Software Construction and Evolution CSSE 375 Defensive Programming
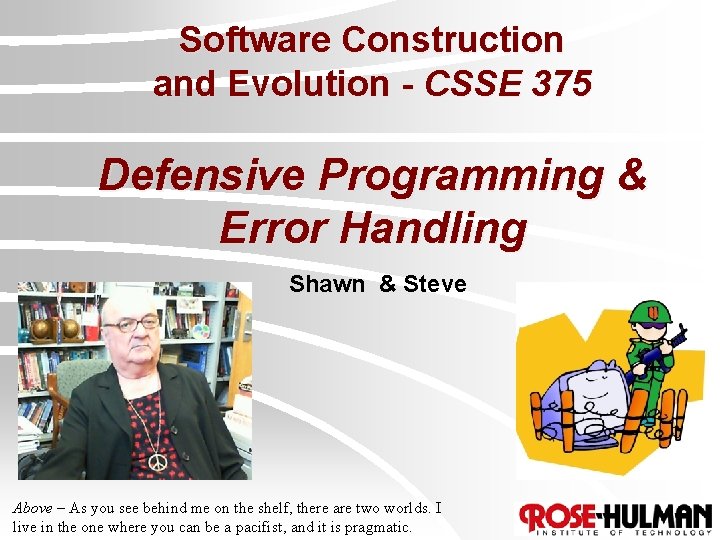
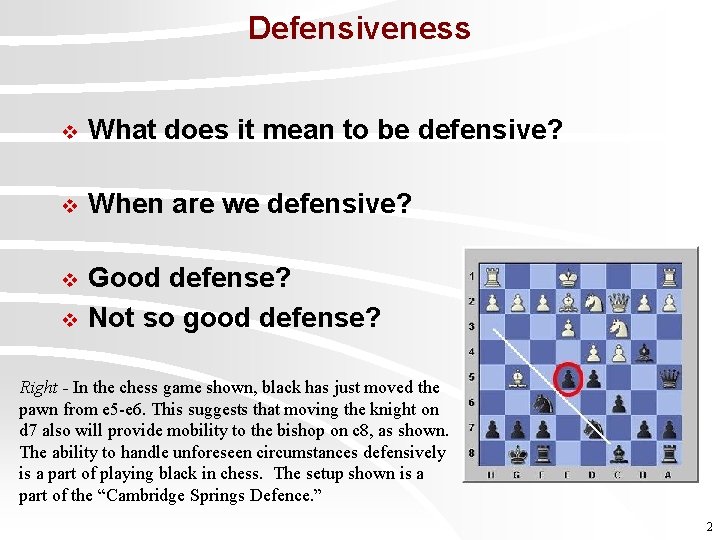
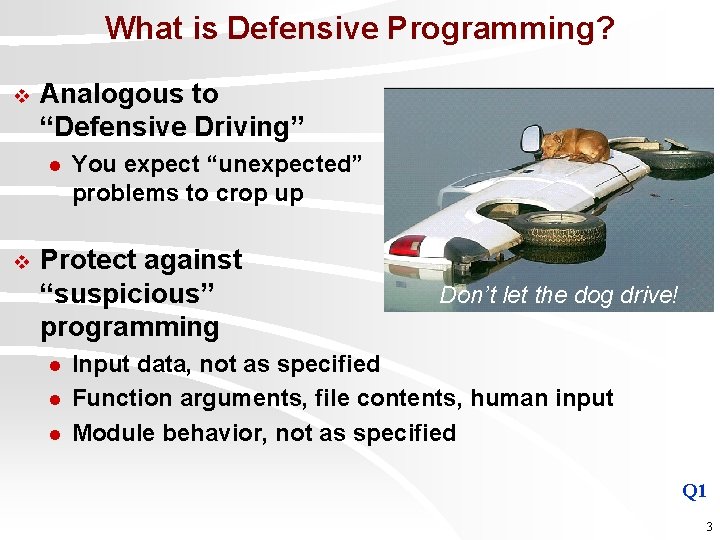
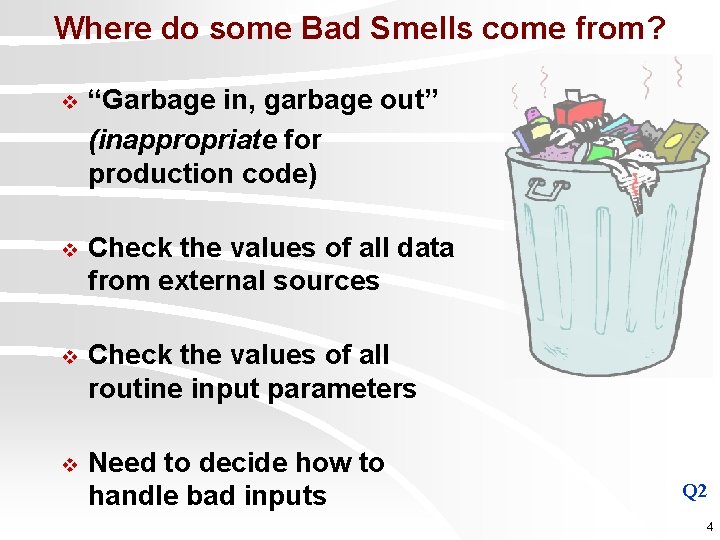
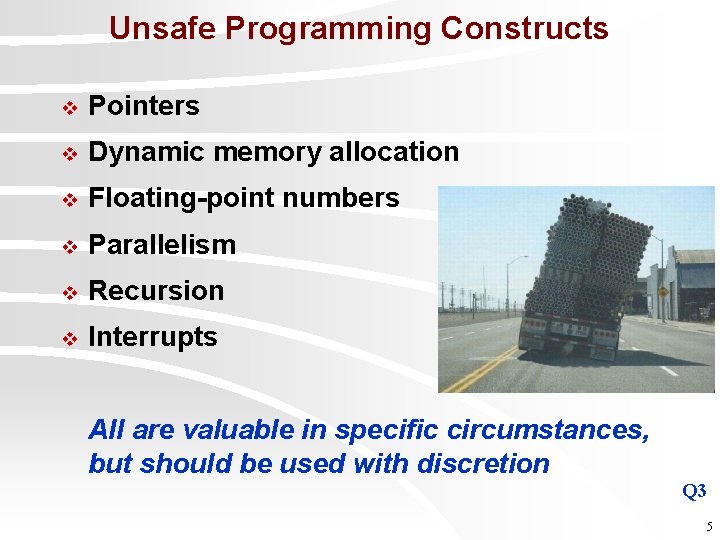
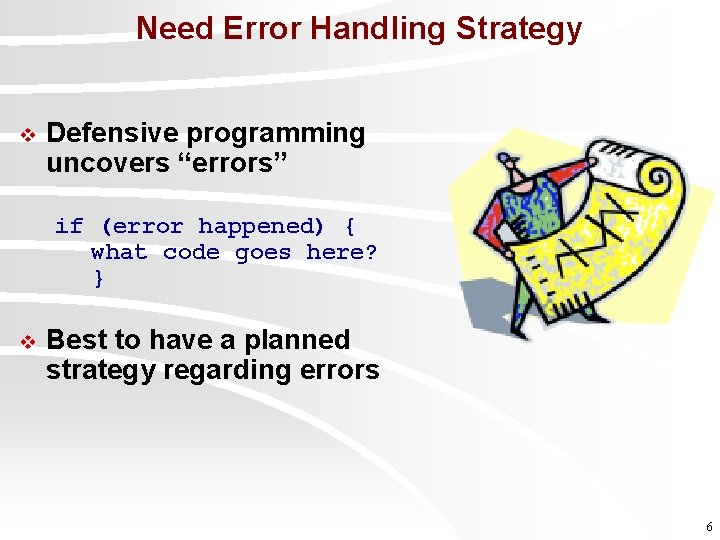
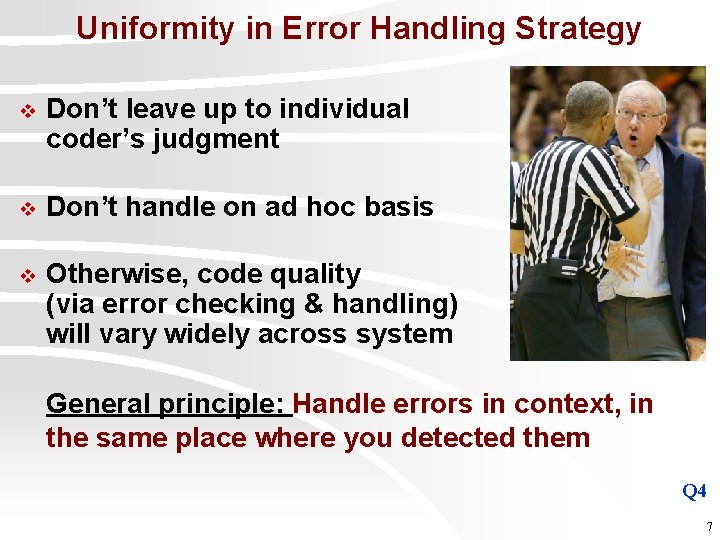
