C Plus Data Structures Nell Dale Chapter 2
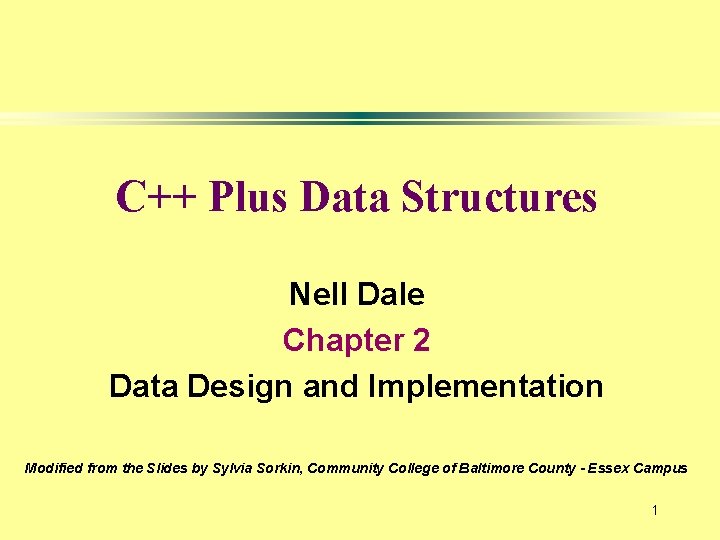
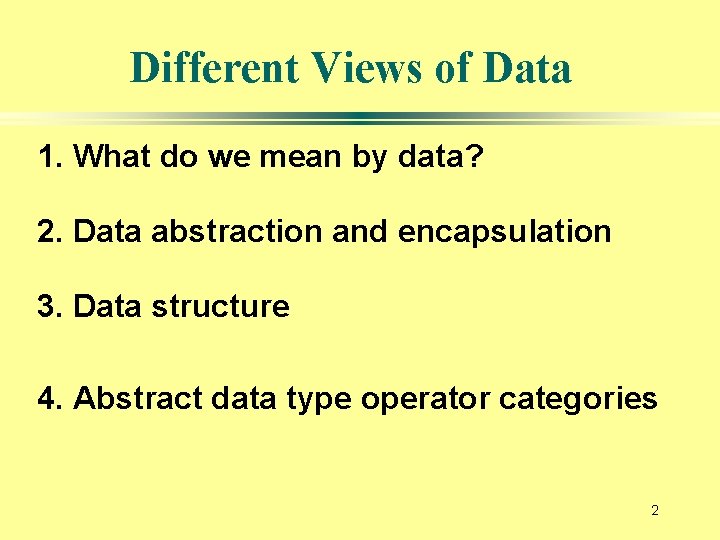
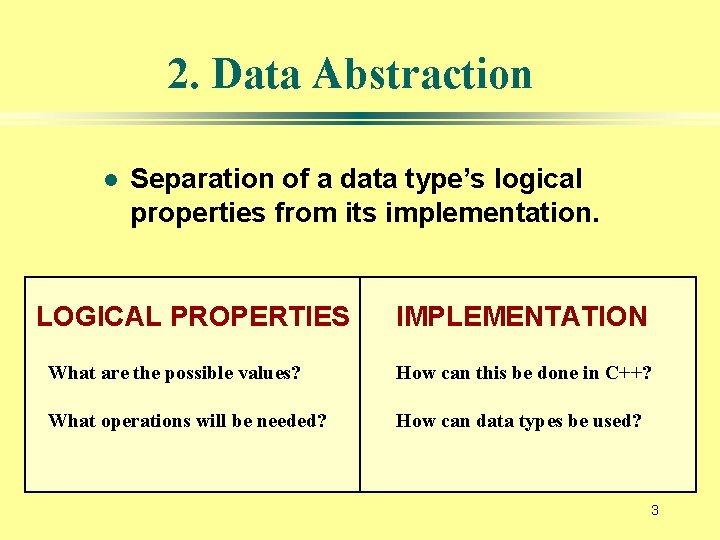
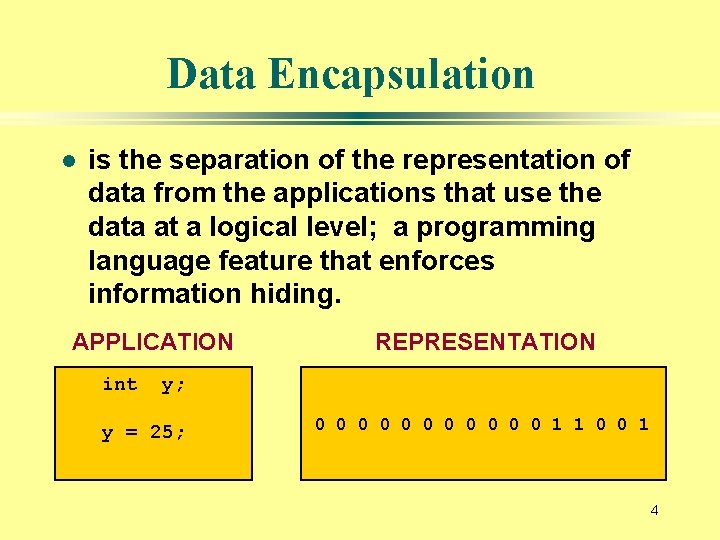
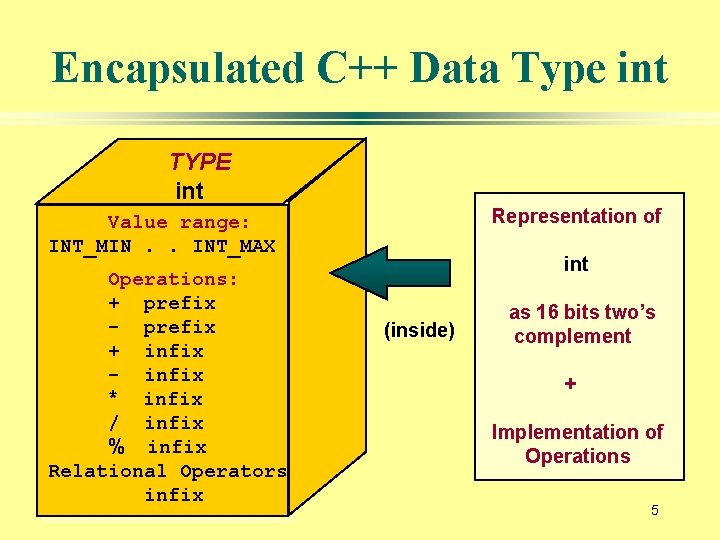
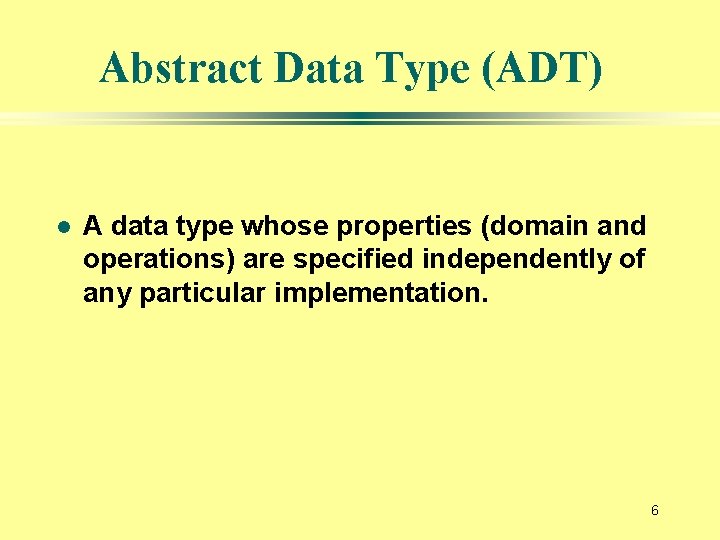
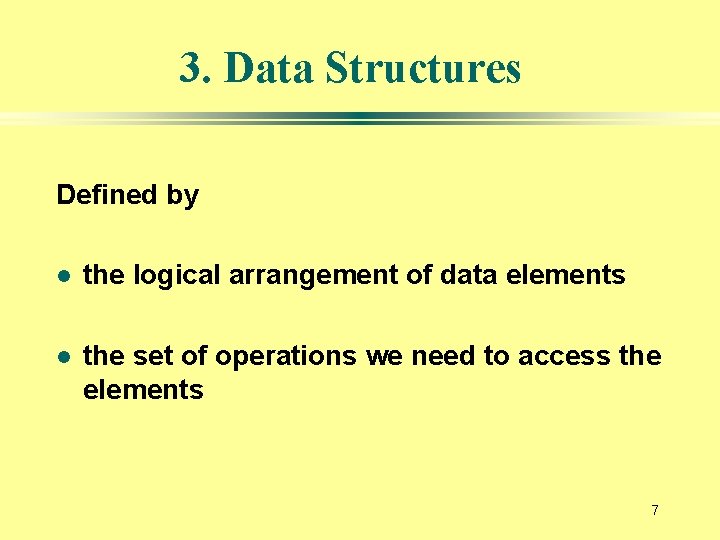
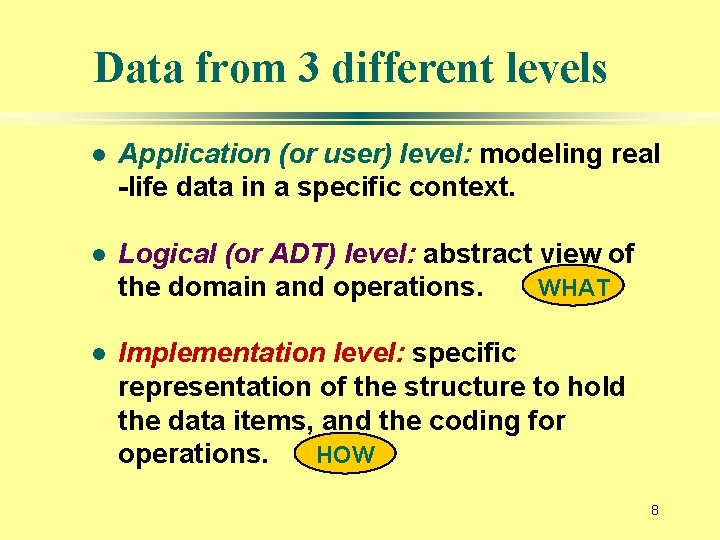
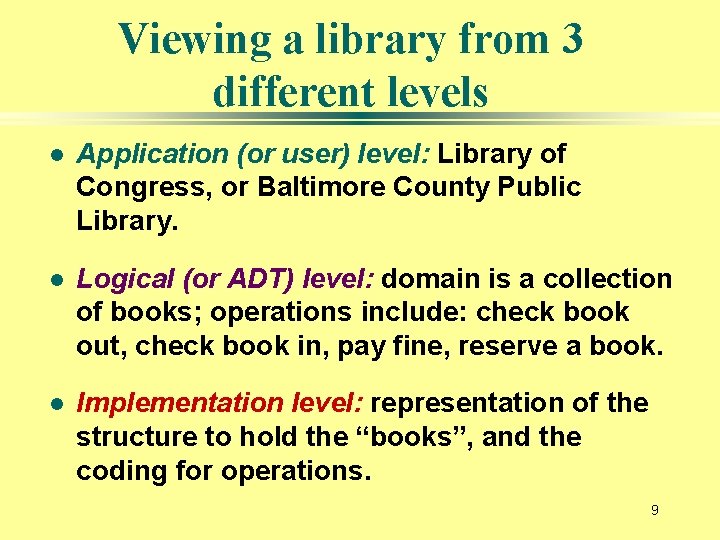
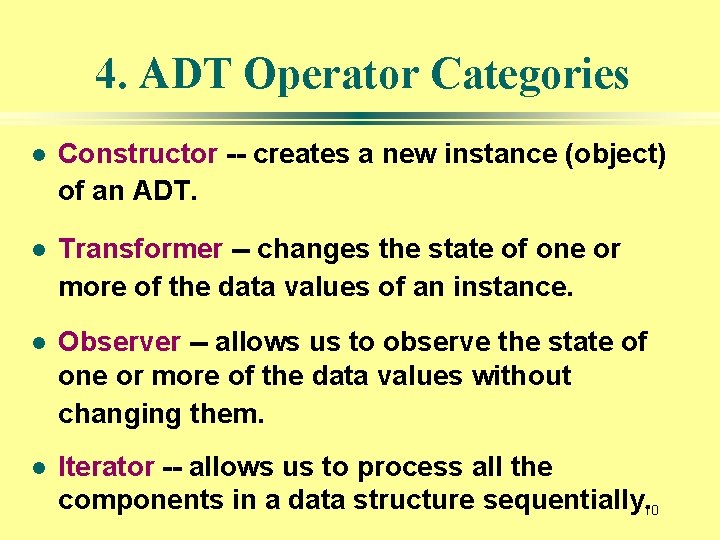
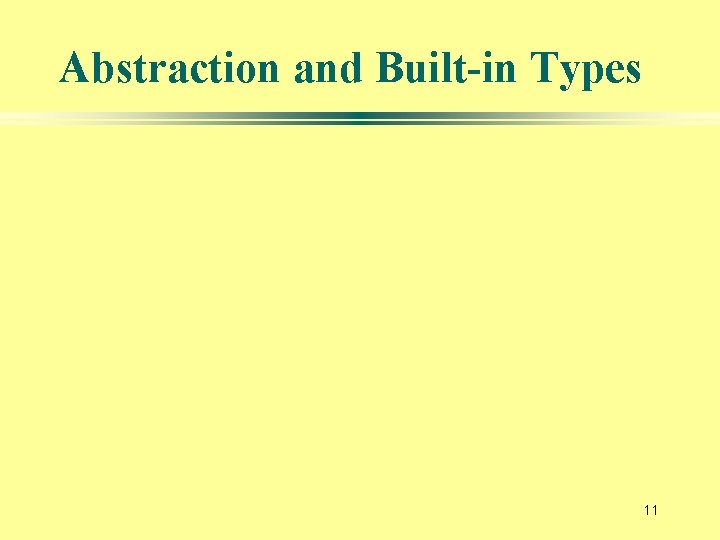
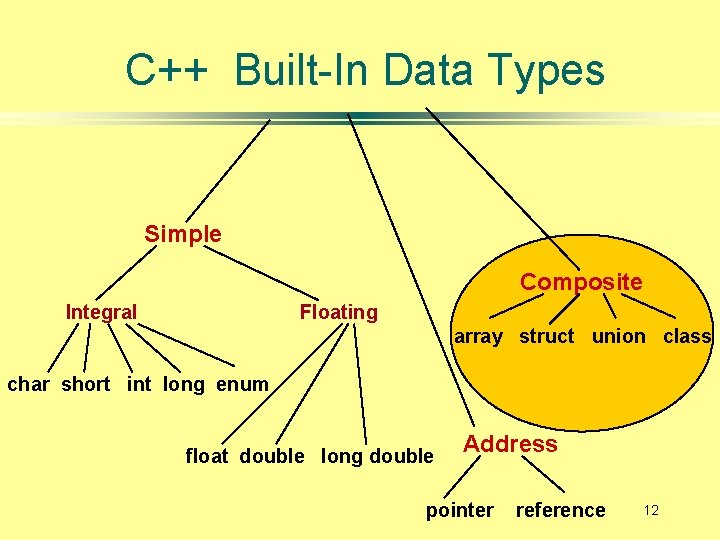
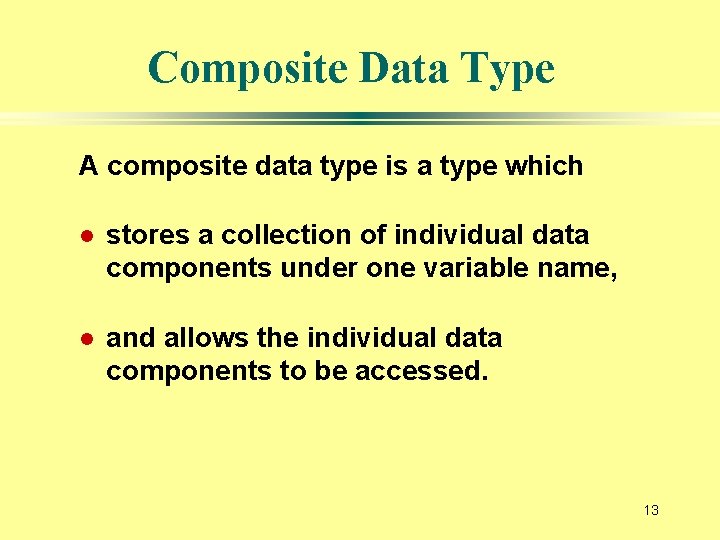
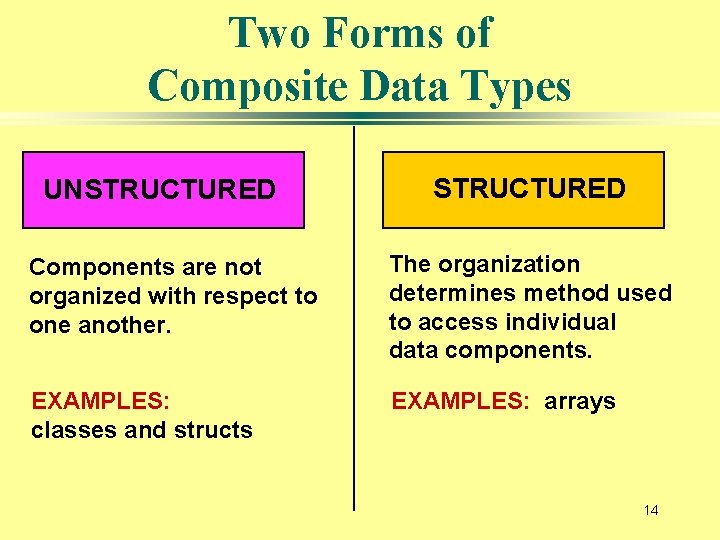
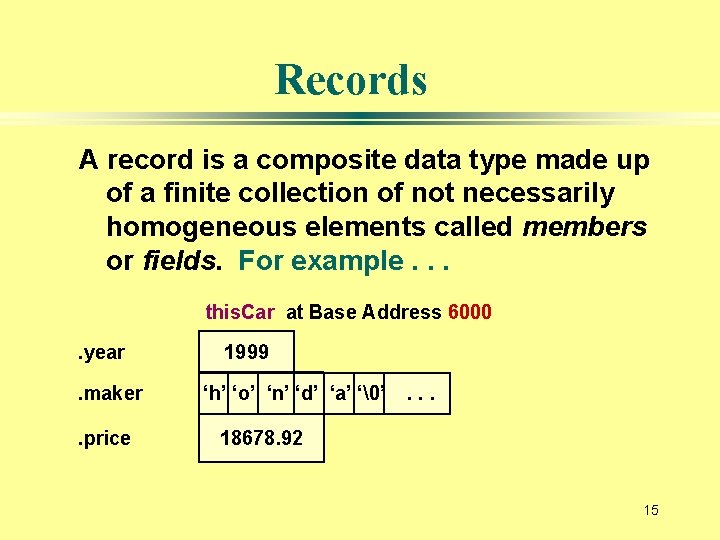
![struct Car. Type struct { Car. Type int char float year ; maker[10]; price struct Car. Type struct { Car. Type int char float year ; maker[10]; price](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-16.jpg)
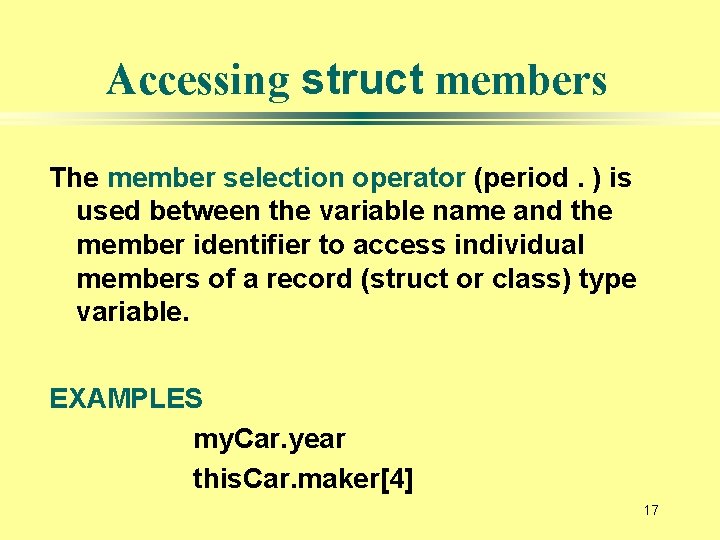
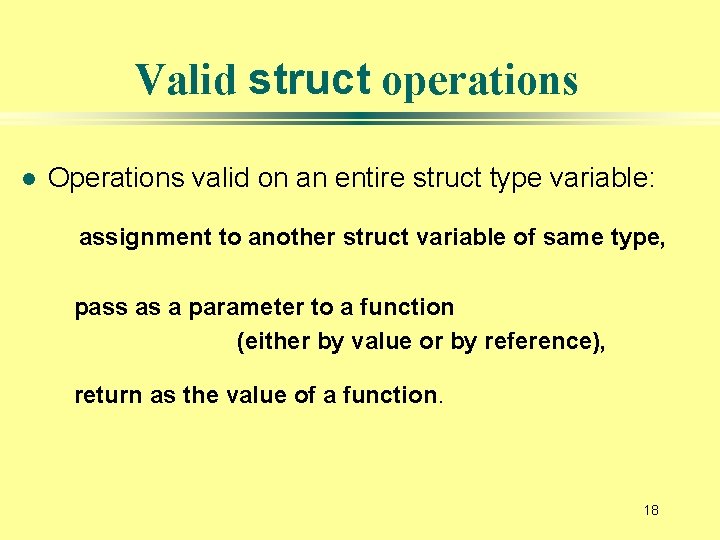
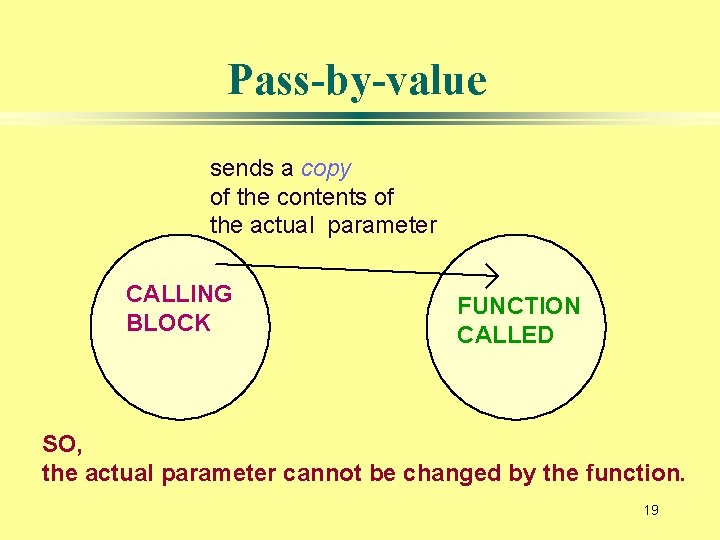
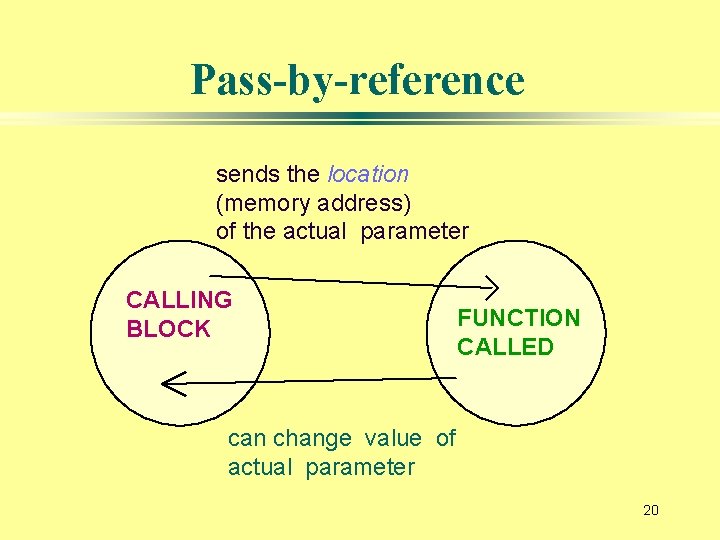
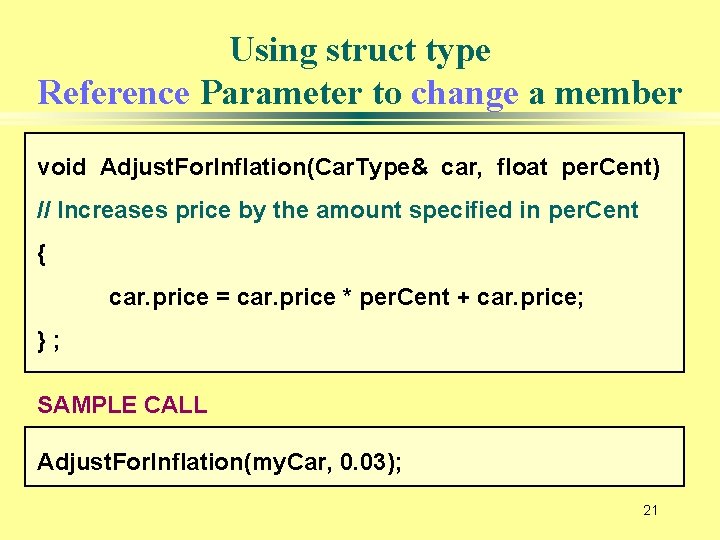
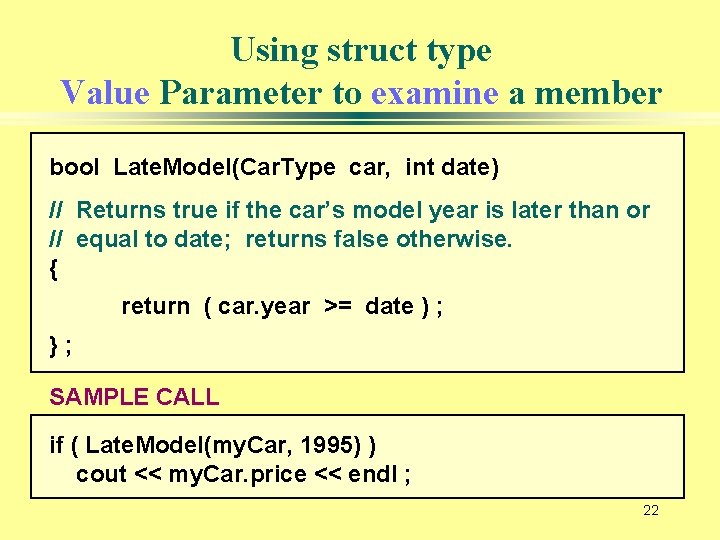
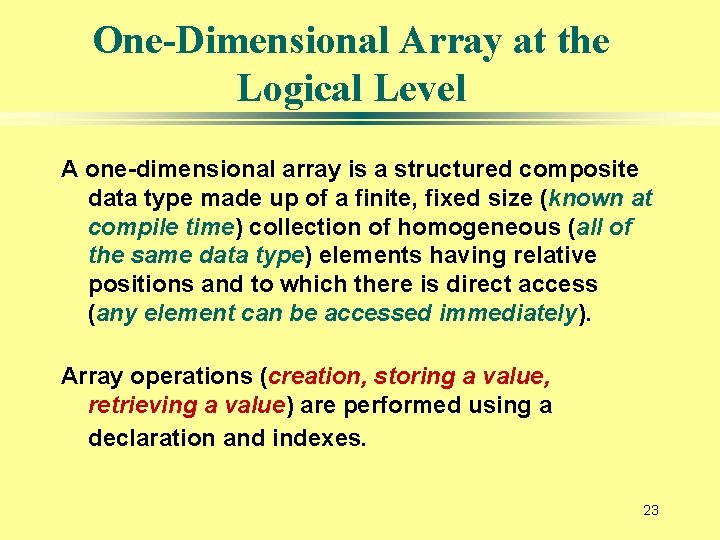
![Implementation Example This ACCESSING FUNCTION gives position of values[Index] Address(Index) = Base. Address + Implementation Example This ACCESSING FUNCTION gives position of values[Index] Address(Index) = Base. Address +](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-24.jpg)
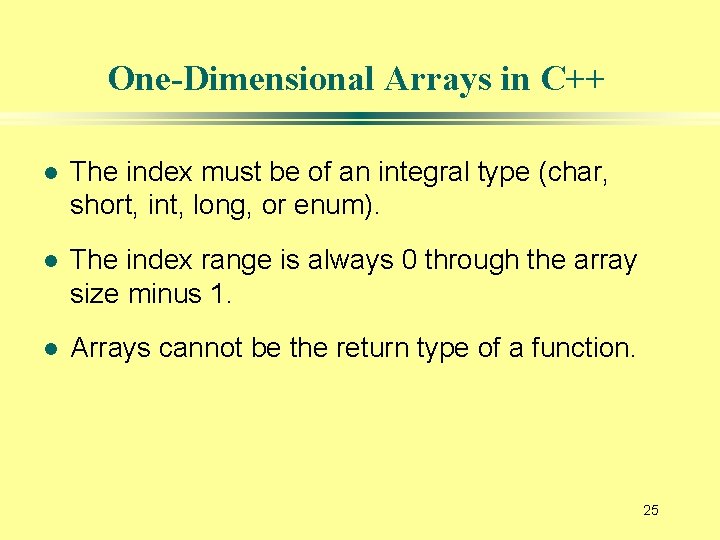
![Another Example This ACCESSING FUNCTION gives position of name[Index] Address(Index) = Base. Address + Another Example This ACCESSING FUNCTION gives position of name[Index] Address(Index) = Base. Address +](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-26.jpg)
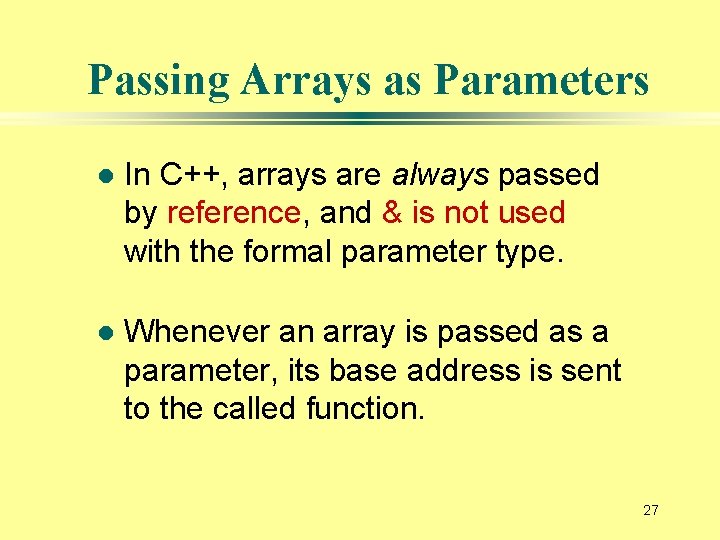
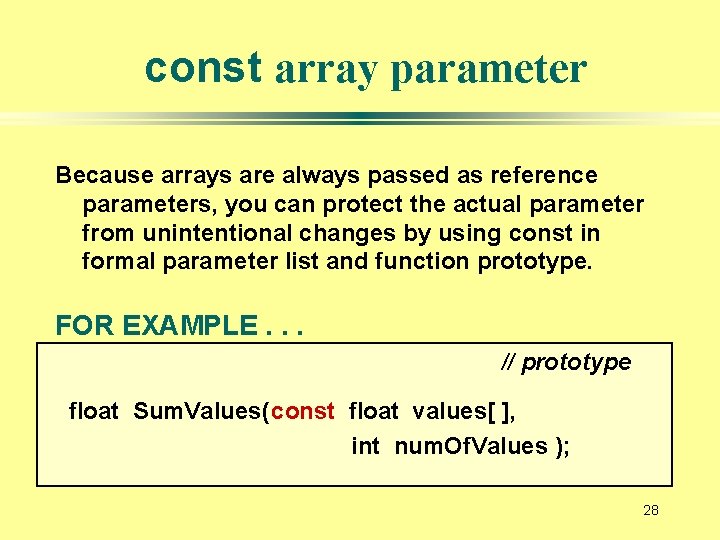
![float Sum. Values (const float values[ ], int num. Of. Values ) // Pre: float Sum. Values (const float values[ ], int num. Of. Values ) // Pre:](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-29.jpg)
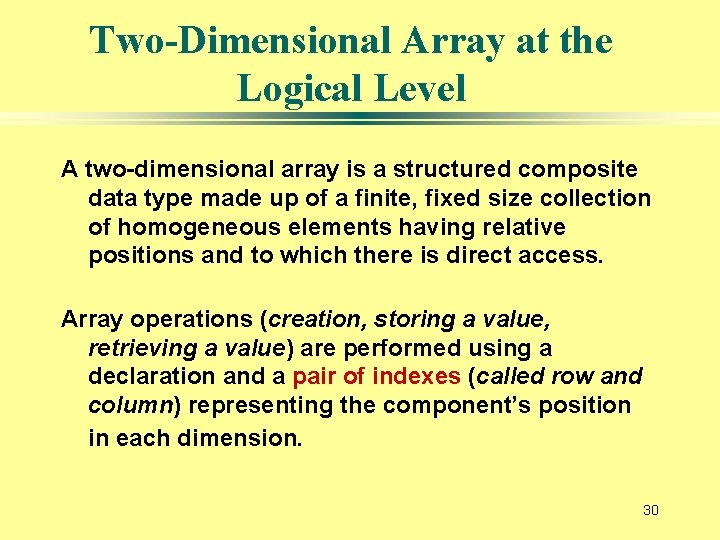
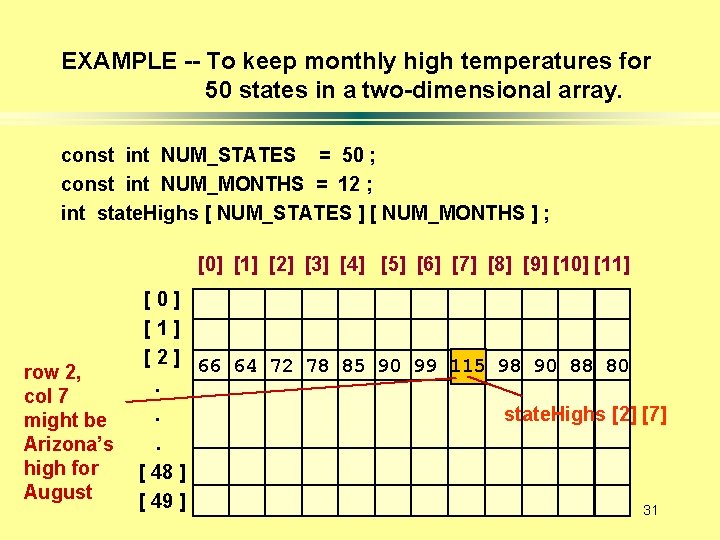
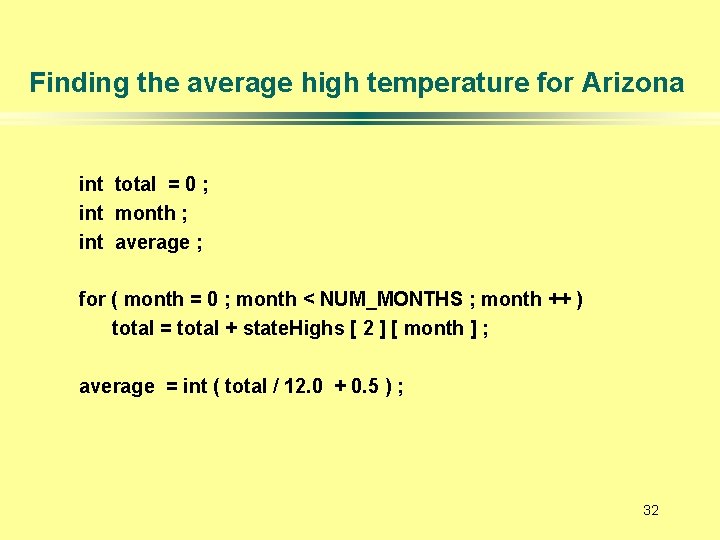
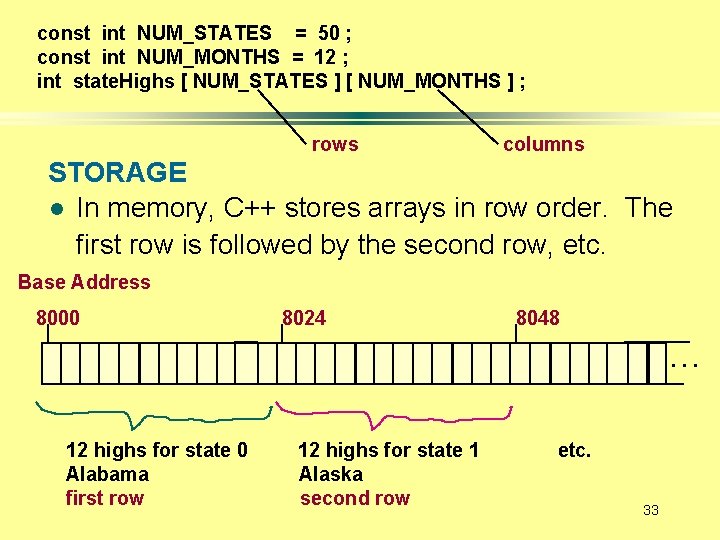
![Implementation Level View state. Highs[ 0 ] [ 1 ] state. Highs[ 0 ] Implementation Level View state. Highs[ 0 ] [ 1 ] state. Highs[ 0 ]](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-34.jpg)
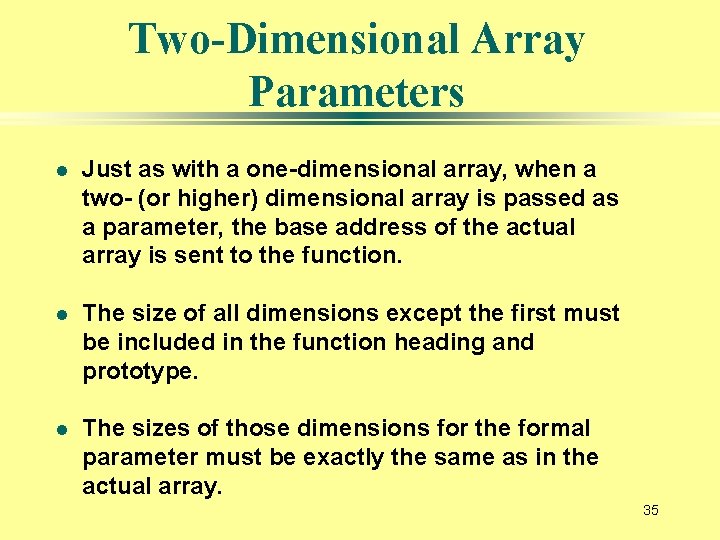
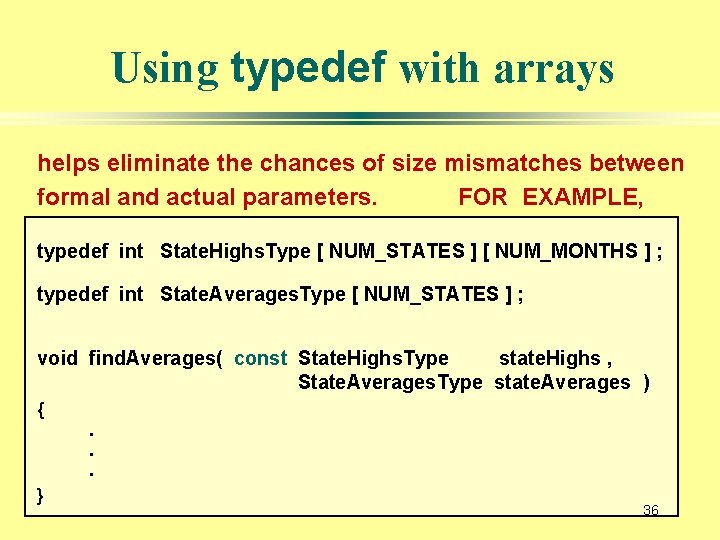
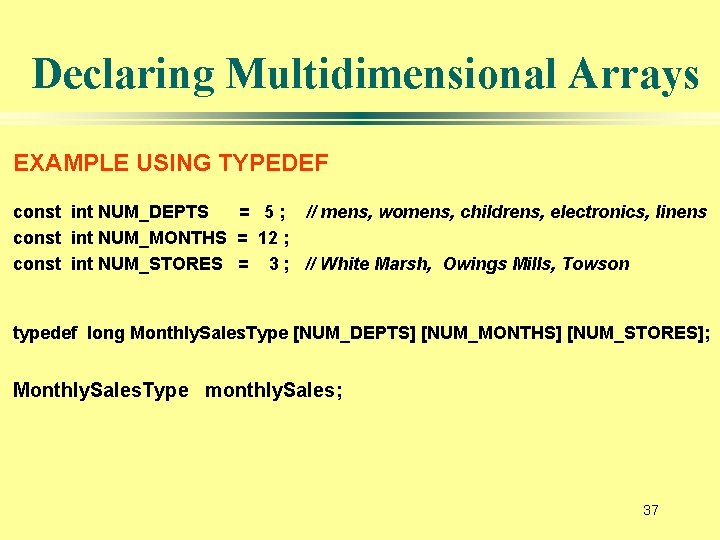
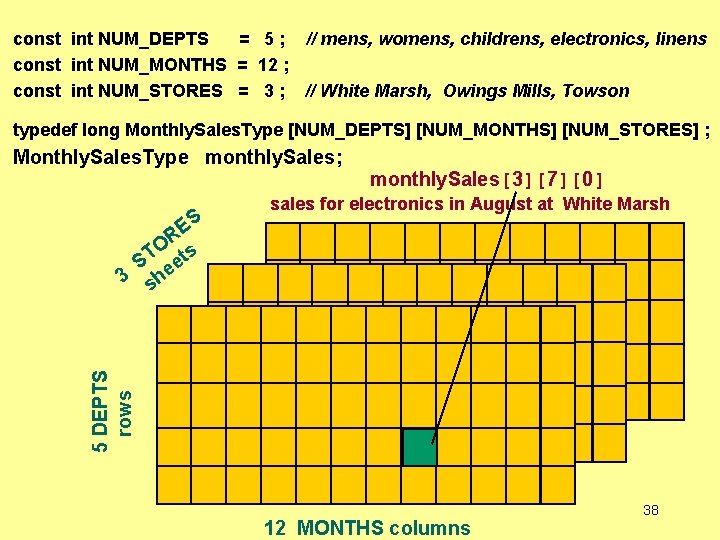
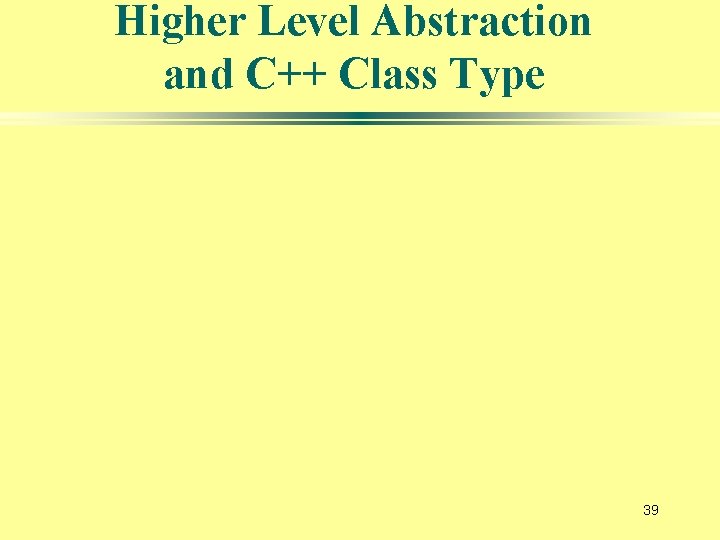
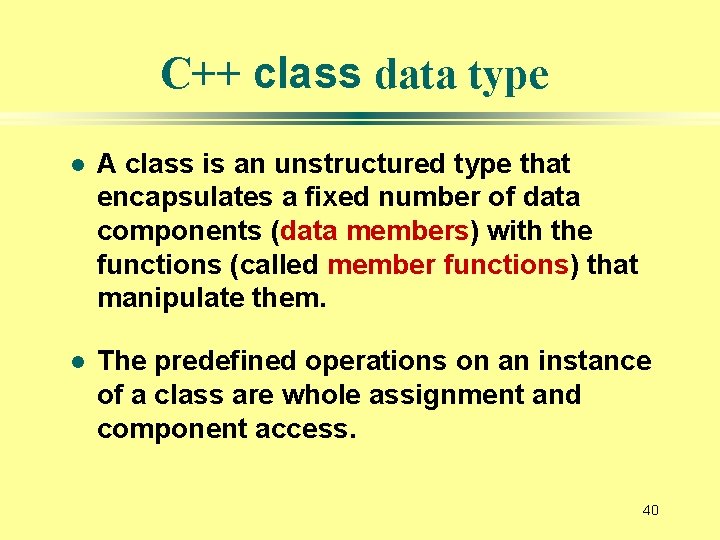
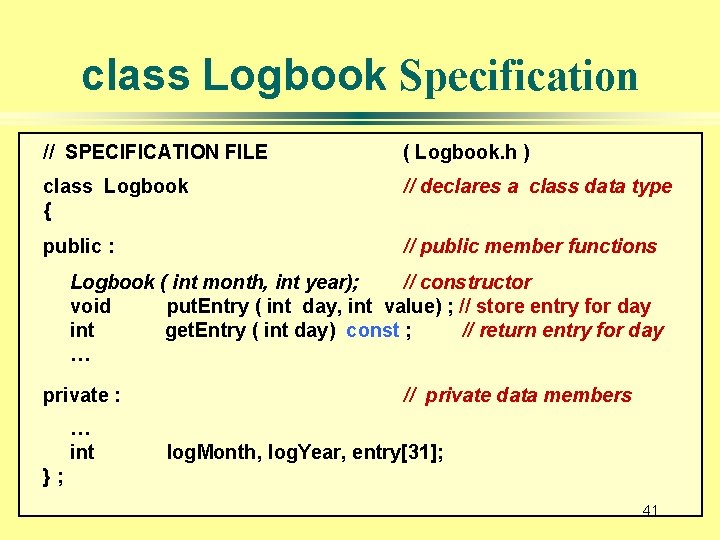
