Chapter 16 2 Linked Structures DaleWeems 1 Using
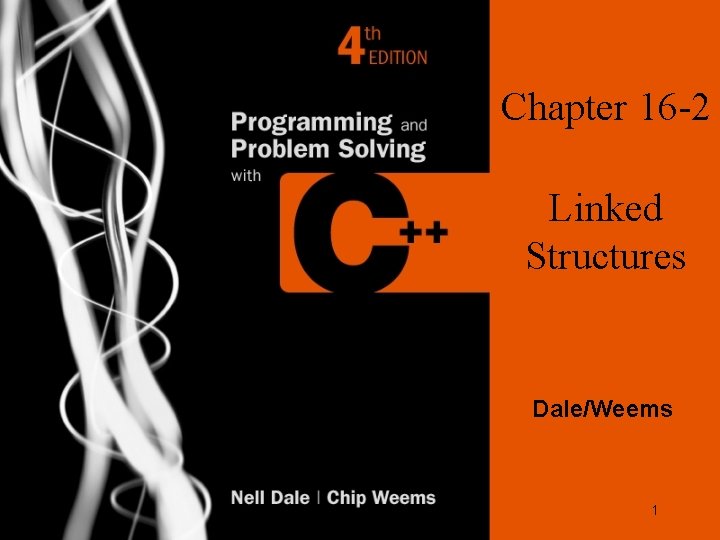
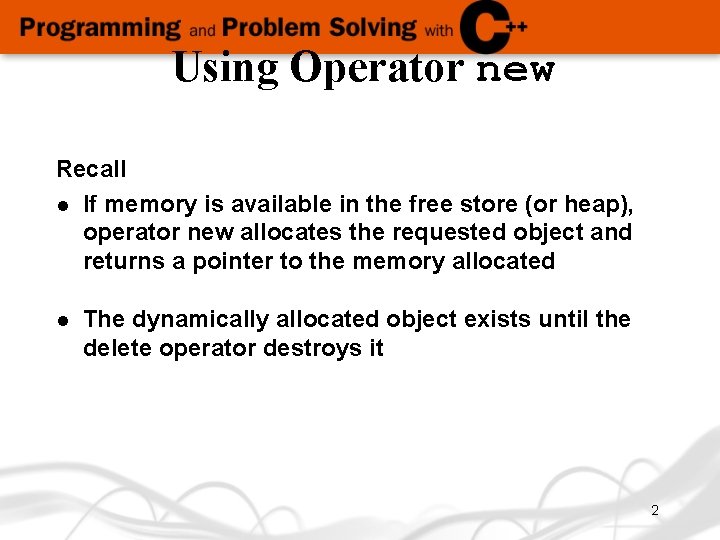
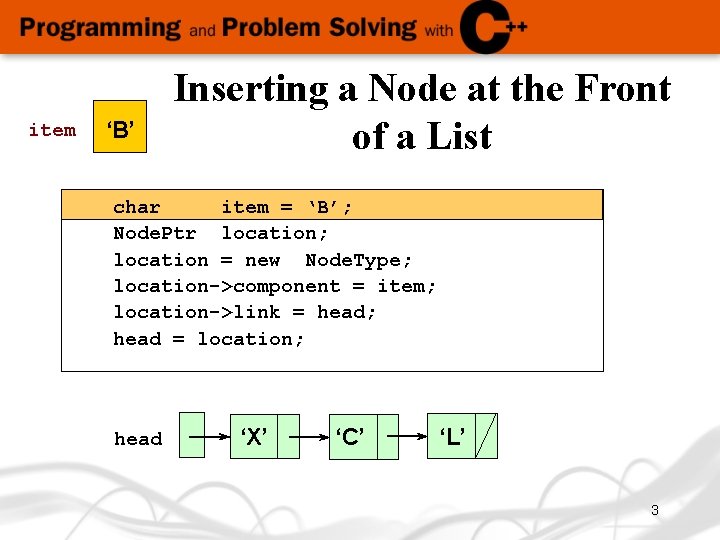
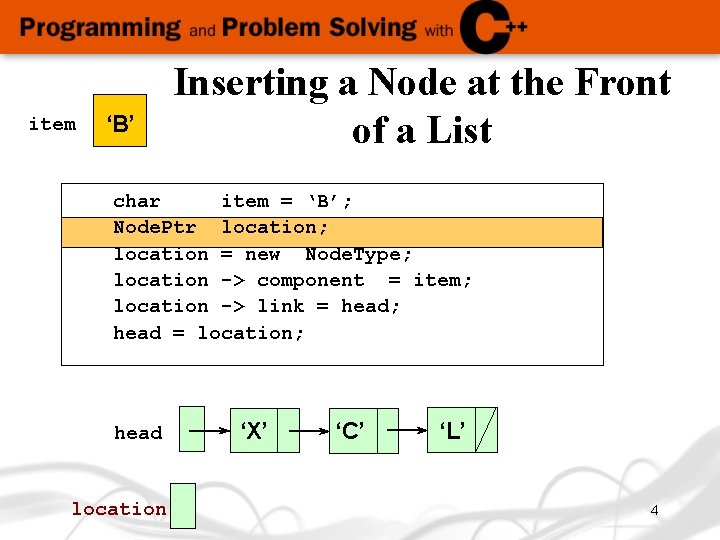
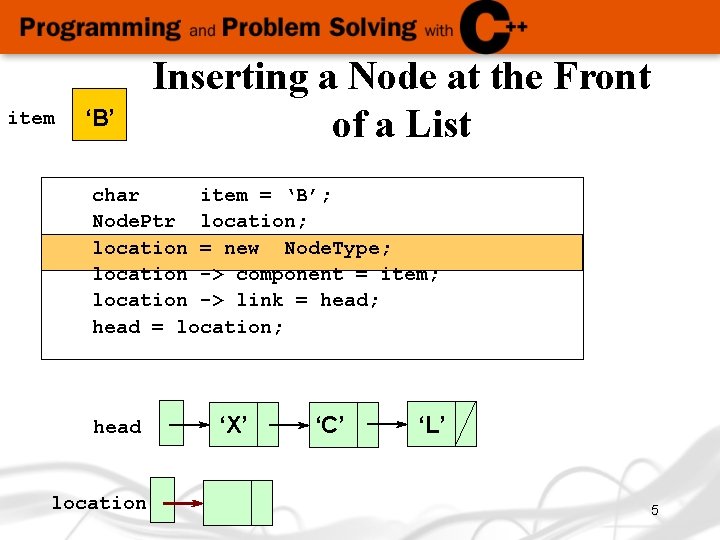
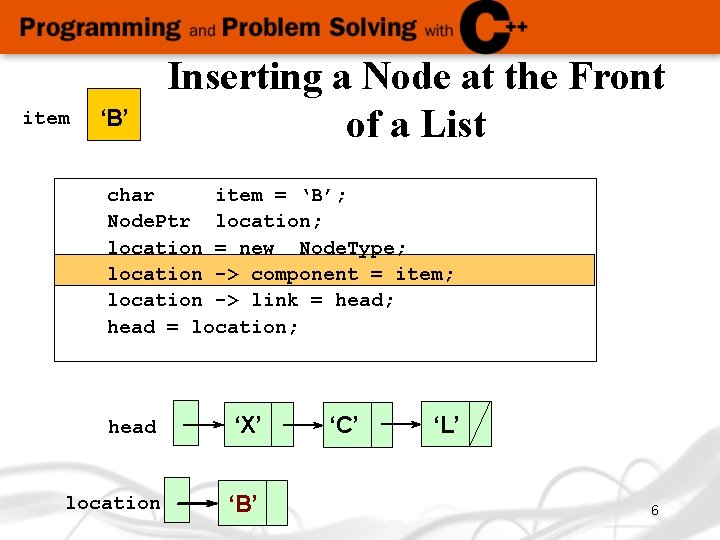
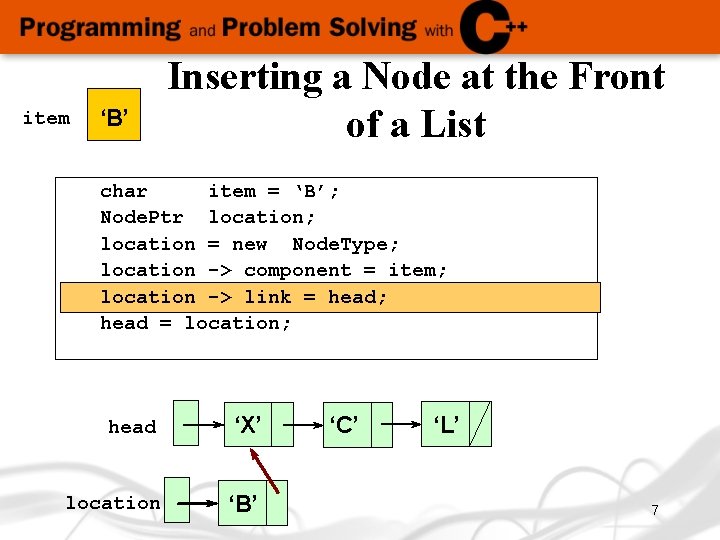
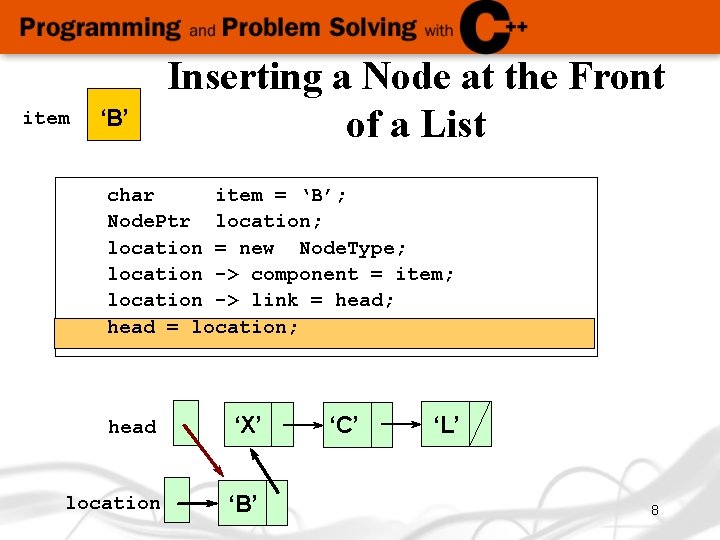
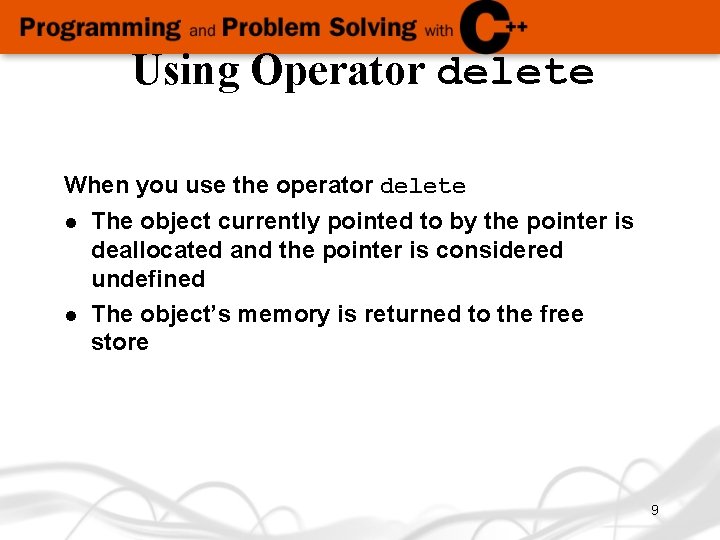
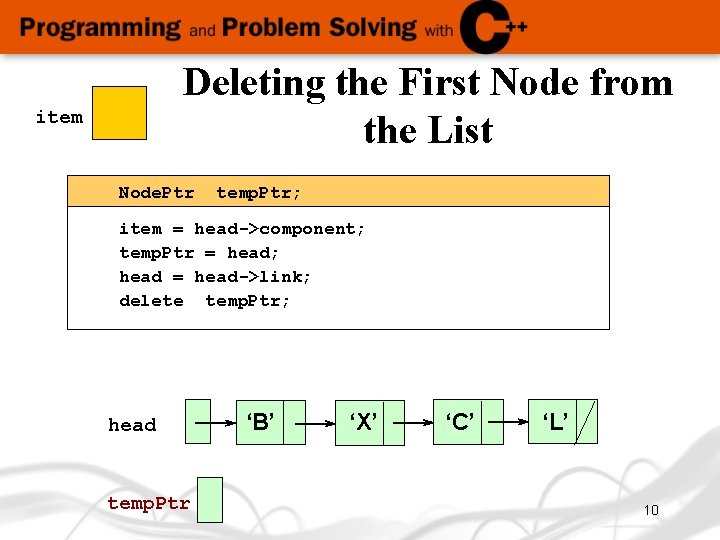
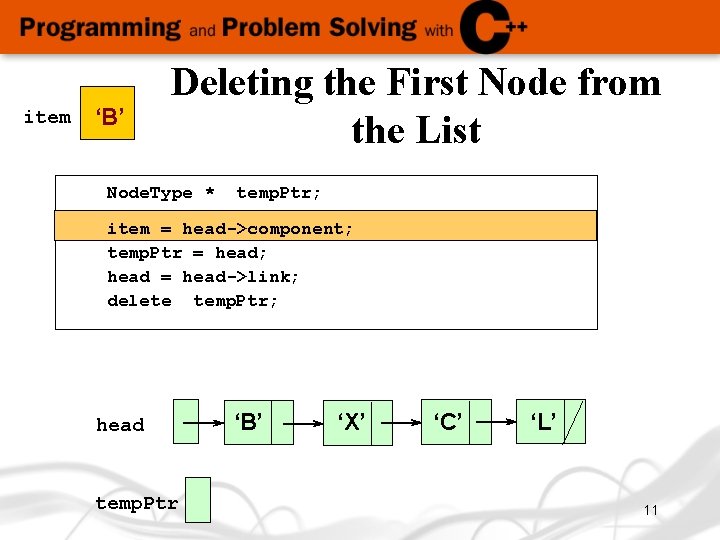
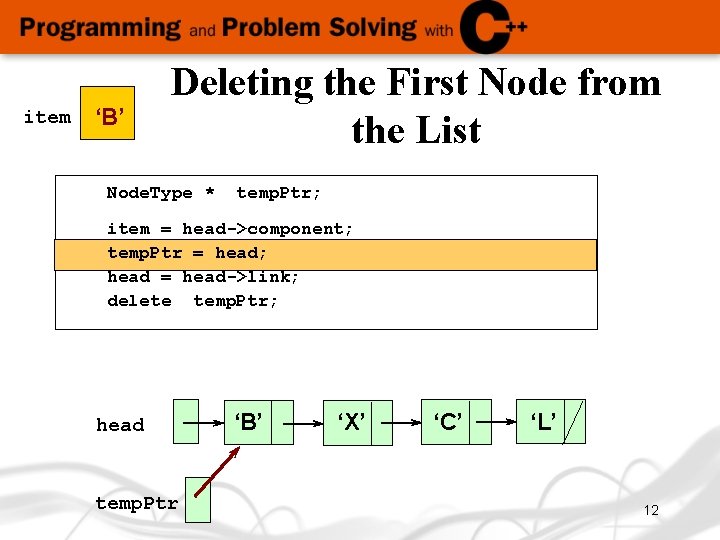
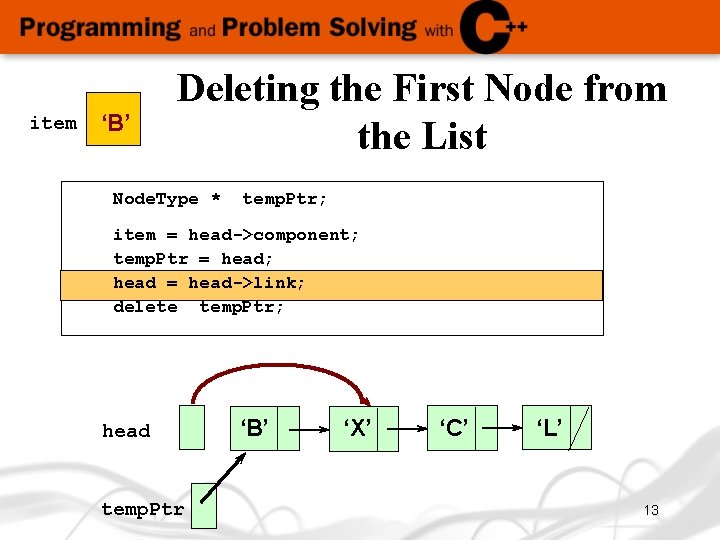
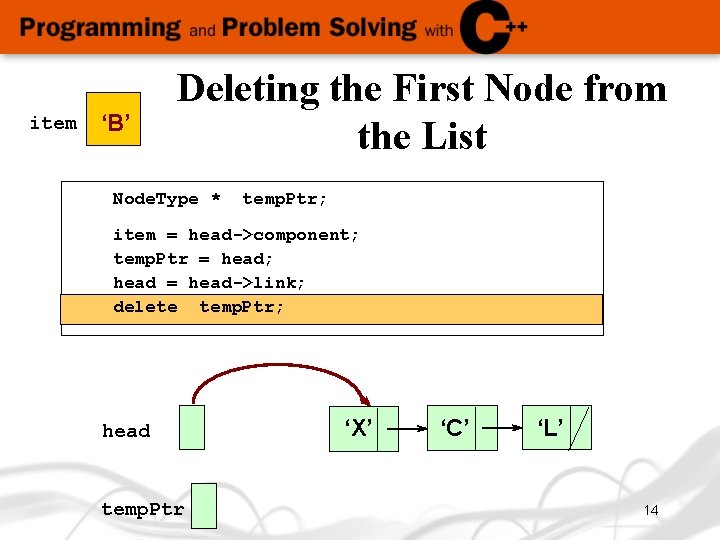
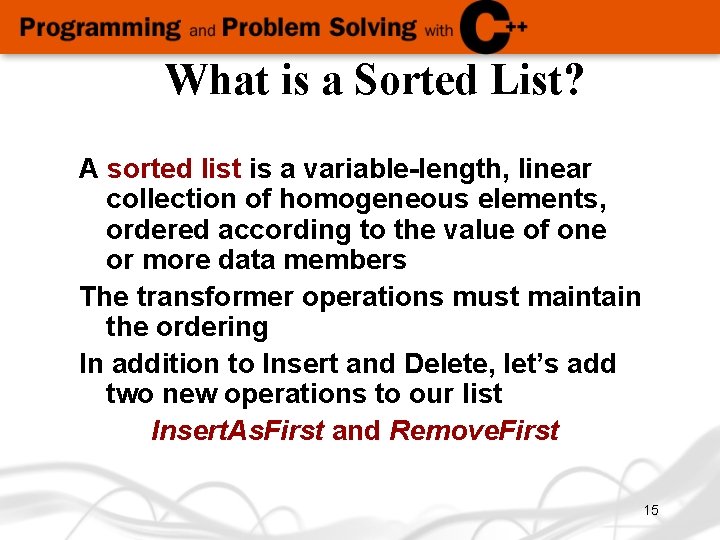
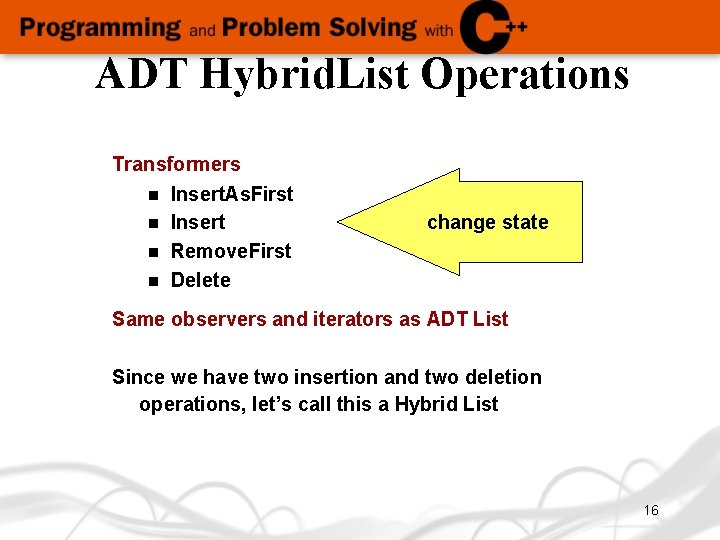
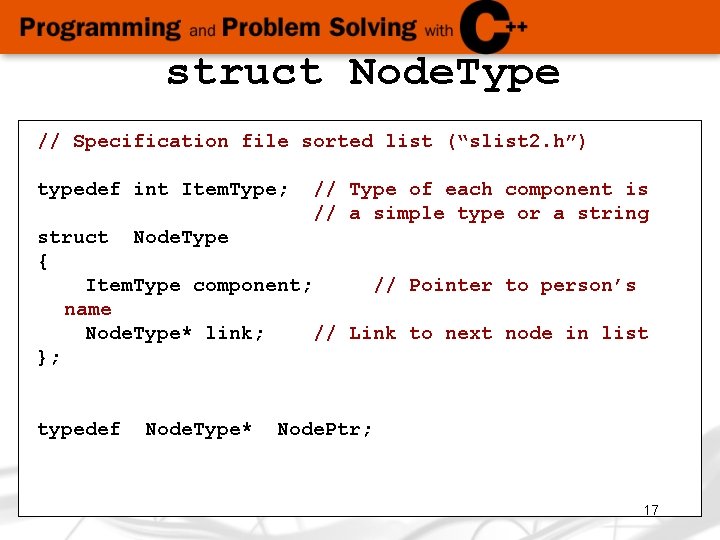
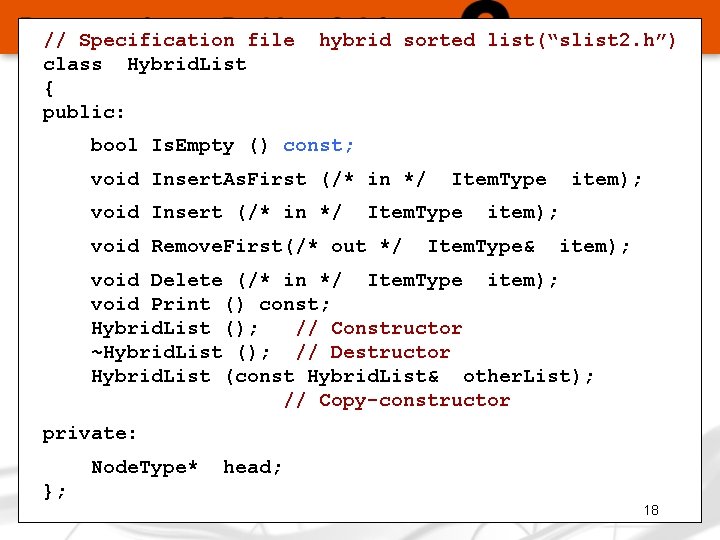
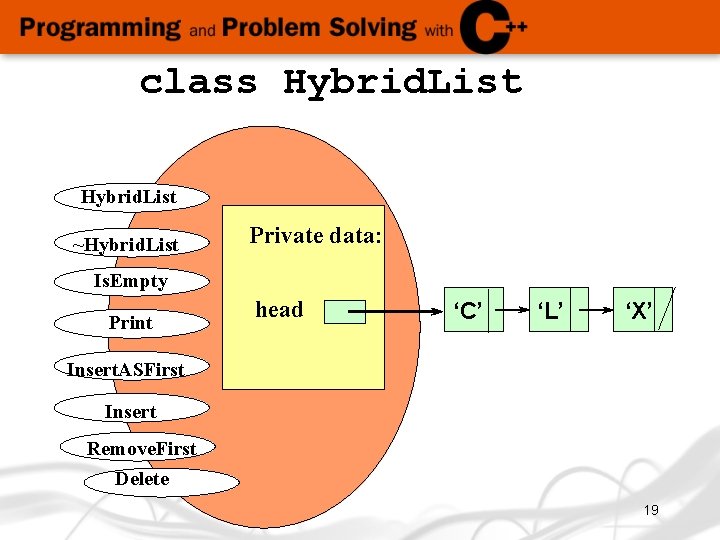
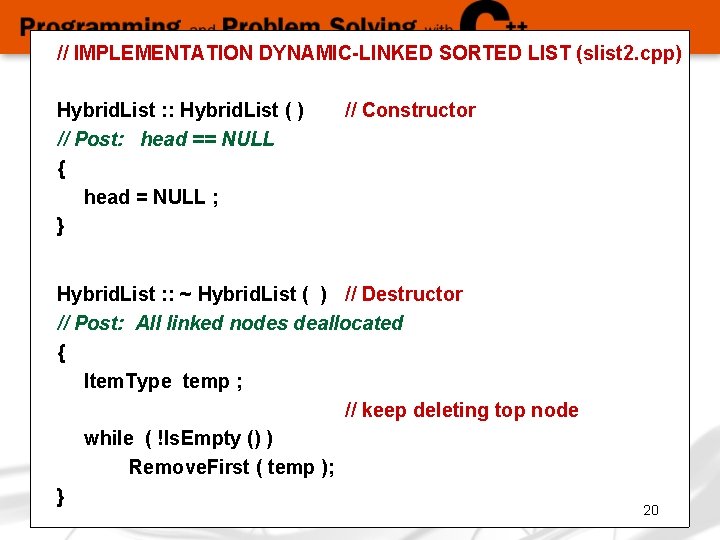
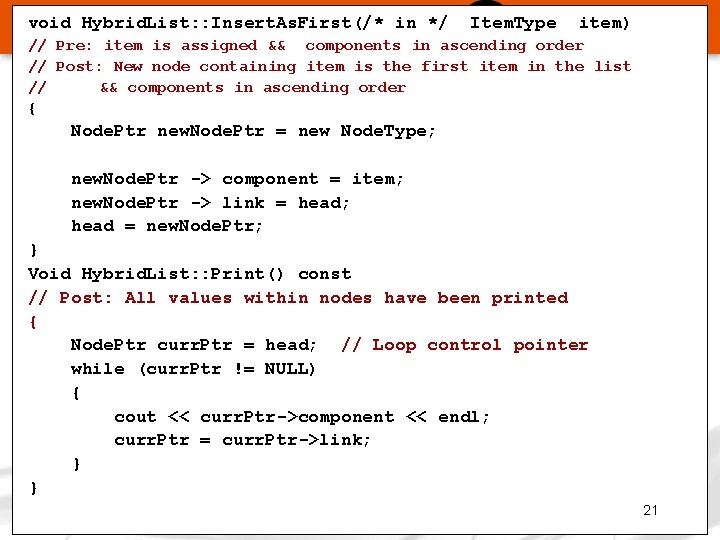
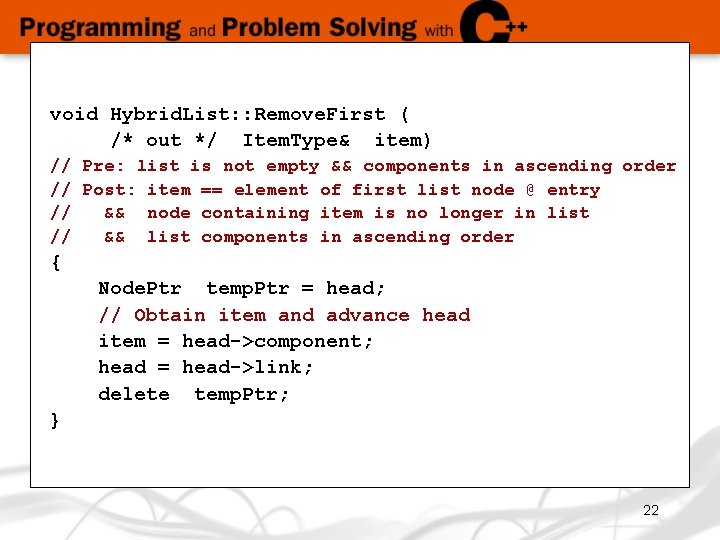
