Todays topics Java Arrays Upcoming Functions Reading Great
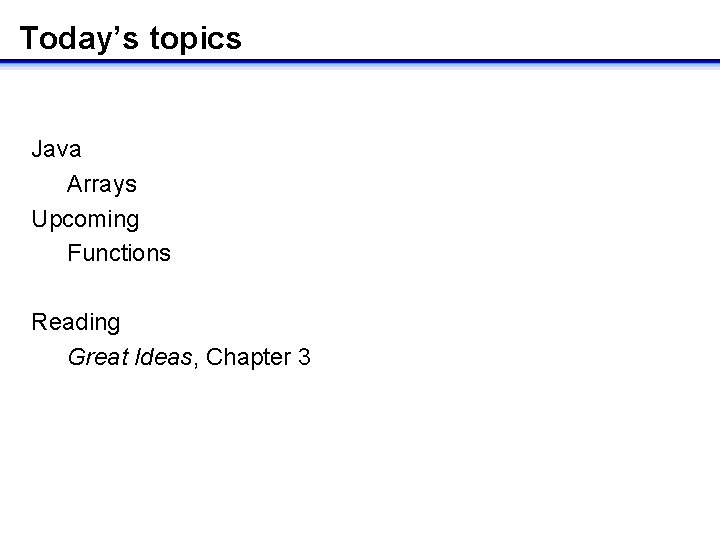
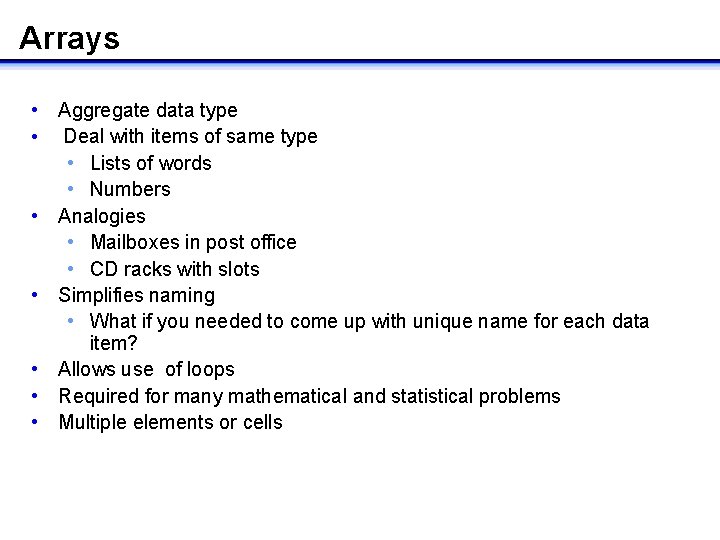
![Using arrays • Use subscript or index to access an element x[5] = 20; Using arrays • Use subscript or index to access an element x[5] = 20;](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-3.jpg)
![Creating Arrays • Declaration double weights[]; • Definition weights = new double[50]; • Combine Creating Arrays • Declaration double weights[]; • Definition weights = new double[50]; • Combine](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-4.jpg)
![Arrays & Loops num[0] = 0; int k = 2; while(k < num. length) Arrays & Loops num[0] = 0; int k = 2; while(k < num. length)](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-5.jpg)
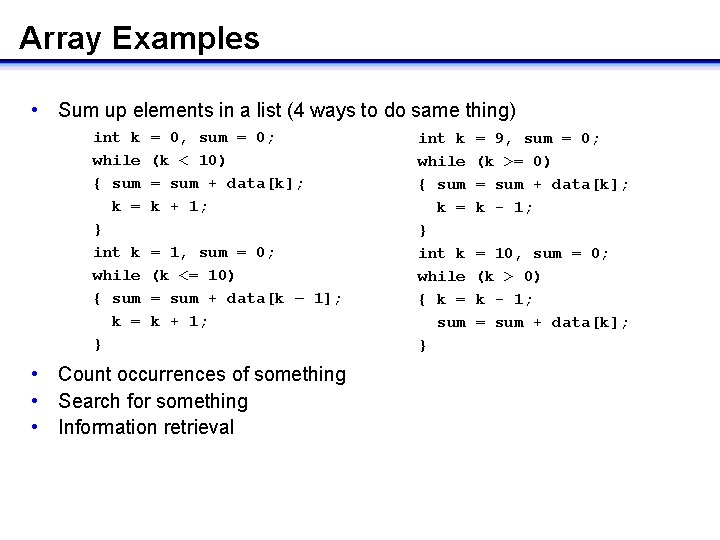
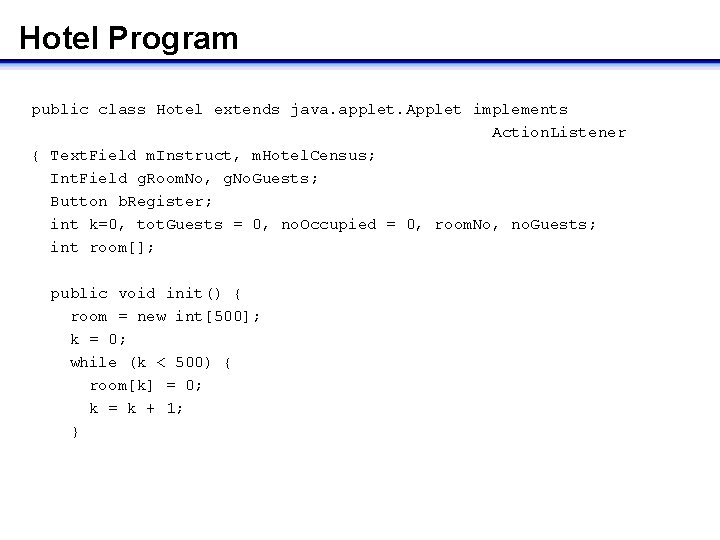
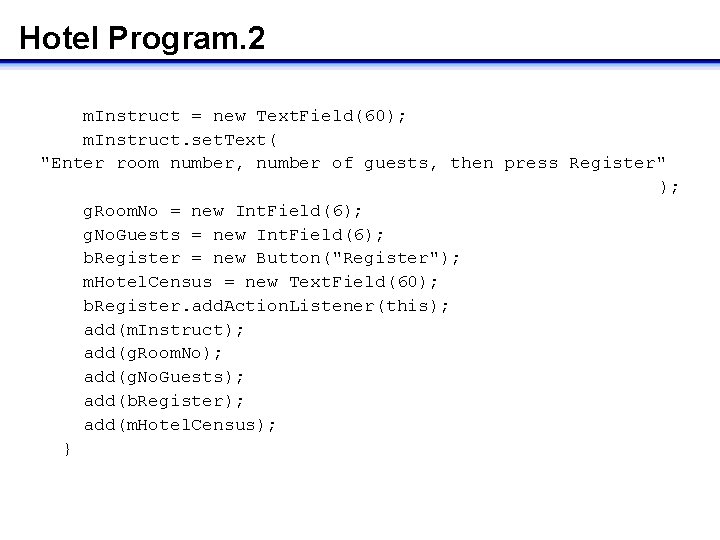
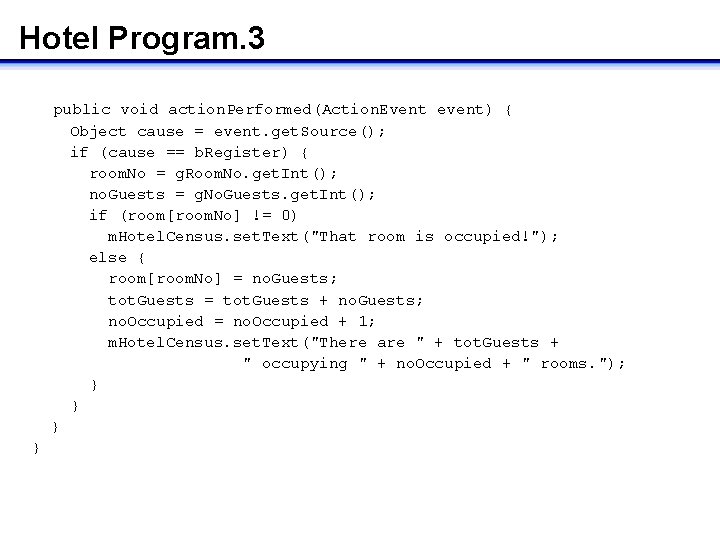
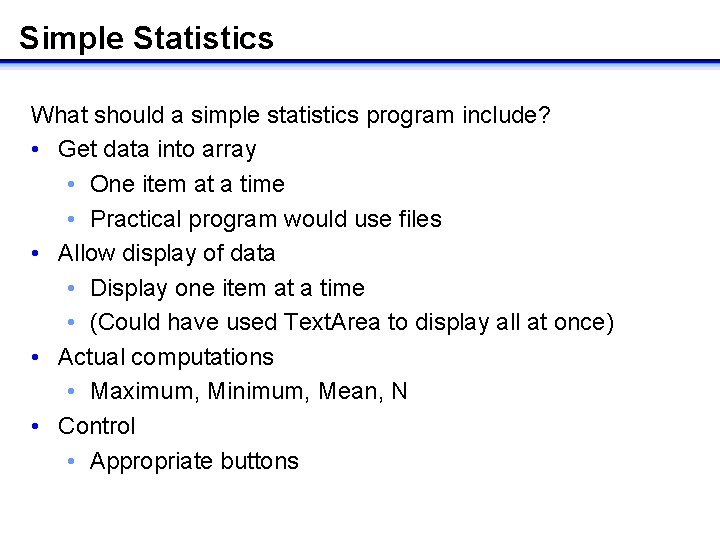
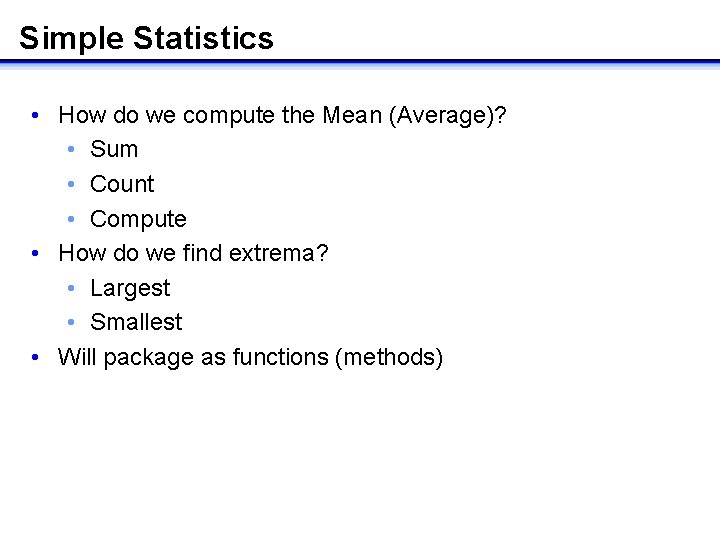
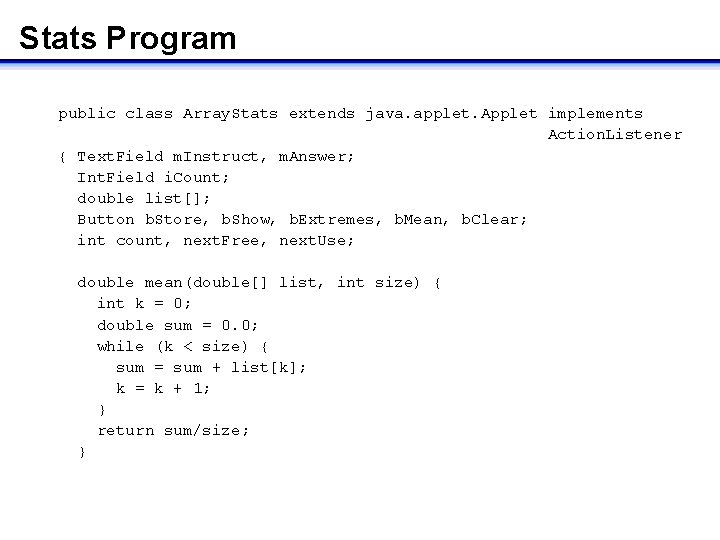
![Stats Program. 2 double max(double[] list, int size) { int k = 1; double Stats Program. 2 double max(double[] list, int size) { int k = 1; double](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-13.jpg)