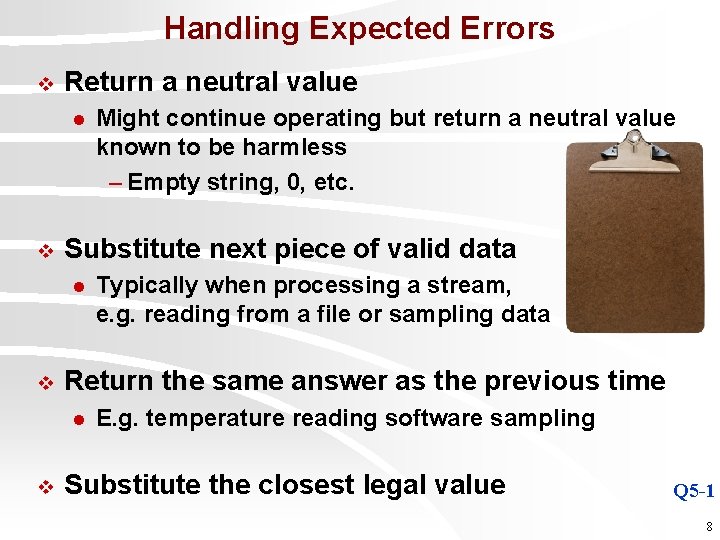
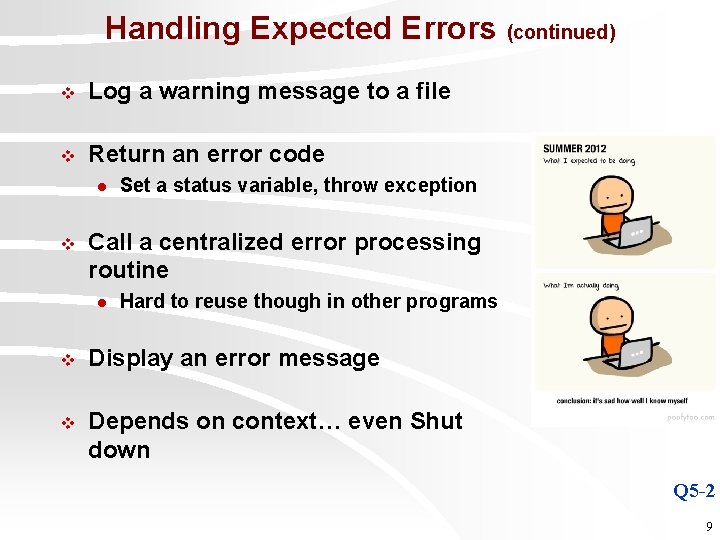
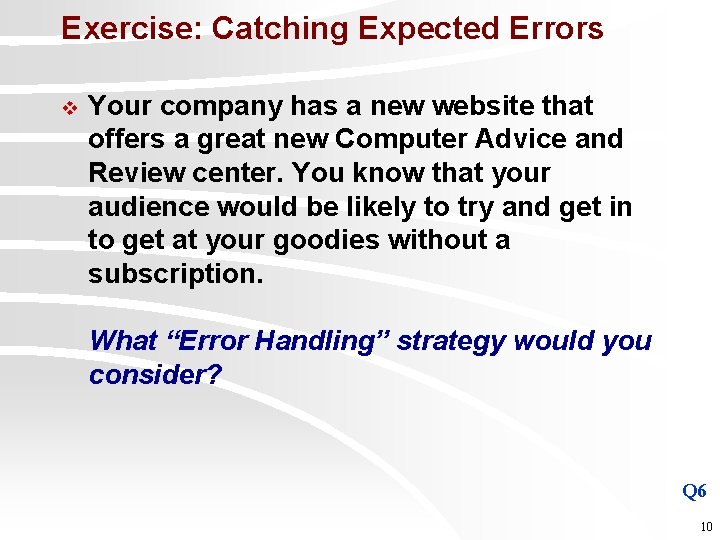
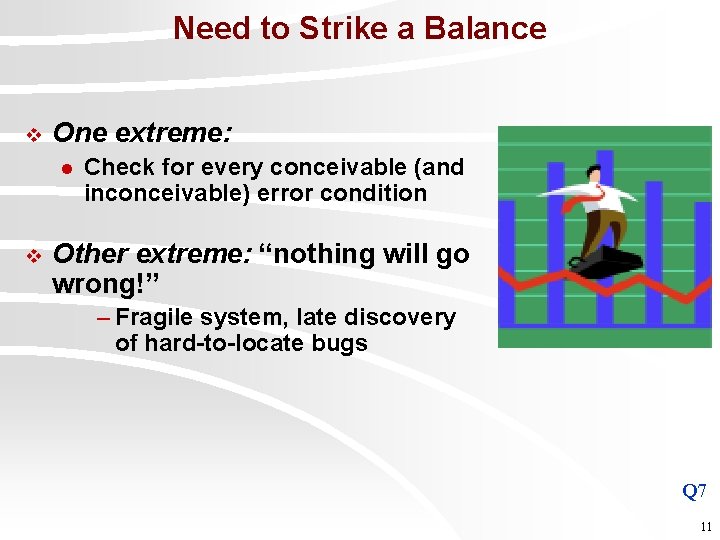
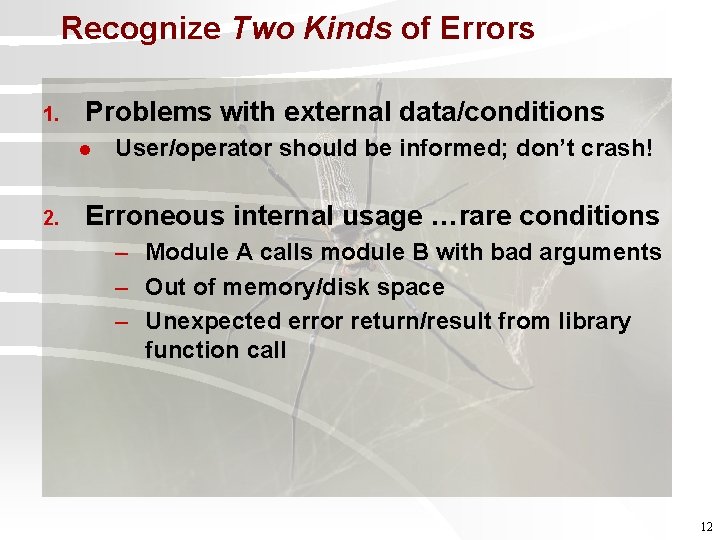
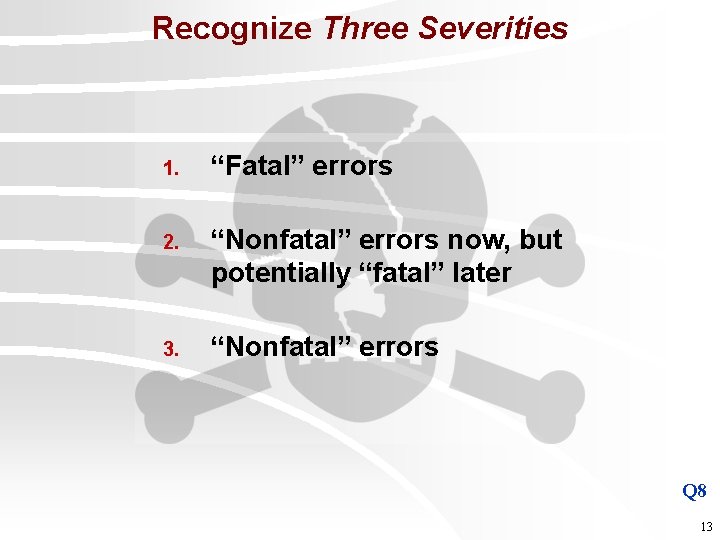
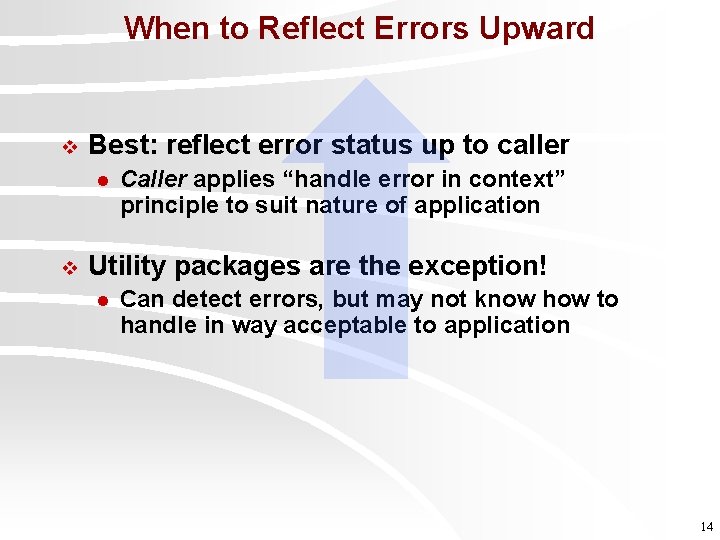
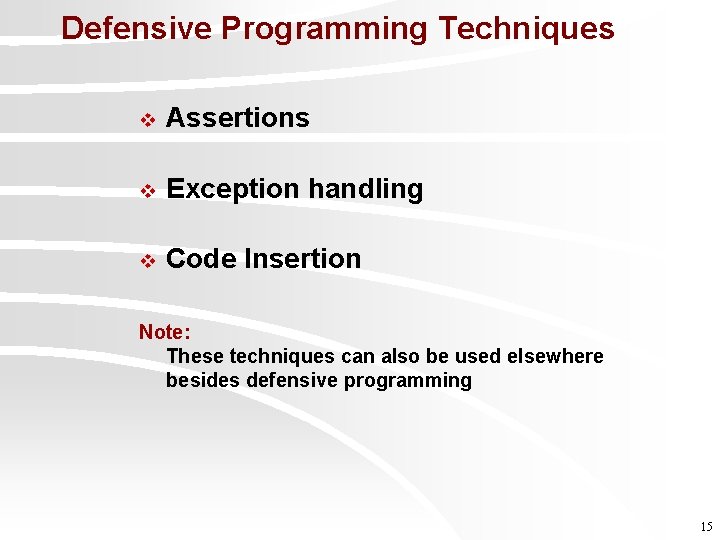