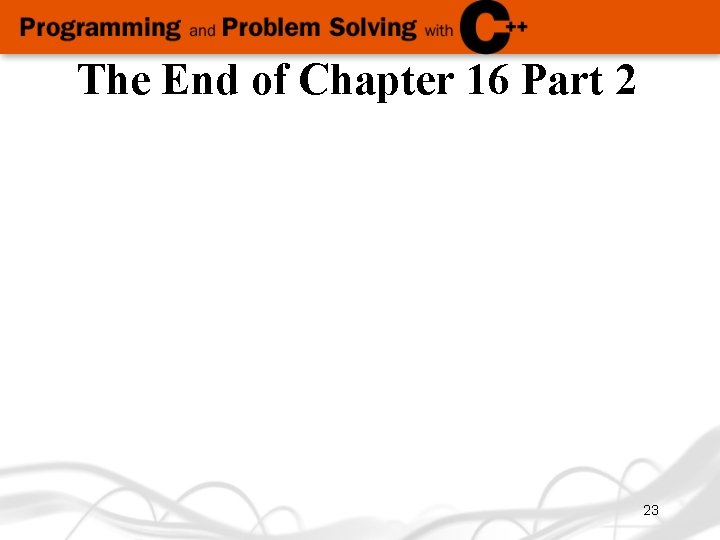
- Slides: 23
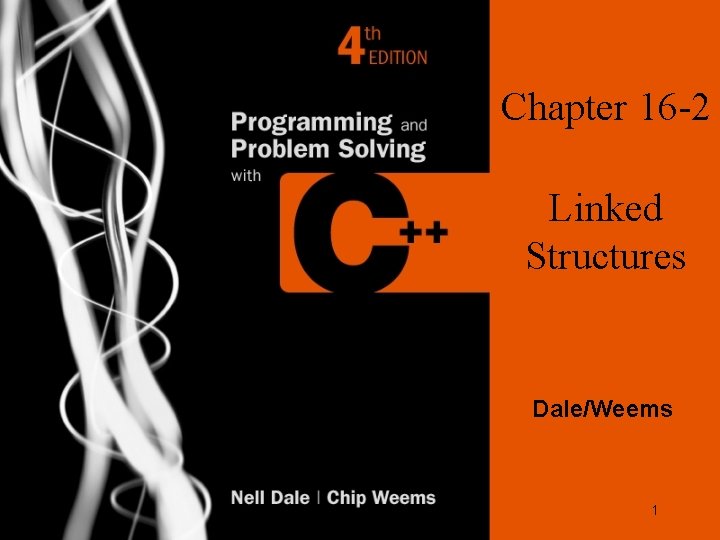
Chapter 16 -2 Linked Structures Dale/Weems 1
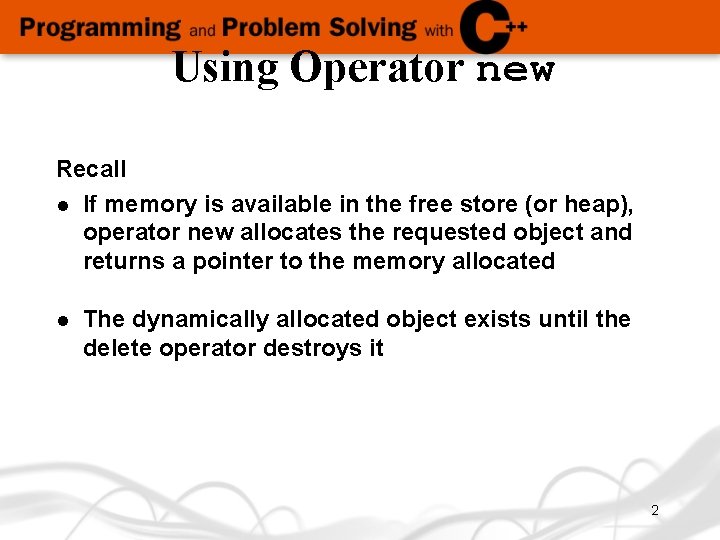
Using Operator new Recall l If memory is available in the free store (or heap), operator new allocates the requested object and returns a pointer to the memory allocated l The dynamically allocated object exists until the delete operator destroys it 2
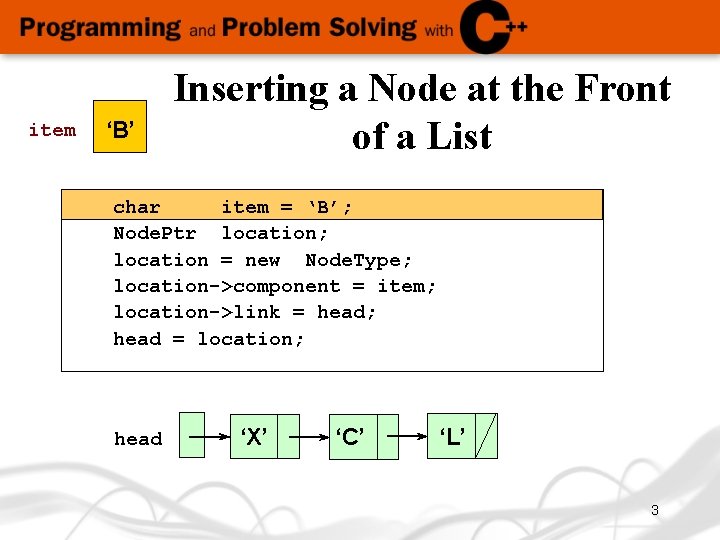
item ‘B’ Inserting a Node at the Front of a List char item = ‘B’; Node. Ptr location; location = new Node. Type; location->component = item; location->link = head; head = location; head ‘X’ ‘C’ ‘L’ 3
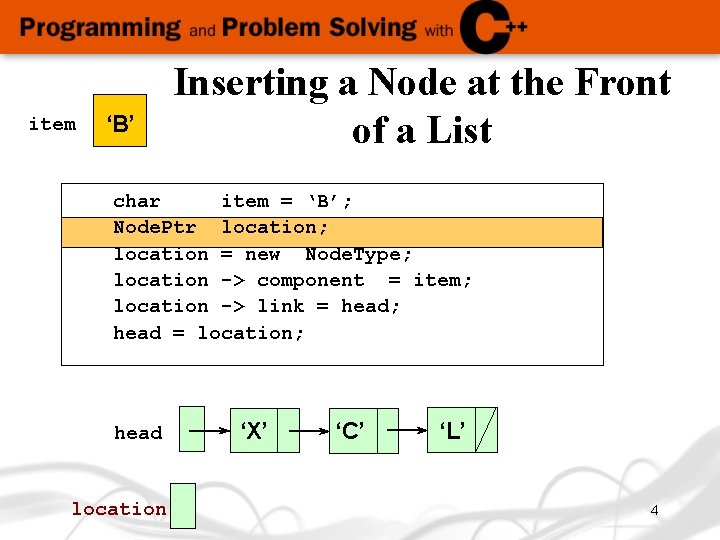
item ‘B’ Inserting a Node at the Front of a List char item = ‘B’; Node. Ptr location; location = new Node. Type; location -> component = item; location -> link = head; head = location; head location ‘X’ ‘C’ ‘L’ 4
