Chapter 16 1 Linked Structures DaleWeems 1 Chapter
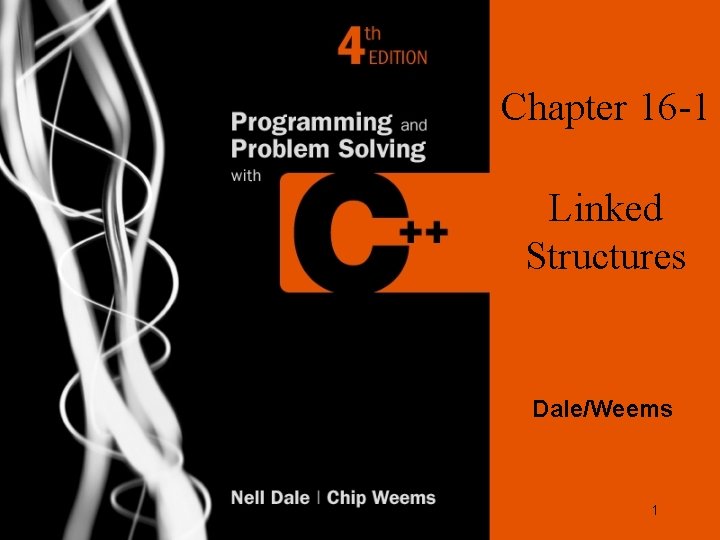
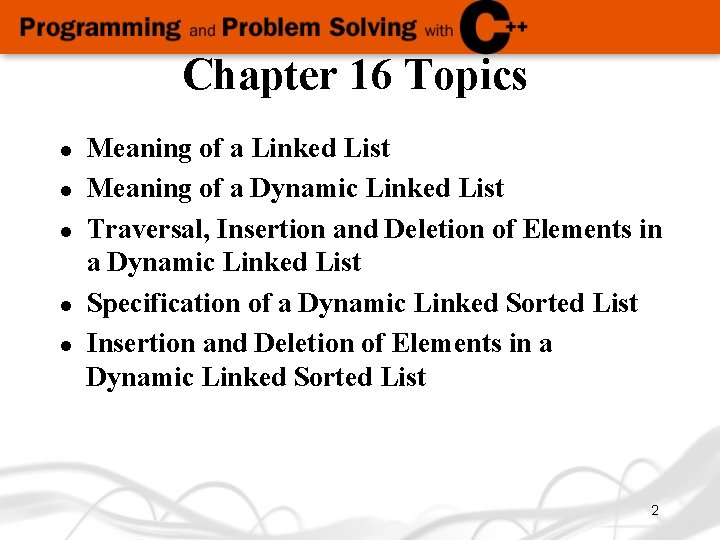
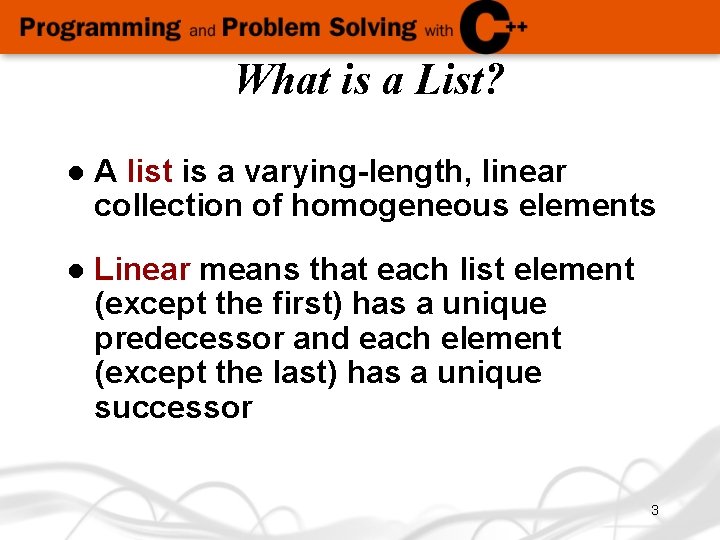
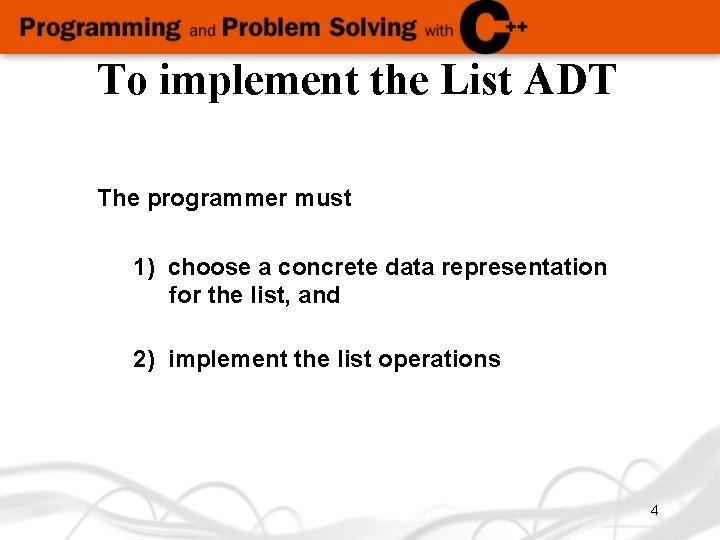
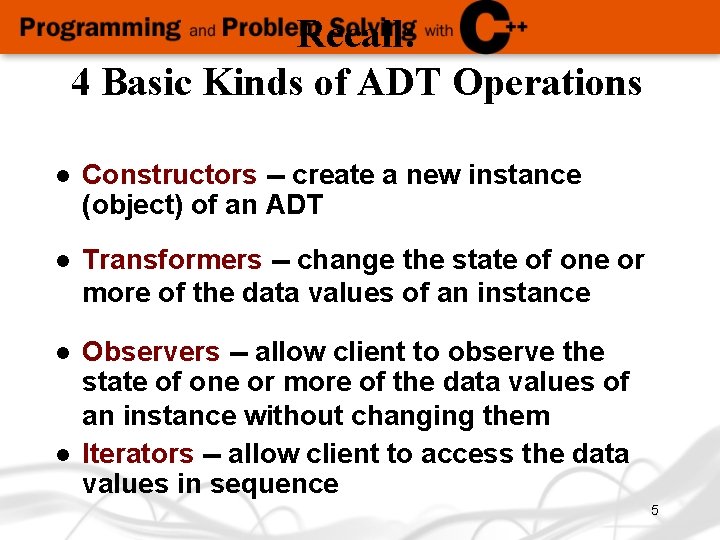
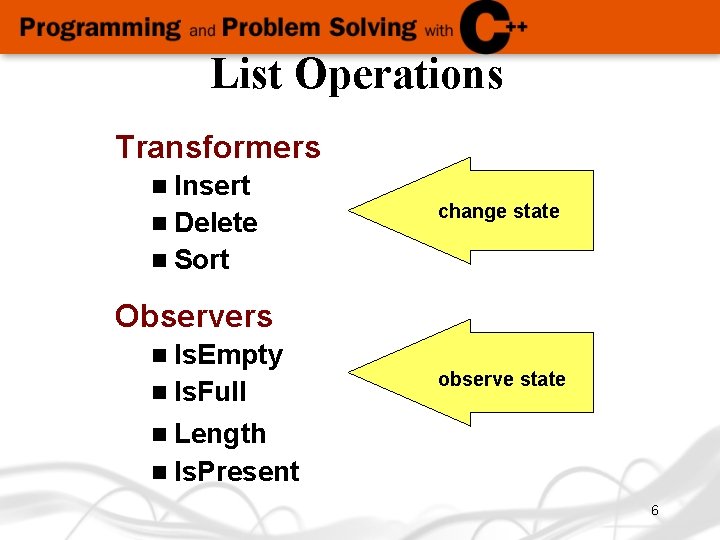
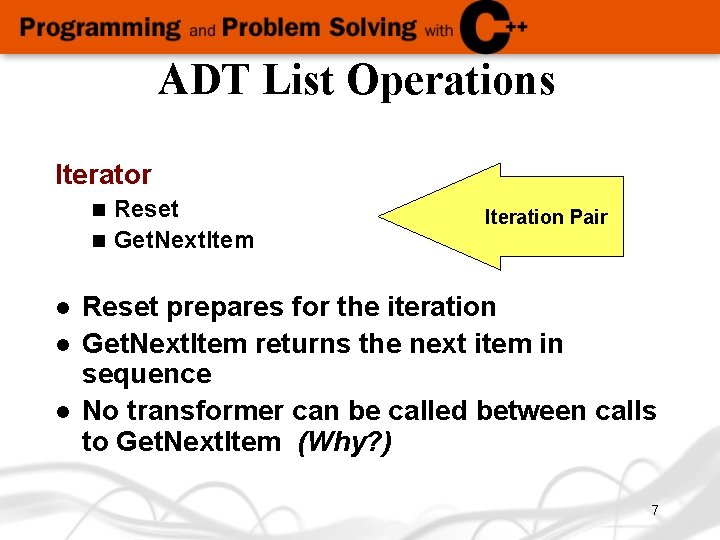
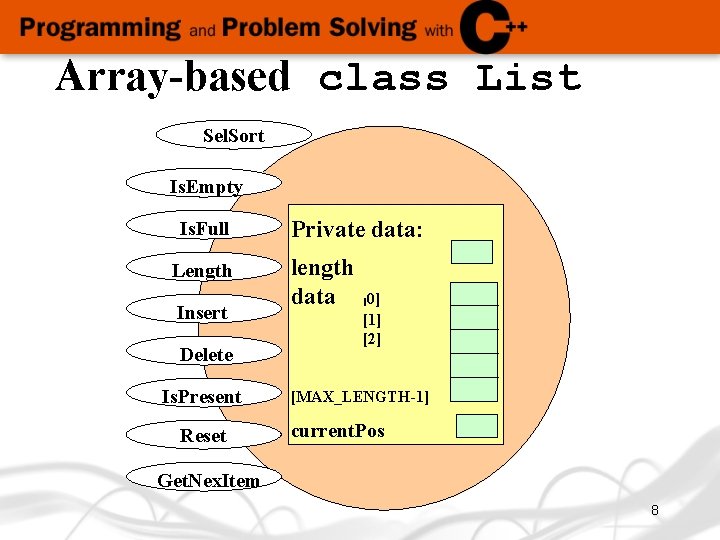
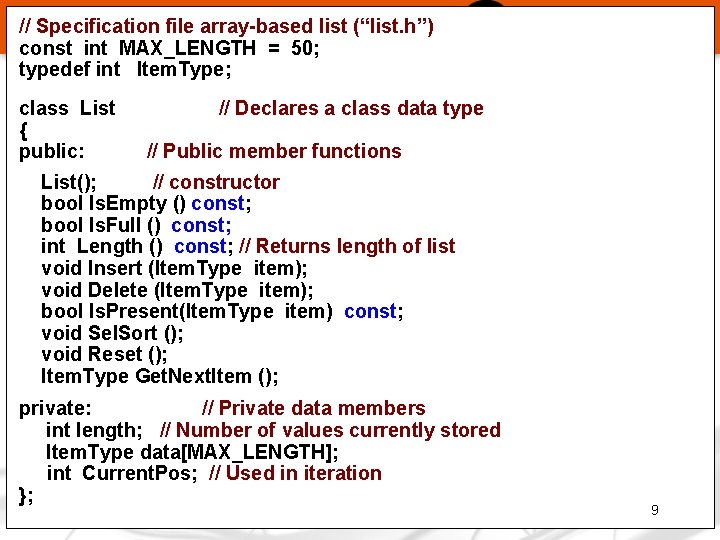
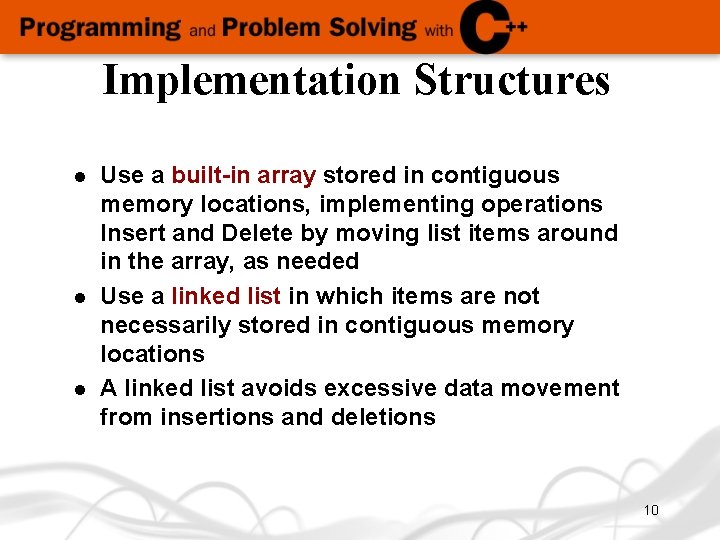
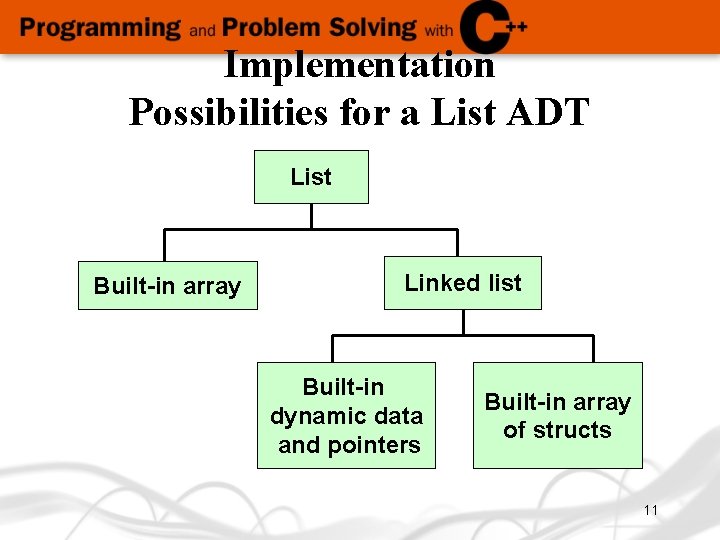
![Array Representation of a Linked List component link head 2 Node[0] 58 -1 Node[1] Array Representation of a Linked List component link head 2 Node[0] 58 -1 Node[1]](https://slidetodoc.com/presentation_image_h2/6dbb982debd59de2651351a48091c898/image-12.jpg)
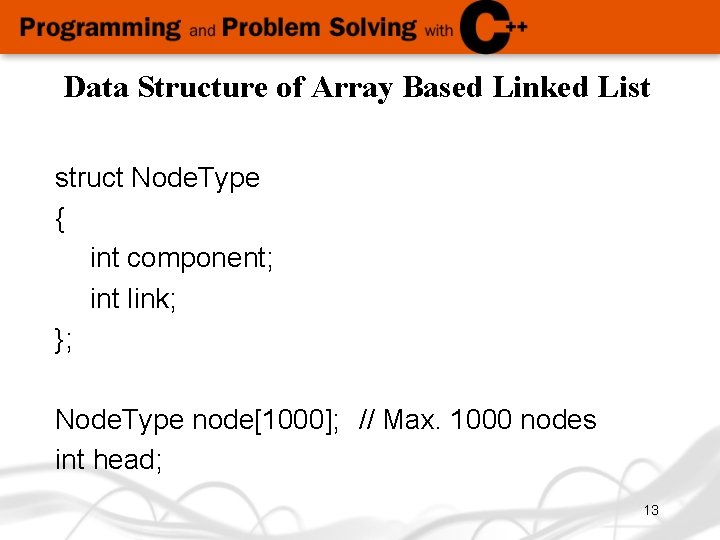
![Insert a New Node into a Linked List component link head Node[0] 58 2 Insert a New Node into a Linked List component link head Node[0] 58 2](https://slidetodoc.com/presentation_image_h2/6dbb982debd59de2651351a48091c898/image-14.jpg)
![Delete a Node from a Linked List component link head Node[0] 58 2 Node[1] Delete a Node from a Linked List component link head Node[0] 58 2 Node[1]](https://slidetodoc.com/presentation_image_h2/6dbb982debd59de2651351a48091c898/image-15.jpg)
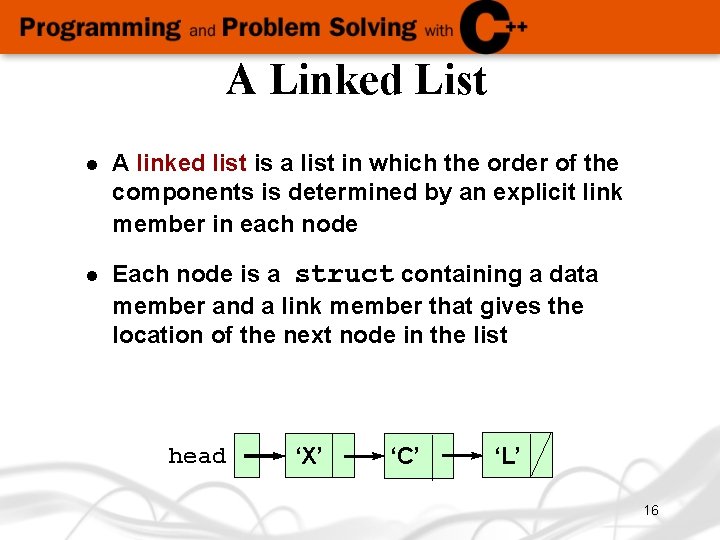
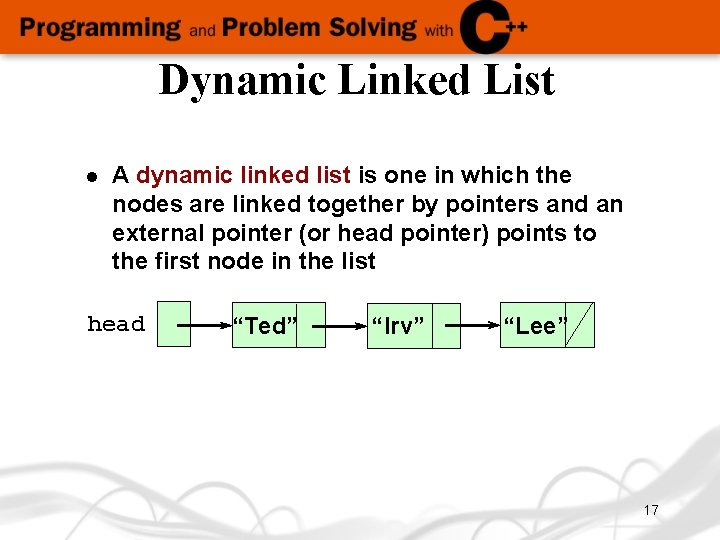
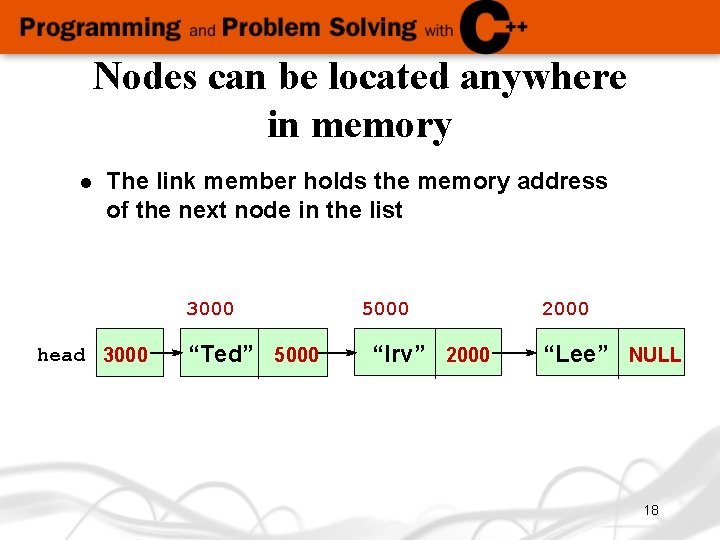
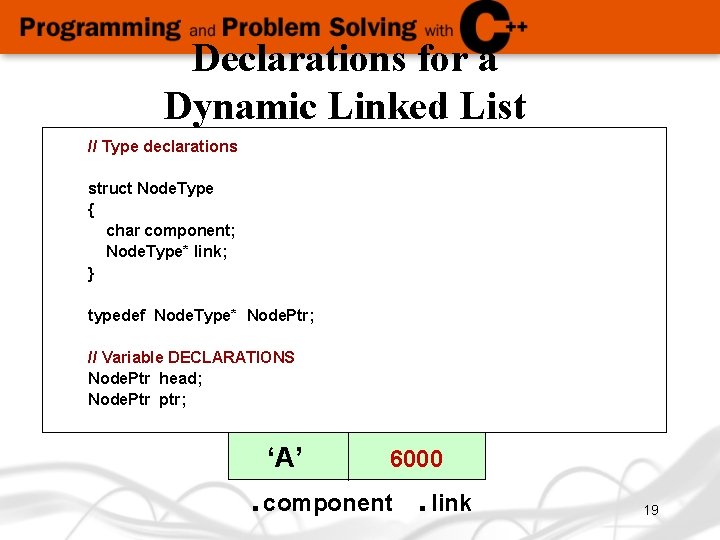