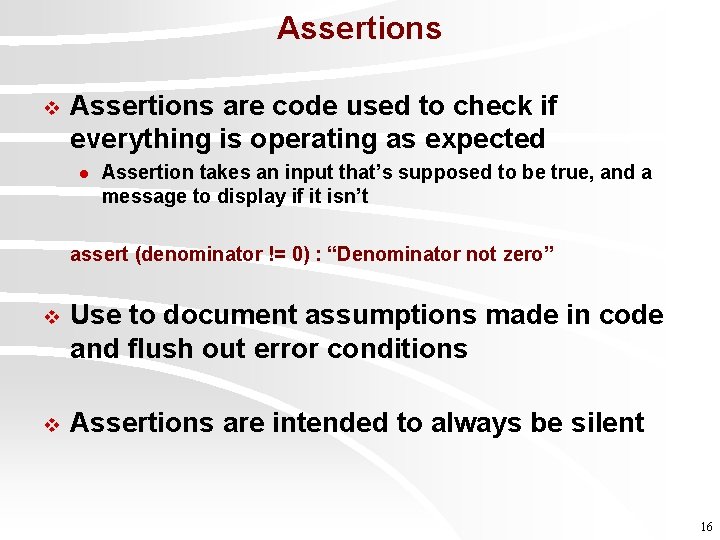
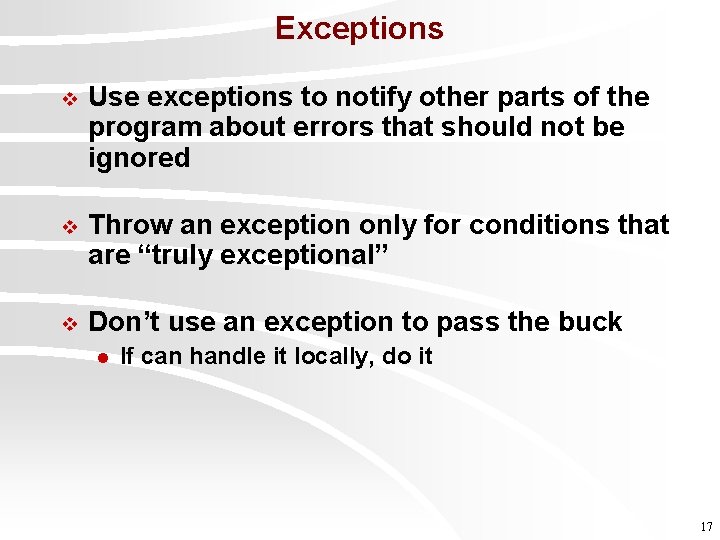
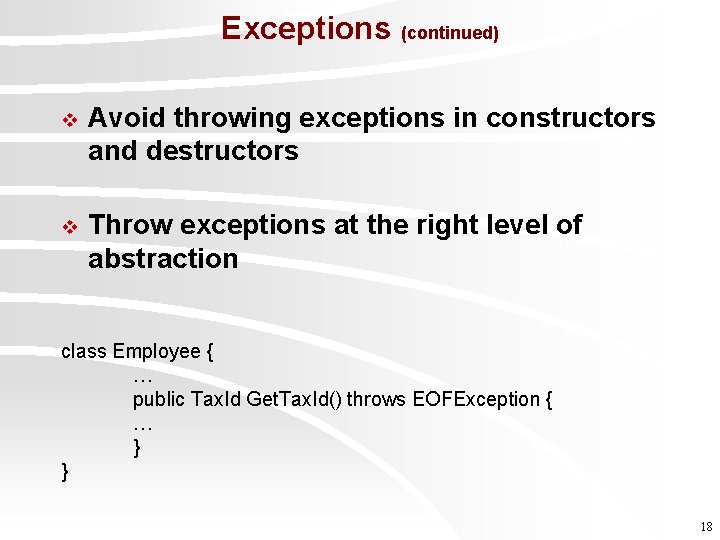
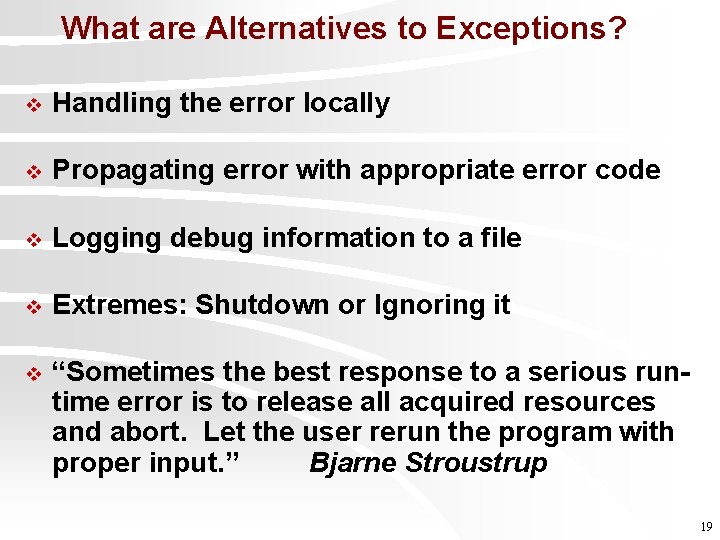
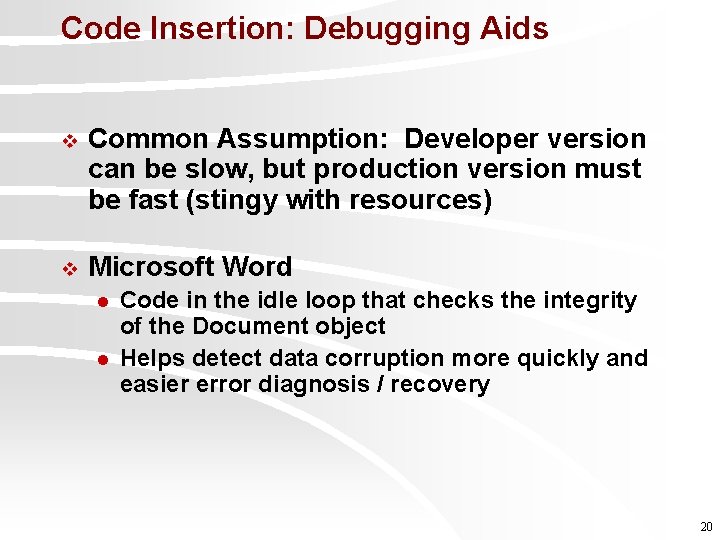
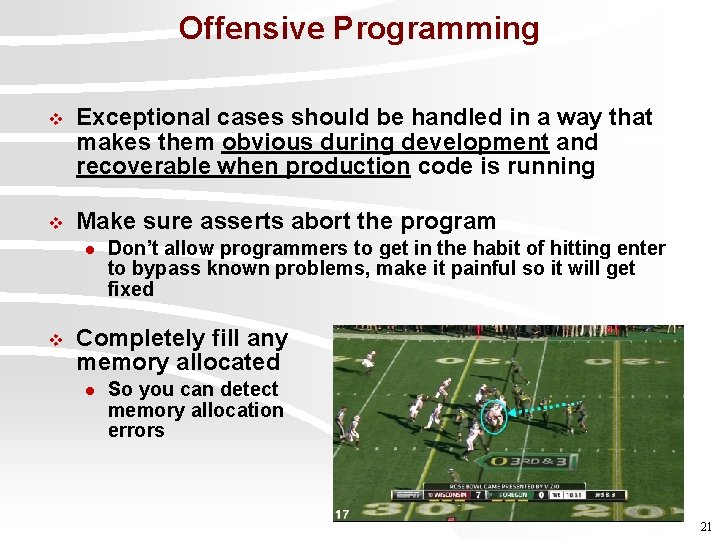
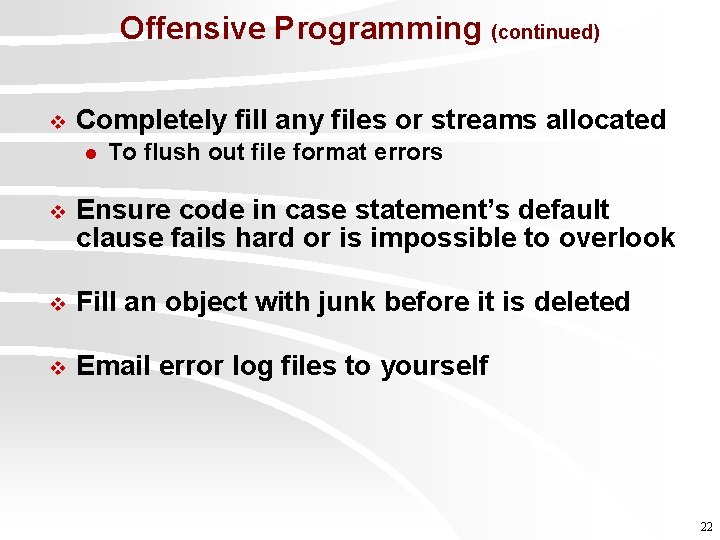
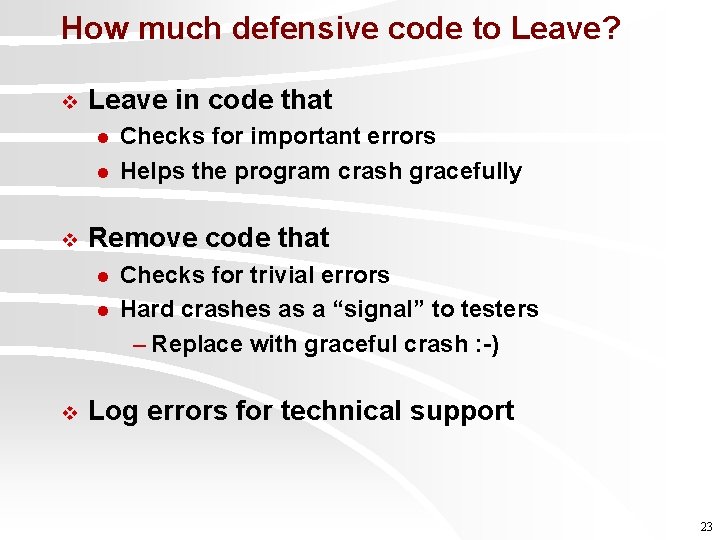