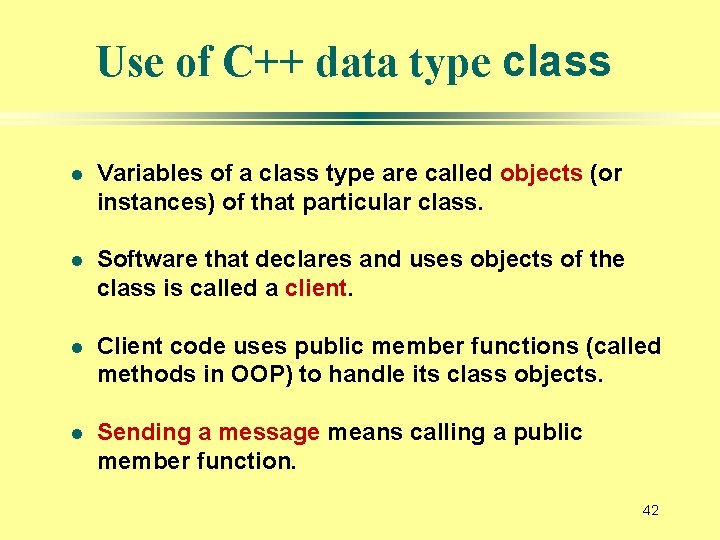
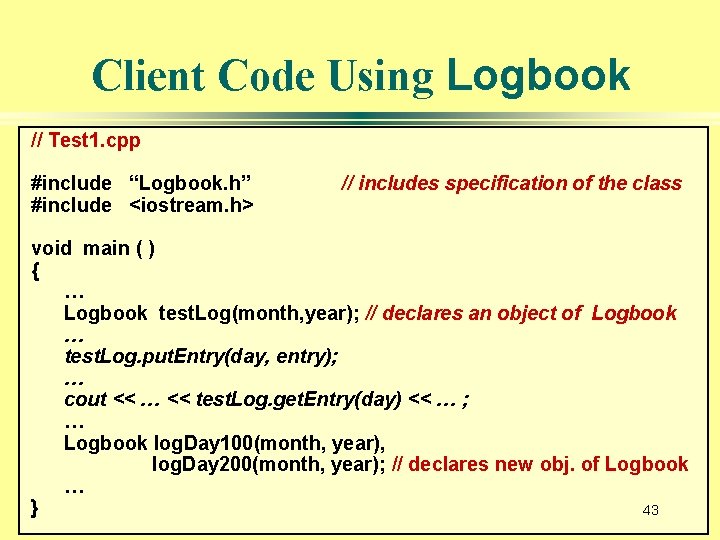
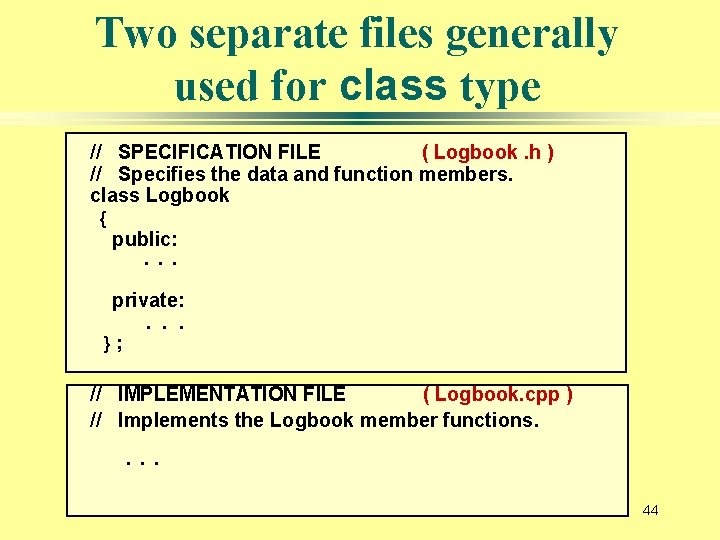
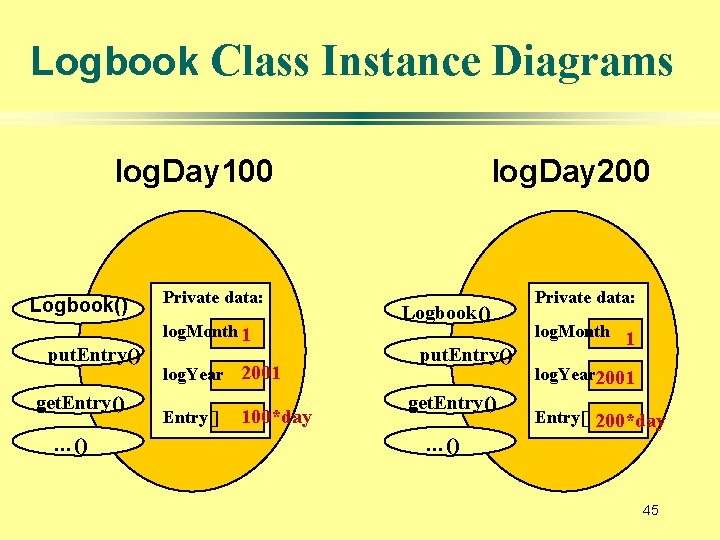
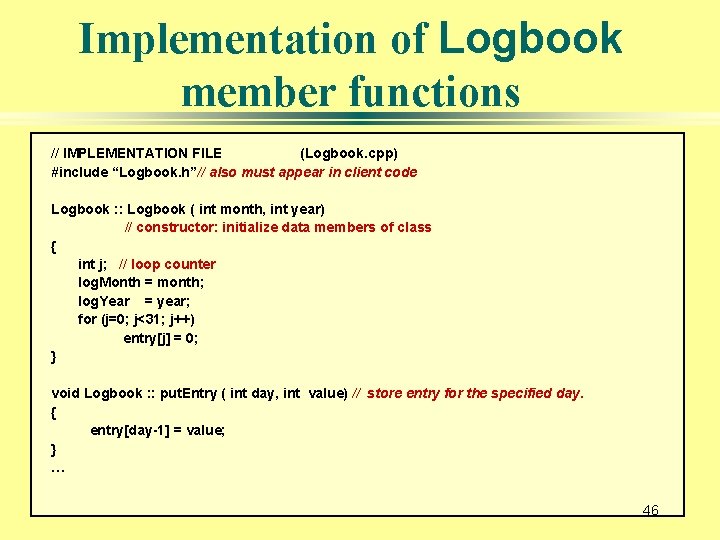
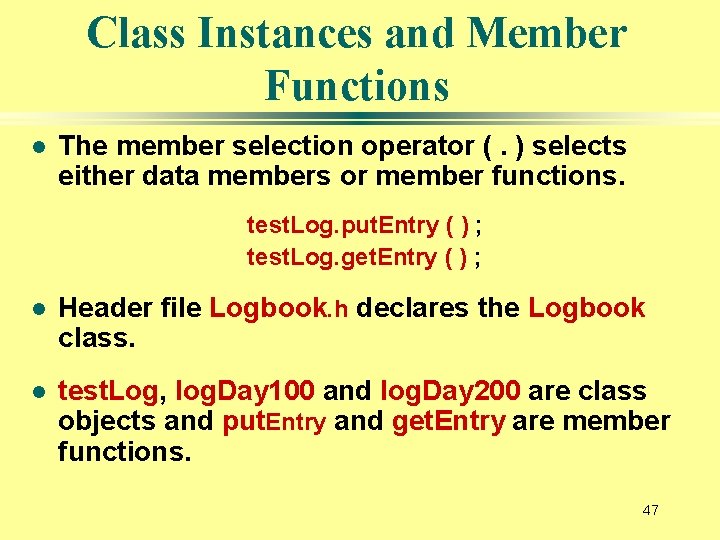
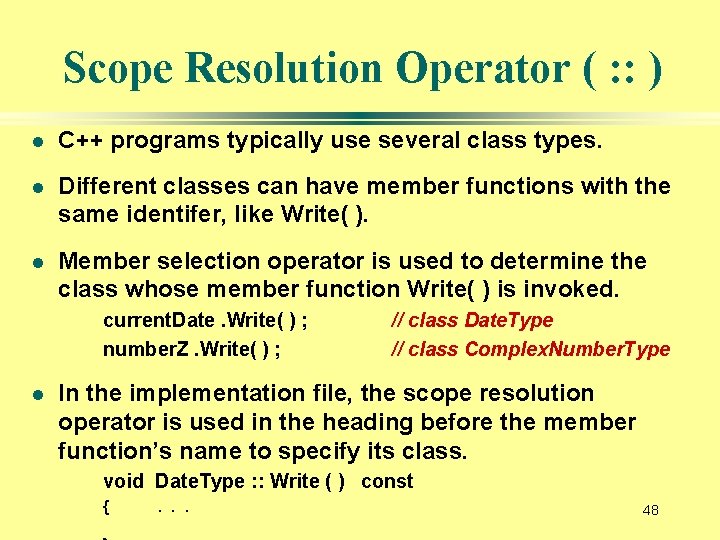
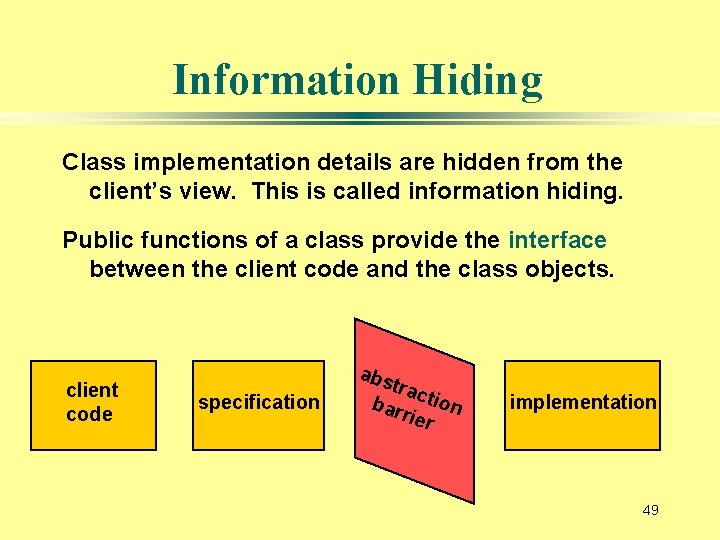
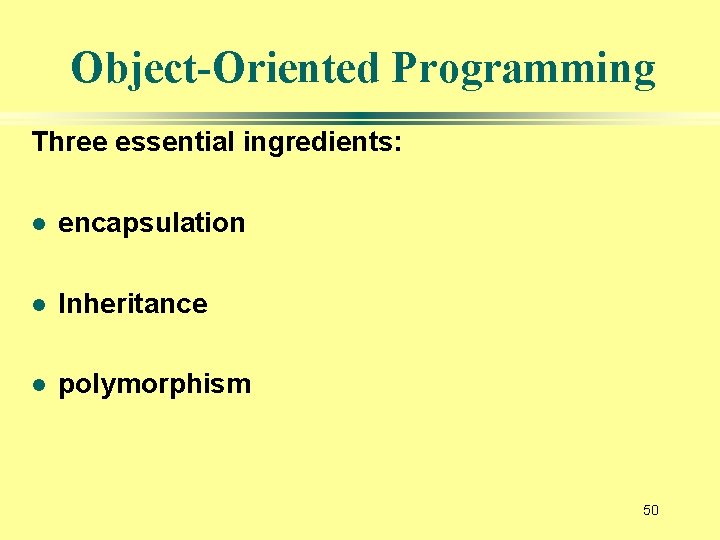
- Slides: 50
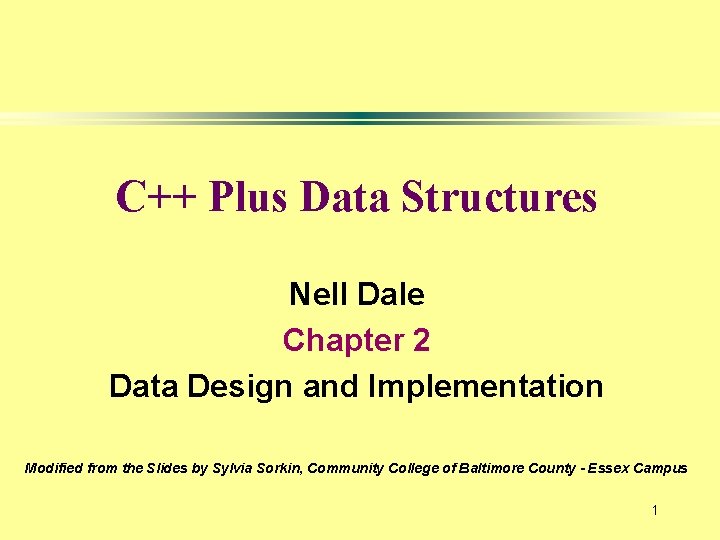
C++ Plus Data Structures Nell Dale Chapter 2 Data Design and Implementation Modified from the Slides by Sylvia Sorkin, Community College of Baltimore County - Essex Campus 1
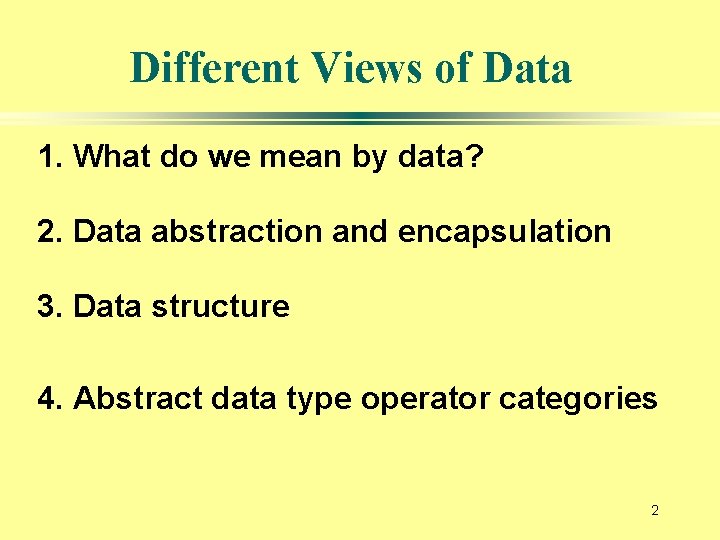
Different Views of Data 1. What do we mean by data? 2. Data abstraction and encapsulation 3. Data structure 4. Abstract data type operator categories 2
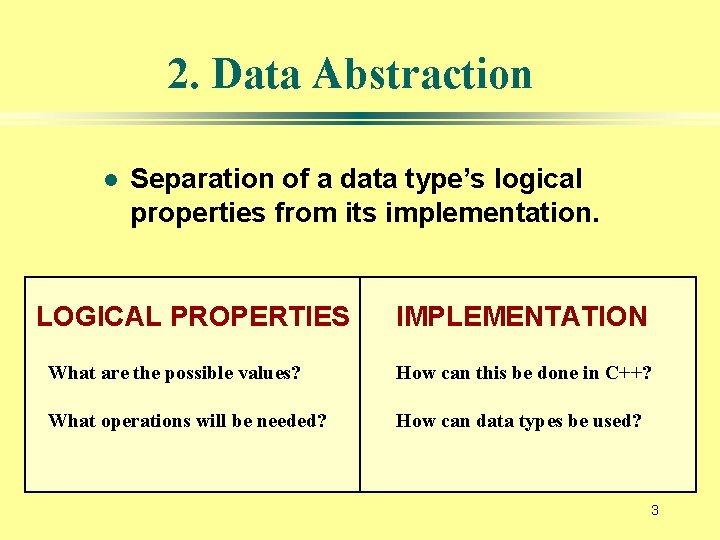
2. Data Abstraction l Separation of a data type’s logical properties from its implementation. LOGICAL PROPERTIES IMPLEMENTATION What are the possible values? How can this be done in C++? What operations will be needed? How can data types be used? 3
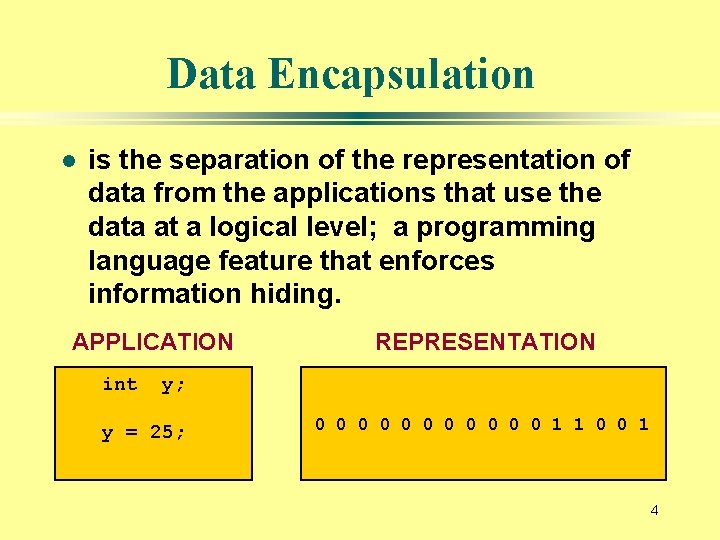
Data Encapsulation l is the separation of the representation of data from the applications that use the data at a logical level; a programming language feature that enforces information hiding. APPLICATION int REPRESENTATION y; y = 25; 0 0 0 1 1 0 0 1 4
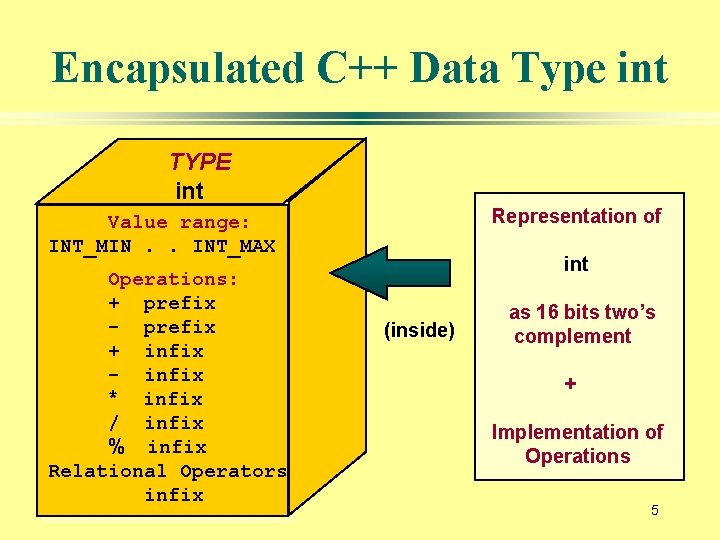
Encapsulated C++ Data Type int TYPE int Representation of Value range: INT_MIN. . INT_MAX Operations: + prefix - prefix + infix - infix * infix / infix % infix Relational Operators infix int (inside) as 16 bits two’s complement + Implementation of Operations 5
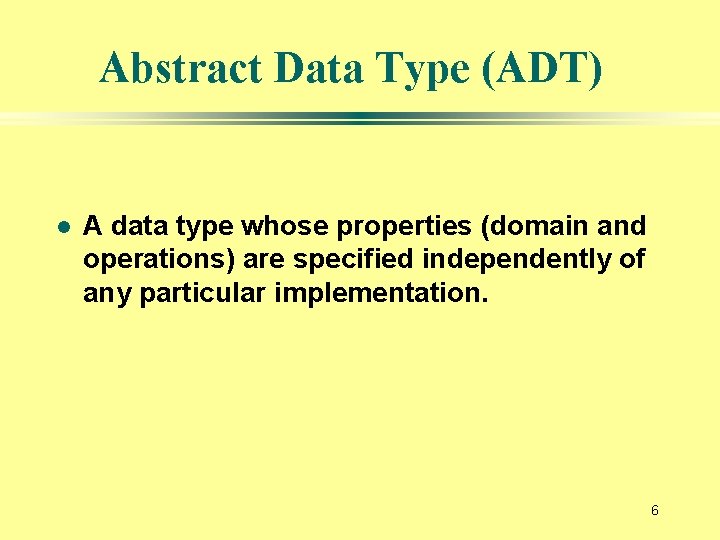
Abstract Data Type (ADT) l A data type whose properties (domain and operations) are specified independently of any particular implementation. 6
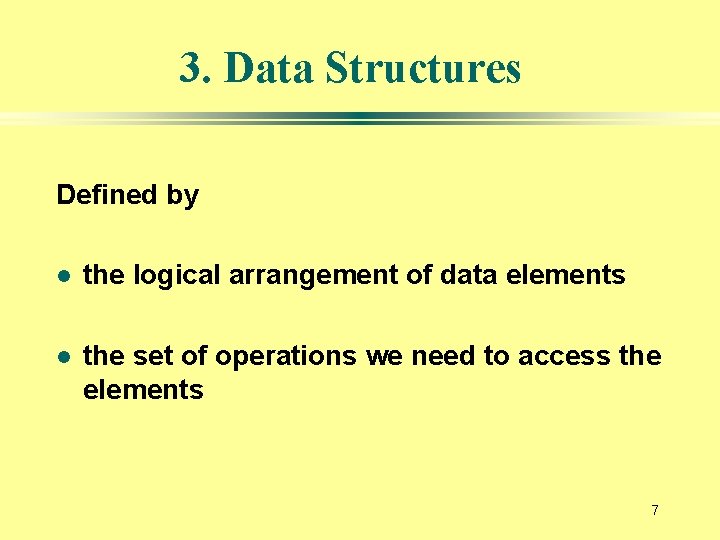
3. Data Structures Defined by l the logical arrangement of data elements l the set of operations we need to access the elements 7
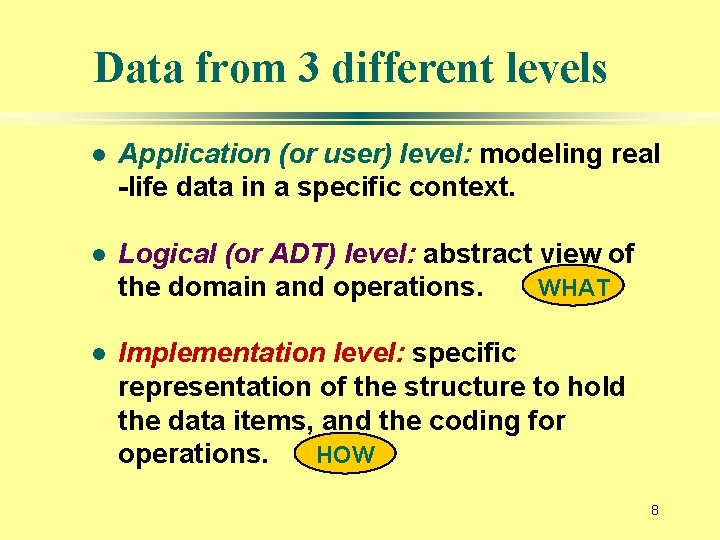
Data from 3 different levels l Application (or user) level: modeling real -life data in a specific context. l Logical (or ADT) level: abstract view of the domain and operations. WHAT l Implementation level: specific representation of the structure to hold the data items, and the coding for operations. HOW 8
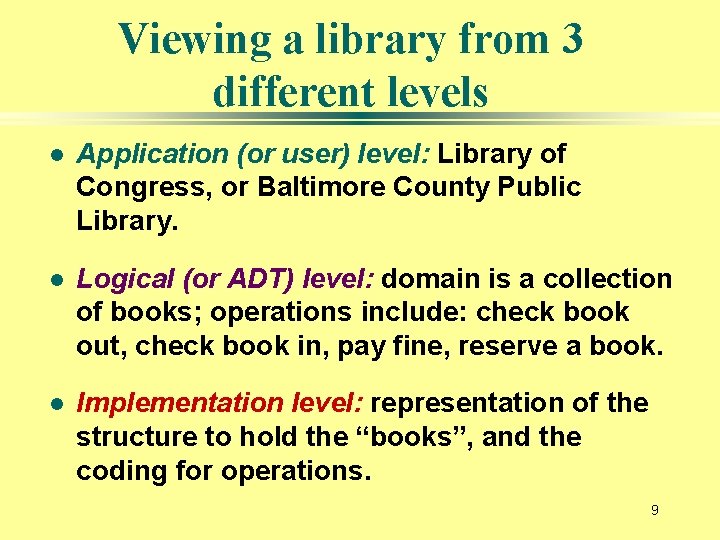
Viewing a library from 3 different levels l Application (or user) level: Library of Congress, or Baltimore County Public Library. l Logical (or ADT) level: domain is a collection of books; operations include: check book out, check book in, pay fine, reserve a book. l Implementation level: representation of the structure to hold the “books”, and the coding for operations. 9
