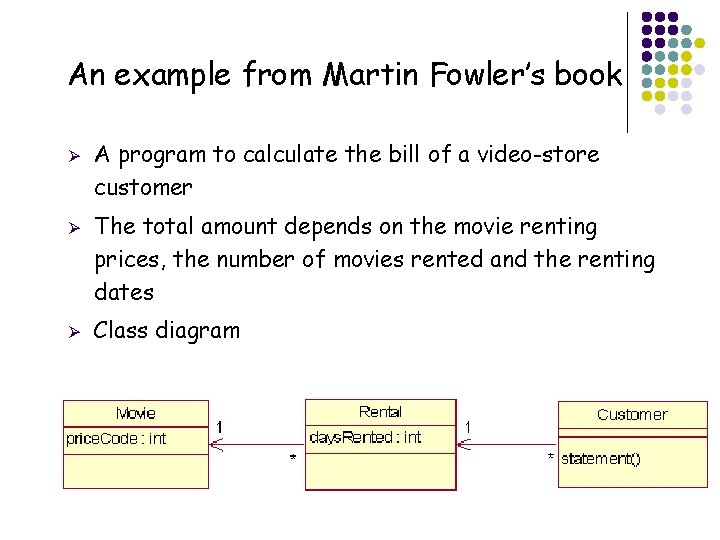
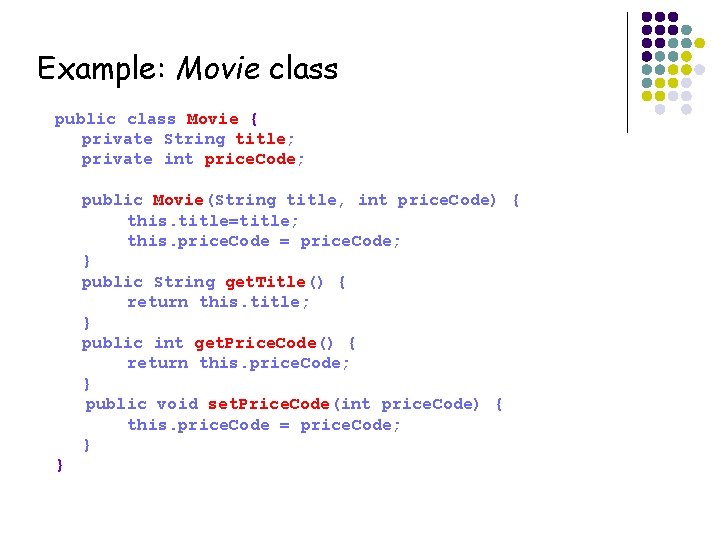
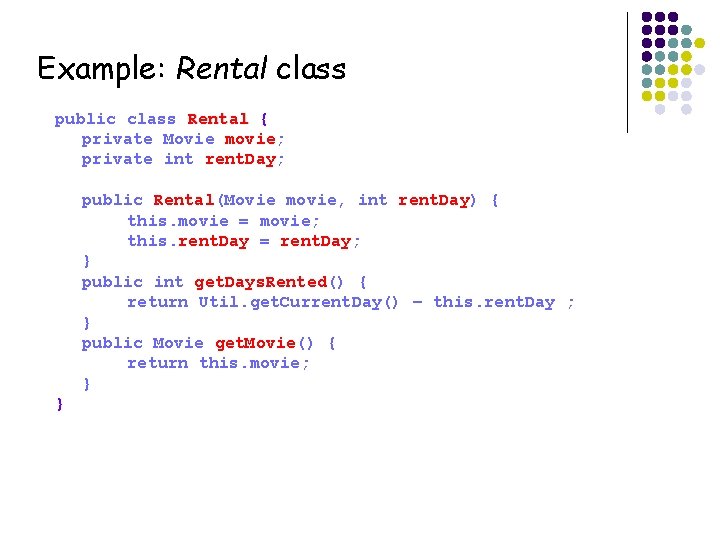
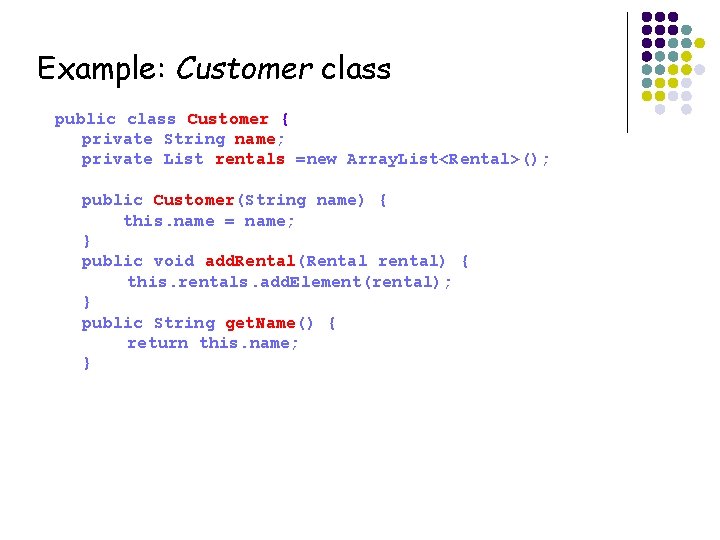
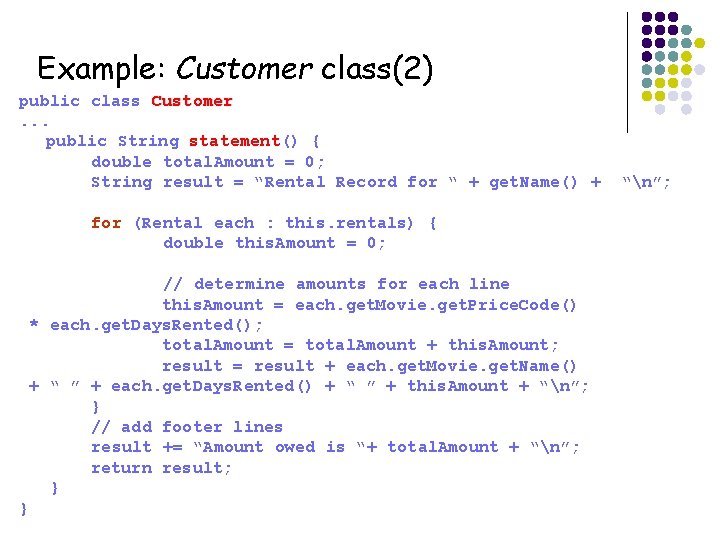
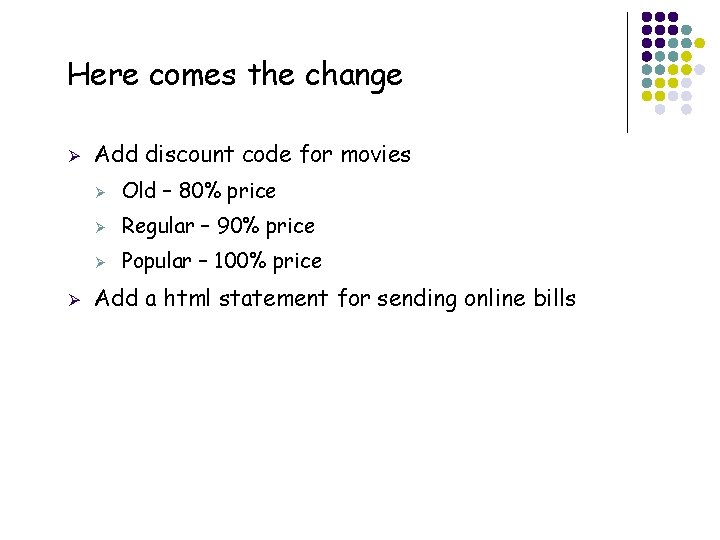
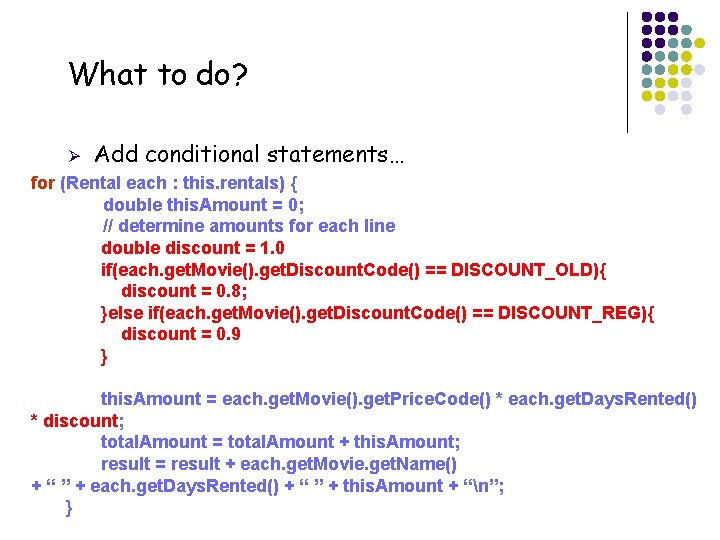
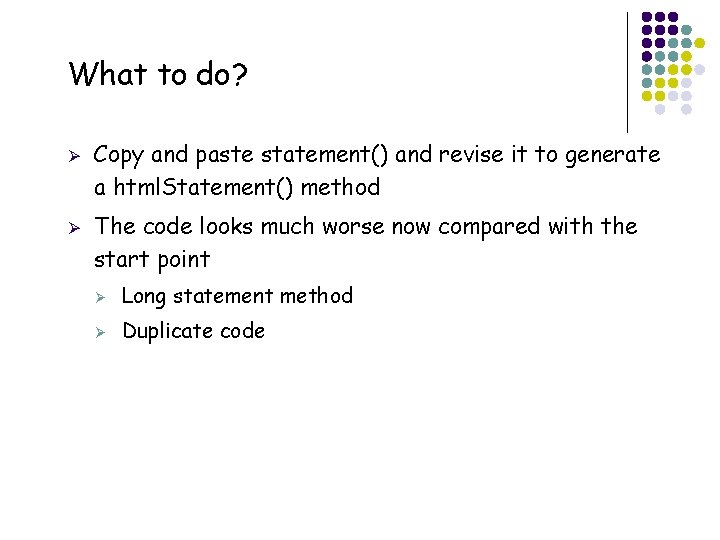
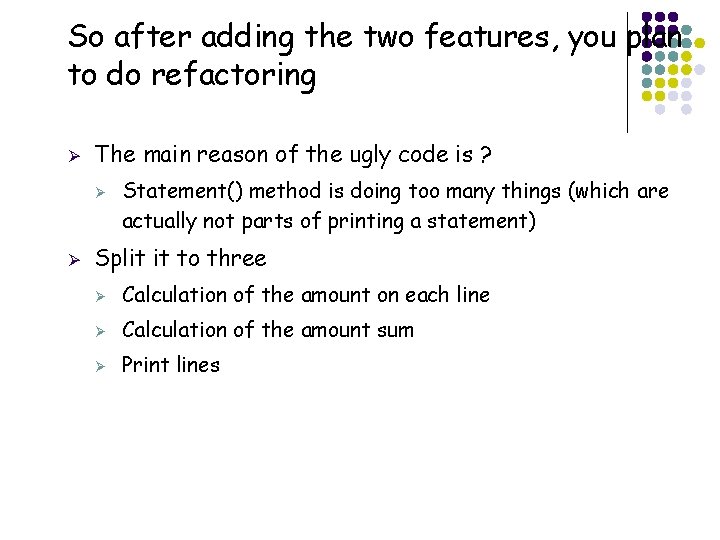
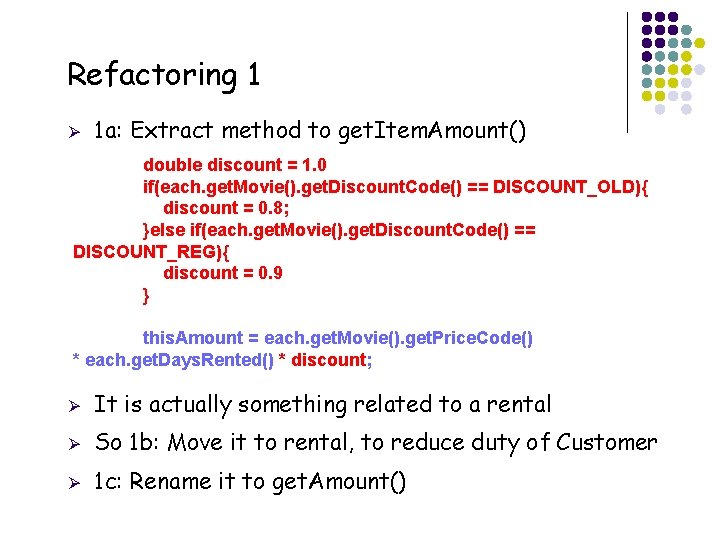
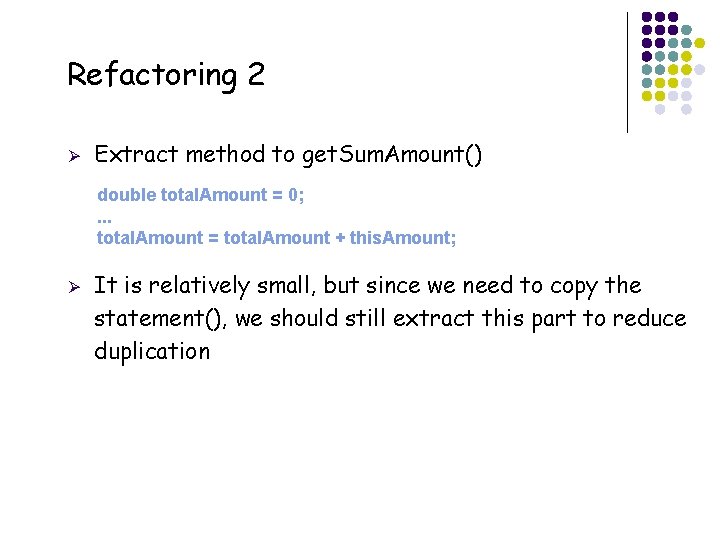
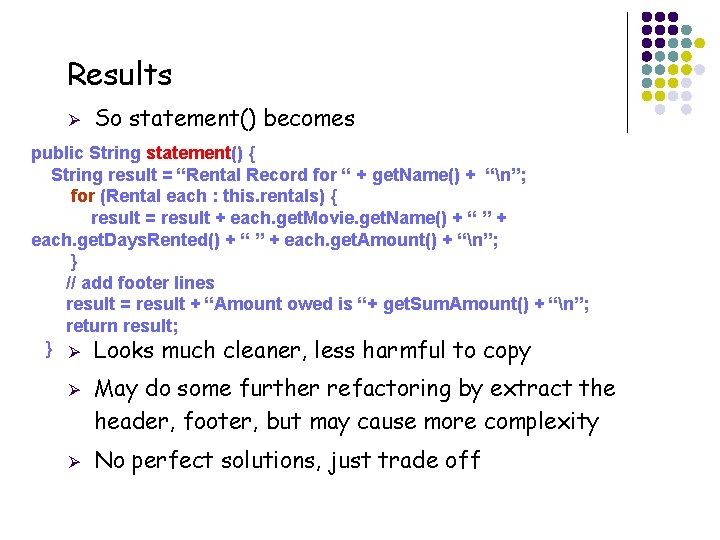
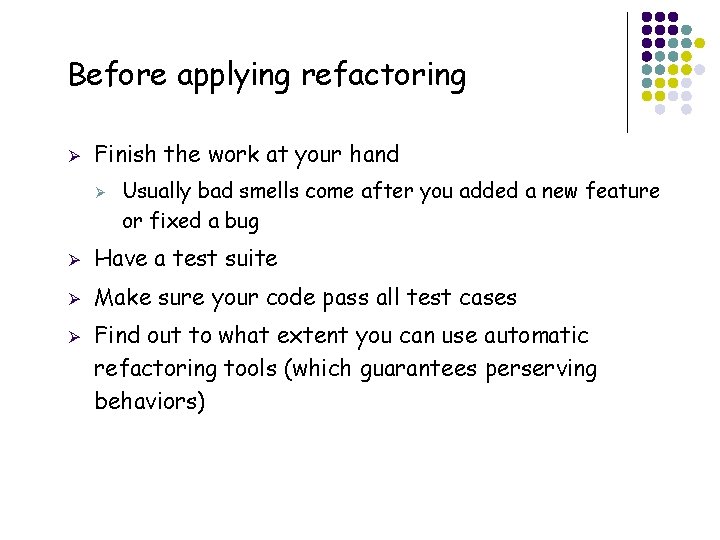
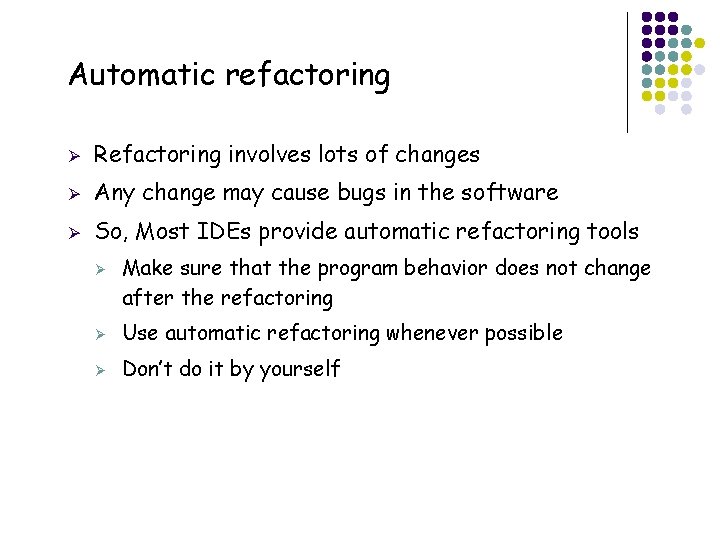
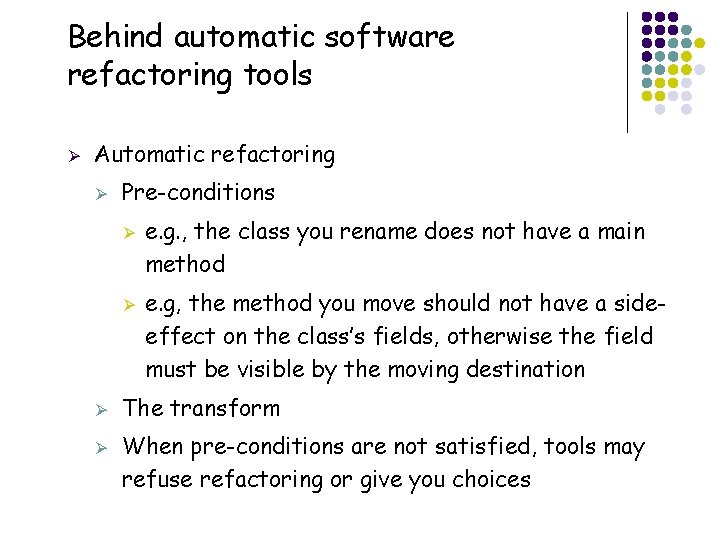
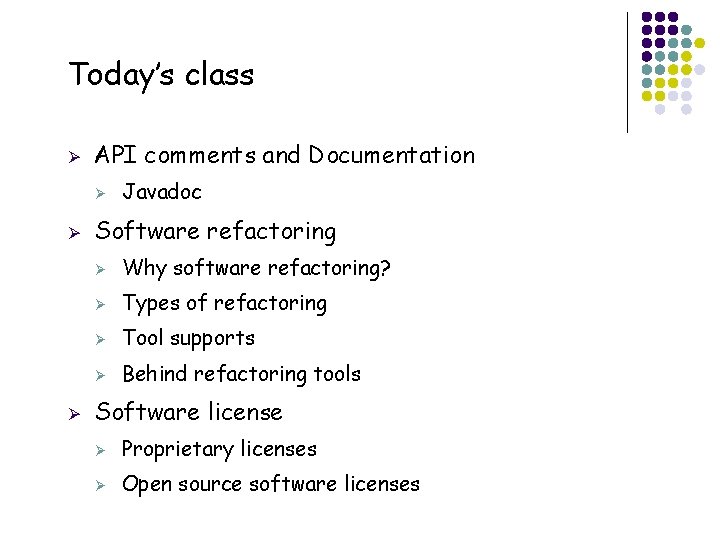
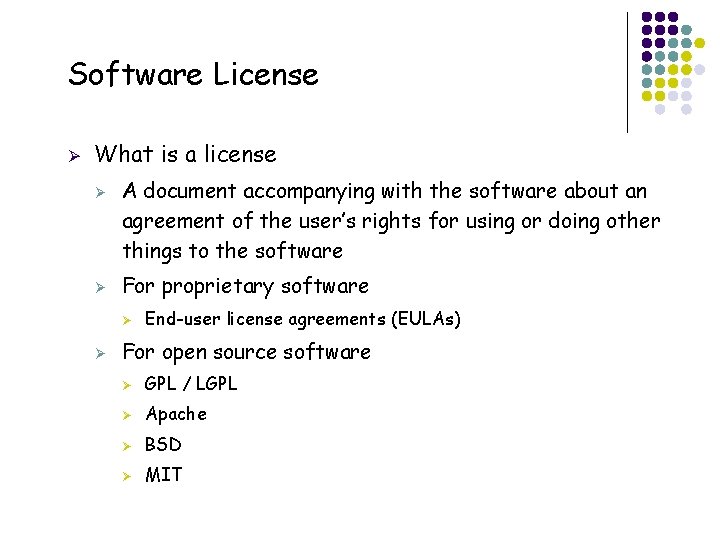
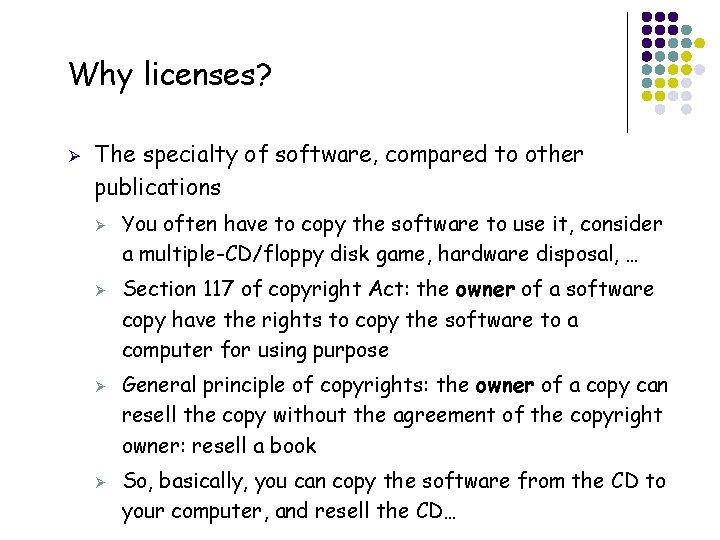
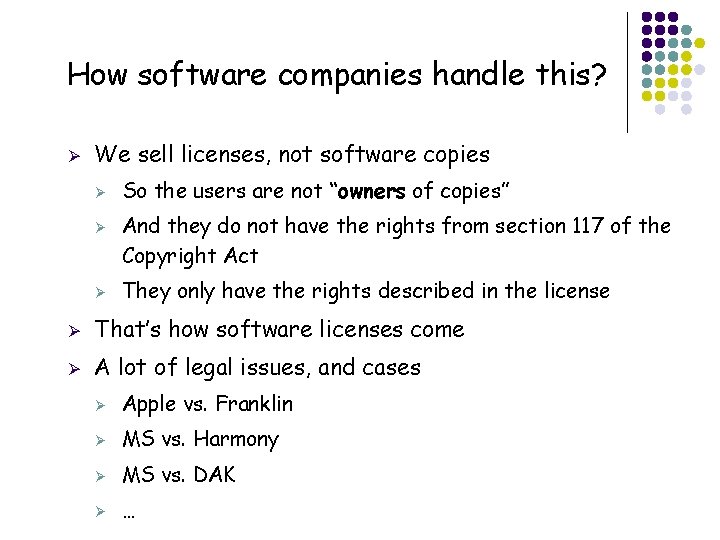
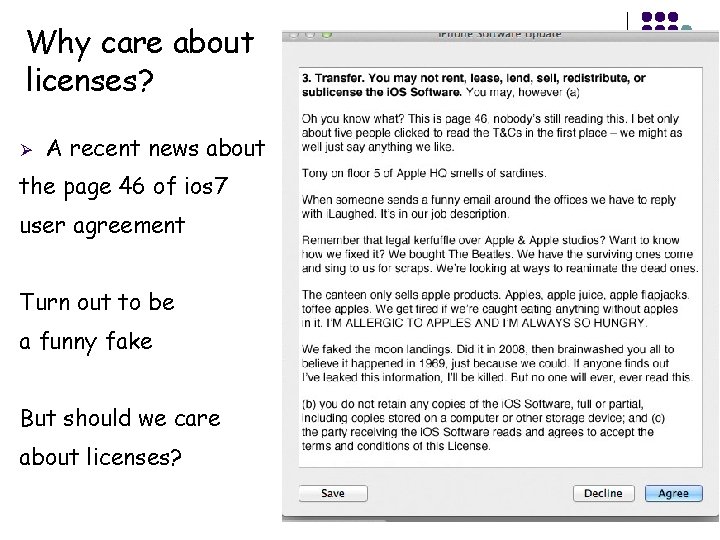
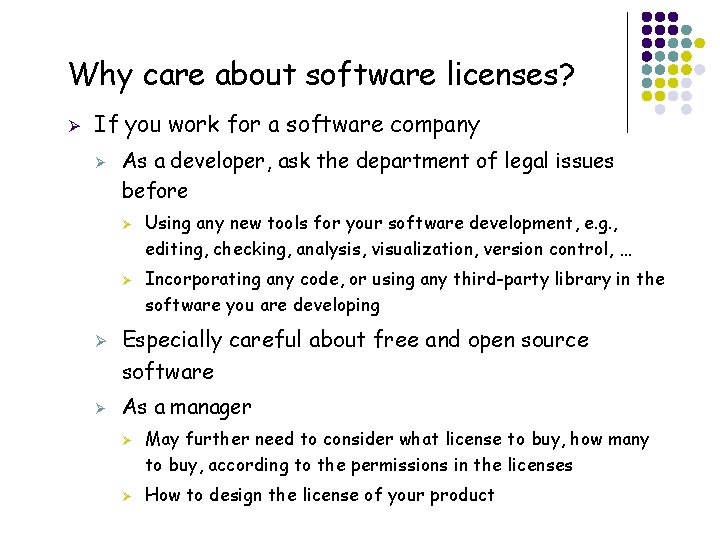
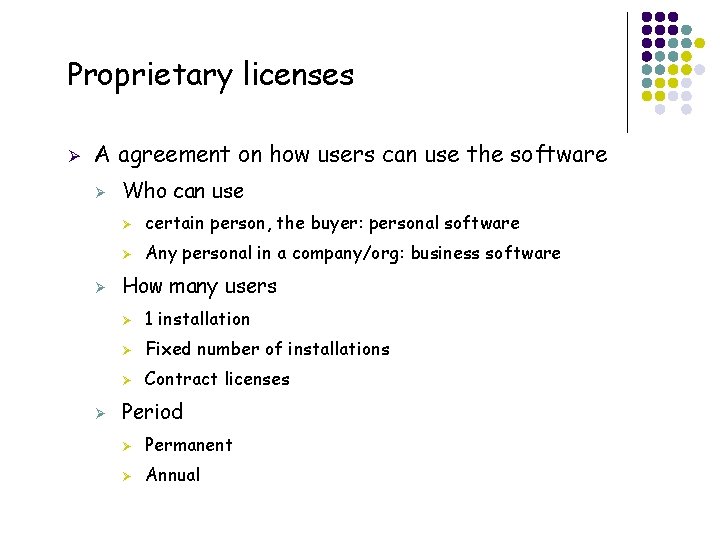
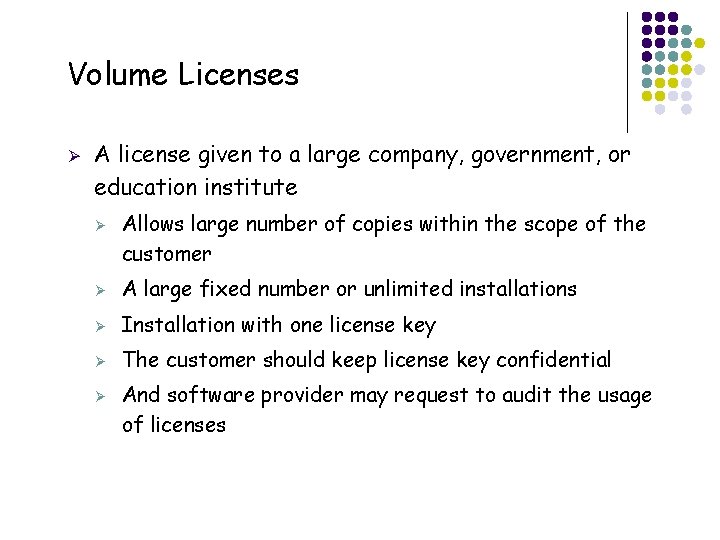
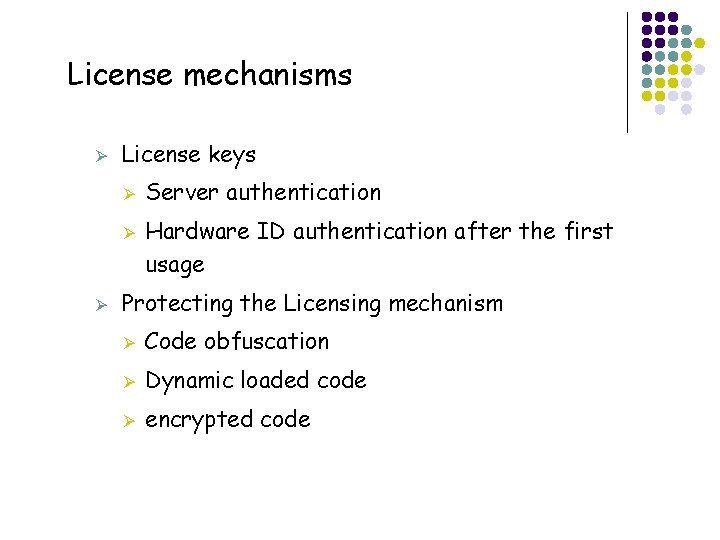
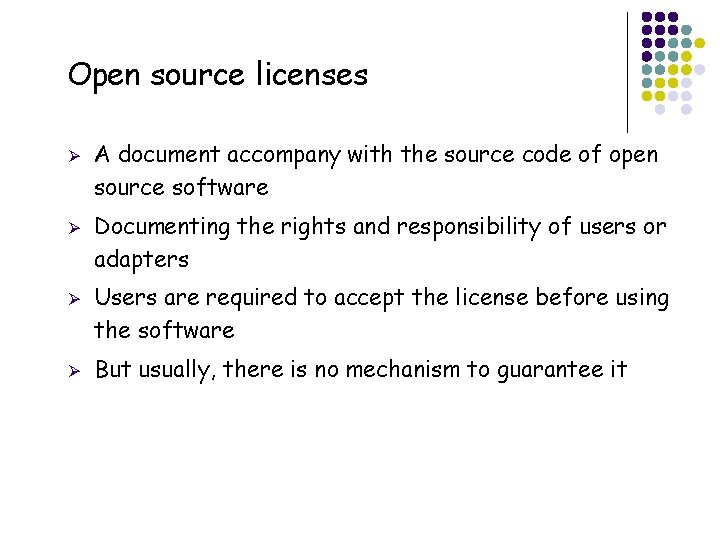
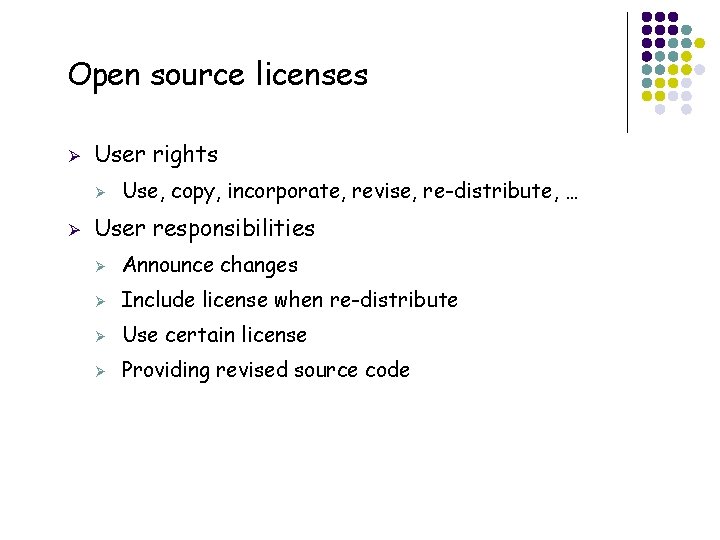
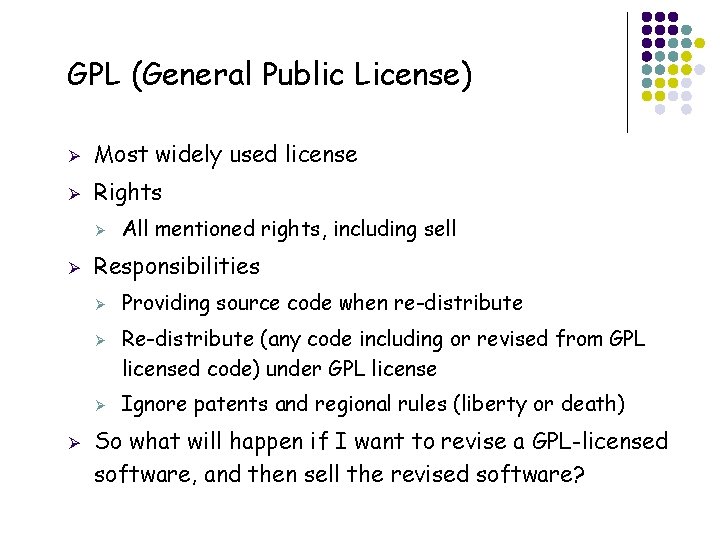
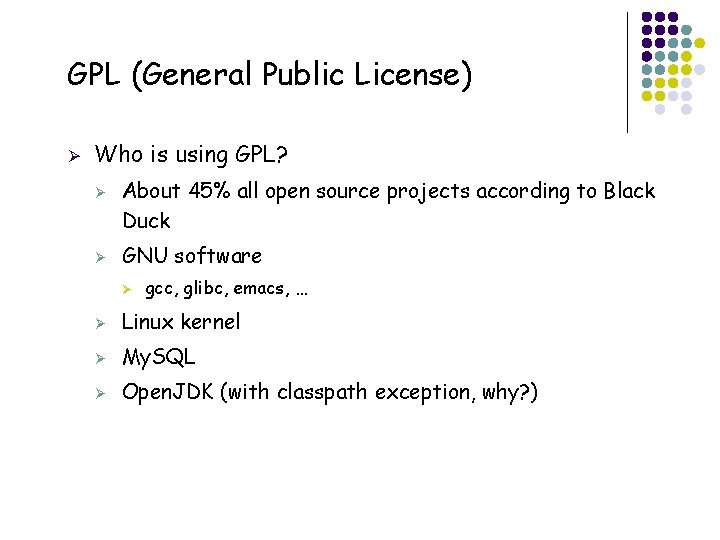
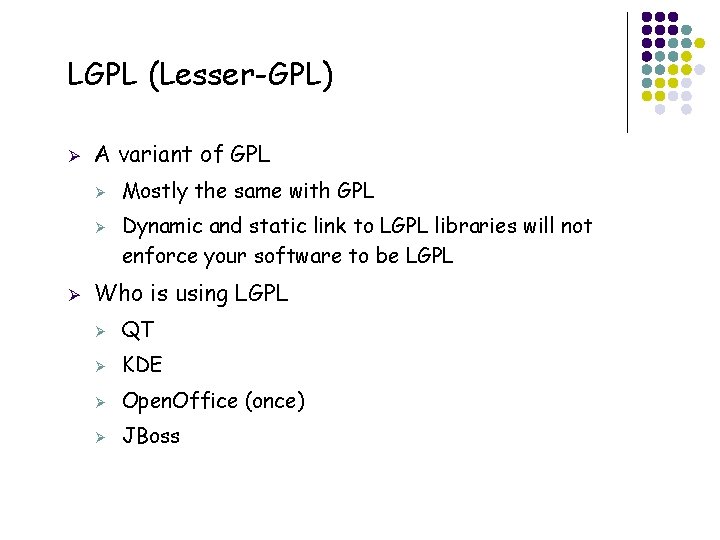
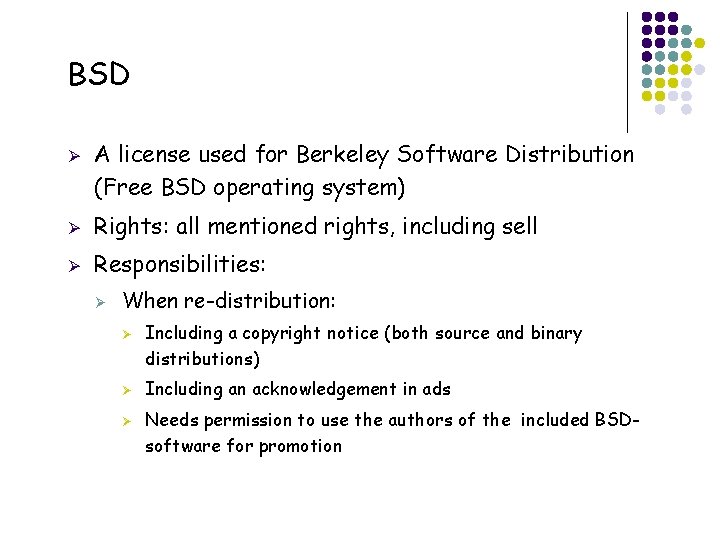
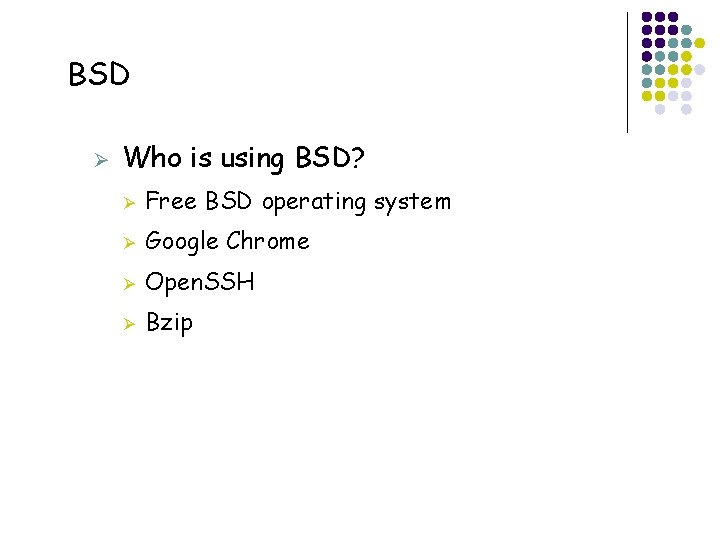
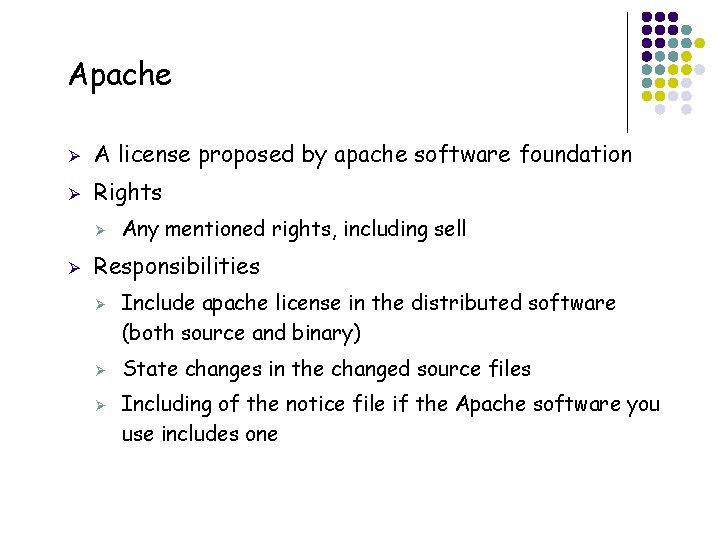
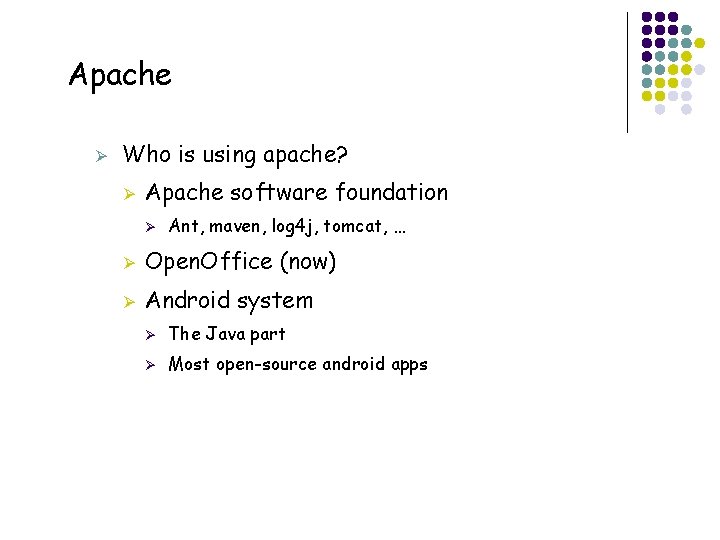
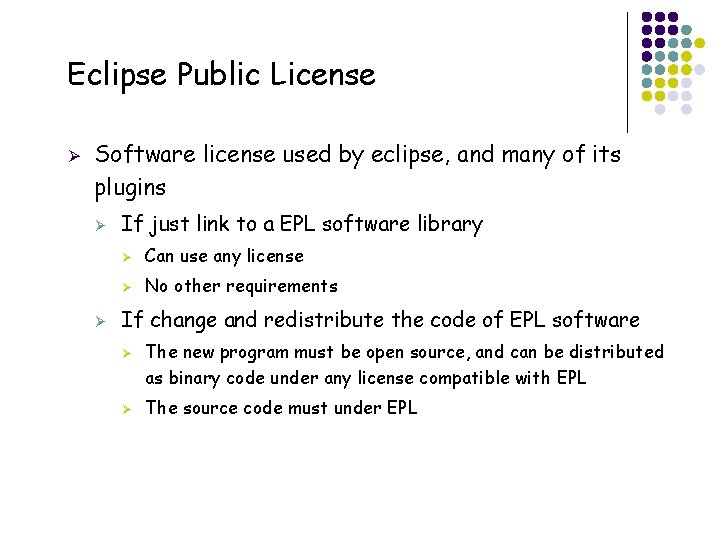
