Todays topics Parsing Java Programming Reading Great Ideas
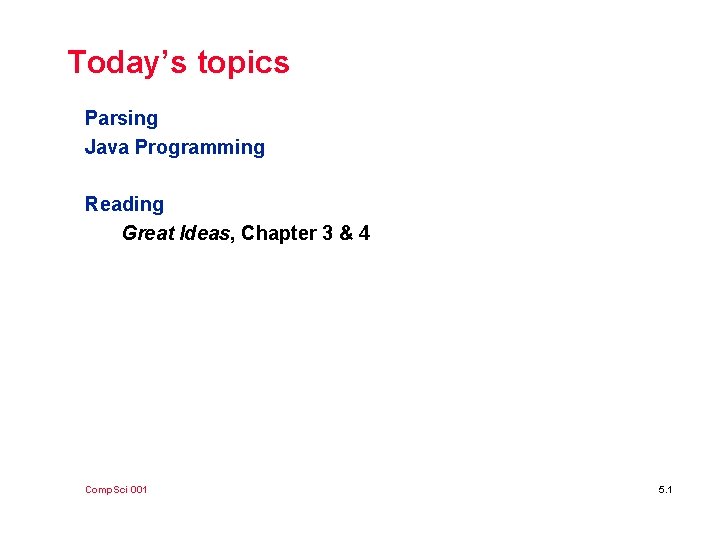
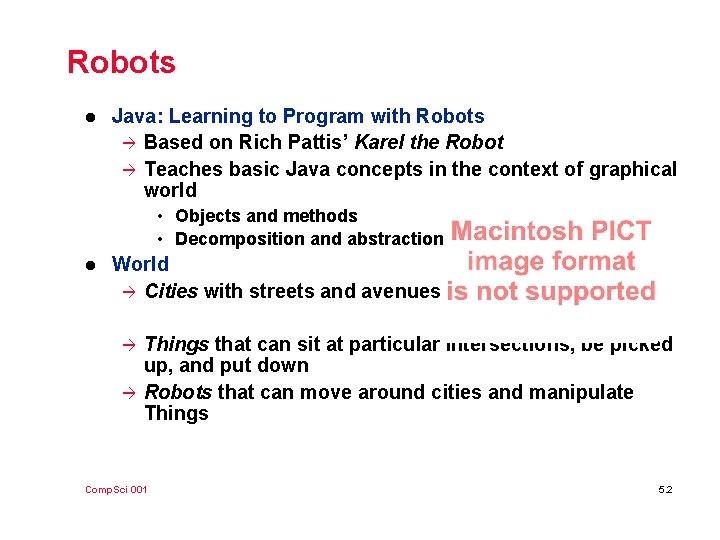
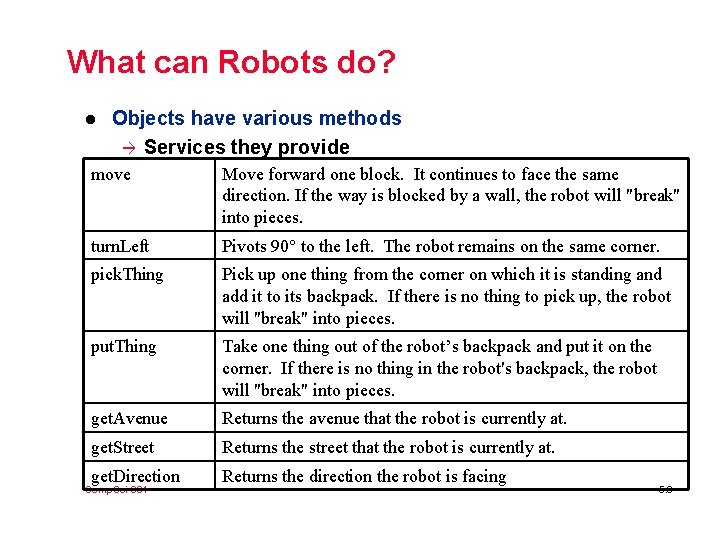
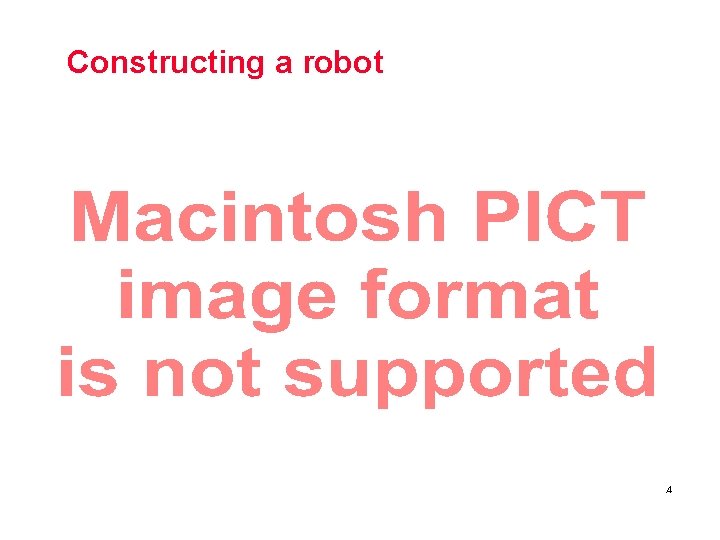
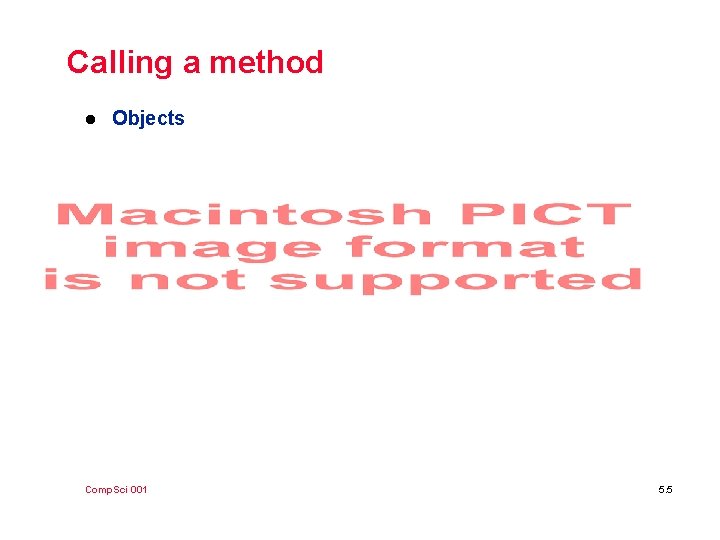
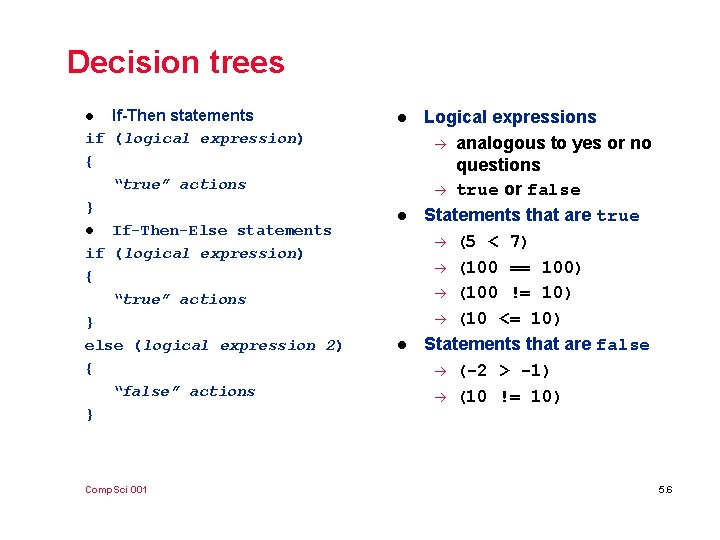
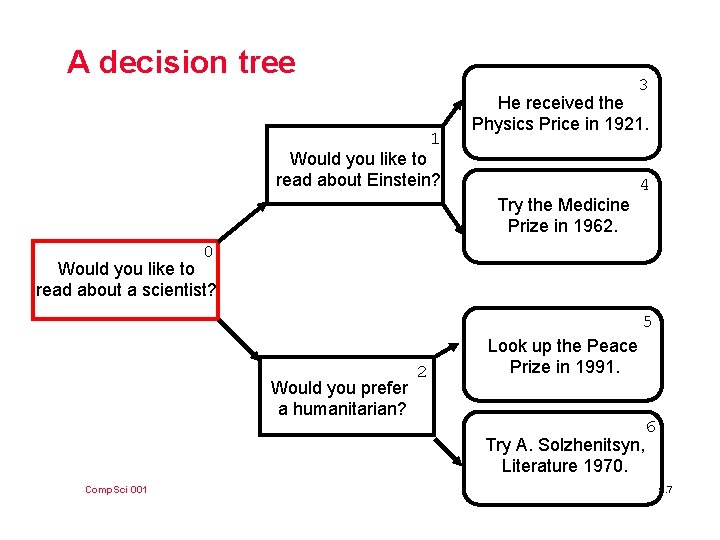
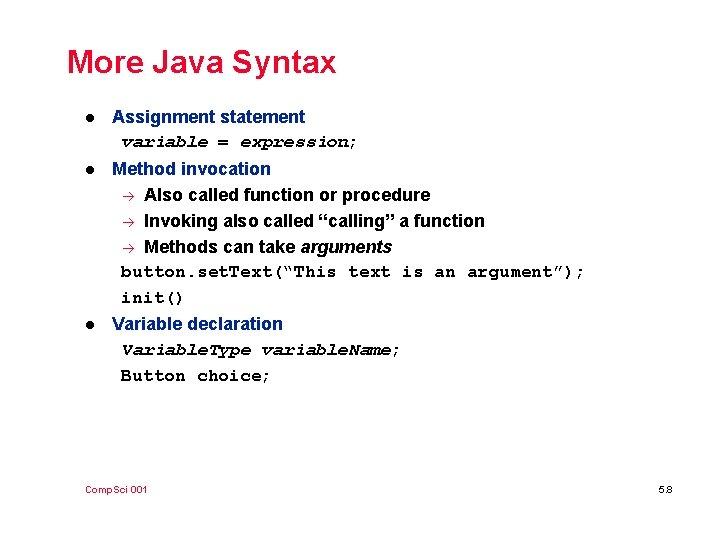
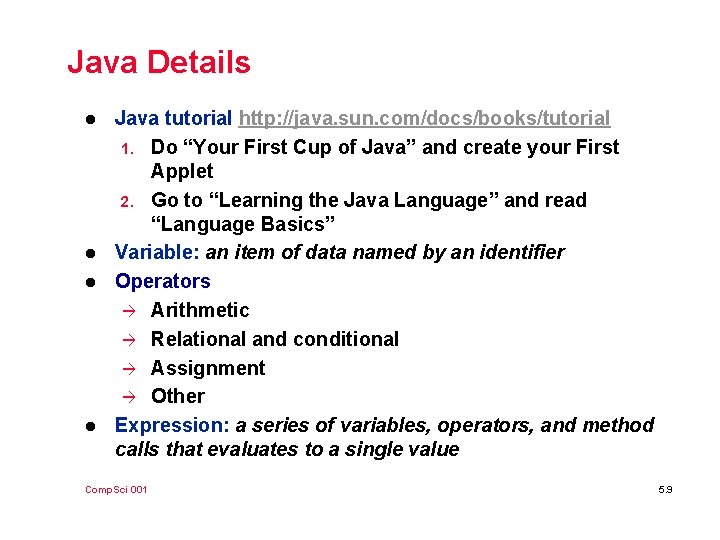
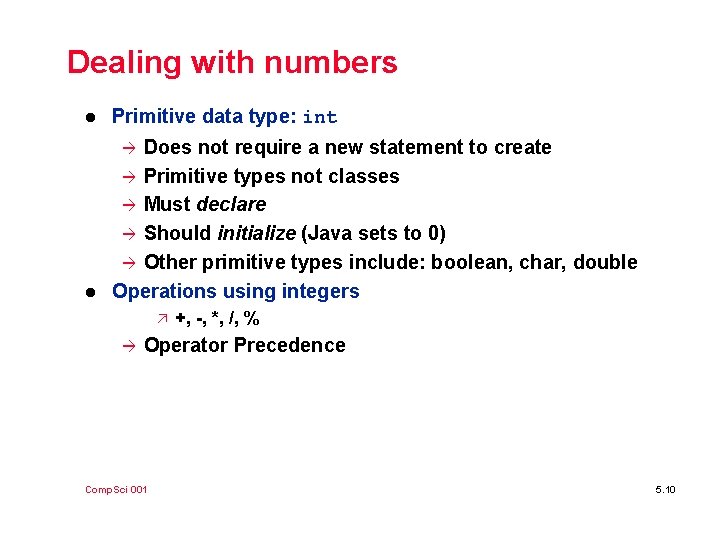
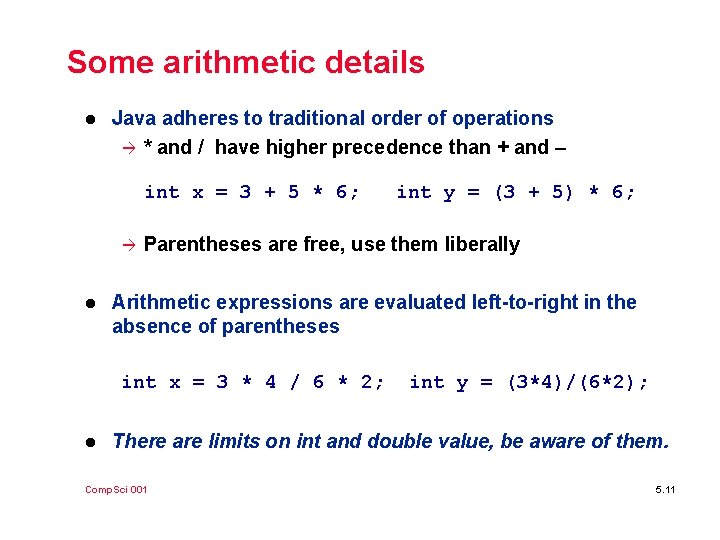
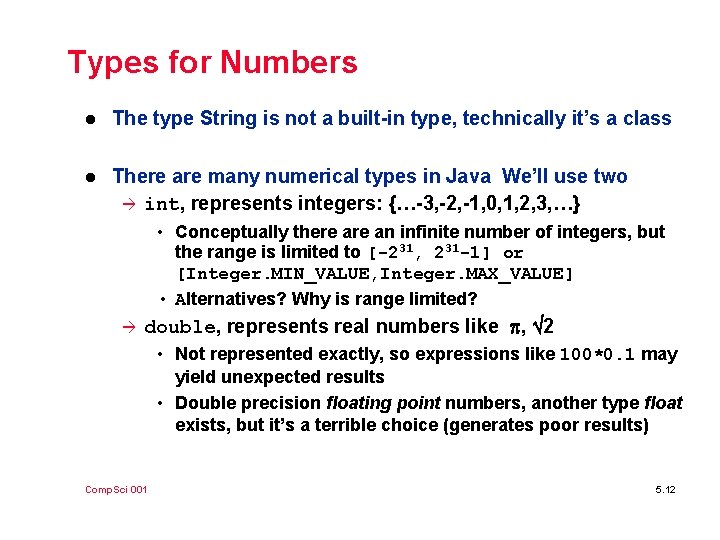
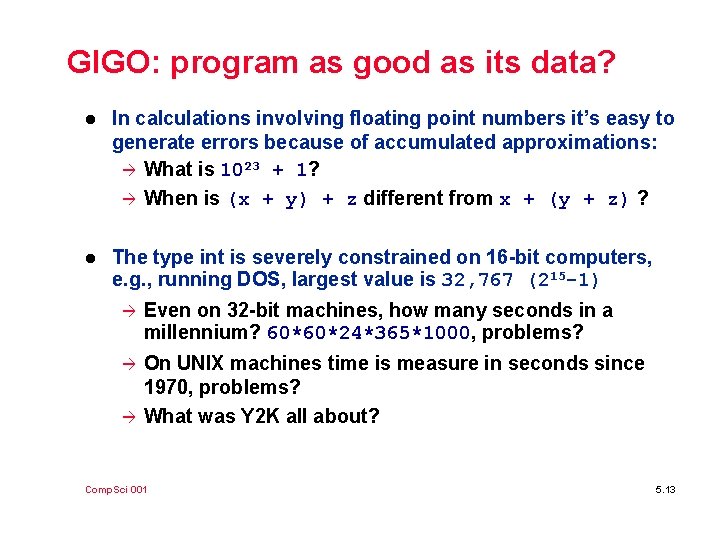
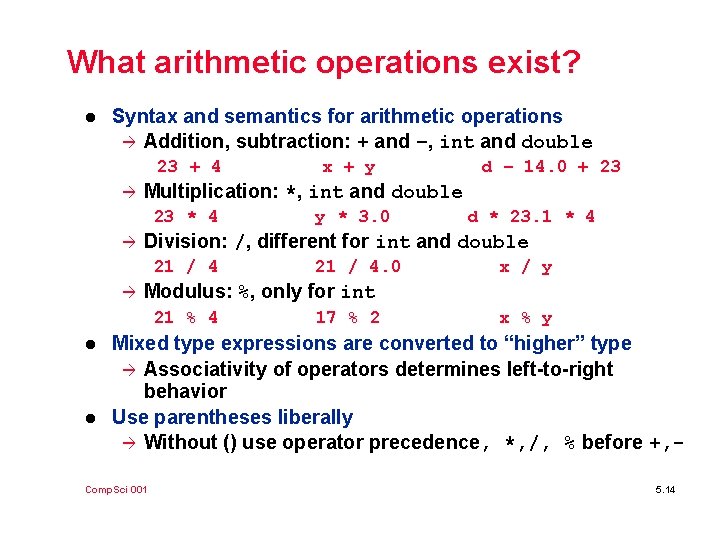
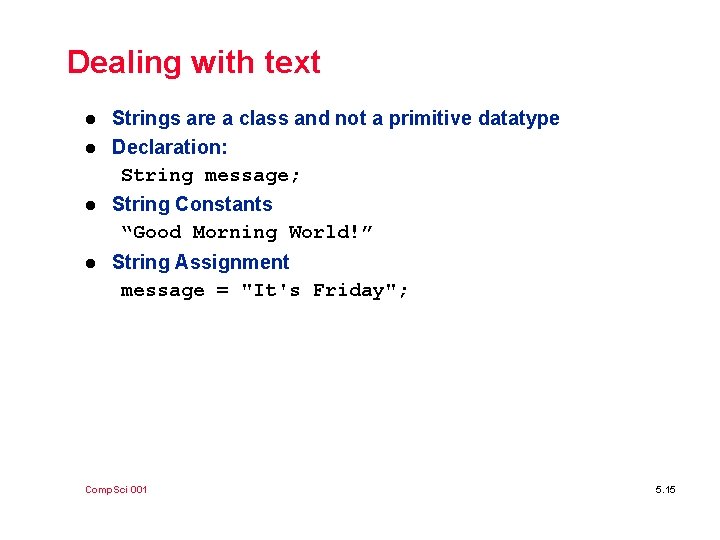
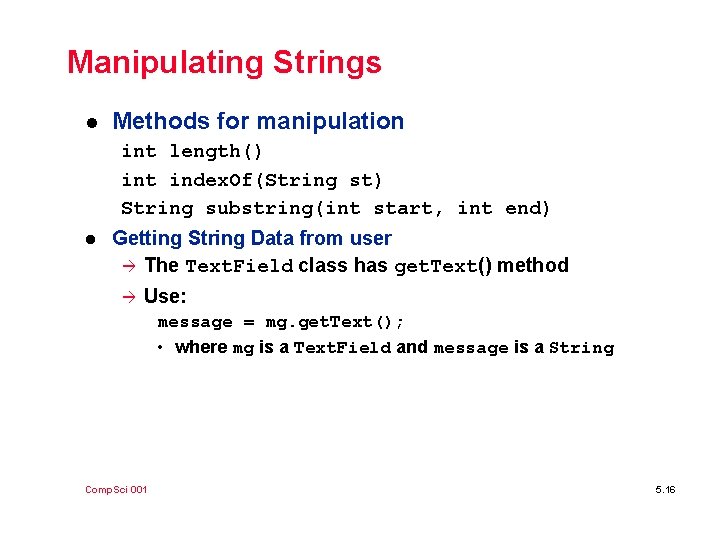
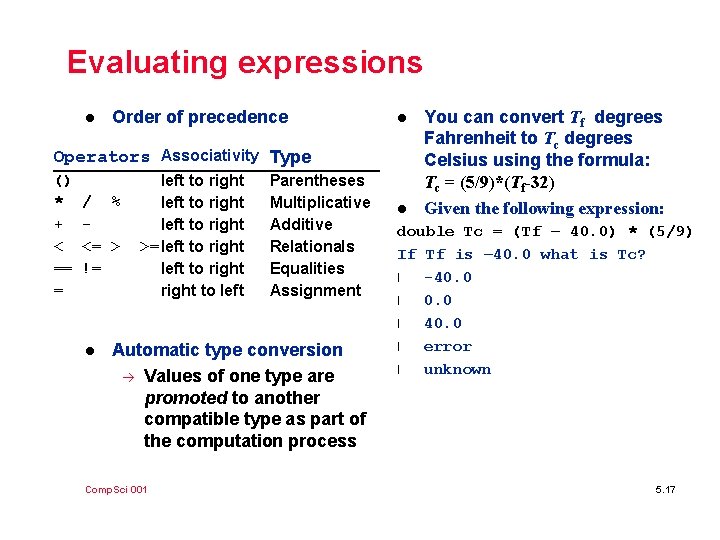
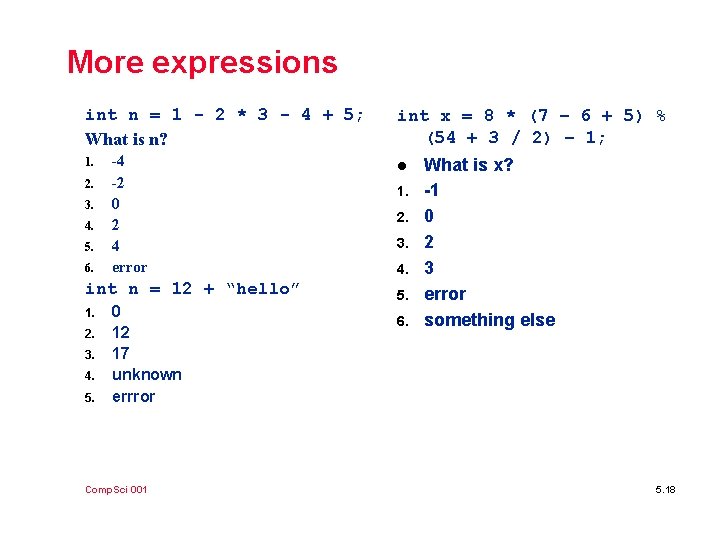
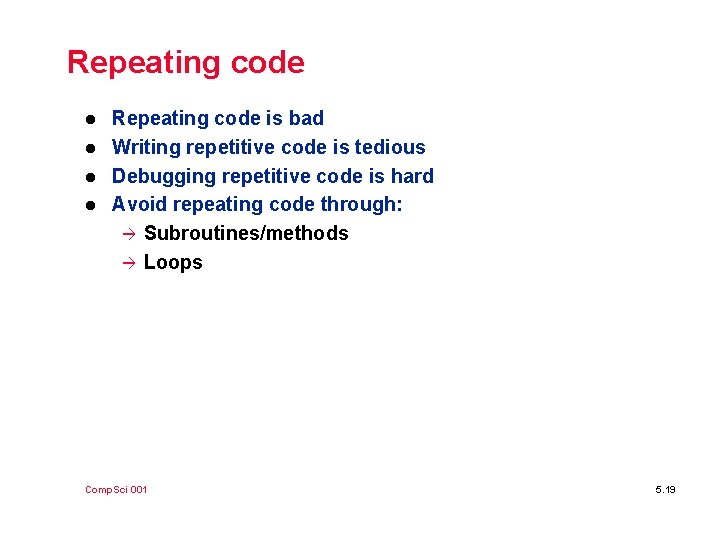
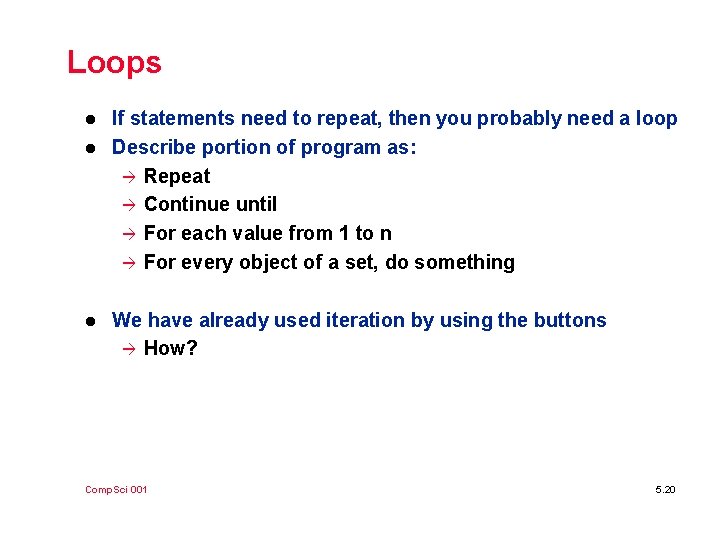
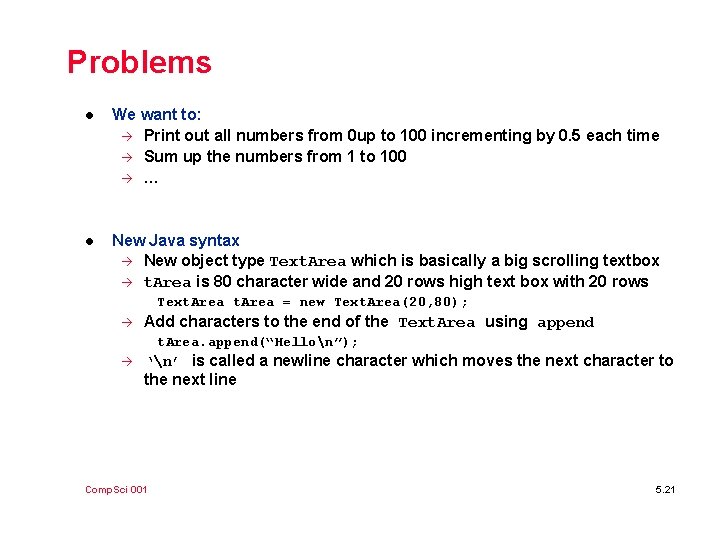
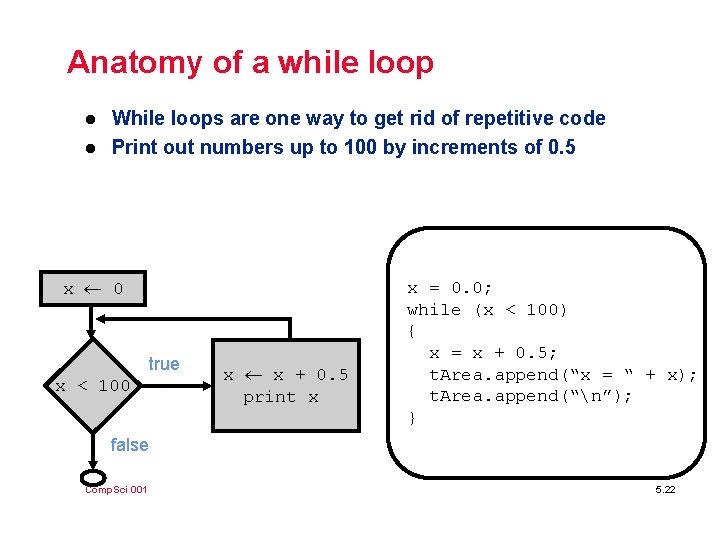
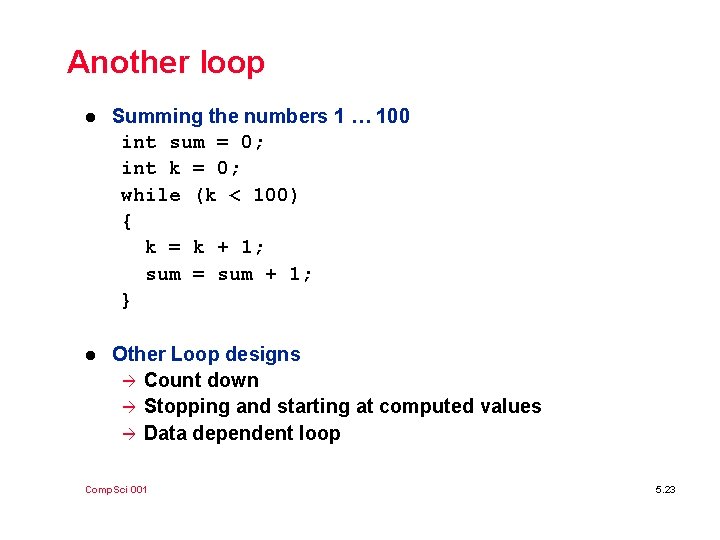
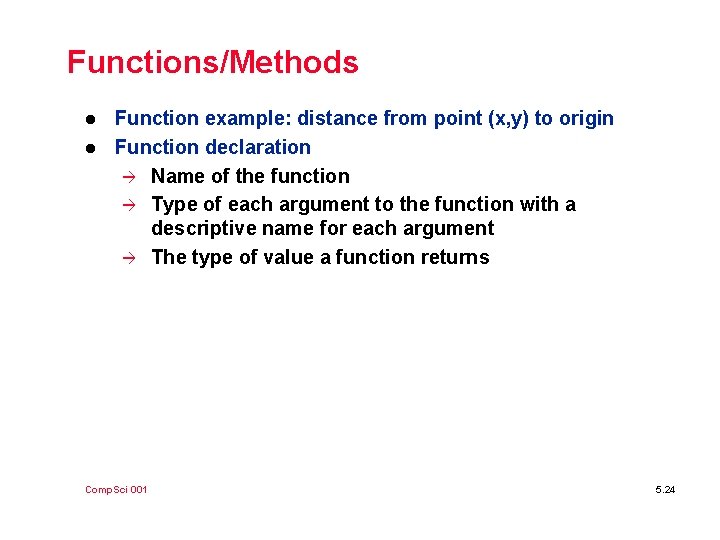
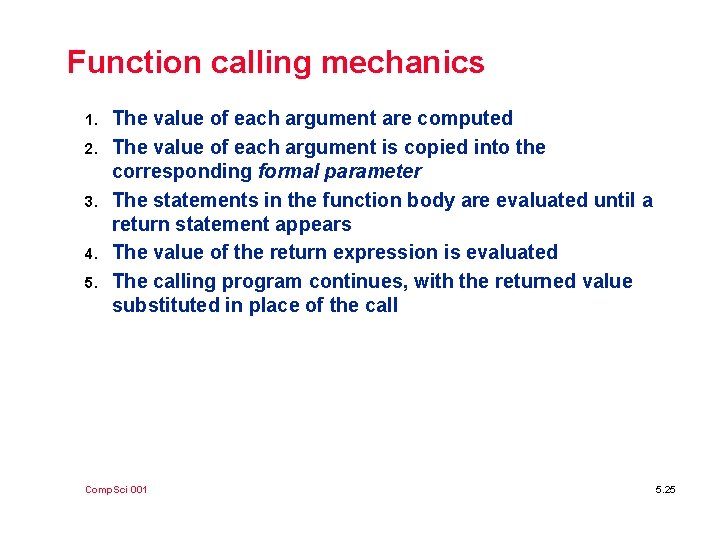
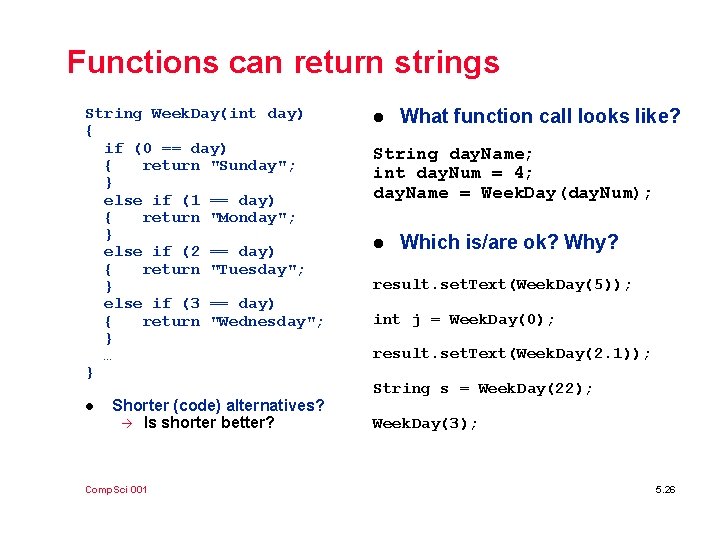
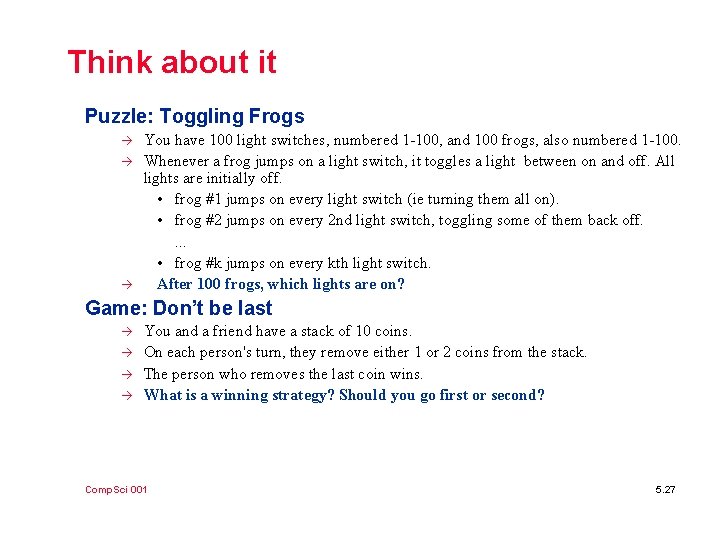
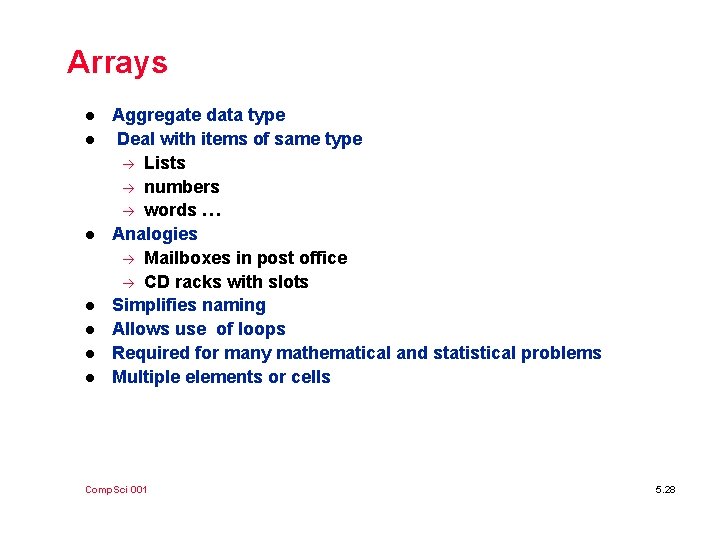
![Using arrays l l subscript or index to access element x[5] = 20; foo. Using arrays l l subscript or index to access element x[5] = 20; foo.](https://slidetodoc.com/presentation_image_h/0cb8523d612236cba1f25e65133a26d5/image-29.jpg)
![Creating Arrays l Declaration double weights[]; l Definition weights = new double[50]; Combine double Creating Arrays l Declaration double weights[]; l Definition weights = new double[50]; Combine double](https://slidetodoc.com/presentation_image_h/0cb8523d612236cba1f25e65133a26d5/image-30.jpg)
![Arrays & Loops int k = 2; while(k<6) { num[k] = k*k; k = Arrays & Loops int k = 2; while(k<6) { num[k] = k*k; k =](https://slidetodoc.com/presentation_image_h/0cb8523d612236cba1f25e65133a26d5/image-31.jpg)
- Slides: 31
