Code style Jordi Cortadella Department of Computer Science
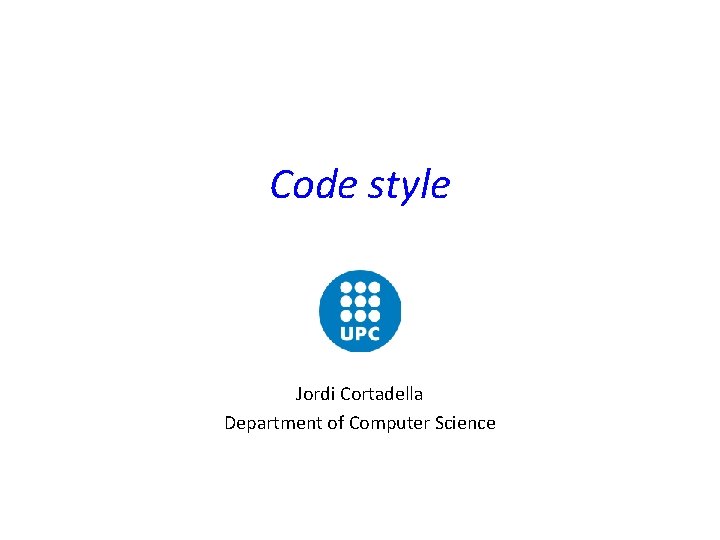
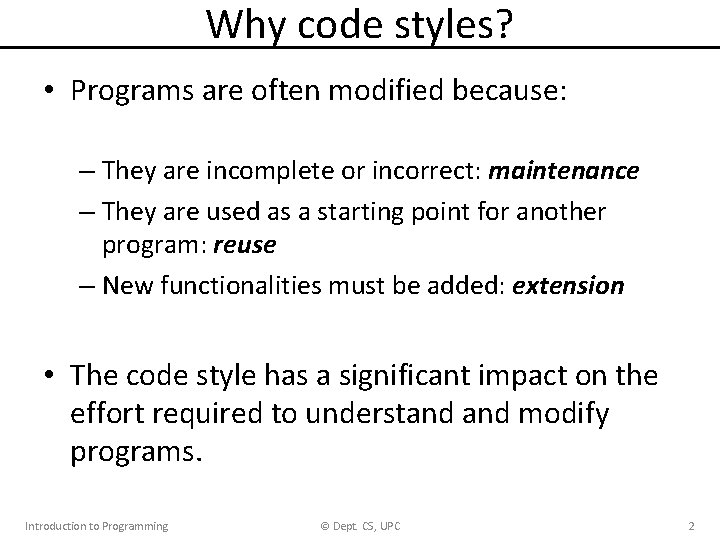
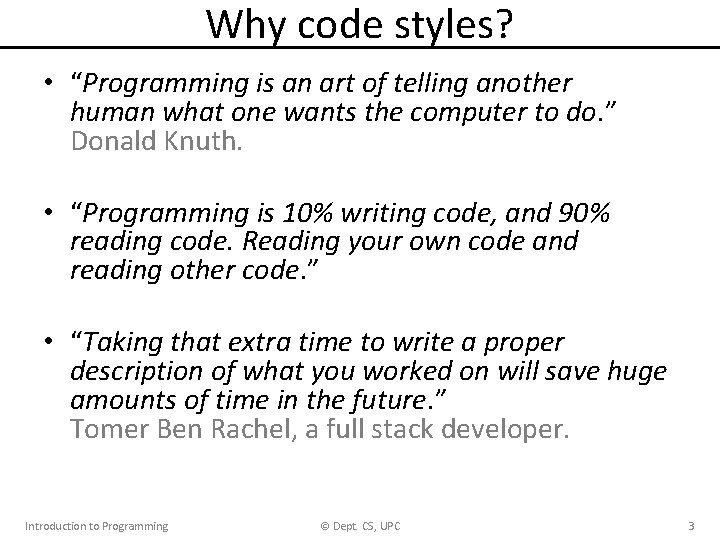
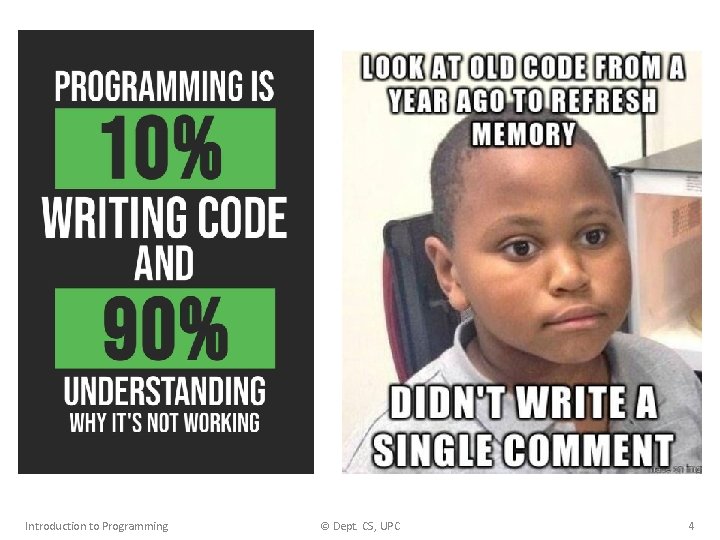
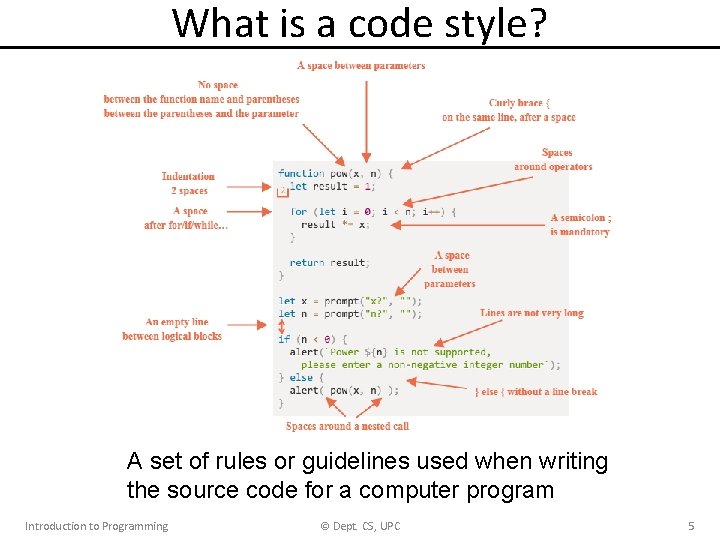
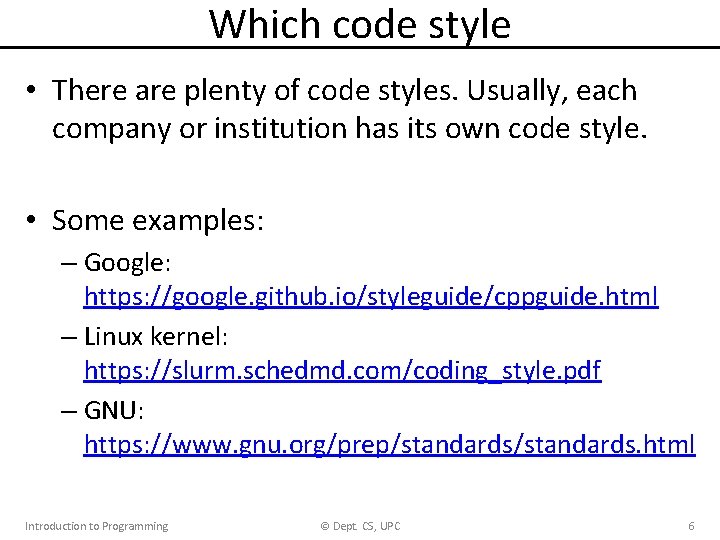
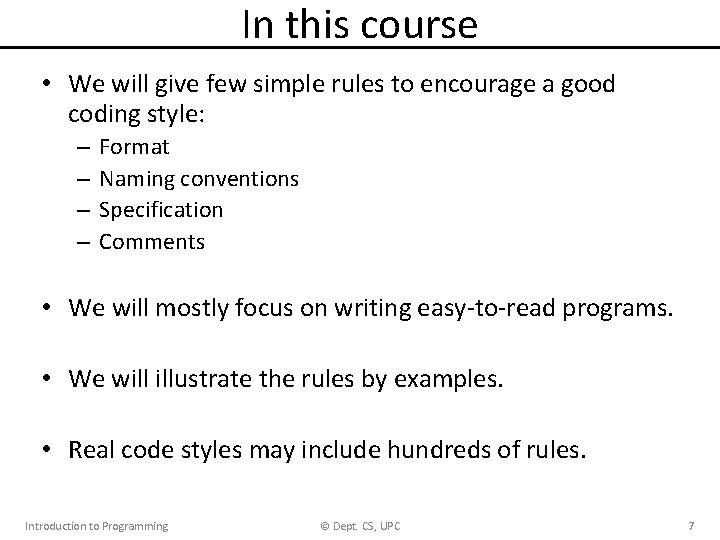
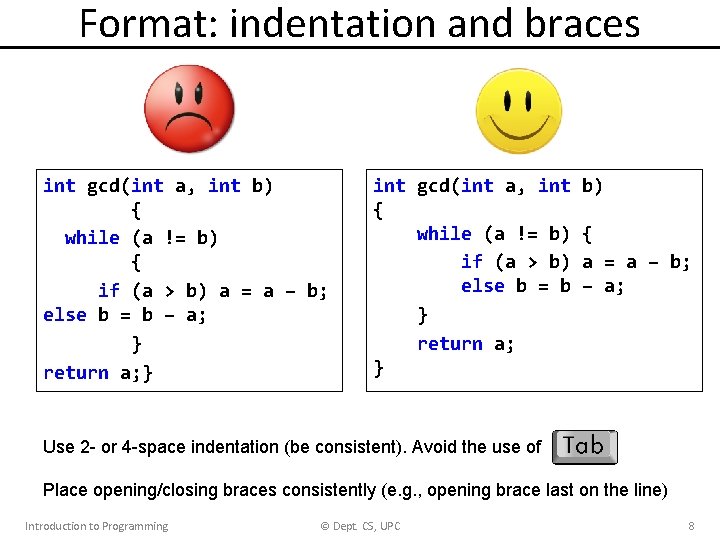
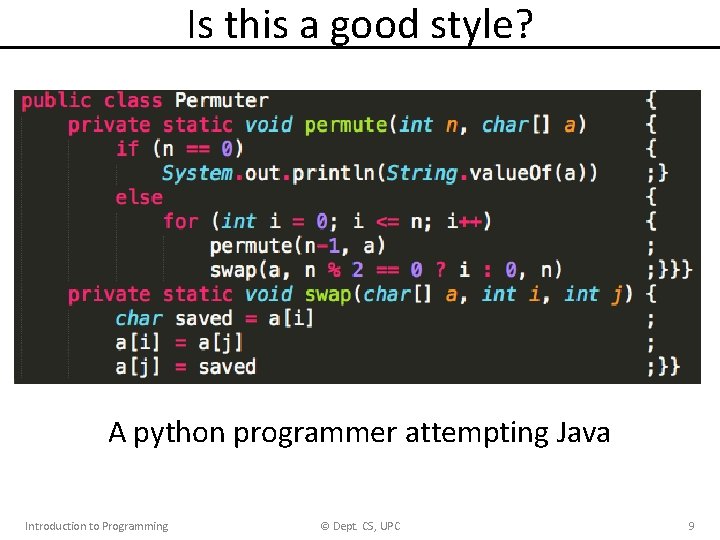
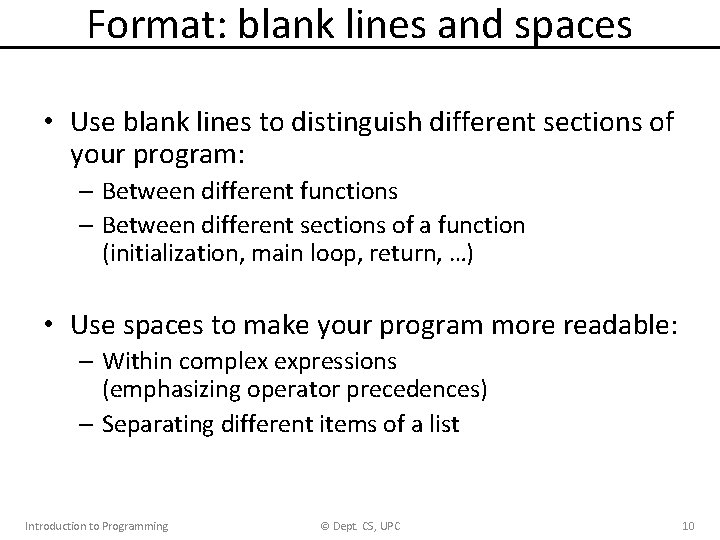
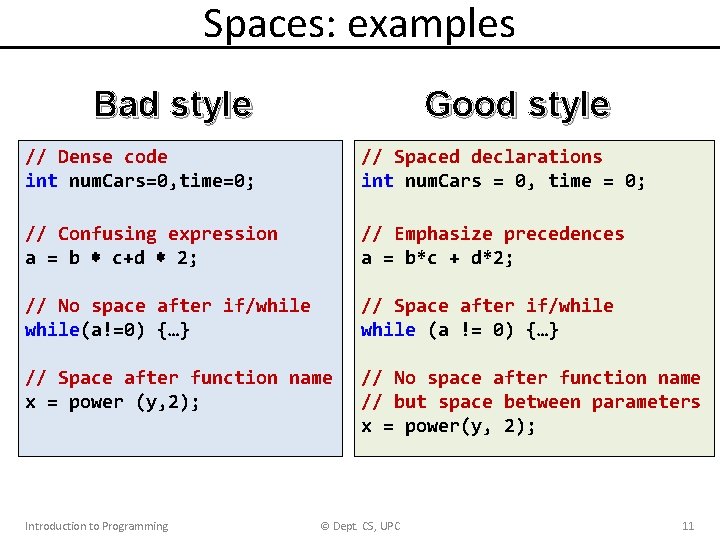
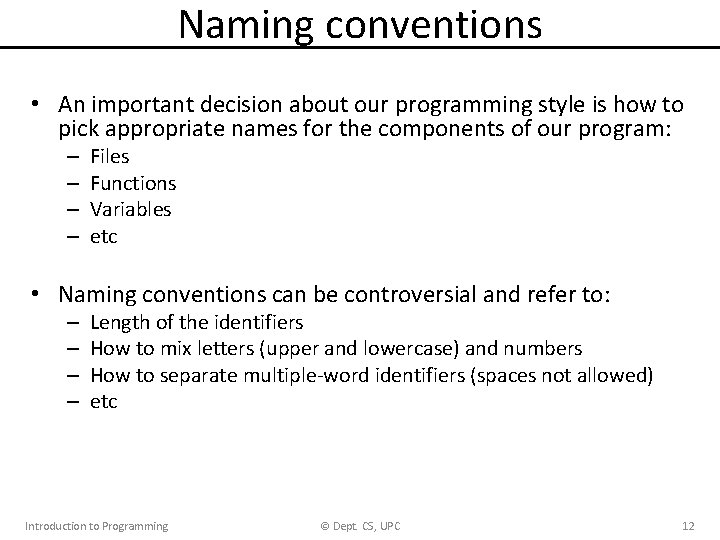
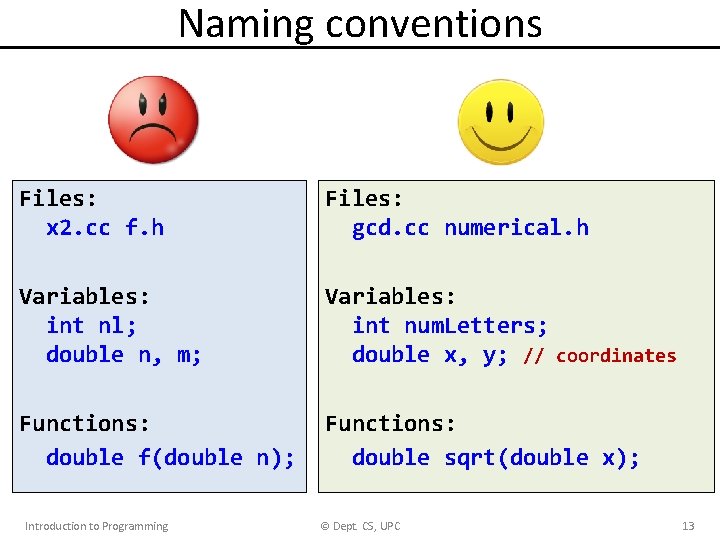
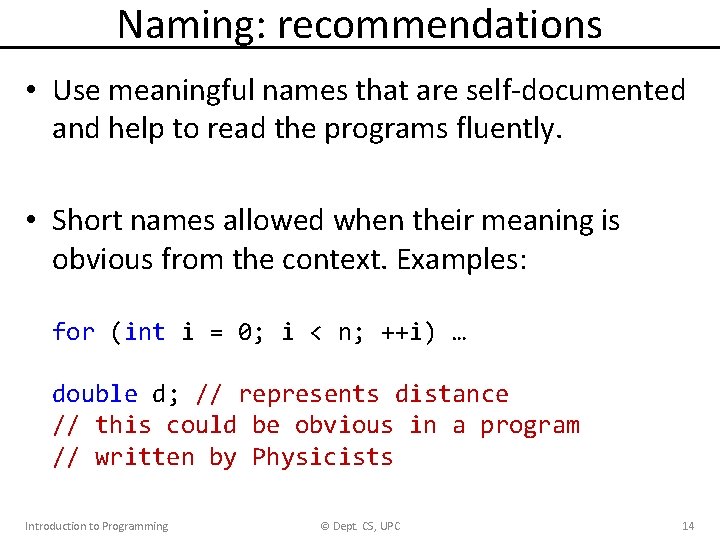
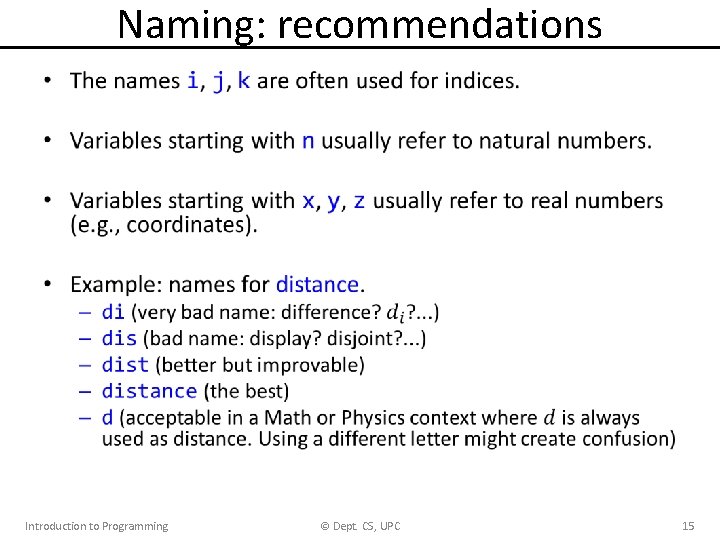
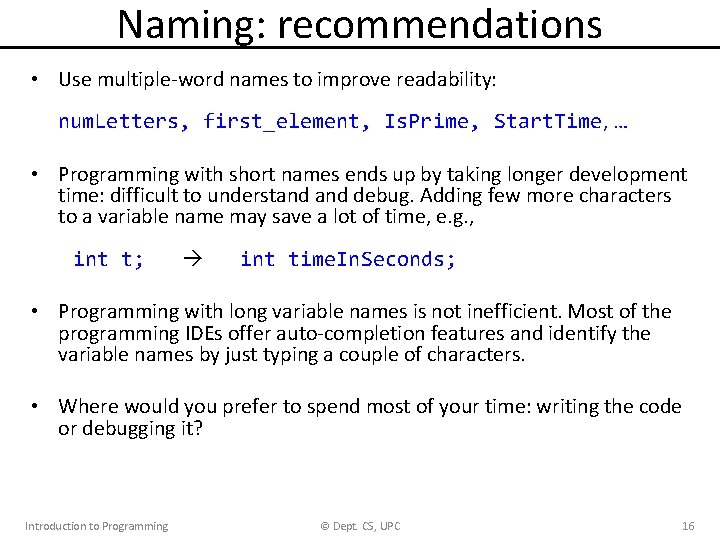
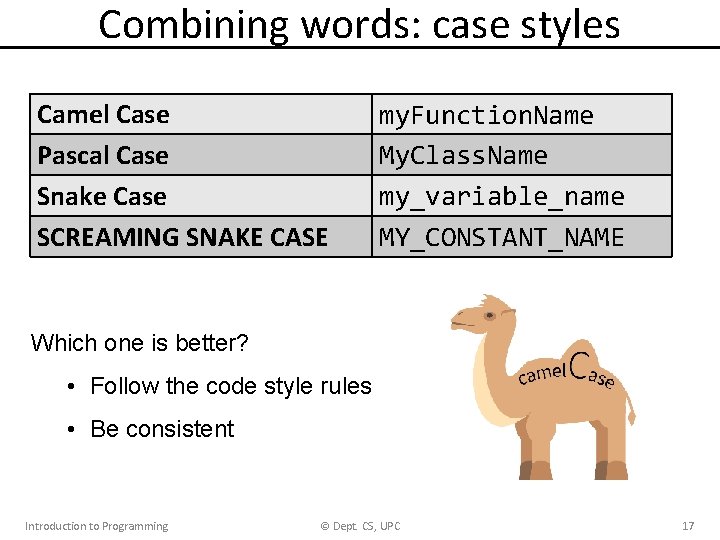
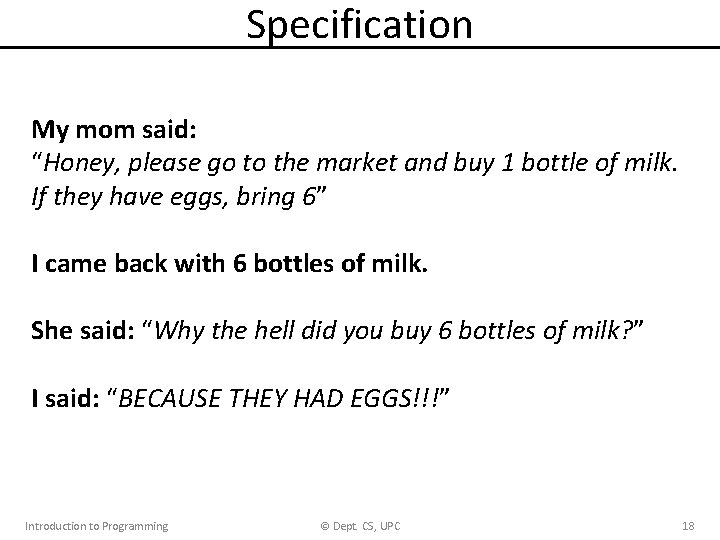
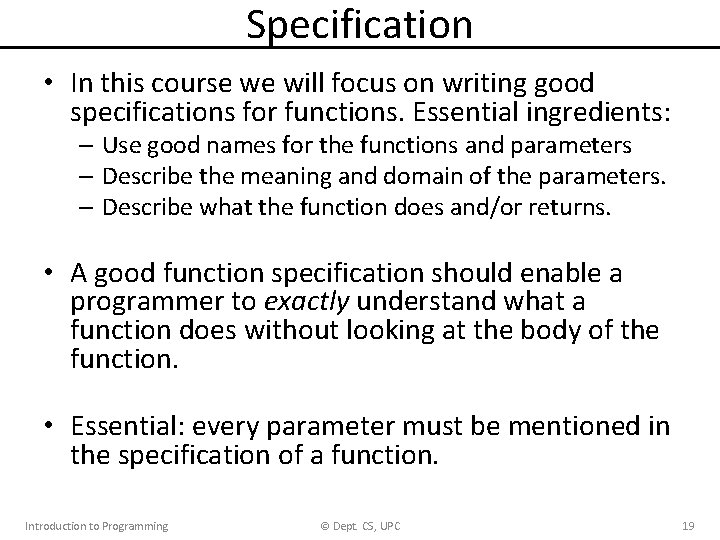
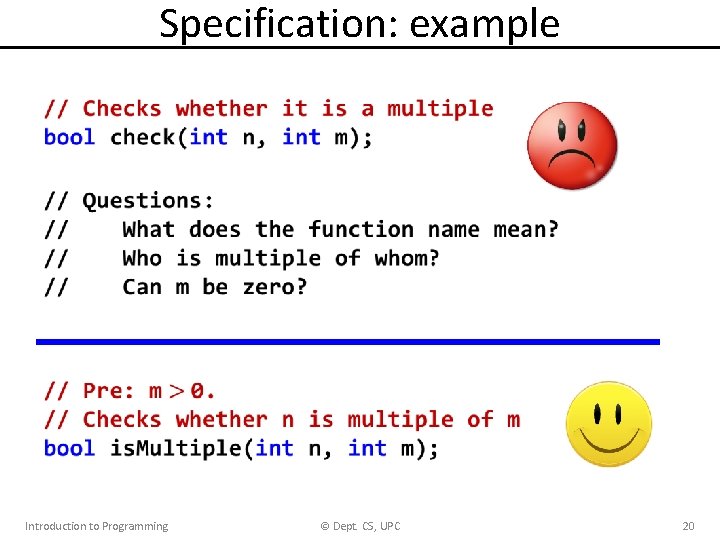
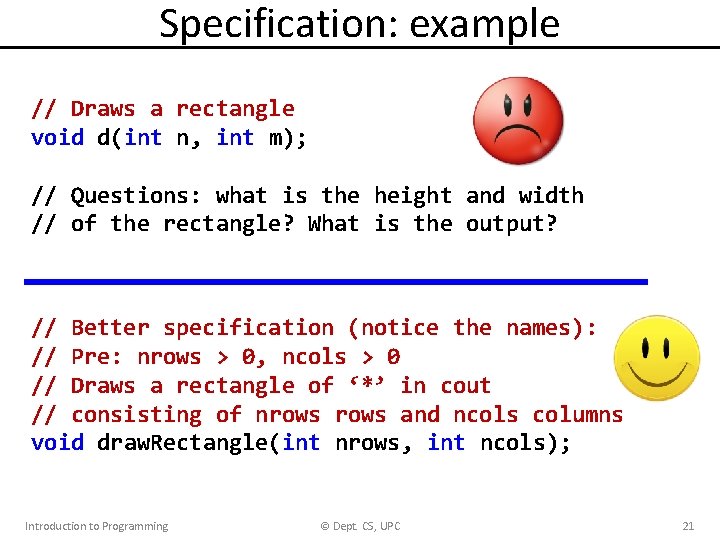
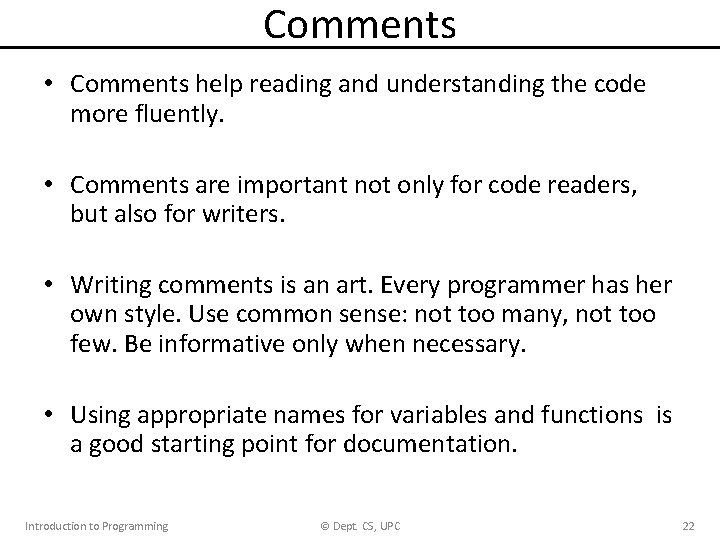
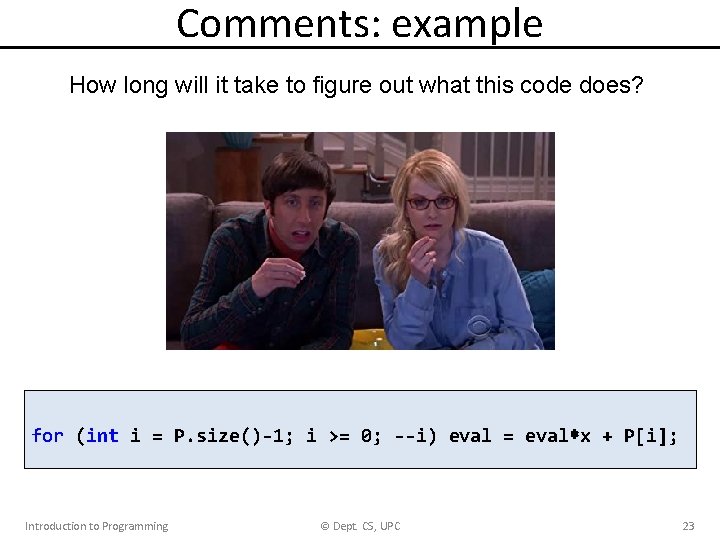
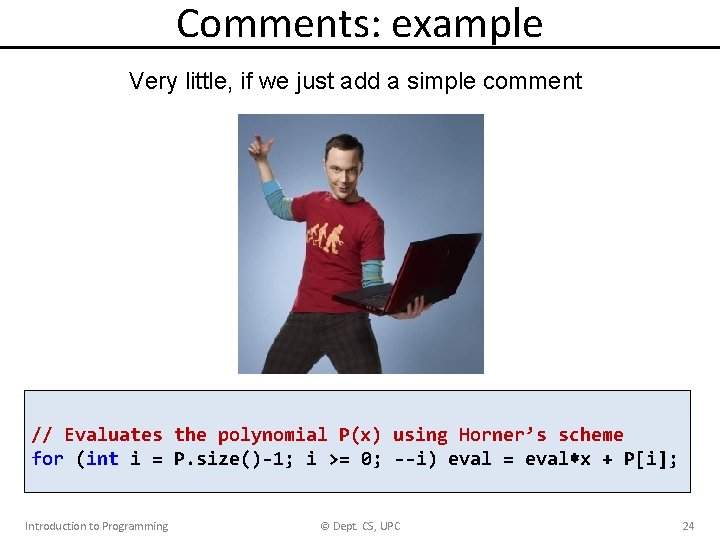
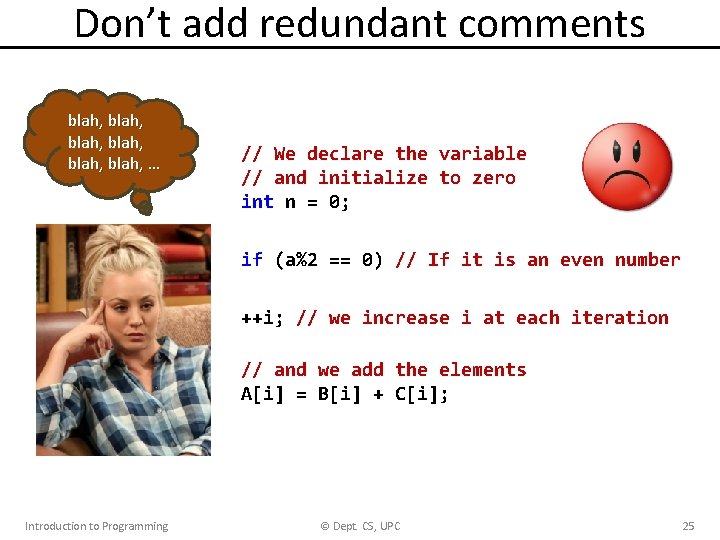
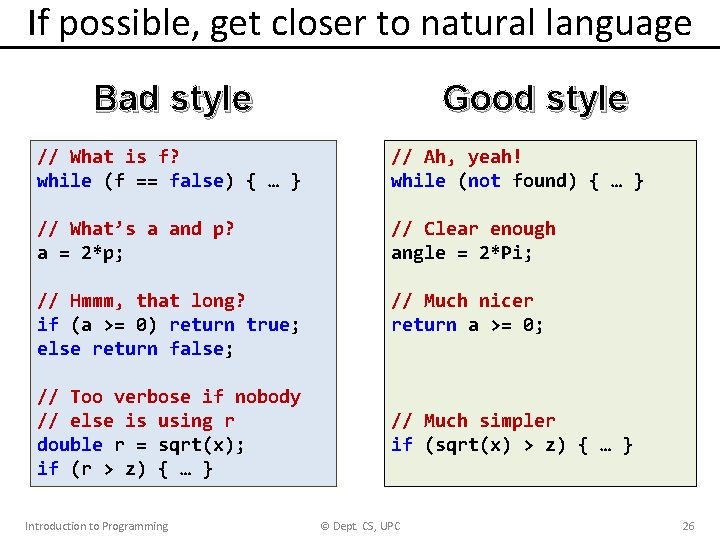
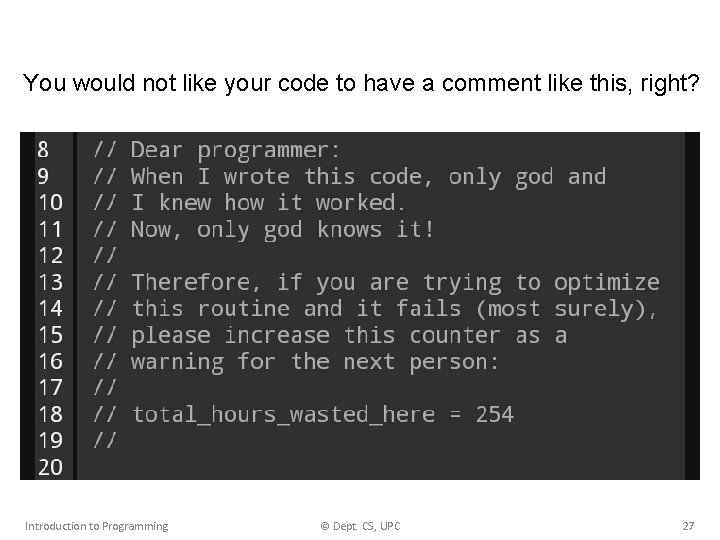
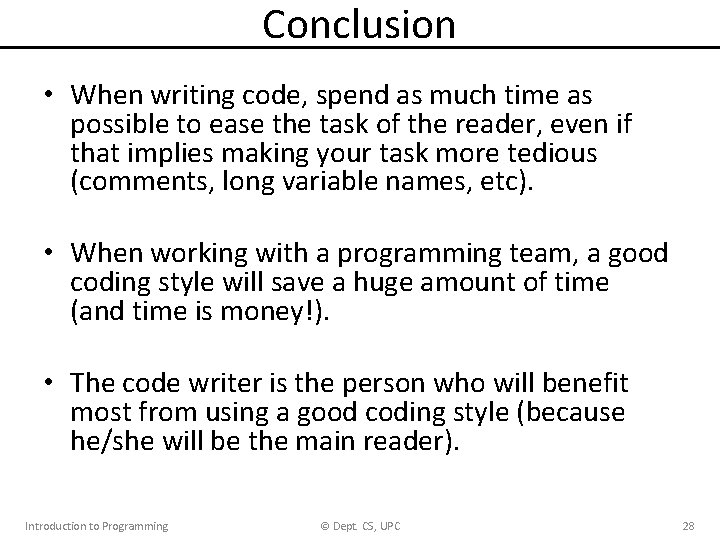
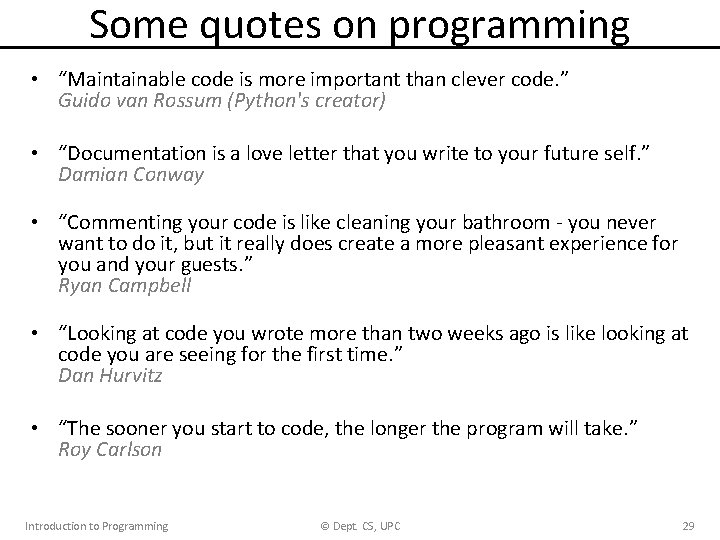
- Slides: 29
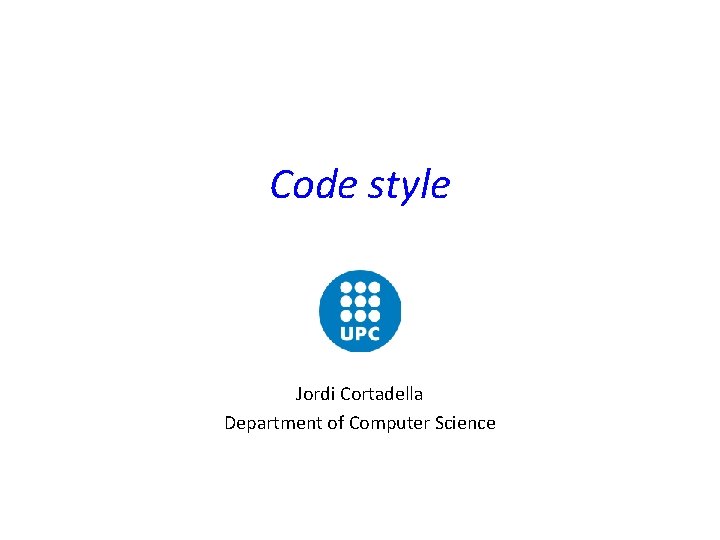
Code style Jordi Cortadella Department of Computer Science
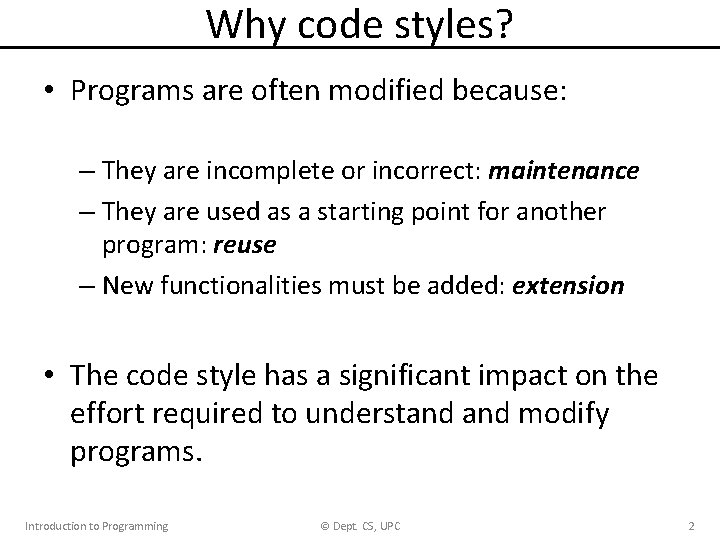
