Aspect J aspectoriented programming using Java technology Gregor
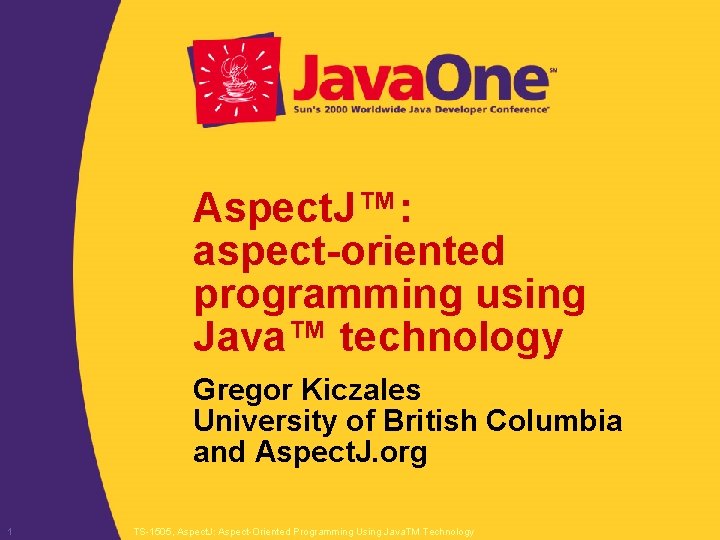
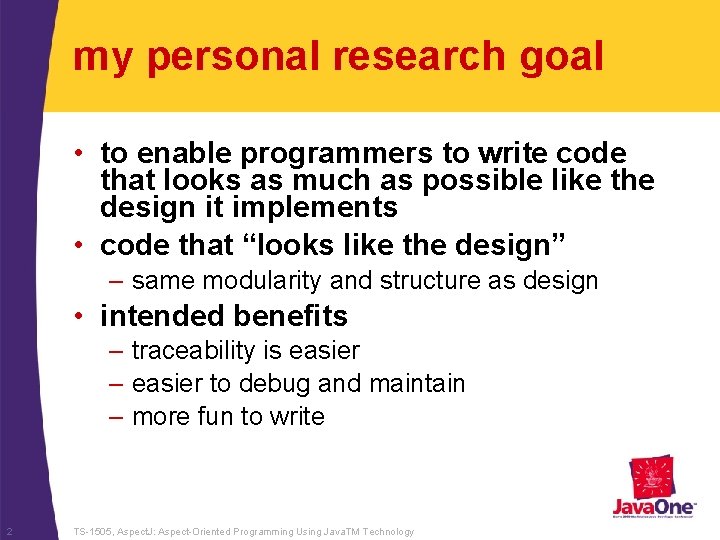
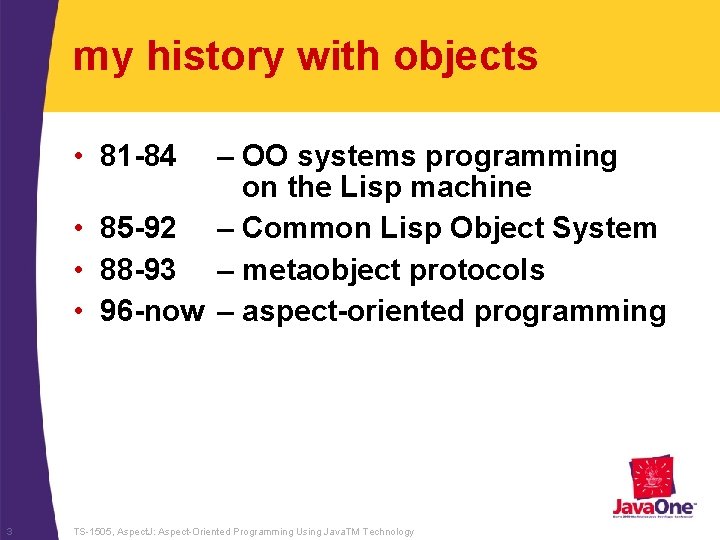
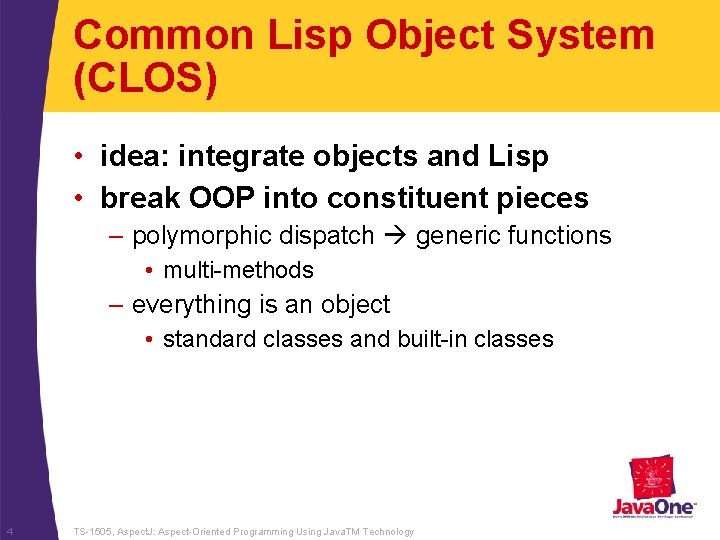
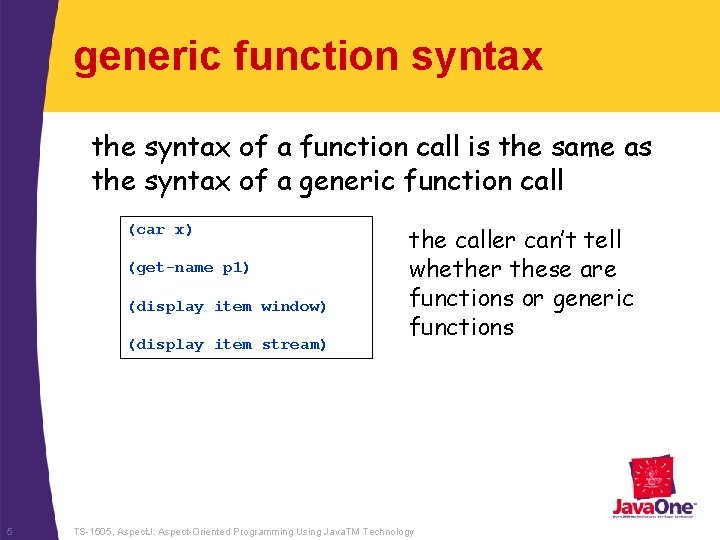
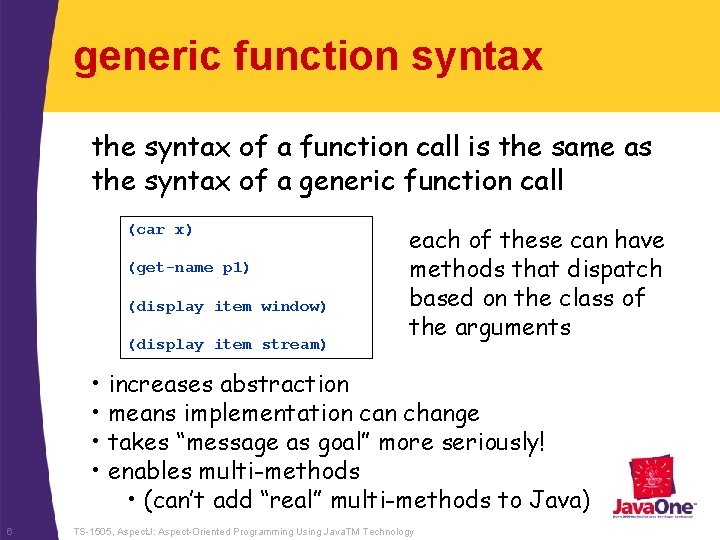
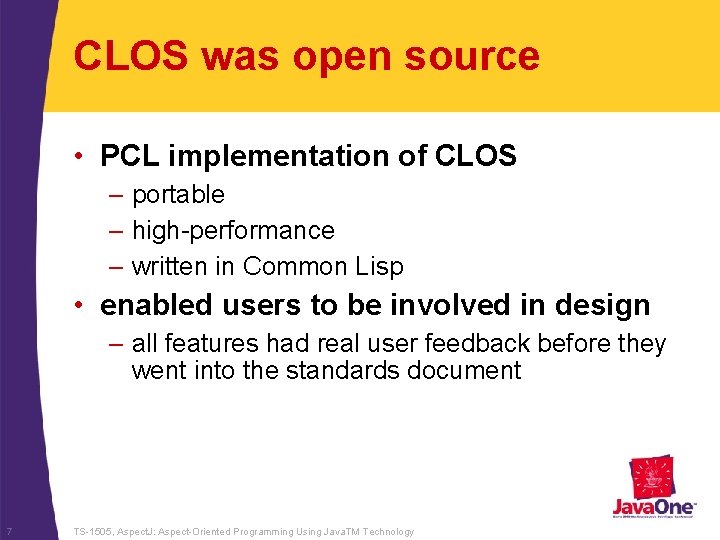
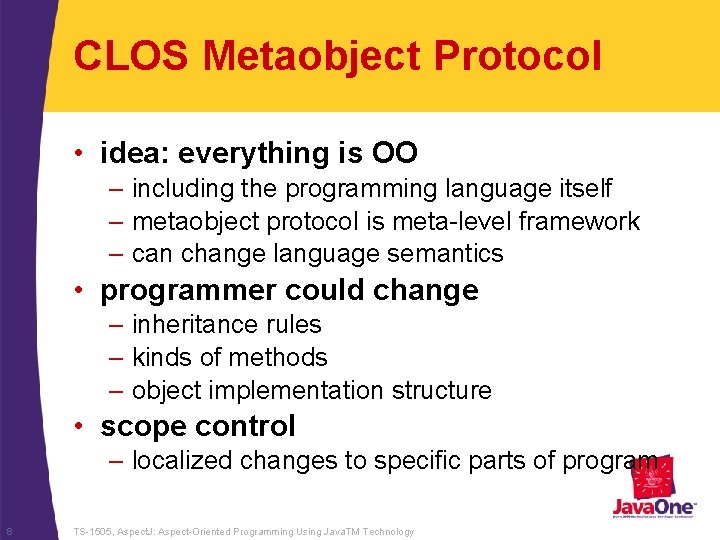
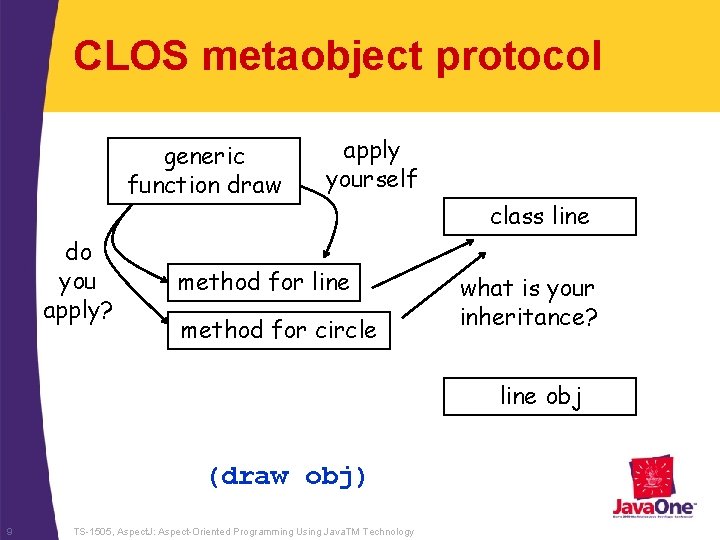
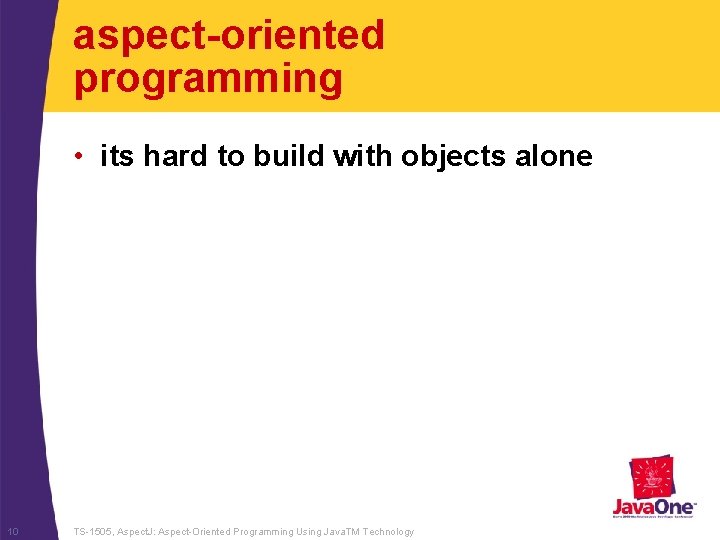
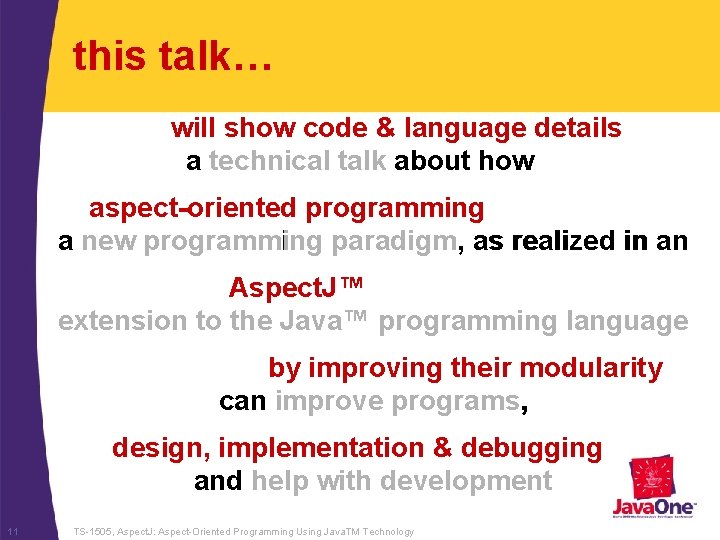
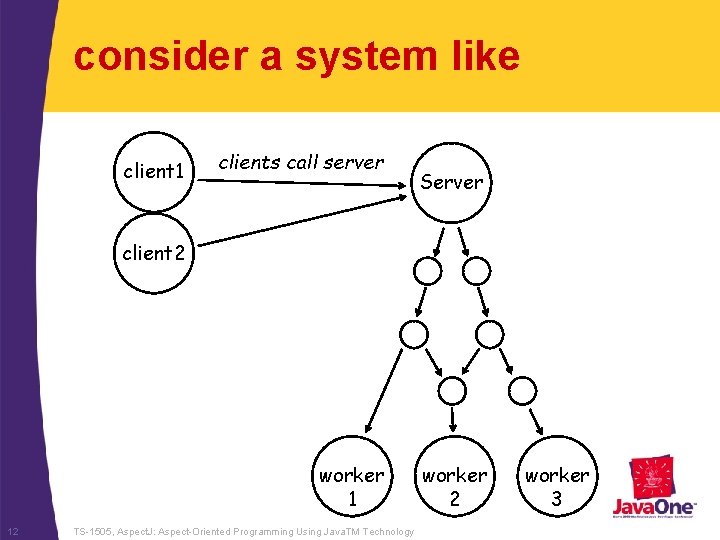
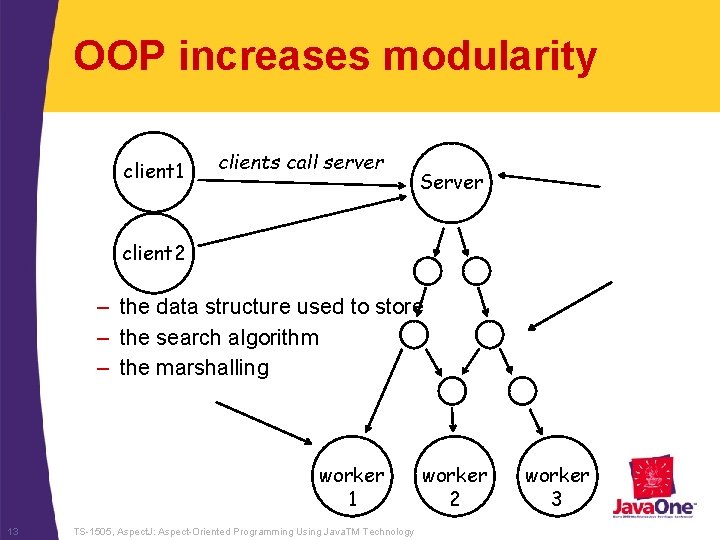
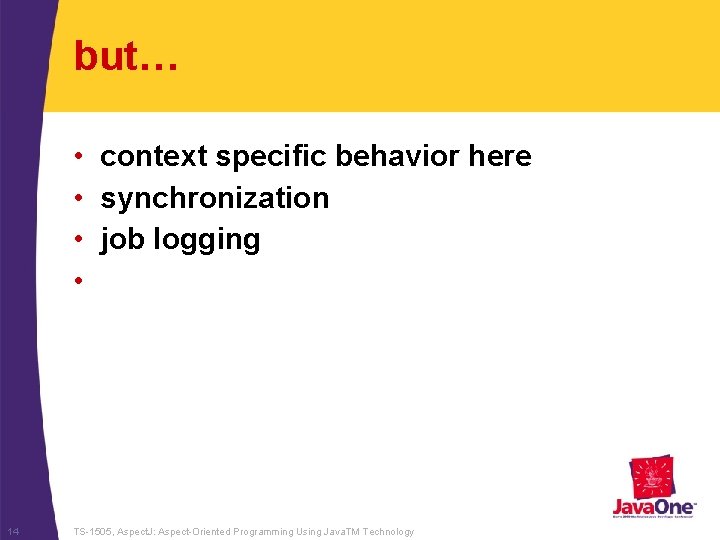
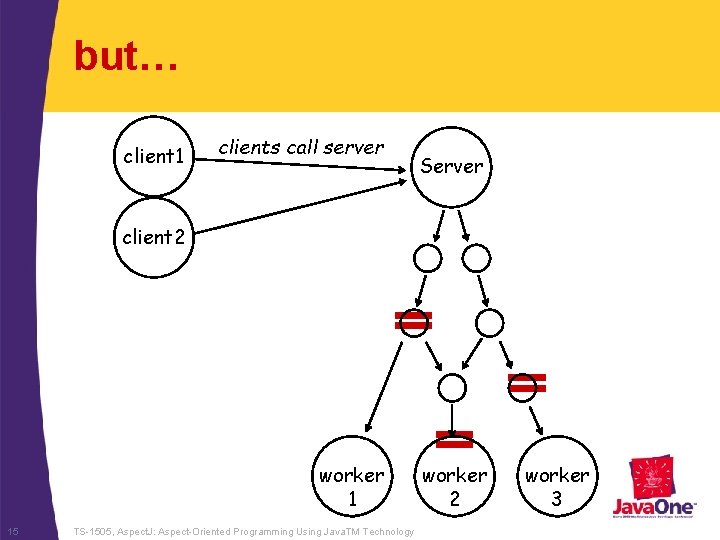
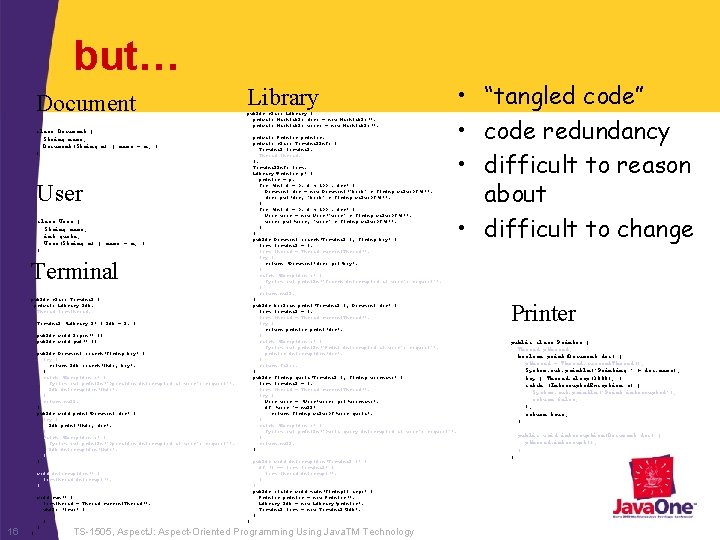
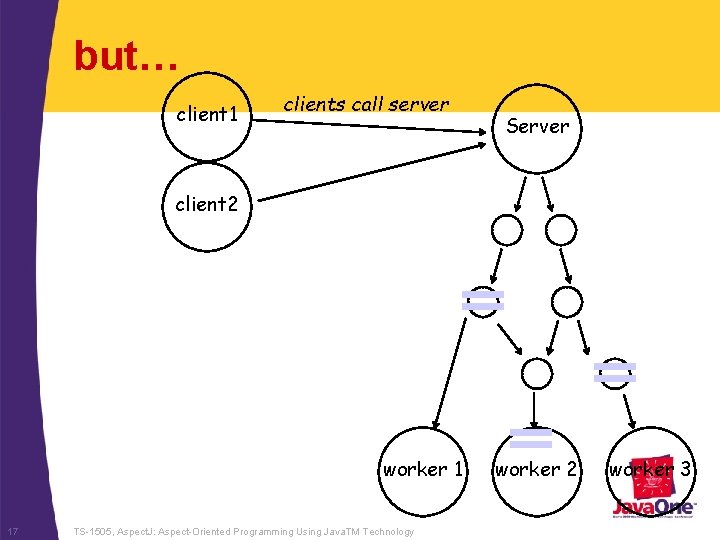
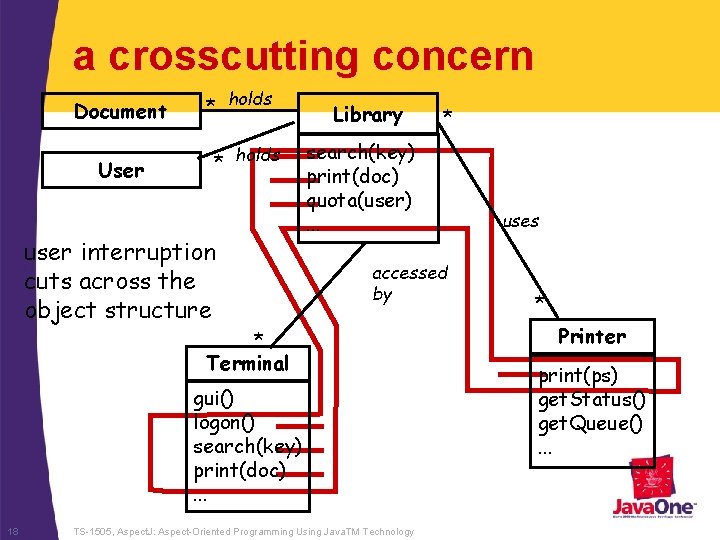
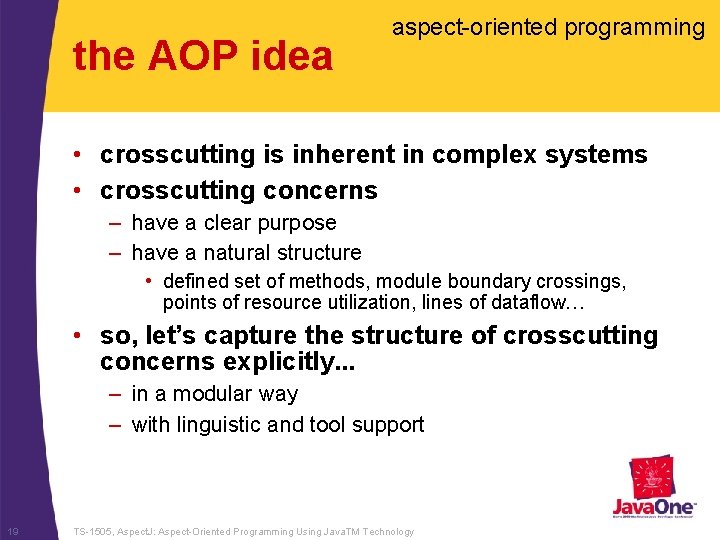
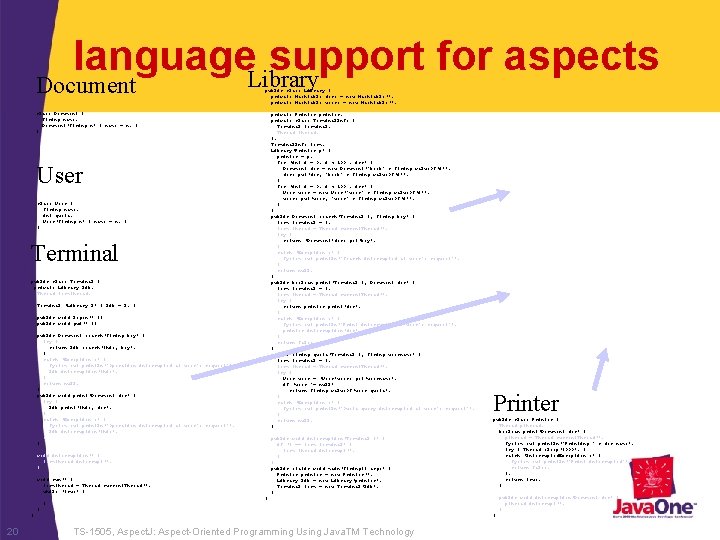
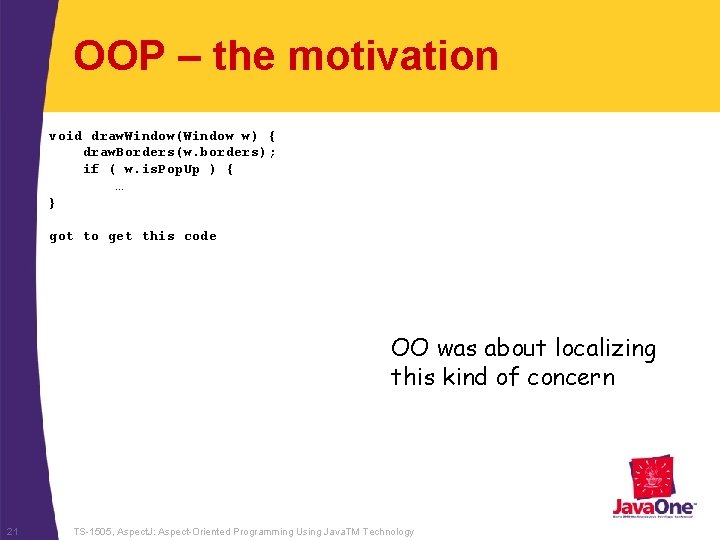
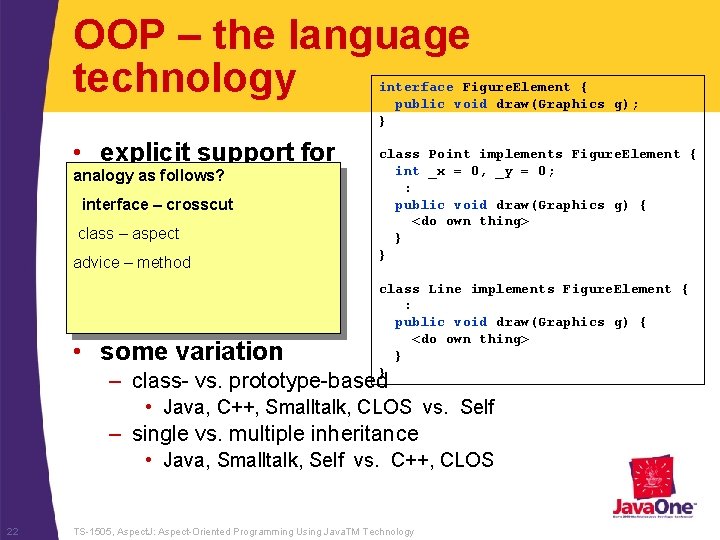
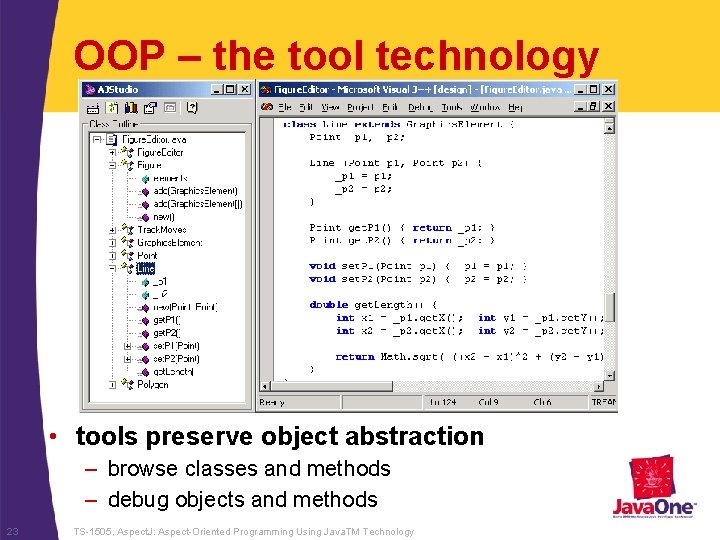
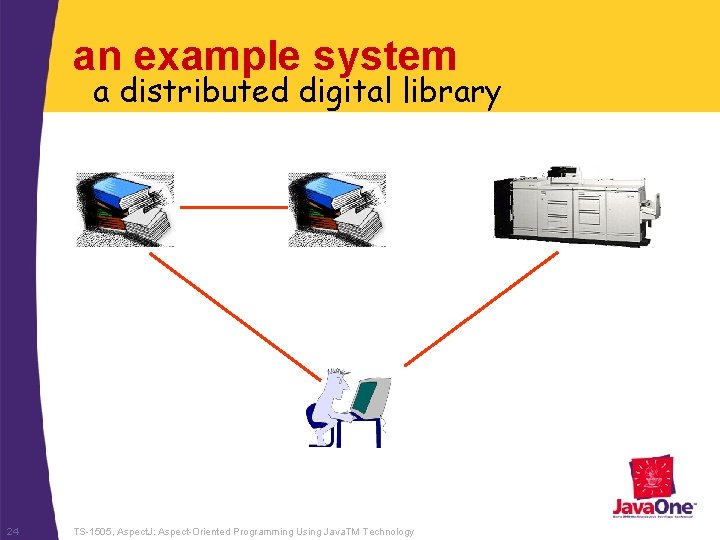
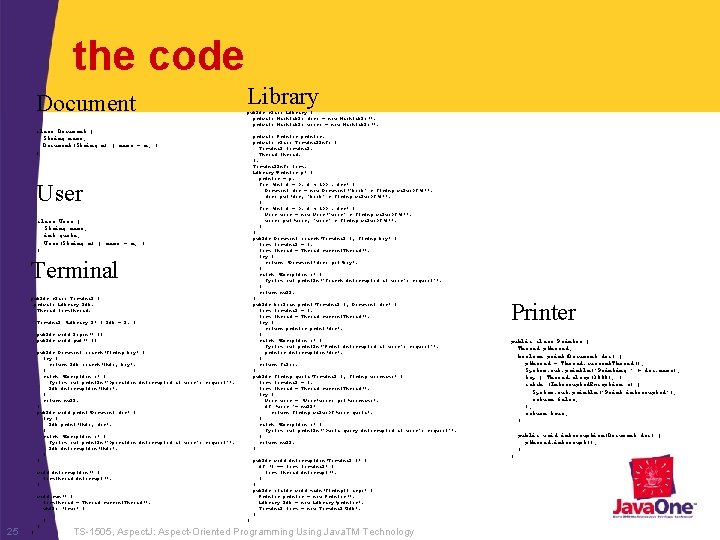
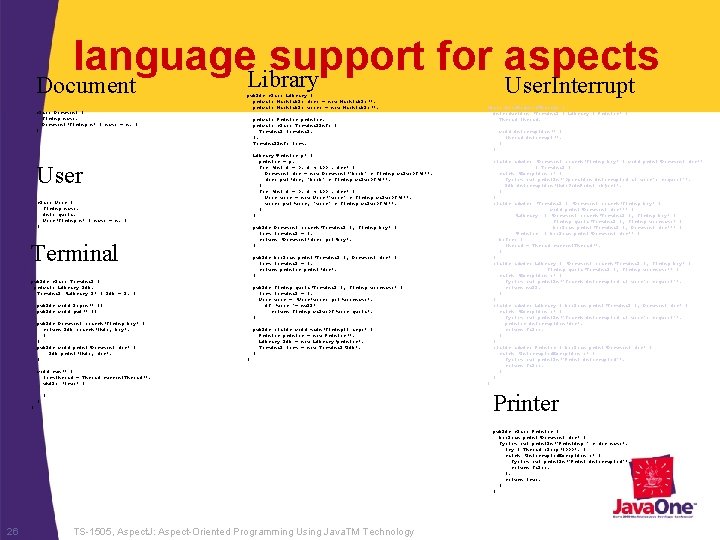
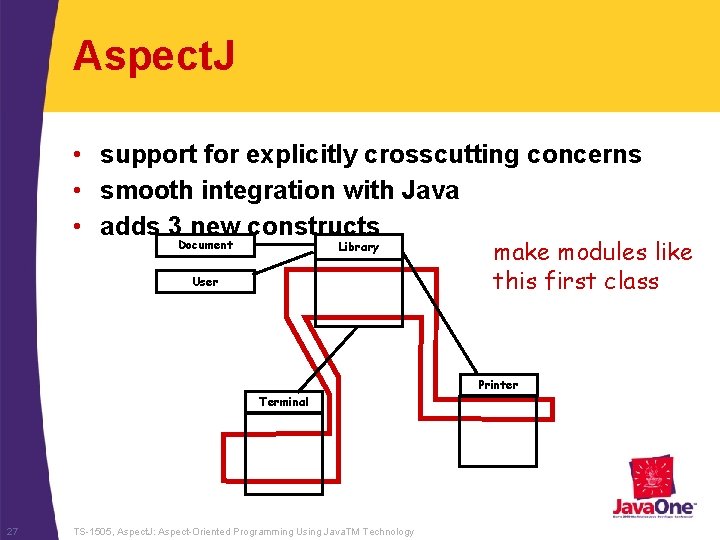
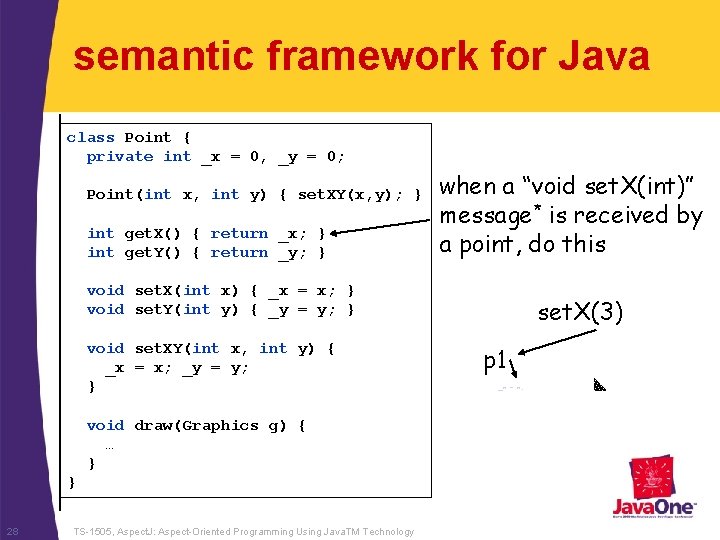
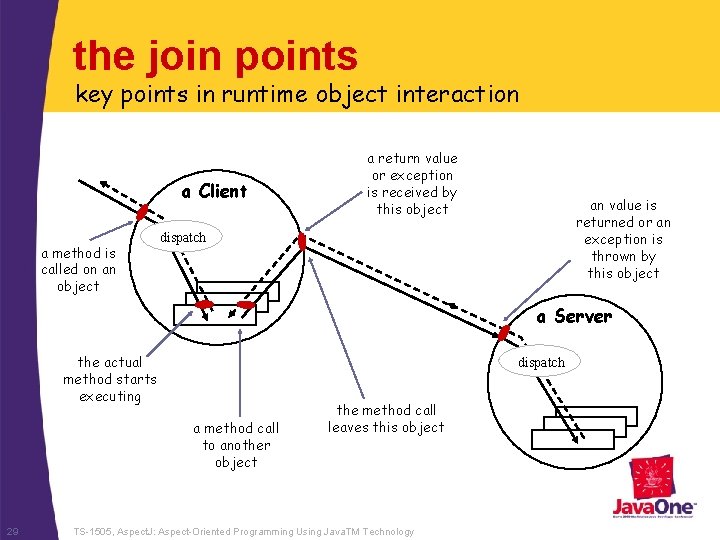
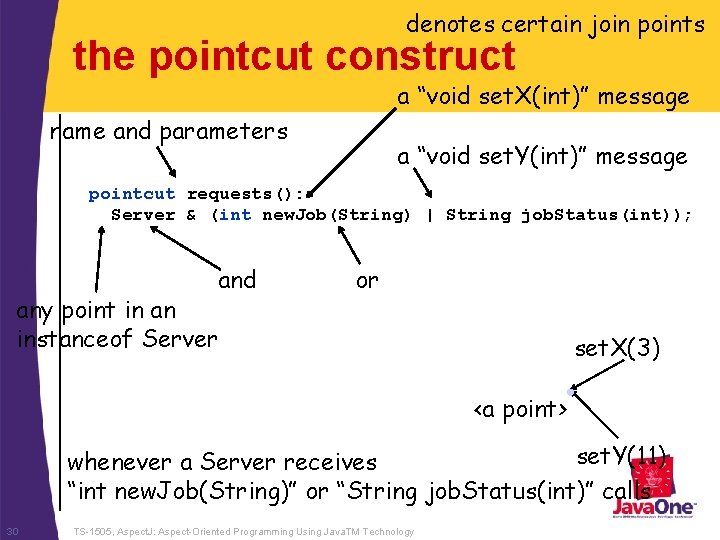
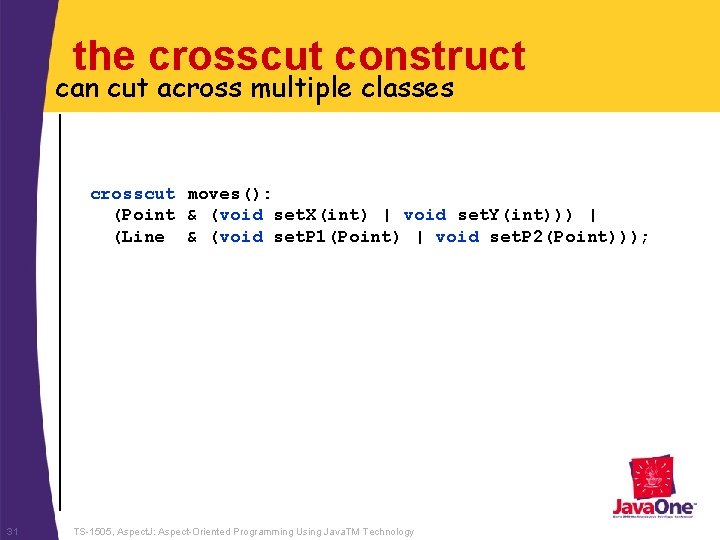
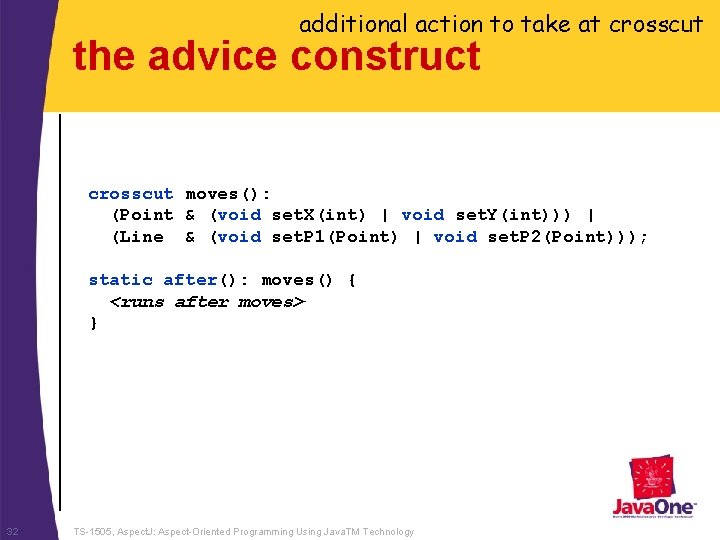
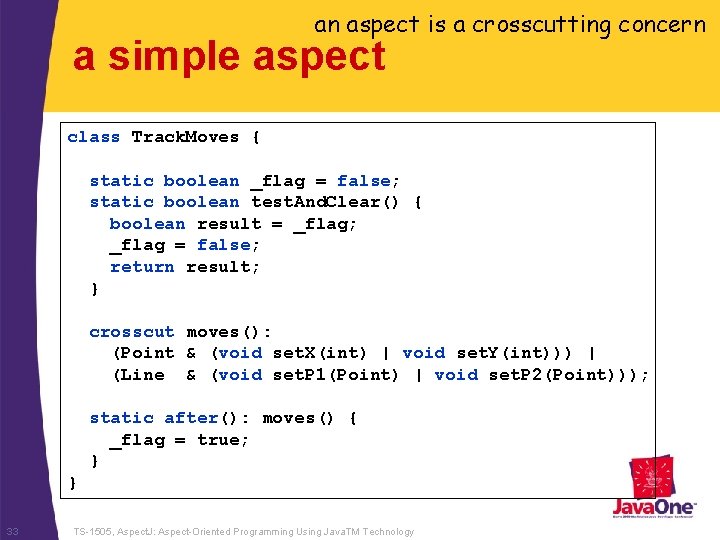
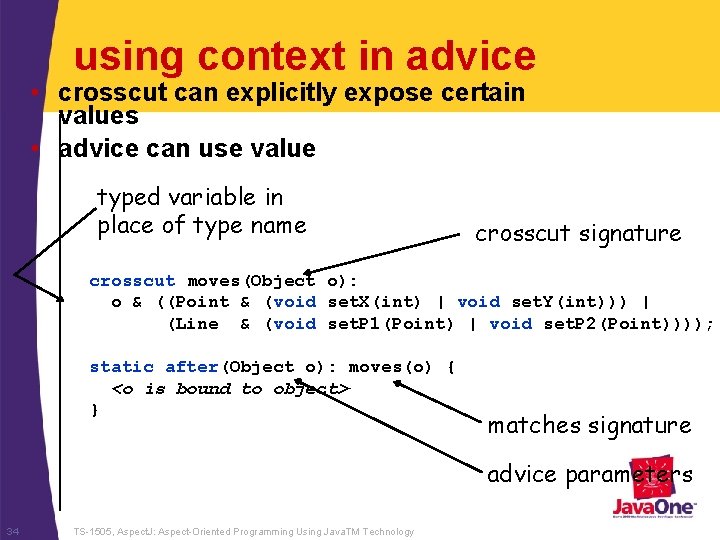
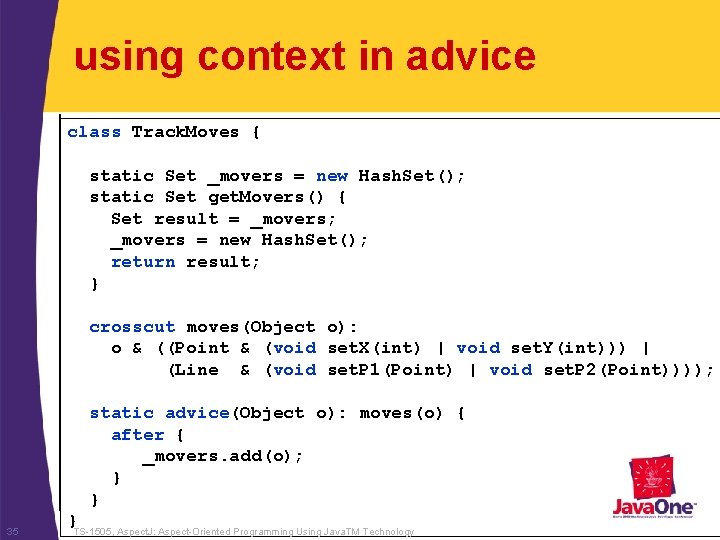
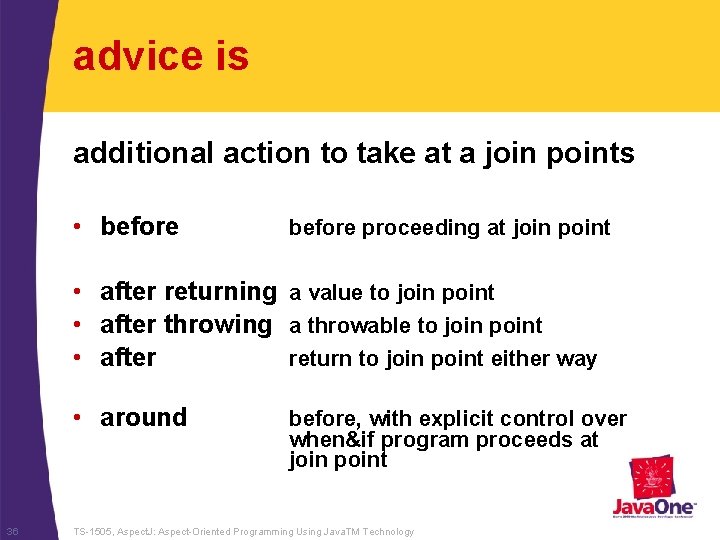
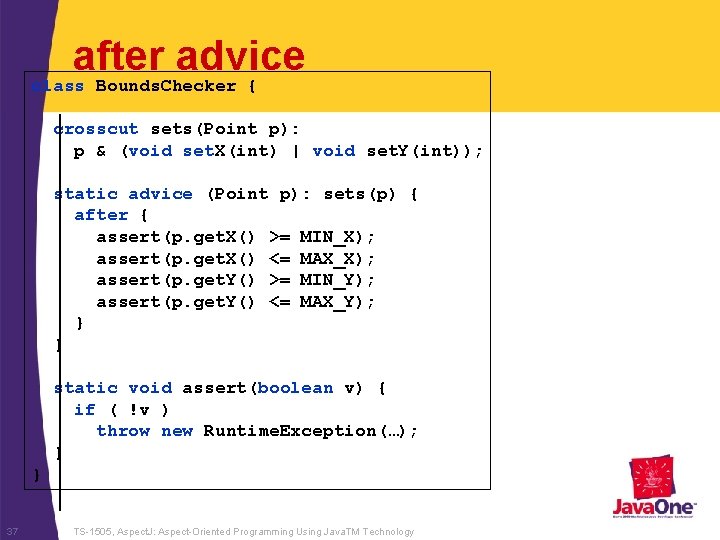
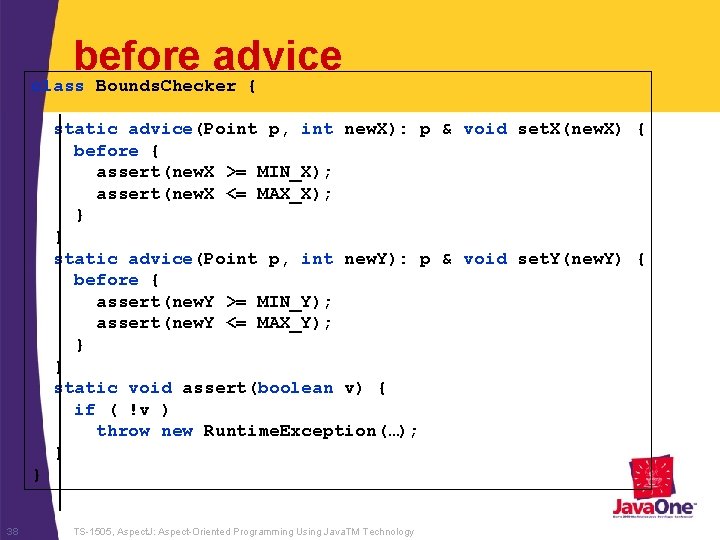
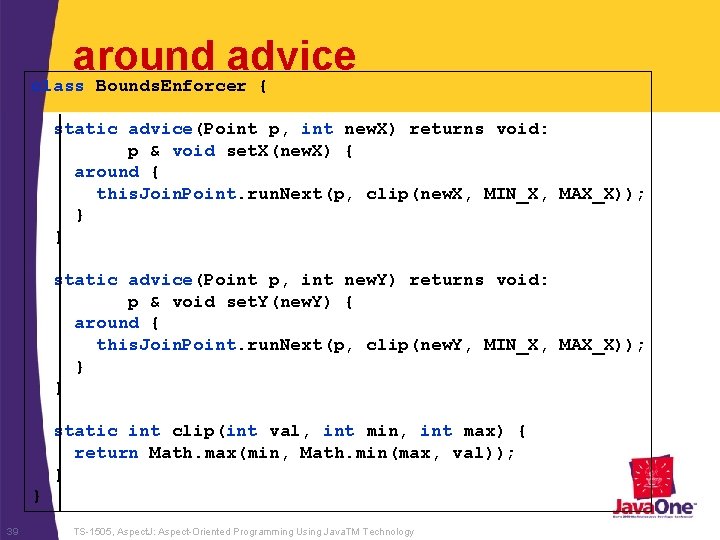
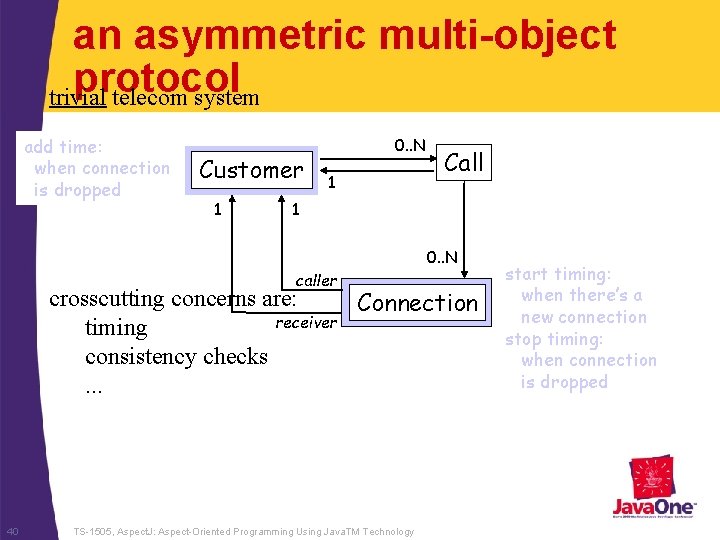
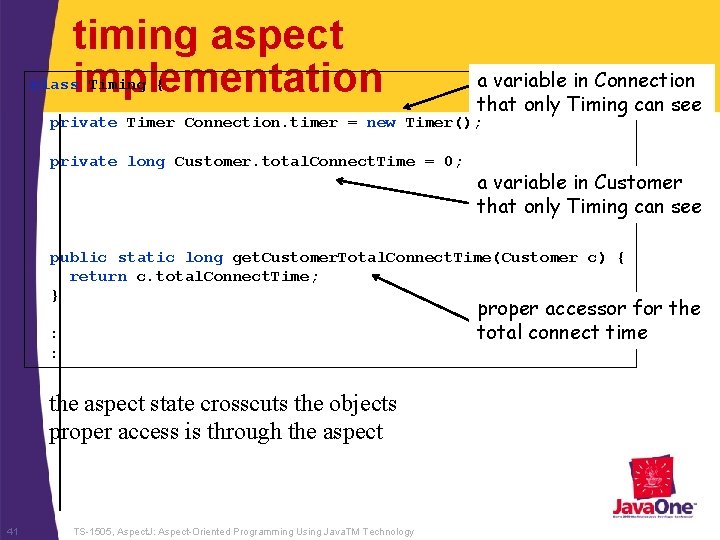
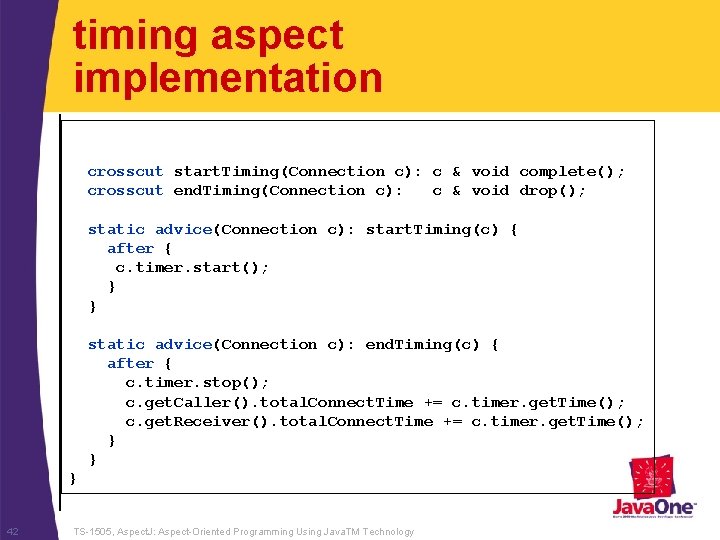
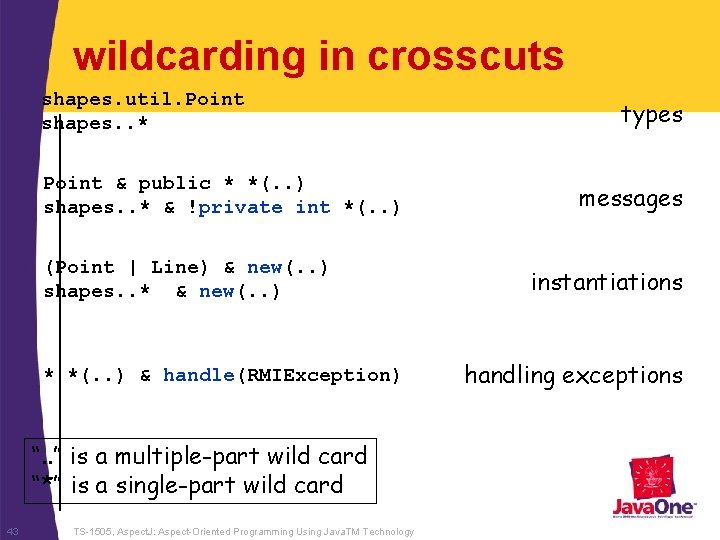
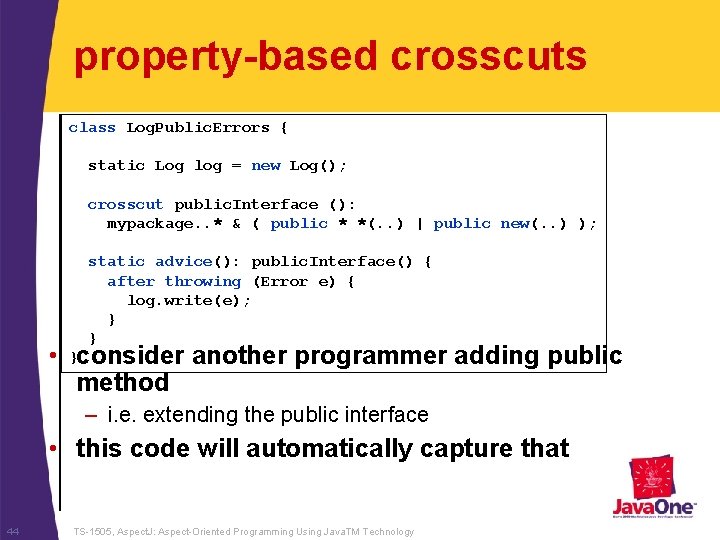
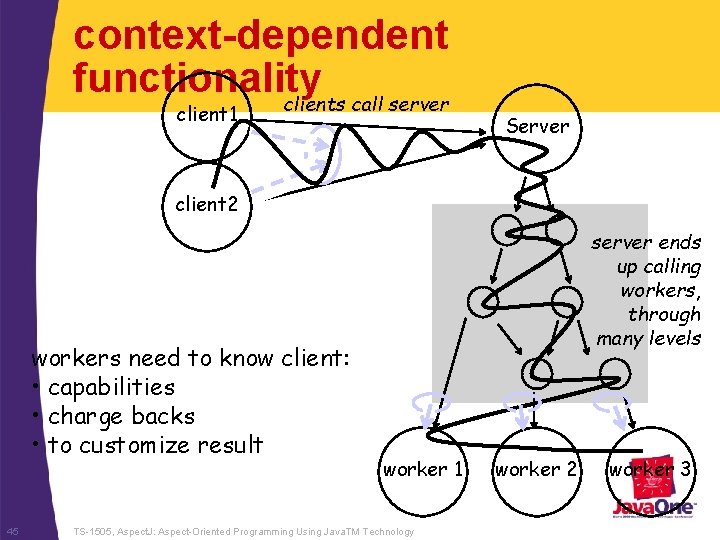
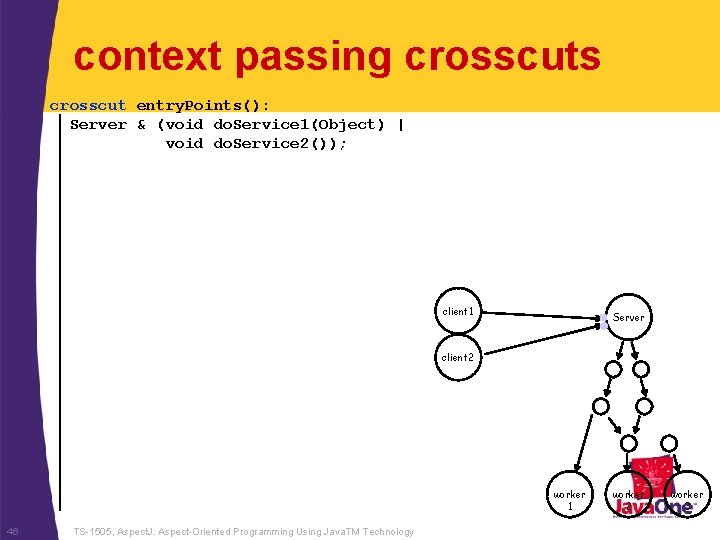
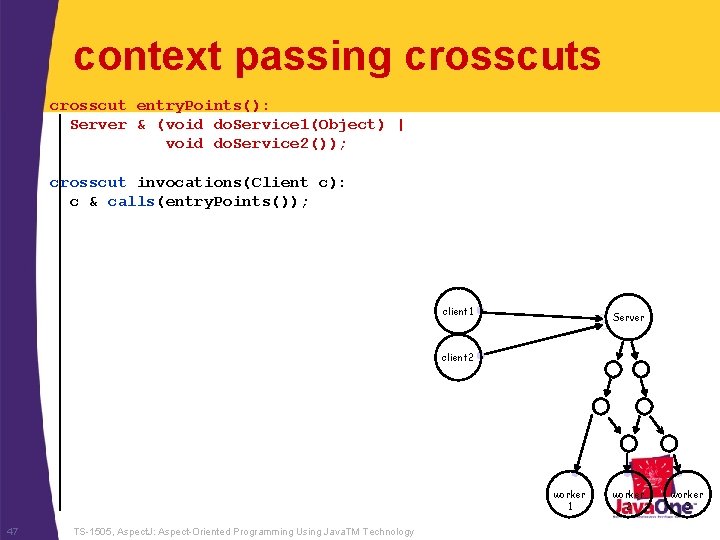
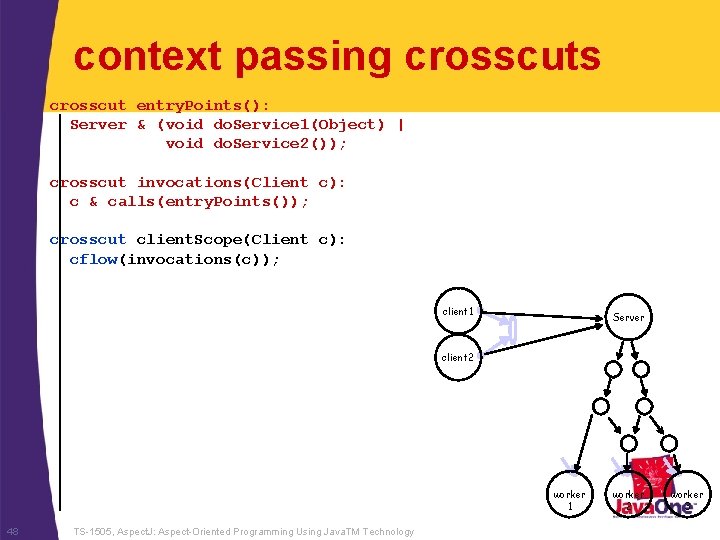
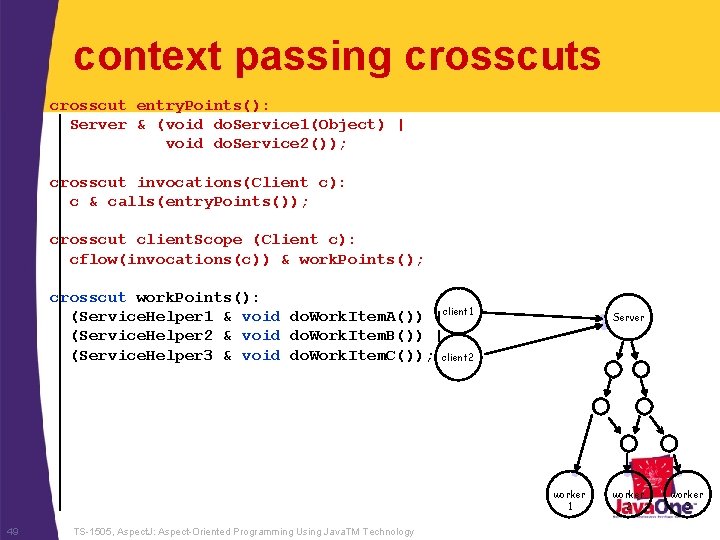
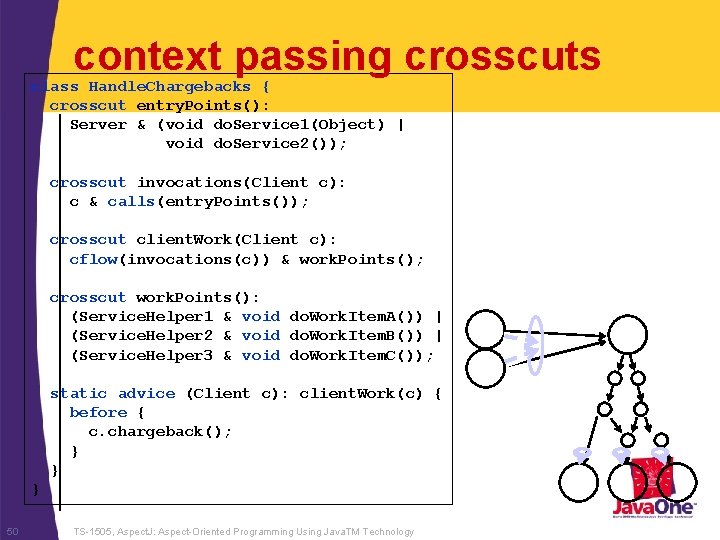
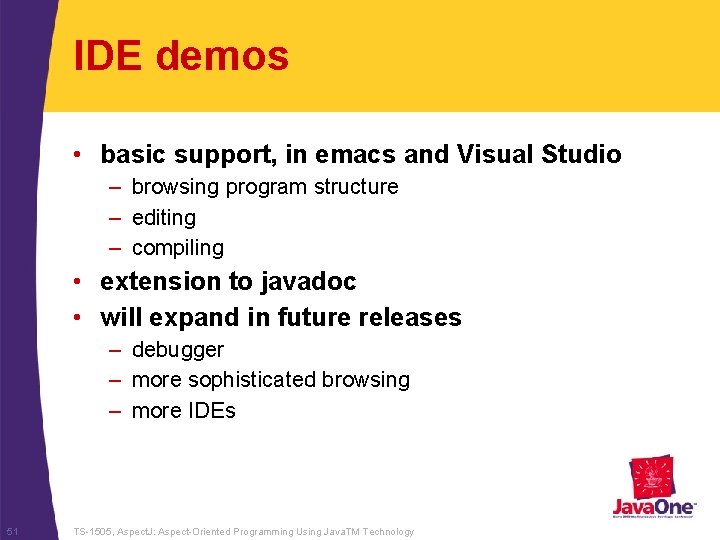
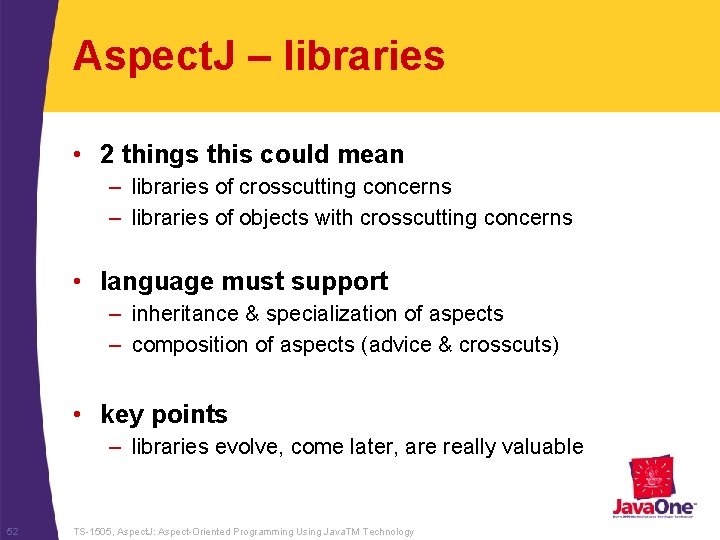
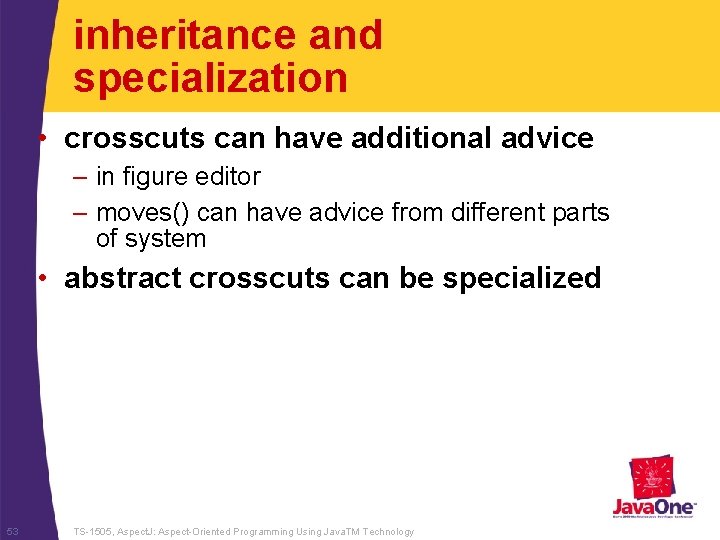
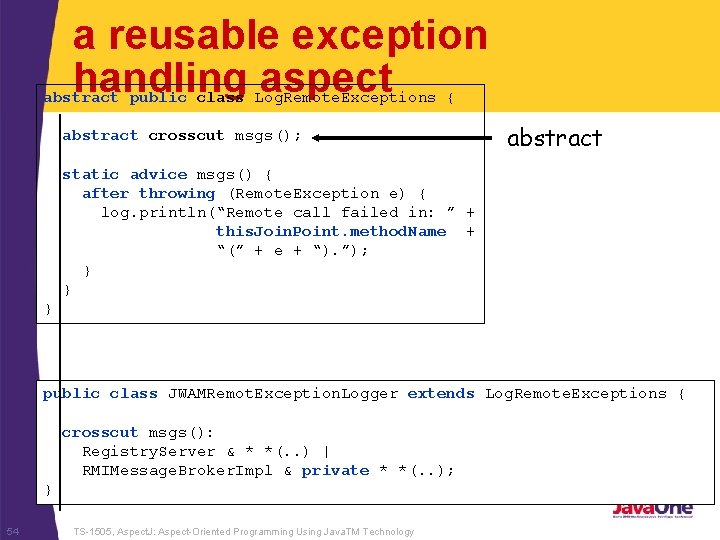
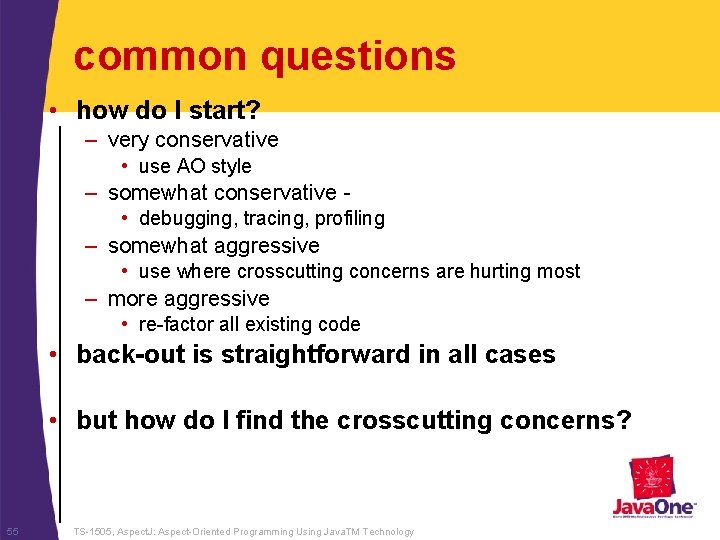
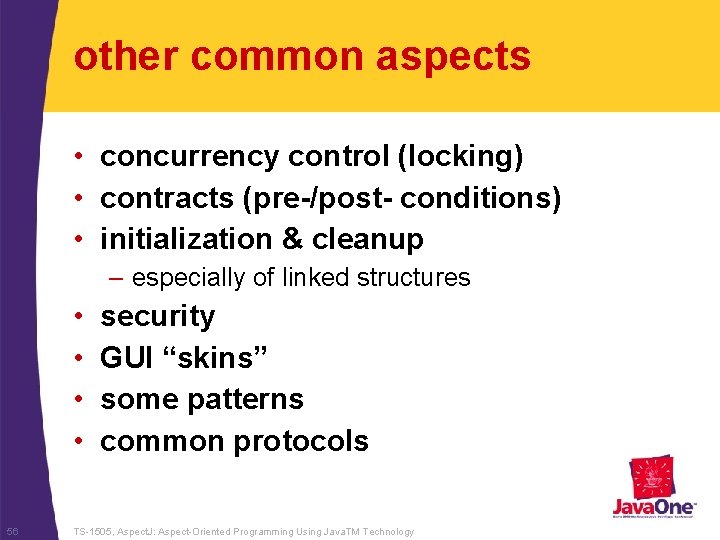
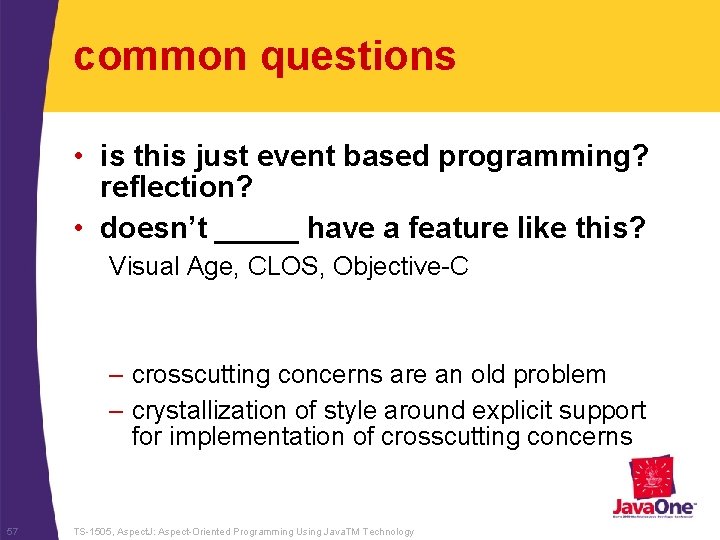
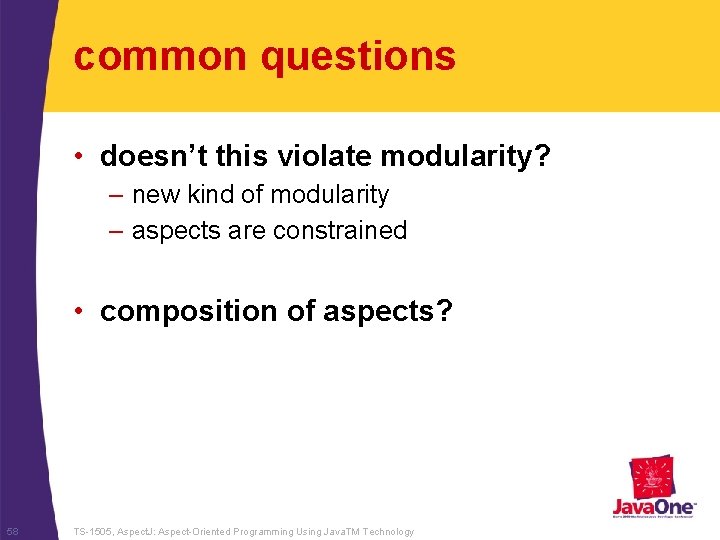
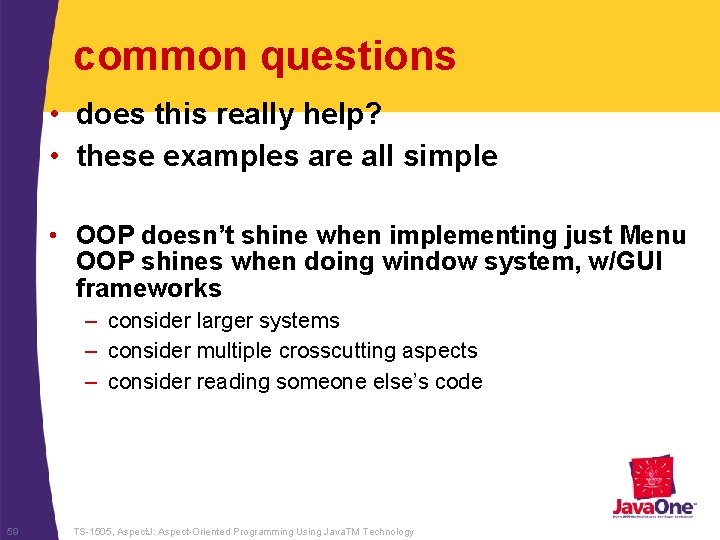
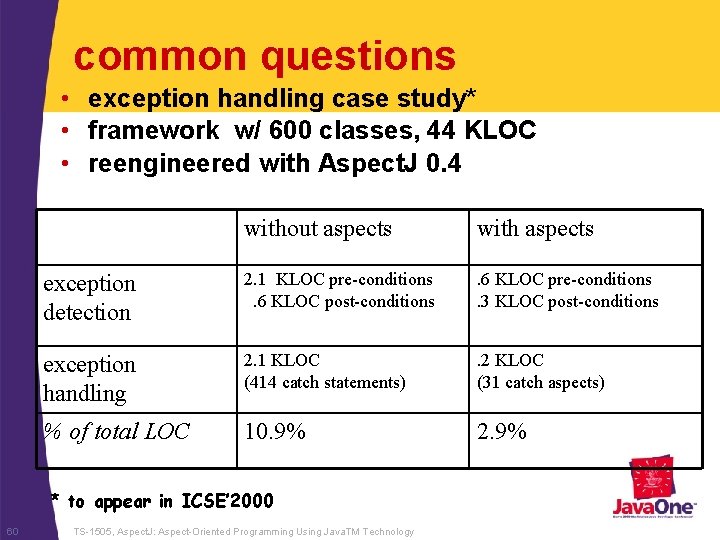
![related work in separation of crosscutting concerns • Hyper. J [Ossher, Tarr et. al] related work in separation of crosscutting concerns • Hyper. J [Ossher, Tarr et. al]](https://slidetodoc.com/presentation_image_h2/5ac0226281ee68062a0e0fc24872df14/image-61.jpg)
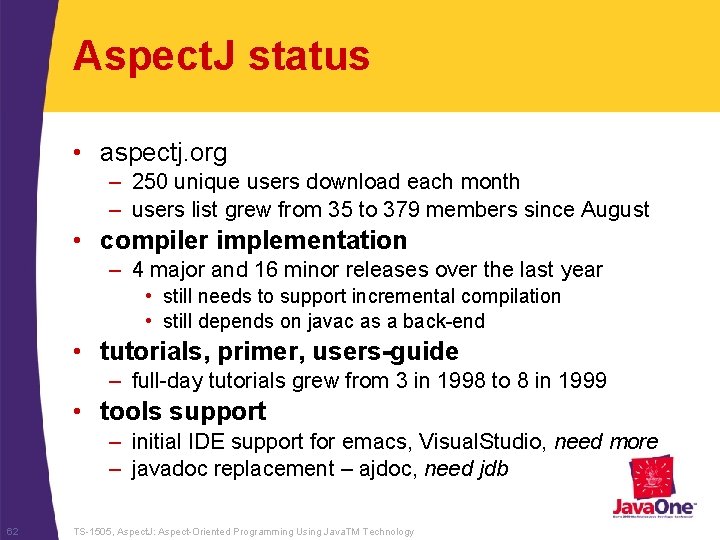
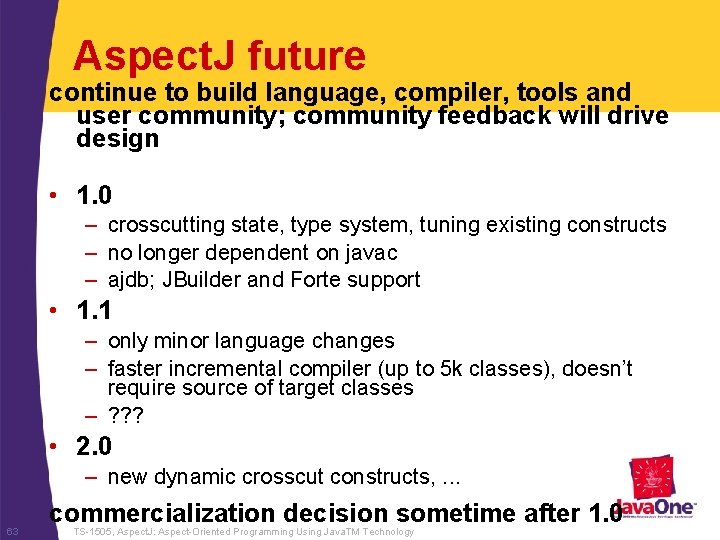
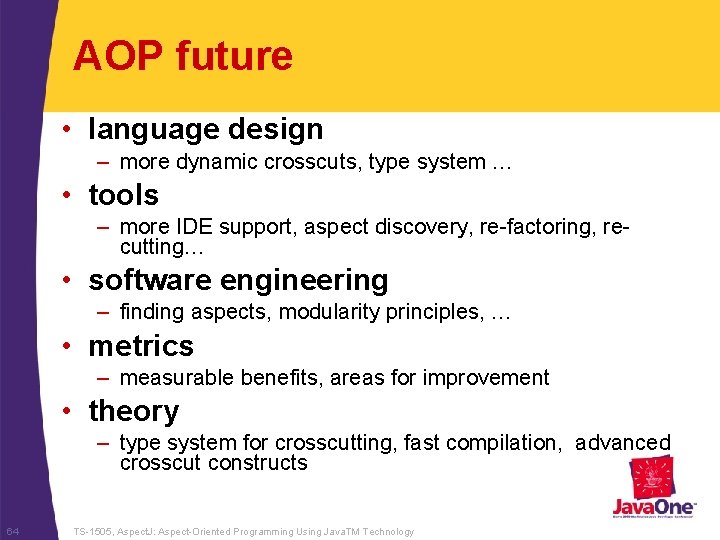
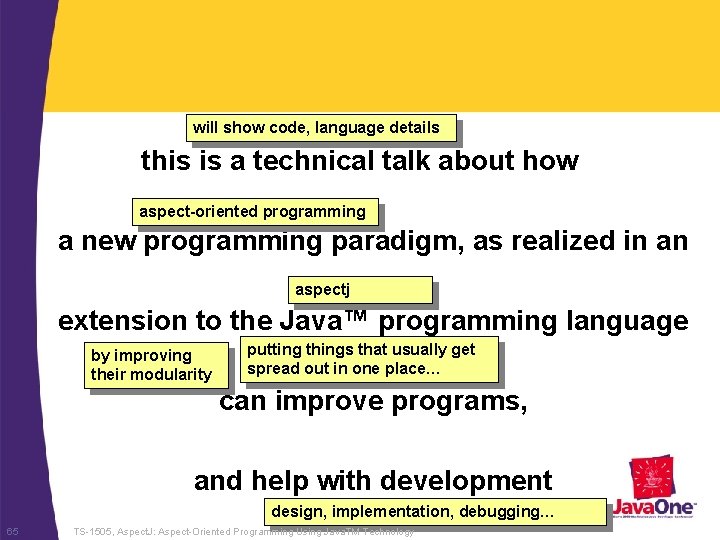
- Slides: 65
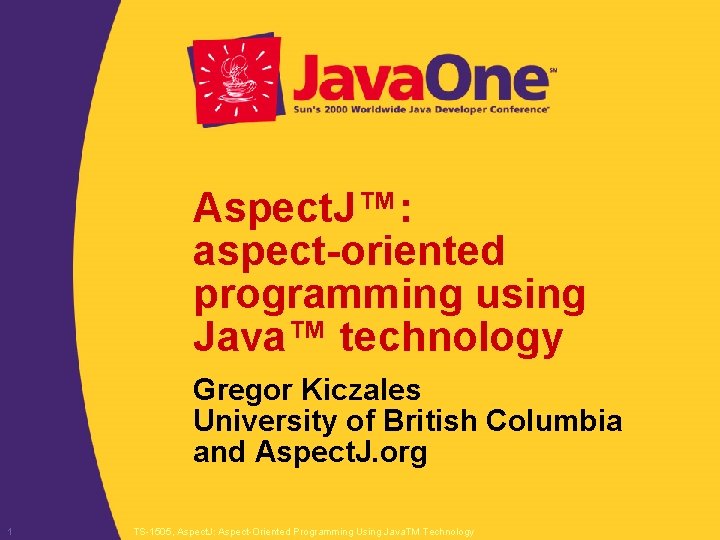
Aspect. J™: aspect-oriented programming using Java™ technology Gregor Kiczales University of British Columbia and Aspect. J. org 1 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
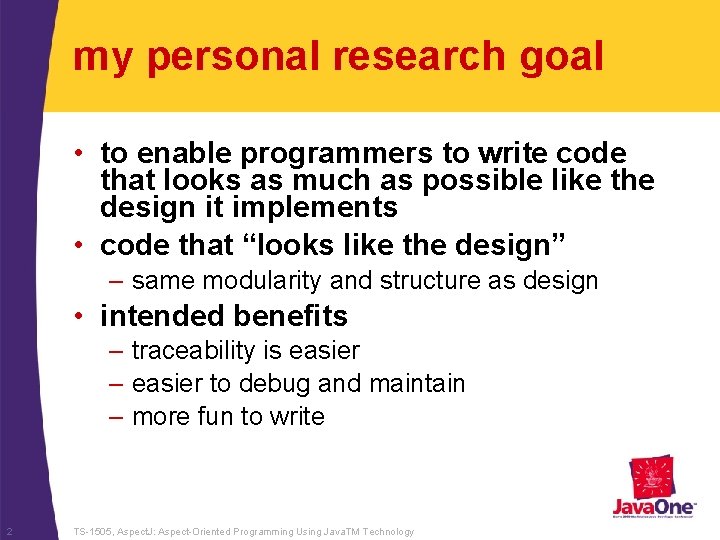
my personal research goal • to enable programmers to write code that looks as much as possible like the design it implements • code that “looks like the design” – same modularity and structure as design • intended benefits – traceability is easier – easier to debug and maintain – more fun to write 2 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
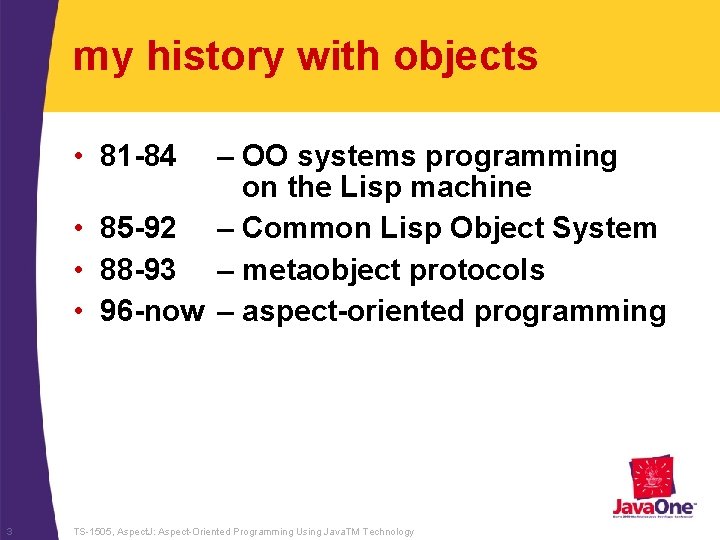
my history with objects • 81 -84 – OO systems programming on the Lisp machine • 85 -92 – Common Lisp Object System • 88 -93 – metaobject protocols • 96 -now – aspect-oriented programming 3 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
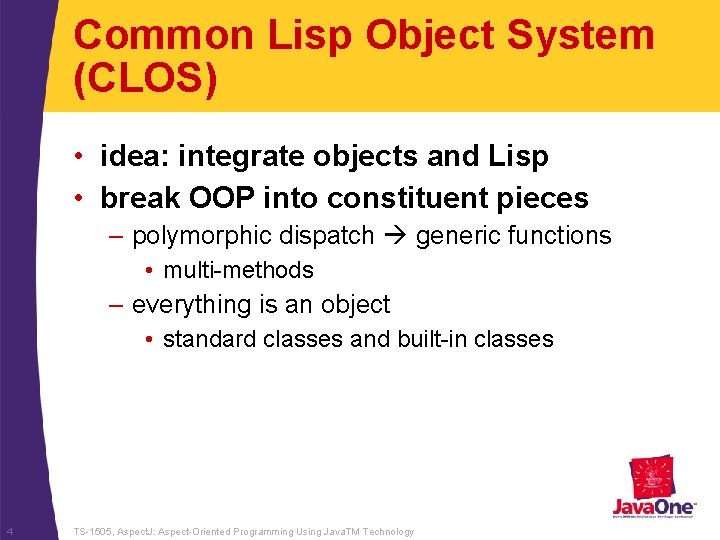
Common Lisp Object System (CLOS) • idea: integrate objects and Lisp • break OOP into constituent pieces – polymorphic dispatch generic functions • multi-methods – everything is an object • standard classes and built-in classes 4 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
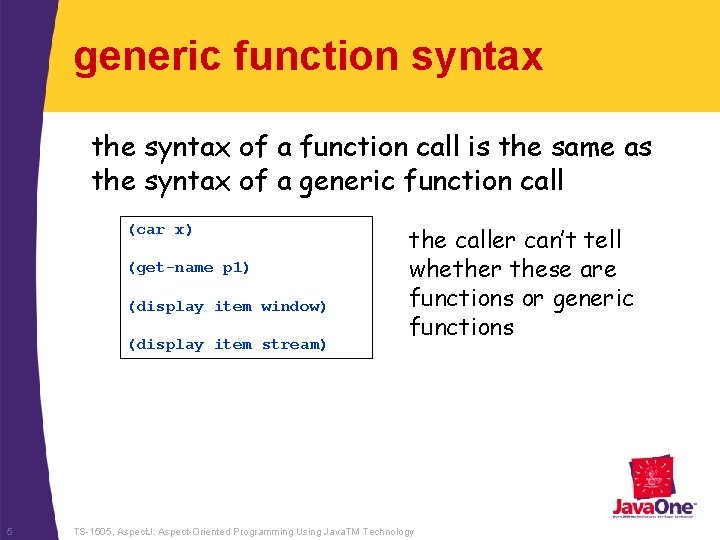
generic function syntax the syntax of a function call is the same as the syntax of a generic function call (car x) (get-name p 1) (display item window) (display item stream) 5 the caller can’t tell whether these are functions or generic functions TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
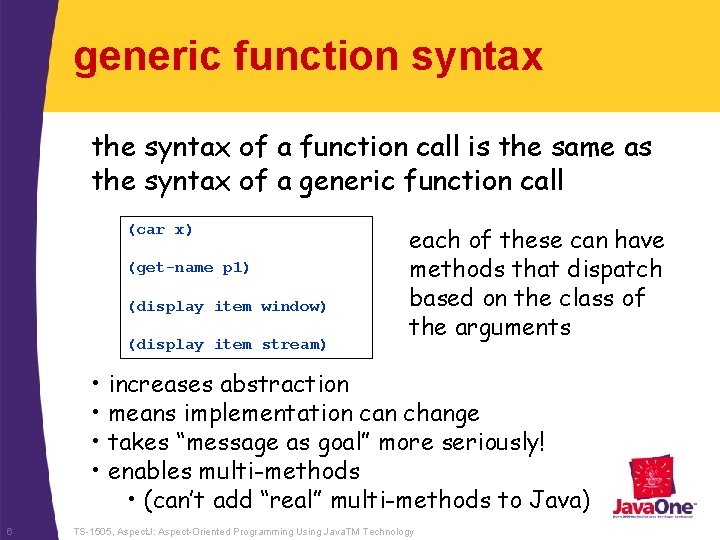
generic function syntax the syntax of a function call is the same as the syntax of a generic function call (car x) (get-name p 1) (display item window) (display item stream) each of these can have methods that dispatch based on the class of the arguments • increases abstraction • means implementation can change • takes “message as goal” more seriously! • enables multi-methods • (can’t add “real” multi-methods to Java) 6 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