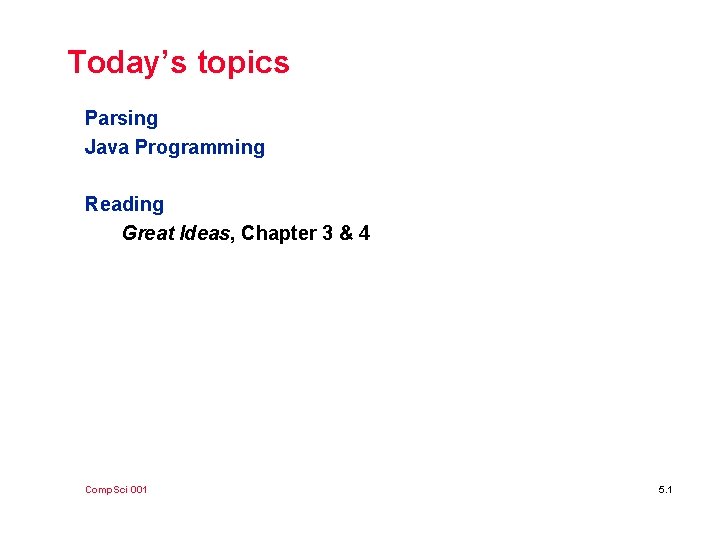
Today’s topics Parsing Java Programming Reading Great Ideas, Chapter 3 & 4 Comp. Sci 001 5. 1
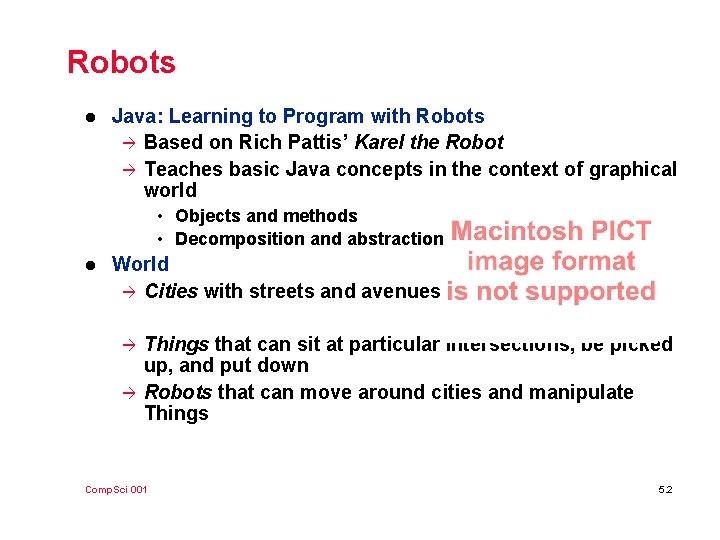
Robots l Java: Learning to Program with Robots à Based on Rich Pattis’ Karel the Robot à Teaches basic Java concepts in the context of graphical world • Objects and methods • Decomposition and abstraction l World à Cities with streets and avenues à à Things that can sit at particular intersections, be picked up, and put down Robots that can move around cities and manipulate Things Comp. Sci 001 5. 2
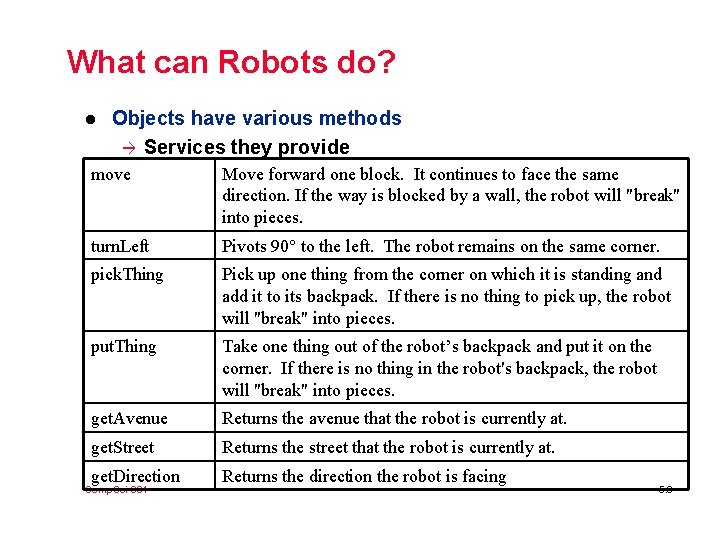
What can Robots do? l Objects have various methods à Services they provide move Move forward one block. It continues to face the same direction. If the way is blocked by a wall, the robot will "break" into pieces. turn. Left Pivots 90 to the left. The robot remains on the same corner. pick. Thing Pick up one thing from the corner on which it is standing and add it to its backpack. If there is no thing to pick up, the robot will "break" into pieces. put. Thing Take one thing out of the robot’s backpack and put it on the corner. If there is no thing in the robot's backpack, the robot will "break" into pieces. get. Avenue Returns the avenue that the robot is currently at. get. Street Returns the street that the robot is currently at. get. Direction Returns the direction the robot is facing Comp. Sci 001 5. 3
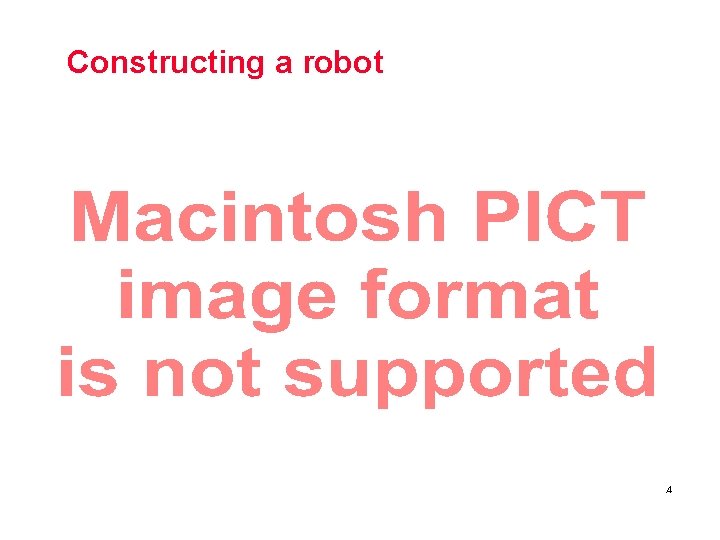
Constructing a robot Comp. Sci 001 5. 4
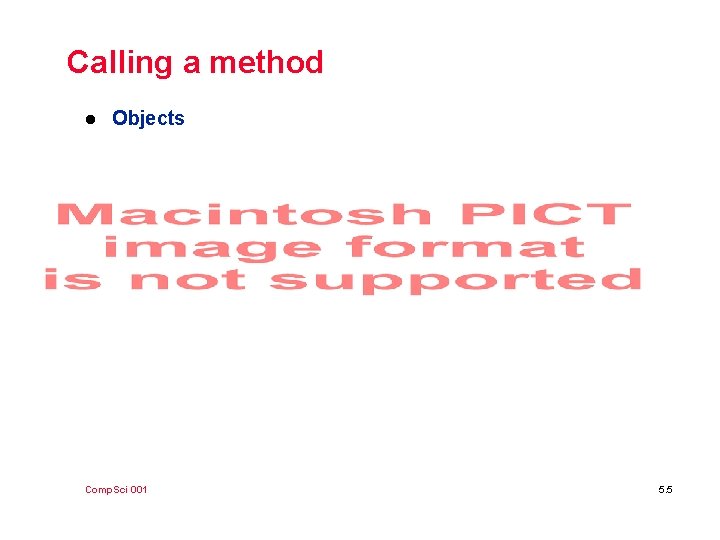
Calling a method l Objects Comp. Sci 001 5. 5
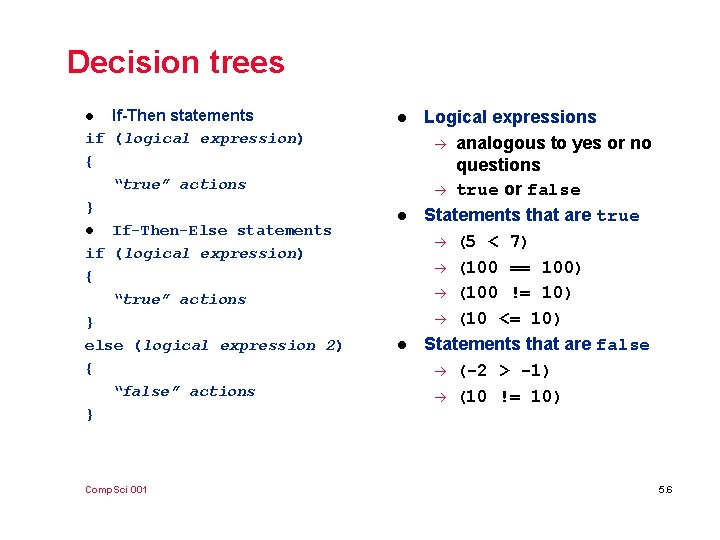
Decision trees If-Then statements if (logical expression) { “true” actions } l If-Then-Else statements if (logical expression) { “true” actions } else (logical expression 2) { “false” actions } l Comp. Sci 001 l l l Logical expressions à analogous to yes or no questions à true or false Statements that are true à (5 < 7) à (100 == 100) à (100 != 10) à (10 <= 10) Statements that are false à (-2 > -1) à (10 != 10) 5. 6