Why code styles? • Programs are often modified because: – They are incomplete or incorrect: maintenance – They are used as a starting point for another program: reuse – New functionalities must be added: extension • The code style has a significant impact on the effort required to understand modify programs. Introduction to Programming © Dept. CS, UPC 2
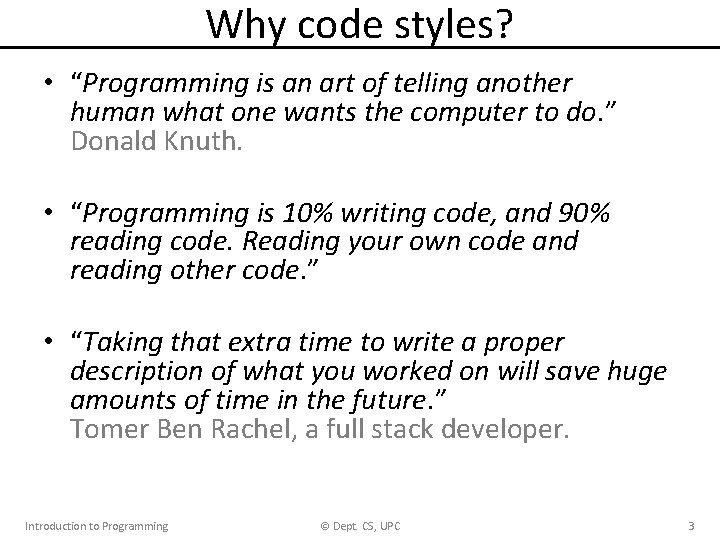
Why code styles? • “Programming is an art of telling another human what one wants the computer to do. ” Donald Knuth. • “Programming is 10% writing code, and 90% reading code. Reading your own code and reading other code. ” • “Taking that extra time to write a proper description of what you worked on will save huge amounts of time in the future. ” Tomer Ben Rachel, a full stack developer. Introduction to Programming © Dept. CS, UPC 3
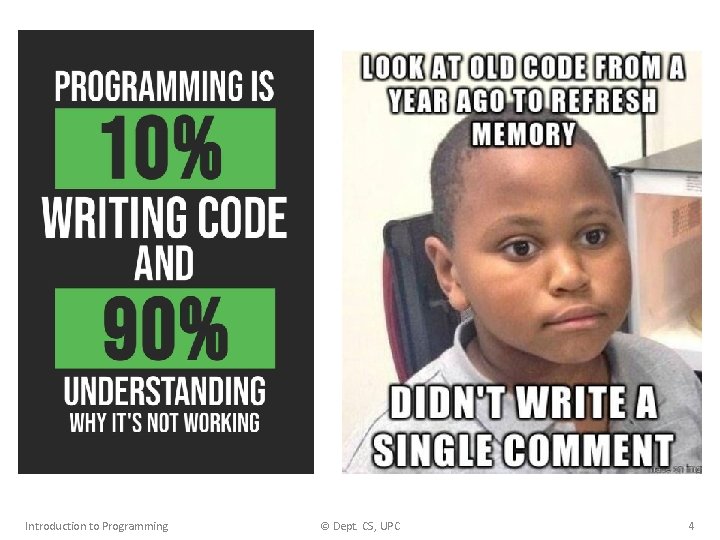
Introduction to Programming © Dept. CS, UPC 4
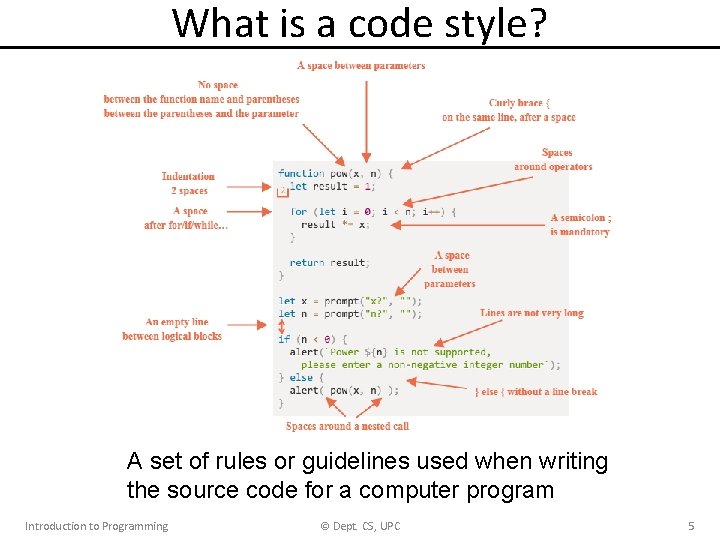
What is a code style? A set of rules or guidelines used when writing the source code for a computer program Introduction to Programming © Dept. CS, UPC 5
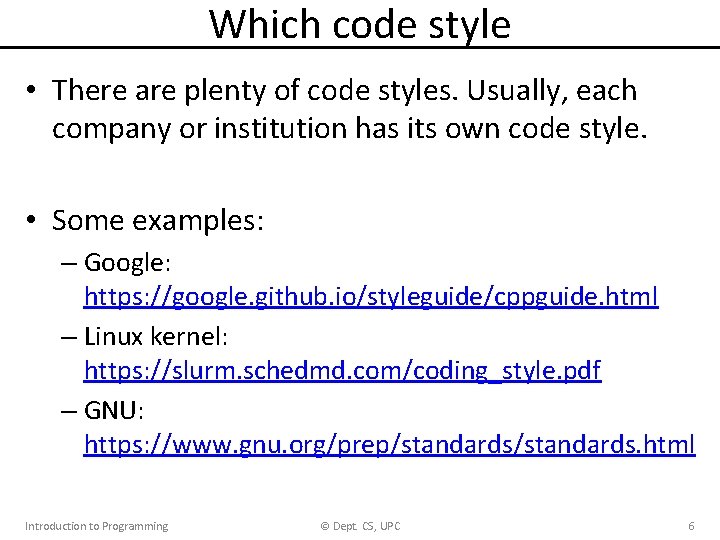
Which code style • There are plenty of code styles. Usually, each company or institution has its own code style. • Some examples: – Google: https: //google. github. io/styleguide/cppguide. html – Linux kernel: https: //slurm. schedmd. com/coding_style. pdf – GNU: https: //www. gnu. org/prep/standards. html Introduction to Programming © Dept. CS, UPC 6
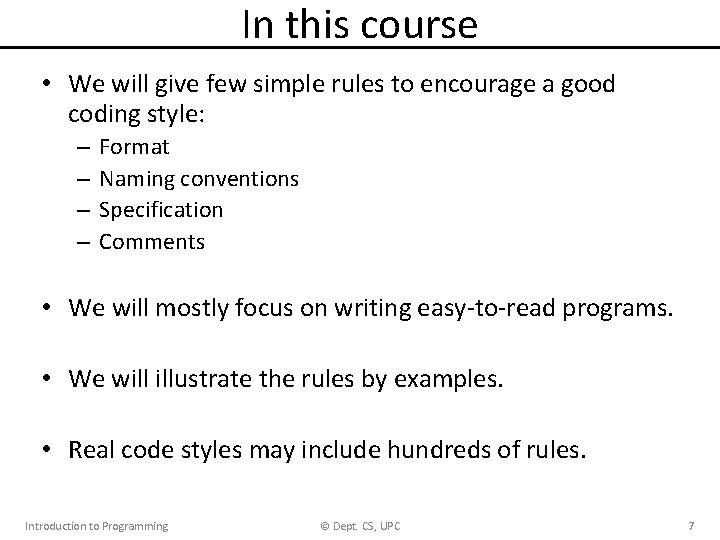