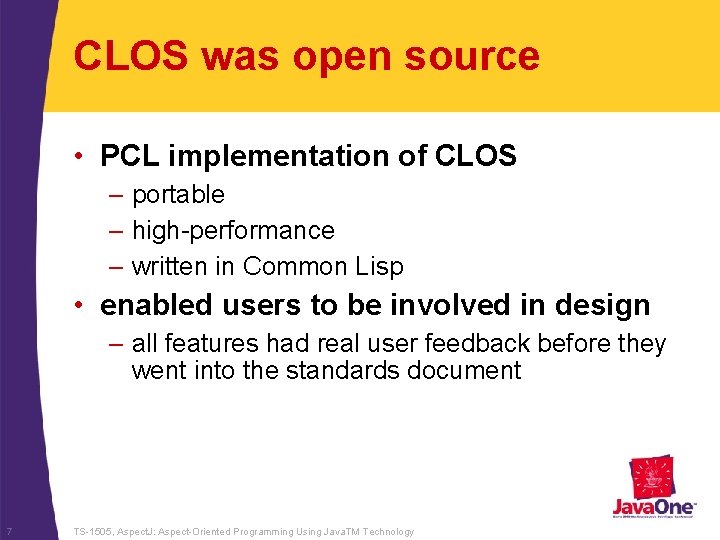
CLOS was open source • PCL implementation of CLOS – portable – high-performance – written in Common Lisp • enabled users to be involved in design – all features had real user feedback before they went into the standards document 7 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
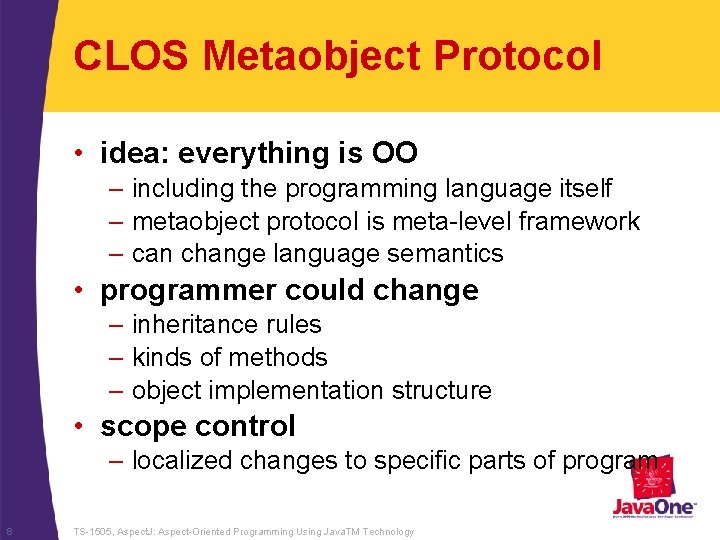
CLOS Metaobject Protocol • idea: everything is OO – including the programming language itself – metaobject protocol is meta-level framework – can change language semantics • programmer could change – inheritance rules – kinds of methods – object implementation structure • scope control – localized changes to specific parts of program 8 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
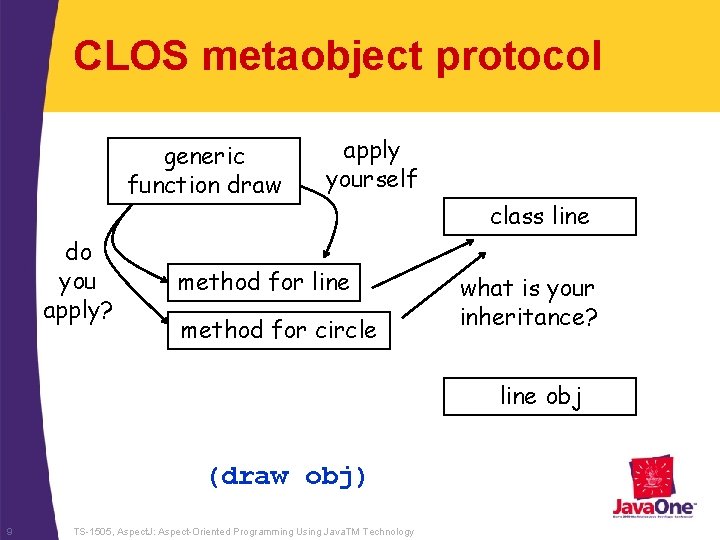
CLOS metaobject protocol generic function draw do you apply? apply yourself method for line method for circle class line what is your inheritance? line obj (draw obj) 9 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
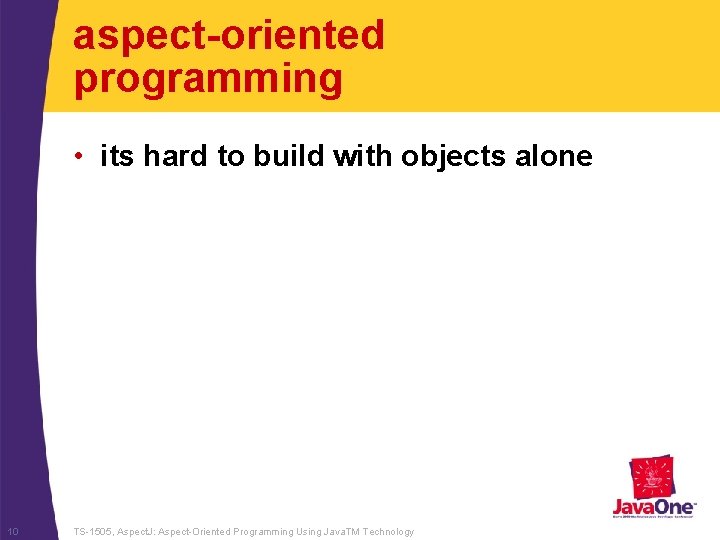
aspect-oriented programming • its hard to build with objects alone 10 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
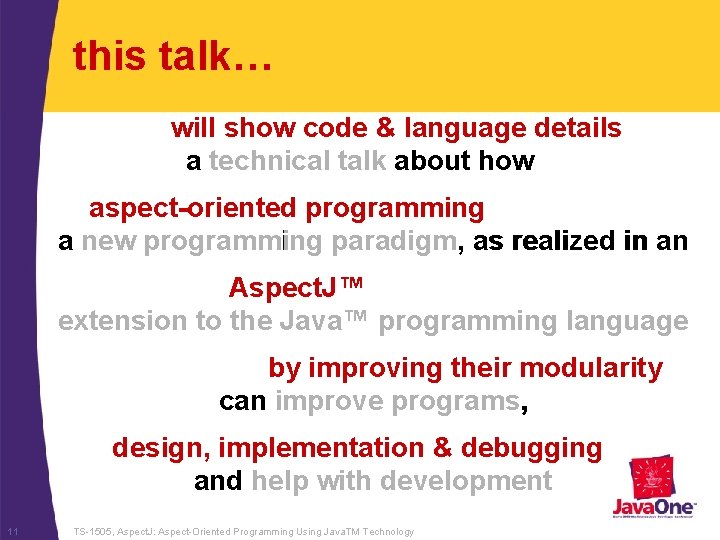
this talk… will show code & language details a technical talk about how aspect-oriented programming a new programming paradigm, as realized in an Aspect. J™ extension to the Java™ programming language by improving their modularity can improve programs, design, implementation & debugging and help with development 11 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
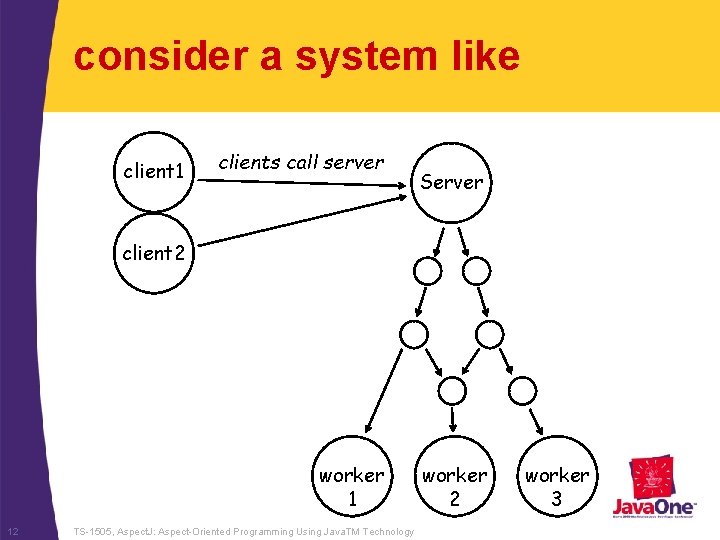
consider a system like client 1 clients call server Server client 2 worker 1 12 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
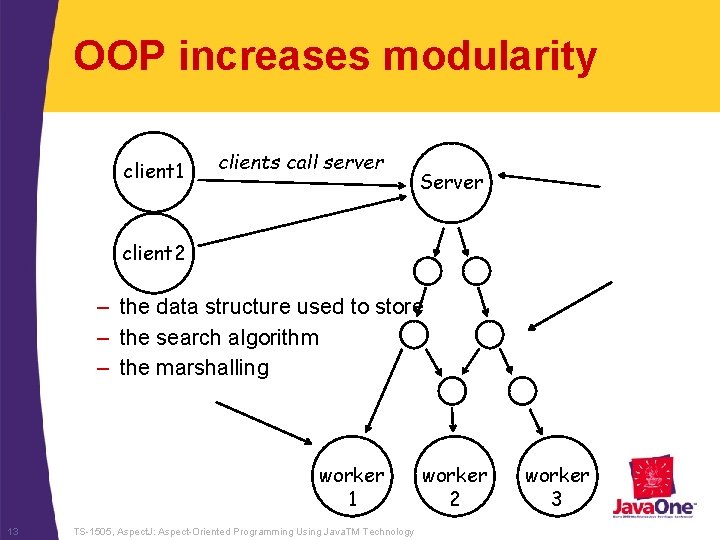
OOP increases modularity client 1 clients call server Server client 2 – the data structure used to store – the search algorithm – the marshalling worker 1 13 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
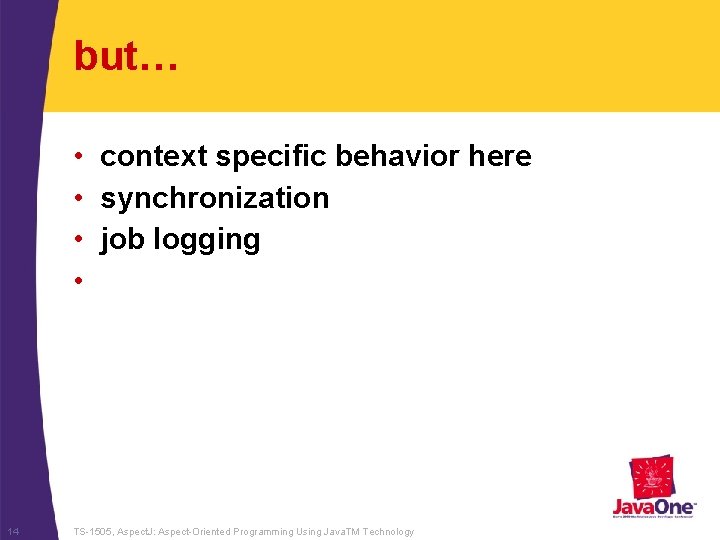
but… • context specific behavior here • synchronization • job logging • 14 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
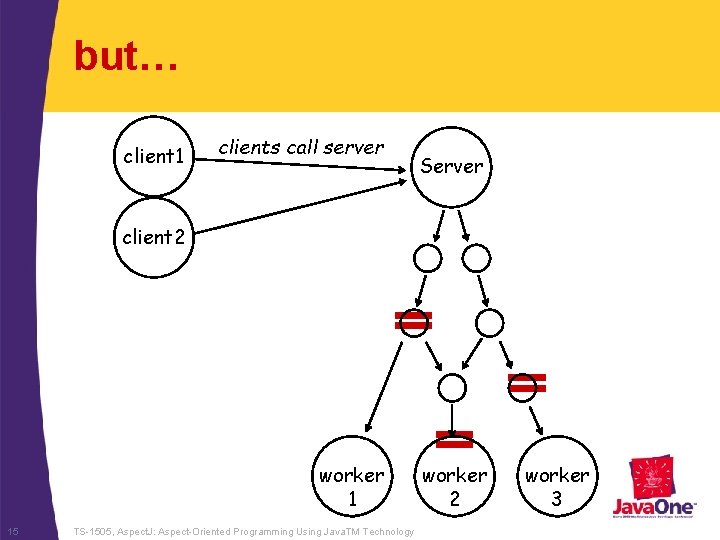
but… client 1 clients call server Server client 2 worker 1 15 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
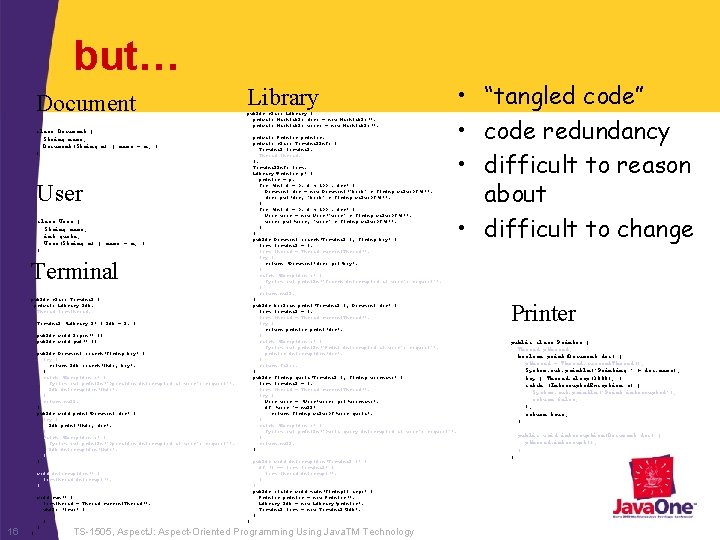
but… Document Library public class Library { private Hashtable docs = new Hashtable(); private Hashtable users = new Hashtable(); class Document { String name; Document(String n) { name = n; } } private Printer printer; private class Terminal. Info { Terminal terminal; Thread thread; }; Terminal. Info term; Library(Printer p) { printer = p; for (int i = 0; i < 100 ; i++) { Document doc = new Document("book" + String. value. Of(i)); docs. put(doc, "book" + String. value. Of(i)); } for (int i = 0; i < 100 ; i++) { User user = new User("user" + String. value. Of(i)); users. put(user, "user" + String. value. Of(i)); } } public Document search(Terminal t, String key) { terminal = t; term. thread = Thread. current. Thread(); try { return (Document)docs. get(key); } catch (Exception e) { System. out. println("Search interrupted at user's request"); } return null; } public boolean print(Terminal t, Document doc) { terminal = t; term. thread = Thread. current. Thread(); try { return printer. print(doc); } catch (Exception e) { System. out. println("Print interrupted at user's request"); printerruption(doc); } return false; } public String quota(Terminal t, String username) { terminal = t; term. thread = Thread. current. Thread(); try { User user = (User)users. get(username); if (user != null) return String. value. Of(user. quota); } catch (Exception e) { System. out. println("Quota query interrupted at user's request"); } return null; } User class User { String name; int quota; User(String n) { name = n; } } Terminal public class Terminal { private Library lib; Thread termthread; Terminal (Library l) { lib = l; } public void logon() {} public void gui() {} public Document search(String key) { try { return lib. search(this, key); } catch (Exception e) { System. out. println("Operation interrupted at user's request"); lib. interruption(this); } return null; } public void print(Document doc) { try { lib. print(this, doc); } catch (Exception e) { System. out. println("Operation interrupted at user's request"); lib. interruption(this); } } public void interruption(Terminal t) { if (t == terminal) { term. thread. interrupt(); } } public static void main(String[] args) { Printer printer = new Printer(); Library lib = new Library(printer); Terminal term = new Terminal(lib); } void interruption() { termthread. interrupt(); } void run() { termthread = Thread. current. Thread(); while (true) { } 16 } } • “tangled code” • code redundancy • difficult to reason about • difficult to change } TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology Printer public class Printer { Thread pthread; boolean print(Document doc) { pthread = Thread. current. Thread(); System. out. println("Printing " + doc. name); try { Thread. sleep(3000); } catch (Interrupted. Exception e) { System. out. println("Print interrupted"); return false; }; return true; } public void interruption(Document doc) { pthread. interrupt(); } }