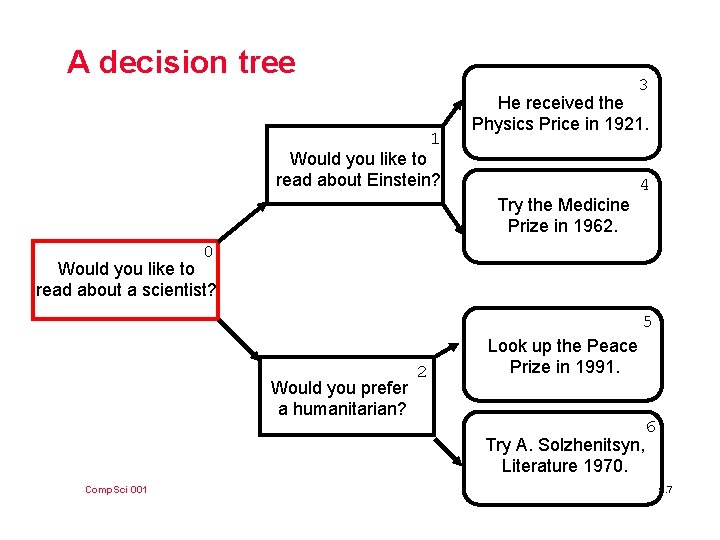
A decision tree 3 1 He received the Physics Price in 1921. Would you like to read about Einstein? Try the Medicine Prize in 1962. 4 0 Would you like to read about a scientist? 5 Would you prefer a humanitarian? Comp. Sci 001 2 Look up the Peace Prize in 1991. 6 Try A. Solzhenitsyn, Literature 1970. 5. 7
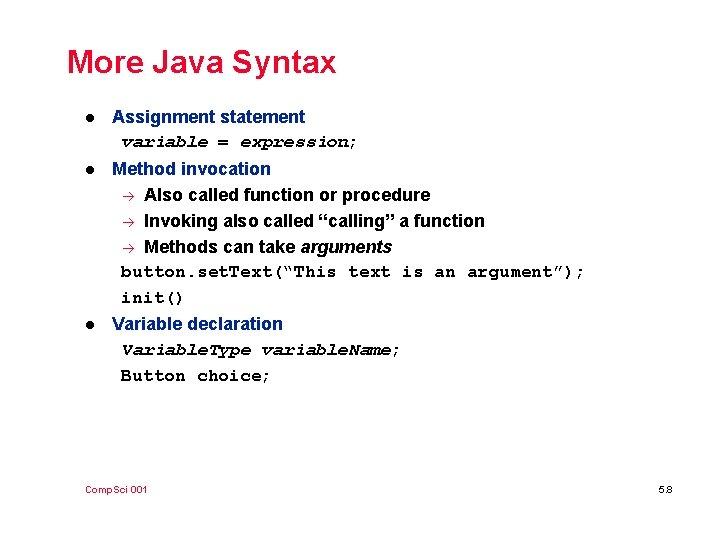
More Java Syntax l Assignment statement variable = expression; l Method invocation à Also called function or procedure à Invoking also called “calling” a function à Methods can take arguments button. set. Text(“This text is an argument”); init() l Variable declaration Variable. Type variable. Name; Button choice; Comp. Sci 001 5. 8
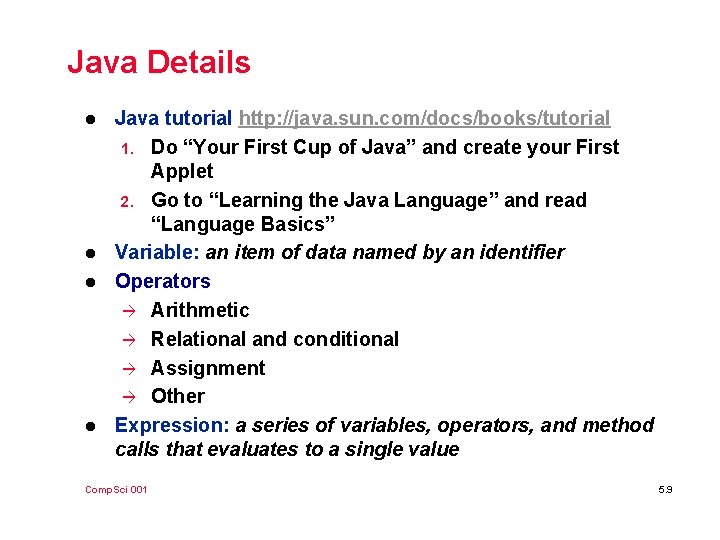
Java Details l l Java tutorial http: //java. sun. com/docs/books/tutorial 1. Do “Your First Cup of Java” and create your First Applet 2. Go to “Learning the Java Language” and read “Language Basics” Variable: an item of data named by an identifier Operators à Arithmetic à Relational and conditional à Assignment à Other Expression: a series of variables, operators, and method calls that evaluates to a single value Comp. Sci 001 5. 9
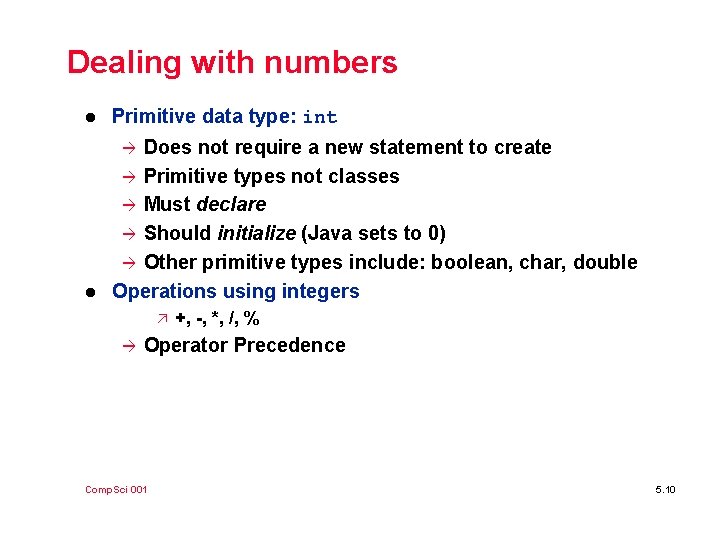
Dealing with numbers l Primitive data type: int l Does not require a new statement to create à Primitive types not classes à Must declare à Should initialize (Java sets to 0) à Other primitive types include: boolean, char, double Operations using integers à ä à +, -, *, /, % Operator Precedence Comp. Sci 001 5. 10
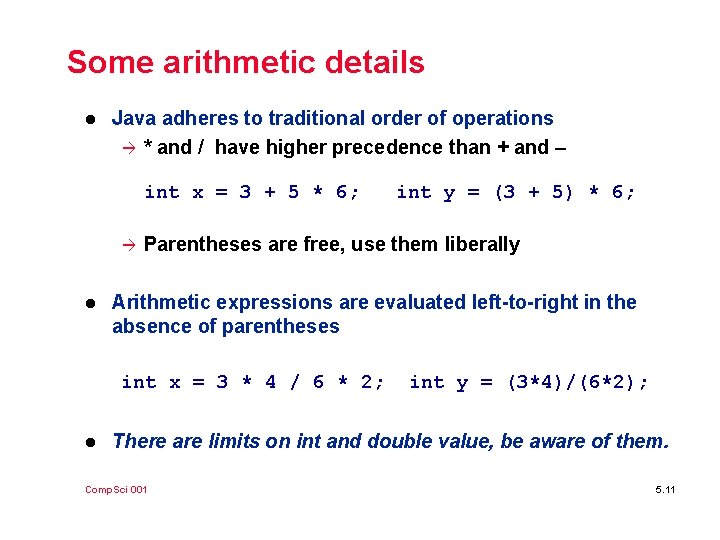
Some arithmetic details l Java adheres to traditional order of operations à * and / have higher precedence than + and – int x = 3 + 5 * 6; à l Parentheses are free, use them liberally Arithmetic expressions are evaluated left-to-right in the absence of parentheses int x = 3 * 4 / 6 * 2; l int y = (3 + 5) * 6; int y = (3*4)/(6*2); There are limits on int and double value, be aware of them. Comp. Sci 001 5. 11