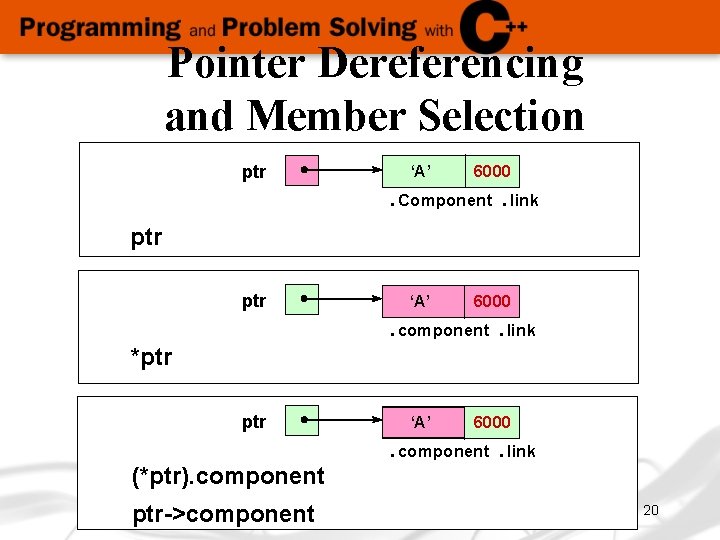
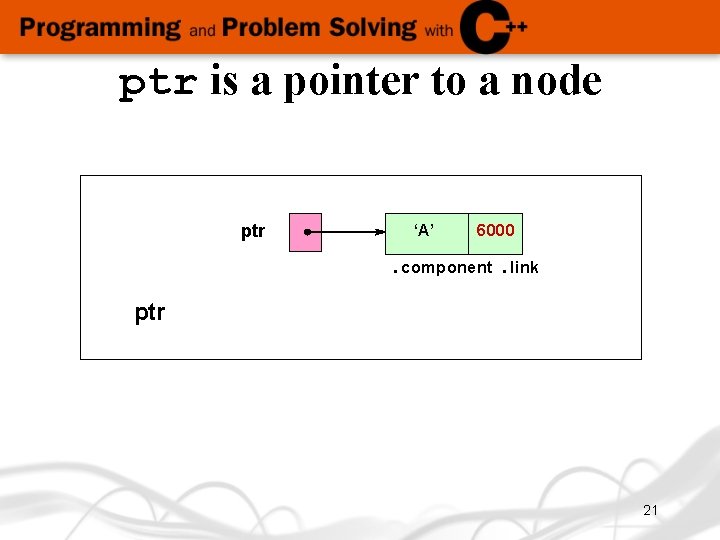
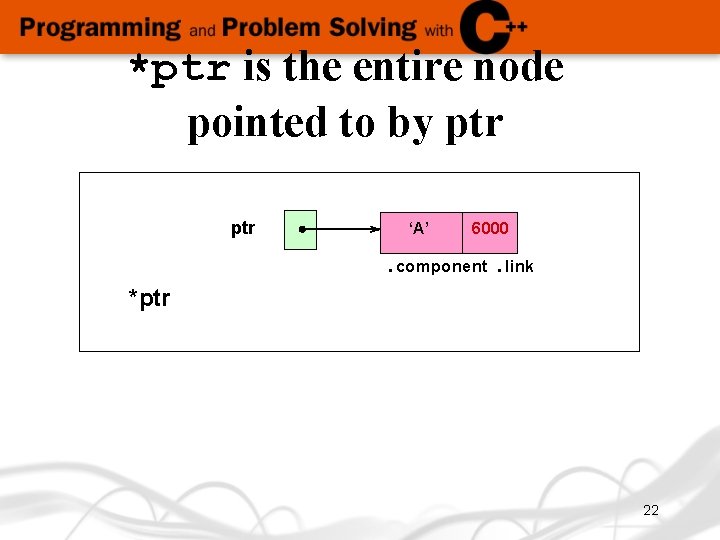
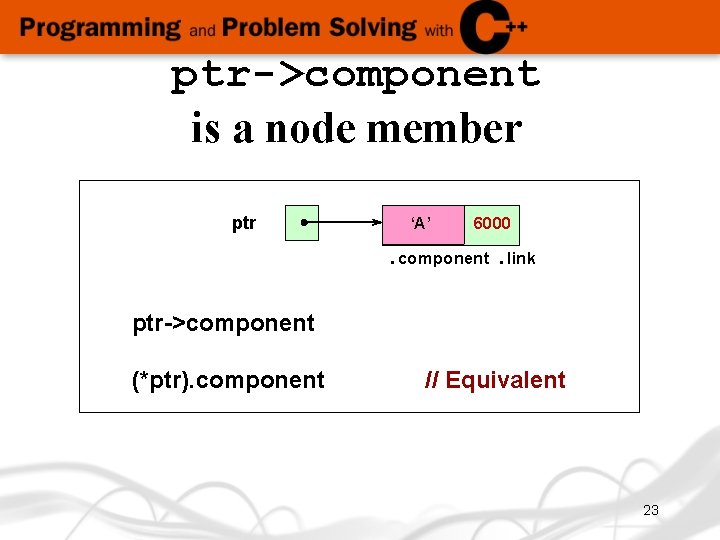
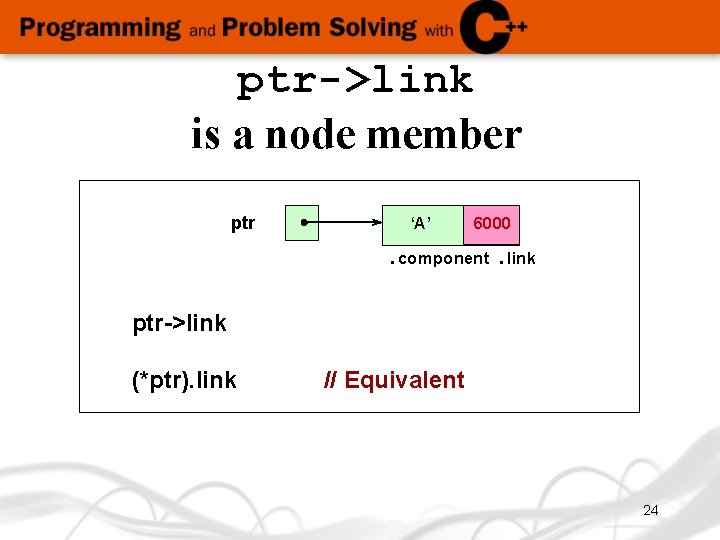
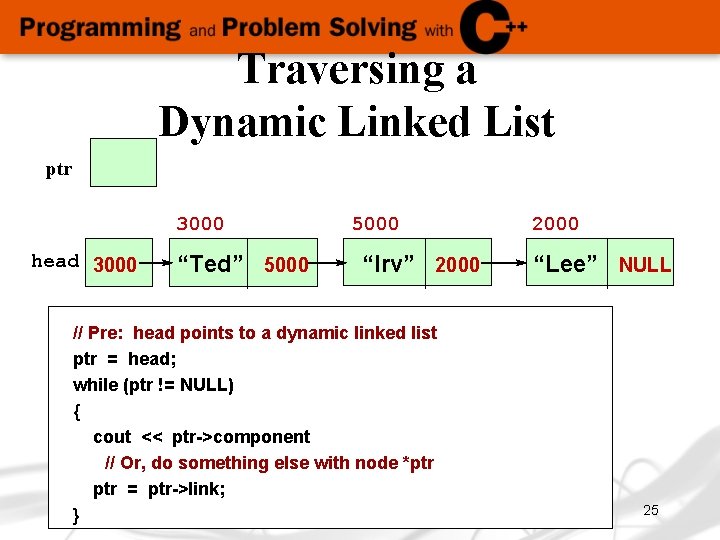
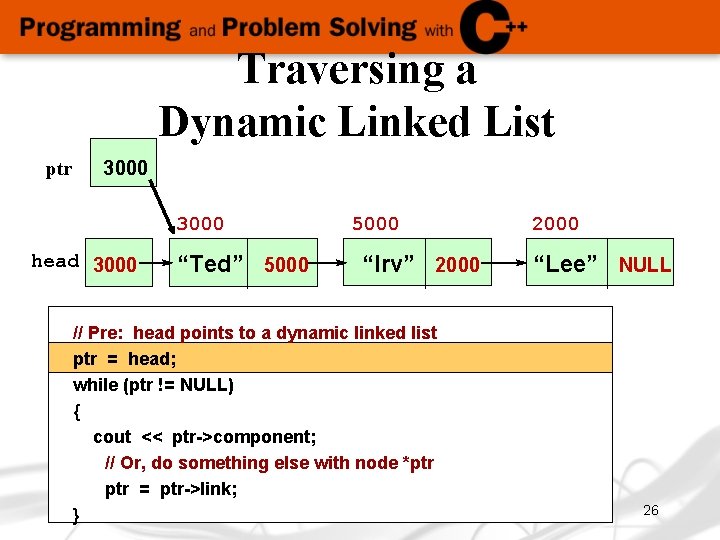
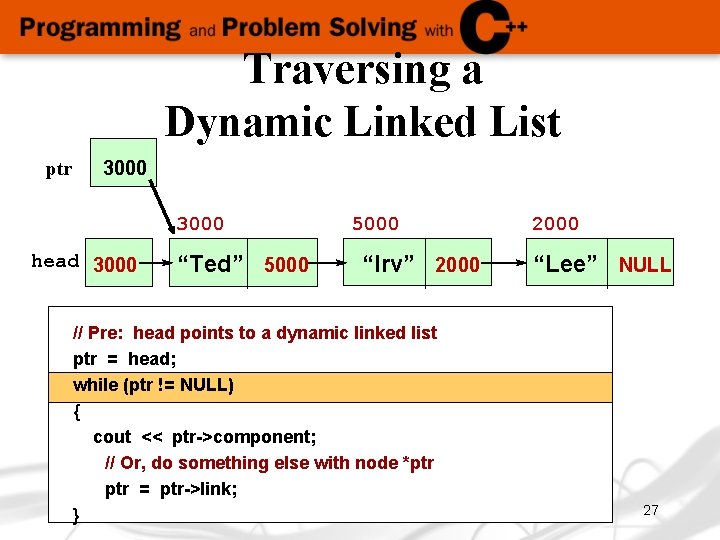
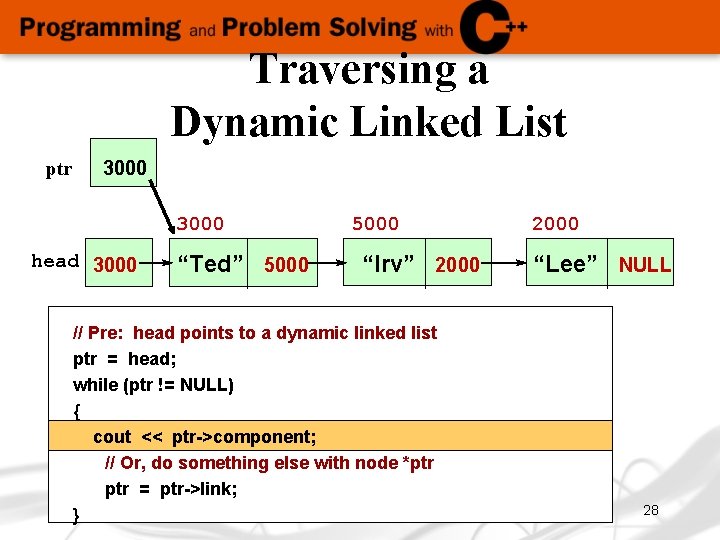
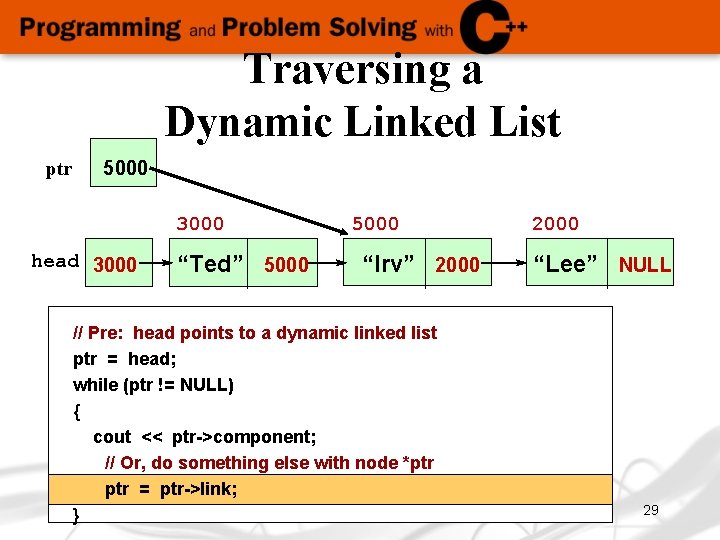
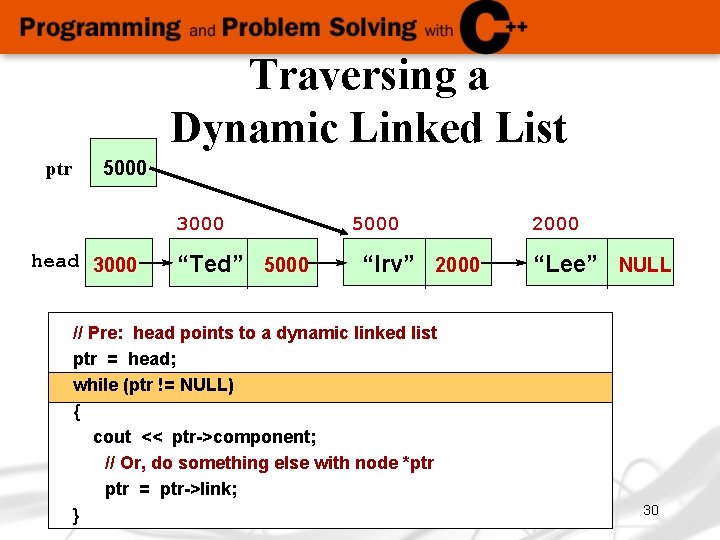
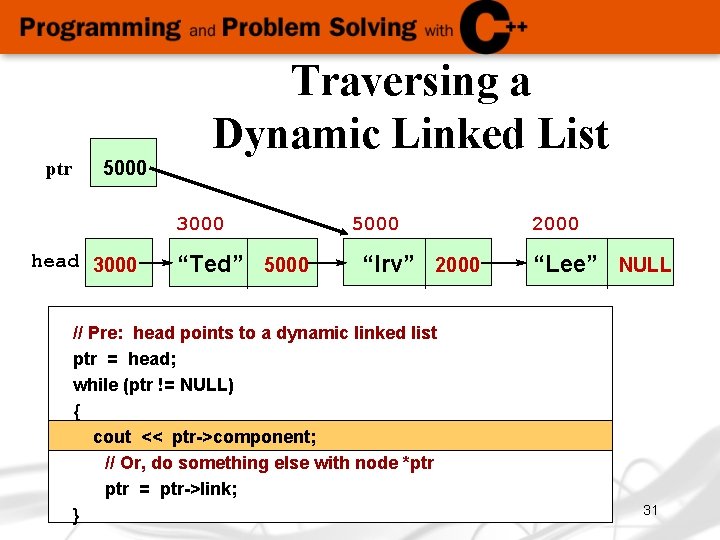
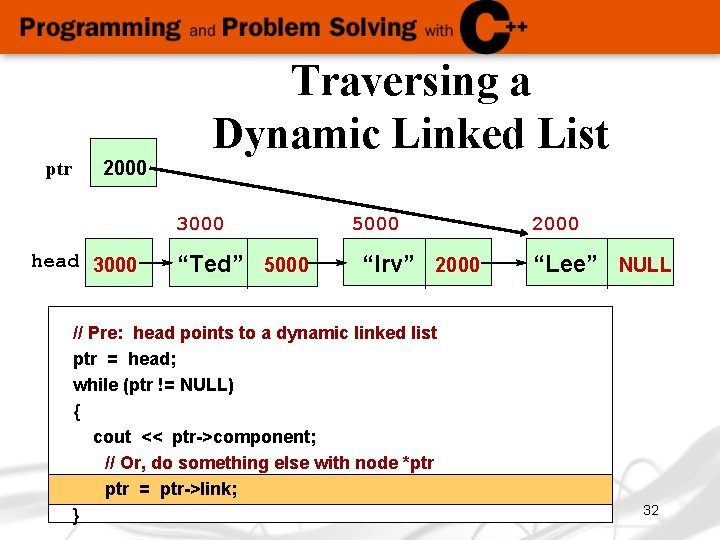
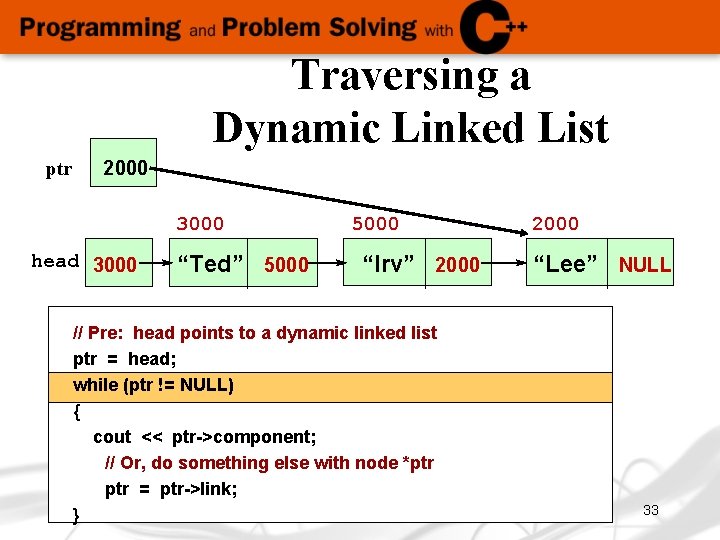
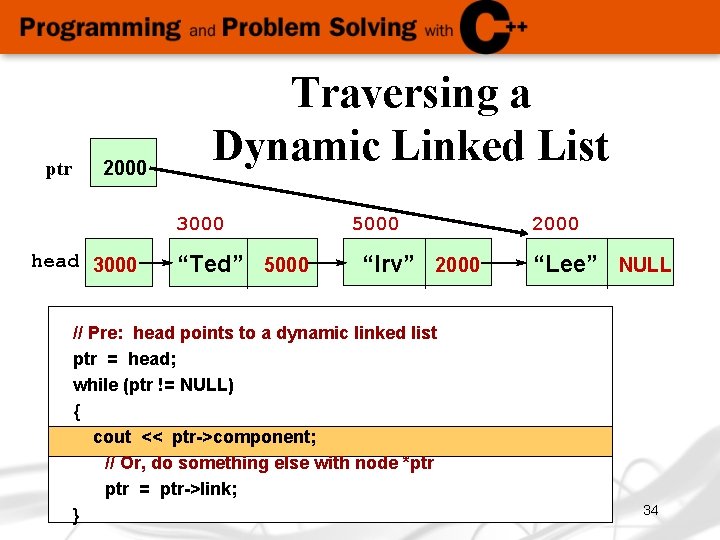
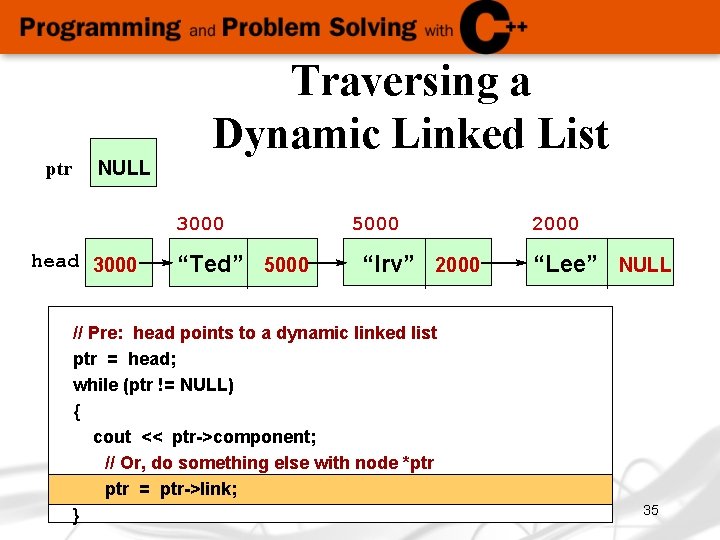
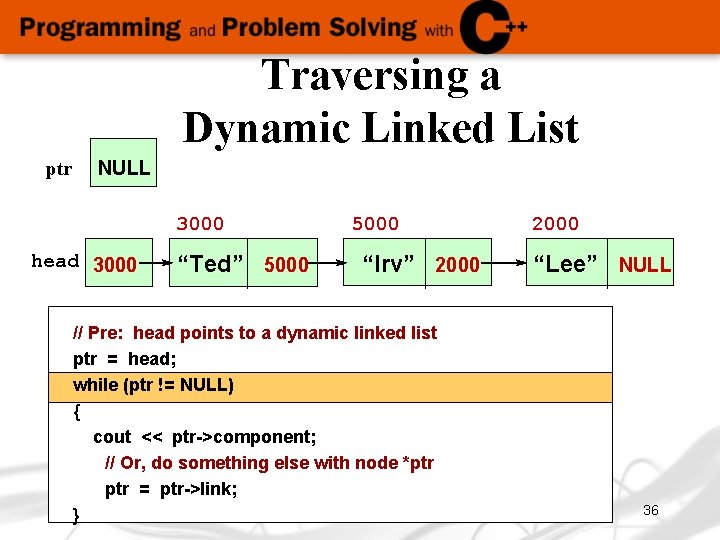
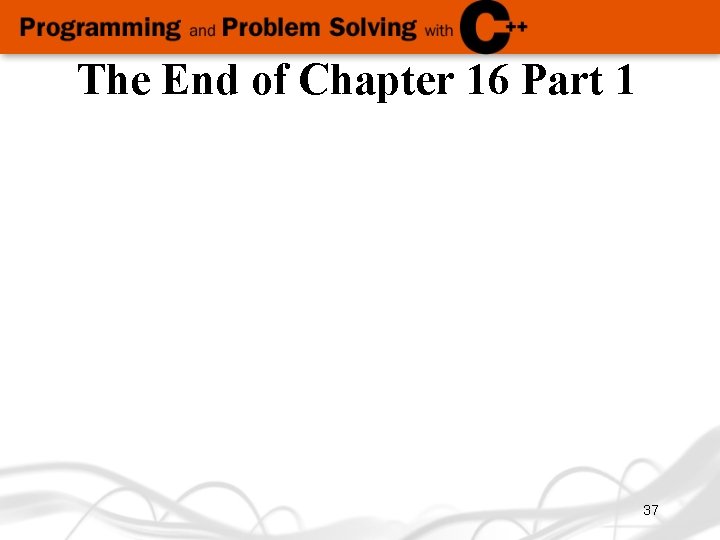
- Slides: 37
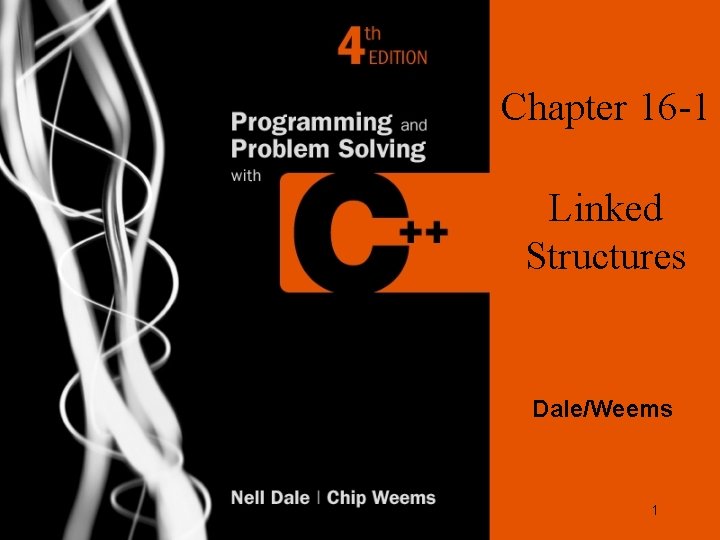
Chapter 16 -1 Linked Structures Dale/Weems 1
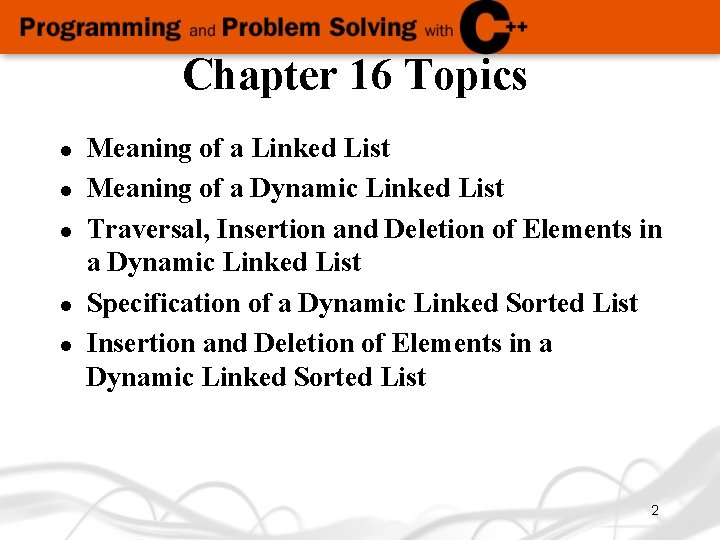
Chapter 16 Topics l l l Meaning of a Linked List Meaning of a Dynamic Linked List Traversal, Insertion and Deletion of Elements in a Dynamic Linked List Specification of a Dynamic Linked Sorted List Insertion and Deletion of Elements in a Dynamic Linked Sorted List 2
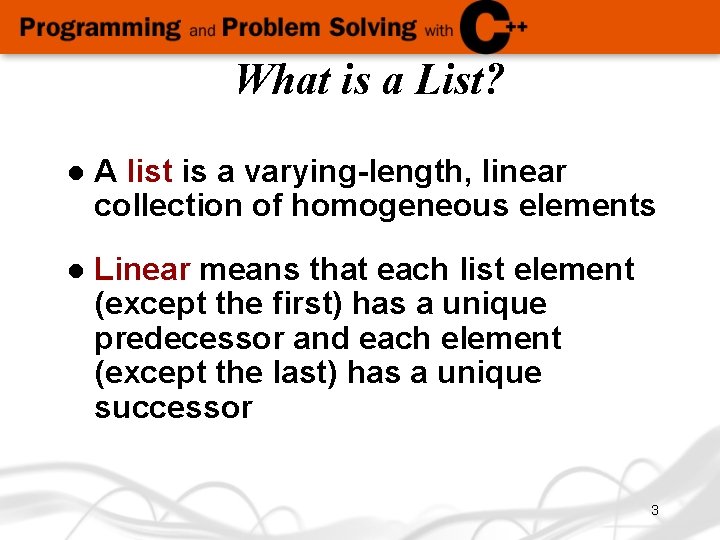
What is a List? l A list is a varying-length, linear collection of homogeneous elements l Linear means that each list element (except the first) has a unique predecessor and each element (except the last) has a unique successor 3
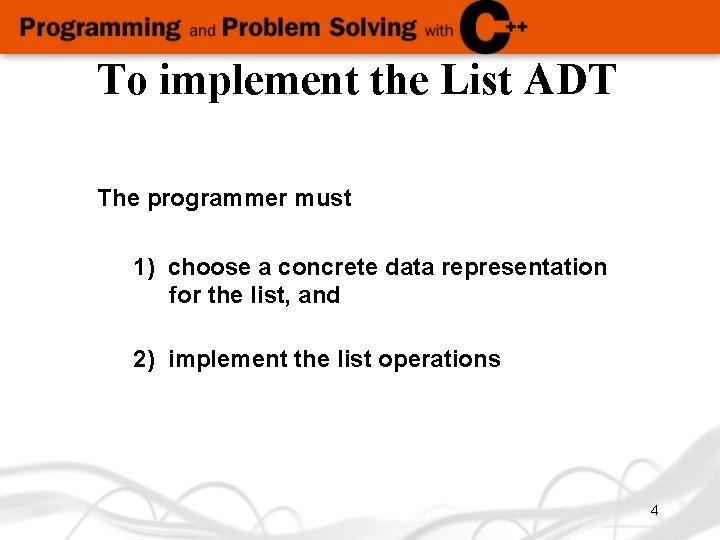