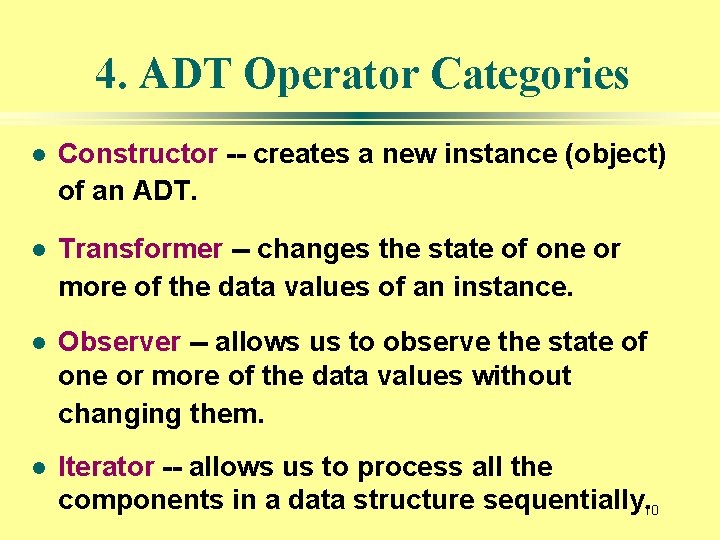
4. ADT Operator Categories l Constructor -- creates a new instance (object) of an ADT. l Transformer -- changes the state of one or more of the data values of an instance. l Observer -- allows us to observe the state of one or more of the data values without changing them. l Iterator -- allows us to process all the components in a data structure sequentially. 10
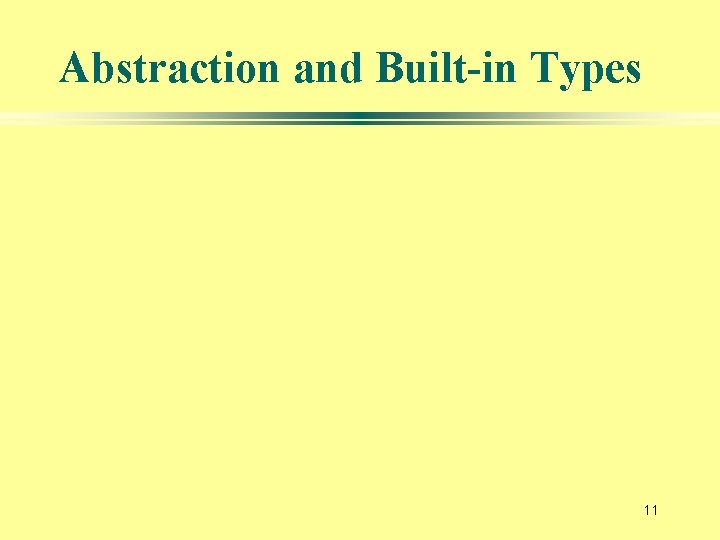
Abstraction and Built-in Types 11
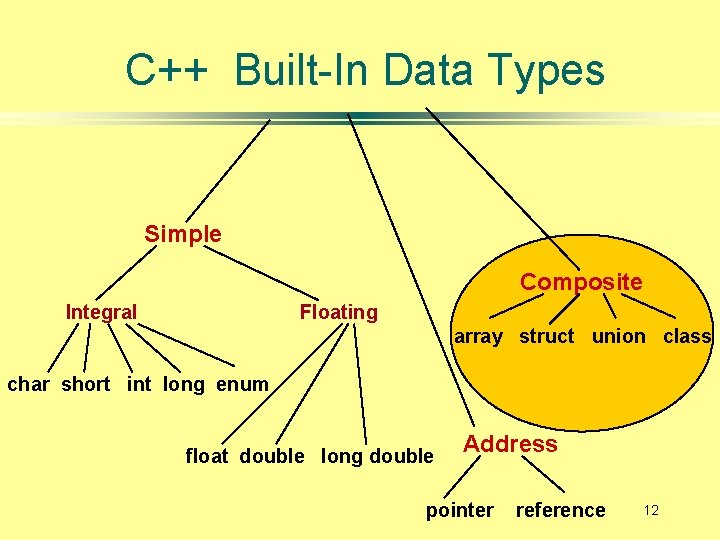
C++ Built-In Data Types Simple Composite Integral Floating array struct union class char short int long enum float double long double Address pointer reference 12
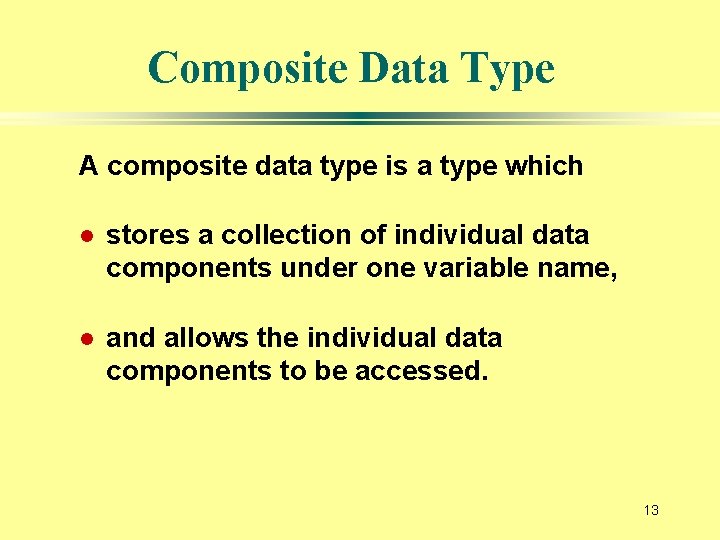
Composite Data Type A composite data type is a type which l stores a collection of individual data components under one variable name, l and allows the individual data components to be accessed. 13
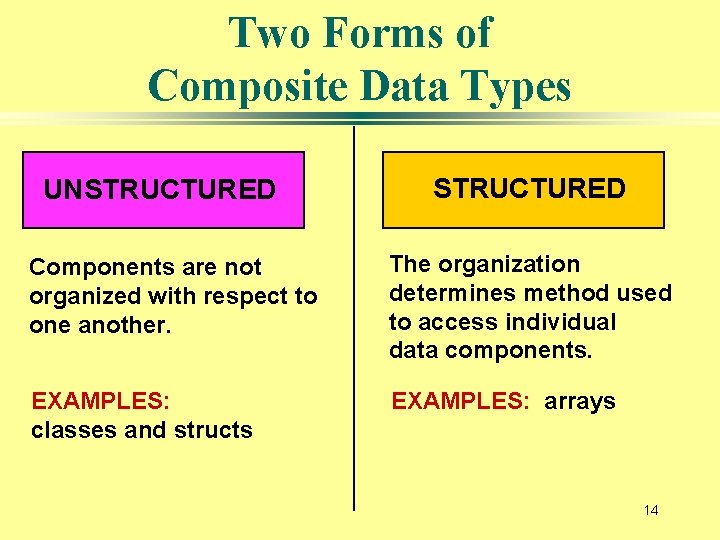
Two Forms of Composite Data Types UNSTRUCTURED Components are not organized with respect to one another. The organization determines method used to access individual data components. EXAMPLES: classes and structs EXAMPLES: arrays 14
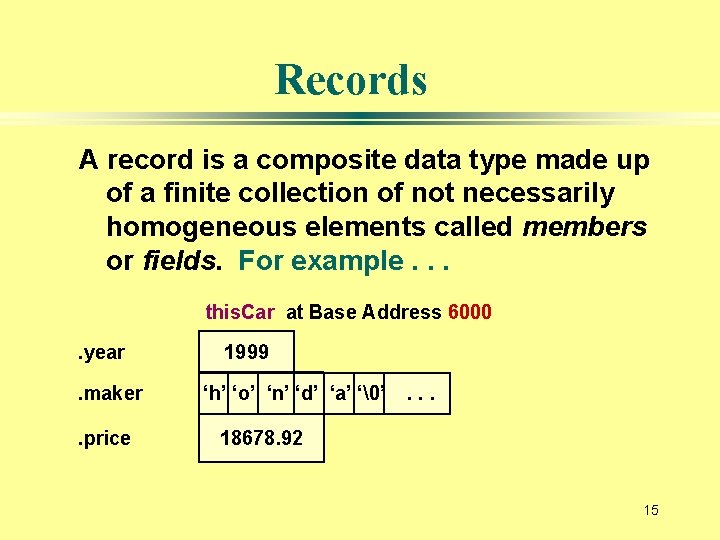
Records A record is a composite data type made up of a finite collection of not necessarily homogeneous elements called members or fields. For example. . . this. Car at Base Address 6000. year. maker. price 1999 ‘h’ ‘o’ ‘n’ ‘d’ ‘a’ ‘ ’ . . . 18678. 92 15
![struct Car Type struct Car Type int char float year maker10 price struct Car. Type struct { Car. Type int char float year ; maker[10]; price](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-16.jpg)
struct Car. Type struct { Car. Type int char float year ; maker[10]; price ; } ; Car. Type this. Car; my. Car; //Car. Type variables 16
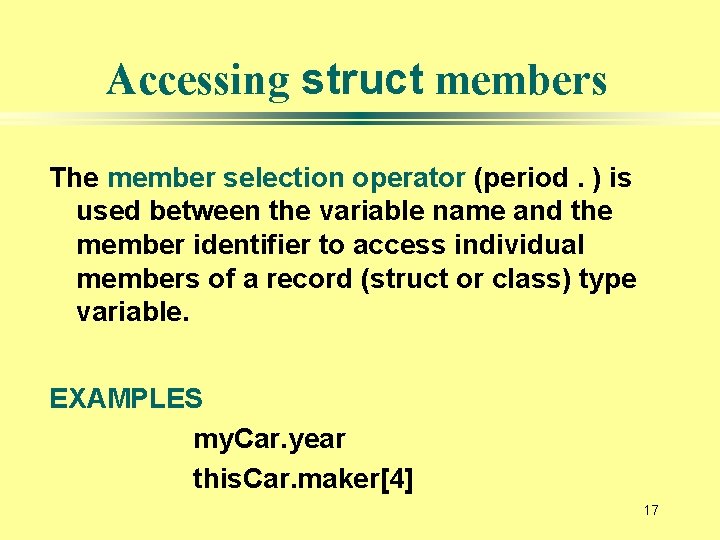
Accessing struct members The member selection operator (period. ) is used between the variable name and the member identifier to access individual members of a record (struct or class) type variable. EXAMPLES my. Car. year this. Car. maker[4] 17
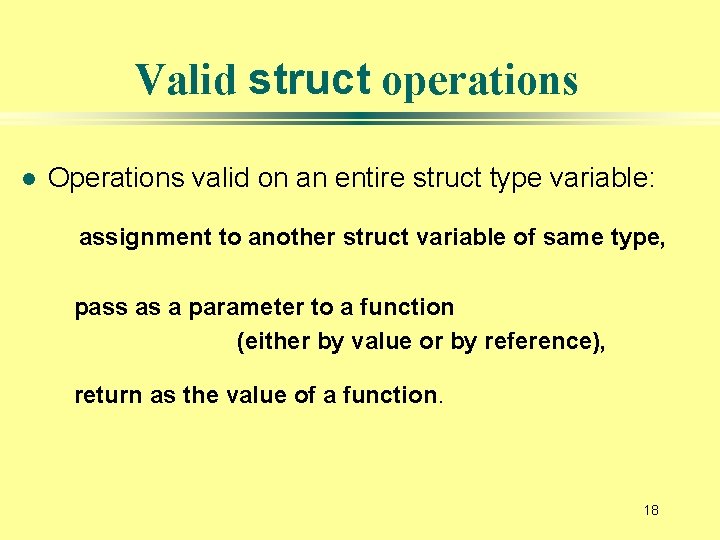
Valid struct operations l Operations valid on an entire struct type variable: assignment to another struct variable of same type, pass as a parameter to a function (either by value or by reference), return as the value of a function. 18
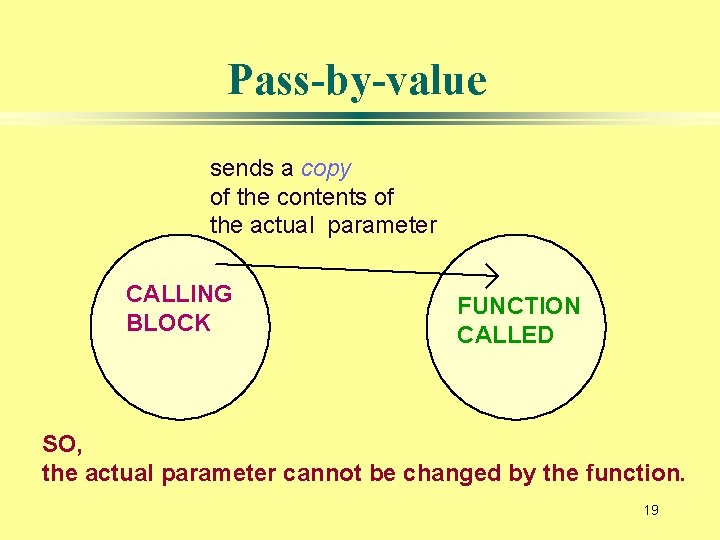
Pass-by-value sends a copy of the contents of the actual parameter CALLING BLOCK FUNCTION CALLED SO, the actual parameter cannot be changed by the function. 19
