Chapter 12 GUIs Java and Swing Overview n
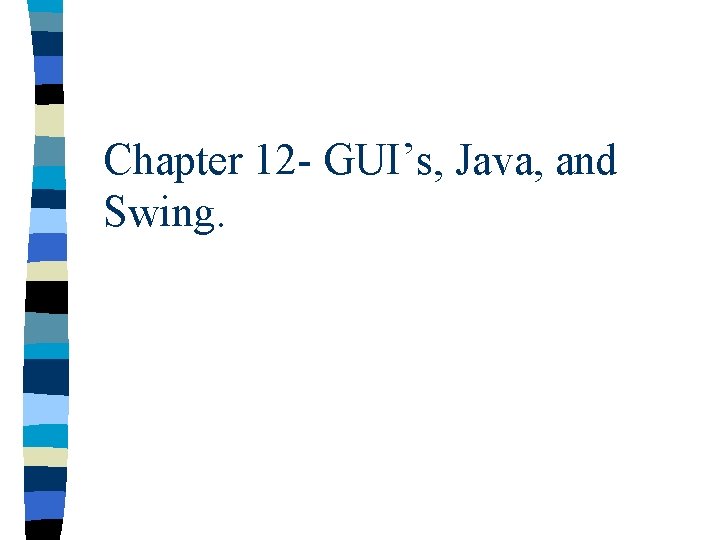
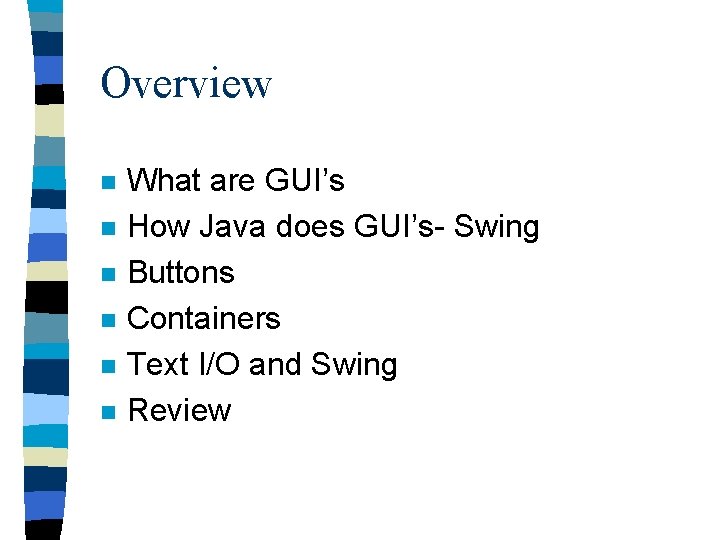
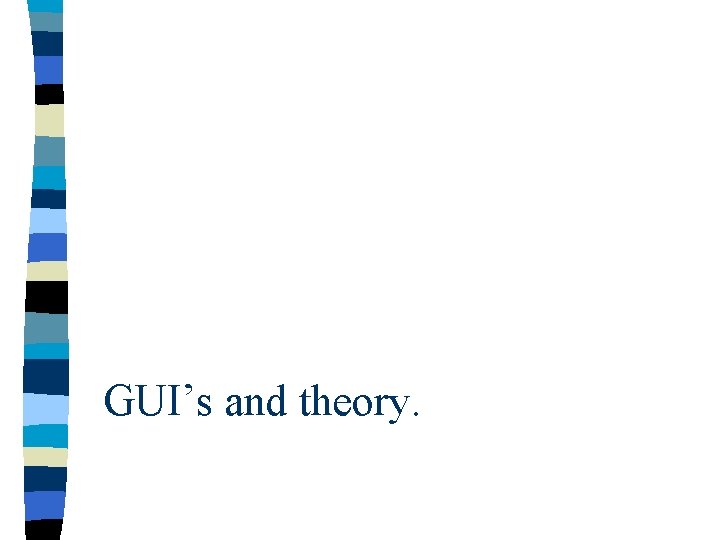
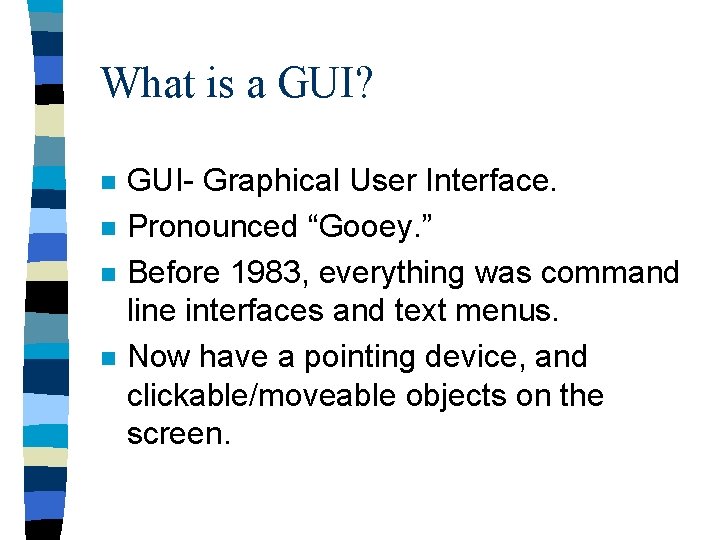
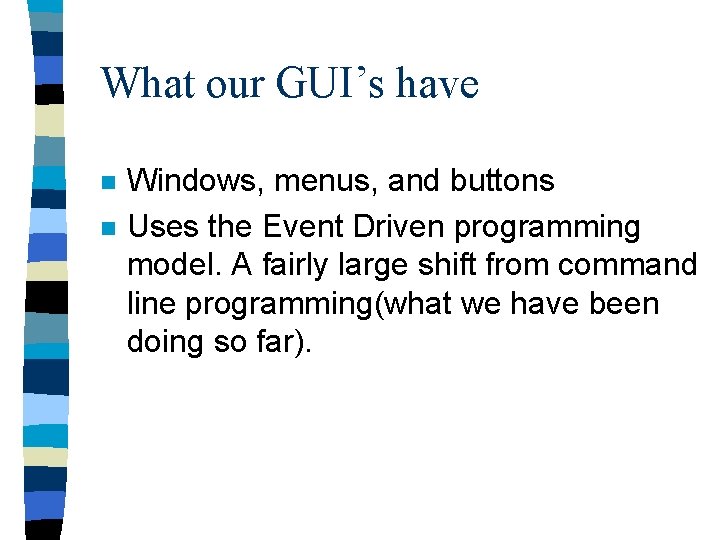
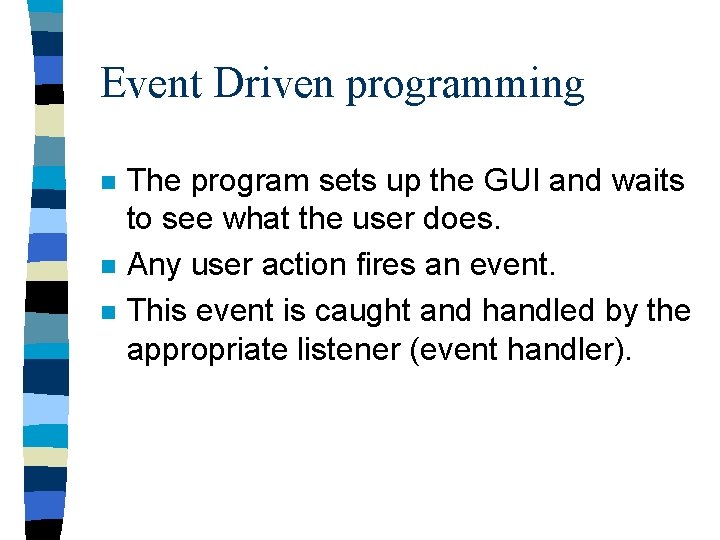
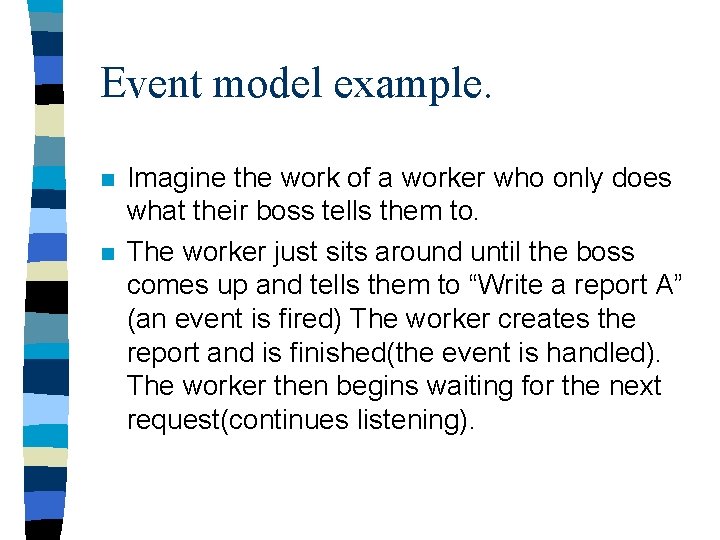
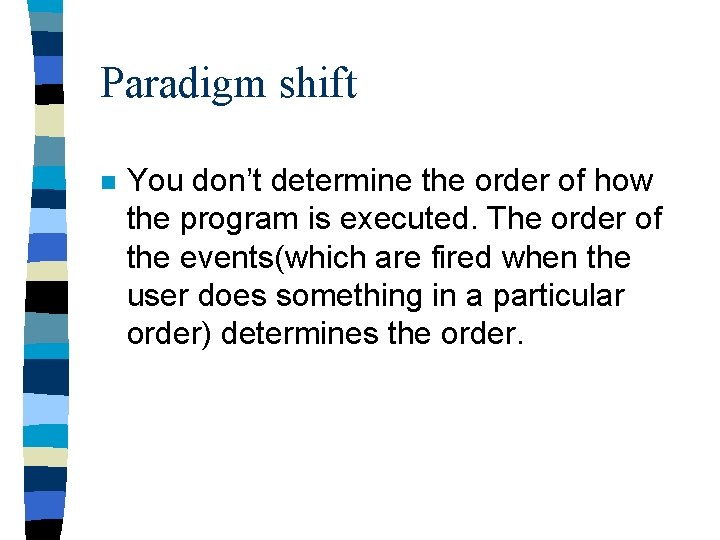
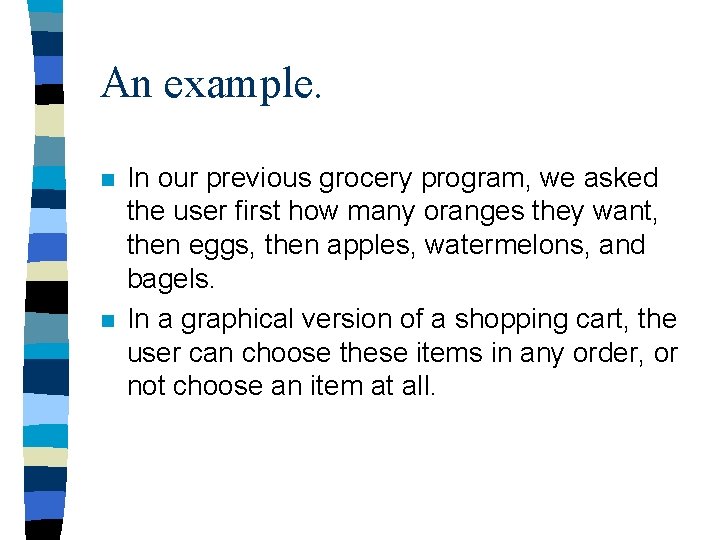
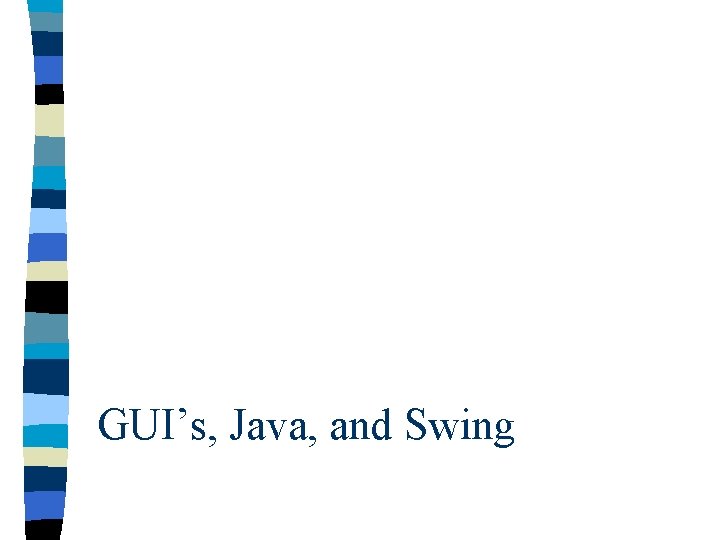
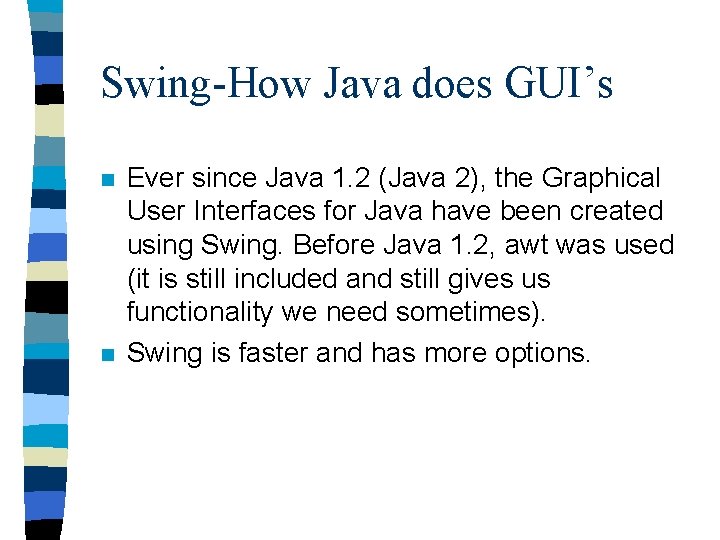
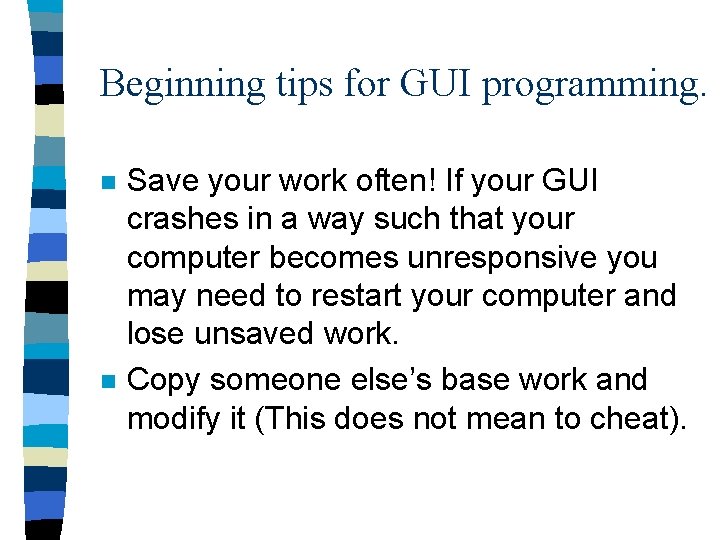
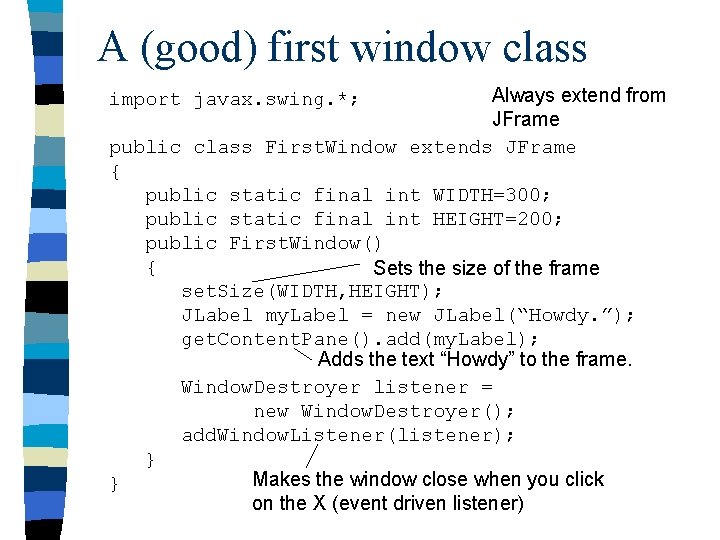
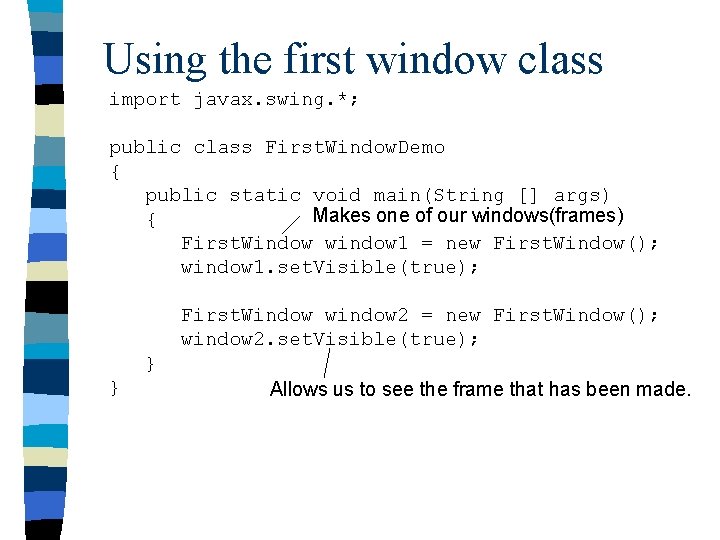
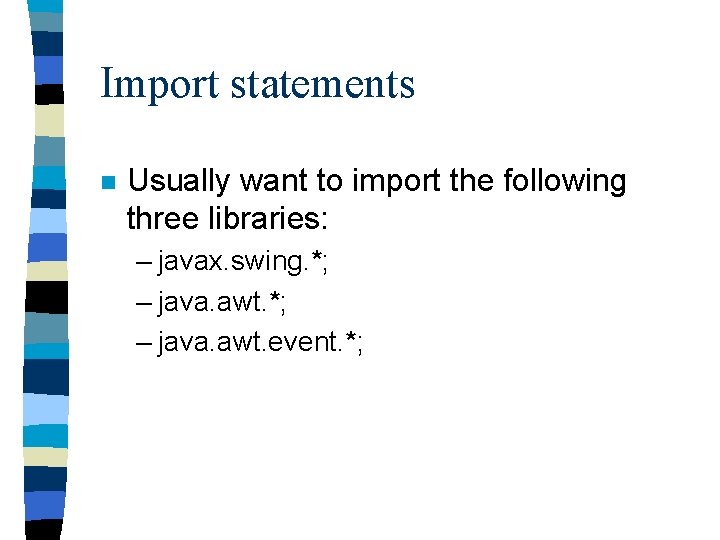
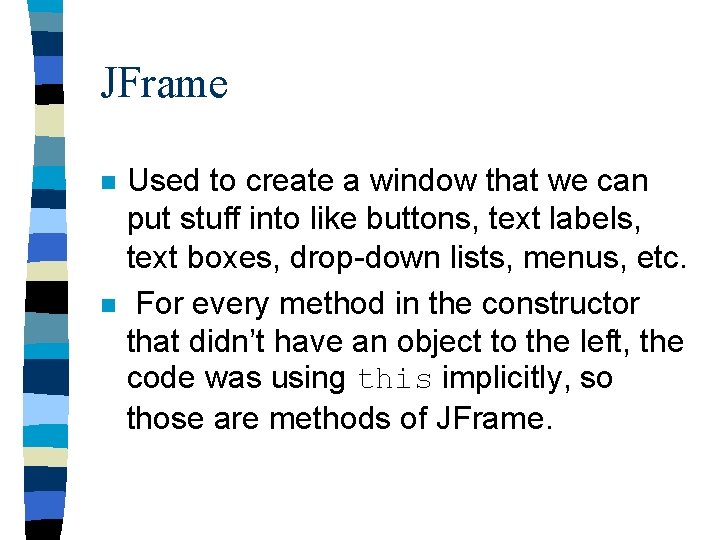
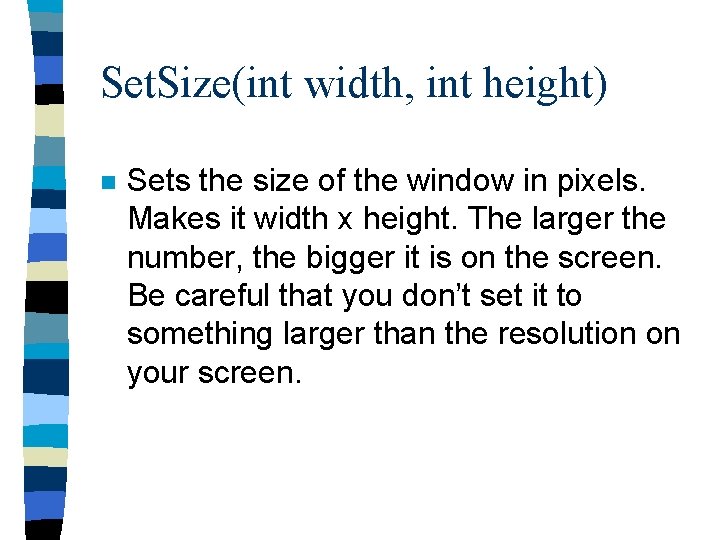
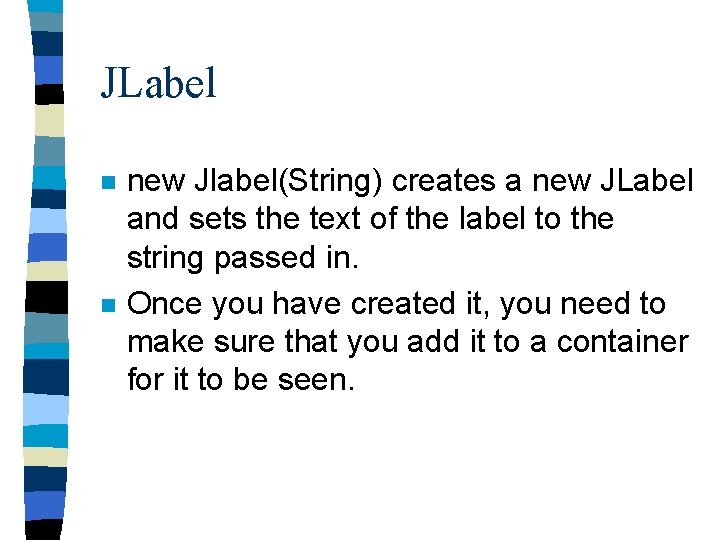
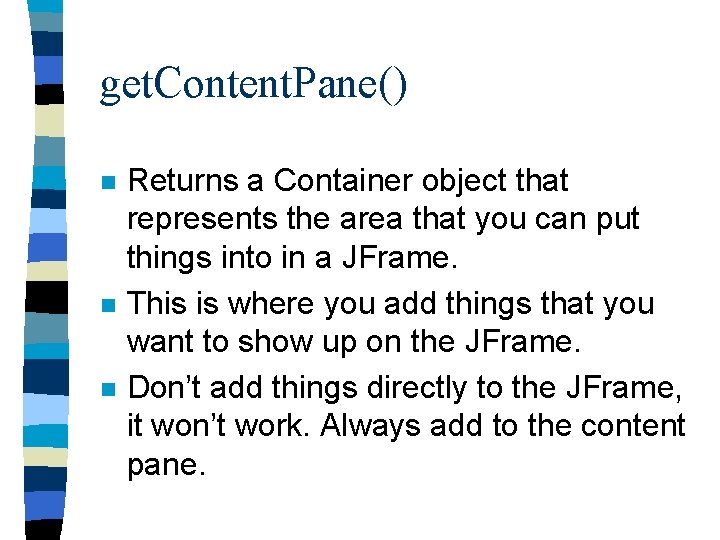
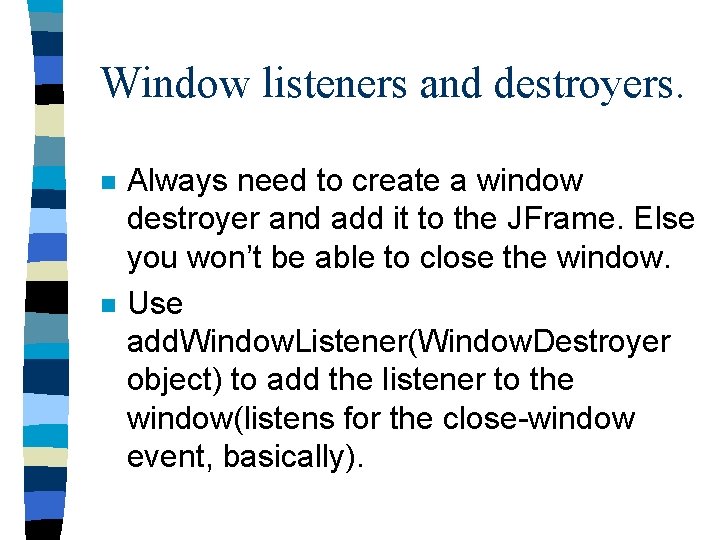
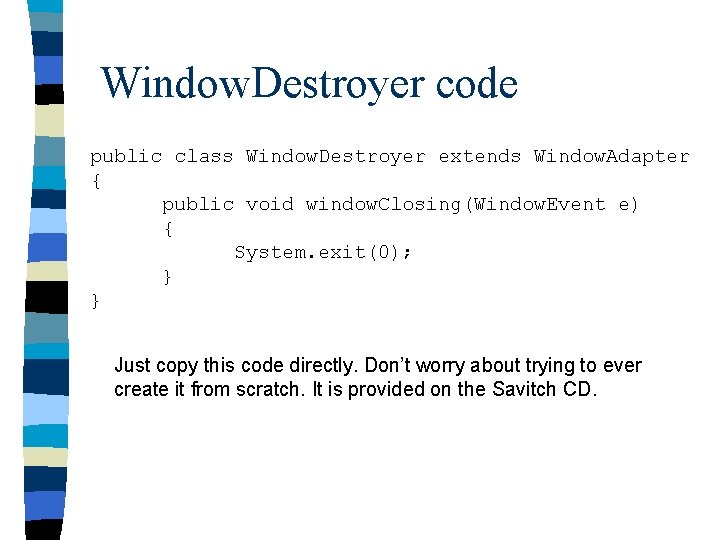
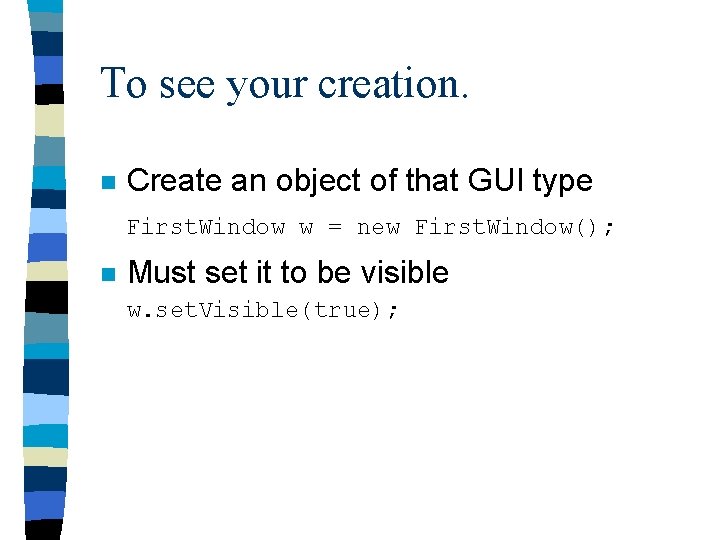
