Reusing computations Jordi Cortadella Department of Computer Science
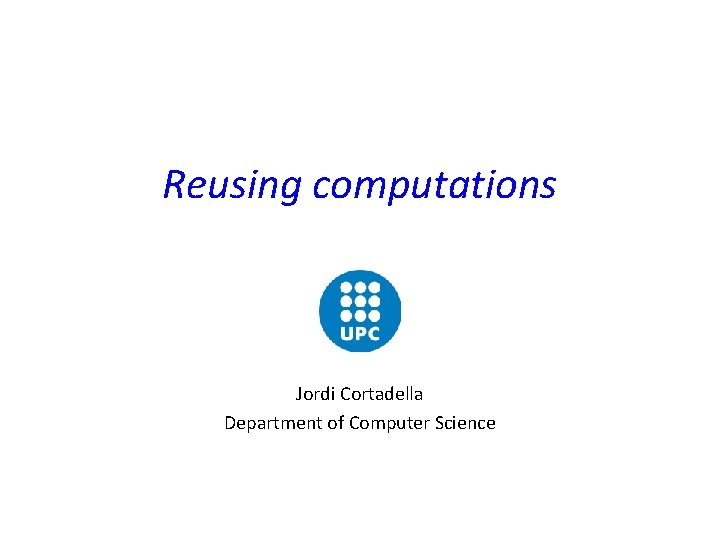
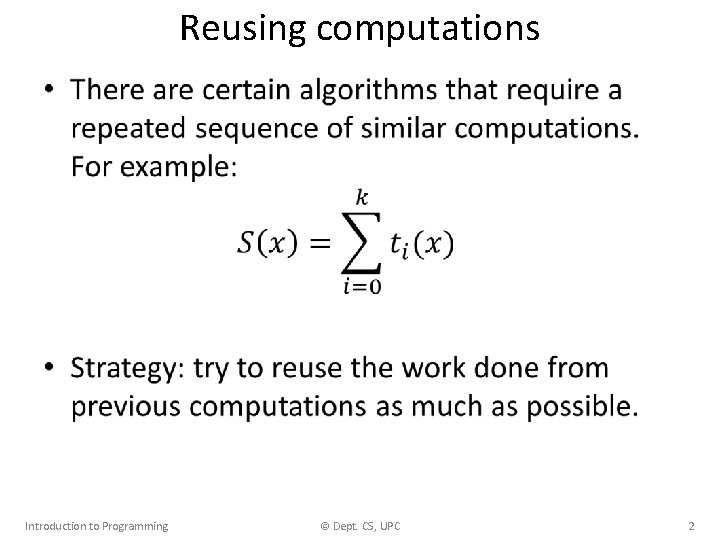
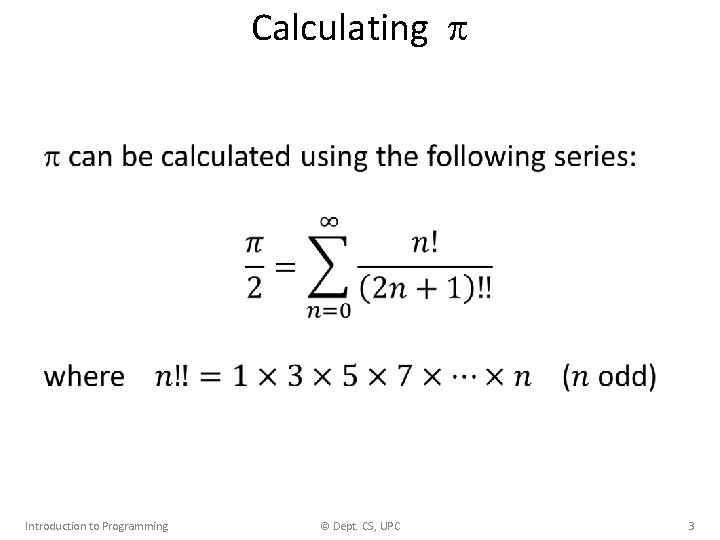
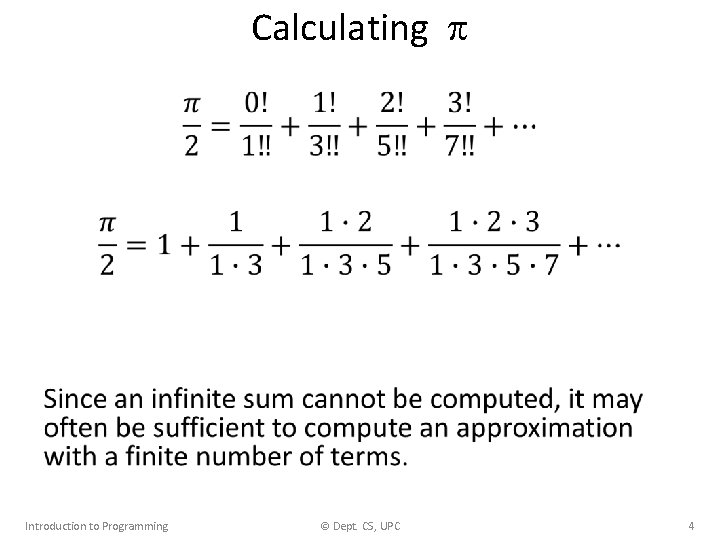
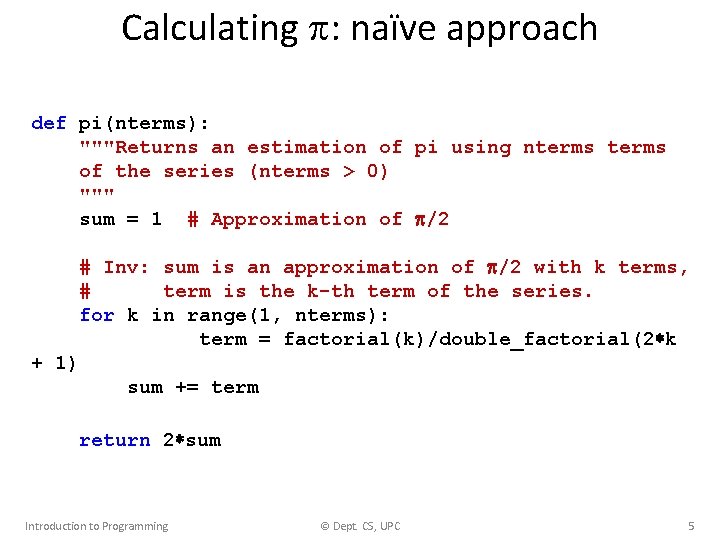
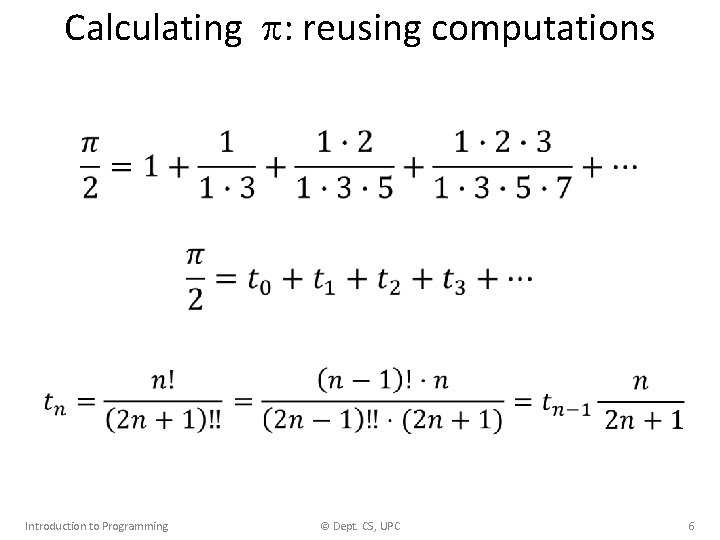
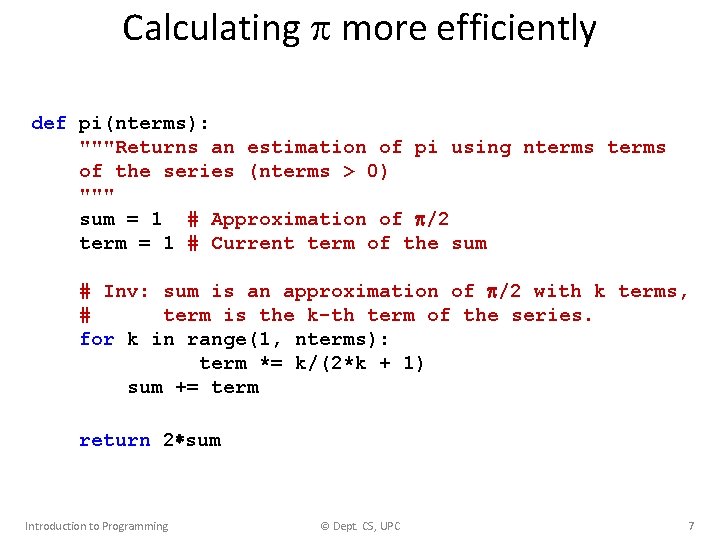
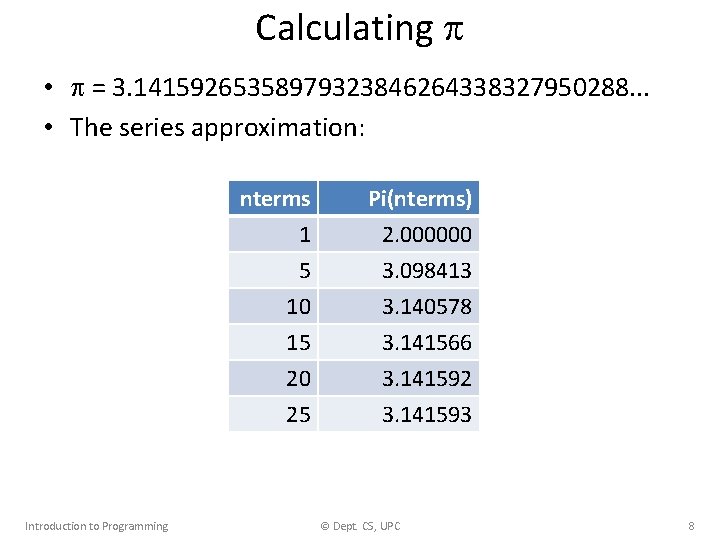
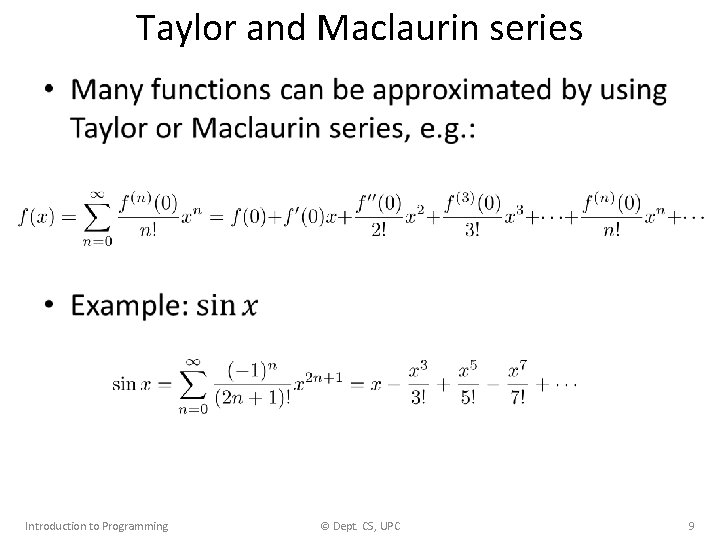
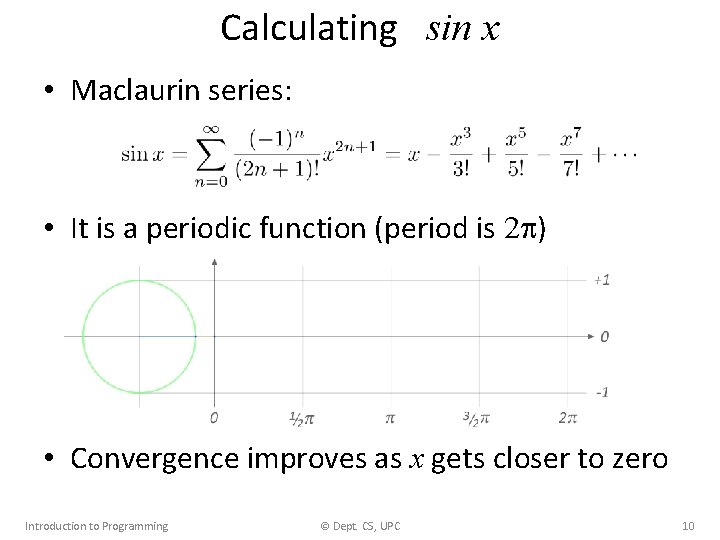
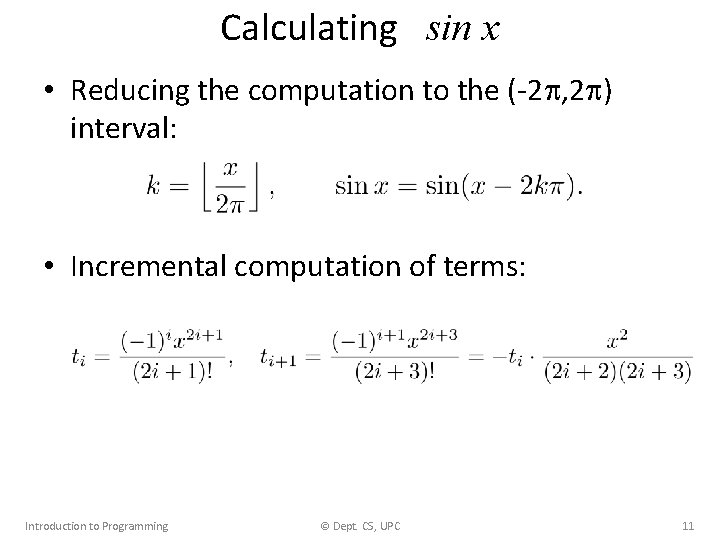
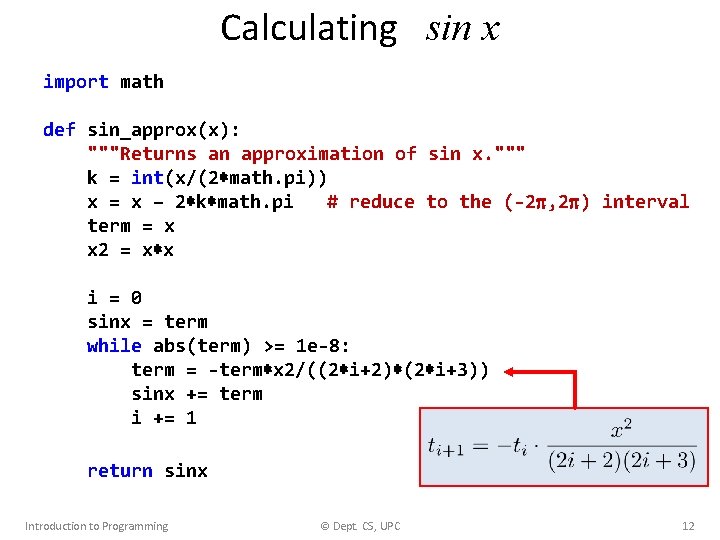
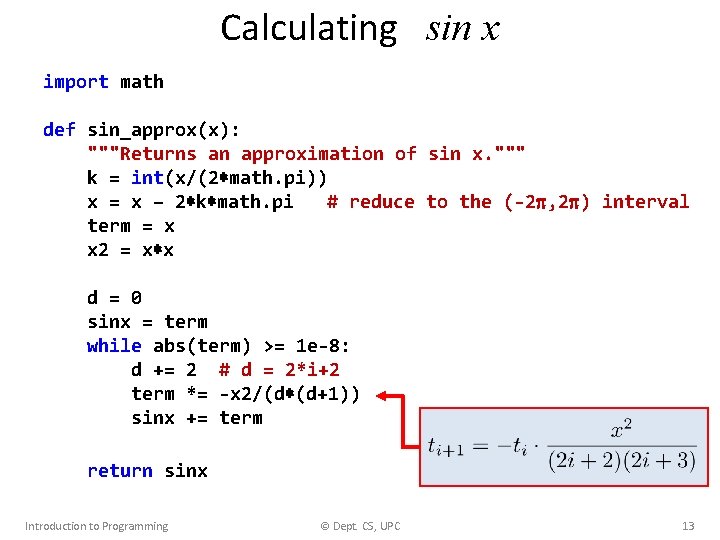
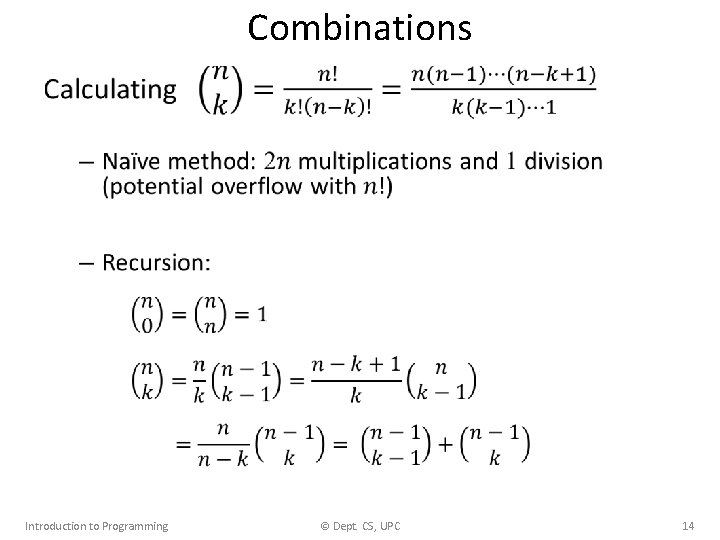