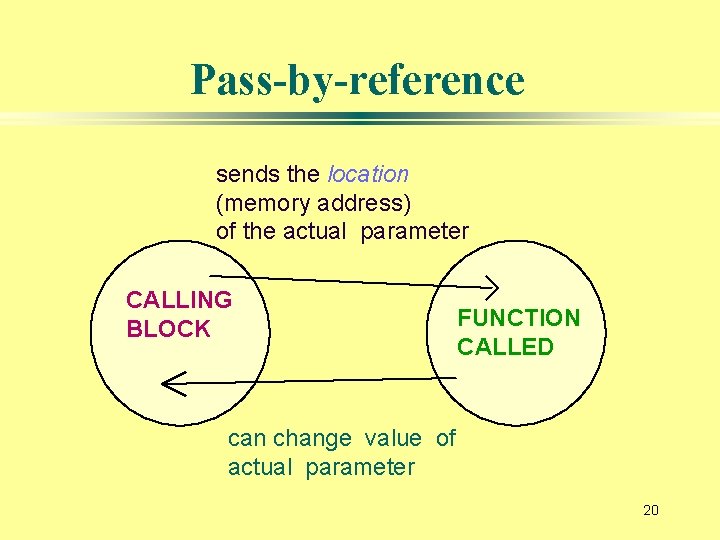
Pass-by-reference sends the location (memory address) of the actual parameter CALLING BLOCK FUNCTION CALLED can change value of actual parameter 20
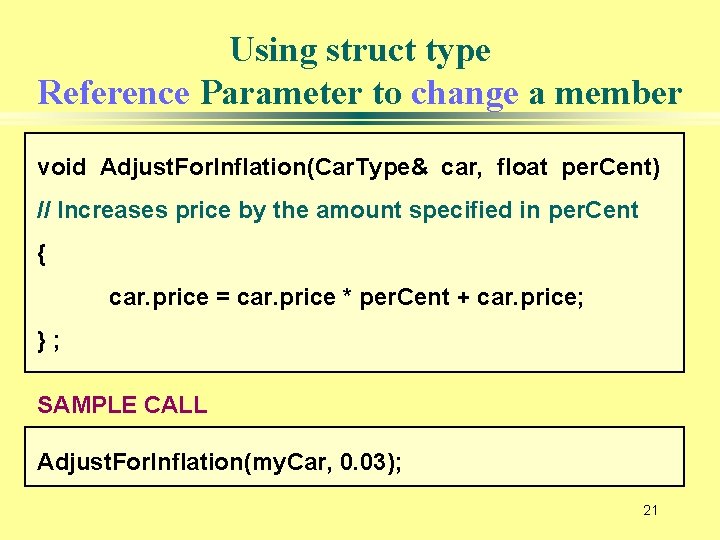
Using struct type Reference Parameter to change a member void Adjust. For. Inflation(Car. Type& car, float per. Cent) // Increases price by the amount specified in per. Cent { car. price = car. price * per. Cent + car. price; }; SAMPLE CALL Adjust. For. Inflation(my. Car, 0. 03); 21
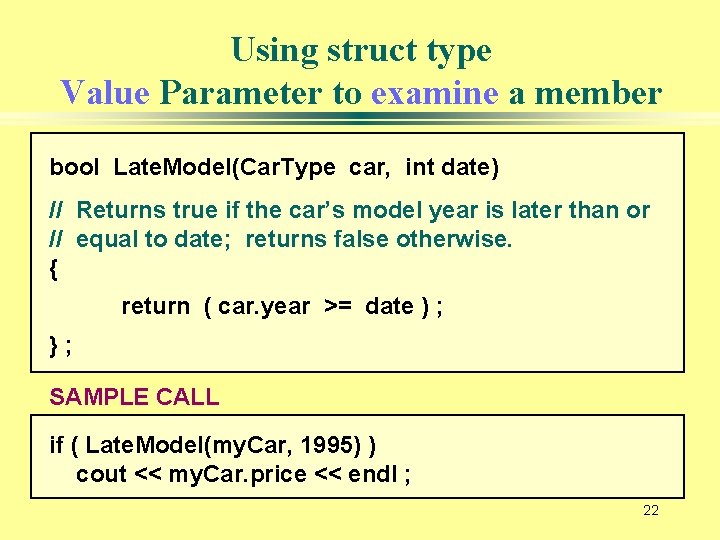
Using struct type Value Parameter to examine a member bool Late. Model(Car. Type car, int date) // Returns true if the car’s model year is later than or // equal to date; returns false otherwise. { return ( car. year >= date ) ; }; SAMPLE CALL if ( Late. Model(my. Car, 1995) ) cout << my. Car. price << endl ; 22
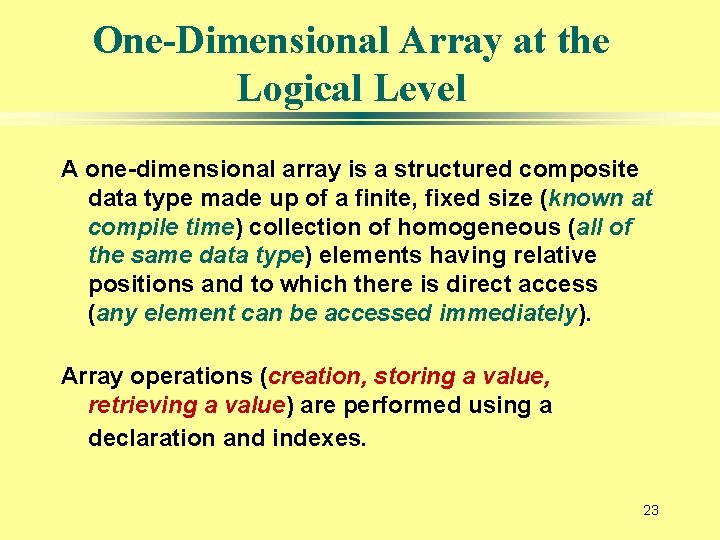
One-Dimensional Array at the Logical Level A one-dimensional array is a structured composite data type made up of a finite, fixed size (known at compile time) collection of homogeneous (all of the same data type) elements having relative positions and to which there is direct access (any element can be accessed immediately). Array operations (creation, storing a value, retrieving a value) are performed using a declaration and indexes. 23
![Implementation Example This ACCESSING FUNCTION gives position of valuesIndex AddressIndex Base Address Implementation Example This ACCESSING FUNCTION gives position of values[Index] Address(Index) = Base. Address +](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-24.jpg)
Implementation Example This ACCESSING FUNCTION gives position of values[Index] Address(Index) = Base. Address + Index * Size. Of. Element float values[5]; // assume element size is 4 bytes Base Address 7000 7004 7008 7012 values[0] values[1] values[2] values[3] Indexes 7016 values[4] 24
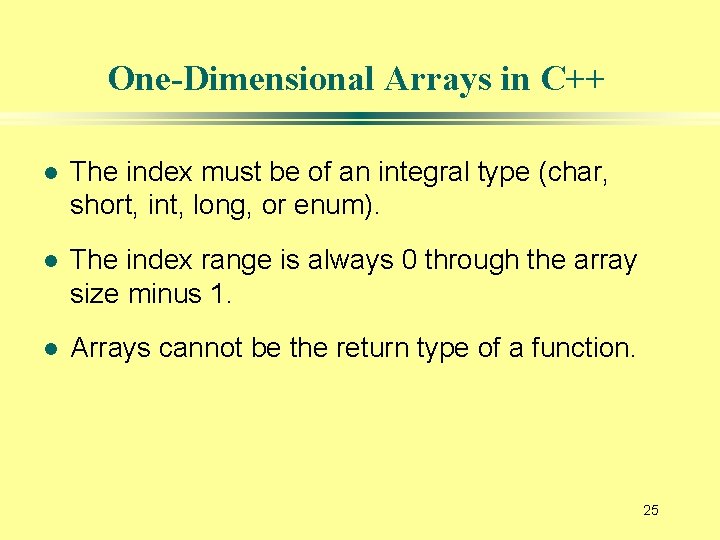
One-Dimensional Arrays in C++ l The index must be of an integral type (char, short, int, long, or enum). l The index range is always 0 through the array size minus 1. l Arrays cannot be the return type of a function. 25
![Another Example This ACCESSING FUNCTION gives position of nameIndex AddressIndex Base Address Another Example This ACCESSING FUNCTION gives position of name[Index] Address(Index) = Base. Address +](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-26.jpg)
Another Example This ACCESSING FUNCTION gives position of name[Index] Address(Index) = Base. Address + Index * Size. Of. Element char name[10]; // assume element size is 1 byte Base Address 6000 6001 6002 6003 6004 name[0] name[1] name[2] name[3] name[4] 6005 6006 6007 . . . 6008 6009 name[9] 26
