Creating GUIs in Java Using AWT and Swing
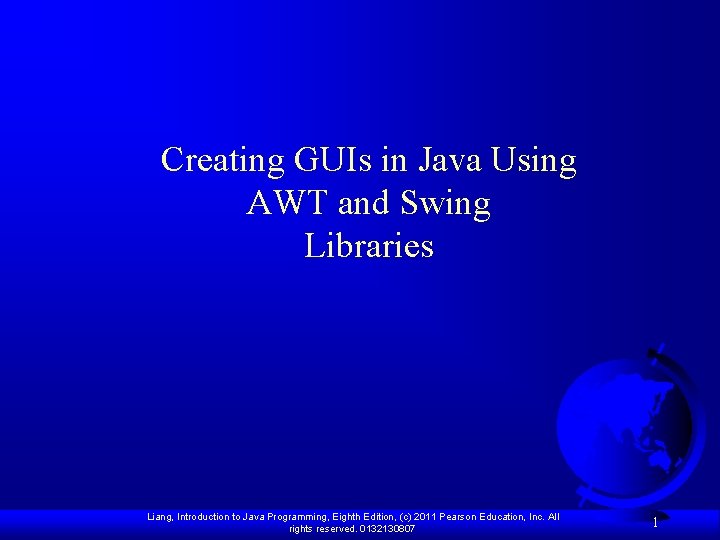
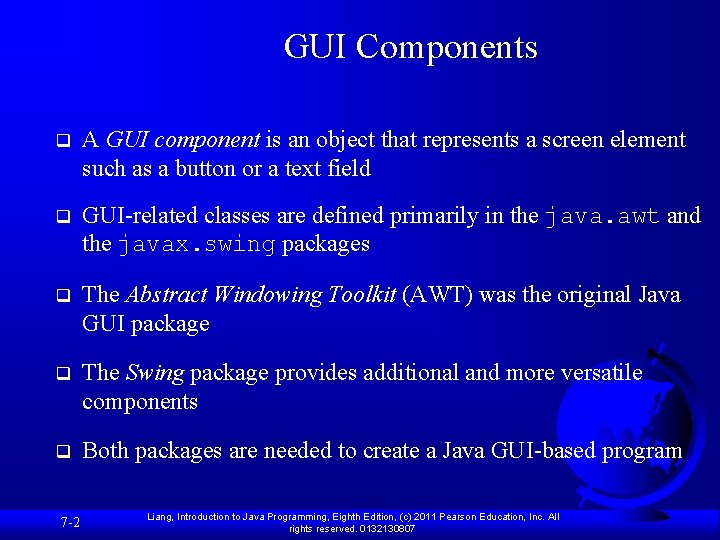
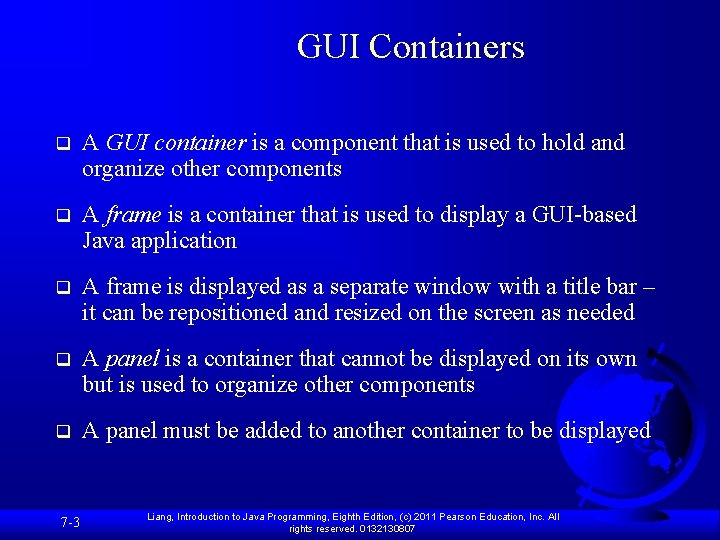
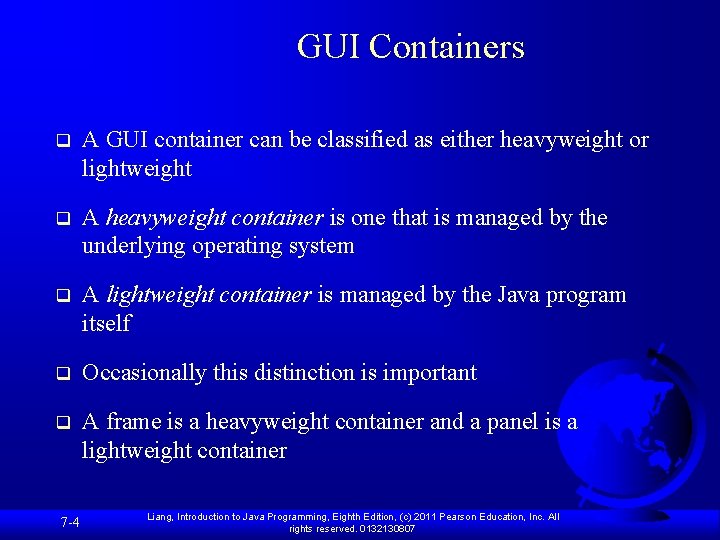
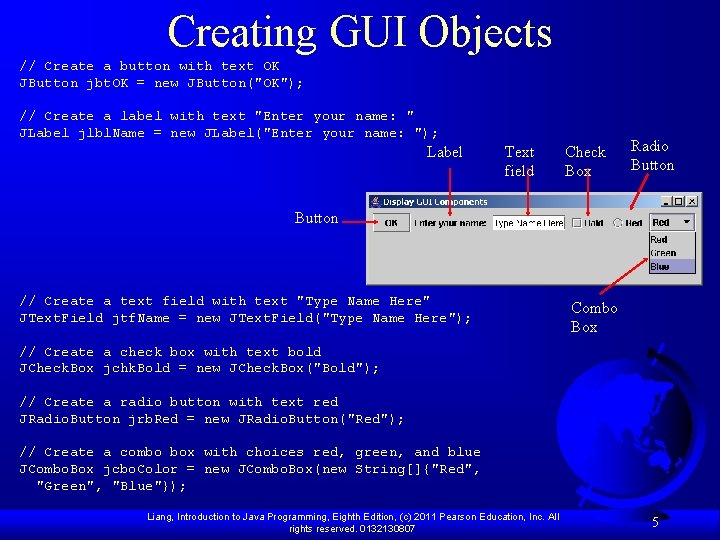
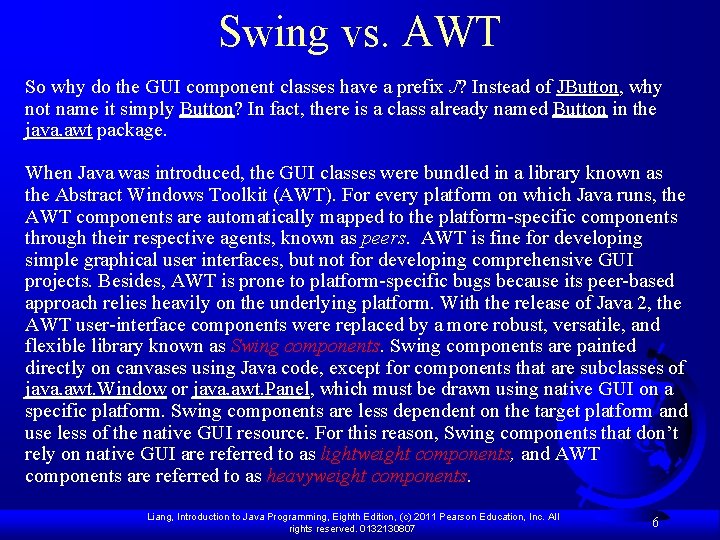
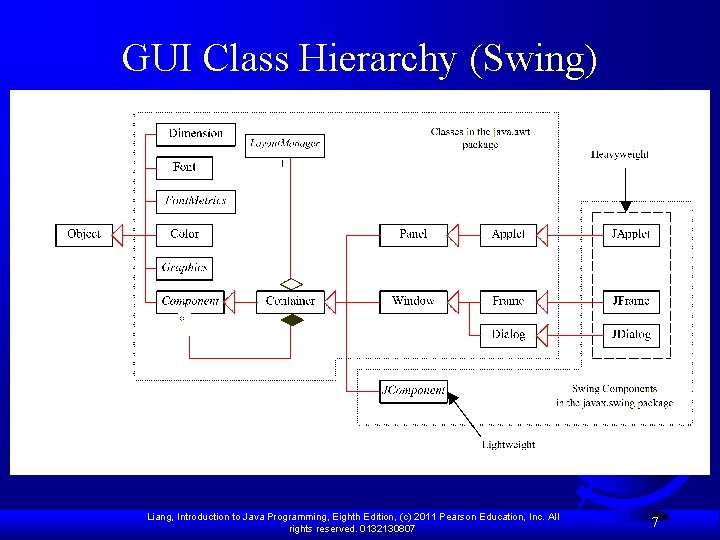
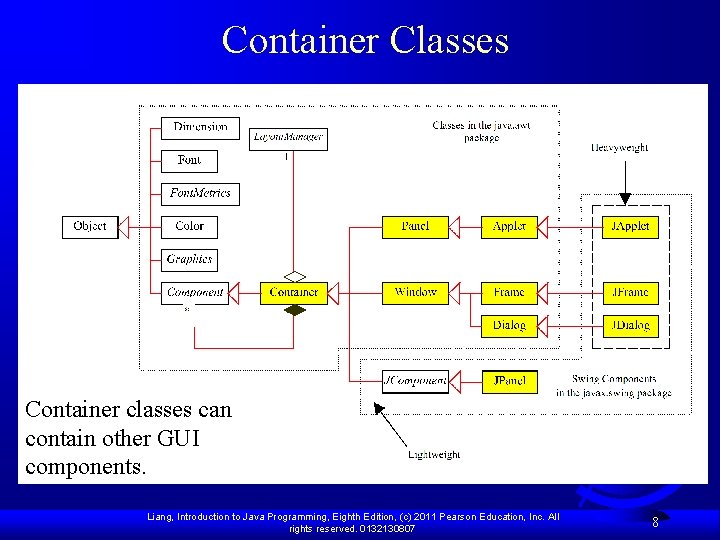
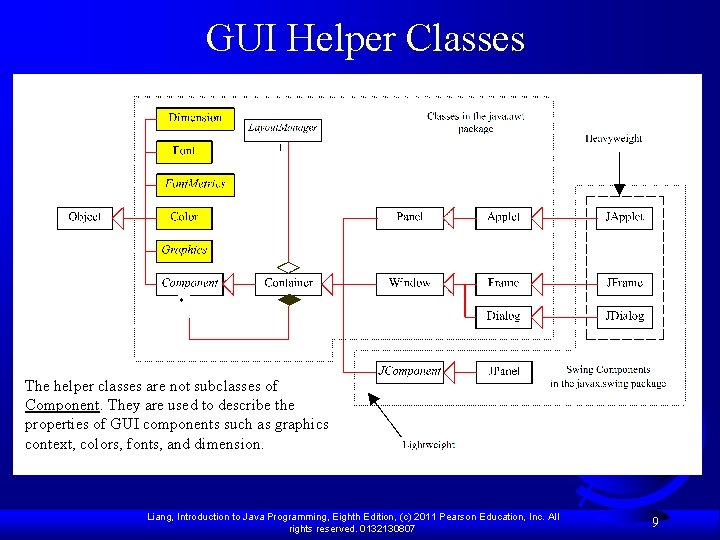
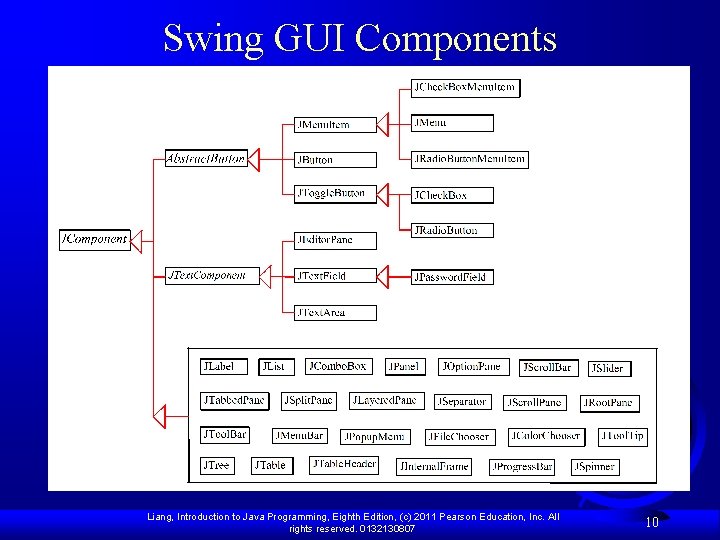
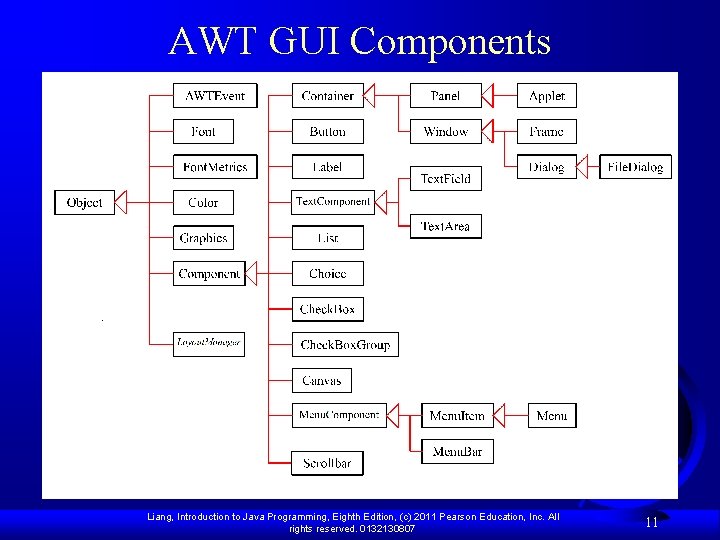
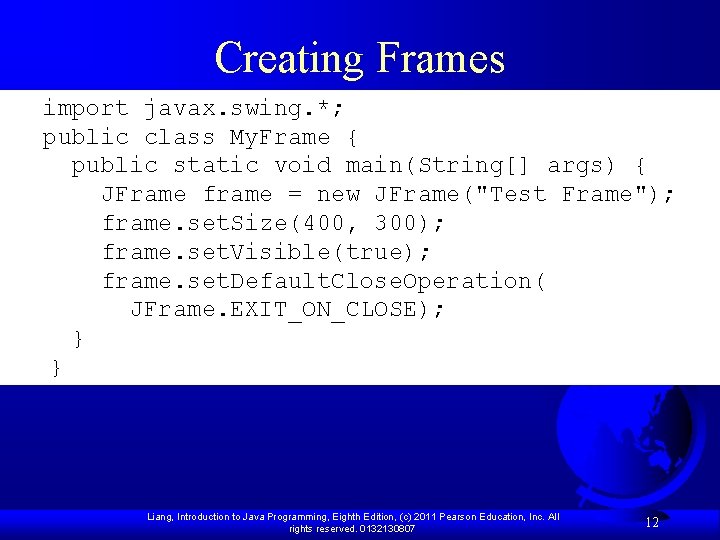
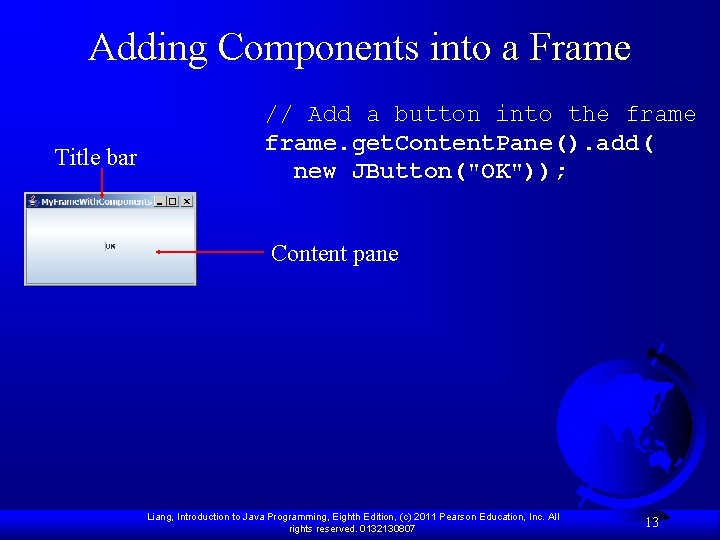
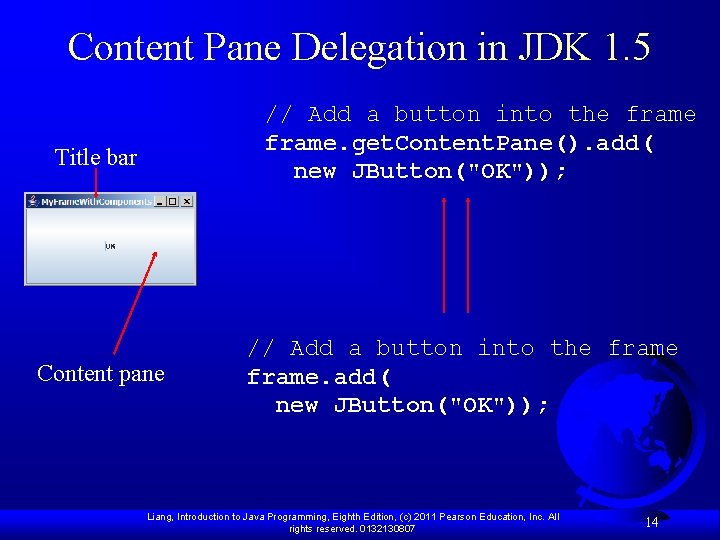
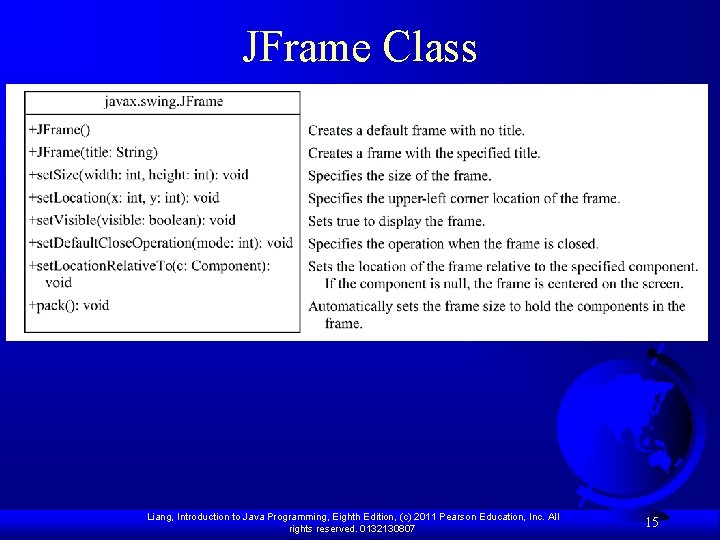
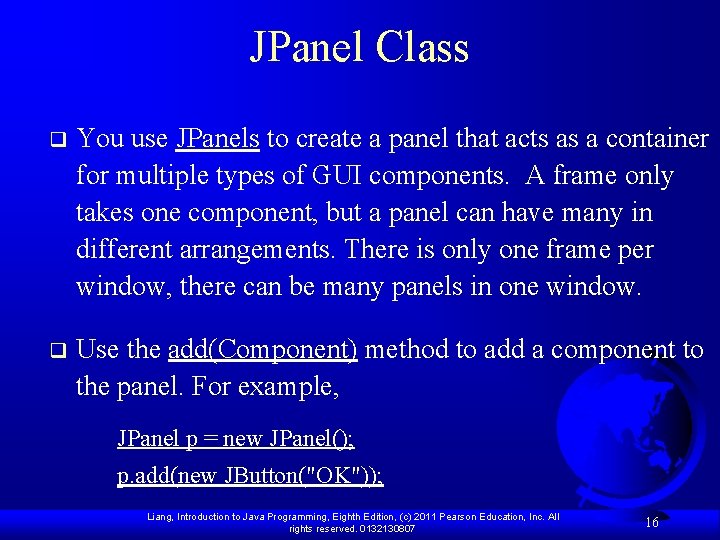
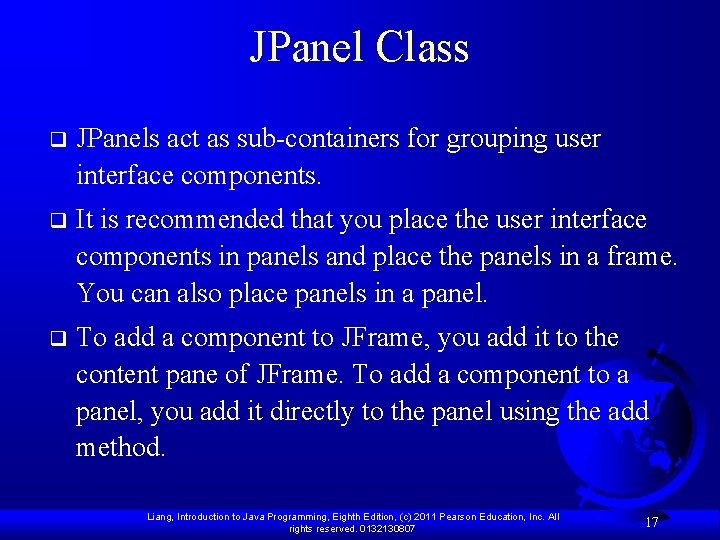
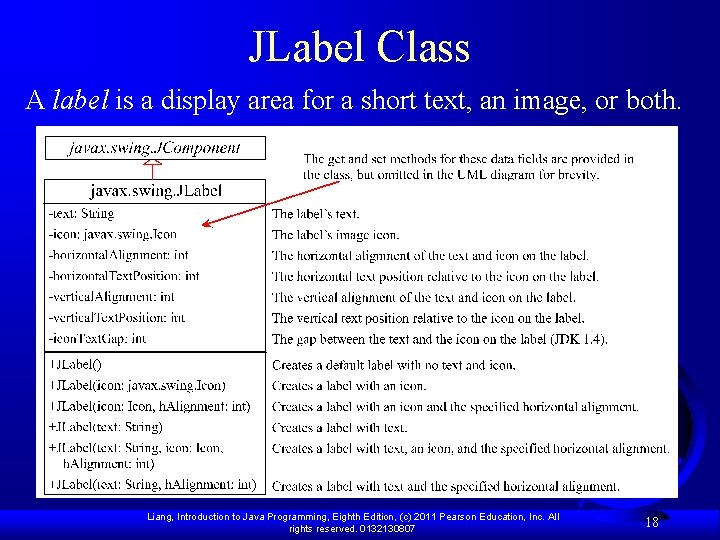
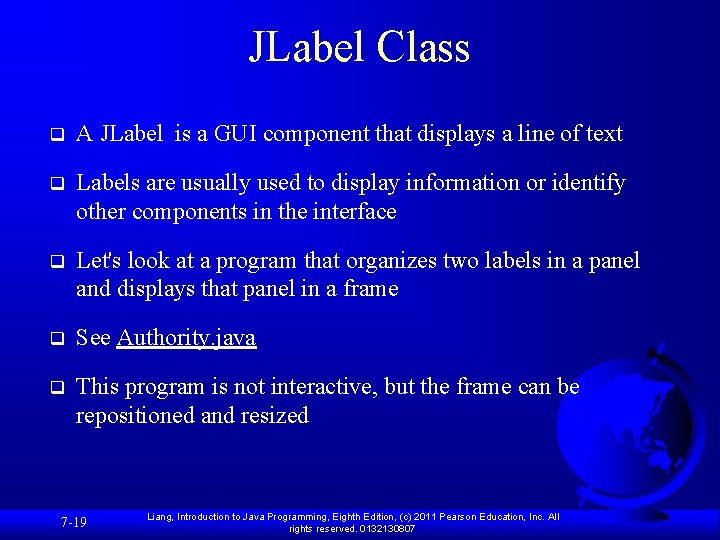
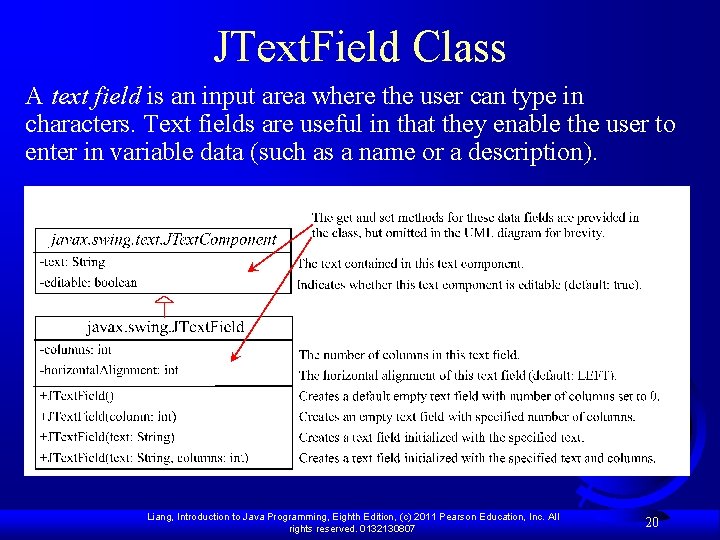
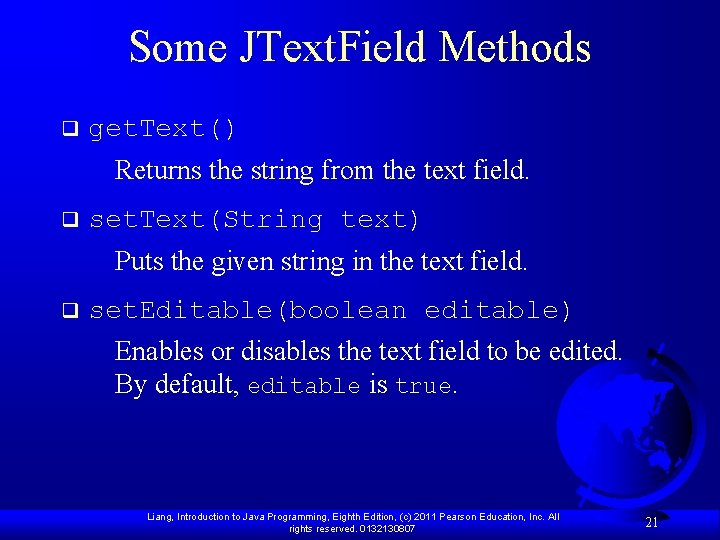
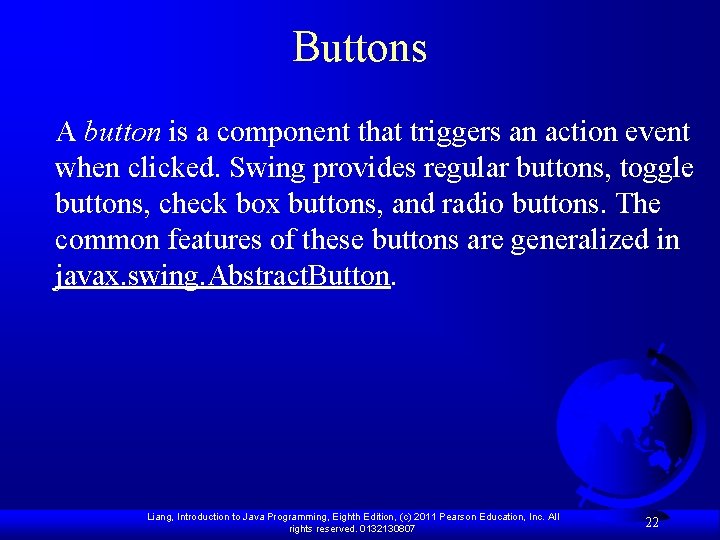
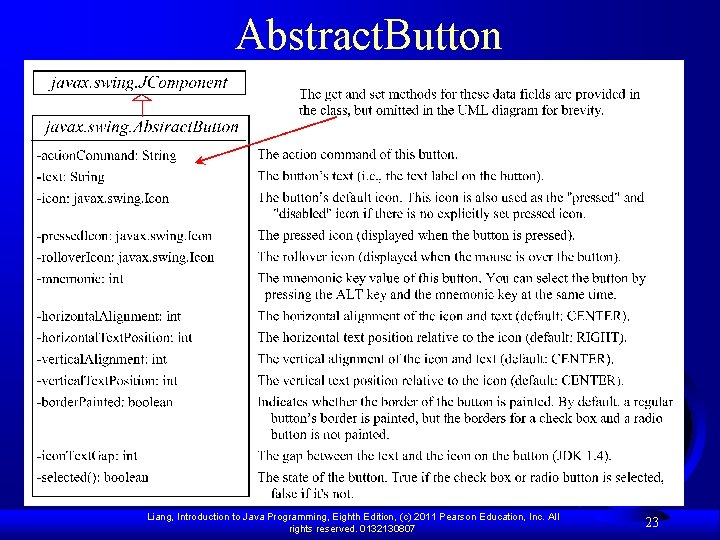
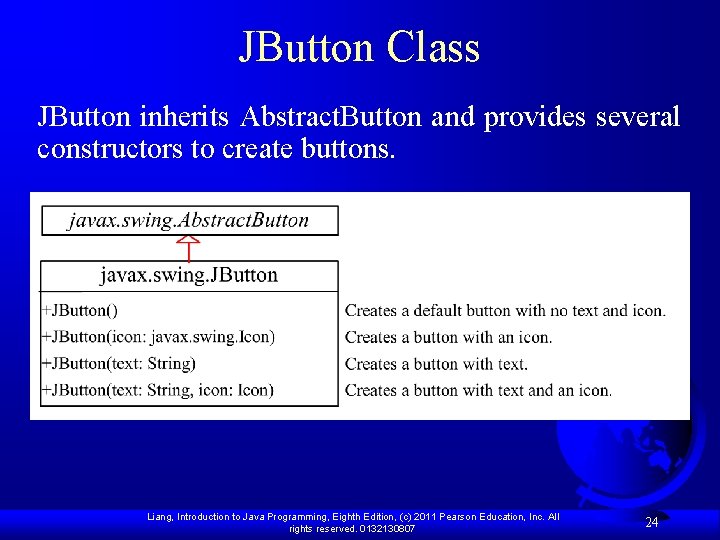
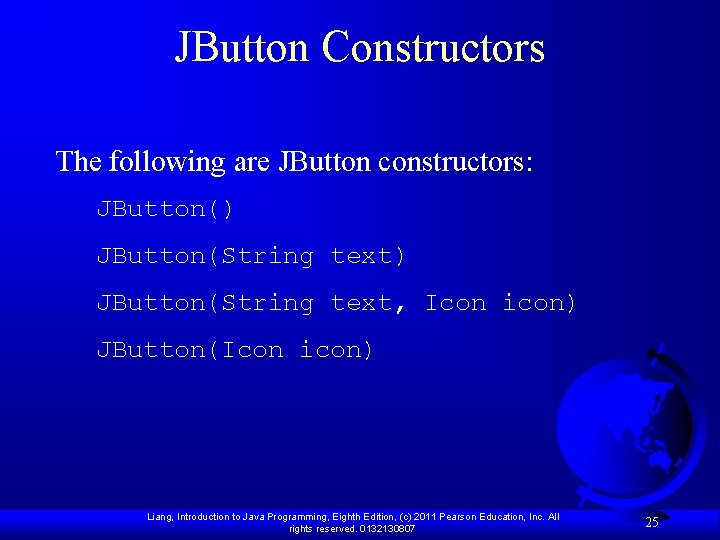
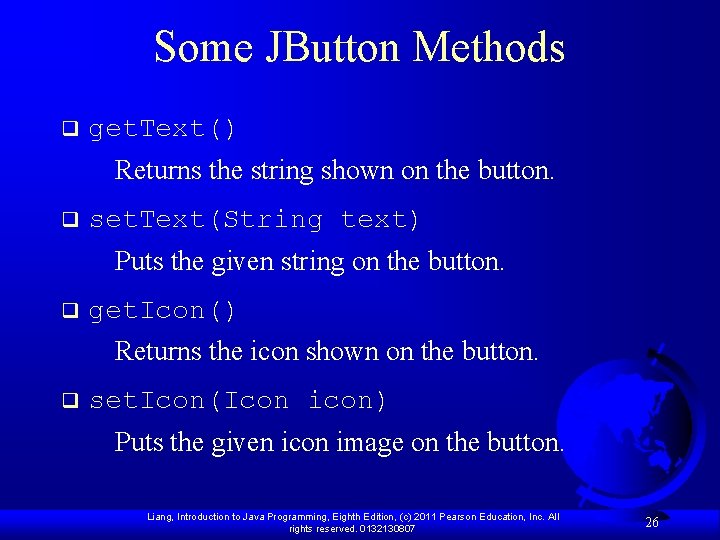
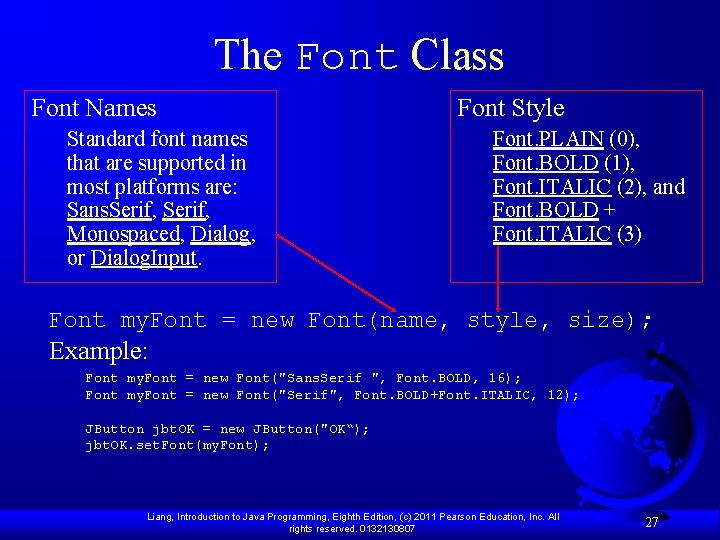
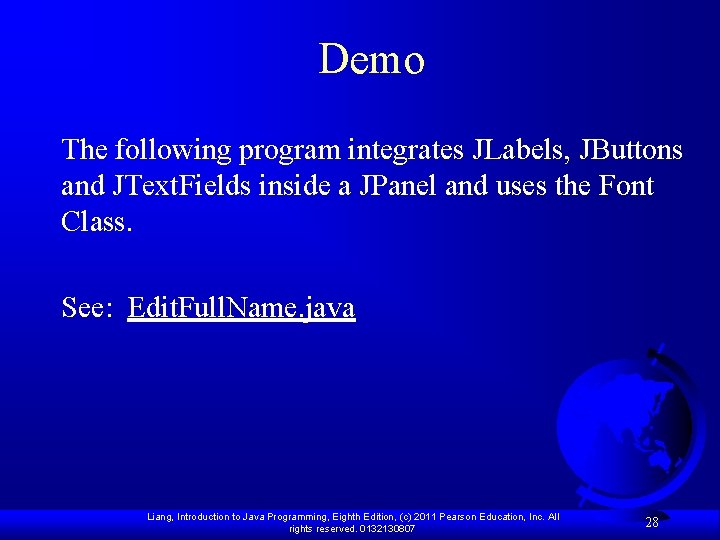
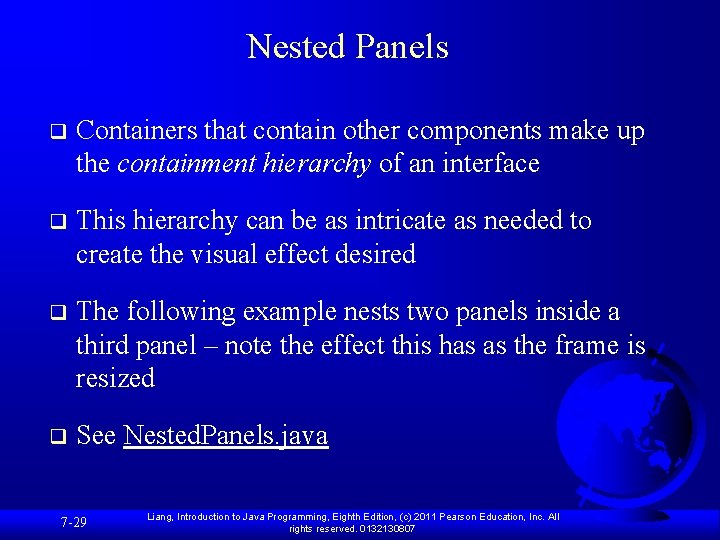
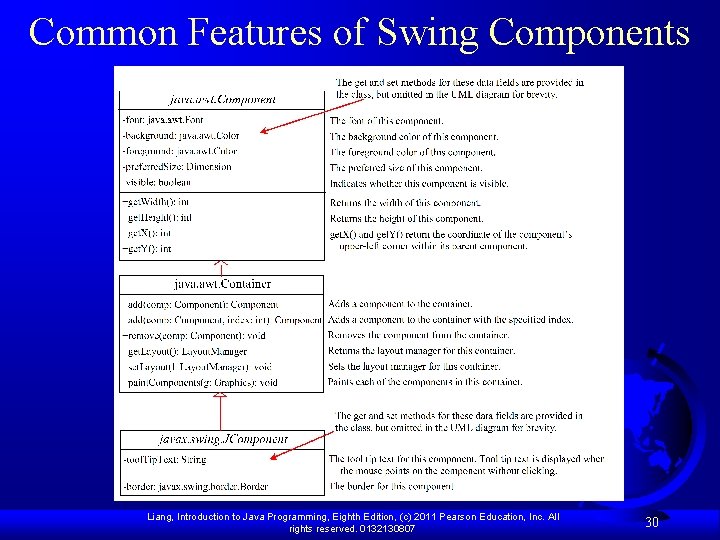
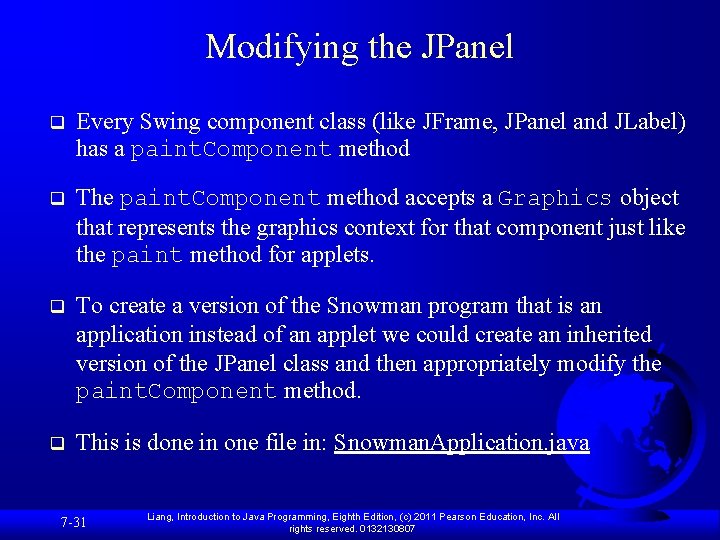
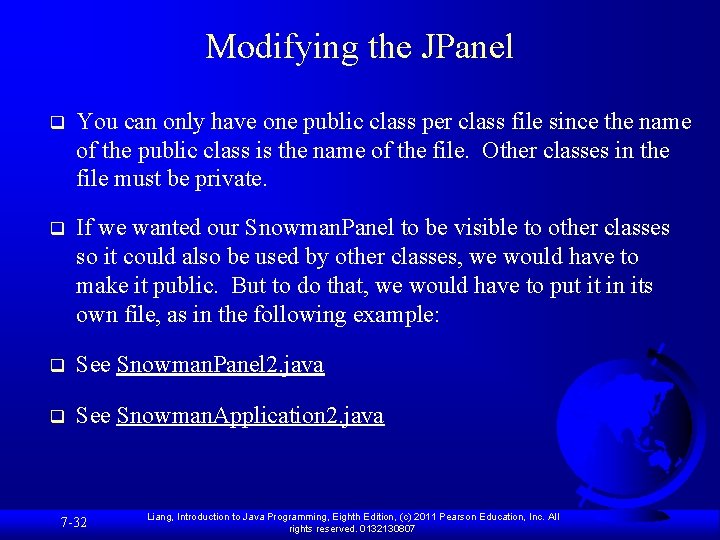
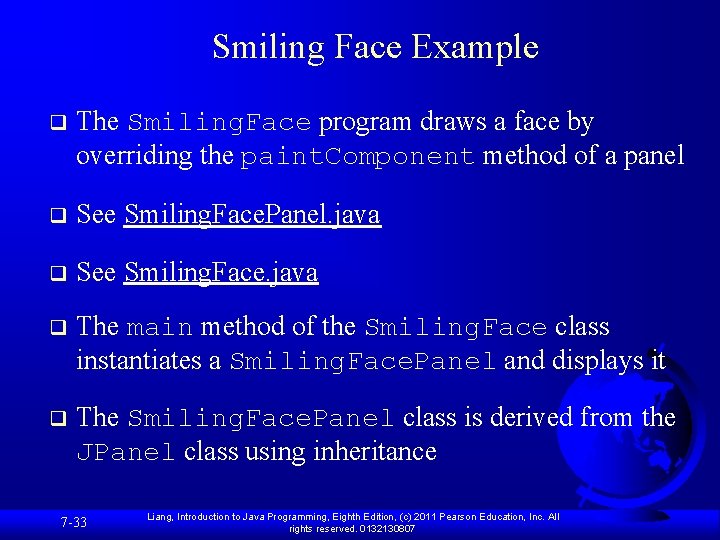
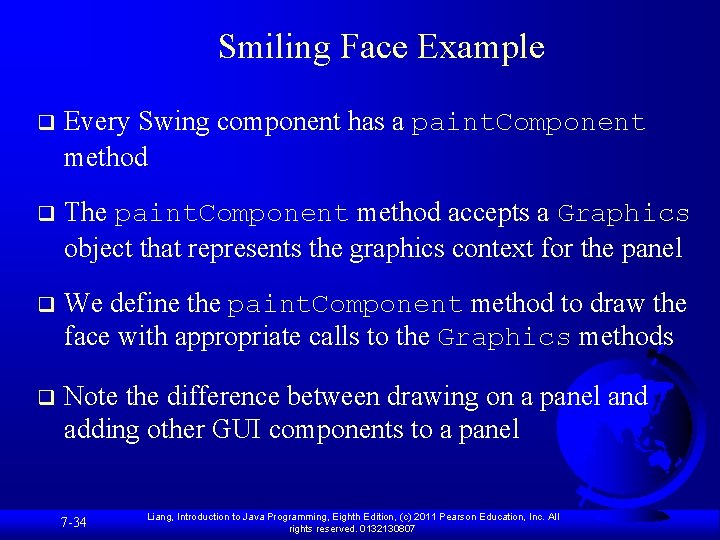
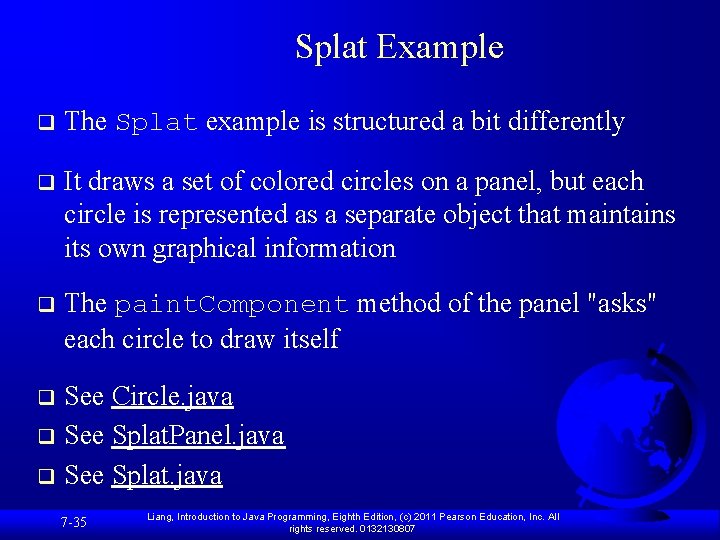
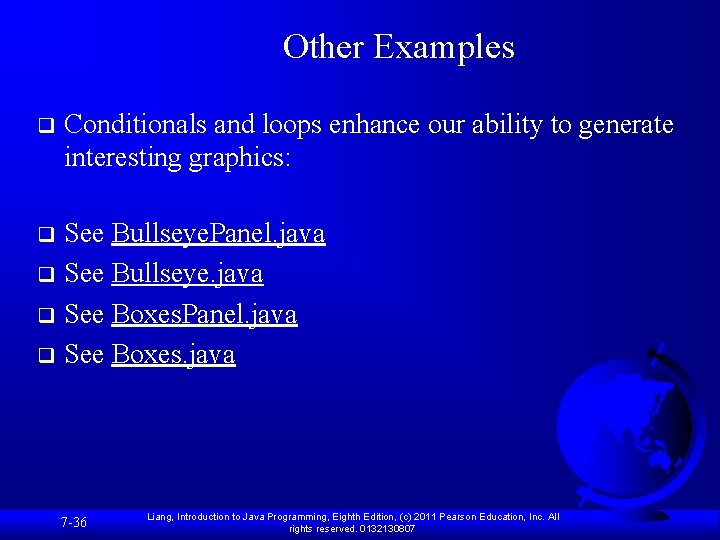
- Slides: 36
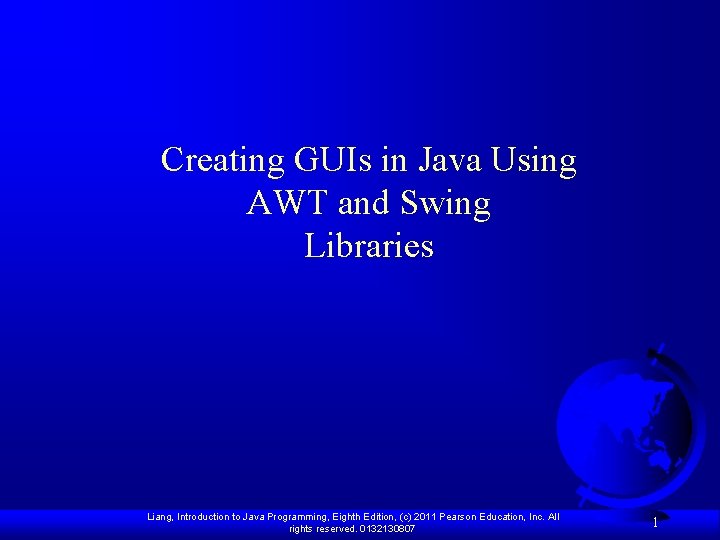
Creating GUIs in Java Using AWT and Swing Libraries Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 1
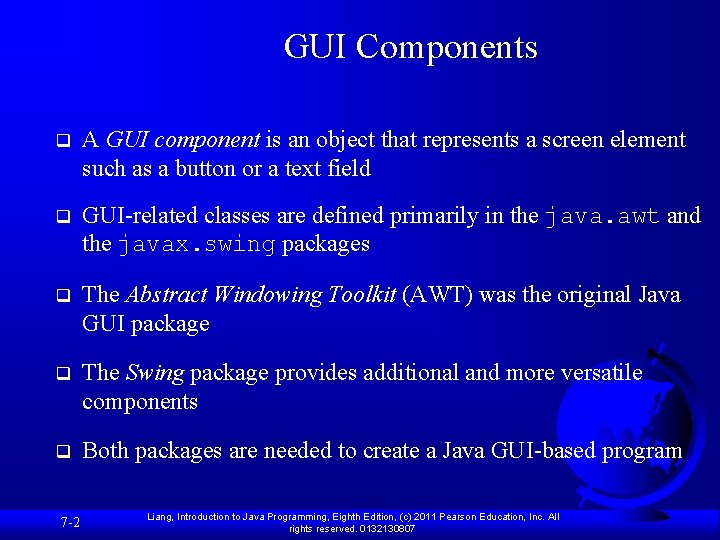
GUI Components q A GUI component is an object that represents a screen element such as a button or a text field q GUI-related classes are defined primarily in the java. awt and the javax. swing packages q The Abstract Windowing Toolkit (AWT) was the original Java GUI package q The Swing package provides additional and more versatile components q Both packages are needed to create a Java GUI-based program 7 -2 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
