Repetition and Iteration ANSIC Repetition We need a
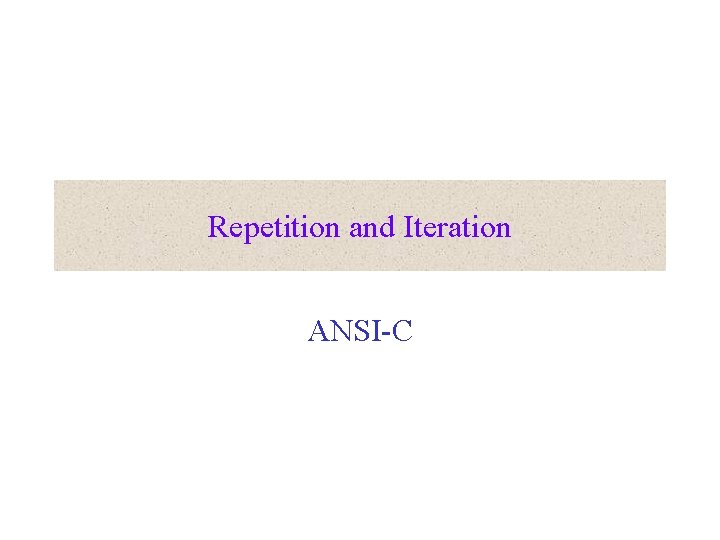
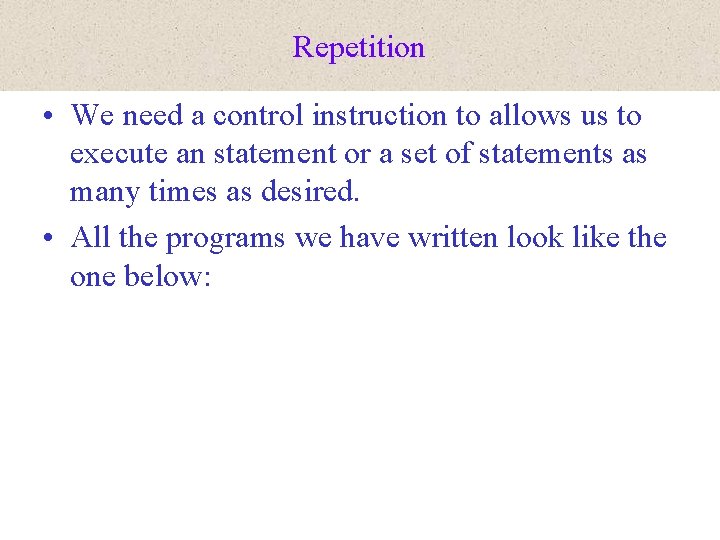
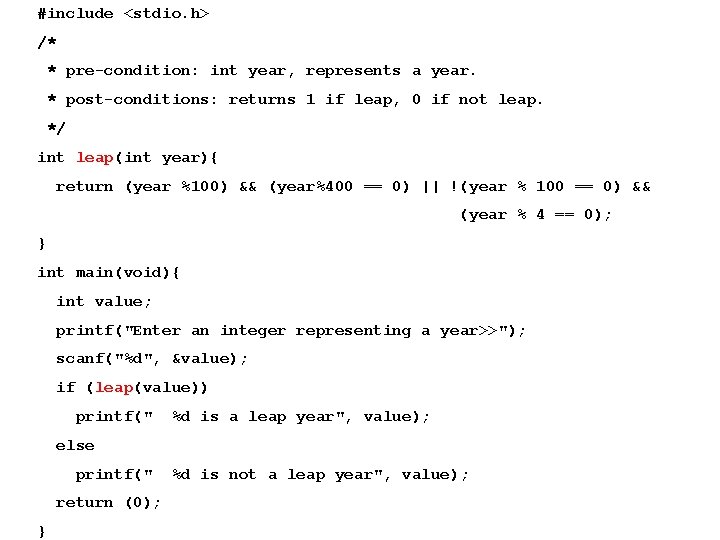
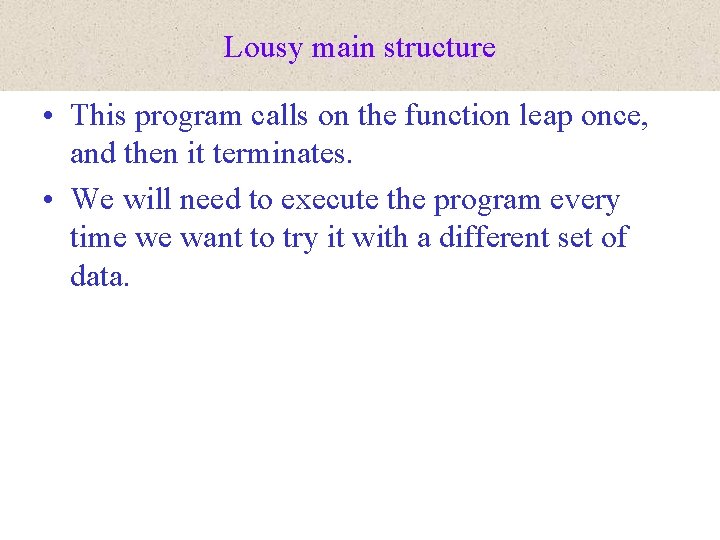
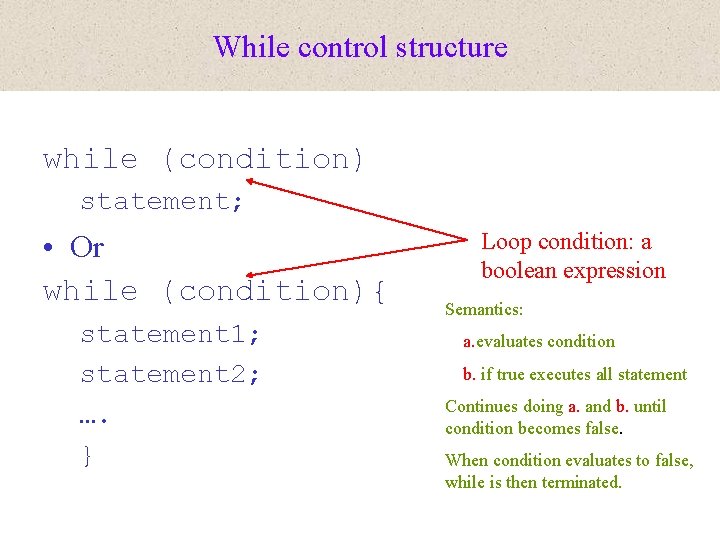
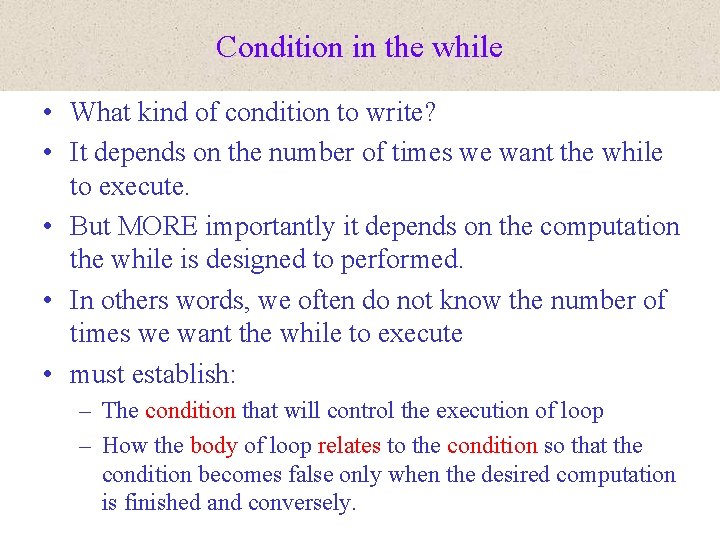
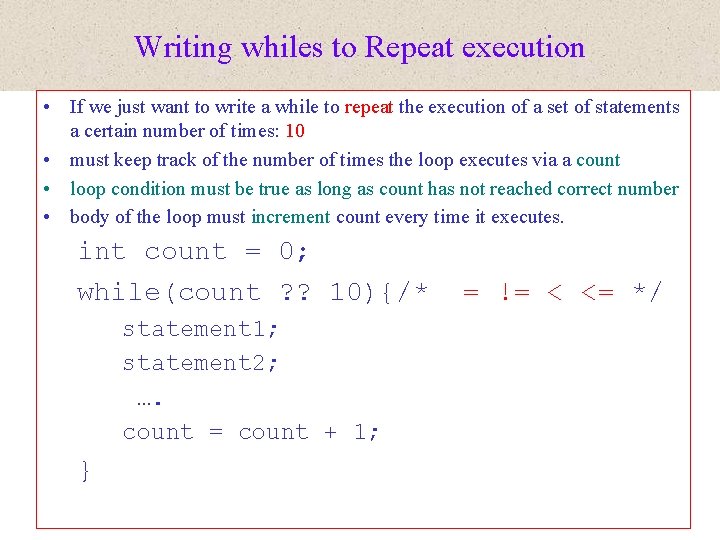
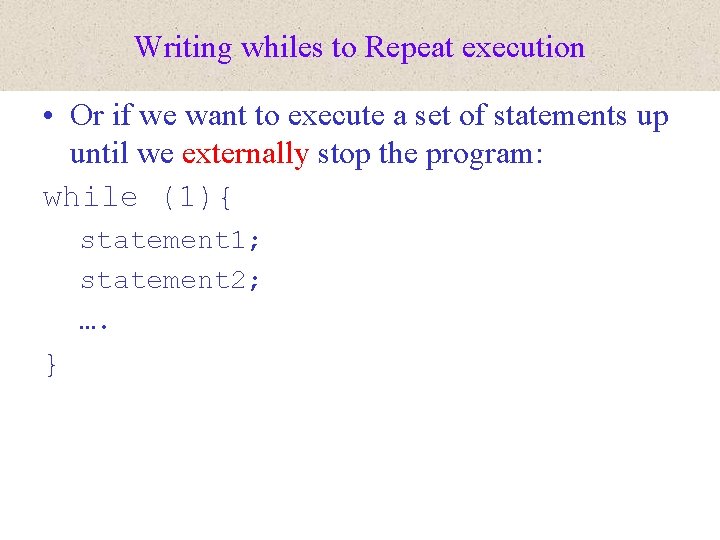
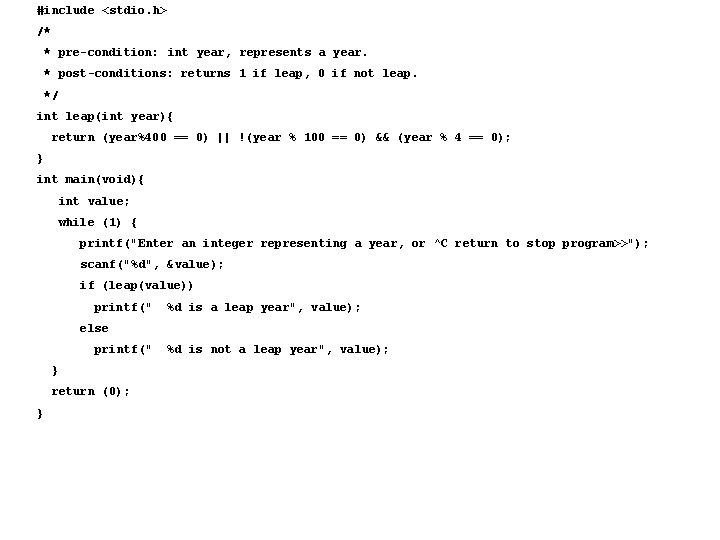
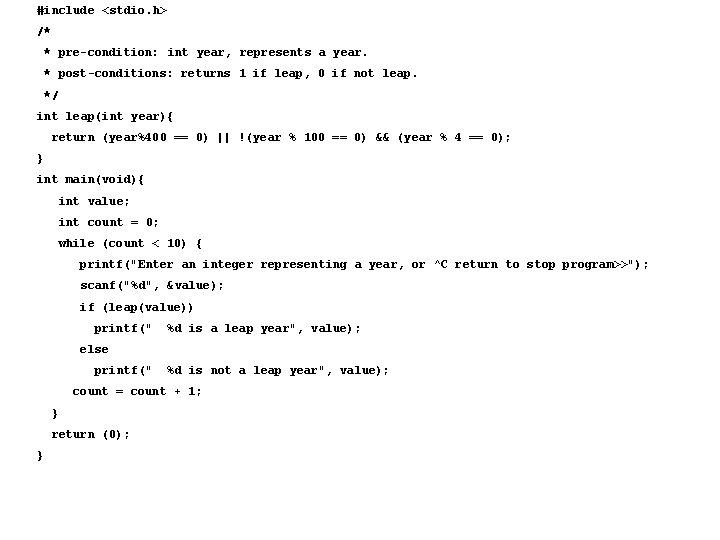
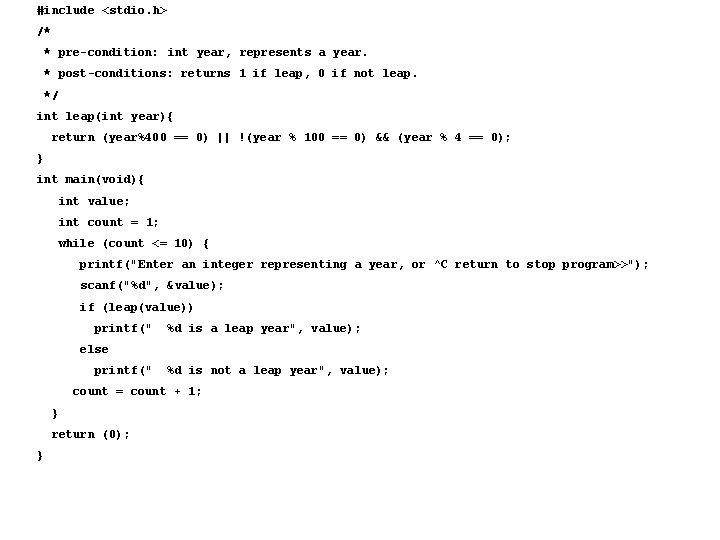
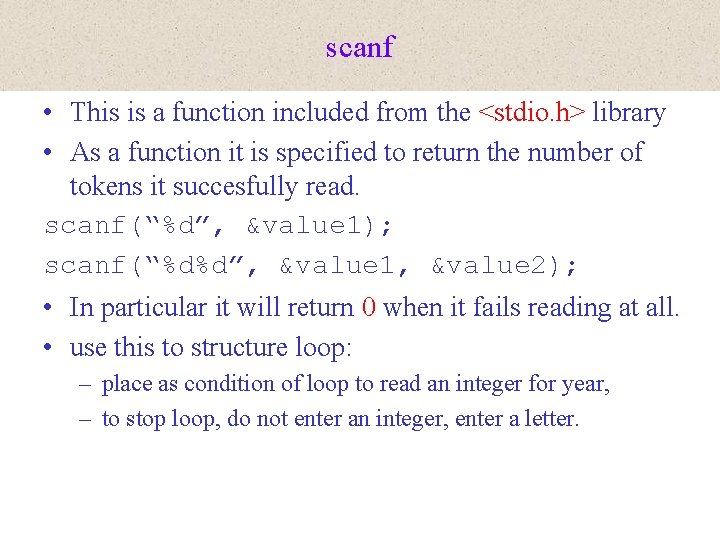
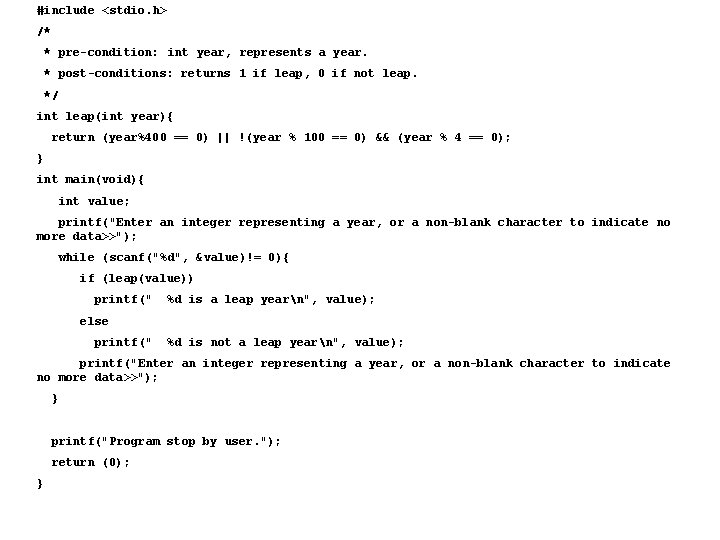