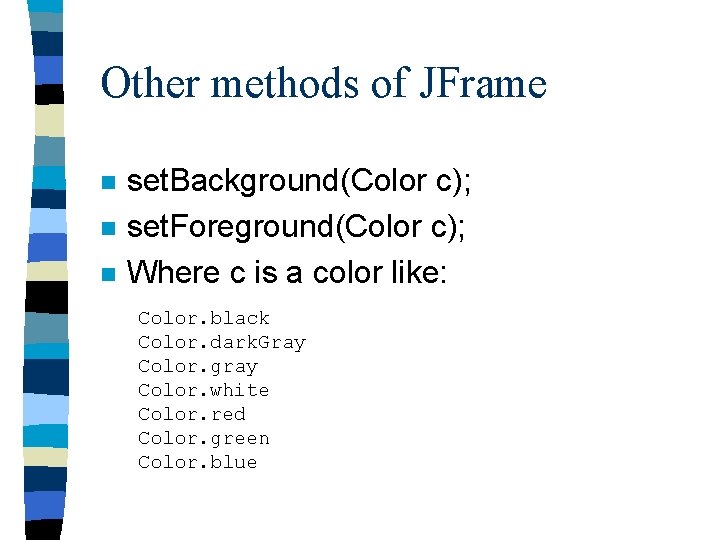
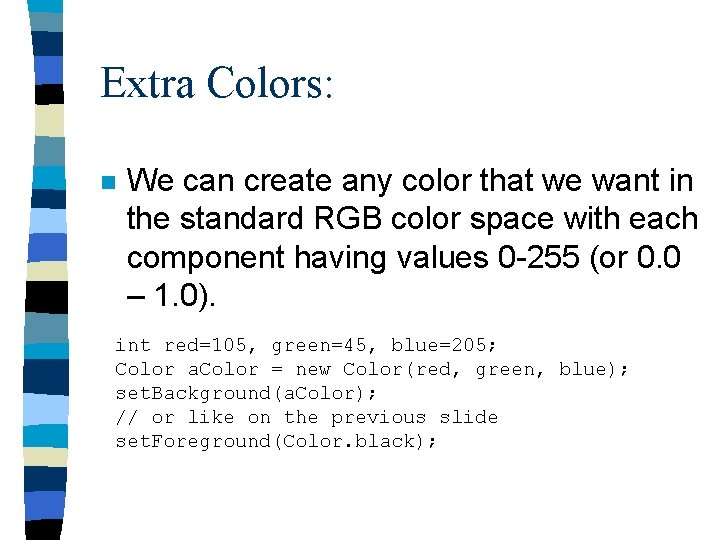
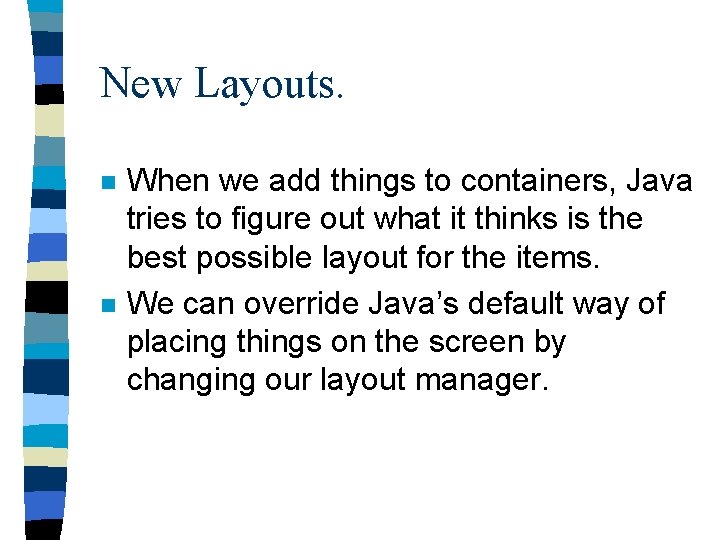
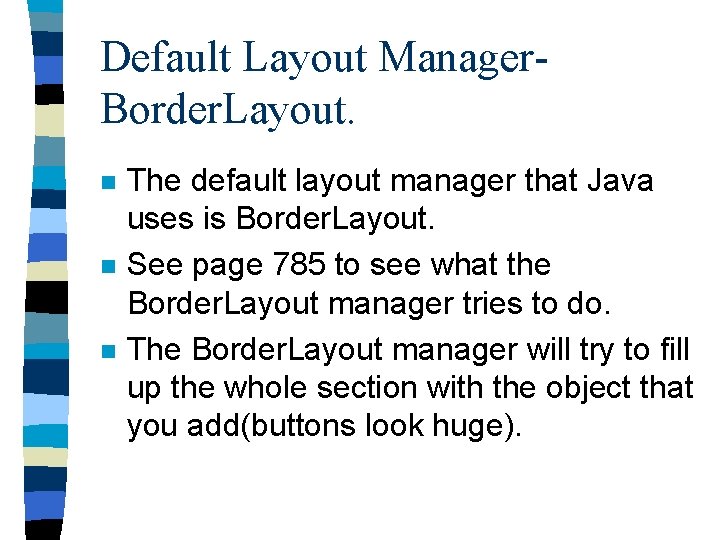
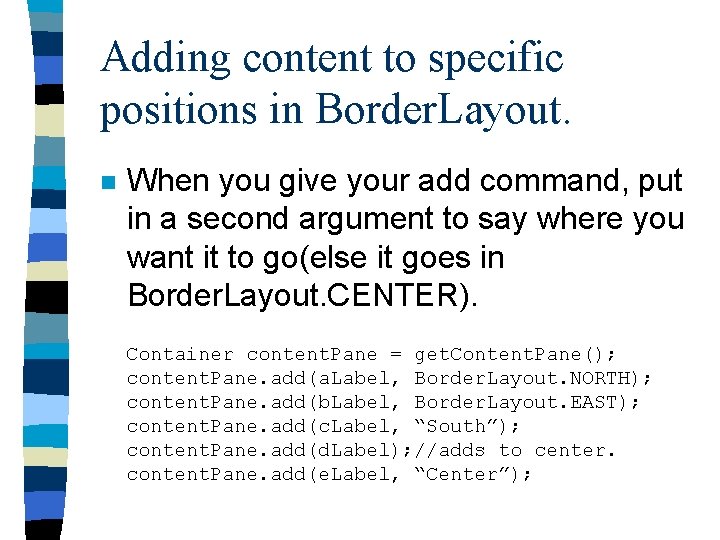
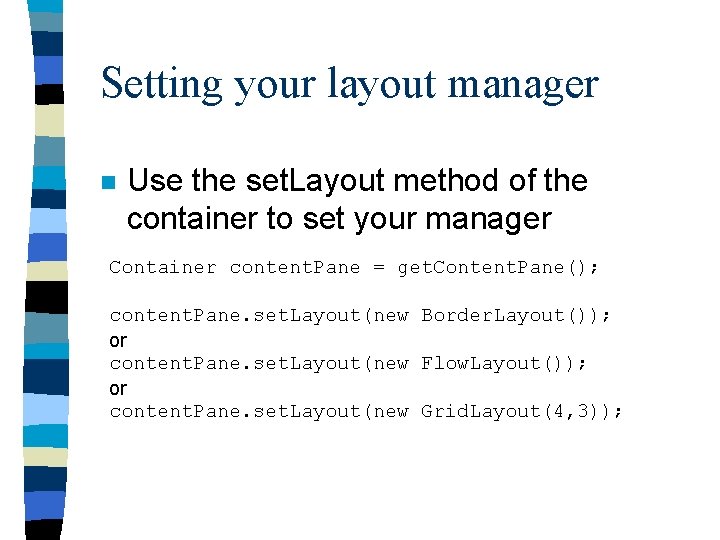
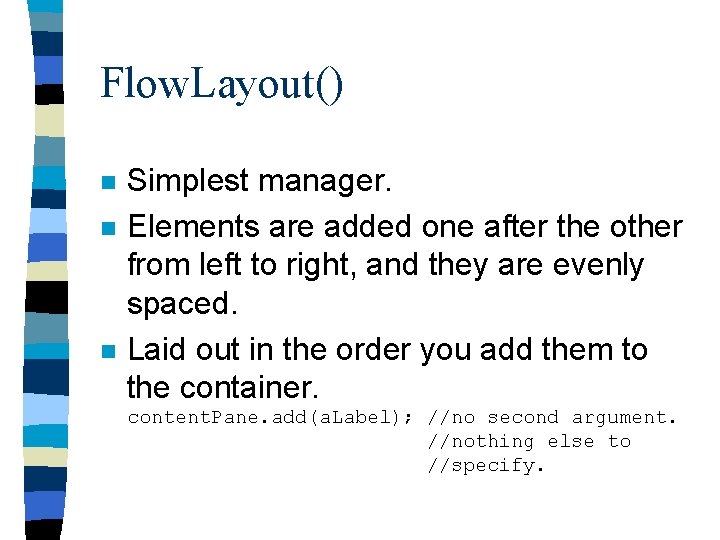
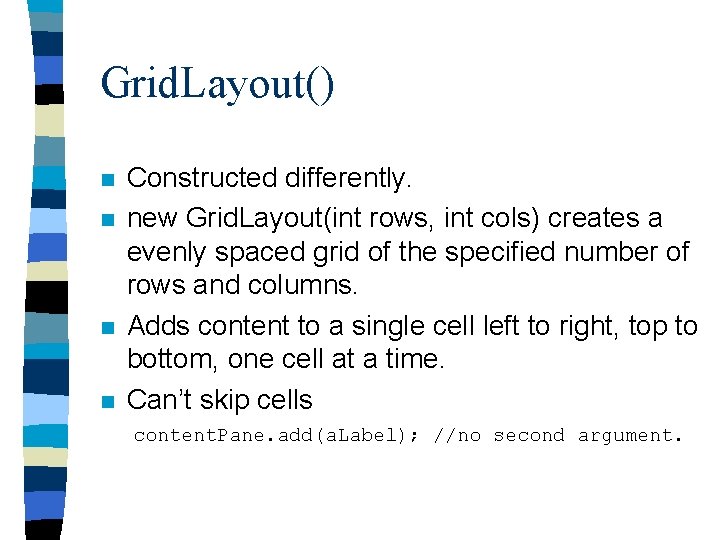
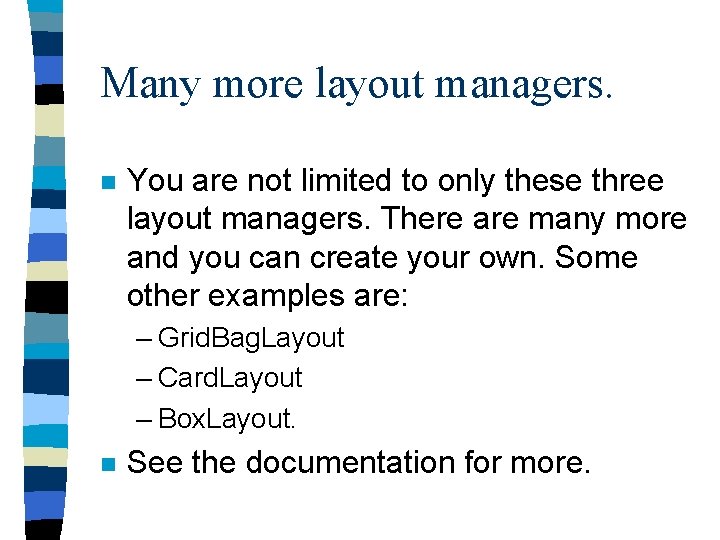
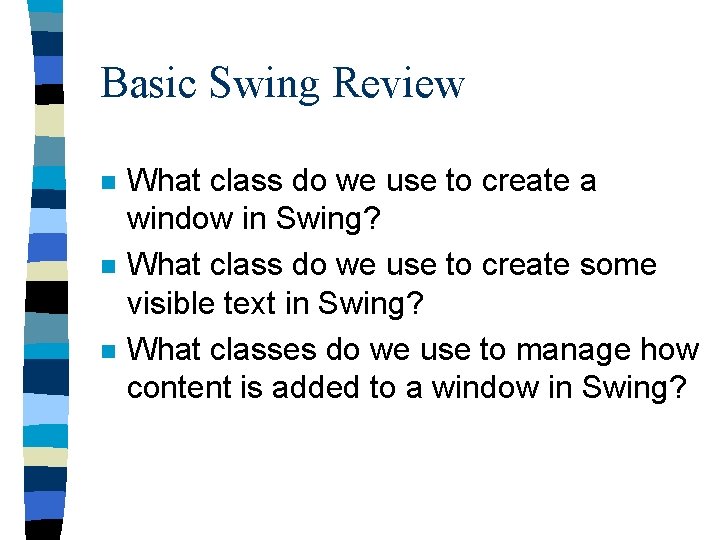
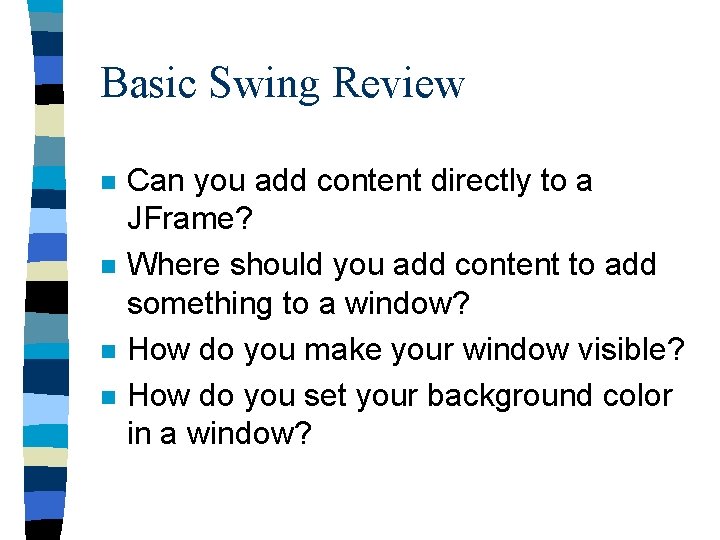
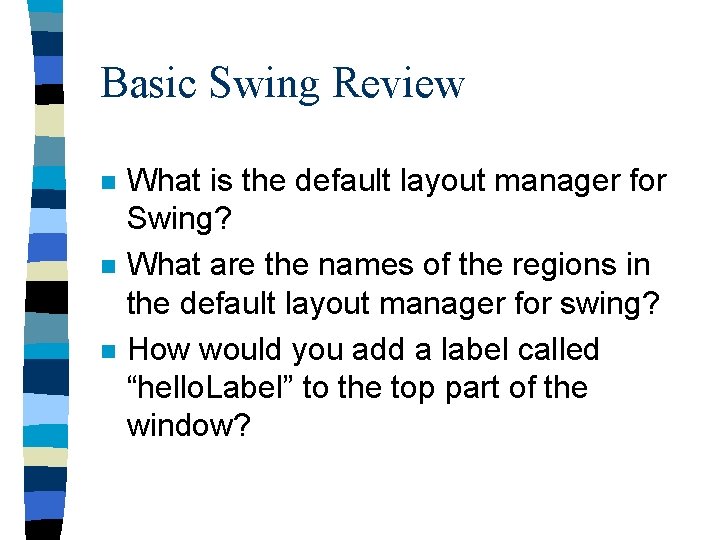
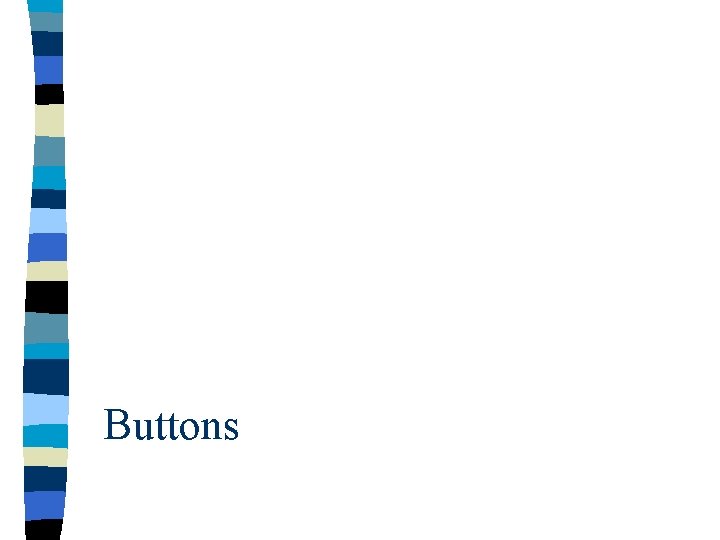
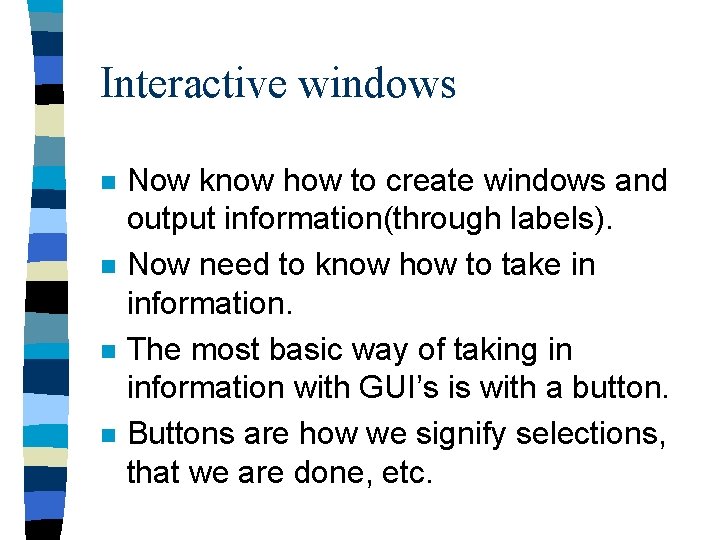
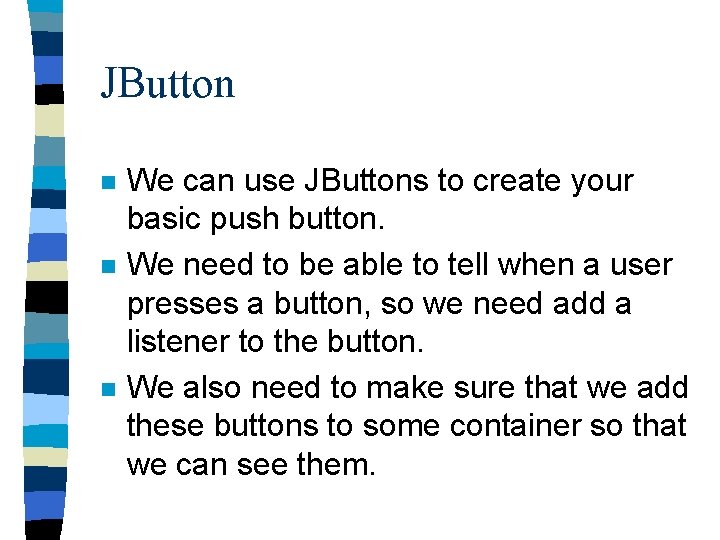
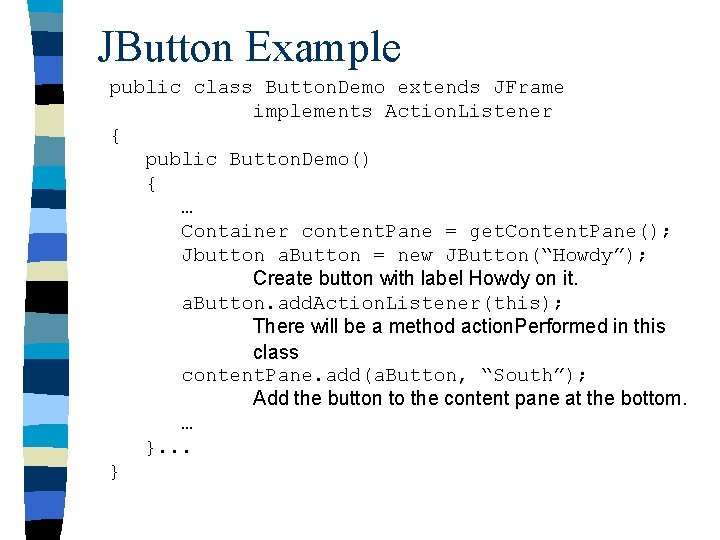
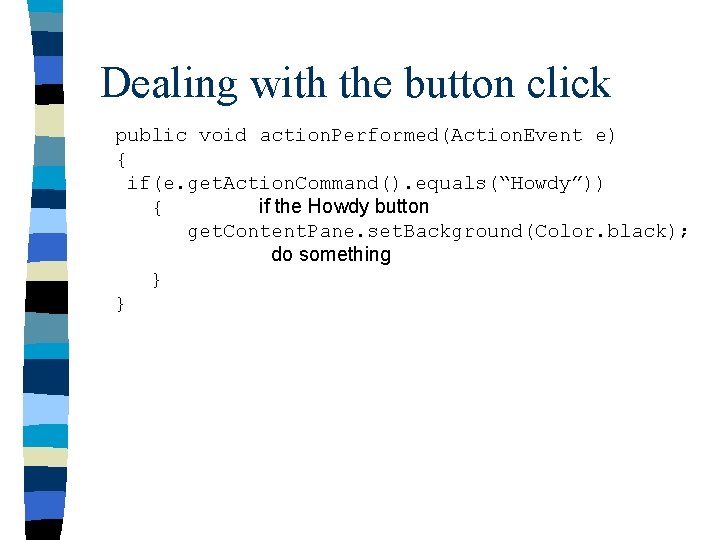
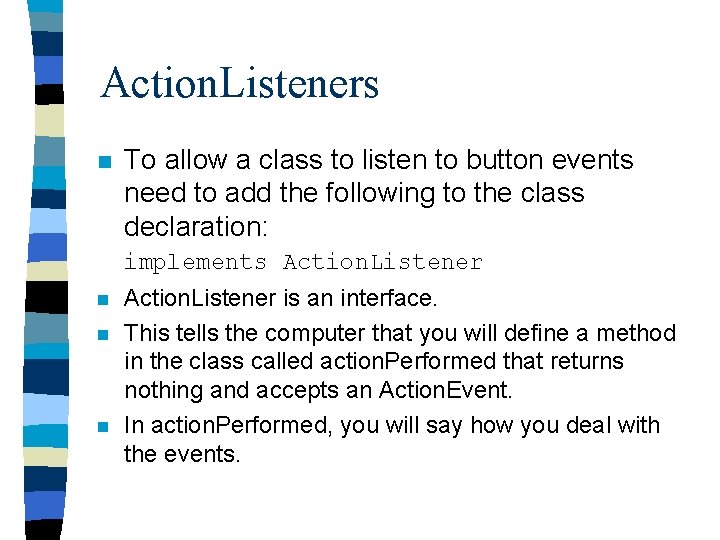
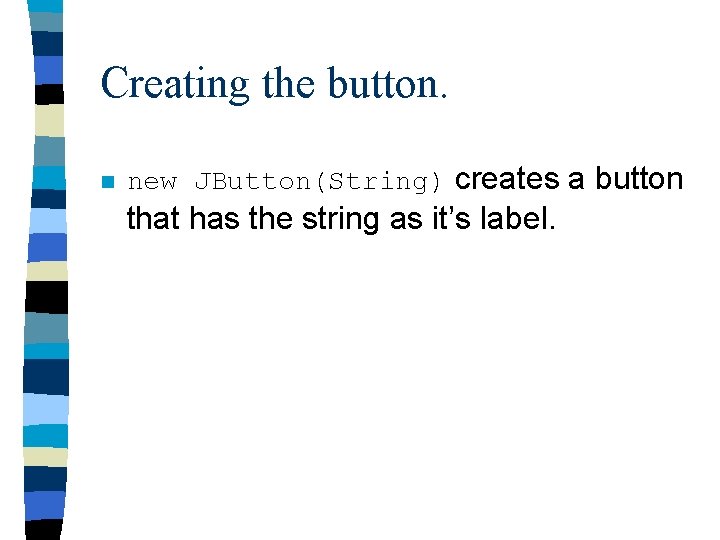
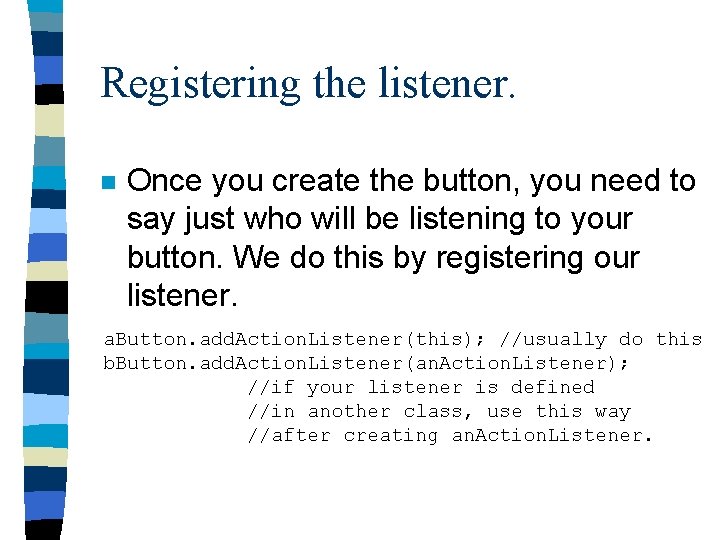
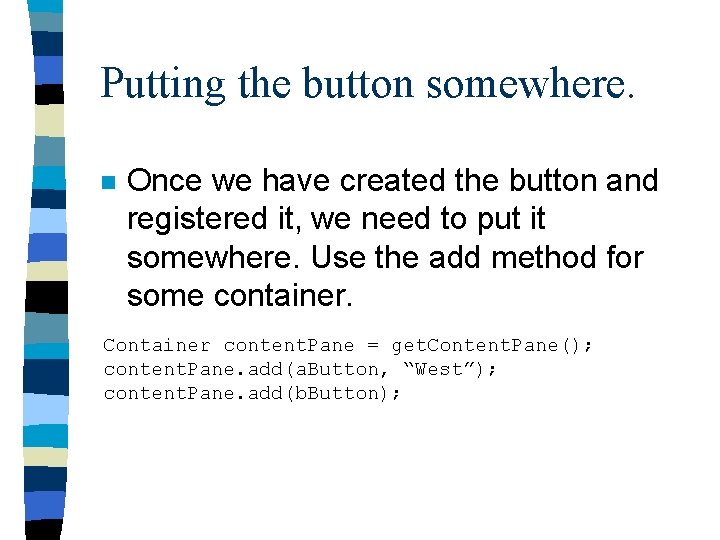
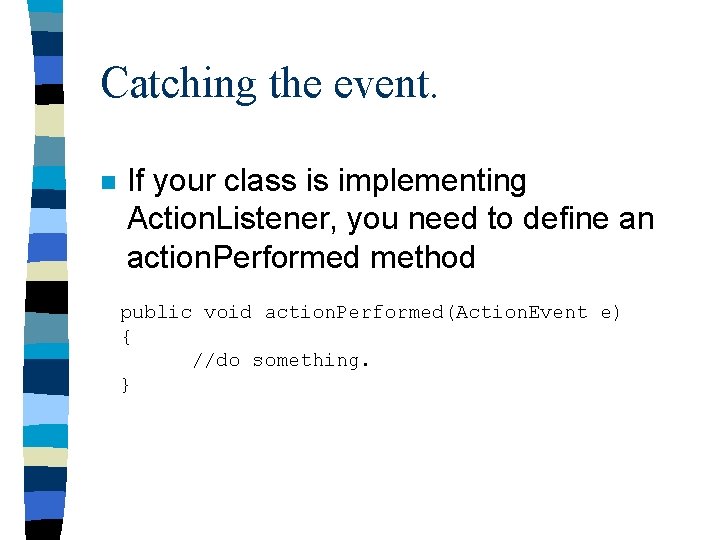
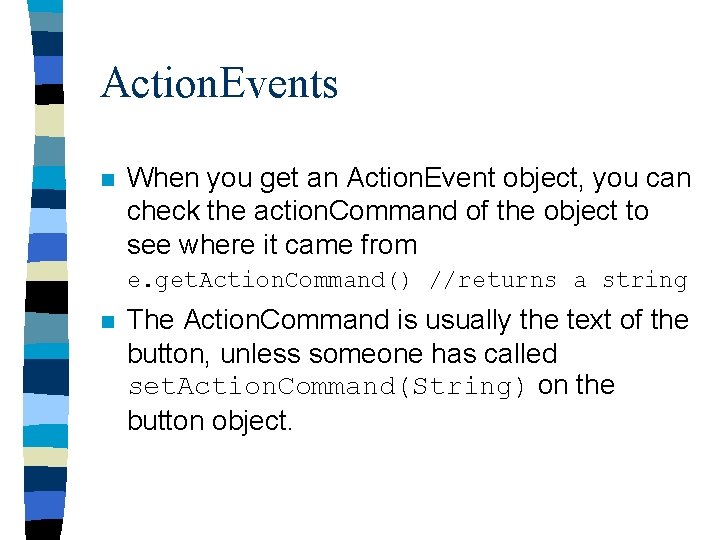