![Stats Program. 3 double min(double[] list, int size) { int k = 1; double Stats Program. 3 double min(double[] list, int size) { int k = 1; double](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-14.jpg)
![Stats Program. 4 public void init() { list = new double[100]; m. Instruct = Stats Program. 4 public void init() { list = new double[100]; m. Instruct =](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-15.jpg)
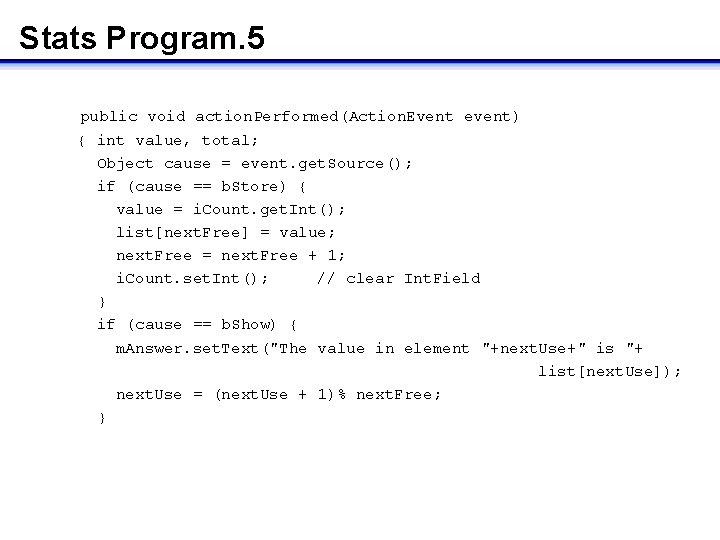
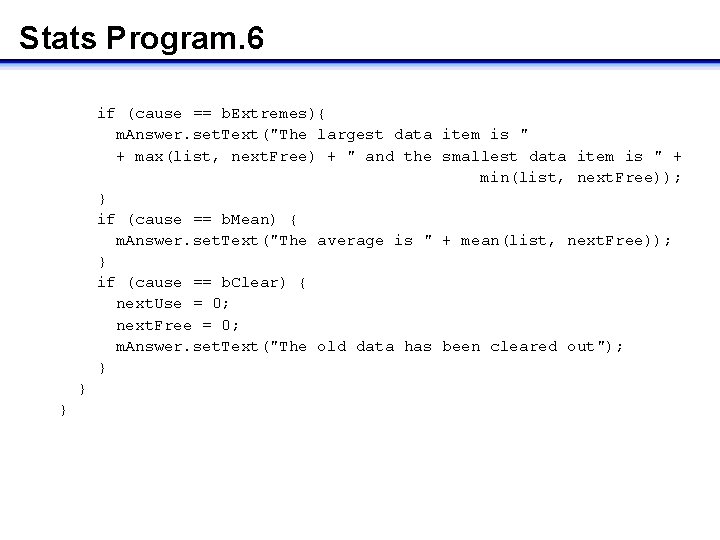
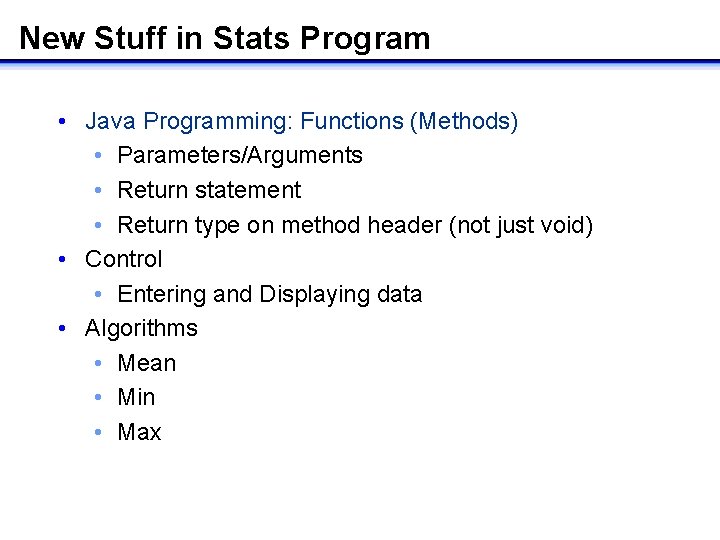
- Slides: 18
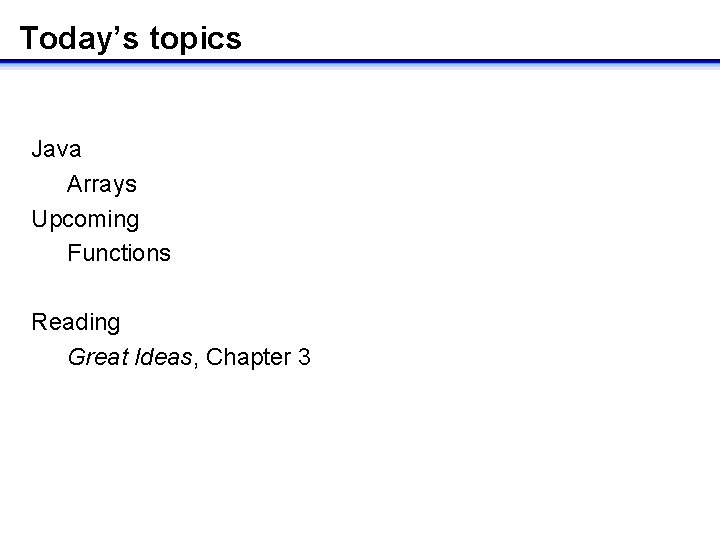
Today’s topics Java Arrays Upcoming Functions Reading Great Ideas, Chapter 3
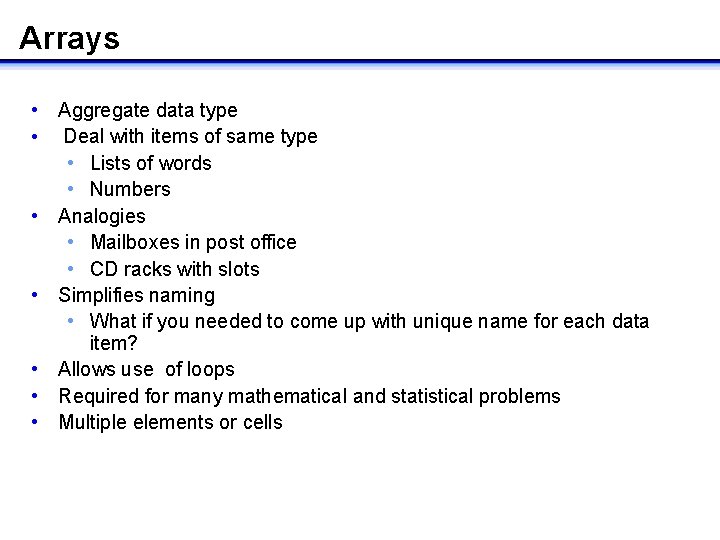
Arrays • Aggregate data type • Deal with items of same type • Lists of words • Numbers • Analogies • Mailboxes in post office • CD racks with slots • Simplifies naming • What if you needed to come up with unique name for each data item? • Allows use of loops • Required for many mathematical and statistical problems • Multiple elements or cells
![Using arrays Use subscript or index to access an element x5 20 Using arrays • Use subscript or index to access an element x[5] = 20;](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-3.jpg)