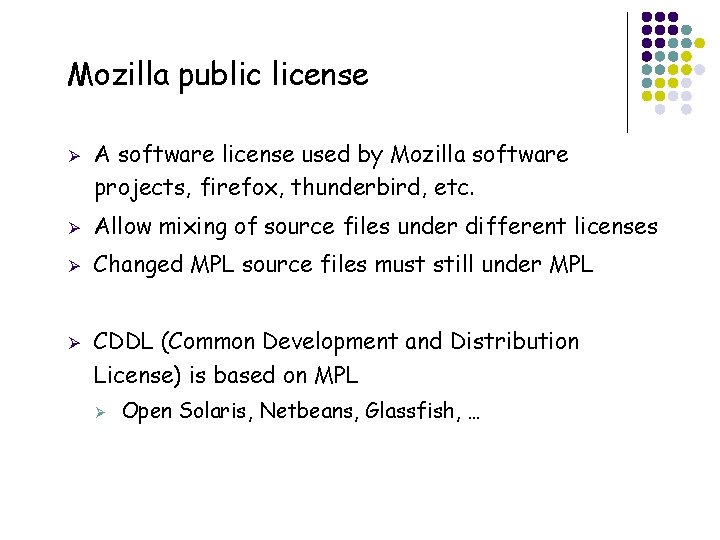
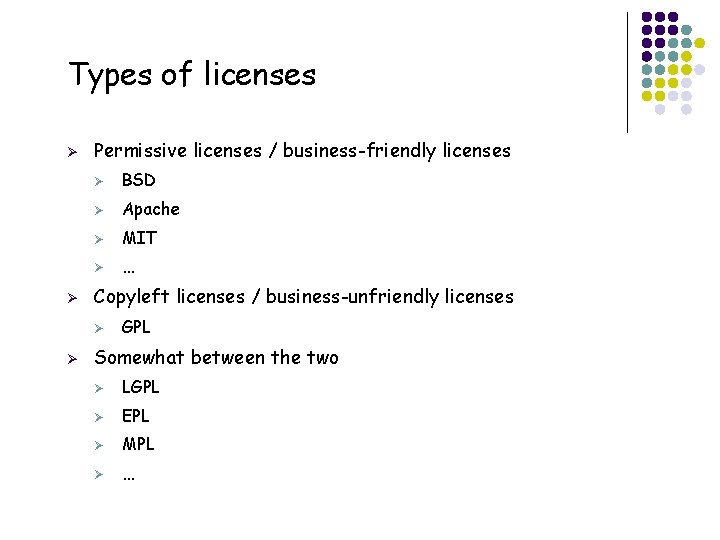
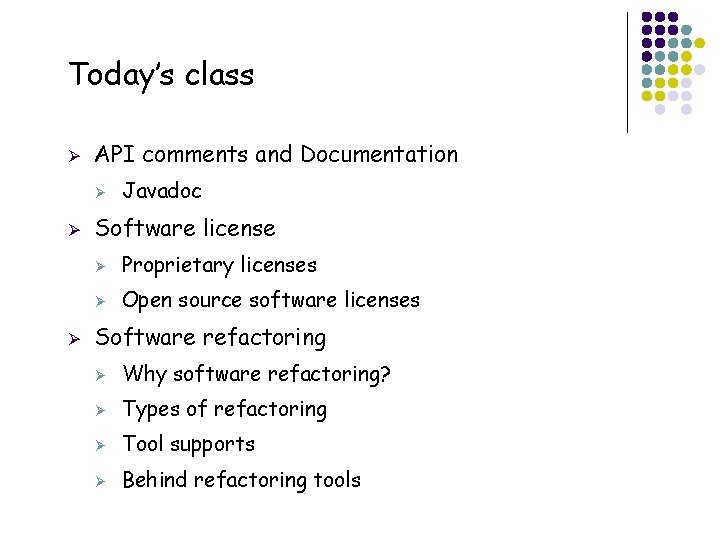
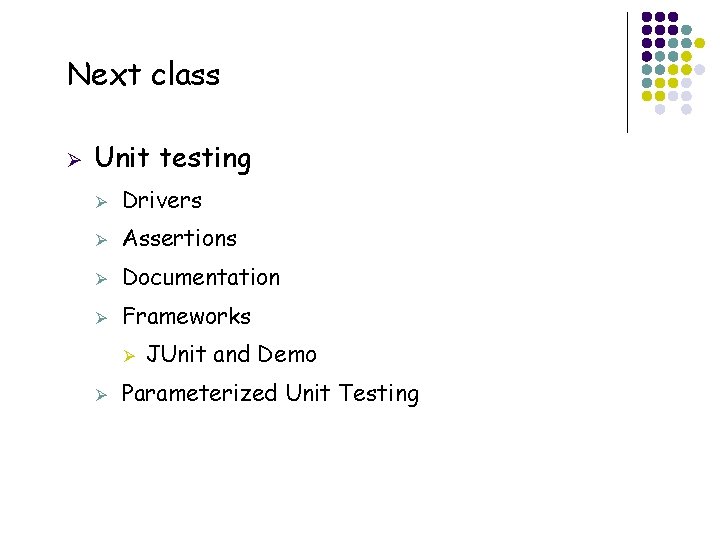
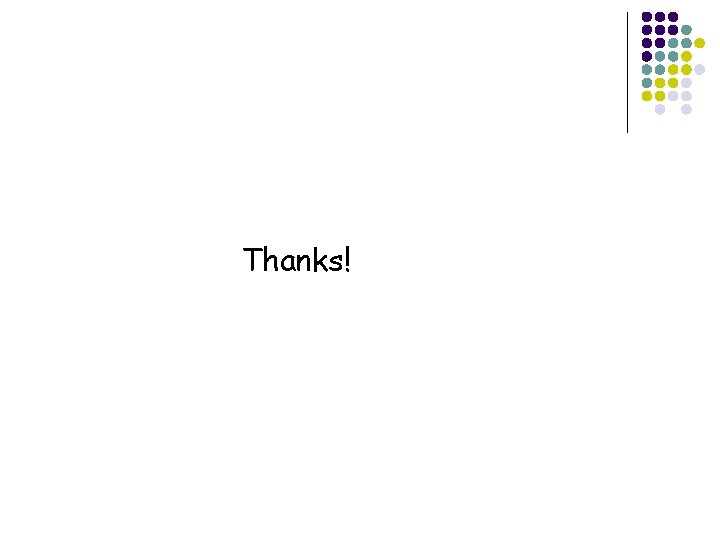
- Slides: 55
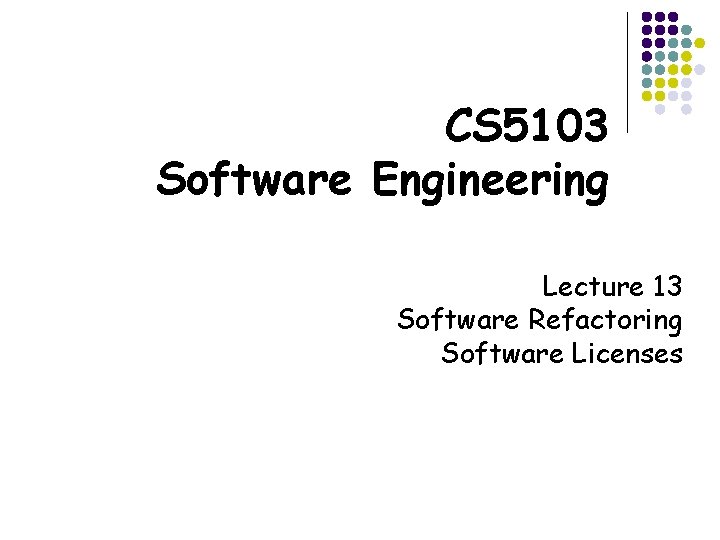
CS 5103 Software Engineering Lecture 13 Software Refactoring Software Licenses
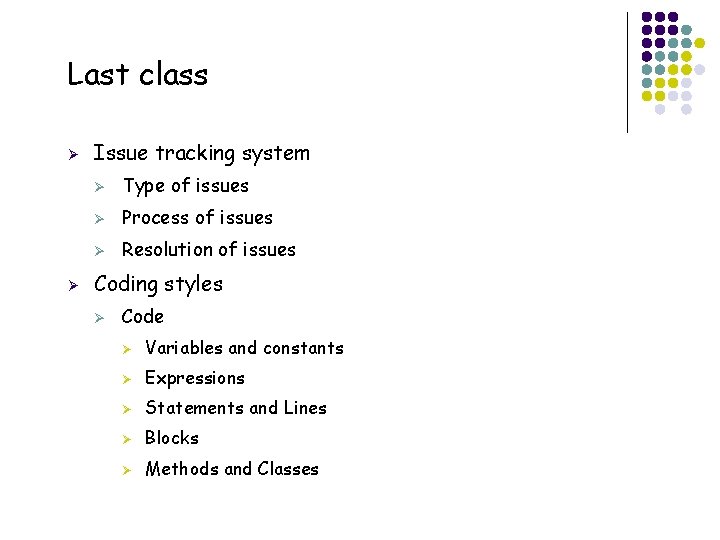
Last class Ø Ø Issue tracking system Ø Type of issues Ø Process of issues Ø Resolution of issues Coding styles Ø 2 Code Ø Variables and constants Ø Expressions Ø Statements and Lines Ø Blocks Ø Methods and Classes
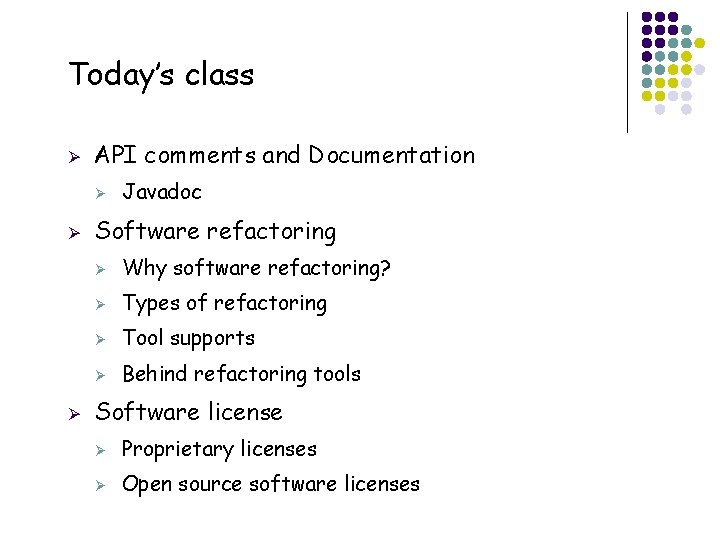
Today’s class Ø API comments and Documentation Ø Ø Ø 3 Javadoc Software refactoring Ø Why software refactoring? Ø Types of refactoring Ø Tool supports Ø Behind refactoring tools Software license Ø Proprietary licenses Ø Open source software licenses
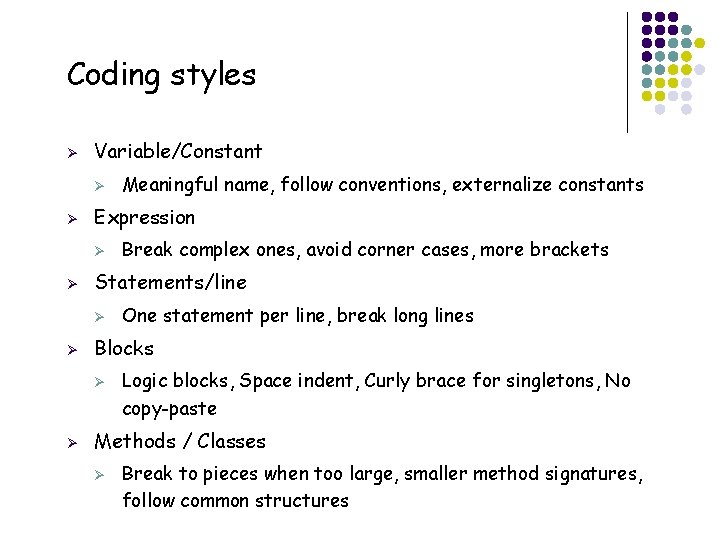
Coding styles Ø Variable/Constant Ø Ø Expression Ø Ø Logic blocks, Space indent, Curly brace for singletons, No copy-paste Methods / Classes Ø 4 One statement per line, break long lines Blocks Ø Ø Break complex ones, avoid corner cases, more brackets Statements/line Ø Ø Meaningful name, follow conventions, externalize constants Break to pieces when too large, smaller method signatures, follow common structures
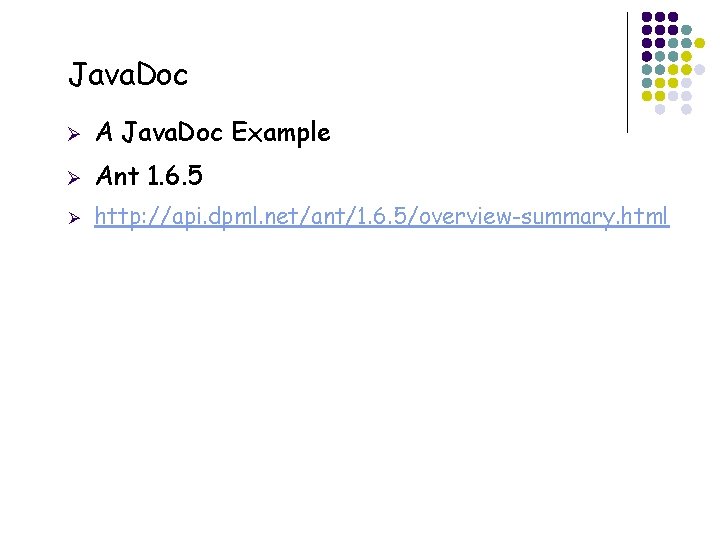