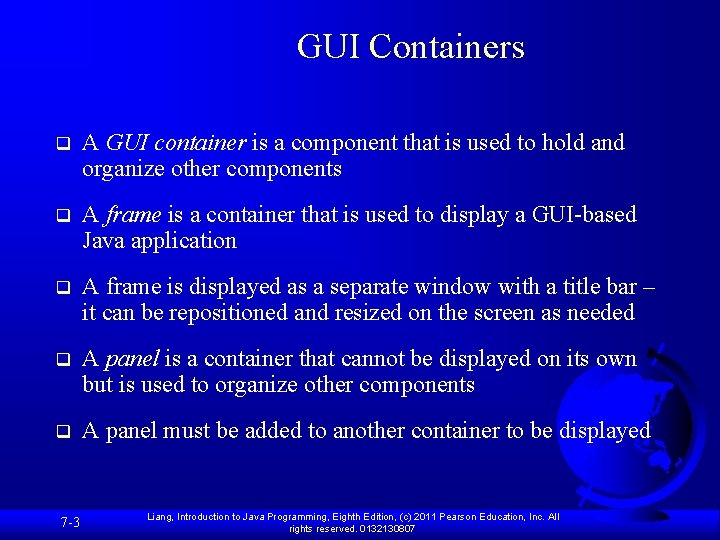
GUI Containers q A GUI container is a component that is used to hold and organize other components q A frame is a container that is used to display a GUI-based Java application q A frame is displayed as a separate window with a title bar – it can be repositioned and resized on the screen as needed q A panel is a container that cannot be displayed on its own but is used to organize other components q A panel must be added to another container to be displayed 7 -3 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
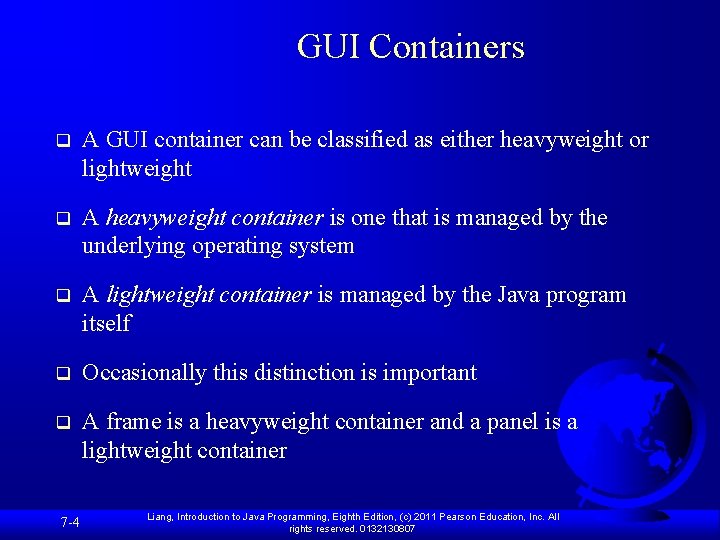
GUI Containers q A GUI container can be classified as either heavyweight or lightweight q A heavyweight container is one that is managed by the underlying operating system q A lightweight container is managed by the Java program itself q Occasionally this distinction is important q A frame is a heavyweight container and a panel is a lightweight container 7 -4 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
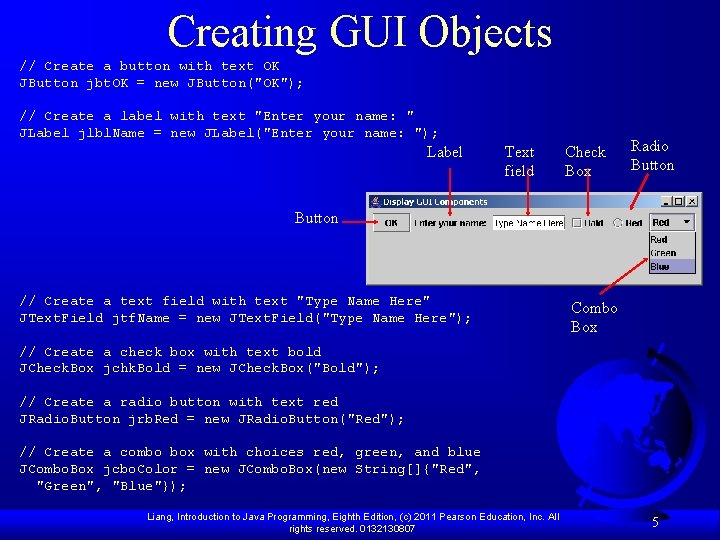
Creating GUI Objects // Create a button with text OK JButton jbt. OK = new JButton("OK"); // Create a label with text "Enter your name: " JLabel jlbl. Name = new JLabel("Enter your name: "); Label Text field Check Box Radio Button // Create a text field with text "Type Name Here" JText. Field jtf. Name = new JText. Field("Type Name Here"); Combo Box // Create a check box with text bold JCheck. Box jchk. Bold = new JCheck. Box("Bold"); // Create a radio button with text red JRadio. Button jrb. Red = new JRadio. Button("Red"); // Create a combo box with choices red, green, and blue JCombo. Box jcbo. Color = new JCombo. Box(new String[]{"Red", "Green", "Blue"}); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 5