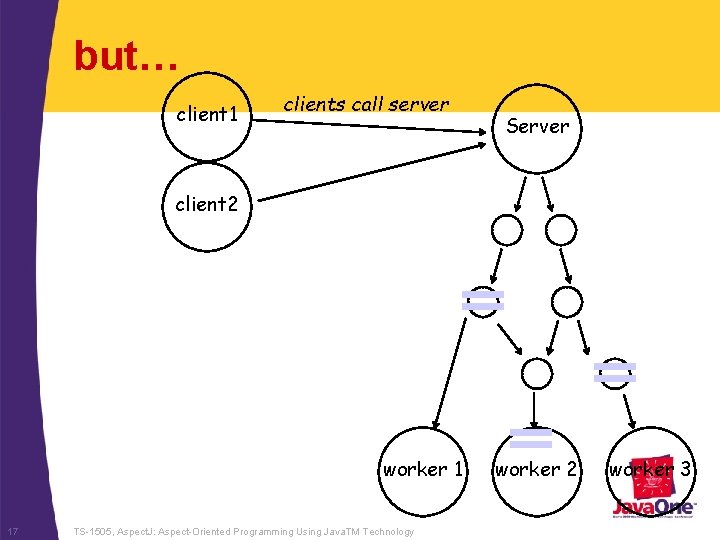
but… client 1 clients call server Server client 2 worker 1 17 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
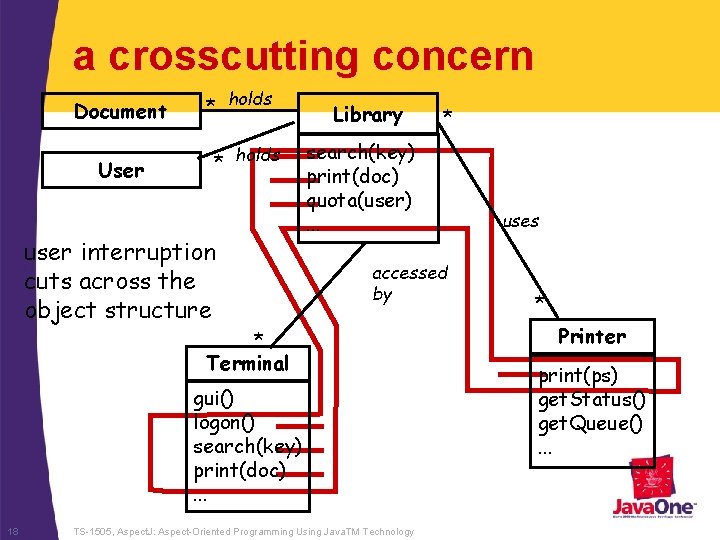
a crosscutting concern Document User * holds user interruption cuts across the object structure Library search(key) print(doc) quota(user). . . accessed by * Terminal gui() logon() search(key) print(doc). . . 18 * TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology uses * Printer print(ps) get. Status() get. Queue(). . .
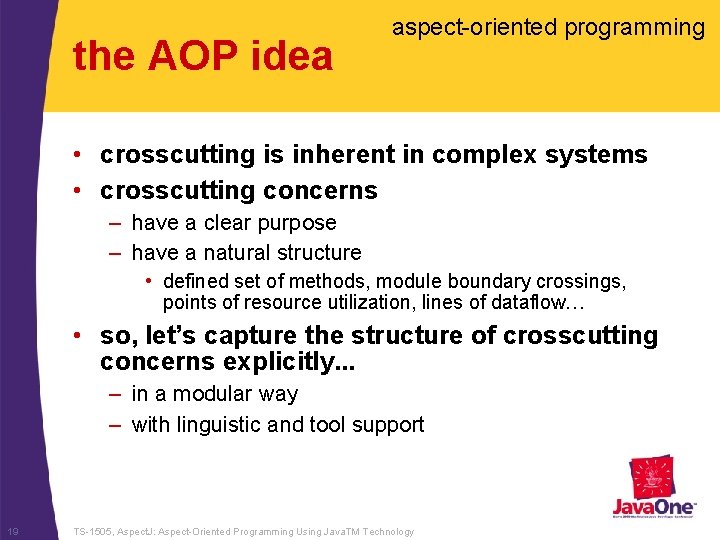
the AOP idea aspect-oriented programming • crosscutting is inherent in complex systems • crosscutting concerns – have a clear purpose – have a natural structure • defined set of methods, module boundary crossings, points of resource utilization, lines of dataflow… • so, let’s capture the structure of crosscutting concerns explicitly. . . – in a modular way – with linguistic and tool support 19 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
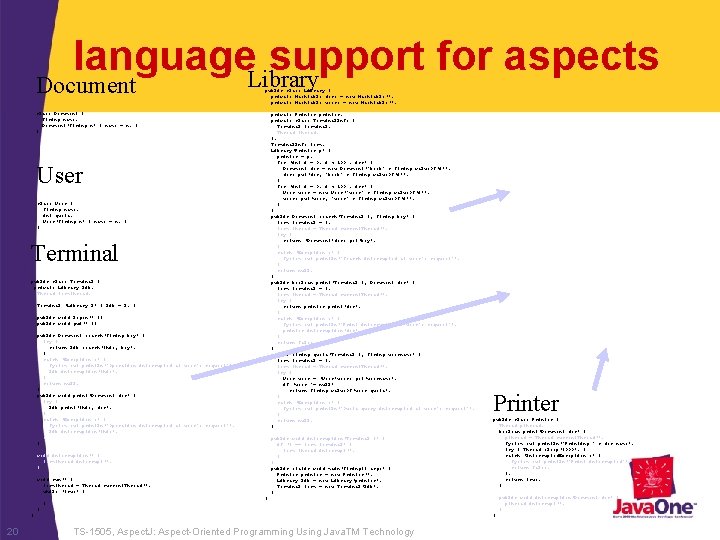
language. Library support for aspects Document public class Library { private Hashtable docs = new Hashtable(); private Hashtable users = new Hashtable(); class Document { String name; Document(String n) { name = n; } } private Printer printer; private class Terminal. Info { Terminal terminal; Thread thread; }; Terminal. Info term; Library(Printer p) { printer = p; for (int i = 0; i < 100 ; i++) { Document doc = new Document("book" + String. value. Of(i)); docs. put(doc, "book" + String. value. Of(i)); } for (int i = 0; i < 100 ; i++) { User user = new User("user" + String. value. Of(i)); users. put(user, "user" + String. value. Of(i)); } } public Document search(Terminal t, String key) { terminal = t; term. thread = Thread. current. Thread(); try { return (Document)docs. get(key); } catch (Exception e) { System. out. println("Search interrupted at user's request"); } return null; } public boolean print(Terminal t, Document doc) { terminal = t; term. thread = Thread. current. Thread(); try { return printer. print(doc); } catch (Exception e) { System. out. println("Print interrupted at user's request"); printerruption(doc); } return false; } public String quota(Terminal t, String username) { terminal = t; term. thread = Thread. current. Thread(); try { User user = (User)users. get(username); if (user != null) return String. value. Of(user. quota); } catch (Exception e) { System. out. println("Quota query interrupted at user's request"); } return null; } User class User { String name; int quota; User(String n) { name = n; } } Terminal public class Terminal { private Library lib; Thread termthread; Terminal (Library l) { lib = l; } public void logon() {} public void gui() {} public Document search(String key) { try { return lib. search(this, key); } catch (Exception e) { System. out. println("Operation interrupted at user's request"); lib. interruption(this); } return null; } public void print(Document doc) { try { lib. print(this, doc); } catch (Exception e) { System. out. println("Operation interrupted at user's request"); lib. interruption(this); } } public void interruption(Terminal t) { if (t == terminal) { term. thread. interrupt(); } } public static void main(String[] args) { Printer printer = new Printer(); Library lib = new Library(printer); Terminal term = new Terminal(lib); } void interruption() { termthread. interrupt(); } void run() { termthread = Thread. current. Thread(); while (true) { } Printer public class Printer { Thread pthread; boolean print(Document doc) { pthread = Thread. current. Thread(); System. out. println("Printing " + doc. name); try { Thread. sleep(3000); } catch (Interrupted. Exception e) { System. out. println("Print interrupted"); return false; }; return true; } public void interruption(Document doc) { pthread. interrupt(); } } 20 } TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
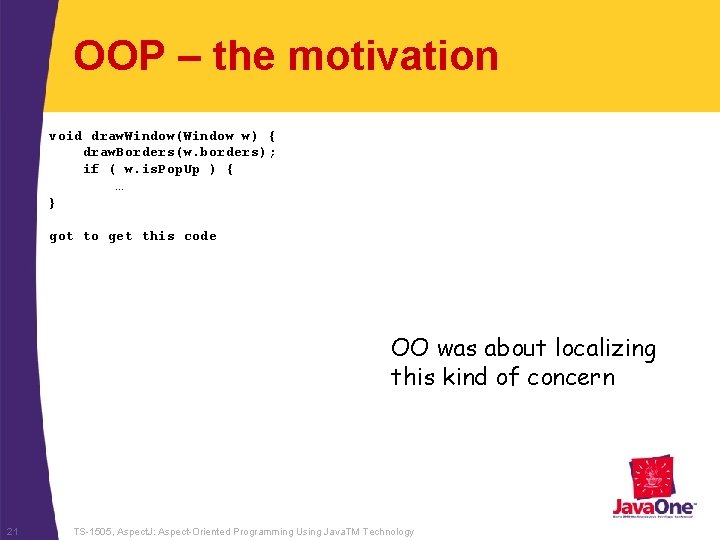
OOP – the motivation void draw. Window(Window w) { draw. Borders(w. borders); if ( w. is. Pop. Up ) { … } got to get this code OO was about localizing this kind of concern 21 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
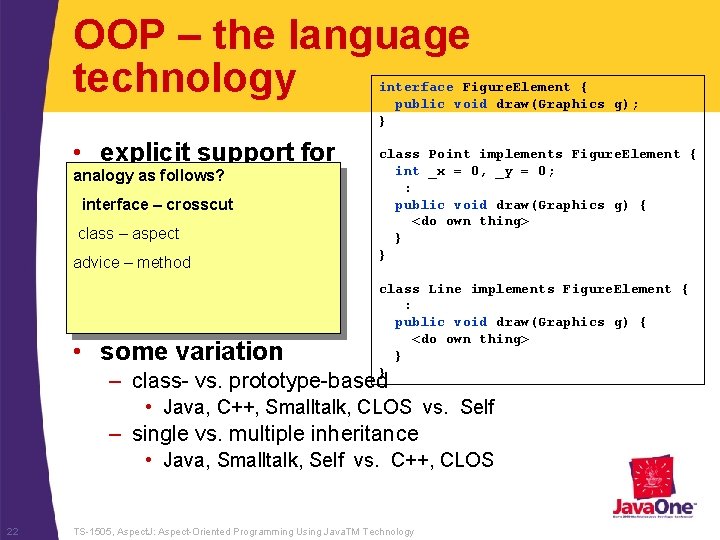
OOP – the language technology interface Figure. Element { public void draw(Graphics g); } • explicit support for analogy as follows? as – message goal interface – crosscut – encapsulation class–– polymorphism aspect advice method – –inheritance • some variation class Point implements Figure. Element { int _x = 0, _y = 0; : public void draw(Graphics g) { <do own thing> } } class Line implements Figure. Element { : public void draw(Graphics g) { <do own thing> } } – class- vs. prototype-based • Java, C++, Smalltalk, CLOS vs. Self – single vs. multiple inheritance • Java, Smalltalk, Self vs. C++, CLOS 22 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
