Topics 14 1 What Is Inheritance 14 2
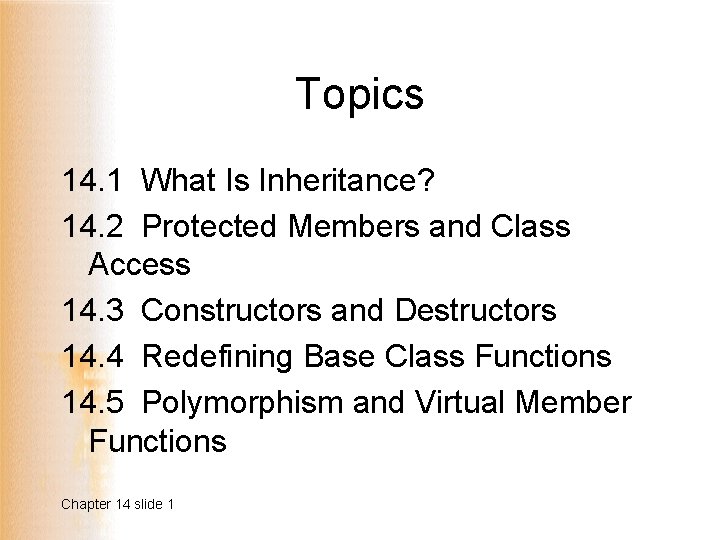
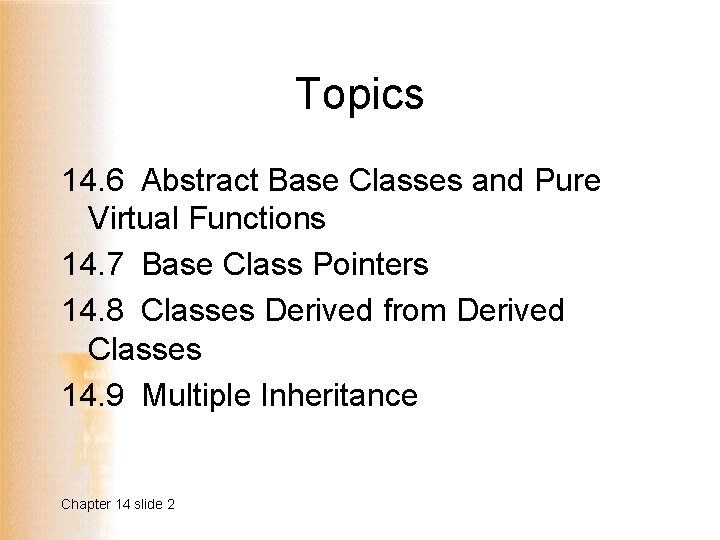
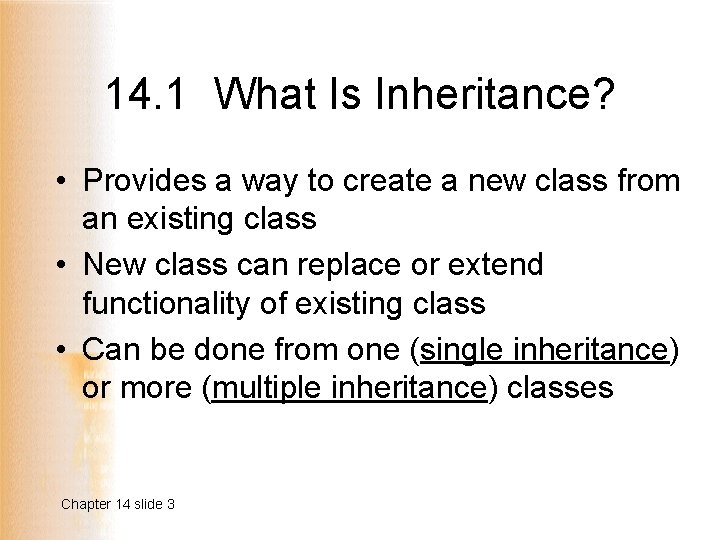