Java. Doc 5 Ø A Java. Doc Example Ø Ant 1. 6. 5 Ø http: //api. dpml. net/ant/1. 6. 5/overview-summary. html
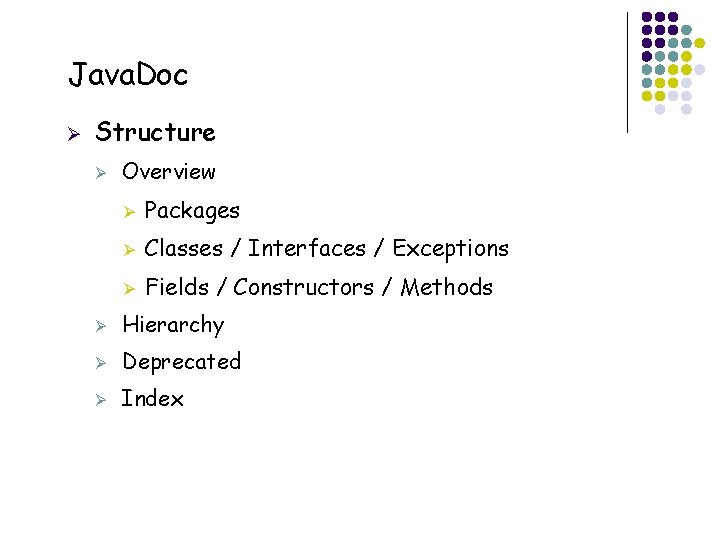
Java. Doc Ø Structure Ø 6 Overview Ø Packages Ø Classes / Interfaces / Exceptions Ø Fields / Constructors / Methods Ø Hierarchy Ø Deprecated Ø Index
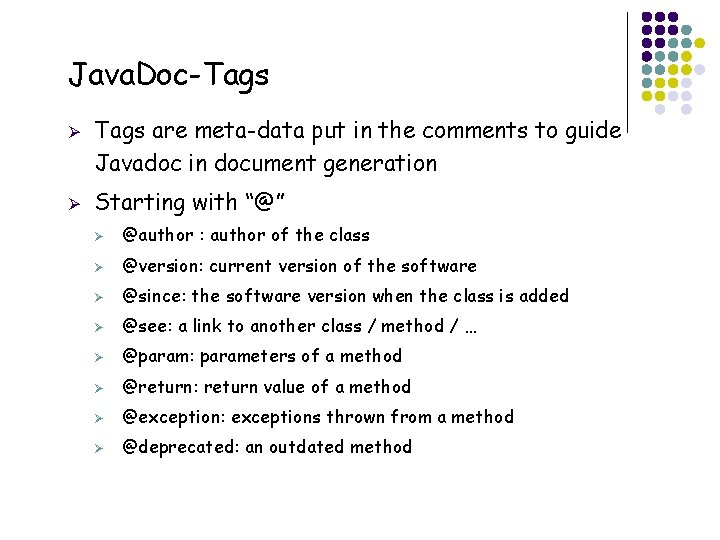
Java. Doc-Tags Ø Ø 7 Tags are meta-data put in the comments to guide Javadoc in document generation Starting with “@” Ø @author : author of the class Ø @version: current version of the software Ø @since: the software version when the class is added Ø @see: a link to another class / method / … Ø @param: parameters of a method Ø @return: return value of a method Ø @exception: exceptions thrown from a method Ø @deprecated: an outdated method
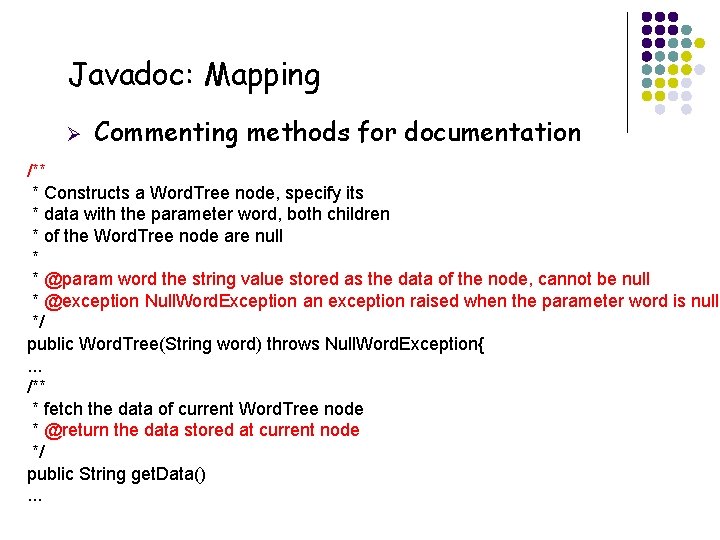
Javadoc: Mapping Ø Commenting methods for documentation /** * Constructs a Word. Tree node, specify its * data with the parameter word, both children * of the Word. Tree node are null * * @param word the string value stored as the data of the node, cannot be null * @exception Null. Word. Exception an exception raised when the parameter word is null */ public Word. Tree(String word) throws Null. Word. Exception{. . . /** * fetch the data of current Word. Tree node * @return the data stored at current node */ public String get. Data(). . . 8