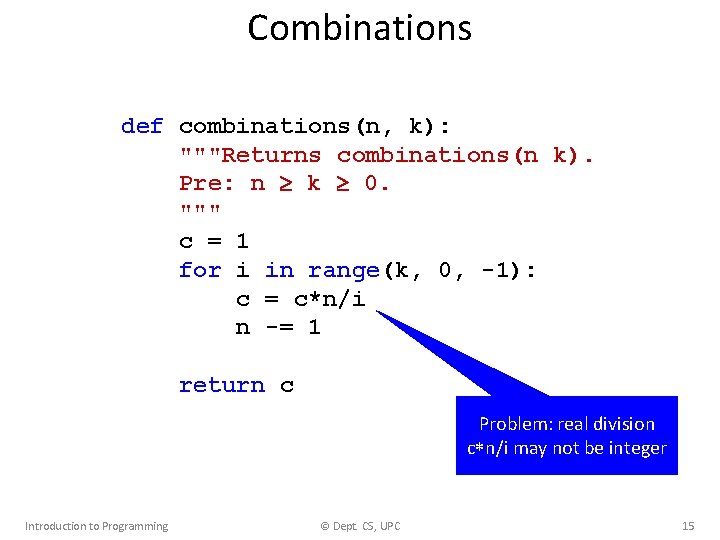
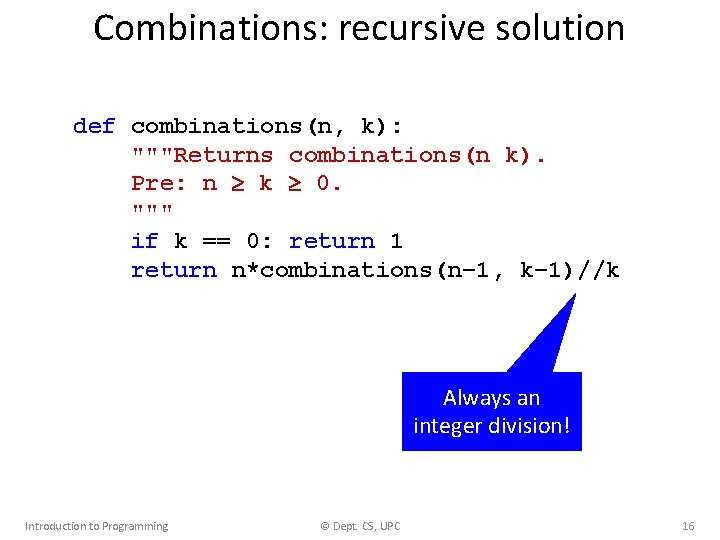
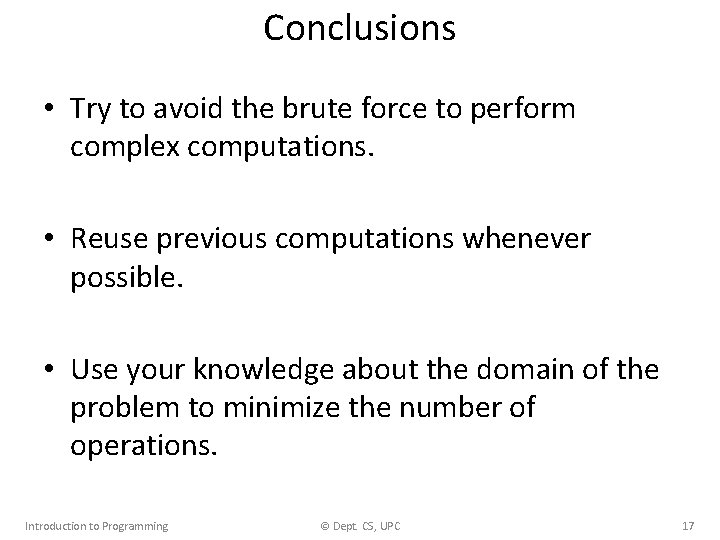
- Slides: 17
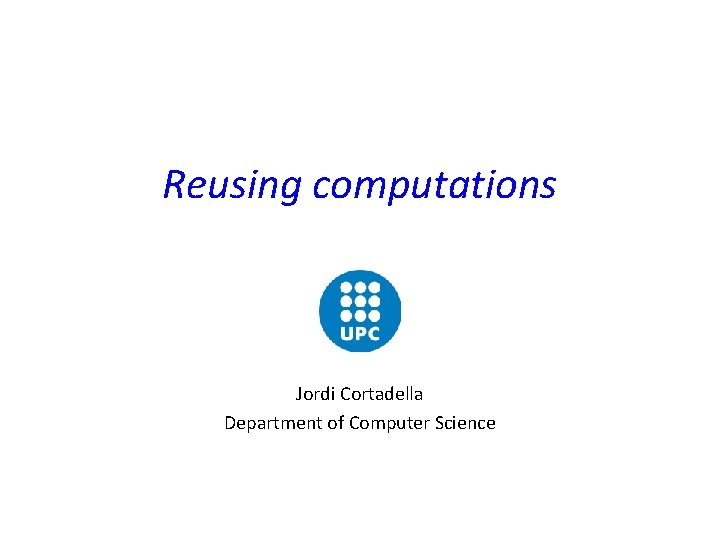
Reusing computations Jordi Cortadella Department of Computer Science
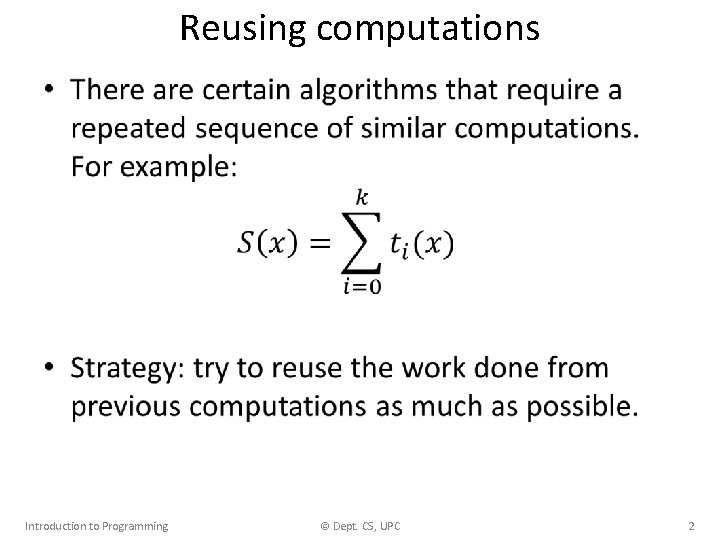
Reusing computations • Introduction to Programming © Dept. CS, UPC 2
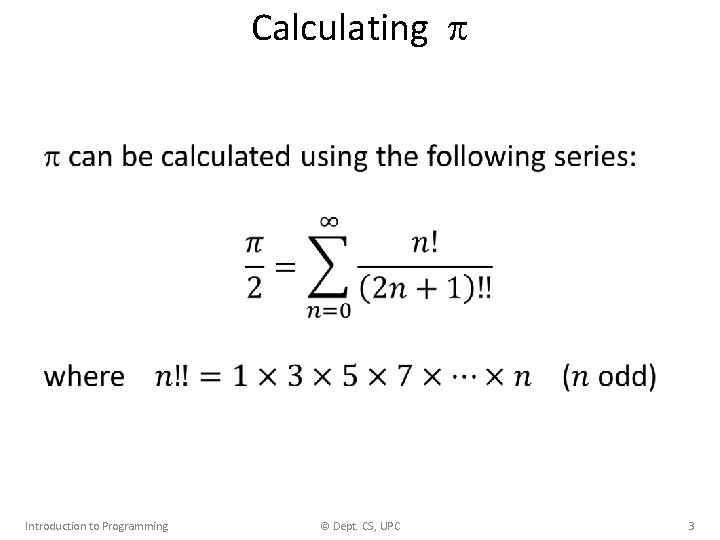
Calculating • Introduction to Programming © Dept. CS, UPC 3
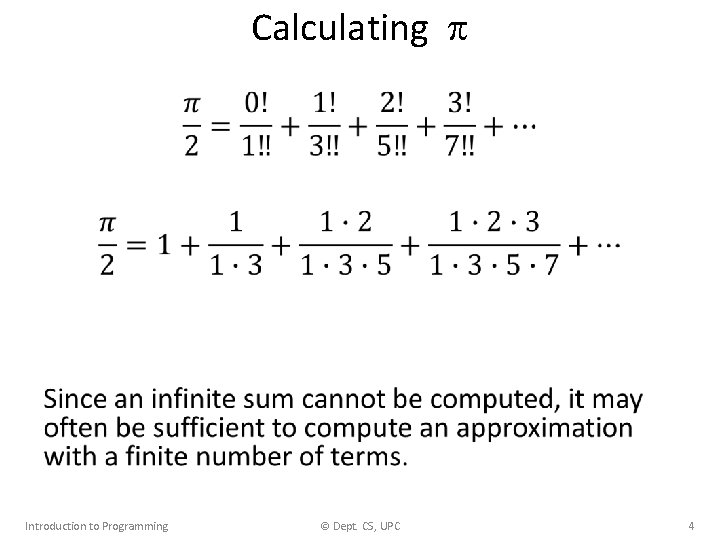
Calculating • Introduction to Programming © Dept. CS, UPC 4
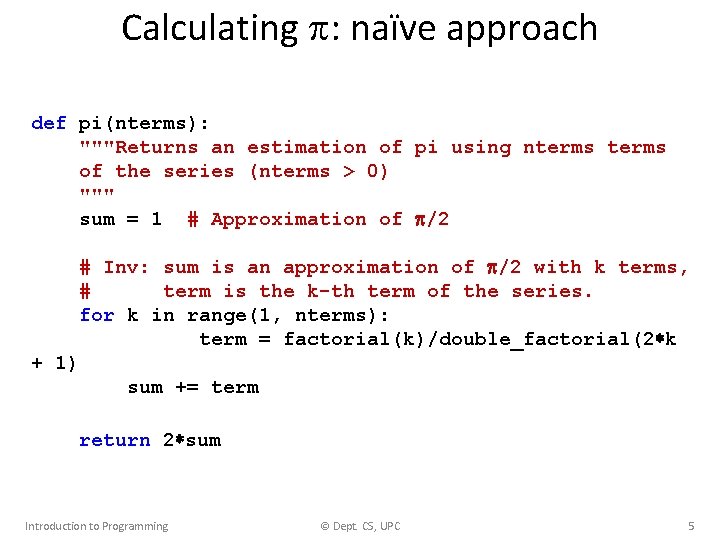