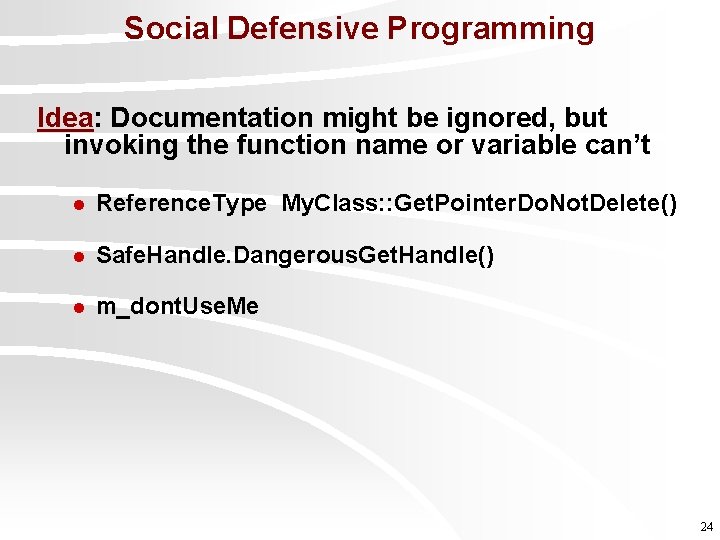
- Slides: 24
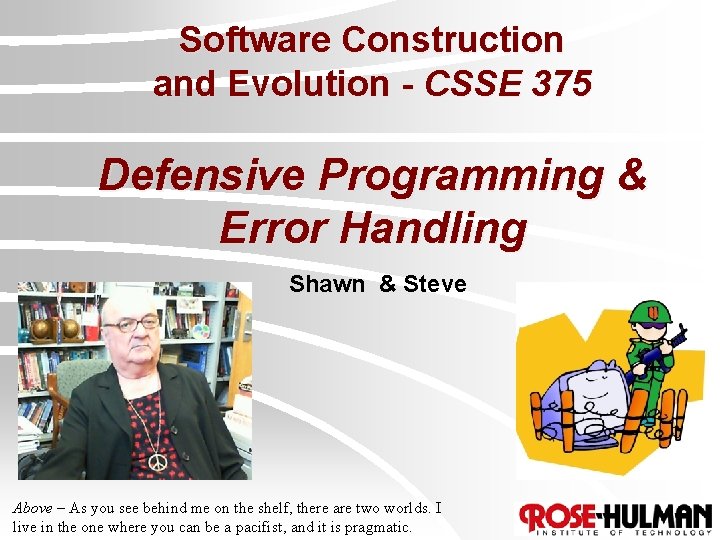
Software Construction and Evolution - CSSE 375 Defensive Programming & Error Handling Shawn & Steve Above – As you see behind me on the shelf, there are two worlds. I live in the one where you can be a pacifist, and it is pragmatic.