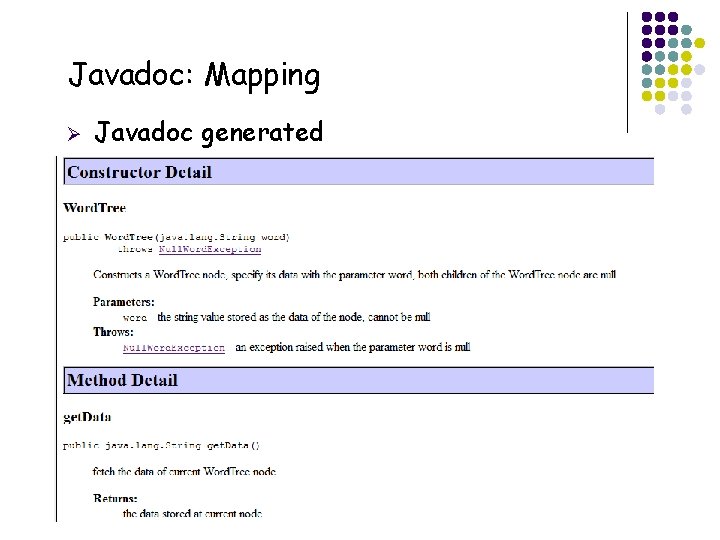
Javadoc: Mapping Ø 9 Javadoc generated
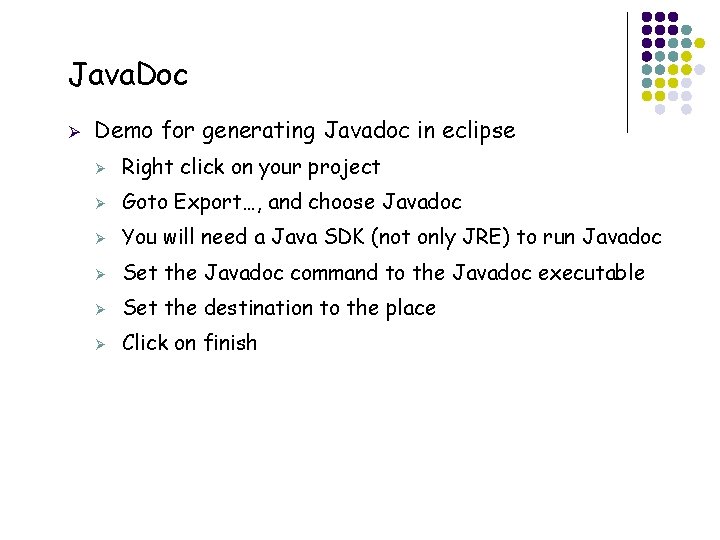
Java. Doc Ø 10 Demo for generating Javadoc in eclipse Ø Right click on your project Ø Goto Export…, and choose Javadoc Ø You will need a Java SDK (not only JRE) to run Javadoc Ø Set the Javadoc command to the Javadoc executable Ø Set the destination to the place Ø Click on finish
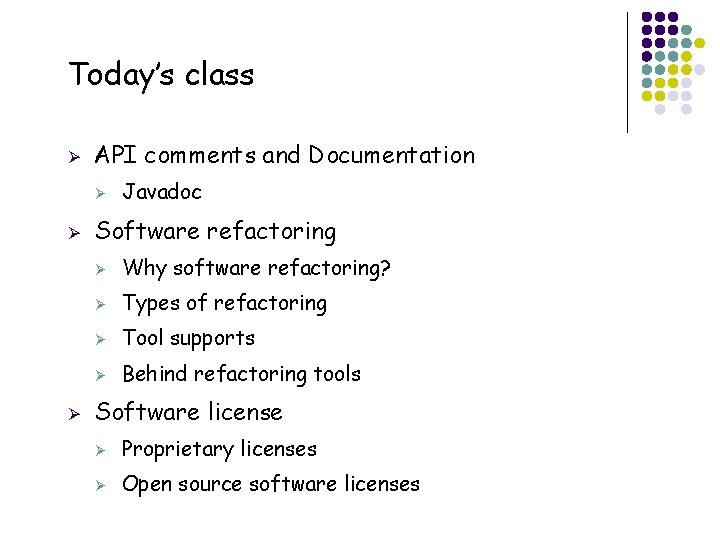
Today’s class Ø API comments and Documentation Ø Ø Ø 11 Javadoc Software refactoring Ø Why software refactoring? Ø Types of refactoring Ø Tool supports Ø Behind refactoring tools Software license Ø Proprietary licenses Ø Open source software licenses
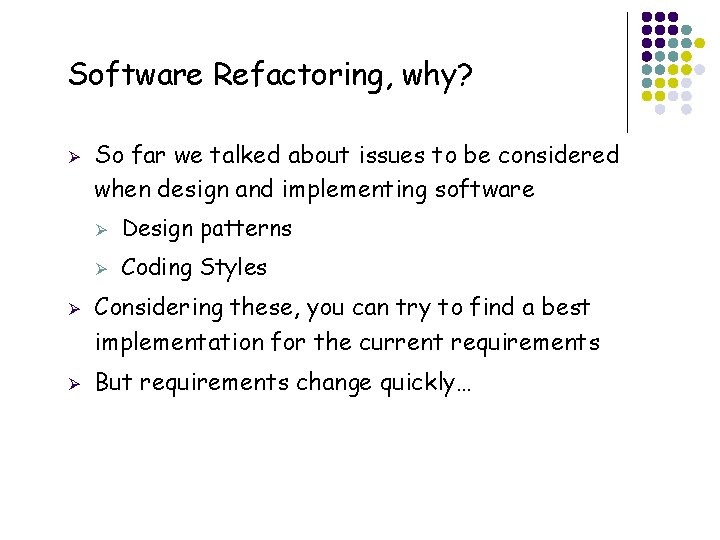
Software Refactoring, why? Ø Ø Ø 12 So far we talked about issues to be considered when design and implementing software Ø Design patterns Ø Coding Styles Considering these, you can try to find a best implementation for the current requirements But requirements change quickly…
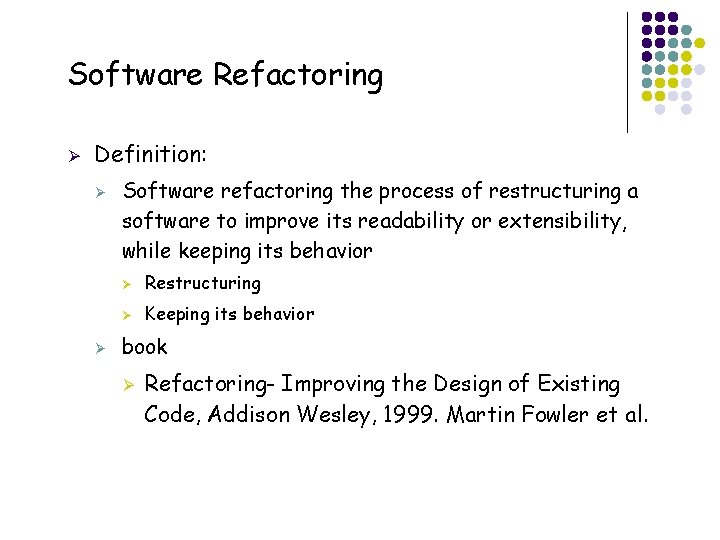
Software Refactoring Ø Definition: Ø Ø Software refactoring the process of restructuring a software to improve its readability or extensibility, while keeping its behavior Ø Restructuring Ø Keeping its behavior book Ø 13 Refactoring- Improving the Design of Existing Code, Addison Wesley, 1999. Martin Fowler et al.