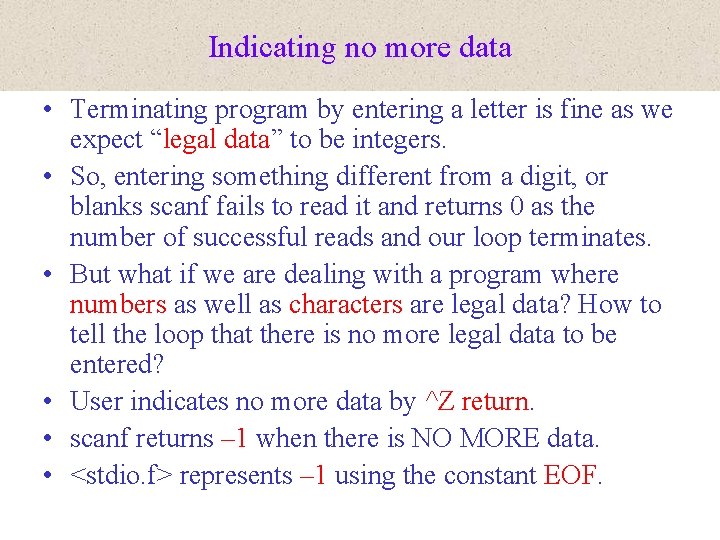
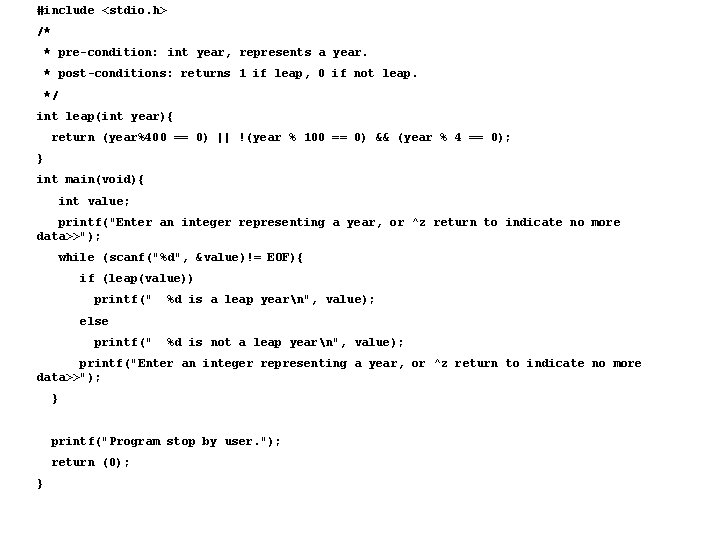
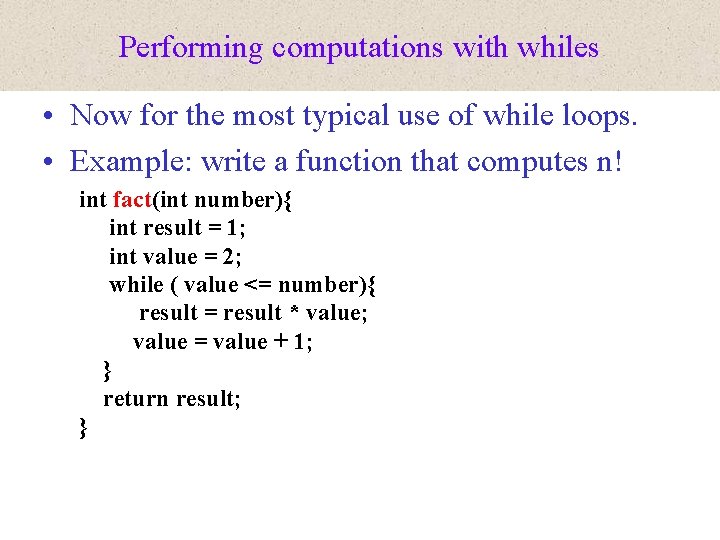
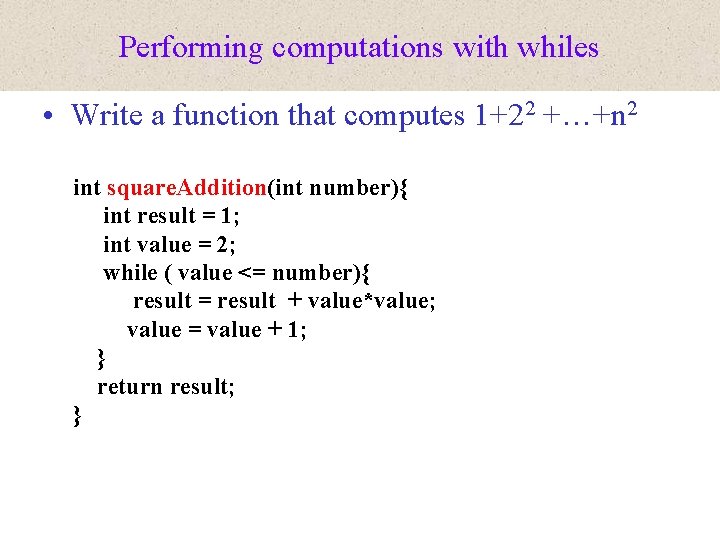
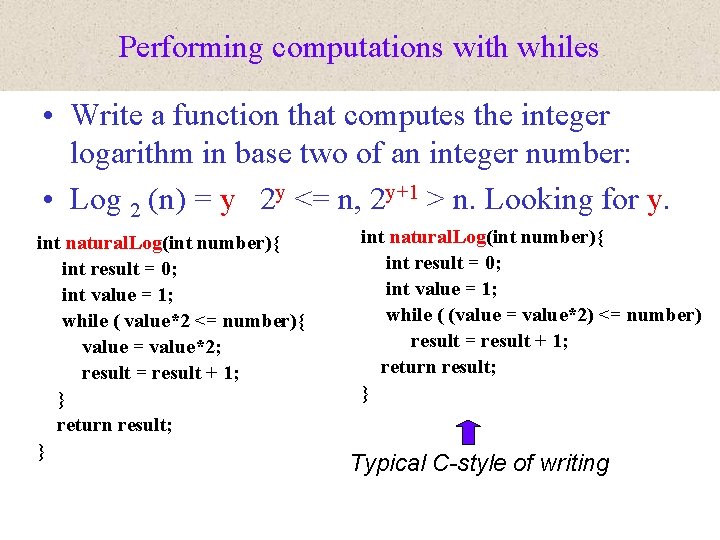
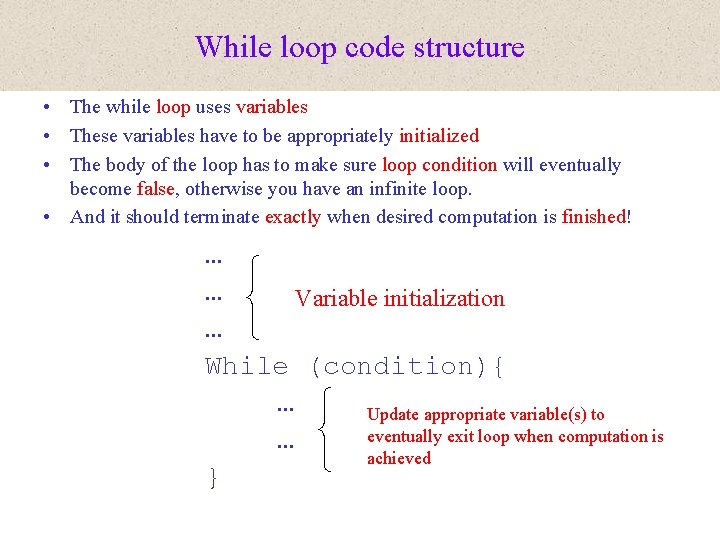
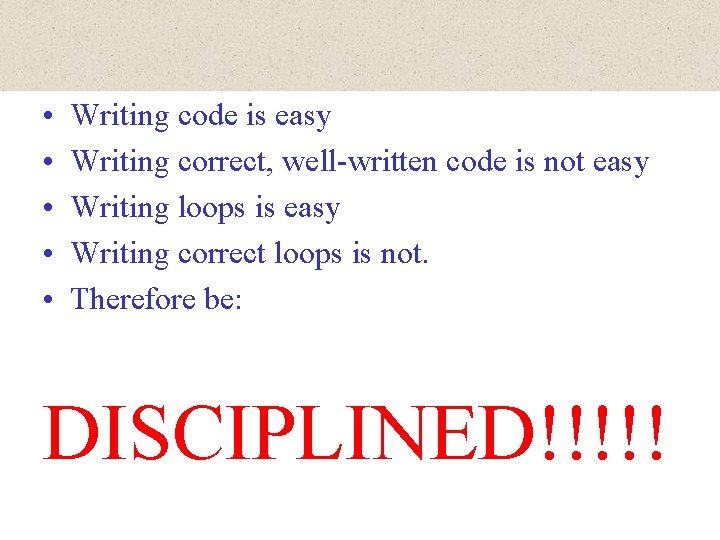
- Slides: 20
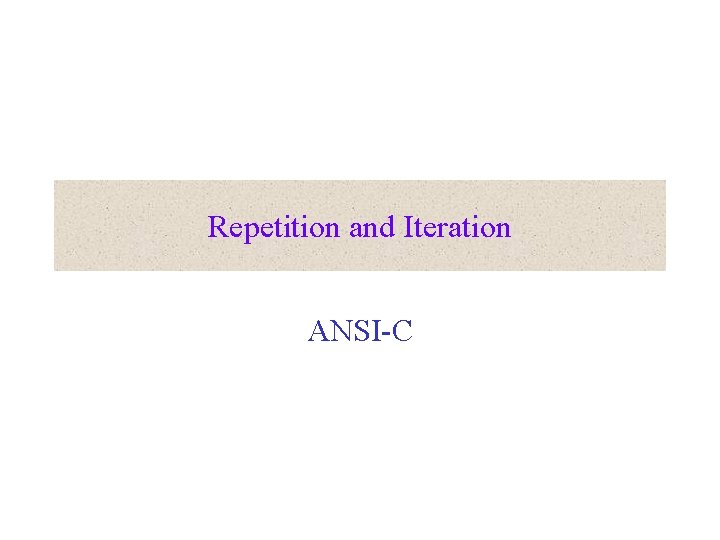
Repetition and Iteration ANSI-C
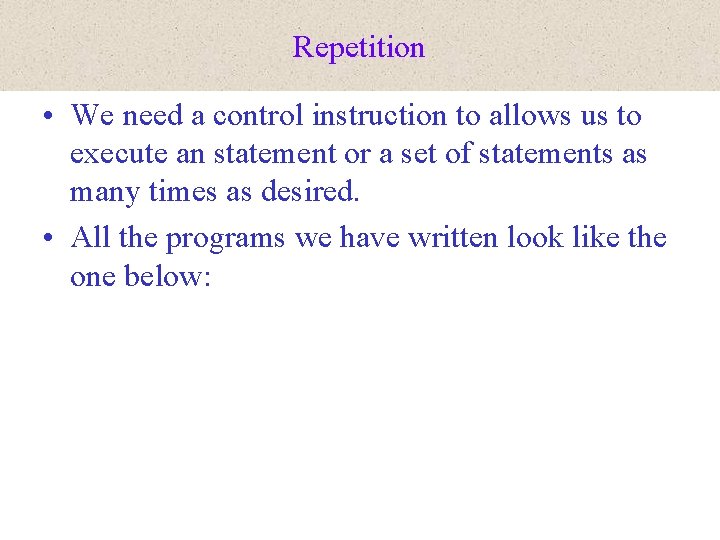
Repetition • We need a control instruction to allows us to execute an statement or a set of statements as many times as desired. • All the programs we have written look like the one below:
