1 Fig 3 3 fig 0303 cpp 2
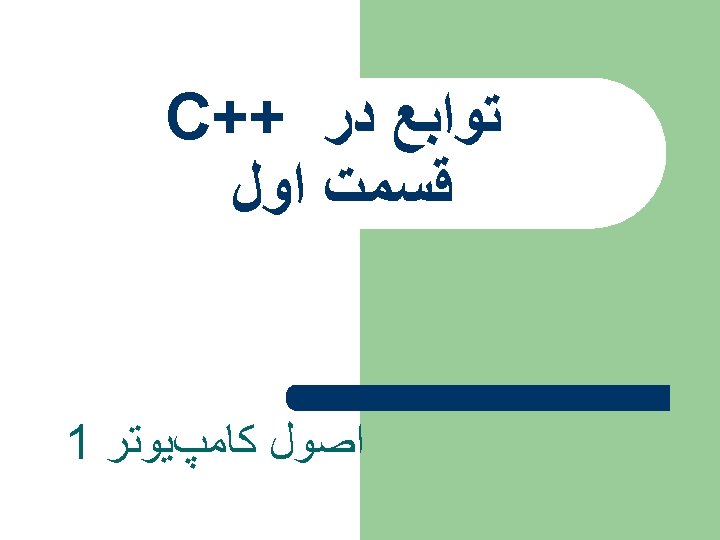
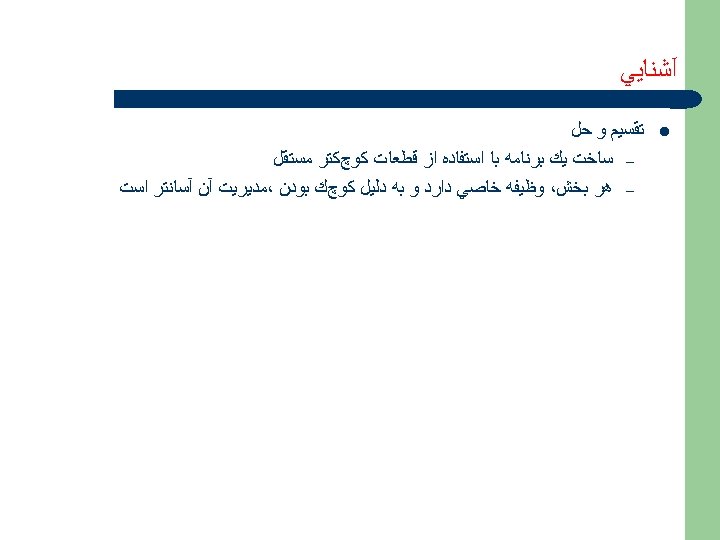
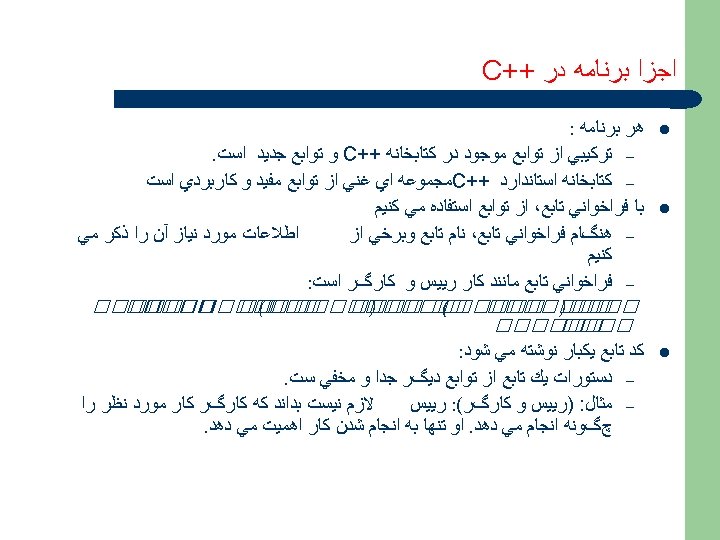
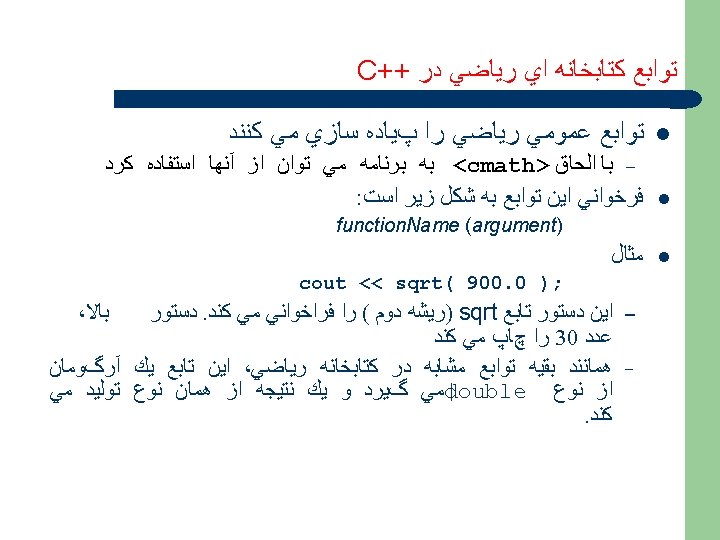
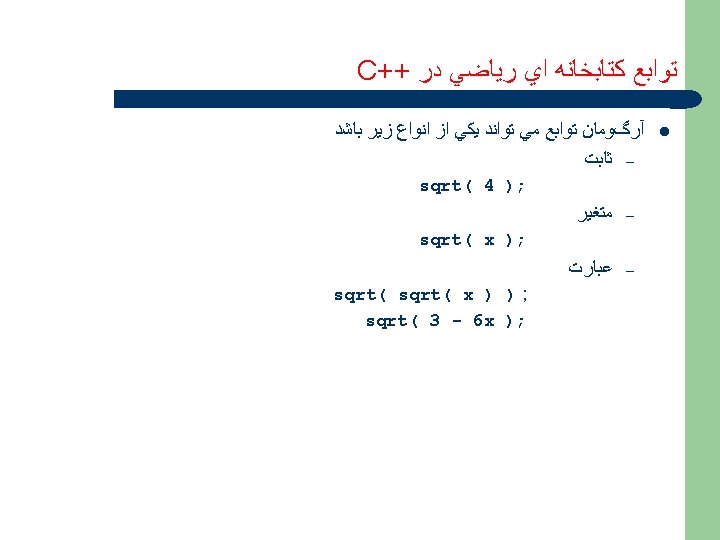
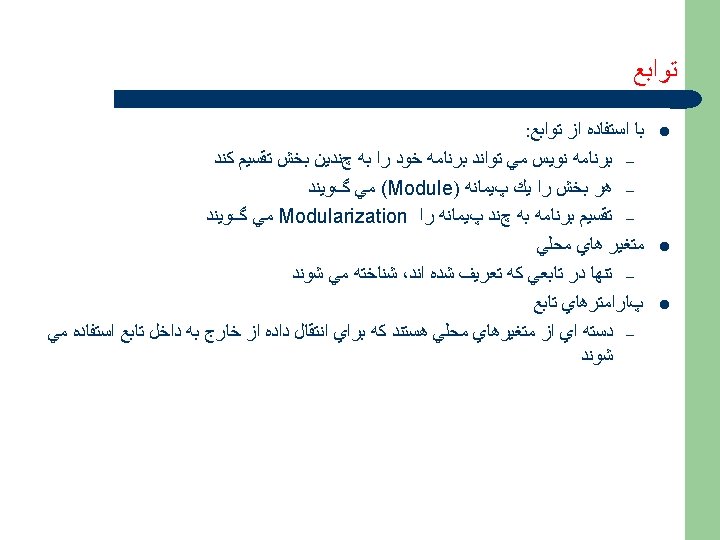
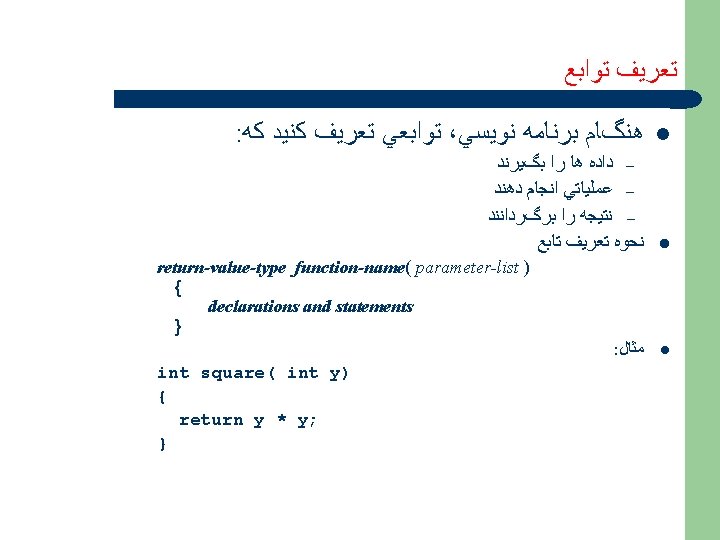
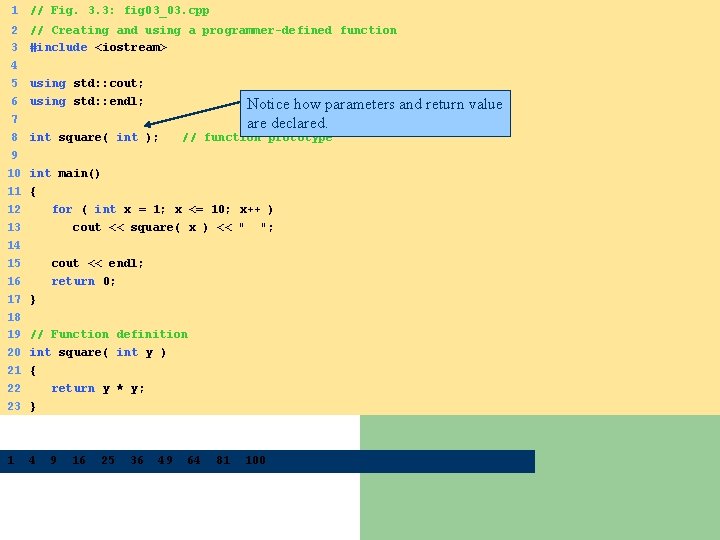
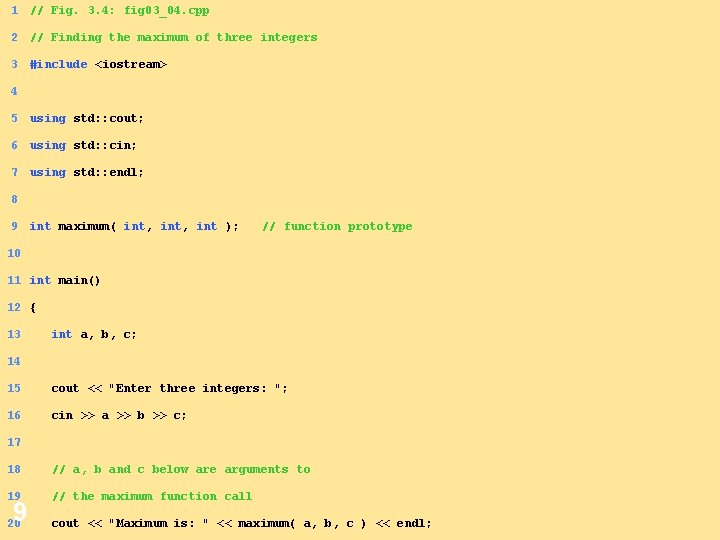
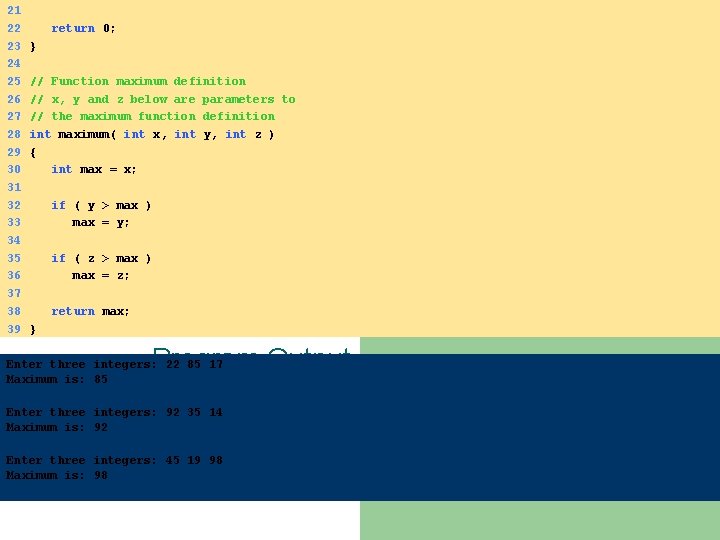
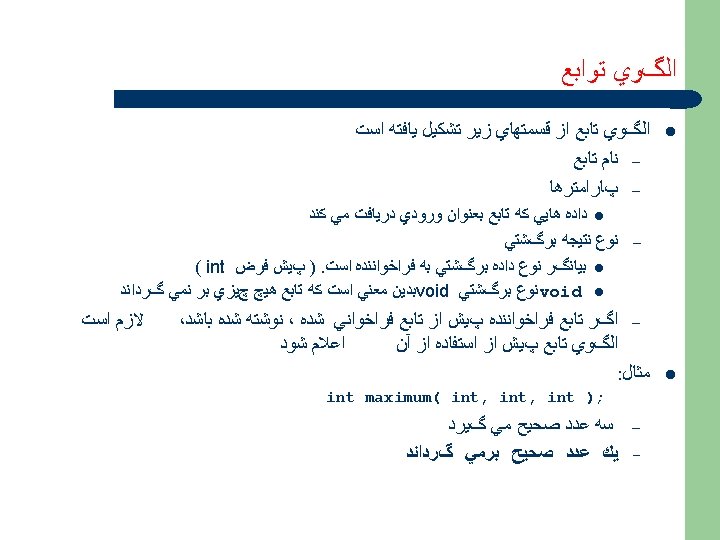
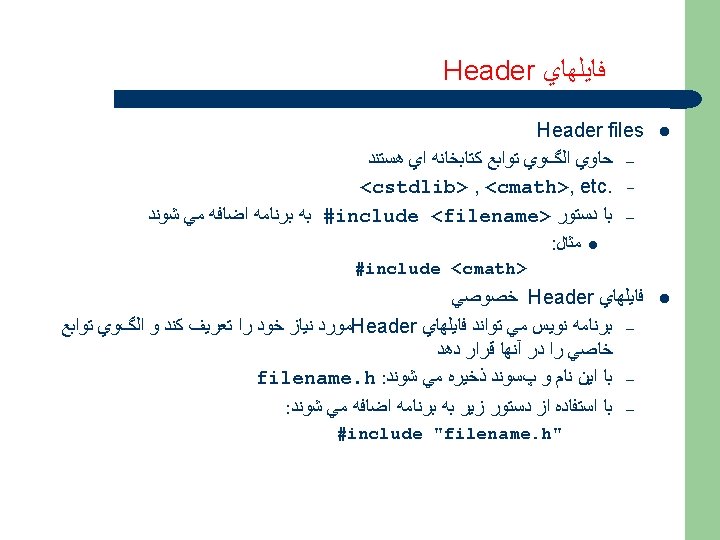
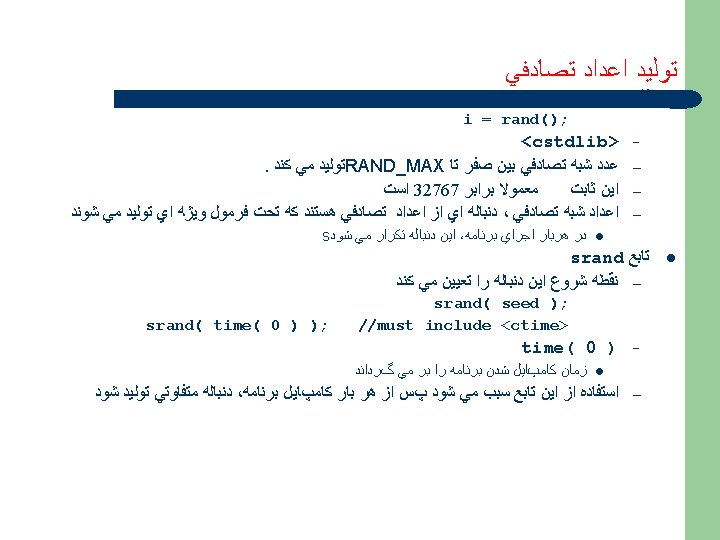
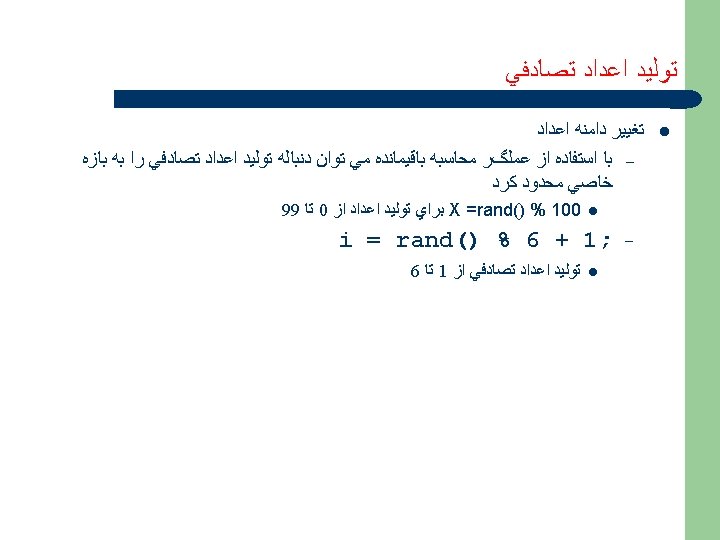
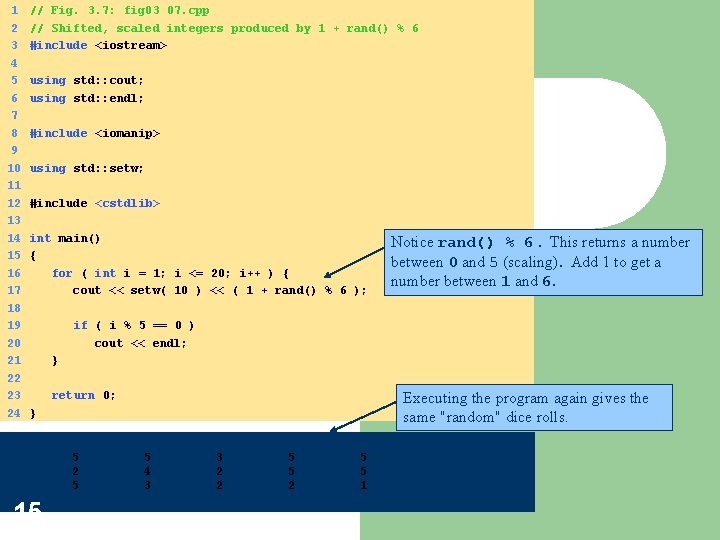
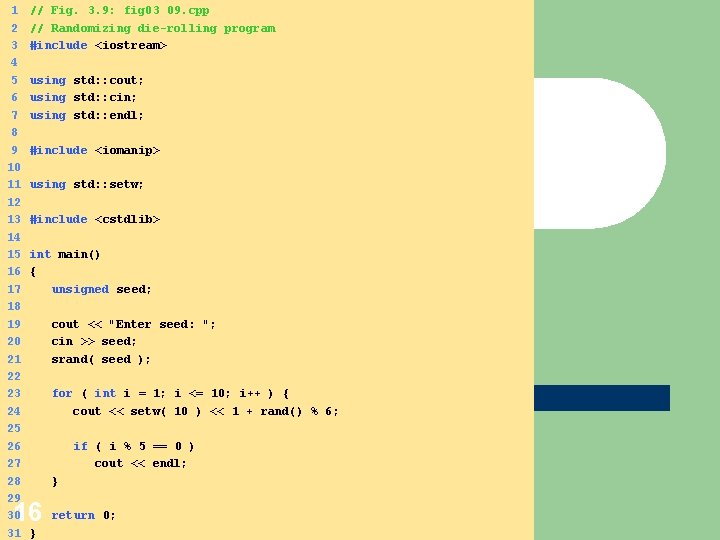
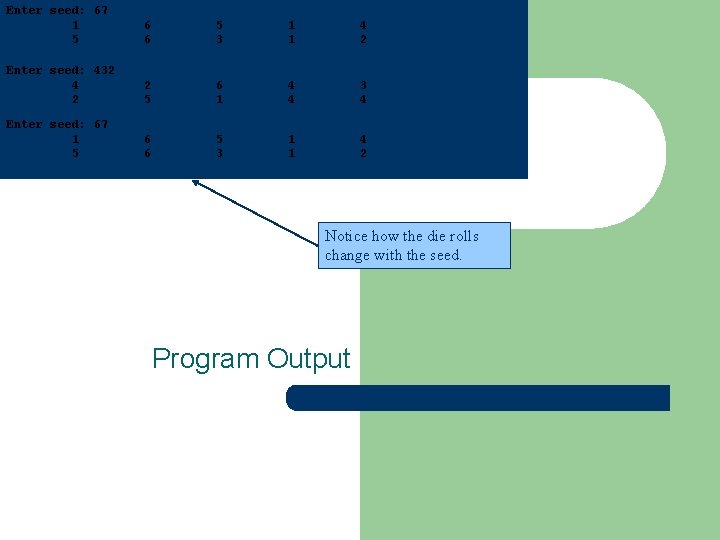
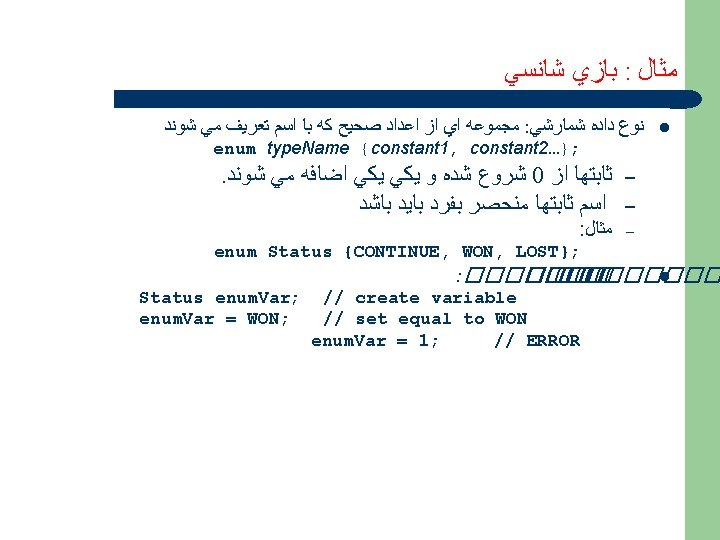
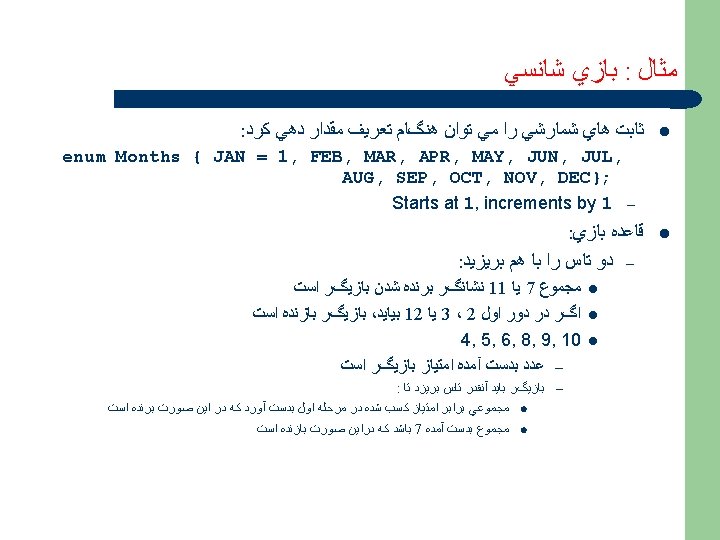
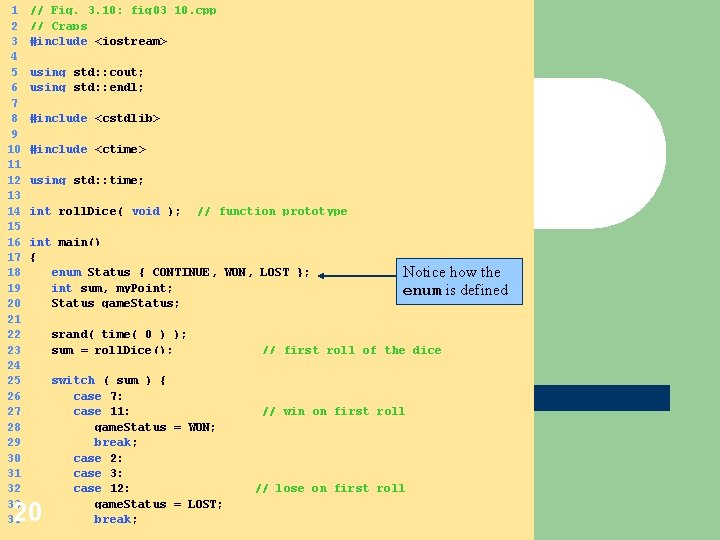
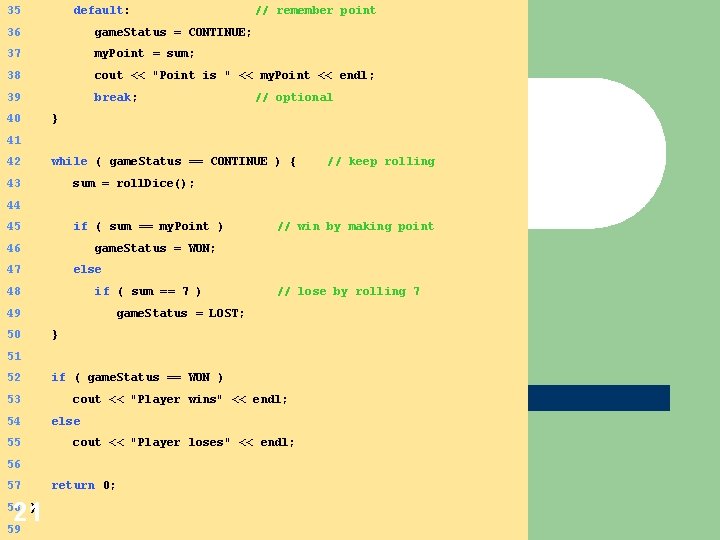