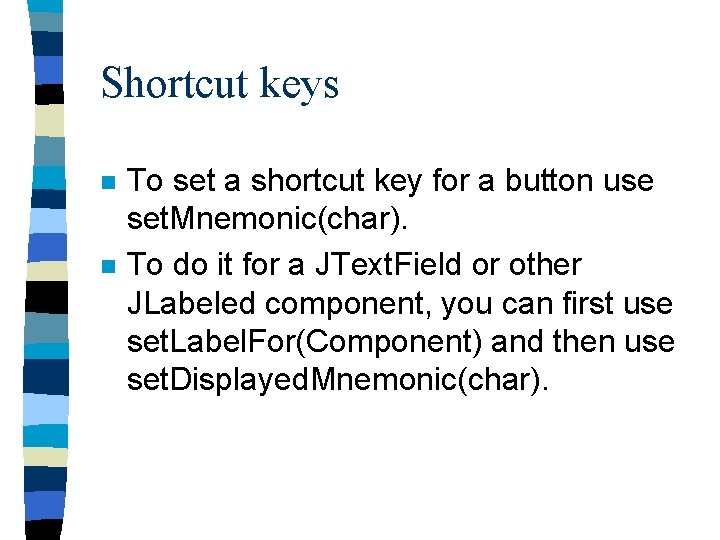
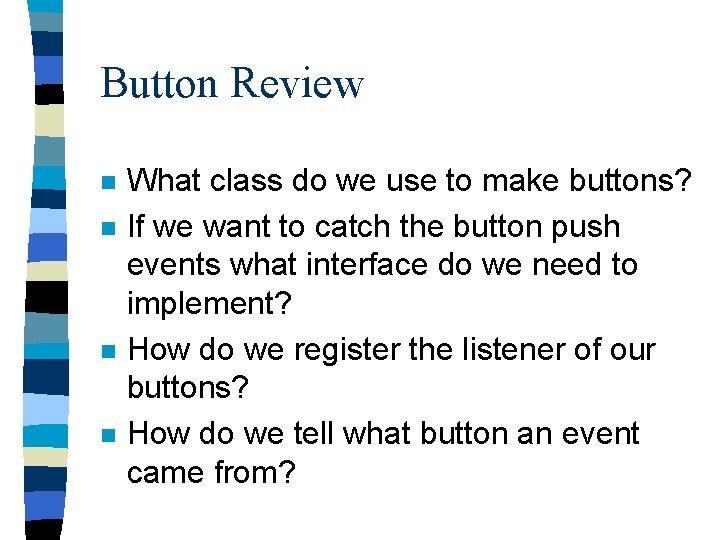
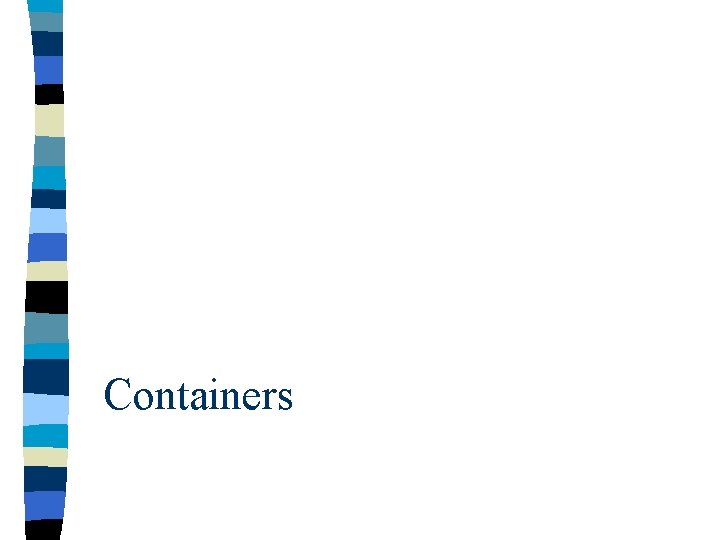
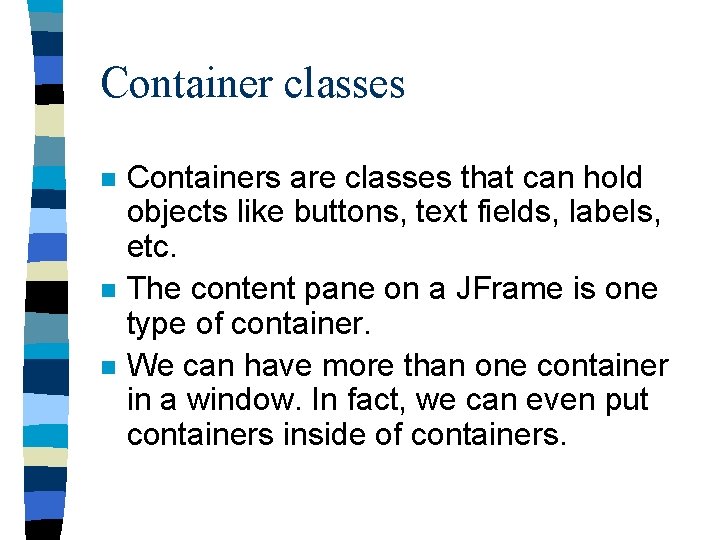
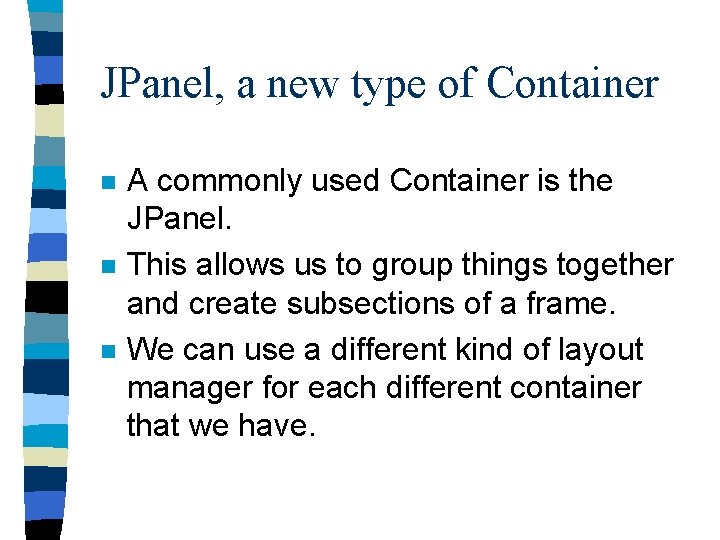
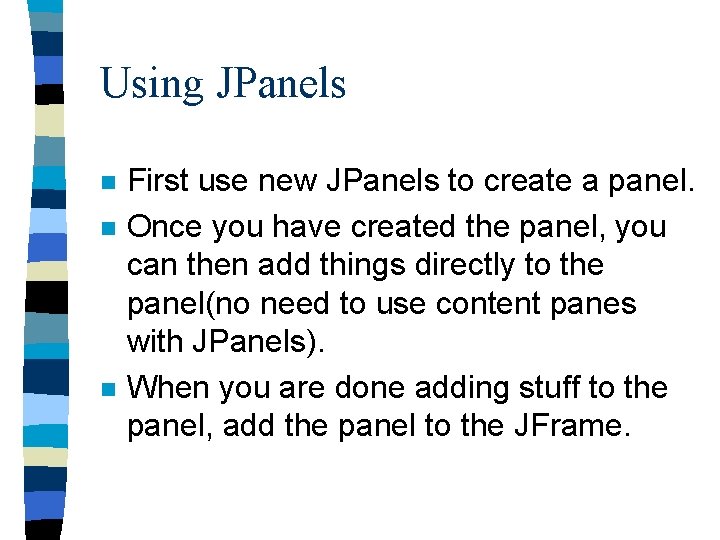
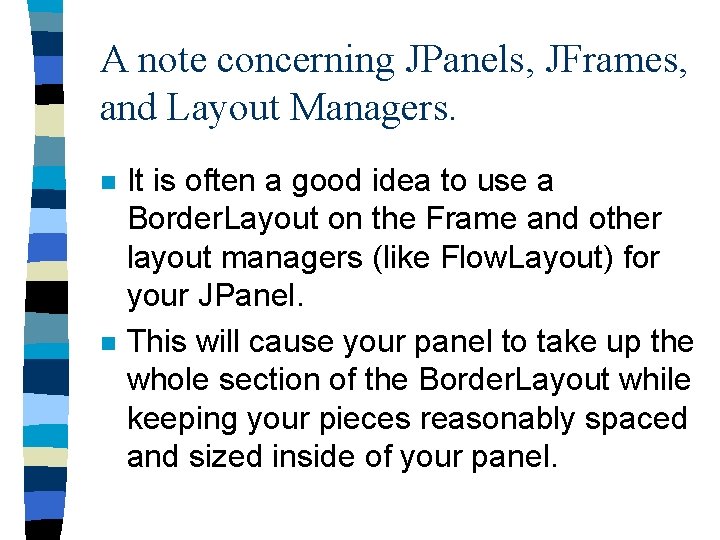
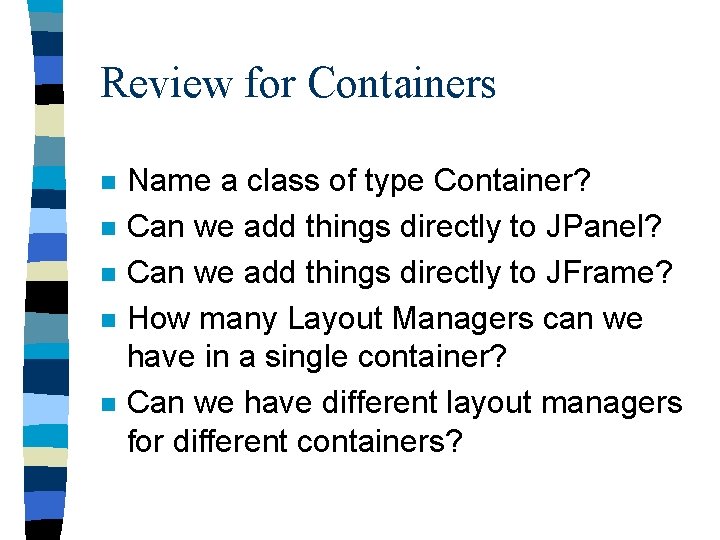
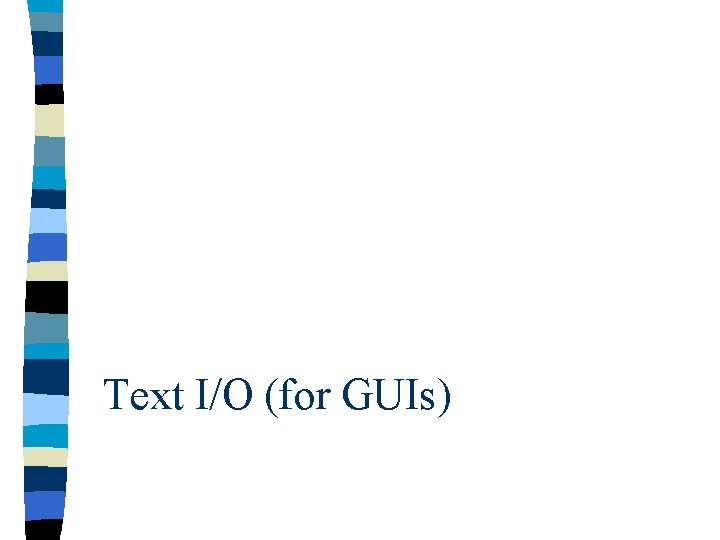
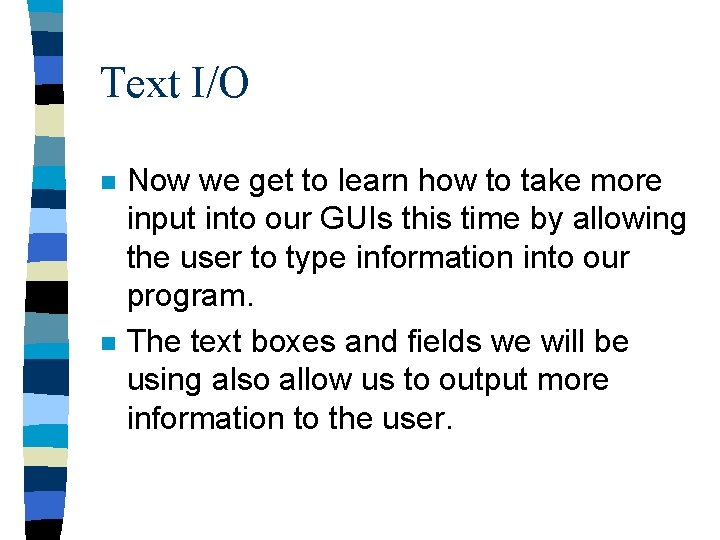
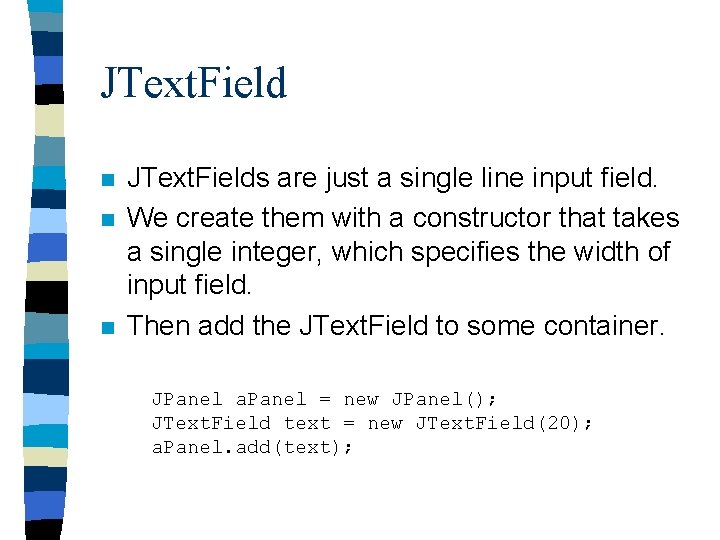
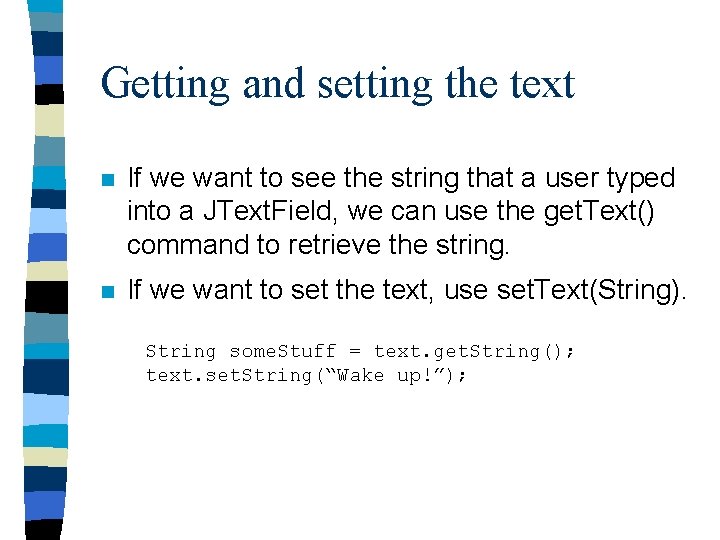
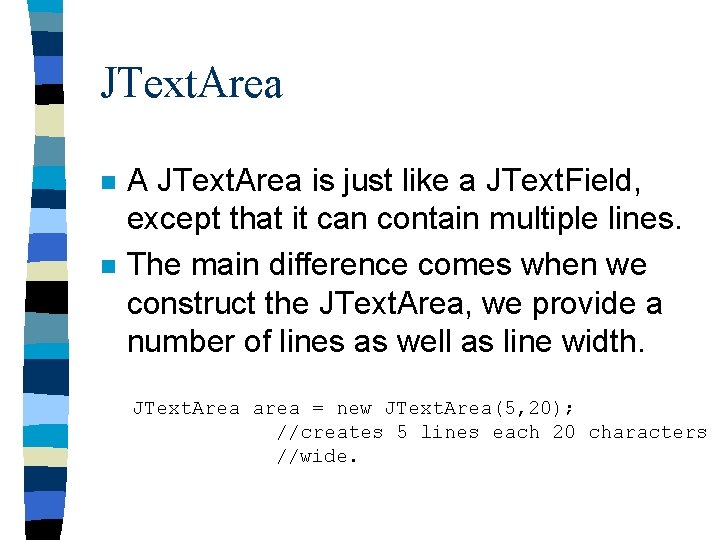
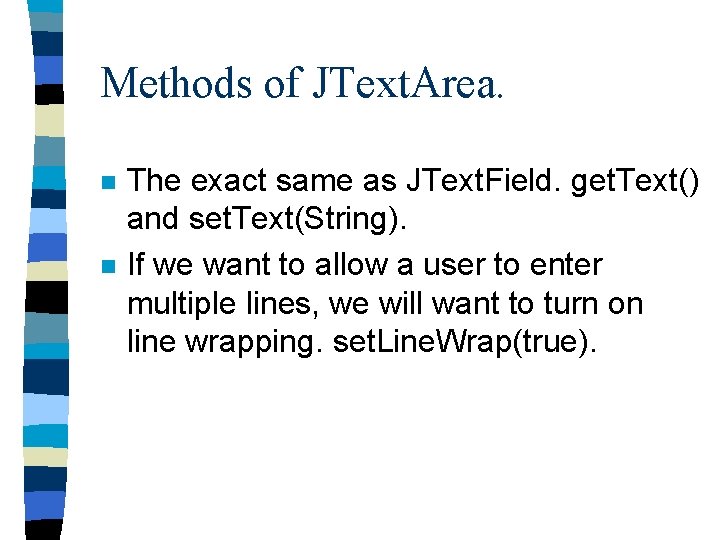
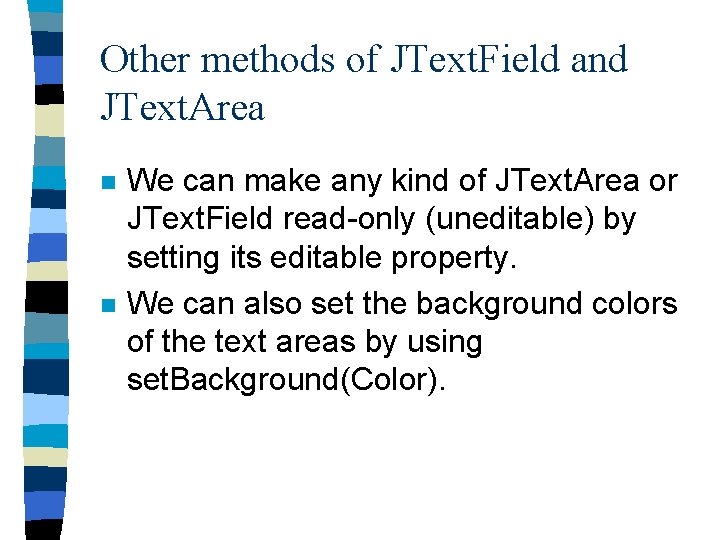
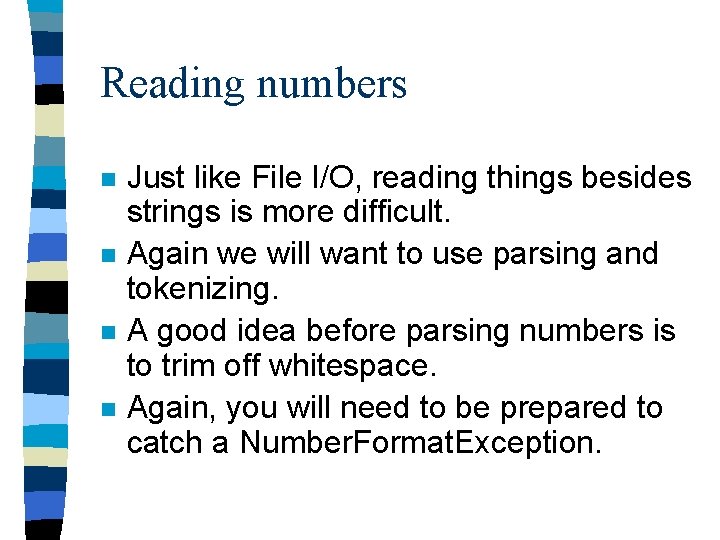
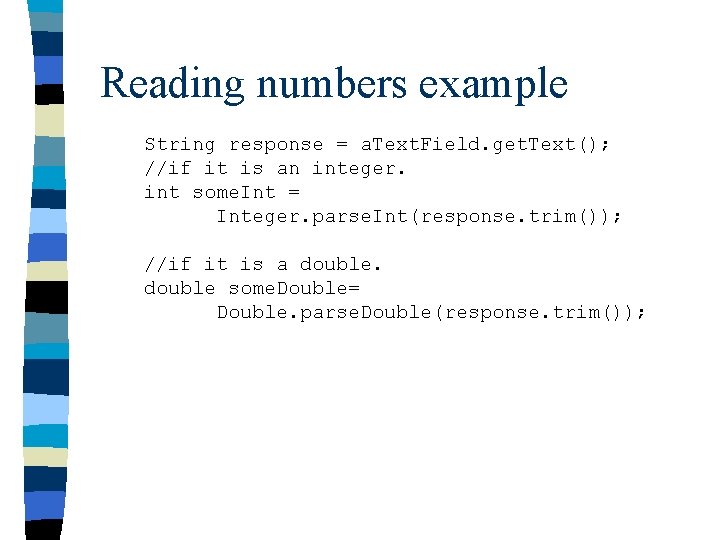
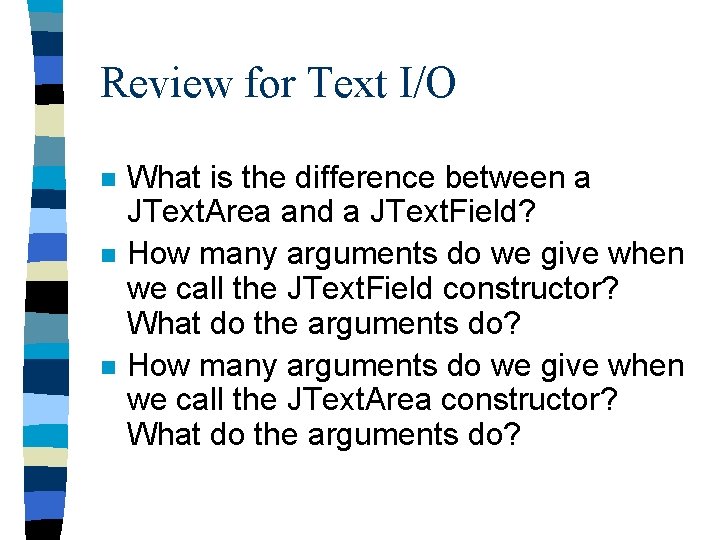
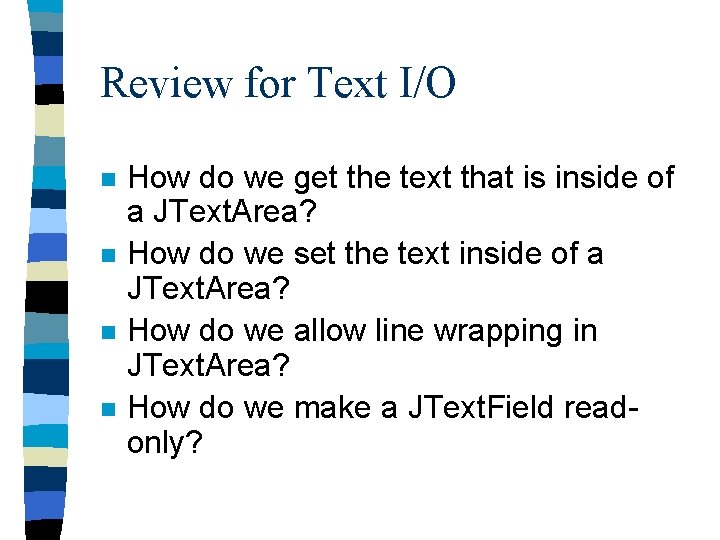
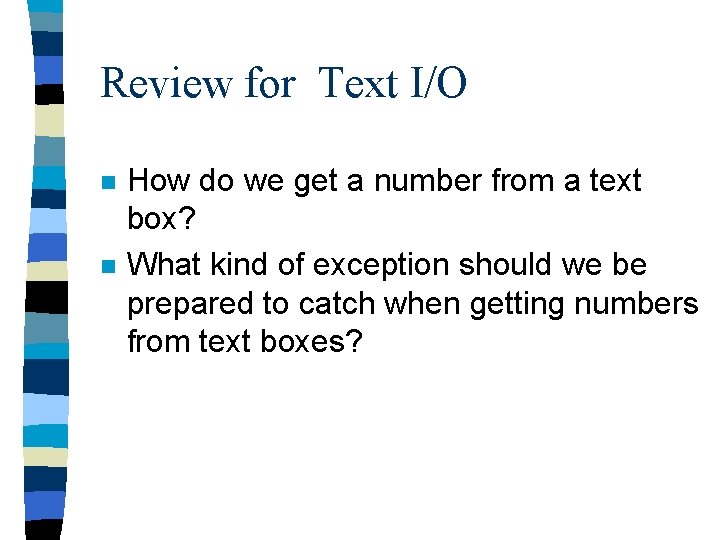
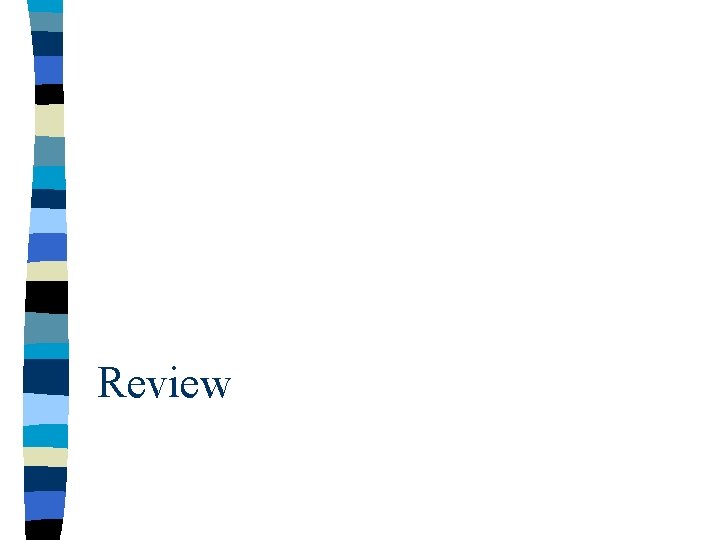
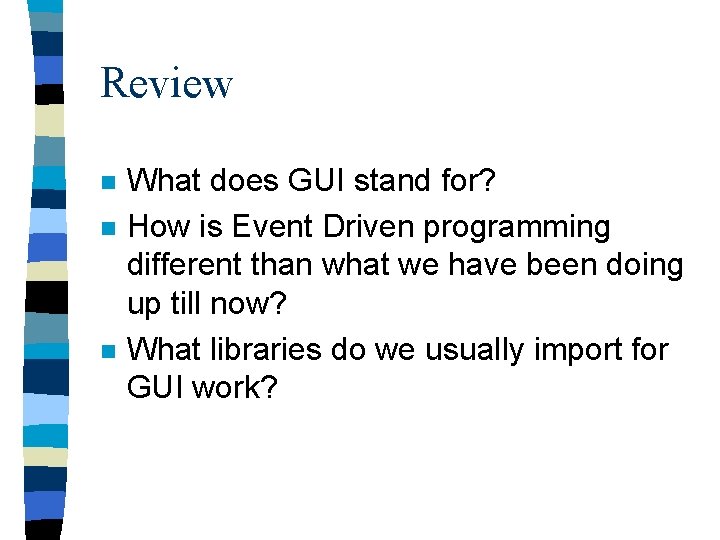
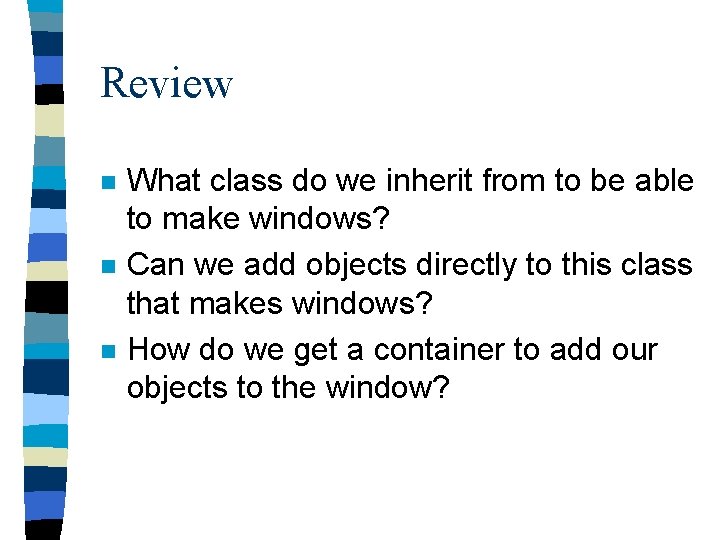