Using arrays • Use subscript or index to access an element x[5] = 20; foo. set. Text(“Result is " + x[5]); • First element is element 0, not 1!!! • Often used in loops int k = 0, sum = 0; while ( k < 10 ) { sum = sum + measurements[k]; k = k + 1; } • Note that subscript is a variable, k
![Creating Arrays Declaration double weights Definition weights new double50 Combine Creating Arrays • Declaration double weights[]; • Definition weights = new double[50]; • Combine](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-4.jpg)
Creating Arrays • Declaration double weights[]; • Definition weights = new double[50]; • Combine declaration and definition double weights[] = new double[50]; int num[] = new int[6]; ? ? ? num[1] = 21; num[5] = 13; ? 21 ? ? ? 13
![Arrays Loops num0 0 int k 2 whilek num length Arrays & Loops num[0] = 0; int k = 2; while(k < num. length)](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-5.jpg)
Arrays & Loops num[0] = 0; int k = 2; while(k < num. length) { num[k] = k * k; k = k + 1; } 0 21 4 9 16 25 • Subscript range errors! ! ! • Java checks (many languages do not) • Costs & tradeoffs
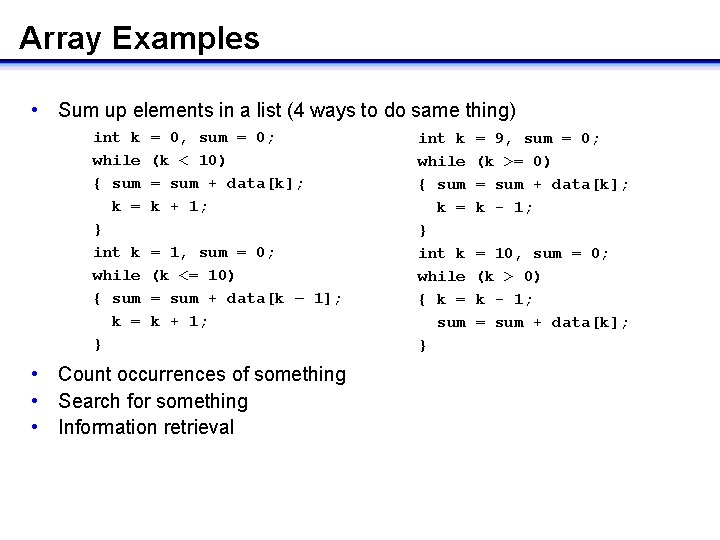