In this course • We will give few simple rules to encourage a good coding style: – – Format Naming conventions Specification Comments • We will mostly focus on writing easy-to-read programs. • We will illustrate the rules by examples. • Real code styles may include hundreds of rules. Introduction to Programming © Dept. CS, UPC 7
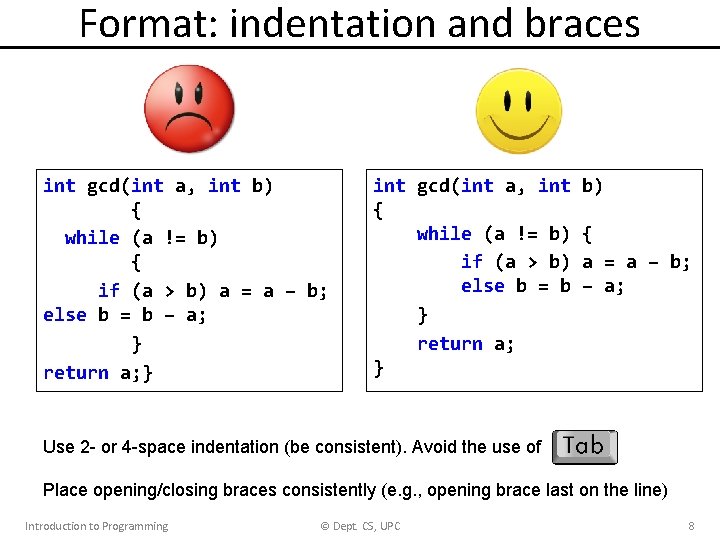
Format: indentation and braces int gcd(int a, int b) { while (a != b) { if (a > b) a = a – b; else b = b – a; } return a; } int gcd(int a, int { while (a != b) if (a > b) else b = b } return a; } b) { a = a – b; – a; Use 2 - or 4 -space indentation (be consistent). Avoid the use of Place opening/closing braces consistently (e. g. , opening brace last on the line) Introduction to Programming © Dept. CS, UPC 8
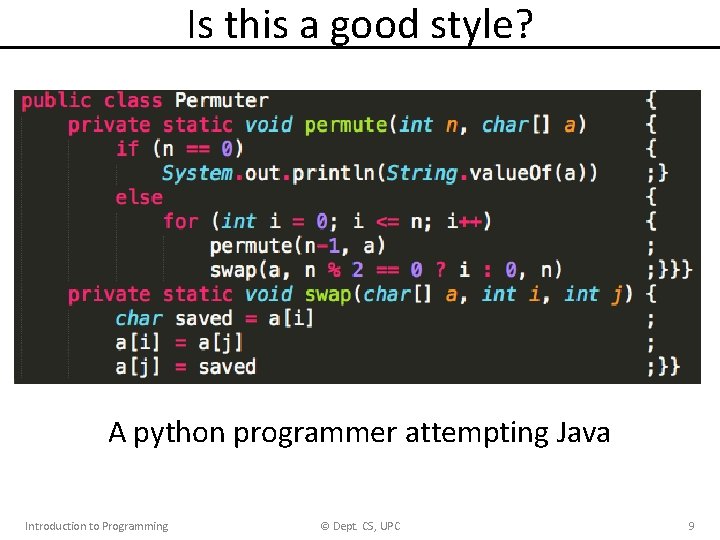
Is this a good style? A python programmer attempting Java Introduction to Programming © Dept. CS, UPC 9
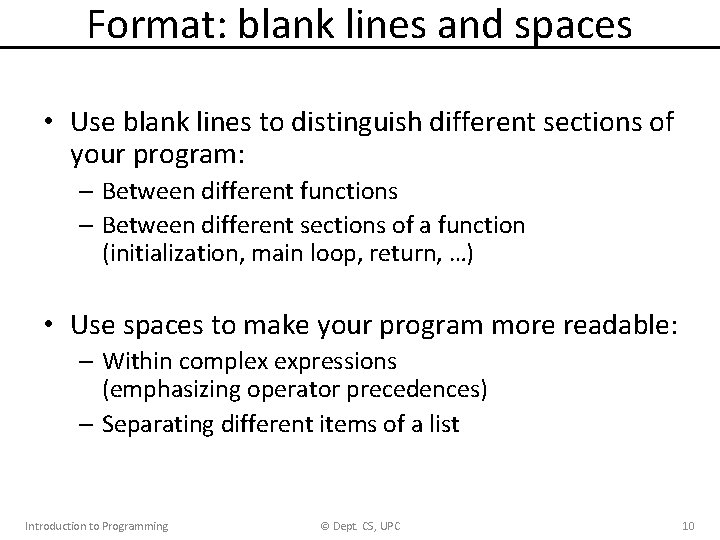
Format: blank lines and spaces • Use blank lines to distinguish different sections of your program: – Between different functions – Between different sections of a function (initialization, main loop, return, …) • Use spaces to make your program more readable: – Within complex expressions (emphasizing operator precedences) – Separating different items of a list Introduction to Programming © Dept. CS, UPC 10
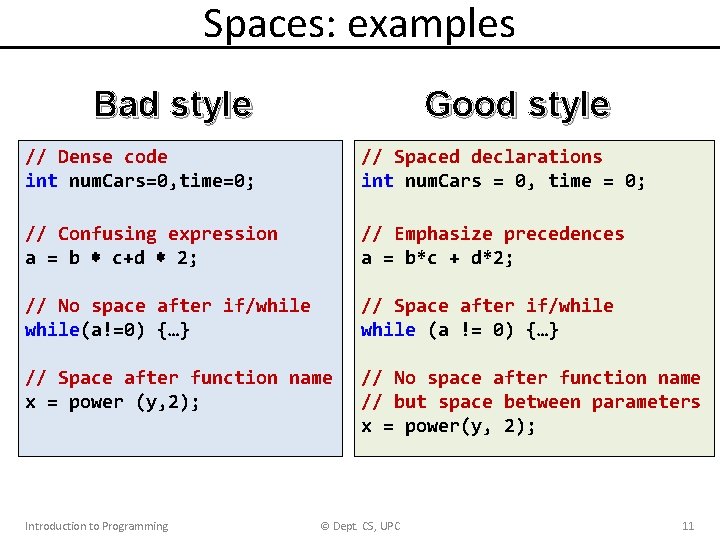
Spaces: examples Bad style Good style // Dense code int num. Cars=0, time=0; // Spaced declarations int num. Cars = 0, time = 0; // Confusing expression a = b c+d 2; // Emphasize precedences a = b*c + d*2; // No space after if/while(a!=0) {…} // Space after if/while (a != 0) {…} // Space after function name x = power (y, 2); // No space after function name // but space between parameters x = power(y, 2); Introduction to Programming © Dept. CS, UPC 11