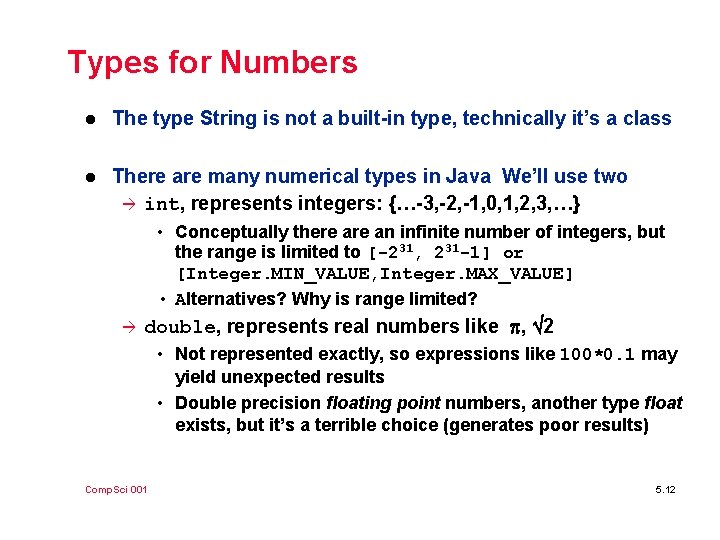
Types for Numbers l The type String is not a built-in type, technically it’s a class l There are many numerical types in Java We’ll use two à int, represents integers: {…-3, -2, -1, 0, 1, 2, 3, …} • Conceptually there an infinite number of integers, but the range is limited to [-231, 231 -1] or [Integer. MIN_VALUE, Integer. MAX_VALUE] • Alternatives? Why is range limited? à double, represents real numbers like , 2 • Not represented exactly, so expressions like 100*0. 1 may yield unexpected results • Double precision floating point numbers, another type float exists, but it’s a terrible choice (generates poor results) Comp. Sci 001 5. 12
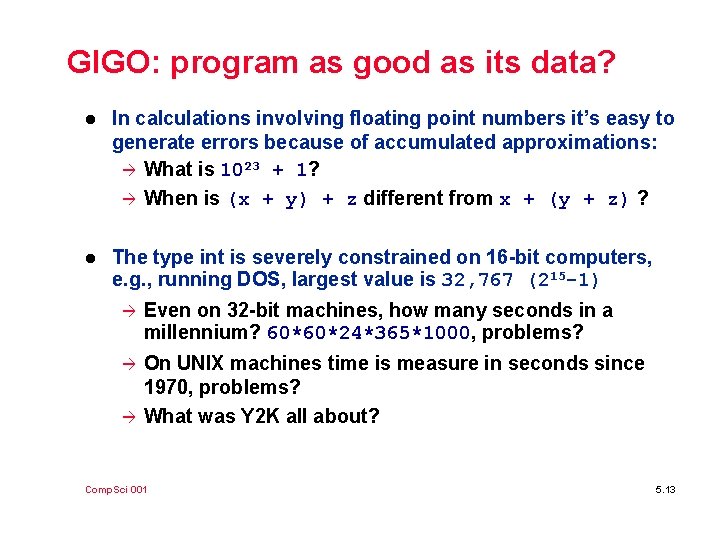
GIGO: program as good as its data? l In calculations involving floating point numbers it’s easy to generate errors because of accumulated approximations: à What is 1023 + 1? à When is (x + y) + z different from x + (y + z) ? l The type int is severely constrained on 16 -bit computers, e. g. , running DOS, largest value is 32, 767 (215 -1) à Even on 32 -bit machines, how many seconds in a millennium? 60*60*24*365*1000, problems? à On UNIX machines time is measure in seconds since 1970, problems? What was Y 2 K all about? à Comp. Sci 001 5. 13
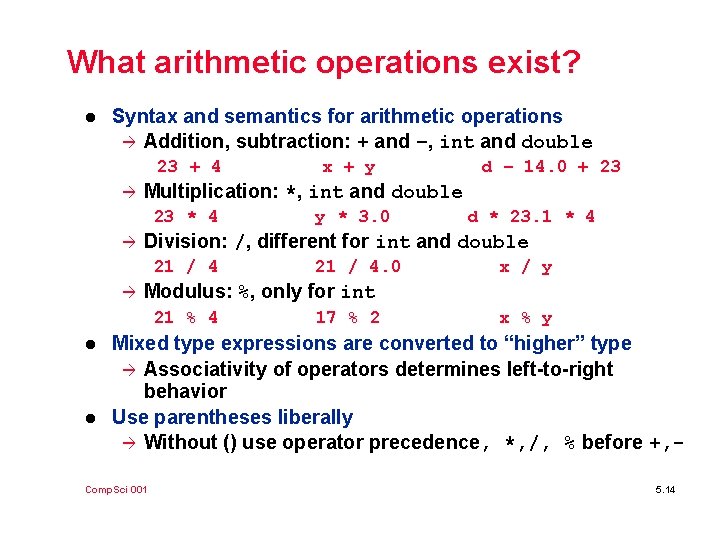
What arithmetic operations exist? l Syntax and semantics for arithmetic operations à Addition, subtraction: + and –, int and double 23 + 4 à l d * 23. 1 * 4 21 / 4. 0 x / y Modulus: %, only for int 21 % 4 l y * 3. 0 Division: /, different for int and double 21 / 4 à d – 14. 0 + 23 Multiplication: *, int and double 23 * 4 à x + y 17 % 2 x % y Mixed type expressions are converted to “higher” type à Associativity of operators determines left-to-right behavior Use parentheses liberally à Without () use operator precedence, *, /, % before +, - Comp. Sci 001 5. 14