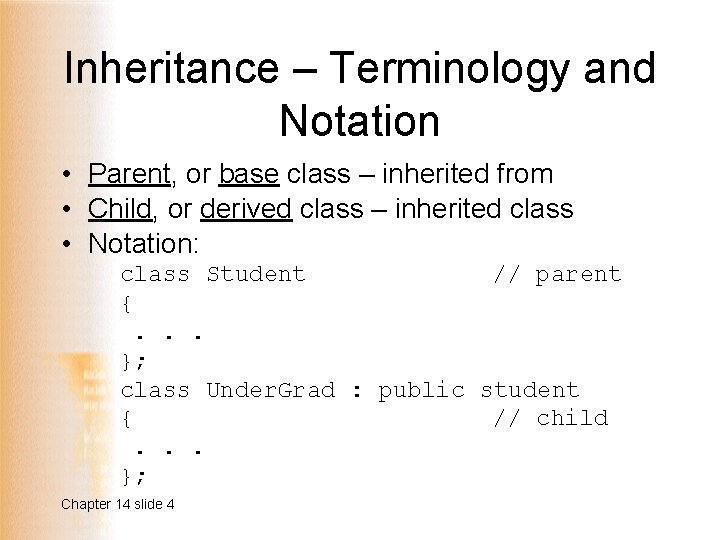
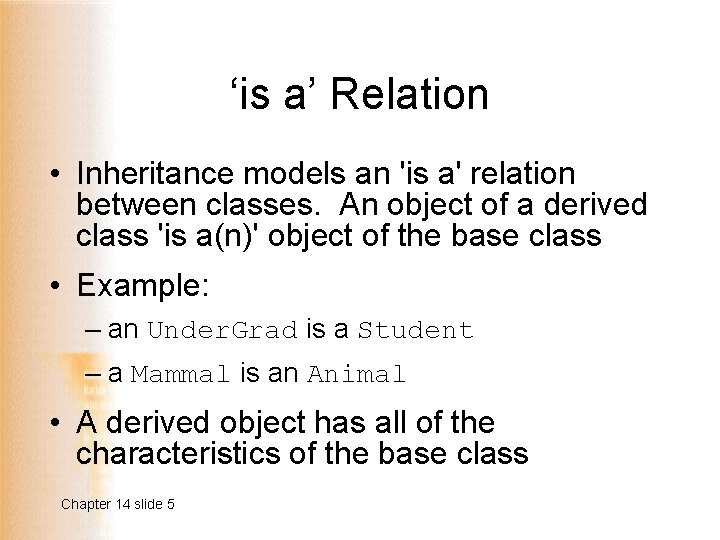
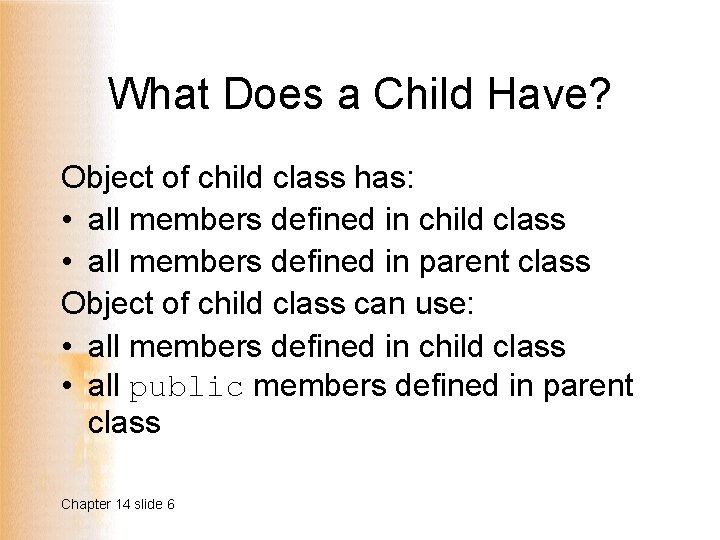