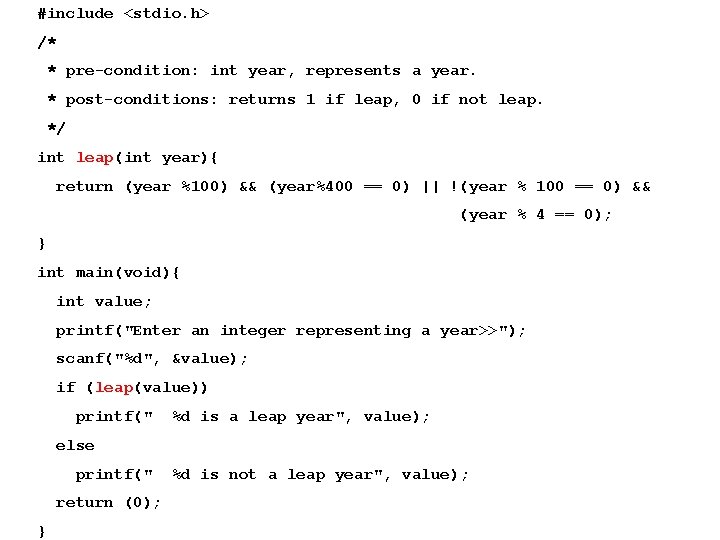
#include <stdio. h> /* * pre-condition: int year, represents a year. * post-conditions: returns 1 if leap, 0 if not leap. */ int leap(int year){ return (year %100) && (year%400 == 0) || !(year % 100 == 0) && (year % 4 == 0); } int main(void){ int value; printf("Enter an integer representing a year>>"); scanf("%d", &value); if (leap(value)) printf(" %d is a leap year", value); else printf(" return (0); } %d is not a leap year", value);
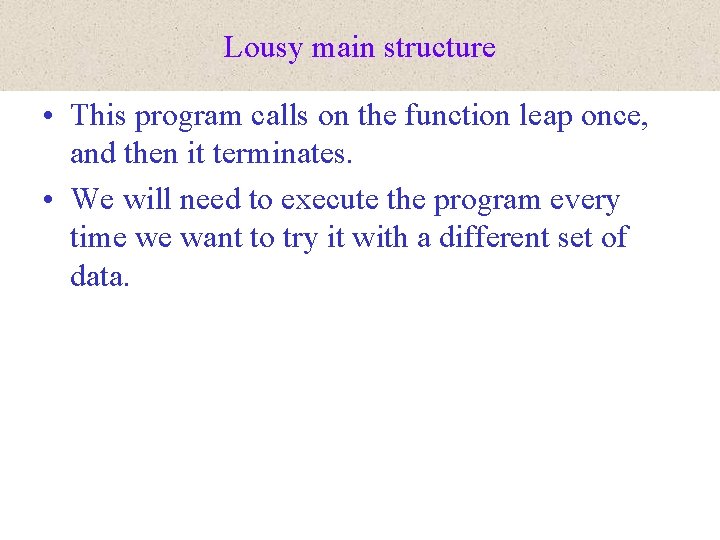