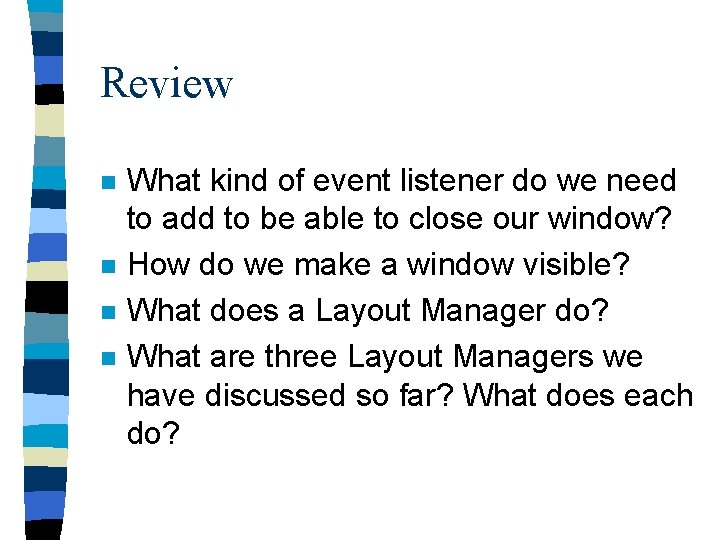
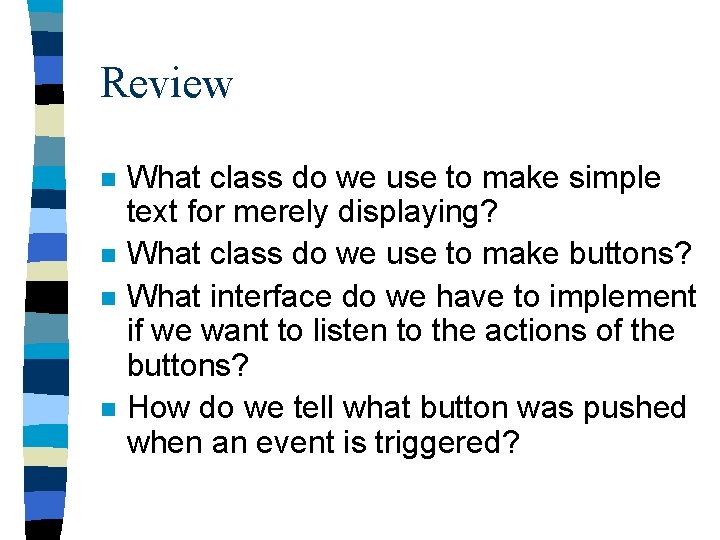
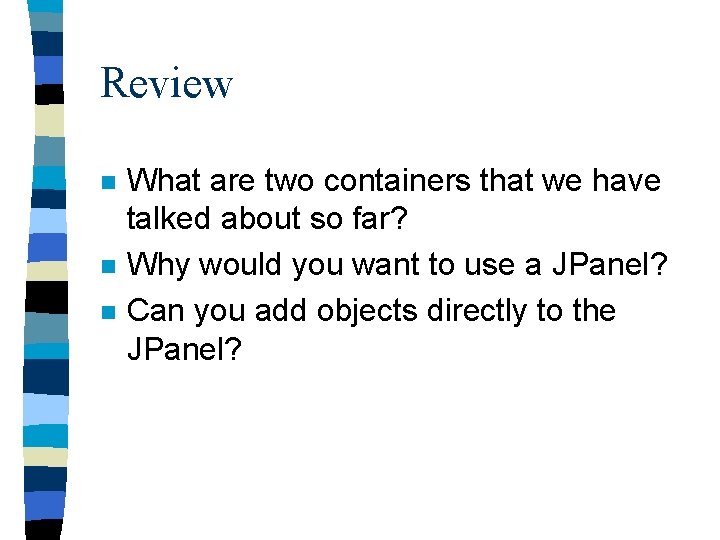
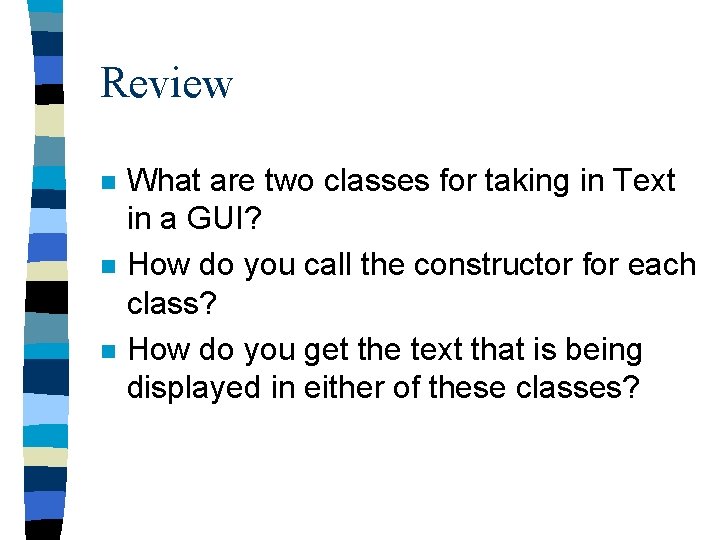
- Slides: 72
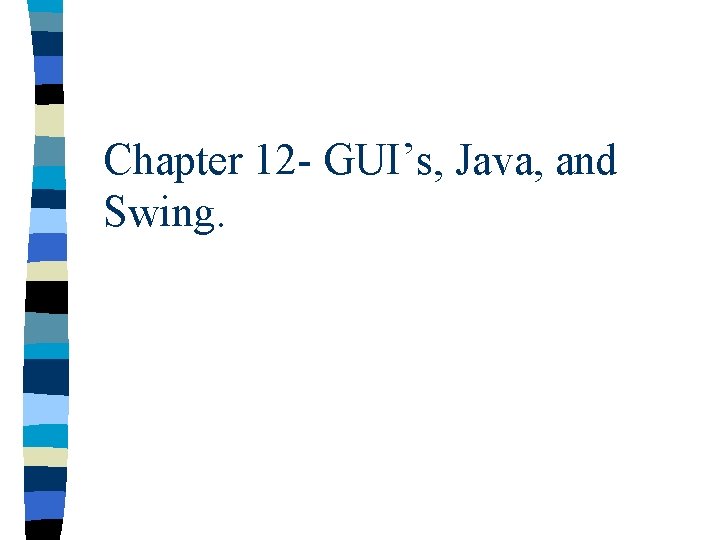
Chapter 12 - GUI’s, Java, and Swing.
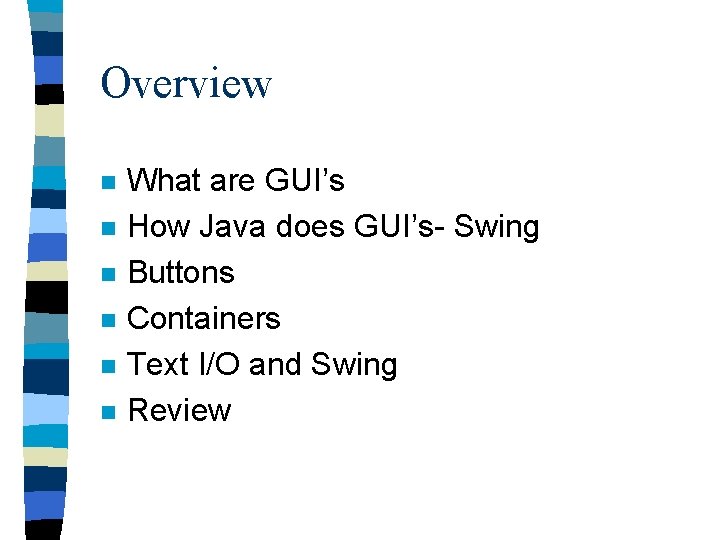
Overview n n n What are GUI’s How Java does GUI’s- Swing Buttons Containers Text I/O and Swing Review
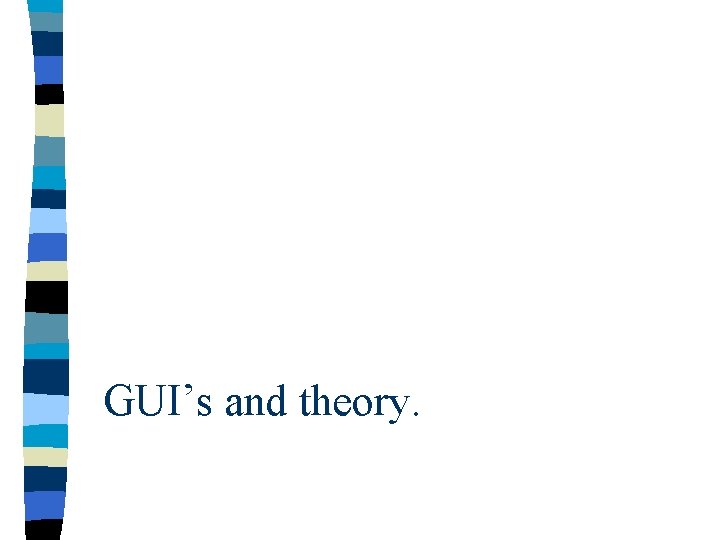
GUI’s and theory.
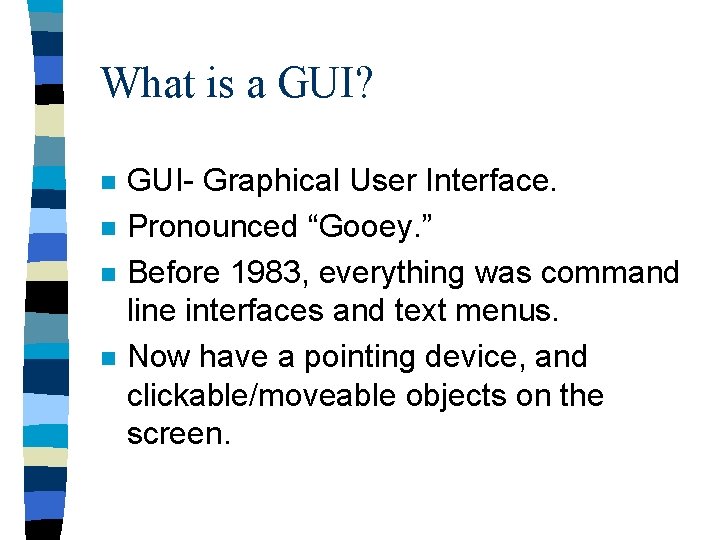
What is a GUI? n n GUI- Graphical User Interface. Pronounced “Gooey. ” Before 1983, everything was command line interfaces and text menus. Now have a pointing device, and clickable/moveable objects on the screen.
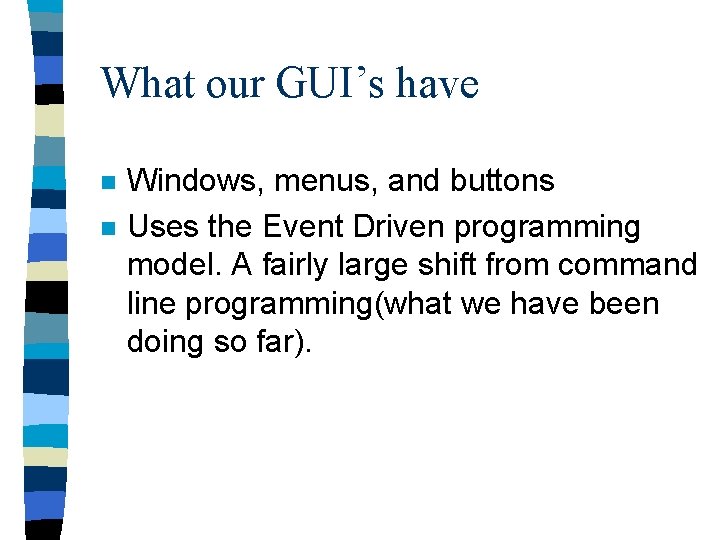
What our GUI’s have n n Windows, menus, and buttons Uses the Event Driven programming model. A fairly large shift from command line programming(what we have been doing so far).