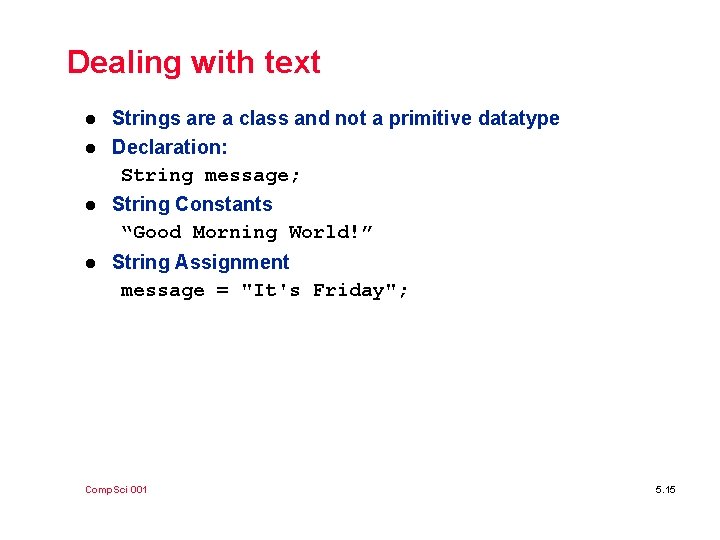
Dealing with text l l Strings are a class and not a primitive datatype Declaration: String message; l String Constants “Good Morning World!” l String Assignment message = "It's Friday"; Comp. Sci 001 5. 15
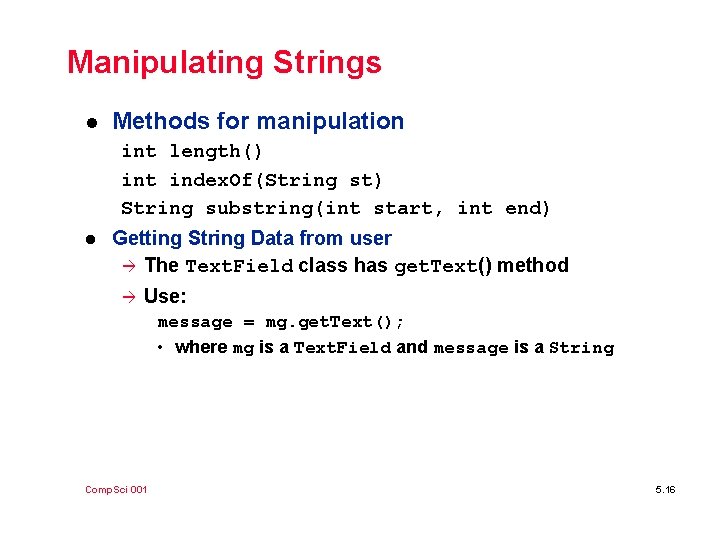
Manipulating Strings l Methods for manipulation int length() int index. Of(String st) String substring(int start, int end) l Getting String Data from user à The Text. Field class has get. Text() method à Use: message = mg. get. Text(); • where mg is a Text. Field and message is a String Comp. Sci 001 5. 16
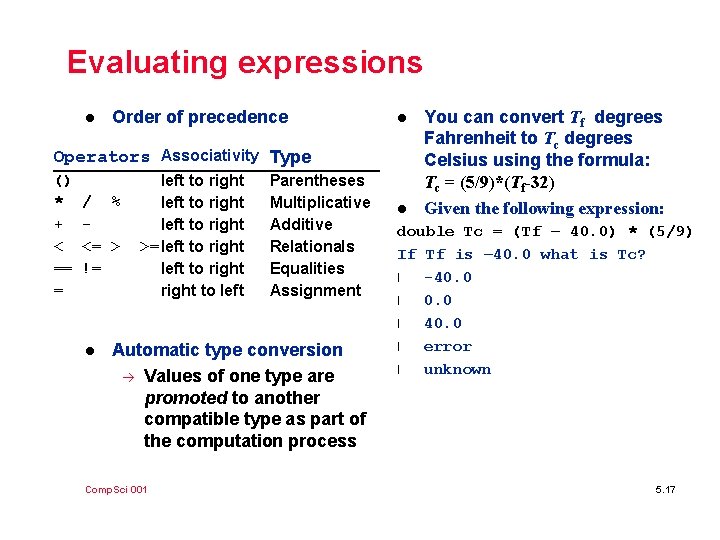
Evaluating expressions l Order of precedence l Operators Associativity Type () * + < == = / % <= > != l left to right >= left to right to left Parentheses Multiplicative Additive Relationals Equalities Assignment Automatic type conversion à Values of one type are promoted to another compatible type as part of the computation process Comp. Sci 001 l You can convert Tf degrees Fahrenheit to Tc degrees Celsius using the formula: Tc = (5/9)*(Tf-32) Given the following expression: double Tc = (Tf – 40. 0) * (5/9) If Tf is – 40. 0 what is Tc? l -40. 0 l error l unknown 5. 17
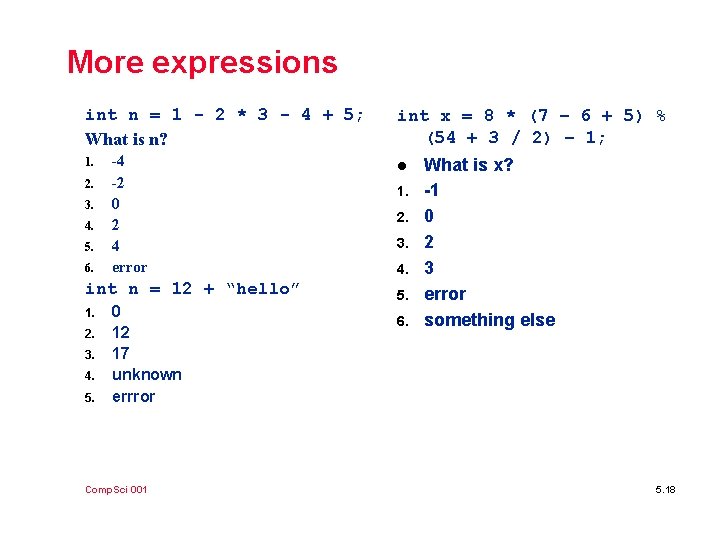
More expressions int n = 1 - 2 * 3 - 4 + 5; What is n? 1. 2. 3. 4. 5. 6. -4 -2 0 2 4 error int n = 12 + “hello” 1. 2. 3. 4. 5. 0 12 17 unknown errror Comp. Sci 001 int x = 8 * (7 – 6 + 5) % (54 + 3 / 2) – 1; l 1. 2. 3. 4. 5. 6. What is x? -1 0 2 3 error something else 5. 18
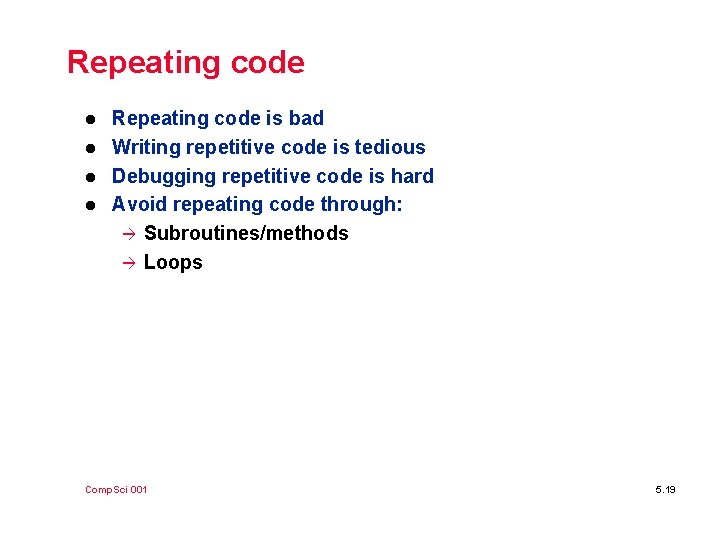
Repeating code l l Repeating code is bad Writing repetitive code is tedious Debugging repetitive code is hard Avoid repeating code through: à Subroutines/methods à Loops Comp. Sci 001 5. 19
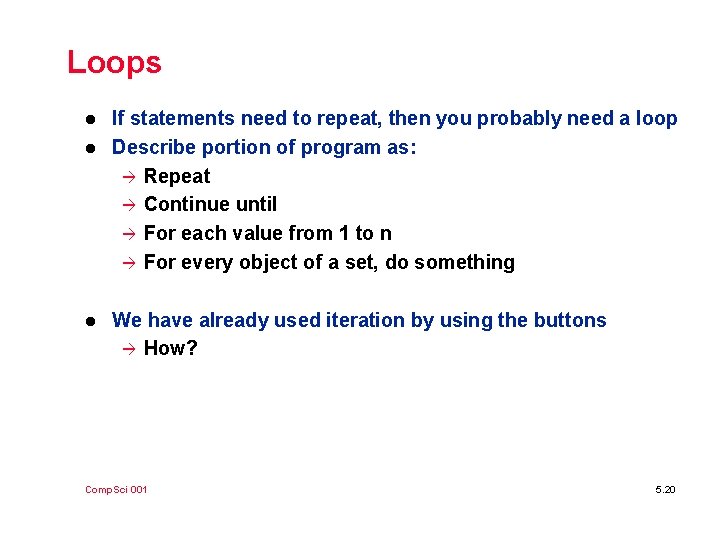