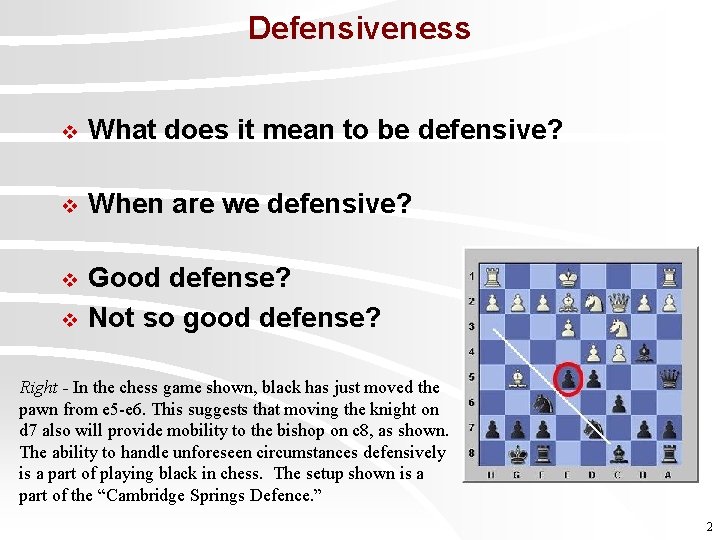
Defensiveness v What does it mean to be defensive? v When are we defensive? v Good defense? Not so good defense? v Right - In the chess game shown, black has just moved the pawn from e 5 -e 6. This suggests that moving the knight on d 7 also will provide mobility to the bishop on c 8, as shown. The ability to handle unforeseen circumstances defensively is a part of playing black in chess. The setup shown is a part of the “Cambridge Springs Defence. ” 2
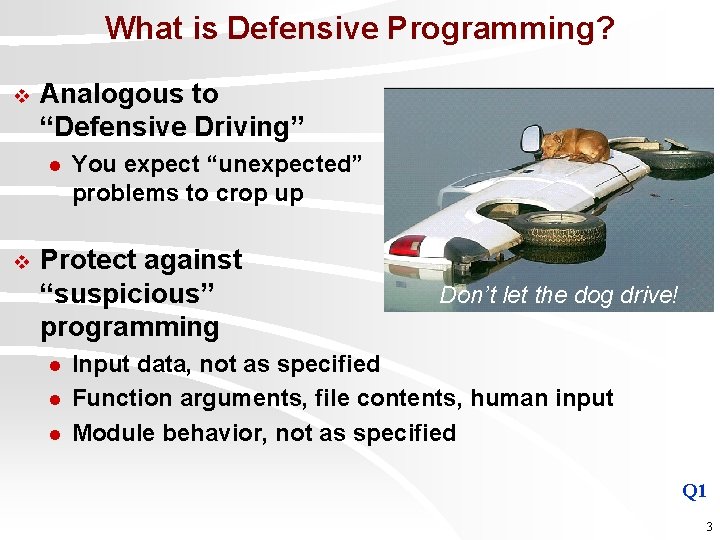
What is Defensive Programming? v Analogous to “Defensive Driving” l v You expect “unexpected” problems to crop up Protect against “suspicious” programming l l l Don’t let the dog drive! Input data, not as specified Function arguments, file contents, human input Module behavior, not as specified Q 1 3
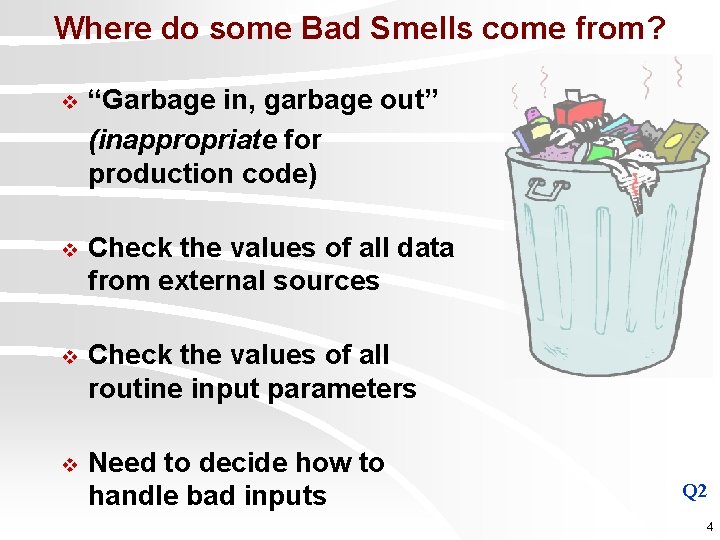
Where do some Bad Smells come from? v “Garbage in, garbage out” (inappropriate for production code) v Check the values of all data from external sources v Check the values of all routine input parameters v Need to decide how to handle bad inputs Q 2 4
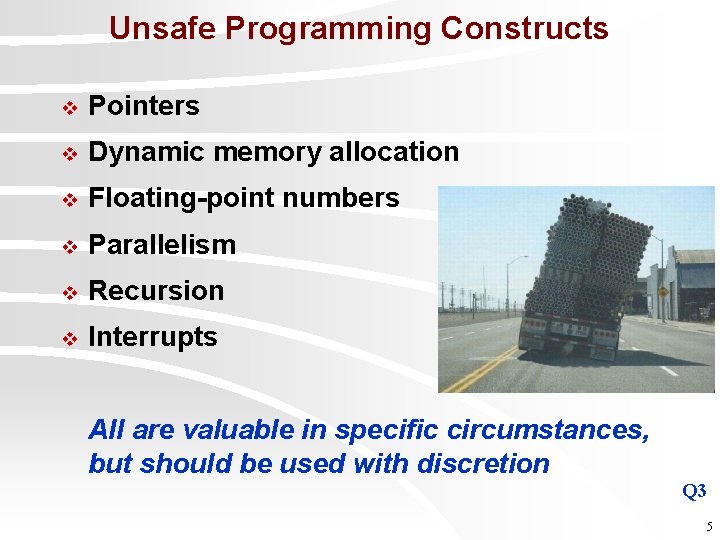
Unsafe Programming Constructs v Pointers v Dynamic memory allocation v Floating-point numbers v Parallelism v Recursion v Interrupts All are valuable in specific circumstances, but should be used with discretion Q 3 5
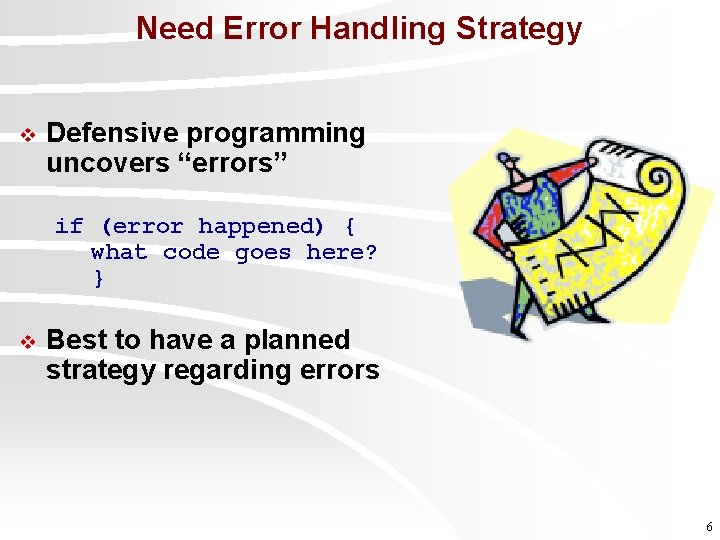
Need Error Handling Strategy v Defensive programming uncovers “errors” if (error happened) { what code goes here? } v Best to have a planned strategy regarding errors 6
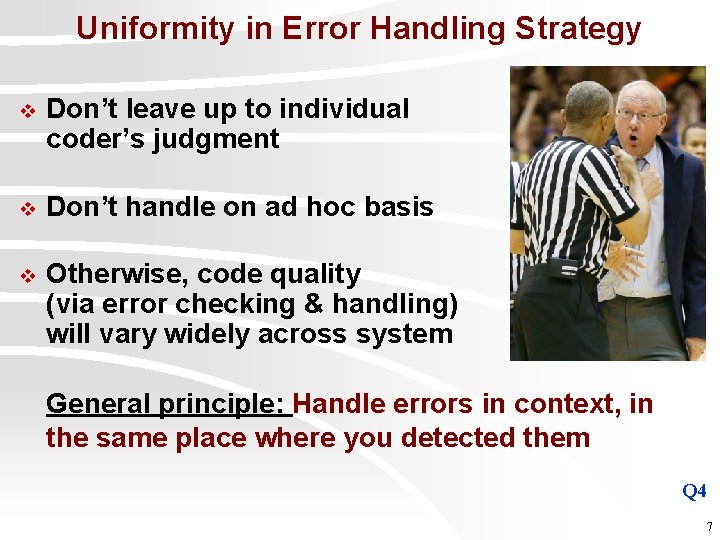
Uniformity in Error Handling Strategy v Don’t leave up to individual coder’s judgment v Don’t handle on ad hoc basis v Otherwise, code quality (via error checking & handling) will vary widely across system General principle: Handle errors in context, in the same place where you detected them Q 4 7
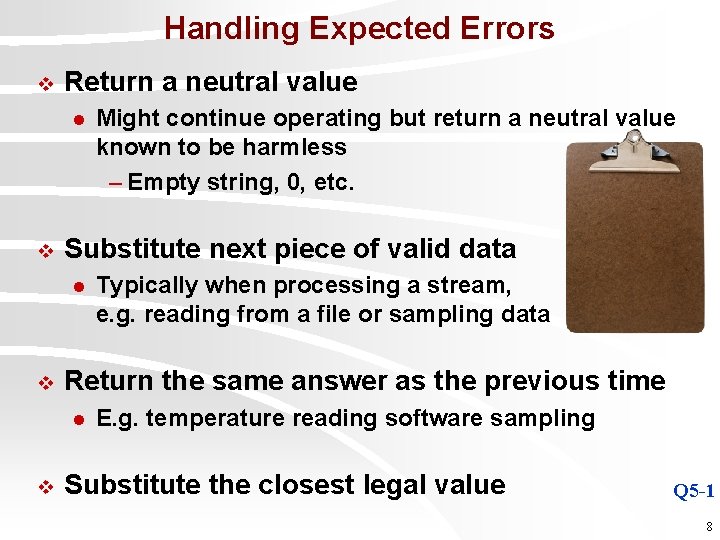