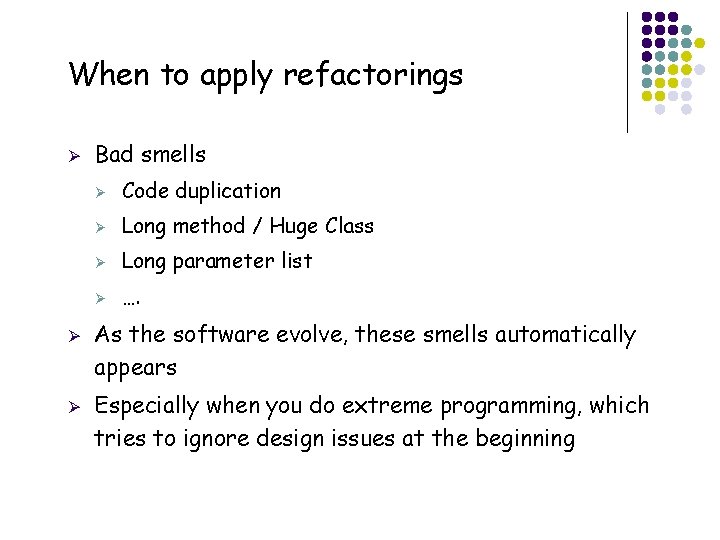
When to apply refactorings Ø Ø Ø 14 Bad smells Ø Code duplication Ø Long method / Huge Class Ø Long parameter list Ø …. As the software evolve, these smells automatically appears Especially when you do extreme programming, which tries to ignore design issues at the beginning
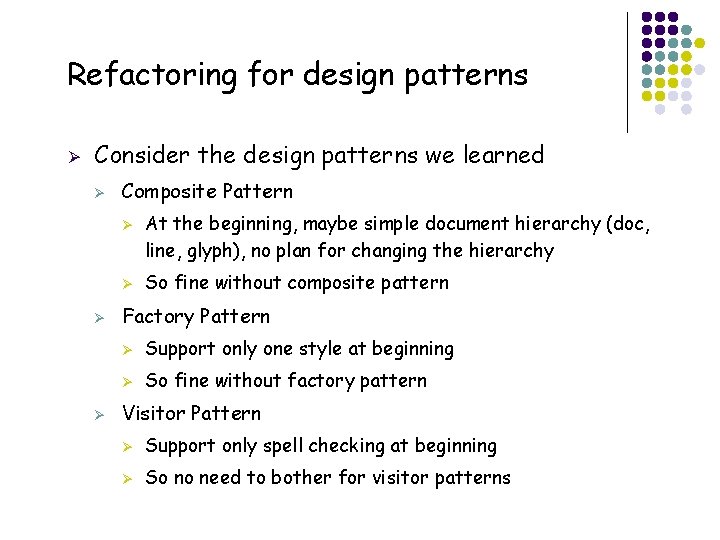
Refactoring for design patterns Ø Consider the design patterns we learned Ø Composite Pattern Ø Ø 15 At the beginning, maybe simple document hierarchy (doc, line, glyph), no plan for changing the hierarchy So fine without composite pattern Factory Pattern Ø Support only one style at beginning Ø So fine without factory pattern Visitor Pattern Ø Support only spell checking at beginning Ø So no need to bother for visitor patterns
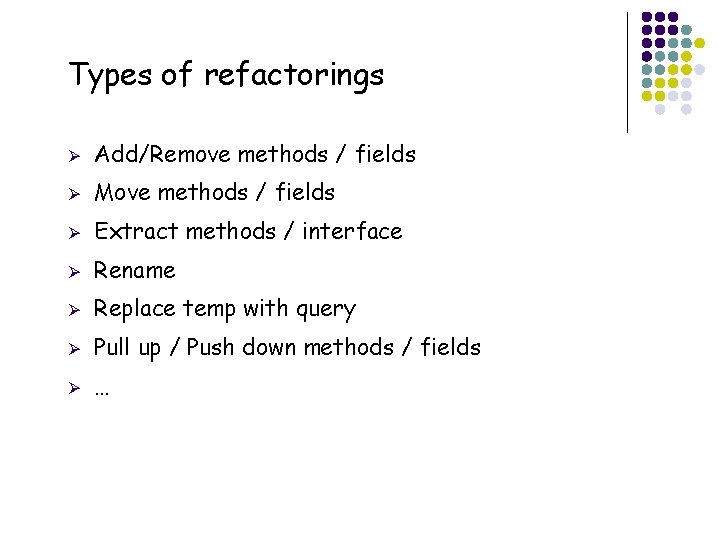
Types of refactorings 16 Ø Add/Remove methods / fields Ø Move methods / fields Ø Extract methods / interface Ø Rename Ø Replace temp with query Ø Pull up / Push down methods / fields Ø …
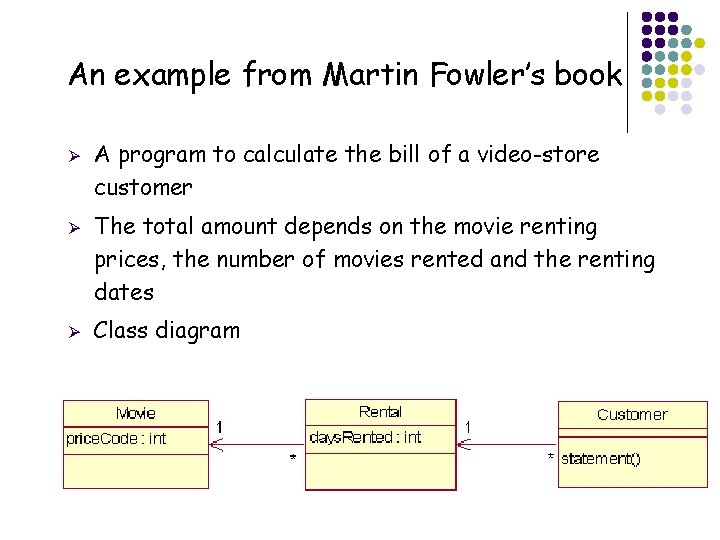
An example from Martin Fowler’s book Ø Ø Ø 17 A program to calculate the bill of a video-store customer The total amount depends on the movie renting prices, the number of movies rented and the renting dates Class diagram
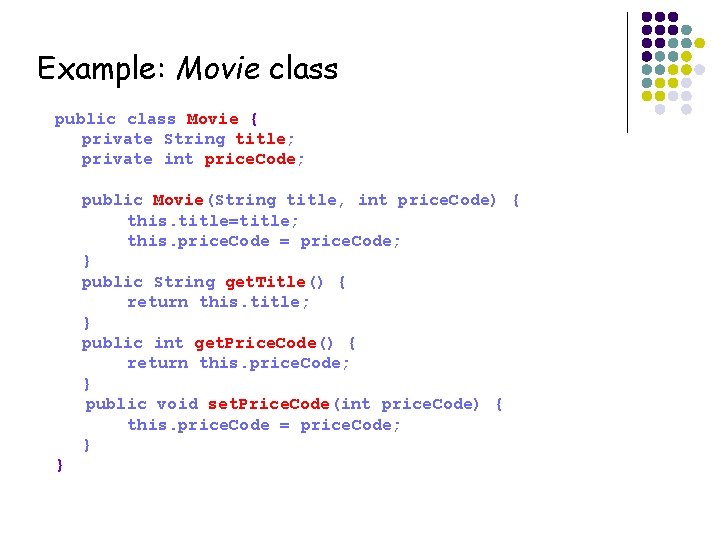
Example: Movie class public class Movie { private String title; private int price. Code; public Movie(String title, int price. Code) { this. title=title; this. price. Code = price. Code; } public String get. Title() { return this. title; } public int get. Price. Code() { return this. price. Code; } public void set. Price. Code(int price. Code) { this. price. Code = price. Code; } }
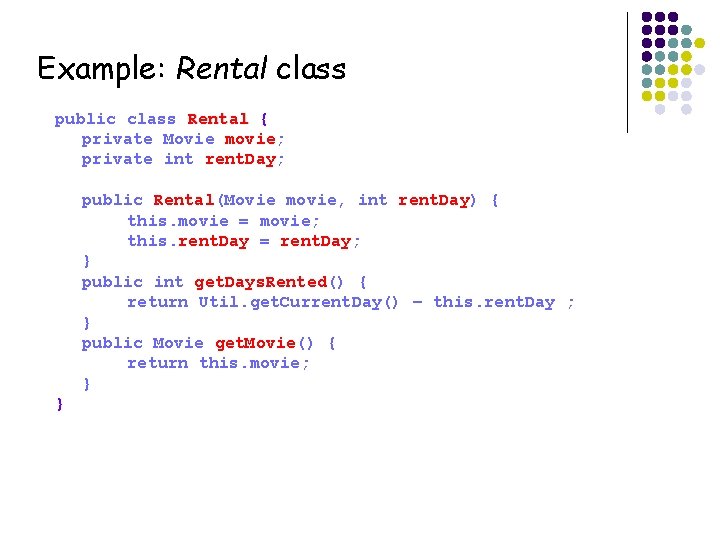
Example: Rental class public class Rental { private Movie movie; private int rent. Day; public Rental(Movie movie, int rent. Day) { this. movie = movie; this. rent. Day = rent. Day; } public int get. Days. Rented() { return Util. get. Current. Day() – this. rent. Day ; } public Movie get. Movie() { return this. movie; } }
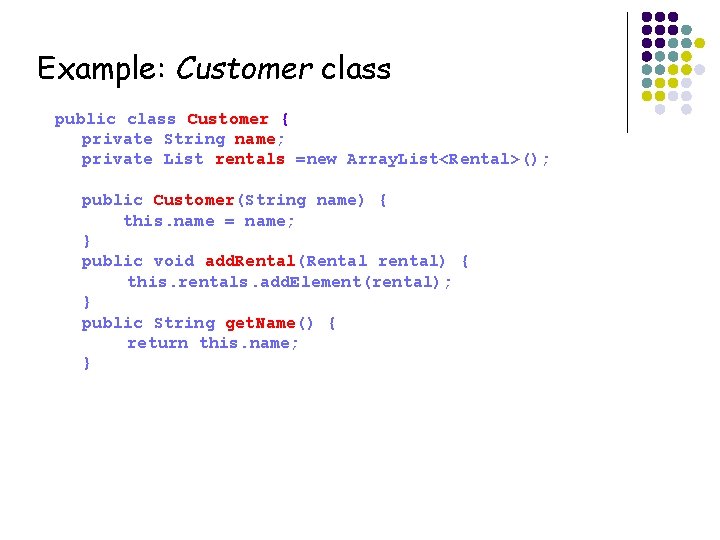
Example: Customer class public class Customer { private String name; private List rentals =new Array. List<Rental>(); public Customer(String name) { this. name = name; } public void add. Rental(Rental rental) { this. rentals. add. Element(rental); } public String get. Name() { return this. name; }
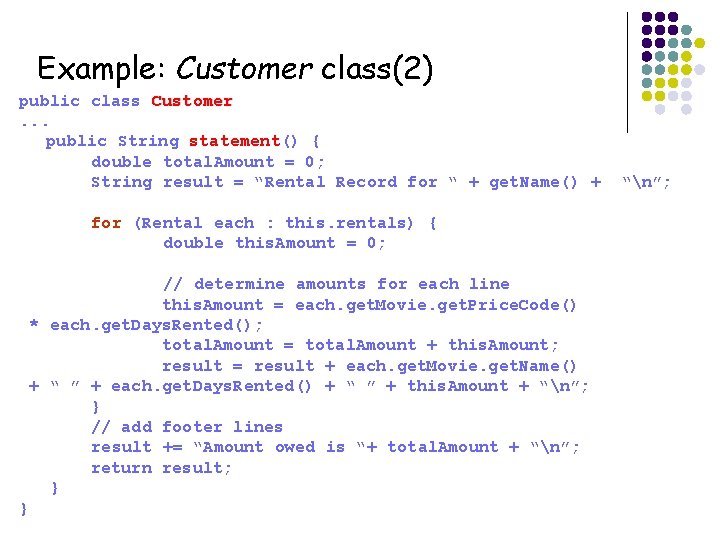
Example: Customer class(2) public class Customer. . . public String statement() { double total. Amount = 0; String result = “Rental Record for “ + get. Name() + for (Rental each : this. rentals) { double this. Amount = 0; // determine amounts for each line this. Amount = each. get. Movie. get. Price. Code() * each. get. Days. Rented(); total. Amount = total. Amount + this. Amount; result = result + each. get. Movie. get. Name() + “ ” + each. get. Days. Rented() + “ ” + this. Amount + “n”; } // add footer lines result += “Amount owed is “+ total. Amount + “n”; return result; } } “n”;
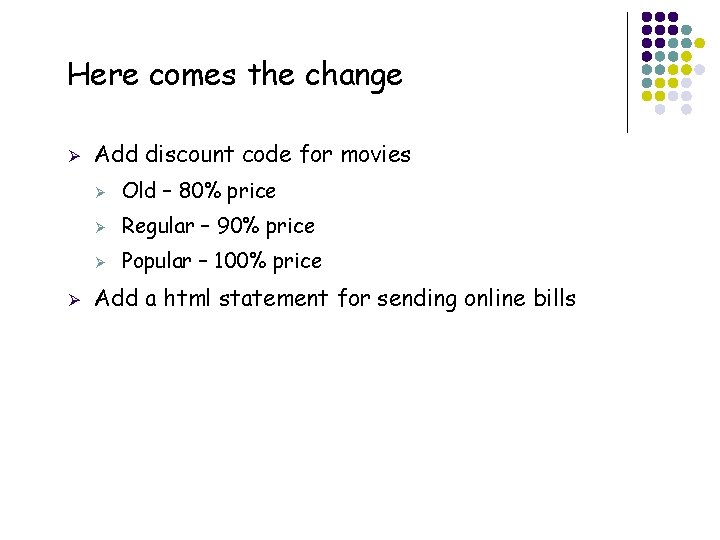
Here comes the change Ø Ø 22 Add discount code for movies Ø Old – 80% price Ø Regular – 90% price Ø Popular – 100% price Add a html statement for sending online bills
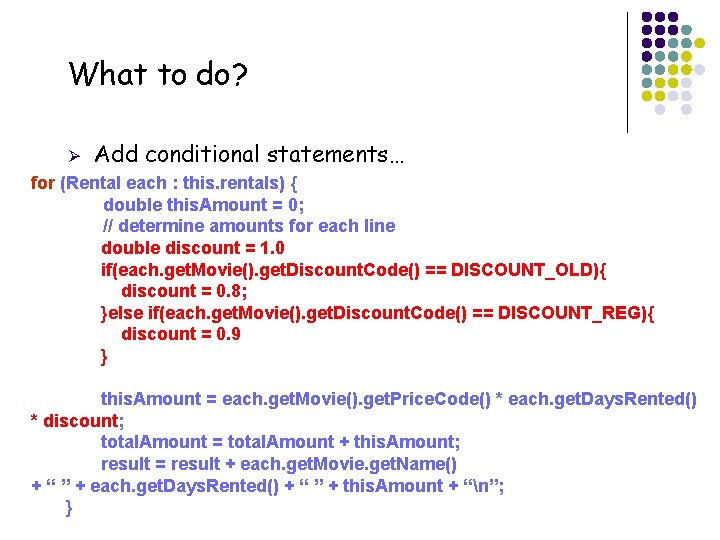
What to do? Ø Add conditional statements… for (Rental each : this. rentals) { double this. Amount = 0; // determine amounts for each line double discount = 1. 0 if(each. get. Movie(). get. Discount. Code() == DISCOUNT_OLD){ discount = 0. 8; }else if(each. get. Movie(). get. Discount. Code() == DISCOUNT_REG){ discount = 0. 9 } this. Amount = each. get. Movie(). get. Price. Code() * each. get. Days. Rented() * discount; total. Amount = total. Amount + this. Amount; result = result + each. get. Movie. get. Name() + “ ” + each. get. Days. Rented() + “ ” + this. Amount + “n”; } 23