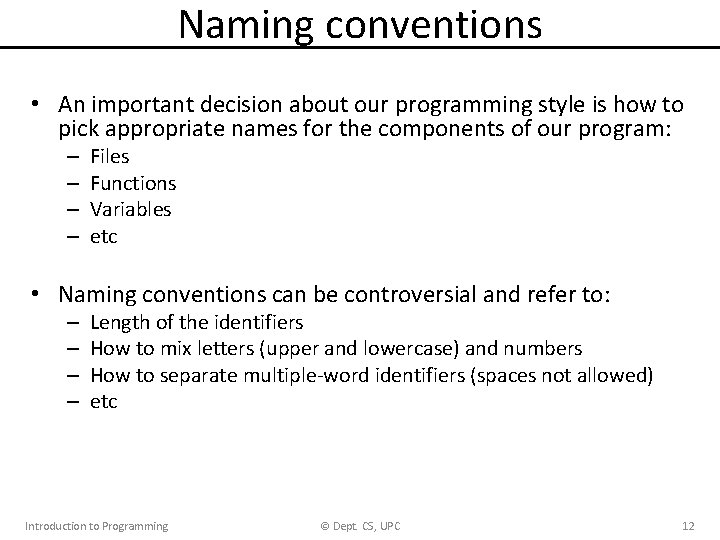
Naming conventions • An important decision about our programming style is how to pick appropriate names for the components of our program: – – Files Functions Variables etc • Naming conventions can be controversial and refer to: – – Length of the identifiers How to mix letters (upper and lowercase) and numbers How to separate multiple-word identifiers (spaces not allowed) etc Introduction to Programming © Dept. CS, UPC 12
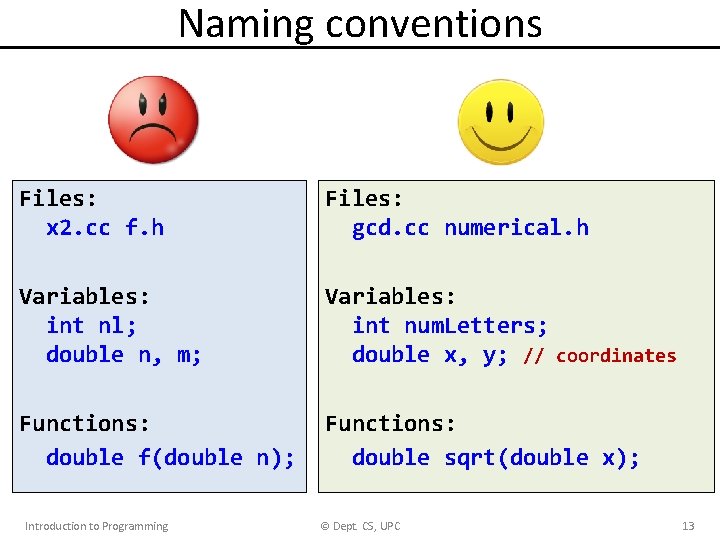
Naming conventions Files: x 2. cc f. h Files: gcd. cc numerical. h Variables: int nl; double n, m; Variables: int num. Letters; double x, y; // coordinates Functions: double f(double n); Functions: double sqrt(double x); Introduction to Programming © Dept. CS, UPC 13
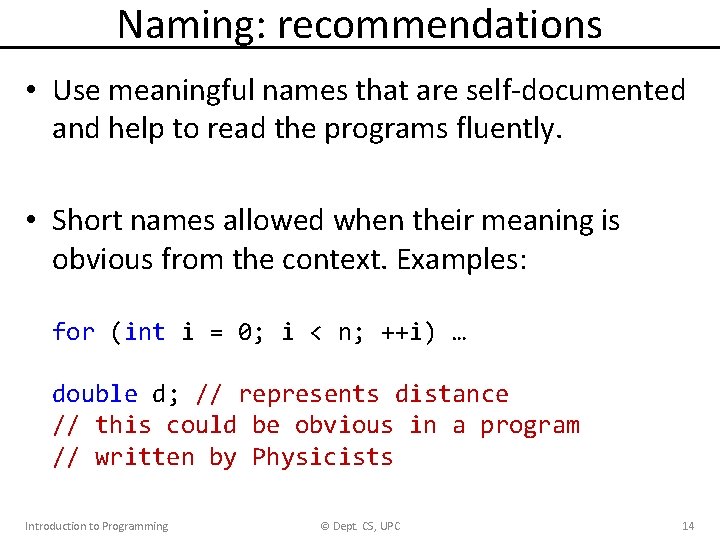
Naming: recommendations • Use meaningful names that are self-documented and help to read the programs fluently. • Short names allowed when their meaning is obvious from the context. Examples: for (int i = 0; i < n; ++i) … double d; // represents distance // this could be obvious in a program // written by Physicists Introduction to Programming © Dept. CS, UPC 14
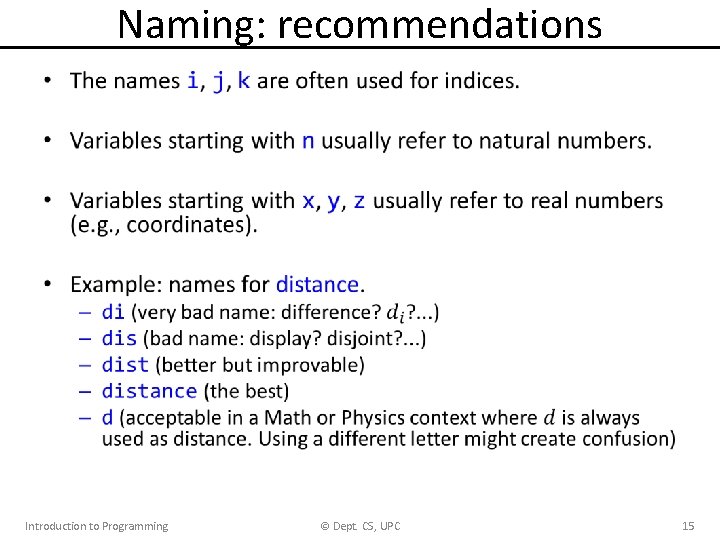
Naming: recommendations • Introduction to Programming © Dept. CS, UPC 15
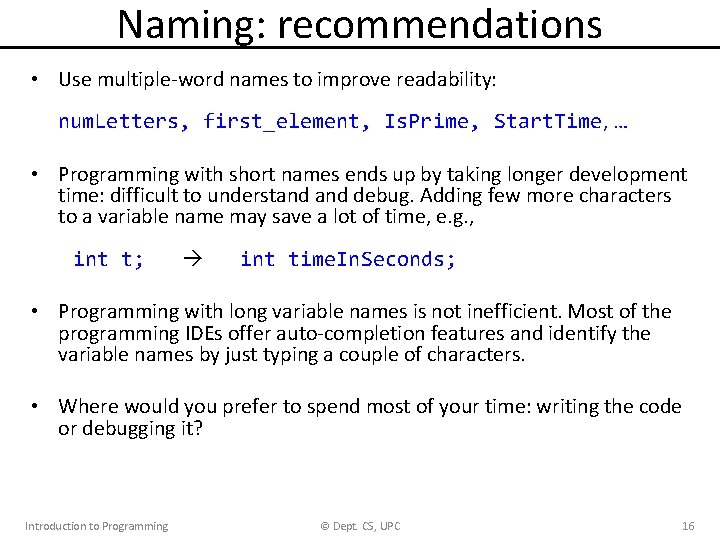