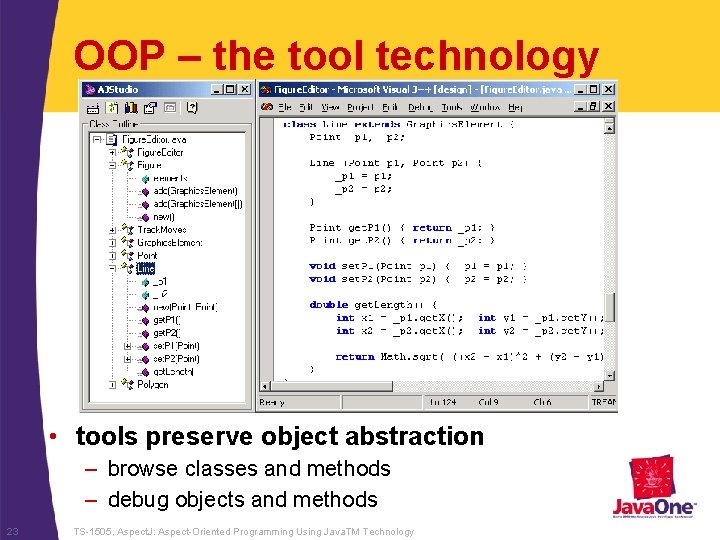
OOP – the tool technology • tools preserve object abstraction – browse classes and methods – debug objects and methods 23 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
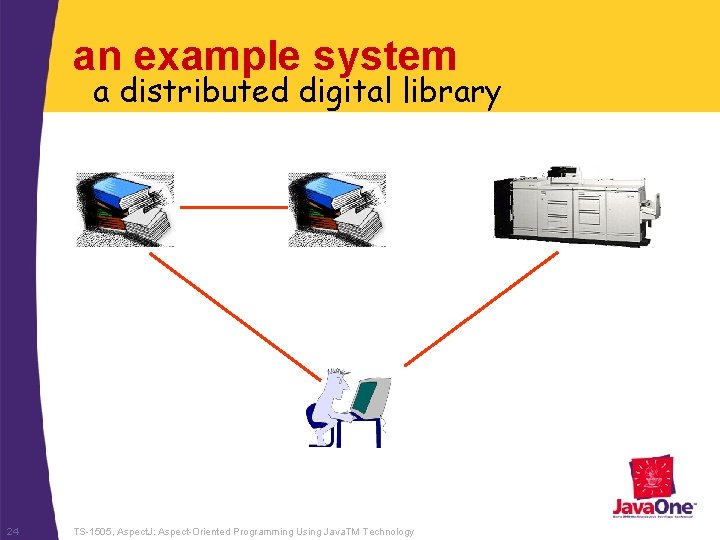
an example system a distributed digital library 24 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
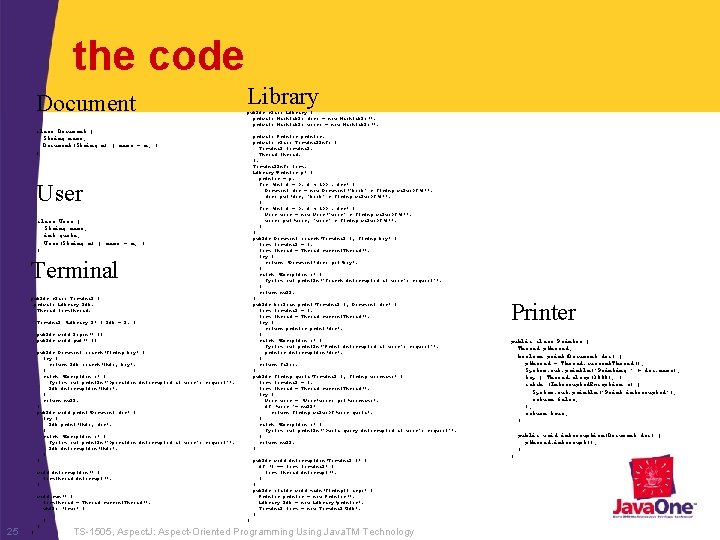
the code Document Library public class Library { private Hashtable docs = new Hashtable(); private Hashtable users = new Hashtable(); class Document { String name; Document(String n) { name = n; } } private Printer printer; private class Terminal. Info { Terminal terminal; Thread thread; }; Terminal. Info term; Library(Printer p) { printer = p; for (int i = 0; i < 100 ; i++) { Document doc = new Document("book" + String. value. Of(i)); docs. put(doc, "book" + String. value. Of(i)); } for (int i = 0; i < 100 ; i++) { User user = new User("user" + String. value. Of(i)); users. put(user, "user" + String. value. Of(i)); } } public Document search(Terminal t, String key) { terminal = t; term. thread = Thread. current. Thread(); try { return (Document)docs. get(key); } catch (Exception e) { System. out. println("Search interrupted at user's request"); } return null; } public boolean print(Terminal t, Document doc) { terminal = t; term. thread = Thread. current. Thread(); try { return printer. print(doc); } catch (Exception e) { System. out. println("Print interrupted at user's request"); printerruption(doc); } return false; } public String quota(Terminal t, String username) { terminal = t; term. thread = Thread. current. Thread(); try { User user = (User)users. get(username); if (user != null) return String. value. Of(user. quota); } catch (Exception e) { System. out. println("Quota query interrupted at user's request"); } return null; } User class User { String name; int quota; User(String n) { name = n; } } Terminal public class Terminal { private Library lib; Thread termthread; Terminal (Library l) { lib = l; } public void logon() {} public void gui() {} public Document search(String key) { try { return lib. search(this, key); } catch (Exception e) { System. out. println("Operation interrupted at user's request"); lib. interruption(this); } return null; } public void print(Document doc) { try { lib. print(this, doc); } catch (Exception e) { System. out. println("Operation interrupted at user's request"); lib. interruption(this); } } public void interruption(Terminal t) { if (t == terminal) { term. thread. interrupt(); } } public static void main(String[] args) { Printer printer = new Printer(); Library lib = new Library(printer); Terminal term = new Terminal(lib); } void interruption() { termthread. interrupt(); } void run() { termthread = Thread. current. Thread(); while (true) { } 25 } } } TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology Printer public class Printer { Thread pthread; boolean print(Document doc) { pthread = Thread. current. Thread(); System. out. println("Printing " + doc. name); try { Thread. sleep(3000); } catch (Interrupted. Exception e) { System. out. println("Print interrupted"); return false; }; return true; } public void interruption(Document doc) { pthread. interrupt(); } }
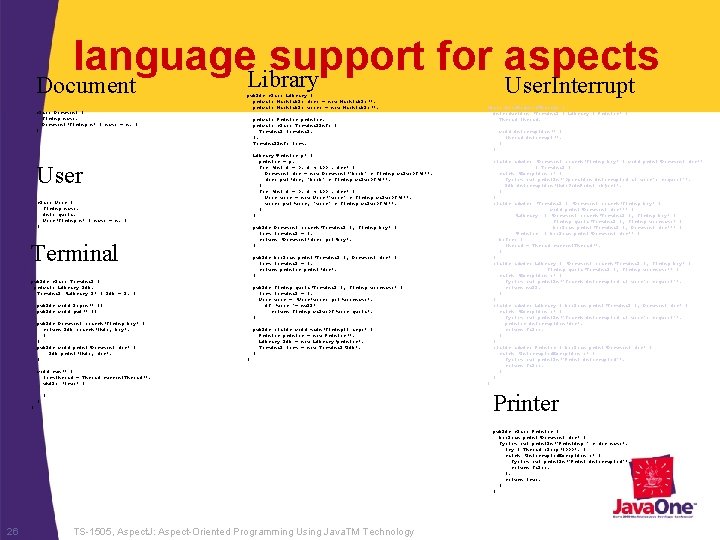
language. Library support for aspects Document class Document { String name; Document(String n) { name = n; } } public class Library { private Hashtable docs = new Hashtable(); private Hashtable users = new Hashtable(); private Printer printer; private class Terminal. Info { Terminal terminal; }; Terminal. Info term; class User. Request. Threads { introduction (Terminal | Library | Printer) { Thread thread; void interruption() { thread. interrupt(); } } Library(Printer p) { printer = p; for (int i = 0; i < 100 ; i++) { Document doc = new Document("book" + String. value. Of(i)); docs. put(doc, "book" + String. value. Of(i)); } for (int i = 0; i < 100 ; i++) { User user = new User("user" + String. value. Of(i)); users. put(user, "user" + String. value. Of(i)); } } User class User { String name; into quota; User(String n) { name = n; } } static advice (Document search(String key) | void print(Document doc)) & Terminal { catch (Exception e) { System. out. println("Operation interrupted at user's request"); lib. interruption(this. Join. Point. object); } } static advice (Terminal & (Document search(String key) | void print(Document doc))) | (Library & (Document search(Terminal t, String key) | String quota(Terminal t, String username) | boolean print(Terminal t, Document doc))) | (Printer & boolean print(Document doc)) { before { thread = Thread. current. Thread(); } } static advice Library & (Document search(Terminal t, String key) | String quota(Terminal t, String username)) { catch (Exception e) { System. out. println("Search interrupted at user's request"); return null; } } static