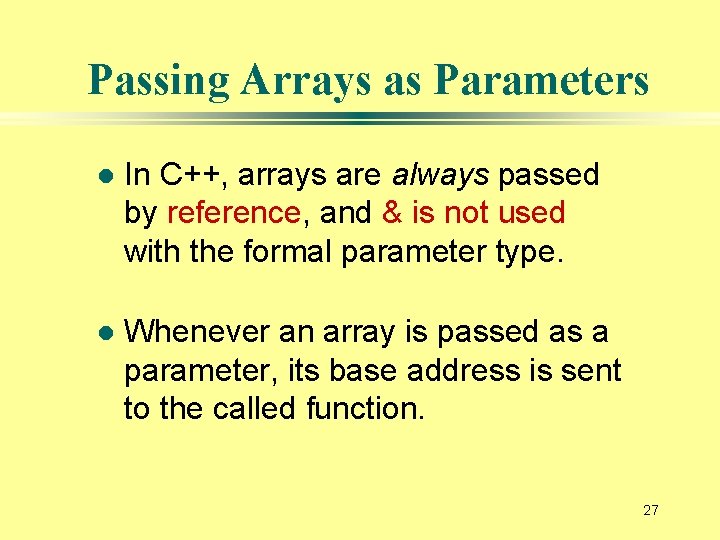
Passing Arrays as Parameters l In C++, arrays are always passed by reference, and & is not used with the formal parameter type. l Whenever an array is passed as a parameter, its base address is sent to the called function. 27
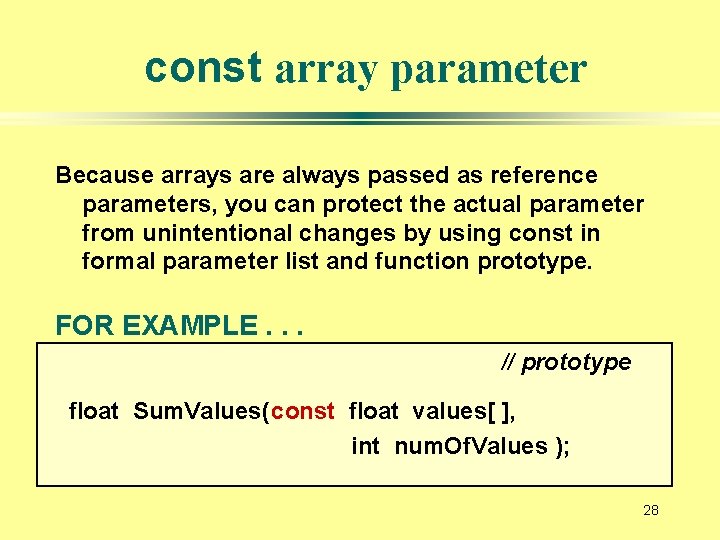
const array parameter Because arrays are always passed as reference parameters, you can protect the actual parameter from unintentional changes by using const in formal parameter list and function prototype. FOR EXAMPLE. . . // prototype float Sum. Values(const float values[ ], int num. Of. Values ); 28
![float Sum Values const float values int num Of Values Pre float Sum. Values (const float values[ ], int num. Of. Values ) // Pre:](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-29.jpg)
float Sum. Values (const float values[ ], int num. Of. Values ) // Pre: values[ 0] through values[num. Of. Values-1] // have been assigned // Returns the sum of values[0] through // values[num. Of. Values-1] { float sum = 0; for ( int index = 0; index < num. Of. Values; index++ ) { sum += values [ index ] ; } return sum; } 29
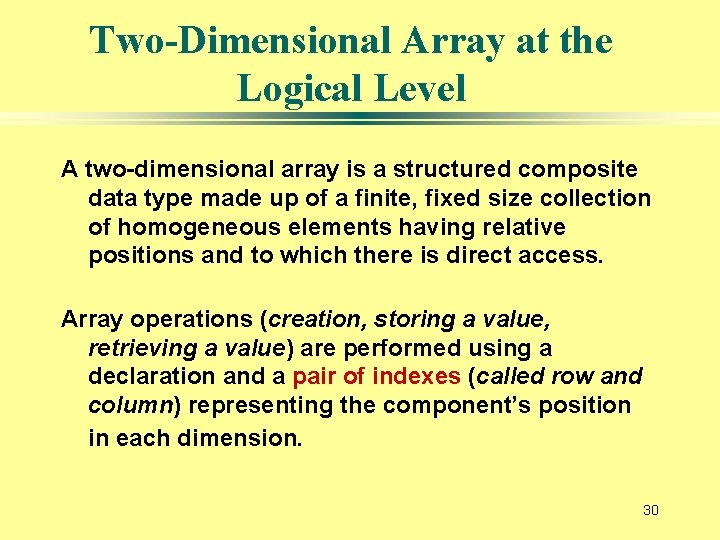
Two-Dimensional Array at the Logical Level A two-dimensional array is a structured composite data type made up of a finite, fixed size collection of homogeneous elements having relative positions and to which there is direct access. Array operations (creation, storing a value, retrieving a value) are performed using a declaration and a pair of indexes (called row and column) representing the component’s position in each dimension. 30
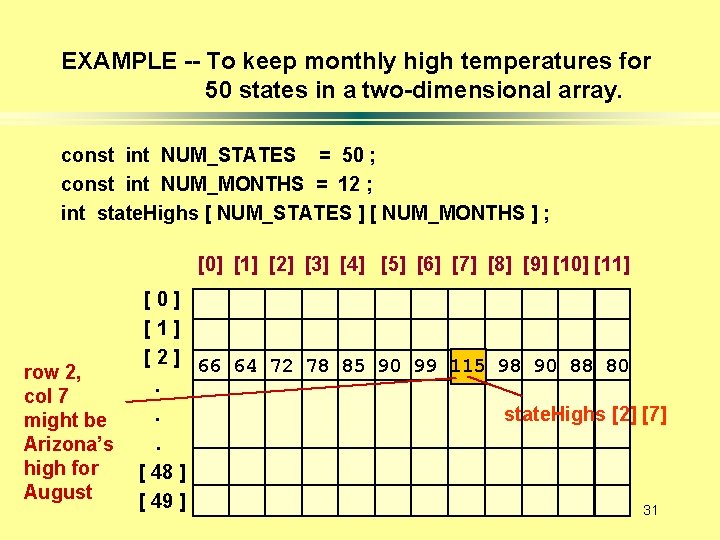
EXAMPLE -- To keep monthly high temperatures for 50 states in a two-dimensional array. const int NUM_STATES = 50 ; const int NUM_MONTHS = 12 ; int state. Highs [ NUM_STATES ] [ NUM_MONTHS ] ; [0] [1] [2] [3] [4] [5] [6] [7] [8] [9] [10] [11] row 2, col 7 might be Arizona’s high for August [0] [1] [ 2 ] 66 64 72 78 85 90 99 115 98 90 88 80. . state. Highs [2] [7]. [ 48 ] [ 49 ] 31
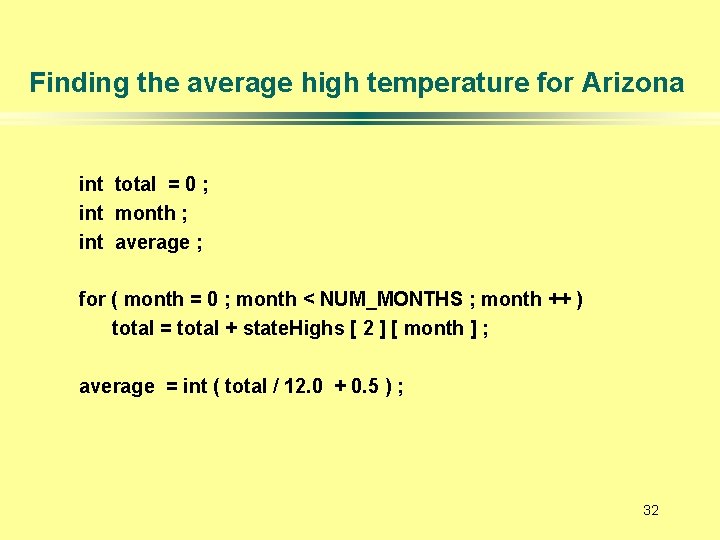
Finding the average high temperature for Arizona int total = 0 ; int month ; int average ; for ( month = 0 ; month < NUM_MONTHS ; month ++ ) total = total + state. Highs [ 2 ] [ month ] ; average = int ( total / 12. 0 + 0. 5 ) ; 32
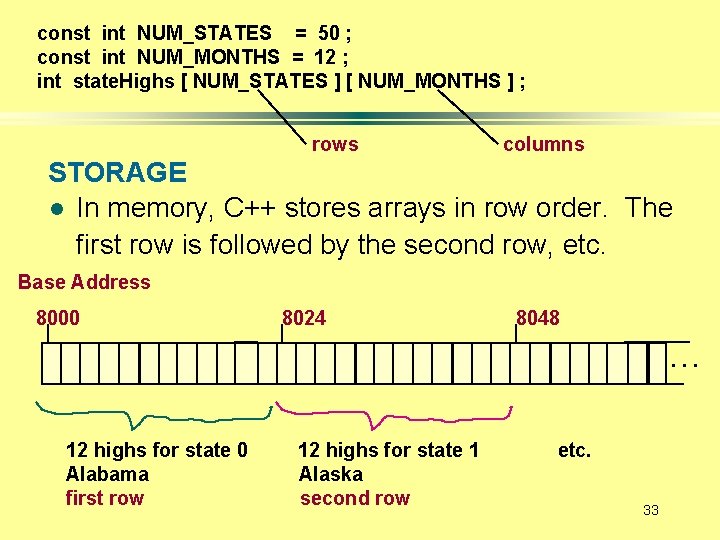
const int NUM_STATES = 50 ; const int NUM_MONTHS = 12 ; int state. Highs [ NUM_STATES ] [ NUM_MONTHS ] ; rows columns STORAGE l In memory, C++ stores arrays in row order. The first row is followed by the second row, etc. Base Address 8000 8024 8048. . . 12 highs for state 0 Alabama first row 12 highs for state 1 Alaska second row etc. 33