Calculating : naïve approach def pi(nterms): """Returns an estimation of pi using nterms of the series (nterms > 0) """ sum = 1 # Approximation of /2 # Inv: sum is an approximation of /2 with k terms, # term is the k-th term of the series. for k in range(1, nterms): term = factorial(k)/double_factorial(2 k + 1) sum += term return 2 sum Introduction to Programming © Dept. CS, UPC 5
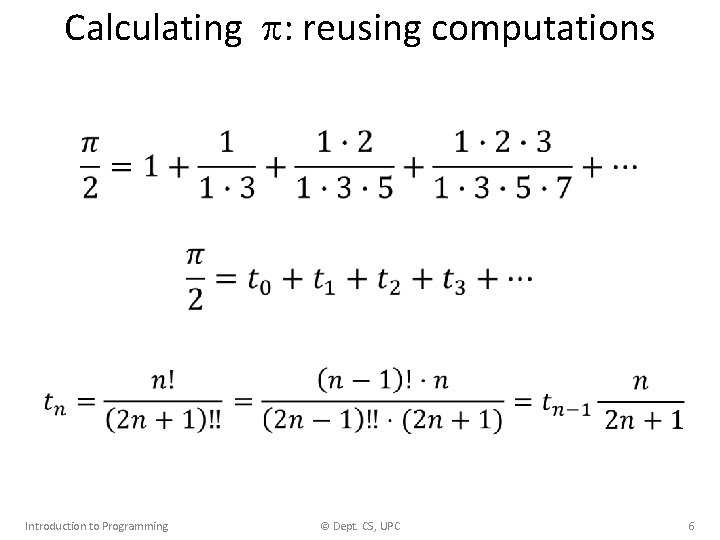
Calculating : reusing computations • Introduction to Programming © Dept. CS, UPC 6
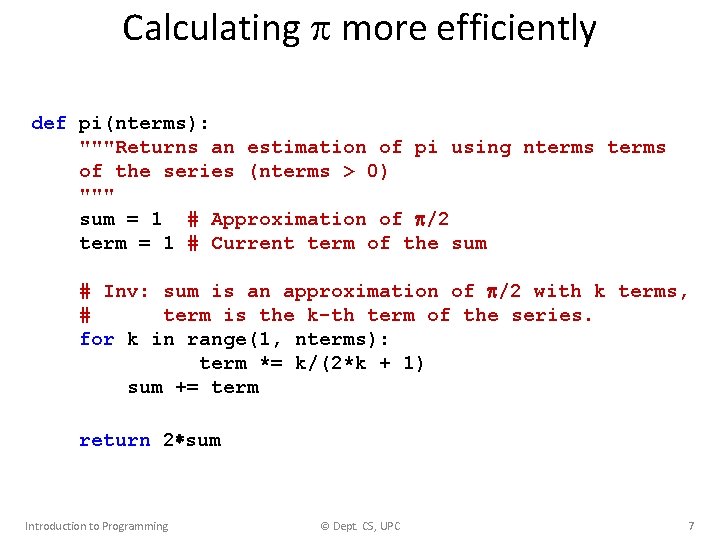
Calculating more efficiently def pi(nterms): """Returns an estimation of pi using nterms of the series (nterms > 0) """ sum = 1 # Approximation of /2 