Lousy main structure • This program calls on the function leap once, and then it terminates. • We will need to execute the program every time we want to try it with a different set of data.
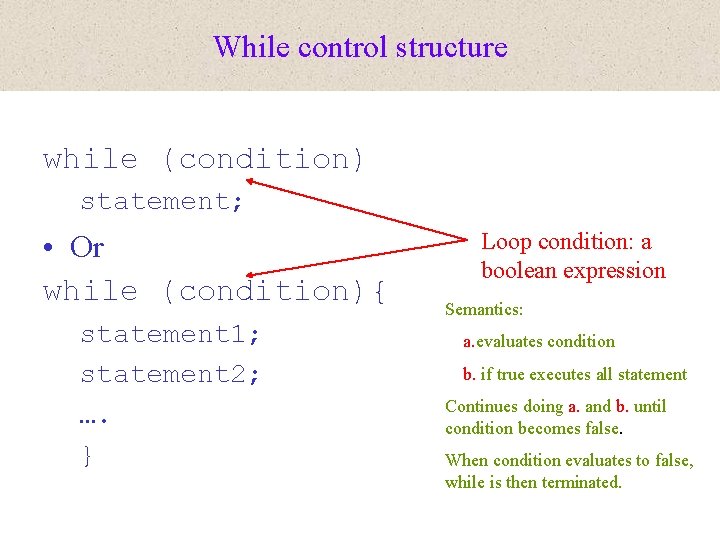
While control structure while (condition) statement; • Or while (condition){ statement 1; statement 2; …. } Loop condition: a boolean expression Semantics: a. evaluates condition b. if true executes all statement Continues doing a. and b. until condition becomes false. When condition evaluates to false, while is then terminated.