![Implementation Level View state Highs 0 1 state Highs 0 Implementation Level View state. Highs[ 0 ] [ 1 ] state. Highs[ 0 ]](https://slidetodoc.com/presentation_image_h2/4cc5851da70d6540923d6de1b3626802/image-34.jpg)
Implementation Level View state. Highs[ 0 ] [ 1 ] state. Highs[ 0 ] [ 2 ] state. Highs[ 0 ] [ 3 ] state. Highs[ 0 ] [ 4 ] state. Highs[ 0 ] [ 5 ] state. Highs[ 0 ] [ 6 ] state. Highs[ 0 ] [ 7 ] state. Highs[ 0 ] [ 8 ] state. Highs[ 0 ] [ 9 ] state. Highs[ 0 ] [10 ] state. Highs[ 0 ] [11 ] state. Highs[ 1 ] [ 0 ] state. Highs[ 1 ] [ 2 ] state. Highs[ 1 ] [ 3 ]. . Base Address 8000 To locate an element such as state. Highs [ 2 ] [ 7] the compiler needs to know that there are 12 columns in this two-dimensional array. At what address will state. Highs [ 2 ] [ 7 ] be found? Assume 2 bytes for type int. 34
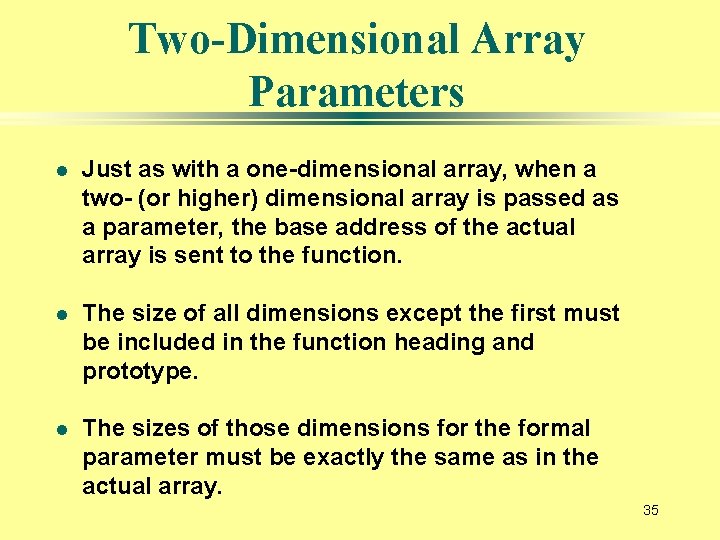
Two-Dimensional Array Parameters l Just as with a one-dimensional array, when a two- (or higher) dimensional array is passed as a parameter, the base address of the actual array is sent to the function. l The size of all dimensions except the first must be included in the function heading and prototype. l The sizes of those dimensions for the formal parameter must be exactly the same as in the actual array. 35
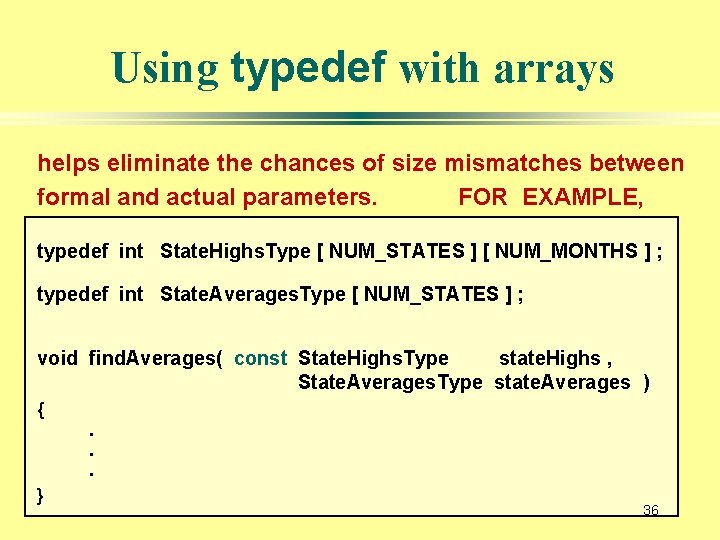
Using typedef with arrays helps eliminate the chances of size mismatches between formal and actual parameters. FOR EXAMPLE, typedef int State. Highs. Type [ NUM_STATES ] [ NUM_MONTHS ] ; typedef int State. Averages. Type [ NUM_STATES ] ; void find. Averages( const State. Highs. Type state. Highs , State. Averages. Type state. Averages ) {. . . } 36
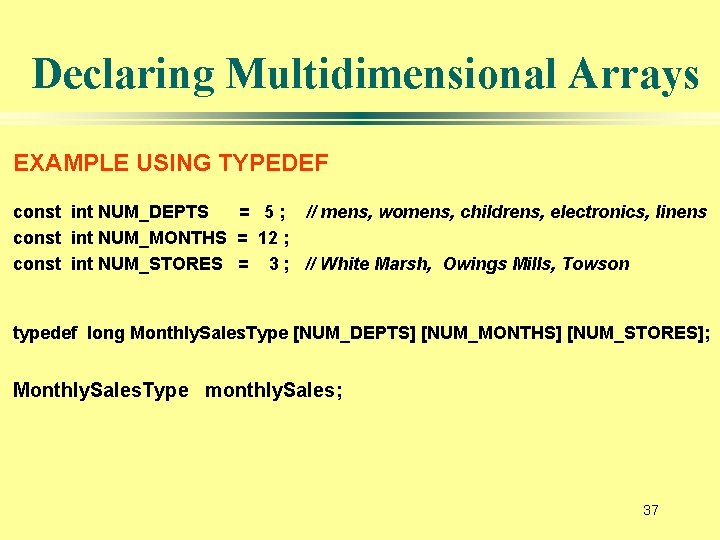
Declaring Multidimensional Arrays EXAMPLE USING TYPEDEF const int NUM_DEPTS = 5 ; // mens, womens, childrens, electronics, linens const int NUM_MONTHS = 12 ; const int NUM_STORES = 3 ; // White Marsh, Owings Mills, Towson typedef long Monthly. Sales. Type [NUM_DEPTS] [NUM_MONTHS] [NUM_STORES]; Monthly. Sales. Type monthly. Sales; 37
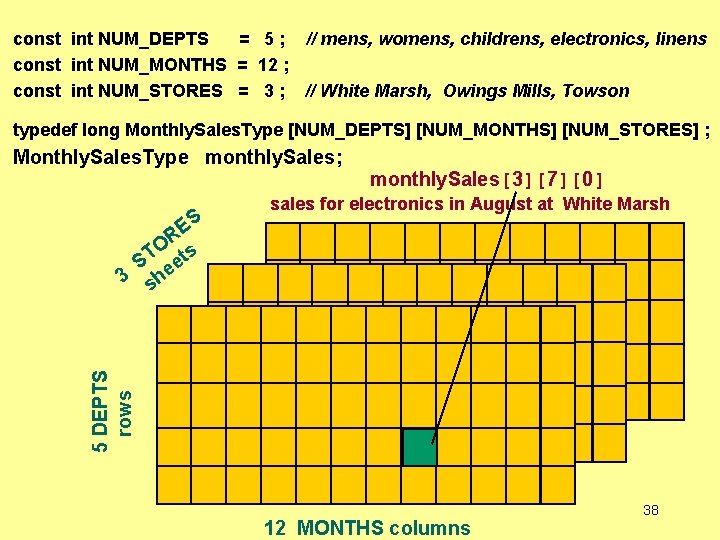
const int NUM_DEPTS = 5 ; // mens, womens, childrens, electronics, linens const int NUM_MONTHS = 12 ; const int NUM_STORES = 3 ; // White Marsh, Owings Mills, Towson typedef long Monthly. Sales. Type [NUM_DEPTS] [NUM_MONTHS] [NUM_STORES] ; Monthly. Sales. Type monthly. Sales; sales for electronics in August at White Marsh 5 DEPTS rows S E R O s ST eet 3 sh monthly. Sales[3][7][0] 12 MONTHS columns 38
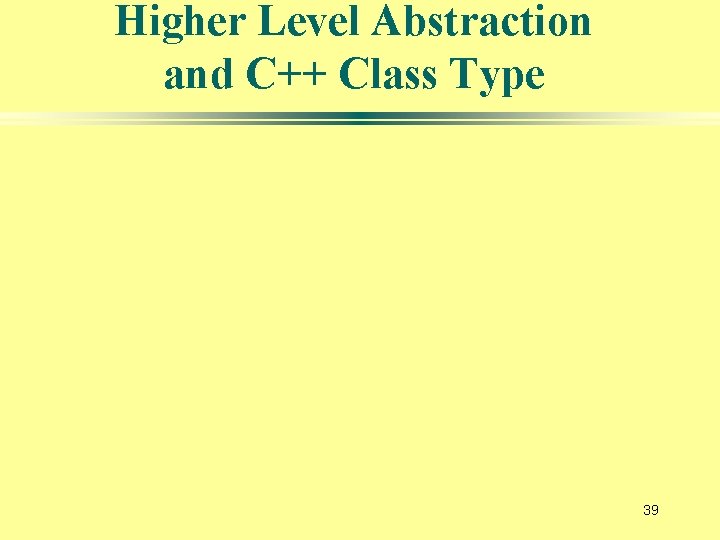
Higher Level Abstraction and C++ Class Type 39
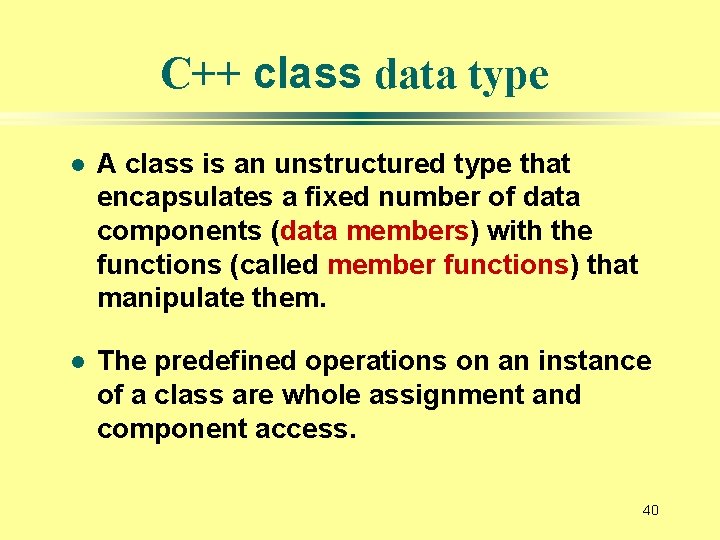