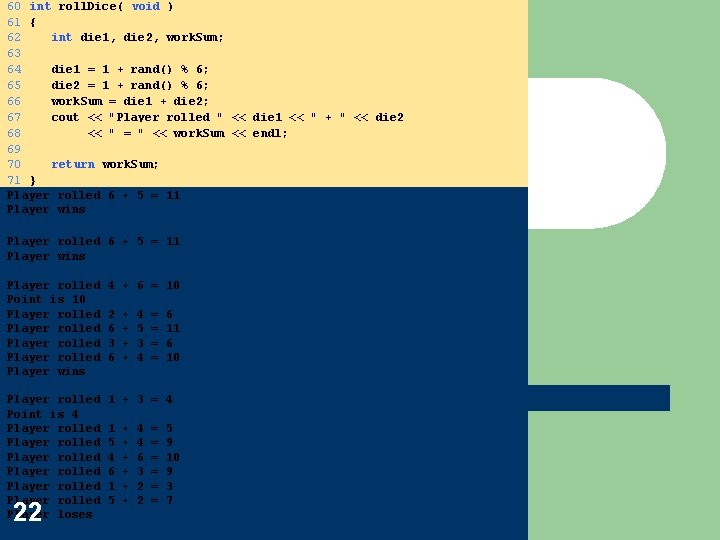
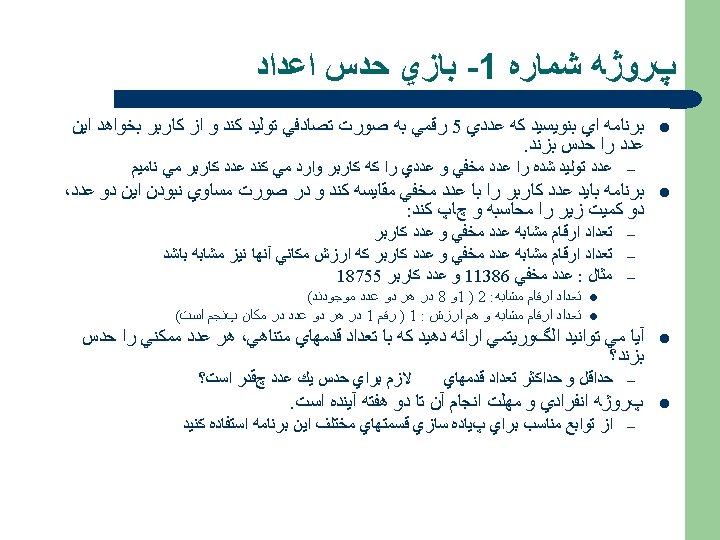
- Slides: 23
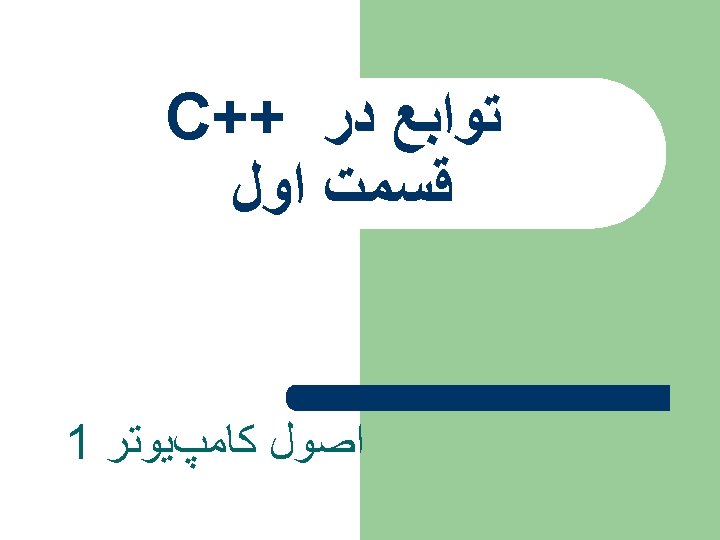
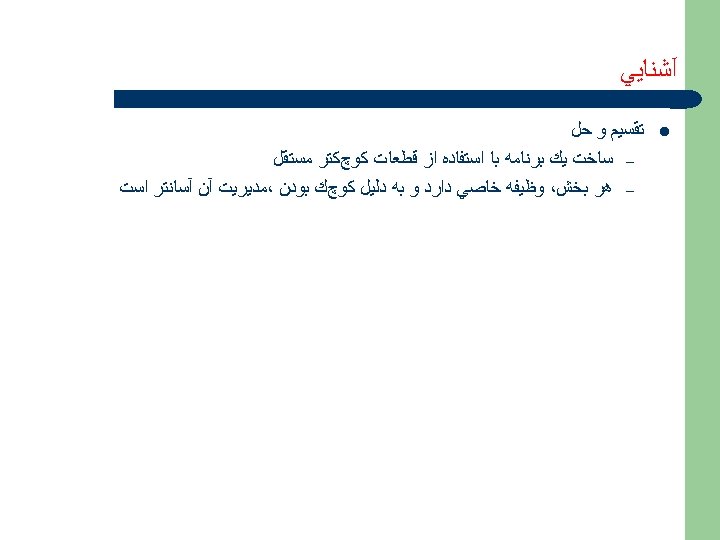
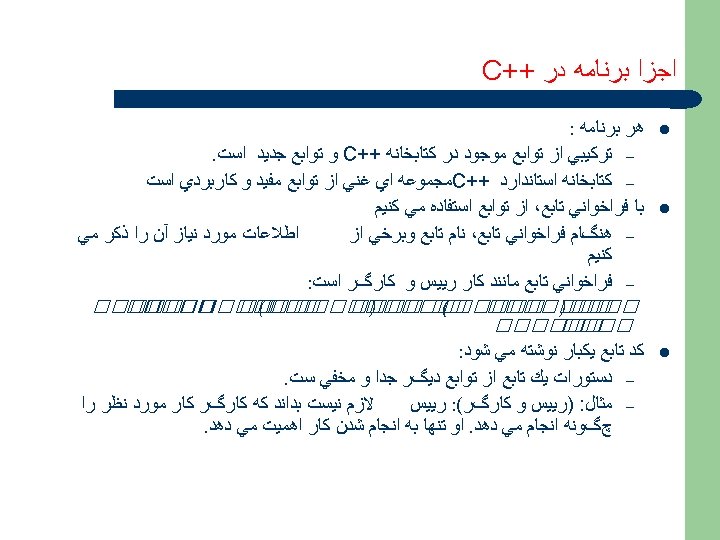
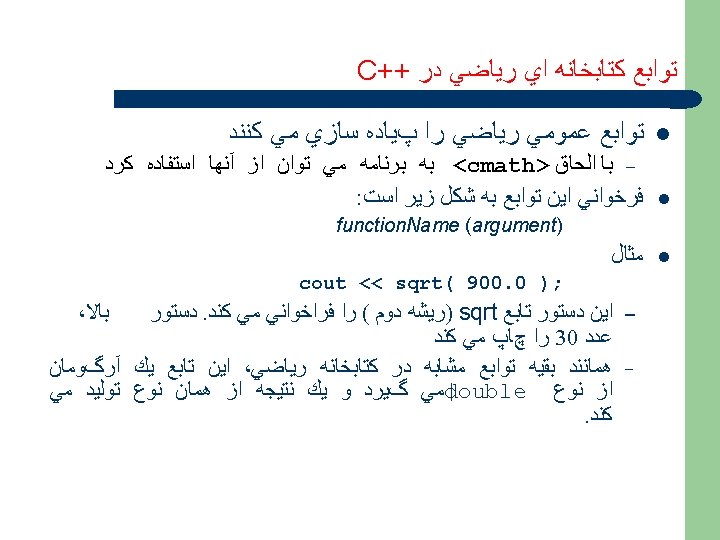
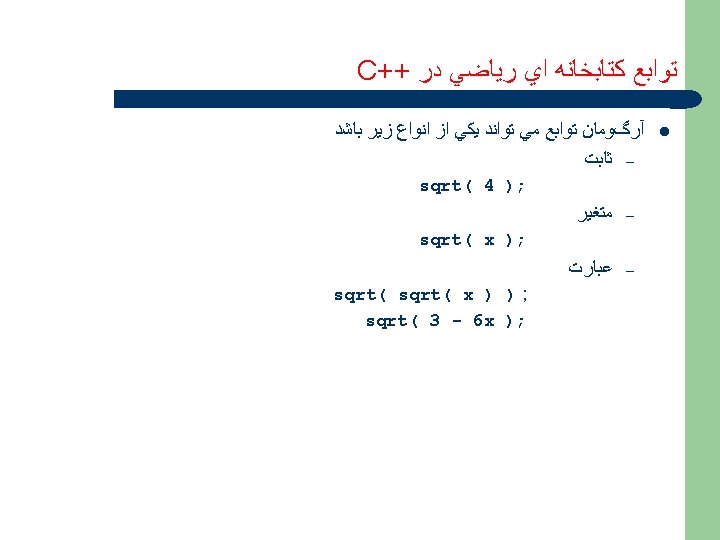
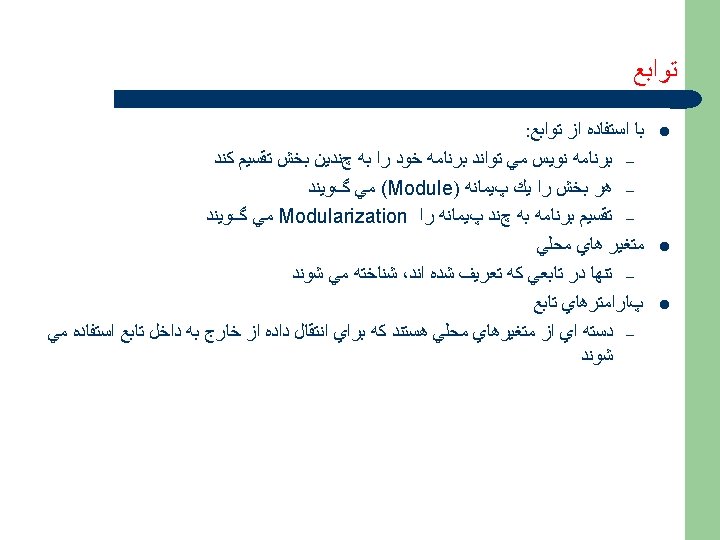
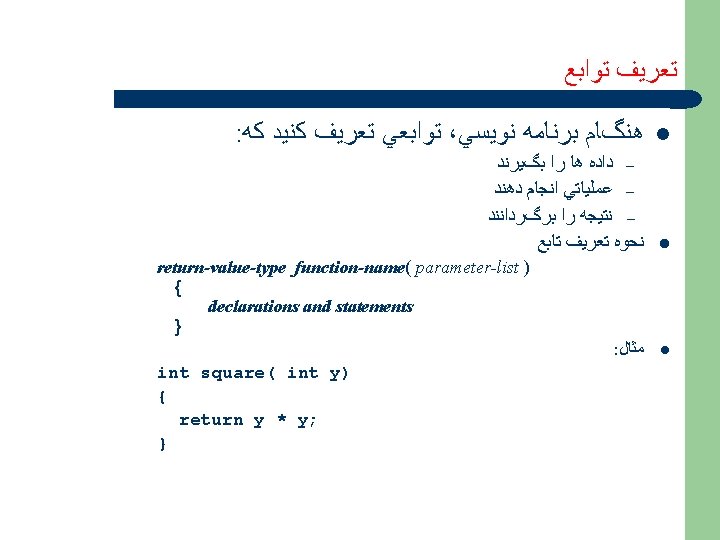
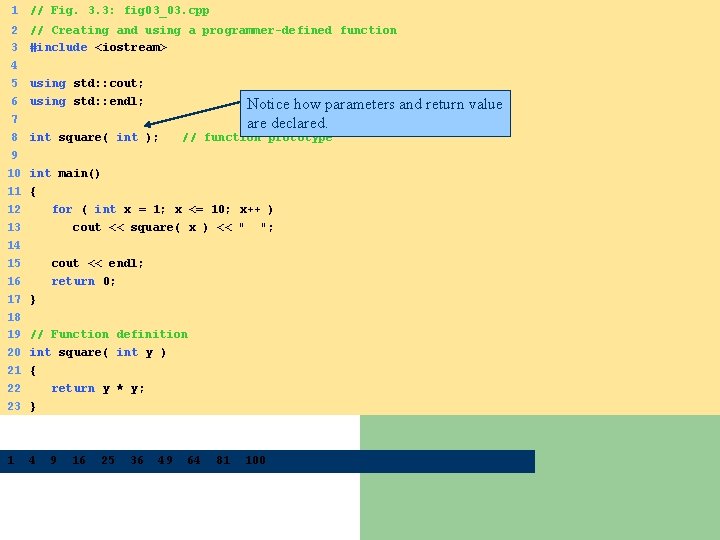
1 // Fig. 3. 3: fig 03_03. cpp 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 // Creating and using a programmer-defined function #include <iostream> 1 4 8 using std: : cout; using std: : endl; int square( int ); Notice how parameters and return value are declared. // function prototype int main() { for ( int x = 1; x <= 10; x++ ) cout << square( x ) << " "; cout << endl; return 0; } // Function definition int square( int y ) { return y * y; } Program Output 9 16 25 36 49 64 81 100