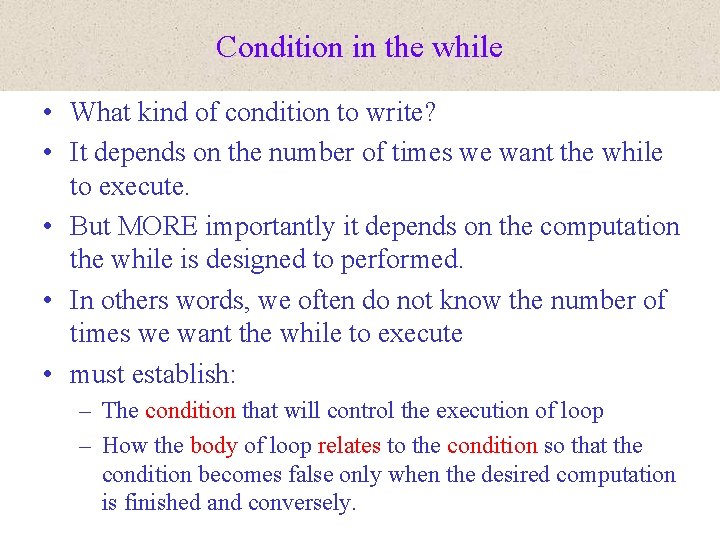
Condition in the while • What kind of condition to write? • It depends on the number of times we want the while to execute. • But MORE importantly it depends on the computation the while is designed to performed. • In others words, we often do not know the number of times we want the while to execute • must establish: – The condition that will control the execution of loop – How the body of loop relates to the condition so that the condition becomes false only when the desired computation is finished and conversely.