C++ class data type l A class is an unstructured type that encapsulates a fixed number of data components (data members) with the functions (called member functions) that manipulate them. l The predefined operations on an instance of a class are whole assignment and component access. 40
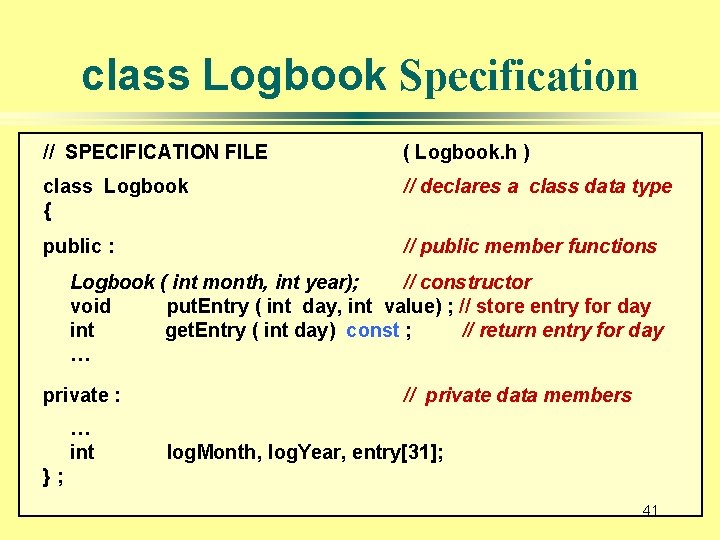
class Logbook Specification // SPECIFICATION FILE ( Logbook. h ) class Logbook { // declares a class data type public : // public member functions Logbook ( int month, int year); // constructor void put. Entry ( int day, int value) ; // store entry for day int get. Entry ( int day) const ; // return entry for day … private : … int // private data members log. Month, log. Year, entry[31]; }; 41
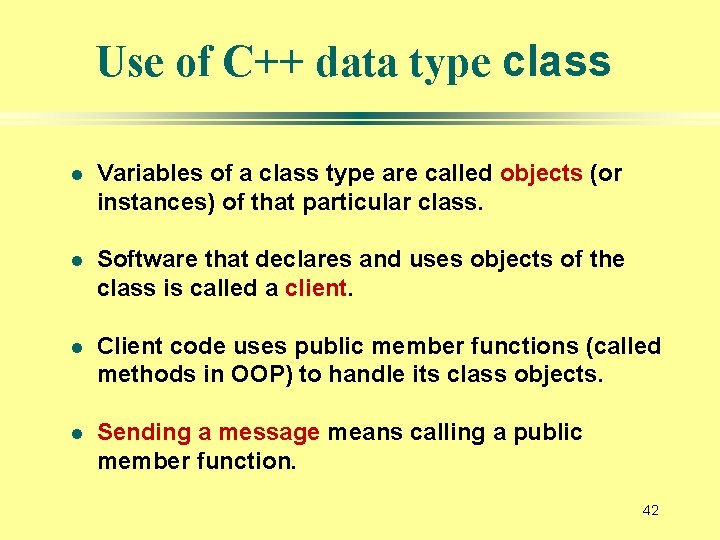
Use of C++ data type class l Variables of a class type are called objects (or instances) of that particular class. l Software that declares and uses objects of the class is called a client. l Client code uses public member functions (called methods in OOP) to handle its class objects. l Sending a message means calling a public member function. 42
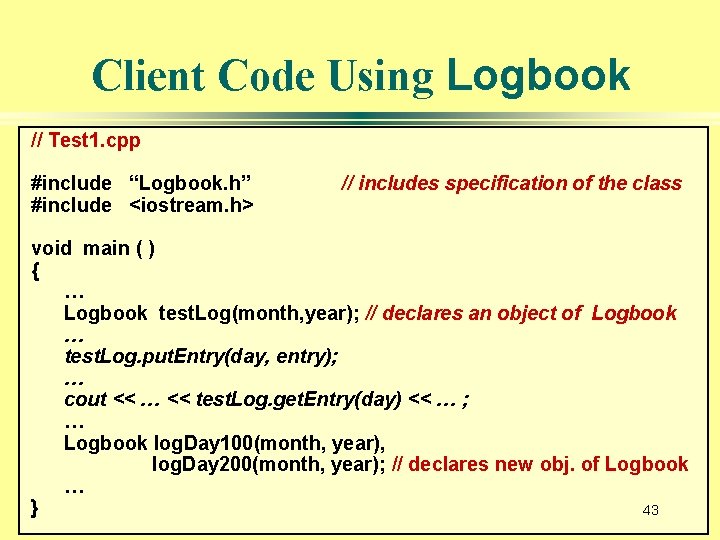
Client Code Using Logbook // Test 1. cpp #include “Logbook. h” #include <iostream. h> // includes specification of the class void main ( ) { … Logbook test. Log(month, year); // declares an object of Logbook … test. Log. put. Entry(day, entry); … cout << … << test. Log. get. Entry(day) << … ; … Logbook log. Day 100(month, year), log. Day 200(month, year); // declares new obj. of Logbook … } 43
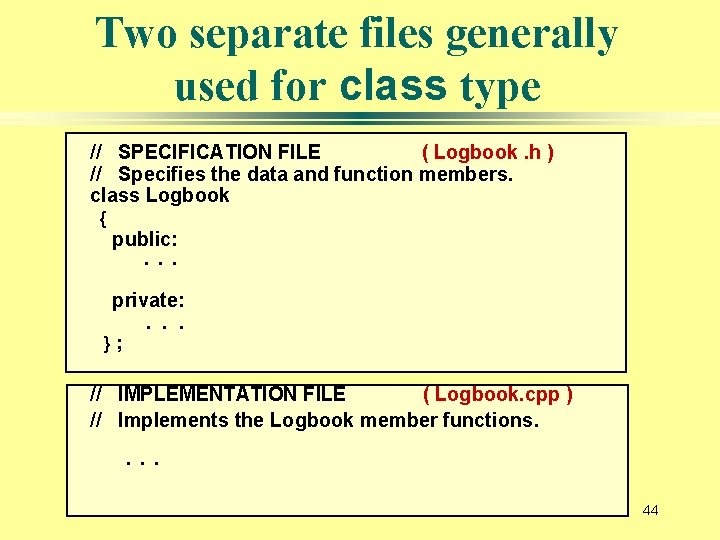
Two separate files generally used for class type // SPECIFICATION FILE ( Logbook. h ) // Specifies the data and function members. class Logbook { public: . . . private: . . . }; // IMPLEMENTATION FILE ( Logbook. cpp ) // Implements the Logbook member functions. . 44
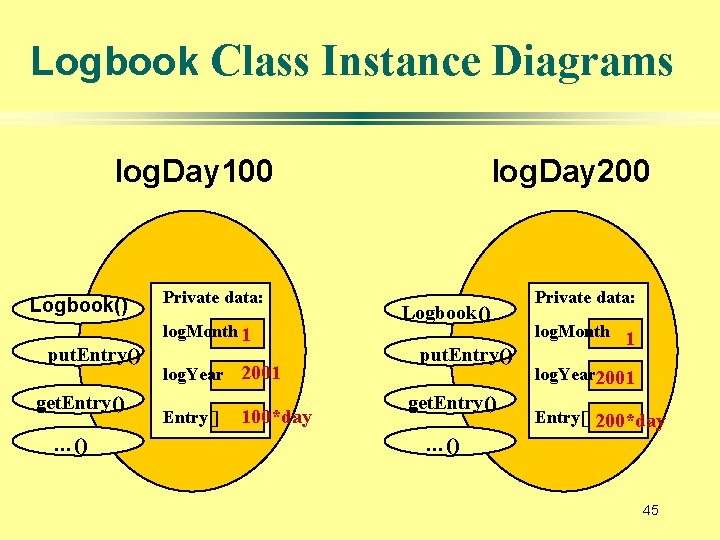
Logbook Class Instance Diagrams log. Day 100 Logbook() put. Entry() get. Entry() …() Private data: log. Month 1 log. Year 2001 Entry[] 100*day log. Day 200 Logbook() put. Entry() get. Entry() Private data: log. Month 1 log. Year 2001 Entry[] 200*day …() 45
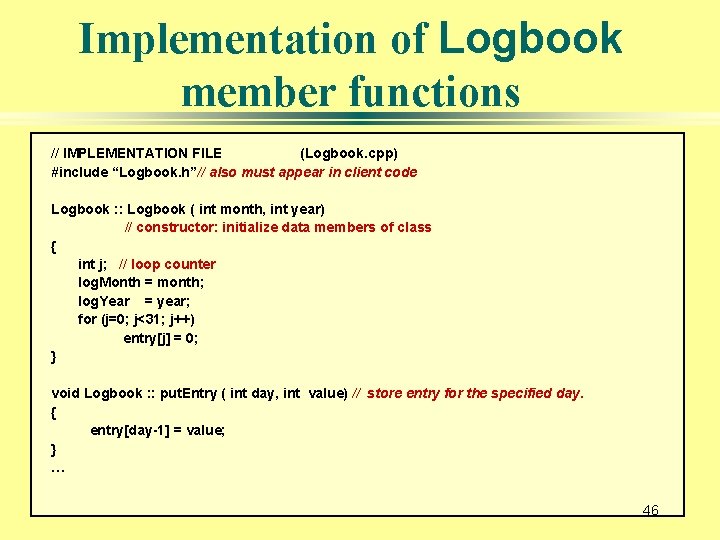
Implementation of Logbook member functions // IMPLEMENTATION FILE (Logbook. cpp) #include “Logbook. h”// also must appear in client code Logbook : : Logbook ( int month, int year) // constructor: initialize data members of class { int j; // loop counter log. Month = month; log. Year = year; for (j=0; j<31; j++) entry[j] = 0; } void Logbook : : put. Entry ( int day, int value) // store entry for the specified day. { entry[day-1] = value; } … 46
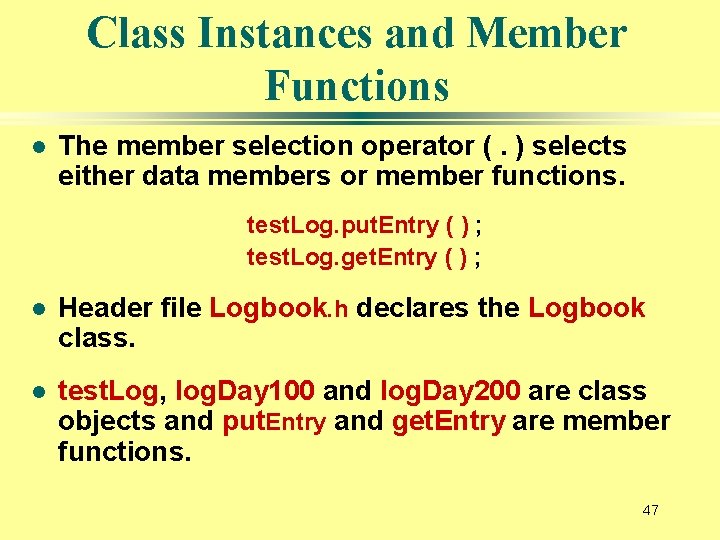