advice Library & boolean print(Terminal t, Document doc) { catch (Exception e) { System. out. println("Search interrupted at user's request"); printerruption(doc); return false; } } static advice Printer & boolean print(Document doc) { catch (Interrupted. Exception e) { System. out. println("Print interrupted"); return false; } } public Document search(Terminal t, String key) { terminal = t; return (Document)docs. get(key); } Terminal public boolean print(Terminal t, Document doc) { terminal = t; return printer. print(doc); } public class Terminal { private Library lib; Terminal (Library l) { lib = l; } public String quota(Terminal t, String username) { terminal = t; User user = (User)users. get(username); if (user != null) return String. value. Of(user. quota); } public void logon() {} public void gui() {} public Document search(String key) { return lib. search(this, key); } } public void print(Document doc) { lib. print(this, doc); } User. Interrupt public static void main(String[] args) { Printer printer = new Printer(); Library lib = new Library(printer); Terminal term = new Terminal(lib); } } void run() { termthread = Thread. current. Thread(); while (true) { } Printer } } } public class Printer { boolean print(Document doc) { System. out. println("Printing " + doc. name); try { Thread. sleep(3000); } catch (Interrupted. Exception e) { System. out. println("Print interrupted"); return false; }; return true; } } 26 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
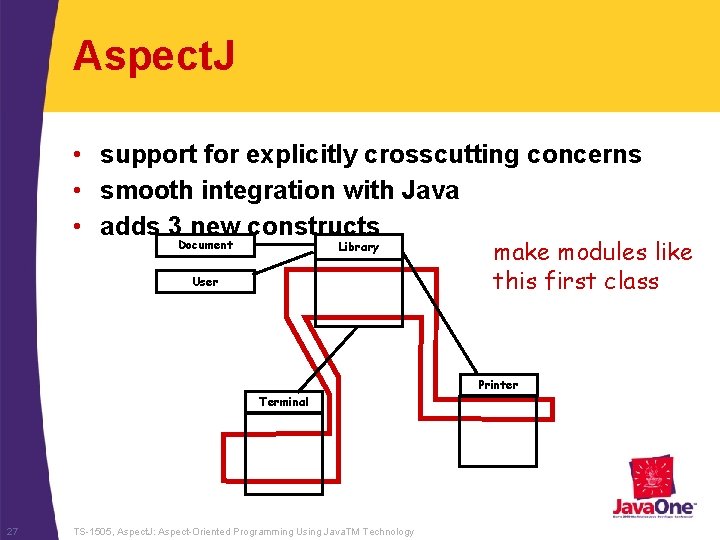
Aspect. J • support for explicitly crosscutting concerns • smooth integration with Java • adds 3 new constructs Document Library User make modules like this first class Printer Terminal 27 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
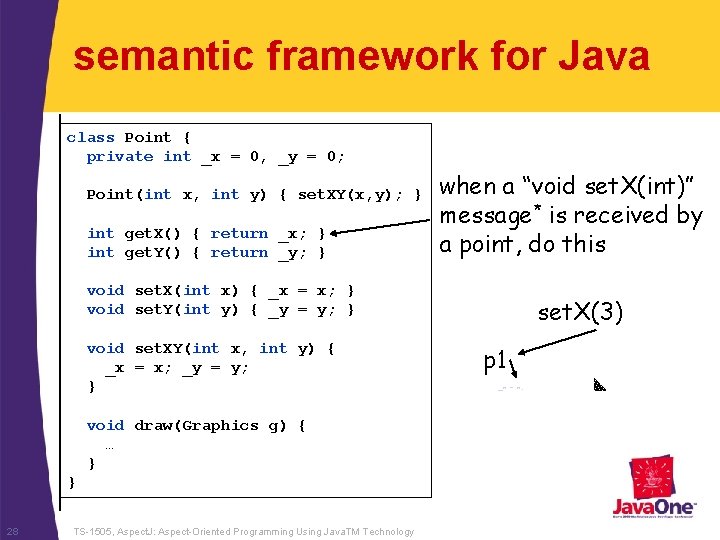
semantic framework for Java class Point { private int _x = 0, _y = 0; Point(int x, int y) { set. XY(x, y); } int get. X() { return _x; } int get. Y() { return _y; } when a “void set. X(int)” message* is received by a point, do this void set. X(int x) { _x = x; } void set. Y(int y) { _y = y; } void set. XY(int x, int y) { _x = x; _y = y; } void draw(Graphics g) { … } } 28 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology set. X(3) p 1 _x = x; return _y; return _x;
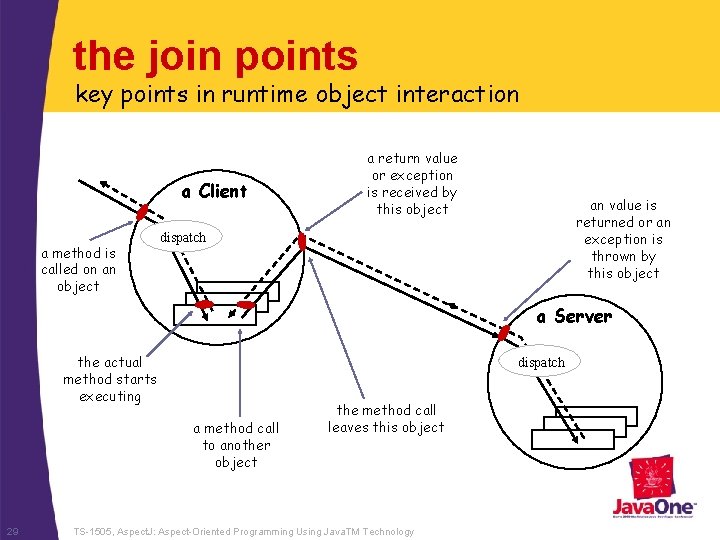
the join points key points in runtime object interaction a Client a method is called on an object a return value or exception is received by this object an value is returned or an exception is thrown by this object dispatch a Server the actual method starts executing dispatch a method call to another object 29 the method call leaves this object TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
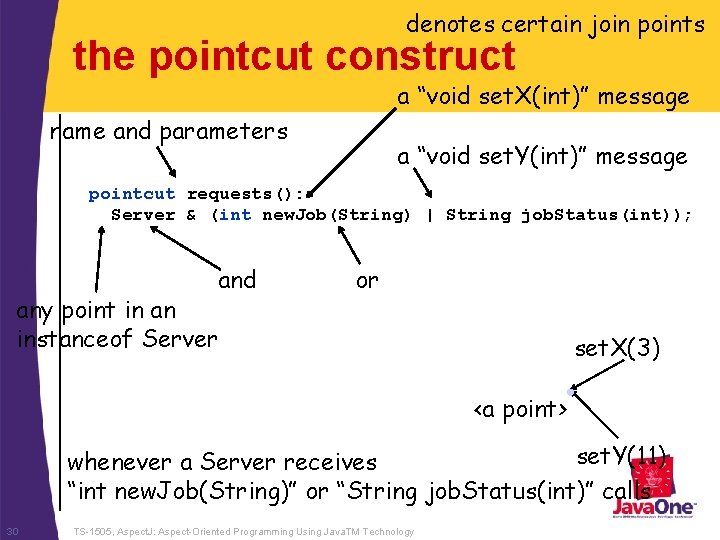
denotes certain join points the pointcut construct a “void set. X(int)” message name and parameters a “void set. Y(int)” message pointcut requests(): Server & (int new. Job(String) | String job. Status(int)); and any point in an instanceof Server or set. X(3) <a point> set. Y(11) whenever a Server receives “int new. Job(String)” or “String job. Status(int)” calls 30 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
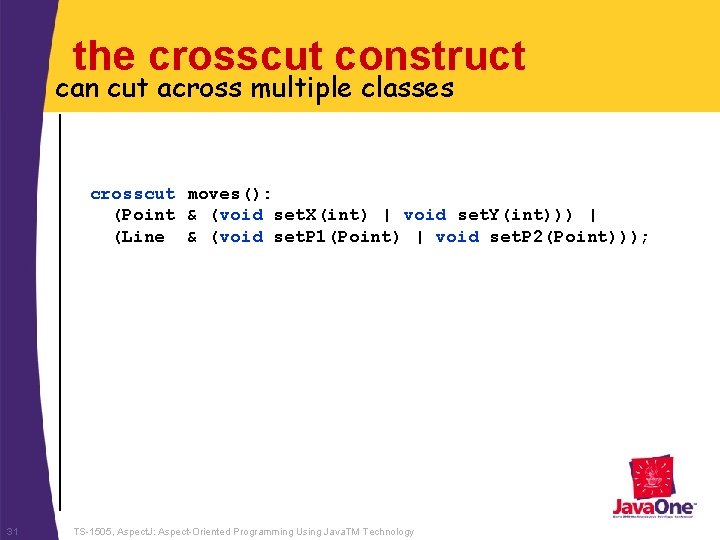
the crosscut construct can cut across multiple classes crosscut moves(): (Point & (void set. X(int) | void set. Y(int))) | (Line & (void set. P 1(Point) | void set. P 2(Point))); 31 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
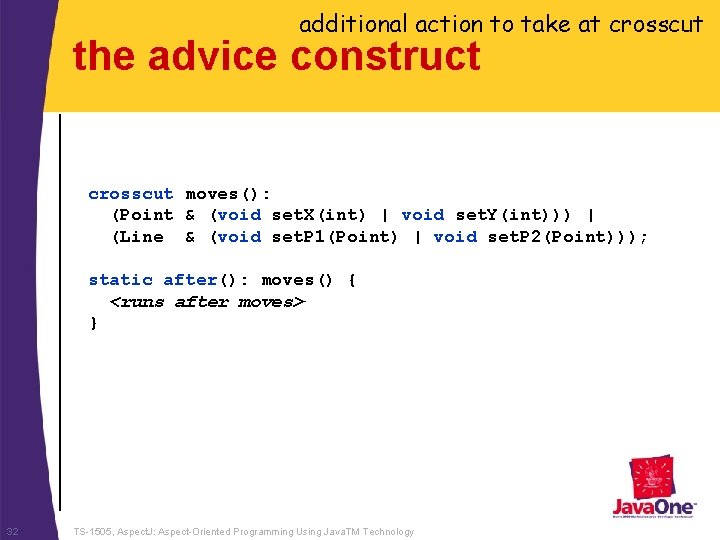
additional action to take at crosscut the advice construct crosscut moves(): (Point & (void set. X(int) | void set. Y(int))) | (Line & (void set. P 1(Point) | void set. P 2(Point))); static after(): moves() { <runs after moves> } 32 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
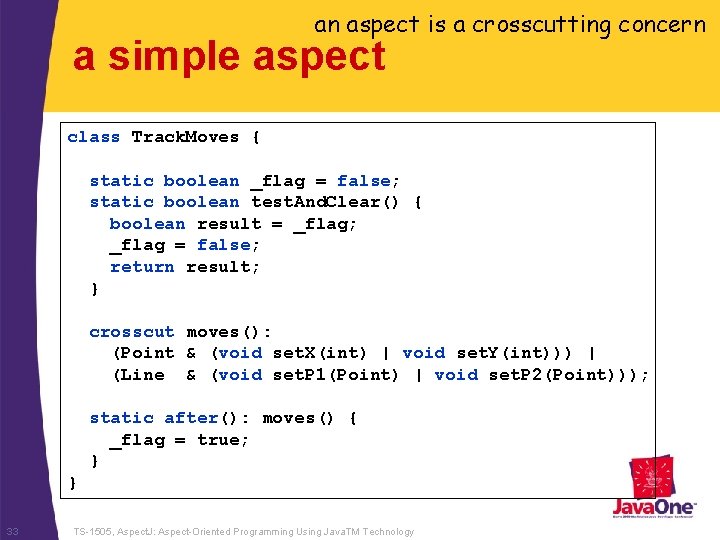
an aspect is a crosscutting concern a simple aspect class Track. Moves { static boolean _flag = false; static boolean test. And. Clear() { boolean result = _flag; _flag = false; return result; } crosscut moves(): (Point & (void set. X(int) | void set. Y(int))) | (Line & (void set. P 1(Point) | void set. P 2(Point))); static after(): moves() { _flag = true; } } 33 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
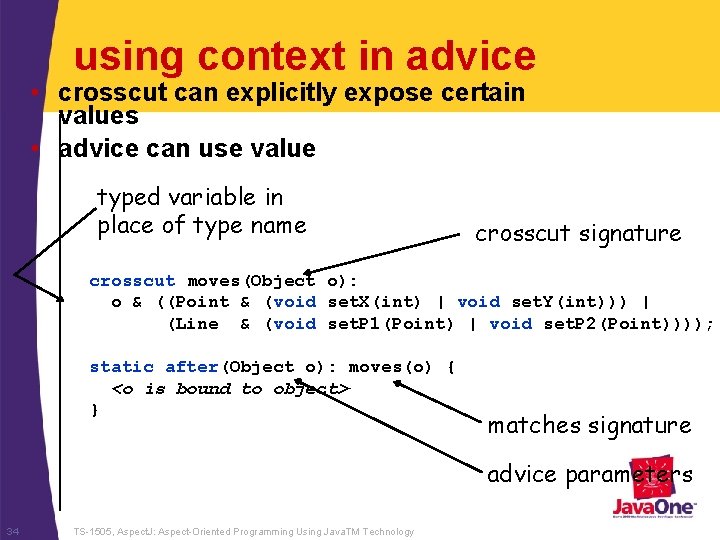
using context in advice • crosscut can explicitly expose certain values • advice can use value typed variable in place of type name crosscut signature crosscut moves(Object o): o & ((Point & (void set. X(int) | void set. Y(int))) | (Line & (void set. P 1(Point) | void set. P 2(Point)))); static after(Object o): moves(o) { <o is bound to object> } matches signature advice parameters 34 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
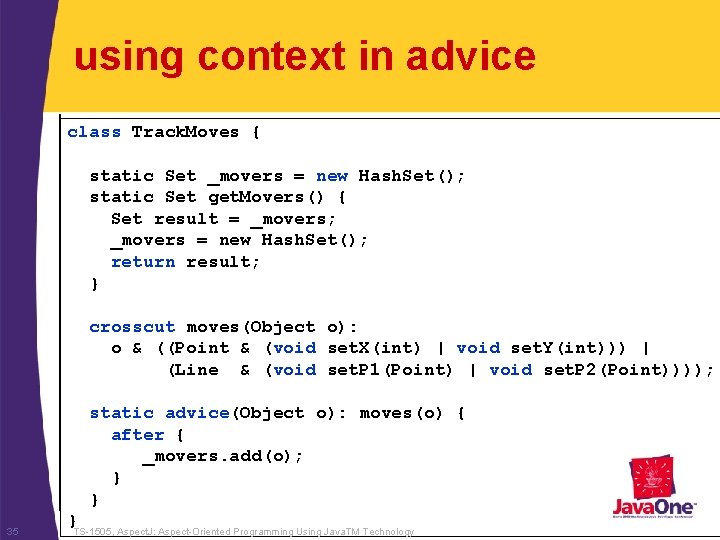
using context in advice class Track. Moves { static Set _movers = new Hash. Set(); static Set get. Movers() { Set result = _movers; _movers = new Hash. Set(); return result; } crosscut moves(Object o): o & ((Point & (void set. X(int) | void set. Y(int))) | (Line & (void set. P 1(Point) | void set. P 2(Point)))); static advice(Object o): moves(o) { after { _movers. add(o); } } 35 } TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