Array Examples • Sum up elements in a list (4 ways to do same thing) int k = 0, sum = 0; int k = 9, sum = 0; while (k < 10) while (k >= 0) { sum = sum + data[k]; k = k + 1; k = k - 1; } } int k = 1, sum = 0; int k = 10, sum = 0; while (k <= 10) while (k > 0) { sum = sum + data[k – 1]; { k = k - 1; k = k + 1; sum = sum + data[k]; } } • Count occurrences of something • Search for something • Information retrieval
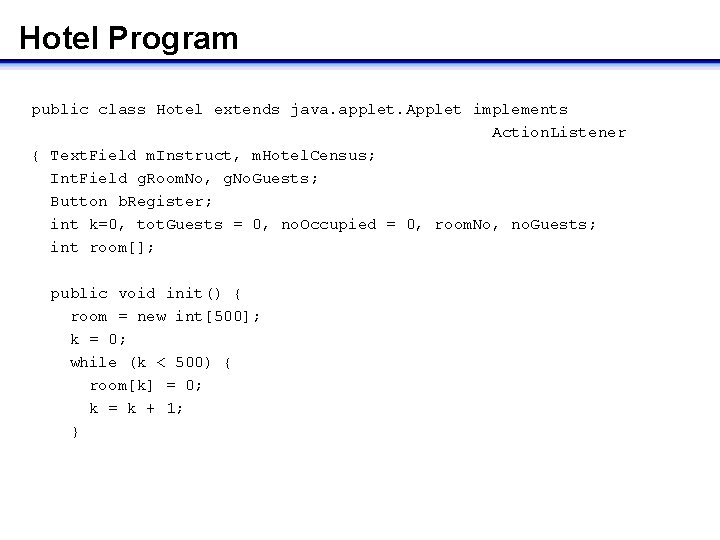
Hotel Program public class Hotel extends java. applet. Applet implements Action. Listener { Text. Field m. Instruct, m. Hotel. Census; Int. Field g. Room. No, g. No. Guests; Button b. Register; int k=0, tot. Guests = 0, no. Occupied = 0, room. No, no. Guests; int room[]; public void init() { room = new int[500]; k = 0; while (k < 500) { room[k] = 0; k = k + 1; }
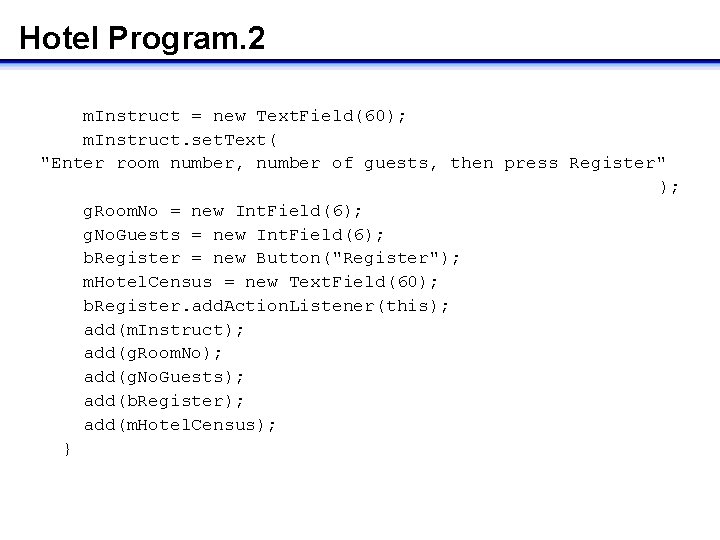
Hotel Program. 2 m. Instruct = new Text. Field(60); m. Instruct. set. Text( "Enter room number, number of guests, then press Register" ); g. Room. No = new Int. Field(6); g. No. Guests = new Int. Field(6); b. Register = new Button("Register"); m. Hotel. Census = new Text. Field(60); b. Register. add. Action. Listener(this); add(m. Instruct); add(g. Room. No); add(g. No. Guests); add(b. Register); add(m. Hotel. Census); }