term = 1 # Current term of the sum # Inv: sum is an approximation of /2 with k terms, # term is the k-th term of the series. for k in range(1, nterms): term *= k/(2*k + 1) sum += term return 2 sum Introduction to Programming © Dept. CS, UPC 7
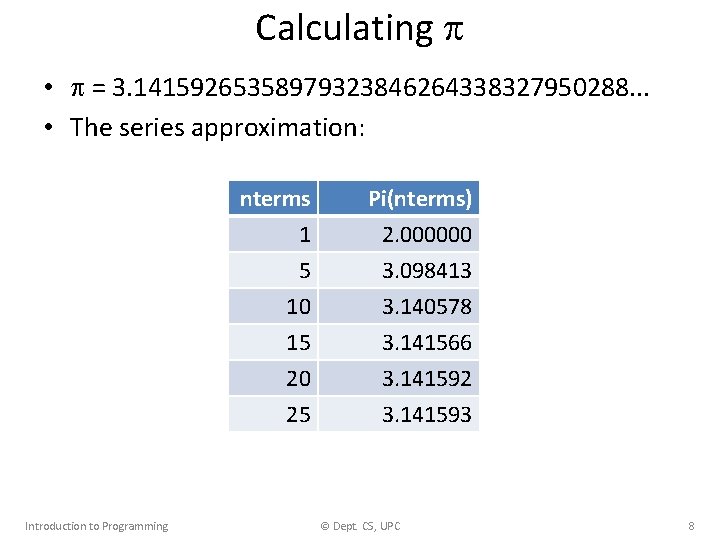
Calculating • = 3. 14159265358979323846264338327950288. . . • The series approximation: Introduction to Programming nterms 1 5 10 Pi(nterms) 2. 000000 3. 098413 3. 140578 15 20 25 3. 141566 3. 141592 3. 141593 © Dept. CS, UPC 8
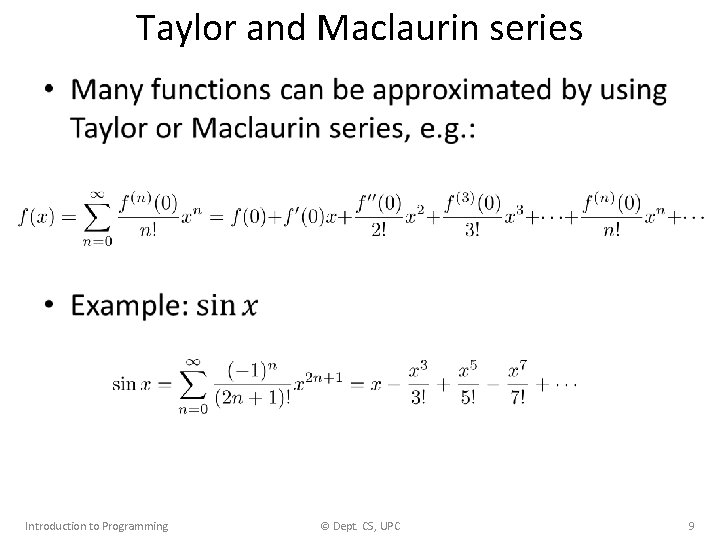
Taylor and Maclaurin series • Introduction to Programming © Dept. CS, UPC 9
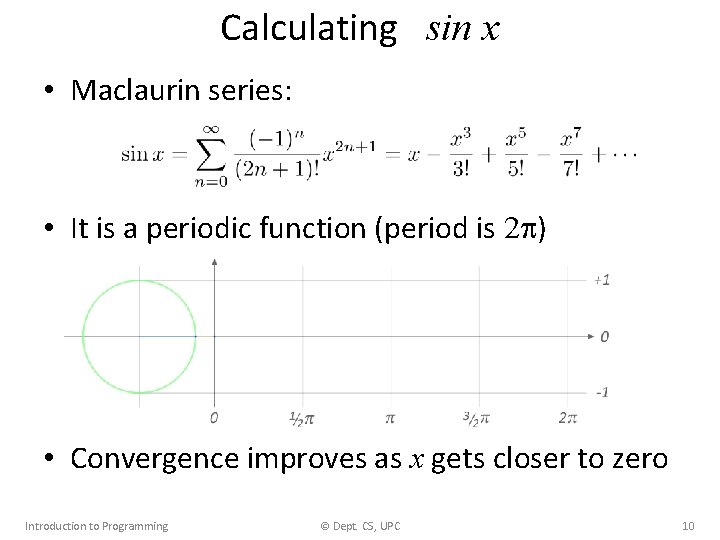