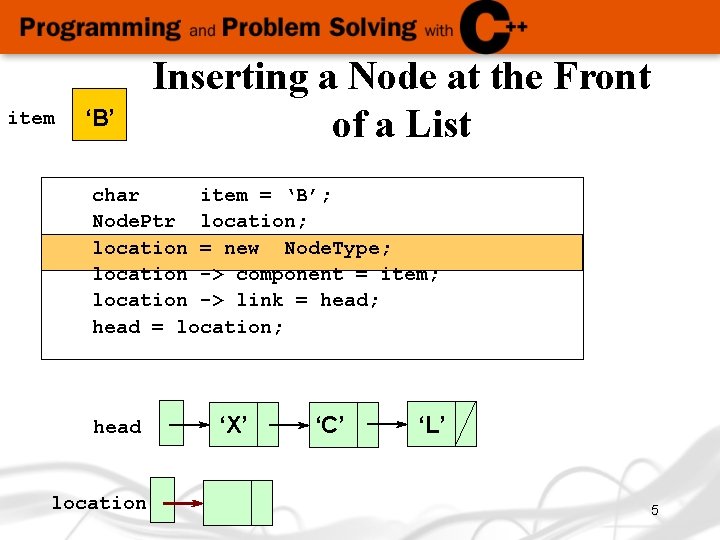
item ‘B’ Inserting a Node at the Front of a List char item = ‘B’; Node. Ptr location; location = new Node. Type; location -> component = item; location -> link = head; head = location; head location ‘X’ ‘C’ ‘L’ 5
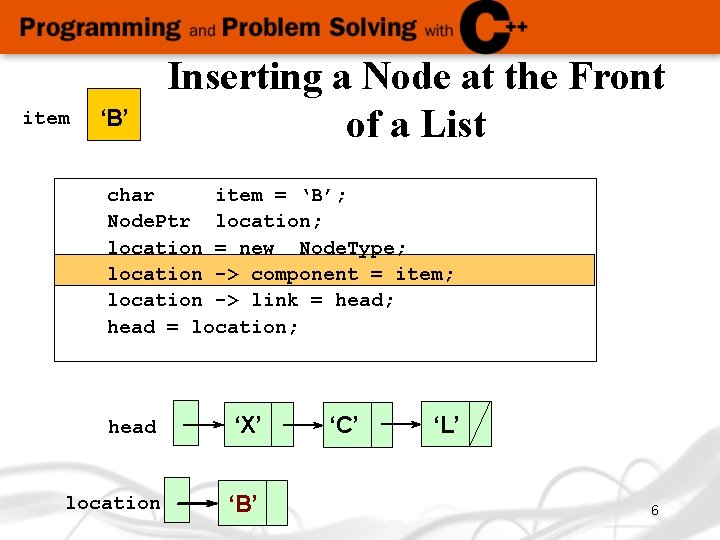
item ‘B’ Inserting a Node at the Front of a List char item = ‘B’; Node. Ptr location; location = new Node. Type; location -> component = item; location -> link = head; head = location; head ‘X’ location ‘B’ ‘C’ ‘L’ 6
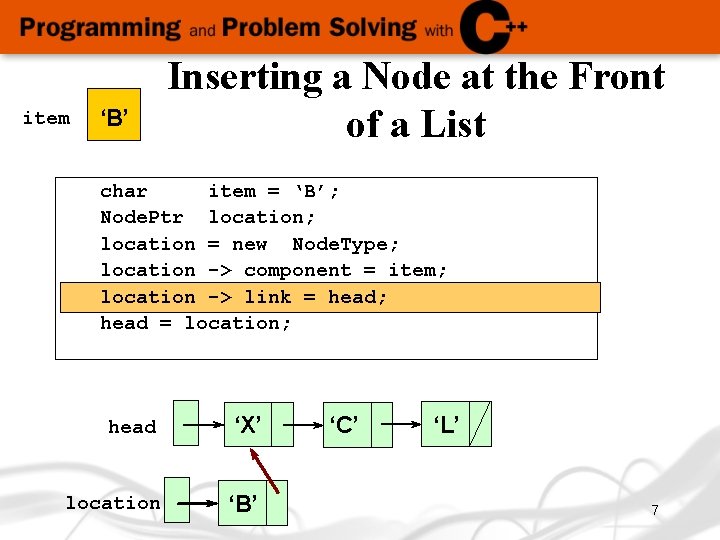
item ‘B’ Inserting a Node at the Front of a List char item = ‘B’; Node. Ptr location; location = new Node. Type; location -> component = item; location -> link = head; head = location; head ‘X’ location ‘B’ ‘C’ ‘L’ 7
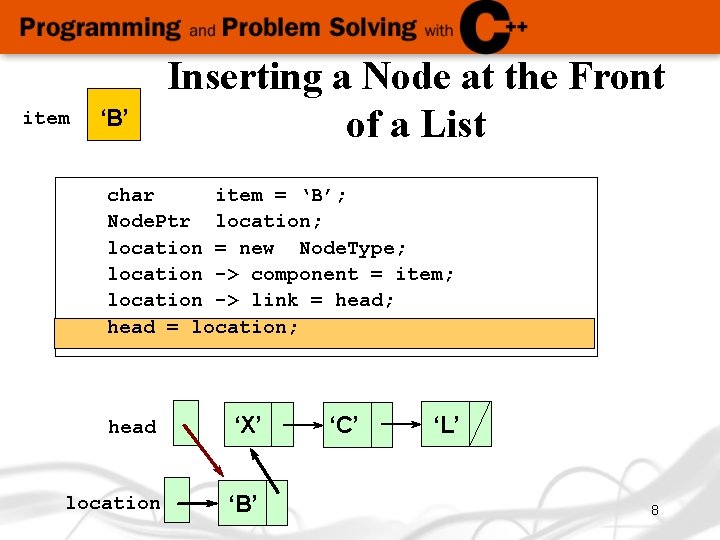