Class Instances and Member Functions l The member selection operator (. ) selects either data members or member functions. test. Log. put. Entry ( ) ; test. Log. get. Entry ( ) ; l Header file Logbook. h declares the Logbook class. l test. Log, log. Day 100 and log. Day 200 are class objects and put. Entry and get. Entry are member functions. 47
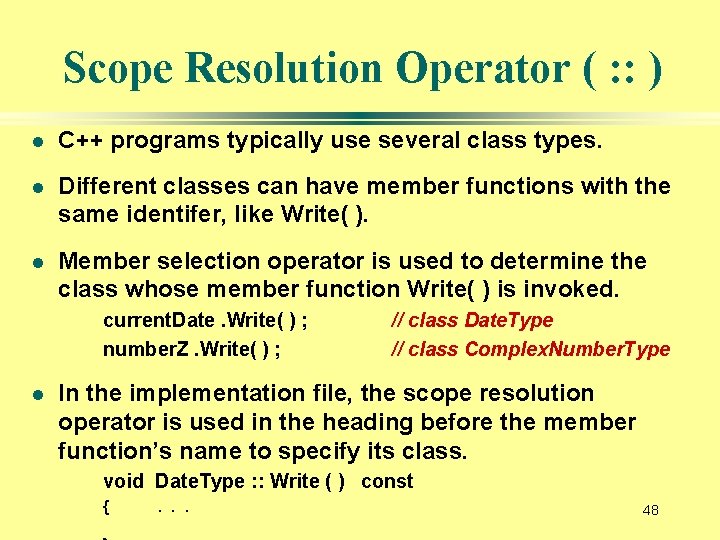
Scope Resolution Operator ( : : ) l C++ programs typically use several class types. l Different classes can have member functions with the same identifer, like Write( ). l Member selection operator is used to determine the class whose member function Write( ) is invoked. current. Date. Write( ) ; number. Z. Write( ) ; l // class Date. Type // class Complex. Number. Type In the implementation file, the scope resolution operator is used in the heading before the member function’s name to specify its class. void Date. Type : : Write ( ) const { . . . 48
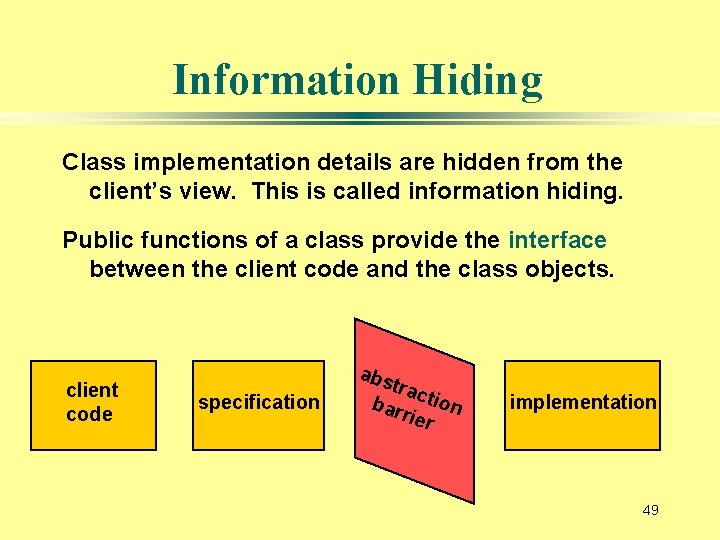
Information Hiding Class implementation details are hidden from the client’s view. This is called information hiding. Public functions of a class provide the interface between the client code and the class objects. client code specification abs trac bar tion rier implementation 49
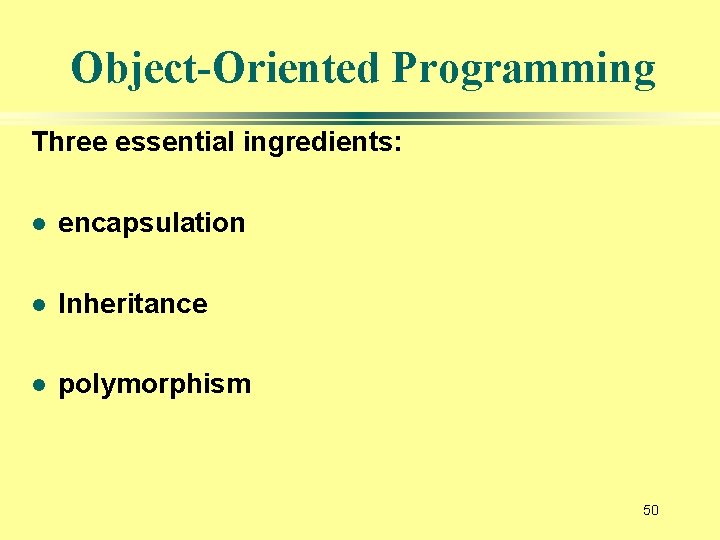
Object-Oriented Programming Three essential ingredients: l encapsulation l Inheritance l polymorphism 50
How are the whale flipper and the human arm different
Data structures chapter 1
La sofferenza nell'arte
Caratteri mendeliani nell'uomo
Cipro è nell'unione europea
Il triangolo nell'architettura
Alimenti di facile digestione
La valle d'aosta si trova nell'italia settentrionale
Modulo e ritmo in arte
Superbia
Odissea nell'arte
La parola nell'arte
La liguria si trova nell'italia settentrionale
Nell'andare se ne va piangendo
Contrasti nell'europa del seicento zanichelli
Parabola nell'architettura
Lussuria nell'arte
Acqua significato simbolico
Postura
Nostalgia nell'arte
Jerusalem cite de dieu chant
Je contiens du sucre sans être sucré
Jura trias
Plus j'apprends plus je me rends compte de mon ignorance
Dale ormond
Verbal symbols cone of experience example
Edgar dale’s cone of experience
Example of games in contrived experiences
Vasomotor reversal of dale
Kerucut pengalaman edgar dale
Kerucut pengalaman edgar dale
Tord dale
Kerucut pengalaman edgar dale
Dale brunner
Dale botting
Innate defination
Kon pengalaman pembelajaran dale
Dale county high school softball
Dale nin yaşantı konisi
Kerucut pengalaman edgar dale
Puntos acupuntura abdomen
Dale meade
Yaant
Susan dale barker
Dale larousse
Dale carnegie conversation stack
Kerucut pengalaman edgar dale
Dale limosna mujer
Kerucut pengalaman edgar dale hd
When was dale chihuly born
Ernest dale aportaciones a la administracion