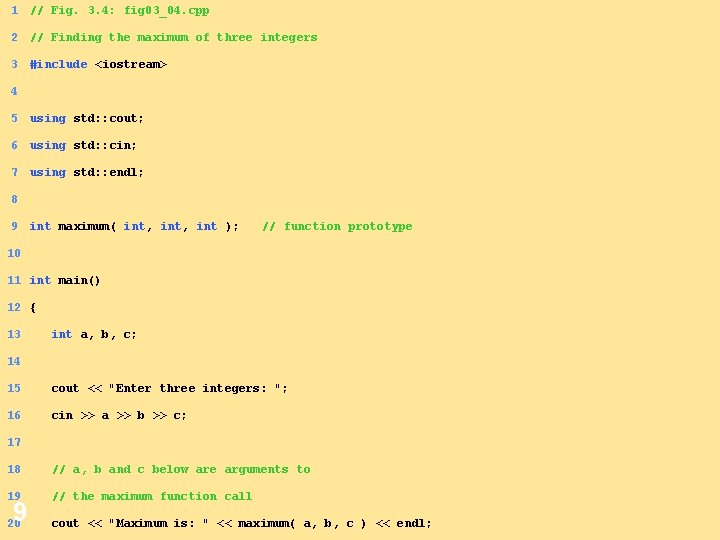
1 // Fig. 3. 4: fig 03_04. cpp 2 // Finding the maximum of three integers 3 #include <iostream> 5 1. Function prototype (3 using std: : cout; parameters) 6 using std: : cin; 7 using std: : endl; 4 2. Input values 8 9 int maximum( int, int ); // function prototype 10 11 int main() 2. 1 Call function 12 { 13 int a, b, c; 14 15 cout << "Enter three integers: "; 16 cin >> a >> b >> c; 17 18 // a, b and c below are arguments to 19 // the maximum function call 20 cout << "Maximum is: " << maximum( a, b, c ) << endl; 9
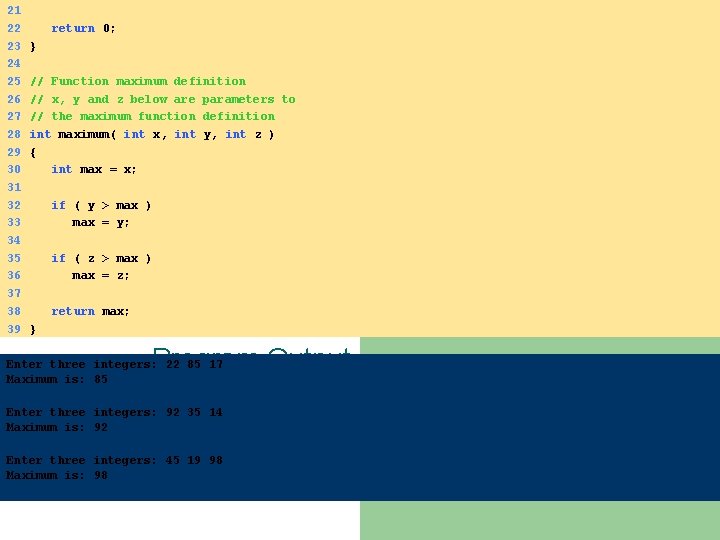
21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 return 0; } // Function maximum definition // x, y and z below are parameters to // the maximum function definition int maximum( int x, int y, int z ) { int max = x; if ( y > max ) max = y; if ( z > max ) max = z; return max; } Program Output Enter three integers: 22 85 17 Maximum is: 85 Enter three integers: 92 35 14 Maximum is: 92 Enter three integers: 45 19 98 Maximum is: 98 10
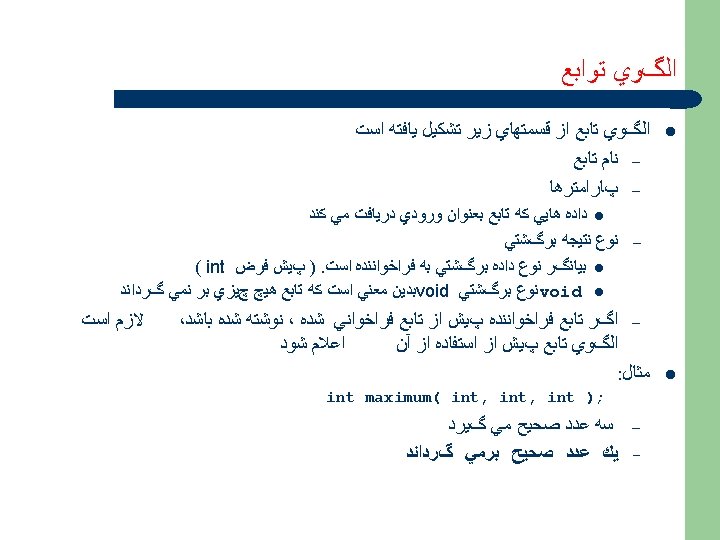
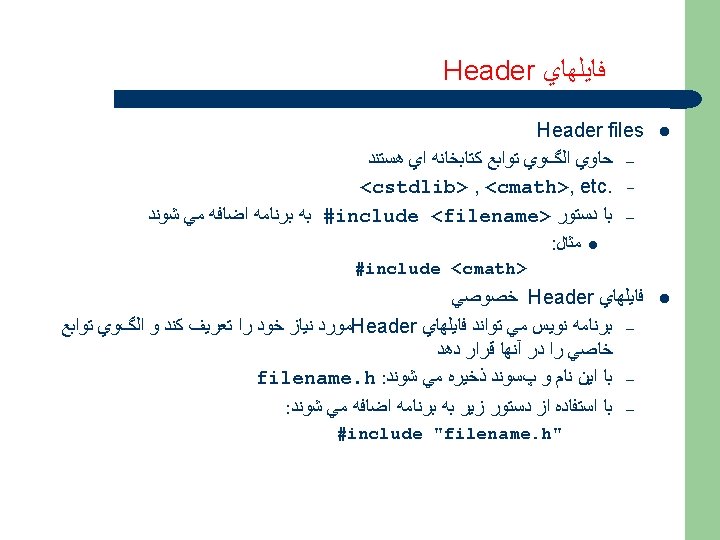
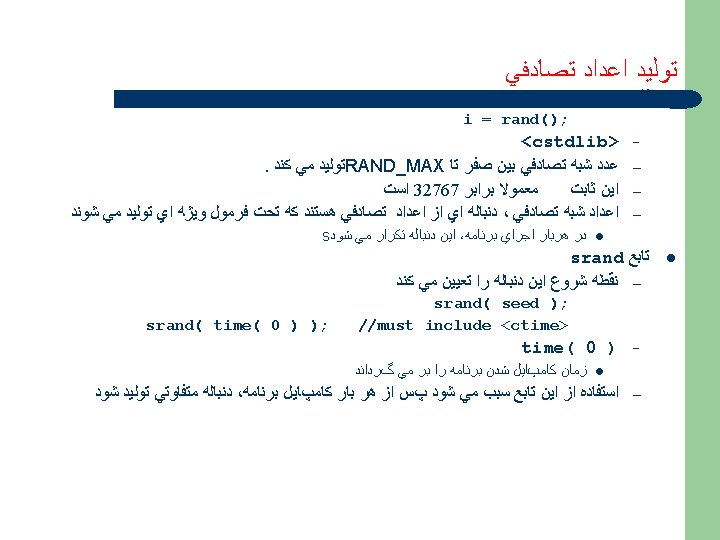
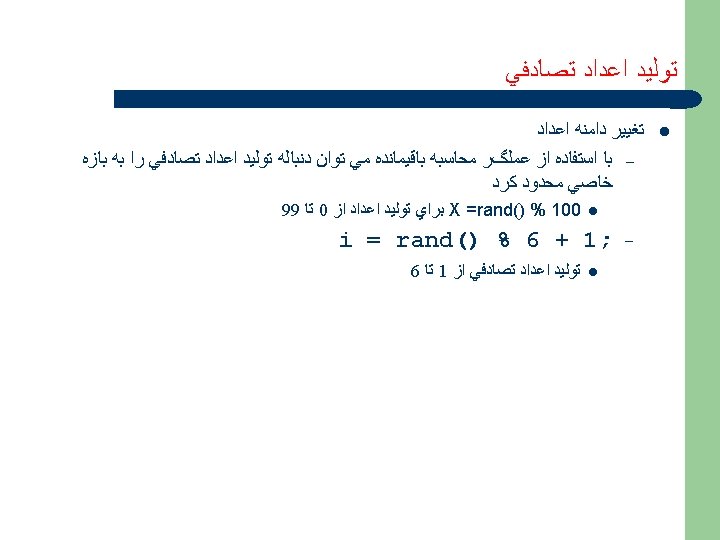
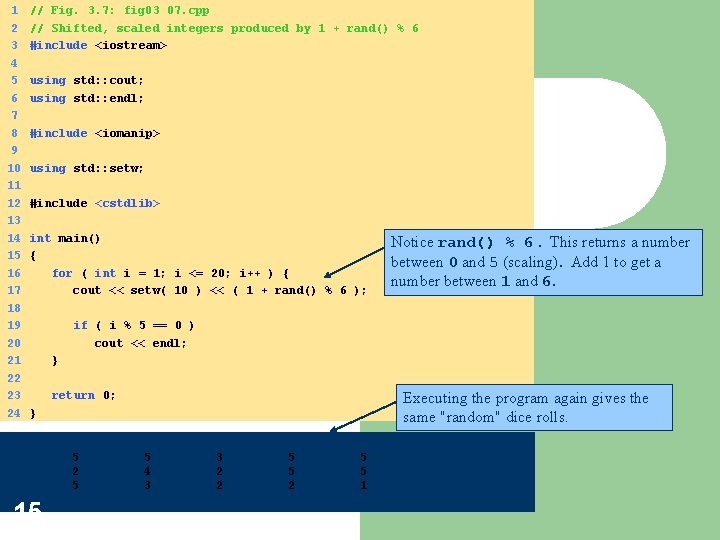
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 // Fig. 3. 7: fig 03_07. cpp // Shifted, scaled integers produced by 1 + rand() % 6 #include <iostream> using std: : cout; using std: : endl; #include <iomanip> using std: : setw; #include <cstdlib> int main() { for ( int i = 1; i <= 20; i++ ) { cout << setw( 10 ) << ( 1 + rand() % 6 ); Program Output. 