Naming: recommendations • Use multiple-word names to improve readability: num. Letters, first_element, Is. Prime, Start. Time, … • Programming with short names ends up by taking longer development time: difficult to understand debug. Adding few more characters to a variable name may save a lot of time, e. g. , int t; int time. In. Seconds; • Programming with long variable names is not inefficient. Most of the programming IDEs offer auto-completion features and identify the variable names by just typing a couple of characters. • Where would you prefer to spend most of your time: writing the code or debugging it? Introduction to Programming © Dept. CS, UPC 16
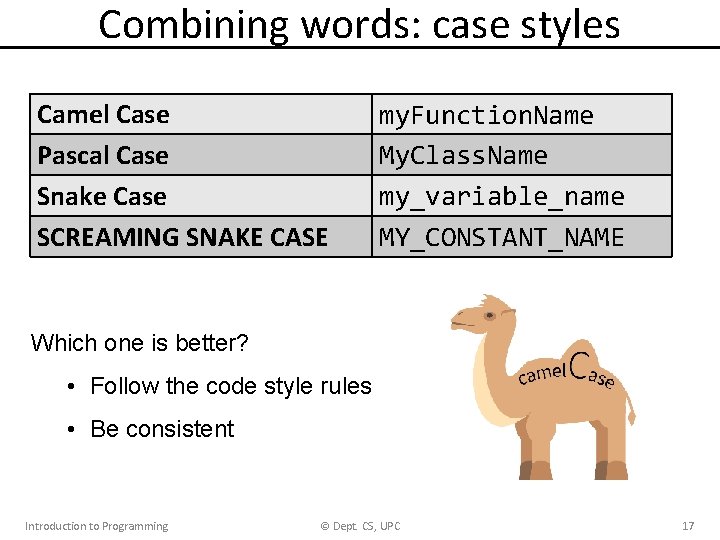
Combining words: case styles Camel Case Pascal Case Snake Case SCREAMING SNAKE CASE my. Function. Name My. Class. Name my_variable_name MY_CONSTANT_NAME Which one is better? • Follow the code style rules • Be consistent Introduction to Programming © Dept. CS, UPC 17
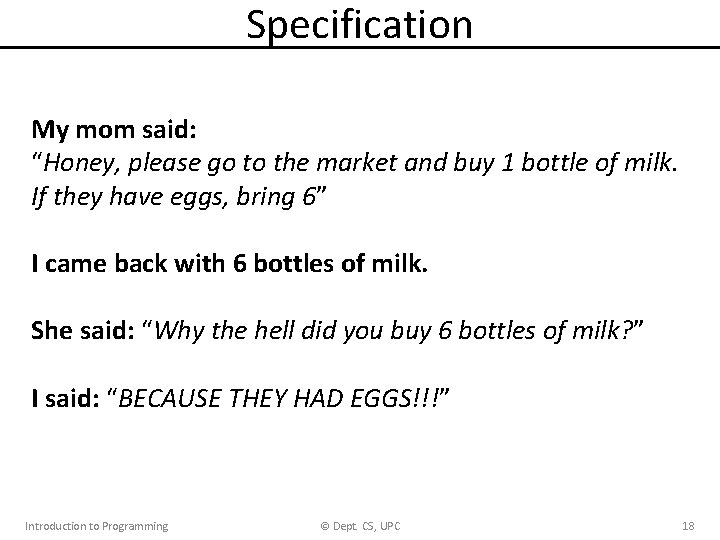
Specification My mom said: “Honey, please go to the market and buy 1 bottle of milk. If they have eggs, bring 6” I came back with 6 bottles of milk. She said: “Why the hell did you buy 6 bottles of milk? ” I said: “BECAUSE THEY HAD EGGS!!!” Introduction to Programming © Dept. CS, UPC 18
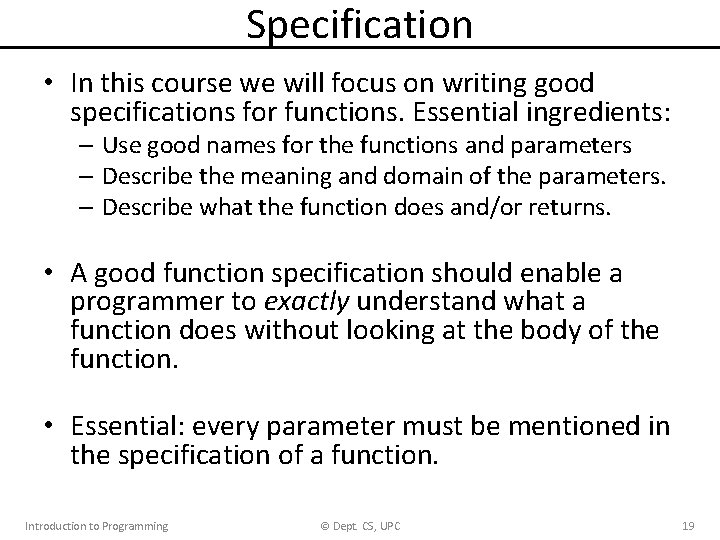
