refercence www netacad com CHAPTER 6 Inheritance refercence
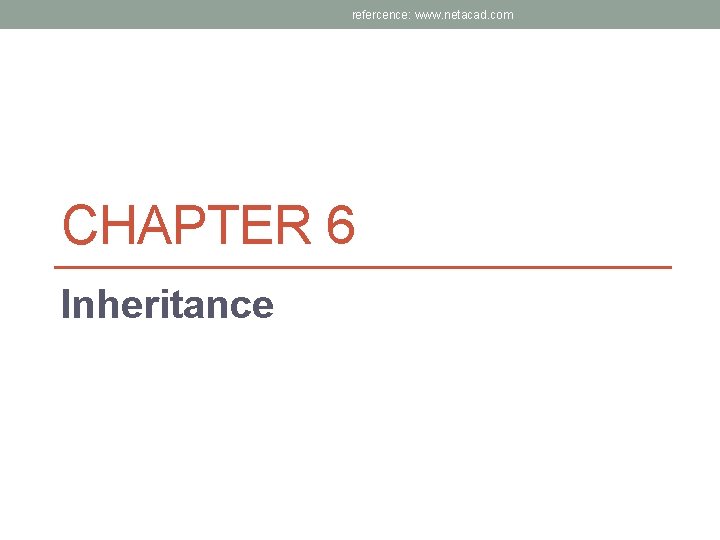
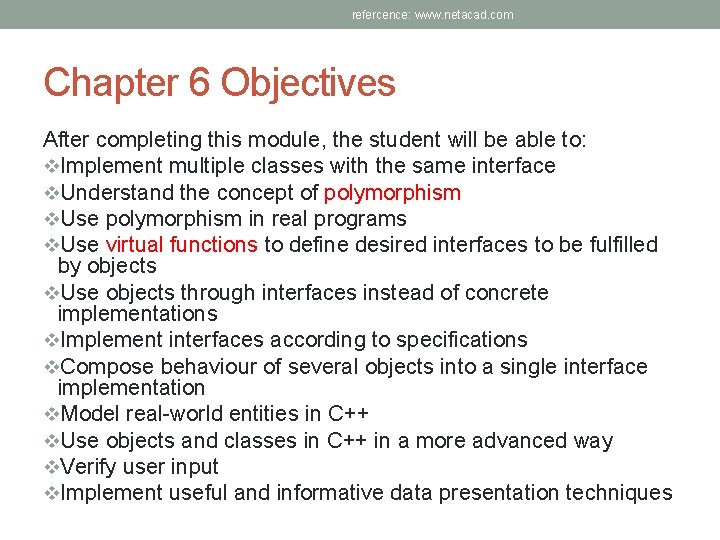
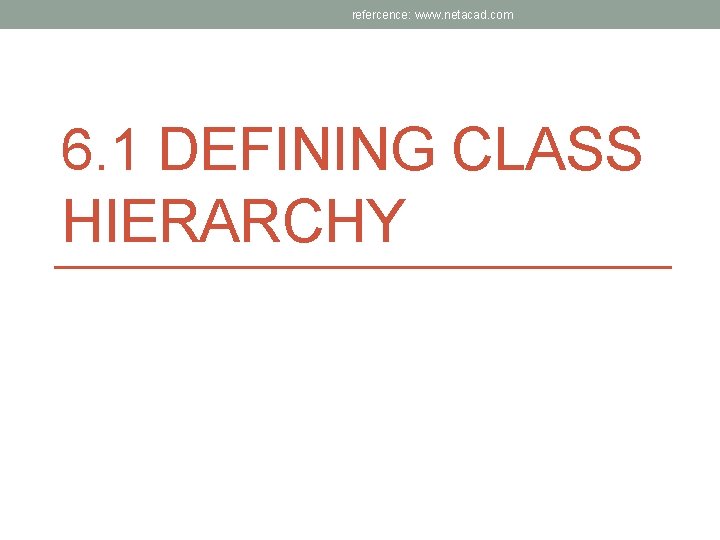
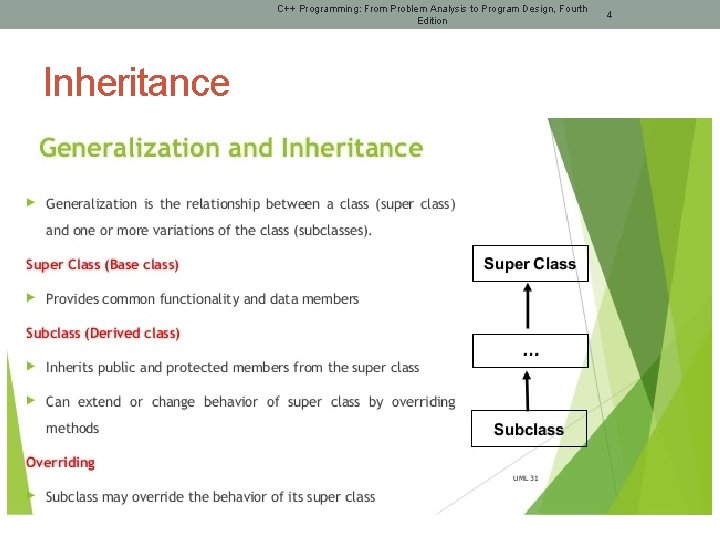
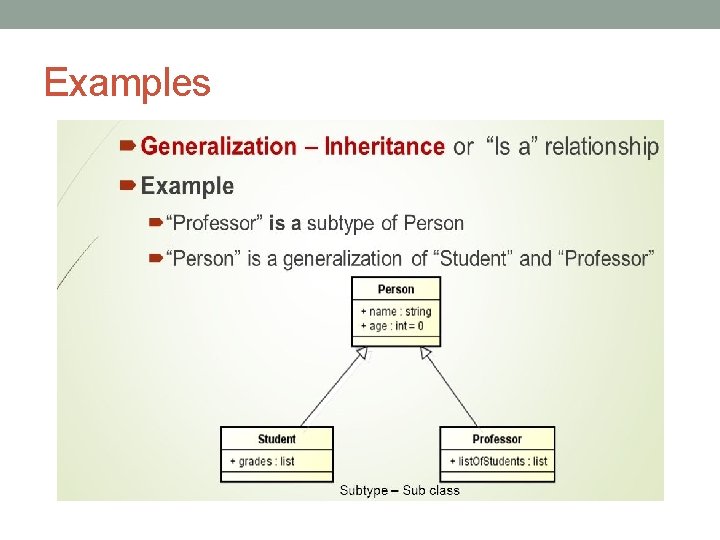
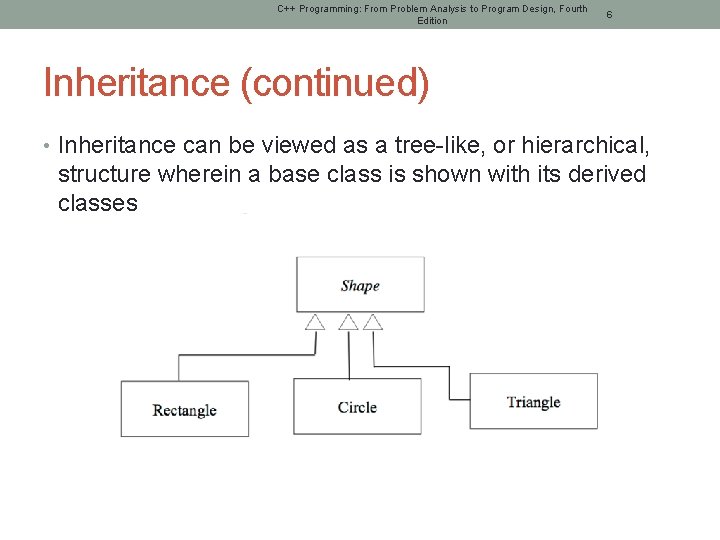
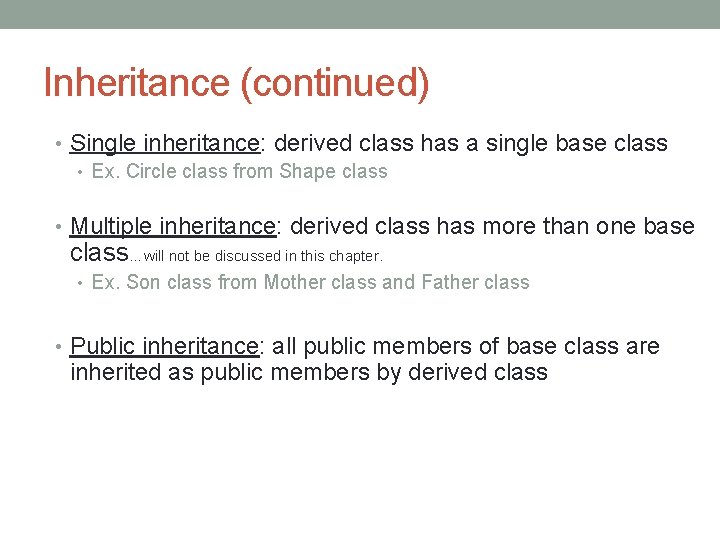
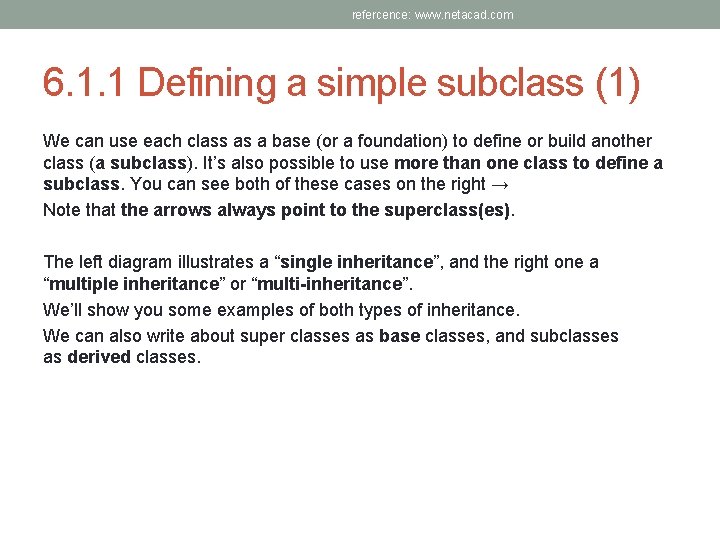
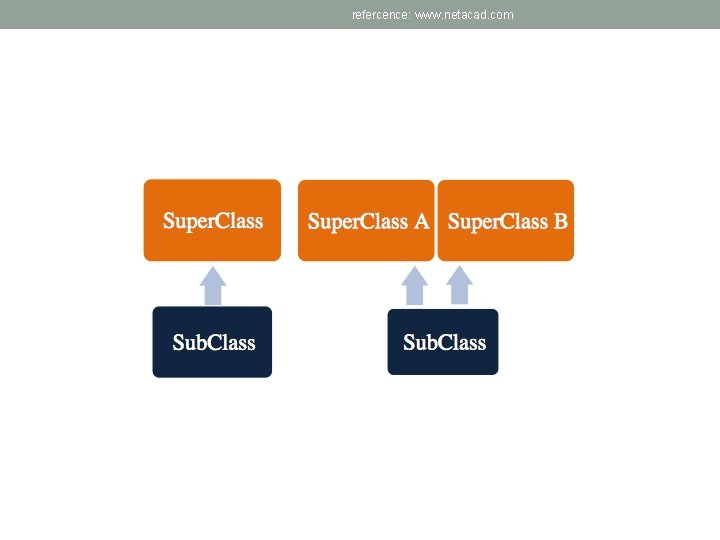
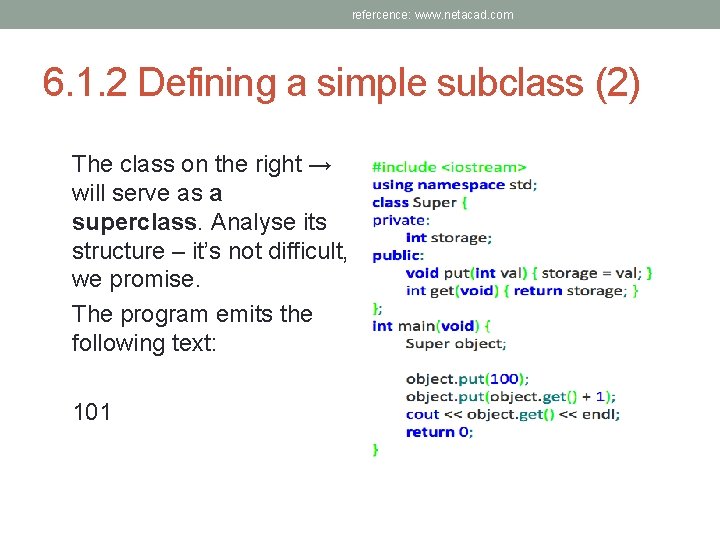
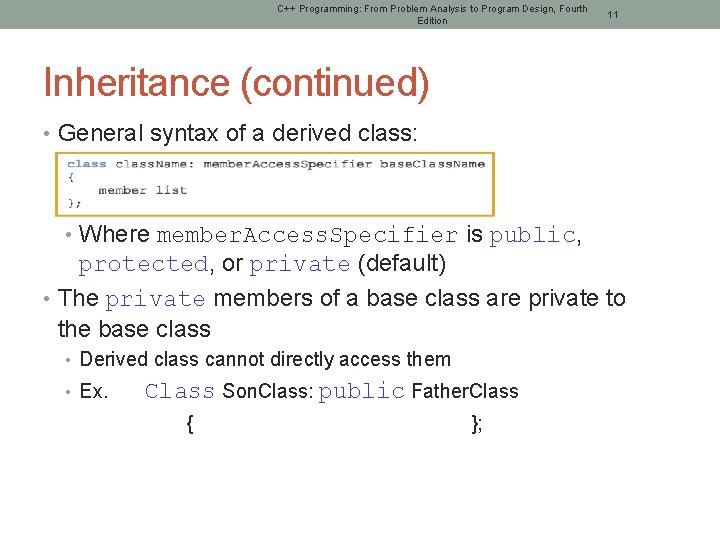
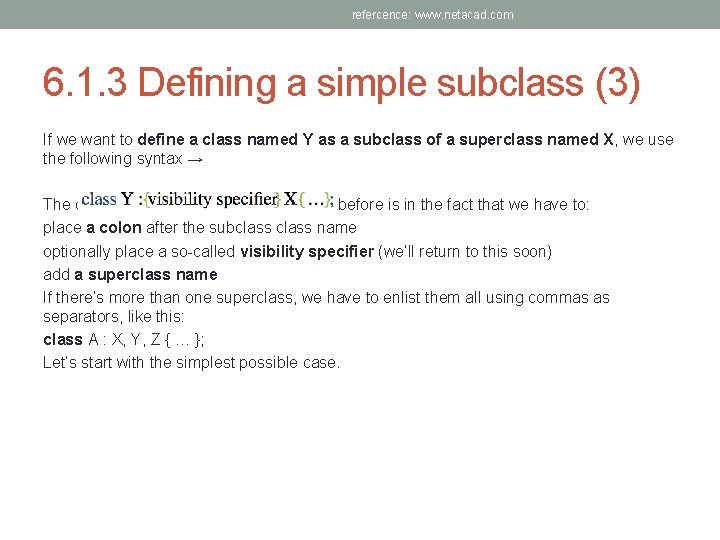
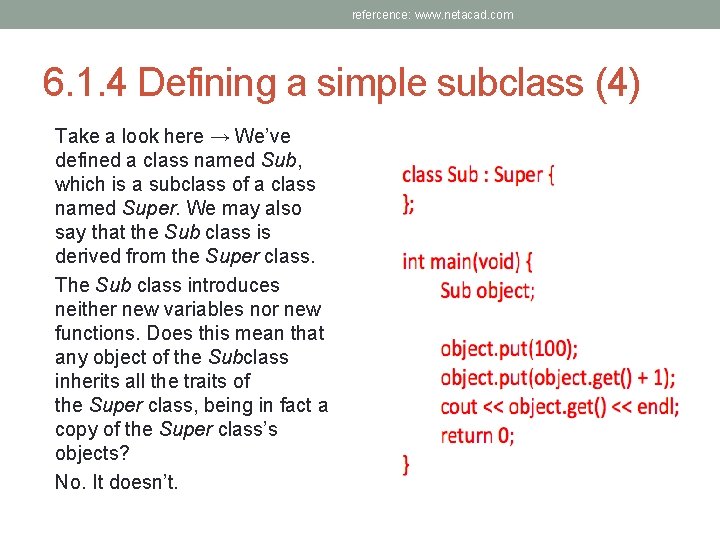
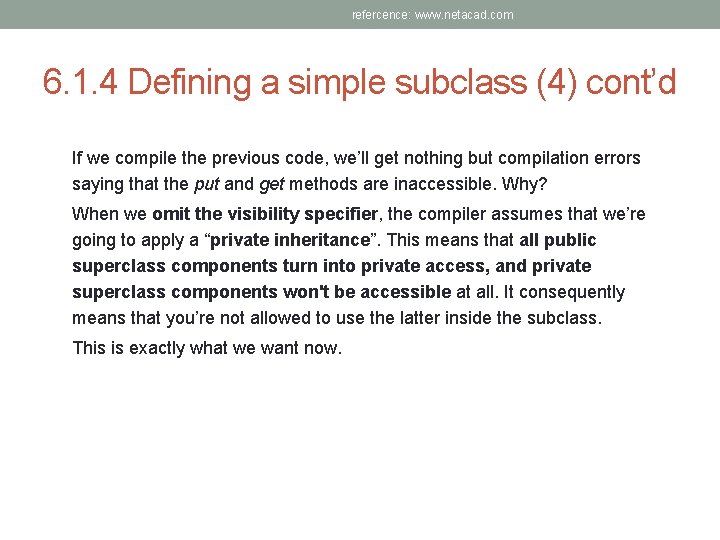
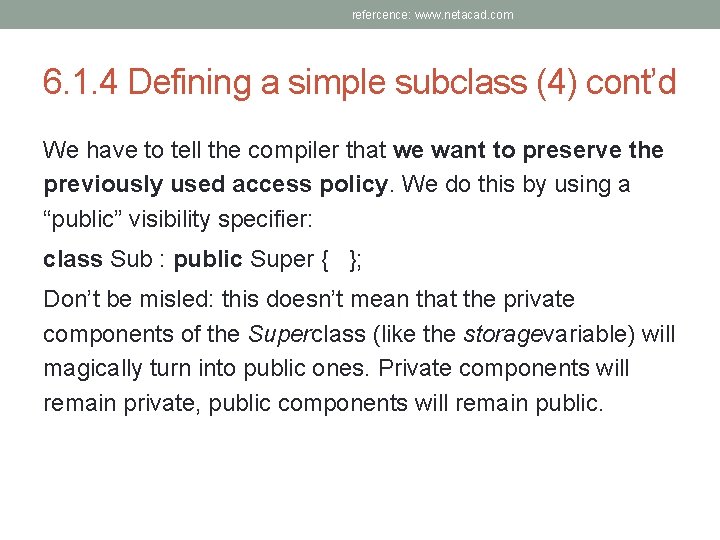
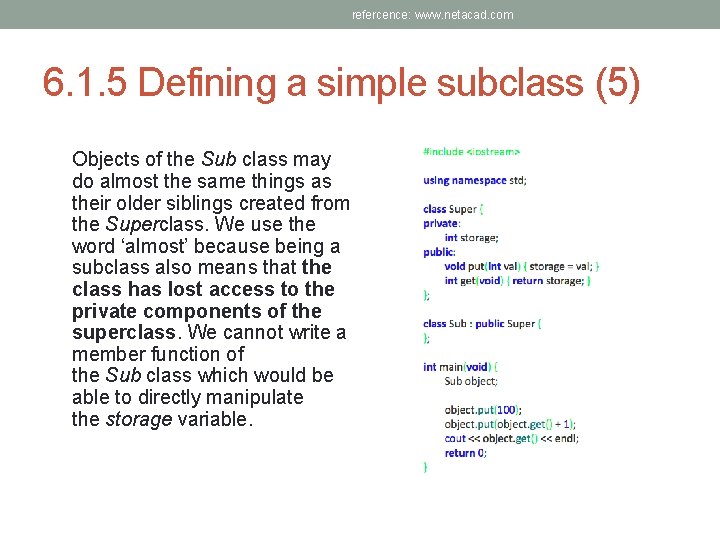
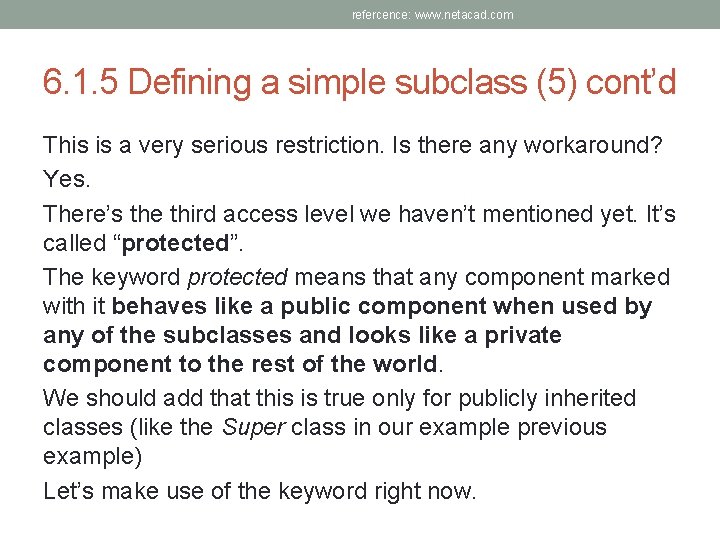
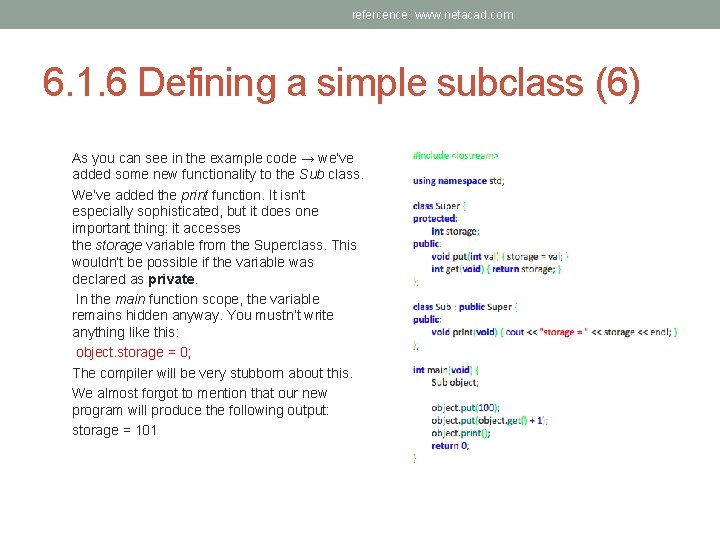
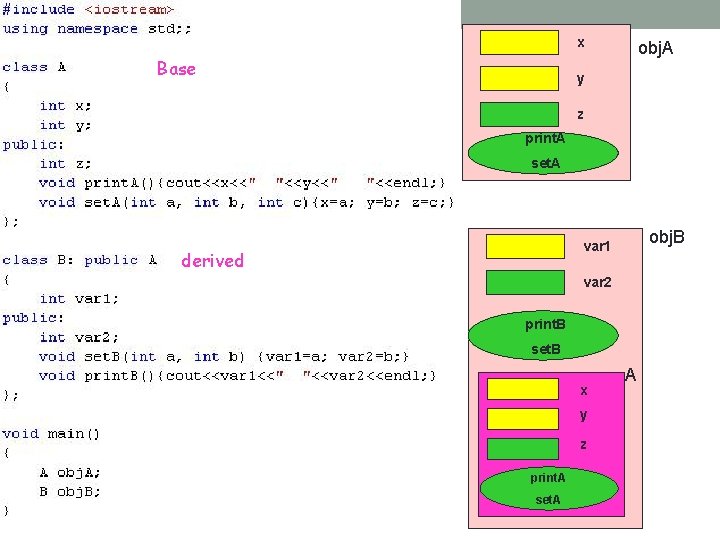
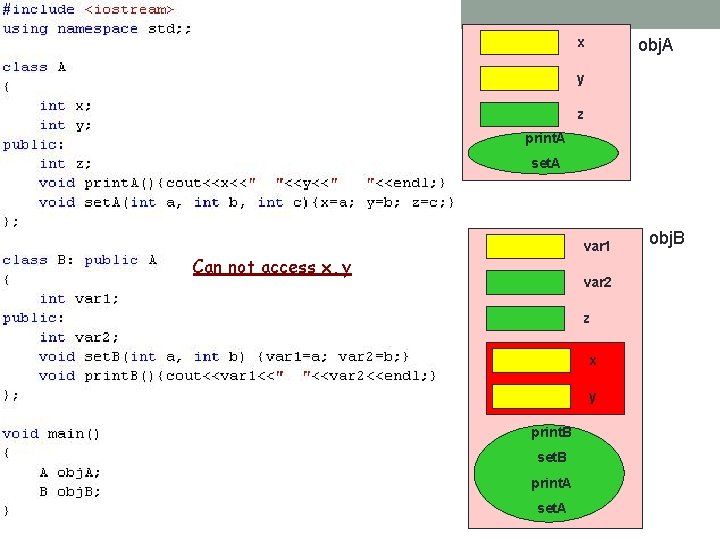
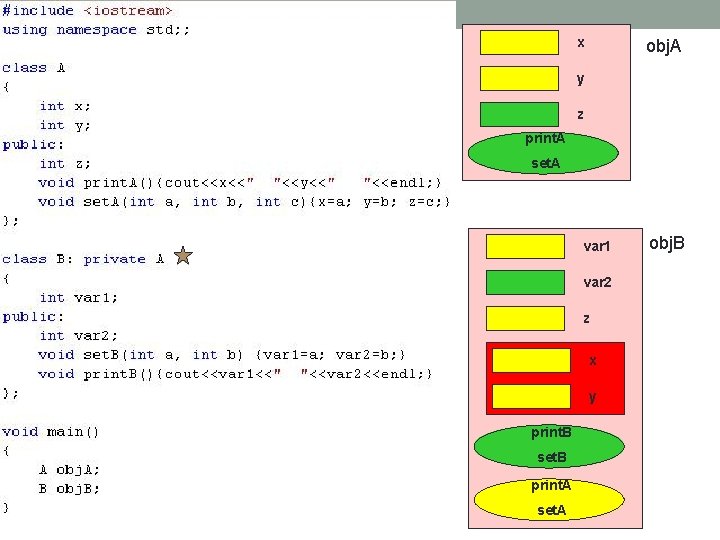
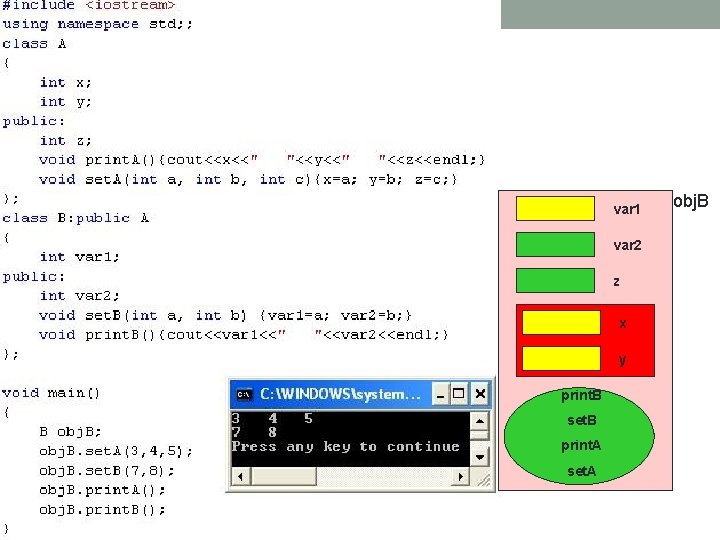
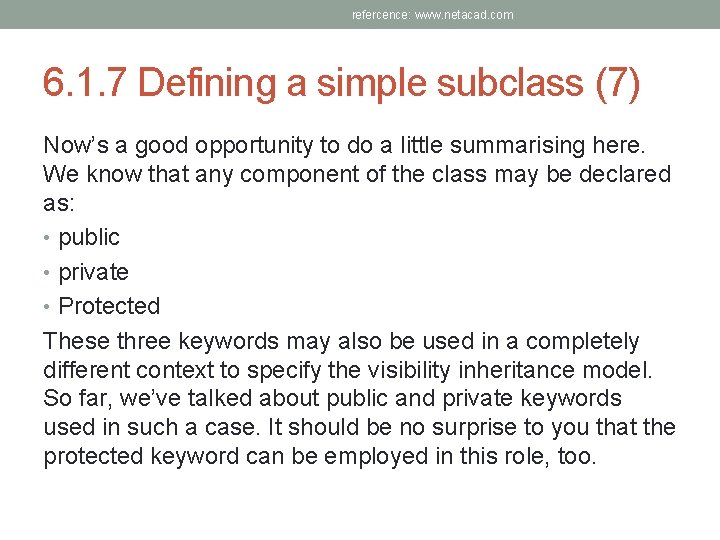
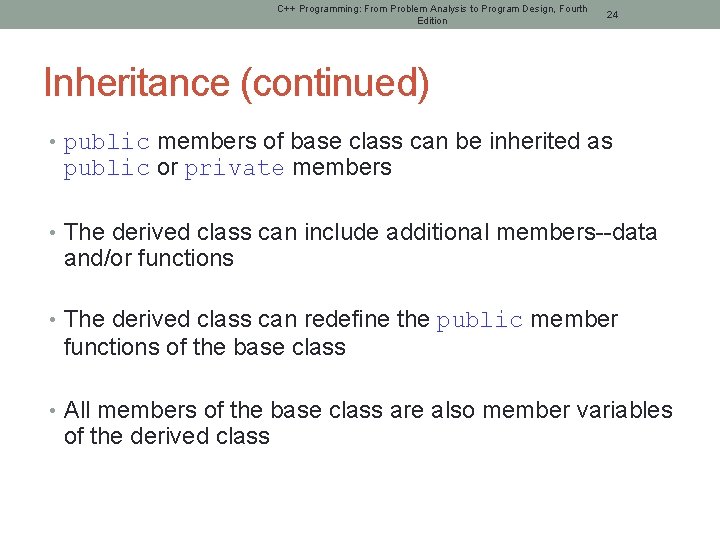
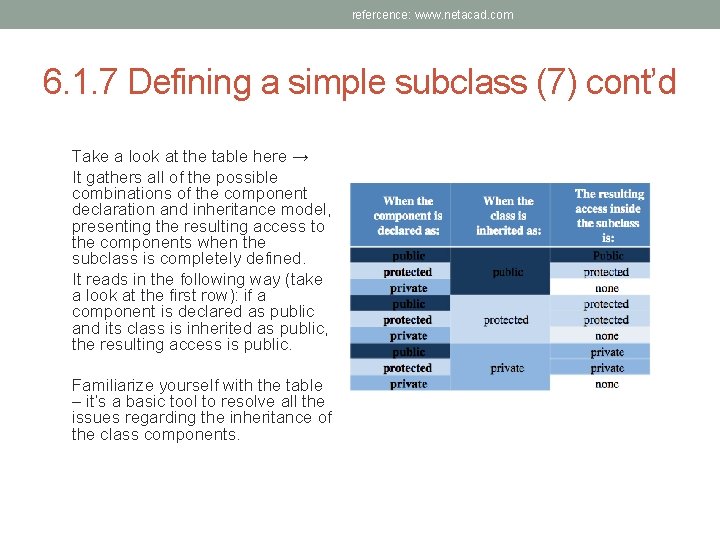
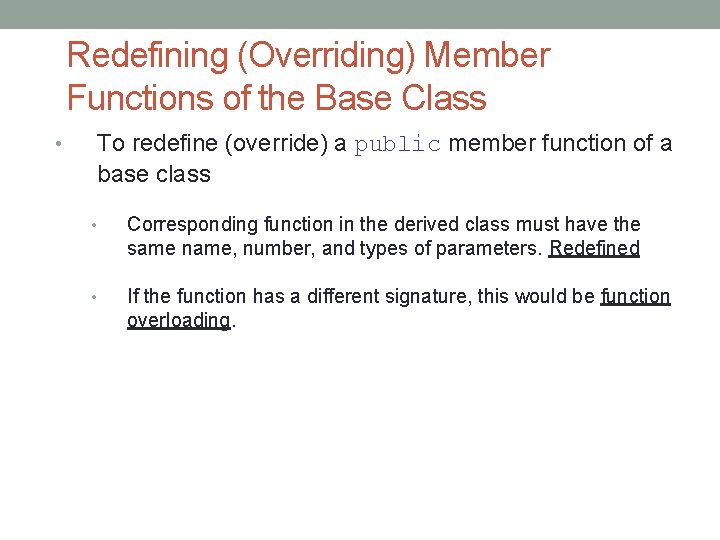
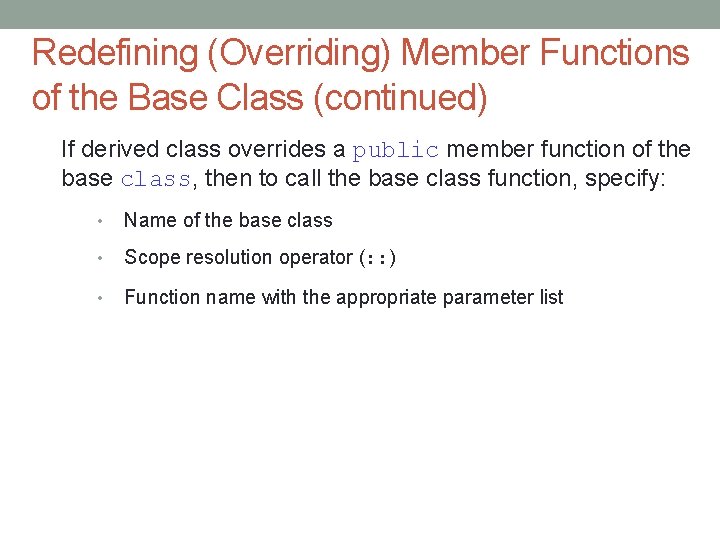
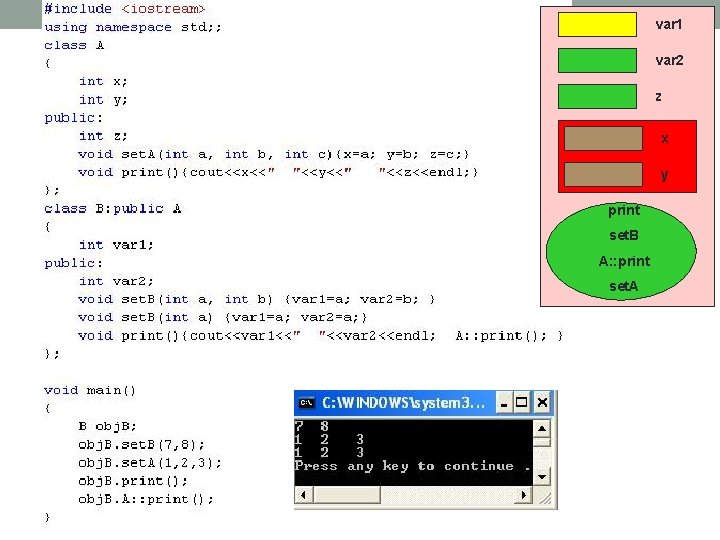
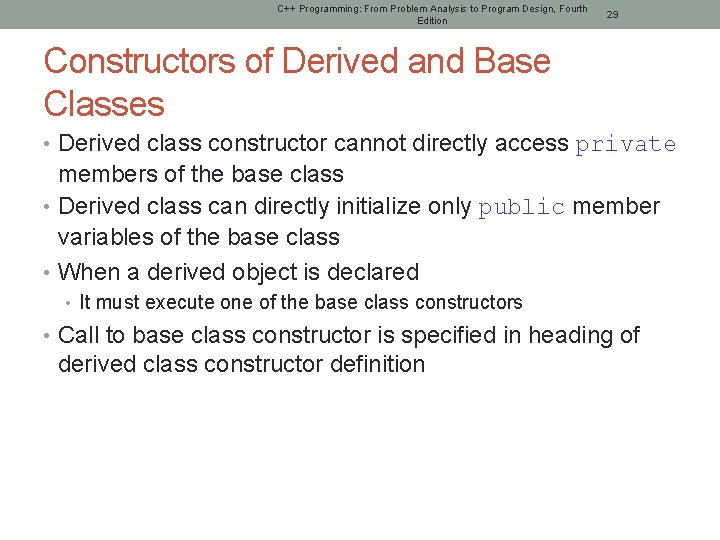
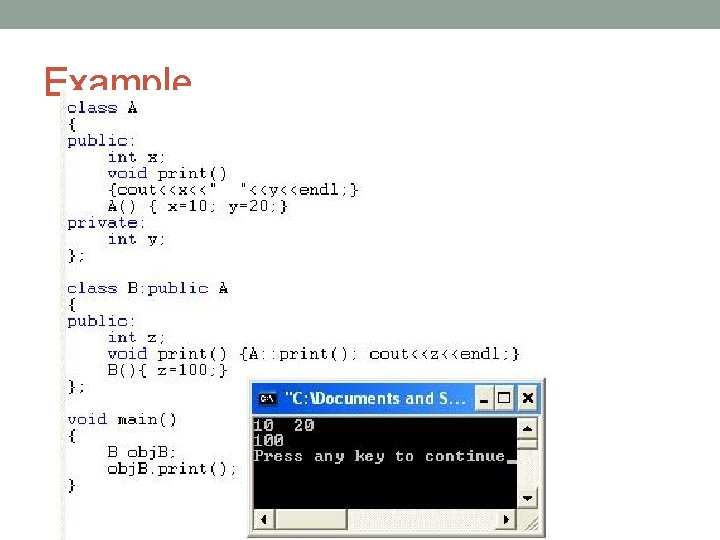
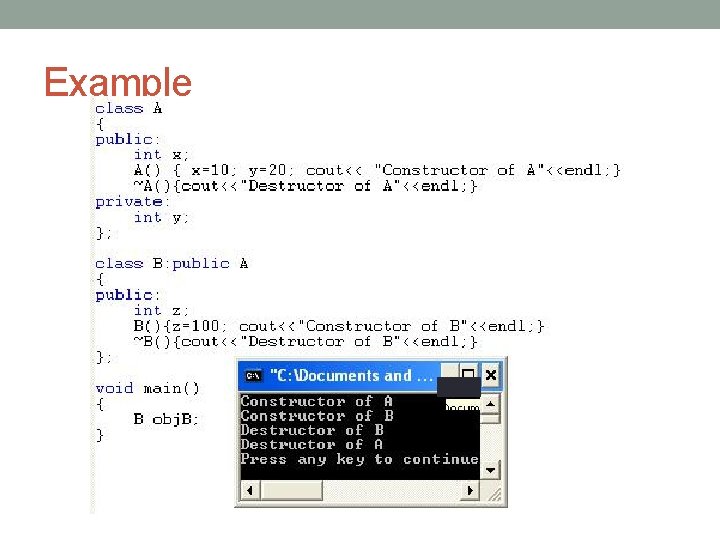
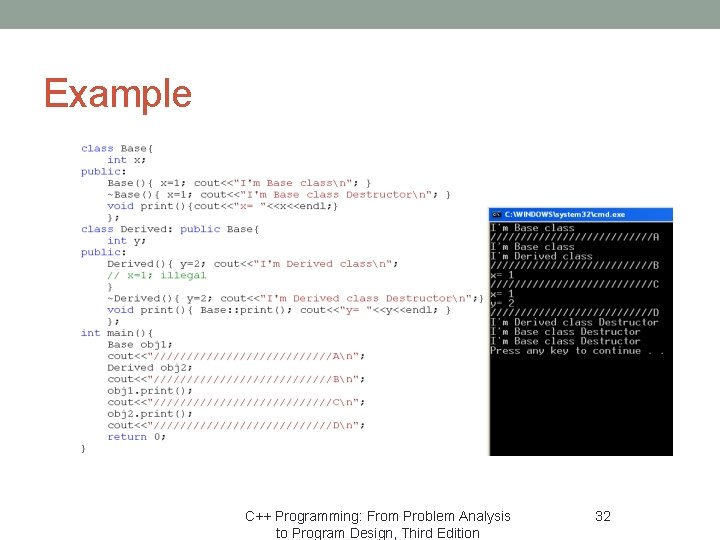
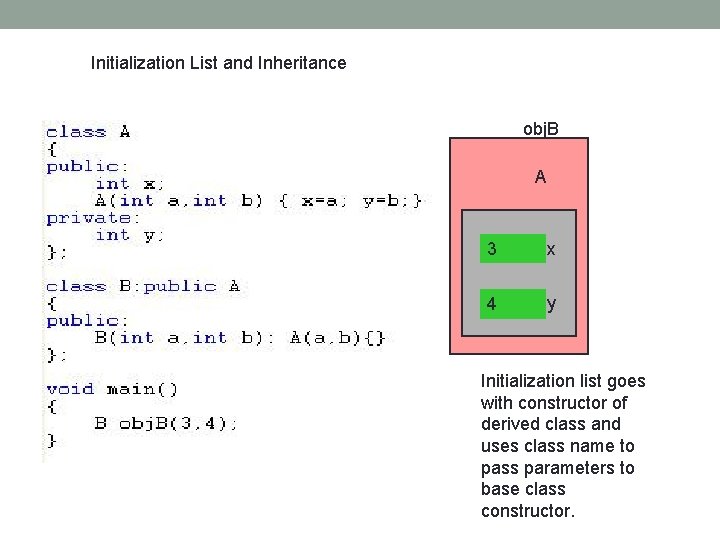
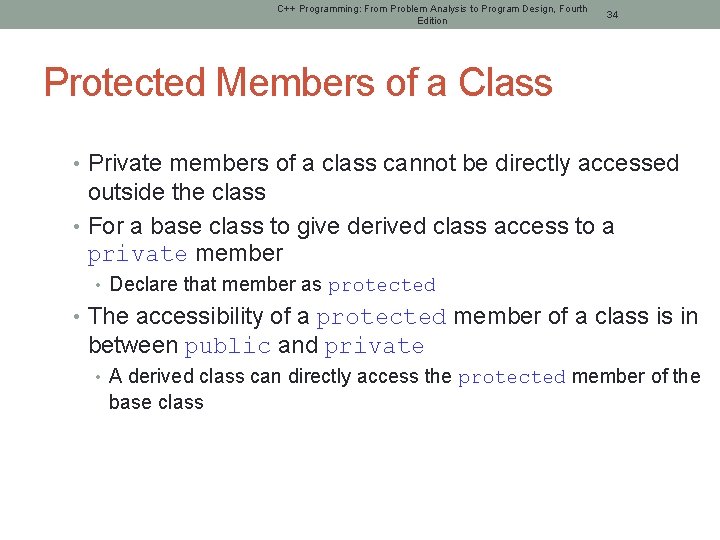
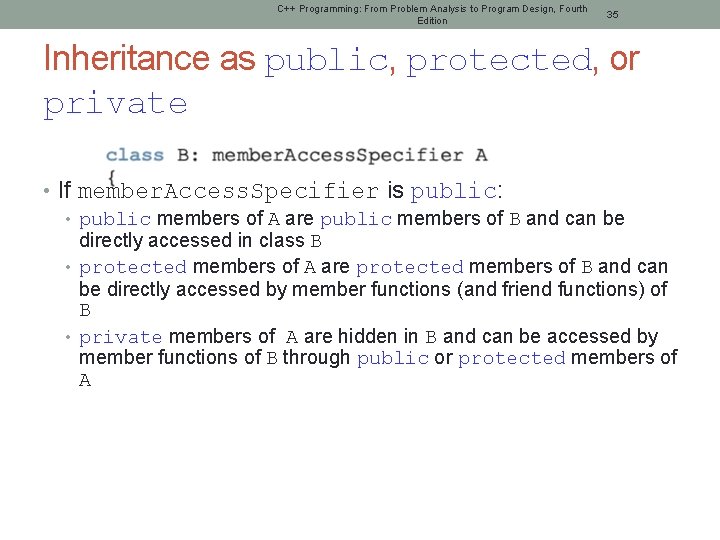
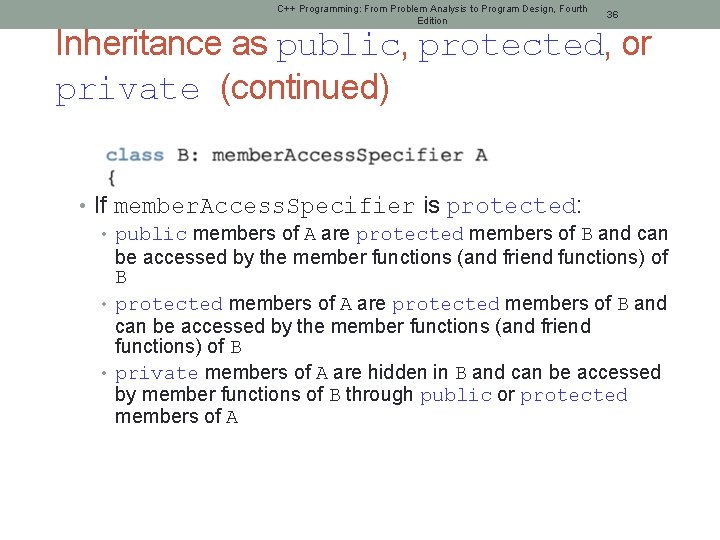
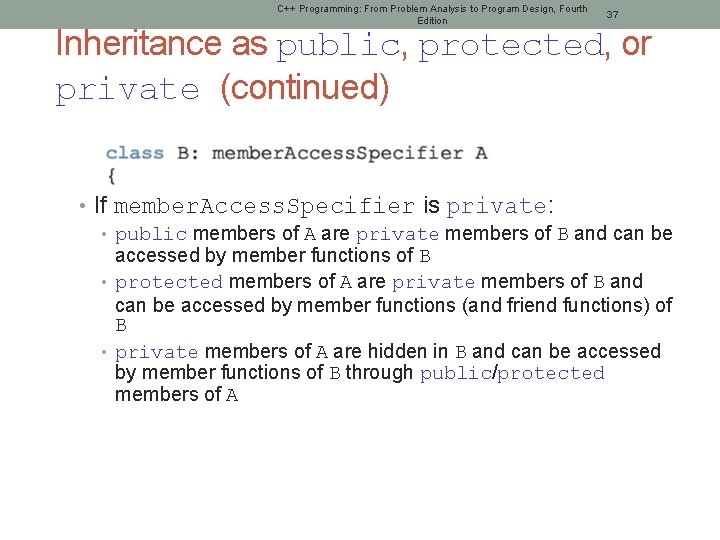
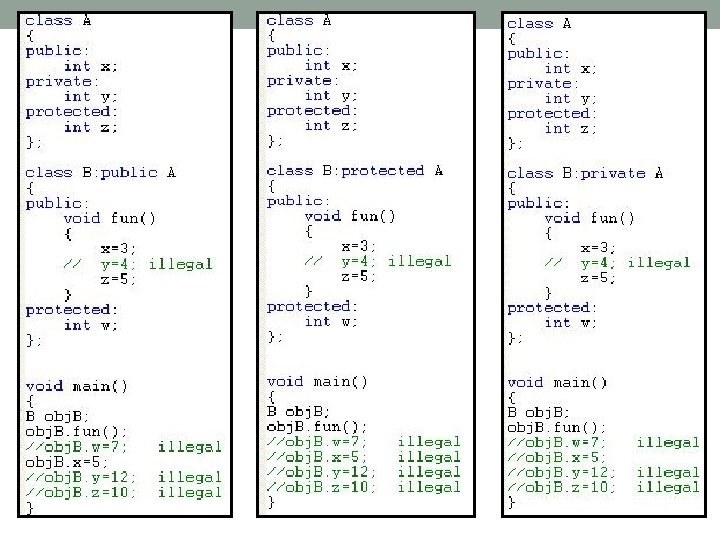
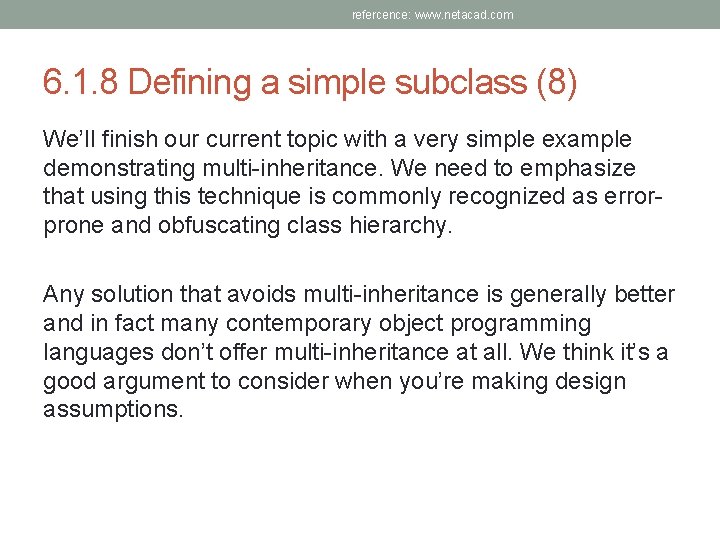
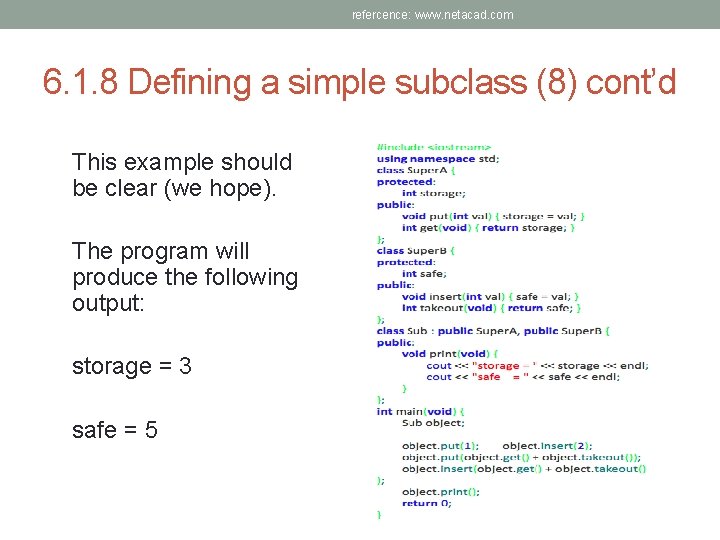
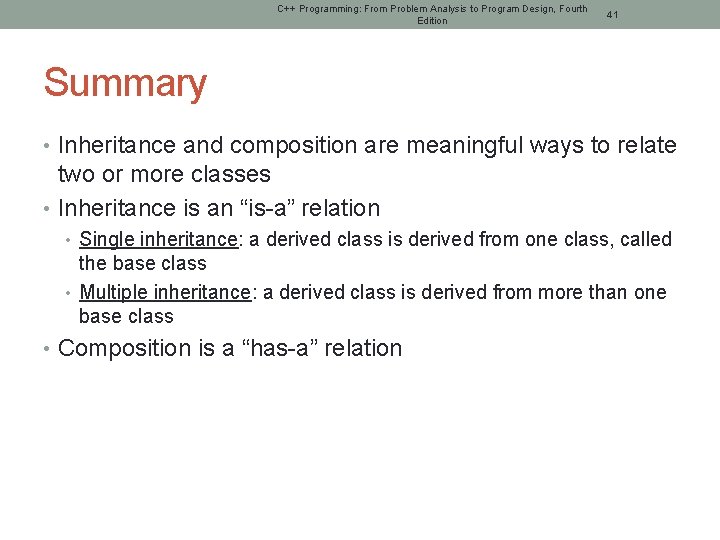
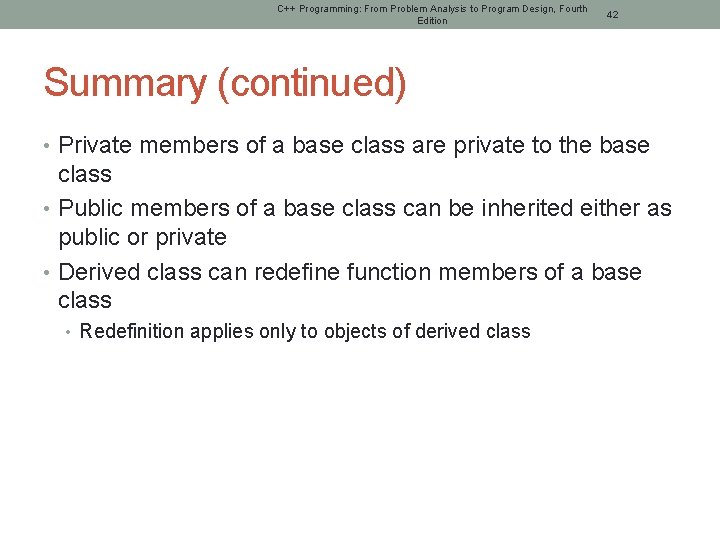
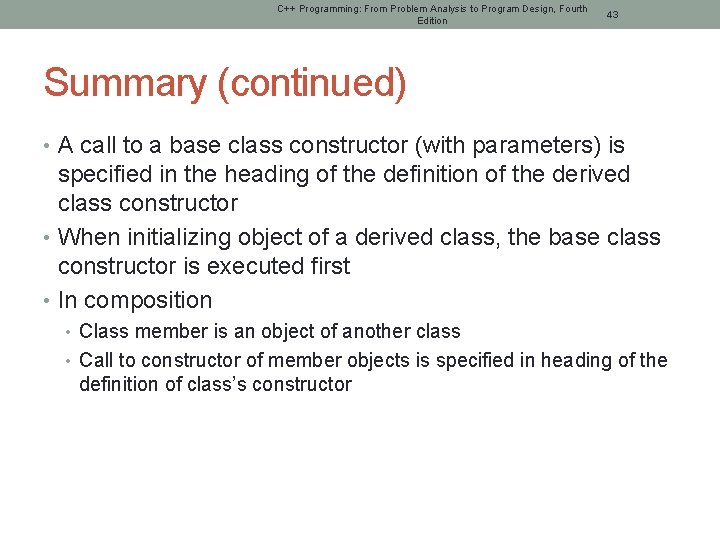
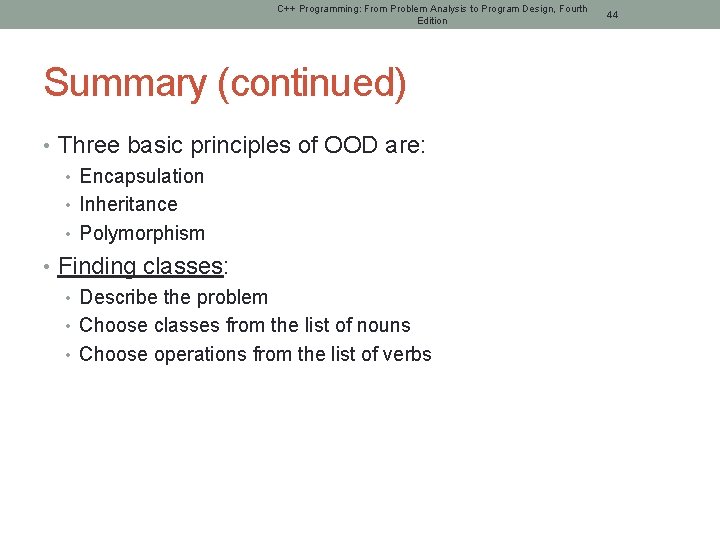
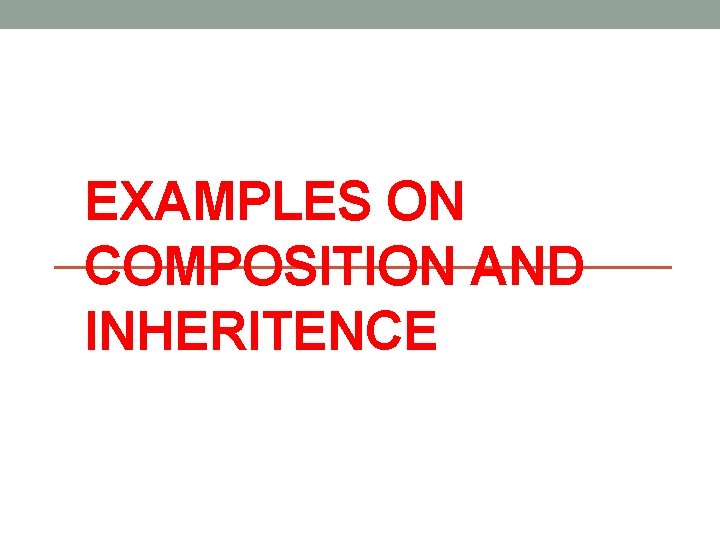
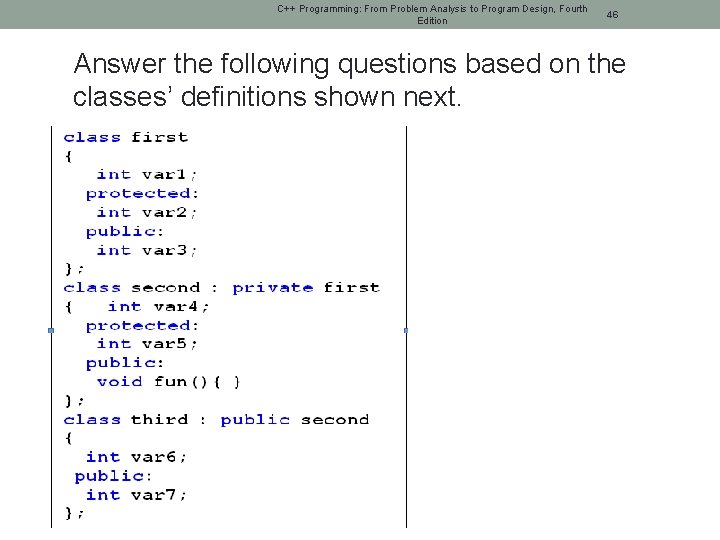
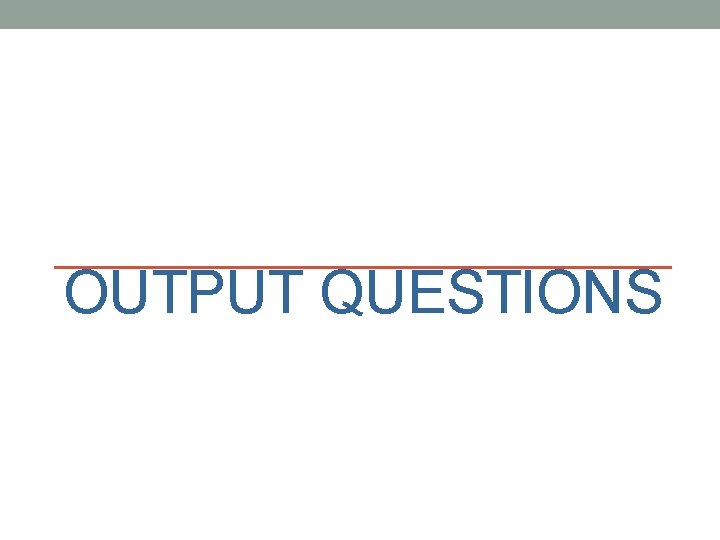
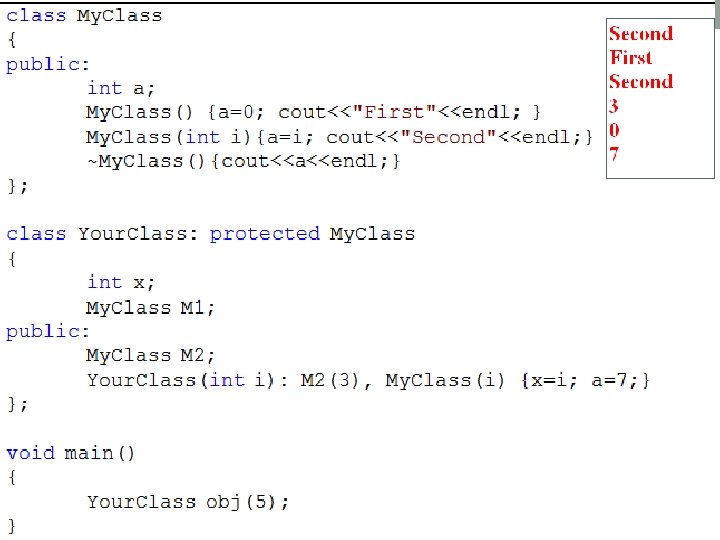
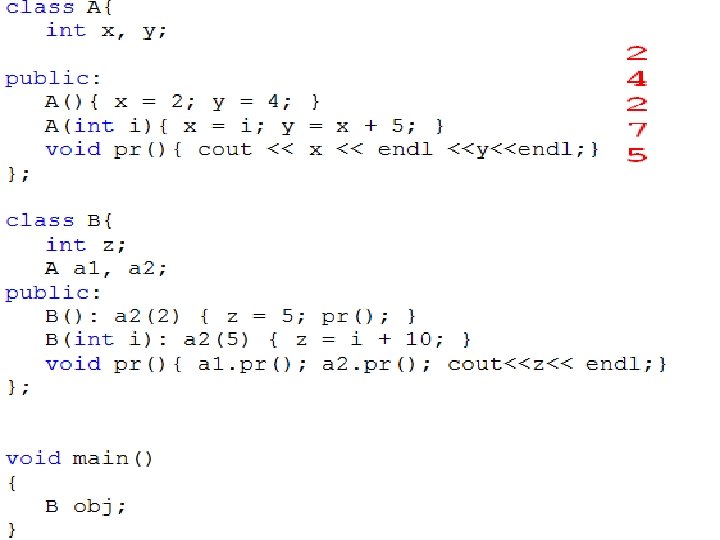
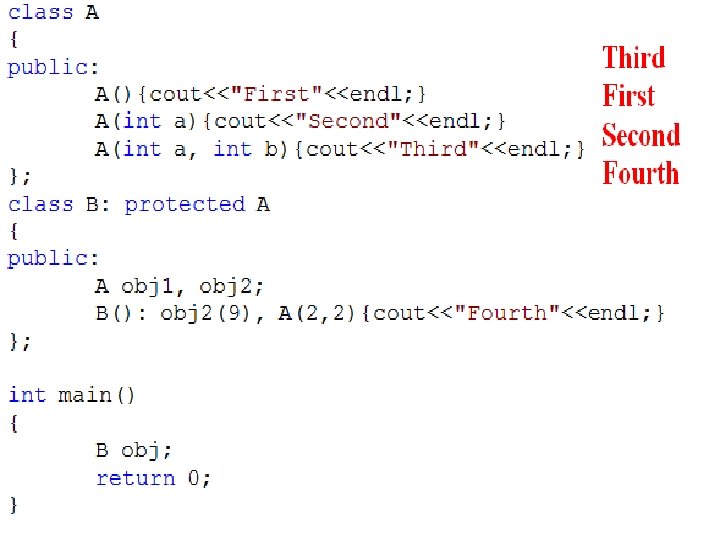
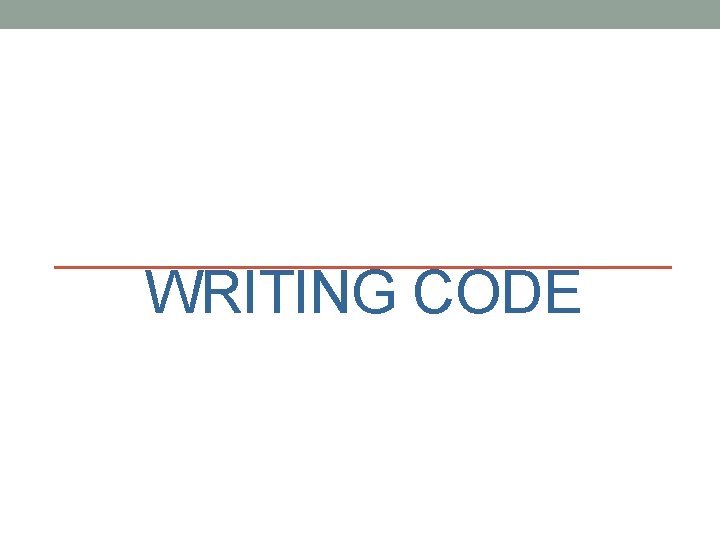
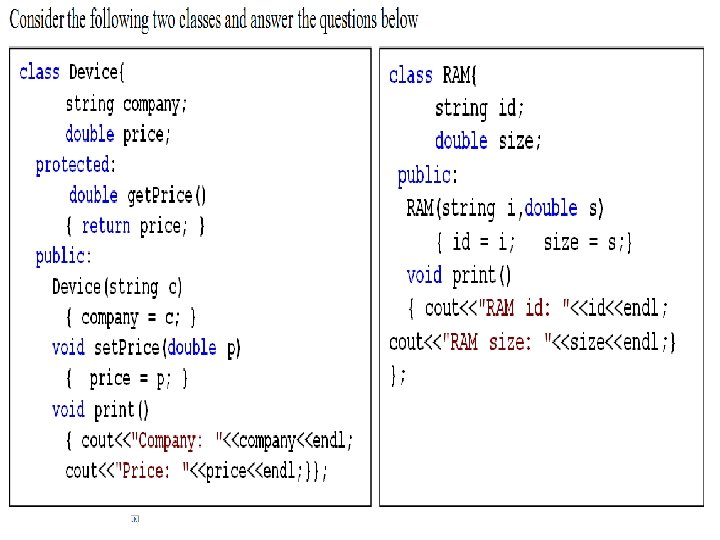
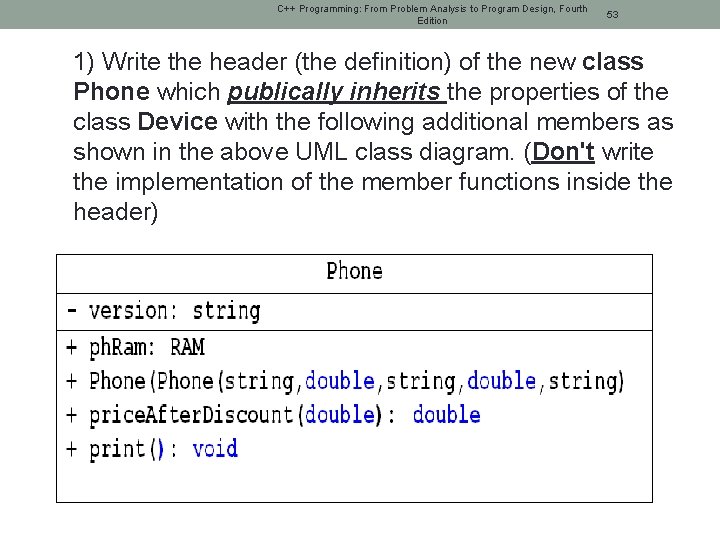
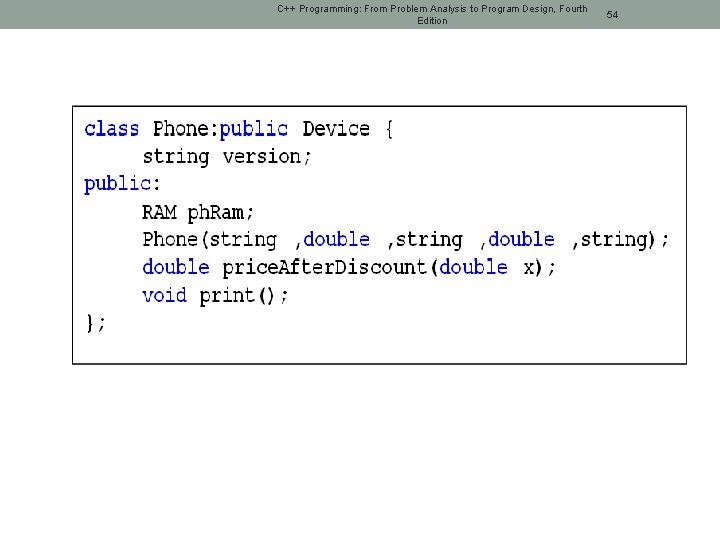
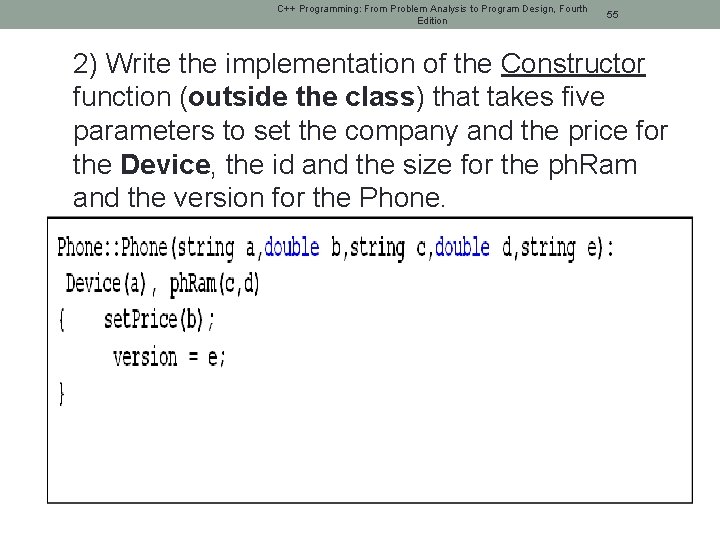
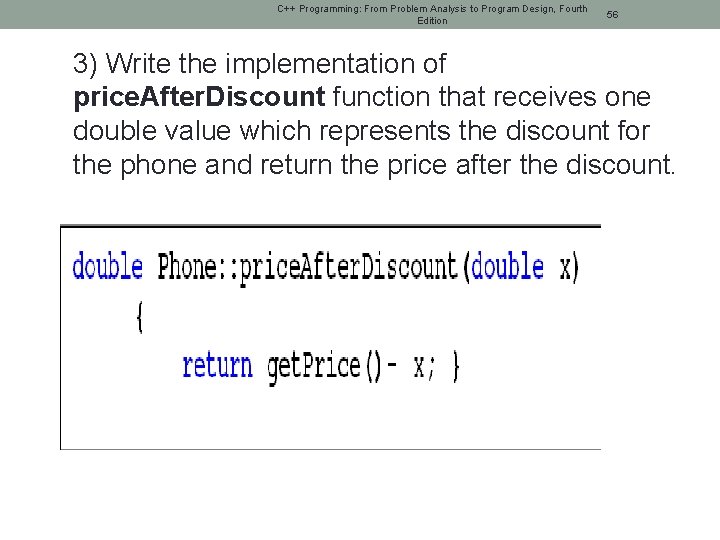
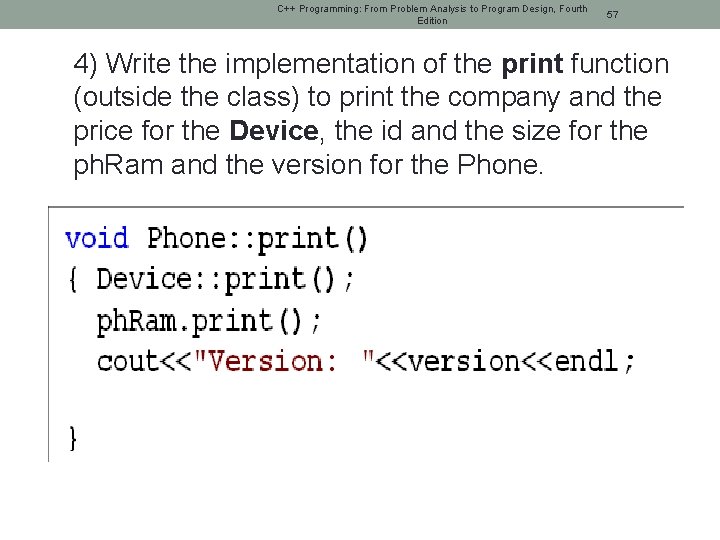
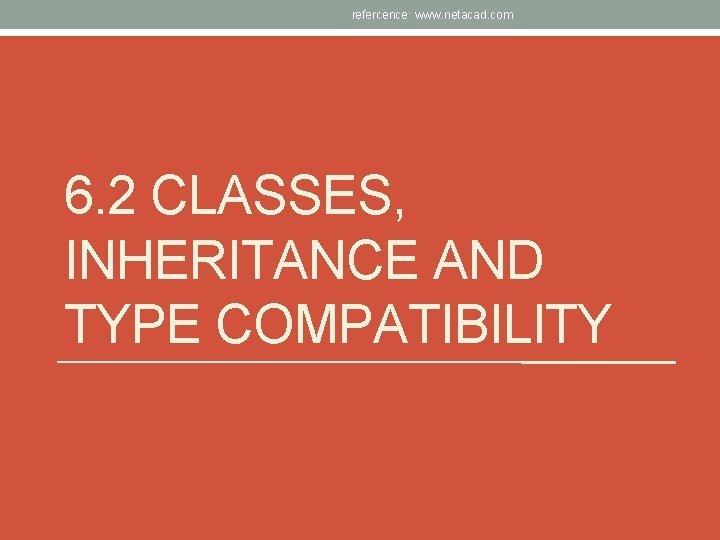
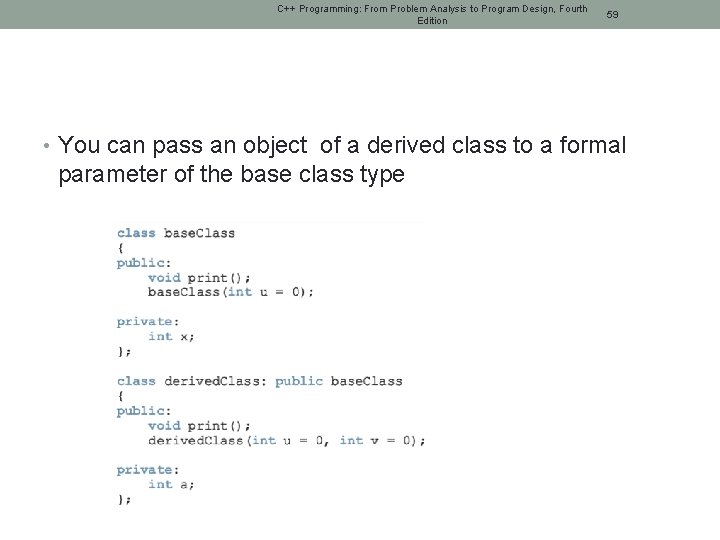
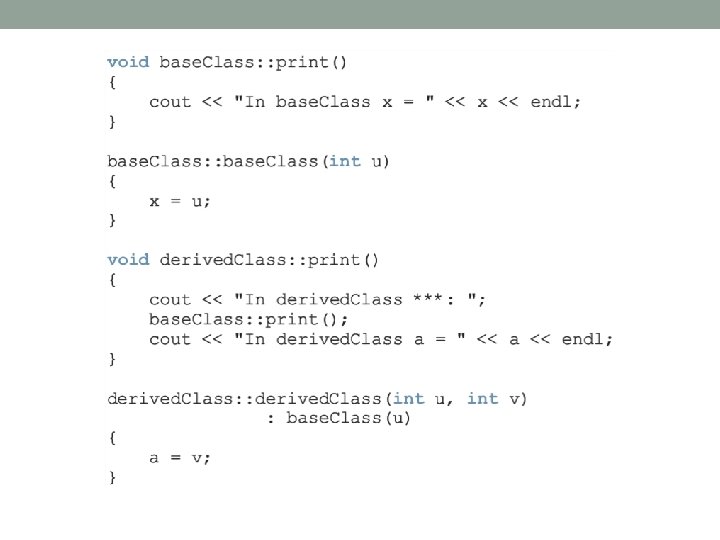
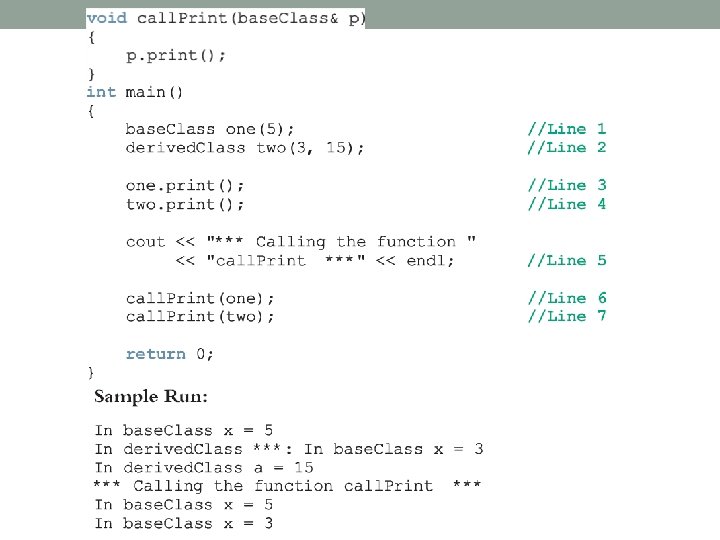
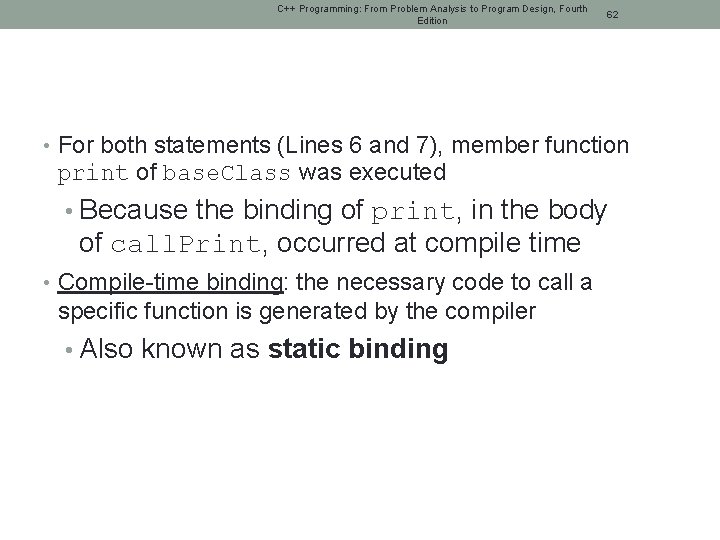
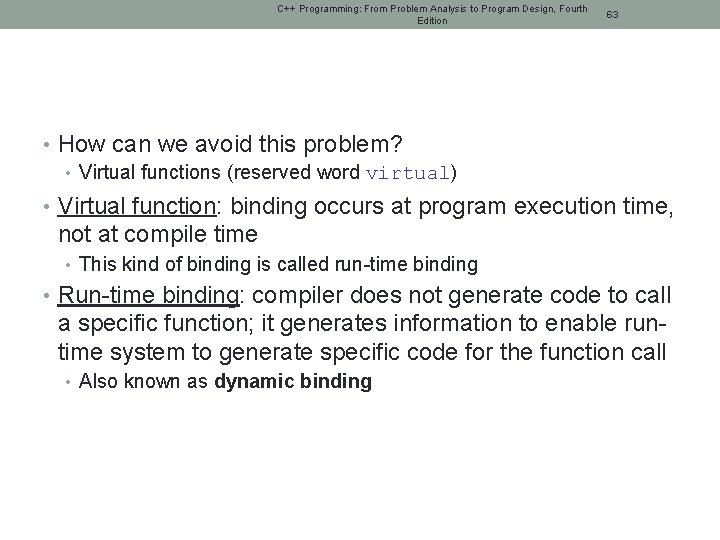
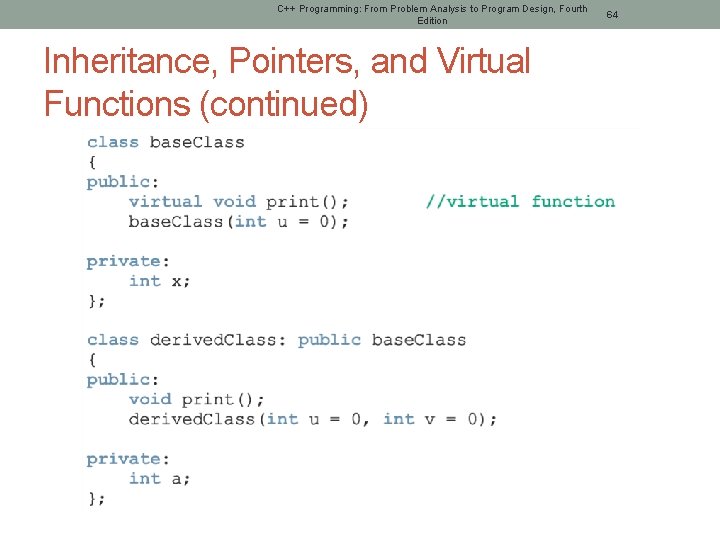
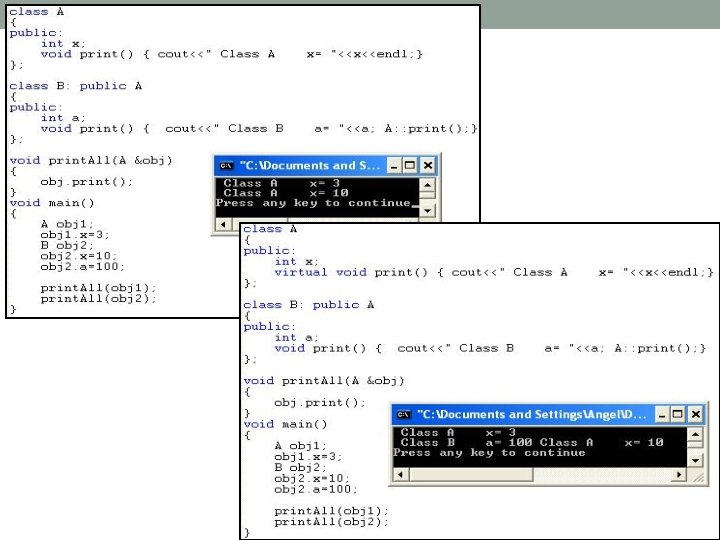