Handling Expected Errors v Return a neutral value l v Substitute next piece of valid data l v Typically when processing a stream, e. g. reading from a file or sampling data Return the same answer as the previous time l v Might continue operating but return a neutral value known to be harmless – Empty string, 0, etc. E. g. temperature reading software sampling Substitute the closest legal value Q 5 -1 8
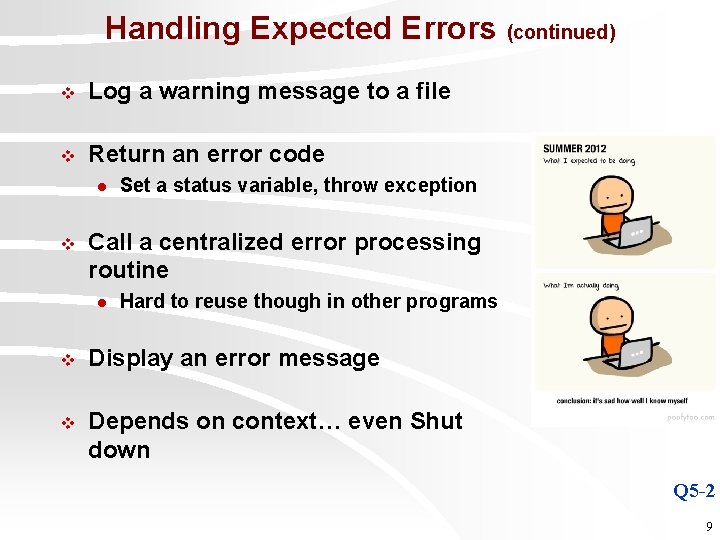
Handling Expected Errors (continued) v Log a warning message to a file v Return an error code l v Set a status variable, throw exception Call a centralized error processing routine l Hard to reuse though in other programs v Display an error message v Depends on context… even Shut down Q 5 -2 9
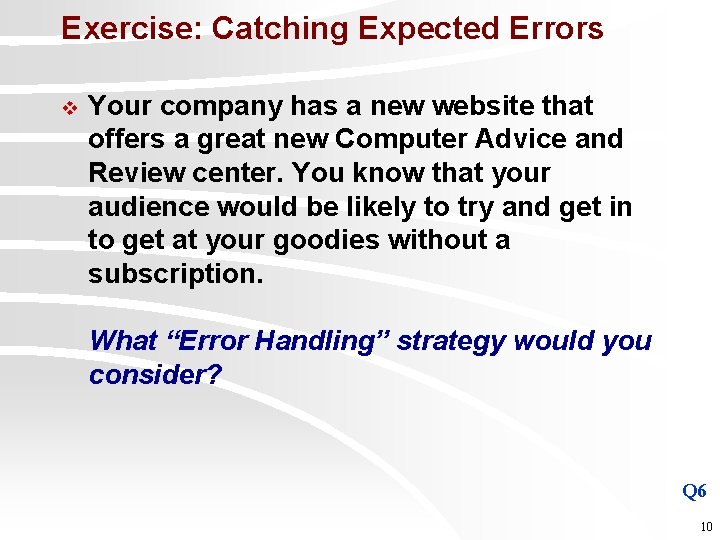
Exercise: Catching Expected Errors v Your company has a new website that offers a great new Computer Advice and Review center. You know that your audience would be likely to try and get in to get at your goodies without a subscription. What “Error Handling” strategy would you consider? Q 6 10
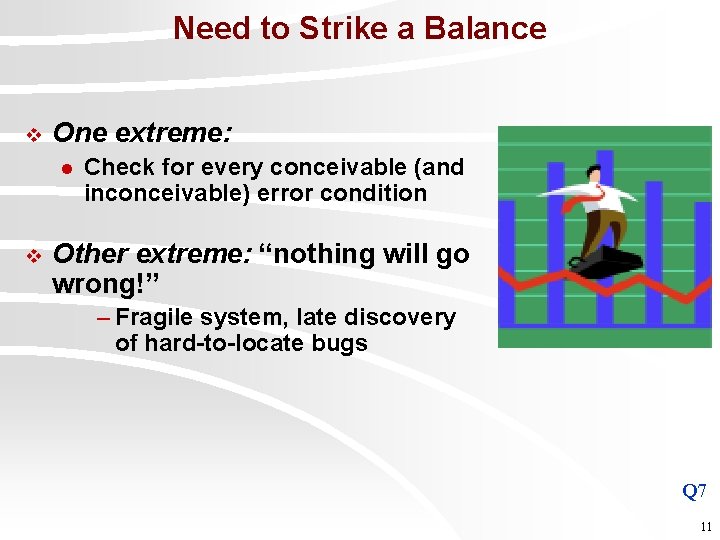
Need to Strike a Balance v One extreme: l v Check for every conceivable (and inconceivable) error condition Other extreme: “nothing will go wrong!” – Fragile system, late discovery of hard-to-locate bugs Q 7 11
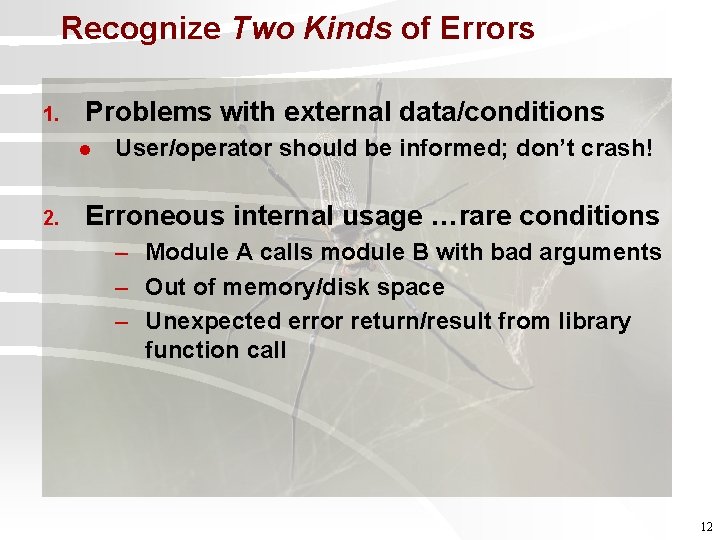
Recognize Two Kinds of Errors 1. Problems with external data/conditions l 2. User/operator should be informed; don’t crash! Erroneous internal usage …rare conditions – Module A calls module B with bad arguments – Out of memory/disk space – Unexpected error return/result from library function call 12
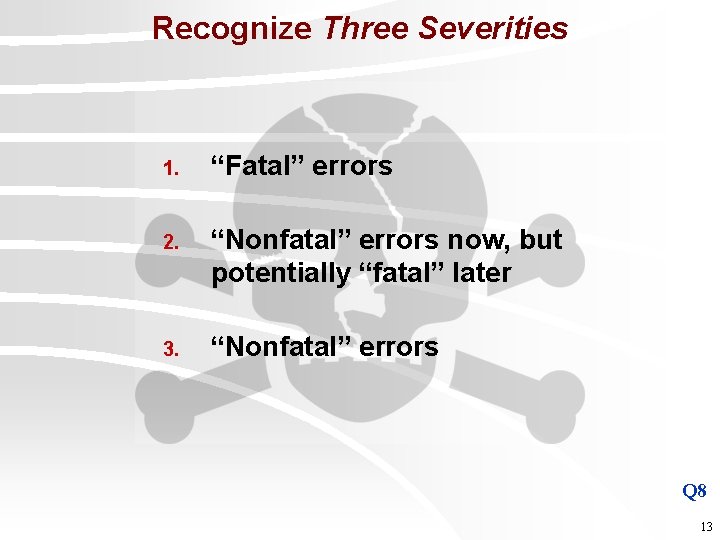
Recognize Three Severities 1. “Fatal” errors 2. “Nonfatal” errors now, but potentially “fatal” later 3. “Nonfatal” errors Q 8 13
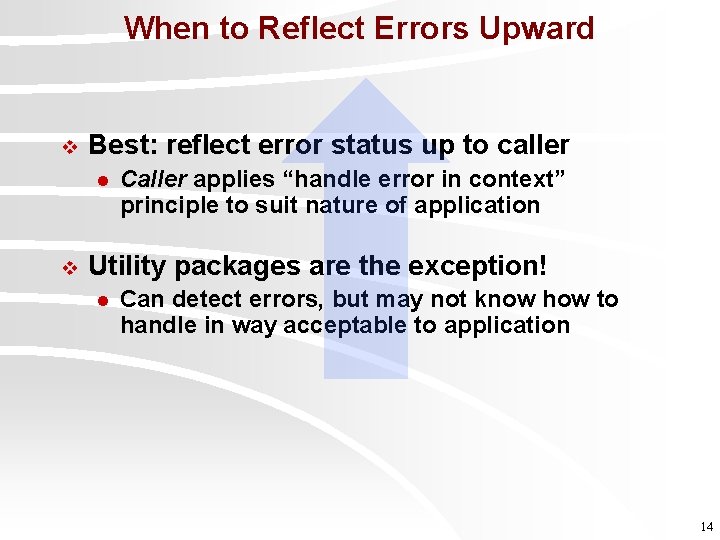
When to Reflect Errors Upward v Best: reflect error status up to caller l v Caller applies “handle error in context” principle to suit nature of application Utility packages are the exception! l Can detect errors, but may not know how to handle in way acceptable to application 14