To implement the List ADT The programmer must 1) choose a concrete data representation for the list, and 2) implement the list operations 4
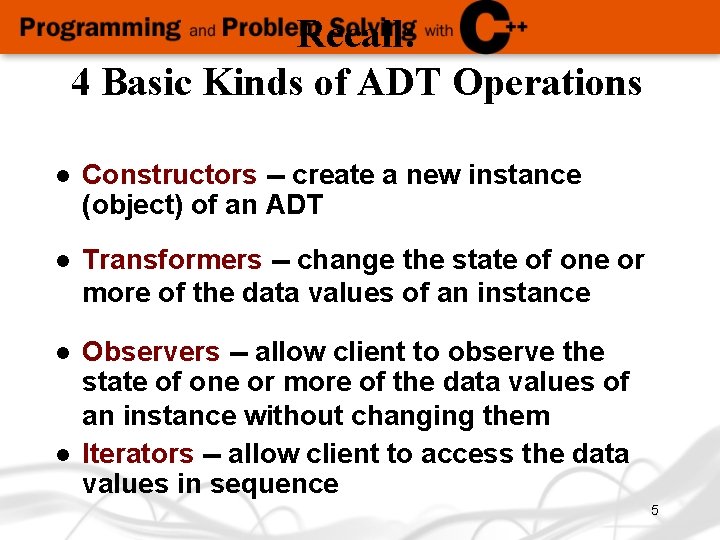
Recall: 4 Basic Kinds of ADT Operations l Constructors -- create a new instance (object) of an ADT l Transformers -- change the state of one or more of the data values of an instance l Observers -- allow client to observe the state of one or more of the data values of an instance without changing them Iterators -- allow client to access the data values in sequence l 5
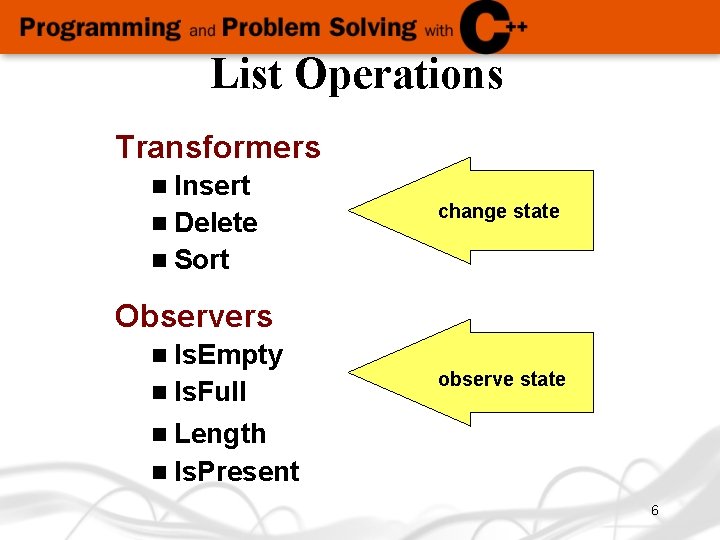
List Operations Transformers n Insert n Delete change state n Sort Observers n Is. Empty n Is. Full observe state n Length n Is. Present 6
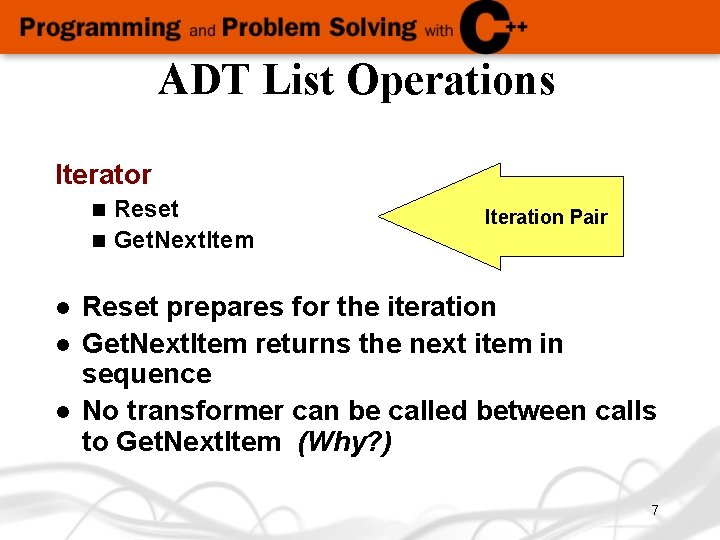
ADT List Operations Iterator Reset n Get. Next. Item n l l l Iteration Pair Reset prepares for the iteration Get. Next. Item returns the next item in sequence No transformer can be called between calls to Get. Next. Item (Why? ) 7
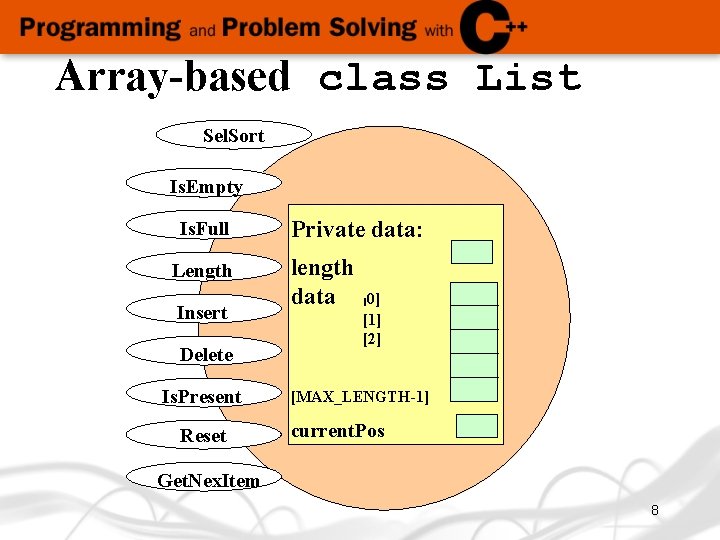
Array-based class List Sel. Sort Is. Empty Is. Full Length Insert Delete Is. Present Reset Private data: length data 0] [1] [2] [ [MAX_LENGTH-1] current. Pos Get. Nex. Item 8
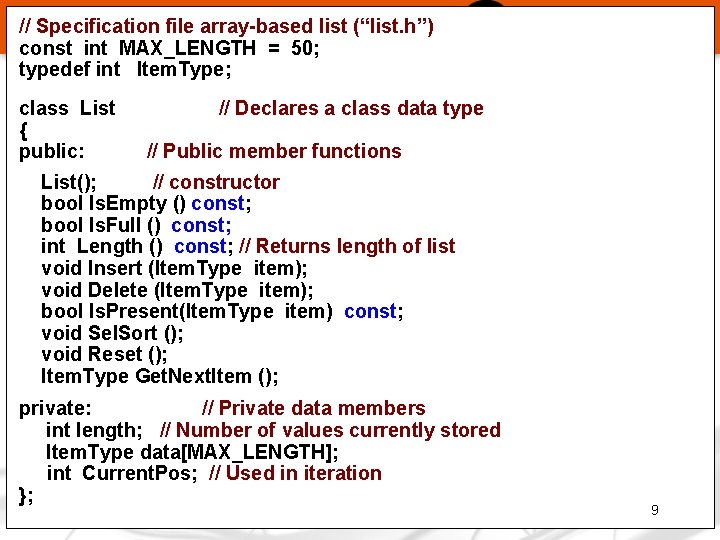