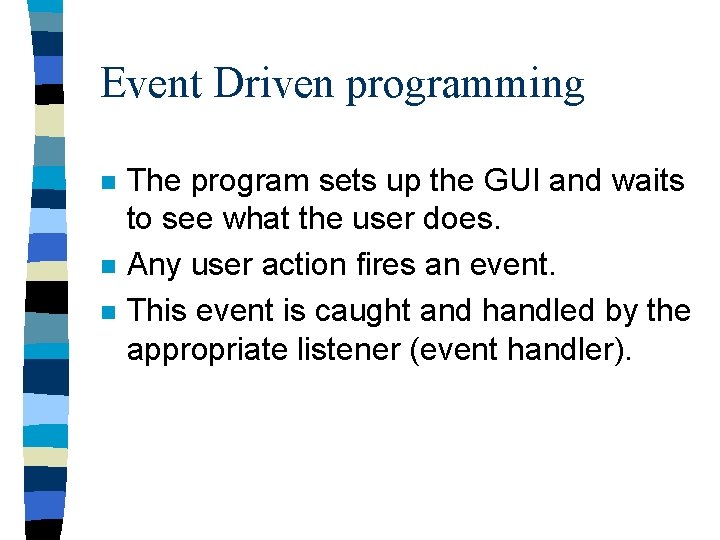
Event Driven programming n n n The program sets up the GUI and waits to see what the user does. Any user action fires an event. This event is caught and handled by the appropriate listener (event handler).
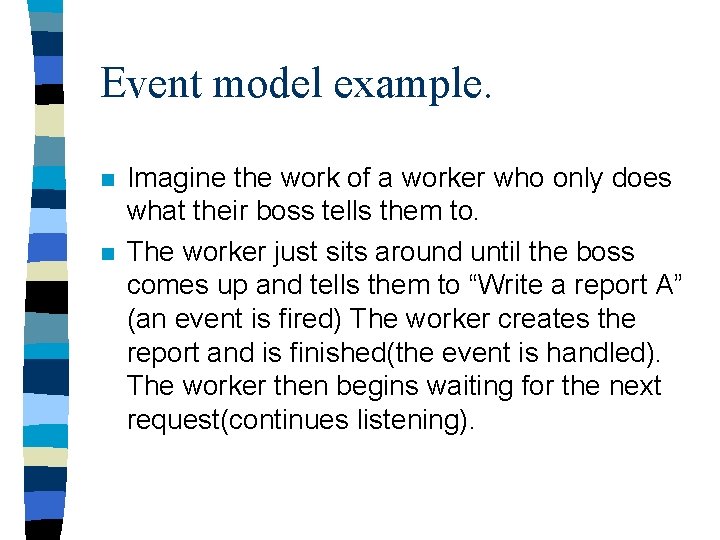
Event model example. n n Imagine the work of a worker who only does what their boss tells them to. The worker just sits around until the boss comes up and tells them to “Write a report A” (an event is fired) The worker creates the report and is finished(the event is handled). The worker then begins waiting for the next request(continues listening).
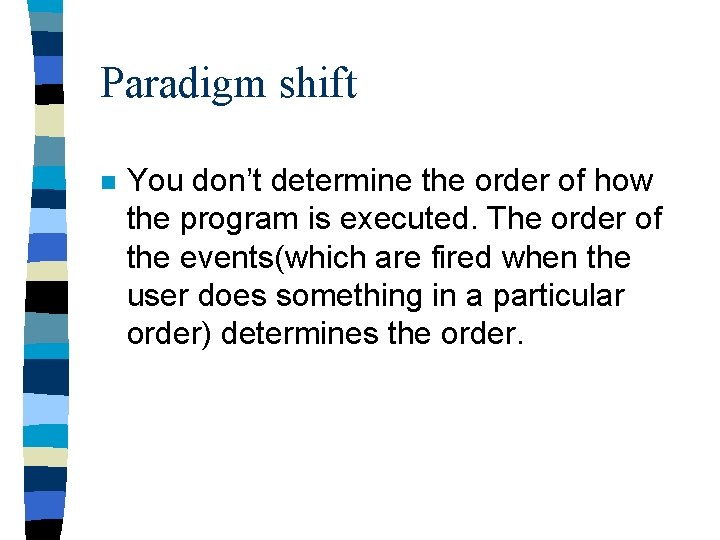
Paradigm shift n You don’t determine the order of how the program is executed. The order of the events(which are fired when the user does something in a particular order) determines the order.
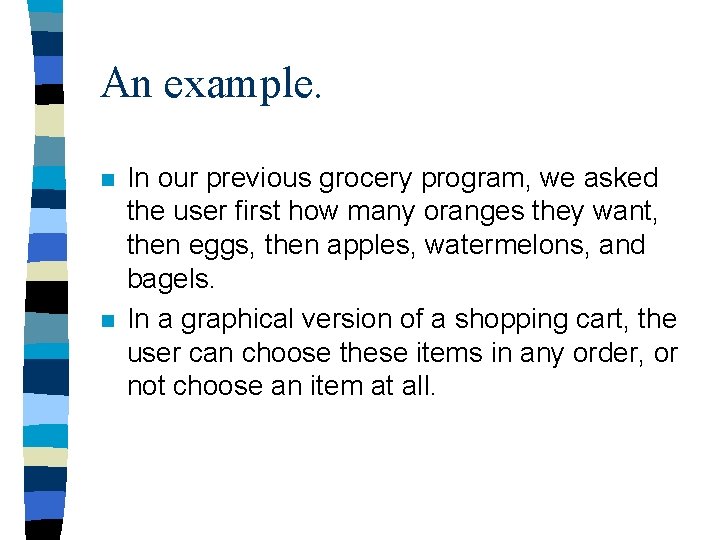
An example. n n In our previous grocery program, we asked the user first how many oranges they want, then eggs, then apples, watermelons, and bagels. In a graphical version of a shopping cart, the user can choose these items in any order, or not choose an item at all.
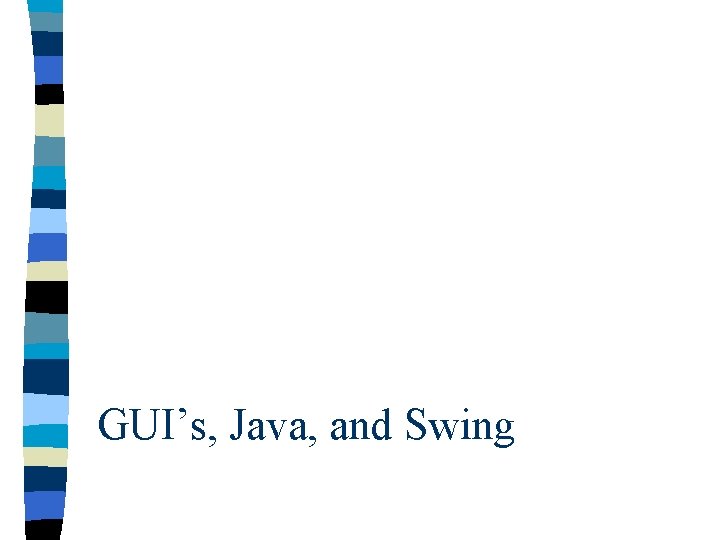
GUI’s, Java, and Swing
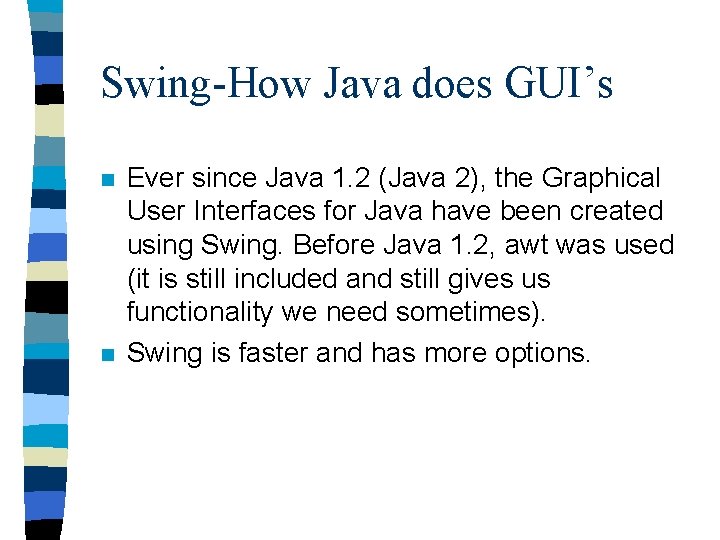
Swing-How Java does GUI’s n n Ever since Java 1. 2 (Java 2), the Graphical User Interfaces for Java have been created using Swing. Before Java 1. 2, awt was used (it is still included and still gives us functionality we need sometimes). Swing is faster and has more options.
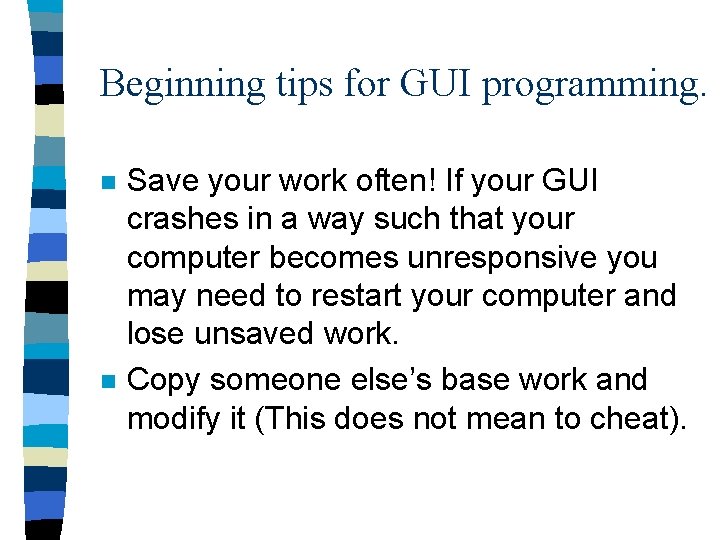
Beginning tips for GUI programming. n n Save your work often! If your GUI crashes in a way such that your computer becomes unresponsive you may need to restart your computer and lose unsaved work. Copy someone else’s base work and modify it (This does not mean to cheat).
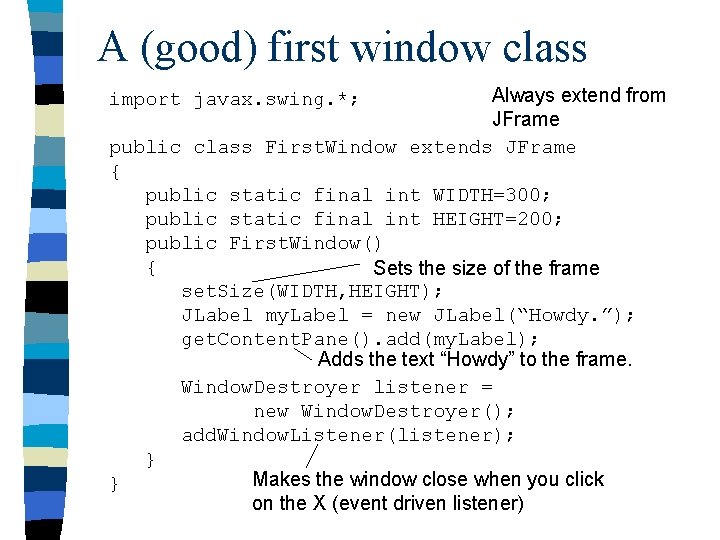
A (good) first window class Always extend from JFrame public class First. Window extends JFrame { public static final int WIDTH=300; public static final int HEIGHT=200; public First. Window() { Sets the size of the frame set. Size(WIDTH, HEIGHT); JLabel my. Label = new JLabel(“Howdy. ”); get. Content. Pane(). add(my. Label); Adds the text “Howdy” to the frame. Window. Destroyer listener = new Window. Destroyer(); add. Window. Listener(listener); } Makes the window close when you click } on the X (event driven listener) import javax. swing. *;
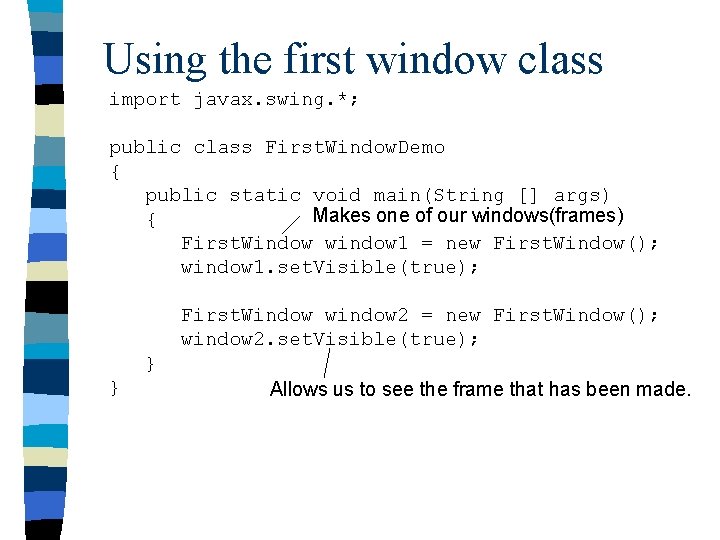
Using the first window class import javax. swing. *; public class First. Window. Demo { public static void main(String [] args) Makes one of our windows(frames) { First. Window window 1 = new First. Window(); window 1. set. Visible(true); First. Window window 2 = new First. Window(); window 2. set. Visible(true); } } Allows us to see the frame that has been made.
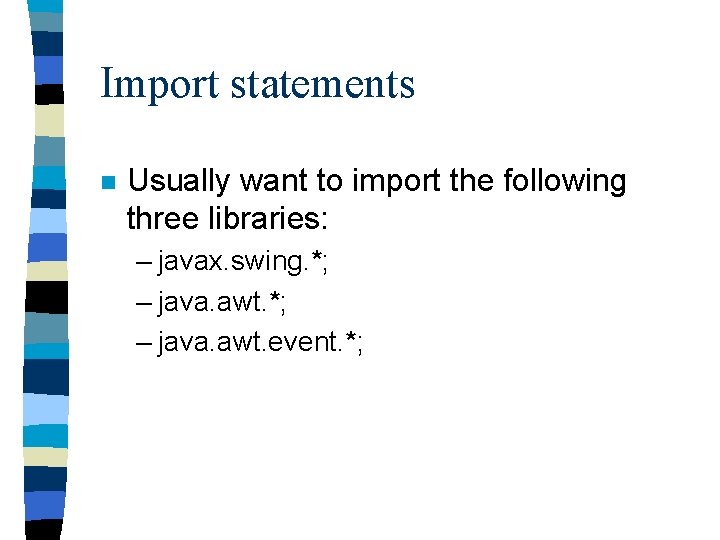
Import statements n Usually want to import the following three libraries: – javax. swing. *; – java. awt. event. *;
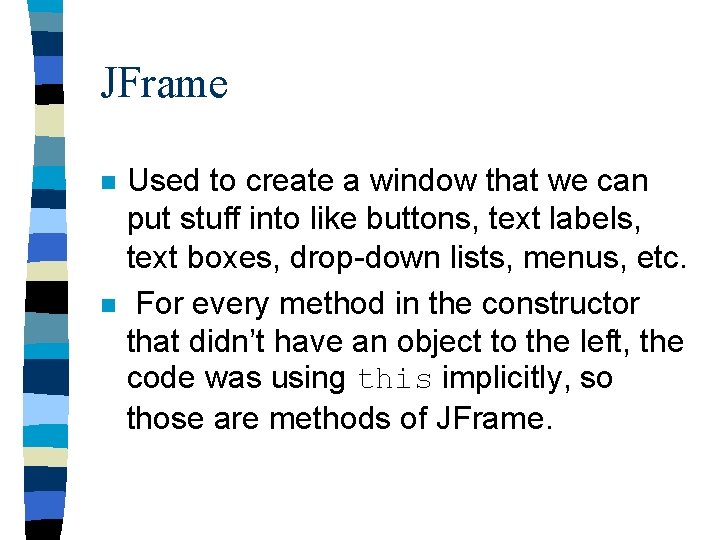
JFrame n n Used to create a window that we can put stuff into like buttons, text labels, text boxes, drop-down lists, menus, etc. For every method in the constructor that didn’t have an object to the left, the code was using this implicitly, so those are methods of JFrame.
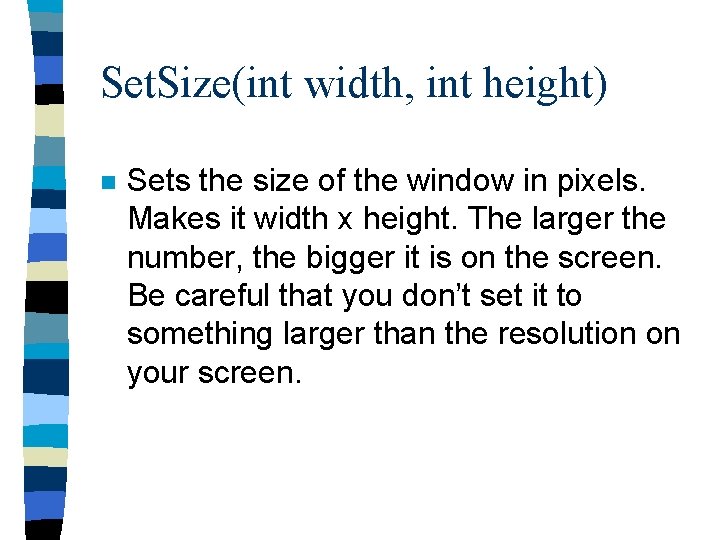
Set. Size(int width, int height) n Sets the size of the window in pixels. Makes it width x height. The larger the number, the bigger it is on the screen. Be careful that you don’t set it to something larger than the resolution on your screen.
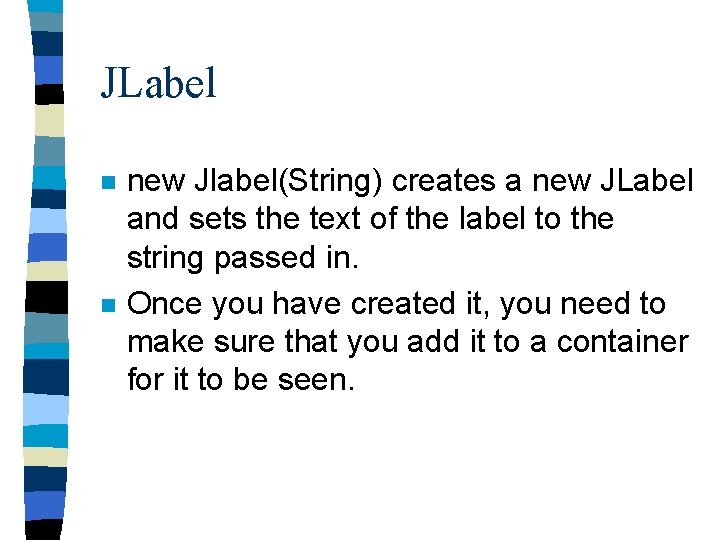
JLabel n n new Jlabel(String) creates a new JLabel and sets the text of the label to the string passed in. Once you have created it, you need to make sure that you add it to a container for it to be seen.
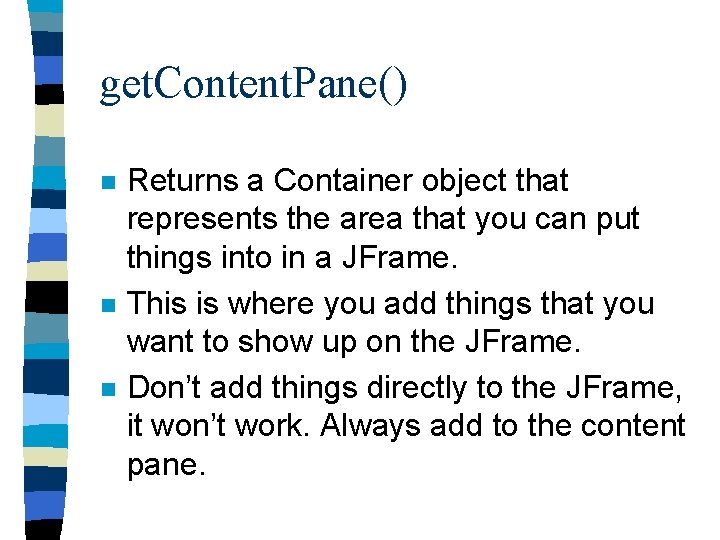
get. Content. Pane() n n n Returns a Container object that represents the area that you can put things into in a JFrame. This is where you add things that you want to show up on the JFrame. Don’t add things directly to the JFrame, it won’t work. Always add to the content pane.
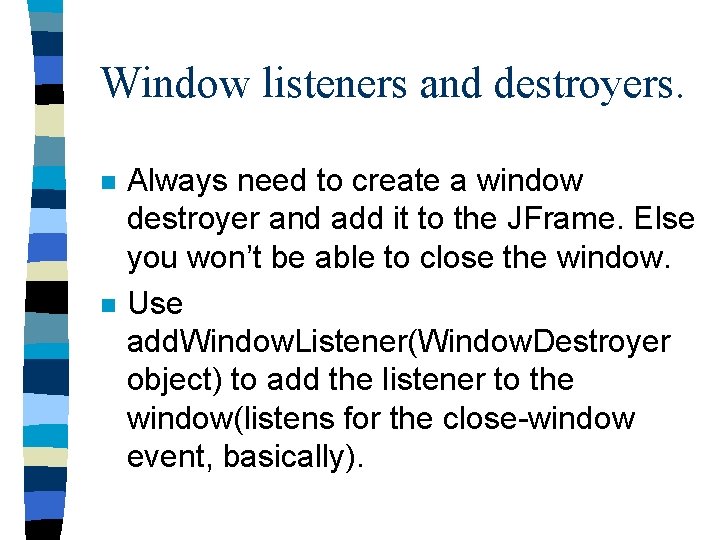
Window listeners and destroyers. n n Always need to create a window destroyer and add it to the JFrame. Else you won’t be able to close the window. Use add. Window. Listener(Window. Destroyer object) to add the listener to the window(listens for the close-window event, basically).
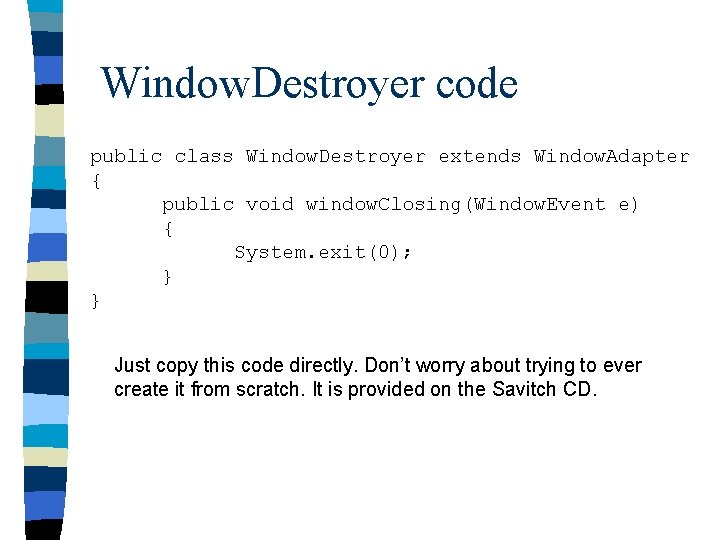
Window. Destroyer code public class Window. Destroyer extends Window. Adapter { public void window. Closing(Window. Event e) { System. exit(0); } } Just copy this code directly. Don’t worry about trying to ever create it from scratch. It is provided on the Savitch CD.