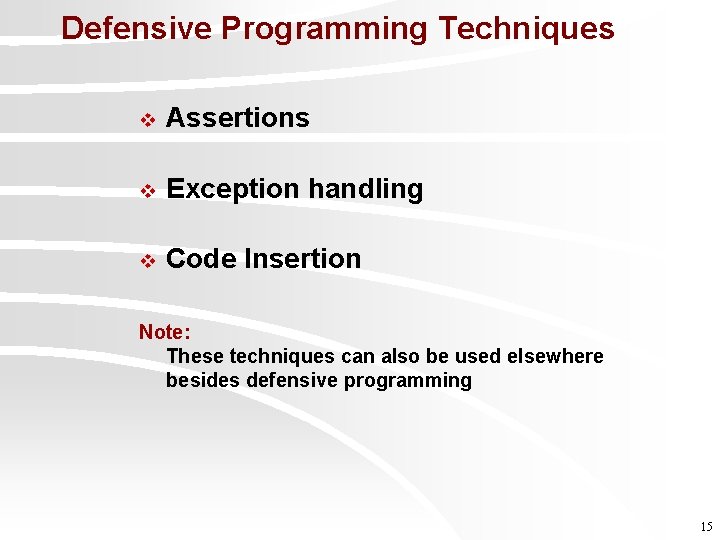
Defensive Programming Techniques v Assertions v Exception handling v Code Insertion Note: These techniques can also be used elsewhere besides defensive programming 15
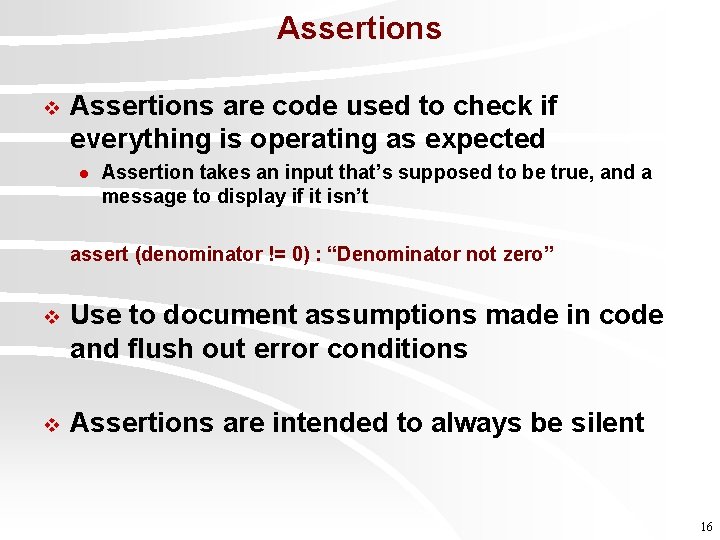
Assertions v Assertions are code used to check if everything is operating as expected l Assertion takes an input that’s supposed to be true, and a message to display if it isn’t assert (denominator != 0) : “Denominator not zero” v Use to document assumptions made in code and flush out error conditions v Assertions are intended to always be silent 16
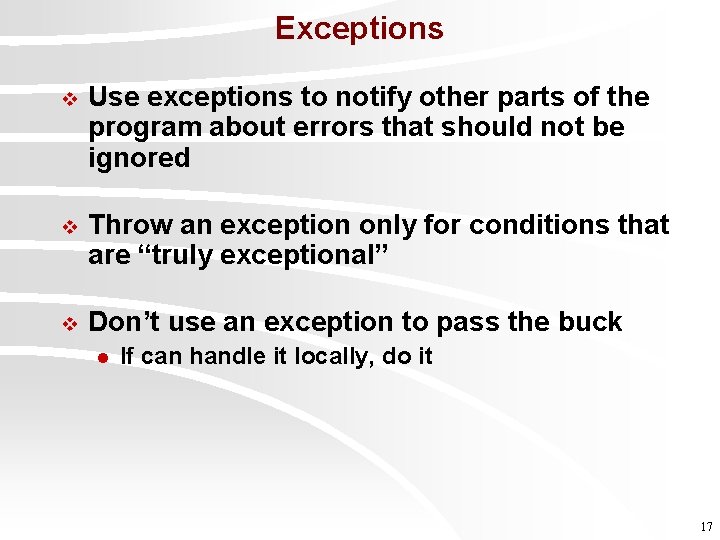
Exceptions v Use exceptions to notify other parts of the program about errors that should not be ignored v Throw an exception only for conditions that are “truly exceptional” v Don’t use an exception to pass the buck l If can handle it locally, do it 17
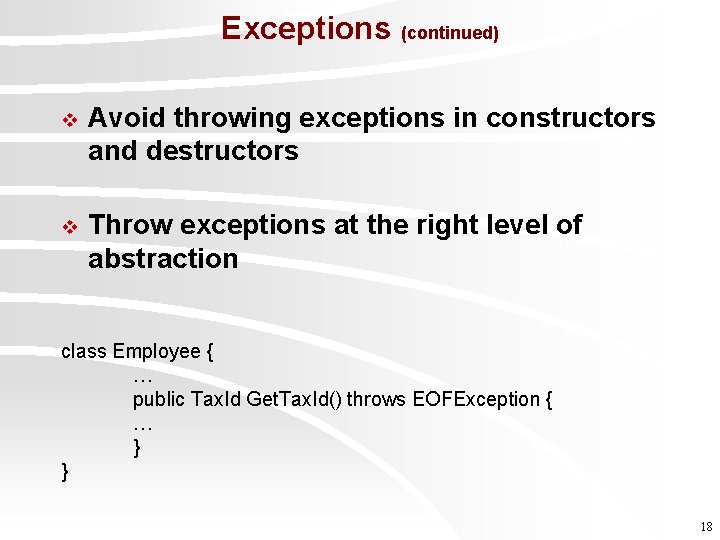
Exceptions (continued) v Avoid throwing exceptions in constructors and destructors v Throw exceptions at the right level of abstraction class Employee { … public Tax. Id Get. Tax. Id() throws EOFException { … } } 18
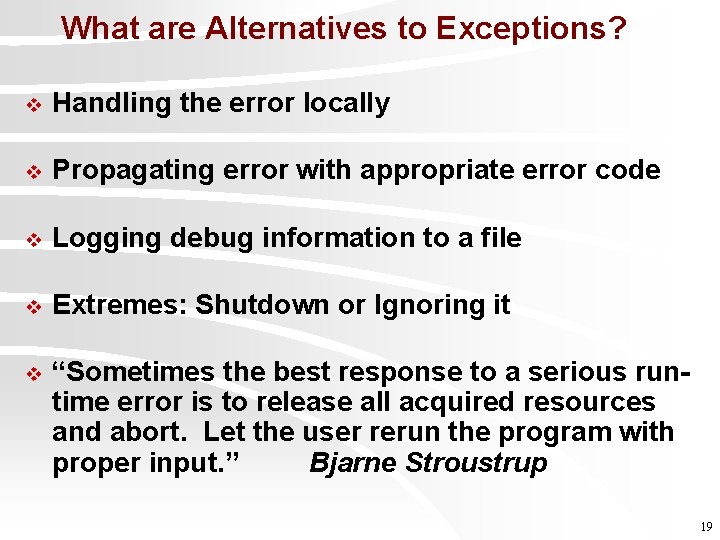
What are Alternatives to Exceptions? v Handling the error locally v Propagating error with appropriate error code v Logging debug information to a file v Extremes: Shutdown or Ignoring it v “Sometimes the best response to a serious runtime error is to release all acquired resources and abort. Let the user rerun the program with proper input. ” Bjarne Stroustrup 19
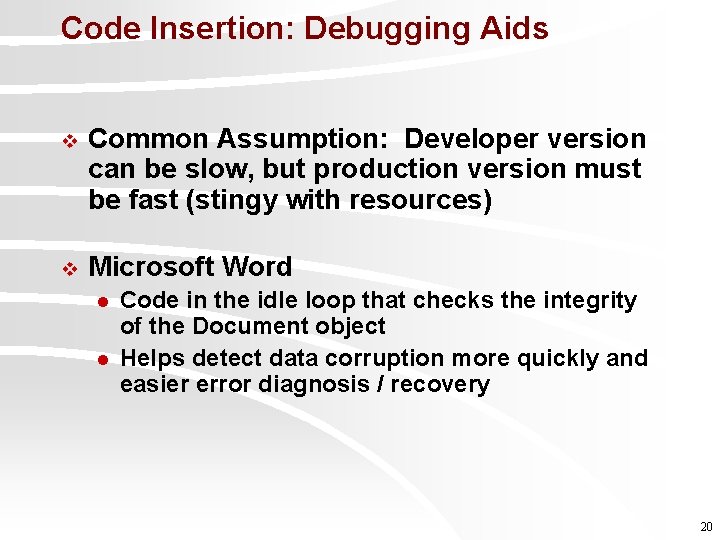
Code Insertion: Debugging Aids v Common Assumption: Developer version can be slow, but production version must be fast (stingy with resources) v Microsoft Word l l Code in the idle loop that checks the integrity of the Document object Helps detect data corruption more quickly and easier error diagnosis / recovery 20