// Specification file array-based list (“list. h”) const int MAX_LENGTH = 50; typedef int Item. Type; class List { public: // Declares a class data type // Public member functions List(); // constructor bool Is. Empty () const; bool Is. Full () const; int Length () const; // Returns length of list void Insert (Item. Type item); void Delete (Item. Type item); bool Is. Present(Item. Type item) const; void Sel. Sort (); void Reset (); Item. Type Get. Next. Item (); private: // Private data members int length; // Number of values currently stored Item. Type data[MAX_LENGTH]; int Current. Pos; // Used in iteration }; 9
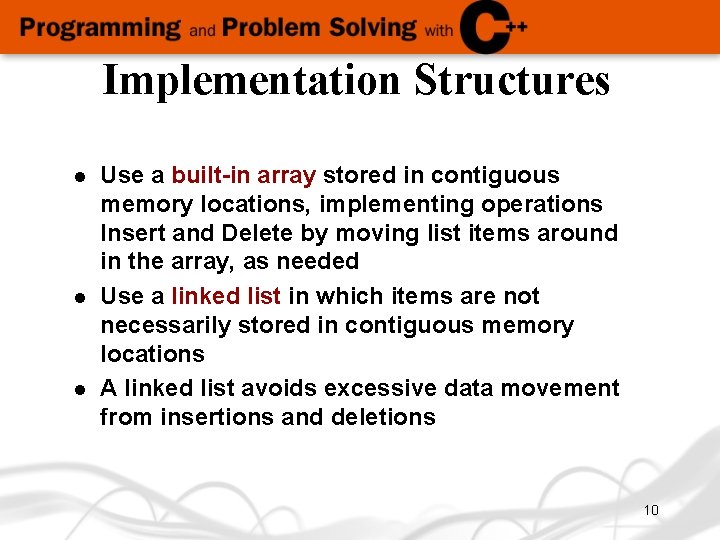
Implementation Structures l l l Use a built-in array stored in contiguous memory locations, implementing operations Insert and Delete by moving list items around in the array, as needed Use a linked list in which items are not necessarily stored in contiguous memory locations A linked list avoids excessive data movement from insertions and deletions 10
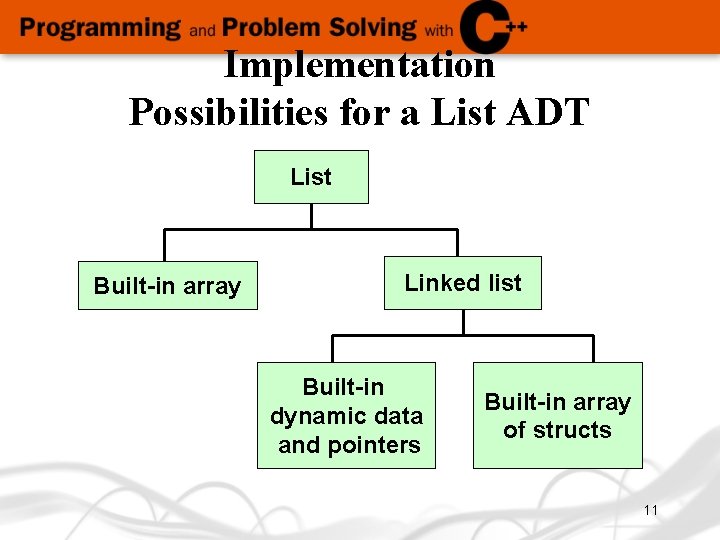
Implementation Possibilities for a List ADT List Built-in array Linked list Built-in dynamic data and pointers Built-in array of structs 11
![Array Representation of a Linked List component link head 2 Node0 58 1 Node1 Array Representation of a Linked List component link head 2 Node[0] 58 -1 Node[1]](https://slidetodoc.com/presentation_image_h2/6dbb982debd59de2651351a48091c898/image-12.jpg)
Array Representation of a Linked List component link head 2 Node[0] 58 -1 Node[1] Node[2] 4 Node[3] Node[4] 46 Node[5] 16 5 0 7 Node[6] Node[7] 39 4 12
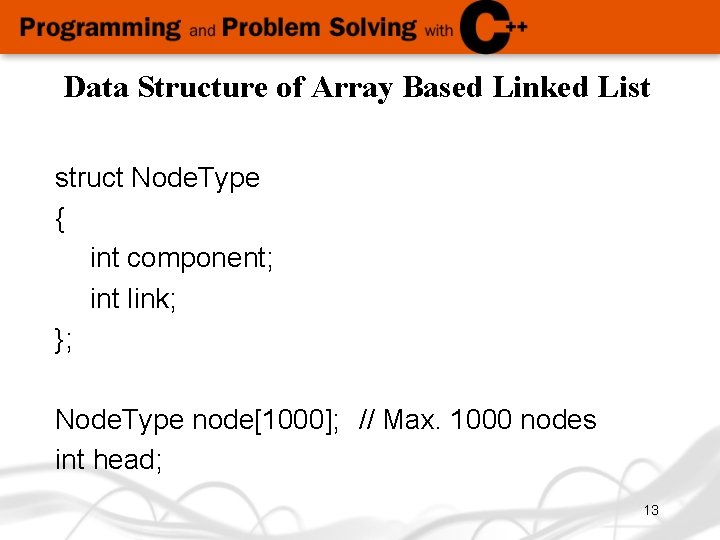