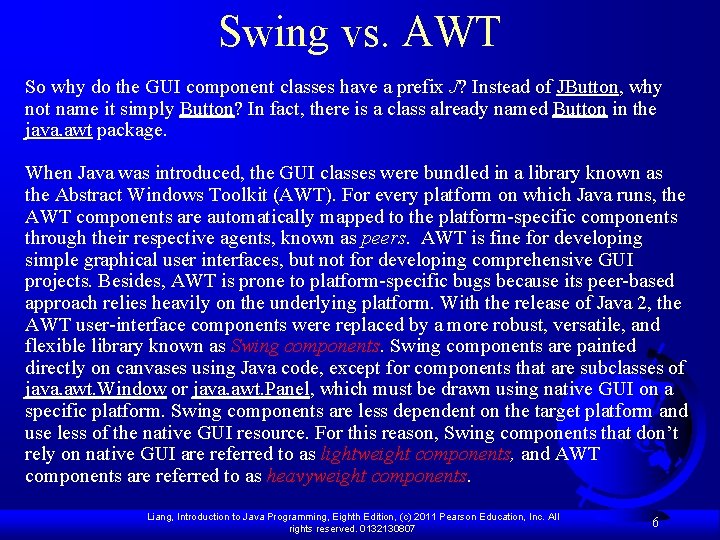
Swing vs. AWT So why do the GUI component classes have a prefix J? Instead of JButton, why not name it simply Button? In fact, there is a class already named Button in the java. awt package. When Java was introduced, the GUI classes were bundled in a library known as the Abstract Windows Toolkit (AWT). For every platform on which Java runs, the AWT components are automatically mapped to the platform-specific components through their respective agents, known as peers. AWT is fine for developing simple graphical user interfaces, but not for developing comprehensive GUI projects. Besides, AWT is prone to platform-specific bugs because its peer-based approach relies heavily on the underlying platform. With the release of Java 2, the AWT user-interface components were replaced by a more robust, versatile, and flexible library known as Swing components are painted directly on canvases using Java code, except for components that are subclasses of java. awt. Window or java. awt. Panel, which must be drawn using native GUI on a specific platform. Swing components are less dependent on the target platform and use less of the native GUI resource. For this reason, Swing components that don’t rely on native GUI are referred to as lightweight components, and AWT components are referred to as heavyweight components. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 6
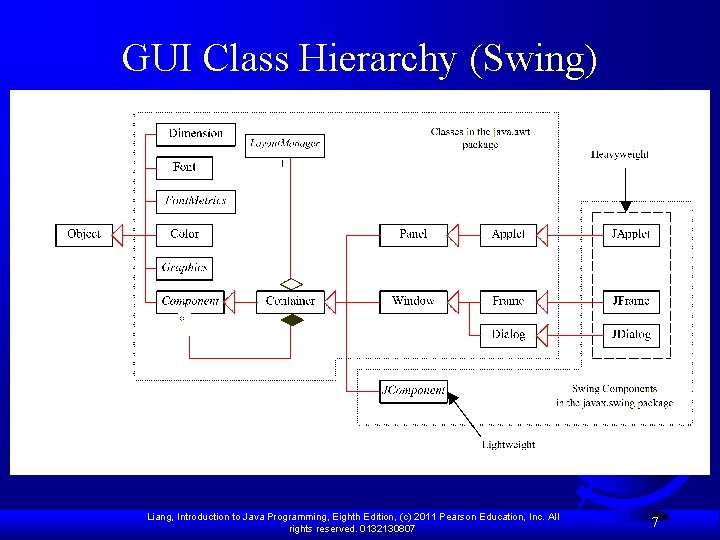
GUI Class Hierarchy (Swing) Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 7
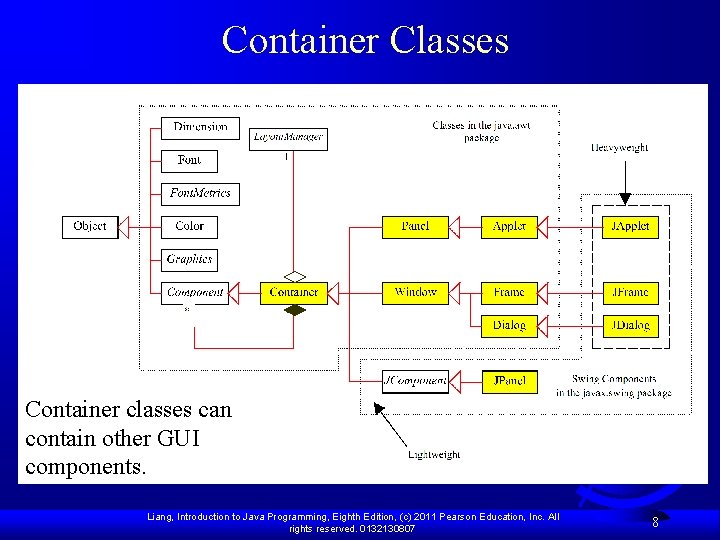
Container Classes Container classes can contain other GUI components. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 8
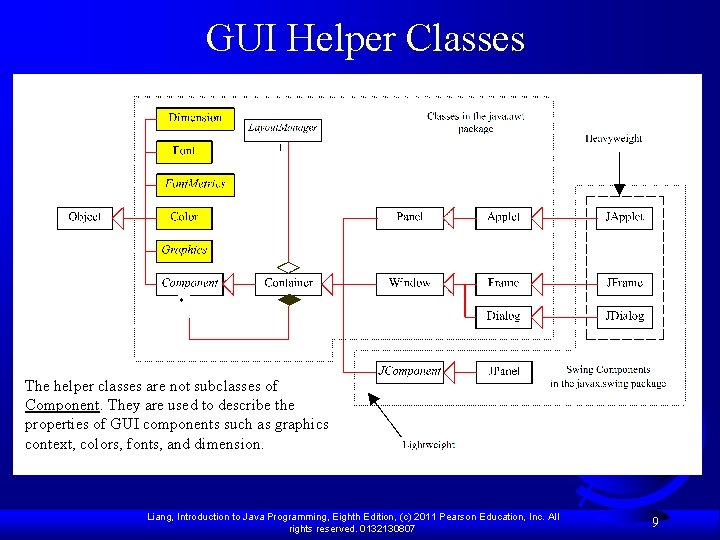
GUI Helper Classes The helper classes are not subclasses of Component. They are used to describe the properties of GUI components such as graphics context, colors, fonts, and dimension. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 9