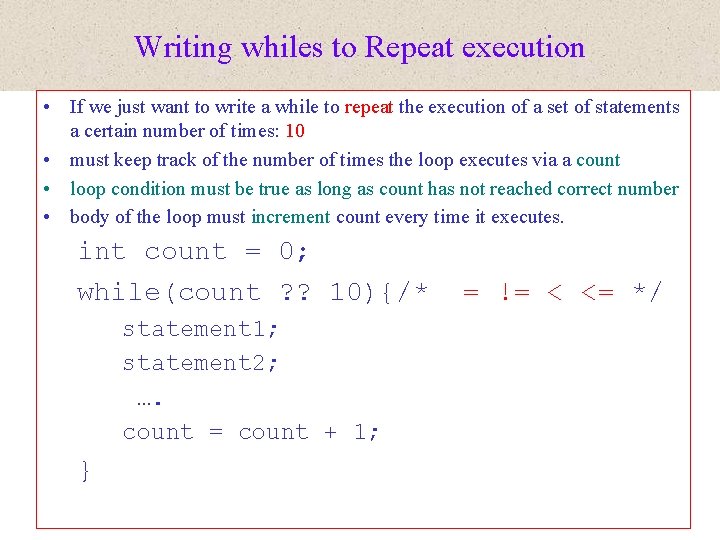
Writing whiles to Repeat execution • If we just want to write a while to repeat the execution of a set of statements a certain number of times: 10 • must keep track of the number of times the loop executes via a count • loop condition must be true as long as count has not reached correct number • body of the loop must increment count every time it executes. int count = 0; while(count ? ? 10){/* statement 1; statement 2; …. count = count + 1; } = != < <= */
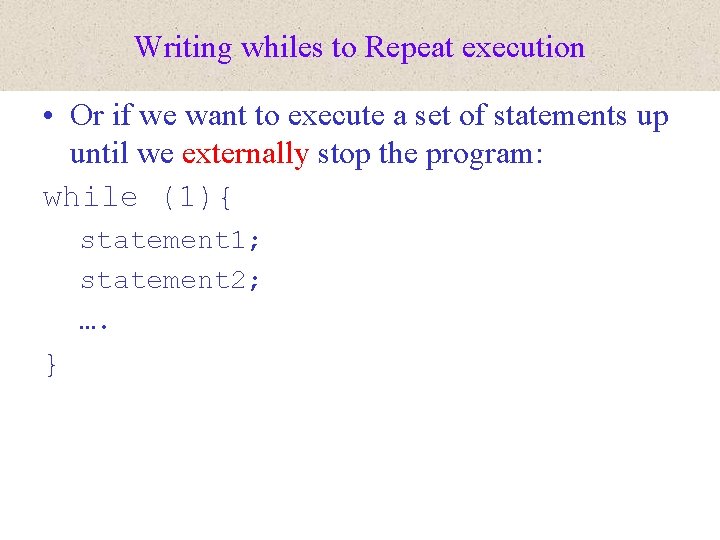
Writing whiles to Repeat execution • Or if we want to execute a set of statements up until we externally stop the program: while (1){ statement 1; statement 2; …. }
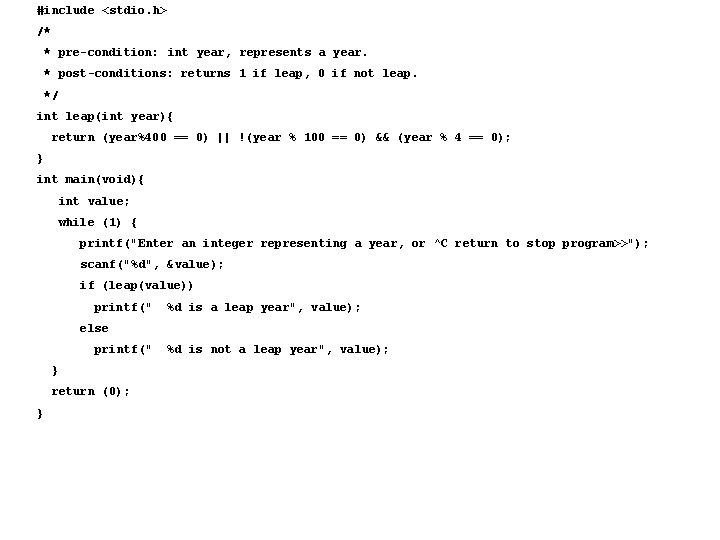
#include <stdio. h> /* * pre-condition: int year, represents a year. * post-conditions: returns 1 if leap, 0 if not leap. */ int leap(int year){ return (year%400 == 0) || !(year % 100 == 0) && (year % 4 == 0); } int main(void){ int value; while (1) { printf("Enter an integer representing a year, or ^C return to stop program>>"); scanf("%d", &value); if (leap(value)) printf(" %d is a leap year", value); else printf(" } return (0); } %d is not a leap year", value);
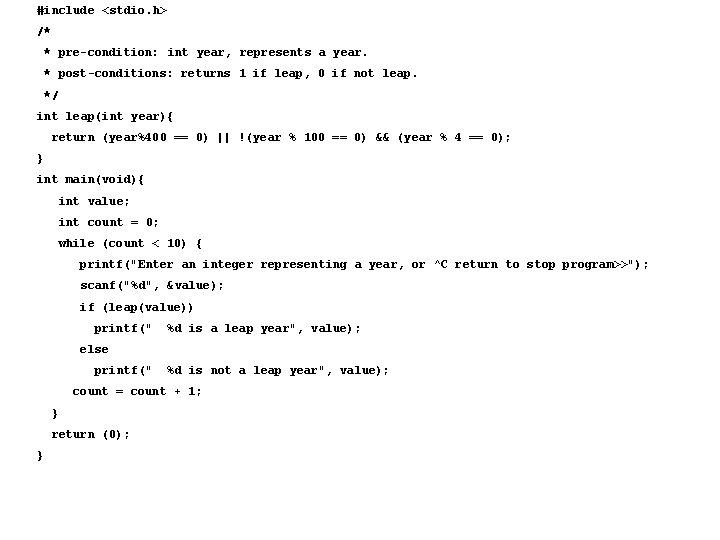
#include <stdio. h> /* * pre-condition: int year, represents a year. * post-conditions: returns 1 if leap, 0 if not leap. */ int leap(int year){ return (year%400 == 0) || !(year % 100 == 0) && (year % 4 == 0); } int main(void){ int value; int count = 0; while (count < 10) { printf("Enter an integer representing a year, or ^C return to stop program>>"); scanf("%d", &value); if (leap(value)) printf(" %d is a leap year", value); else printf(" %d is not a leap year", value); count = count + 1; } return (0); }
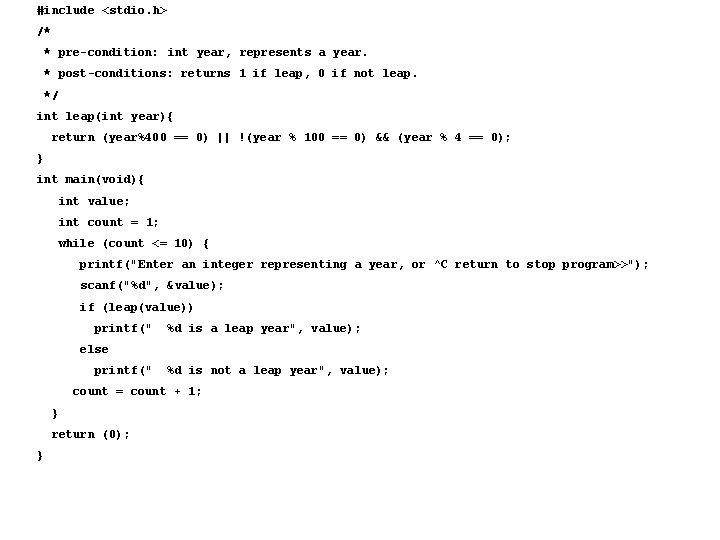
#include <stdio. h> /* * pre-condition: int year, represents a year. * post-conditions: returns 1 if leap, 0 if not leap. */ int leap(int year){ return (year%400 == 0) || !(year % 100 == 0) && (year % 4 == 0); } int main(void){ int value; int count = 1; while (count <= 10) { printf("Enter an integer representing a year, or ^C return to stop program>>"); scanf("%d", &value); if (leap(value)) printf(" %d is a leap year", value); else printf(" %d is not a leap year", value); count = count + 1; } return (0); }
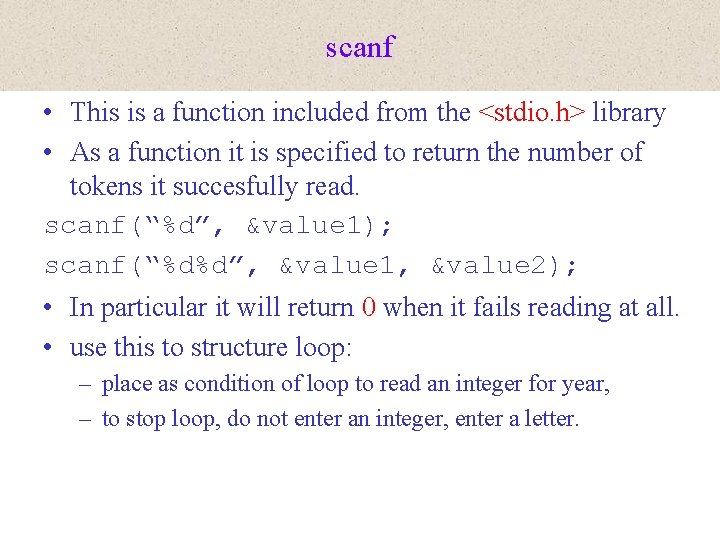
scanf • This is a function included from the <stdio. h> library • As a function it is specified to return the number of tokens it succesfully read. scanf(“%d”, &value 1); scanf(“%d%d”, &value 1, &value 2); • In particular it will return 0 when it fails reading at all. • use this to structure loop: – place as condition of loop to read an integer for year, – to stop loop, do not enter an integer, enter a letter.