Data Structure of Array Based Linked List struct Node. Type { int component; int link; }; Node. Type node[1000]; // Max. 1000 nodes int head; 13
![Insert a New Node into a Linked List component link head Node0 58 2 Insert a New Node into a Linked List component link head Node[0] 58 2](https://slidetodoc.com/presentation_image_h2/6dbb982debd59de2651351a48091c898/image-14.jpg)
Insert a New Node into a Linked List component link head Node[0] 58 2 Node[1] 25 Node[2] 4 Node[3] Node[4] 46 Node[5] 16 -1 7 5 0 1 Node[6] Node[7] 39 Insert 25, Setting link to 7 Change link from 7 to 1 4 14
![Delete a Node from a Linked List component link head Node0 58 2 Node1 Delete a Node from a Linked List component link head Node[0] 58 2 Node[1]](https://slidetodoc.com/presentation_image_h2/6dbb982debd59de2651351a48091c898/image-15.jpg)
Delete a Node from a Linked List component link head Node[0] 58 2 Node[1] 25 Node[2] 4 Node[3] Node[4] 46 Node[5] 16 -1 7 5 0 1 Deleted 0 Change the link From 4 to 15 0 Node[6] Node[7] 39
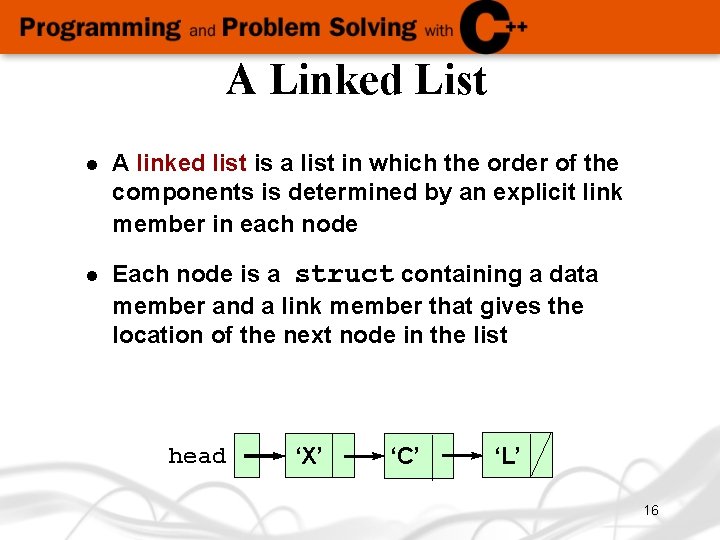
A Linked List l A linked list is a list in which the order of the components is determined by an explicit link member in each node l Each node is a struct containing a data member and a link member that gives the location of the next node in the list head ‘X’ ‘C’ ‘L’ 16
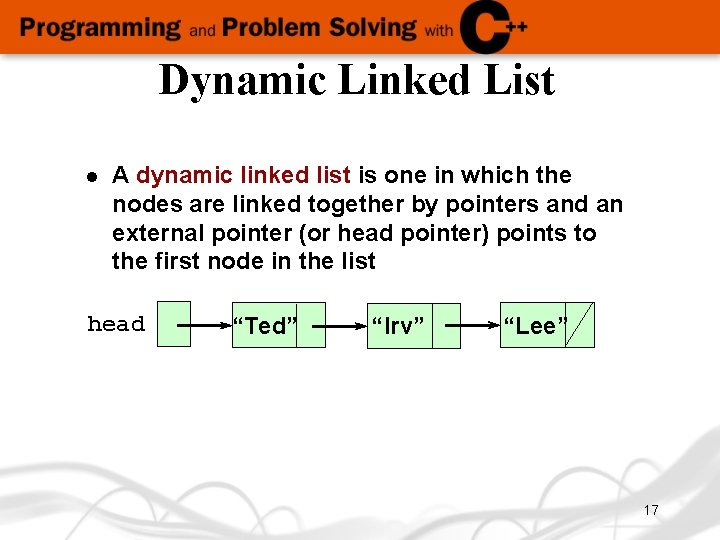