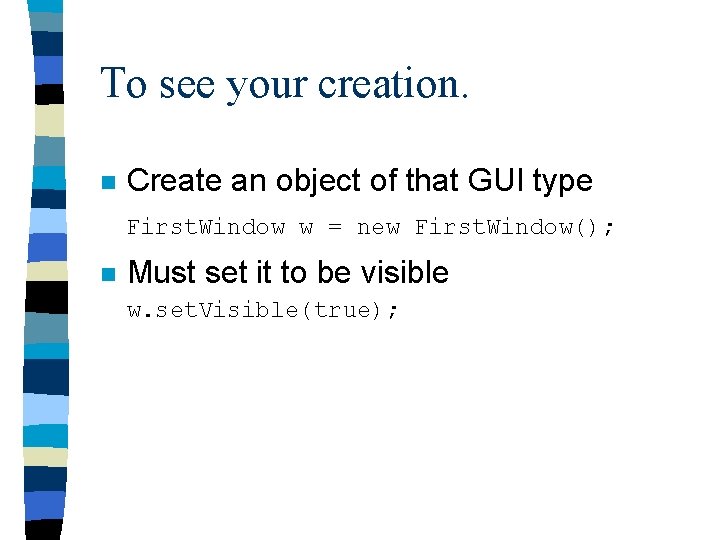
To see your creation. n Create an object of that GUI type First. Window w = new First. Window(); n Must set it to be visible w. set. Visible(true);
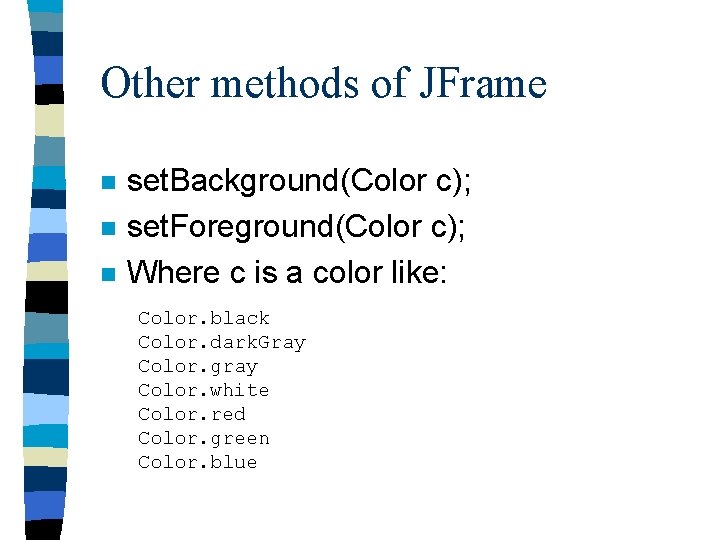
Other methods of JFrame n n n set. Background(Color c); set. Foreground(Color c); Where c is a color like: Color. black Color. dark. Gray Color. gray Color. white Color. red Color. green Color. blue
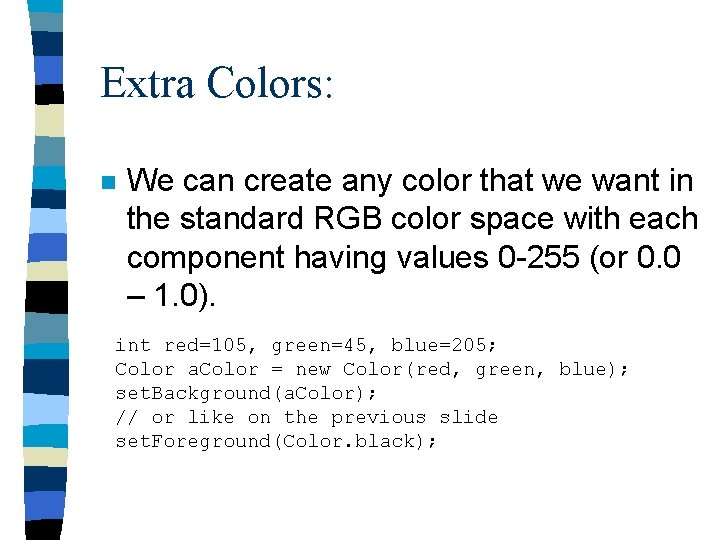
Extra Colors: n We can create any color that we want in the standard RGB color space with each component having values 0 -255 (or 0. 0 – 1. 0). int red=105, green=45, blue=205; Color a. Color = new Color(red, green, blue); set. Background(a. Color); // or like on the previous slide set. Foreground(Color. black);
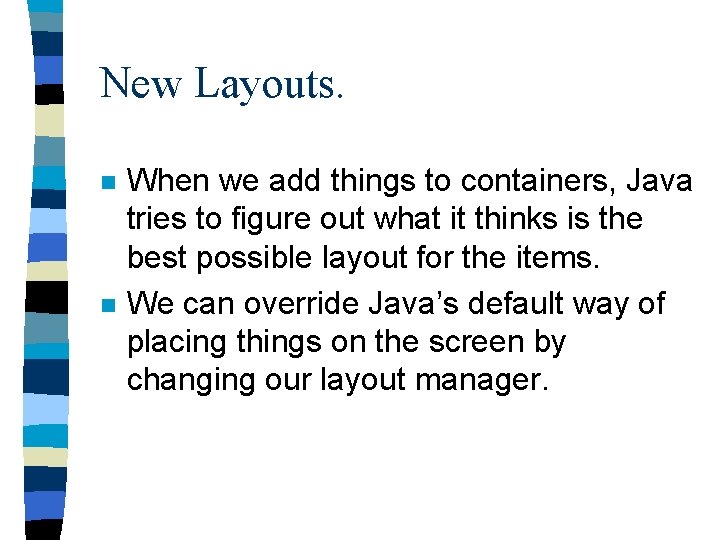
New Layouts. n n When we add things to containers, Java tries to figure out what it thinks is the best possible layout for the items. We can override Java’s default way of placing things on the screen by changing our layout manager.
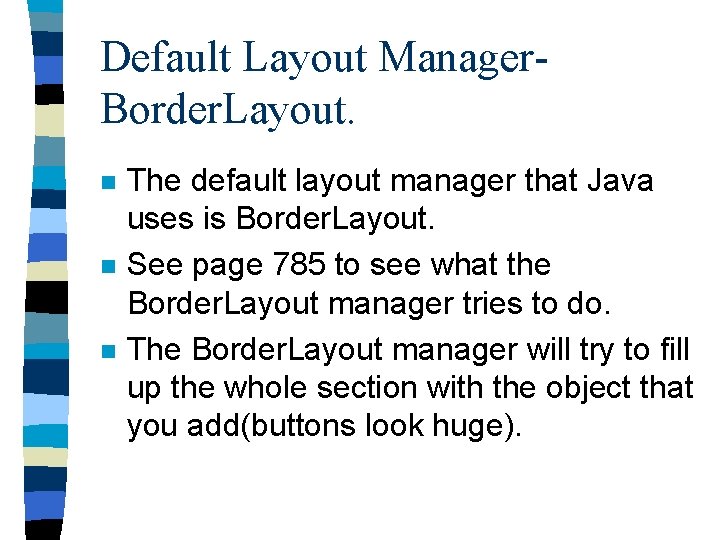
Default Layout Manager. Border. Layout. n n n The default layout manager that Java uses is Border. Layout. See page 785 to see what the Border. Layout manager tries to do. The Border. Layout manager will try to fill up the whole section with the object that you add(buttons look huge).
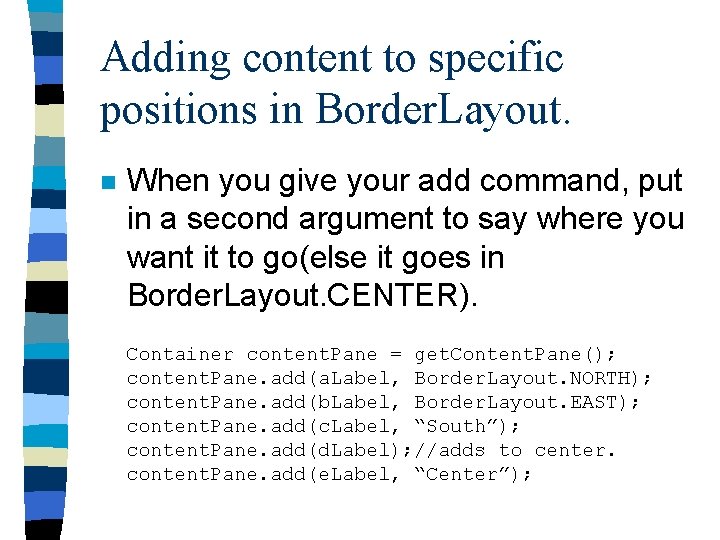
Adding content to specific positions in Border. Layout. n When you give your add command, put in a second argument to say where you want it to go(else it goes in Border. Layout. CENTER). Container content. Pane = get. Content. Pane(); content. Pane. add(a. Label, Border. Layout. NORTH); content. Pane. add(b. Label, Border. Layout. EAST); content. Pane. add(c. Label, “South”); content. Pane. add(d. Label); //adds to center. content. Pane. add(e. Label, “Center”);
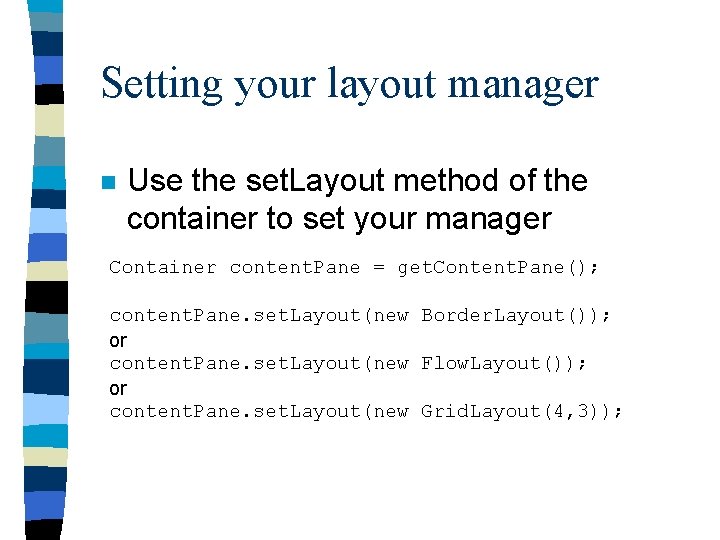
Setting your layout manager n Use the set. Layout method of the container to set your manager Container content. Pane = get. Content. Pane(); content. Pane. set. Layout(new Border. Layout()); or content. Pane. set. Layout(new Flow. Layout()); or content. Pane. set. Layout(new Grid. Layout(4, 3));
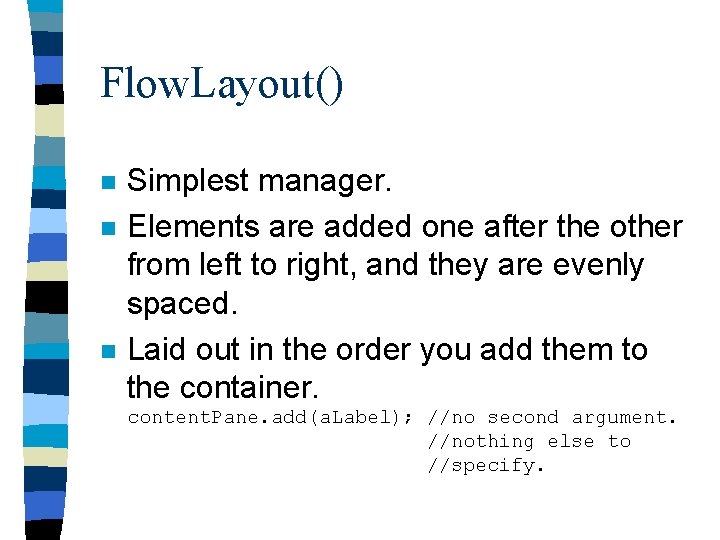
Flow. Layout() n n n Simplest manager. Elements are added one after the other from left to right, and they are evenly spaced. Laid out in the order you add them to the container. content. Pane. add(a. Label); //no second argument. //nothing else to //specify.
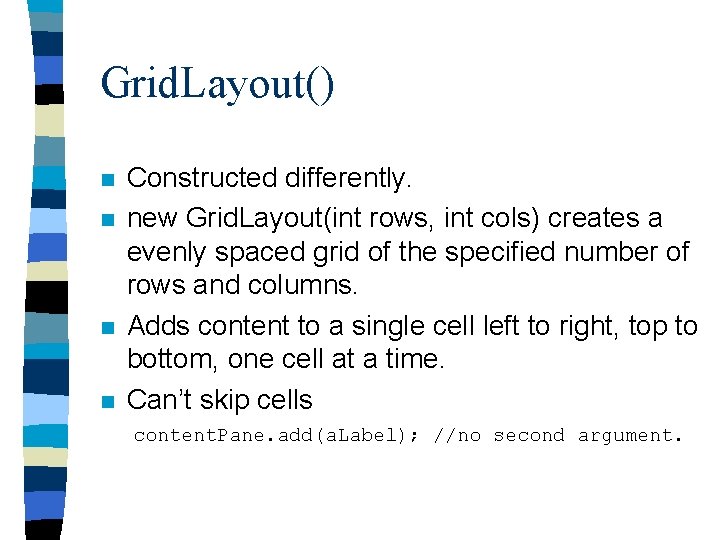
Grid. Layout() n n Constructed differently. new Grid. Layout(int rows, int cols) creates a evenly spaced grid of the specified number of rows and columns. Adds content to a single cell left to right, top to bottom, one cell at a time. Can’t skip cells content. Pane. add(a. Label); //no second argument.
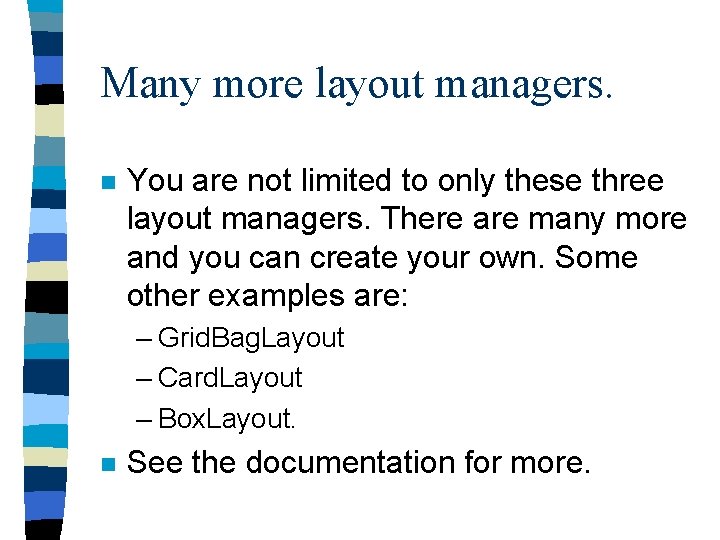
Many more layout managers. n You are not limited to only these three layout managers. There are many more and you can create your own. Some other examples are: – Grid. Bag. Layout – Card. Layout – Box. Layout. n See the documentation for more.
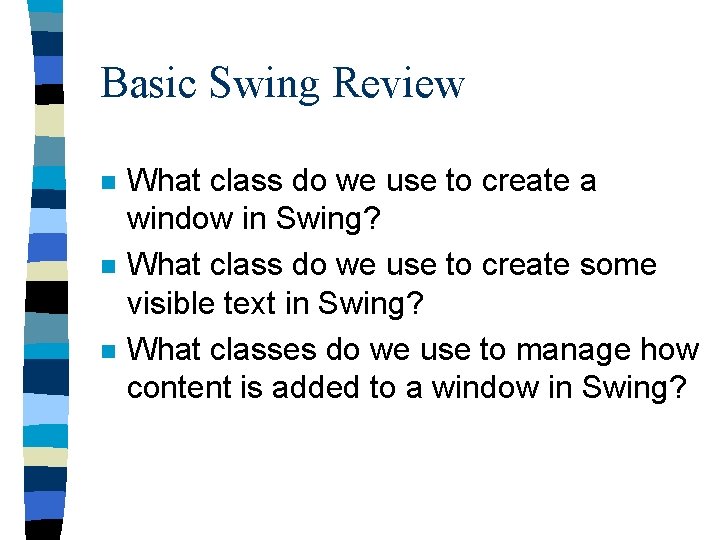
Basic Swing Review n n n What class do we use to create a window in Swing? What class do we use to create some visible text in Swing? What classes do we use to manage how content is added to a window in Swing?
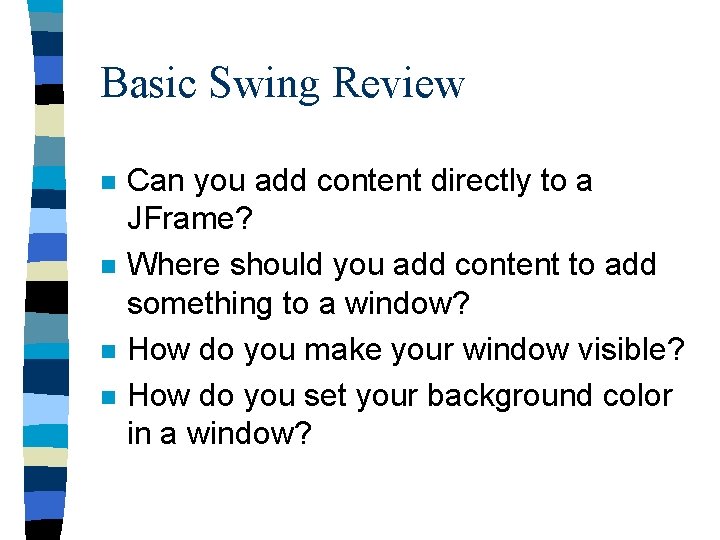
Basic Swing Review n n Can you add content directly to a JFrame? Where should you add content to add something to a window? How do you make your window visible? How do you set your background color in a window?
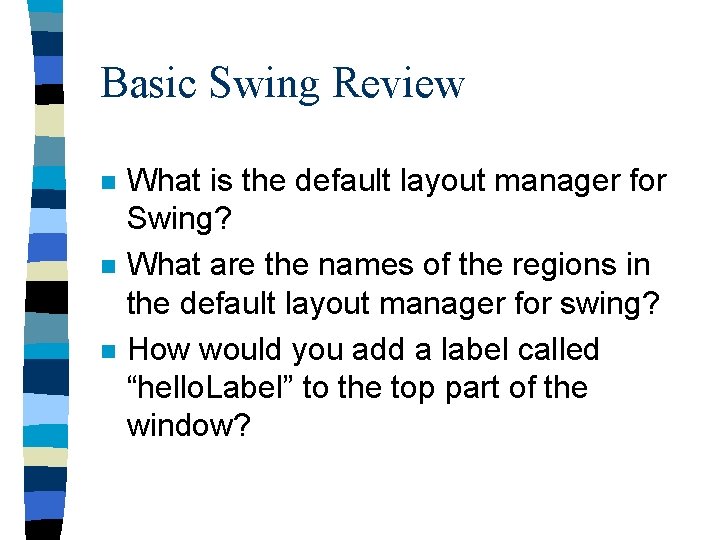
Basic Swing Review n n n What is the default layout manager for Swing? What are the names of the regions in the default layout manager for swing? How would you add a label called “hello. Label” to the top part of the window?
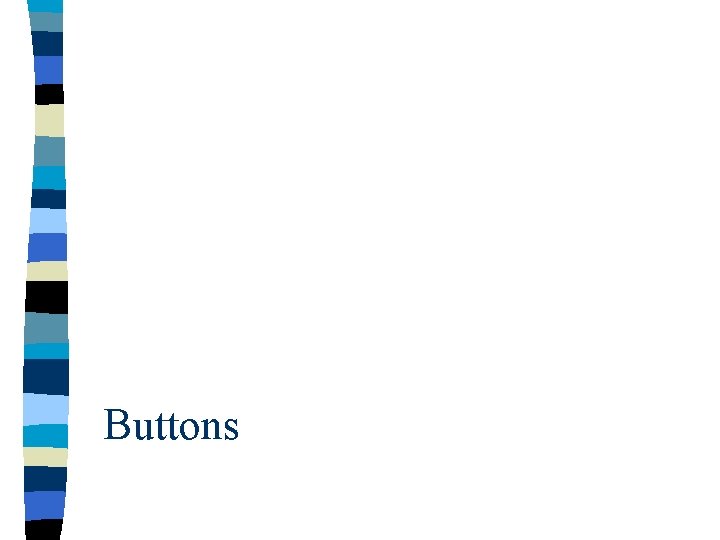
Buttons
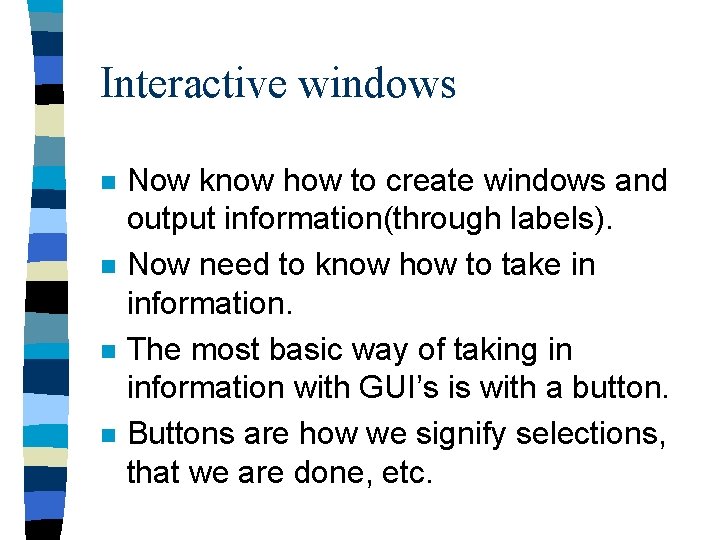
Interactive windows n n Now know how to create windows and output information(through labels). Now need to know how to take in information. The most basic way of taking in information with GUI’s is with a button. Buttons are how we signify selections, that we are done, etc.
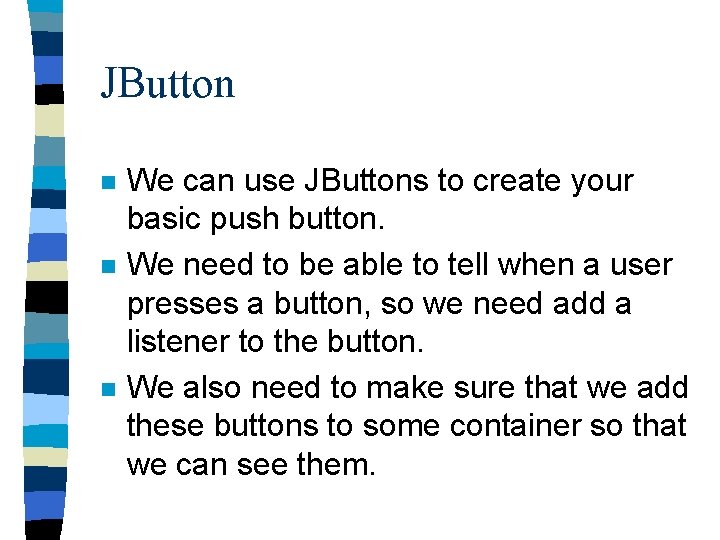
JButton n We can use JButtons to create your basic push button. We need to be able to tell when a user presses a button, so we need add a listener to the button. We also need to make sure that we add these buttons to some container so that we can see them.
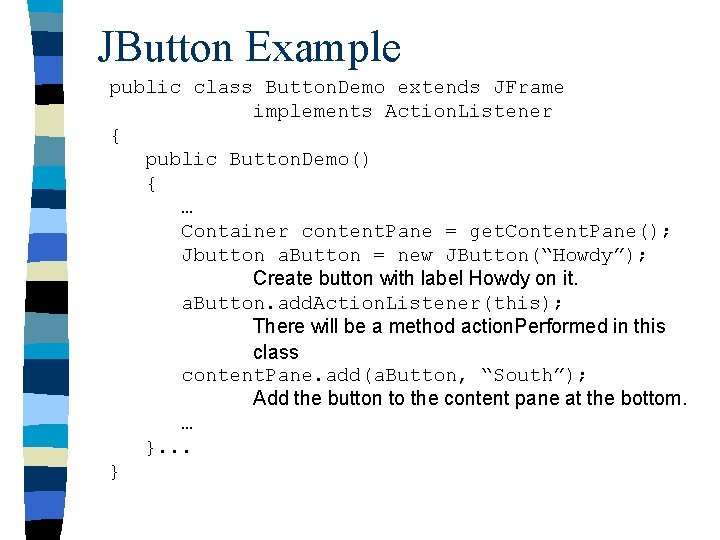
JButton Example public class Button. Demo extends JFrame implements Action. Listener { public Button. Demo() { … Container content. Pane = get. Content. Pane(); Jbutton a. Button = new JButton(“Howdy”); Create button with label Howdy on it. a. Button. add. Action. Listener(this); There will be a method action. Performed in this class content. Pane. add(a. Button, “South”); Add the button to the content pane at the bottom. … }. . . }
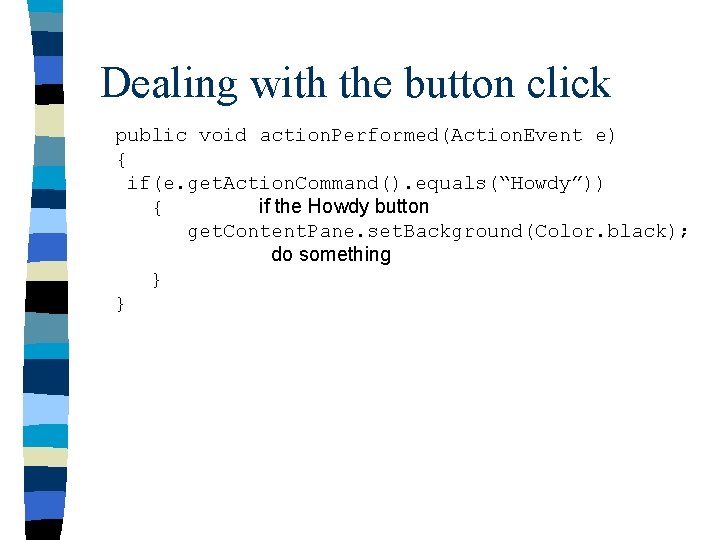
Dealing with the button click public void action. Performed(Action. Event e) { if(e. get. Action. Command(). equals(“Howdy”)) { if the Howdy button get. Content. Pane. set. Background(Color. black); do something } }
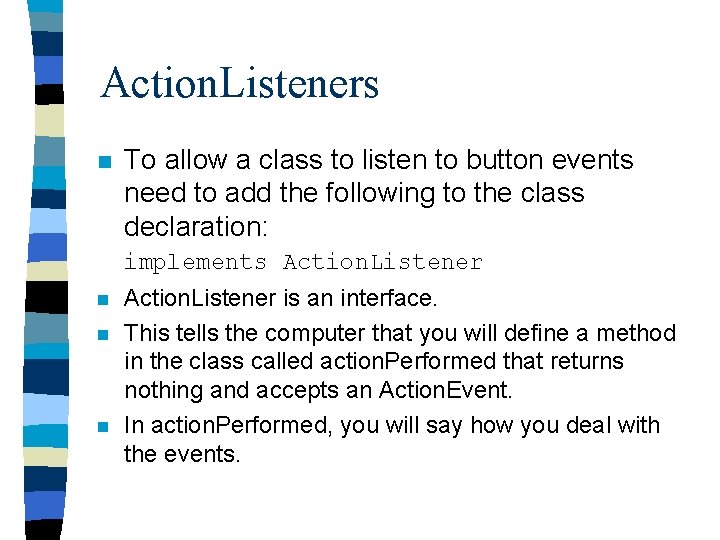
Action. Listeners n To allow a class to listen to button events need to add the following to the class declaration: implements Action. Listener n n n Action. Listener is an interface. This tells the computer that you will define a method in the class called action. Performed that returns nothing and accepts an Action. Event. In action. Performed, you will say how you deal with the events.