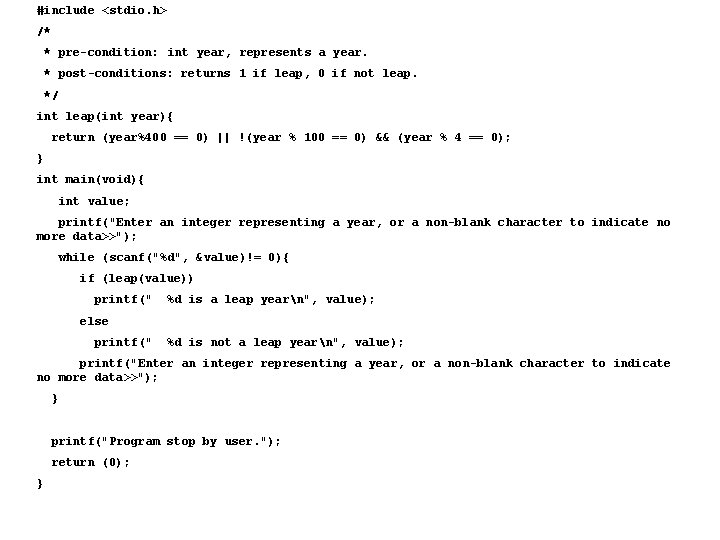
#include <stdio. h> /* * pre-condition: int year, represents a year. * post-conditions: returns 1 if leap, 0 if not leap. */ int leap(int year){ return (year%400 == 0) || !(year % 100 == 0) && (year % 4 == 0); } int main(void){ int value; printf("Enter an integer representing a year, or a non-blank character to indicate no more data>>"); while (scanf("%d", &value)!= 0){ if (leap(value)) printf(" %d is a leap yearn", value); else printf(" %d is not a leap yearn", value); printf("Enter an integer representing a year, or a non-blank character to indicate no more data>>"); } printf("Program stop by user. "); return (0); }
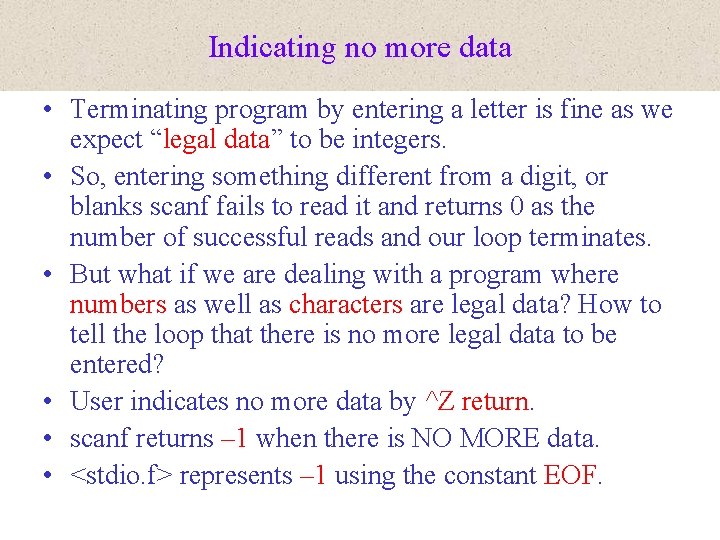
Indicating no more data • Terminating program by entering a letter is fine as we expect “legal data” to be integers. • So, entering something different from a digit, or blanks scanf fails to read it and returns 0 as the number of successful reads and our loop terminates. • But what if we are dealing with a program where numbers as well as characters are legal data? How to tell the loop that there is no more legal data to be entered? • User indicates no more data by ^Z return. • scanf returns – 1 when there is NO MORE data. • <stdio. f> represents – 1 using the constant EOF.
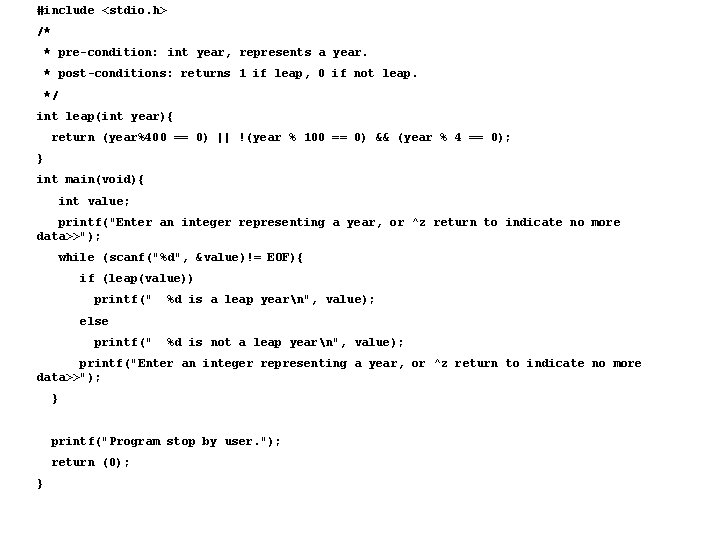
#include <stdio. h> /* * pre-condition: int year, represents a year. * post-conditions: returns 1 if leap, 0 if not leap. */ int leap(int year){ return (year%400 == 0) || !(year % 100 == 0) && (year % 4 == 0); } int main(void){ int value; printf("Enter an integer representing a year, or ^z return to indicate no more data>>"); while (scanf("%d", &value)!= EOF){ if (leap(value)) printf(" %d is a leap yearn", value); else printf(" %d is not a leap yearn", value); printf("Enter an integer representing a year, or ^z return to indicate no more data>>"); } printf("Program stop by user. "); return (0); }
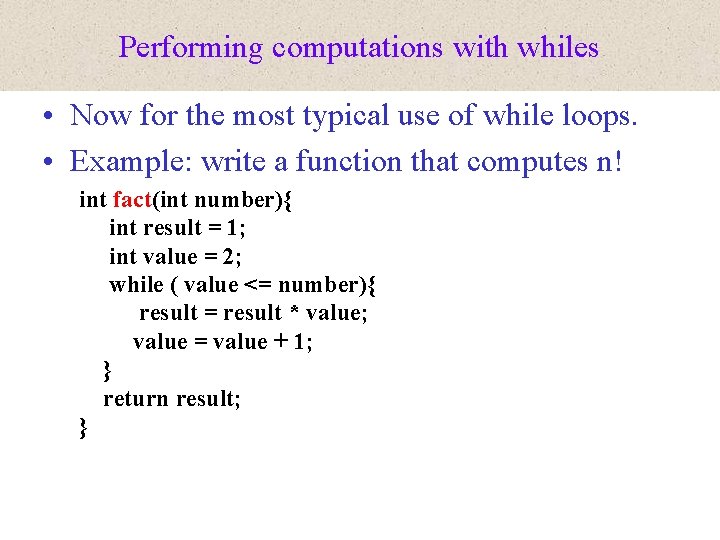
Performing computations with whiles • Now for the most typical use of while loops. • Example: write a function that computes n! int fact(int number){ int result = 1; int value = 2; while ( value <= number){ result = result * value; value = value + 1; } return result; }