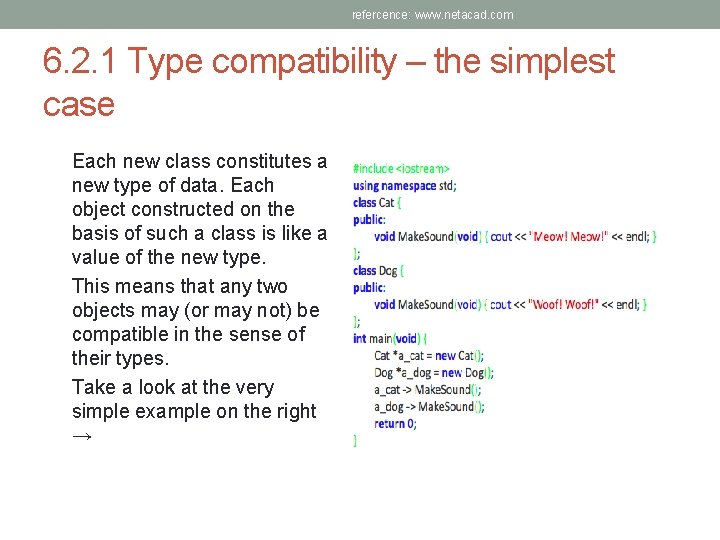
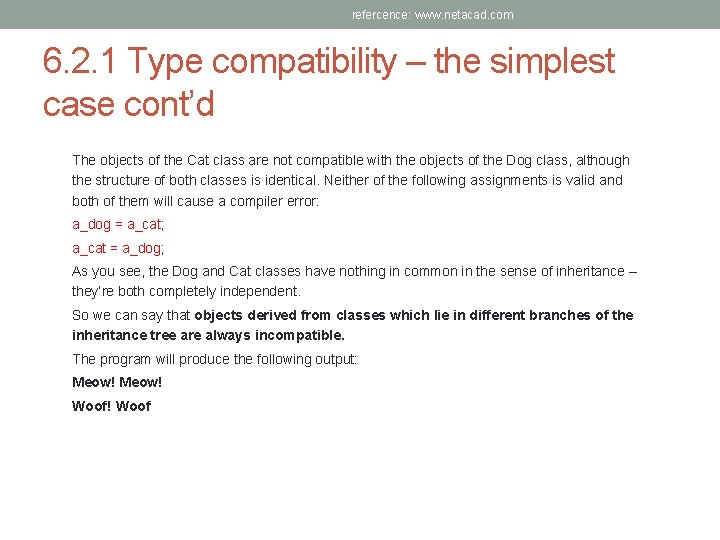
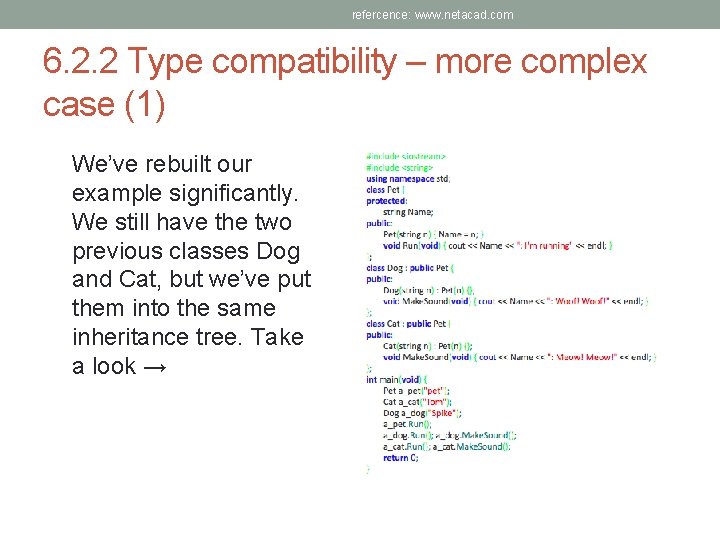
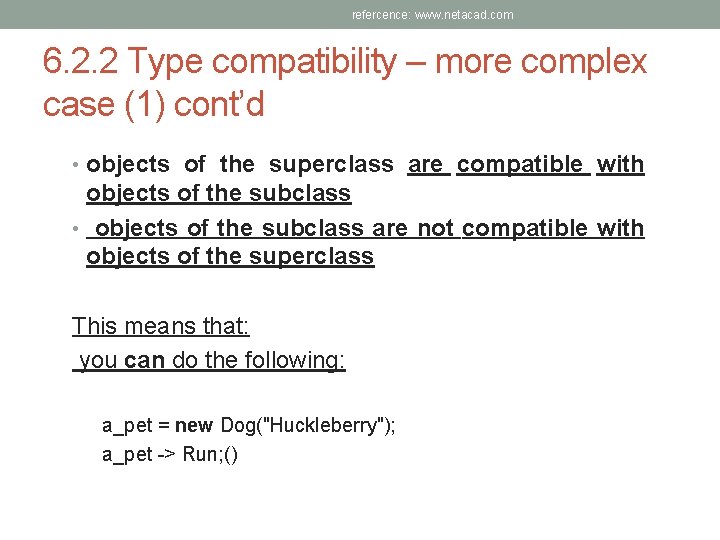
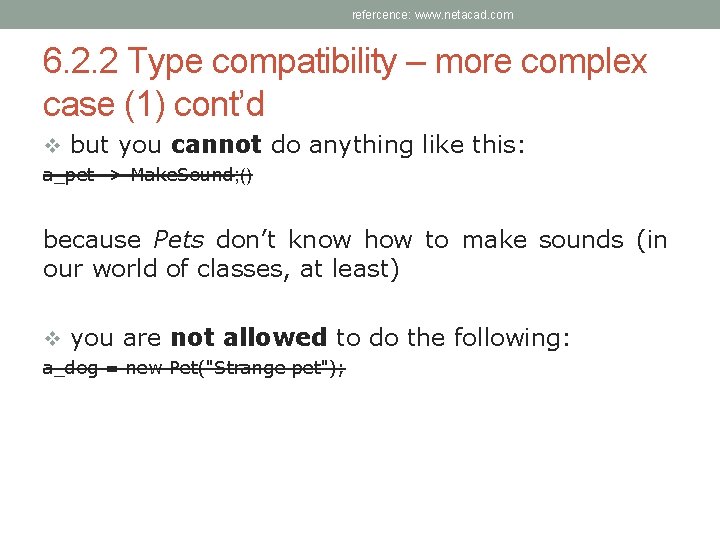
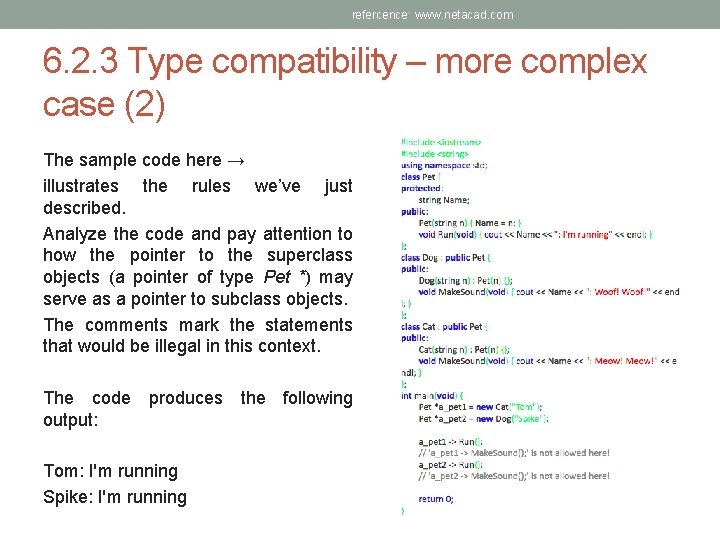
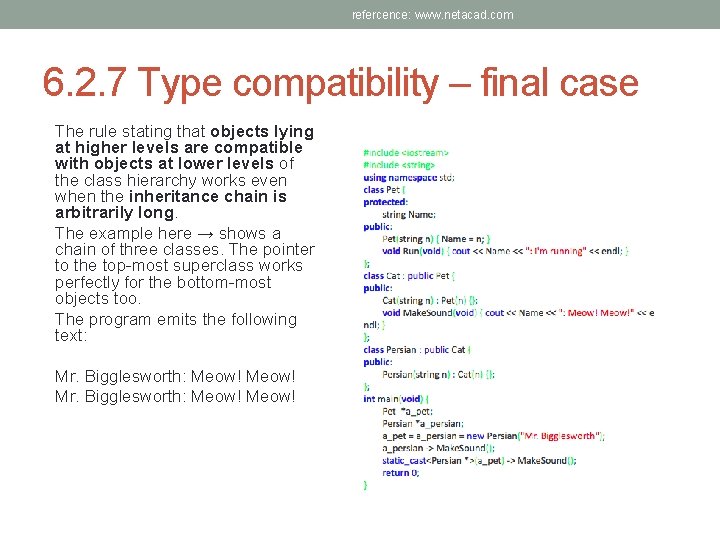
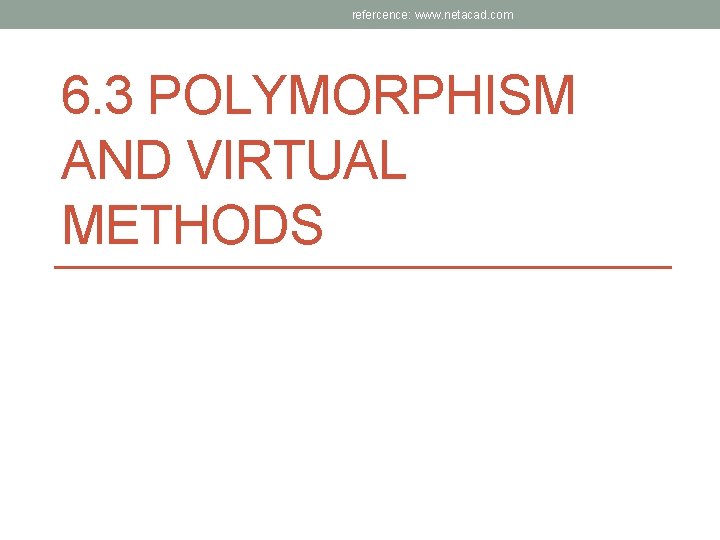
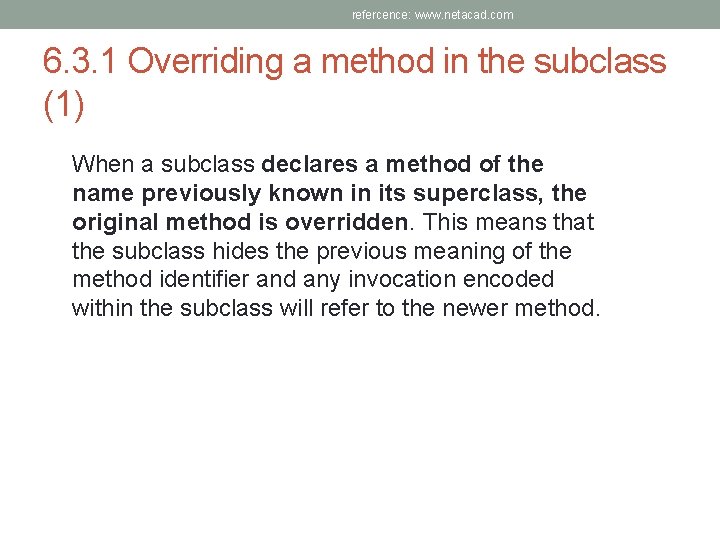
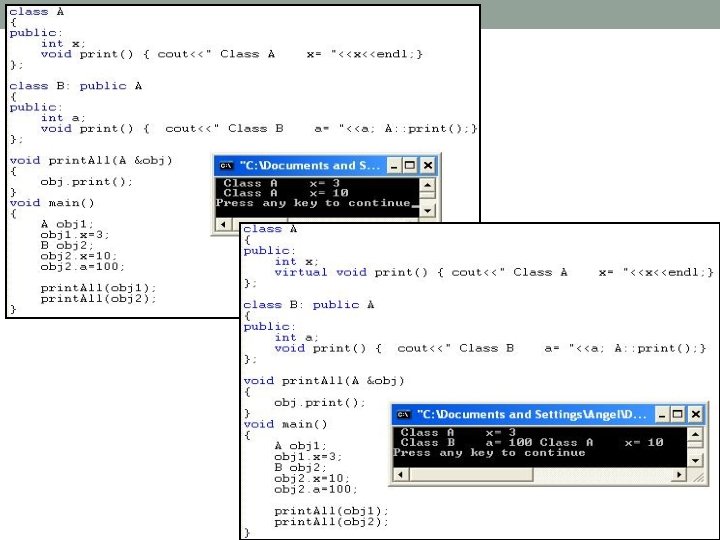
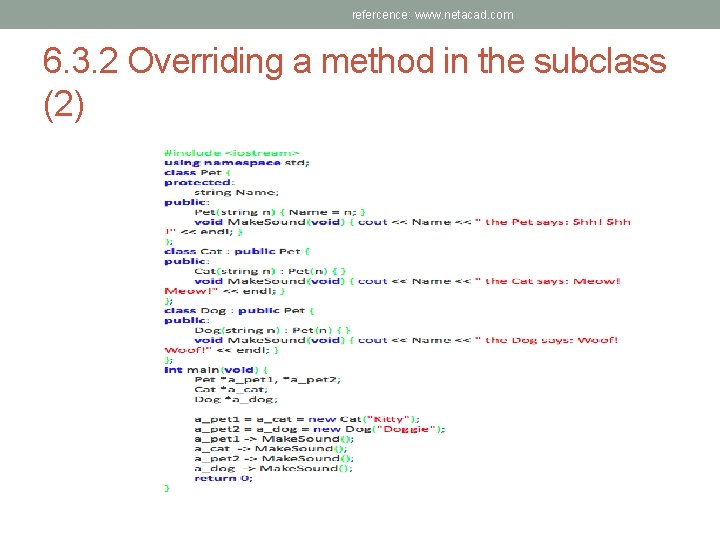
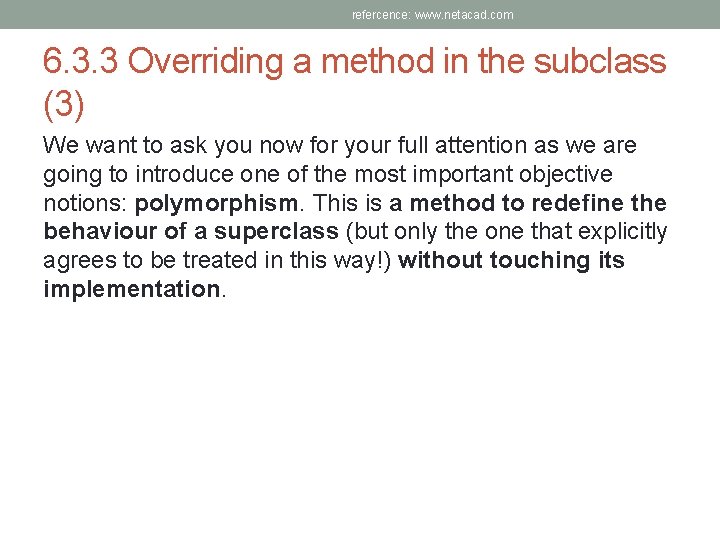
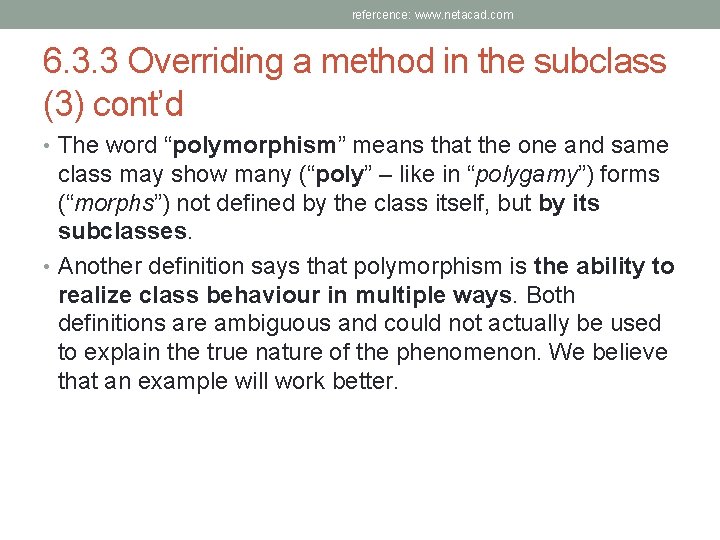
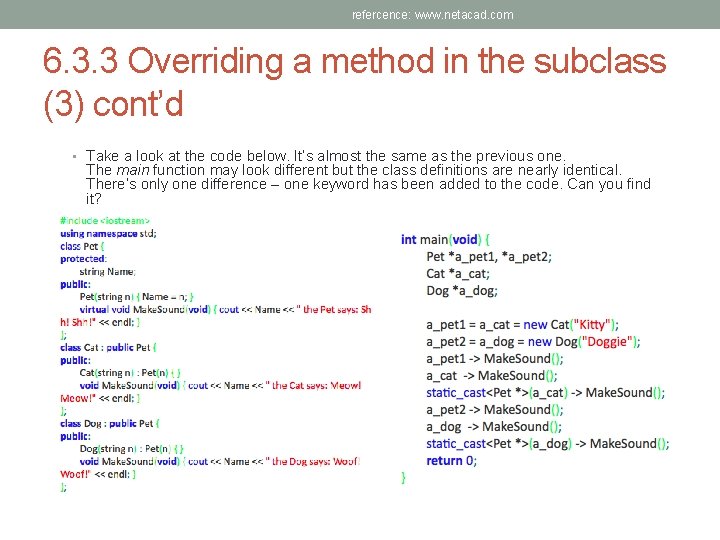
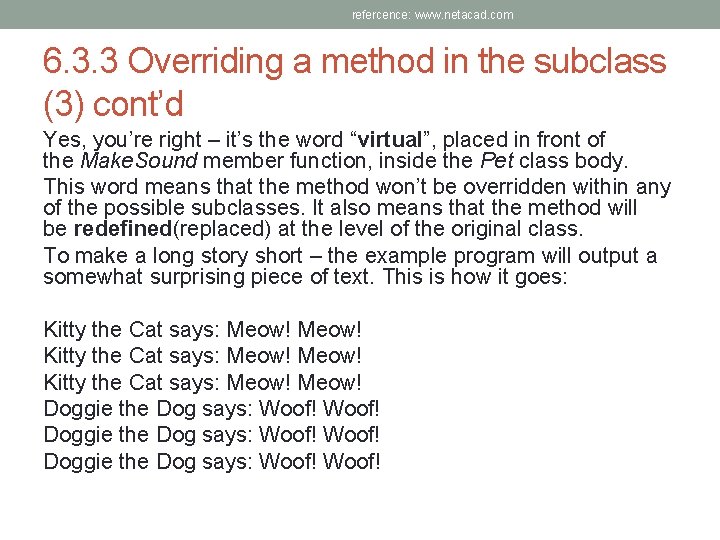
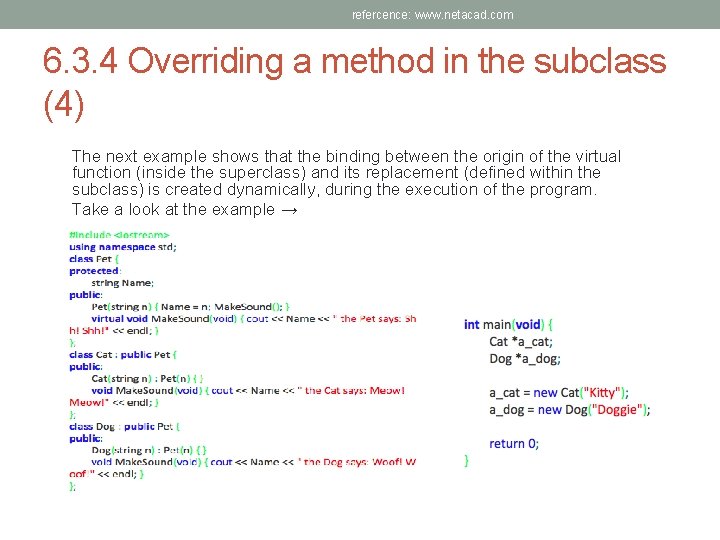
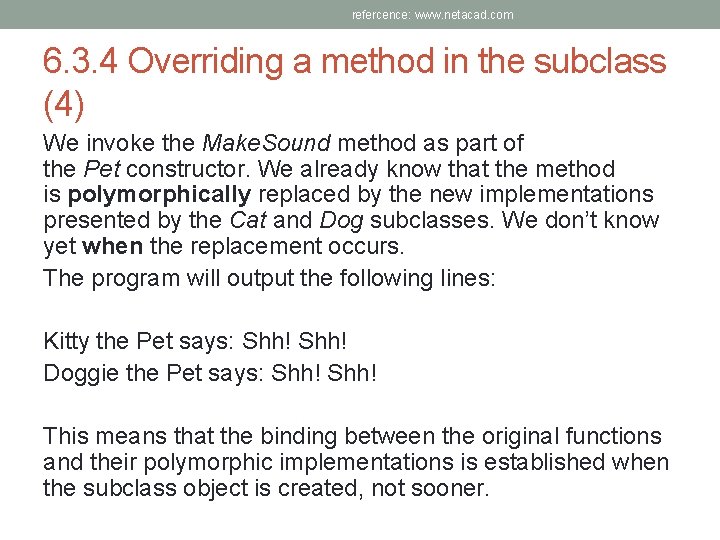
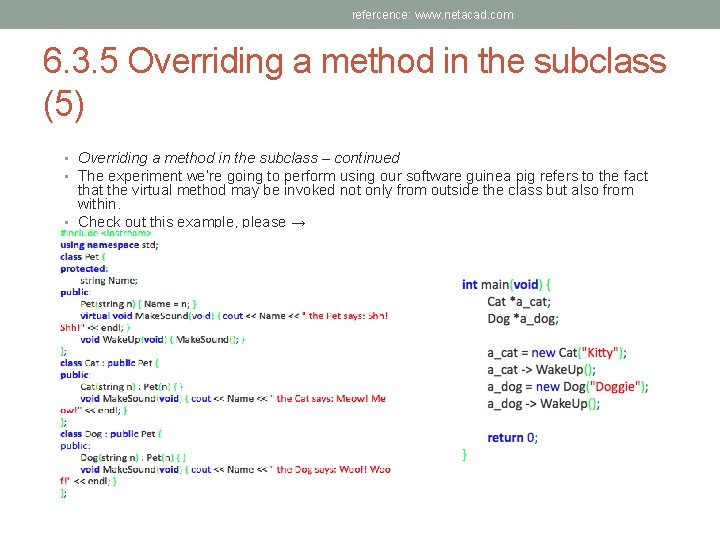
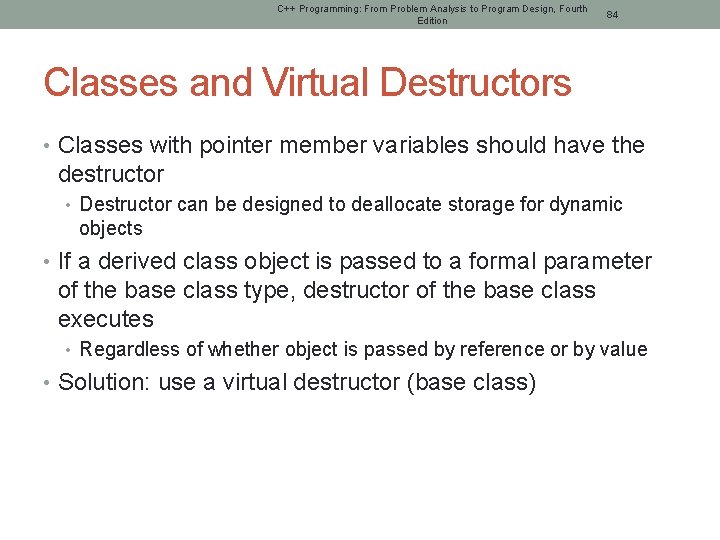
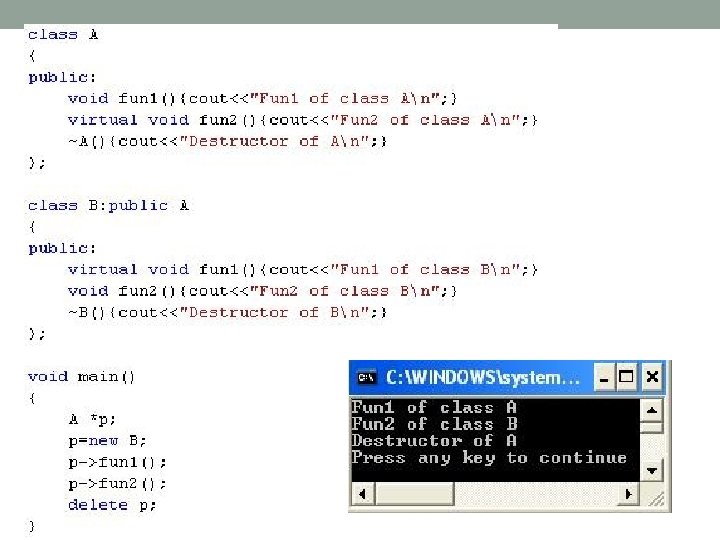
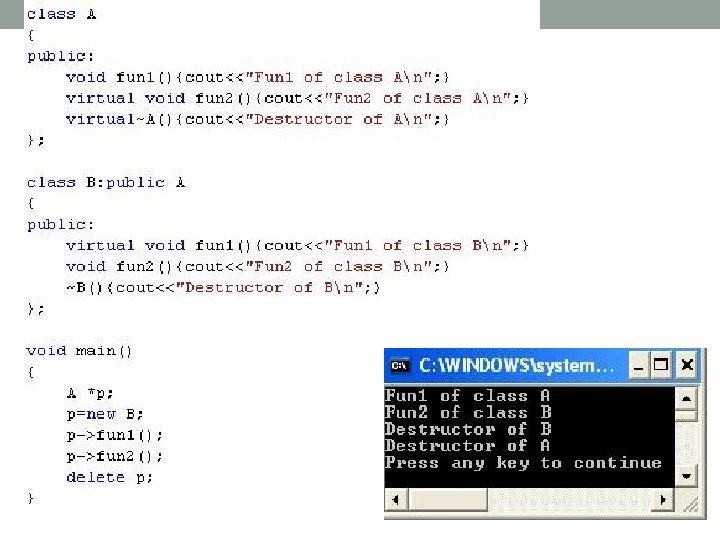
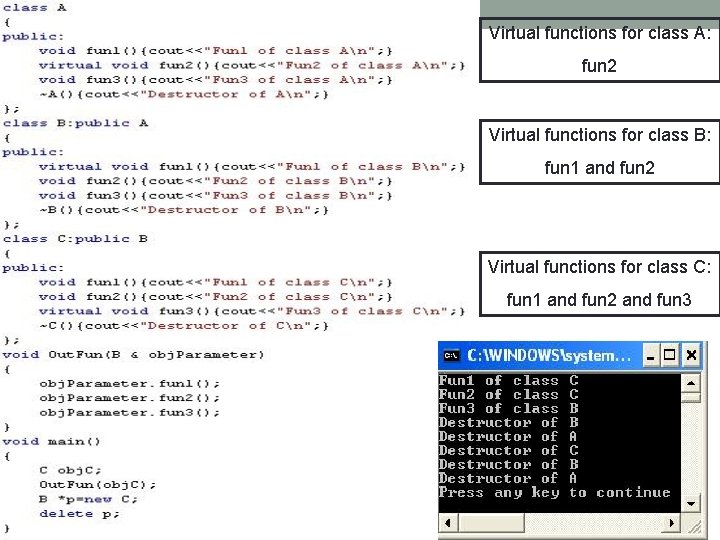
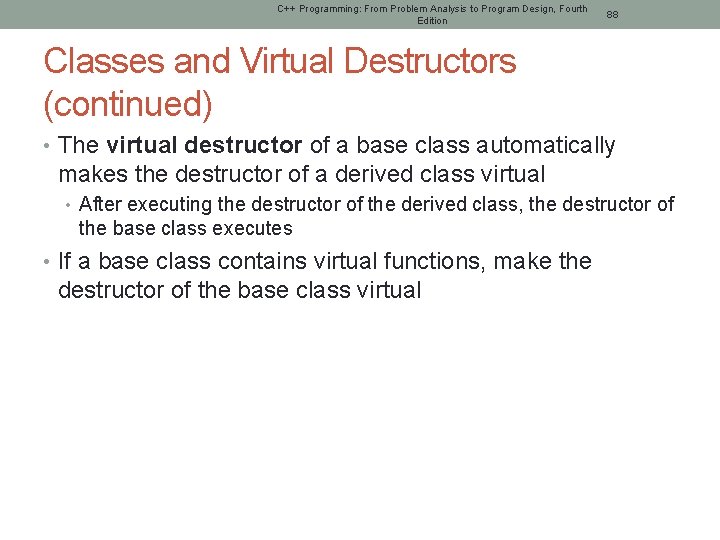
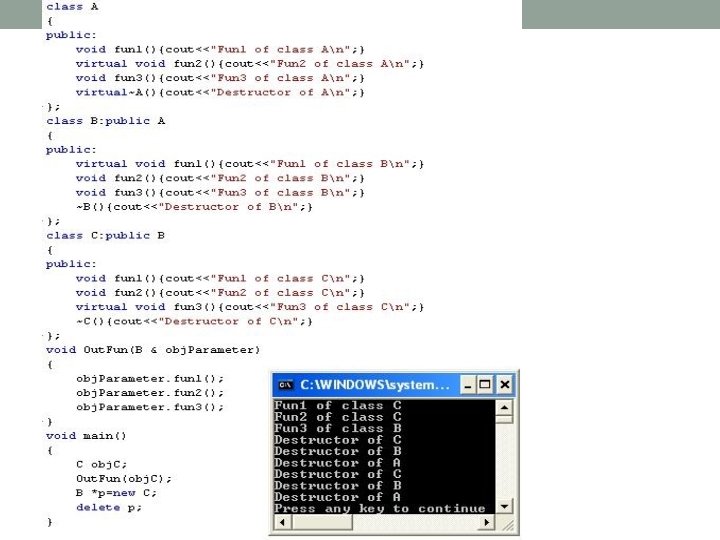
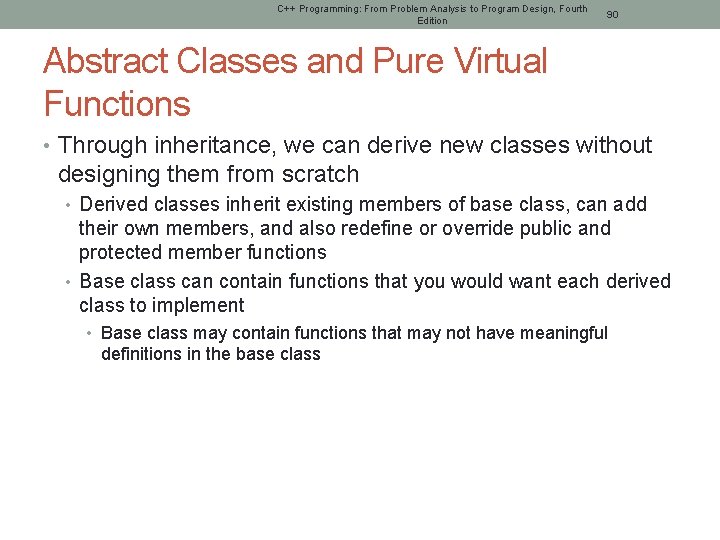
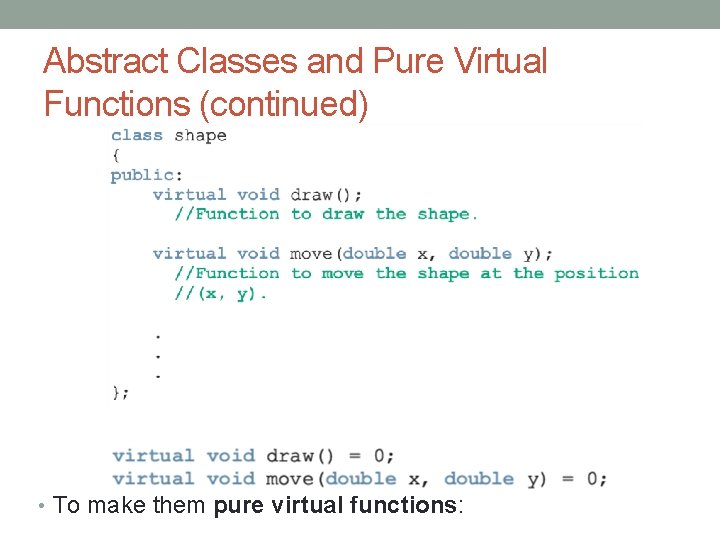
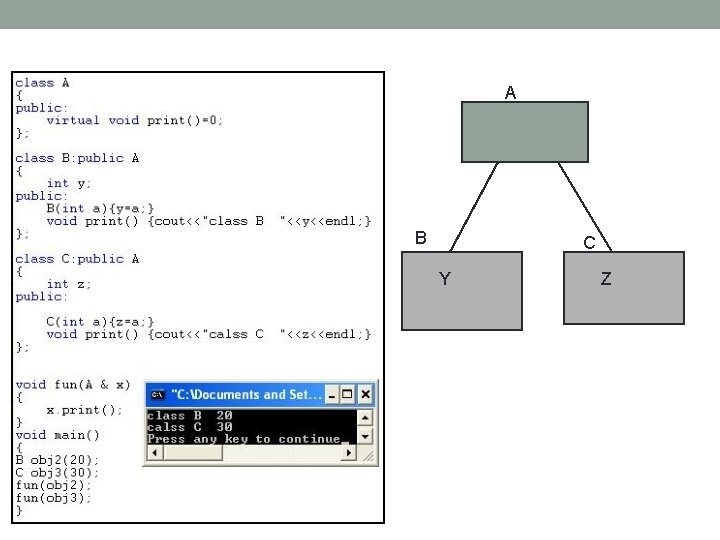
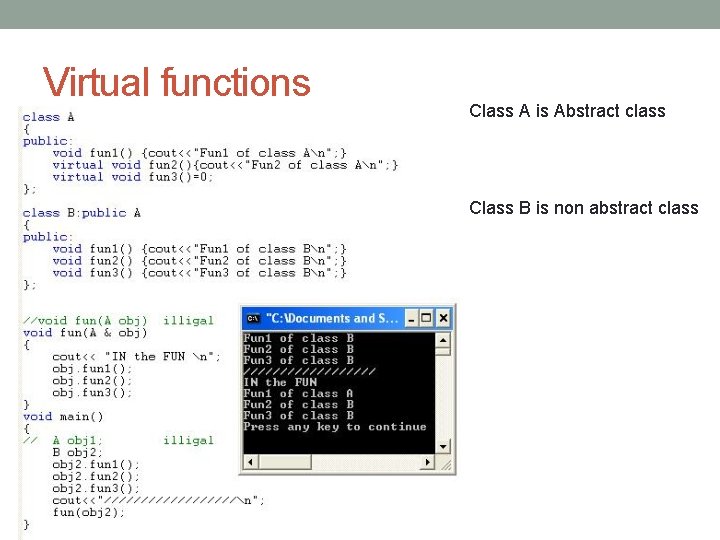
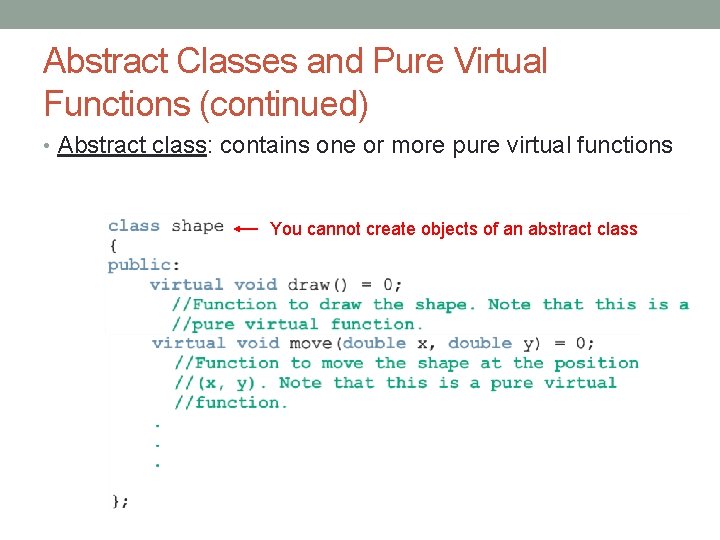
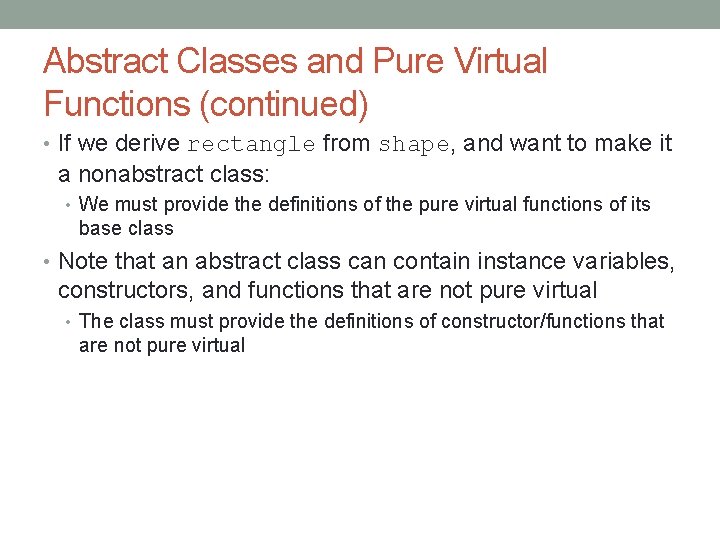
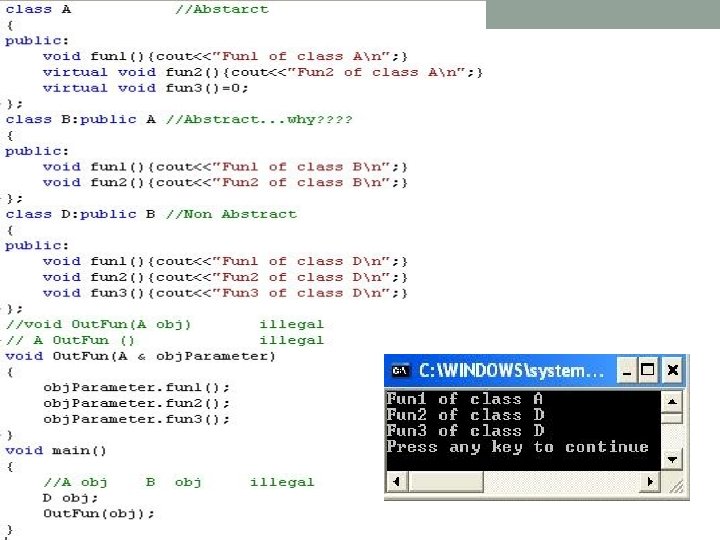
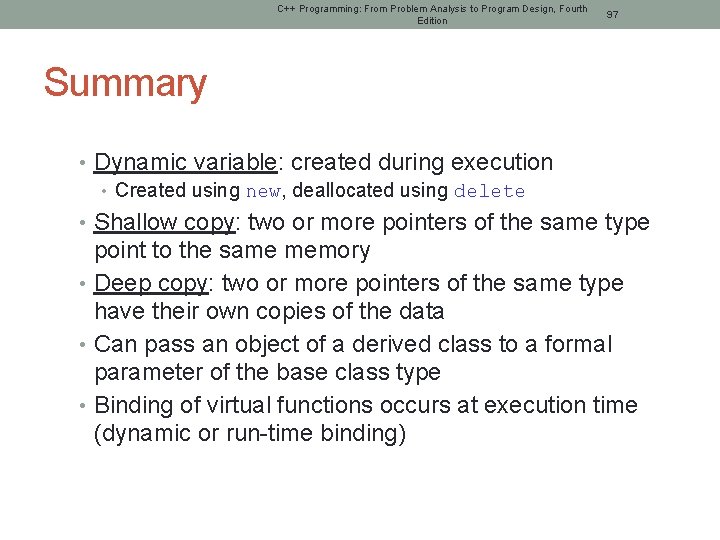
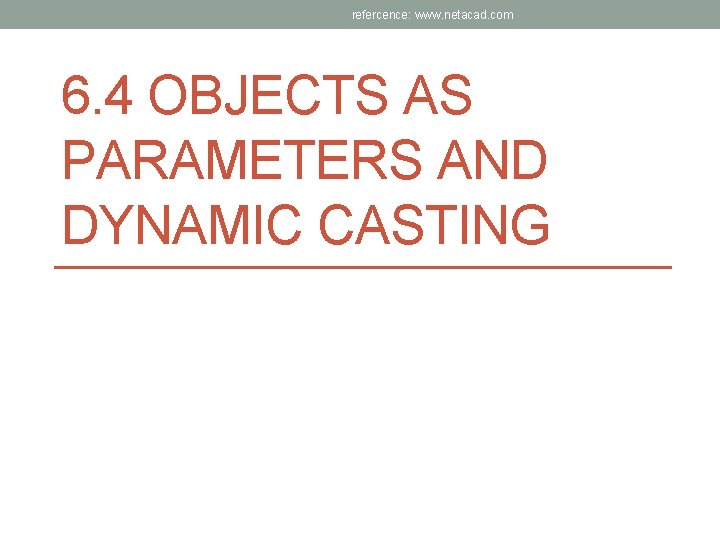
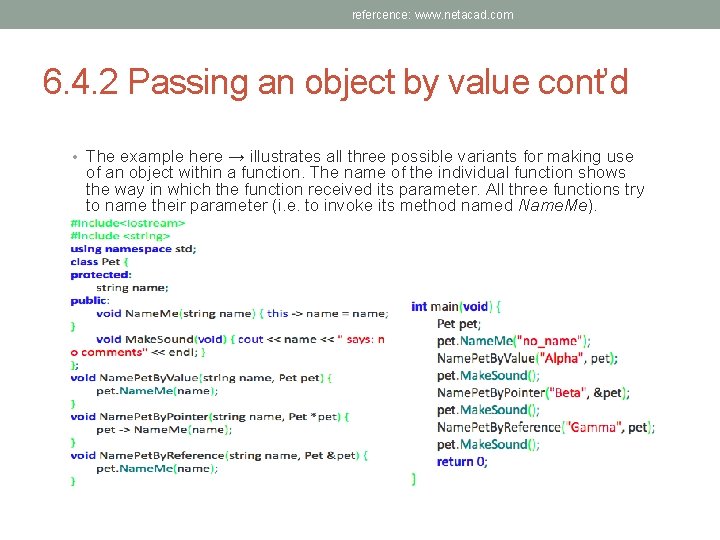
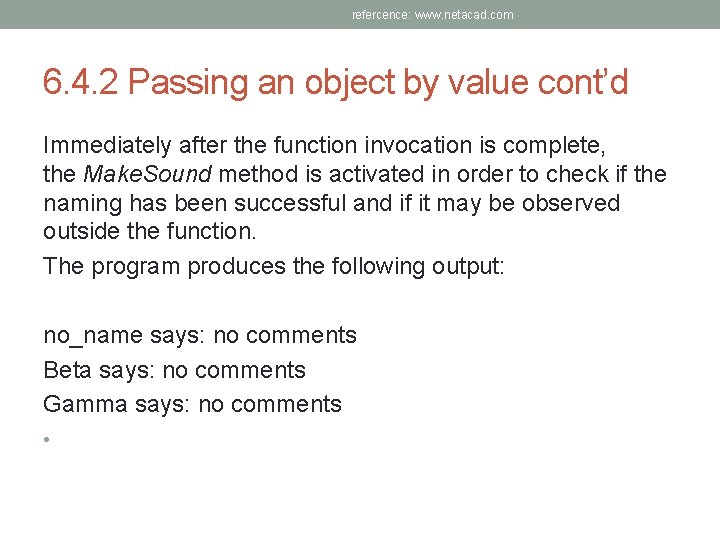
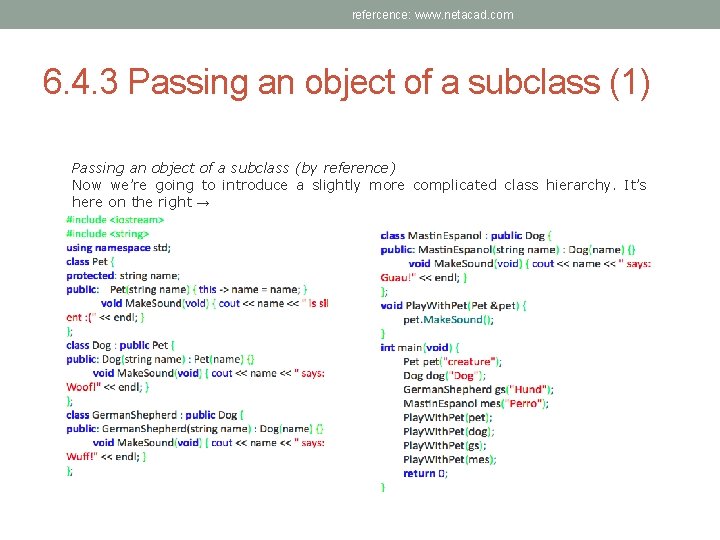
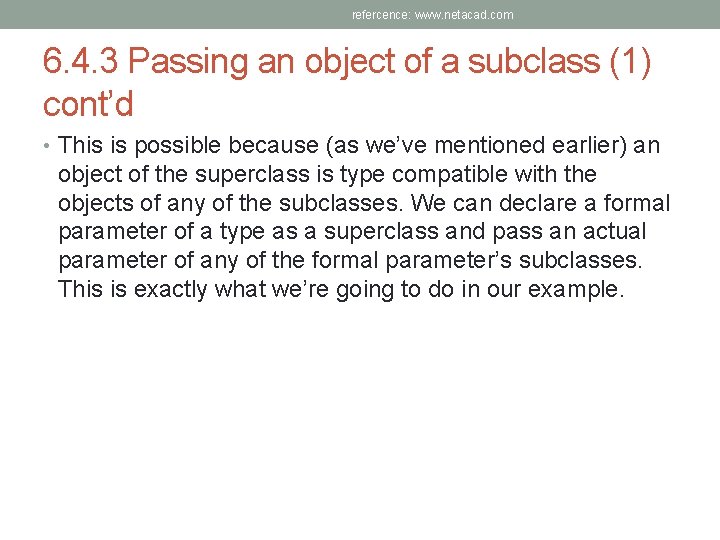
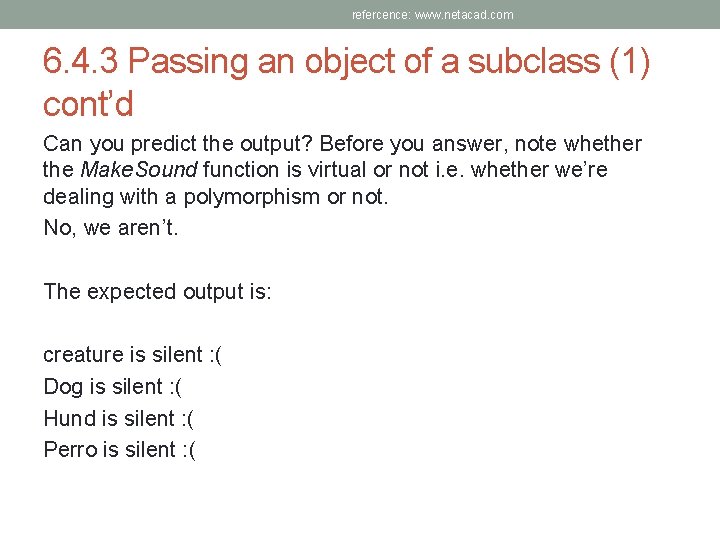
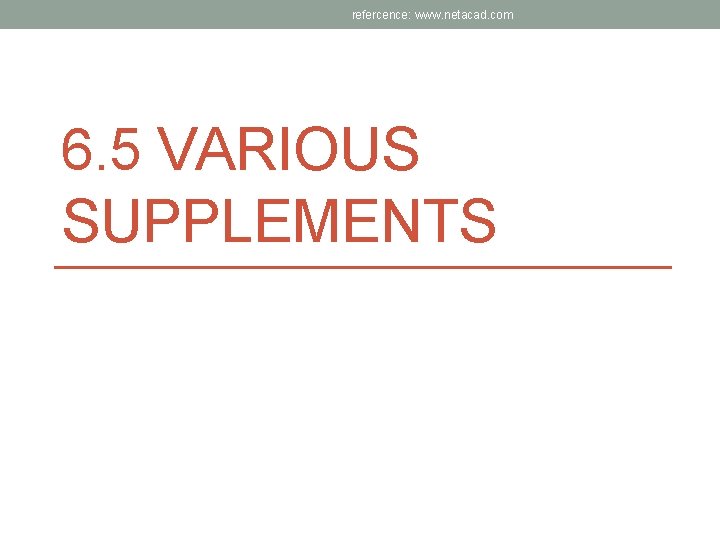
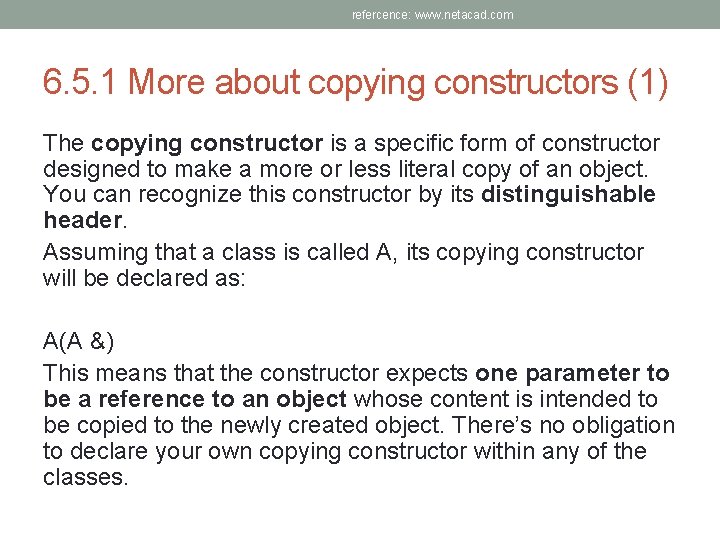
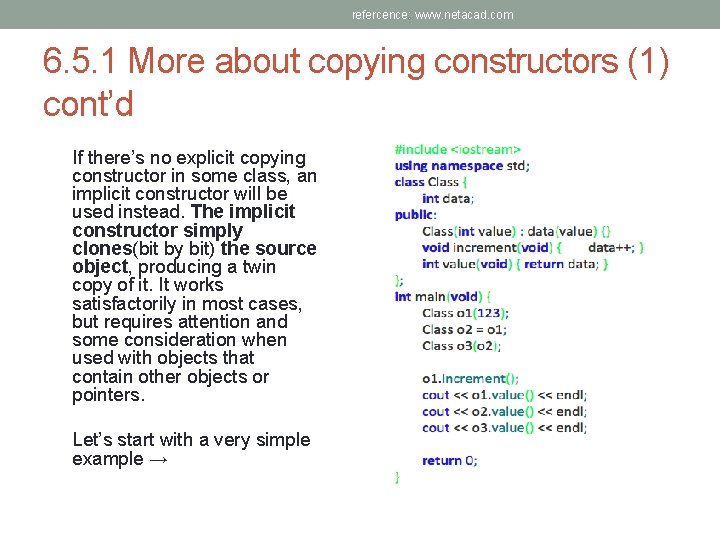
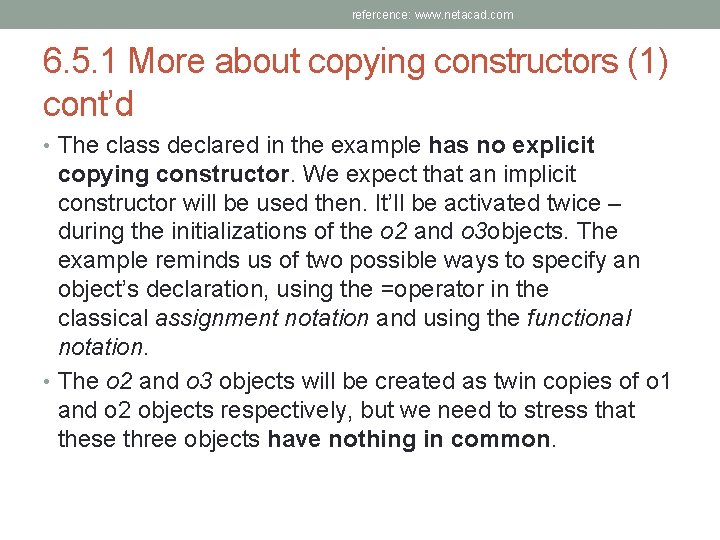
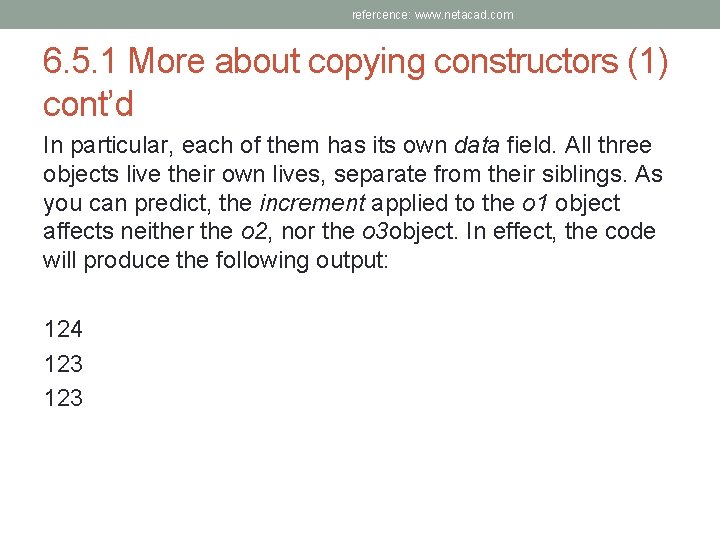
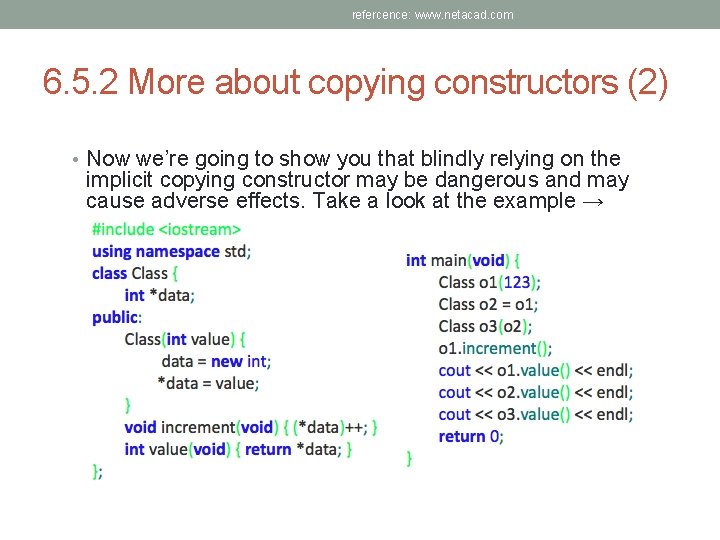
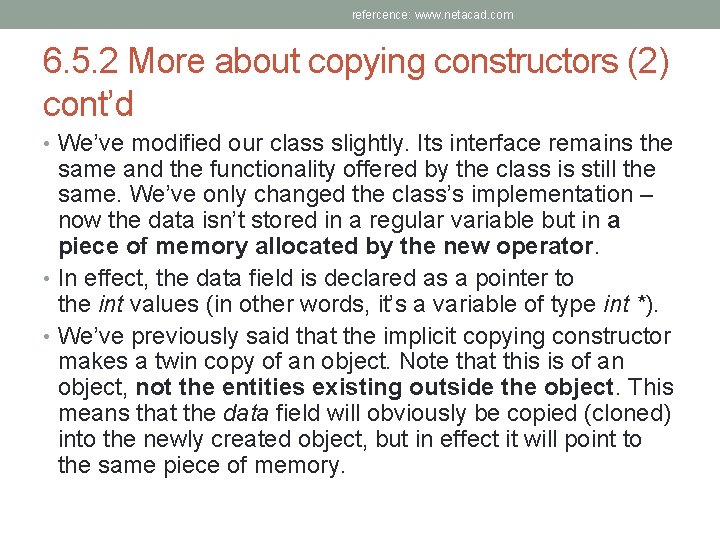
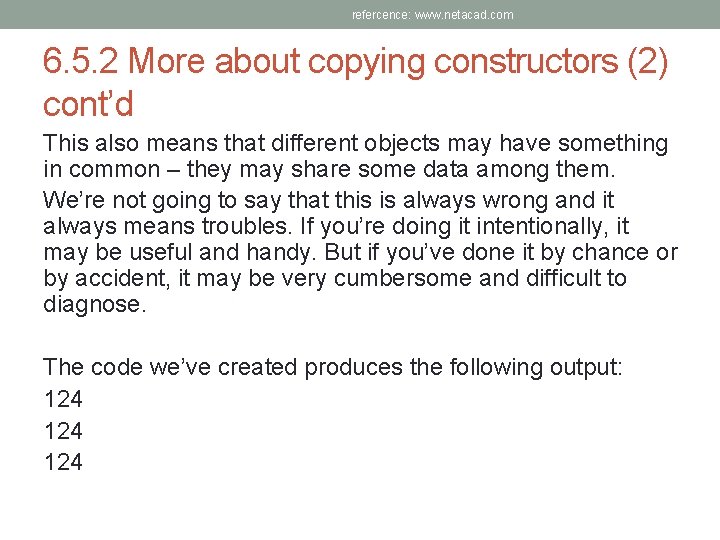
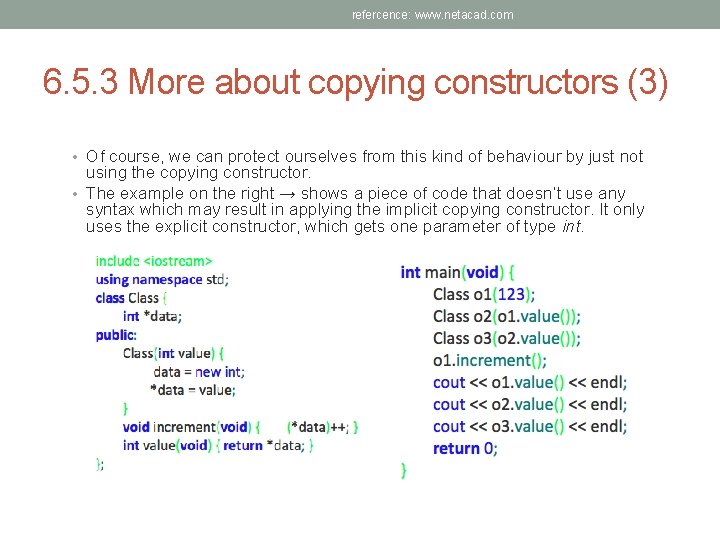
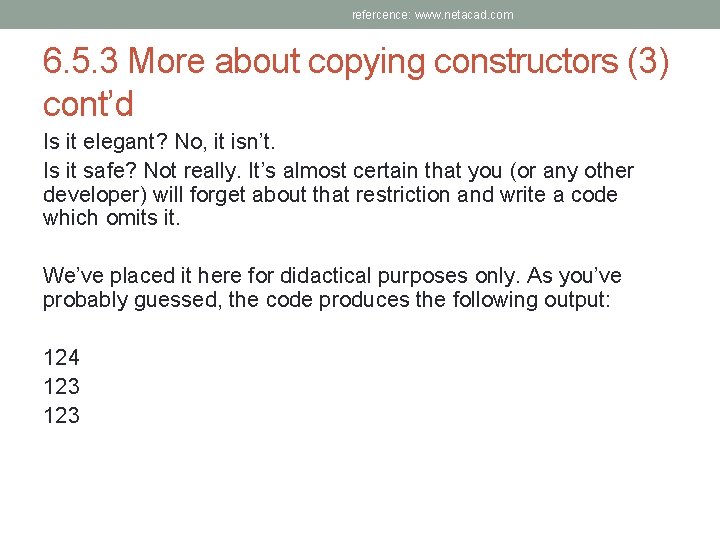
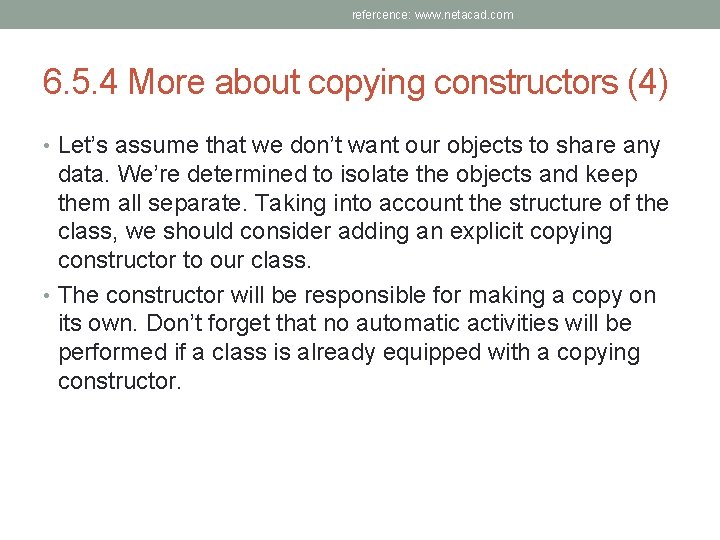
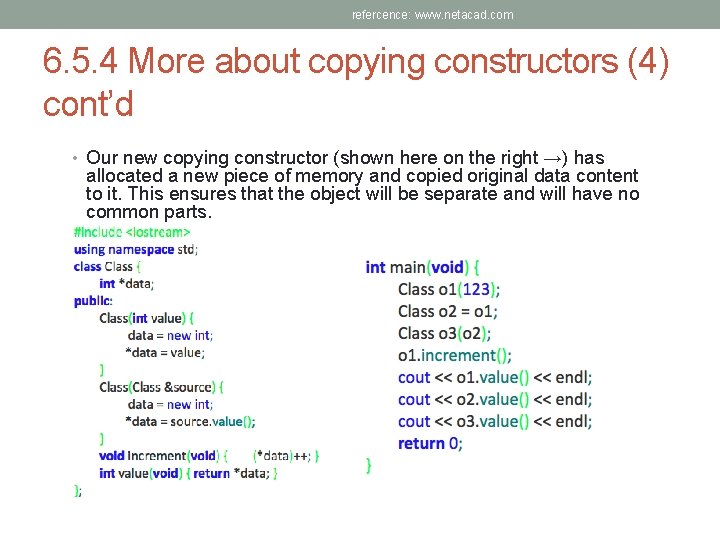
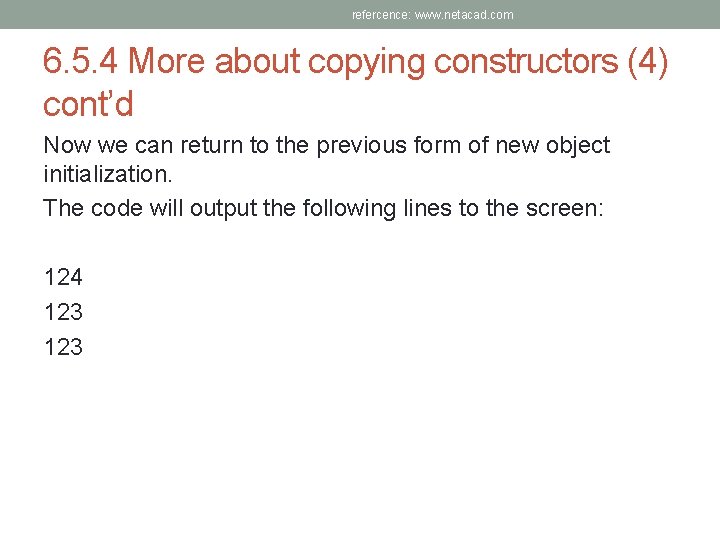
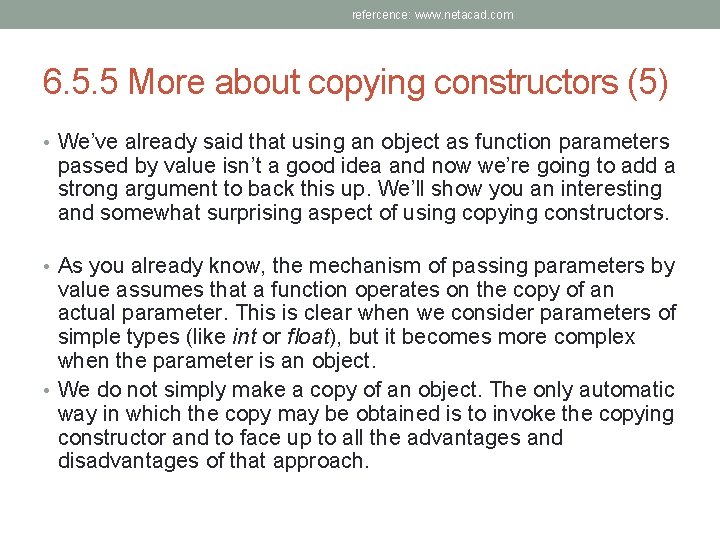
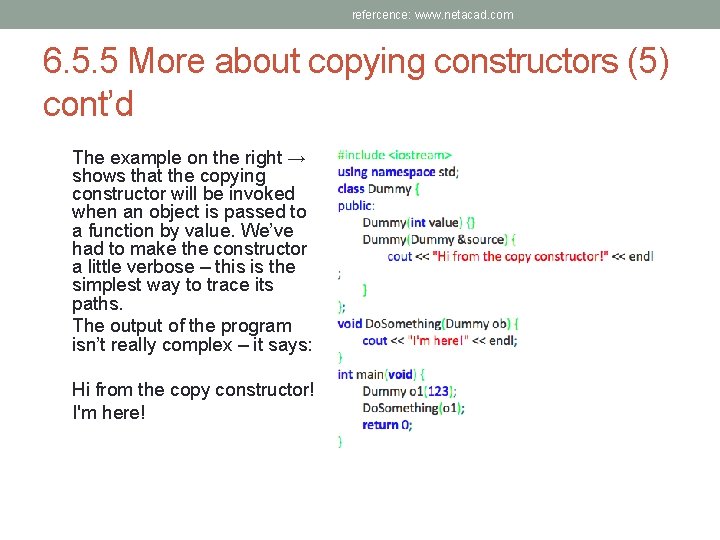
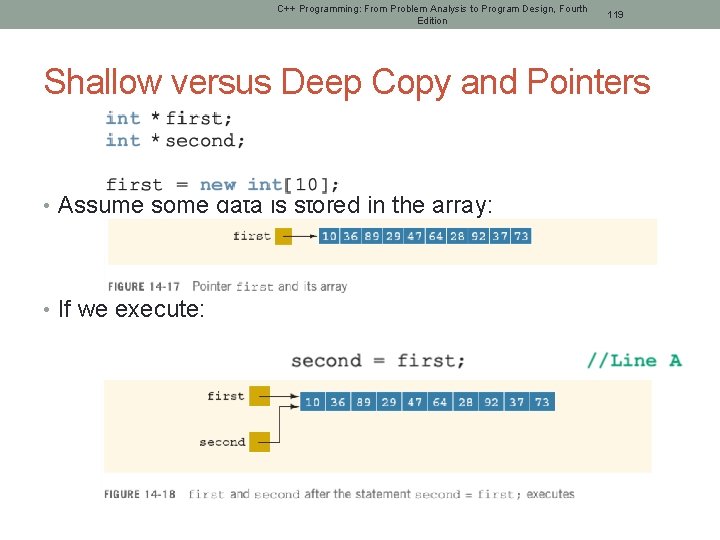
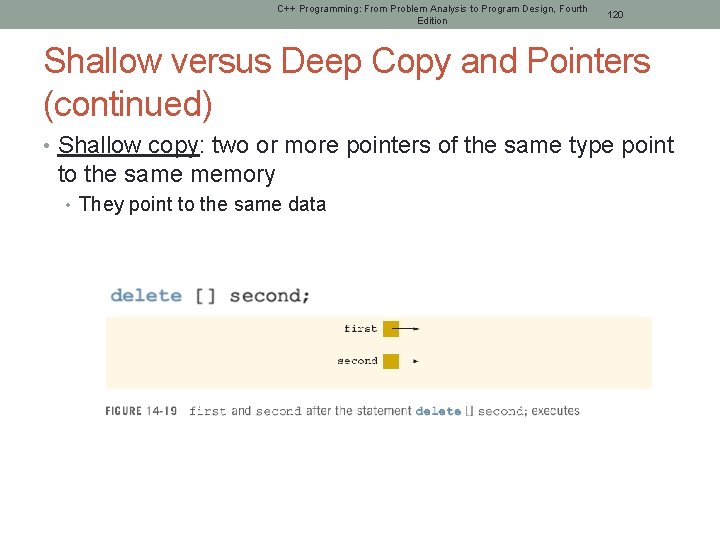
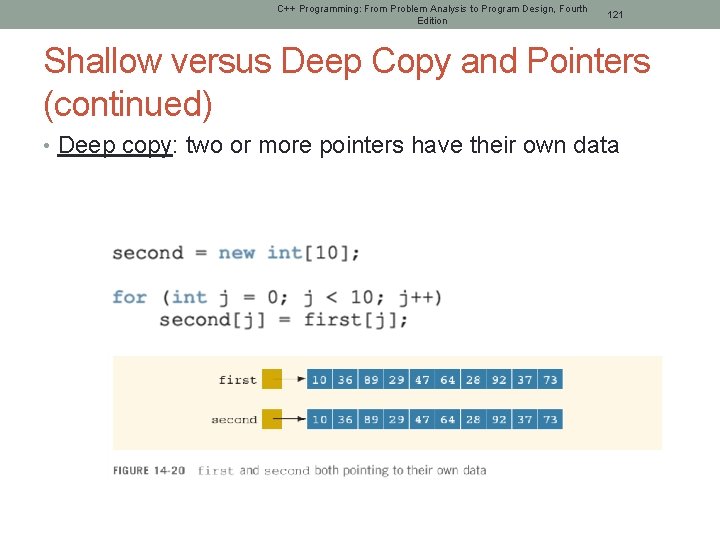
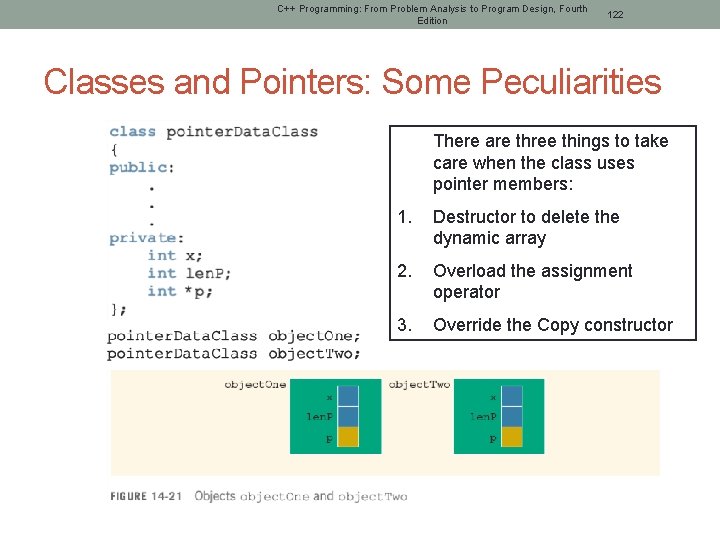
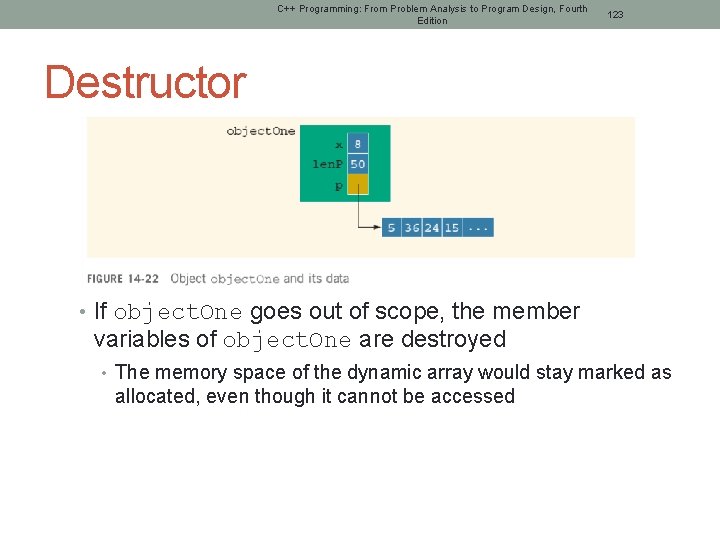
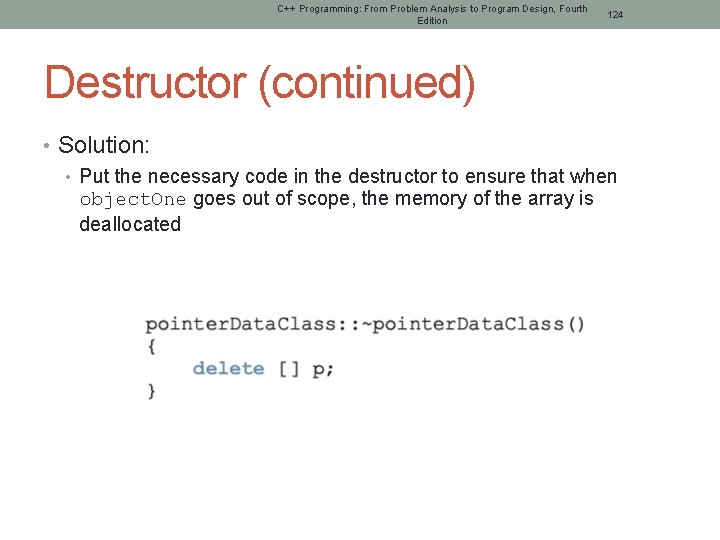
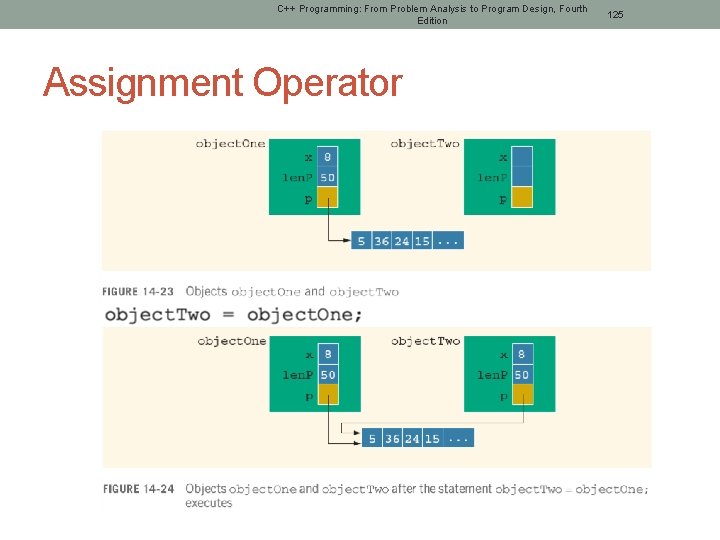
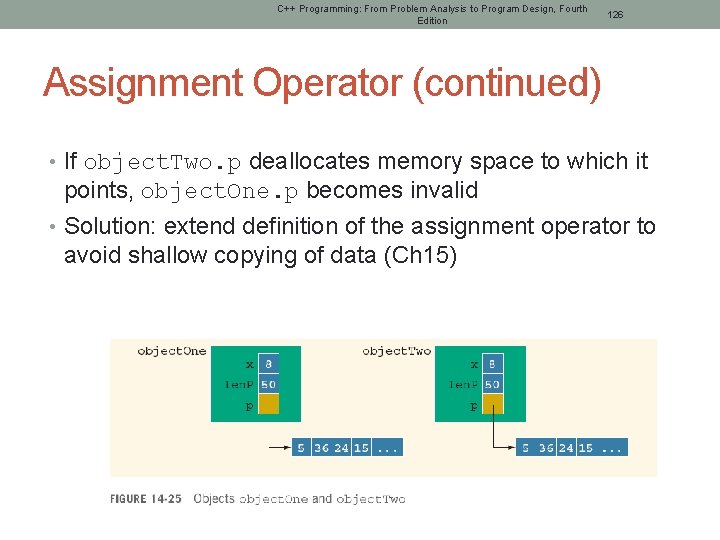
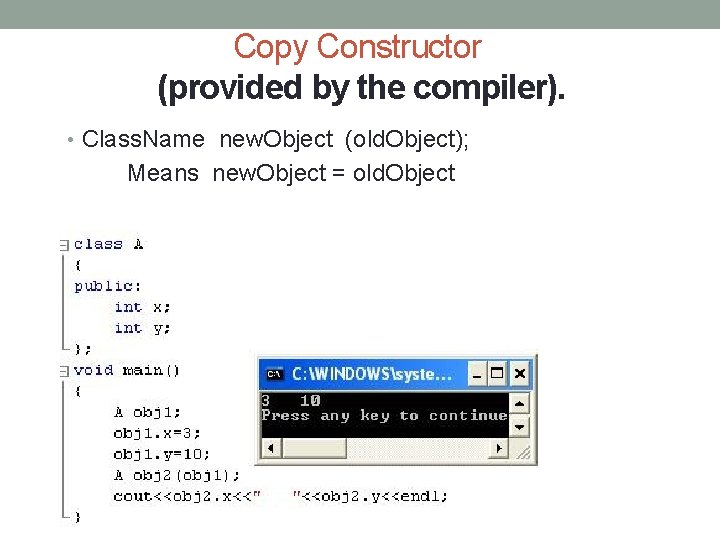
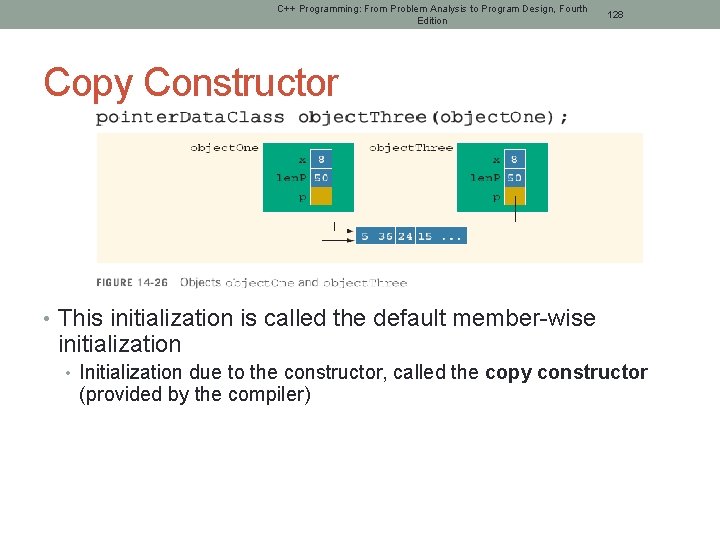
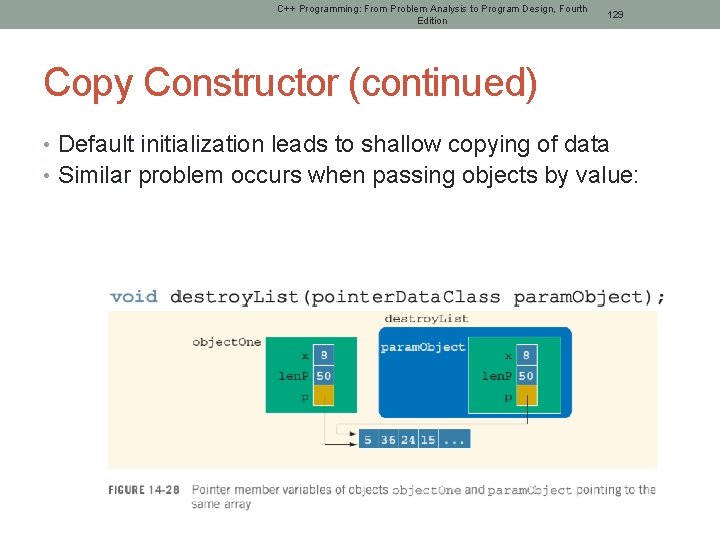
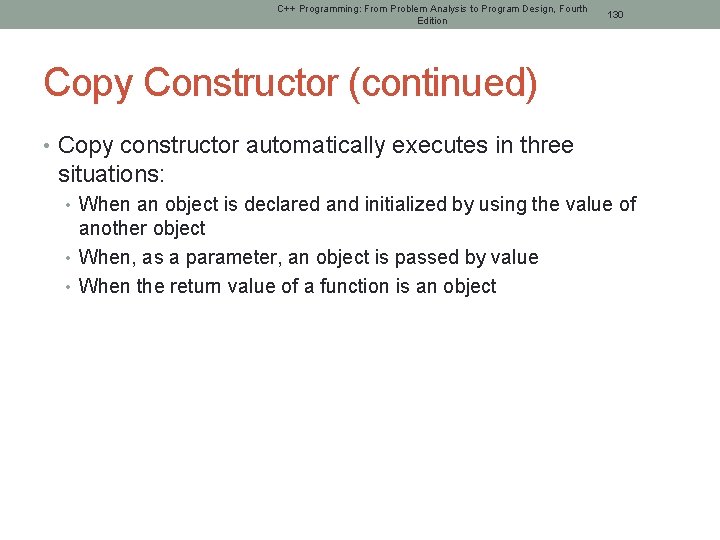
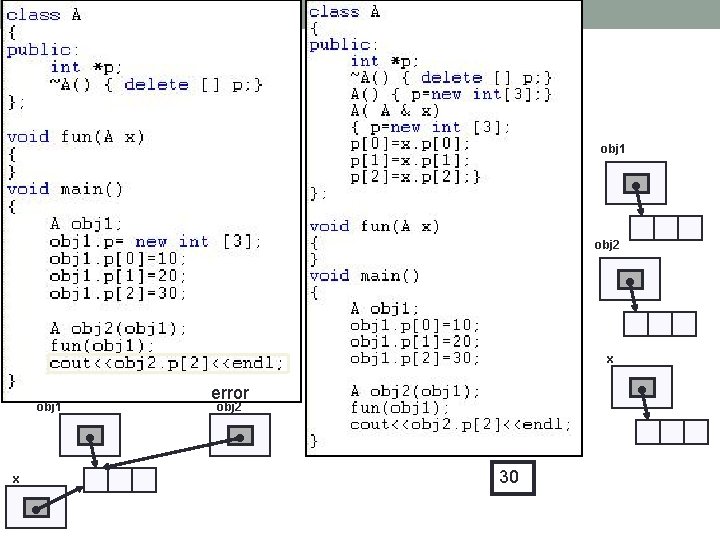