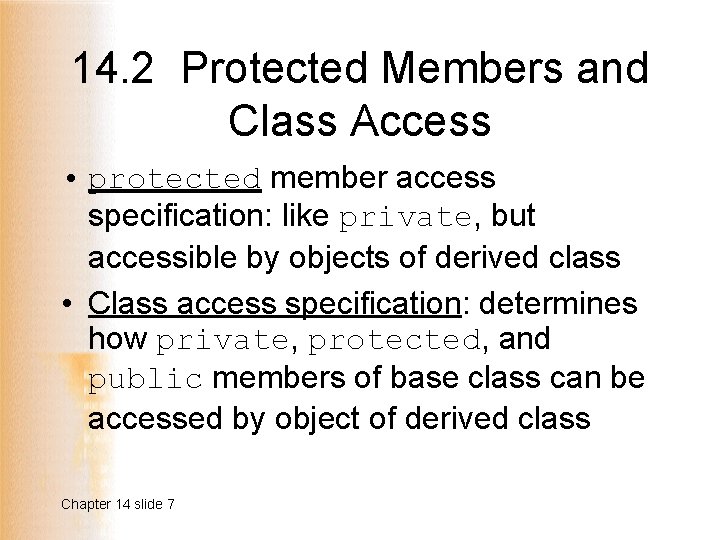
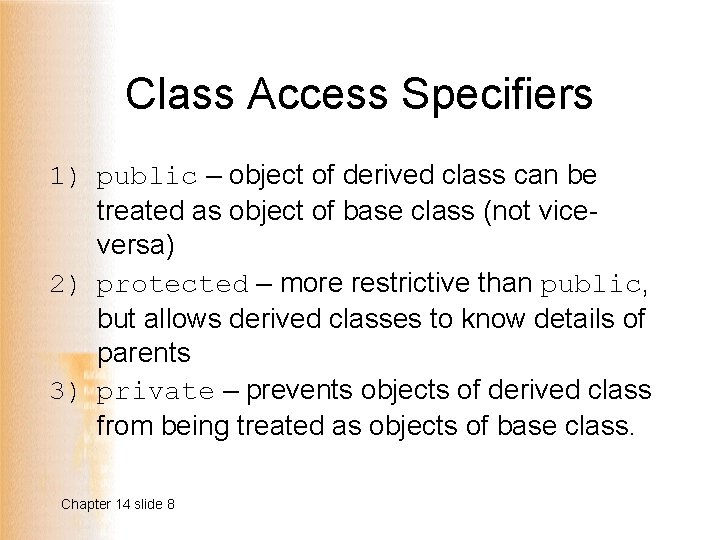
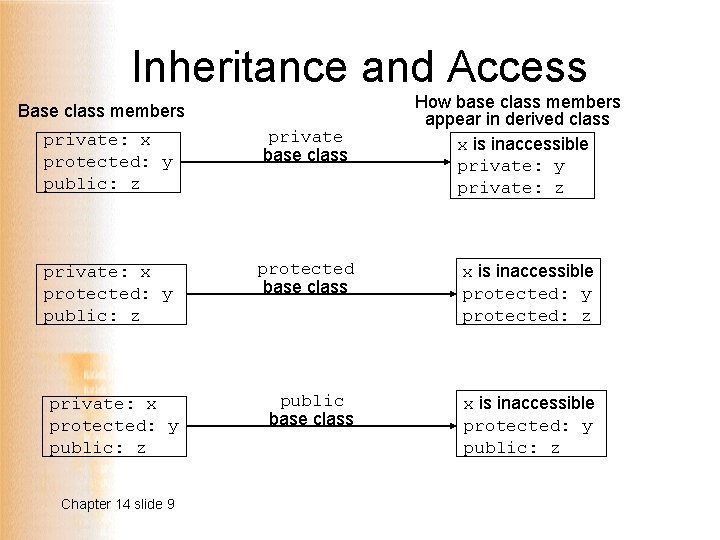
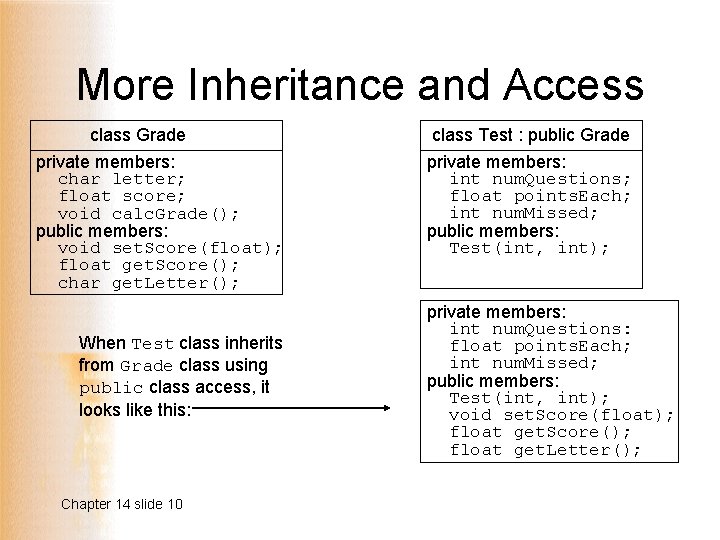