3 Notice rand() % 6. This returns a number between 0 and 5 (scaling). Add 1 to get a number between 1 and 6. if ( i % 5 == 0 ) cout << endl; } Executing the program again gives the same "random" dice rolls. return 0; } 15 5 2 5 5 5 4 3 1 3 2 2 4 5 5 2 6 5 5 1 4
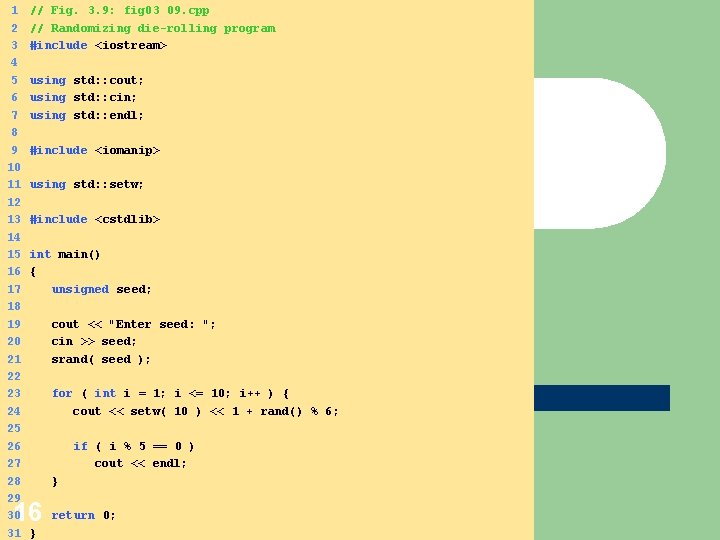
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 2. Input value for seed // Fig. 3. 9: fig 03_09. cpp // Randomizing die-rolling program #include <iostream> using std: : cout; using std: : cin; using std: : endl; 2. 1 Use srand to change random #include <iomanip> sequence using std: : setw; #include <cstdlib> 2. 2 Define Loop int main() { unsigned seed; cout << "Enter seed: "; 3. Generate and output cin >> seed; srand( seed ); random numbers for ( int i = 1; i <= 10; i++ ) { cout << setw( 10 ) << 1 + rand() % 6; if ( i % 5 == 0 ) cout << endl; } 16 } return 0;
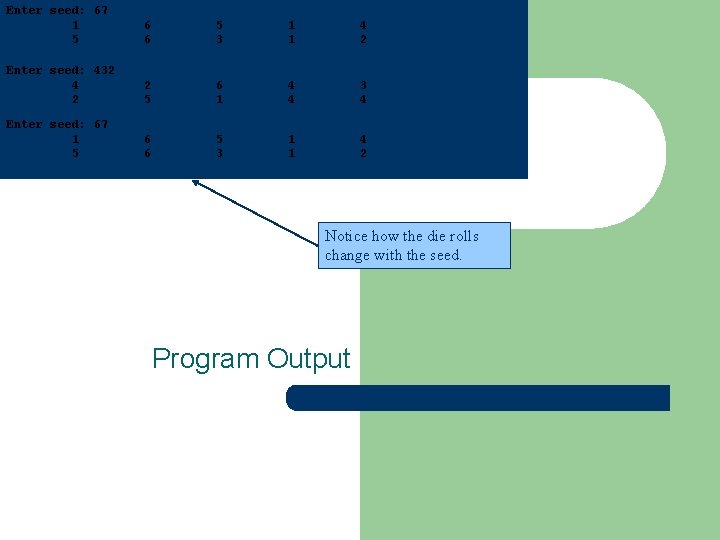