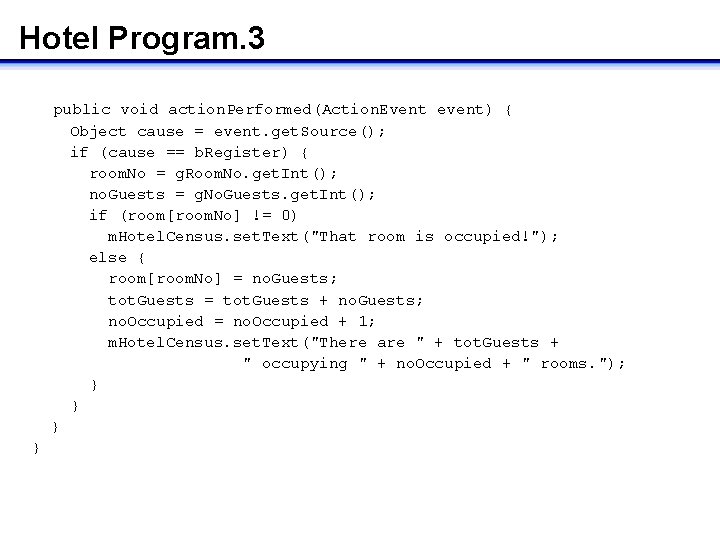
Hotel Program. 3 public void action. Performed(Action. Event event) { Object cause = event. get. Source(); if (cause == b. Register) { room. No = g. Room. No. get. Int(); no. Guests = g. No. Guests. get. Int(); if (room[room. No] != 0) m. Hotel. Census. set. Text("That room is occupied!"); else { room[room. No] = no. Guests; tot. Guests = tot. Guests + no. Guests; no. Occupied = no. Occupied + 1; m. Hotel. Census. set. Text("There are " + tot. Guests + " occupying " + no. Occupied + " rooms. "); } }
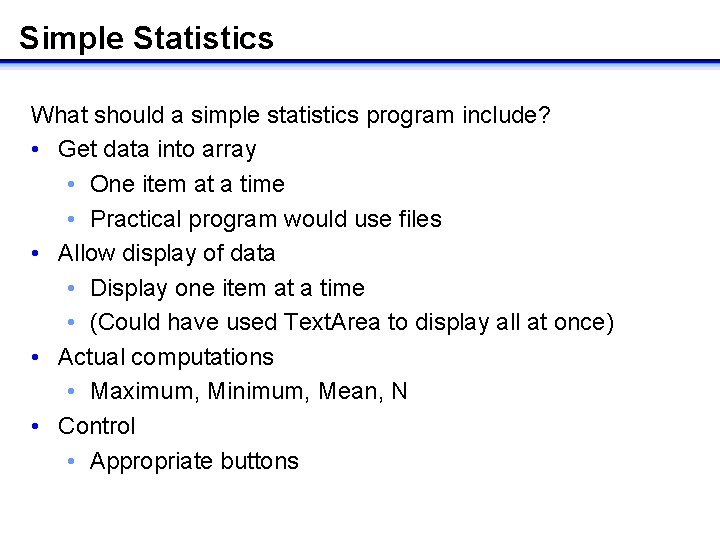
Simple Statistics What should a simple statistics program include? • Get data into array • One item at a time • Practical program would use files • Allow display of data • Display one item at a time • (Could have used Text. Area to display all at once) • Actual computations • Maximum, Minimum, Mean, N • Control • Appropriate buttons
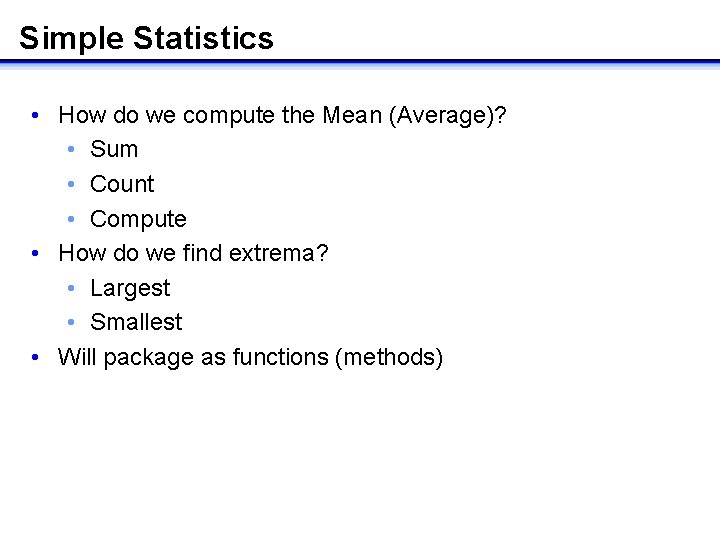
Simple Statistics • How do we compute the Mean (Average)? • Sum • Count • Compute • How do we find extrema? • Largest • Smallest • Will package as functions (methods)
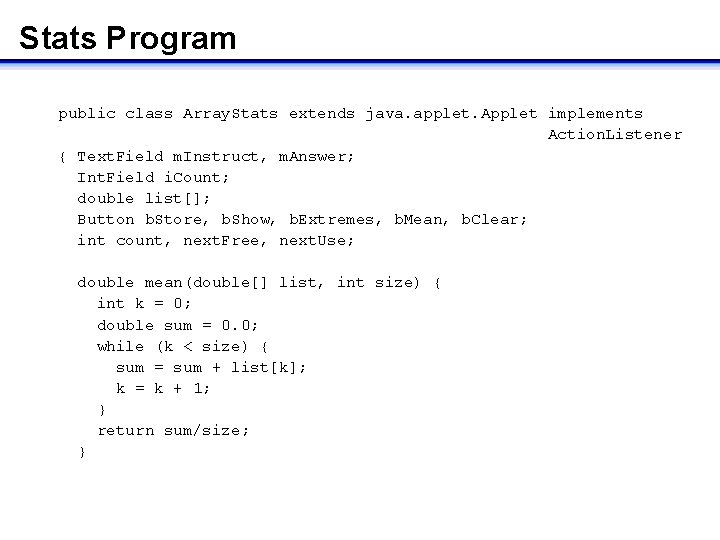