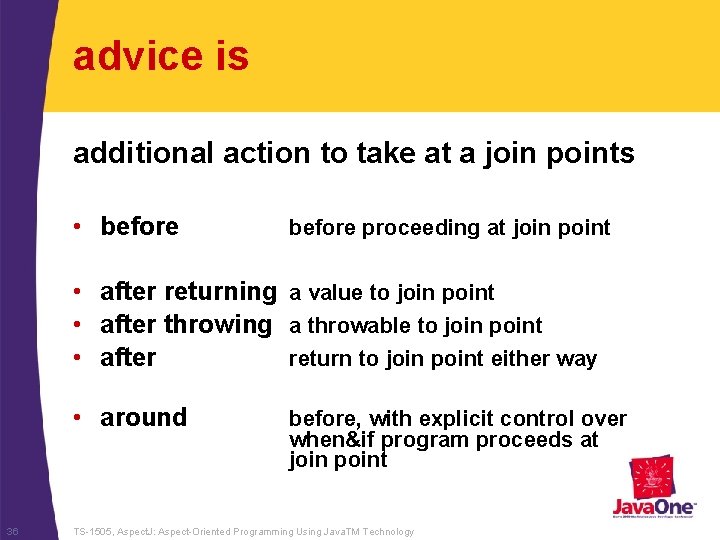
advice is additional action to take at a join points • before proceeding at join point • after returning a value to join point • after throwing a throwable to join point • after return to join point either way • around 36 before, with explicit control over when&if program proceeds at join point TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
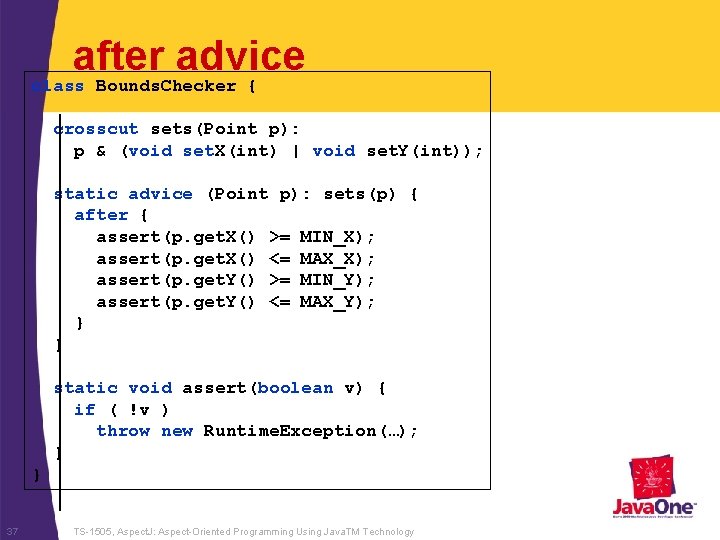
after advice class Bounds. Checker { crosscut sets(Point p): p & (void set. X(int) | void set. Y(int)); static advice (Point p): sets(p) { after { assert(p. get. X() >= MIN_X); assert(p. get. X() <= MAX_X); assert(p. get. Y() >= MIN_Y); assert(p. get. Y() <= MAX_Y); } } static void assert(boolean v) { if ( !v ) throw new Runtime. Exception(…); } } 37 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
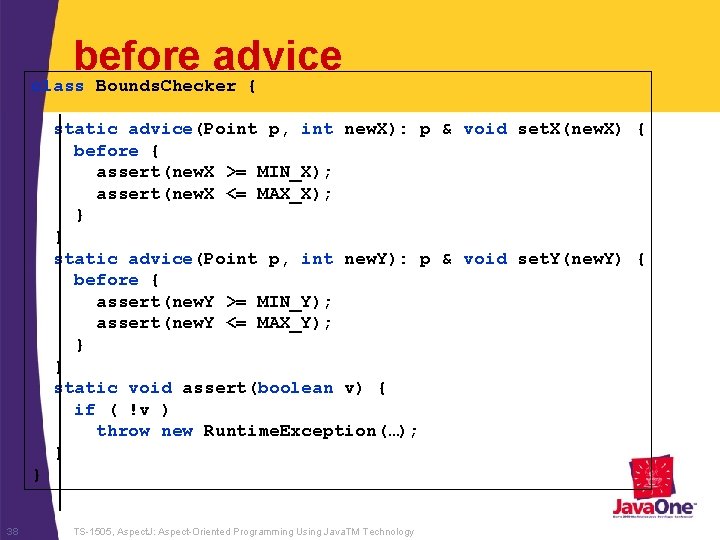
before advice class Bounds. Checker { static advice(Point p, int new. X): p & void set. X(new. X) { before { assert(new. X >= MIN_X); assert(new. X <= MAX_X); } } static advice(Point p, int new. Y): p & void set. Y(new. Y) { before { assert(new. Y >= MIN_Y); assert(new. Y <= MAX_Y); } } static void assert(boolean v) { if ( !v ) throw new Runtime. Exception(…); } } 38 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
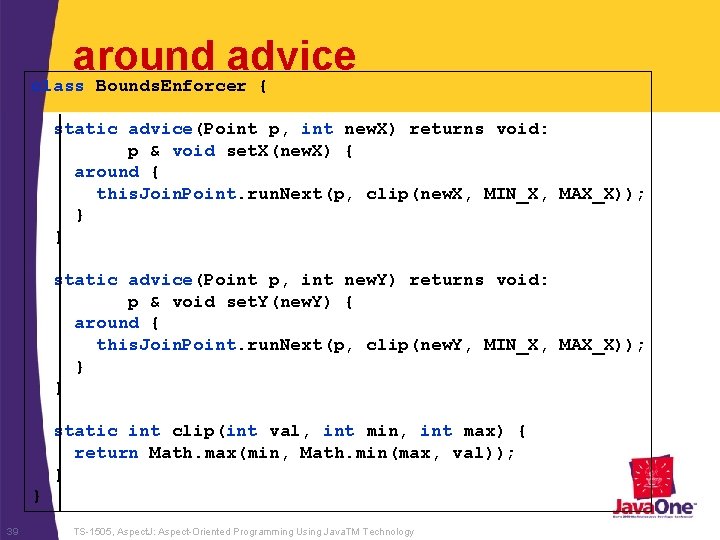
around advice class Bounds. Enforcer { static advice(Point p, int new. X) returns void: p & void set. X(new. X) { around { this. Join. Point. run. Next(p, clip(new. X, MIN_X, MAX_X)); } } static advice(Point p, int new. Y) returns void: p & void set. Y(new. Y) { around { this. Join. Point. run. Next(p, clip(new. Y, MIN_X, MAX_X)); } } static int clip(int val, int min, int max) { return Math. max(min, Math. min(max, val)); } } 39 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
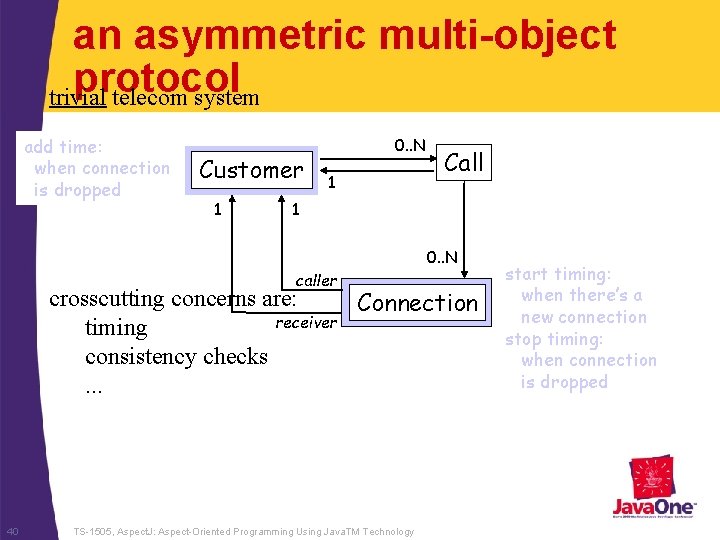
an asymmetric multi-object protocol trivial telecom system add time: total time when connection connected is dropped Customer 1 0. . N 1 Call 1 0. . N caller crosscutting concerns are: Connection receiver timing consistency checks. . . 40 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology start timing: time each when there’s a connection new connection stop timing: when connection is dropped
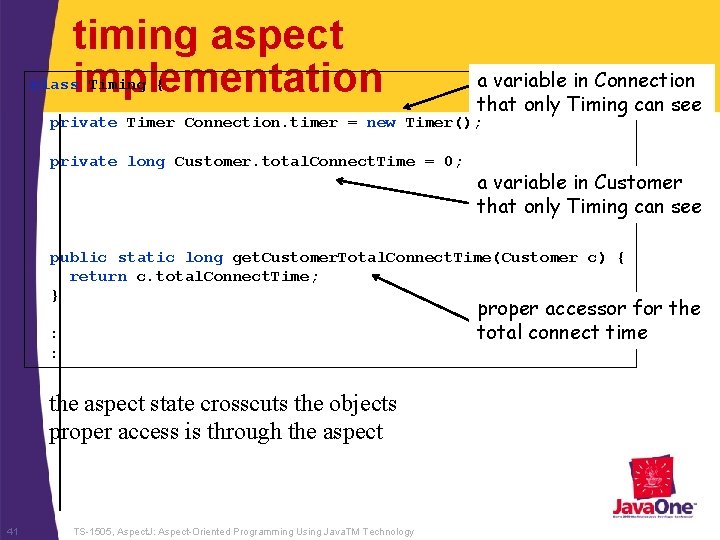
timing aspect implementation class Timing { a variable in Connection that only Timing can see private Timer Connection. timer = new Timer(); private long Customer. total. Connect. Time = 0; a variable in Customer that only Timing can see public static long get. Customer. Total. Connect. Time(Customer c) { return c. total. Connect. Time; } proper accessor for the total connect time : : the aspect state crosscuts the objects proper access is through the aspect 41 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
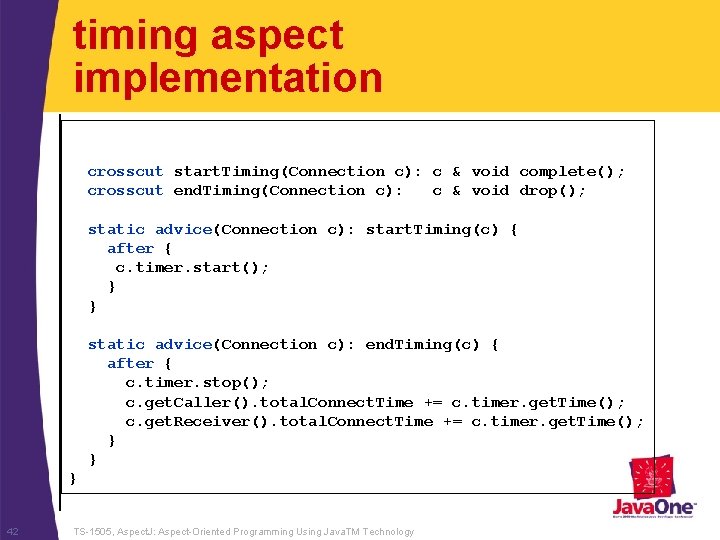
timing aspect implementation crosscut start. Timing(Connection c): c & void complete(); crosscut end. Timing(Connection c): c & void drop(); static advice(Connection c): start. Timing(c) { after { c. timer. start(); } } static advice(Connection c): end. Timing(c) { after { c. timer. stop(); c. get. Caller(). total. Connect. Time += c. timer. get. Time(); c. get. Receiver(). total. Connect. Time += c. timer. get. Time(); } } } 42 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
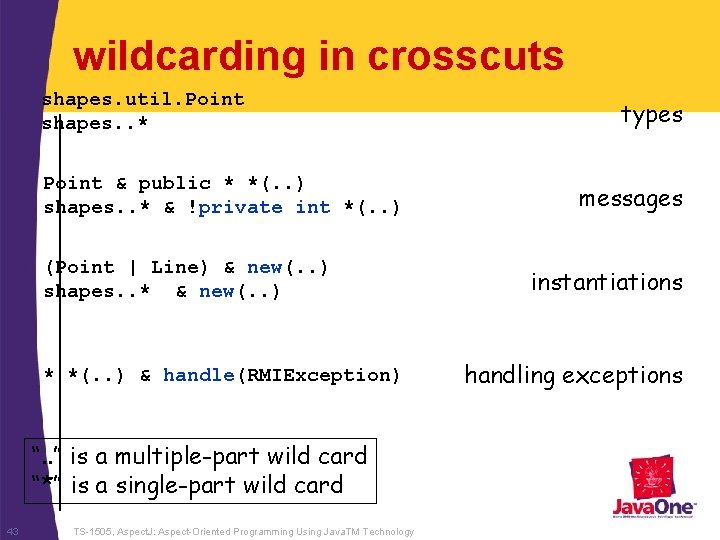
wildcarding in crosscuts shapes. util. Point shapes. . * Point & public * *(. . ) shapes. . * & !private int *(. . ) (Point | Line) & new(. . ) shapes. . * & new(. . ) * *(. . ) & handle(RMIException) “. . ” is a multiple-part wild card “*” is a single-part wild card 43 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology types messages instantiations handling exceptions
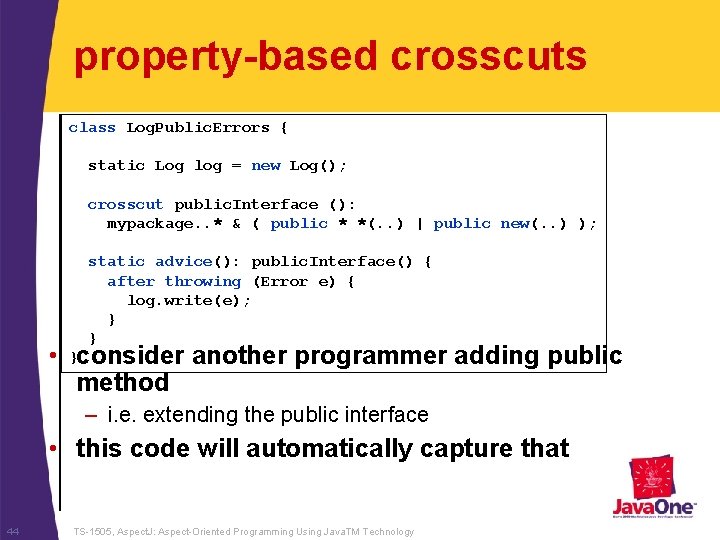
property-based crosscuts class Log. Public. Errors { static Log log = new Log(); crosscut public. Interface (): mypackage. . * & ( public * *(. . ) | public new(. . ) ); static advice(): public. Interface() { after throwing (Error e) { log. write(e); } } • }consider another programmer adding public method – i. e. extending the public interface • this code will automatically capture that 44 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
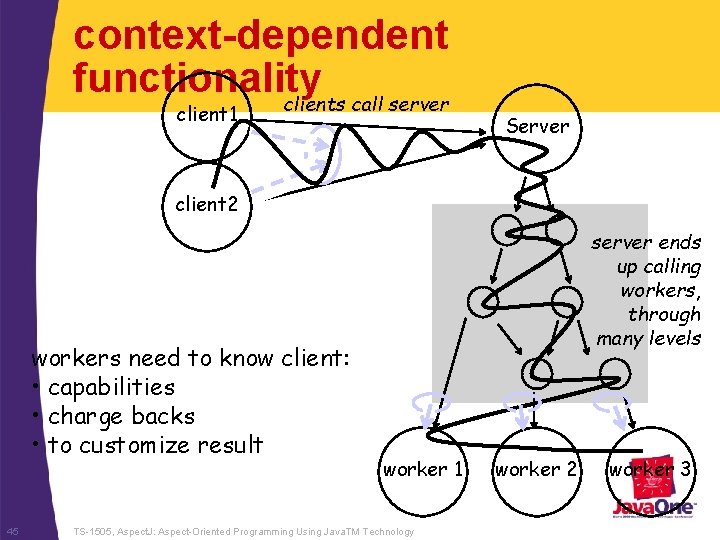
context-dependent functionality clients call server client 1 Server client 2 workers need to know client: • capabilities • charge backs • to customize result 45 server ends up calling workers, through many levels worker 1 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
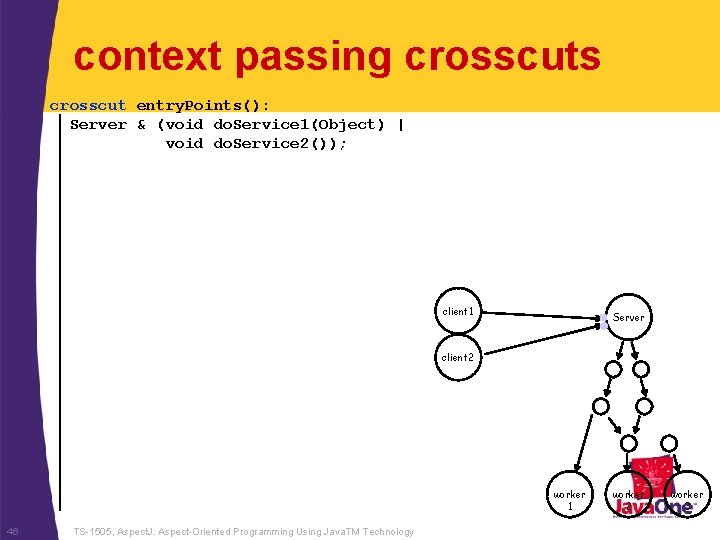
context passing crosscuts crosscut entry. Points(): Server & (void do. Service 1(Object) | void do. Service 2()); client 1 Server client 2 worker 1 46 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
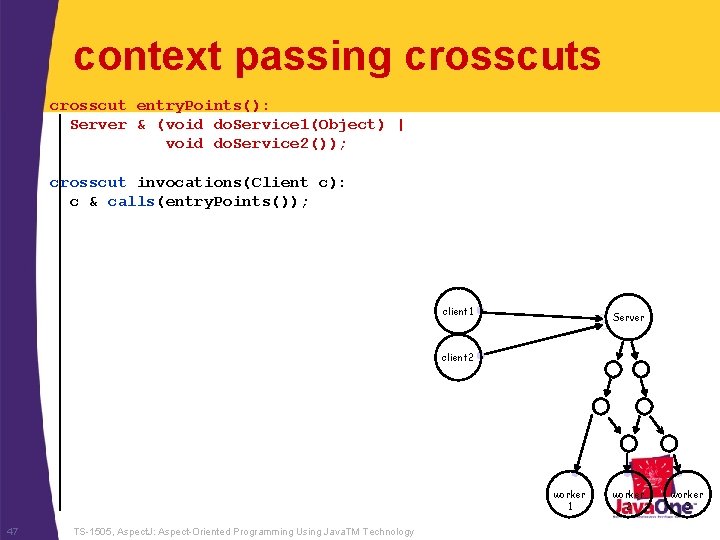