Calculating sin x • Maclaurin series: • It is a periodic function (period is 2 ) • Convergence improves as x gets closer to zero Introduction to Programming © Dept. CS, UPC 10
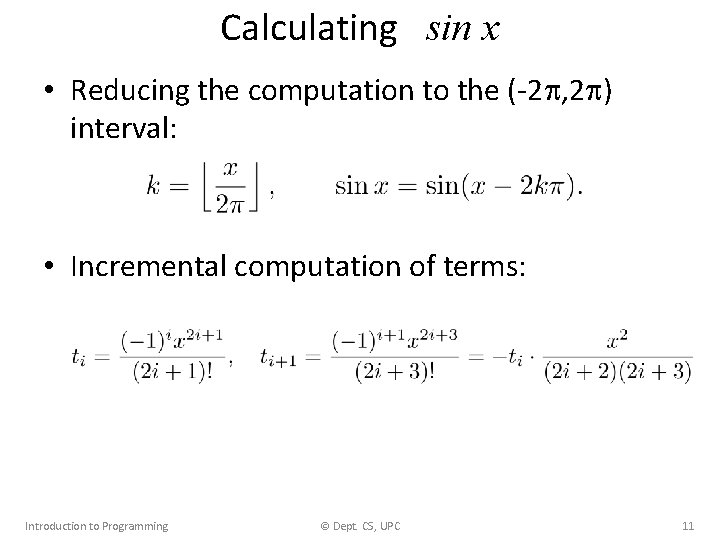
Calculating sin x • Reducing the computation to the (-2 , 2 ) interval: • Incremental computation of terms: Introduction to Programming © Dept. CS, UPC 11
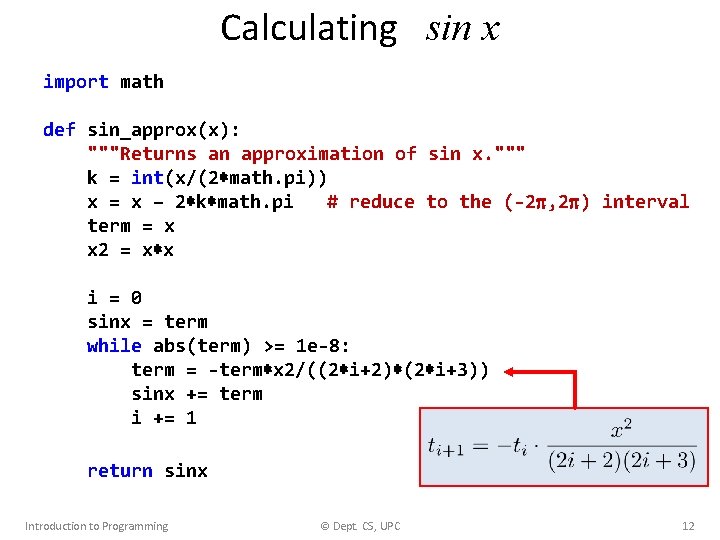
Calculating sin x import math def sin_approx(x): """Returns an approximation of sin x. """ k = int(x/(2 math. pi)) x = x – 2 k math. pi # reduce to the (-2 , 2 ) interval term = x x 2 = x x i = 0 sinx = term while