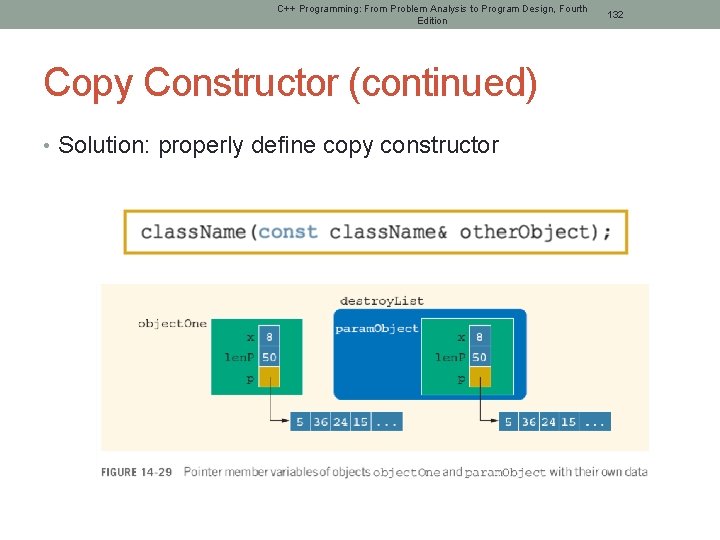
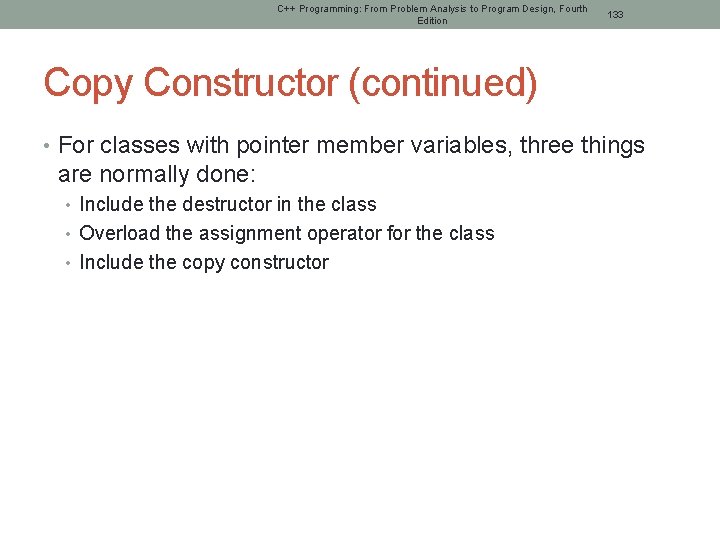
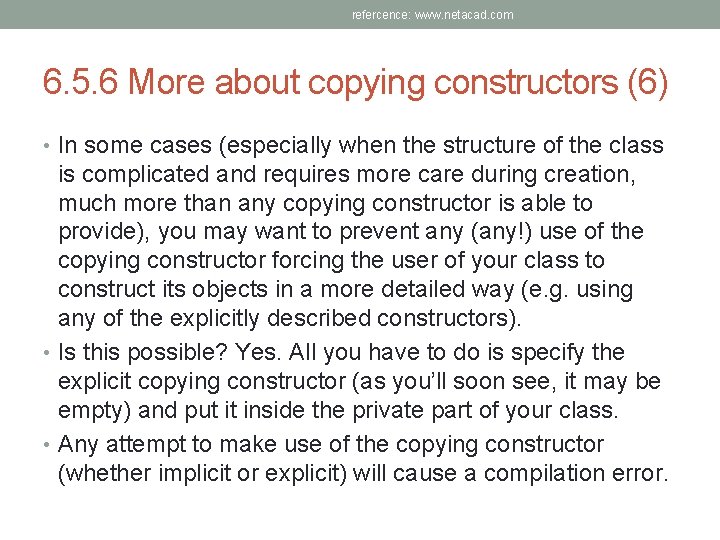
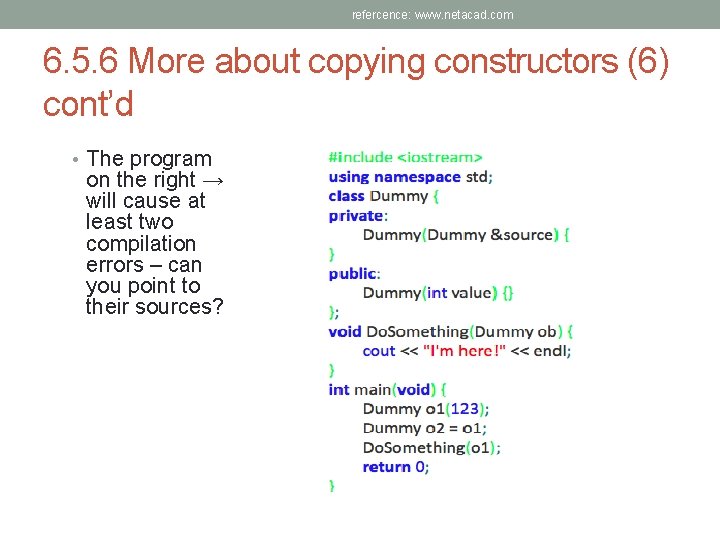
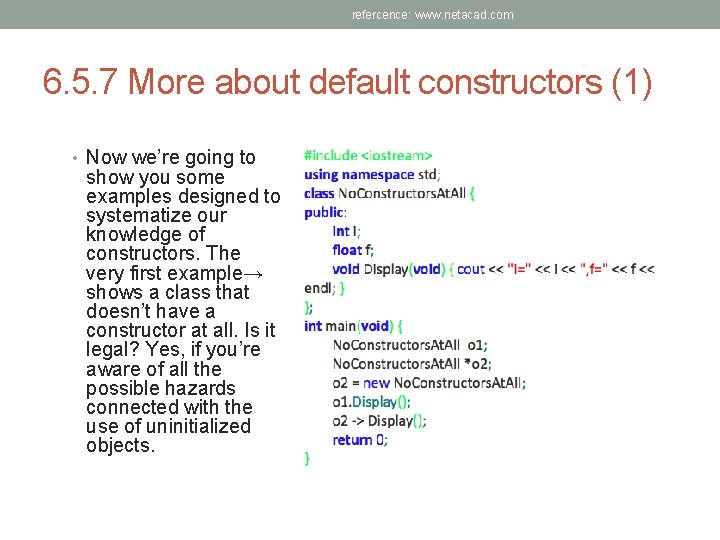
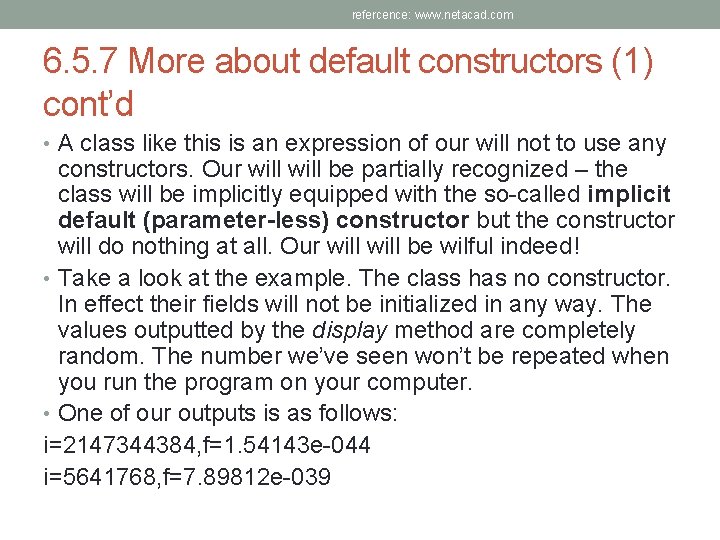
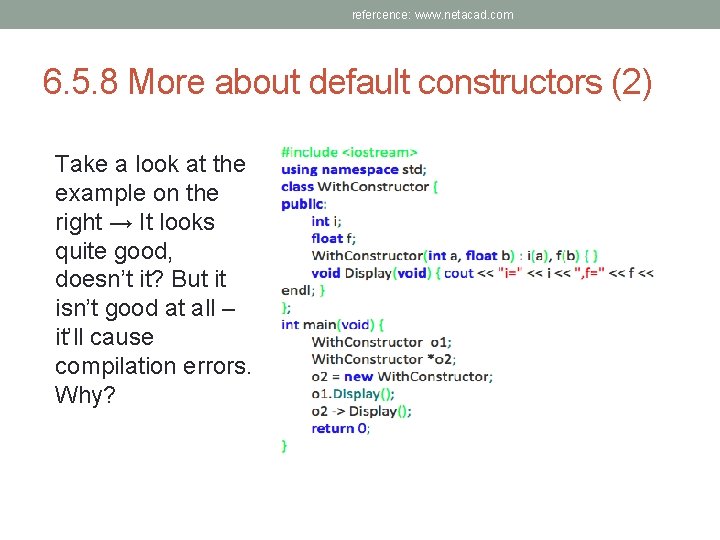
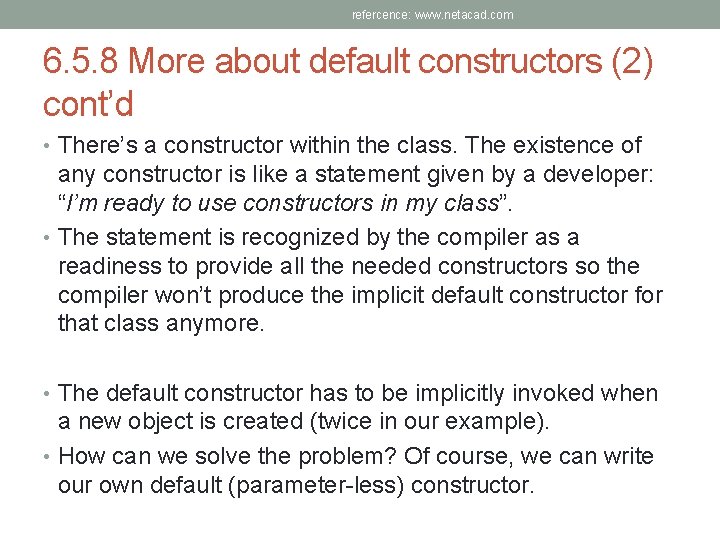
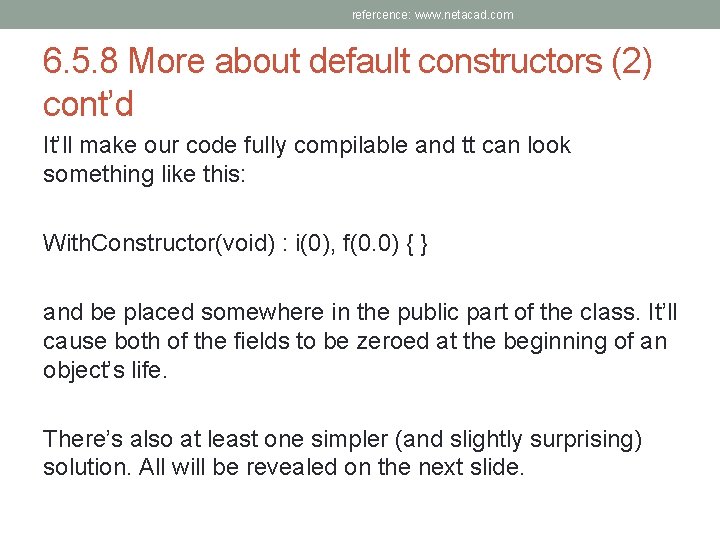
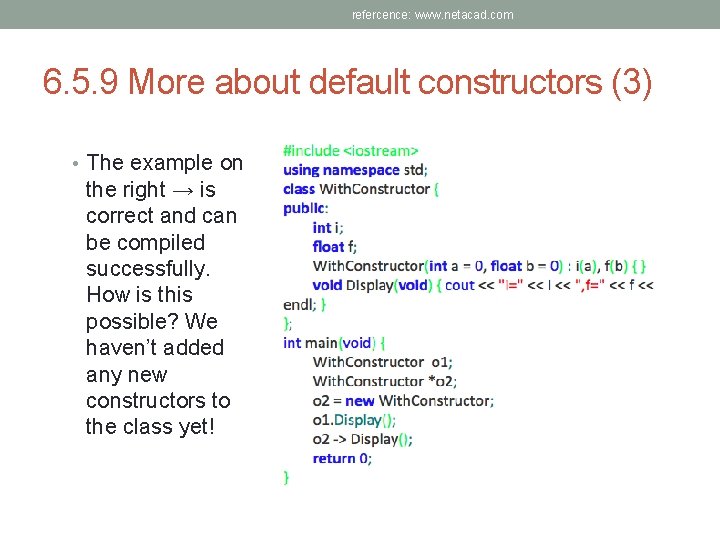
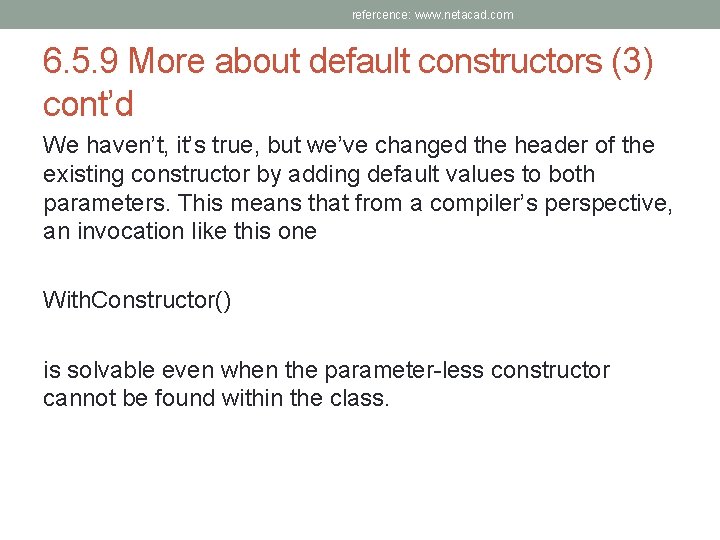
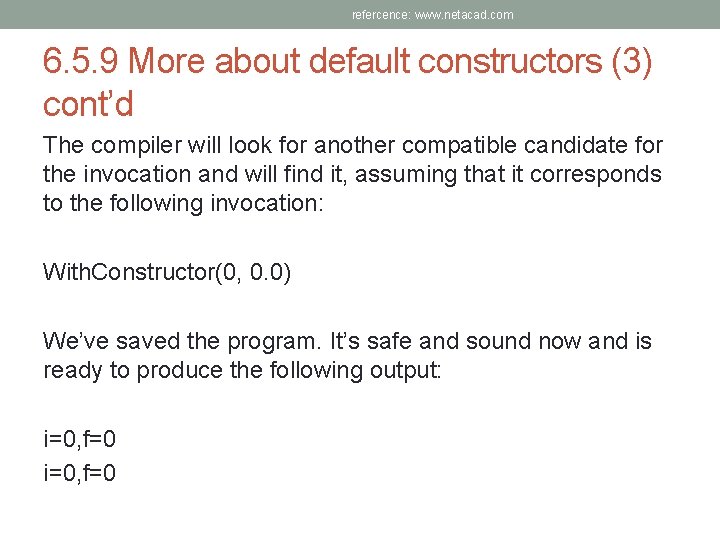
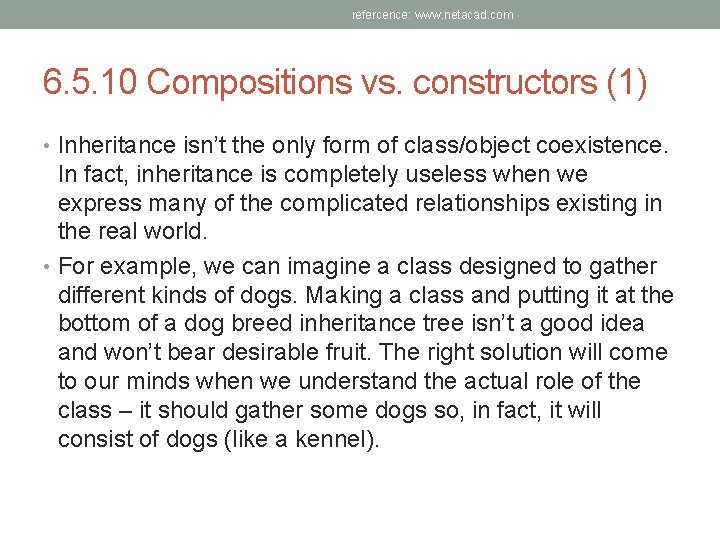
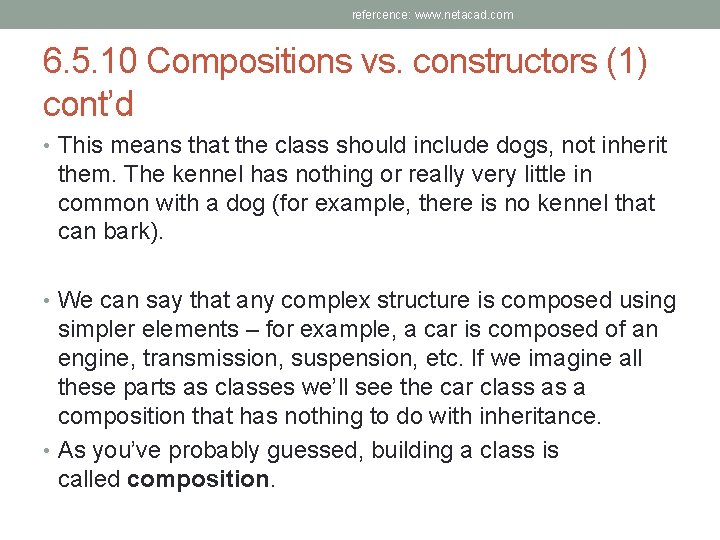
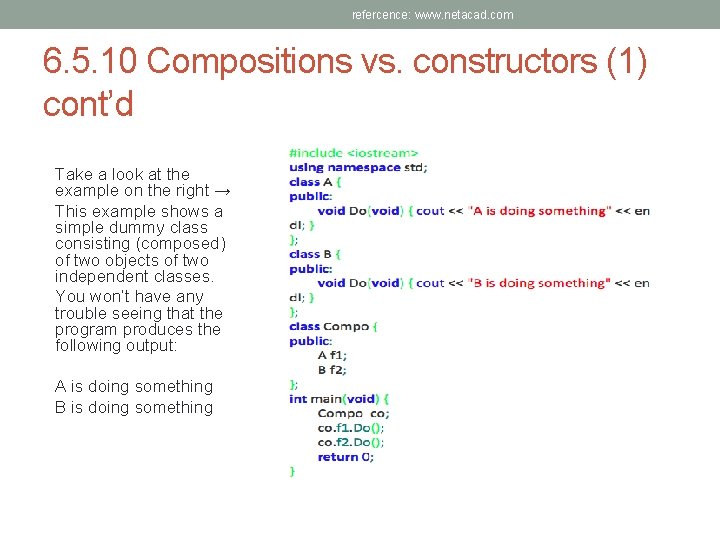
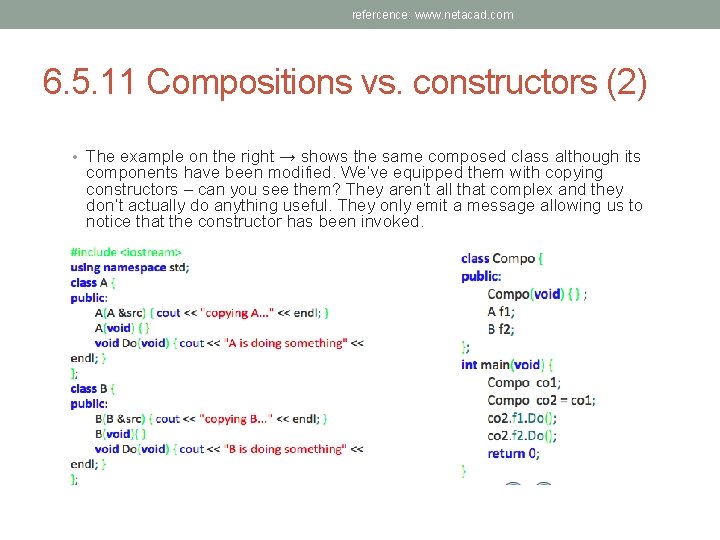
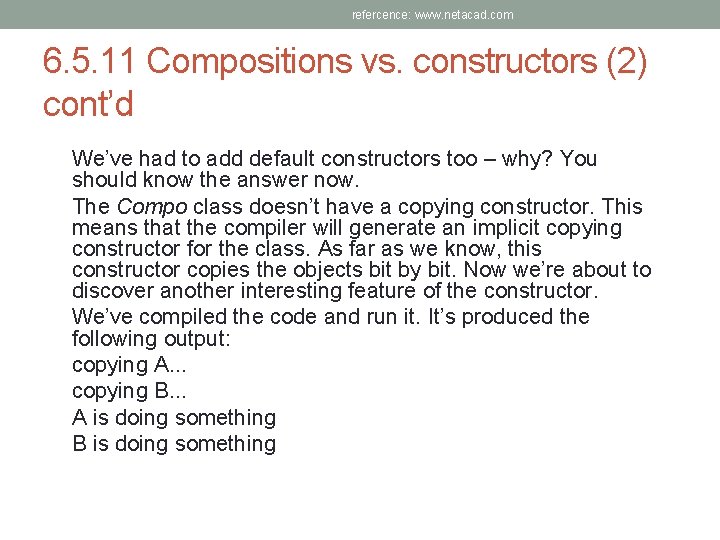
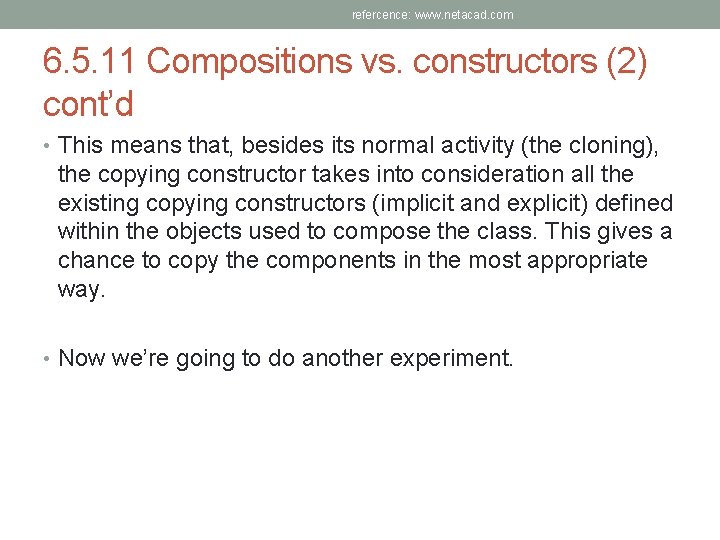
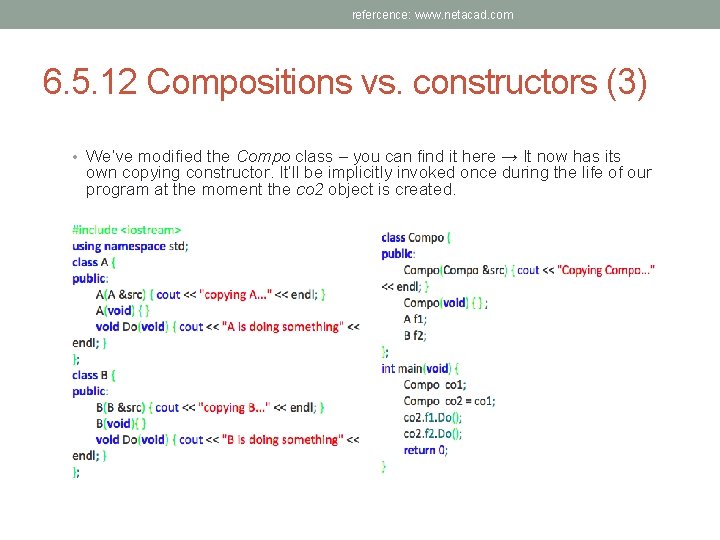
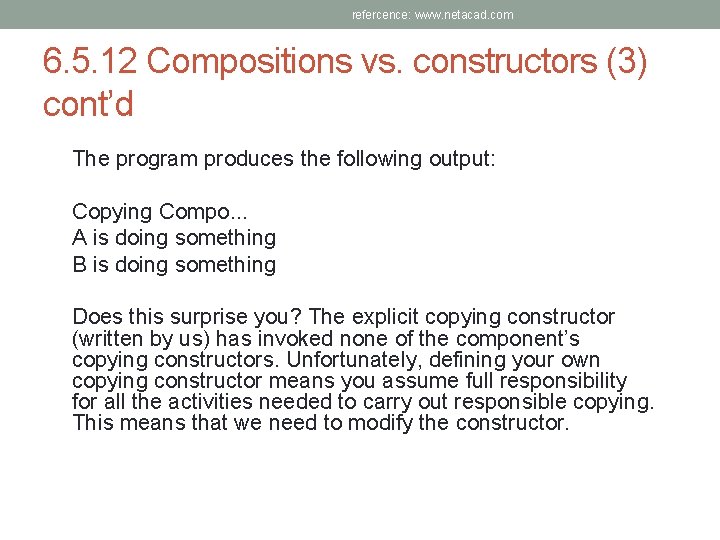
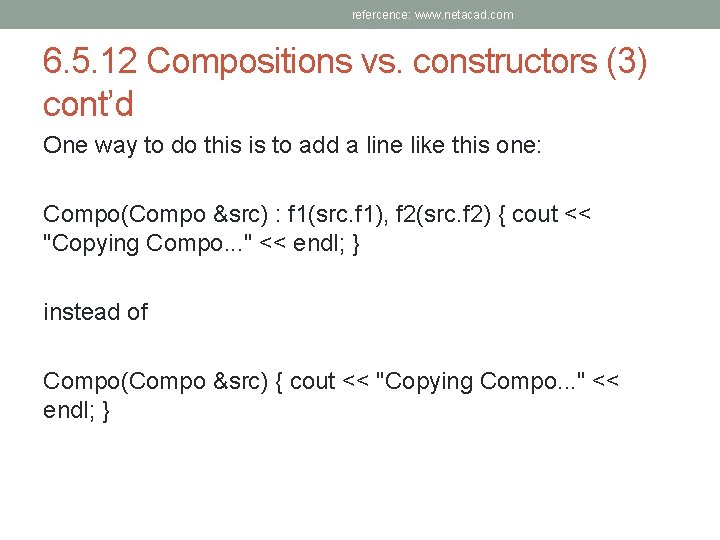
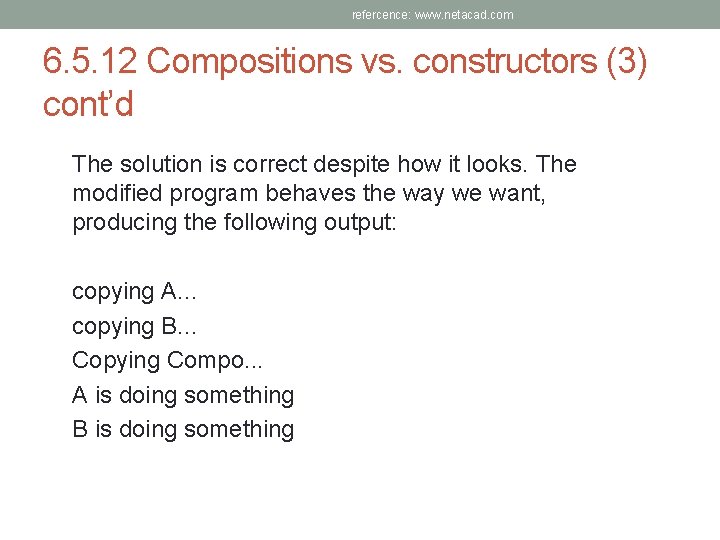
- Slides: 153
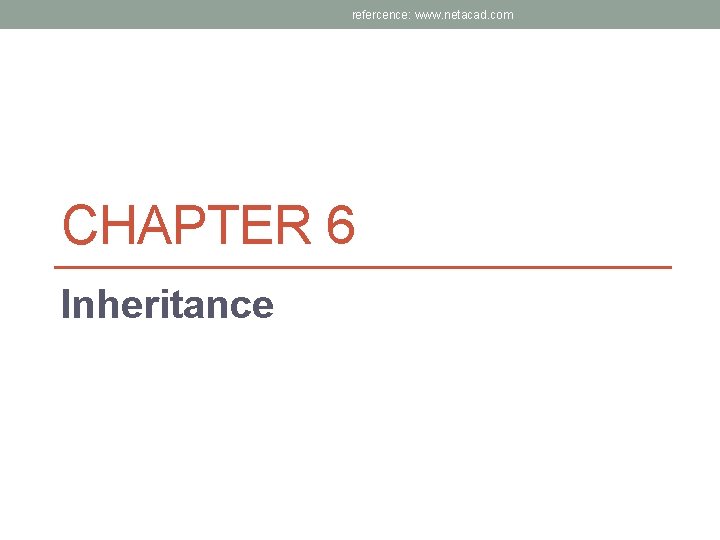
refercence: www. netacad. com CHAPTER 6 Inheritance
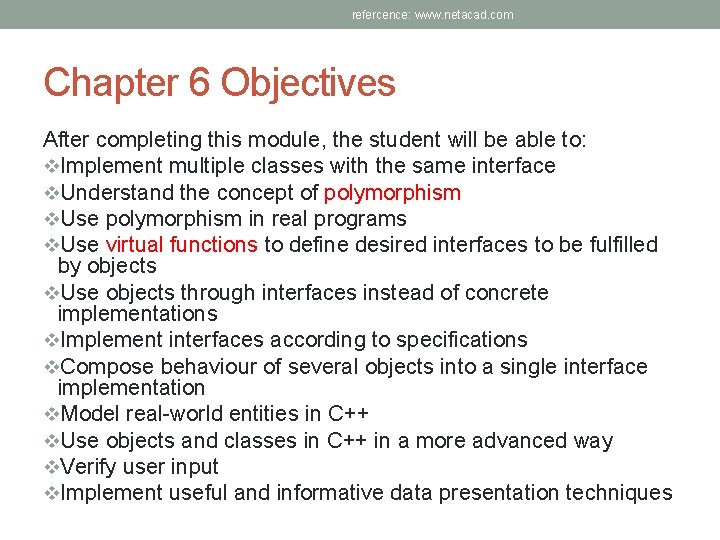
refercence: www. netacad. com Chapter 6 Objectives After completing this module, the student will be able to: v. Implement multiple classes with the same interface v. Understand the concept of polymorphism v. Use polymorphism in real programs v. Use virtual functions to define desired interfaces to be fulfilled by objects v. Use objects through interfaces instead of concrete implementations v. Implement interfaces according to specifications v. Compose behaviour of several objects into a single interface implementation v. Model real-world entities in C++ v. Use objects and classes in C++ in a more advanced way v. Verify user input v. Implement useful and informative data presentation techniques
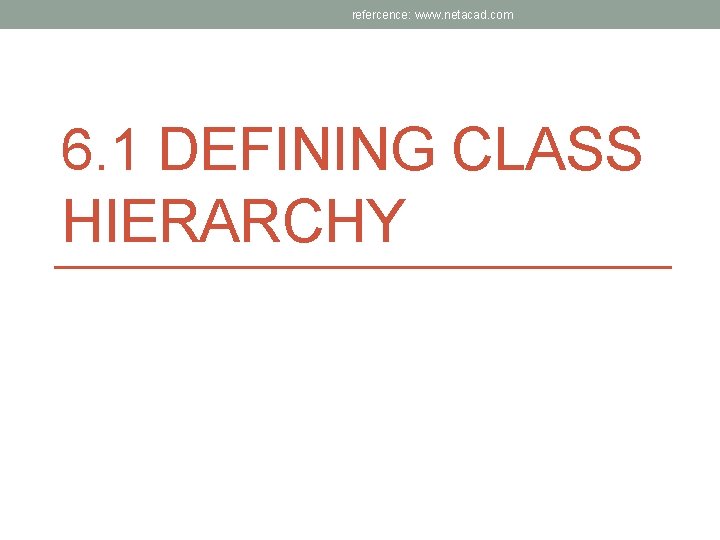
refercence: www. netacad. com 6. 1 DEFINING CLASS HIERARCHY
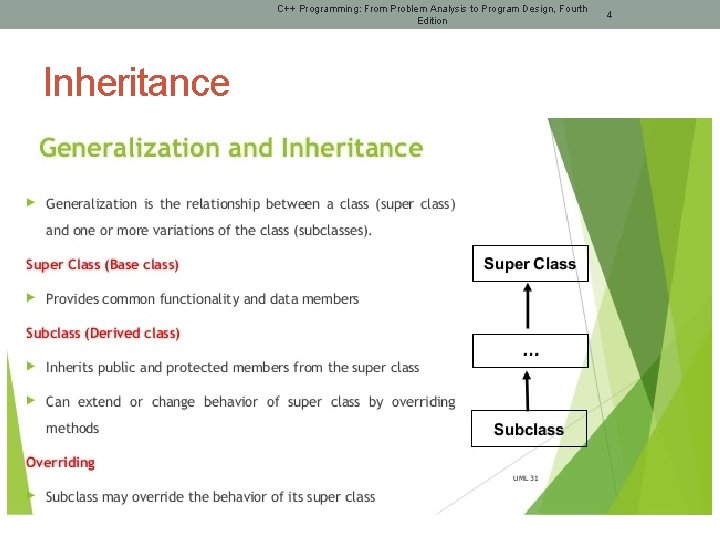
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 4 Inheritance • Inheritance is an “is-a” relationship • Example: “every employee is a person” • Inheritance lets us create new classes from existing classes • New classes are called the derived classes • Existing classes are called the base classes • Derived classes inherit the properties of the base classes
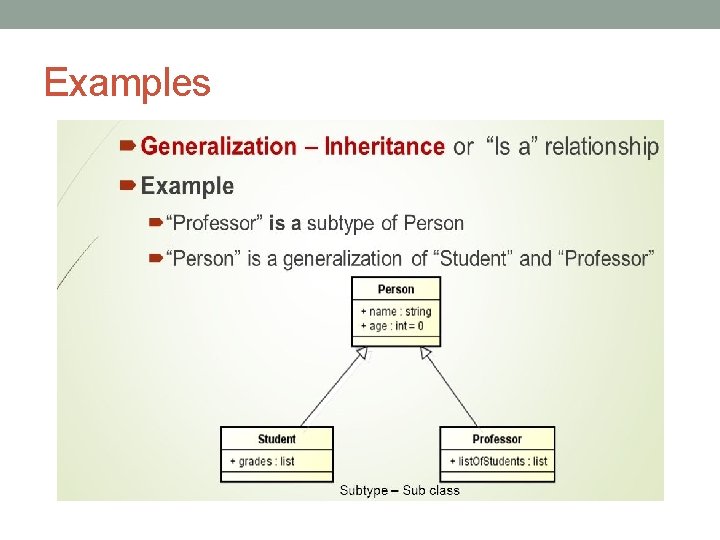
Examples
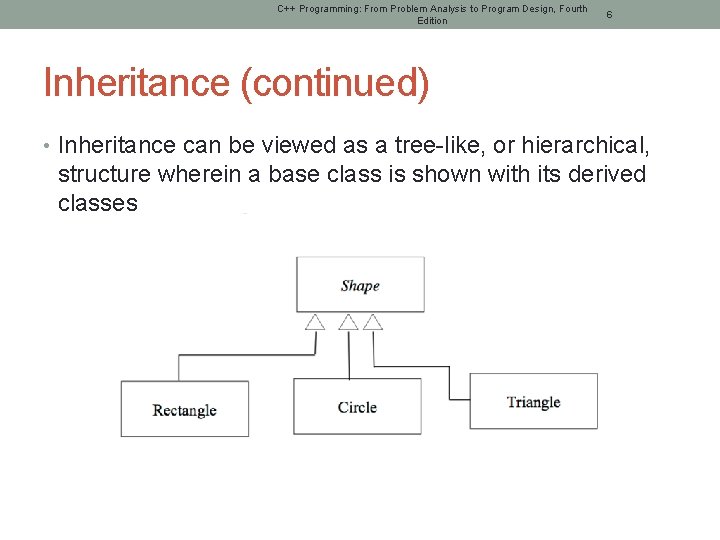
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 6 Inheritance (continued) • Inheritance can be viewed as a tree-like, or hierarchical, structure wherein a base class is shown with its derived classes
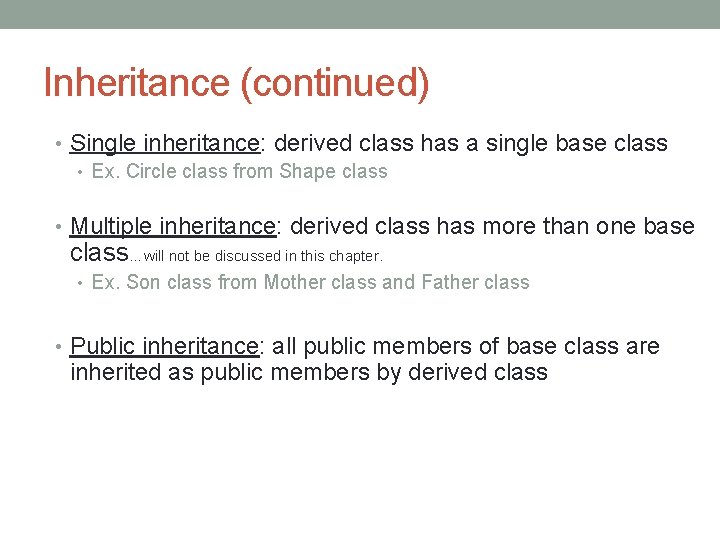