context passing crosscuts crosscut entry. Points(): Server & (void do. Service 1(Object) | void do. Service 2()); crosscut invocations(Client c): c & calls(entry. Points()); client 1 Server client 2 worker 1 47 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
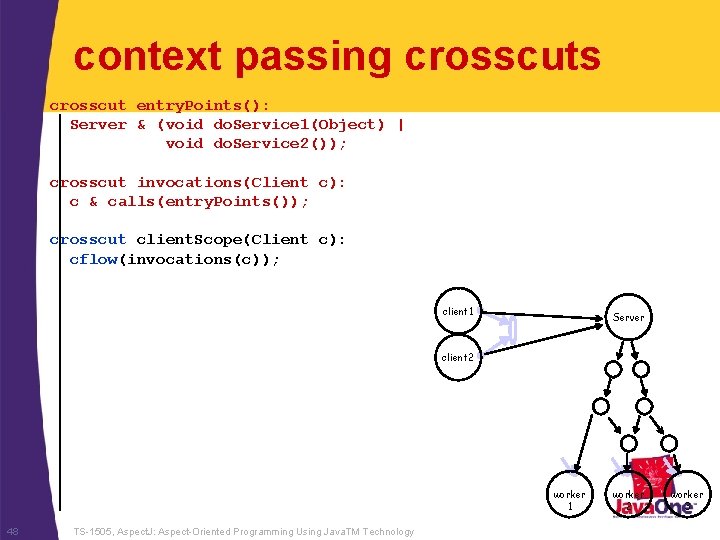
context passing crosscuts crosscut entry. Points(): Server & (void do. Service 1(Object) | void do. Service 2()); crosscut invocations(Client c): c & calls(entry. Points()); crosscut client. Scope(Client c): cflow(invocations(c)); client 1 Server client 2 worker 1 48 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
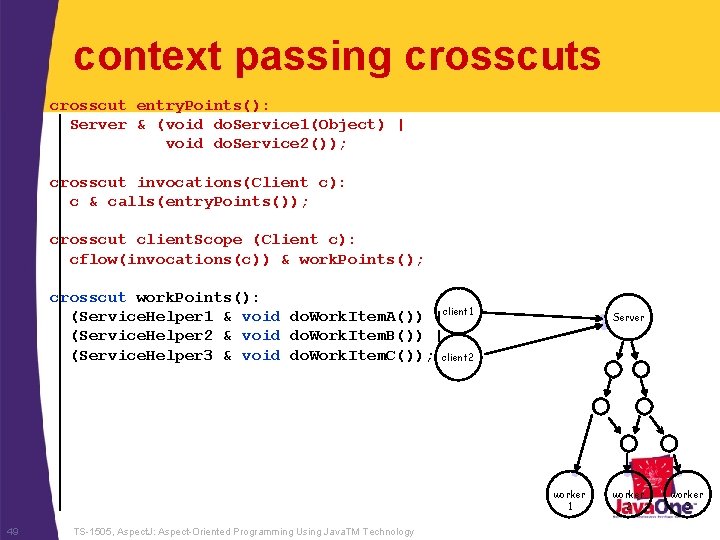
context passing crosscuts crosscut entry. Points(): Server & (void do. Service 1(Object) | void do. Service 2()); crosscut invocations(Client c): c & calls(entry. Points()); crosscut client. Scope (Client c): cflow(invocations(c)) & work. Points(); crosscut work. Points(): (Service. Helper 1 & void do. Work. Item. A()) |client 1 (Service. Helper 2 & void do. Work. Item. B()) | (Service. Helper 3 & void do. Work. Item. C()); client 2 Server worker 1 49 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology worker 2 worker 3
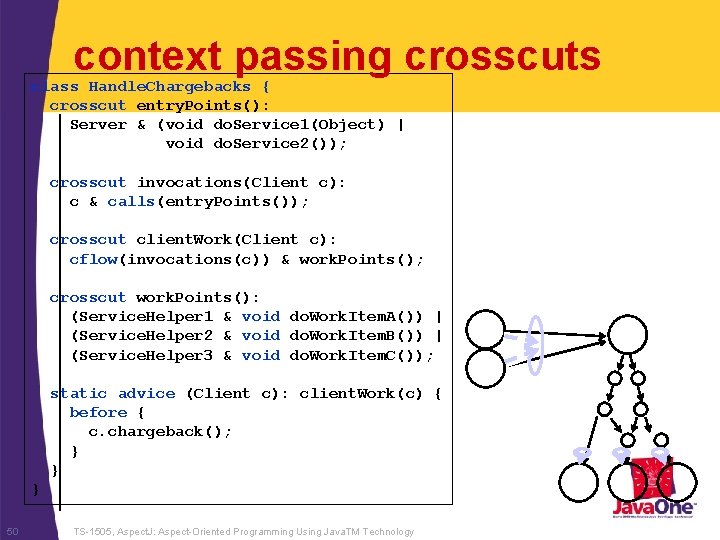
context passing crosscuts class Handle. Chargebacks { crosscut entry. Points(): Server & (void do. Service 1(Object) | void do. Service 2()); crosscut invocations(Client c): c & calls(entry. Points()); crosscut client. Work(Client c): cflow(invocations(c)) & work. Points(); crosscut work. Points(): (Service. Helper 1 & void do. Work. Item. A()) | (Service. Helper 2 & void do. Work. Item. B()) | (Service. Helper 3 & void do. Work. Item. C()); static advice (Client c): client. Work(c) { before { c. chargeback(); } } } 50 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
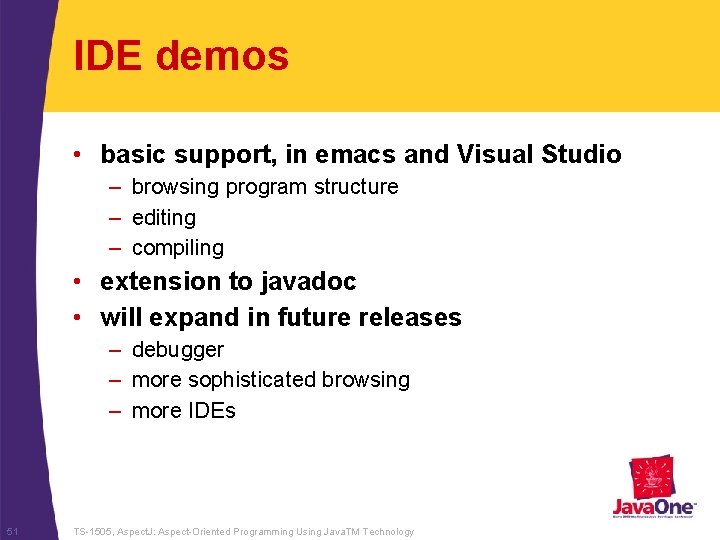
IDE demos • basic support, in emacs and Visual Studio – browsing program structure – editing – compiling • extension to javadoc • will expand in future releases – debugger – more sophisticated browsing – more IDEs 51 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
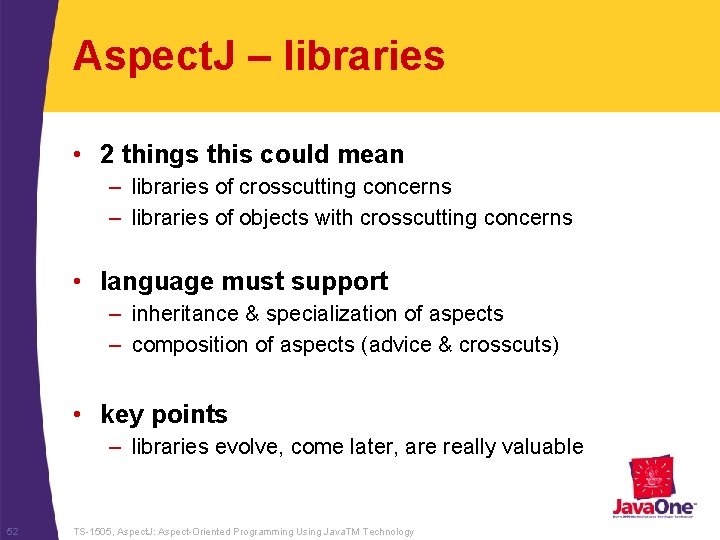
Aspect. J – libraries • 2 things this could mean – libraries of crosscutting concerns – libraries of objects with crosscutting concerns • language must support – inheritance & specialization of aspects – composition of aspects (advice & crosscuts) • key points – libraries evolve, come later, are really valuable 52 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
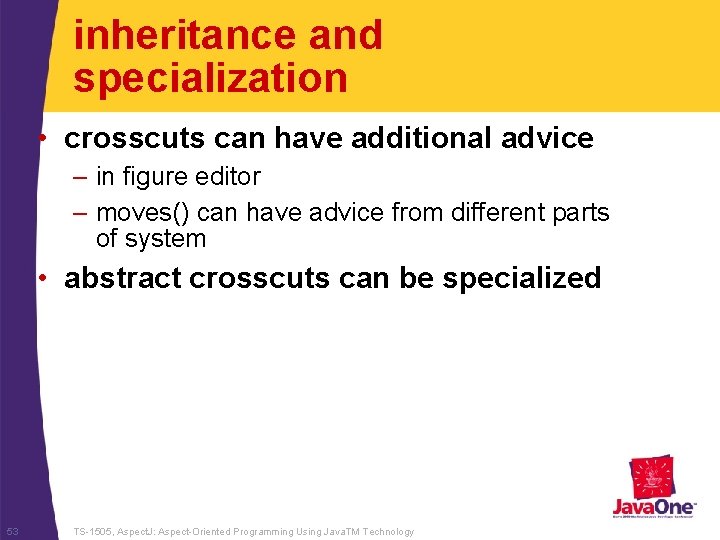
inheritance and specialization • crosscuts can have additional advice – in figure editor – moves() can have advice from different parts of system • abstract crosscuts can be specialized 53 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
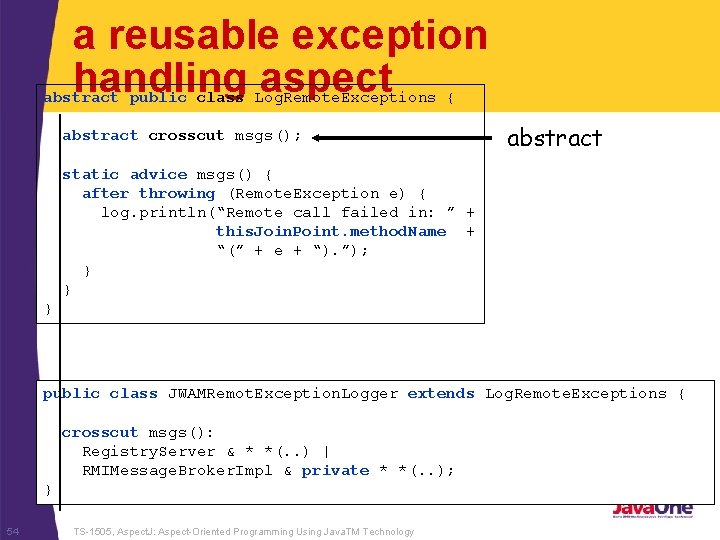
a reusable exception handling aspect abstract public class Log. Remote. Exceptions { abstract crosscut msgs(); abstract static advice msgs() { after throwing (Remote. Exception e) { log. println(“Remote call failed in: ” + this. Join. Point. method. Name + “(” + e + “). ”); } } } public class JWAMRemot. Exception. Logger extends Log. Remote. Exceptions { crosscut msgs(): Registry. Server & * *(. . ) | RMIMessage. Broker. Impl & private * *(. . ); } 54 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
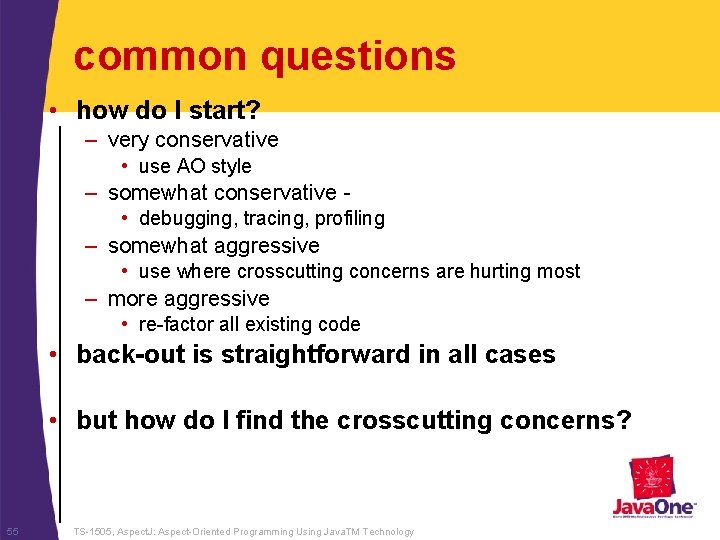
common questions • how do I start? – very conservative • use AO style – somewhat conservative • debugging, tracing, profiling – somewhat aggressive • use where crosscutting concerns are hurting most – more aggressive • re-factor all existing code • back-out is straightforward in all cases • but how do I find the crosscutting concerns? 55 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
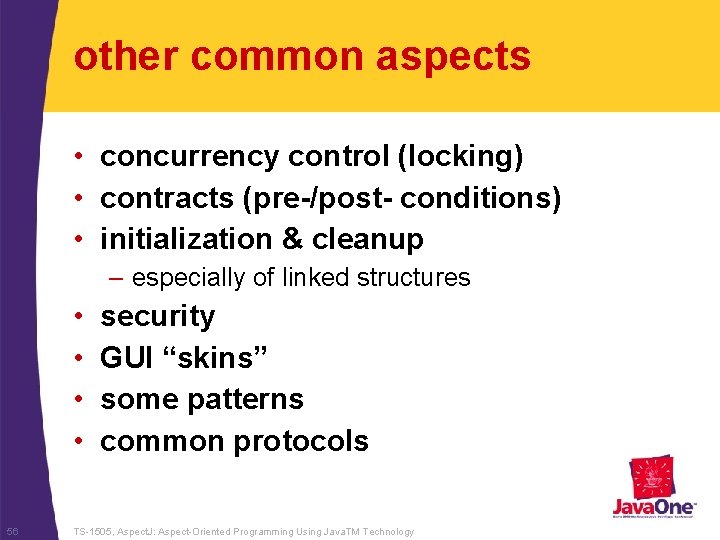
other common aspects • concurrency control (locking) • contracts (pre-/post- conditions) • initialization & cleanup – especially of linked structures • • 56 security GUI “skins” some patterns common protocols TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
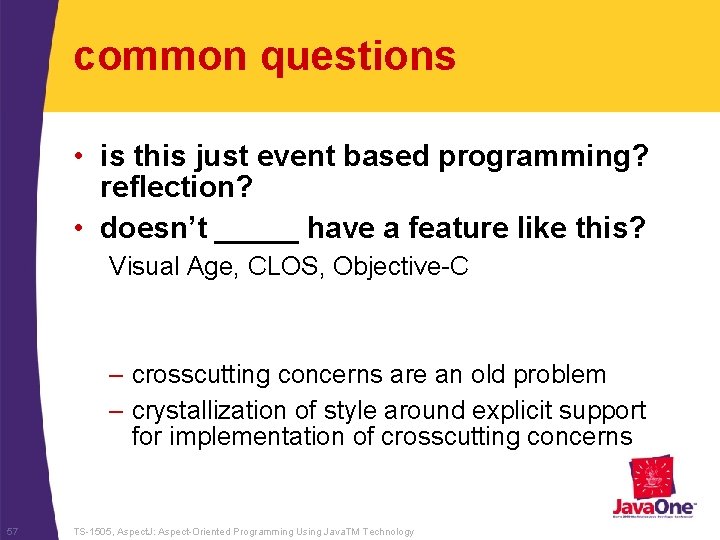
common questions • is this just event based programming? reflection? • doesn’t _____ have a feature like this? Visual Age, CLOS, Objective-C – crosscutting concerns are an old problem – crystallization of style around explicit support for implementation of crosscutting concerns 57 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
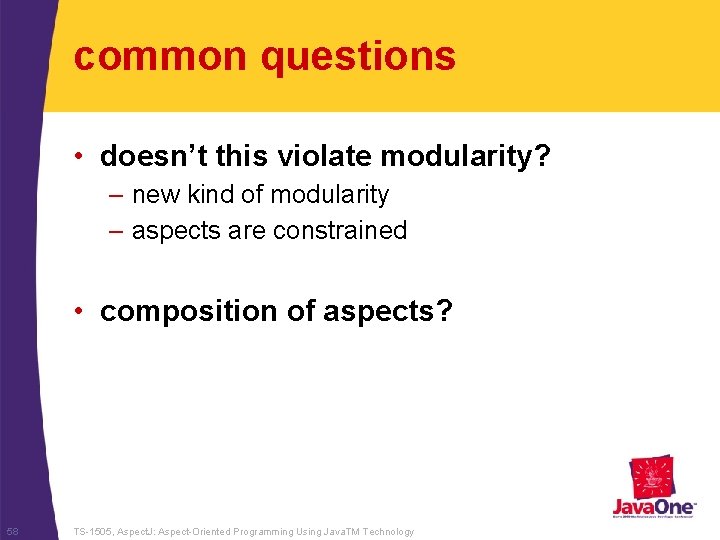
common questions • doesn’t this violate modularity? – new kind of modularity – aspects are constrained • composition of aspects? 58 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
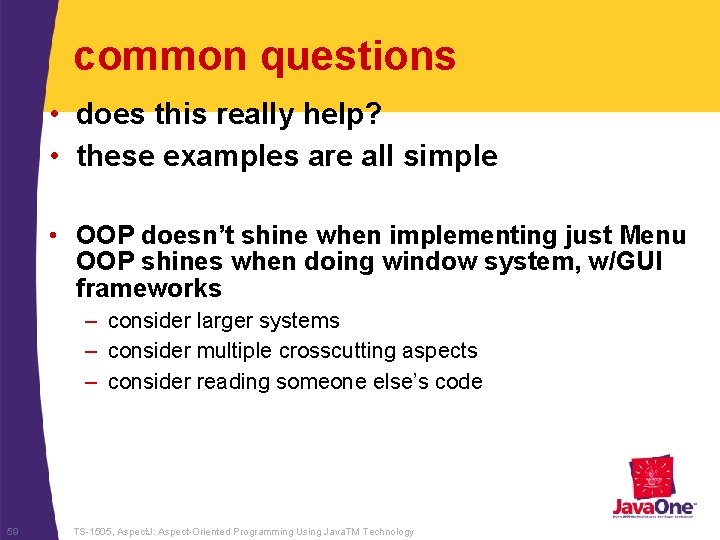
common questions • does this really help? • these examples are all simple • OOP doesn’t shine when implementing just Menu OOP shines when doing window system, w/GUI frameworks – consider larger systems – consider multiple crosscutting aspects – consider reading someone else’s code 59 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