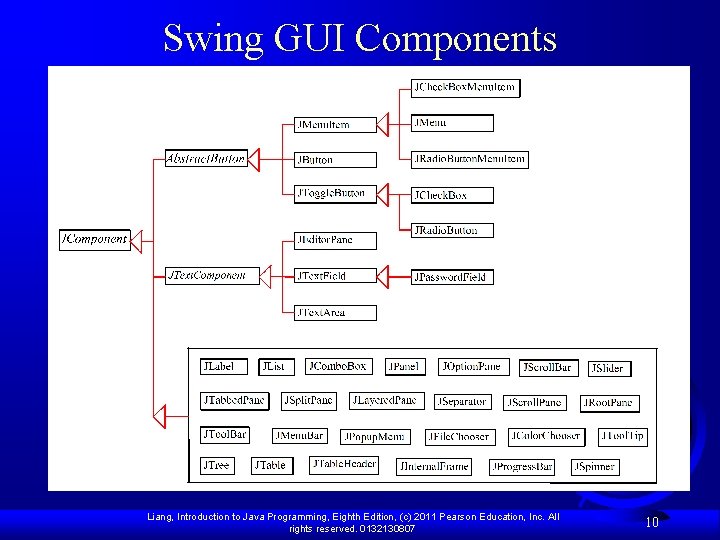
Swing GUI Components Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 10
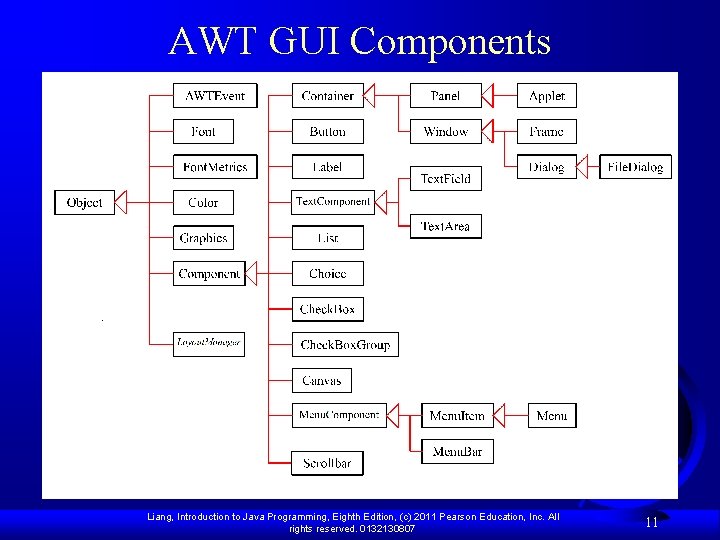
AWT GUI Components Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 11
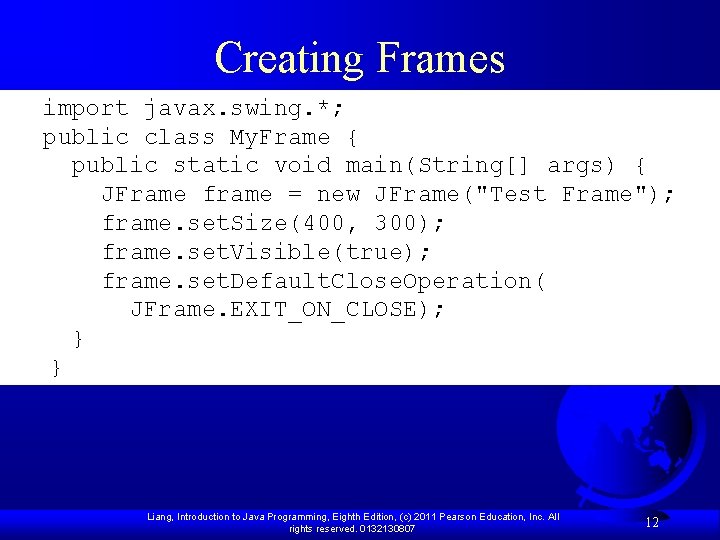
Creating Frames import javax. swing. *; public class My. Frame { public static void main(String[] args) { JFrame frame = new JFrame("Test Frame"); frame. set. Size(400, 300); frame. set. Visible(true); frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE); } } Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 12
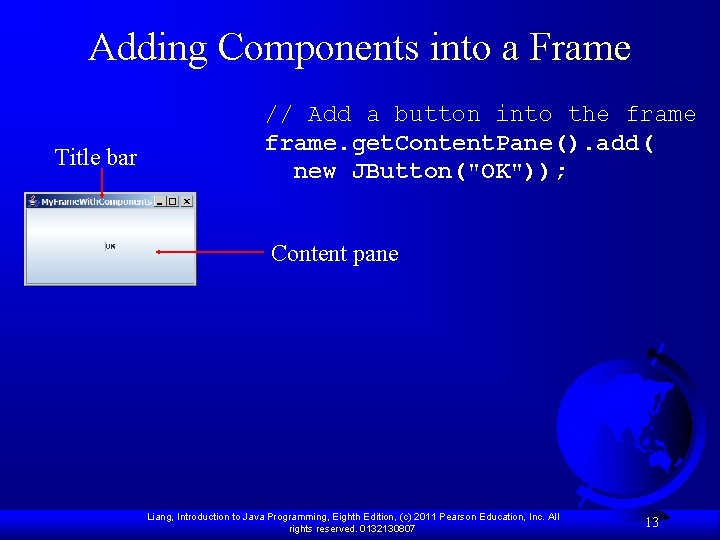
Adding Components into a Frame Title bar // Add a button into the frame. get. Content. Pane(). add( new JButton("OK")); Content pane Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 13
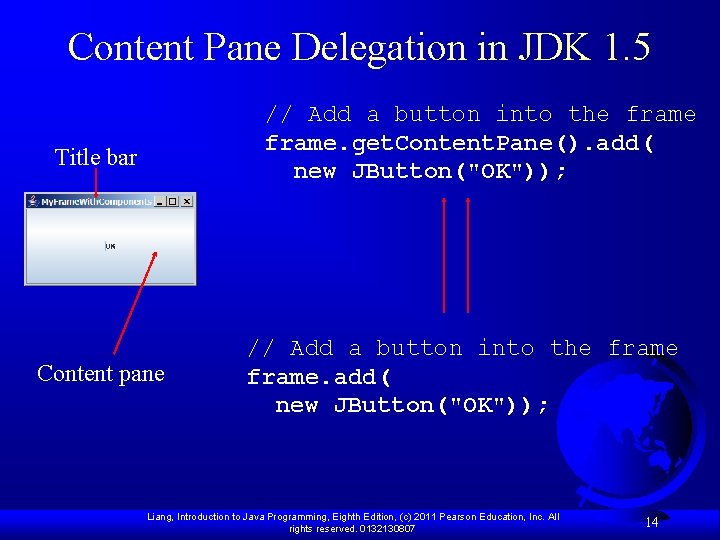
Content Pane Delegation in JDK 1. 5 // Add a button into the frame. get. Content. Pane(). add( new JButton("OK")); Title bar Content pane // Add a button into the frame. add( new JButton("OK")); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 14
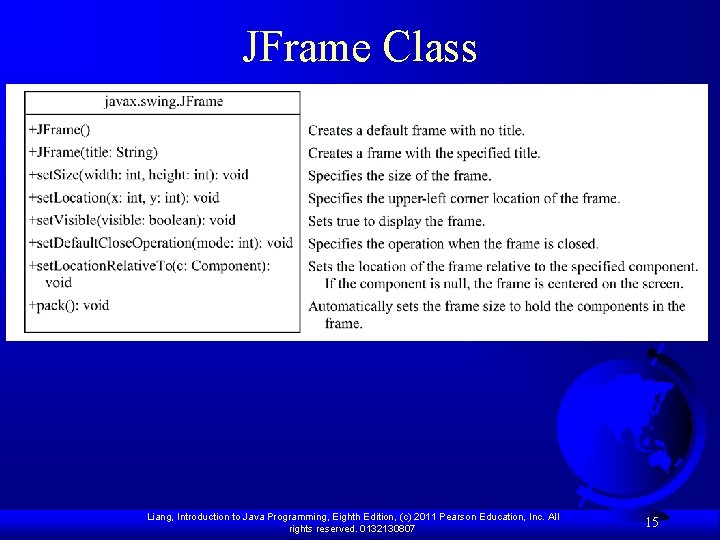
JFrame Class Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 15
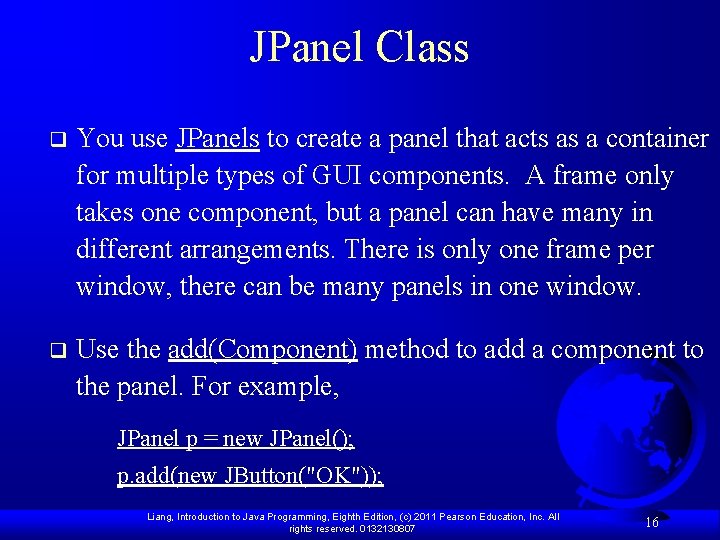
JPanel Class q You use JPanels to create a panel that acts as a container for multiple types of GUI components. A frame only takes one component, but a panel can have many in different arrangements. There is only one frame per window, there can be many panels in one window. q Use the add(Component) method to add a component to the panel. For example, JPanel p = new JPanel(); p. add(new JButton("OK")); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 16
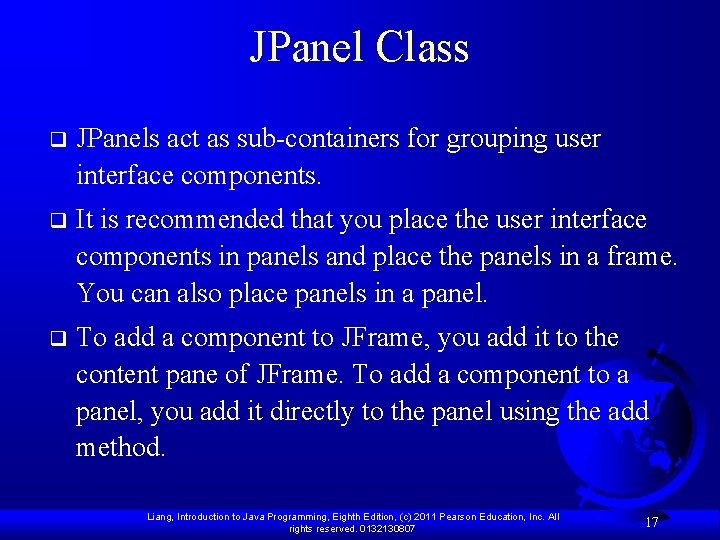