item ‘B’ Inserting a Node at the Front of a List char item = ‘B’; Node. Ptr location; location = new Node. Type; location -> component = item; location -> link = head; head = location; head ‘X’ location ‘B’ ‘C’ ‘L’ 8
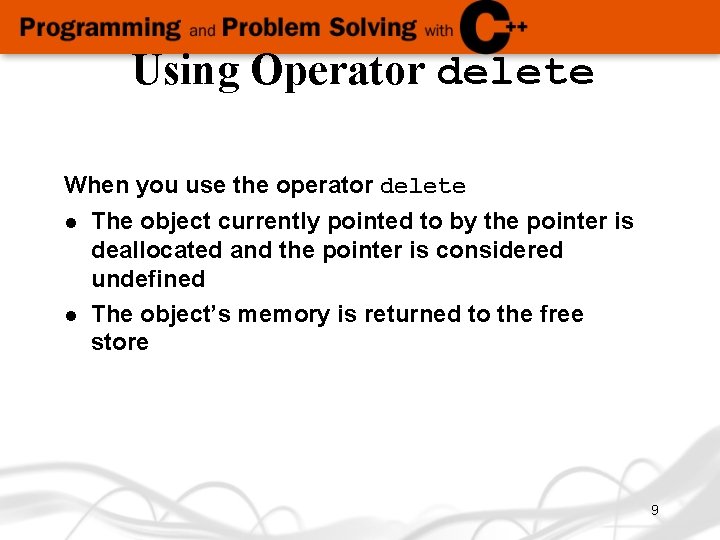
Using Operator delete When you use the operator delete l l The object currently pointed to by the pointer is deallocated and the pointer is considered undefined The object’s memory is returned to the free store 9
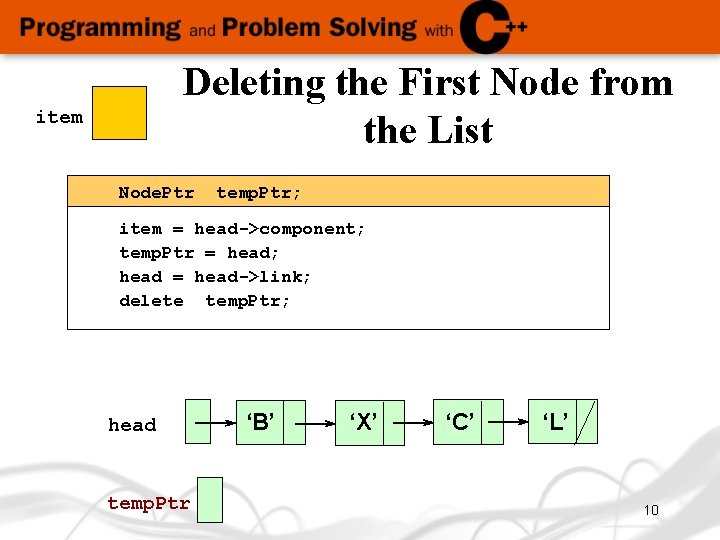
Deleting the First Node from the List item Node. Ptr temp. Ptr; item = head->component; temp. Ptr = head; head = head->link; delete temp. Ptr; head temp. Ptr ‘B’ ‘X’ ‘C’ ‘L’ 10
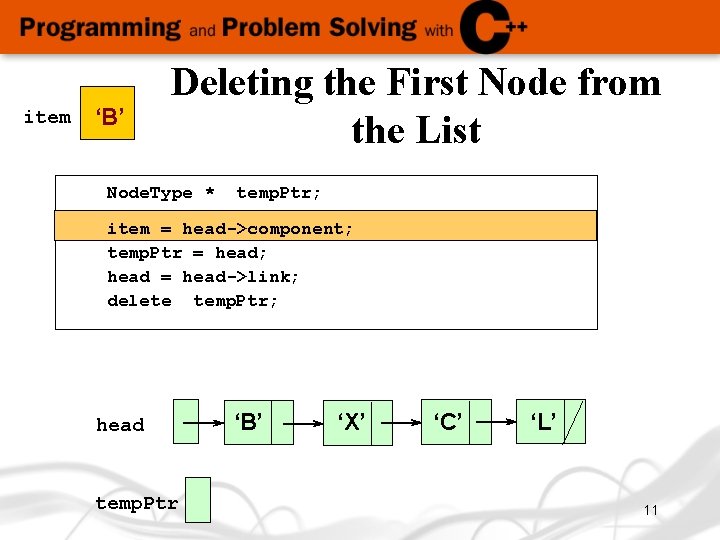
item ‘B’ Deleting the First Node from the List Node. Type * temp. Ptr; item = head->component; temp. Ptr = head; head = head->link; delete temp. Ptr; head temp. Ptr ‘B’ ‘X’ ‘C’ ‘L’ 11
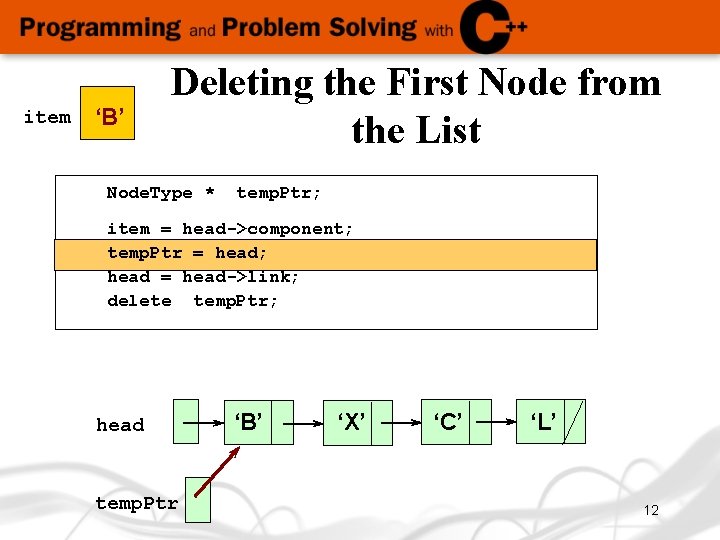