Loops l l l If statements need to repeat, then you probably need a loop Describe portion of program as: à Repeat à Continue until à For each value from 1 to n à For every object of a set, do something We have already used iteration by using the buttons à How? Comp. Sci 001 5. 20
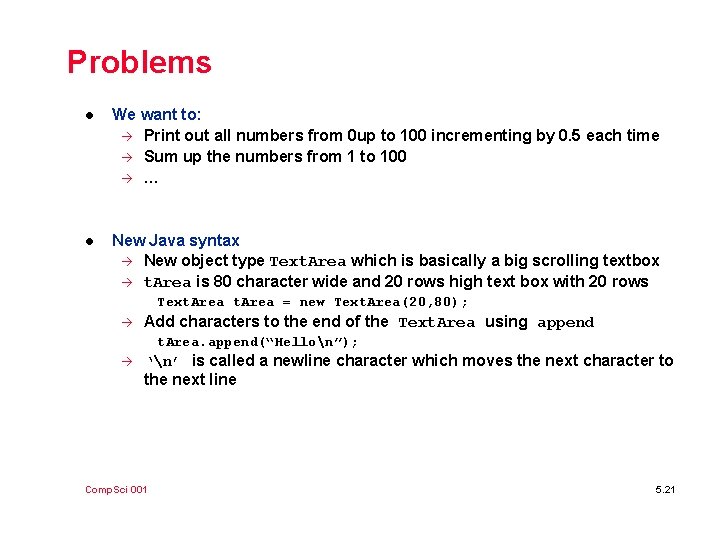
Problems l We want to: à Print out all numbers from 0 up to 100 incrementing by 0. 5 each time à Sum up the numbers from 1 to 100 à … l New Java syntax à New object type Text. Area which is basically a big scrolling textbox à t. Area is 80 character wide and 20 rows high text box with 20 rows Text. Area = new Text. Area(20, 80); à Add characters to the end of the Text. Area using append t. Area. append(“Hellon”); à ‘n’ is called a newline character which moves the next character to the next line Comp. Sci 001 5. 21
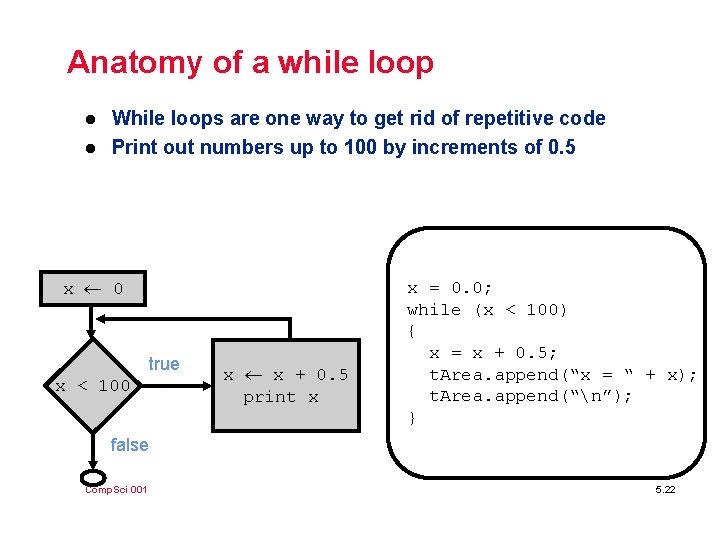
Anatomy of a while loop l l While loops are one way to get rid of repetitive code Print out numbers up to 100 by increments of 0. 5 x 0 true x < 100 x x + 0. 5 print x x = 0. 0; while (x < 100) { x = x + 0. 5; t. Area. append(“x = “ + x); t. Area. append(“n”); } false Comp. Sci 001 5. 22
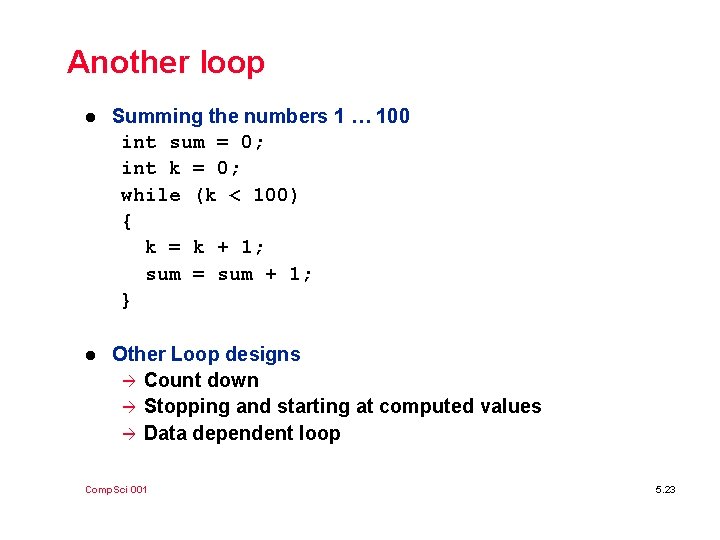
Another loop l Summing the numbers 1 … 100 int sum = 0; int k = 0; while (k < 100) { k = k + 1; sum = sum + 1; } l Other Loop designs à Count down à Stopping and starting at computed values à Data dependent loop Comp. Sci 001 5. 23
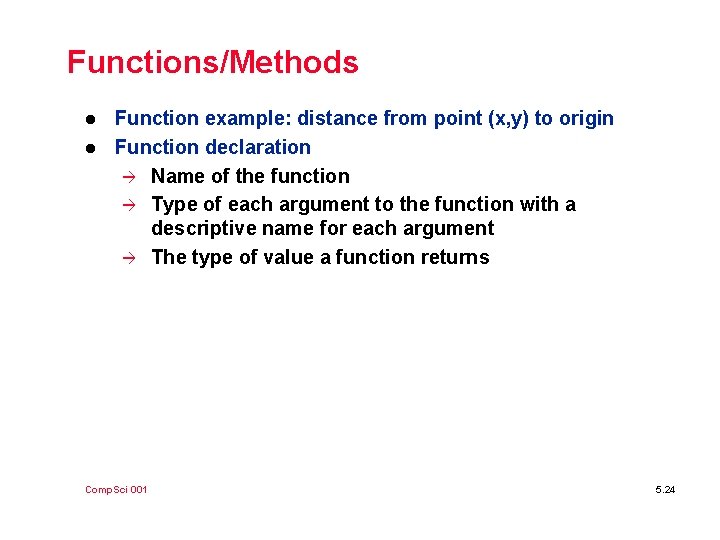
Functions/Methods l l Function example: distance from point (x, y) to origin Function declaration à Name of the function à Type of each argument to the function with a descriptive name for each argument à The type of value a function returns Comp. Sci 001 5. 24
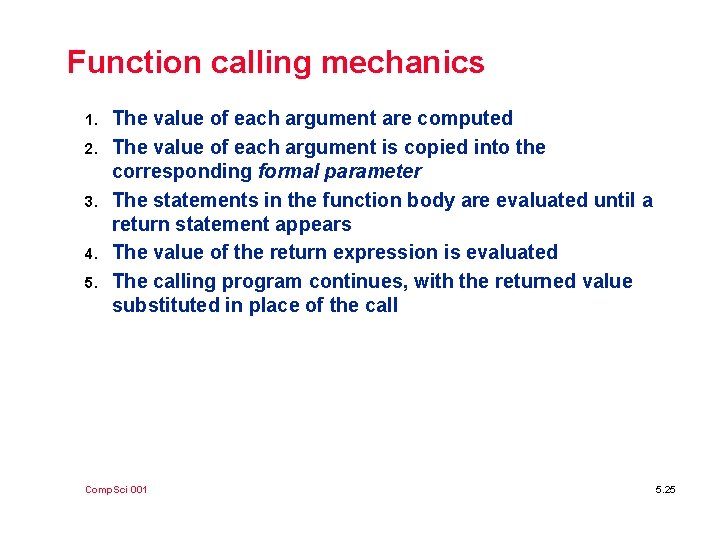
Function calling mechanics 1. 2. 3. 4. 5. The value of each argument are computed The value of each argument is copied into the corresponding formal parameter The statements in the function body are evaluated until a return statement appears The value of the return expression is evaluated The calling program continues, with the returned value substituted in place of the call Comp. Sci 001 5. 25