JPanel Class q JPanels act as sub-containers for grouping user interface components. q It is recommended that you place the user interface components in panels and place the panels in a frame. You can also place panels in a panel. q To add a component to JFrame, you add it to the content pane of JFrame. To add a component to a panel, you add it directly to the panel using the add method. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 17
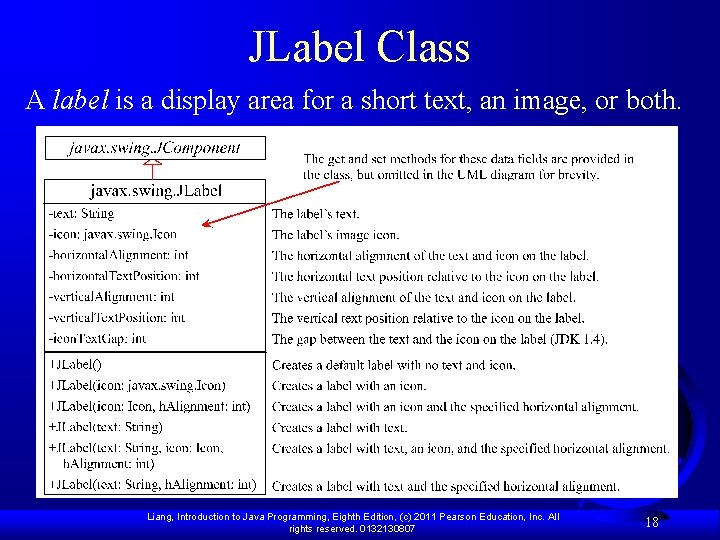
JLabel Class A label is a display area for a short text, an image, or both. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 18
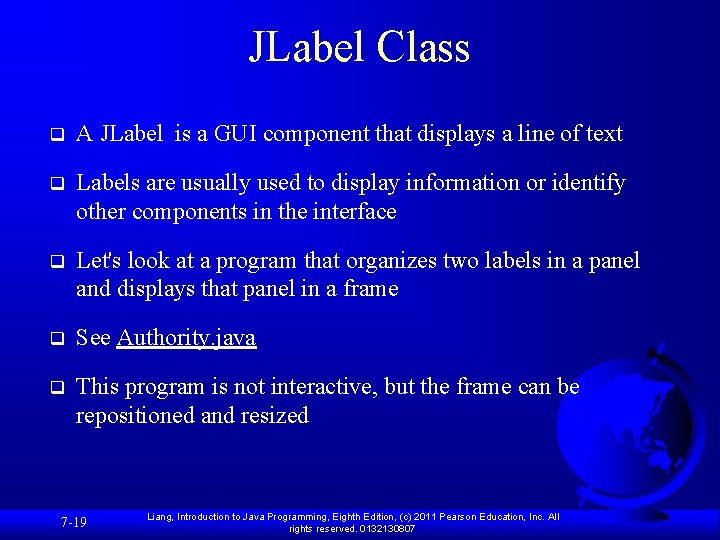
JLabel Class q A JLabel is a GUI component that displays a line of text q Labels are usually used to display information or identify other components in the interface q Let's look at a program that organizes two labels in a panel and displays that panel in a frame q See Authority. java q This program is not interactive, but the frame can be repositioned and resized 7 -19 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
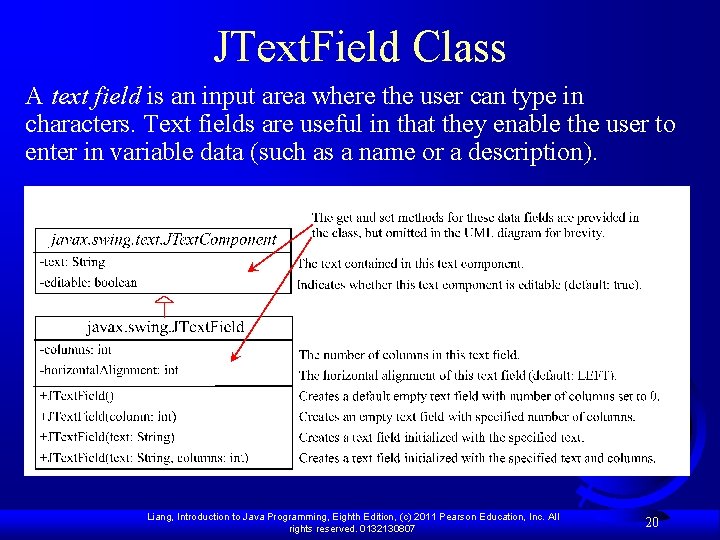
JText. Field Class A text field is an input area where the user can type in characters. Text fields are useful in that they enable the user to enter in variable data (such as a name or a description). Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 20
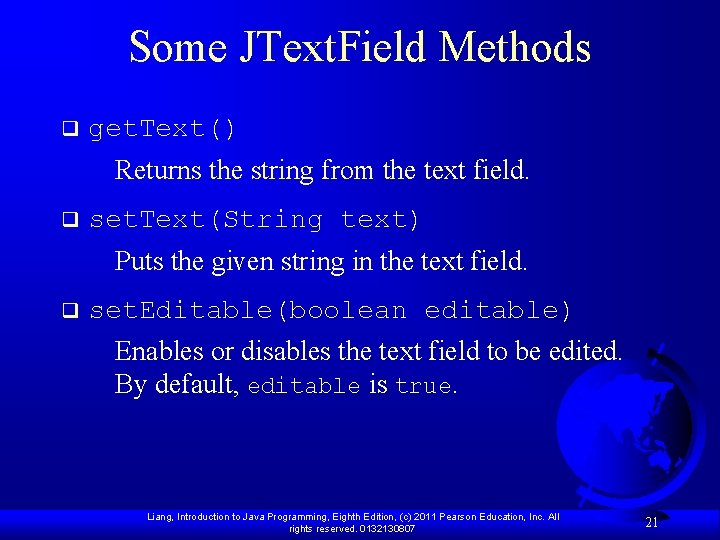
Some JText. Field Methods q get. Text() Returns the string from the text field. q set. Text(String text) Puts the given string in the text field. q set. Editable(boolean editable) Enables or disables the text field to be edited. By default, editable is true. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 21
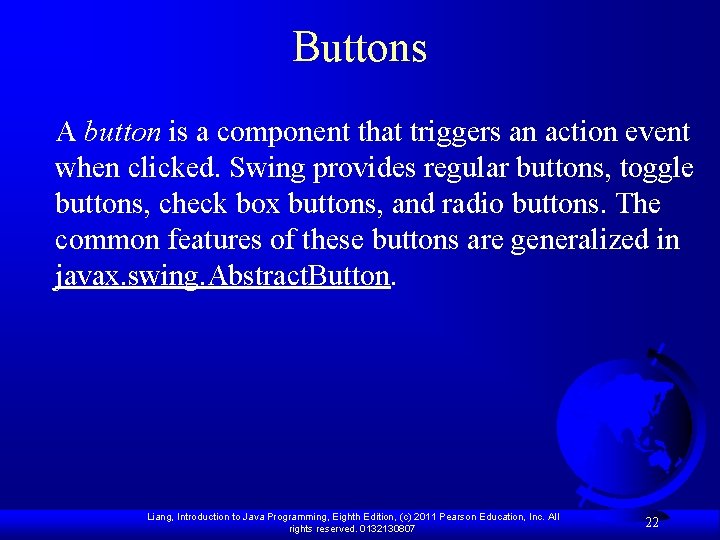
Buttons A button is a component that triggers an action event when clicked. Swing provides regular buttons, toggle buttons, check box buttons, and radio buttons. The common features of these buttons are generalized in javax. swing. Abstract. Button. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 22