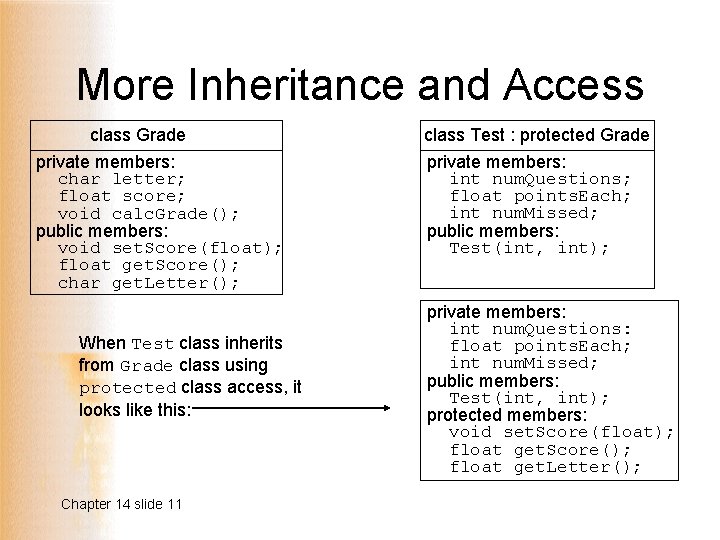
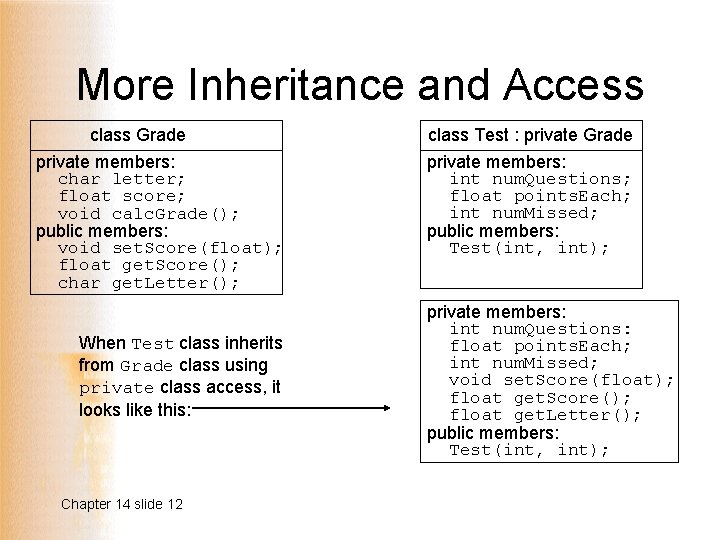
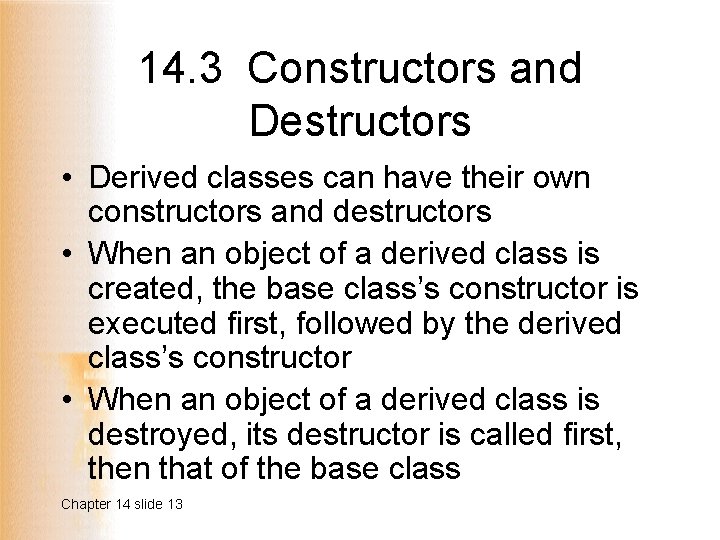