Inheritance (continued) • Single inheritance: derived class has a single base class • Ex. Circle class from Shape class • Multiple inheritance: derived class has more than one base class…will not be discussed in this chapter. • Ex. Son class from Mother class and Father class • Public inheritance: all public members of base class are inherited as public members by derived class
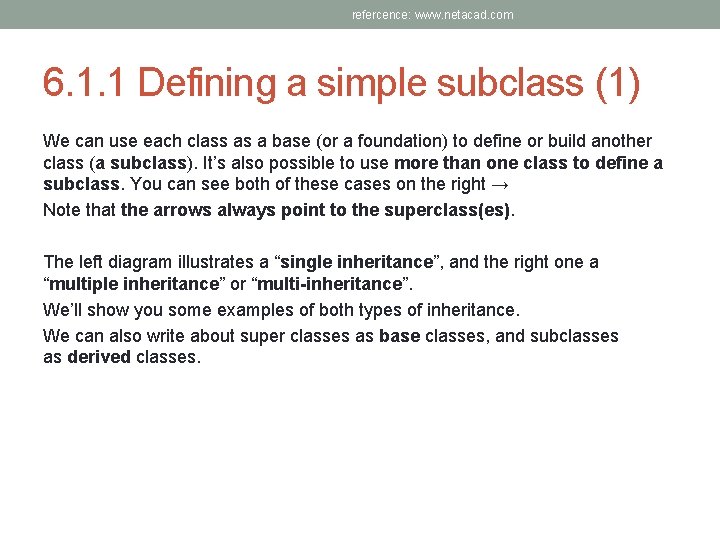
refercence: www. netacad. com 6. 1. 1 Defining a simple subclass (1) We can use each class as a base (or a foundation) to define or build another class (a subclass). It’s also possible to use more than one class to define a subclass. You can see both of these cases on the right → Note that the arrows always point to the superclass(es). The left diagram illustrates a “single inheritance”, and the right one a “multiple inheritance” or “multi-inheritance”. We’ll show you some examples of both types of inheritance. We can also write about super classes as base classes, and subclasses as derived classes.
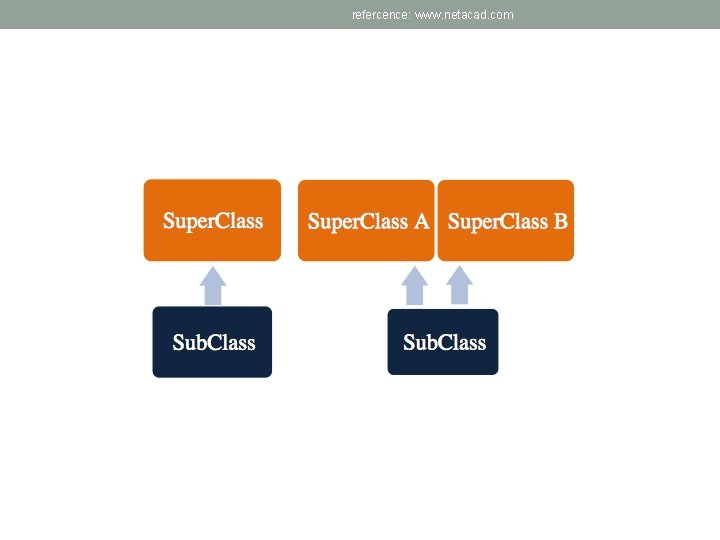
refercence: www. netacad. com
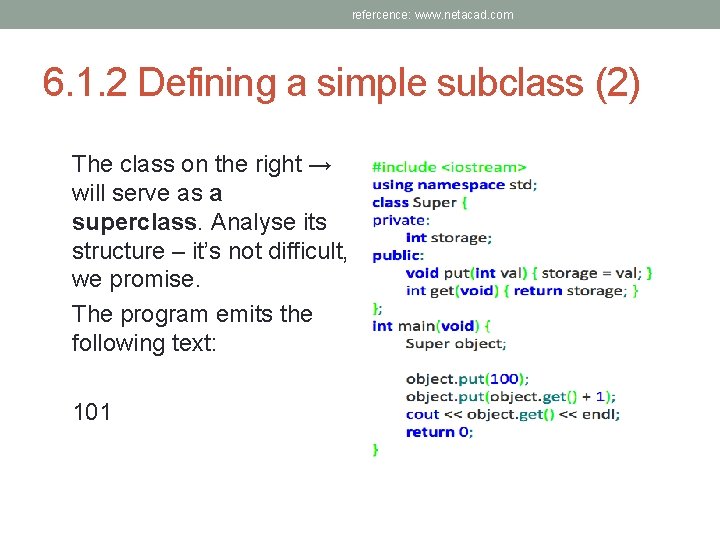
refercence: www. netacad. com 6. 1. 2 Defining a simple subclass (2) The class on the right → will serve as a superclass. Analyse its structure – it’s not difficult, we promise. The program emits the following text: 101
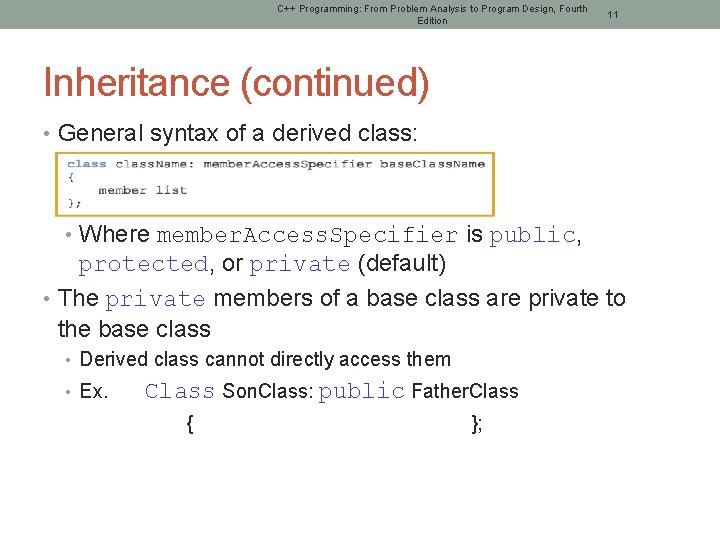
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 11 Inheritance (continued) • General syntax of a derived class: • Where member. Access. Specifier is public, protected, or private (default) • The private members of a base class are private to the base class • Derived class cannot directly access them • Ex. Class Son. Class: public Father. Class { };
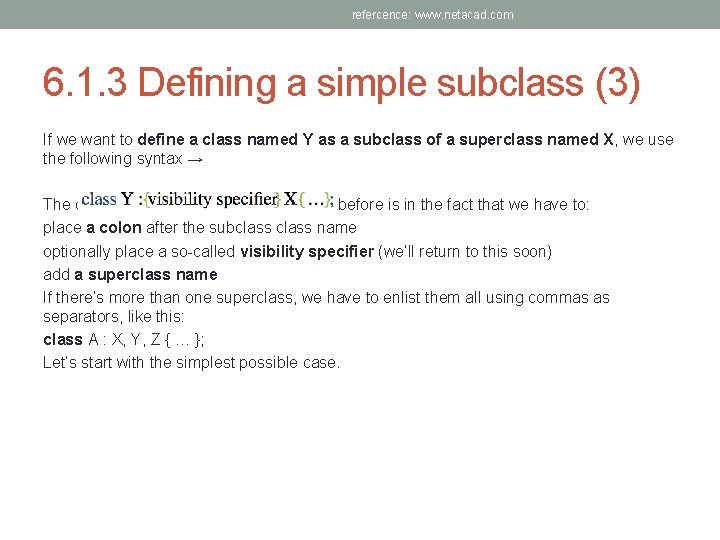
refercence: www. netacad. com 6. 1. 3 Defining a simple subclass (3) If we want to define a class named Y as a subclass of a superclass named X, we use the following syntax → The difference from the notation we used before is in the fact that we have to: place a colon after the subclass name optionally place a so-called visibility specifier (we’ll return to this soon) add a superclass name If there’s more than one superclass, we have to enlist them all using commas as separators, like this: class A : X, Y, Z { … }; Let’s start with the simplest possible case.
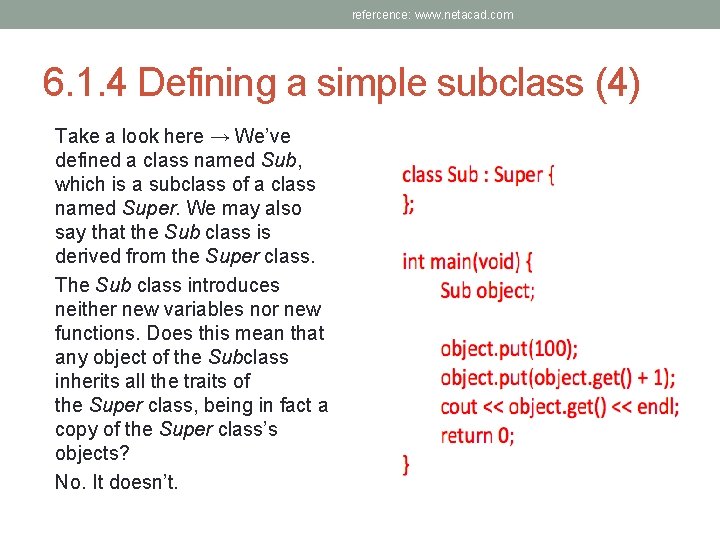
refercence: www. netacad. com 6. 1. 4 Defining a simple subclass (4) Take a look here → We’ve defined a class named Sub, which is a subclass of a class named Super. We may also say that the Sub class is derived from the Super class. The Sub class introduces neither new variables nor new functions. Does this mean that any object of the Subclass inherits all the traits of the Super class, being in fact a copy of the Super class’s objects? No. It doesn’t.