Specification • In this course we will focus on writing good specifications for functions. Essential ingredients: – Use good names for the functions and parameters – Describe the meaning and domain of the parameters. – Describe what the function does and/or returns. • A good function specification should enable a programmer to exactly understand what a function does without looking at the body of the function. • Essential: every parameter must be mentioned in the specification of a function. Introduction to Programming © Dept. CS, UPC 19
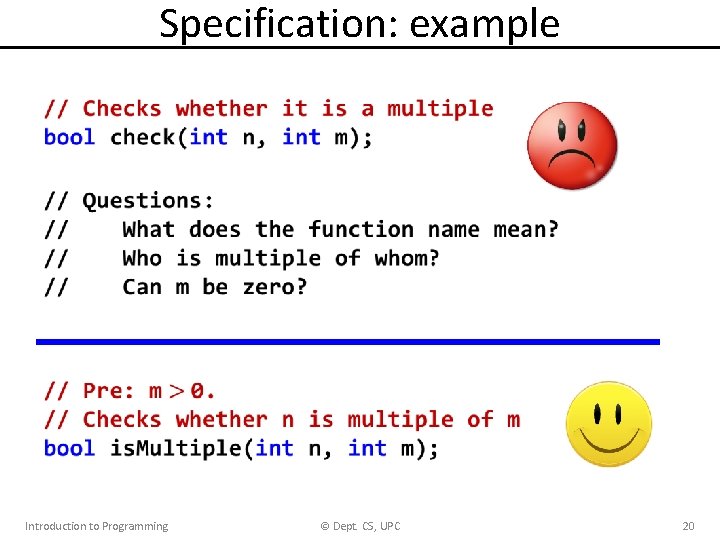
Specification: example Introduction to Programming © Dept. CS, UPC 20
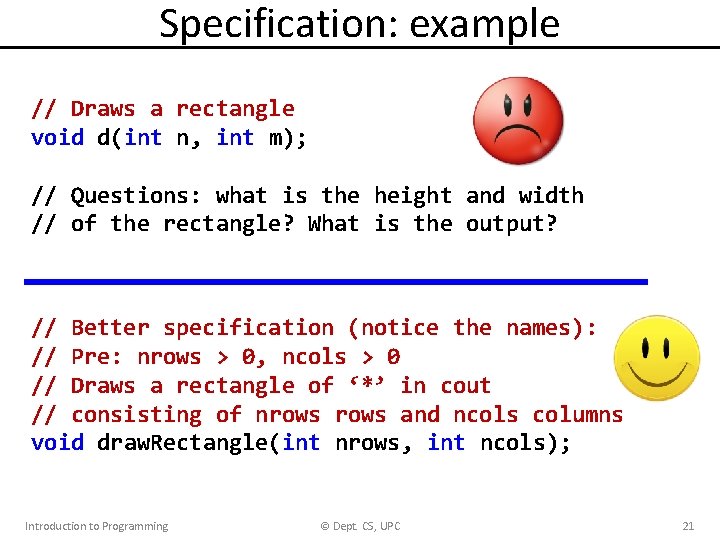
Specification: example // Draws a rectangle void d(int n, int m); // Questions: what is the height and width // of the rectangle? What is the output? // Better specification (notice the names): // Pre: nrows > 0, ncols > 0 // Draws a rectangle of ‘*’ in cout // consisting of nrows and ncols columns void draw. Rectangle(int nrows, int ncols); Introduction to Programming © Dept. CS, UPC 21
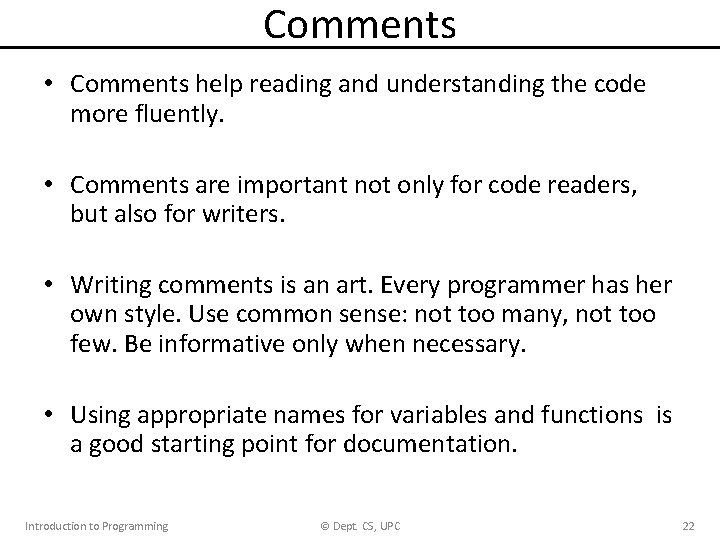
Comments • Comments help reading and understanding the code more fluently. • Comments are important not only for code readers, but also for writers. • Writing comments is an art. Every programmer has her own style. Use common sense: not too many, not too few. Be informative only when necessary. • Using appropriate names for variables and functions is a good starting point for documentation. Introduction to Programming © Dept. CS, UPC 22
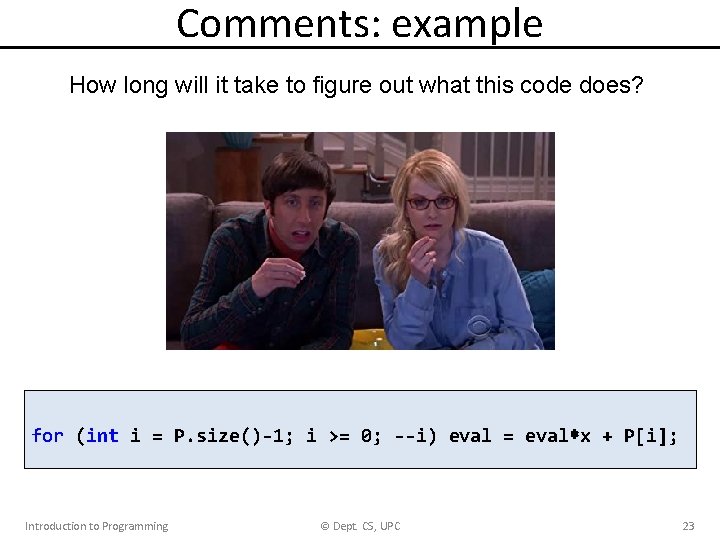
Comments: example How long will it take to figure out what this code does? for (int i = P. size()-1; i >= 0; --i) eval = eval x + P[i]; Introduction to Programming © Dept. CS, UPC 23