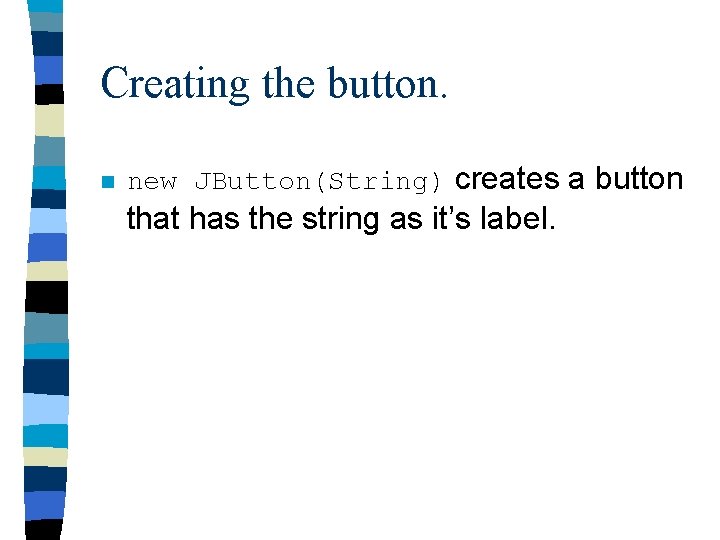
Creating the button. n new JButton(String) creates a button that has the string as it’s label.
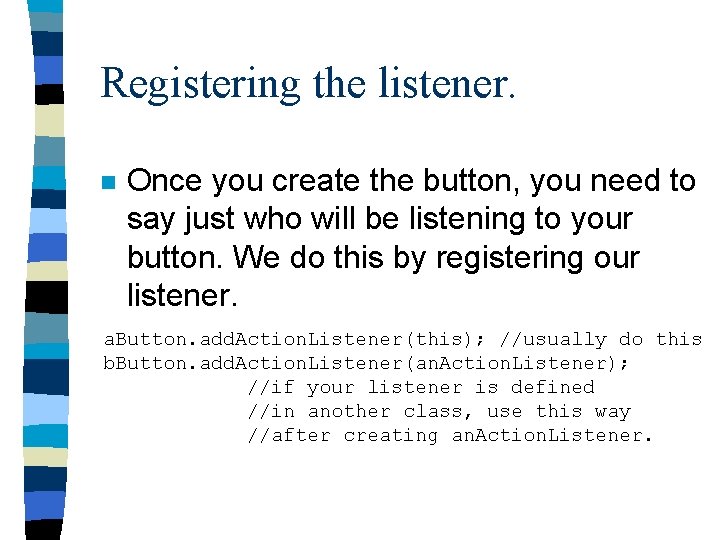
Registering the listener. n Once you create the button, you need to say just who will be listening to your button. We do this by registering our listener. a. Button. add. Action. Listener(this); //usually do this b. Button. add. Action. Listener(an. Action. Listener); //if your listener is defined //in another class, use this way //after creating an. Action. Listener.
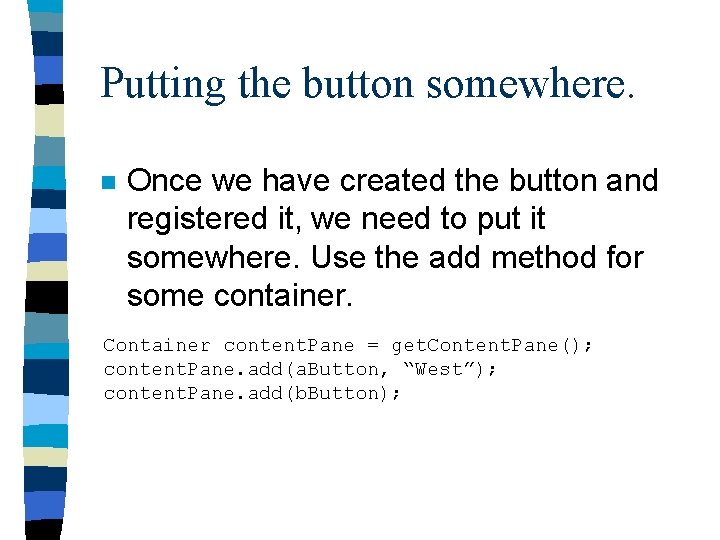
Putting the button somewhere. n Once we have created the button and registered it, we need to put it somewhere. Use the add method for some container. Container content. Pane = get. Content. Pane(); content. Pane. add(a. Button, “West”); content. Pane. add(b. Button);
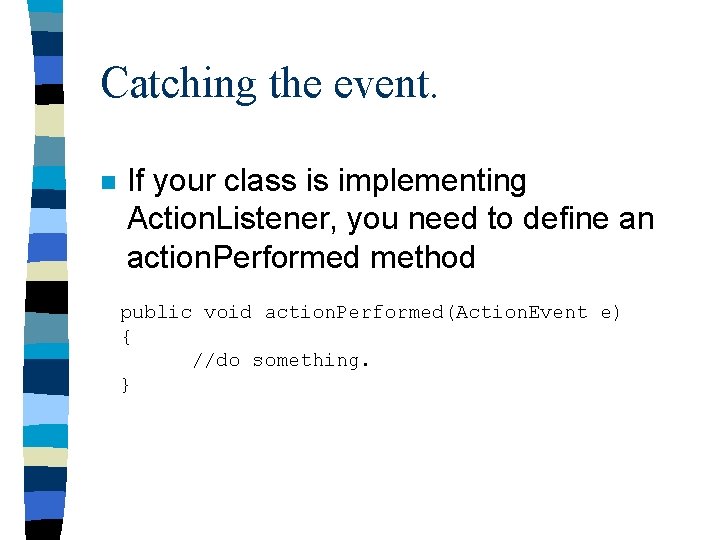
Catching the event. n If your class is implementing Action. Listener, you need to define an action. Performed method public void action. Performed(Action. Event e) { //do something. }
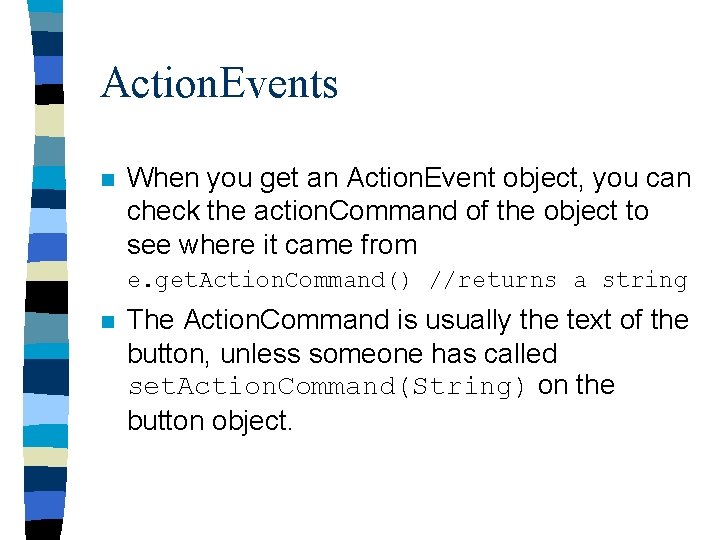
Action. Events n When you get an Action. Event object, you can check the action. Command of the object to see where it came from e. get. Action. Command() //returns a string n The Action. Command is usually the text of the button, unless someone has called set. Action. Command(String) on the button object.
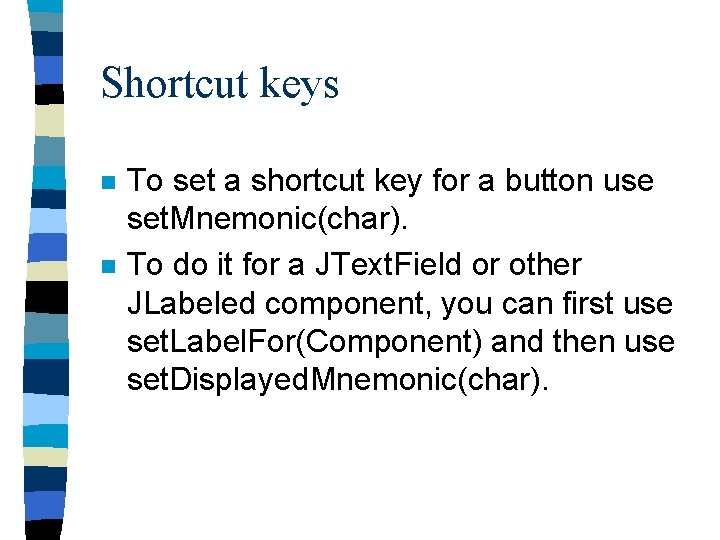
Shortcut keys n n To set a shortcut key for a button use set. Mnemonic(char). To do it for a JText. Field or other JLabeled component, you can first use set. Label. For(Component) and then use set. Displayed. Mnemonic(char).
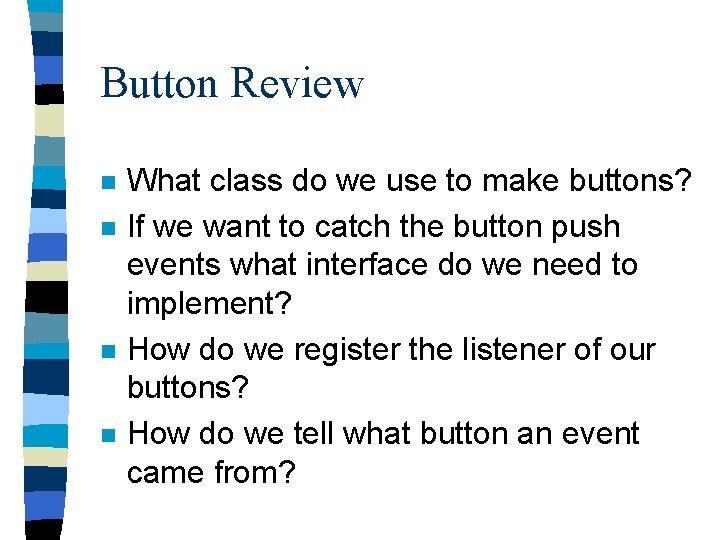
Button Review n n What class do we use to make buttons? If we want to catch the button push events what interface do we need to implement? How do we register the listener of our buttons? How do we tell what button an event came from?
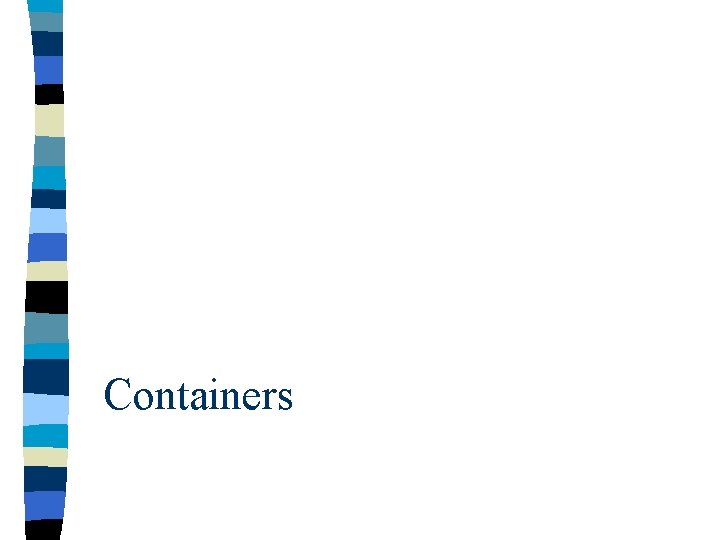
Containers
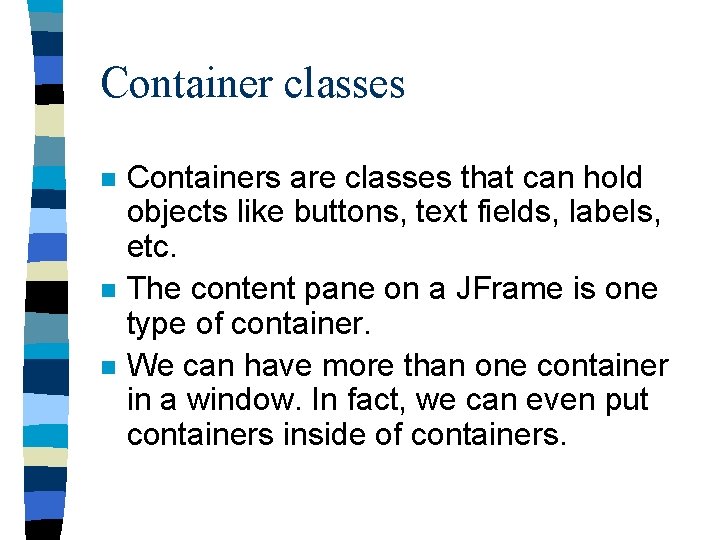
Container classes n n n Containers are classes that can hold objects like buttons, text fields, labels, etc. The content pane on a JFrame is one type of container. We can have more than one container in a window. In fact, we can even put containers inside of containers.
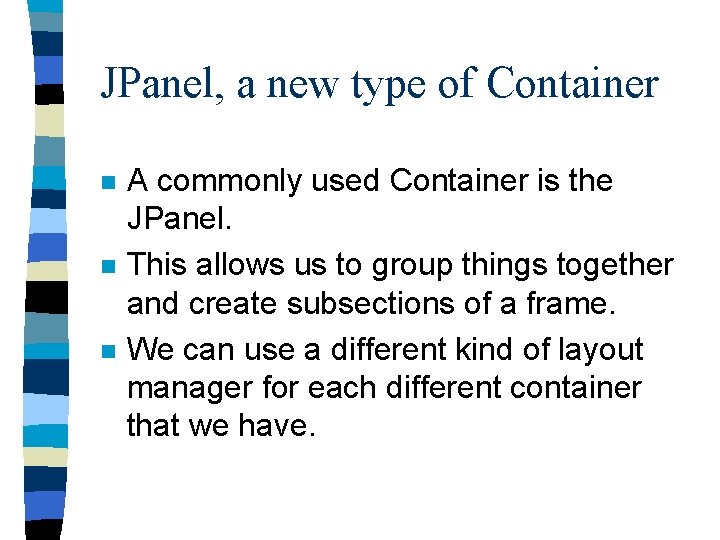
JPanel, a new type of Container n n n A commonly used Container is the JPanel. This allows us to group things together and create subsections of a frame. We can use a different kind of layout manager for each different container that we have.
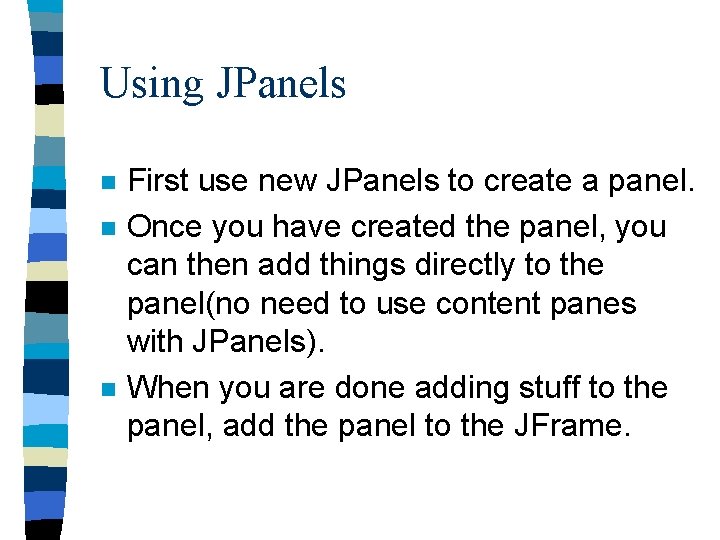
Using JPanels n n n First use new JPanels to create a panel. Once you have created the panel, you can then add things directly to the panel(no need to use content panes with JPanels). When you are done adding stuff to the panel, add the panel to the JFrame.
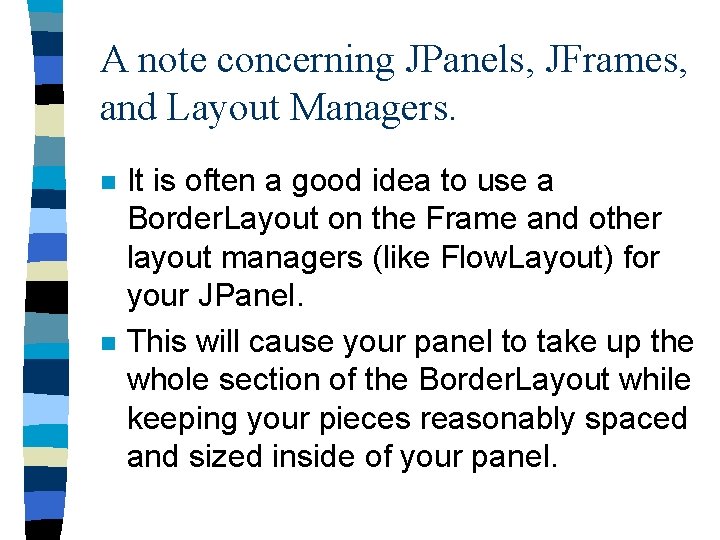
A note concerning JPanels, JFrames, and Layout Managers. n n It is often a good idea to use a Border. Layout on the Frame and other layout managers (like Flow. Layout) for your JPanel. This will cause your panel to take up the whole section of the Border. Layout while keeping your pieces reasonably spaced and sized inside of your panel.
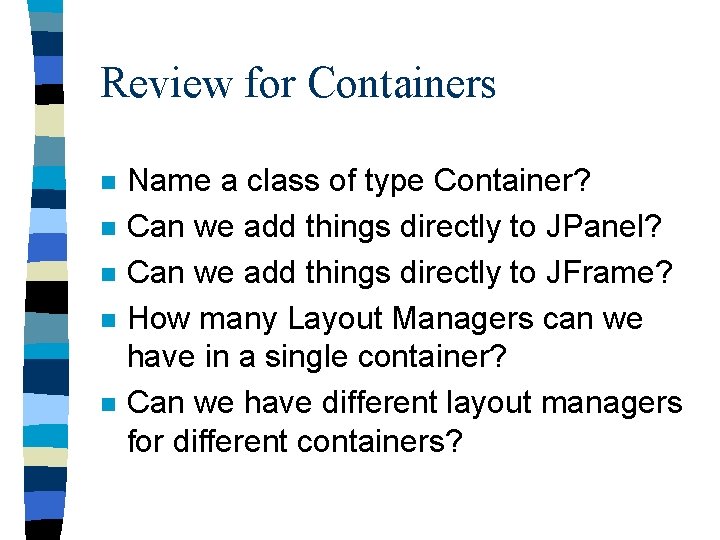
Review for Containers n n n Name a class of type Container? Can we add things directly to JPanel? Can we add things directly to JFrame? How many Layout Managers can we have in a single container? Can we have different layout managers for different containers?
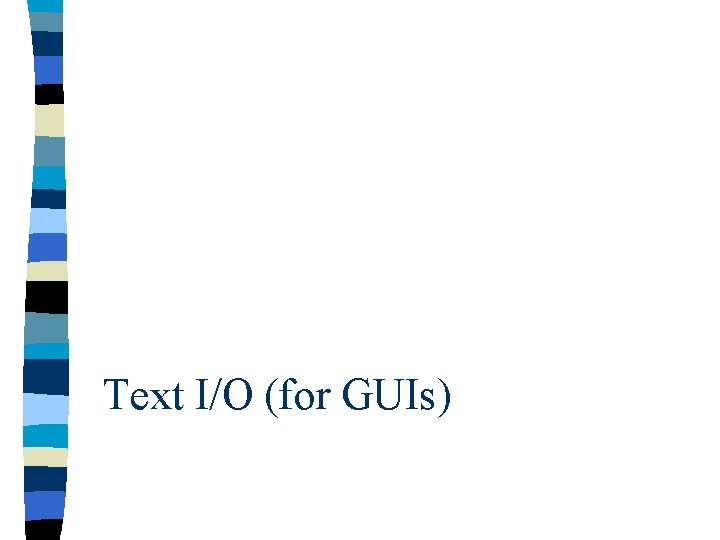
Text I/O (for GUIs)
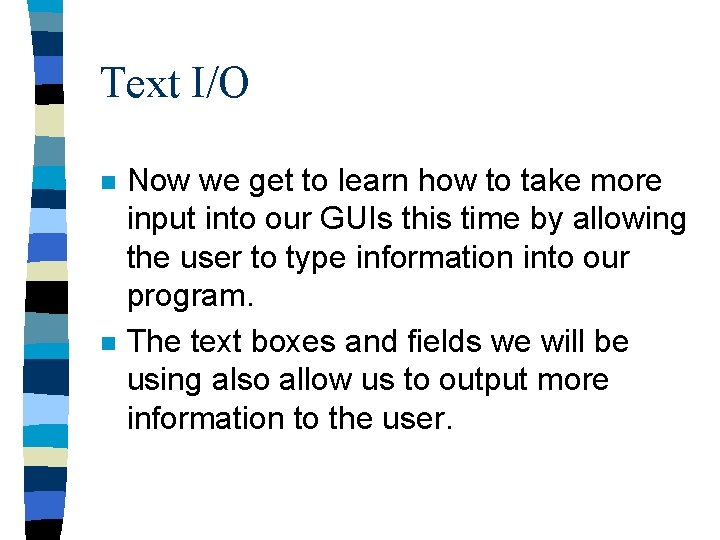
Text I/O n n Now we get to learn how to take more input into our GUIs this time by allowing the user to type information into our program. The text boxes and fields we will be using also allow us to output more information to the user.