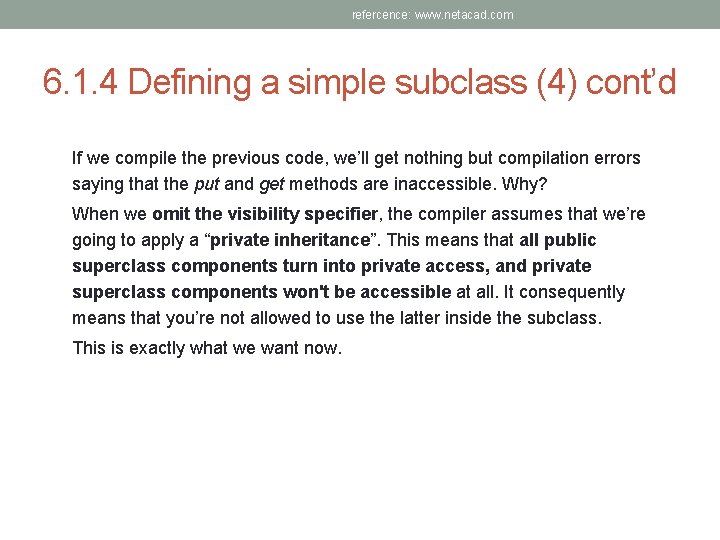
refercence: www. netacad. com 6. 1. 4 Defining a simple subclass (4) cont’d If we compile the previous code, we’ll get nothing but compilation errors saying that the put and get methods are inaccessible. Why? When we omit the visibility specifier, the compiler assumes that we’re going to apply a “private inheritance”. This means that all public superclass components turn into private access, and private superclass components won't be accessible at all. It consequently means that you’re not allowed to use the latter inside the subclass. This is exactly what we want now.
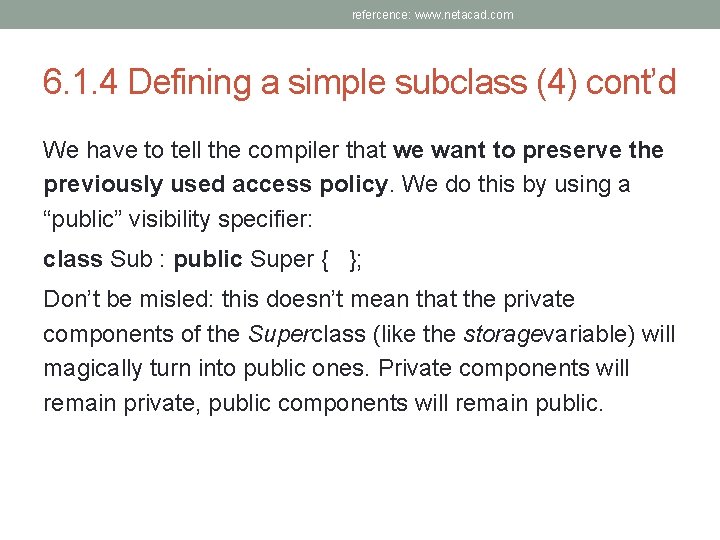
refercence: www. netacad. com 6. 1. 4 Defining a simple subclass (4) cont’d We have to tell the compiler that we want to preserve the previously used access policy. We do this by using a “public” visibility specifier: class Sub : public Super { }; Don’t be misled: this doesn’t mean that the private components of the Superclass (like the storagevariable) will magically turn into public ones. Private components will remain private, public components will remain public.
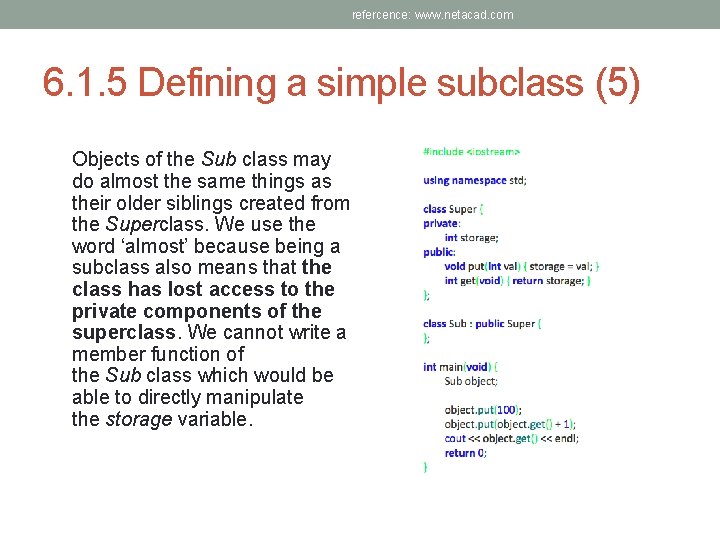
refercence: www. netacad. com 6. 1. 5 Defining a simple subclass (5) Objects of the Sub class may do almost the same things as their older siblings created from the Superclass. We use the word ‘almost’ because being a subclass also means that the class has lost access to the private components of the superclass. We cannot write a member function of the Sub class which would be able to directly manipulate the storage variable.
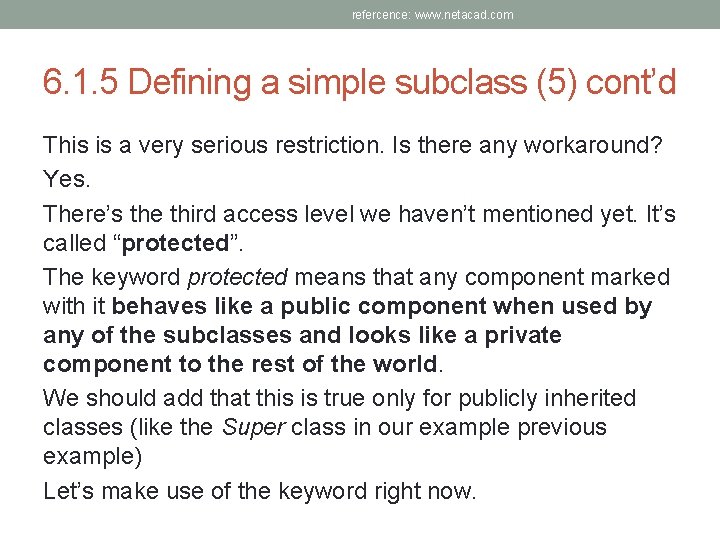
refercence: www. netacad. com 6. 1. 5 Defining a simple subclass (5) cont’d This is a very serious restriction. Is there any workaround? Yes. There’s the third access level we haven’t mentioned yet. It’s called “protected”. The keyword protected means that any component marked with it behaves like a public component when used by any of the subclasses and looks like a private component to the rest of the world. We should add that this is true only for publicly inherited classes (like the Super class in our example previous example) Let’s make use of the keyword right now.
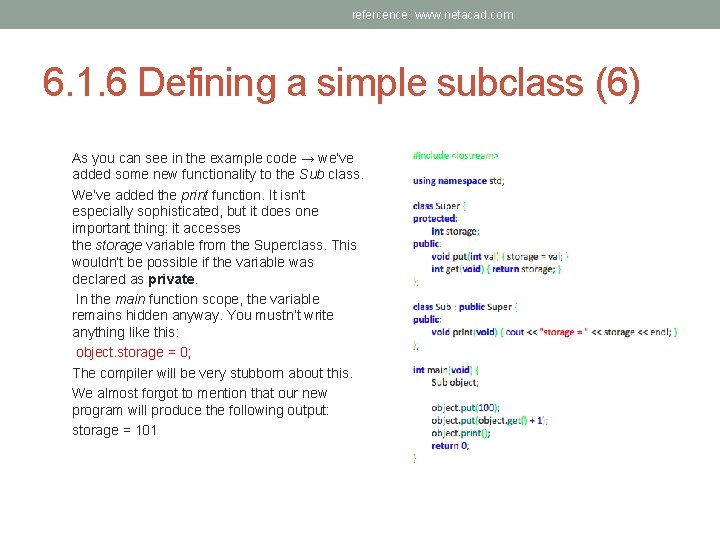
refercence: www. netacad. com 6. 1. 6 Defining a simple subclass (6) As you can see in the example code → we’ve added some new functionality to the Sub class. We’ve added the print function. It isn’t especially sophisticated, but it does one important thing: it accesses the storage variable from the Superclass. This wouldn’t be possible if the variable was declared as private. In the main function scope, the variable remains hidden anyway. You mustn’t write anything like this: object. storage = 0; The compiler will be very stubborn about this. We almost forgot to mention that our new program will produce the following output: storage = 101
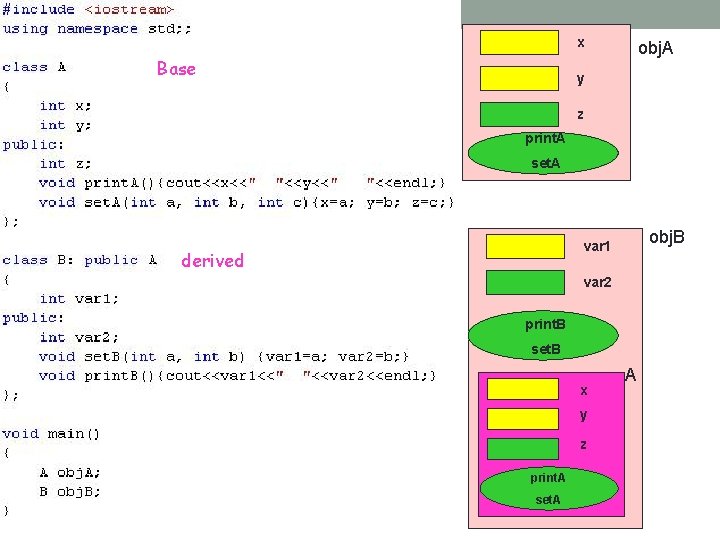
x Base obj. A y z print. A set. A obj. B var 1 derived var 2 print. B set. B x y z print. A set. A A
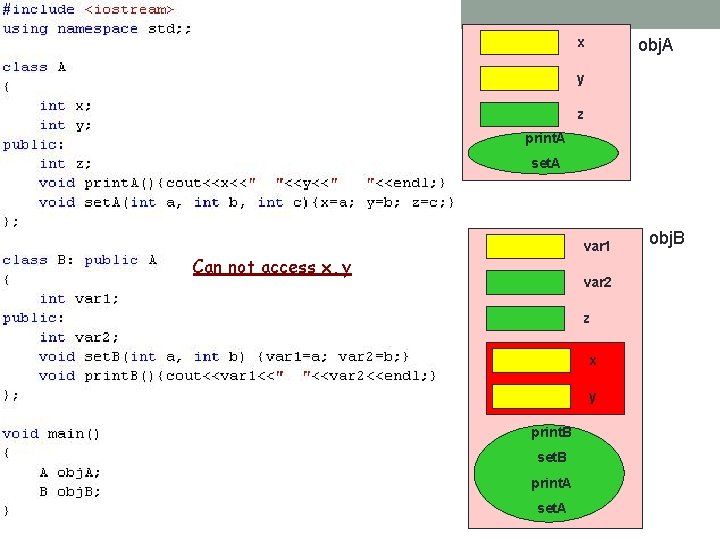
x obj. A y z print. A set. A var 1 Can not access x, y var 2 z x y print. B set. B print. A set. A obj. B
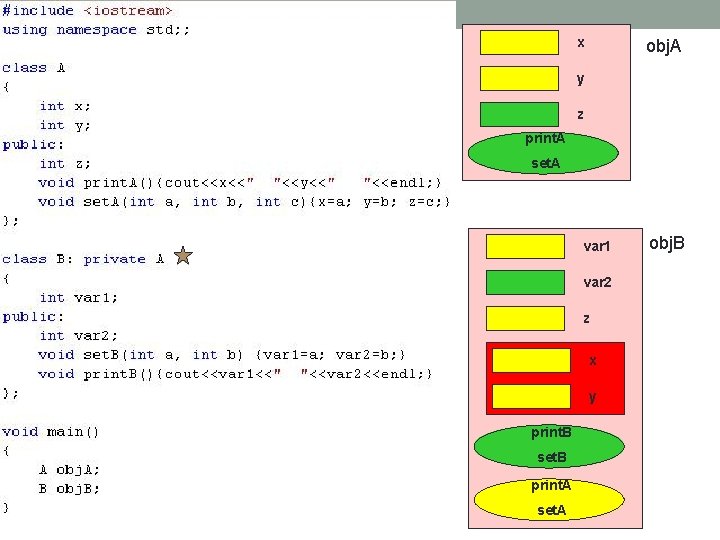
x obj. A y z print. A set. A var 1 var 2 z x y print. B set. B print. A set. A obj. B
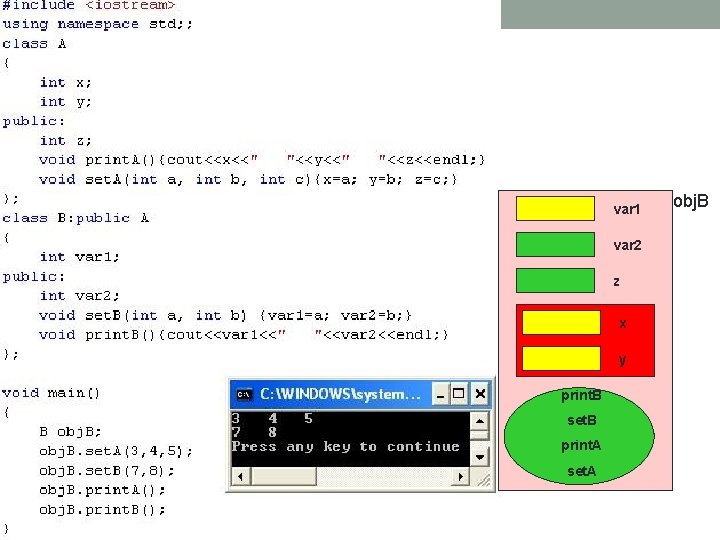
var 1 var 2 z x y print. B set. B print. A set. A obj. B
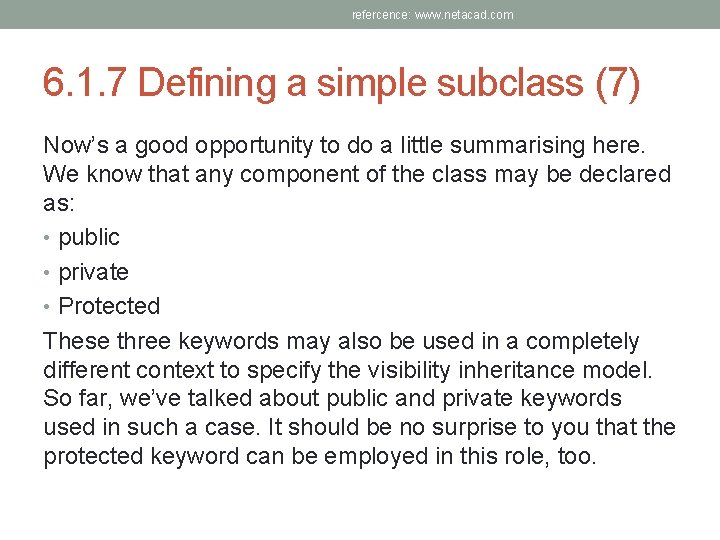
refercence: www. netacad. com 6. 1. 7 Defining a simple subclass (7) Now’s a good opportunity to do a little summarising here. We know that any component of the class may be declared as: • public • private • Protected These three keywords may also be used in a completely different context to specify the visibility inheritance model. So far, we’ve talked about public and private keywords used in such a case. It should be no surprise to you that the protected keyword can be employed in this role, too.
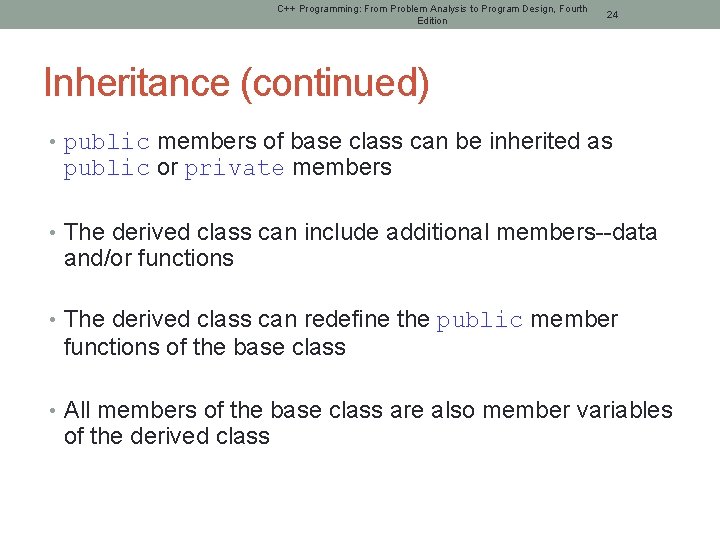
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 24 Inheritance (continued) • public members of base class can be inherited as public or private members • The derived class can include additional members--data and/or functions • The derived class can redefine the public member functions of the base class • All members of the base class are also member variables of the derived class
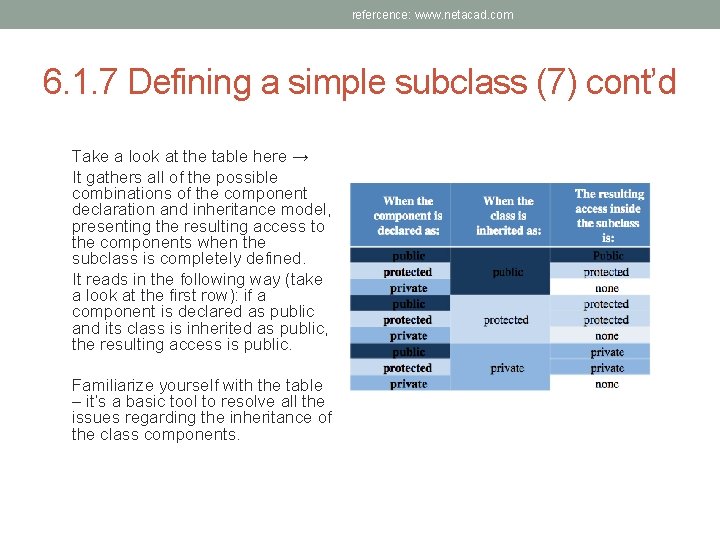
refercence: www. netacad. com 6. 1. 7 Defining a simple subclass (7) cont’d Take a look at the table here → It gathers all of the possible combinations of the component declaration and inheritance model, presenting the resulting access to the components when the subclass is completely defined. It reads in the following way (take a look at the first row): if a component is declared as public and its class is inherited as public, the resulting access is public. Familiarize yourself with the table – it’s a basic tool to resolve all the issues regarding the inheritance of the class components.
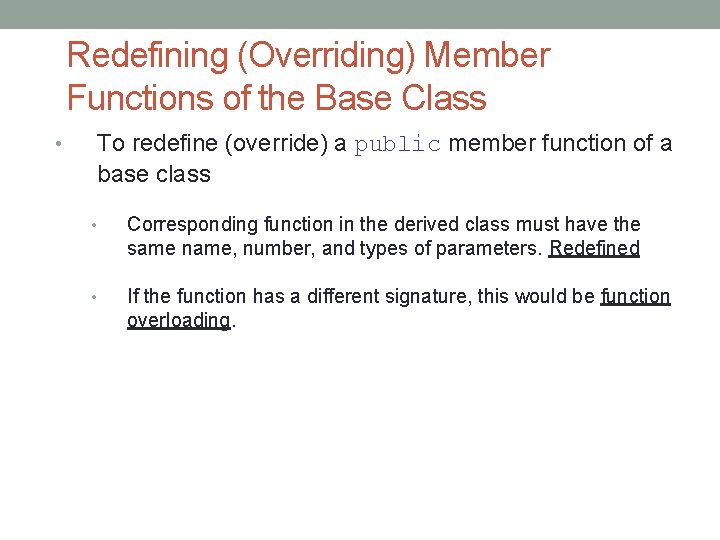
Redefining (Overriding) Member Functions of the Base Class • To redefine (override) a public member function of a base class • Corresponding function in the derived class must have the same name, number, and types of parameters. Redefined • If the function has a different signature, this would be function overloading.
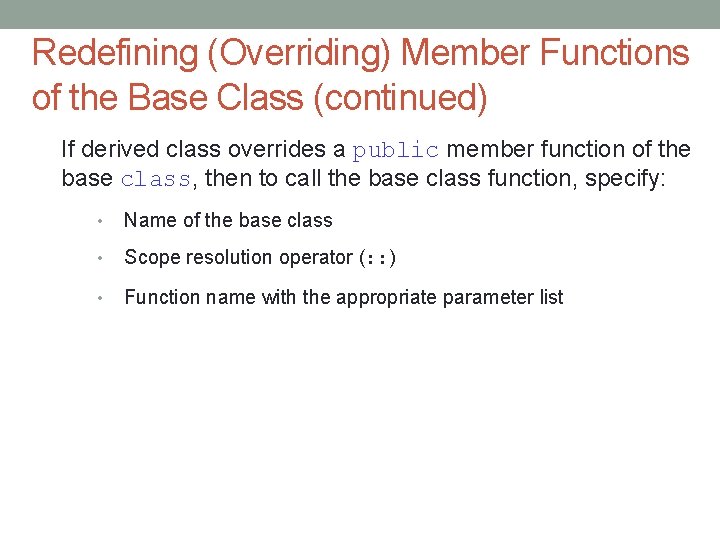
Redefining (Overriding) Member Functions of the Base Class (continued) If derived class overrides a public member function of the base class, then to call the base class function, specify: • Name of the base class • Scope resolution operator (: : ) • Function name with the appropriate parameter list
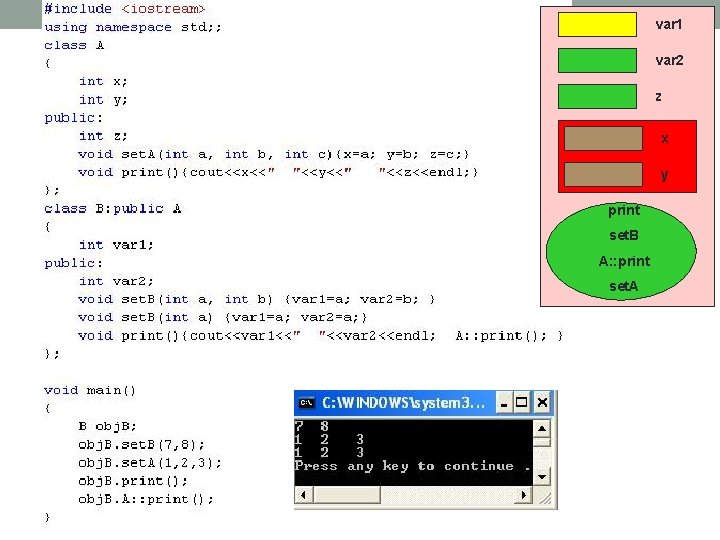
var 1 var 2 z x y print set. B A: : print set. A
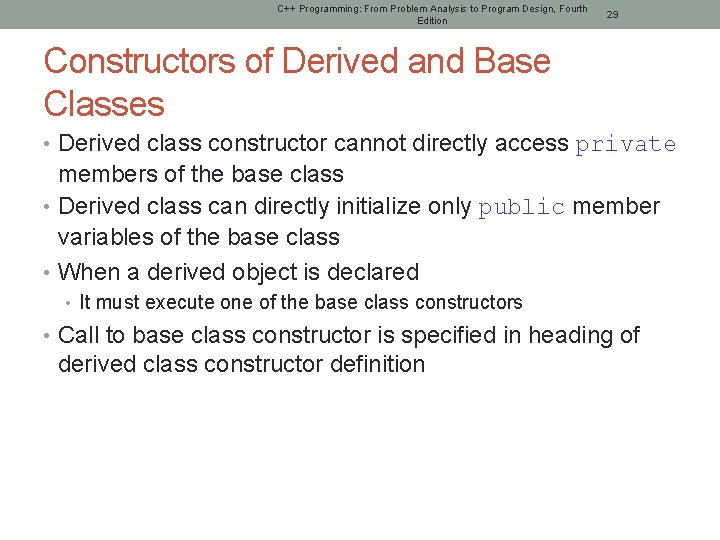
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 29 Constructors of Derived and Base Classes • Derived class constructor cannot directly access private members of the base class • Derived class can directly initialize only public member variables of the base class • When a derived object is declared • It must execute one of the base class constructors • Call to base class constructor is specified in heading of derived class constructor definition
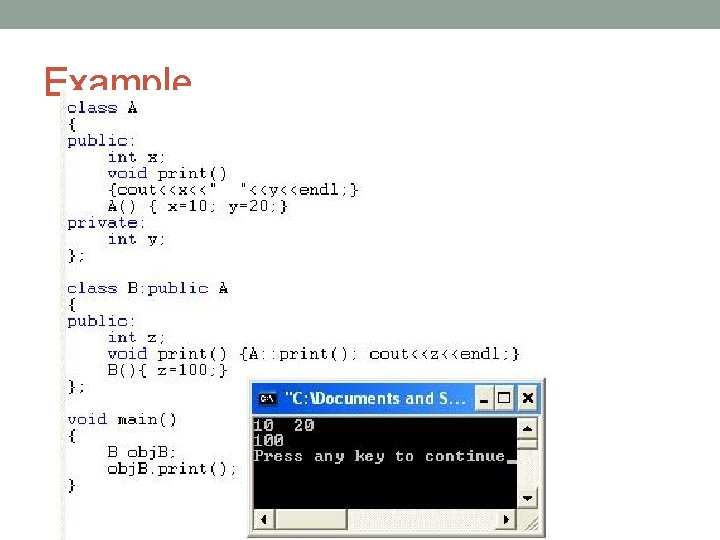
Example
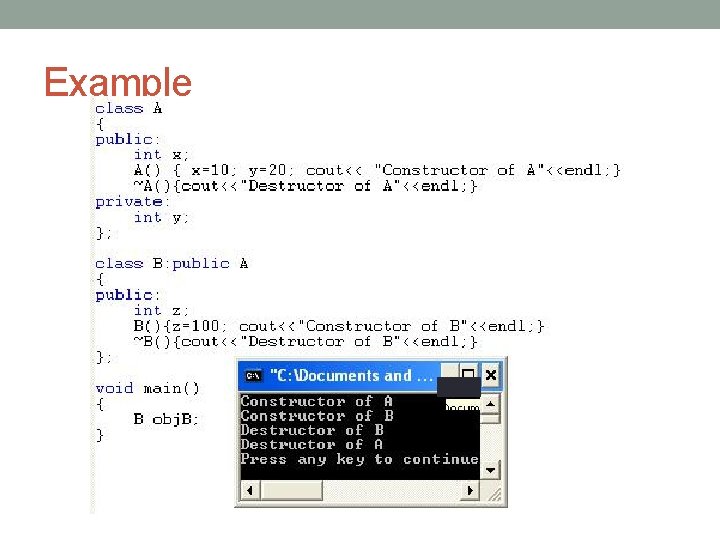
Example
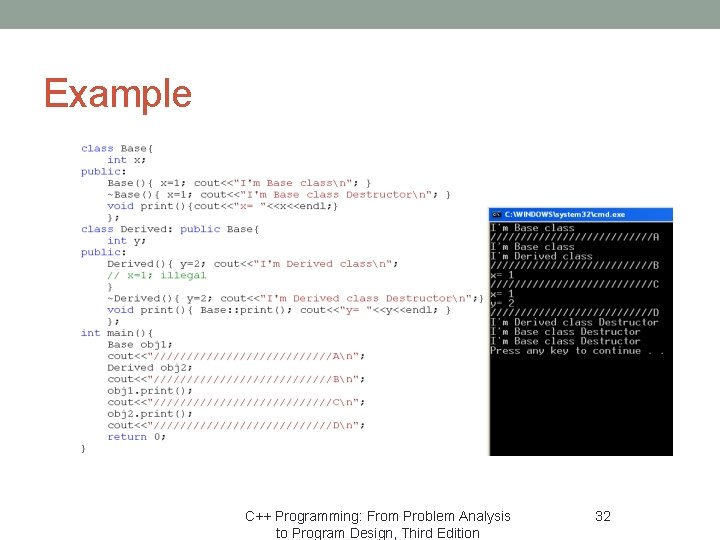
Example C++ Programming: From Problem Analysis to Program Design, Third Edition 32
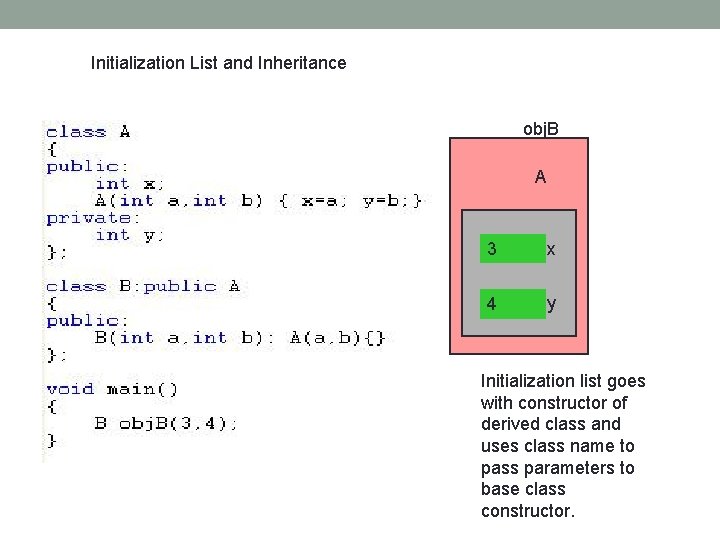
Initialization List and Inheritance obj. B A 3 x 4 y Initialization list goes with constructor of derived class and uses class name to pass parameters to base class constructor.
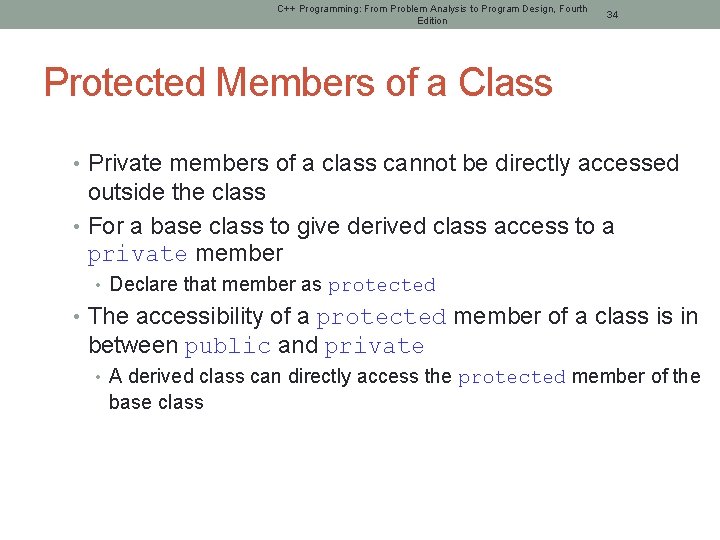
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 34 Protected Members of a Class • Private members of a class cannot be directly accessed outside the class • For a base class to give derived class access to a private member • Declare that member as protected • The accessibility of a protected member of a class is in between public and private • A derived class can directly access the protected member of the base class
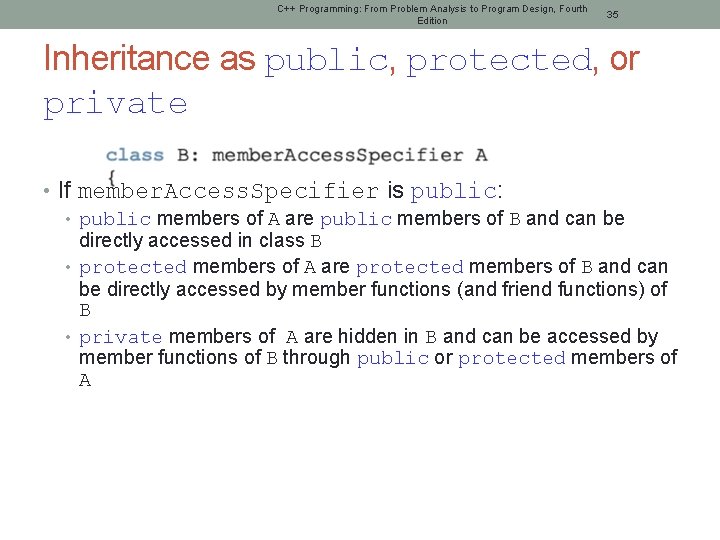
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 35 Inheritance as public, protected, or private • If member. Access. Specifier is public: • public members of A are public members of B and can be directly accessed in class B • protected members of A are protected members of B and can be directly accessed by member functions (and friend functions) of B • private members of A are hidden in B and can be accessed by member functions of B through public or protected members of A
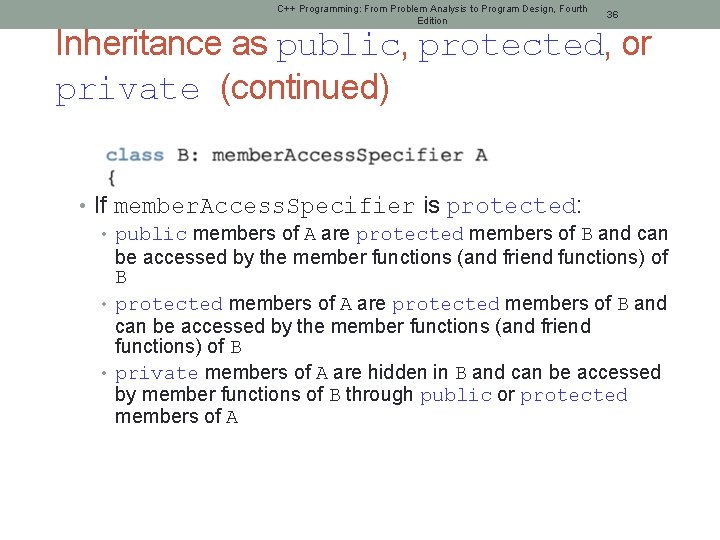
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 36 Inheritance as public, protected, or private (continued) • If member. Access. Specifier is protected: • public members of A are protected members of B and can be accessed by the member functions (and friend functions) of B • protected members of A are protected members of B and can be accessed by the member functions (and friend functions) of B • private members of A are hidden in B and can be accessed by member functions of B through public or protected members of A
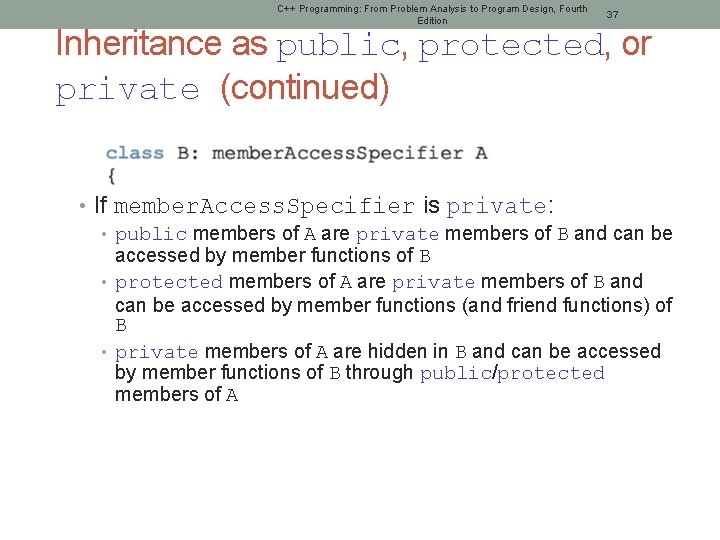
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 37 Inheritance as public, protected, or private (continued) • If member. Access. Specifier is private: • public members of A are private members of B and can be accessed by member functions of B • protected members of A are private members of B and can be accessed by member functions (and friend functions) of B • private members of A are hidden in B and can be accessed by member functions of B through public/protected members of A
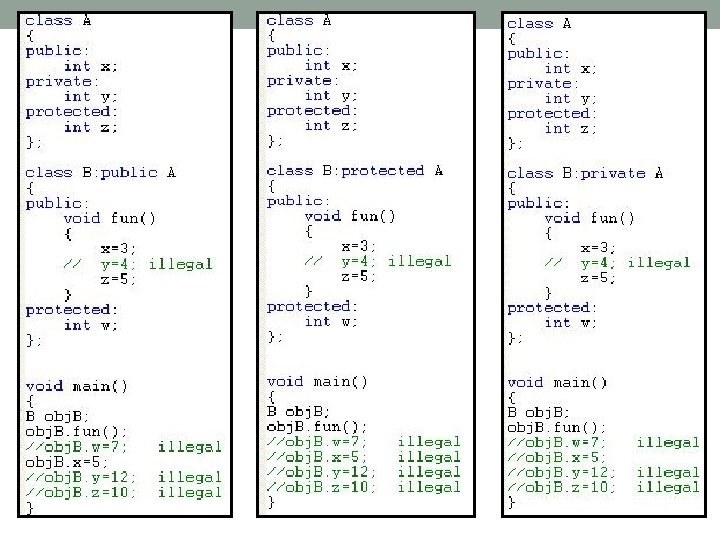
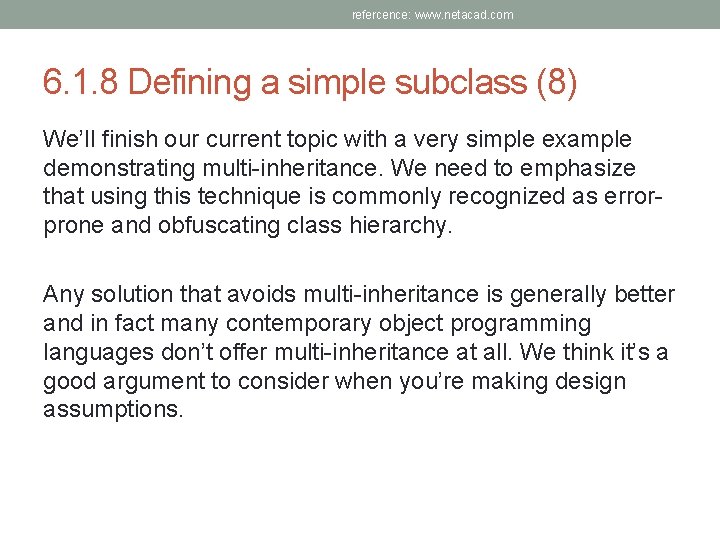
refercence: www. netacad. com 6. 1. 8 Defining a simple subclass (8) We’ll finish our current topic with a very simple example demonstrating multi-inheritance. We need to emphasize that using this technique is commonly recognized as errorprone and obfuscating class hierarchy. Any solution that avoids multi-inheritance is generally better and in fact many contemporary object programming languages don’t offer multi-inheritance at all. We think it’s a good argument to consider when you’re making design assumptions.
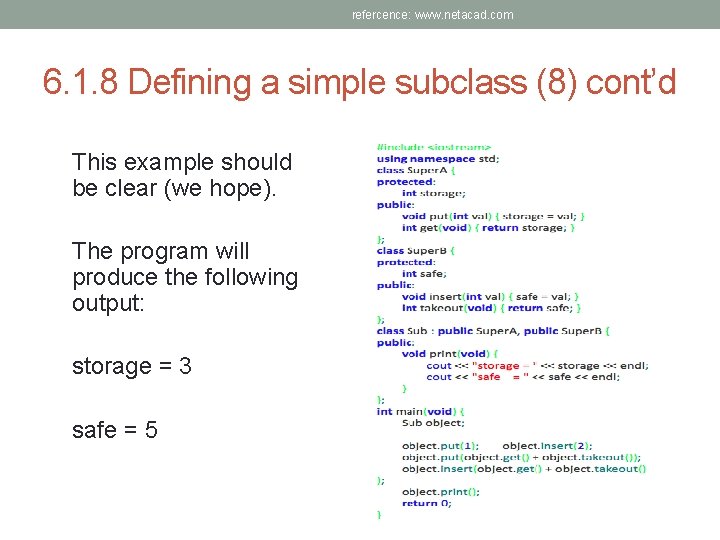
refercence: www. netacad. com 6. 1. 8 Defining a simple subclass (8) cont’d This example should be clear (we hope). The program will produce the following output: storage = 3 safe = 5
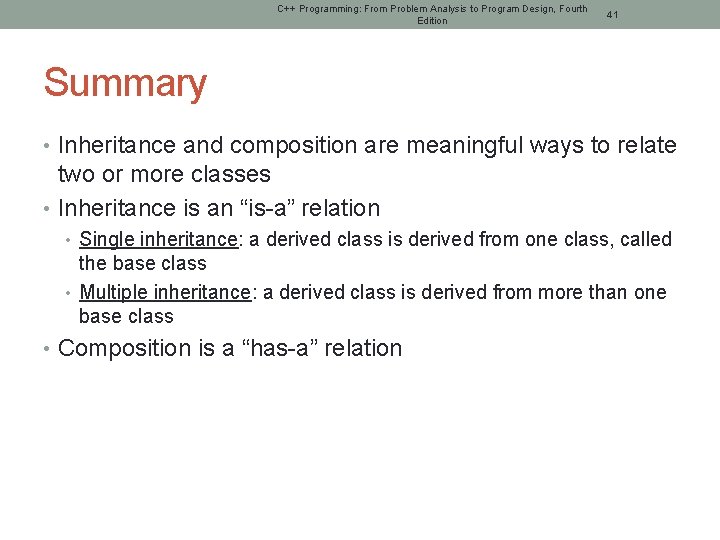
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 41 Summary • Inheritance and composition are meaningful ways to relate two or more classes • Inheritance is an “is-a” relation • Single inheritance: a derived class is derived from one class, called the base class • Multiple inheritance: a derived class is derived from more than one base class • Composition is a “has-a” relation
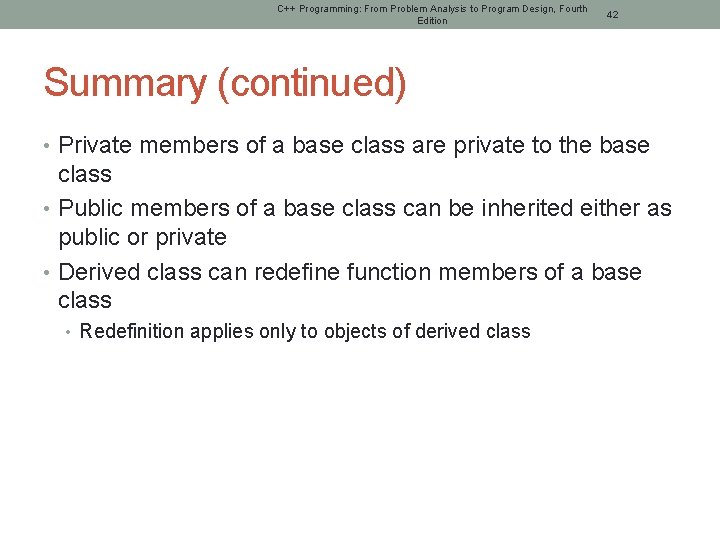
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 42 Summary (continued) • Private members of a base class are private to the base class • Public members of a base class can be inherited either as public or private • Derived class can redefine function members of a base class • Redefinition applies only to objects of derived class
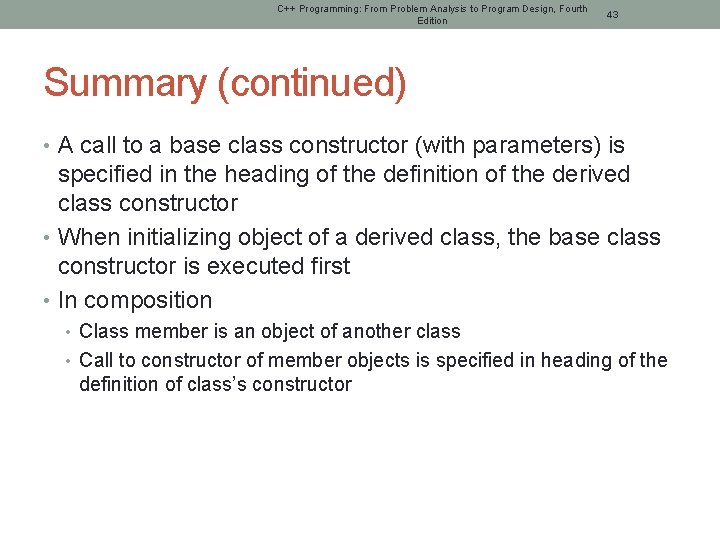
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 43 Summary (continued) • A call to a base class constructor (with parameters) is specified in the heading of the definition of the derived class constructor • When initializing object of a derived class, the base class constructor is executed first • In composition • Class member is an object of another class • Call to constructor of member objects is specified in heading of the definition of class’s constructor
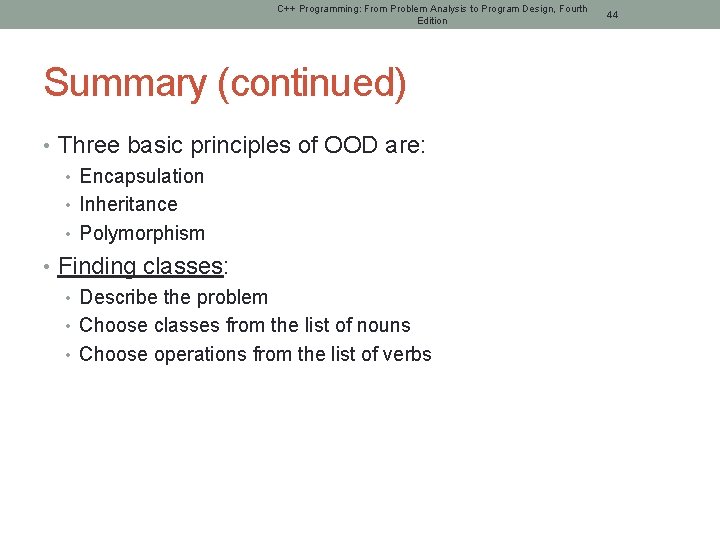
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Summary (continued) • Three basic principles of OOD are: • Encapsulation • Inheritance • Polymorphism • Finding classes: • Describe the problem • Choose classes from the list of nouns • Choose operations from the list of verbs 44
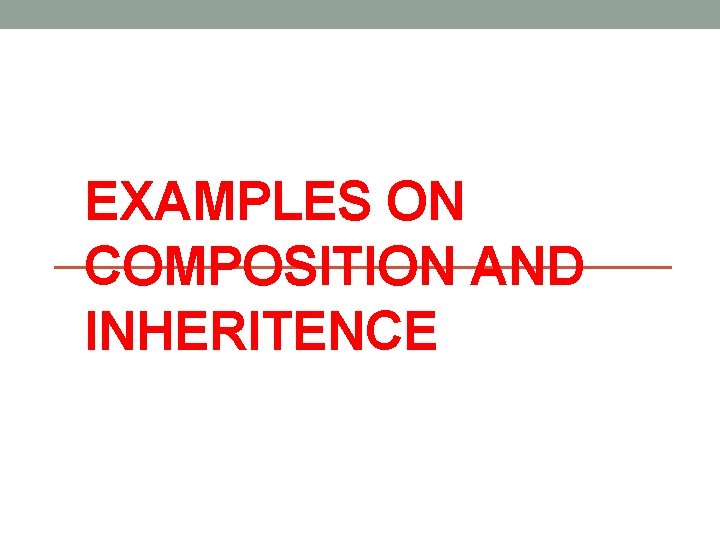
EXAMPLES ON COMPOSITION AND INHERITENCE
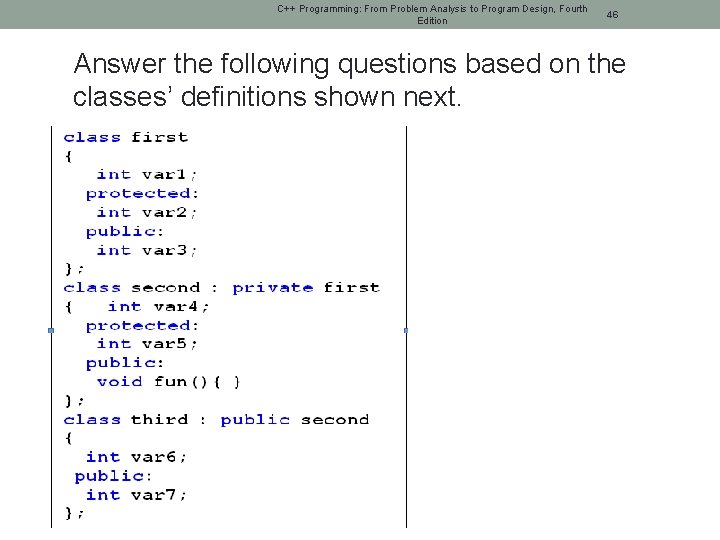
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 46 Answer the following questions based on the classes’ definitions shown next.