Dynamic Linked List l A dynamic linked list is one in which the nodes are linked together by pointers and an external pointer (or head pointer) points to the first node in the list head “Ted” “Irv” “Lee” 17
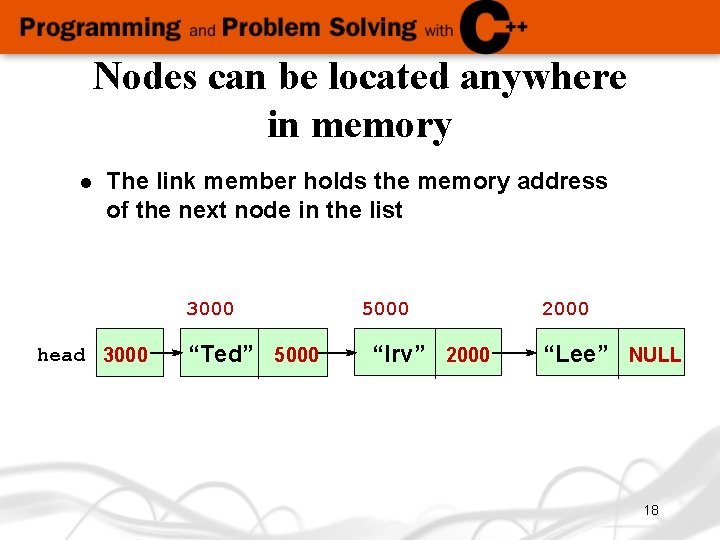
Nodes can be located anywhere in memory l The link member holds the memory address of the next node in the list 3000 head 3000 “Ted” 5000 “Irv” 2000 “Lee” NULL 18
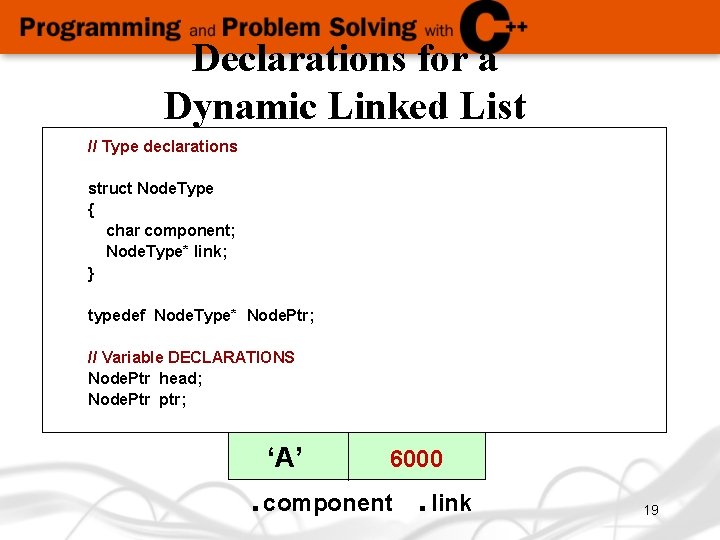
Declarations for a Dynamic Linked List // Type declarations struct Node. Type { char component; Node. Type* link; } typedef Node. Type* Node. Ptr; // Variable DECLARATIONS Node. Ptr head; Node. Ptr ptr; ‘A’ 6000 . component . link 19
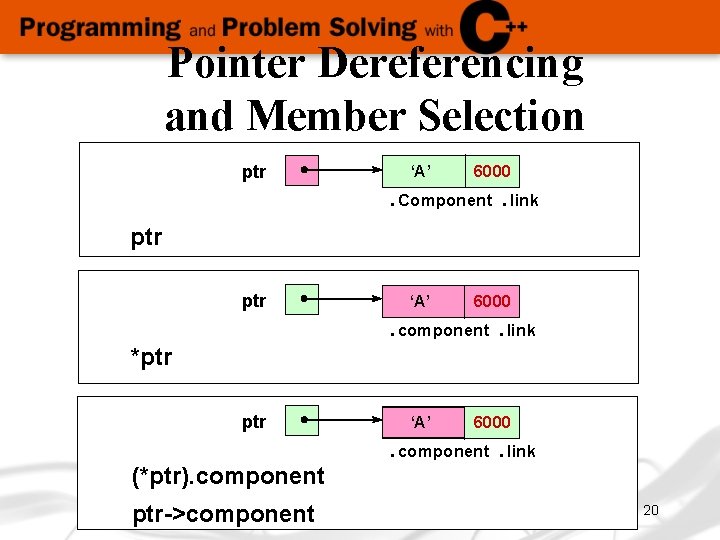
Pointer Dereferencing and Member Selection ptr ‘A’ 6000 . Component. link ptr ‘A’ 6000 . component. link *ptr (*ptr). component ptr->component ‘A’ 6000 . component. link 20
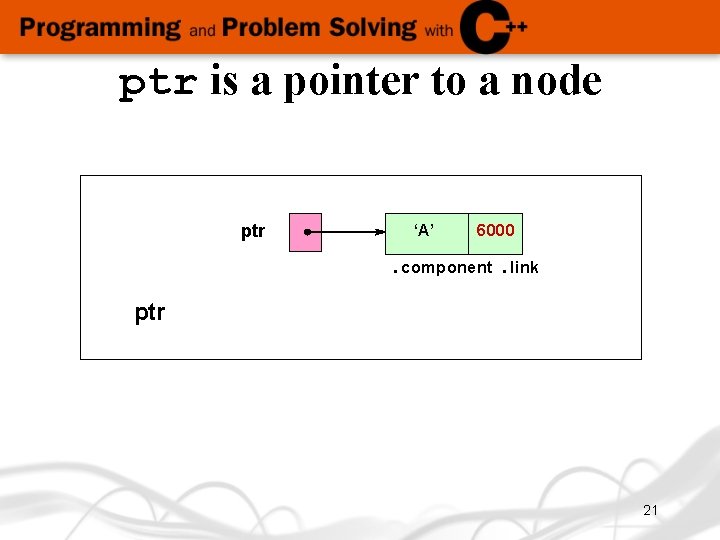
ptr is a pointer to a node ptr ‘A’ 6000 . component. link ptr 21
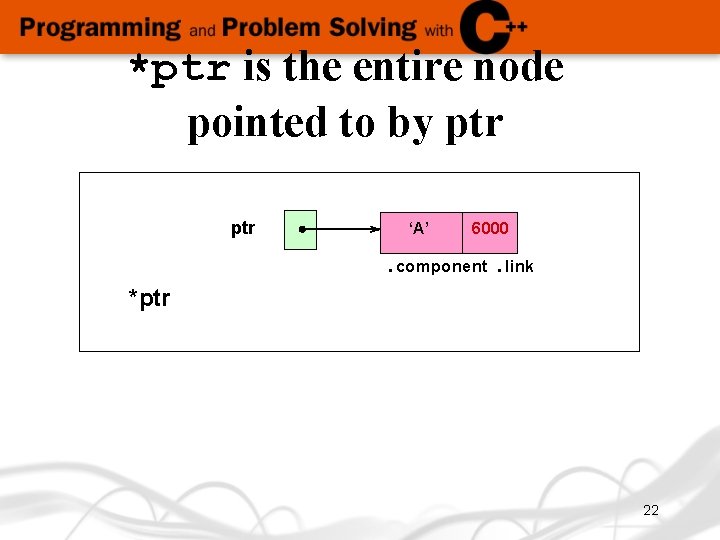
*ptr is the entire node pointed to by ptr ‘A’ 6000 . component. link *ptr 22
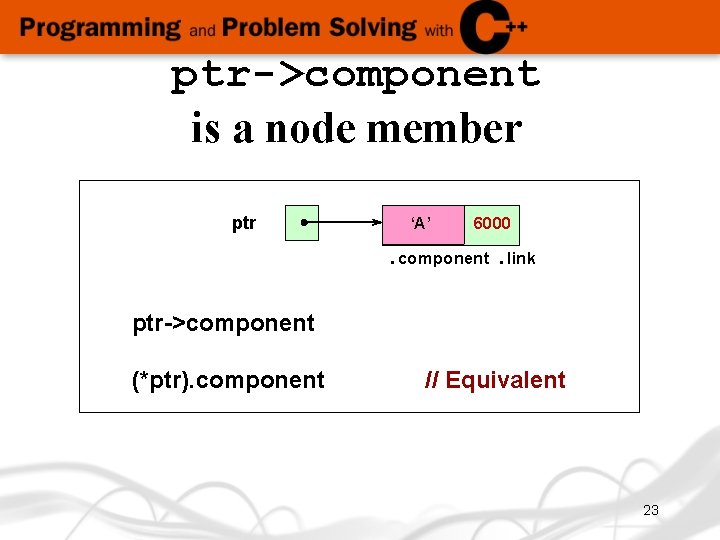
ptr->component is a node member ptr ‘A’ 6000 . component. link ptr->component (*ptr). component // Equivalent 23
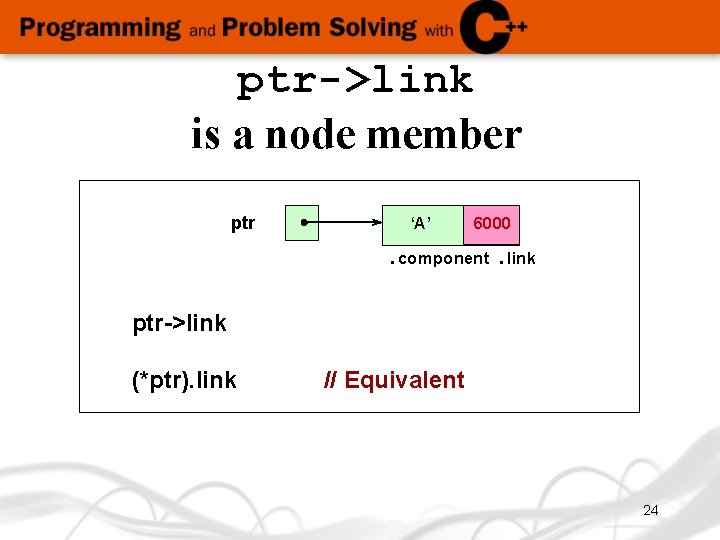
ptr->link is a node member ptr ‘A’ 6000 . component. link ptr->link (*ptr). link // Equivalent 24
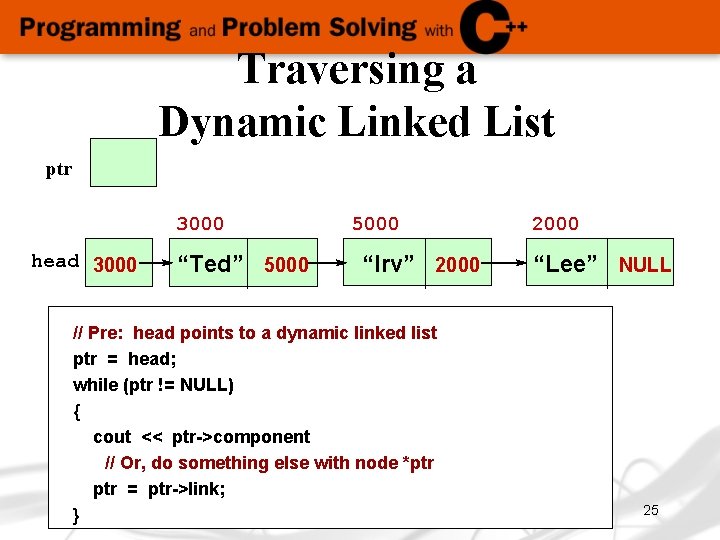
Traversing a Dynamic Linked List ptr 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 25