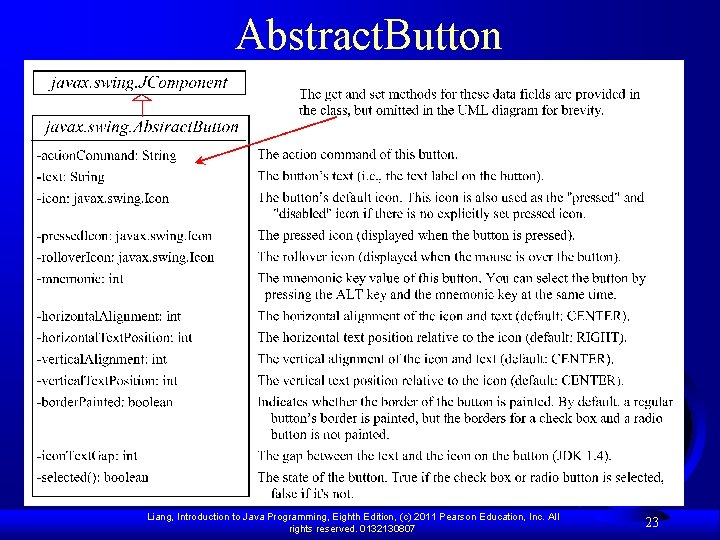
Abstract. Button Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 23
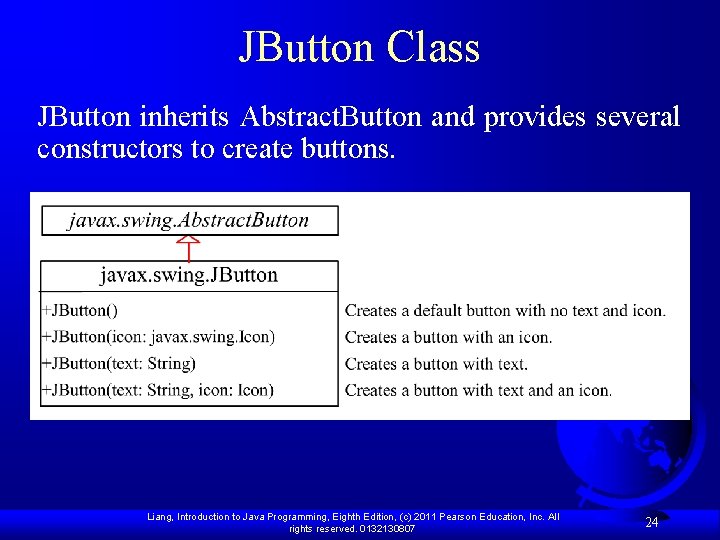
JButton Class JButton inherits Abstract. Button and provides several constructors to create buttons. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 24
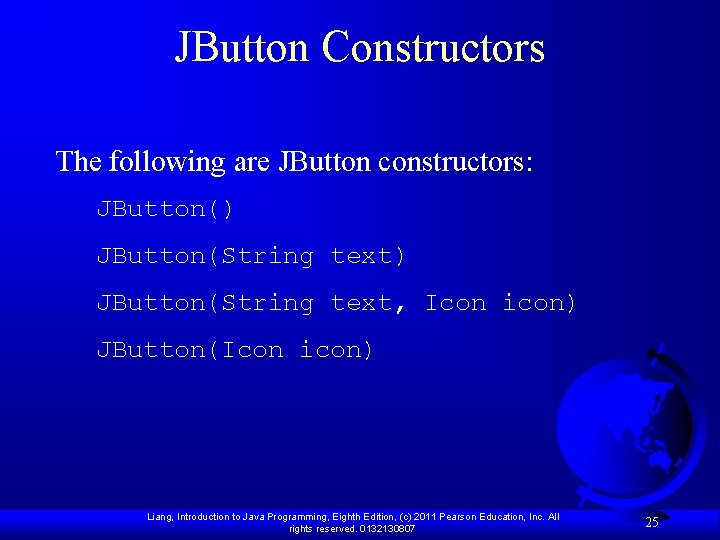
JButton Constructors The following are JButton constructors: JButton() JButton(String text, Icon icon) JButton(Icon icon) Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 25
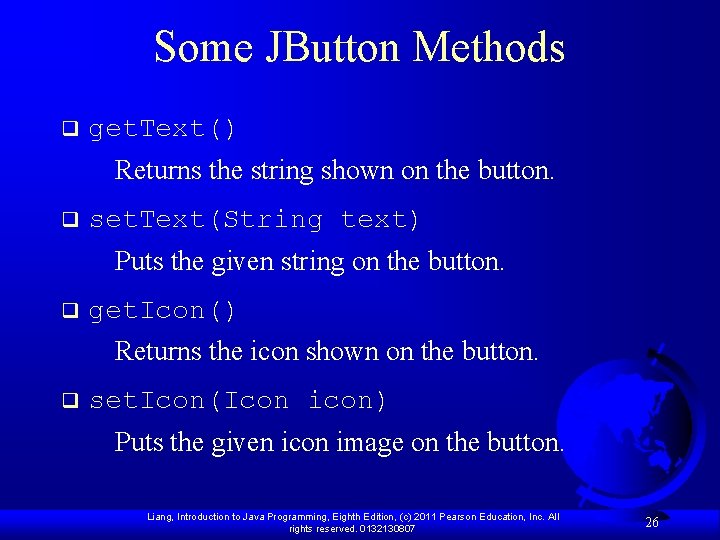
Some JButton Methods q get. Text() Returns the string shown on the button. q set. Text(String text) Puts the given string on the button. q get. Icon() Returns the icon shown on the button. q set. Icon(Icon icon) Puts the given icon image on the button. Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 26
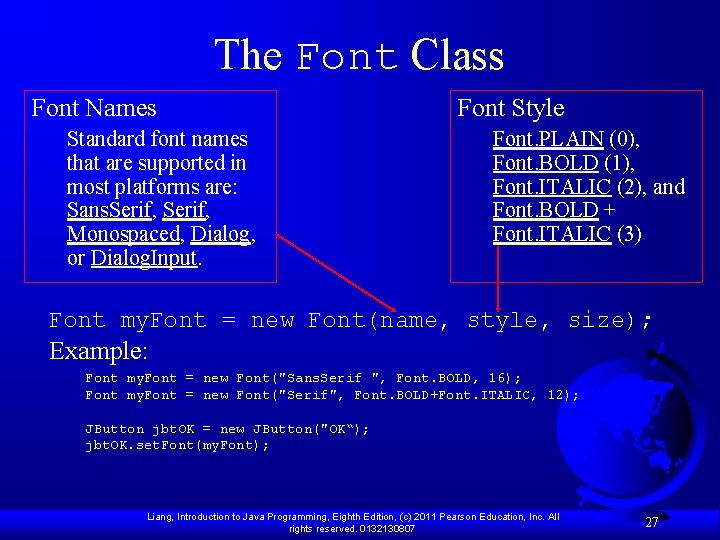
The Font Class Font Names Standard font names that are supported in most platforms are: Sans. Serif, Monospaced, Dialog, or Dialog. Input. Font Style Font. PLAIN (0), Font. BOLD (1), Font. ITALIC (2), and Font. BOLD + Font. ITALIC (3) Font my. Font = new Font(name, style, size); Example: Font my. Font = new Font("Sans. Serif ", Font. BOLD, 16); Font my. Font = new Font("Serif", Font. BOLD+Font. ITALIC, 12); JButton jbt. OK = new JButton("OK“); jbt. OK. set. Font(my. Font); Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 27
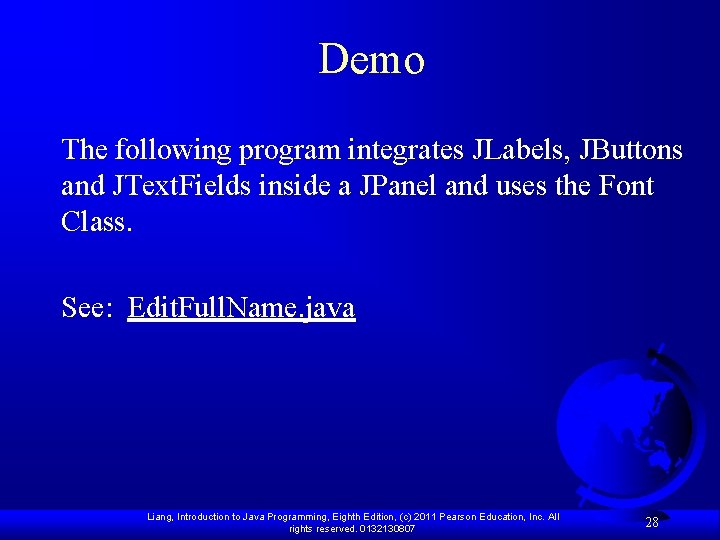
Demo The following program integrates JLabels, JButtons and JText. Fields inside a JPanel and uses the Font Class. See: Edit. Full. Name. java Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 28
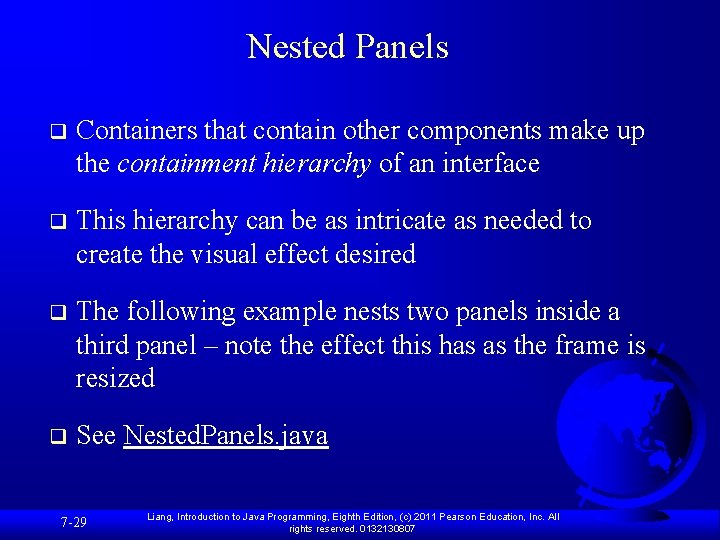
Nested Panels q Containers that contain other components make up the containment hierarchy of an interface q This hierarchy can be as intricate as needed to create the visual effect desired q The following example nests two panels inside a third panel – note the effect this has as the frame is resized q See Nested. Panels. java 7 -29 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