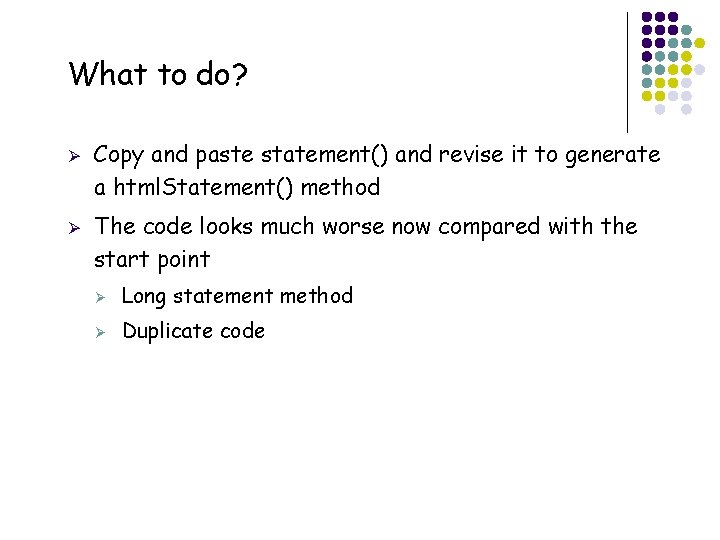
What to do? Ø Ø 24 Copy and paste statement() and revise it to generate a html. Statement() method The code looks much worse now compared with the start point Ø Long statement method Ø Duplicate code
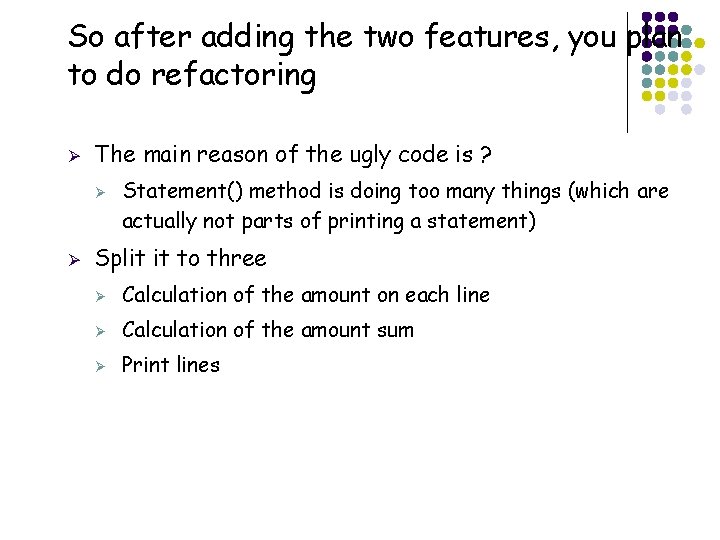
So after adding the two features, you plan to do refactoring Ø The main reason of the ugly code is ? Ø Ø 25 Statement() method is doing too many things (which are actually not parts of printing a statement) Split it to three Ø Calculation of the amount on each line Ø Calculation of the amount sum Ø Print lines
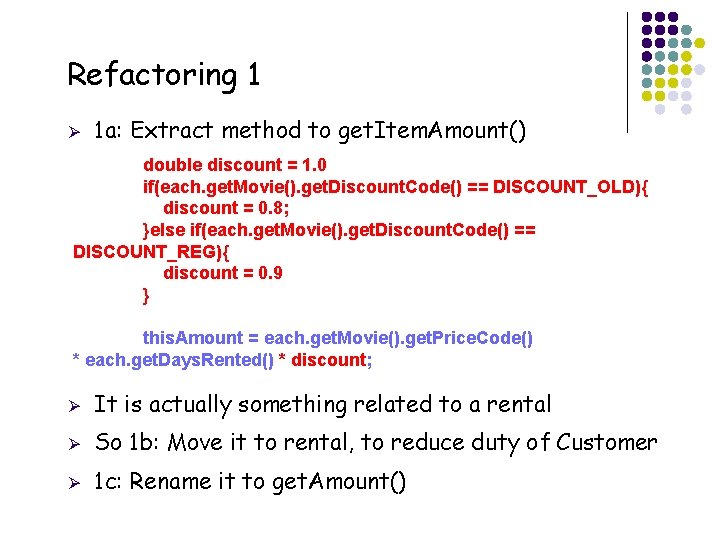
Refactoring 1 Ø 1 a: Extract method to get. Item. Amount() double discount = 1. 0 if(each. get. Movie(). get. Discount. Code() == DISCOUNT_OLD){ discount = 0. 8; }else if(each. get. Movie(). get. Discount. Code() == DISCOUNT_REG){ discount = 0. 9 } this. Amount = each. get. Movie(). get. Price. Code() * each. get. Days. Rented() * discount; 26 Ø It is actually something related to a rental Ø So 1 b: Move it to rental, to reduce duty of Customer Ø 1 c: Rename it to get. Amount()
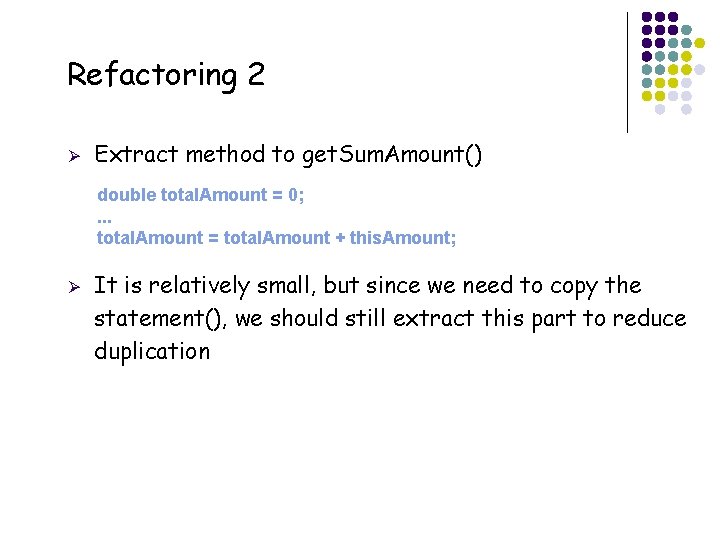
Refactoring 2 Ø Extract method to get. Sum. Amount() double total. Amount = 0; . . . total. Amount = total. Amount + this. Amount; Ø 27 It is relatively small, but since we need to copy the statement(), we should still extract this part to reduce duplication
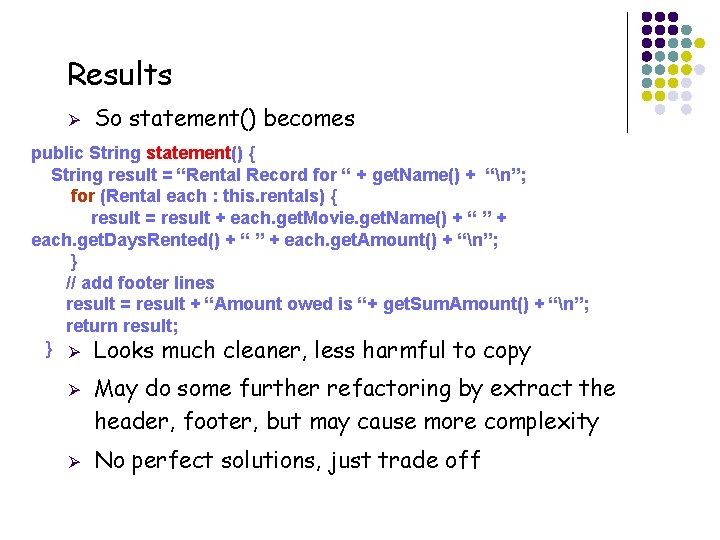
Results Ø So statement() becomes public String statement() { String result = “Rental Record for “ + get. Name() + “n”; for (Rental each : this. rentals) { result = result + each. get. Movie. get. Name() + “ ” + each. get. Days. Rented() + “ ” + each. get. Amount() + “n”; } // add footer lines result = result + “Amount owed is “+ get. Sum. Amount() + “n”; return result; } Ø Looks much cleaner, less harmful to copy Ø Ø 28 May do some further refactoring by extract the header, footer, but may cause more complexity No perfect solutions, just trade off
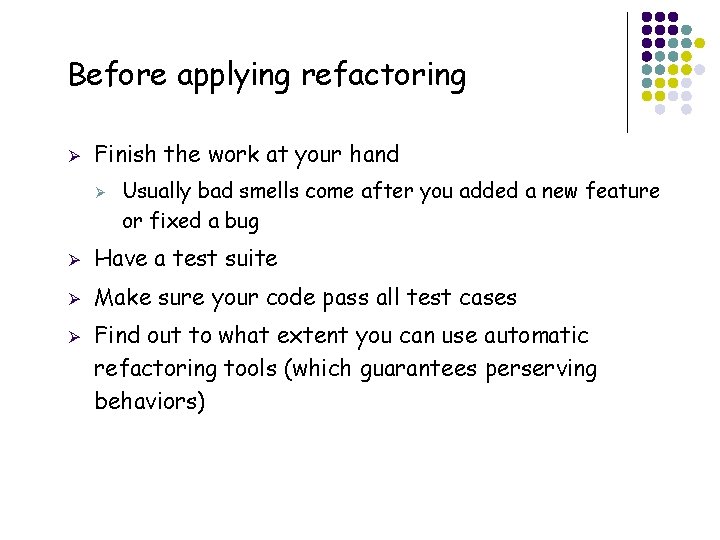
Before applying refactoring Ø Finish the work at your hand Ø Ø Have a test suite Ø Make sure your code pass all test cases Ø 29 Usually bad smells come after you added a new feature or fixed a bug Find out to what extent you can use automatic refactoring tools (which guarantees perserving behaviors)
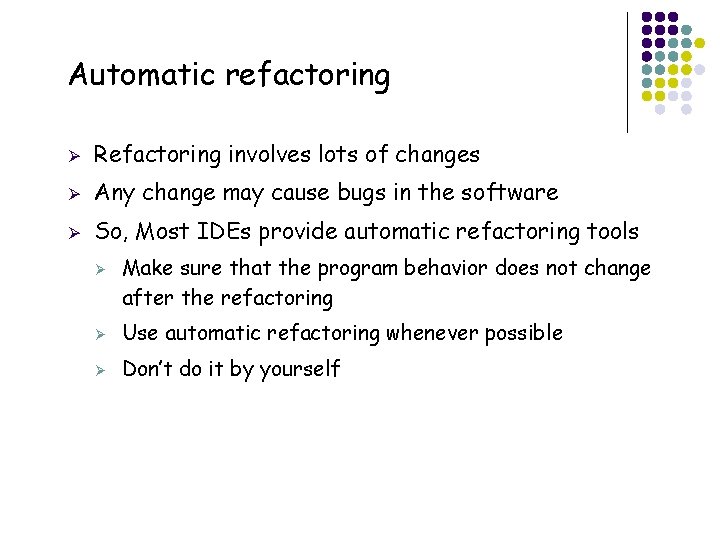
Automatic refactoring Ø Refactoring involves lots of changes Ø Any change may cause bugs in the software Ø So, Most IDEs provide automatic refactoring tools Ø 30 Make sure that the program behavior does not change after the refactoring Ø Use automatic refactoring whenever possible Ø Don’t do it by yourself
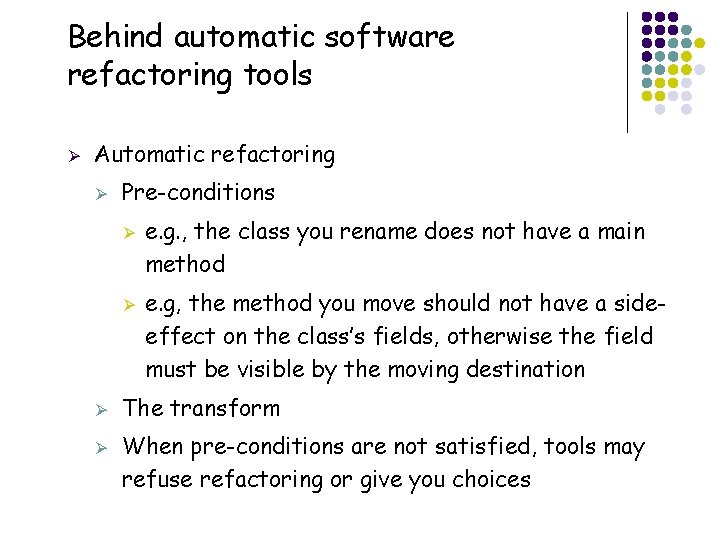
Behind automatic software refactoring tools Ø Automatic refactoring Ø Pre-conditions Ø Ø 31 e. g. , the class you rename does not have a main method e. g, the method you move should not have a sideeffect on the class’s fields, otherwise the field must be visible by the moving destination The transform When pre-conditions are not satisfied, tools may refuse refactoring or give you choices
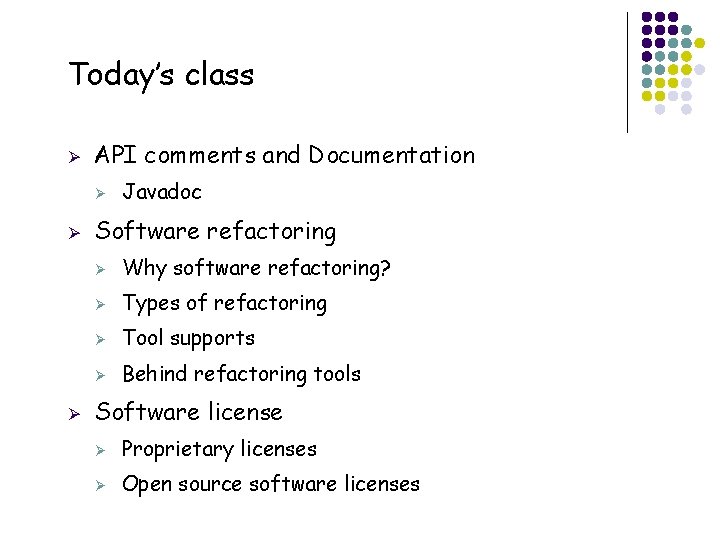
Today’s class Ø API comments and Documentation Ø Ø Ø 32 Javadoc Software refactoring Ø Why software refactoring? Ø Types of refactoring Ø Tool supports Ø Behind refactoring tools Software license Ø Proprietary licenses Ø Open source software licenses
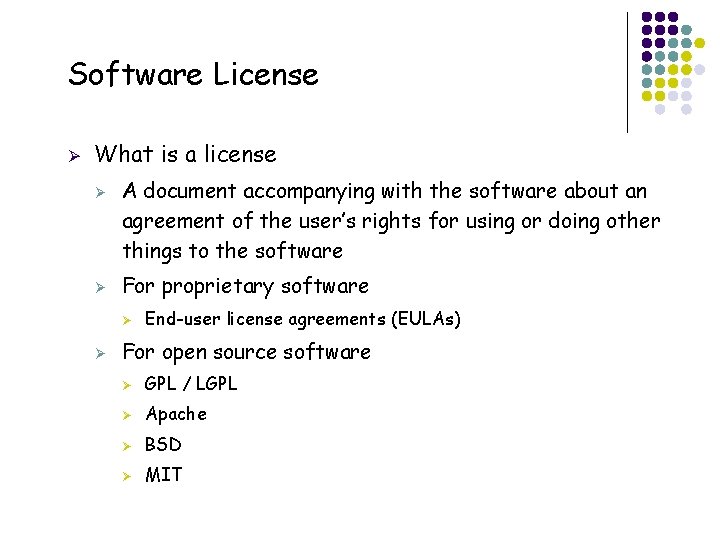
Software License Ø What is a license Ø Ø A document accompanying with the software about an agreement of the user’s rights for using or doing other things to the software For proprietary software Ø Ø 33 End-user license agreements (EULAs) For open source software Ø GPL / LGPL Ø Apache Ø BSD Ø MIT