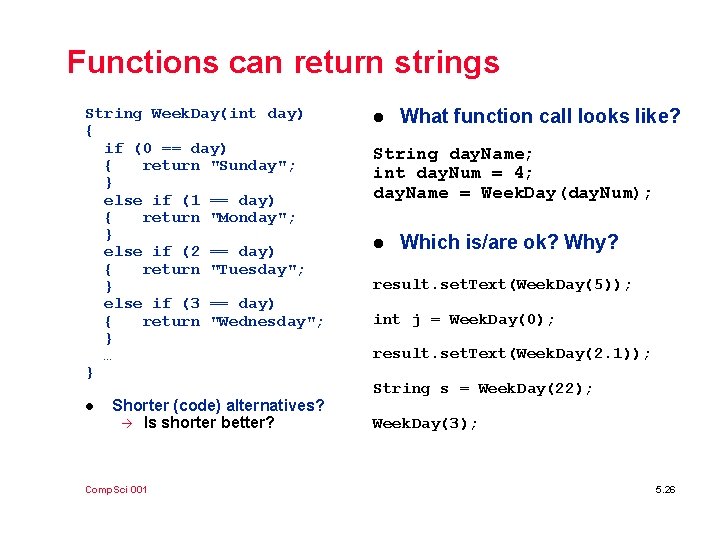
Functions can return strings String Week. Day(int day) { if (0 == day) { return "Sunday"; } else if (1 == day) { return "Monday"; } else if (2 == day) { return "Tuesday"; } else if (3 == day) { return "Wednesday"; } … } l Shorter (code) alternatives? à Is shorter better? Comp. Sci 001 l What function call looks like? String day. Name; int day. Num = 4; day. Name = Week. Day(day. Num); l Which is/are ok? Why? result. set. Text(Week. Day(5)); int j = Week. Day(0); result. set. Text(Week. Day(2. 1)); String s = Week. Day(22); Week. Day(3); 5. 26
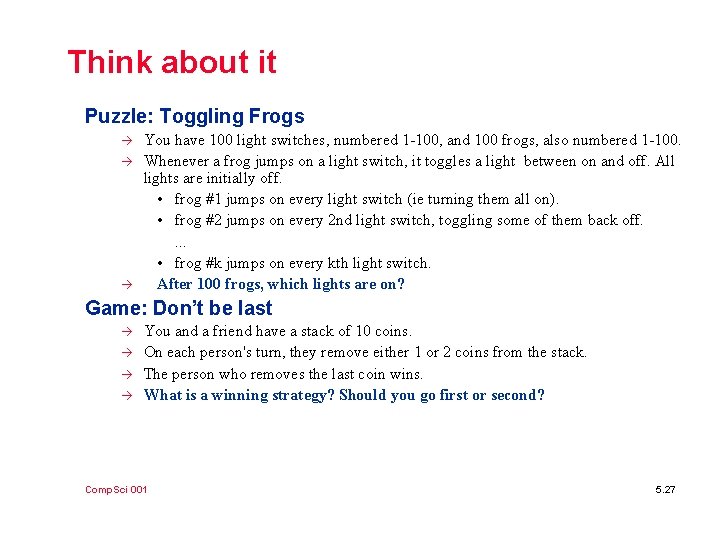
Think about it Puzzle: Toggling Frogs à à à You have 100 light switches, numbered 1 -100, and 100 frogs, also numbered 1 -100. Whenever a frog jumps on a light switch, it toggles a light between on and off. All lights are initially off. • frog #1 jumps on every light switch (ie turning them all on). • frog #2 jumps on every 2 nd light switch, toggling some of them back off. . • frog #k jumps on every kth light switch. After 100 frogs, which lights are on? Game: Don’t be last à à You and a friend have a stack of 10 coins. On each person's turn, they remove either 1 or 2 coins from the stack. The person who removes the last coin wins. What is a winning strategy? Should you go first or second? Comp. Sci 001 5. 27
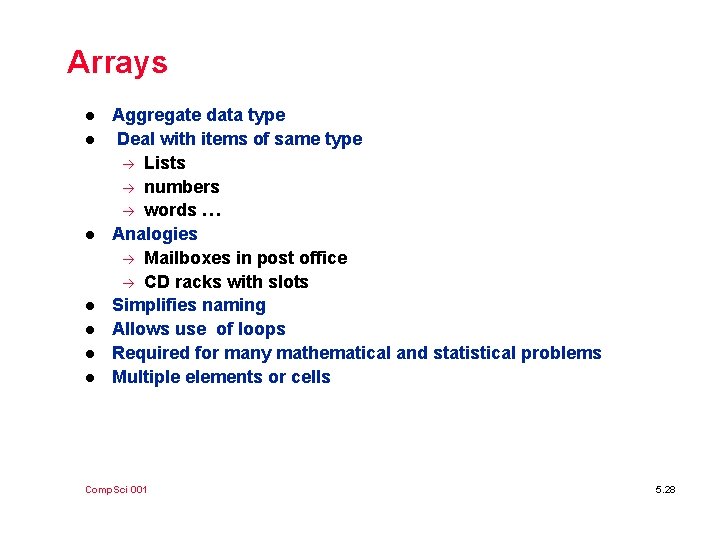
Arrays l l l l Aggregate data type Deal with items of same type à Lists à numbers à words … Analogies à Mailboxes in post office à CD racks with slots Simplifies naming Allows use of loops Required for many mathematical and statistical problems Multiple elements or cells Comp. Sci 001 5. 28
![Using arrays l l subscript or index to access element x5 20 foo Using arrays l l subscript or index to access element x[5] = 20; foo.](https://slidetodoc.com/presentation_image_h/0cb8523d612236cba1f25e65133a26d5/image-29.jpg)