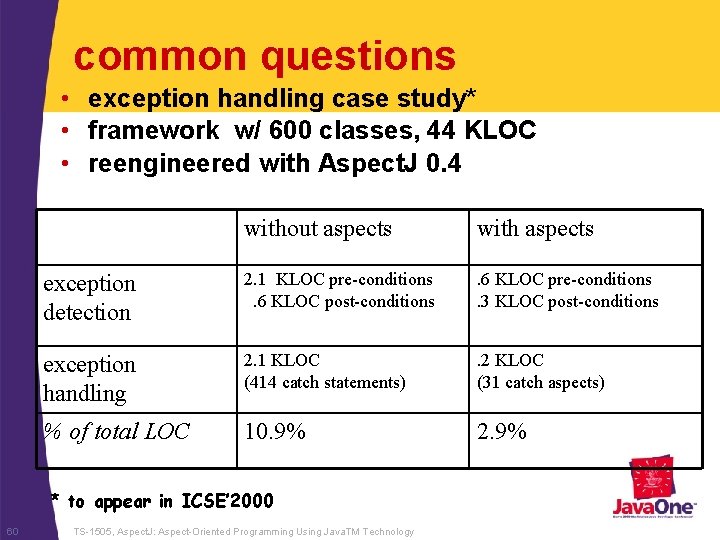
common questions • exception handling case study* • framework w/ 600 classes, 44 KLOC • reengineered with Aspect. J 0. 4 without aspects with aspects exception detection 2. 1 KLOC pre-conditions. 6 KLOC post-conditions . 6 KLOC pre-conditions. 3 KLOC post-conditions exception handling 2. 1 KLOC (414 catch statements) . 2 KLOC (31 catch aspects) % of total LOC 10. 9% 2. 9% * to appear in ICSE’ 2000 60 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
![related work in separation of crosscutting concerns Hyper J Ossher Tarr et al related work in separation of crosscutting concerns • Hyper. J [Ossher, Tarr et. al]](https://slidetodoc.com/presentation_image_h2/5ac0226281ee68062a0e0fc24872df14/image-61.jpg)
related work in separation of crosscutting concerns • Hyper. J [Ossher, Tarr et. al] – multi-dimensional separation of concerns – generator-based approach • • Composition Filters, Demeter/Java Open. C++, Open. JIT, … Intentional Programming active community… – publishes and holds workshops at: OOPSLA, ECOOP and ICSE 61 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
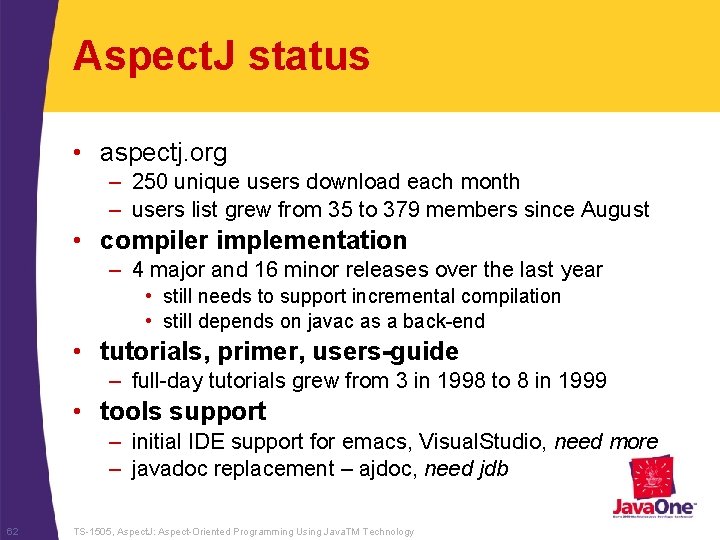
Aspect. J status • aspectj. org – 250 unique users download each month – users list grew from 35 to 379 members since August • compiler implementation – 4 major and 16 minor releases over the last year • still needs to support incremental compilation • still depends on javac as a back-end • tutorials, primer, users-guide – full-day tutorials grew from 3 in 1998 to 8 in 1999 • tools support – initial IDE support for emacs, Visual. Studio, need more – javadoc replacement – ajdoc, need jdb 62 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
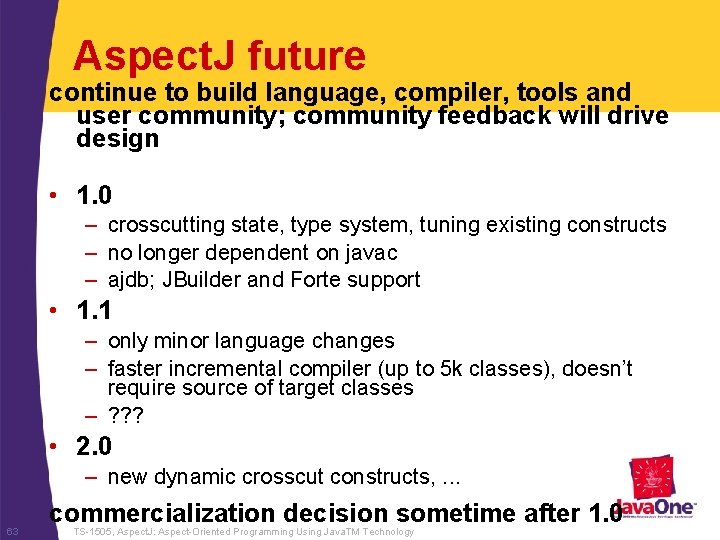
Aspect. J future continue to build language, compiler, tools and user community; community feedback will drive design • 1. 0 – crosscutting state, type system, tuning existing constructs – no longer dependent on javac – ajdb; JBuilder and Forte support • 1. 1 – only minor language changes – faster incremental compiler (up to 5 k classes), doesn’t require source of target classes – ? ? ? • 2. 0 – new dynamic crosscut constructs, . . . 63 commercialization decision sometime after 1. 0 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
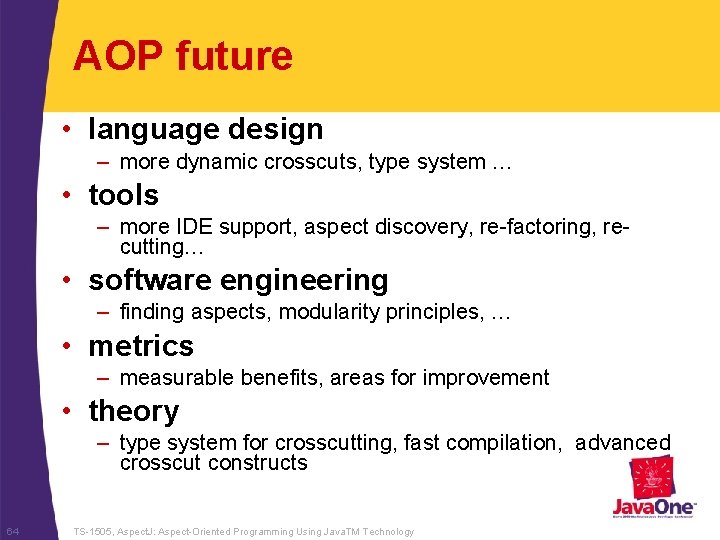
AOP future • language design – more dynamic crosscuts, type system … • tools – more IDE support, aspect discovery, re-factoring, recutting… • software engineering – finding aspects, modularity principles, … • metrics – measurable benefits, areas for improvement • theory – type system for crosscutting, fast compilation, advanced crosscut constructs 64 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
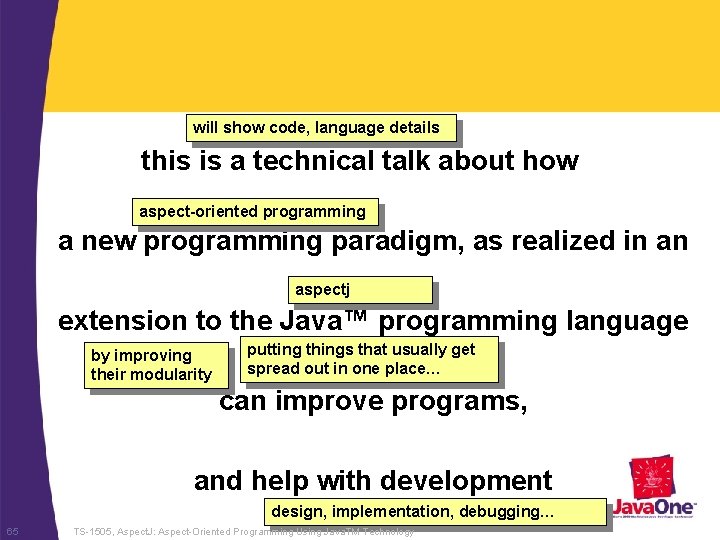
will show code, language details this is a technical talk about how aspect-oriented programming a new programming paradigm, as realized in an aspectj extension to the Java™ programming language by improving their modularity putting things that usually get spread out in one place… can improve programs, and help with development design, implementation, debugging… 65 TS-1505, Aspect. J: Aspect-Oriented Programming Using Java. TM Technology
Aspect et sous aspect
Sesslah
Aspect java
Tom janofsky
Java aspect
Sentihood
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
What is system programming
Linear vs integer programming
Definisi linear
Socket programming tcp udp
Java parallel programming
Java refresher exercises
Problem solving
Java event driven programming example
Elementary programming in java
Java asynchronous programming
Java structured programming
Arne kutzner
R programming khan academy
Event driven programming in java
Defensive programming java
Java refresher course
Java games programming
Java code symbols
Java an introduction to problem solving and programming
Elementary programming in java
Java database programming
Java asynchronous programming
Conclusion of java
Java programming
Elementary programming in java
Programming in java
Advanced programming in java
Java programming language
Java enterprise architecture
Programming from the ground up
Java introduction to problem solving and programming
Introduction to java programming 10th edition quizzes
Interrupt programming in 8051
Binomial coefficient using dynamic programming
Solving goal programming problems using simplex method
Apprenticeship learning using linear programming
Who is gregor mendel and what is he famous for
Section 11–1 the work of gregor mendel
Gregor mohorcic
Gregor mendel plant
Who was gregor mendel
Mendel monk
Rryy x rryy
Wikimpace
Gregor mendal
Gregor mendel chart
Chapter 12 lesson 1 the work of gregor mendel
Who is gregor mendel
Gregor gabriel
What did gregor mendel research
Y chromosome traits
What did gregor mendel do
Gregor mendel conclusion
Chapter 12 lesson 1 the work of gregor mendel
Gregor kiczales ubc
Gregor mendel’s principles of genetics apply to
Apoliena rozprávač
Gregor guron