Stats Program public class Array. Stats extends java. applet. Applet implements Action. Listener { Text. Field m. Instruct, m. Answer; Int. Field i. Count; double list[]; Button b. Store, b. Show, b. Extremes, b. Mean, b. Clear; int count, next. Free, next. Use; double mean(double[] list, int size) { int k = 0; double sum = 0. 0; while (k < size) { sum = sum + list[k]; k = k + 1; } return sum/size; }
![Stats Program 2 double maxdouble list int size int k 1 double Stats Program. 2 double max(double[] list, int size) { int k = 1; double](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-13.jpg)
Stats Program. 2 double max(double[] list, int size) { int k = 1; double largest = list[0]; while (k < size) { if (list[k] > largest) { largest = list[k]; } k = k + 1; } return largest; }
![Stats Program 3 double mindouble list int size int k 1 double Stats Program. 3 double min(double[] list, int size) { int k = 1; double](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-14.jpg)
Stats Program. 3 double min(double[] list, int size) { int k = 1; double smallest = list[0]; while (k < size) { if (list[k] < smallest) { smallest = list[k]; } k = k + 1; } return smallest; }
![Stats Program 4 public void init list new double100 m Instruct Stats Program. 4 public void init() { list = new double[100]; m. Instruct =](https://slidetodoc.com/presentation_image_h/372f4d1d2491bc2e9118b4bd6e05b53a/image-15.jpg)