item ‘B’ Deleting the First Node from the List Node. Type * temp. Ptr; item = head->component; temp. Ptr = head; head = head->link; delete temp. Ptr; head temp. Ptr ‘B’ ‘X’ ‘C’ ‘L’ 12
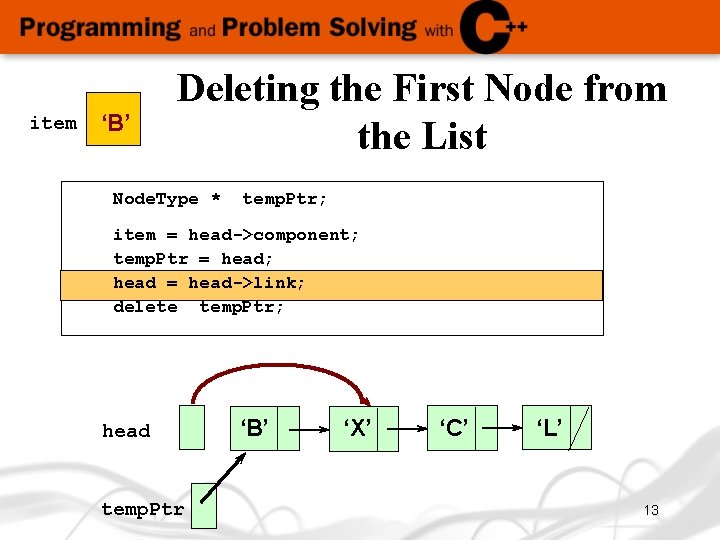
item ‘B’ Deleting the First Node from the List Node. Type * temp. Ptr; item = head->component; temp. Ptr = head; head = head->link; delete temp. Ptr; head temp. Ptr ‘B’ ‘X’ ‘C’ ‘L’ 13
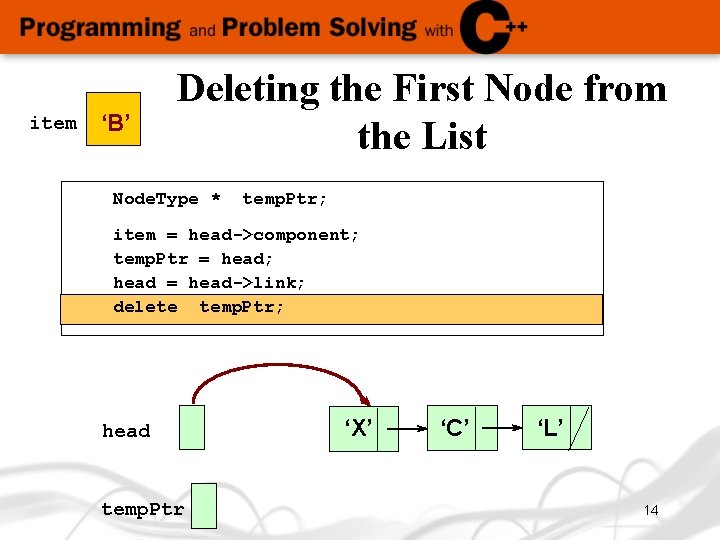
item ‘B’ Deleting the First Node from the List Node. Type * temp. Ptr; item = head->component; temp. Ptr = head; head = head->link; delete temp. Ptr; head temp. Ptr ‘X’ ‘C’ ‘L’ 14
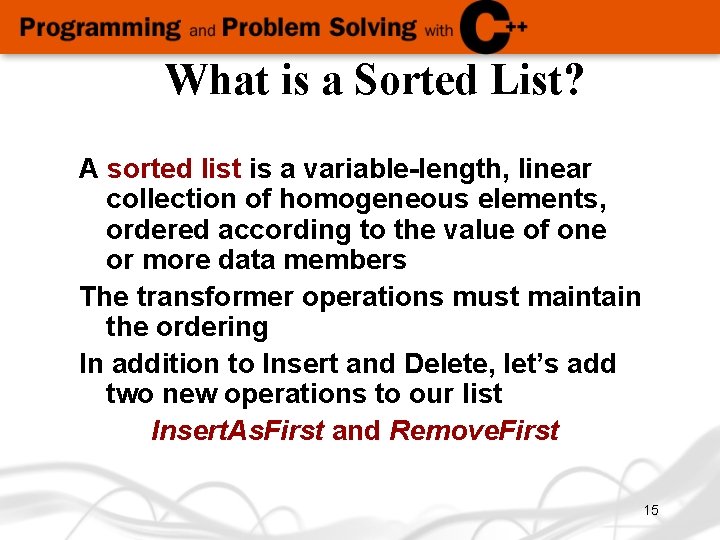
What is a Sorted List? A sorted list is a variable-length, linear collection of homogeneous elements, ordered according to the value of one or more data members The transformer operations must maintain the ordering In addition to Insert and Delete, let’s add two new operations to our list Insert. As. First and Remove. First 15