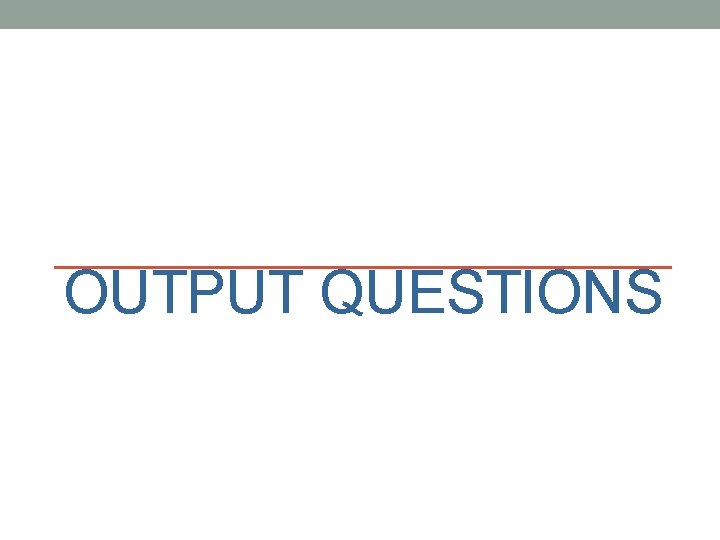
OUTPUT QUESTIONS
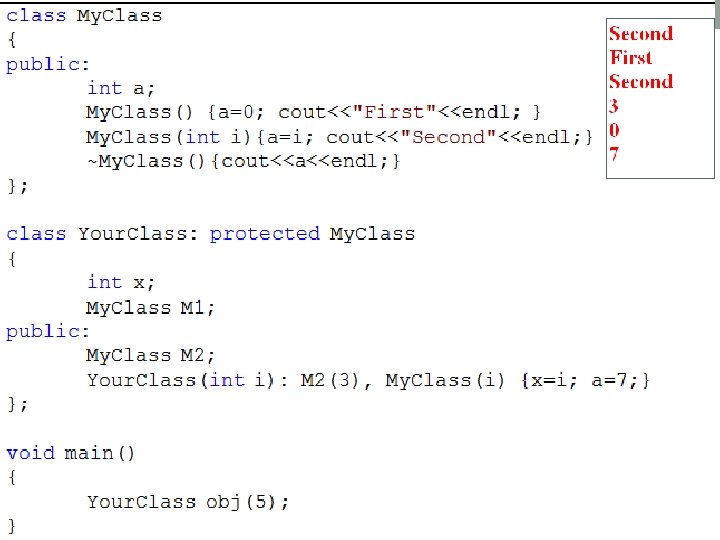
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 48
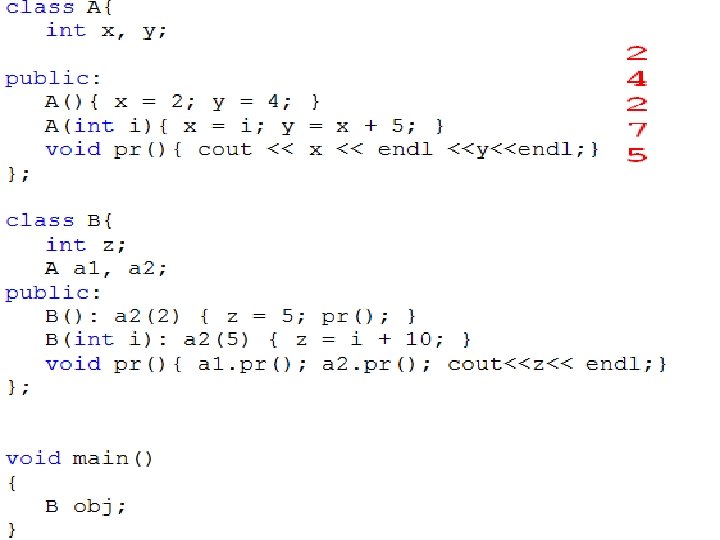
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 49
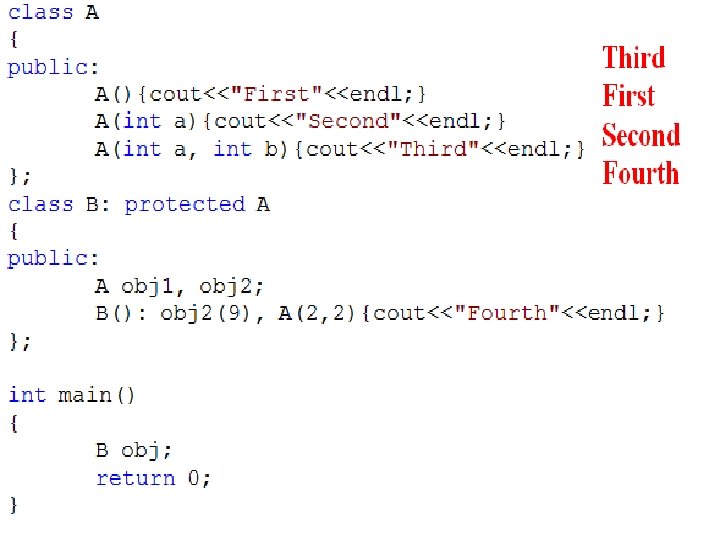
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 50
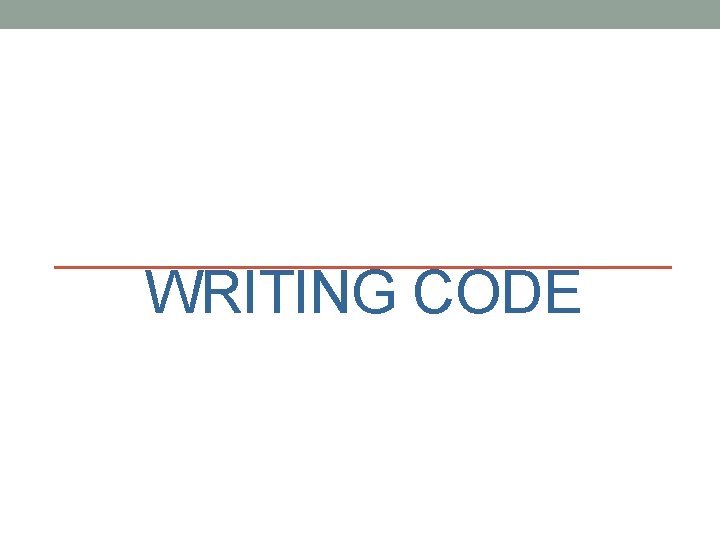
WRITING CODE
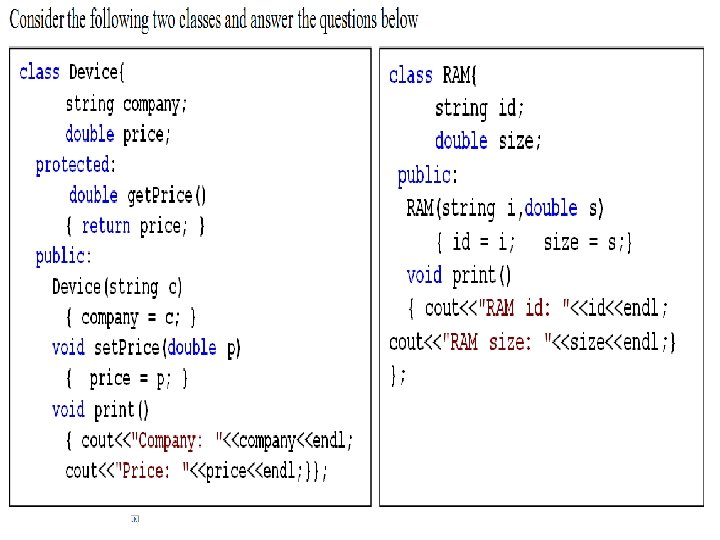
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 52
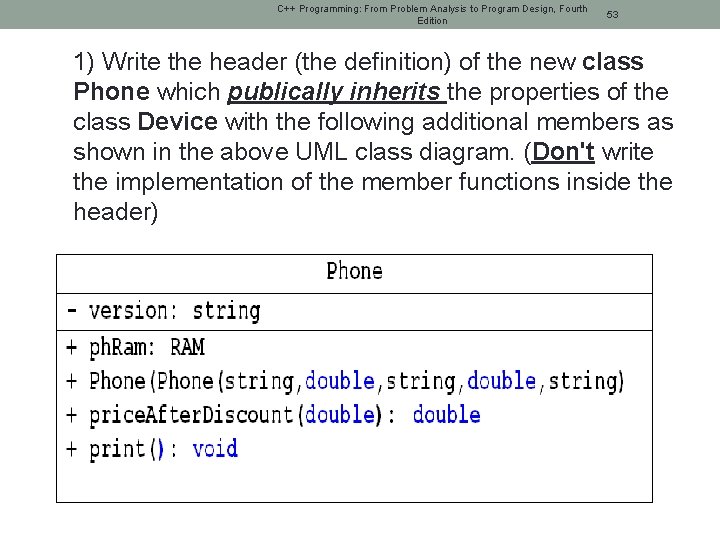
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 53 1) Write the header (the definition) of the new class Phone which publically inherits the properties of the class Device with the following additional members as shown in the above UML class diagram. (Don't write the implementation of the member functions inside the header)
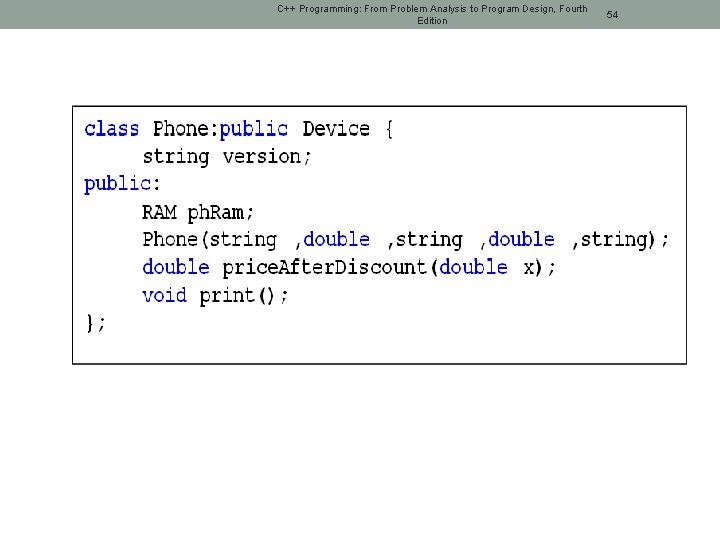
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 54
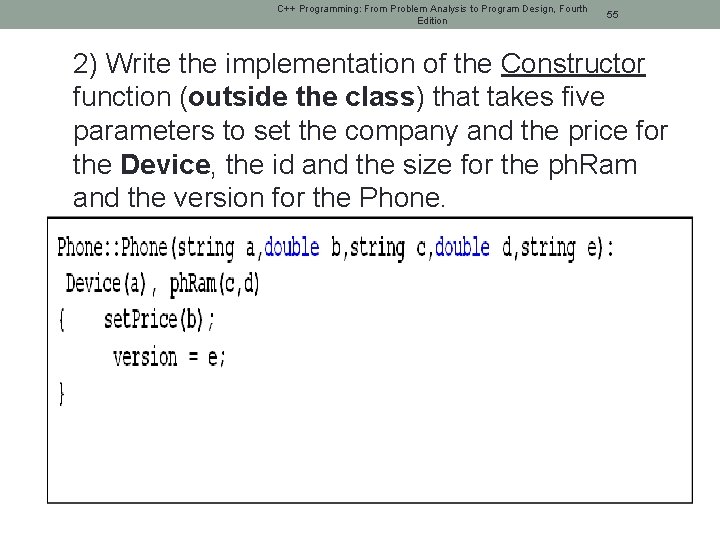
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 55 2) Write the implementation of the Constructor function (outside the class) that takes five parameters to set the company and the price for the Device, the id and the size for the ph. Ram and the version for the Phone.
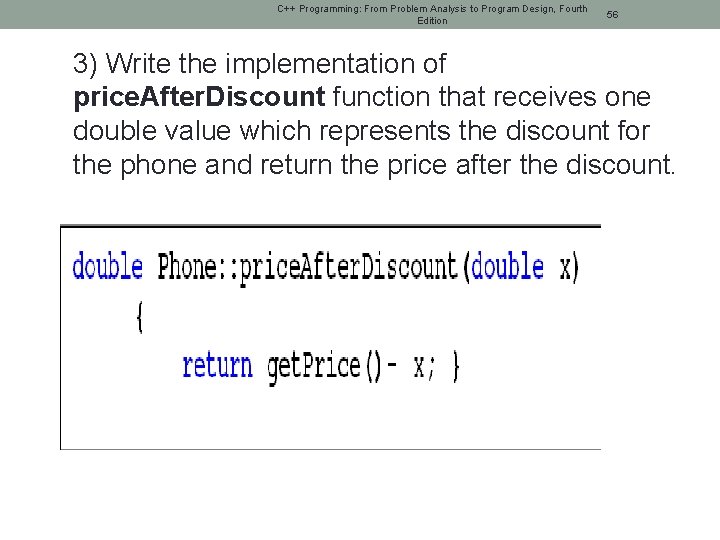
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 56 3) Write the implementation of price. After. Discount function that receives one double value which represents the discount for the phone and return the price after the discount.
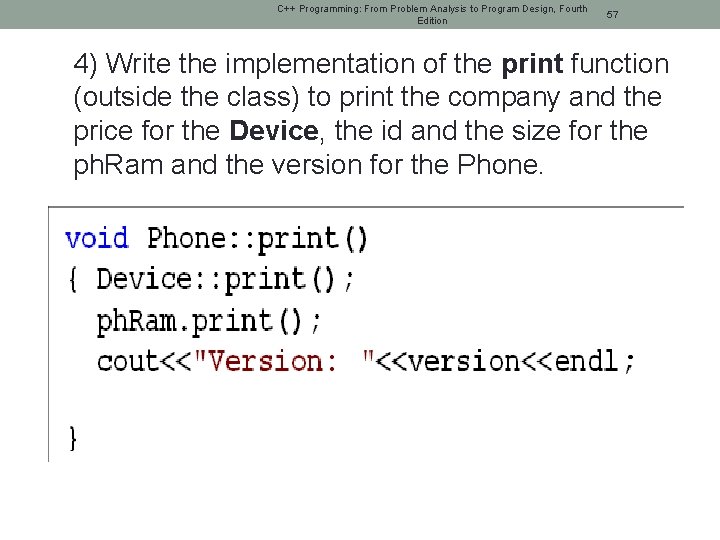
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 57 4) Write the implementation of the print function (outside the class) to print the company and the price for the Device, the id and the size for the ph. Ram and the version for the Phone.
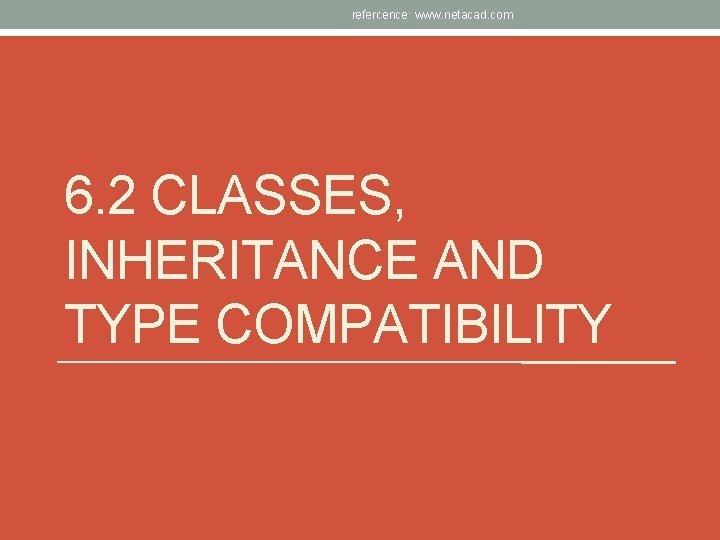
refercence: www. netacad. com 6. 2 CLASSES, INHERITANCE AND TYPE COMPATIBILITY
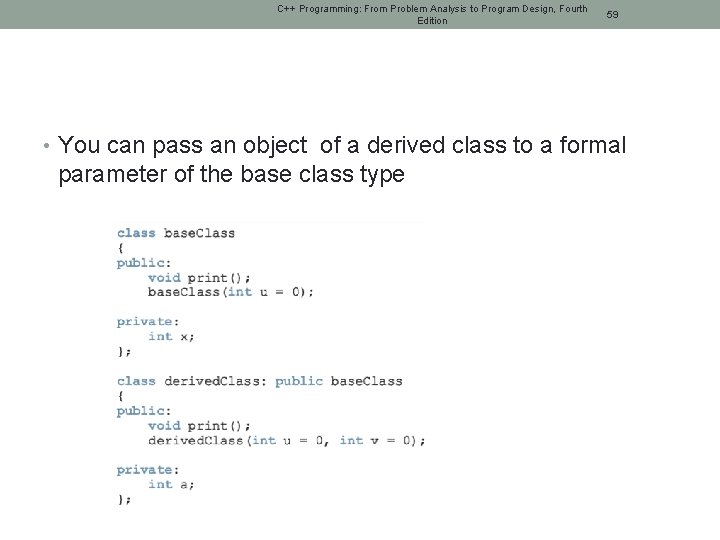
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 59 • You can pass an object of a derived class to a formal parameter of the base class type
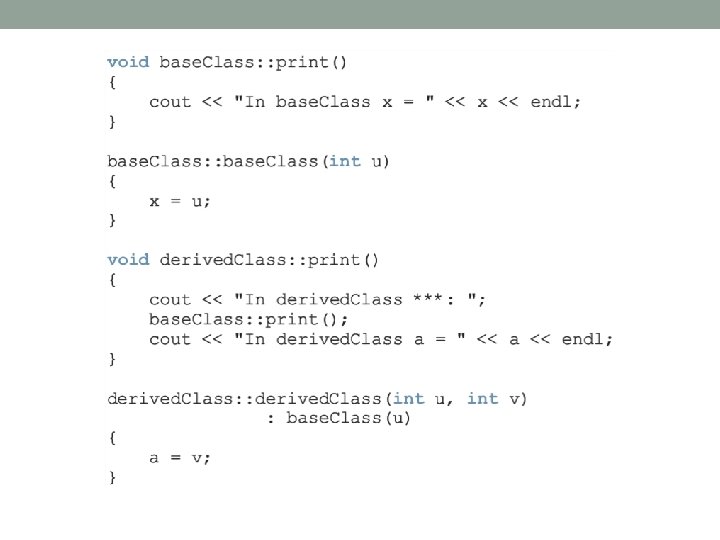
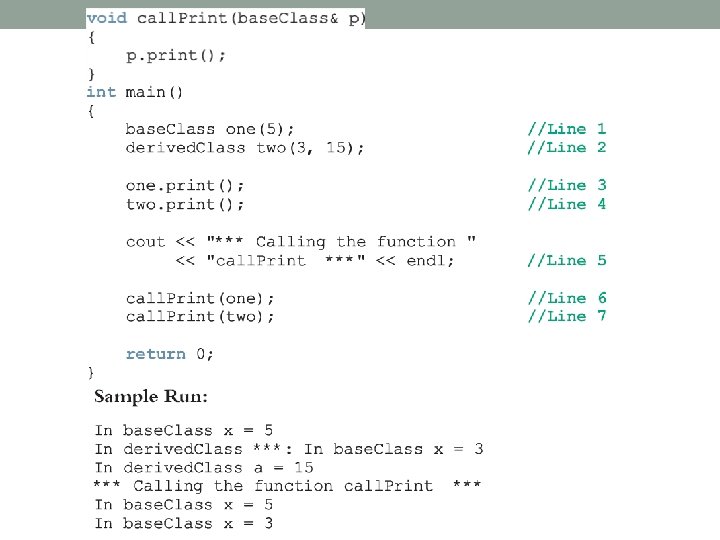
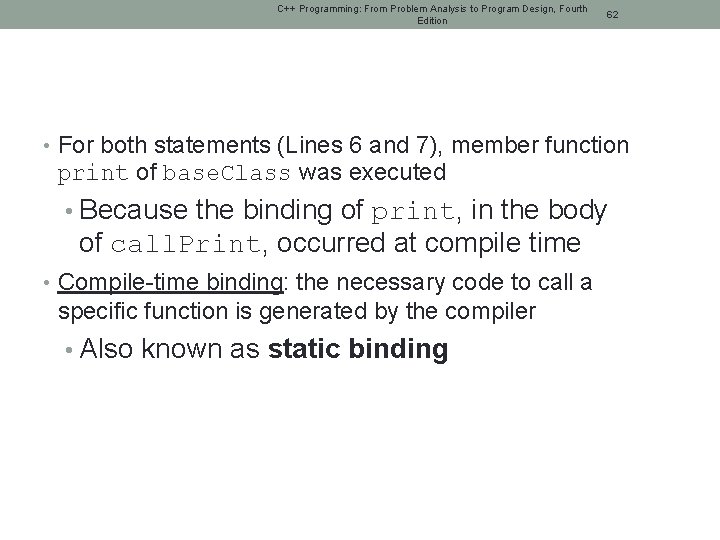
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 62 • For both statements (Lines 6 and 7), member function print of base. Class was executed • Because the binding of print, in the body of call. Print, occurred at compile time • Compile-time binding: the necessary code to call a specific function is generated by the compiler • Also known as static binding
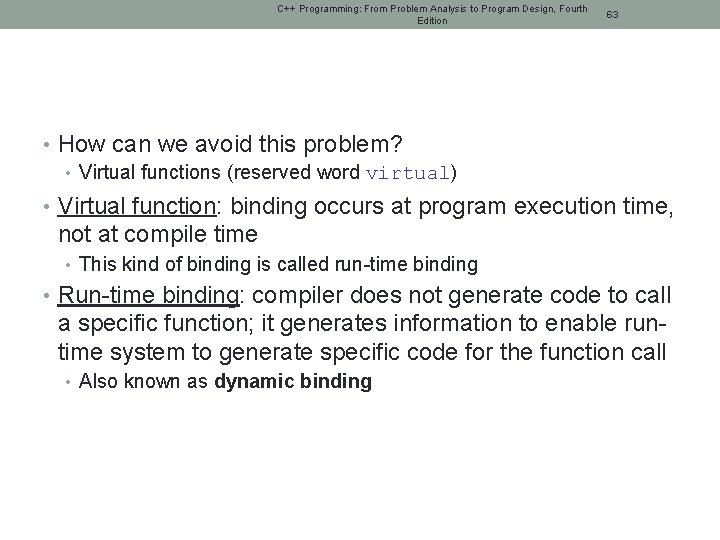
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 63 • How can we avoid this problem? • Virtual functions (reserved word virtual) • Virtual function: binding occurs at program execution time, not at compile time • This kind of binding is called run-time binding • Run-time binding: compiler does not generate code to call a specific function; it generates information to enable runtime system to generate specific code for the function call • Also known as dynamic binding
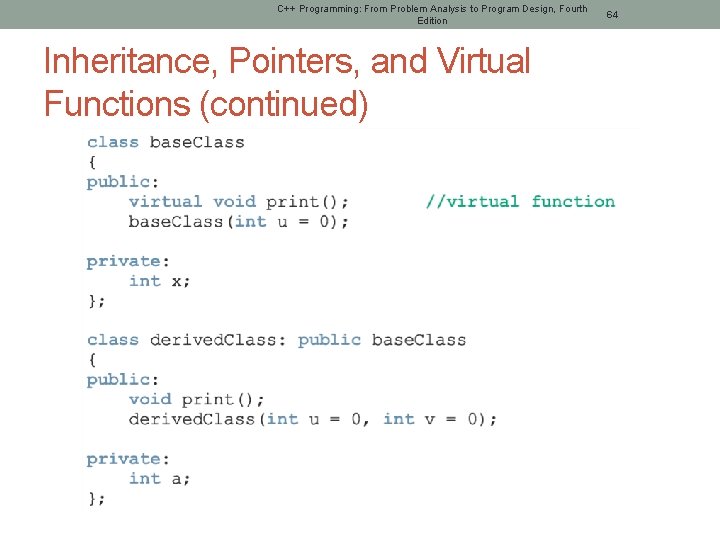
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Inheritance, Pointers, and Virtual Functions (continued) 64
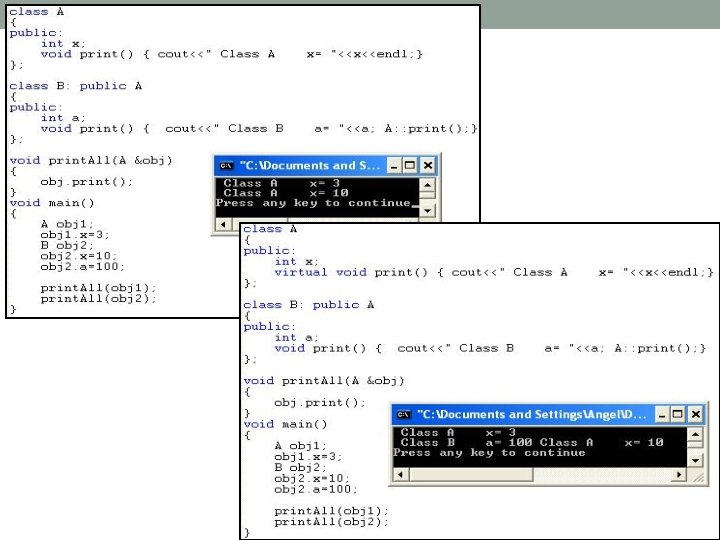
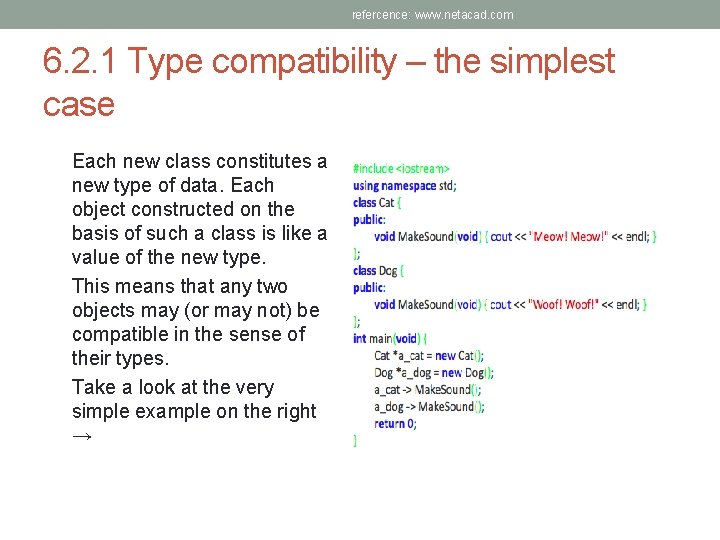
refercence: www. netacad. com 6. 2. 1 Type compatibility – the simplest case Each new class constitutes a new type of data. Each object constructed on the basis of such a class is like a value of the new type. This means that any two objects may (or may not) be compatible in the sense of their types. Take a look at the very simple example on the right →
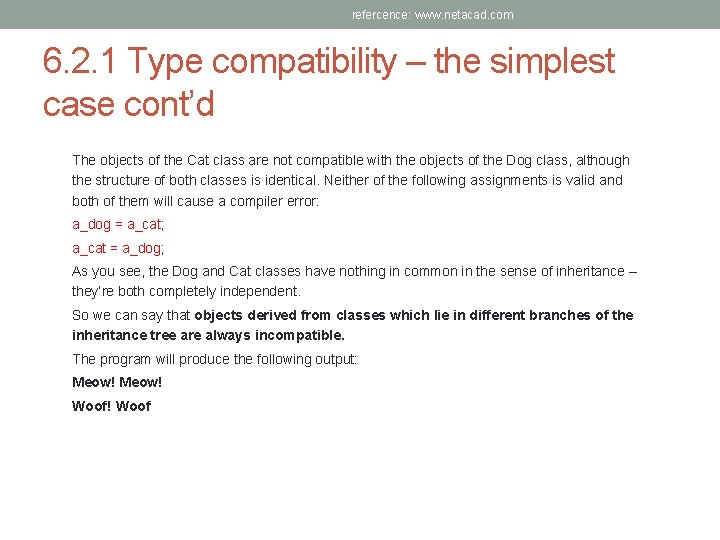
refercence: www. netacad. com 6. 2. 1 Type compatibility – the simplest case cont’d The objects of the Cat class are not compatible with the objects of the Dog class, although the structure of both classes is identical. Neither of the following assignments is valid and both of them will cause a compiler error: a_dog = a_cat; a_cat = a_dog; As you see, the Dog and Cat classes have nothing in common in the sense of inheritance – they’re both completely independent. So we can say that objects derived from classes which lie in different branches of the inheritance tree are always incompatible. The program will produce the following output: Meow! Woof! Woof
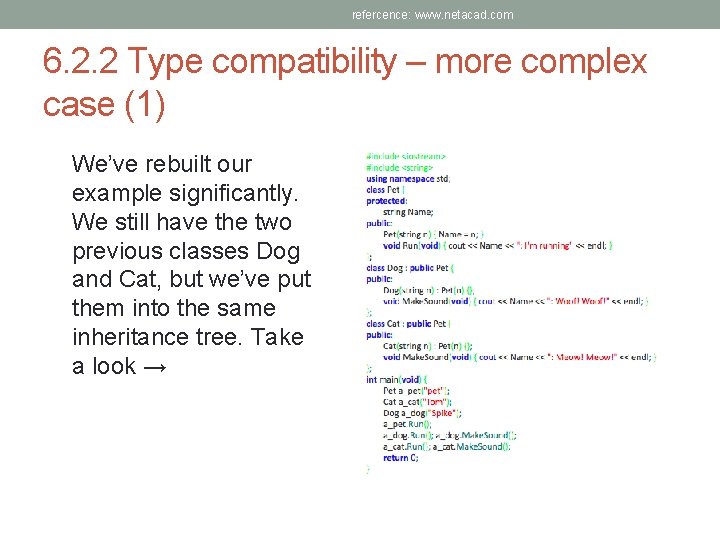
refercence: www. netacad. com 6. 2. 2 Type compatibility – more complex case (1) We’ve rebuilt our example significantly. We still have the two previous classes Dog and Cat, but we’ve put them into the same inheritance tree. Take a look →
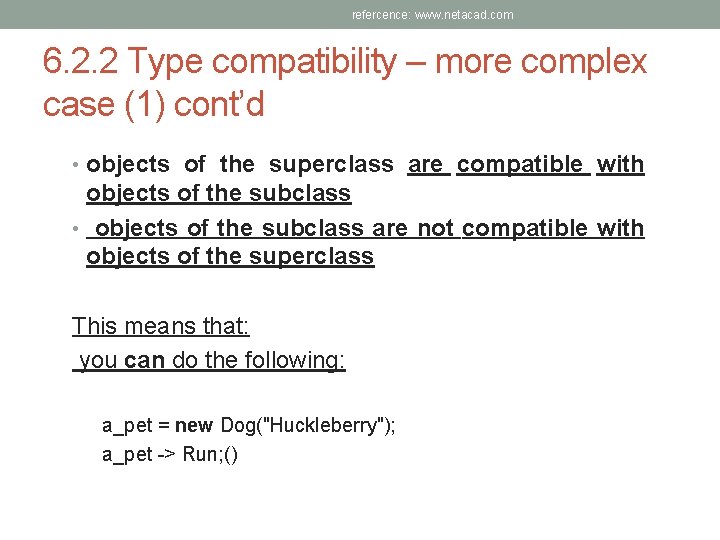
refercence: www. netacad. com 6. 2. 2 Type compatibility – more complex case (1) cont’d • objects of the superclass are compatible with objects of the subclass • objects of the subclass are not compatible with objects of the superclass This means that: you can do the following: a_pet = new Dog("Huckleberry"); a_pet -> Run; ()
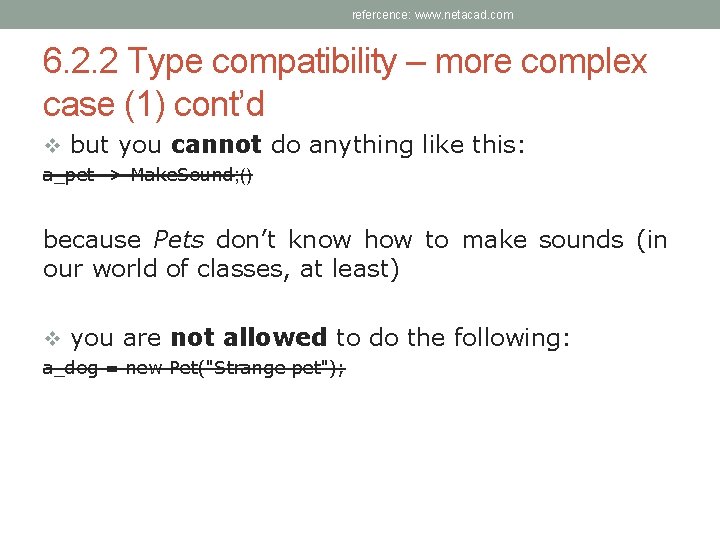
refercence: www. netacad. com 6. 2. 2 Type compatibility – more complex case (1) cont’d v but you cannot do anything like this: a_pet -> Make. Sound; () because Pets don’t know how to make sounds (in our world of classes, at least) v you are not allowed to do the following: a_dog = new Pet("Strange pet");
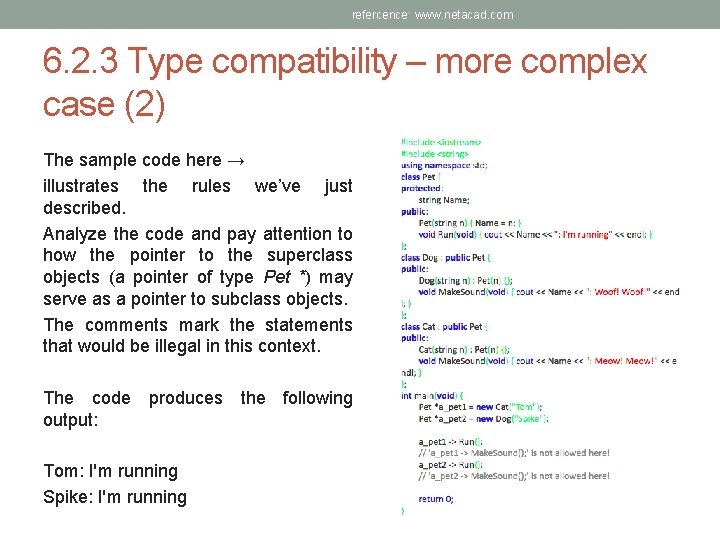
refercence: www. netacad. com 6. 2. 3 Type compatibility – more complex case (2) The sample code here → illustrates the rules we’ve just described. Analyze the code and pay attention to how the pointer to the superclass objects (a pointer of type Pet *) may serve as a pointer to subclass objects. The comments mark the statements that would be illegal in this context. The code produces the following output: Tom: I'm running Spike: I'm running
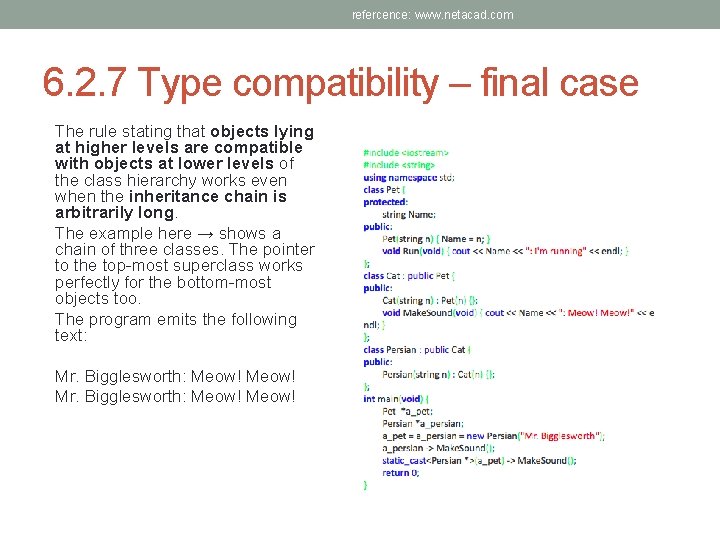
refercence: www. netacad. com 6. 2. 7 Type compatibility – final case The rule stating that objects lying at higher levels are compatible with objects at lower levels of the class hierarchy works even when the inheritance chain is arbitrarily long. The example here → shows a chain of three classes. The pointer to the top-most superclass works perfectly for the bottom-most objects too. The program emits the following text: Mr. Bigglesworth: Meow!
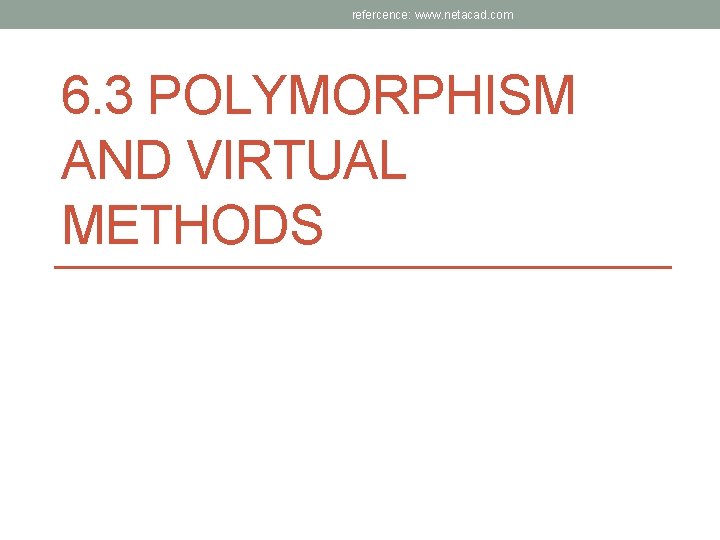
refercence: www. netacad. com 6. 3 POLYMORPHISM AND VIRTUAL METHODS
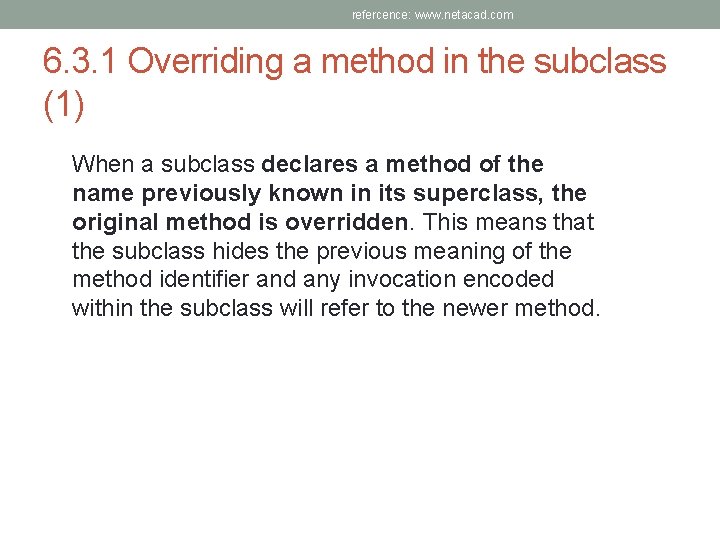
refercence: www. netacad. com 6. 3. 1 Overriding a method in the subclass (1) When a subclass declares a method of the name previously known in its superclass, the original method is overridden. This means that the subclass hides the previous meaning of the method identifier and any invocation encoded within the subclass will refer to the newer method.
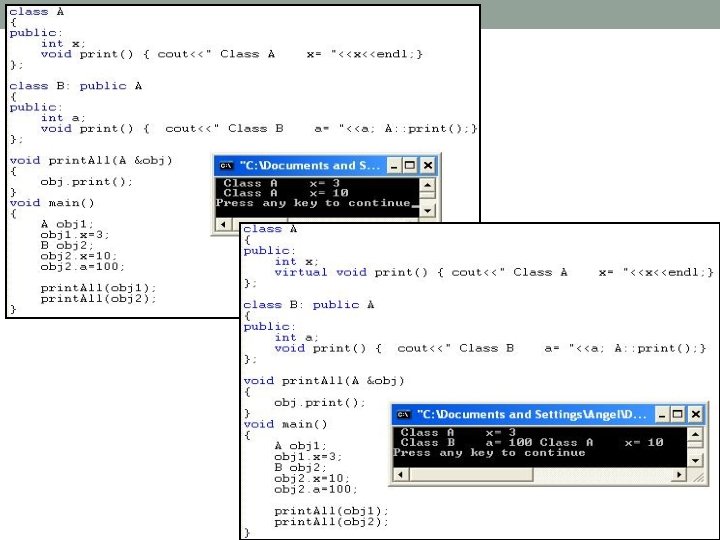
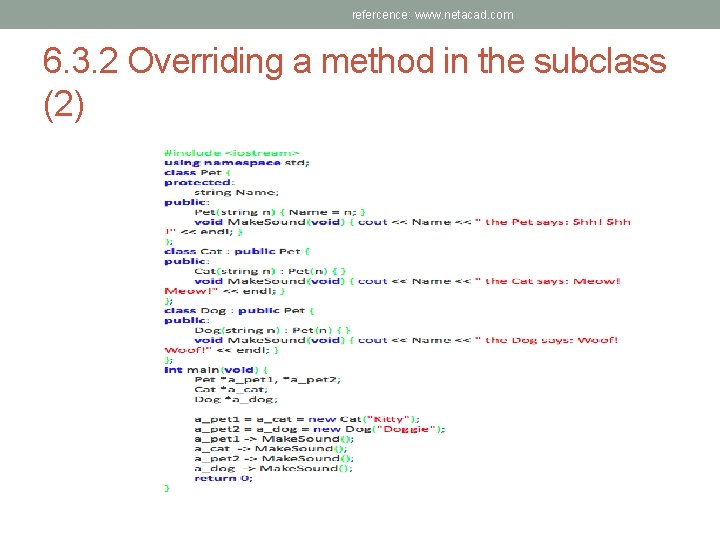
refercence: www. netacad. com 6. 3. 2 Overriding a method in the subclass (2)
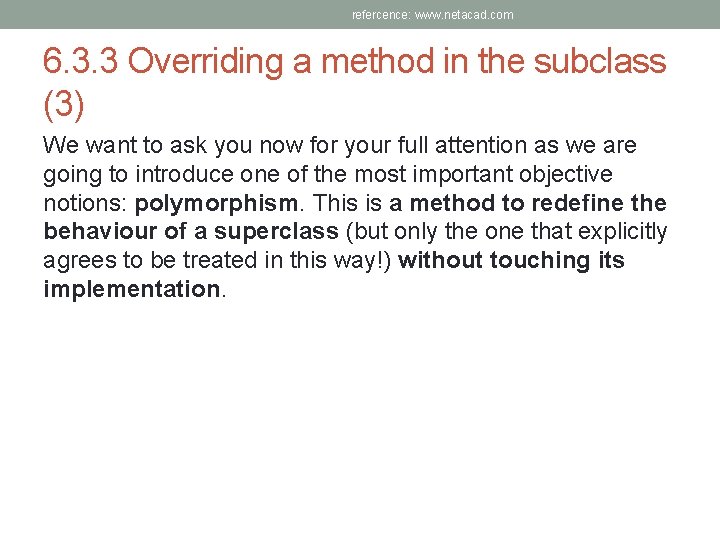
refercence: www. netacad. com 6. 3. 3 Overriding a method in the subclass (3) We want to ask you now for your full attention as we are going to introduce one of the most important objective notions: polymorphism. This is a method to redefine the behaviour of a superclass (but only the one that explicitly agrees to be treated in this way!) without touching its implementation.
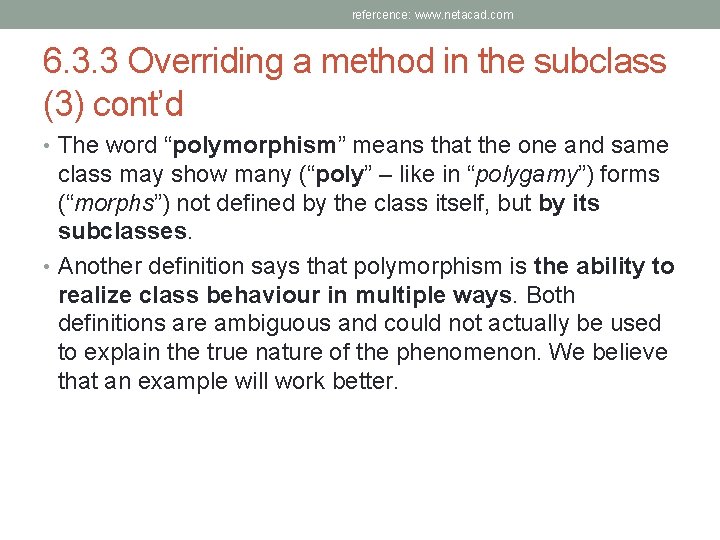
refercence: www. netacad. com 6. 3. 3 Overriding a method in the subclass (3) cont’d • The word “polymorphism” means that the one and same class may show many (“poly” – like in “polygamy”) forms (“morphs”) not defined by the class itself, but by its subclasses. • Another definition says that polymorphism is the ability to realize class behaviour in multiple ways. Both definitions are ambiguous and could not actually be used to explain the true nature of the phenomenon. We believe that an example will work better.
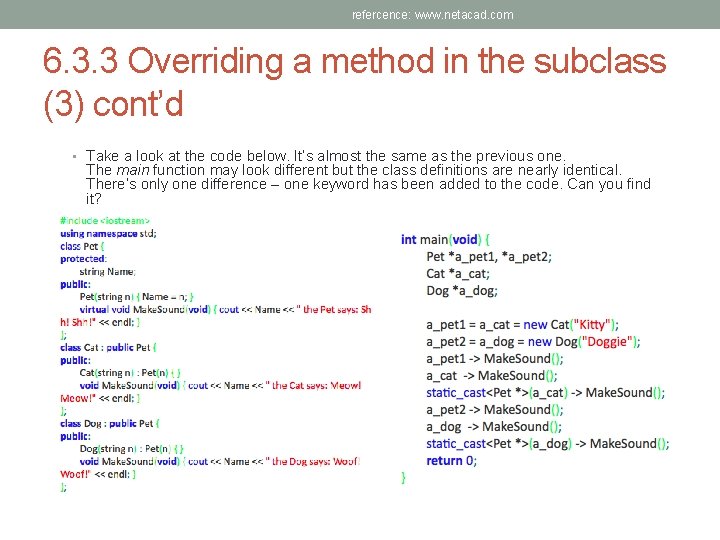
refercence: www. netacad. com 6. 3. 3 Overriding a method in the subclass (3) cont’d • Take a look at the code below. It’s almost the same as the previous one. The main function may look different but the class definitions are nearly identical. There’s only one difference – one keyword has been added to the code. Can you find it?
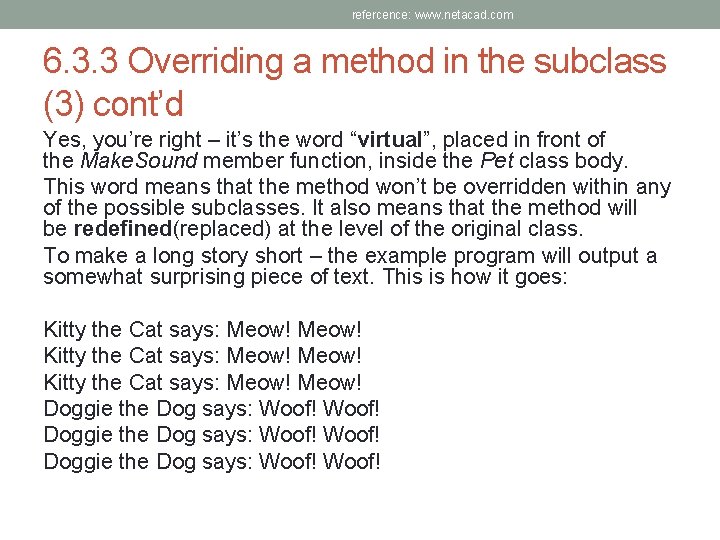
refercence: www. netacad. com 6. 3. 3 Overriding a method in the subclass (3) cont’d Yes, you’re right – it’s the word “virtual”, placed in front of the Make. Sound member function, inside the Pet class body. This word means that the method won’t be overridden within any of the possible subclasses. It also means that the method will be redefined(replaced) at the level of the original class. To make a long story short – the example program will output a somewhat surprising piece of text. This is how it goes: Kitty the Cat says: Meow! Kitty the Cat says: Meow! Doggie the Dog says: Woof! Doggie the Dog says: Woof!
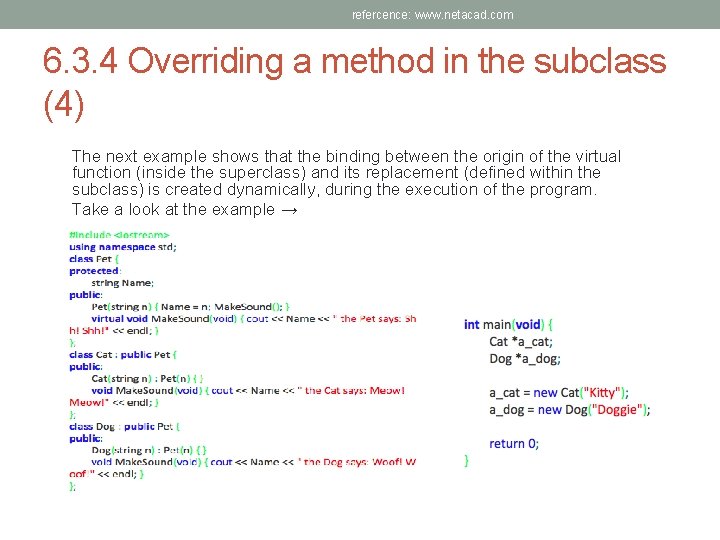
refercence: www. netacad. com 6. 3. 4 Overriding a method in the subclass (4) The next example shows that the binding between the origin of the virtual function (inside the superclass) and its replacement (defined within the subclass) is created dynamically, during the execution of the program. Take a look at the example →
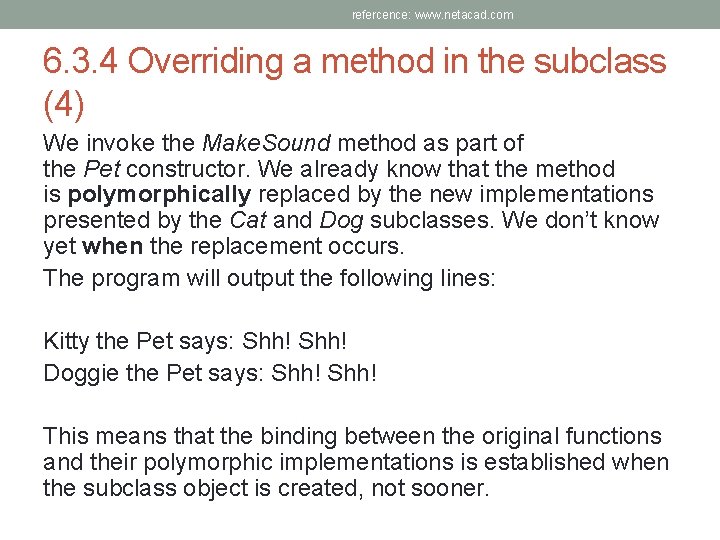
refercence: www. netacad. com 6. 3. 4 Overriding a method in the subclass (4) We invoke the Make. Sound method as part of the Pet constructor. We already know that the method is polymorphically replaced by the new implementations presented by the Cat and Dog subclasses. We don’t know yet when the replacement occurs. The program will output the following lines: Kitty the Pet says: Shh! Doggie the Pet says: Shh! This means that the binding between the original functions and their polymorphic implementations is established when the subclass object is created, not sooner.