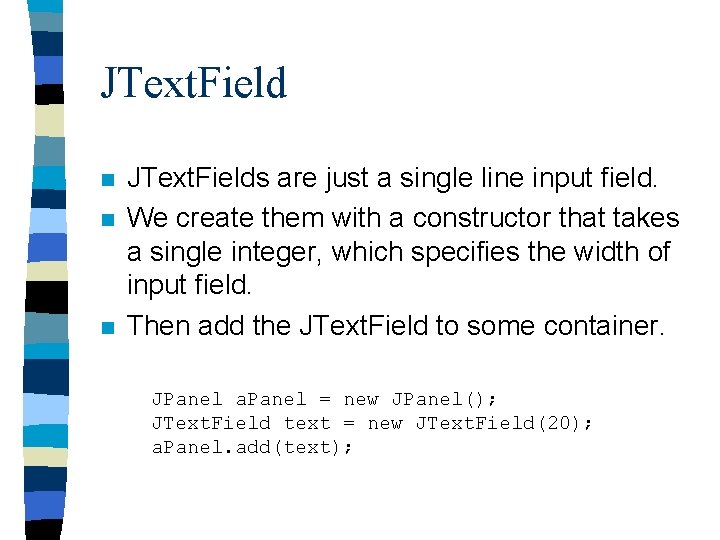
JText. Field n n n JText. Fields are just a single line input field. We create them with a constructor that takes a single integer, which specifies the width of input field. Then add the JText. Field to some container. JPanel a. Panel = new JPanel(); JText. Field text = new JText. Field(20); a. Panel. add(text);
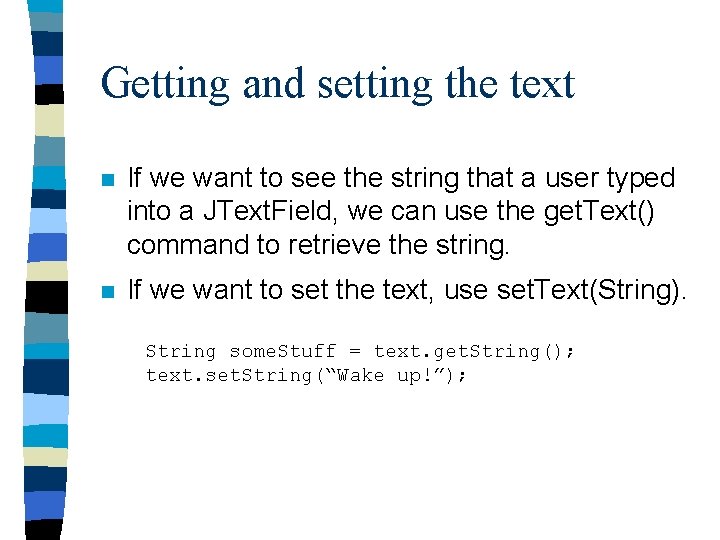
Getting and setting the text n If we want to see the string that a user typed into a JText. Field, we can use the get. Text() command to retrieve the string. n If we want to set the text, use set. Text(String). String some. Stuff = text. get. String(); text. set. String(“Wake up!”);
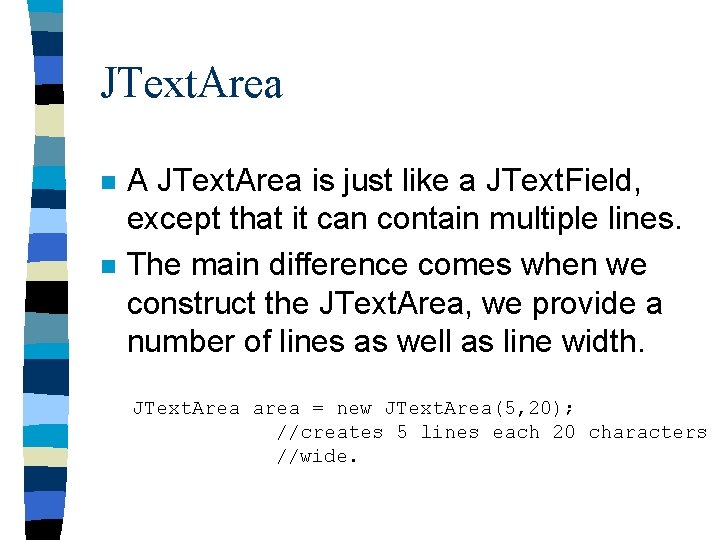
JText. Area n n A JText. Area is just like a JText. Field, except that it can contain multiple lines. The main difference comes when we construct the JText. Area, we provide a number of lines as well as line width. JText. Area area = new JText. Area(5, 20); //creates 5 lines each 20 characters //wide.
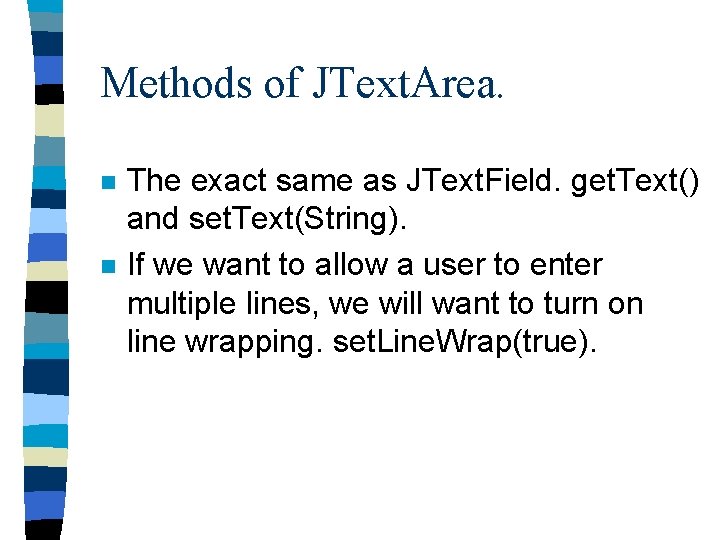
Methods of JText. Area. n n The exact same as JText. Field. get. Text() and set. Text(String). If we want to allow a user to enter multiple lines, we will want to turn on line wrapping. set. Line. Wrap(true).
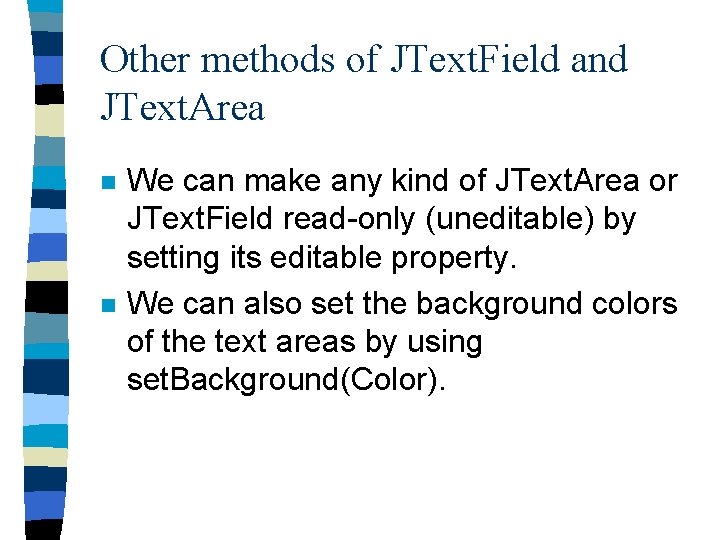
Other methods of JText. Field and JText. Area n n We can make any kind of JText. Area or JText. Field read-only (uneditable) by setting its editable property. We can also set the background colors of the text areas by using set. Background(Color).
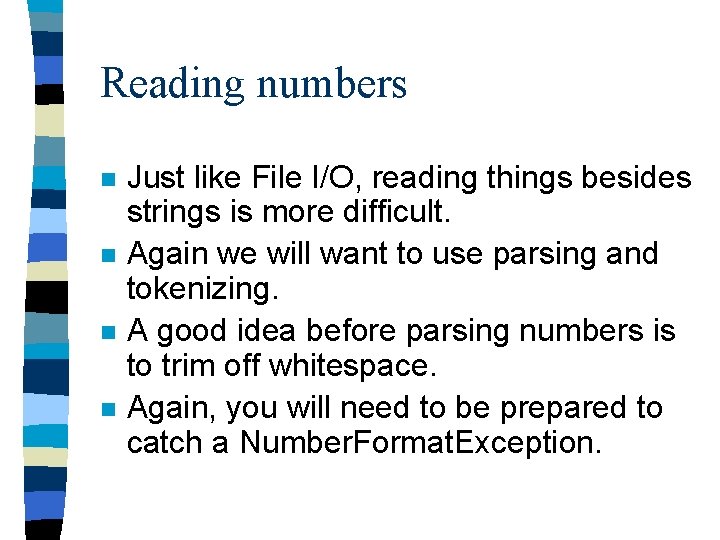
Reading numbers n n Just like File I/O, reading things besides strings is more difficult. Again we will want to use parsing and tokenizing. A good idea before parsing numbers is to trim off whitespace. Again, you will need to be prepared to catch a Number. Format. Exception.
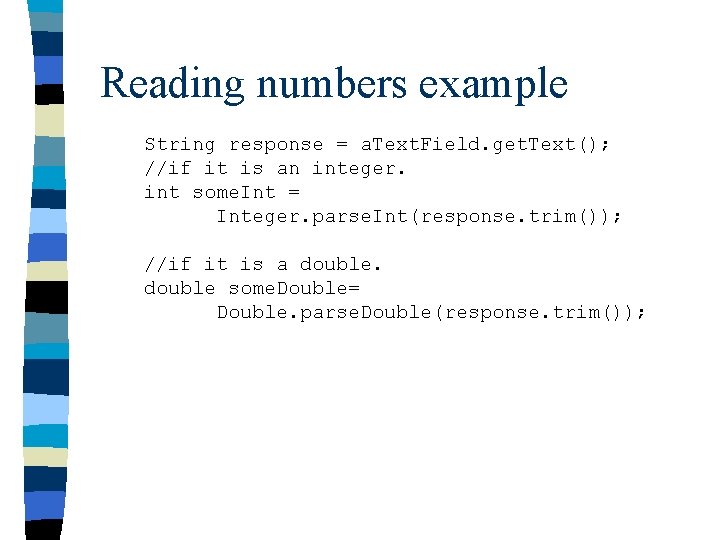
Reading numbers example String response = a. Text. Field. get. Text(); //if it is an integer. int some. Int = Integer. parse. Int(response. trim()); //if it is a double some. Double= Double. parse. Double(response. trim());
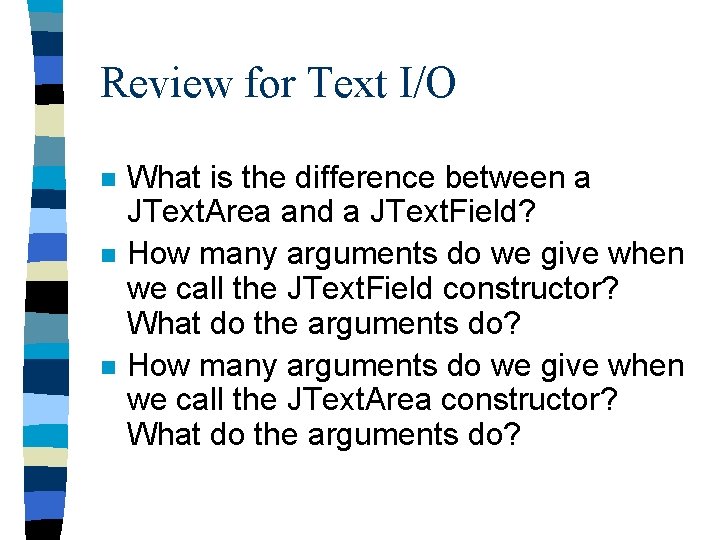
Review for Text I/O n n n What is the difference between a JText. Area and a JText. Field? How many arguments do we give when we call the JText. Field constructor? What do the arguments do? How many arguments do we give when we call the JText. Area constructor? What do the arguments do?
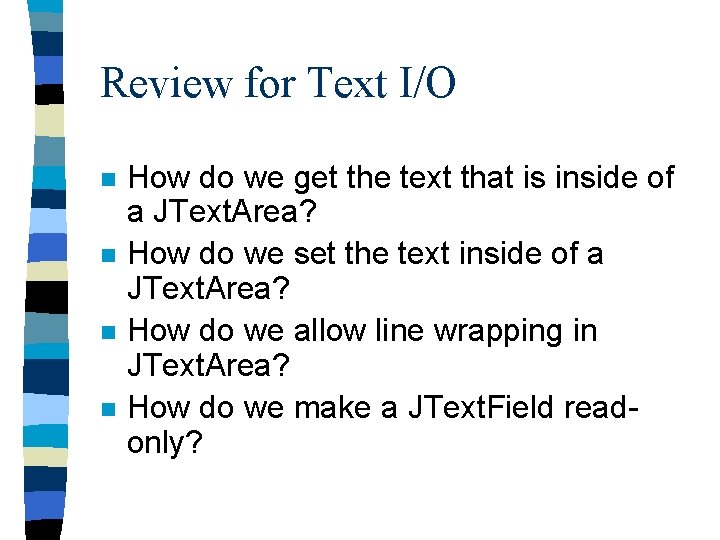
Review for Text I/O n n How do we get the text that is inside of a JText. Area? How do we set the text inside of a JText. Area? How do we allow line wrapping in JText. Area? How do we make a JText. Field readonly?
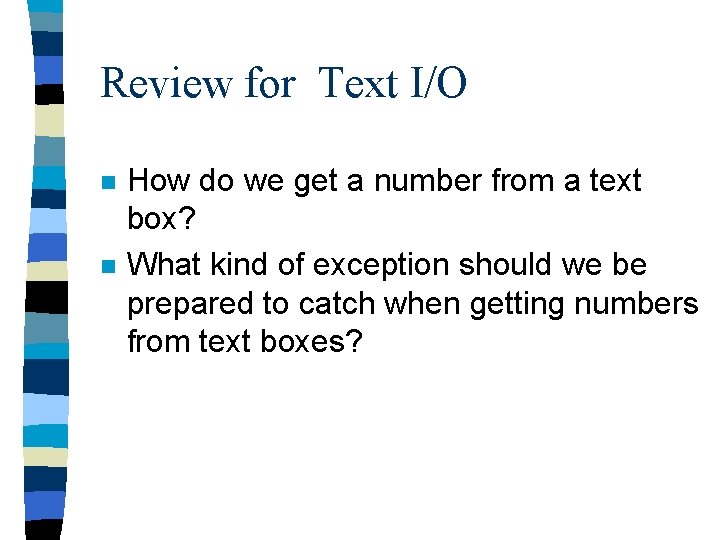
Review for Text I/O n n How do we get a number from a text box? What kind of exception should we be prepared to catch when getting numbers from text boxes?
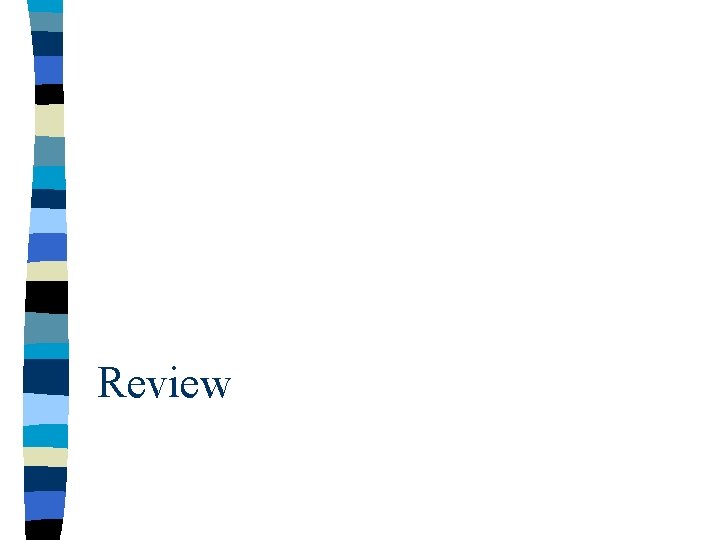
Review
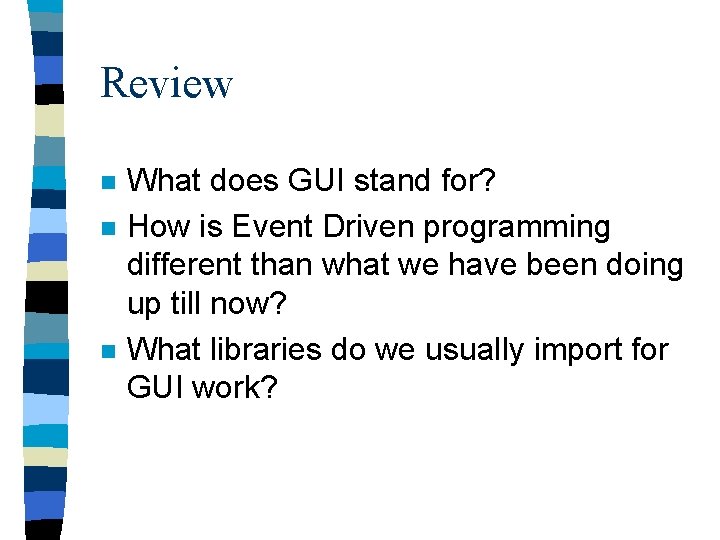
Review n n n What does GUI stand for? How is Event Driven programming different than what we have been doing up till now? What libraries do we usually import for GUI work?
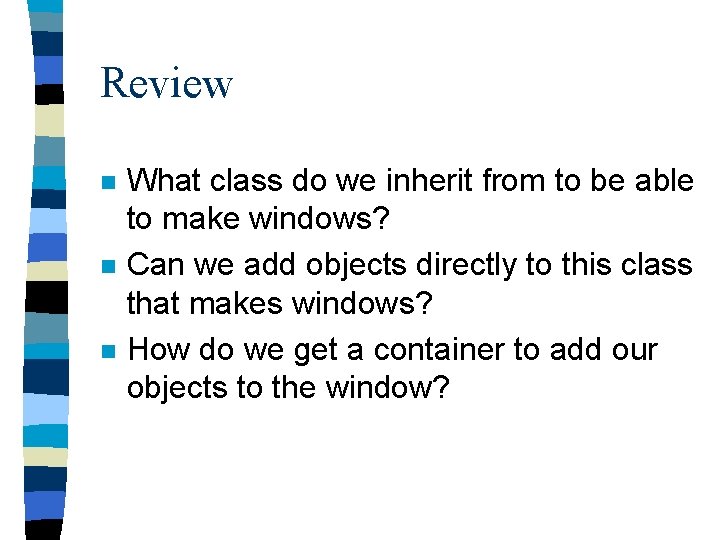
Review n n n What class do we inherit from to be able to make windows? Can we add objects directly to this class that makes windows? How do we get a container to add our objects to the window?
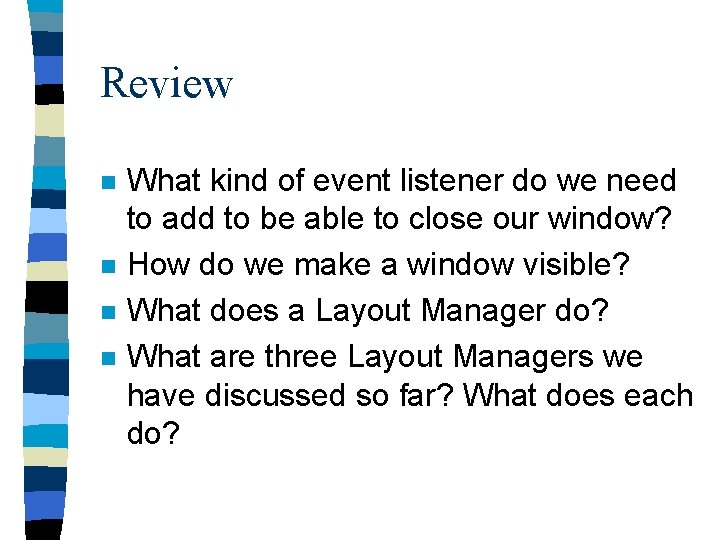
Review n n What kind of event listener do we need to add to be able to close our window? How do we make a window visible? What does a Layout Manager do? What are three Layout Managers we have discussed so far? What does each do?
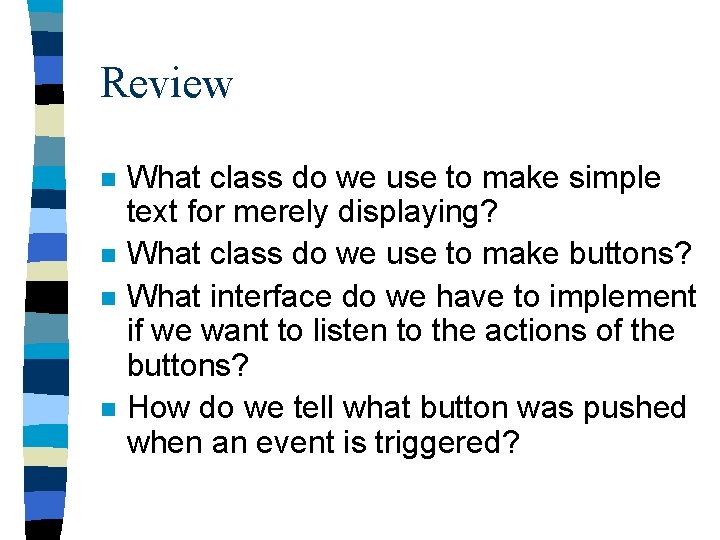
Review n n What class do we use to make simple text for merely displaying? What class do we use to make buttons? What interface do we have to implement if we want to listen to the actions of the buttons? How do we tell what button was pushed when an event is triggered?
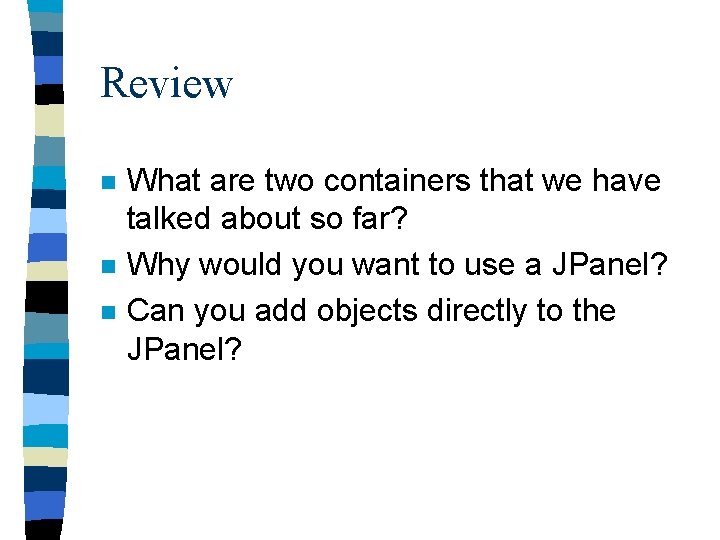
Review n n n What are two containers that we have talked about so far? Why would you want to use a JPanel? Can you add objects directly to the JPanel?
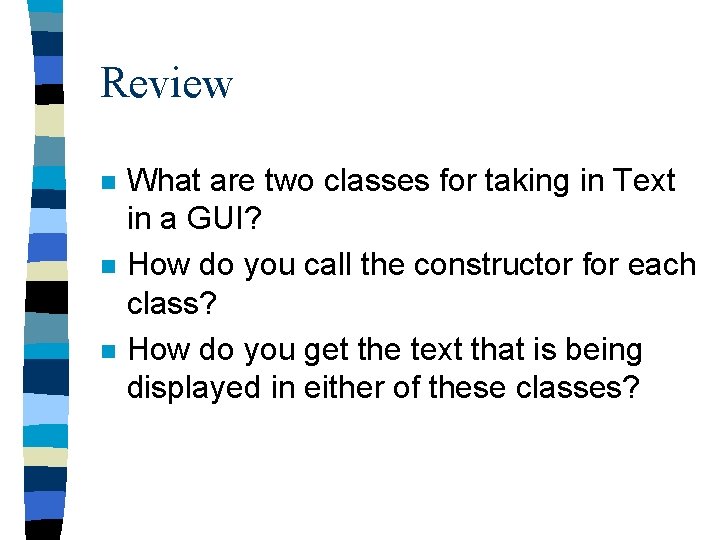
Review n n n What are two classes for taking in Text in a GUI? How do you call the constructor for each class? How do you get the text that is being displayed in either of these classes?
Apa perbedaan antara java swing dengan java awt
Graphics and guis with matlab
Characteristics of gui
Swing vs awt
Java swing responsive
Containment in java
Jscroll demo
Saját képek
Gui programmierung java
Swing mvc example
Swing tutorial java
Java swing draw text
Java swing layout
Java swing actionlistener multiple buttons
자바 스윙
Mvc java swing
Import java.lang.*
Java awt swing
Java swing form example
Import java.awt
Java swing tutorial
Java swing browser
Swing mvc architecture
Java layout 종류
Java swing
Java swing panel
Boxlayout swing
Abstract windowing toolkit
Java graphics set color
Swing layout
Java collections overview
Elements and their properties section 1 metals
Emt chapter 24 trauma overview
Chapter 14 medical overview
Chapter 9 lesson 2 photosynthesis an overview
Chapter 12 selling overview
Financial intermediaries
Chapter 1 overview of verb tenses
Overview of personal finance chapter 1
Distoclusion definition
Chapter 1: introduction to personal finance answer key
Chapter 32 an overview of animal diversity
Chapter 1 an overview of financial management
Chapter 1 overview of financial statement analysis
Java import java.util.*
Java import util
Import java
Gcd java
Import java util random
Import java.io.* in java
Import java.util.*
Java thread import
Import java.awt.event
Java compiler translates java source code into
What is rmi and ejb in java
Theme and topic difference
Diff between awt and swing
Classes salsa and swing implement an interface dance
Merkmale des jazz
Softball ontario rules
Down on the roof so brown
Swing check valve symbol
How the customer explained it
Swing scala
Crismani attachment
Energy release quick check
Layout managers in java
Jazz a swing v české hudbě
Swing prezentace
Joptionpane.showoptiondialog
Swing lock removable partial denture
Swing class hierarchy
Electro swing history