Enter seed: 67 1 5 6 6 5 3 1 1 4 2 Enter seed: 432 4 2 2 5 6 1 4 4 3 4 Enter seed: 67 1 5 6 6 5 3 1 1 4 2 Notice how the die rolls change with the seed. Program Output 17
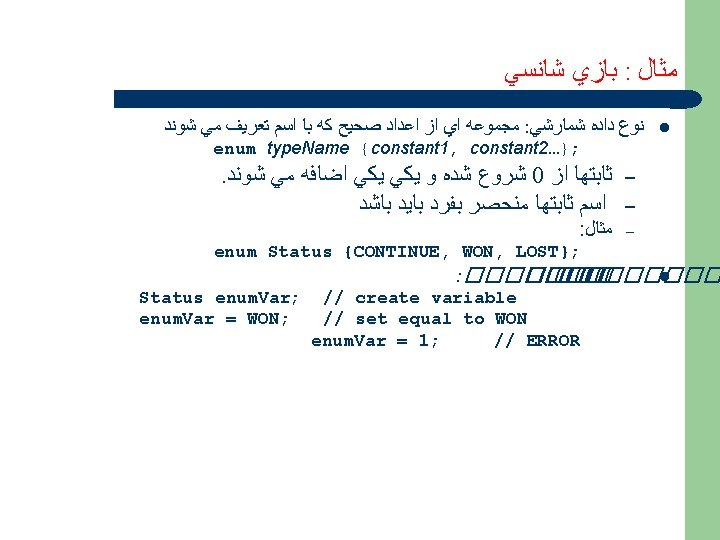
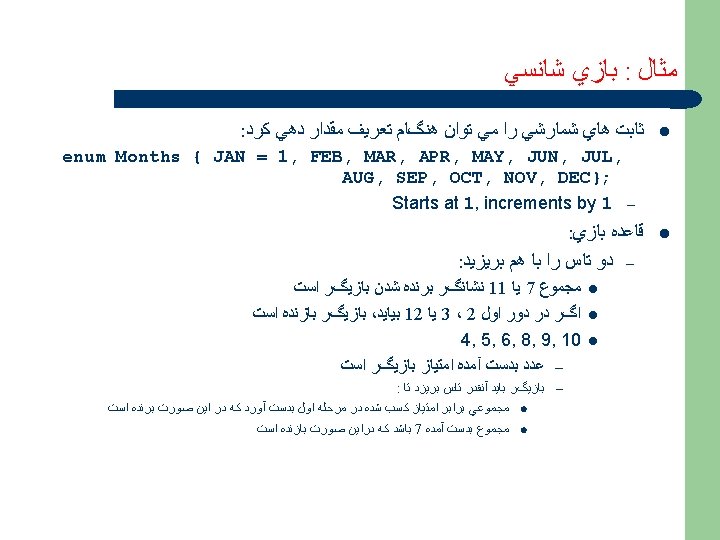
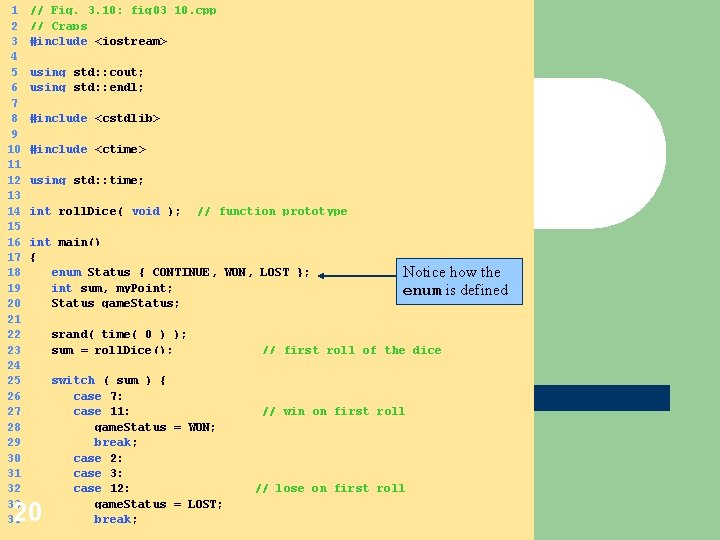
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 // Fig. 3. 10: fig 03_10. cpp // Craps #include <iostream> 1. 1 Initialize variables and enum using std: : cout; using std: : endl; #include <cstdlib> #include <ctime> 1. 2 Seed srand using std: : time; int roll. Dice( void ); // function prototype 2. Define switch statement for win/loss/continue srand( time( 0 ) ); int main() { enum Status { CONTINUE, WON, LOST }; int sum, my. Point; Status game. Status; 20 sum = roll. Dice(); switch ( sum ) { case 7: case 11: game. Status = WON; break; case 2: case 3: case 12: game. Status = LOST; break; Notice how the enum is defined // first roll of the dice // win on first roll // lose on first roll