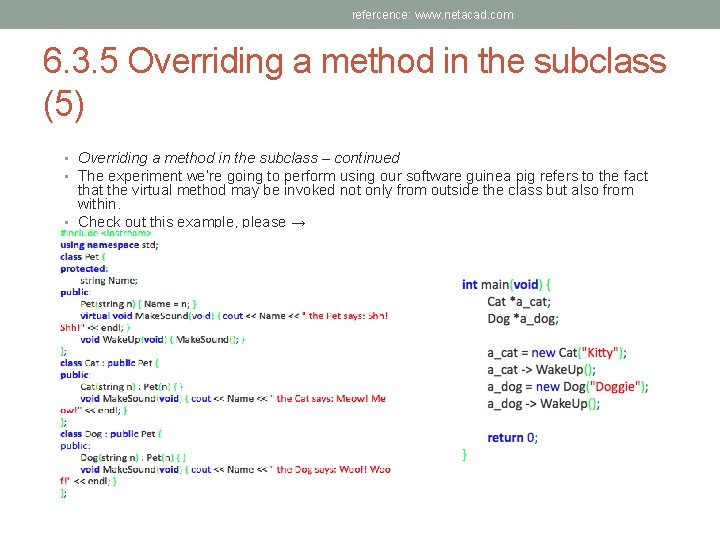
refercence: www. netacad. com 6. 3. 5 Overriding a method in the subclass (5) • Overriding a method in the subclass – continued • The experiment we’re going to perform using our software guinea pig refers to the fact that the virtual method may be invoked not only from outside the class but also from within. • Check out this example, please →
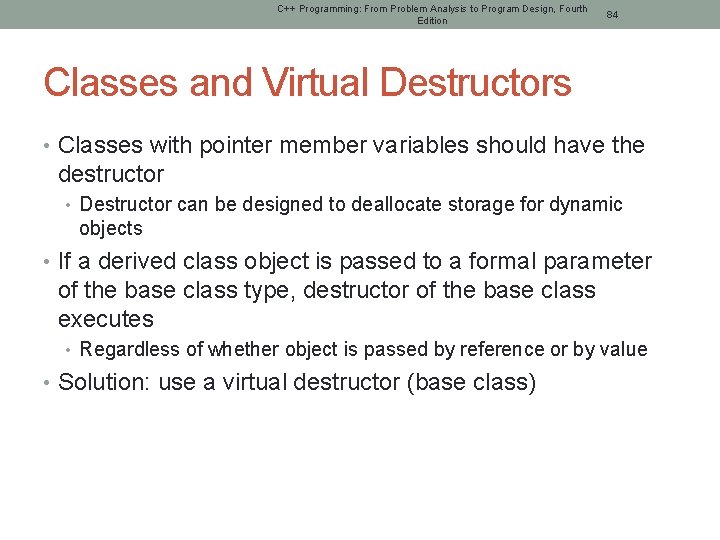
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 84 Classes and Virtual Destructors • Classes with pointer member variables should have the destructor • Destructor can be designed to deallocate storage for dynamic objects • If a derived class object is passed to a formal parameter of the base class type, destructor of the base class executes • Regardless of whether object is passed by reference or by value • Solution: use a virtual destructor (base class)
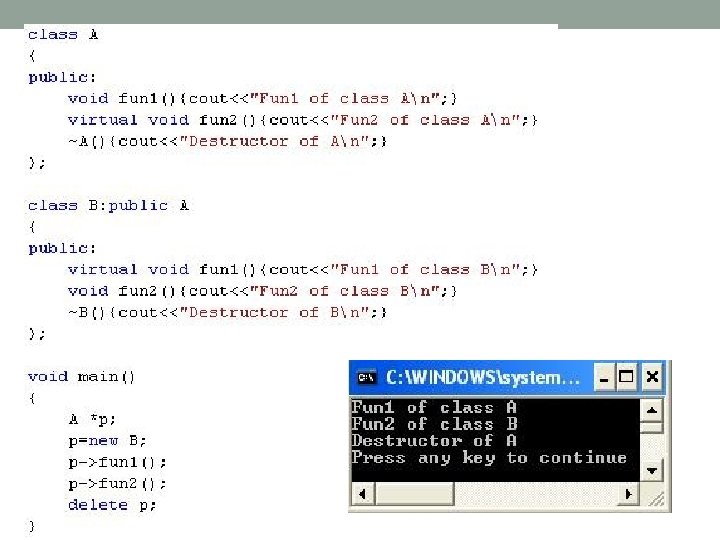
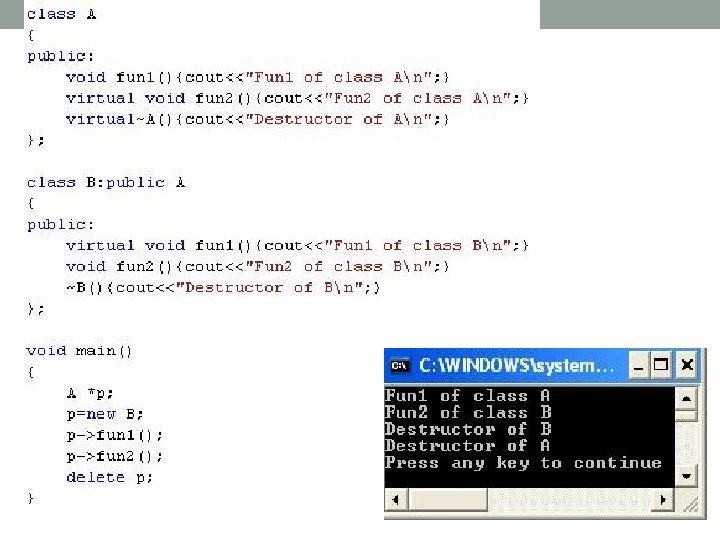
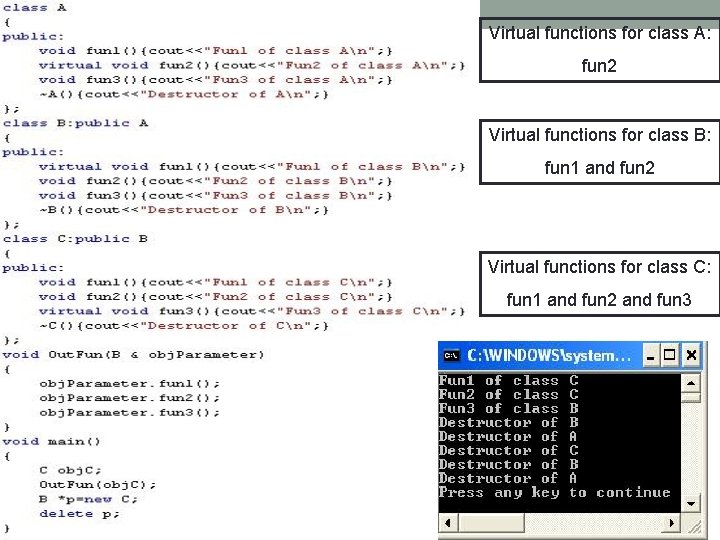
Virtual functions for class A: fun 2 Virtual functions for class B: fun 1 and fun 2 Virtual functions for class C: fun 1 and fun 2 and fun 3
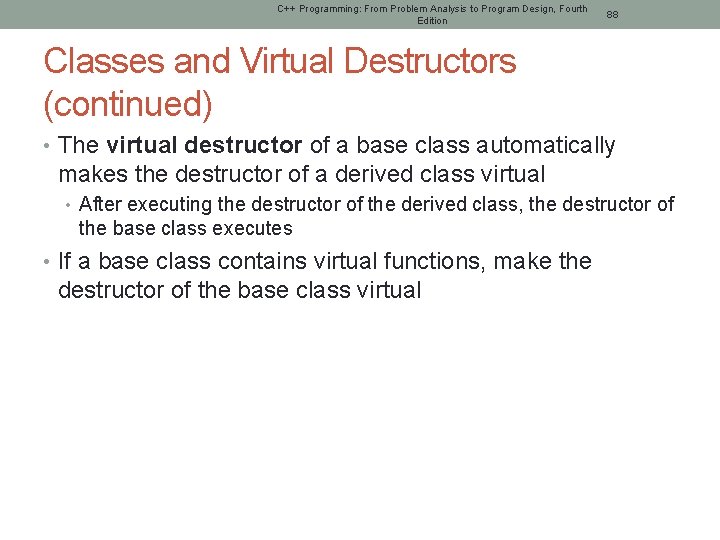
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 88 Classes and Virtual Destructors (continued) • The virtual destructor of a base class automatically makes the destructor of a derived class virtual • After executing the destructor of the derived class, the destructor of the base class executes • If a base class contains virtual functions, make the destructor of the base class virtual
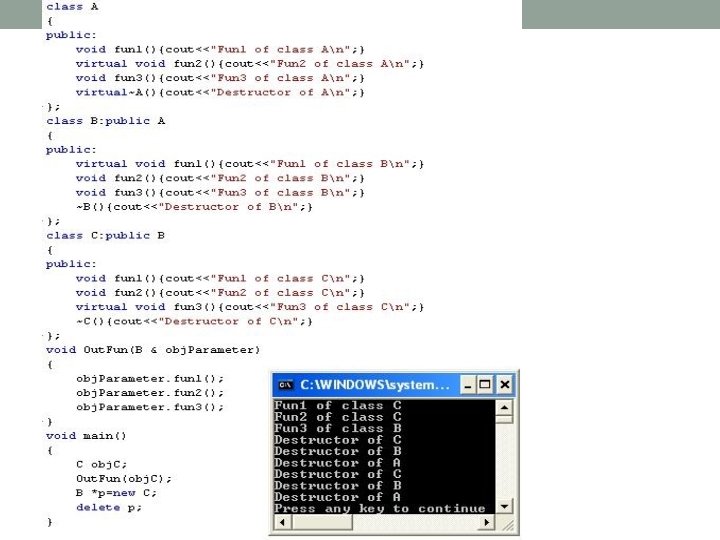
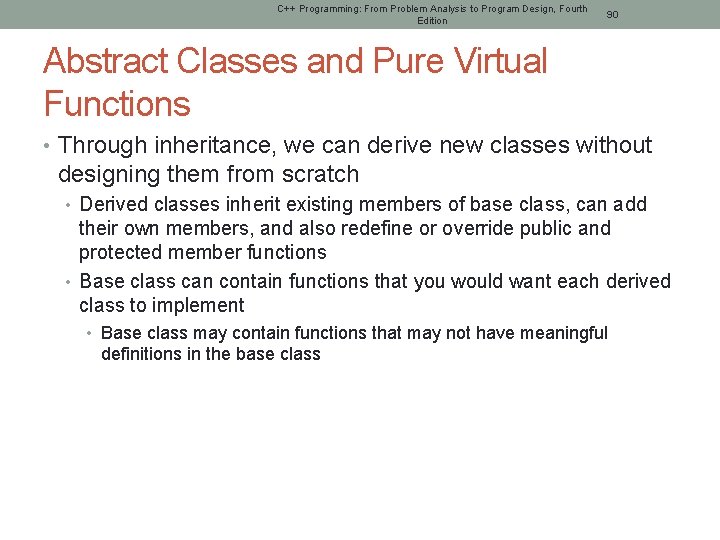
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 90 Abstract Classes and Pure Virtual Functions • Through inheritance, we can derive new classes without designing them from scratch • Derived classes inherit existing members of base class, can add their own members, and also redefine or override public and protected member functions • Base class can contain functions that you would want each derived class to implement • Base class may contain functions that may not have meaningful definitions in the base class
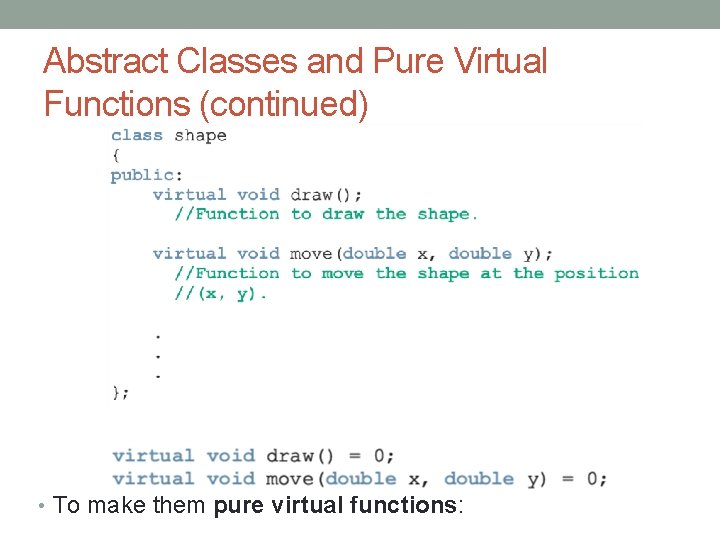
Abstract Classes and Pure Virtual Functions (continued) • To make them pure virtual functions:
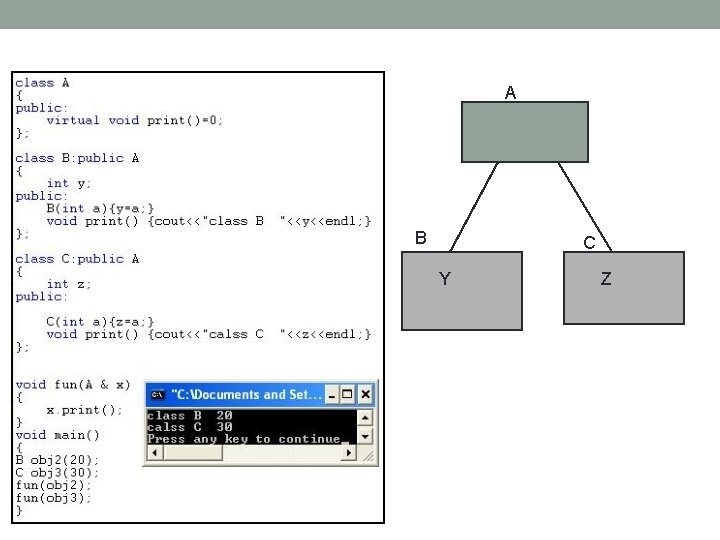
A B C Y Z
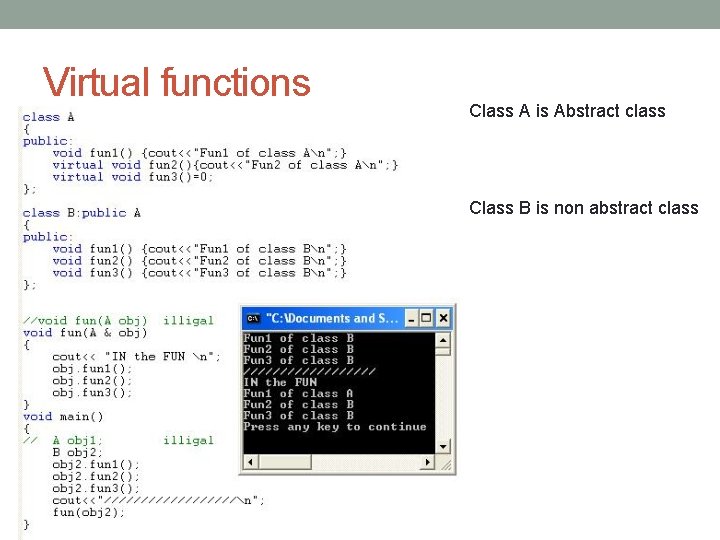
Virtual functions Class A is Abstract class Class B is non abstract class
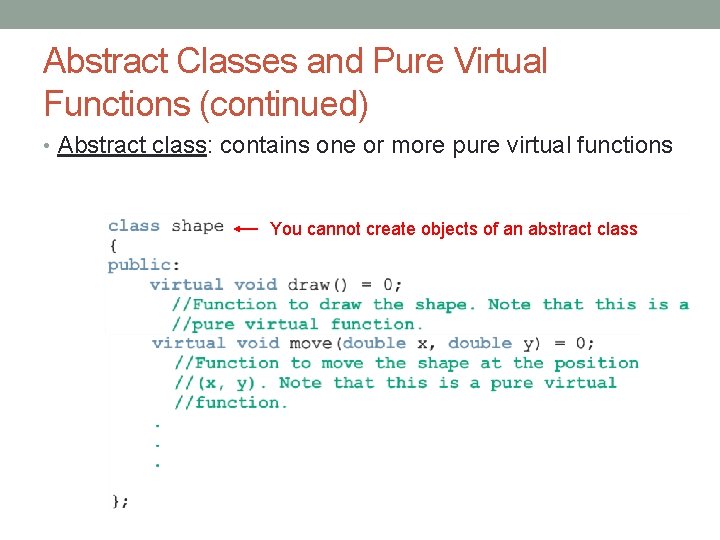
Abstract Classes and Pure Virtual Functions (continued) • Abstract class: contains one or more pure virtual functions You cannot create objects of an abstract class
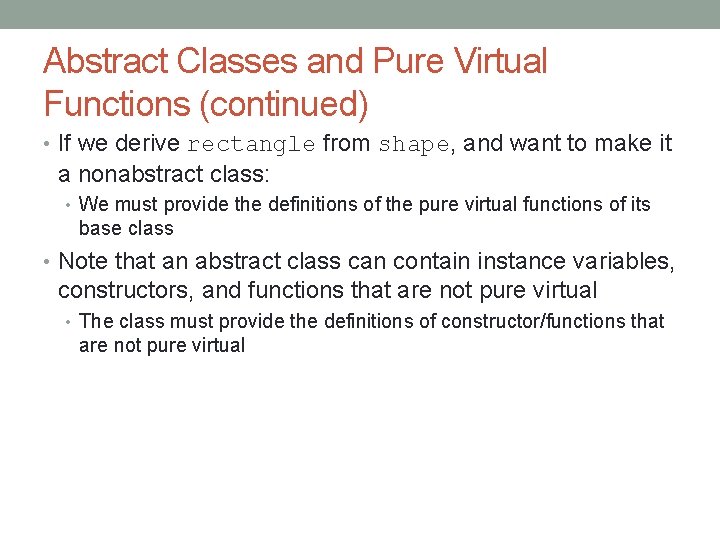
Abstract Classes and Pure Virtual Functions (continued) • If we derive rectangle from shape, and want to make it a nonabstract class: • We must provide the definitions of the pure virtual functions of its base class • Note that an abstract class can contain instance variables, constructors, and functions that are not pure virtual • The class must provide the definitions of constructor/functions that are not pure virtual
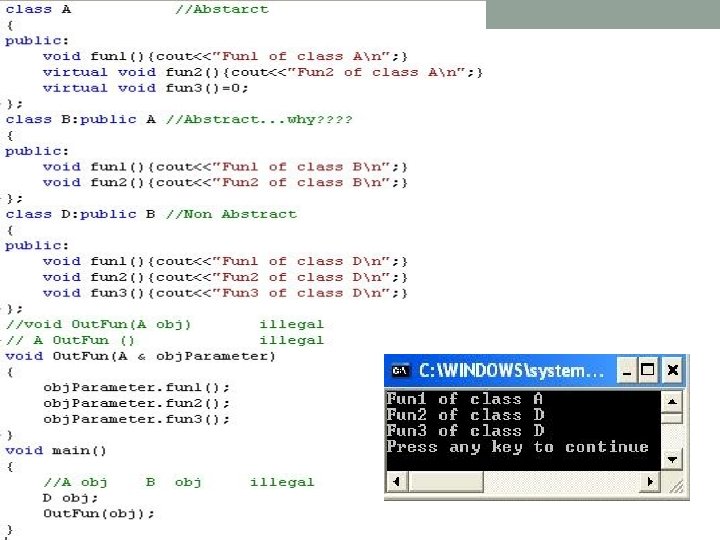
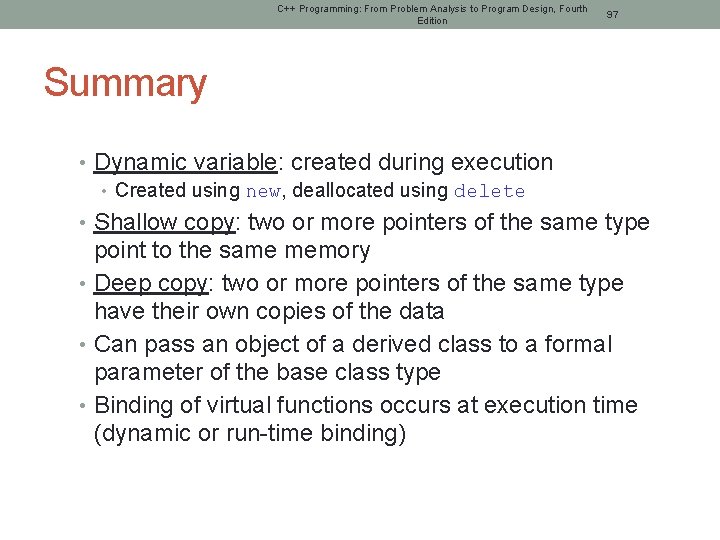
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 97 Summary • Dynamic variable: created during execution • Created using new, deallocated using delete • Shallow copy: two or more pointers of the same type point to the same memory • Deep copy: two or more pointers of the same type have their own copies of the data • Can pass an object of a derived class to a formal parameter of the base class type • Binding of virtual functions occurs at execution time (dynamic or run-time binding)
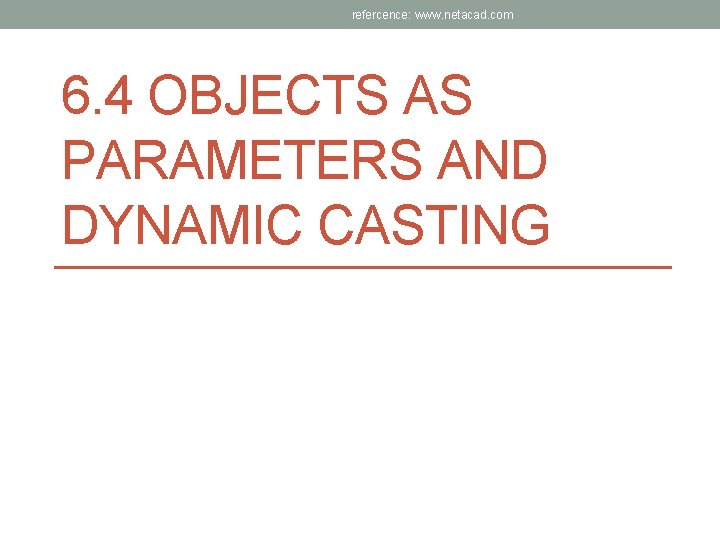
refercence: www. netacad. com 6. 4 OBJECTS AS PARAMETERS AND DYNAMIC CASTING
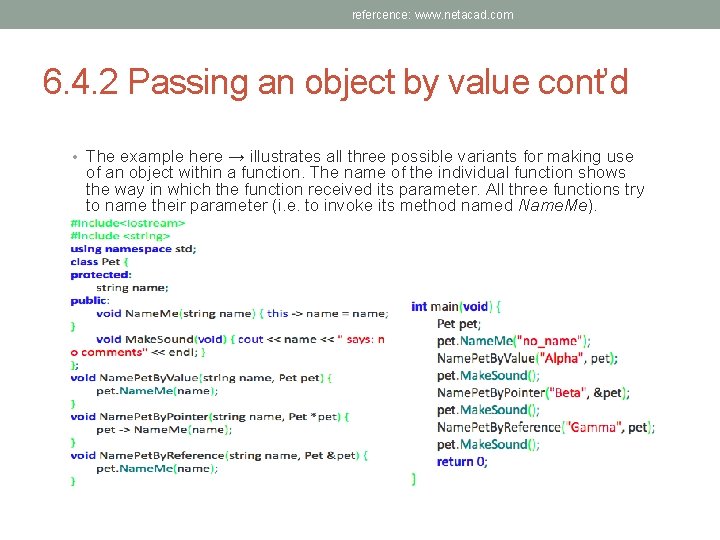
refercence: www. netacad. com 6. 4. 2 Passing an object by value cont’d • The example here → illustrates all three possible variants for making use of an object within a function. The name of the individual function shows the way in which the function received its parameter. All three functions try to name their parameter (i. e. to invoke its method named Name. Me).
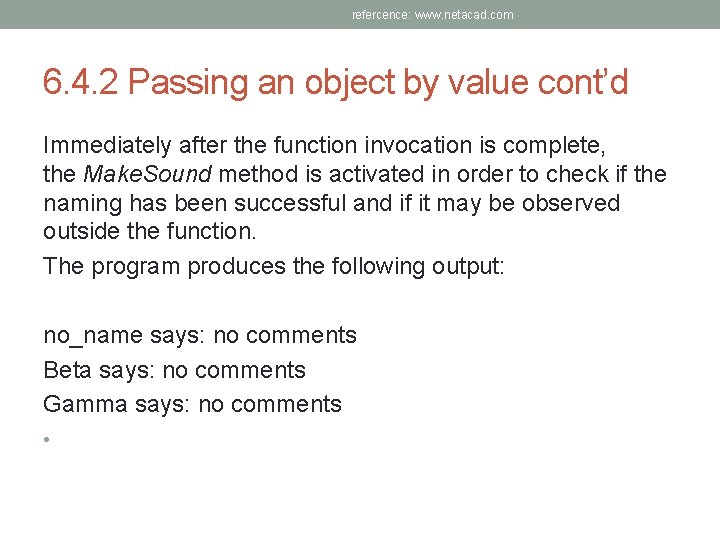
refercence: www. netacad. com 6. 4. 2 Passing an object by value cont’d Immediately after the function invocation is complete, the Make. Sound method is activated in order to check if the naming has been successful and if it may be observed outside the function. The program produces the following output: no_name says: no comments Beta says: no comments Gamma says: no comments •
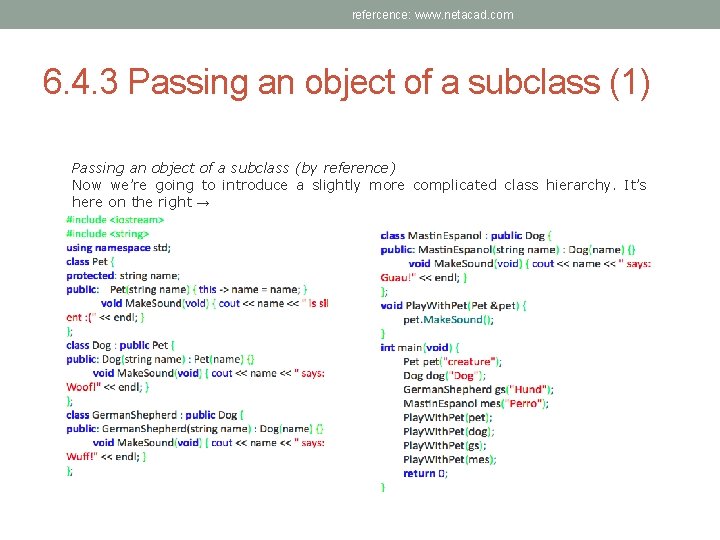
refercence: www. netacad. com 6. 4. 3 Passing an object of a subclass (1) Passing an object of a subclass (by reference) Now we’re going to introduce a slightly more complicated class hierarchy. It’s here on the right →
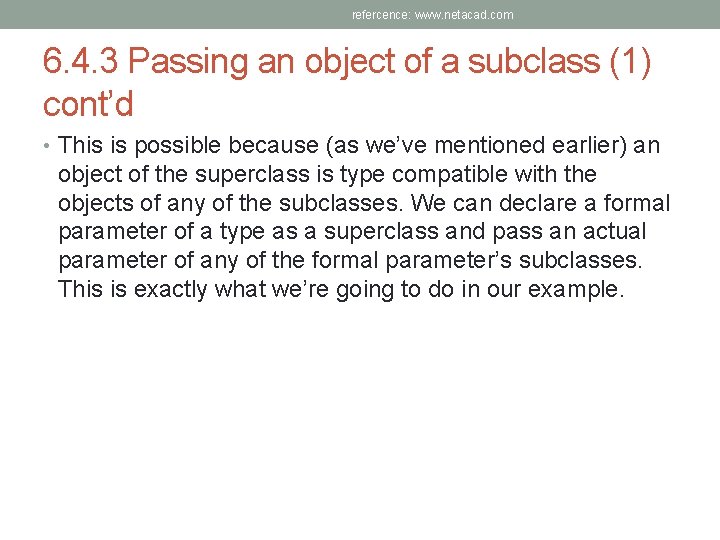
refercence: www. netacad. com 6. 4. 3 Passing an object of a subclass (1) cont’d • This is possible because (as we’ve mentioned earlier) an object of the superclass is type compatible with the objects of any of the subclasses. We can declare a formal parameter of a type as a superclass and pass an actual parameter of any of the formal parameter’s subclasses. This is exactly what we’re going to do in our example.
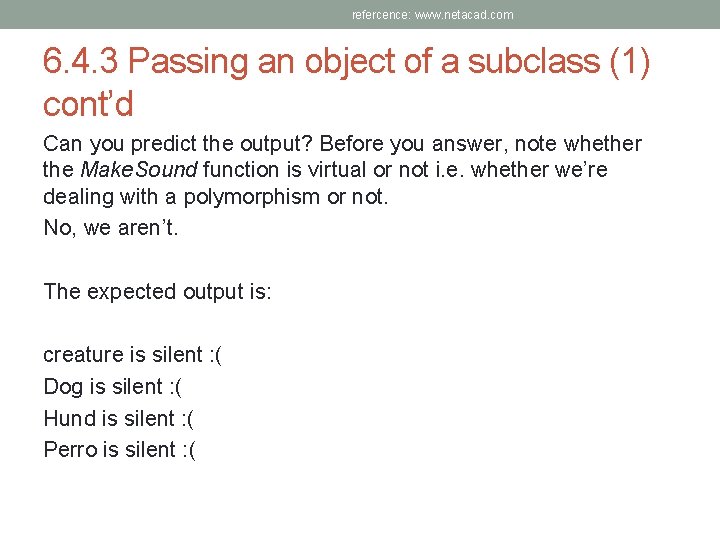
refercence: www. netacad. com 6. 4. 3 Passing an object of a subclass (1) cont’d Can you predict the output? Before you answer, note whether the Make. Sound function is virtual or not i. e. whether we’re dealing with a polymorphism or not. No, we aren’t. The expected output is: creature is silent : ( Dog is silent : ( Hund is silent : ( Perro is silent : (
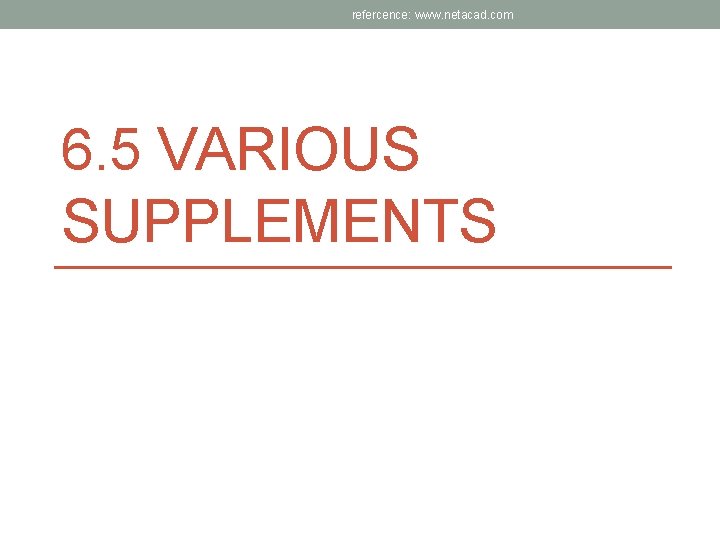
refercence: www. netacad. com 6. 5 VARIOUS SUPPLEMENTS
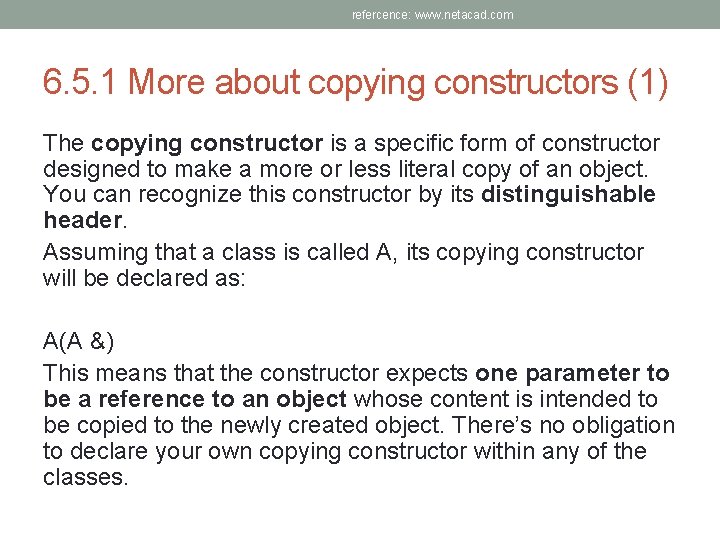
refercence: www. netacad. com 6. 5. 1 More about copying constructors (1) The copying constructor is a specific form of constructor designed to make a more or less literal copy of an object. You can recognize this constructor by its distinguishable header. Assuming that a class is called A, its copying constructor will be declared as: A(A &) This means that the constructor expects one parameter to be a reference to an object whose content is intended to be copied to the newly created object. There’s no obligation to declare your own copying constructor within any of the classes.
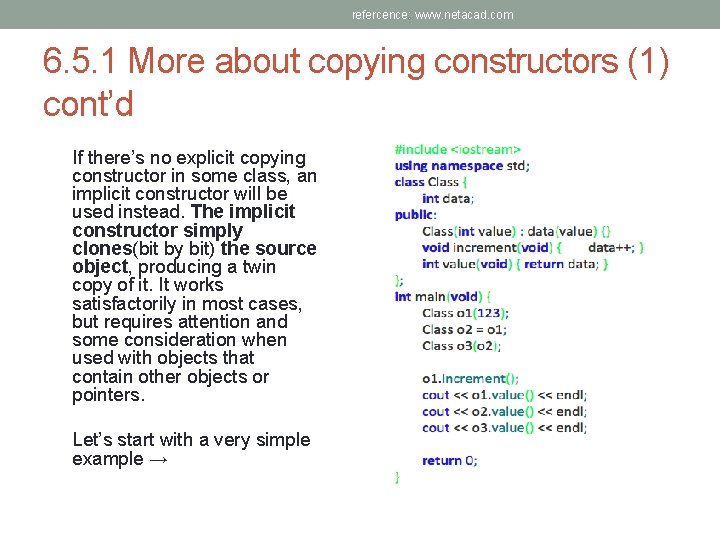
refercence: www. netacad. com 6. 5. 1 More about copying constructors (1) cont’d If there’s no explicit copying constructor in some class, an implicit constructor will be used instead. The implicit constructor simply clones(bit by bit) the source object, producing a twin copy of it. It works satisfactorily in most cases, but requires attention and some consideration when used with objects that contain other objects or pointers. Let’s start with a very simple example →
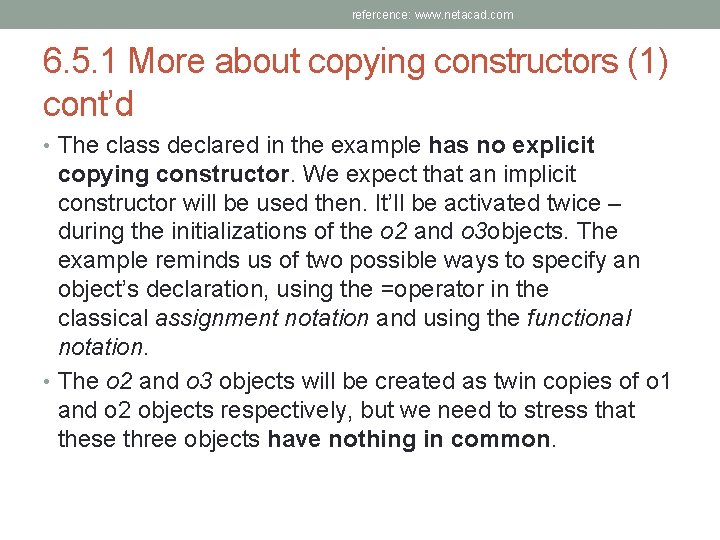
refercence: www. netacad. com 6. 5. 1 More about copying constructors (1) cont’d • The class declared in the example has no explicit copying constructor. We expect that an implicit constructor will be used then. It’ll be activated twice – during the initializations of the o 2 and o 3 objects. The example reminds us of two possible ways to specify an object’s declaration, using the =operator in the classical assignment notation and using the functional notation. • The o 2 and o 3 objects will be created as twin copies of o 1 and o 2 objects respectively, but we need to stress that these three objects have nothing in common.
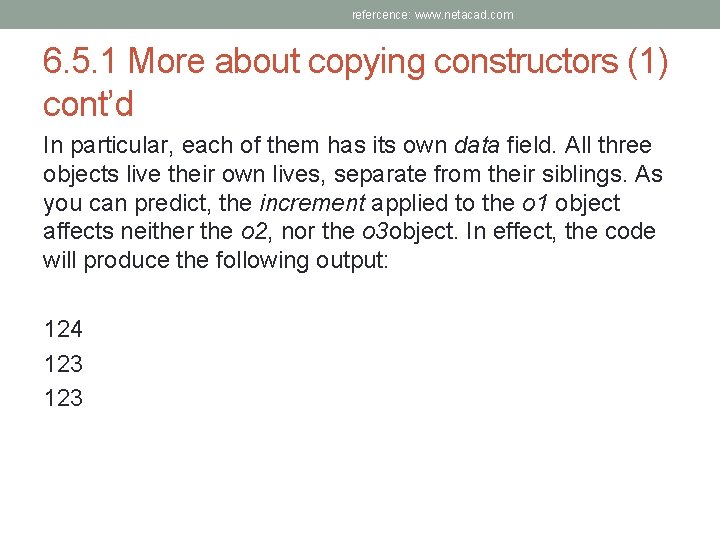
refercence: www. netacad. com 6. 5. 1 More about copying constructors (1) cont’d In particular, each of them has its own data field. All three objects live their own lives, separate from their siblings. As you can predict, the increment applied to the o 1 object affects neither the o 2, nor the o 3 object. In effect, the code will produce the following output: 124 123
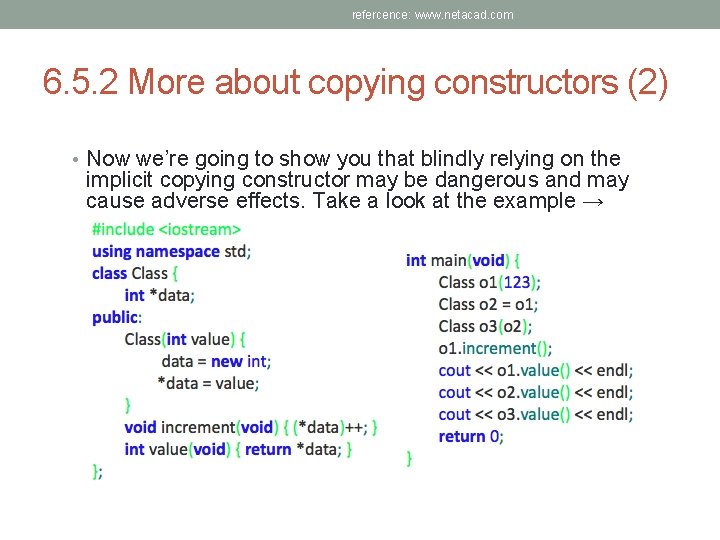
refercence: www. netacad. com 6. 5. 2 More about copying constructors (2) • Now we’re going to show you that blindly relying on the implicit copying constructor may be dangerous and may cause adverse effects. Take a look at the example →
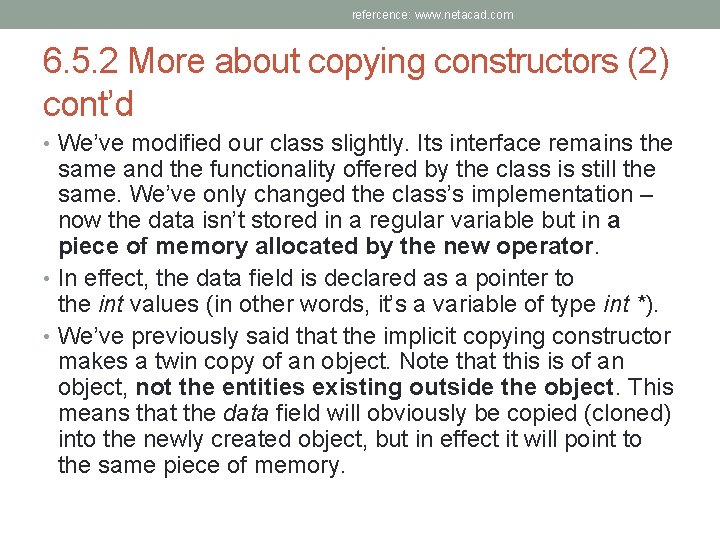
refercence: www. netacad. com 6. 5. 2 More about copying constructors (2) cont’d • We’ve modified our class slightly. Its interface remains the same and the functionality offered by the class is still the same. We’ve only changed the class’s implementation – now the data isn’t stored in a regular variable but in a piece of memory allocated by the new operator. • In effect, the data field is declared as a pointer to the int values (in other words, it’s a variable of type int *). • We’ve previously said that the implicit copying constructor makes a twin copy of an object. Note that this is of an object, not the entities existing outside the object. This means that the data field will obviously be copied (cloned) into the newly created object, but in effect it will point to the same piece of memory.
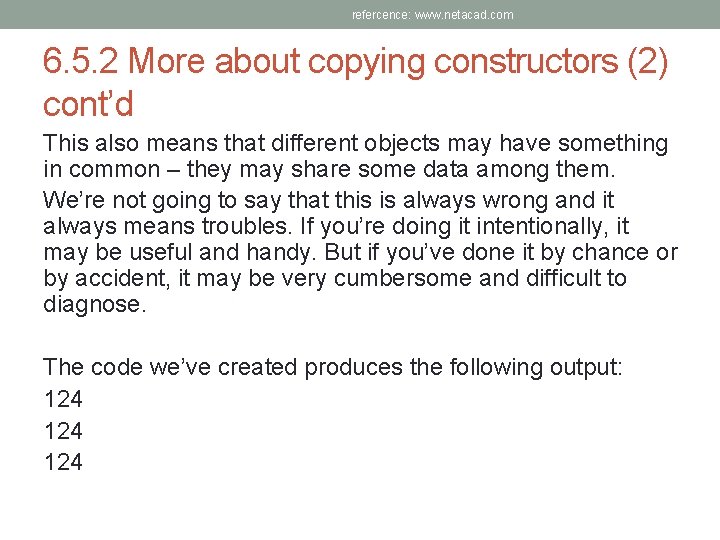
refercence: www. netacad. com 6. 5. 2 More about copying constructors (2) cont’d This also means that different objects may have something in common – they may share some data among them. We’re not going to say that this is always wrong and it always means troubles. If you’re doing it intentionally, it may be useful and handy. But if you’ve done it by chance or by accident, it may be very cumbersome and difficult to diagnose. The code we’ve created produces the following output: 124 124
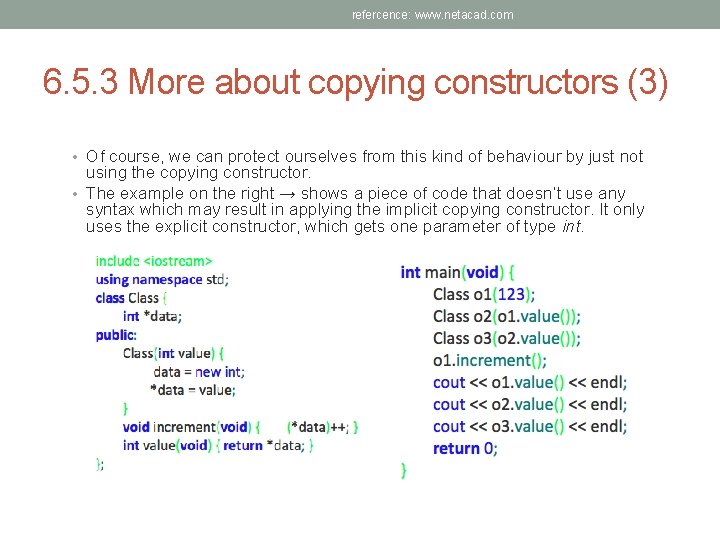
refercence: www. netacad. com 6. 5. 3 More about copying constructors (3) • Of course, we can protect ourselves from this kind of behaviour by just not using the copying constructor. • The example on the right → shows a piece of code that doesn’t use any syntax which may result in applying the implicit copying constructor. It only uses the explicit constructor, which gets one parameter of type int.
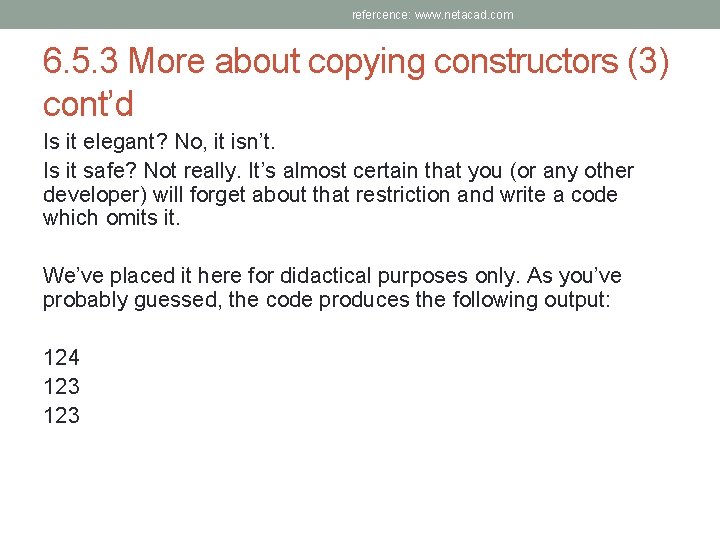
refercence: www. netacad. com 6. 5. 3 More about copying constructors (3) cont’d Is it elegant? No, it isn’t. Is it safe? Not really. It’s almost certain that you (or any other developer) will forget about that restriction and write a code which omits it. We’ve placed it here for didactical purposes only. As you’ve probably guessed, the code produces the following output: 124 123
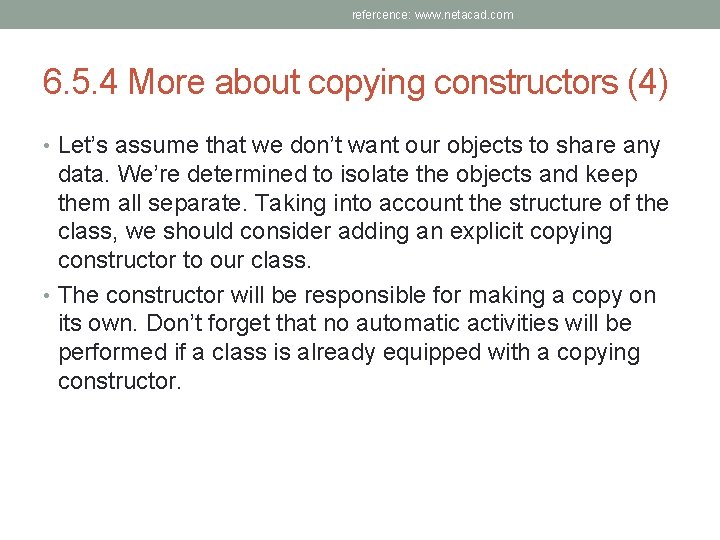
refercence: www. netacad. com 6. 5. 4 More about copying constructors (4) • Let’s assume that we don’t want our objects to share any data. We’re determined to isolate the objects and keep them all separate. Taking into account the structure of the class, we should consider adding an explicit copying constructor to our class. • The constructor will be responsible for making a copy on its own. Don’t forget that no automatic activities will be performed if a class is already equipped with a copying constructor.
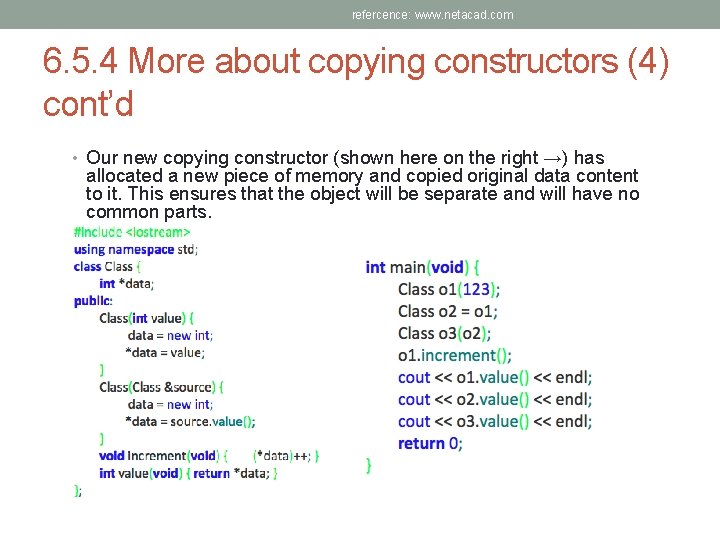
refercence: www. netacad. com 6. 5. 4 More about copying constructors (4) cont’d • Our new copying constructor (shown here on the right →) has allocated a new piece of memory and copied original data content to it. This ensures that the object will be separate and will have no common parts.
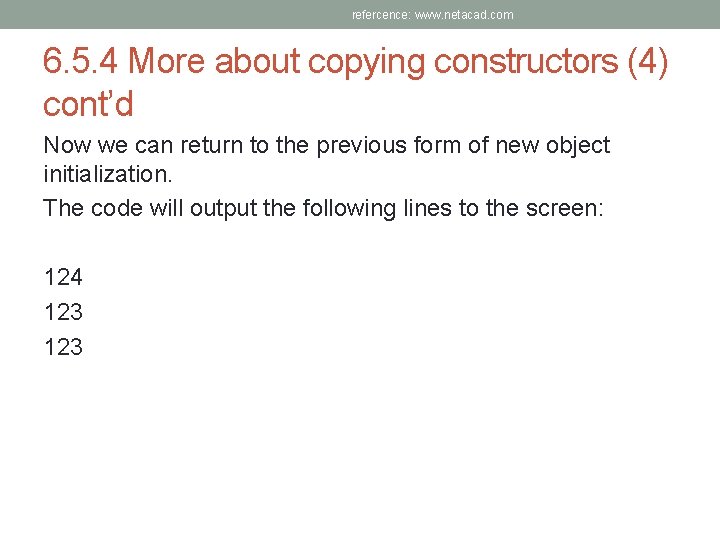
refercence: www. netacad. com 6. 5. 4 More about copying constructors (4) cont’d Now we can return to the previous form of new object initialization. The code will output the following lines to the screen: 124 123
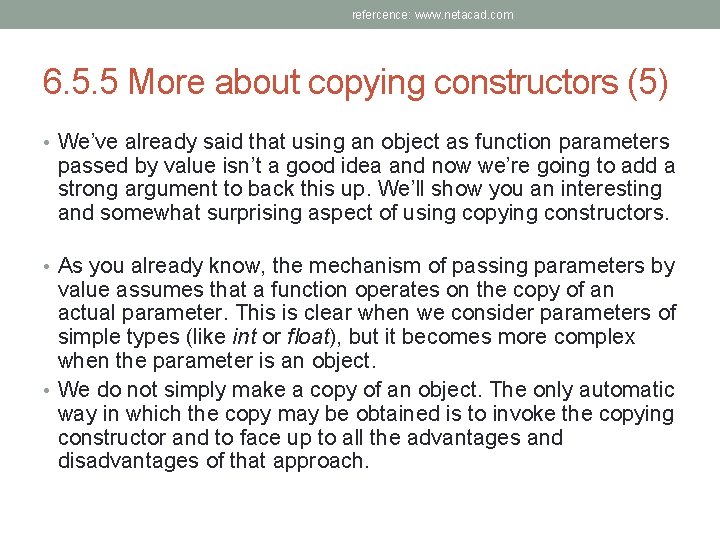
refercence: www. netacad. com 6. 5. 5 More about copying constructors (5) • We’ve already said that using an object as function parameters passed by value isn’t a good idea and now we’re going to add a strong argument to back this up. We’ll show you an interesting and somewhat surprising aspect of using copying constructors. • As you already know, the mechanism of passing parameters by value assumes that a function operates on the copy of an actual parameter. This is clear when we consider parameters of simple types (like int or float), but it becomes more complex when the parameter is an object. • We do not simply make a copy of an object. The only automatic way in which the copy may be obtained is to invoke the copying constructor and to face up to all the advantages and disadvantages of that approach.
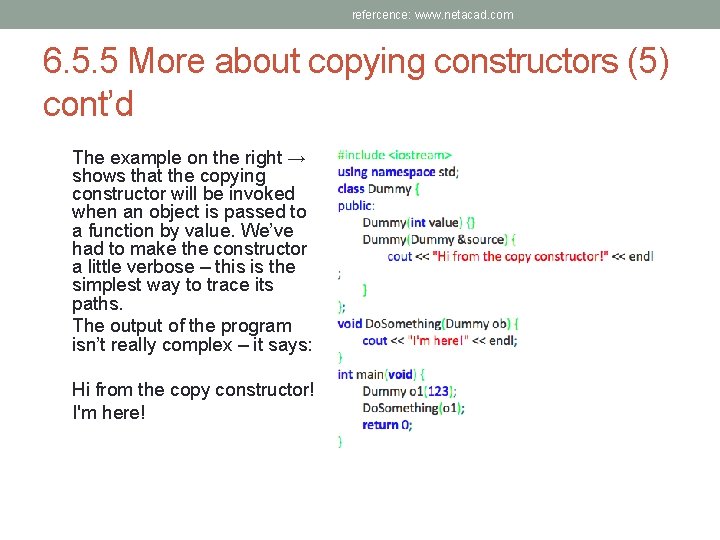
refercence: www. netacad. com 6. 5. 5 More about copying constructors (5) cont’d The example on the right → shows that the copying constructor will be invoked when an object is passed to a function by value. We’ve had to make the constructor a little verbose – this is the simplest way to trace its paths. The output of the program isn’t really complex – it says: Hi from the copy constructor! I'm here!