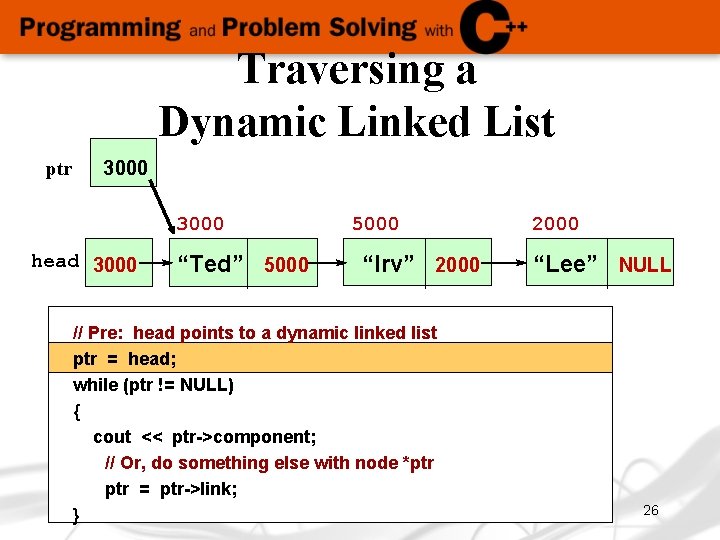
Traversing a Dynamic Linked List ptr 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 26
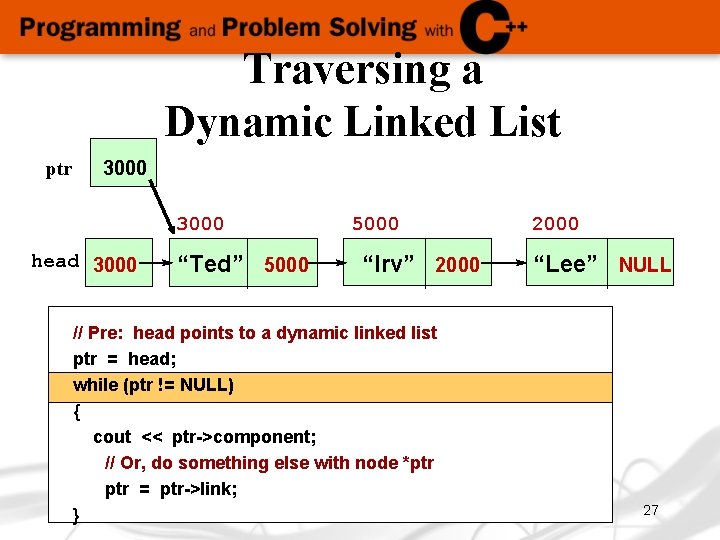
Traversing a Dynamic Linked List ptr 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 27
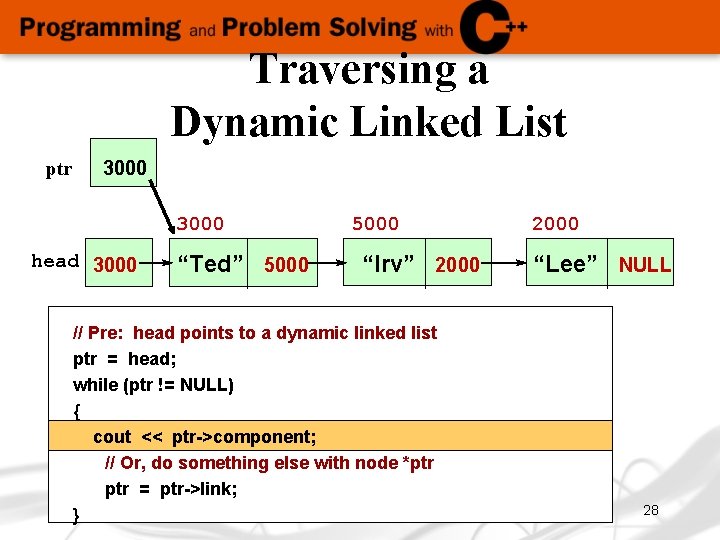
Traversing a Dynamic Linked List ptr 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 28
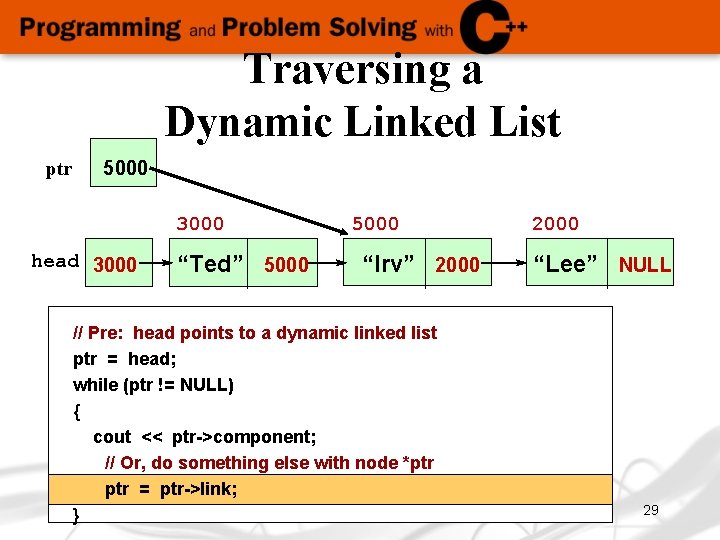
Traversing a Dynamic Linked List ptr 5000 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 29
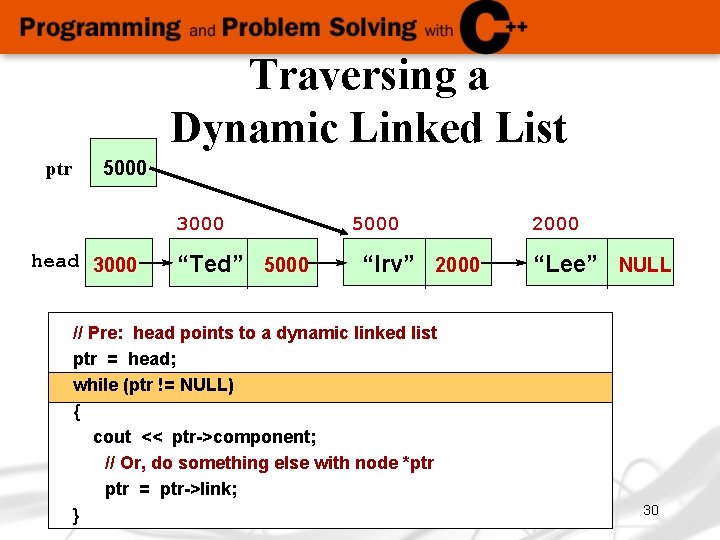
Traversing a Dynamic Linked List ptr 5000 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 30
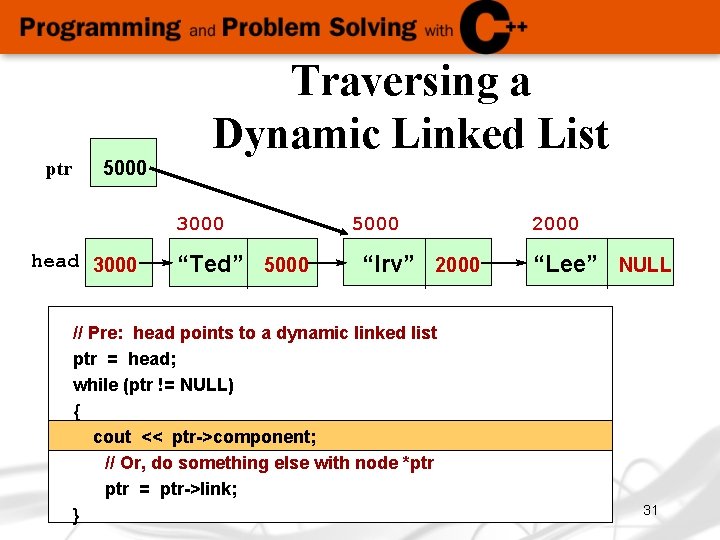
ptr 5000 Traversing a Dynamic Linked List 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 31
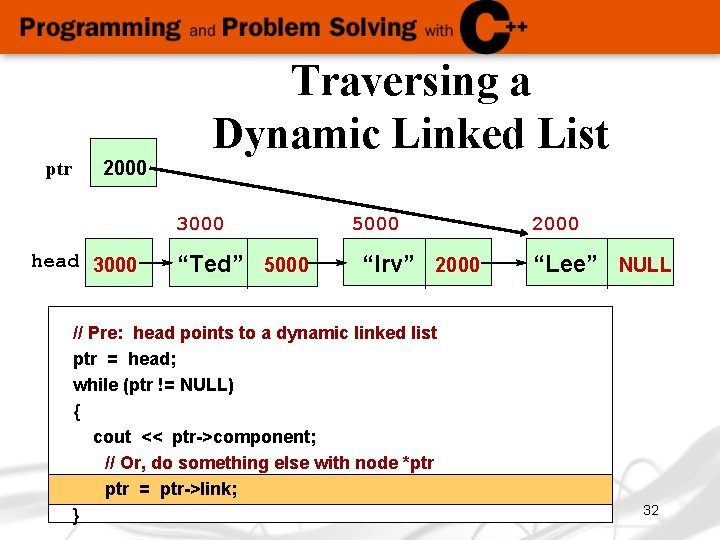
ptr 2000 Traversing a Dynamic Linked List 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 32
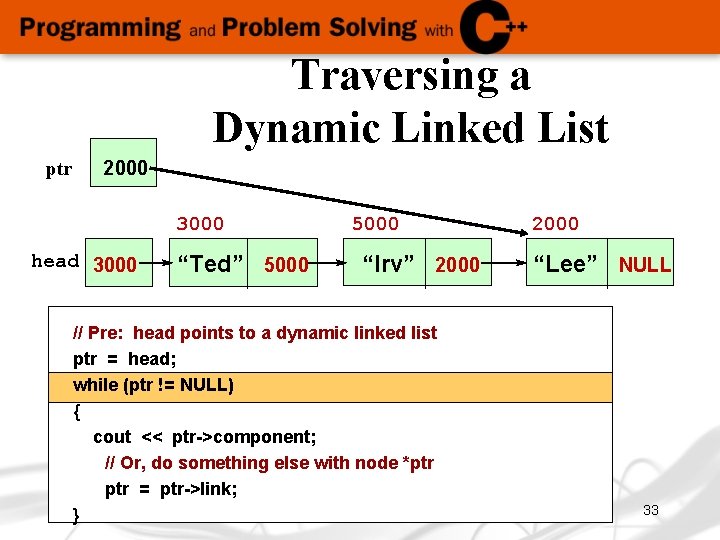
Traversing a Dynamic Linked List ptr 2000 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 33
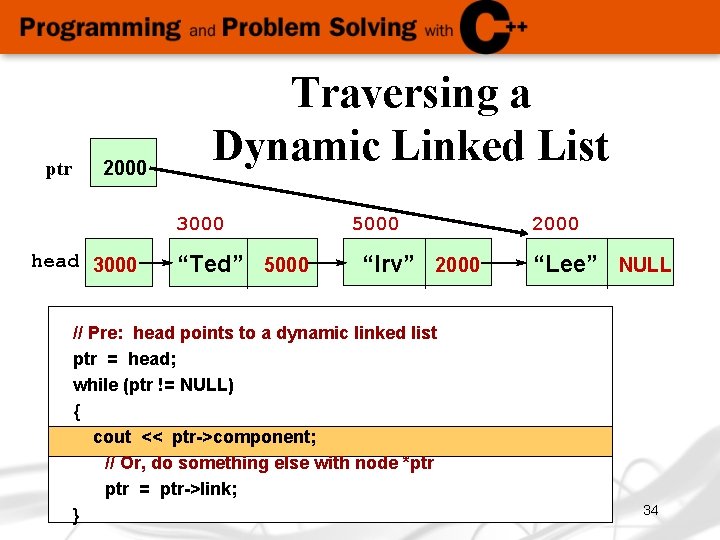
ptr 2000 Traversing a Dynamic Linked List 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 34
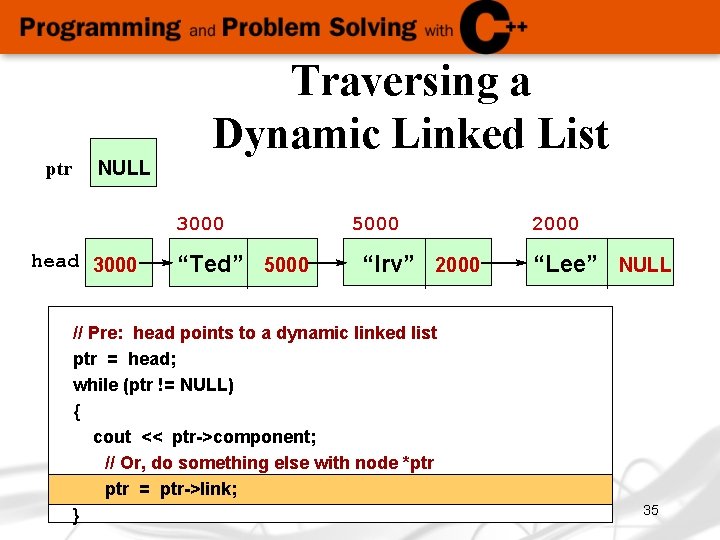
ptr NULL Traversing a Dynamic Linked List 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 35
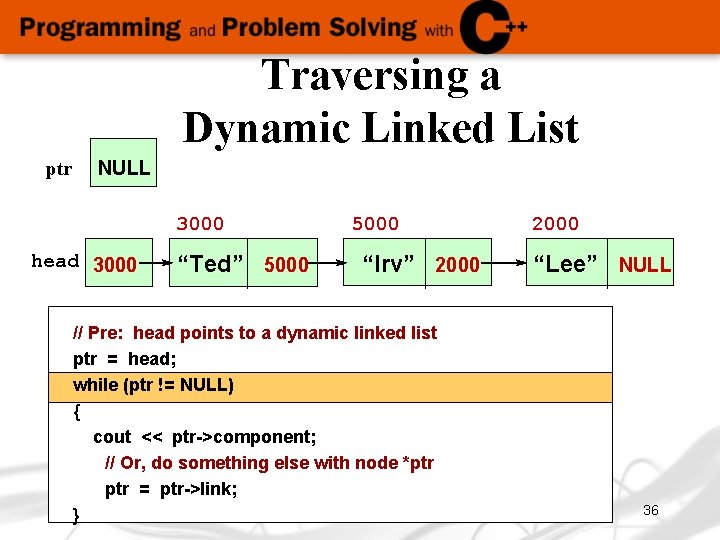