Using arrays l l subscript or index to access element x[5] = 20; foo. set. Text(“Result is " + x[5]); Often used in loops int k while { k = sum } Comp. Sci 001 = 0; sum = 0; ( k < 10 ) k + 1; = sum + name[k]; 5. 29
![Creating Arrays l Declaration double weights l Definition weights new double50 Combine double Creating Arrays l Declaration double weights[]; l Definition weights = new double[50]; Combine double](https://slidetodoc.com/presentation_image_h/0cb8523d612236cba1f25e65133a26d5/image-30.jpg)
Creating Arrays l Declaration double weights[]; l Definition weights = new double[50]; Combine double weights[] = new double[50]; l int num[] = new int[6]; ? ? = 13; ? num[1] =? 21; ? num[5] ? Comp. Sci 001 21 ? ? 13 5. 30
![Arrays Loops int k 2 whilek6 numk kk k Arrays & Loops int k = 2; while(k<6) { num[k] = k*k; k =](https://slidetodoc.com/presentation_image_h/0cb8523d612236cba1f25e65133a26d5/image-31.jpg)
Arrays & Loops int k = 2; while(k<6) { num[k] = k*k; k = k+1; } ? Comp. Sci 001 21 4 9 16 25 5. 31
Pre reading while reading and post reading activities
Conclusion in sba
Reading for today topics 5
Dissatisfaction theme in the great gatsby
Http://www.history.com/topics/great-depression
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
Runtime programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
Recursive descent parsing
Semantic parsing
Recursive descent parsing
Classic parses
Ll1 grammar
Parsing syntax
Error recovery in predictive parsing
The lexical analysis for a modern computer
Recursive descent parser c
Steps of query processing
Difference top down and bottom up
Parsing adalah
Parsing adalah
Probabilistic parsing
End-to-end wireframe parsing
Morphological parsing in nlp
Visual studio regular expression
Cfg adalah
C string parsing
Parsing adalah
Non recursive predictive parsing
Teknik parsing logika informatika