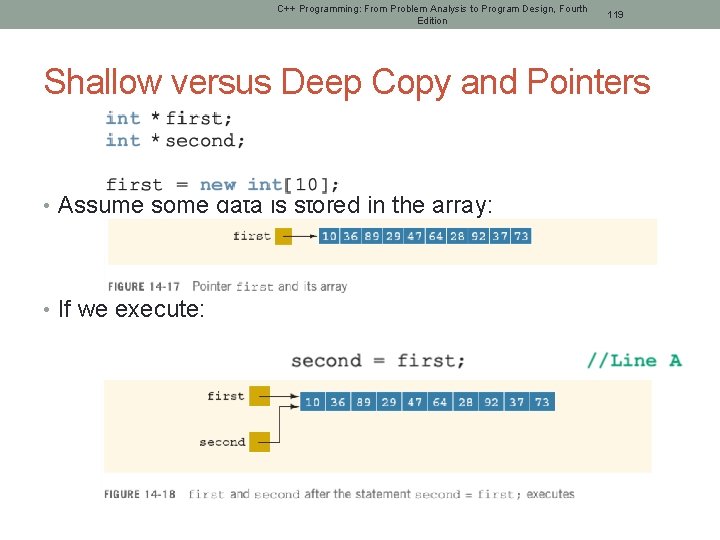
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 119 Shallow versus Deep Copy and Pointers • Assume some data is stored in the array: • If we execute:
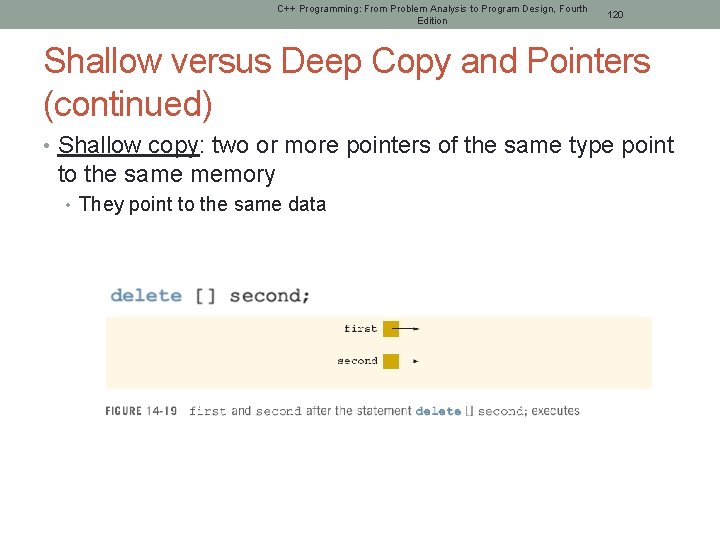
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 120 Shallow versus Deep Copy and Pointers (continued) • Shallow copy: two or more pointers of the same type point to the same memory • They point to the same data
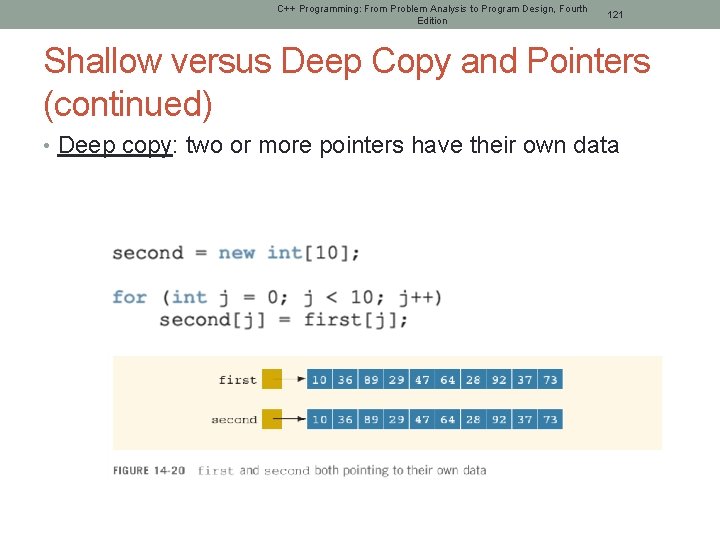
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 121 Shallow versus Deep Copy and Pointers (continued) • Deep copy: two or more pointers have their own data
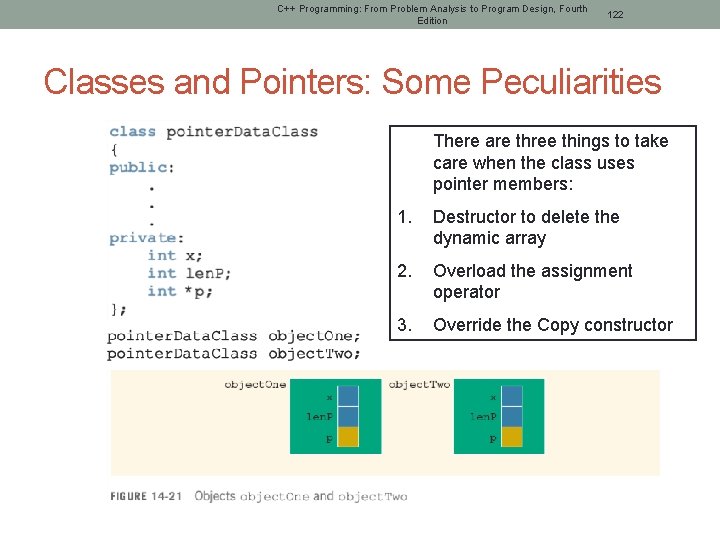
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 122 Classes and Pointers: Some Peculiarities There are three things to take care when the class uses pointer members: 1. Destructor to delete the dynamic array 2. Overload the assignment operator 3. Override the Copy constructor
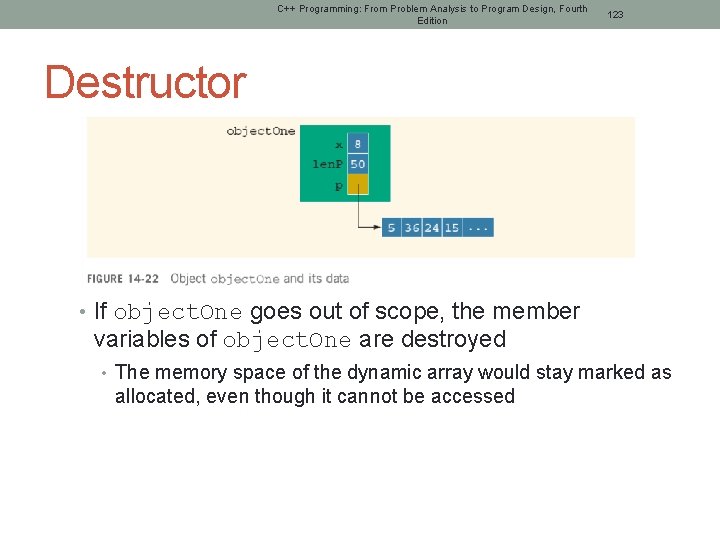
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 123 Destructor • If object. One goes out of scope, the member variables of object. One are destroyed • The memory space of the dynamic array would stay marked as allocated, even though it cannot be accessed
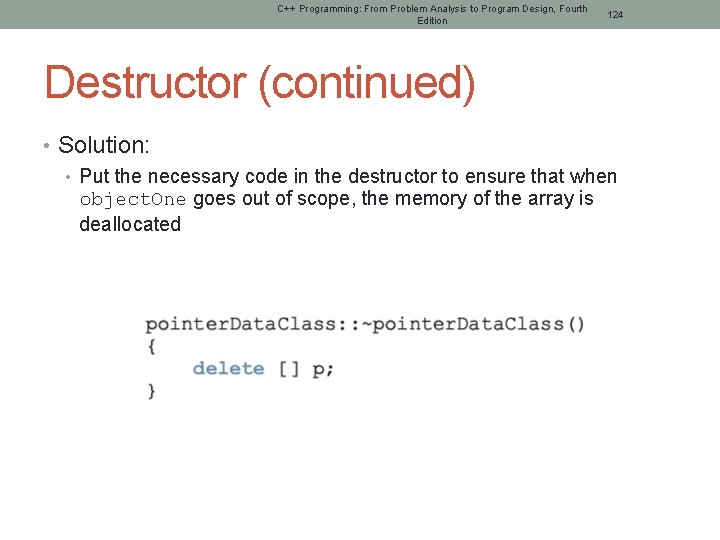
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 124 Destructor (continued) • Solution: • Put the necessary code in the destructor to ensure that when object. One goes out of scope, the memory of the array is deallocated
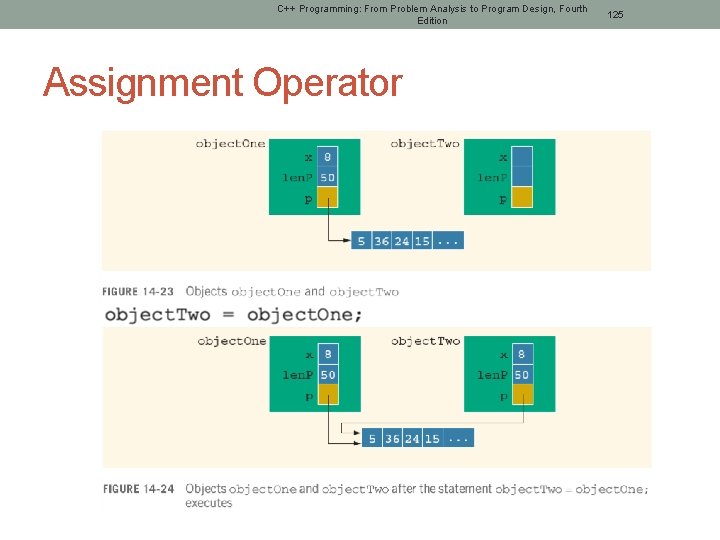
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Assignment Operator 125
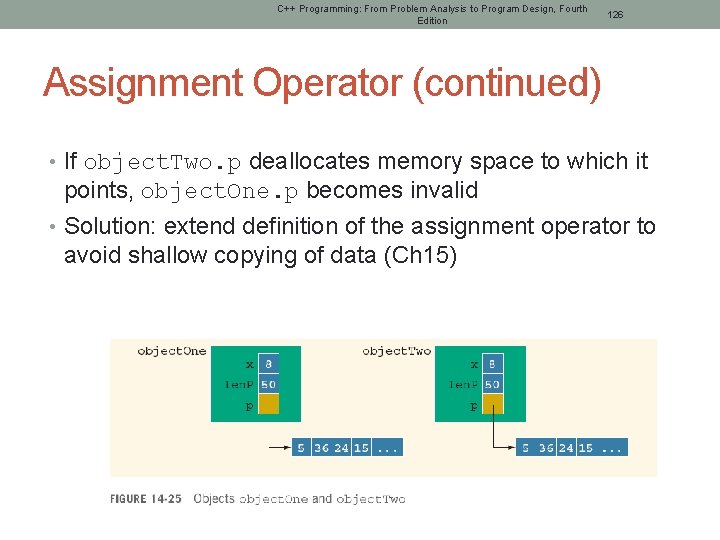
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 126 Assignment Operator (continued) • If object. Two. p deallocates memory space to which it points, object. One. p becomes invalid • Solution: extend definition of the assignment operator to avoid shallow copying of data (Ch 15)
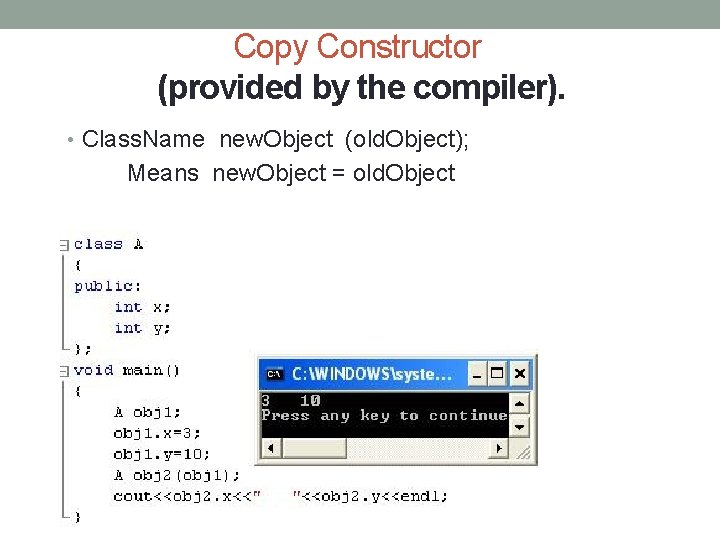
Copy Constructor (provided by the compiler). • Class. Name new. Object (old. Object); Means new. Object = old. Object
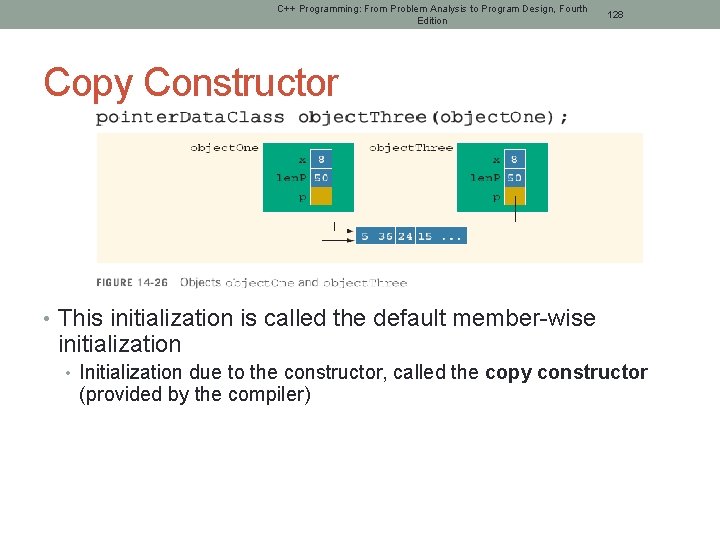
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 128 Copy Constructor • This initialization is called the default member-wise initialization • Initialization due to the constructor, called the copy constructor (provided by the compiler)
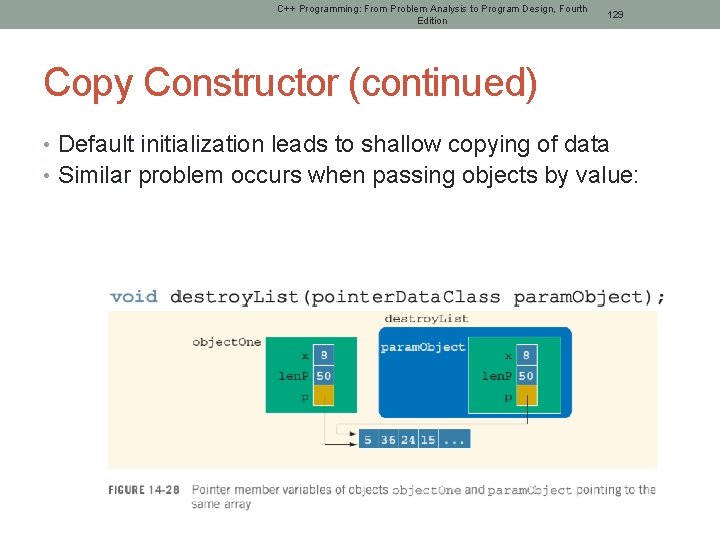
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 129 Copy Constructor (continued) • Default initialization leads to shallow copying of data • Similar problem occurs when passing objects by value:
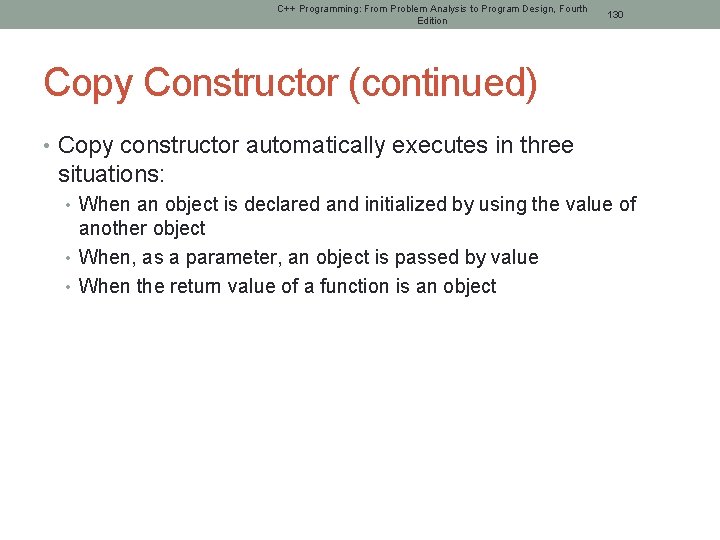
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 130 Copy Constructor (continued) • Copy constructor automatically executes in three situations: • When an object is declared and initialized by using the value of another object • When, as a parameter, an object is passed by value • When the return value of a function is an object
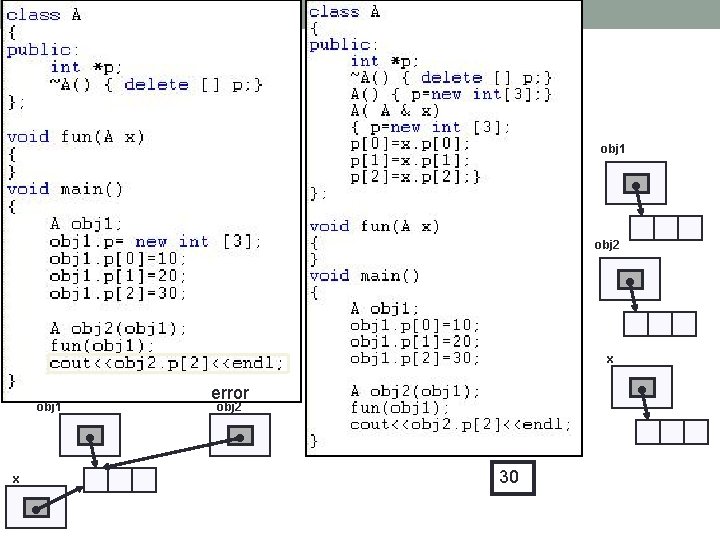
obj 1 obj 2 x obj 1 x error obj 2 30
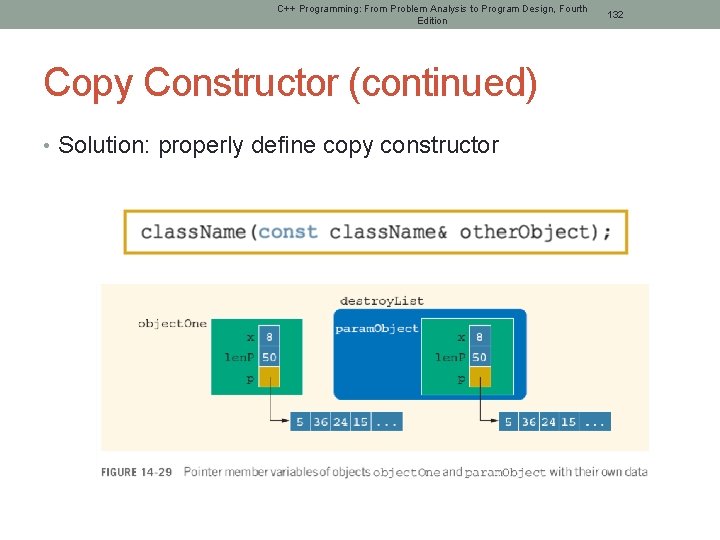
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Copy Constructor (continued) • Solution: properly define copy constructor 132
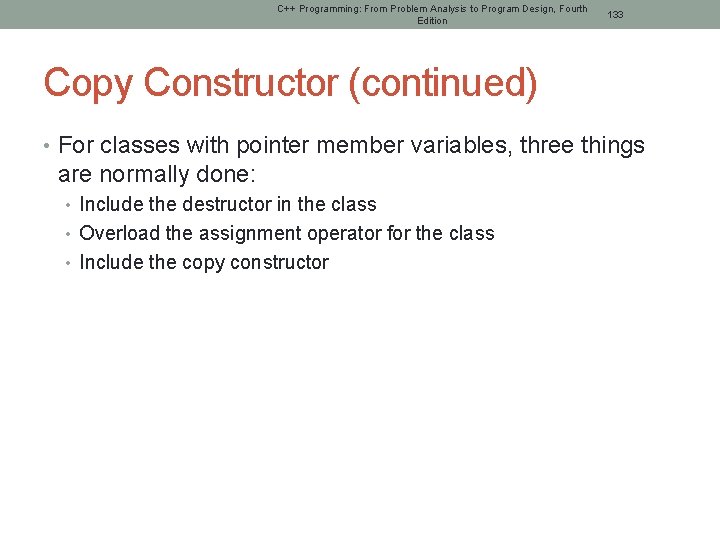
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 133 Copy Constructor (continued) • For classes with pointer member variables, three things are normally done: • Include the destructor in the class • Overload the assignment operator for the class • Include the copy constructor
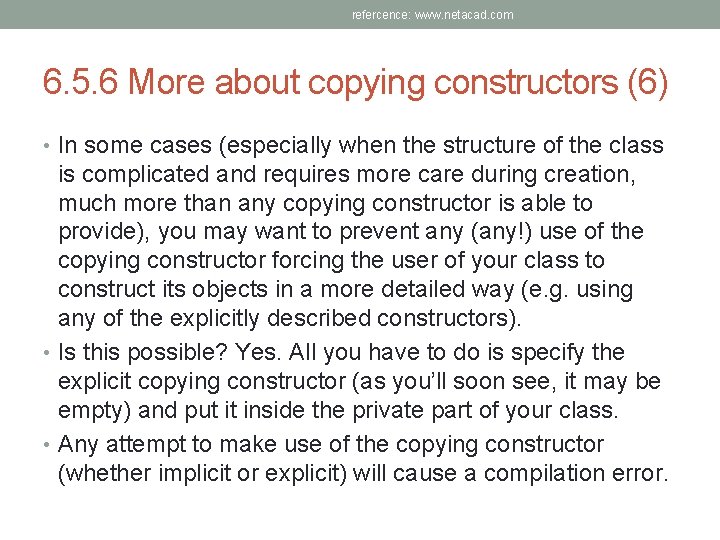
refercence: www. netacad. com 6. 5. 6 More about copying constructors (6) • In some cases (especially when the structure of the class is complicated and requires more care during creation, much more than any copying constructor is able to provide), you may want to prevent any (any!) use of the copying constructor forcing the user of your class to construct its objects in a more detailed way (e. g. using any of the explicitly described constructors). • Is this possible? Yes. All you have to do is specify the explicit copying constructor (as you’ll soon see, it may be empty) and put it inside the private part of your class. • Any attempt to make use of the copying constructor (whether implicit or explicit) will cause a compilation error.
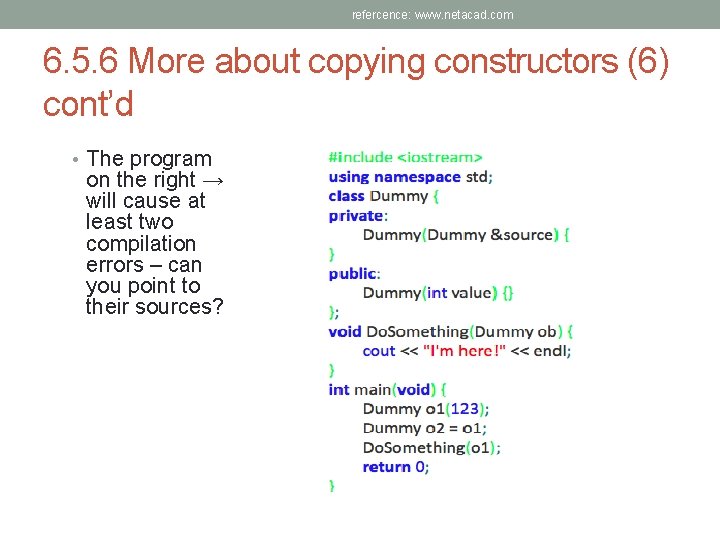
refercence: www. netacad. com 6. 5. 6 More about copying constructors (6) cont’d • The program on the right → will cause at least two compilation errors – can you point to their sources?
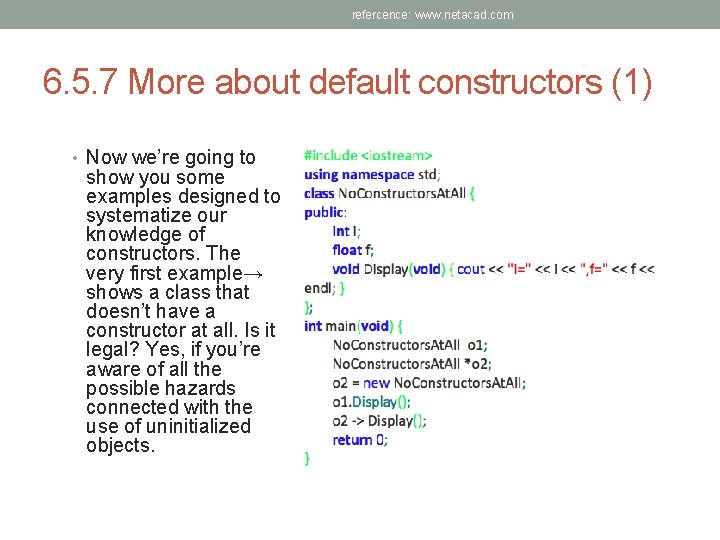
refercence: www. netacad. com 6. 5. 7 More about default constructors (1) • Now we’re going to show you some examples designed to systematize our knowledge of constructors. The very first example→ shows a class that doesn’t have a constructor at all. Is it legal? Yes, if you’re aware of all the possible hazards connected with the use of uninitialized objects.
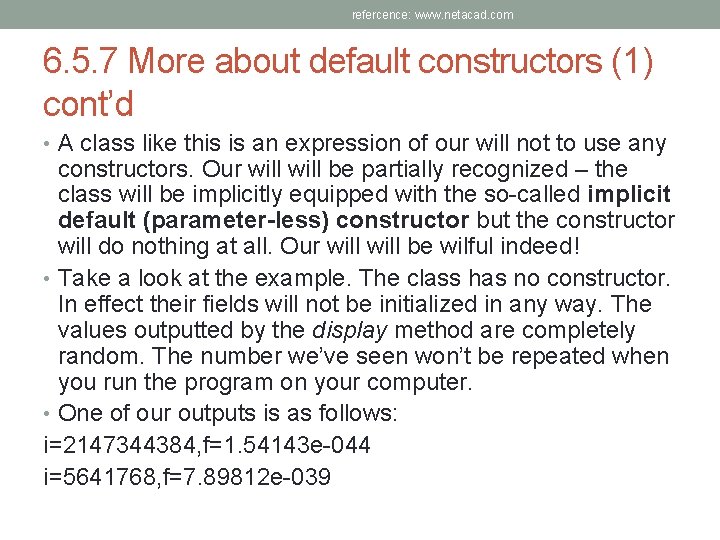
refercence: www. netacad. com 6. 5. 7 More about default constructors (1) cont’d • A class like this is an expression of our will not to use any constructors. Our will be partially recognized – the class will be implicitly equipped with the so-called implicit default (parameter-less) constructor but the constructor will do nothing at all. Our will be wilful indeed! • Take a look at the example. The class has no constructor. In effect their fields will not be initialized in any way. The values outputted by the display method are completely random. The number we’ve seen won’t be repeated when you run the program on your computer. • One of our outputs is as follows: i=2147344384, f=1. 54143 e-044 i=5641768, f=7. 89812 e-039
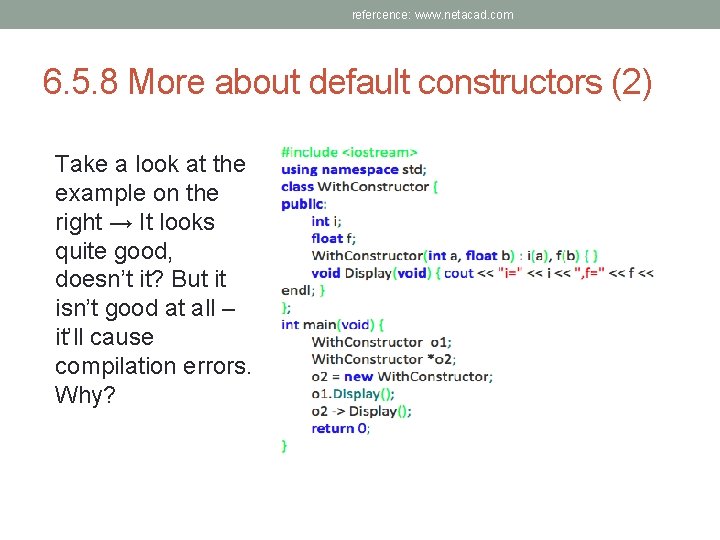
refercence: www. netacad. com 6. 5. 8 More about default constructors (2) Take a look at the example on the right → It looks quite good, doesn’t it? But it isn’t good at all – it’ll cause compilation errors. Why?
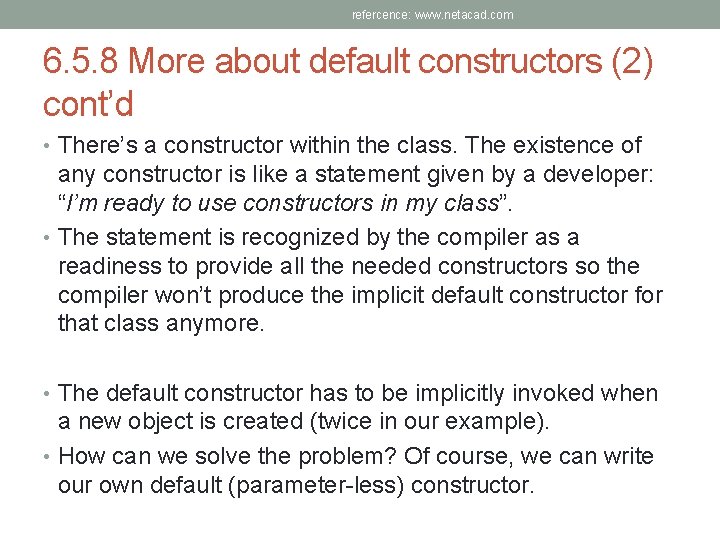
refercence: www. netacad. com 6. 5. 8 More about default constructors (2) cont’d • There’s a constructor within the class. The existence of any constructor is like a statement given by a developer: “I’m ready to use constructors in my class”. • The statement is recognized by the compiler as a readiness to provide all the needed constructors so the compiler won’t produce the implicit default constructor for that class anymore. • The default constructor has to be implicitly invoked when a new object is created (twice in our example). • How can we solve the problem? Of course, we can write our own default (parameter-less) constructor.
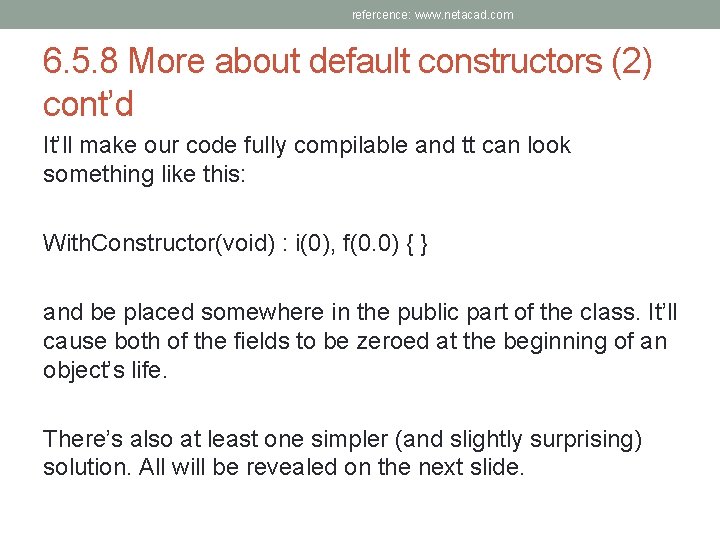
refercence: www. netacad. com 6. 5. 8 More about default constructors (2) cont’d It’ll make our code fully compilable and tt can look something like this: With. Constructor(void) : i(0), f(0. 0) { } and be placed somewhere in the public part of the class. It’ll cause both of the fields to be zeroed at the beginning of an object’s life. There’s also at least one simpler (and slightly surprising) solution. All will be revealed on the next slide.
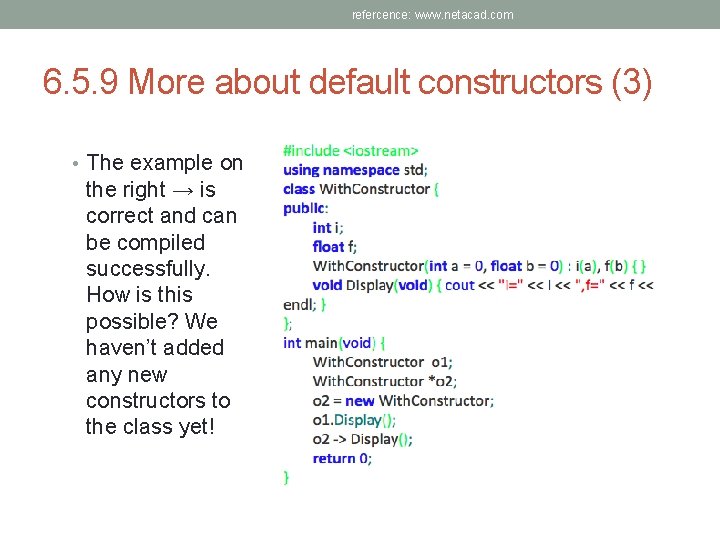
refercence: www. netacad. com 6. 5. 9 More about default constructors (3) • The example on the right → is correct and can be compiled successfully. How is this possible? We haven’t added any new constructors to the class yet!
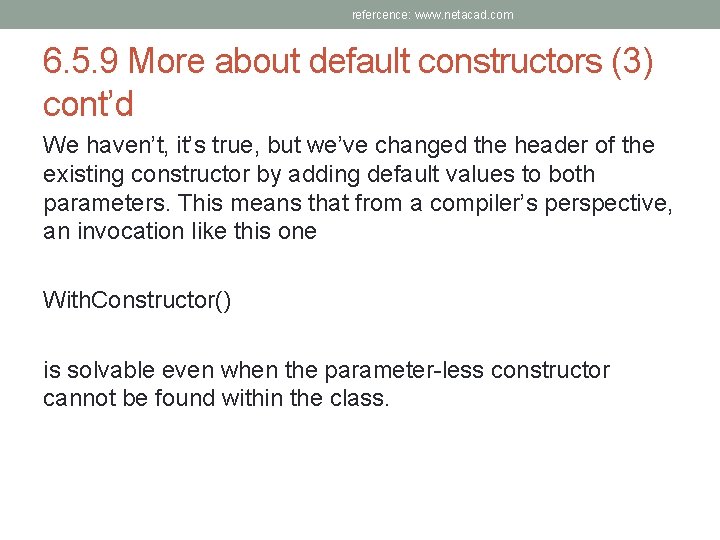
refercence: www. netacad. com 6. 5. 9 More about default constructors (3) cont’d We haven’t, it’s true, but we’ve changed the header of the existing constructor by adding default values to both parameters. This means that from a compiler’s perspective, an invocation like this one With. Constructor() is solvable even when the parameter-less constructor cannot be found within the class.
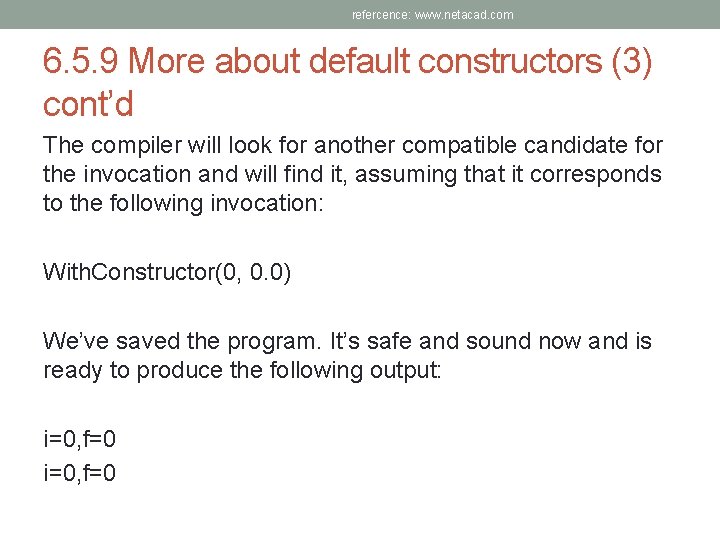
refercence: www. netacad. com 6. 5. 9 More about default constructors (3) cont’d The compiler will look for another compatible candidate for the invocation and will find it, assuming that it corresponds to the following invocation: With. Constructor(0, 0. 0) We’ve saved the program. It’s safe and sound now and is ready to produce the following output: i=0, f=0
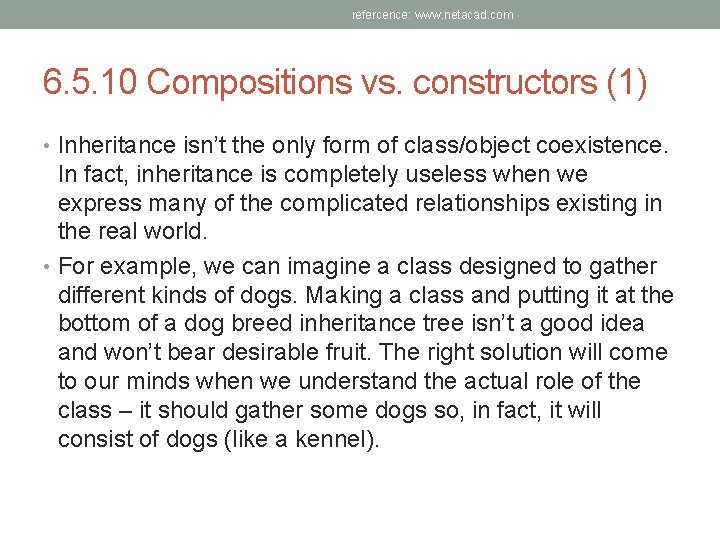
refercence: www. netacad. com 6. 5. 10 Compositions vs. constructors (1) • Inheritance isn’t the only form of class/object coexistence. In fact, inheritance is completely useless when we express many of the complicated relationships existing in the real world. • For example, we can imagine a class designed to gather different kinds of dogs. Making a class and putting it at the bottom of a dog breed inheritance tree isn’t a good idea and won’t bear desirable fruit. The right solution will come to our minds when we understand the actual role of the class – it should gather some dogs so, in fact, it will consist of dogs (like a kennel).
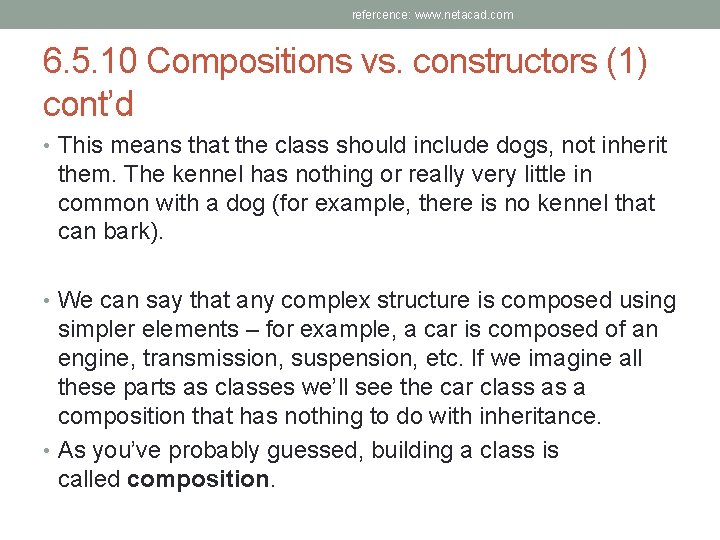
refercence: www. netacad. com 6. 5. 10 Compositions vs. constructors (1) cont’d • This means that the class should include dogs, not inherit them. The kennel has nothing or really very little in common with a dog (for example, there is no kennel that can bark). • We can say that any complex structure is composed using simpler elements – for example, a car is composed of an engine, transmission, suspension, etc. If we imagine all these parts as classes we’ll see the car class as a composition that has nothing to do with inheritance. • As you’ve probably guessed, building a class is called composition.
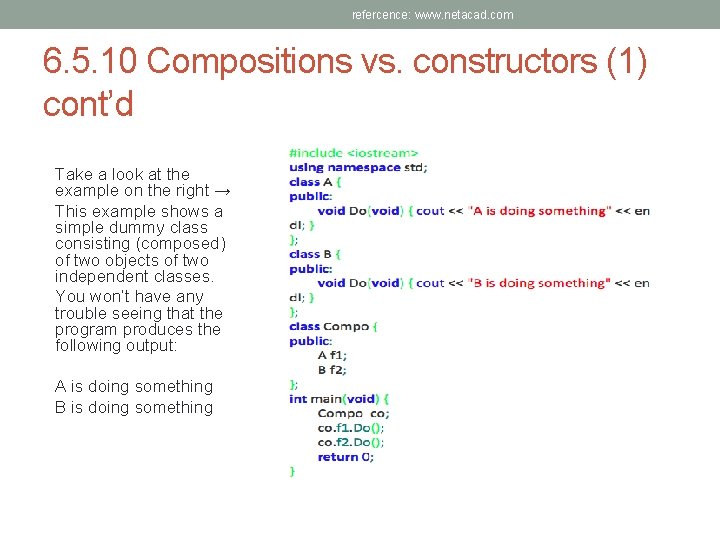
refercence: www. netacad. com 6. 5. 10 Compositions vs. constructors (1) cont’d Take a look at the example on the right → This example shows a simple dummy class consisting (composed) of two objects of two independent classes. You won’t have any trouble seeing that the program produces the following output: A is doing something B is doing something
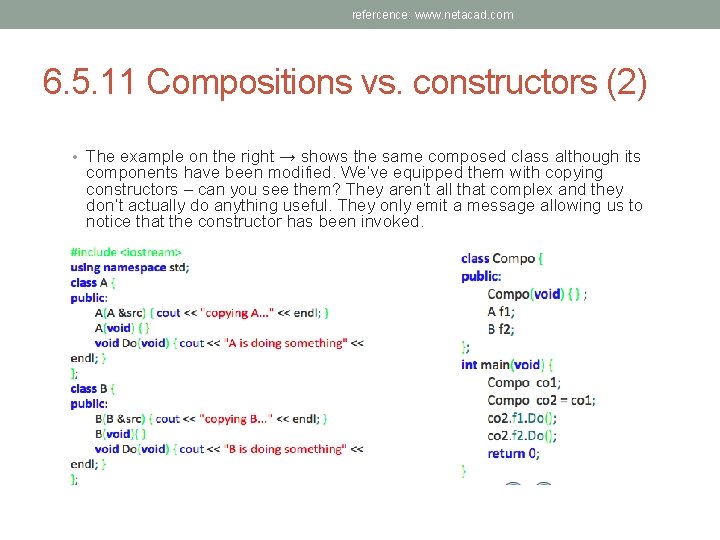
refercence: www. netacad. com 6. 5. 11 Compositions vs. constructors (2) • The example on the right → shows the same composed class although its components have been modified. We’ve equipped them with copying constructors – can you see them? They aren’t all that complex and they don’t actually do anything useful. They only emit a message allowing us to notice that the constructor has been invoked.
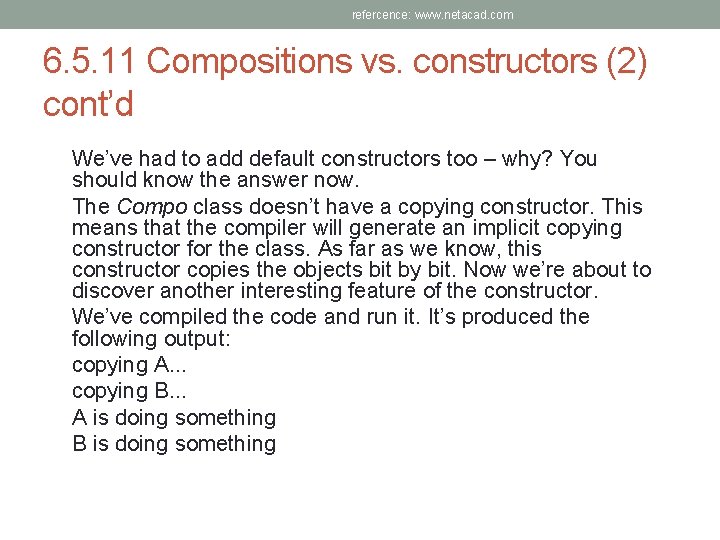
refercence: www. netacad. com 6. 5. 11 Compositions vs. constructors (2) cont’d We’ve had to add default constructors too – why? You should know the answer now. The Compo class doesn’t have a copying constructor. This means that the compiler will generate an implicit copying constructor for the class. As far as we know, this constructor copies the objects bit by bit. Now we’re about to discover another interesting feature of the constructor. We’ve compiled the code and run it. It’s produced the following output: copying A. . . copying B. . . A is doing something B is doing something
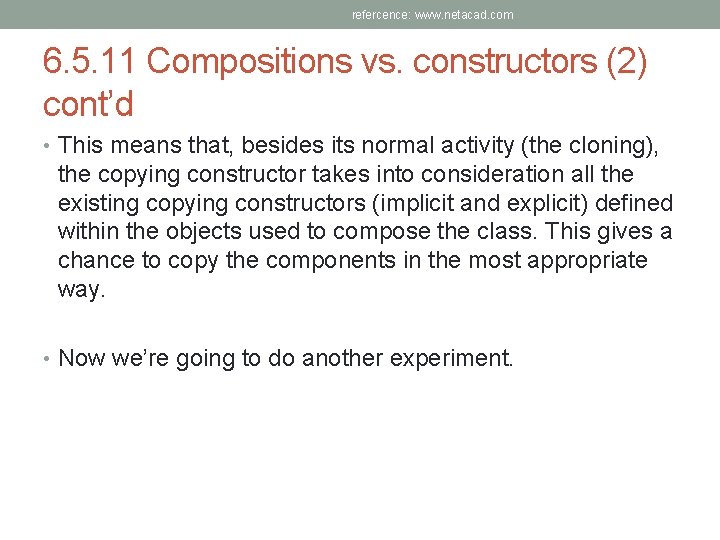
refercence: www. netacad. com 6. 5. 11 Compositions vs. constructors (2) cont’d • This means that, besides its normal activity (the cloning), the copying constructor takes into consideration all the existing copying constructors (implicit and explicit) defined within the objects used to compose the class. This gives a chance to copy the components in the most appropriate way. • Now we’re going to do another experiment.
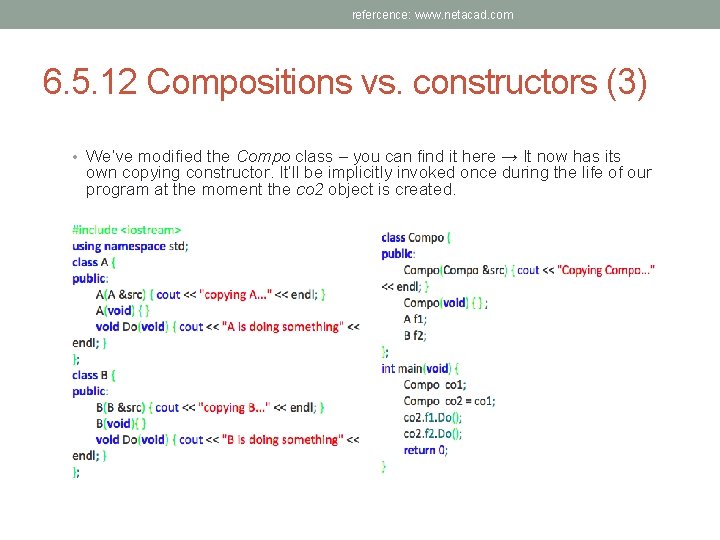
refercence: www. netacad. com 6. 5. 12 Compositions vs. constructors (3) • We’ve modified the Compo class – you can find it here → It now has its own copying constructor. It’ll be implicitly invoked once during the life of our program at the moment the co 2 object is created.
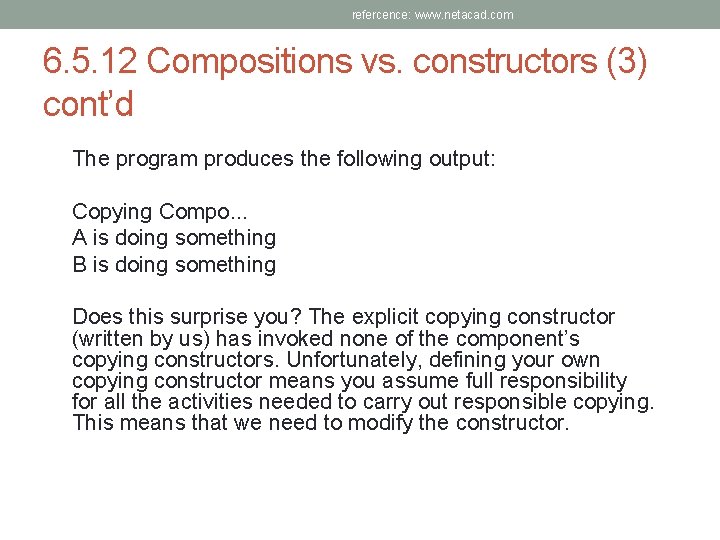
refercence: www. netacad. com 6. 5. 12 Compositions vs. constructors (3) cont’d The program produces the following output: Copying Compo. . . A is doing something B is doing something Does this surprise you? The explicit copying constructor (written by us) has invoked none of the component’s copying constructors. Unfortunately, defining your own copying constructor means you assume full responsibility for all the activities needed to carry out responsible copying. This means that we need to modify the constructor.
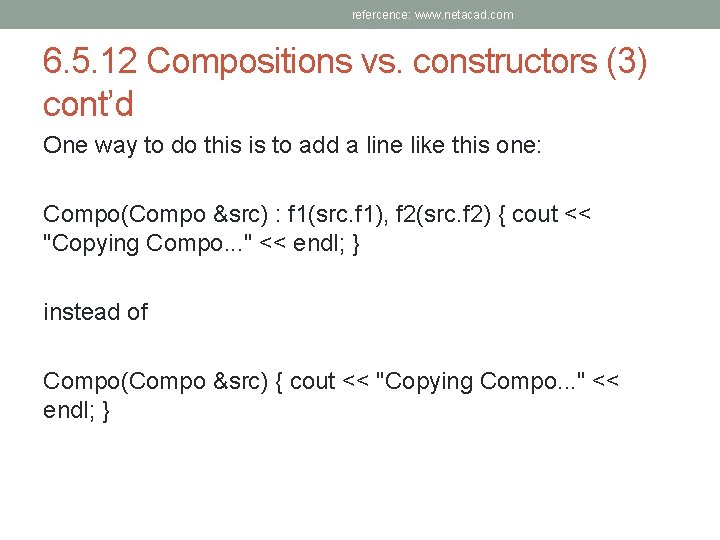
refercence: www. netacad. com 6. 5. 12 Compositions vs. constructors (3) cont’d One way to do this is to add a line like this one: Compo(Compo &src) : f 1(src. f 1), f 2(src. f 2) { cout << "Copying Compo. . . " << endl; } instead of Compo(Compo &src) { cout << "Copying Compo. . . " << endl; }
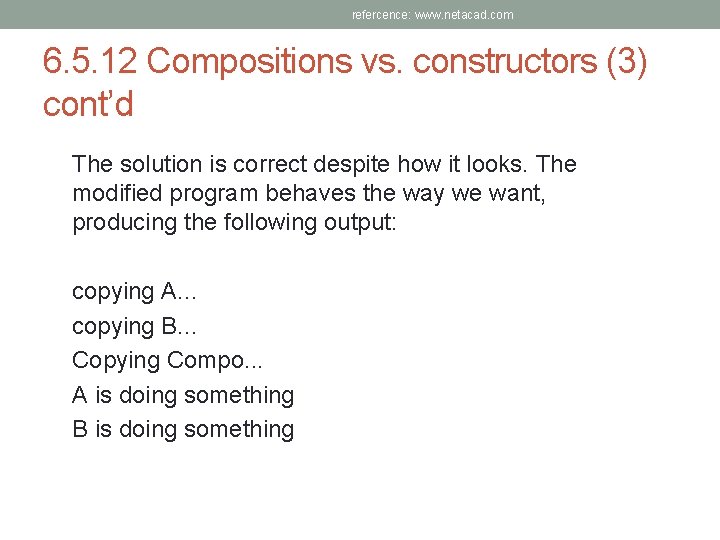
refercence: www. netacad. com 6. 5. 12 Compositions vs. constructors (3) cont’d The solution is correct despite how it looks. The modified program behaves the way we want, producing the following output: copying A. . . copying B. . . Copying Compo. . . A is doing something B is doing something
Netacad com
Bài thơ mẹ đi làm từ sáng sớm
Cơm
What is i d e
Netacad cisco
Cisco netacad
Netacad cisco
Netacad
Sweetcomb
Netacad
Section 2 complex patterns of inheritance
Chapter 16 the molecular basis of inheritance
Chromosomal theory of inheritance
Chapter 11 complex inheritance and human heredity test
A gene locus is
Chapter 16 the molecular basis of inheritance
Chapter 15: the chromosomal basis of inheritance
The chromosomal basis of inheritance chapter 15
Ap biology chapter 15
Chapter 11 section 1 basic patterns of human inheritance
Chapter 11 section 1 basic patterns of human inheritance
Chapter 13-the molecular basis of inheritance
Chapter 9 patterns of inheritance
Chapter 15 the chromosomal basis of inheritance
Prisma inheritance
Class and object diagram in uml
Priority inheritance
Inheritance scala
The inheritance of loss summary
Hydrop fetalis
Rails sti vs polymorphic
Haemophilia punnet square
Sexlinked inheritance
Inheritance of quantitative traits
Proverbs 13 niv
Tujuan inheritance
Apa yang dimaksud dengan inheritance
Pedigree symbols
Complete dominance pattern of inheritance
Oop virtual
Non nuclear inheritance
Non mendelian inheritance
Human inheritance modern genetics answer key
Modern genetics human inheritance answer key
Modern genetics human inheritance answer key
Epistatic inheritance
X linked dominant punnett square
Haemophilia
Perservefamily/status
Inheritance of quantitative traits
Private inheritance
Inheritance tax valencia region spain
Hierarchical inheritance in c++
Java inheritance
Advantages and disadvantages of inheritance in java
Private inheritance
Complete dominance pattern of inheritance
Inheritance of quantitative traits
Sean blaine
Mendelian principles
Use case inheritance
Characteristic of learners
Maternal effect and maternal inheritance
Section 12-1 chromosomes and inheritance
The pedigree below traces the inheritance of alkaptonuria
C++ advantages
Blood transfusion
Solidity multiple inheritance
What is an autosomal recessive trait
Encapsulation abstraction inheritance polymorphism
Inheritance.ilmsummit
Linked genes and unlinked genes
Jenis jenis inheritance
Y linked inheritance
Encapsulation inheritance and polymorphism
The pedigree below traces the inheritance of alkaptonuria
Cystic fibrosis mendelian inheritance
What is inheritance explain with example
Pengertian dari inheritance
Multifactorial inheritance.
Complex patterns of inheritance
Inheritance means
Inheritance board game
The holy spirit is a deposit guaranteeing our inheritance
Interface calculator public int add(int a int b) class test
Oop multiple inheritance
Probability laws govern mendelian inheritance
Bank account java program using inheritance
Single level inheritance is safer than
Contoh multilevel inheritance
Inheritance in java
Inheritance allows
Unity abstract class
Contoh program inheritance java
Encapsulation inheritance polymorphism
Composition vs inheritance vs aggregation
Inheritance
Inheritance presentation
Inheritance timeline
What did mendel conclude determines biological inheritance
Carl jung theory
Java inheritance lab exercise
Encapsulation inheritance polymorphism
Abstraction encapsulation inheritance polymorphism
Simple inheritance
Duchenne muscular dystrophy
Inheritance in c
Define inheritence
Polygenic inheritance
Multifactorial inheritance pedigree
Ppt molecular basis of inheritance
Inheritance
Traits
What is inheritance
Genticas
Is sickle cell anemia genetic
Sex linked punnett squares
Reproduction and inheritance
Qtl mapping
Mendelian pattern of inheritance
Family resemblance test
Mendel experiments in plant hybridization
Polygenic inheritance
Chromosome theory of inheritance
Mendel's first and second law of inheritance
What is the punnet square
Mr men inheritance
Hybrid vs purebred
What is inheritance
What is incomplete dominance give an example
Inheritance vs heritability
Harry potter and the inheritance of sex
Intermediate inheritance
Mosaicism
Inheritance
Polymophism
Incomplete dominance meaning
Hybrid inheritance
Mendel's experimental design
Inheritance in r
Inheritance definition computer science
The cellular basis of reproduction and inheritance
Inheritance logo
Trisomy male
Introduction to inheritance
Red tent summary
The great gatsby summary chapter 8
Chapter 10 chapter assessment chemical reactions answers
Stoichiometry chapter 11 study guide
Chapter 9 study guide chemical reactions
Similarity ratio definition
Chapter 6: career readiness
7 ionic and metallic bonding
Chapter 9 surface water chapter assessment answer key