Stats Program. 4 public void init() { list = new double[100]; m. Instruct = new Text. Field(70); m. Answer = new Text. Field(70); m. Instruct. set. Text("Enter Value, then press Store button"); i. Count = new Int. Field(10); b. Store = new Button("Store"); b. Show = new Button("Show"); b. Extremes = new Button("Extremes"); = new Button("Mean"); b. Mean b. Clear = new Button("Clear"); next. Free = 0; next. Use = 0; b. Store. add. Action. Listener(this); b. Show. add. Action. Listener(this); b. Extremes. add. Action. Listener(this); b. Mean. add. Action. Listener(this); b. Clear. add. Action. Listener(this); add(m. Instruct); add(i. Count); add(b. Store); add(b. Show); add(b. Extremes); add(b. Mean); add(b. Clear); add(m. Answer); }
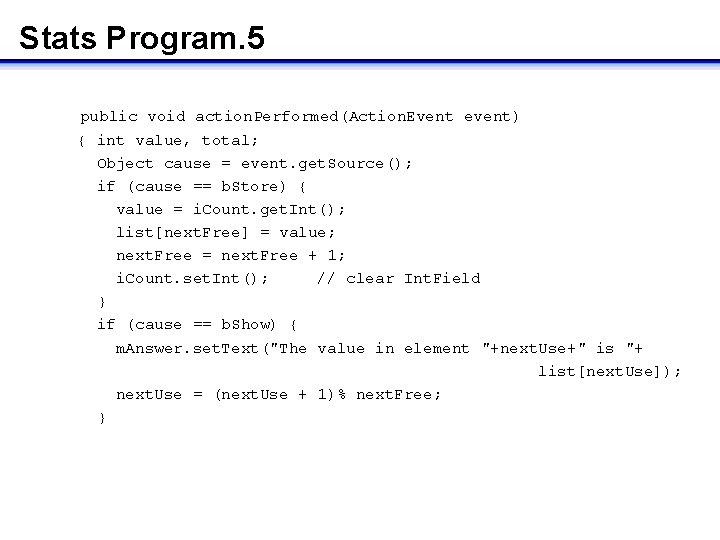
Stats Program. 5 public void action. Performed(Action. Event event) { int value, total; Object cause = event. get. Source(); if (cause == b. Store) { value = i. Count. get. Int(); list[next. Free] = value; next. Free = next. Free + 1; i. Count. set. Int(); // clear Int. Field } if (cause == b. Show) { m. Answer. set. Text("The value in element "+next. Use+" is "+ list[next. Use]); next. Use = (next. Use + 1)% next. Free; }