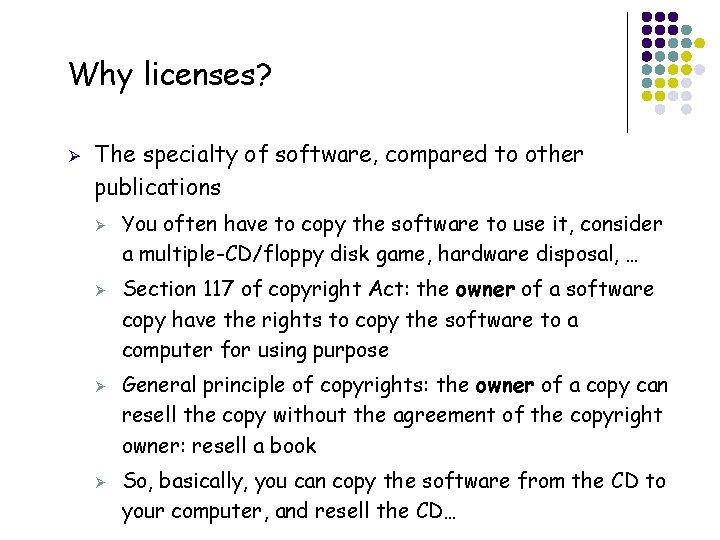
Why licenses? Ø The specialty of software, compared to other publications Ø Ø 34 You often have to copy the software to use it, consider a multiple-CD/floppy disk game, hardware disposal, … Section 117 of copyright Act: the owner of a software copy have the rights to copy the software to a computer for using purpose General principle of copyrights: the owner of a copy can resell the copy without the agreement of the copyright owner: resell a book So, basically, you can copy the software from the CD to your computer, and resell the CD…
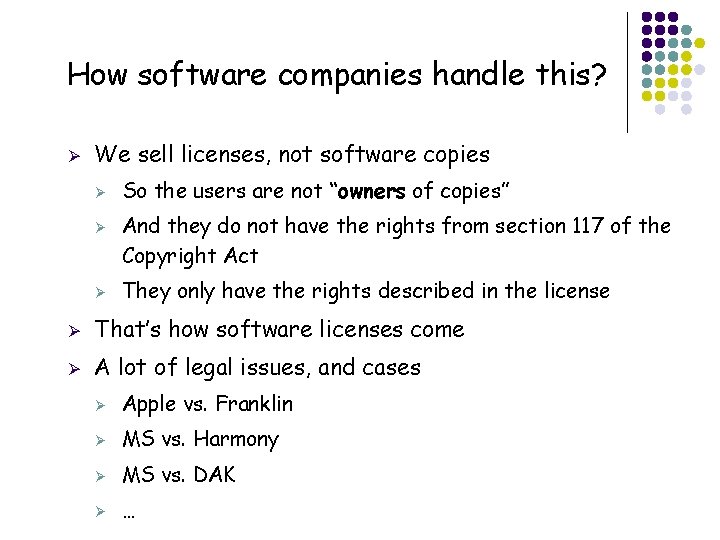
How software companies handle this? Ø We sell licenses, not software copies Ø Ø Ø 35 So the users are not “owners of copies” And they do not have the rights from section 117 of the Copyright Act They only have the rights described in the license Ø That’s how software licenses come Ø A lot of legal issues, and cases Ø Apple vs. Franklin Ø MS vs. Harmony Ø MS vs. DAK Ø …
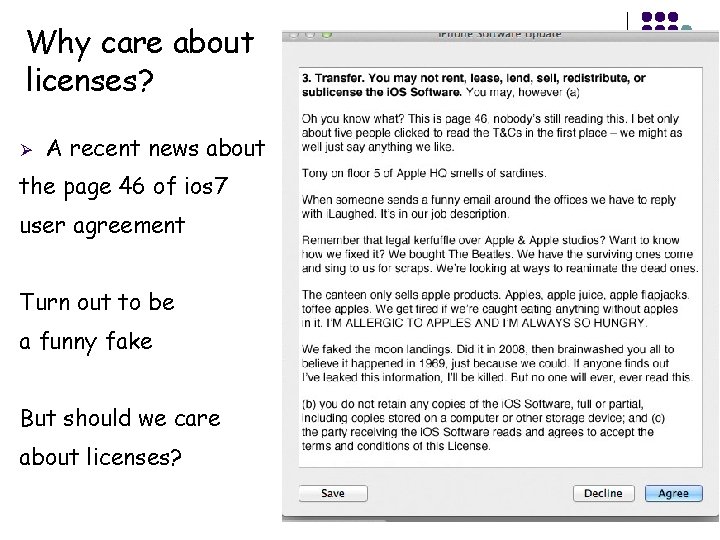
Why care about licenses? Ø A recent news about the page 46 of ios 7 user agreement Turn out to be a funny fake But should we care about licenses? 36
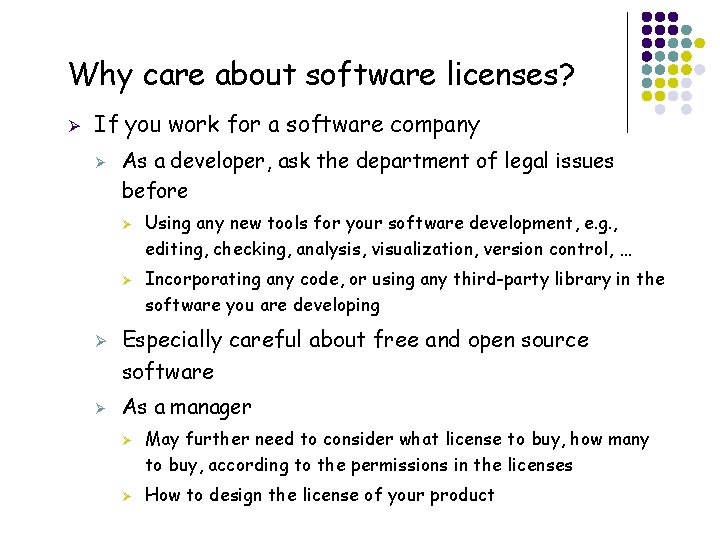
Why care about software licenses? Ø If you work for a software company Ø As a developer, ask the department of legal issues before Ø Ø Incorporating any code, or using any third-party library in the software you are developing Especially careful about free and open source software As a manager Ø Ø 37 Using any new tools for your software development, e. g. , editing, checking, analysis, visualization, version control, … May further need to consider what license to buy, how many to buy, according to the permissions in the licenses How to design the license of your product
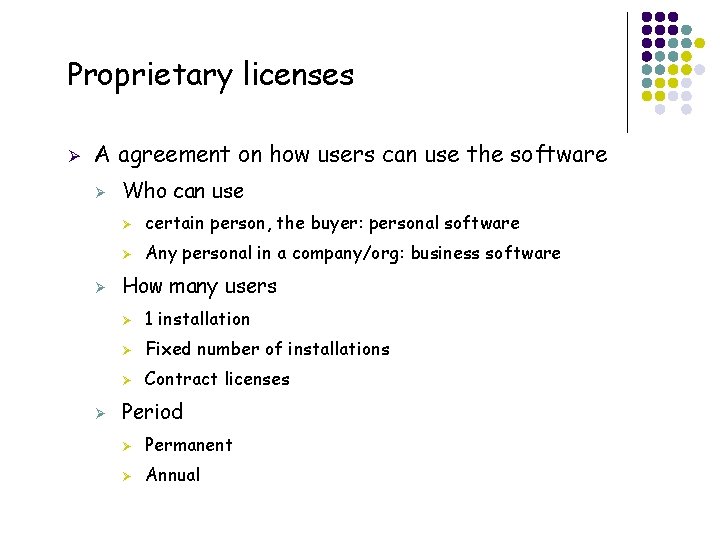
Proprietary licenses Ø A agreement on how users can use the software Ø Ø Ø 38 Who can use Ø certain person, the buyer: personal software Ø Any personal in a company/org: business software How many users Ø 1 installation Ø Fixed number of installations Ø Contract licenses Period Ø Permanent Ø Annual
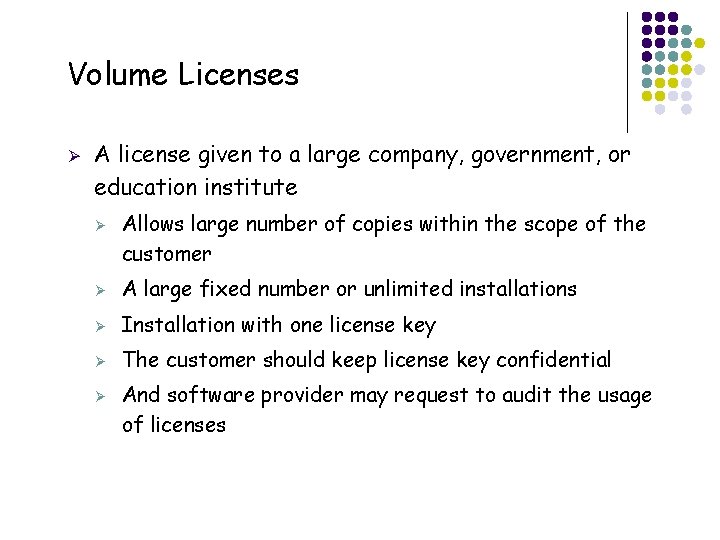
Volume Licenses Ø A license given to a large company, government, or education institute Ø Ø A large fixed number or unlimited installations Ø Installation with one license key Ø The customer should keep license key confidential Ø 39 Allows large number of copies within the scope of the customer And software provider may request to audit the usage of licenses
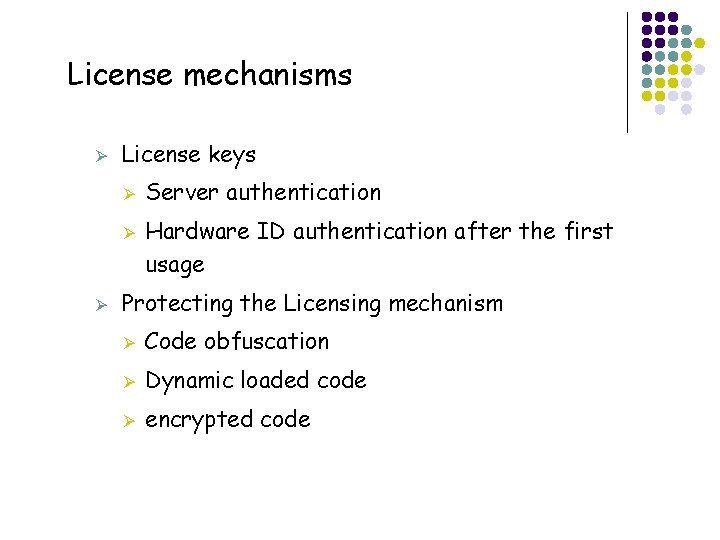
License mechanisms Ø License keys Ø Ø Ø 40 Server authentication Hardware ID authentication after the first usage Protecting the Licensing mechanism Ø Code obfuscation Ø Dynamic loaded code Ø encrypted code
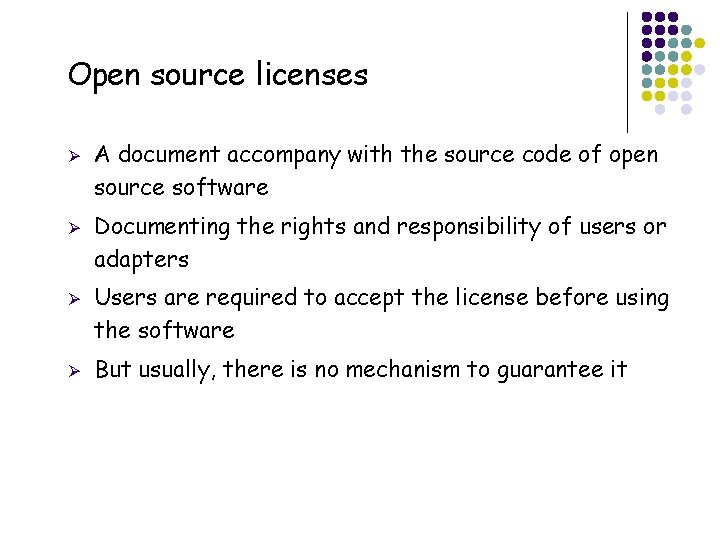
Open source licenses Ø Ø 41 A document accompany with the source code of open source software Documenting the rights and responsibility of users or adapters Users are required to accept the license before using the software But usually, there is no mechanism to guarantee it
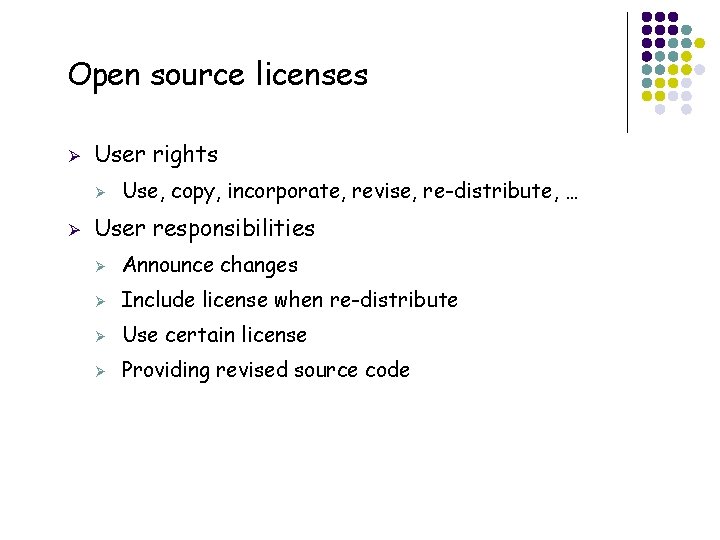
Open source licenses Ø User rights Ø Ø 42 Use, copy, incorporate, revise, re-distribute, … User responsibilities Ø Announce changes Ø Include license when re-distribute Ø Use certain license Ø Providing revised source code
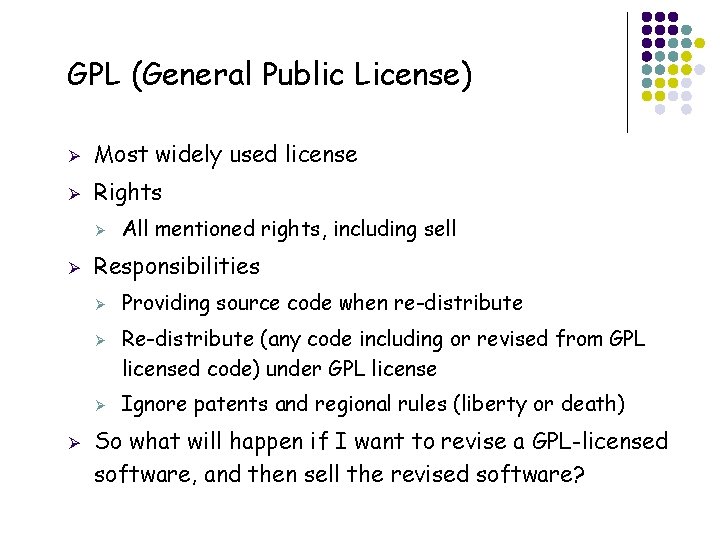
GPL (General Public License) Ø Most widely used license Ø Rights Ø Ø Responsibilities Ø Ø 43 All mentioned rights, including sell Providing source code when re-distribute Re-distribute (any code including or revised from GPL licensed code) under GPL license Ignore patents and regional rules (liberty or death) So what will happen if I want to revise a GPL-licensed software, and then sell the revised software?