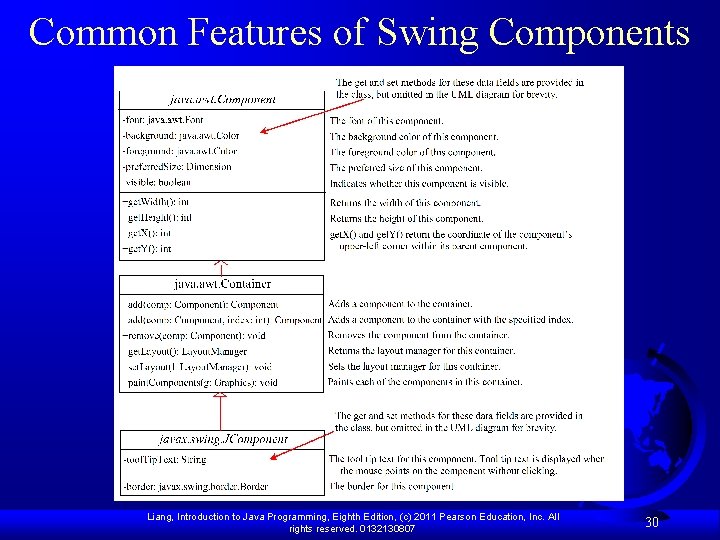
Common Features of Swing Components Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807 30
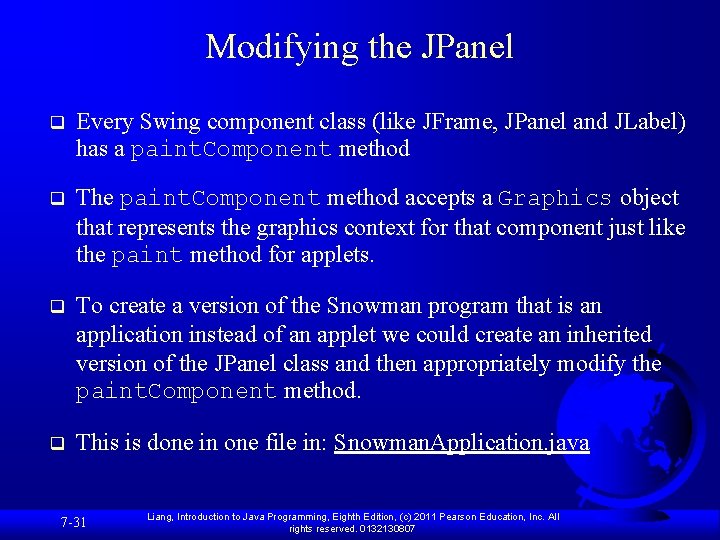
Modifying the JPanel q Every Swing component class (like JFrame, JPanel and JLabel) has a paint. Component method q The paint. Component method accepts a Graphics object that represents the graphics context for that component just like the paint method for applets. q To create a version of the Snowman program that is an application instead of an applet we could create an inherited version of the JPanel class and then appropriately modify the paint. Component method. q This is done in one file in: Snowman. Application. java 7 -31 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
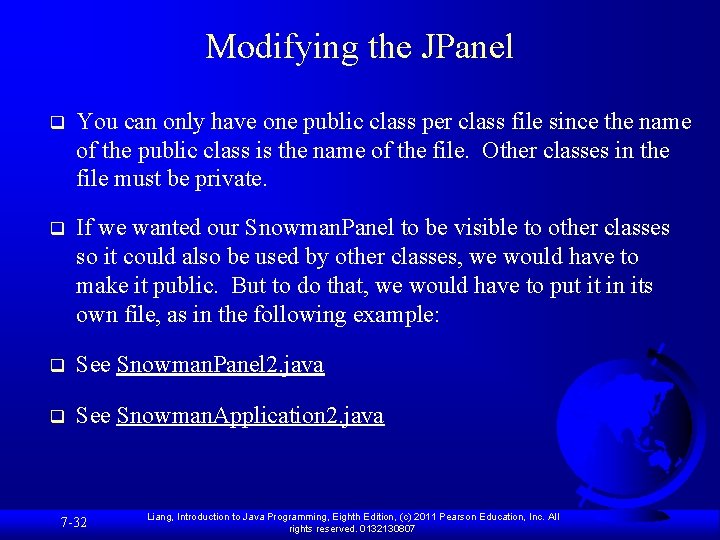
Modifying the JPanel q You can only have one public class per class file since the name of the public class is the name of the file. Other classes in the file must be private. q If we wanted our Snowman. Panel to be visible to other classes so it could also be used by other classes, we would have to make it public. But to do that, we would have to put it in its own file, as in the following example: q See Snowman. Panel 2. java q See Snowman. Application 2. java 7 -32 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
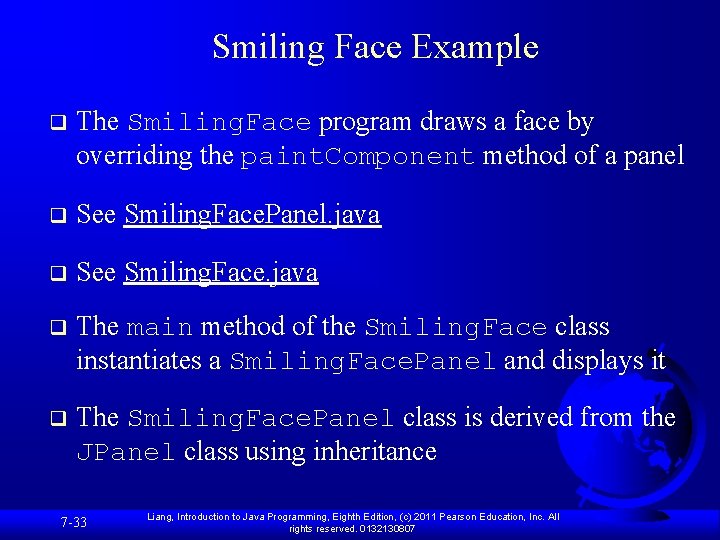
Smiling Face Example q The Smiling. Face program draws a face by overriding the paint. Component method of a panel q See Smiling. Face. Panel. java q See Smiling. Face. java q The main method of the Smiling. Face class instantiates a Smiling. Face. Panel and displays it q The Smiling. Face. Panel class is derived from the JPanel class using inheritance 7 -33 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