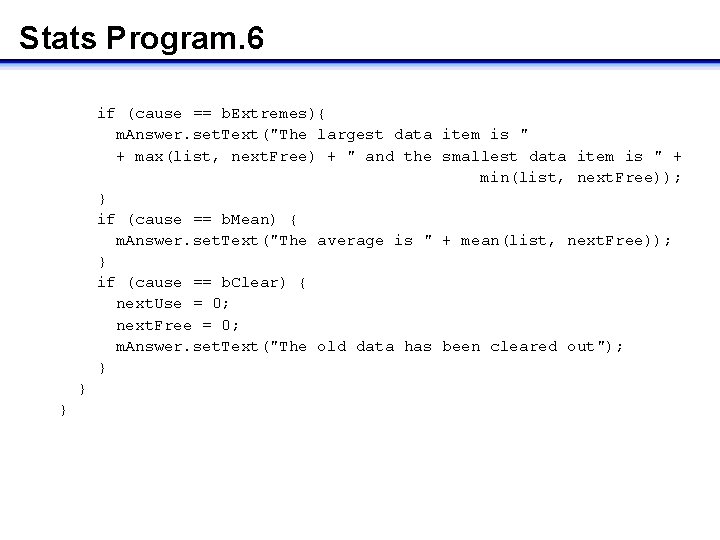
Stats Program. 6 if (cause == b. Extremes){ m. Answer. set. Text("The largest data item is " + max(list, next. Free) + " and the smallest data item is " + min(list, next. Free)); } if (cause == b. Mean) { m. Answer. set. Text("The average is " + mean(list, next. Free)); } if (cause == b. Clear) { next. Use = 0; next. Free = 0; m. Answer. set. Text("The old data has been cleared out"); } } }
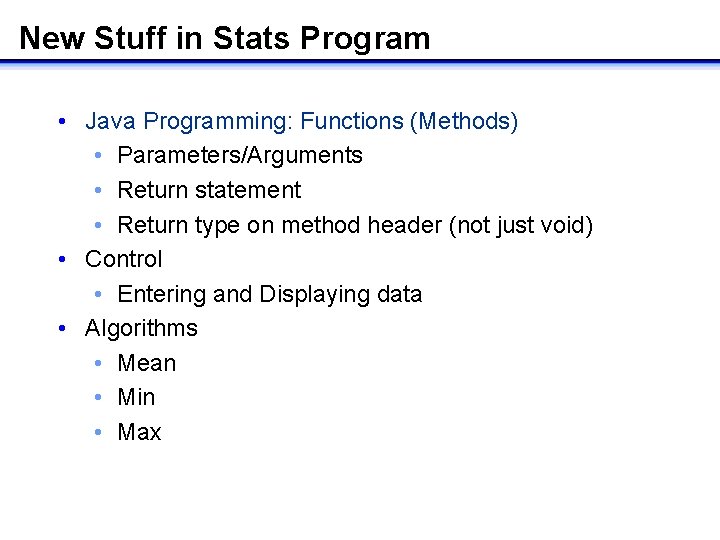
New Stuff in Stats Program • Java Programming: Functions (Methods) • Parameters/Arguments • Return statement • Return type on method header (not just void) • Control • Entering and Displaying data • Algorithms • Mean • Min • Max
Parallel arrays examples
Arreglo unidimensional java
Arreglos bidimensionales java
While reading activities
Reading for today topics 5
Theme of death in the great gatsby chapter 4
Http://www.history.com/topics/great-depression
Array of arrays c++
Java array operations
Partially filled arrays
Parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Array mips
Polynomial representation using arrays
Array of strings assembly
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++