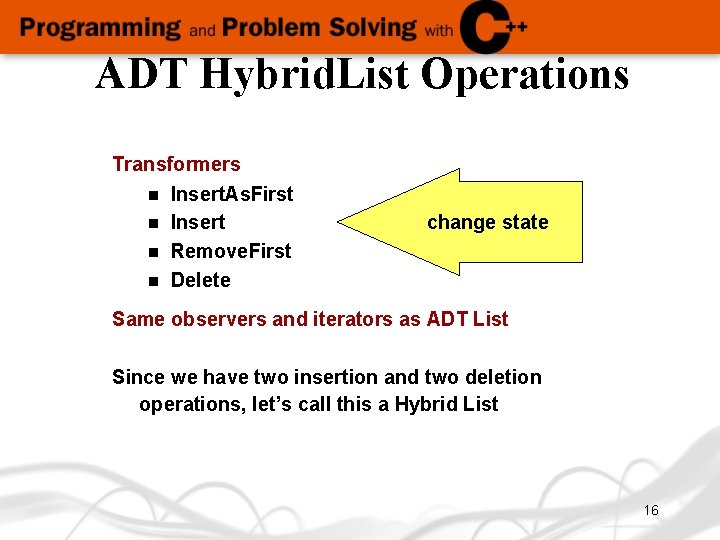
ADT Hybrid. List Operations Transformers n Insert. As. First n Insert n Remove. First n Delete change state Same observers and iterators as ADT List Since we have two insertion and two deletion operations, let’s call this a Hybrid List 16
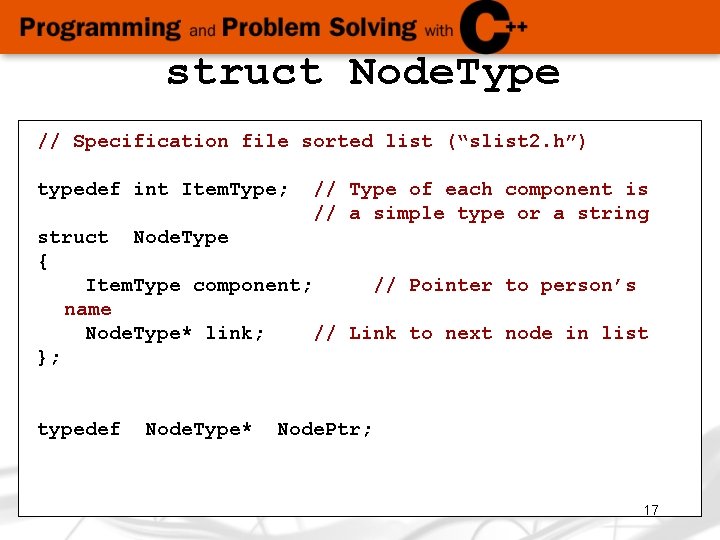
struct Node. Type // Specification file sorted list (“slist 2. h”) typedef int Item. Type; // Type of each component is // a simple type or a string struct Node. Type { Item. Type component; // Pointer to person’s name Node. Type* link; // Link to next node in list }; typedef Node. Type* Node. Ptr; 17
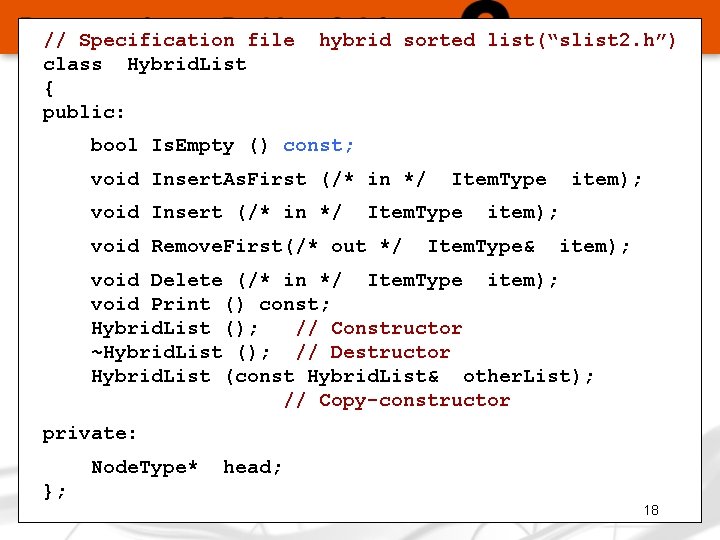
// Specification file class Hybrid. List { public: hybrid sorted list(“slist 2. h”) bool Is. Empty () const; void Insert. As. First (/* in */ void Insert (/* in */ Item. Type void Remove. First(/* out */ item); Item. Type& item); void Delete (/* in */ Item. Type item); void Print () const; Hybrid. List (); // Constructor ~Hybrid. List (); // Destructor Hybrid. List (const Hybrid. List& other. List); // Copy-constructor private: Node. Type* }; head; 18
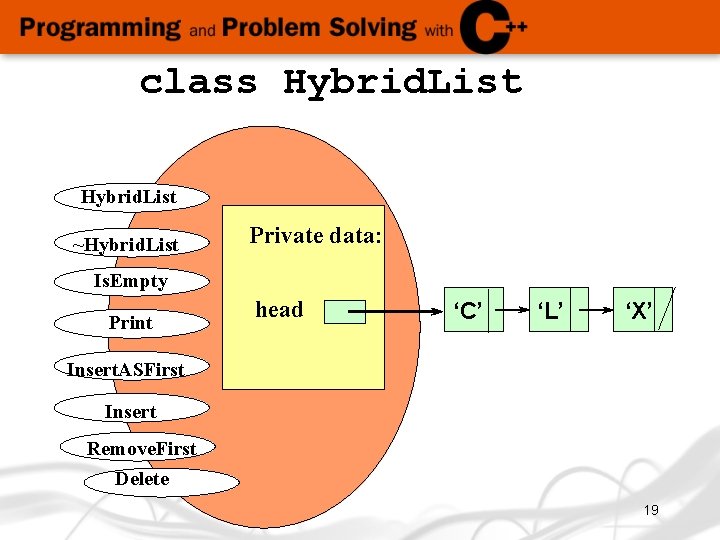
class Hybrid. List ~Hybrid. List Private data: Is. Empty Print head ‘C’ ‘L’ ‘X’ Insert. ASFirst Insert Remove. First Delete 19
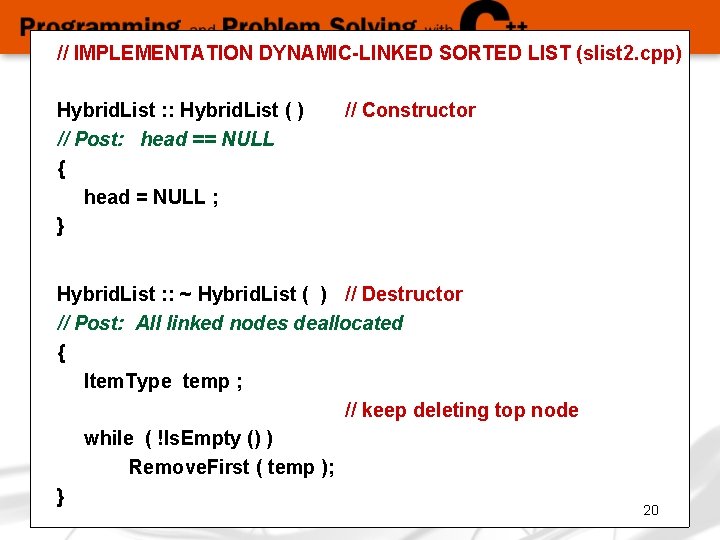
// IMPLEMENTATION DYNAMIC-LINKED SORTED LIST (slist 2. cpp) Hybrid. List : : Hybrid. List ( ) // Post: head == NULL { head = NULL ; } // Constructor Hybrid. List : : ~ Hybrid. List ( ) // Destructor // Post: All linked nodes deallocated { Item. Type temp ; // keep deleting top node while ( !Is. Empty () ) Remove. First ( temp ); } 20
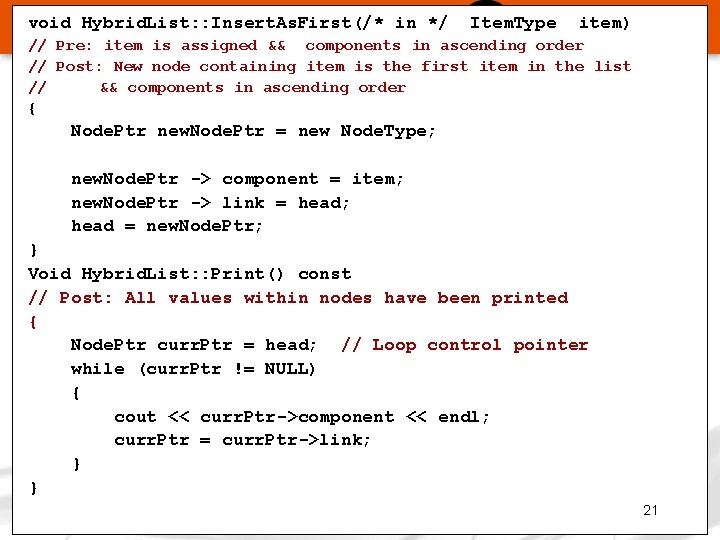
void Hybrid. List: : Insert. As. First(/* in */ Item. Type item) // Pre: item is assigned && components in ascending order // Post: New node containing item is the first item