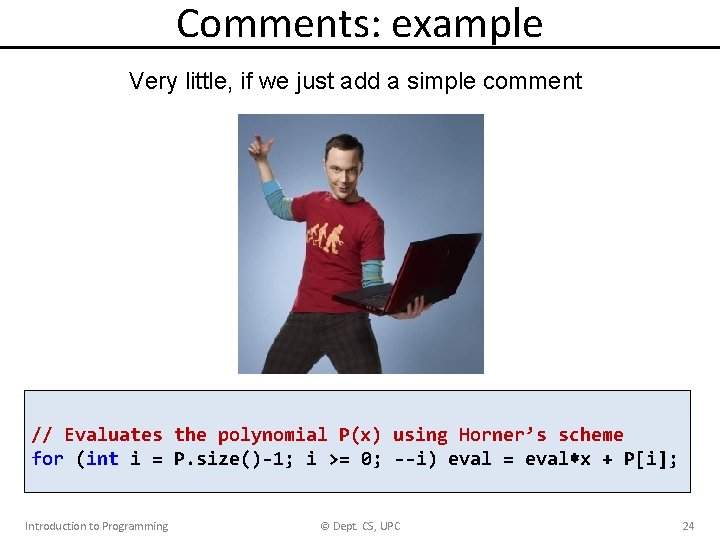
Comments: example Very little, if we just add a simple comment // Evaluates the polynomial P(x) using Horner’s scheme for (int i = P. size()-1; i >= 0; --i) eval = eval x + P[i]; Introduction to Programming © Dept. CS, UPC 24
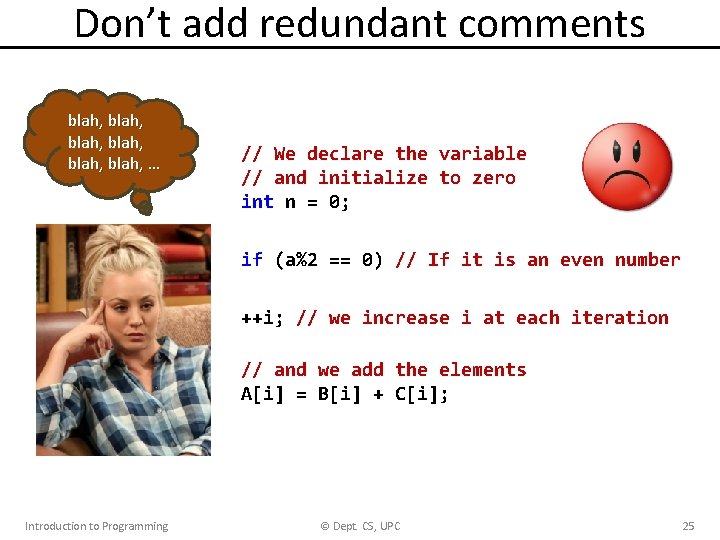
Don’t add redundant comments blah, blah, … // We declare the variable // and initialize to zero int n = 0; if (a%2 == 0) // If it is an even number ++i; // we increase i at each iteration // and we add the elements A[i] = B[i] + C[i]; Introduction to Programming © Dept. CS, UPC 25
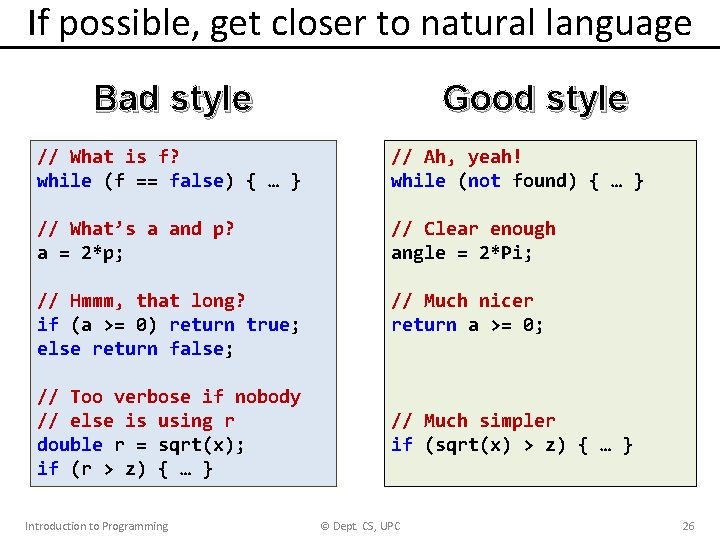
If possible, get closer to natural language Bad style Good style // What is f? while (f == false) { … } // Ah, yeah! while (not found) { … } // What’s a and p? a = 2*p; // Clear enough angle = 2*Pi; // Hmmm, that long? if (a >= 0) return true; else return false; // Much nicer return a >= 0; // Too verbose if nobody // else is using r double r = sqrt(x); if (r > z) { … } Introduction to Programming // Much simpler if (sqrt(x) > z) { … } © Dept. CS, UPC 26
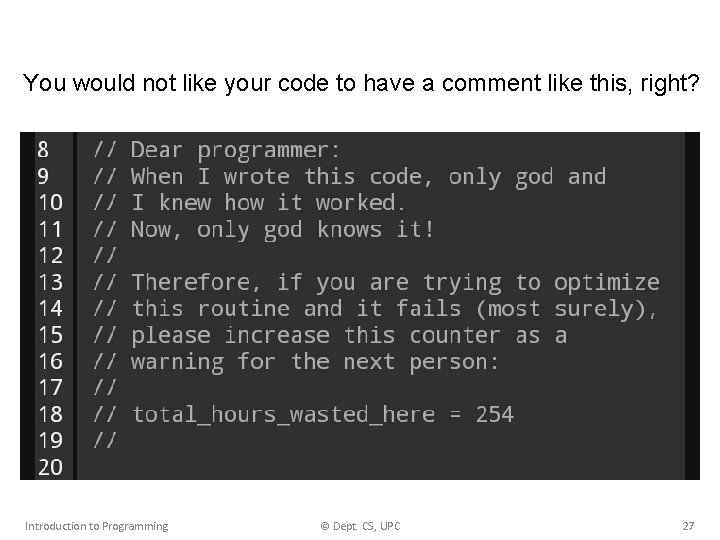
You would not like your code to have a comment like this, right? Introduction to Programming © Dept. CS, UPC 27
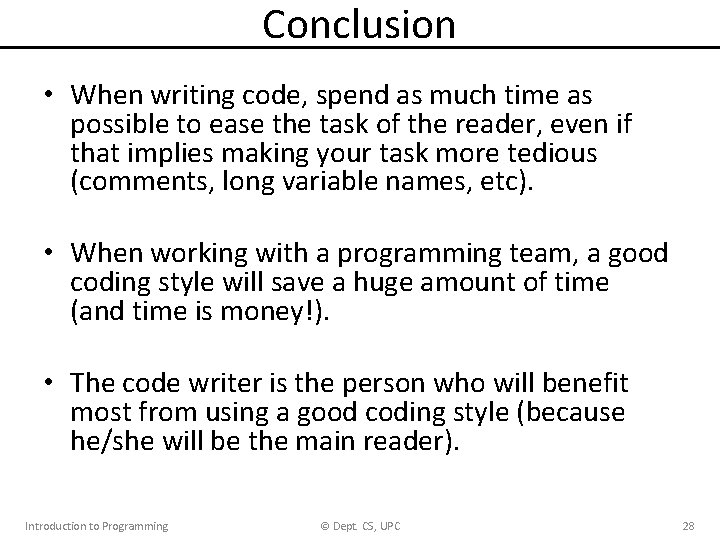
Conclusion • When writing code, spend as much time as possible to ease the task of the reader, even if that implies making your task more tedious (comments, long variable names, etc). • When working with a programming team, a good