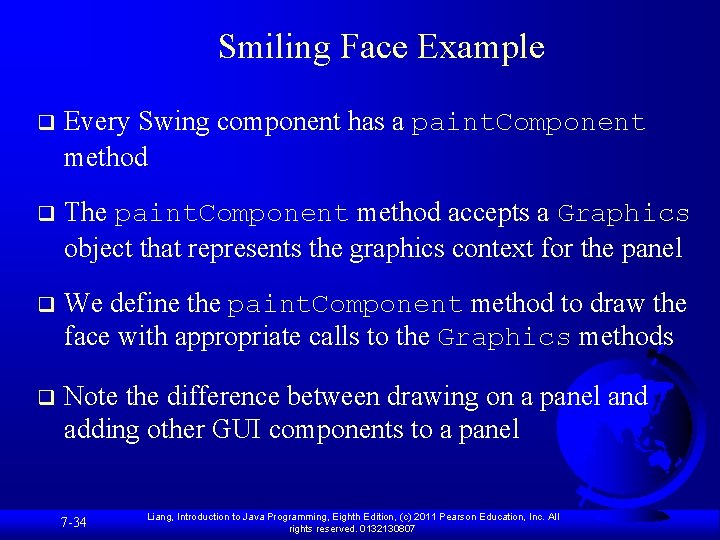
Smiling Face Example q Every Swing component has a paint. Component method q The paint. Component method accepts a Graphics object that represents the graphics context for the panel q We define the paint. Component method to draw the face with appropriate calls to the Graphics methods q Note the difference between drawing on a panel and adding other GUI components to a panel 7 -34 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
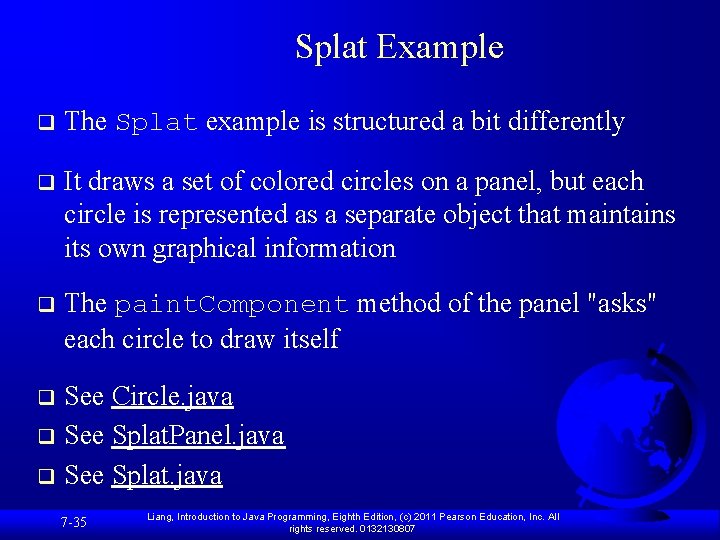
Splat Example q The Splat example is structured a bit differently q It draws a set of colored circles on a panel, but each circle is represented as a separate object that maintains its own graphical information q The paint. Component method of the panel "asks" each circle to draw itself See Circle. java q See Splat. Panel. java q See Splat. java q 7 -35 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
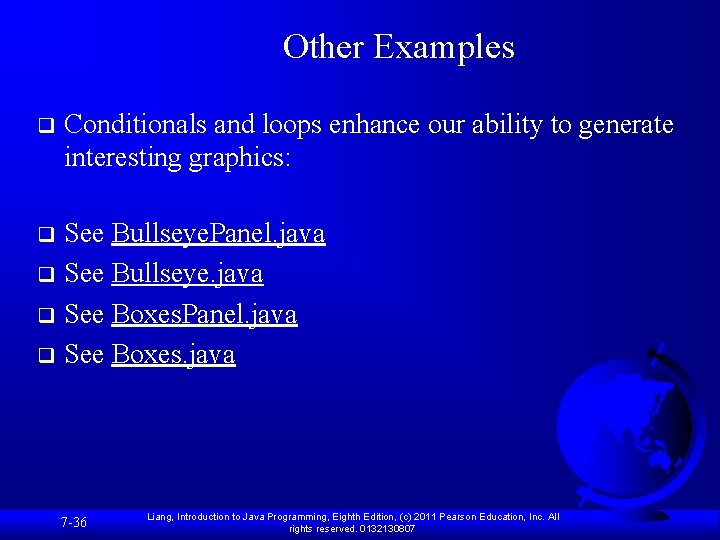
Other Examples q Conditionals and loops enhance our ability to generate interesting graphics: See Bullseye. Panel. java q See Bullseye. java q See Boxes. Panel. java q See Boxes. java q 7 -36 Liang, Introduction to Java Programming, Eighth Edition, (c) 2011 Pearson Education, Inc. All rights reserved. 0132130807
Import java.awt.* import java.awt.event.*
Perbedaan swing dan awt
Abstract
Java awt swing
Java applet swing
Diff between awt and swing
Swing vs awt
Perbedaan xml dan html
Graphics and guis with matlab
Characteristics of a graphical user interface
Java gui awt
Import java.awt.*
Event
Import java.awt.color;
Java awt layout
Import java.awt.*
Java import javax
Event
Java
Cracus
Java awt button
Awt layout
Import java.awt.*
Java
Java 이미지 출력
Java swing responsive gui
Containers in java swing
Import javax.swing
Java swing
Java gui libraries
Java swing mvc example
Java swing tutorial
Java swing draw text
Null layout in java
Java swing actionlistener multiple buttons
Java swing form example
Yoshi