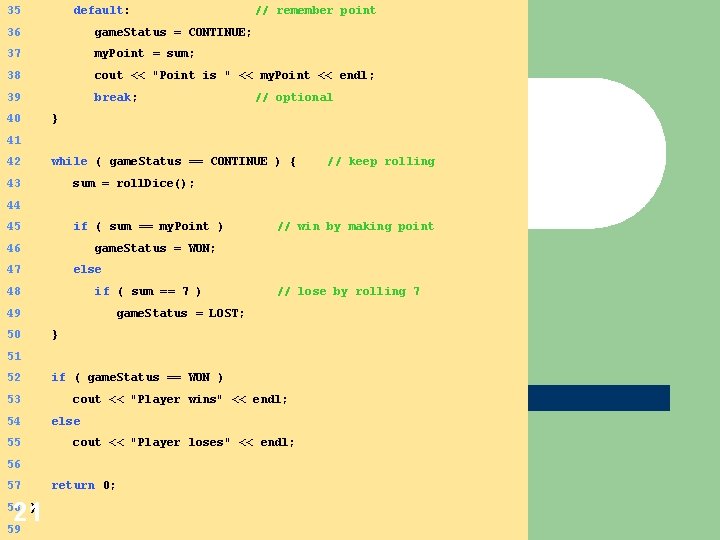
35 default: // remember point 36 game. Status = CONTINUE; 37 my. Point = sum; 38 cout << "Point is " << my. Point << endl; 39 break; 40 // optional } 41 44 // keep rolling 2. 1 Define loop to sum = roll. Dice(); continue playing 45 if ( sum == my. Point ) 42 while ( game. Status == CONTINUE ) { 43 46 game. Status = WON; 47 2. 2 Print win/loss // lose by rolling 7 else 48 if ( sum == 7 ) 49 50 // win by making point game. Status = LOST; } 51 52 53 54 55 if ( game. Status == WON ) cout << "Player wins" << endl; else cout << "Player loses" << endl; 56 57 21 58 } 59 return 0;
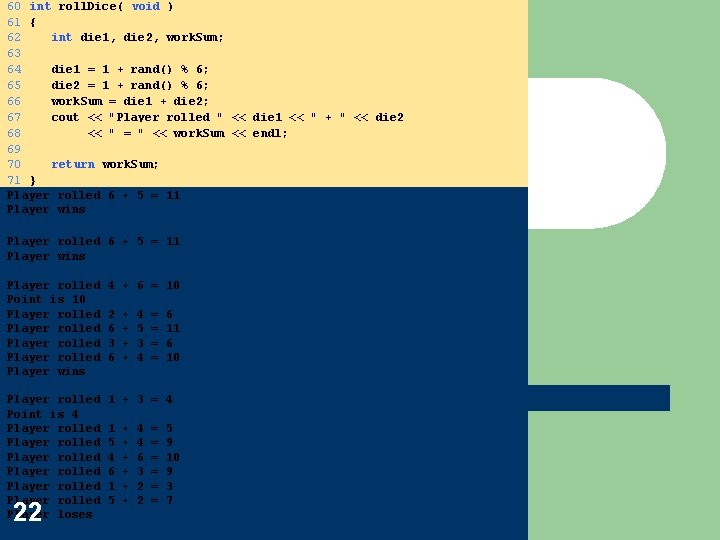
60 int roll. Dice( void ) 61 { 62 int die 1, die 2, work. Sum; 63 64 die 1 = 1 + rand() % 6; 65 die 2 = 1 + rand() % 6; 66 work. Sum = die 1 + die 2; 67 cout << "Player rolled " << die 1 << " + " << die 2 68 << " = " << work. Sum << endl; 69 70 return work. Sum; 71 } Player rolled 6 + 5 = 11 Player wins 3. Define roll. Dice function Player rolled 6 + 5 = 11 Player wins Player rolled Point is 10 Player rolled Player wins 4 + 6 = 10 Player rolled Point is 4 Player rolled Player rolled Player loses 1 + 3 = 4 22 2 6 3 6 1 5 4 6 1 5 + + + + + 4 5 3 4 4 4 6 3 2 2 = = 6 11 6 10 Program Output = = = 5 9 10 9 3 7
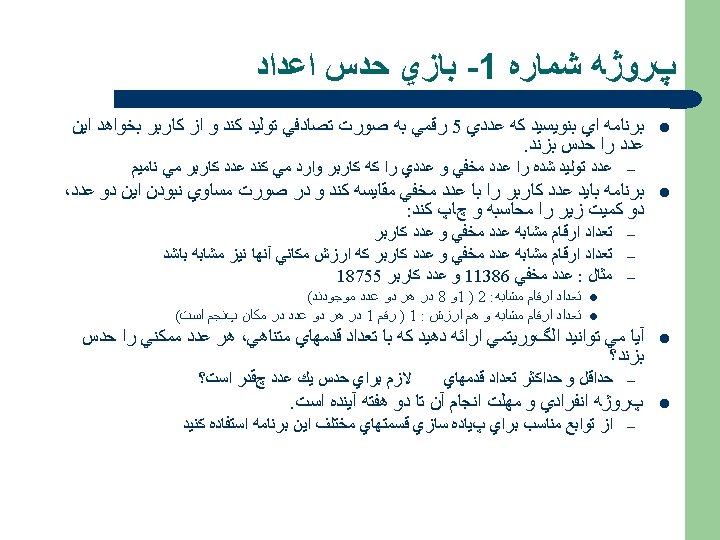
03033033
Fig.2
Cpp formula
Radix sort cpp
Art 639 cpp
Cpp depend
Reformatio in pejus
Cpp program
Maximum cpp benefit 2021
Gabriel kuri cpp
Suprahumeral joint
Extranet cpp
Cpp security certification
Cpp cummins
Cpp program
Kolesarski izpit simulacija
Cpp triangle of explanation
Perizia cpp
Ess portal aai.aero
Cpp certification procurement
Unix cpp sys wipro
Bme c++
Perdão e renuncia
Cpp gpa calculator