Traversing a Dynamic Linked List ptr NULL 3000 head 3000 “Ted” 5000 “Irv” 2000 // Pre: head points to a dynamic linked list ptr = head; while (ptr != NULL) { cout << ptr->component; // Or, do something else with node *ptr = ptr->link; } 2000 “Lee” NULL 36
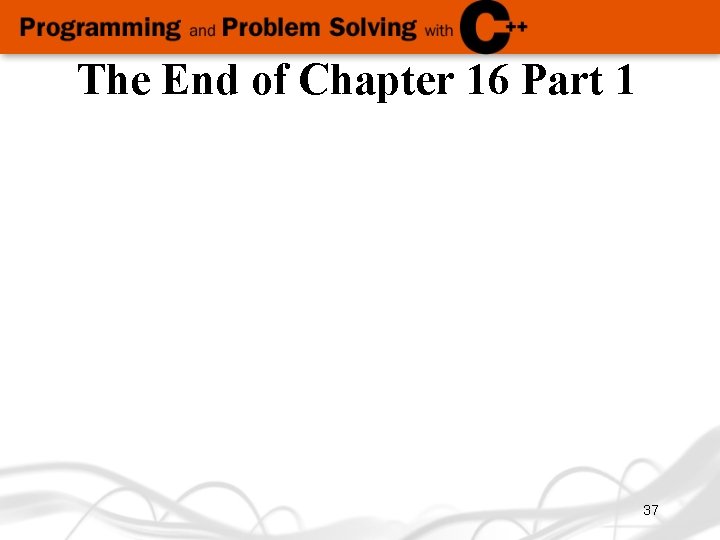
The End of Chapter 16 Part 1 37
In linked list the successive elements
Singly vs doubly linked list
Apa itu single linked list
Function of homologous structure
Chapter 22 genetics and genetically linked diseases
Chapter 7 organizational structures
Monopolistic competition example
Chapter 7 section 3 structures and organelles
Knee anatomy chapter 16 worksheet 1
Chapter 16 worksheet the knee and related structures
Chapter 7 organizational structures
Data structures chapter 1
Chapter 7 market structures vocabulary
Market structure dominated by a few large profitable firms
Section 1 cell discovery and theory
Xlinked dominant
Whats sex linked
Sex linked pedigree
Generic linked list java
Jenis linked list
Singly vs doubly linked list
X linked punnett square
What are sexlinked traits
Sexlinked traits
Sex linked disease punnett square
X linked diseases
Sex-linked traits examples
Linkage
Sex limited traits example
Blood type punnett square
Punnett square blood type
Polynomial division using linked list in c
Book bands linked to phonic phases
Hemophilia punnett square worksheet
Family pedigree symbols
Dominant pedigree
Autosomal vs sex linked
Sex chromosome