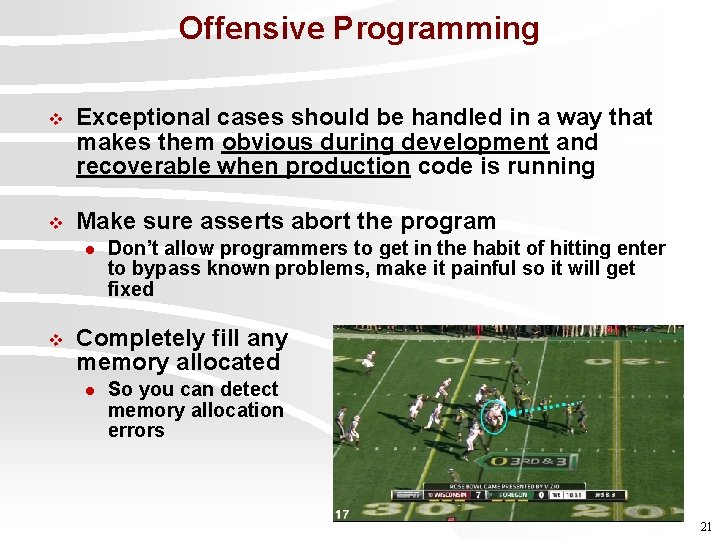
Offensive Programming v Exceptional cases should be handled in a way that makes them obvious during development and recoverable when production code is running v Make sure asserts abort the program l v Don’t allow programmers to get in the habit of hitting enter to bypass known problems, make it painful so it will get fixed Completely fill any memory allocated l So you can detect memory allocation errors 21
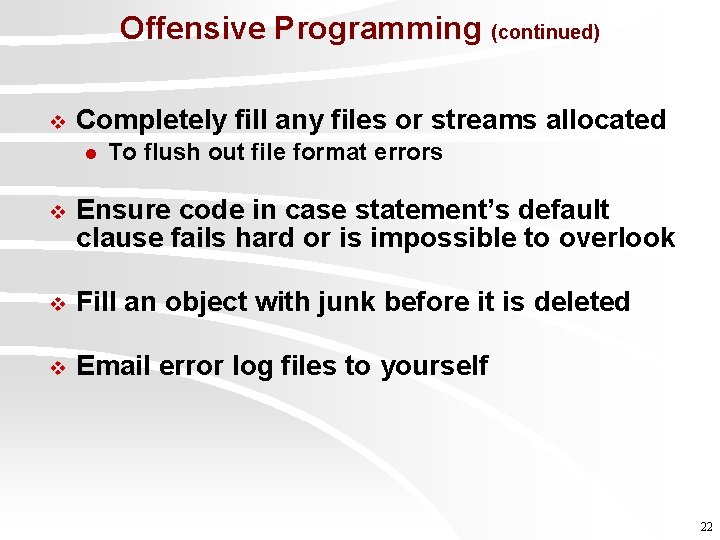
Offensive Programming (continued) v Completely fill any files or streams allocated l To flush out file format errors v Ensure code in case statement’s default clause fails hard or is impossible to overlook v Fill an object with junk before it is deleted v Email error log files to yourself 22
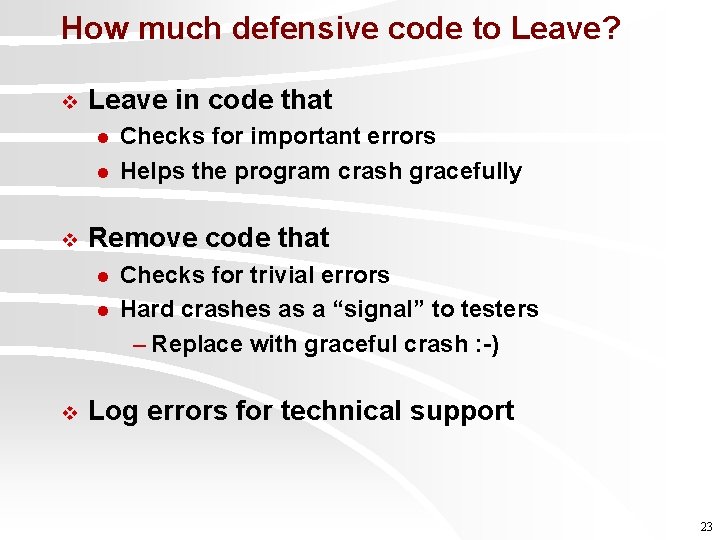
How much defensive code to Leave? v Leave in code that l l v Remove code that l l v Checks for important errors Helps the program crash gracefully Checks for trivial errors Hard crashes as a “signal” to testers – Replace with graceful crash : -) Log errors for technical support 23
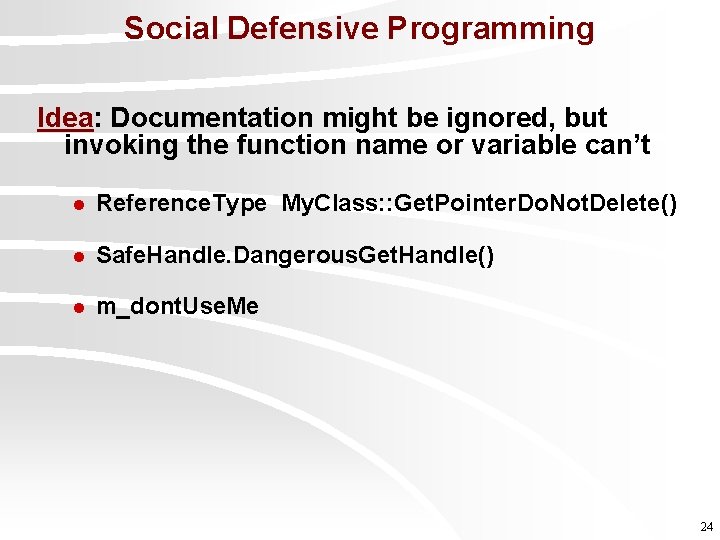
Social Defensive Programming Idea: Documentation might be ignored, but invoking the function name or variable can’t l Reference. Type My. Class: : Get. Pointer. Do. Not. Delete() l Safe. Handle. Dangerous. Get. Handle() l m_dont. Use. Me 24
Csse results
Software architecture cartoon
Representing identity in information security
Csse exam
Defensive programming java
Evolution construction software
Evolution construction software
Friendly armor battalion task force symbol
Rm 375-tr-2008 iluminacion tabla
Zavet 375
Zdjęcie 300 na 375 pikseli
Com(2018) 375 final
1400-375
Half wave plate matrix
Ludlum 375
Csc 375
Cis 375
Suku ke-4 barisan geometri adalah 375
Evolution of oops
Software maintenance process
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Components of system programming
Integer programming vs linear programming
Perbedaan linear programming dan integer programming