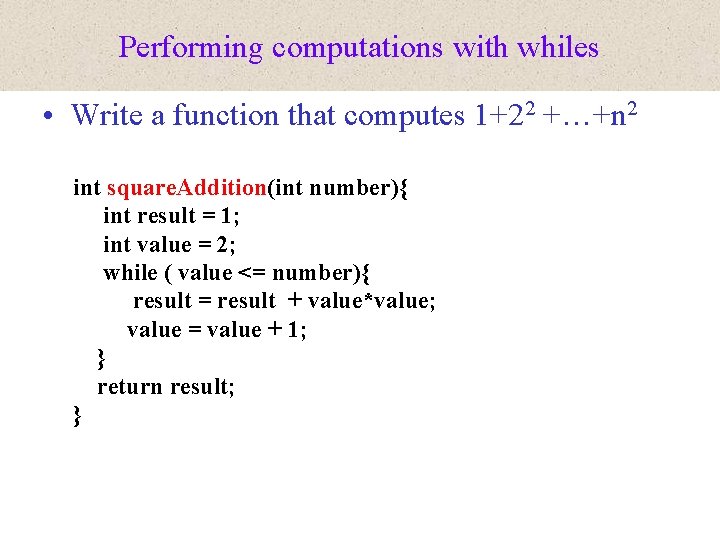
Performing computations with whiles • Write a function that computes 1+22 +…+n 2 int square. Addition(int number){ int result = 1; int value = 2; while ( value <= number){ result = result + value*value; value = value + 1; } return result; }
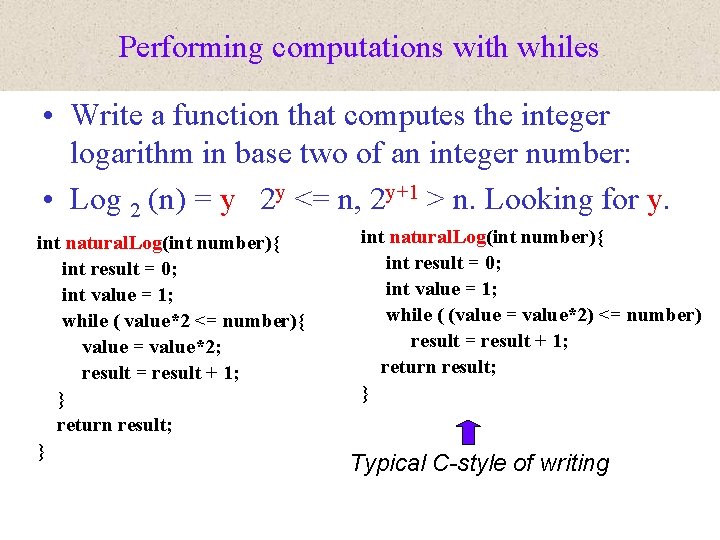
Performing computations with whiles • Write a function that computes the integer logarithm in base two of an integer number: • Log 2 (n) = y 2 y <= n, 2 y+1 > n. Looking for y. int natural. Log(int number){ int result = 0; int value = 1; while ( value*2 <= number){ value = value*2; result = result + 1; } return result; } int natural. Log(int number){ int result = 0; int value = 1; while ( (value = value*2) <= number) result = result + 1; return result; } Typical C-style of writing
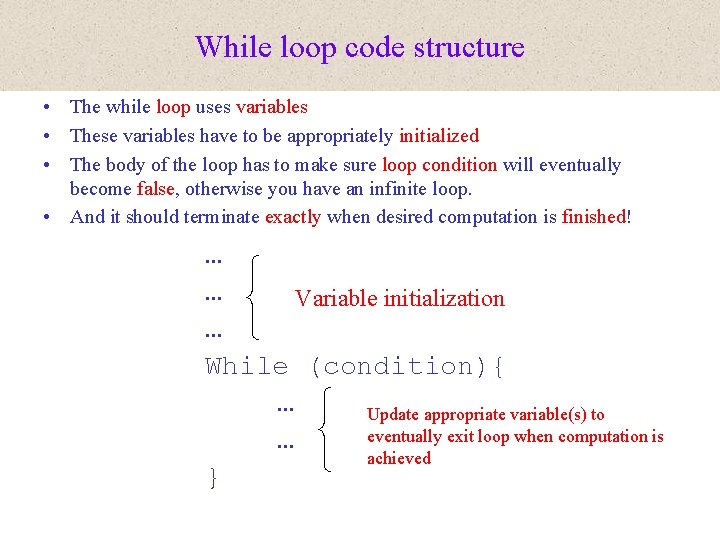
While loop code structure • The while loop uses variables • These variables have to be appropriately initialized • The body of the loop has to make sure loop condition will eventually become false, otherwise you have an infinite loop. • And it should terminate exactly when desired computation is finished! … … Variable initialization … While (condition){ … Update appropriate variable(s) to eventually exit loop when computation is … achieved }
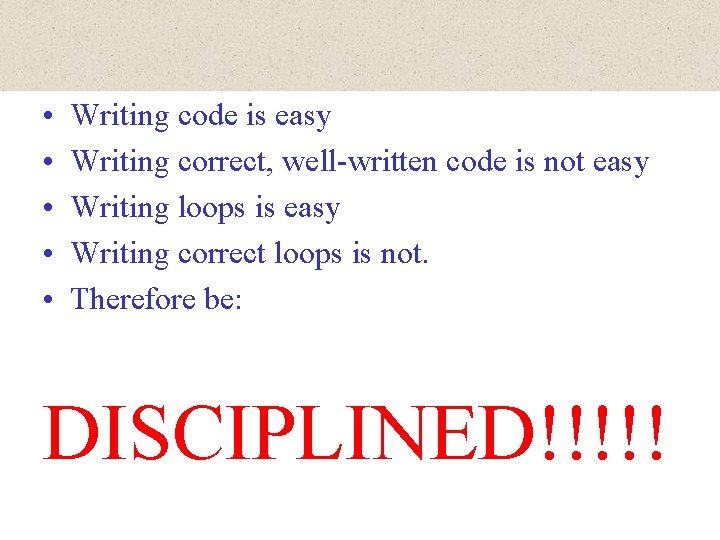
• • • Writing code is easy Writing correct, well-written code is not easy Writing loops is easy Writing correct loops is not. Therefore be: DISCIPLINED!!!!!
Selection sequence iteration
Differentiate between conditional and iterative statements
Selection in pseudocode
Similarities between iteration and recursion
Loaded language ads
Fixed point iteration method
Iteration demo
Recurrence relation solver
Iteration vs recursion
Value iteration
Markov decision process
Orthogonal iteration
Value iteration algorithm
Value iteration algorithm
What is the purpose of an iteration recap
Iterating in math
What is iteration in computer science
Recursion
Iteration definition computer science
What is the purpose of iteration goals?
Birge vieta method uses formula of