in the list // && components in ascending order { Node. Ptr new. Node. Ptr = new Node. Type; new. Node. Ptr -> component = item; new. Node. Ptr -> link = head; head = new. Node. Ptr; } Void Hybrid. List: : Print() const // Post: All values within nodes have been printed { Node. Ptr curr. Ptr = head; // Loop control pointer while (curr. Ptr != NULL) { cout << curr. Ptr->component << endl; curr. Ptr = curr. Ptr->link; } } 21
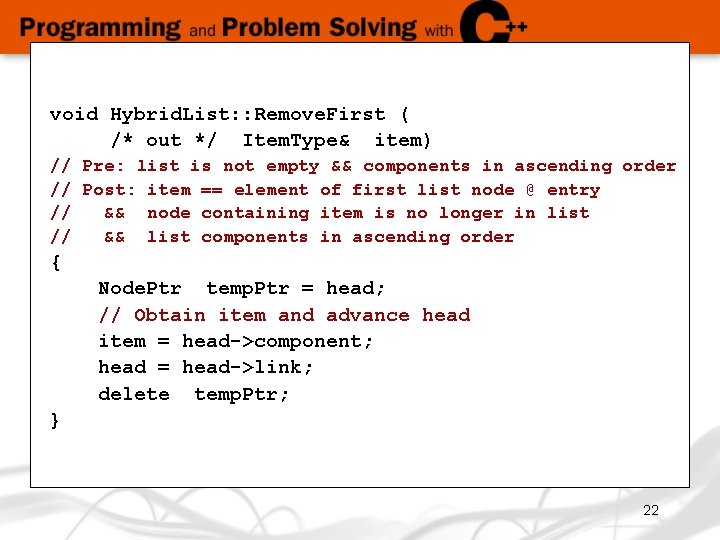
void Hybrid. List: : Remove. First ( /* out */ Item. Type& item) // Pre: list is not empty && components in ascending order // Post: item == element of first list node @ entry // && node containing item is no longer in list // && list components in ascending order { Node. Ptr temp. Ptr = head; // Obtain item and advance head item = head->component; head = head->link; delete temp. Ptr; } 22
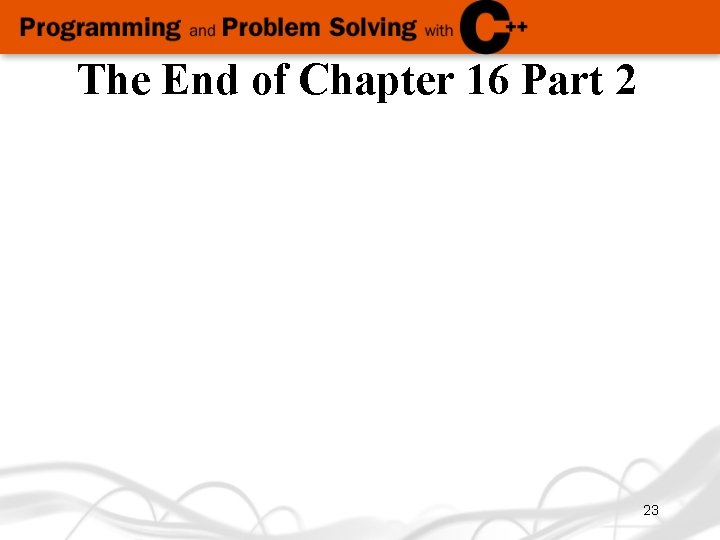
The End of Chapter 16 Part 2 23