abs(term) >= 1 e-8: term = -term x 2/((2 i+2) (2 i+3)) sinx += term i += 1 return sinx Introduction to Programming © Dept. CS, UPC 12
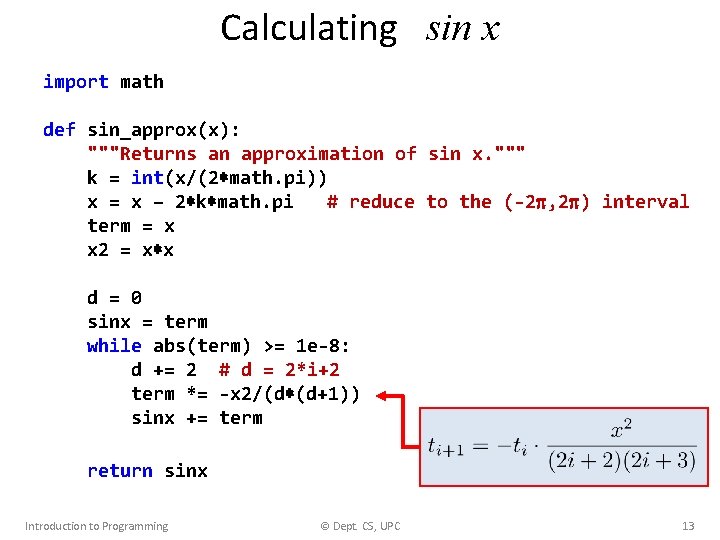
Calculating sin x import math def sin_approx(x): """Returns an approximation of sin x. """ k = int(x/(2 math. pi)) x = x – 2 k math. pi # reduce to the (-2 , 2 ) interval term = x x 2 = x x d = 0 sinx = term while abs(term) >= 1 e-8: d += 2 # d = 2*i+2 term *= -x 2/(d (d+1)) sinx += term return sinx Introduction to Programming © Dept. CS, UPC 13
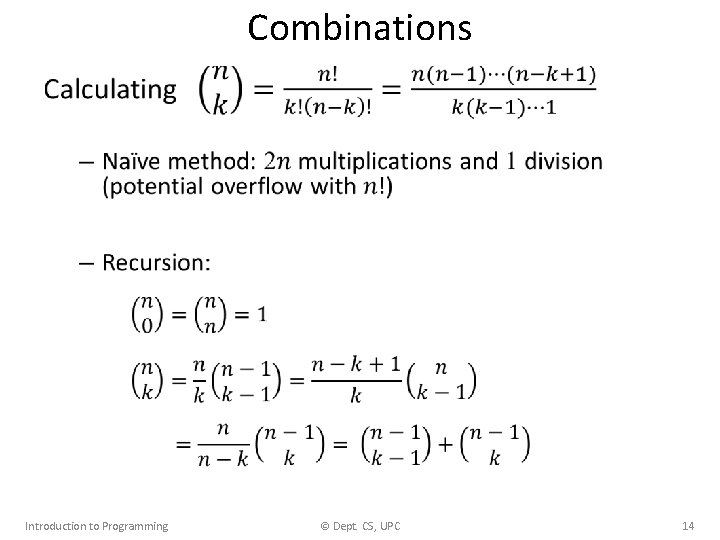
Combinations • Introduction to Programming © Dept. CS, UPC 14
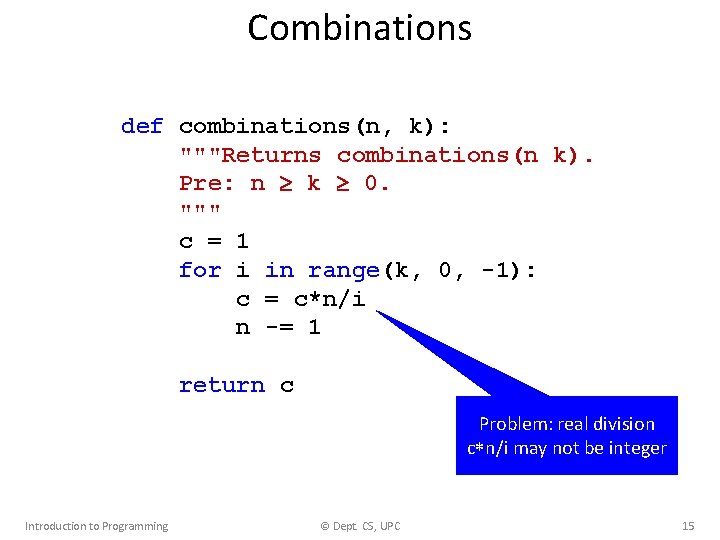