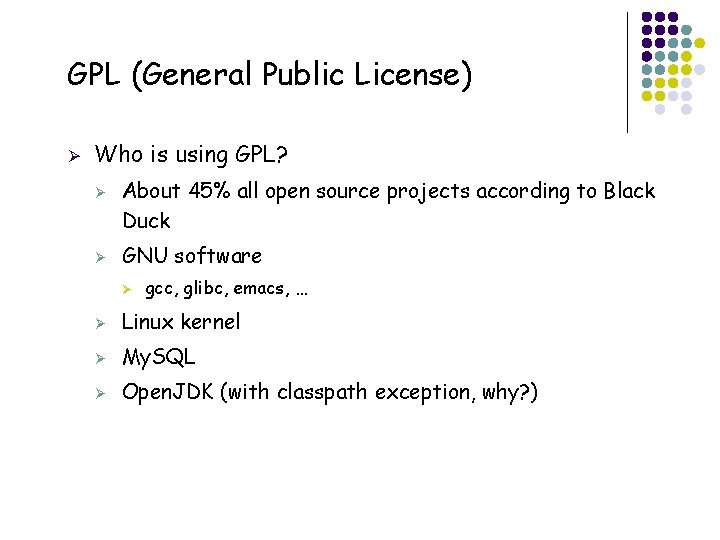
GPL (General Public License) Ø Who is using GPL? Ø Ø About 45% all open source projects according to Black Duck GNU software Ø 44 gcc, glibc, emacs, … Ø Linux kernel Ø My. SQL Ø Open. JDK (with classpath exception, why? )
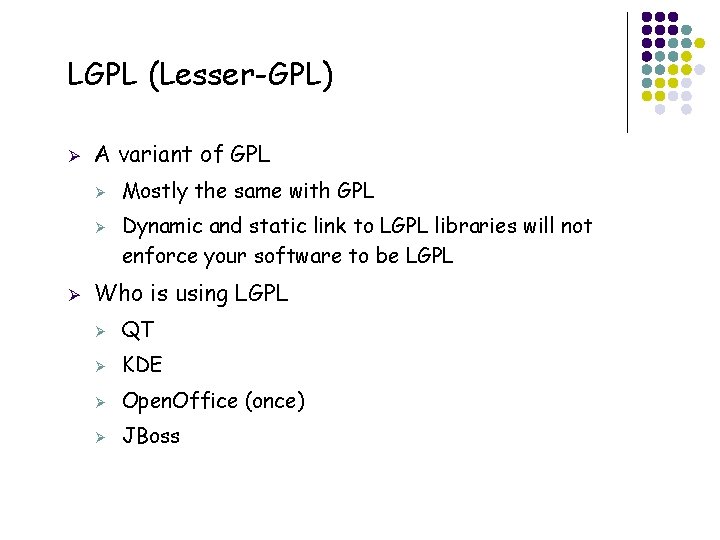
LGPL (Lesser-GPL) Ø A variant of GPL Ø Ø Ø 45 Mostly the same with GPL Dynamic and static link to LGPL libraries will not enforce your software to be LGPL Who is using LGPL Ø QT Ø KDE Ø Open. Office (once) Ø JBoss
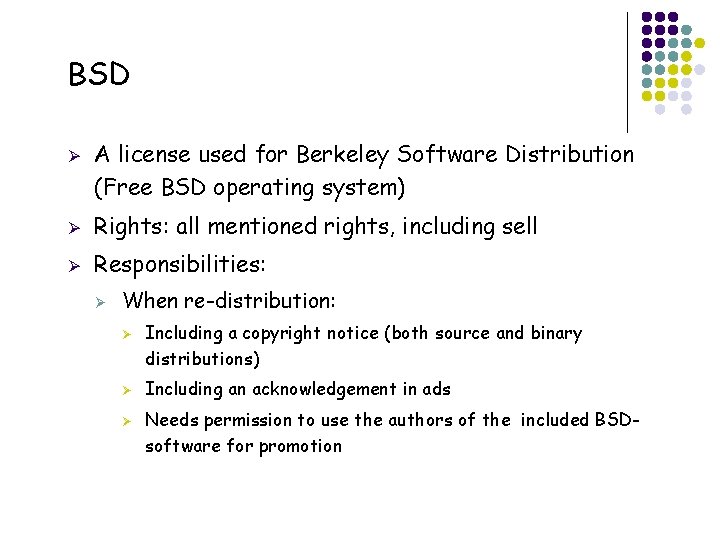
BSD Ø A license used for Berkeley Software Distribution (Free BSD operating system) Ø Rights: all mentioned rights, including sell Ø Responsibilities: Ø When re-distribution: Ø Ø Ø 46 Including a copyright notice (both source and binary distributions) Including an acknowledgement in ads Needs permission to use the authors of the included BSDsoftware for promotion
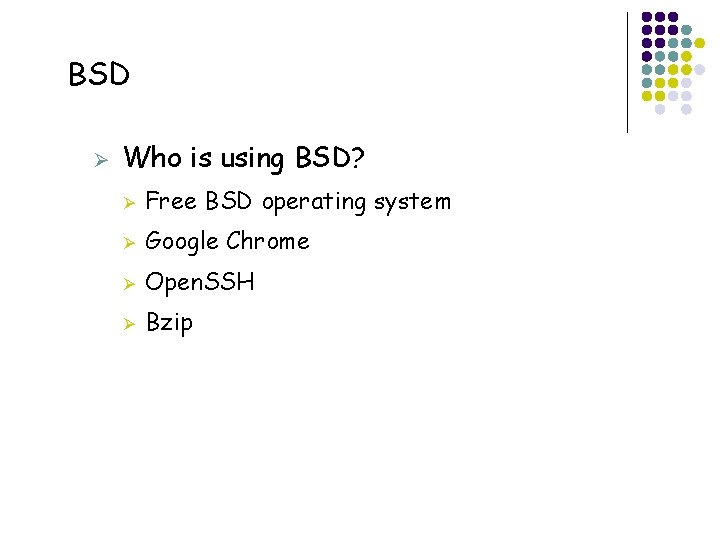
BSD Ø 47 Who is using BSD? Ø Free BSD operating system Ø Google Chrome Ø Open. SSH Ø Bzip
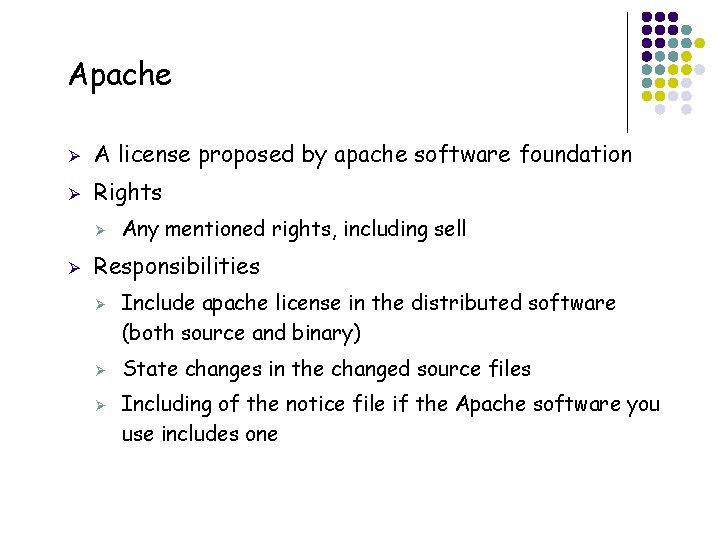
Apache Ø A license proposed by apache software foundation Ø Rights Ø Ø Responsibilities Ø Ø Ø 48 Any mentioned rights, including sell Include apache license in the distributed software (both source and binary) State changes in the changed source files Including of the notice file if the Apache software you use includes one
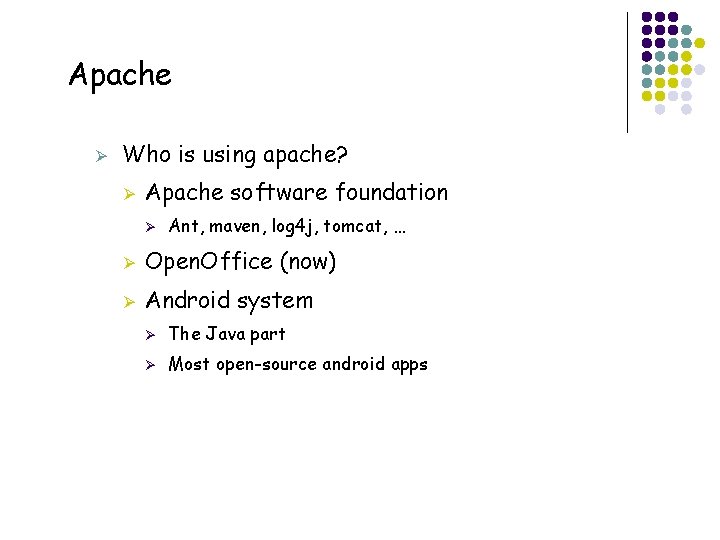
Apache Ø Who is using apache? Ø Apache software foundation Ø 49 Ant, maven, log 4 j, tomcat, … Ø Open. Office (now) Ø Android system Ø The Java part Ø Most open-source android apps
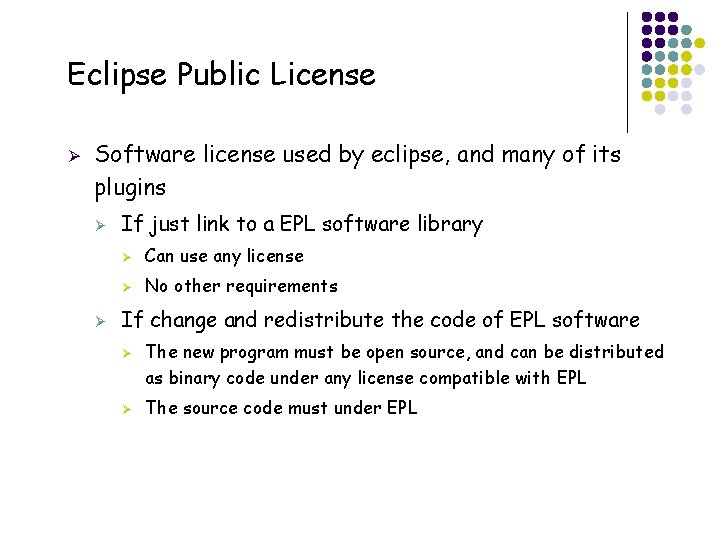
Eclipse Public License Ø Software license used by eclipse, and many of its plugins Ø Ø If just link to a EPL software library Ø Can use any license Ø No other requirements If change and redistribute the code of EPL software Ø Ø 50 The new program must be open source, and can be distributed as binary code under any license compatible with EPL The source code must under EPL
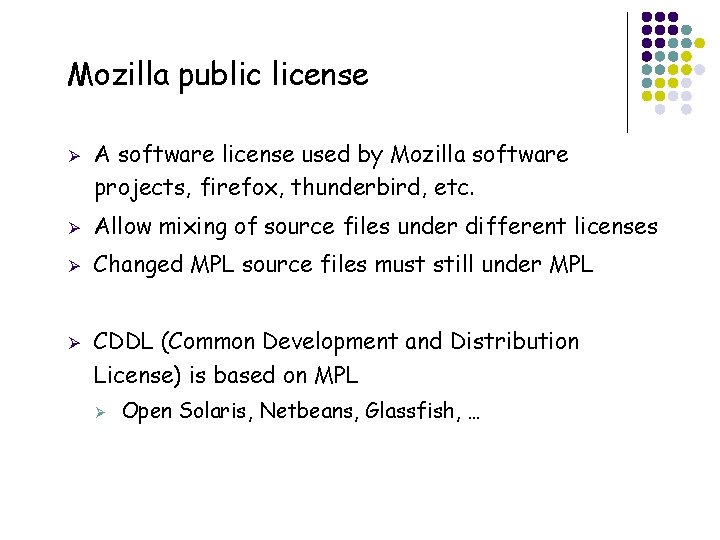
Mozilla public license Ø A software license used by Mozilla software projects, firefox, thunderbird, etc. Ø Allow mixing of source files under different licenses Ø Changed MPL source files must still under MPL Ø CDDL (Common Development and Distribution License) is based on MPL Ø 51 Open Solaris, Netbeans, Glassfish, …
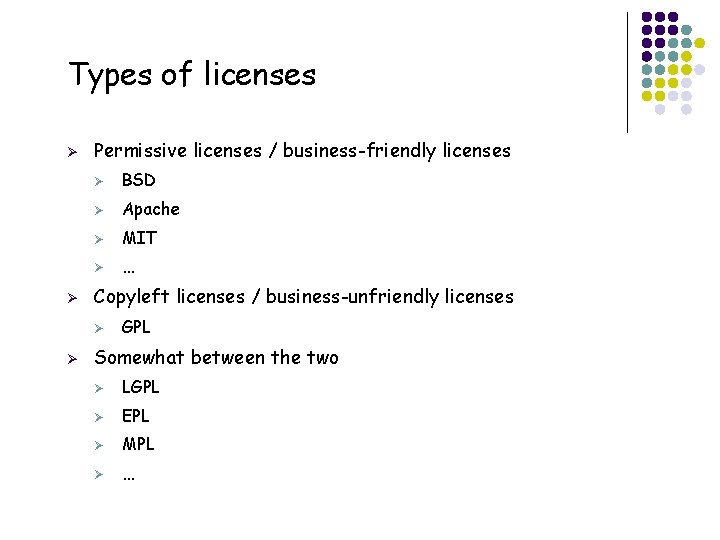
Types of licenses Ø Ø Permissive licenses / business-friendly licenses Ø BSD Ø Apache Ø MIT Ø … Copyleft licenses / business-unfriendly licenses Ø Ø 52 GPL Somewhat between the two Ø LGPL Ø EPL Ø MPL Ø …
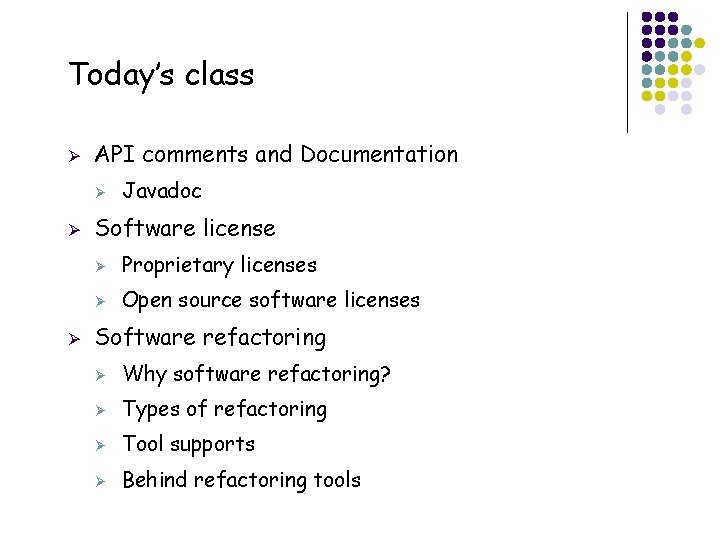
Today’s class Ø API comments and Documentation Ø Ø Ø 53 Javadoc Software license Ø Proprietary licenses Ø Open source software licenses Software refactoring Ø Why software refactoring? Ø Types of refactoring Ø Tool supports Ø Behind refactoring tools
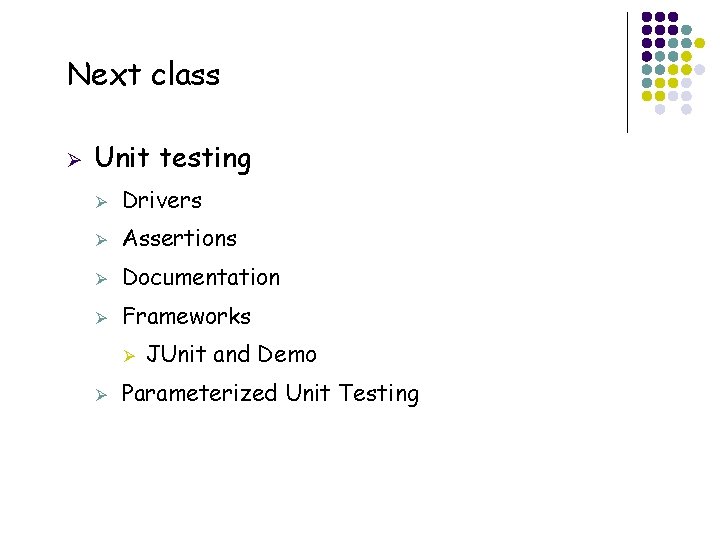
Next class Ø Unit testing Ø Drivers Ø Assertions Ø Documentation Ø Frameworks Ø Ø 54 JUnit and Demo Parameterized Unit Testing
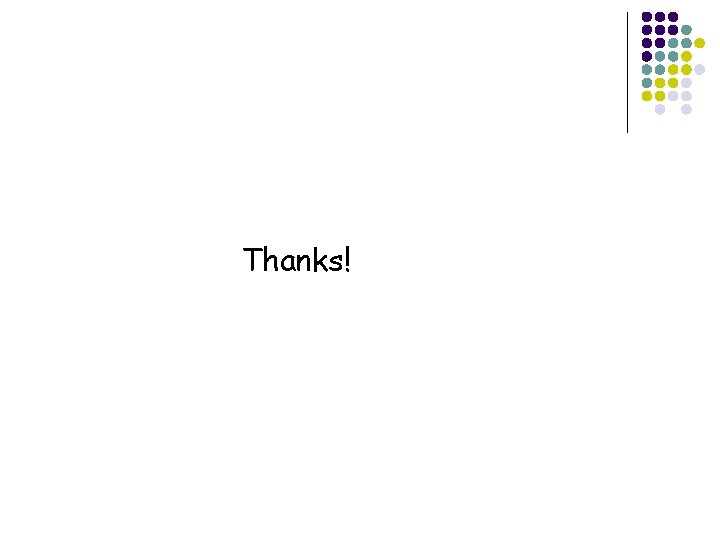
Thanks! 55
Software refactoring beratung
A survey of software refactoring
Requirement analysis in software engineering notes
Refactoring to patterns joshua kerievsky
Refactoring vs shimming
Refactoring
Thoughtworks code witch
Code refactoring
Exterme programming
Refactoring and restructuring methods
01:640:244 lecture notes - lecture 15: plat, idah, farad
Computer based system engineering in software engineering
Forward engineering and reverse engineering
Financial engineering notes
Foundation engineering lecture notes
Descriptive ethics
Software maintenance process models ppt
Who invented software engineering
What is software metrics in software engineering
Software engineering crisis
Software metrics and software metrology
Real time software design in software engineering
Software design fundamentals in software engineering
Software project management handwritten notes pdf
Lecture presentation software
Dicapine
Engineering elegant systems: theory of systems engineering
Reverse engineering vs forward engineering
Computer organization lab experiments
User interface design steps in software engineering
Srs in software engineering
Activity diagram example in software engineering
Software engineering task
4 p's of management spectrum
Ck metrics
Sds table of contents
Supportability of software
Inverse requirements example
Inverse requirements example
Structured specification
Statistical sqa
Rapid prototyping software engineering
Explain rmmm plan with example.
Communication planning modeling construction deployment
Program evolution dynamics
Interface design in software engineering
Use case diagram for covid-19
Rsl/revs in software engineering
Pspec in software engineering
Unified engineering software
User testing software engineering
Types of testing in software engineering
Sw in software engineering
Integration testing software engineering
Design process in software engineering
Project planning begins with the melding of