coding style will save a huge amount of time (and time is money!). • The code writer is the person who will benefit most from using a good coding style (because he/she will be the main reader). Introduction to Programming © Dept. CS, UPC 28
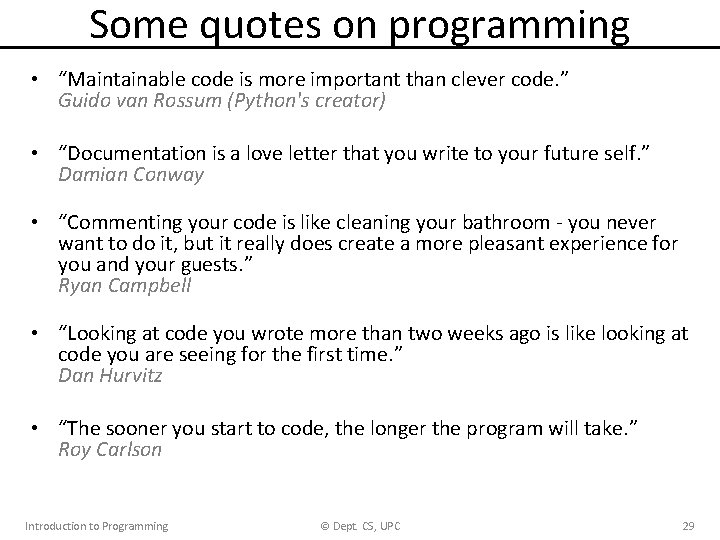
Some quotes on programming • “Maintainable code is more important than clever code. ” Guido van Rossum (Python's creator) • “Documentation is a love letter that you write to your future self. ” Damian Conway • “Commenting your code is like cleaning your bathroom - you never want to do it, but it really does create a more pleasant experience for you and your guests. ” Ryan Campbell • “Looking at code you wrote more than two weeks ago is like looking at code you are seeing for the first time. ” Dan Hurvitz • “The sooner you start to code, the longer the program will take. ” Roy Carlson Introduction to Programming © Dept. CS, UPC 29
Strongly connected components
Ucl computer science meng
Northwestern university computer engineering
Computer science department rutgers
Stanford computer science department
Computer science fsu
Trimentoring
Bhargavi goswami
Mice.cs.columbia
Jordi reviriego
Jordi ustrell
Jordi benlliure
Language telegram
Jordi juanico sabate
Jordi timmers
Jordi ayala
Jordi vives i batlle
Jordi garcia cehic
Jordi graells costa
Jordi npa
Jordi scene
Cmedium
Jordi olivares
Jordi gisbert
Dev23
Busceral
My favourite subject computer
What is block letter style with open punctuation
Informal style of writing examples
Informal vs formal writing