Combinations def combinations(n, k): """Returns combinations(n k). Pre: n k 0. """ c = 1 for i in range(k, 0, -1): c = c*n/i n -= 1 return c Problem: real division c n/i may not be integer Introduction to Programming © Dept. CS, UPC 15
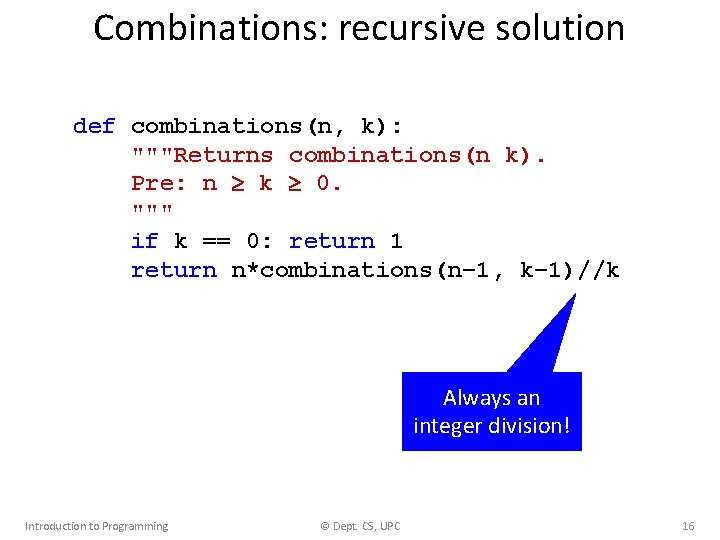
Combinations: recursive solution def combinations(n, k): """Returns combinations(n k). Pre: n k 0. """ if k == 0: return 1 return n*combinations(n– 1, k– 1)//k Always an integer division! Introduction to Programming © Dept. CS, UPC 16
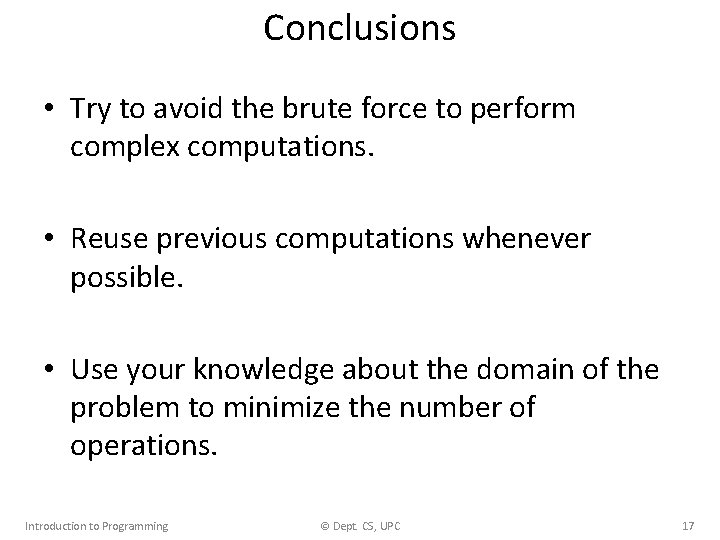
Conclusions • Try to avoid the brute force to perform complex computations. • Reuse previous computations whenever possible. • Use your knowledge about the domain of the problem to minimize the number of operations. Introduction to Programming © Dept. CS, UPC 17
Strongly connected components
Incrementalizing graph algorithms
Embarrassingly parallel
Ucl meng computer science
Electrical engineering northwestern
Computer science department rutgers
Meredith hutchin stanford
Fsu computer science department
Ubc computer science department
Department of computer science christ
Computer science department columbia
Jordi reviriego
Jordi ustrell
Jordi benlliure
Language telegram
Jordi juanico sabate
Jordi timmers
Jordi ayala