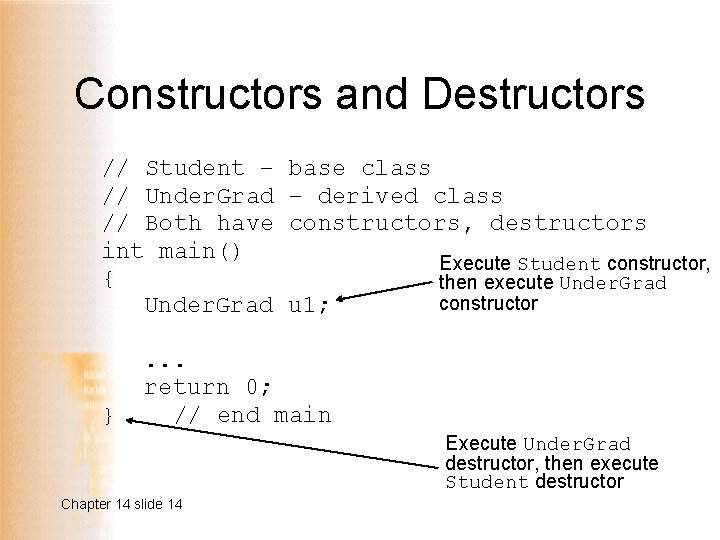
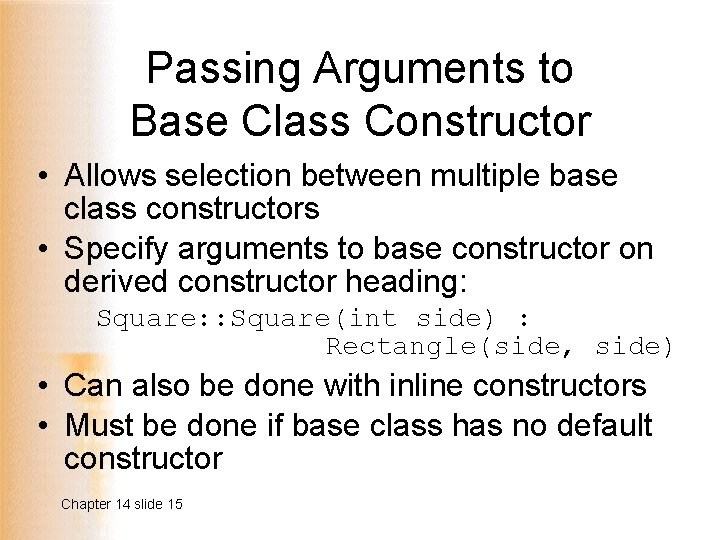
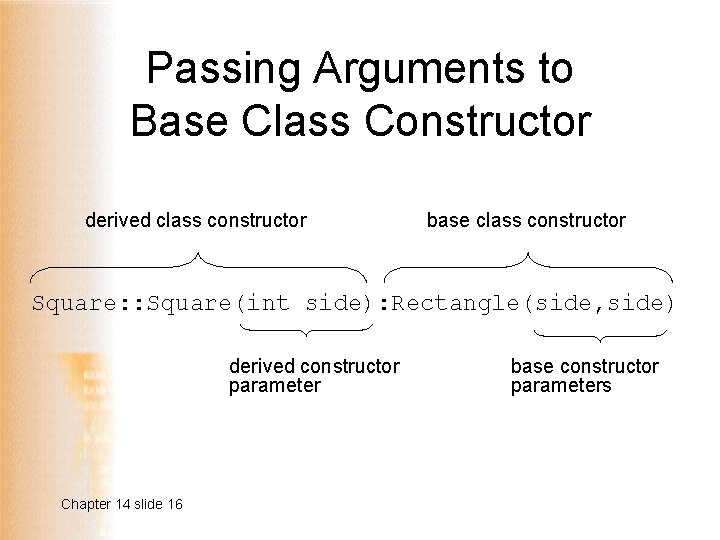
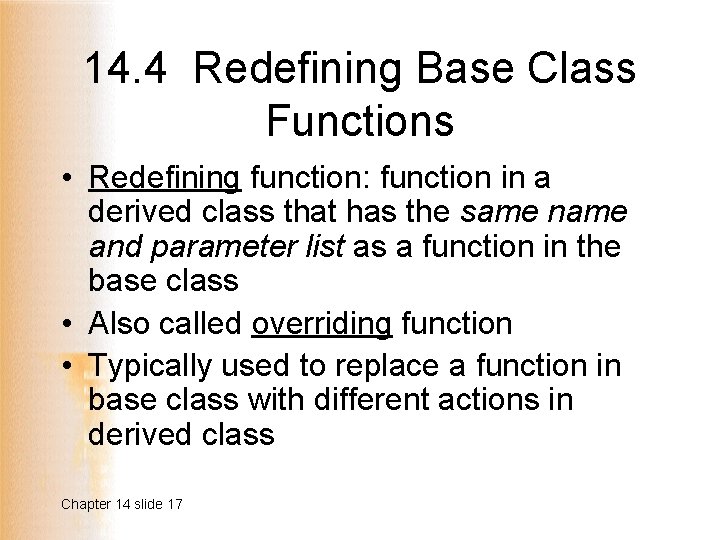
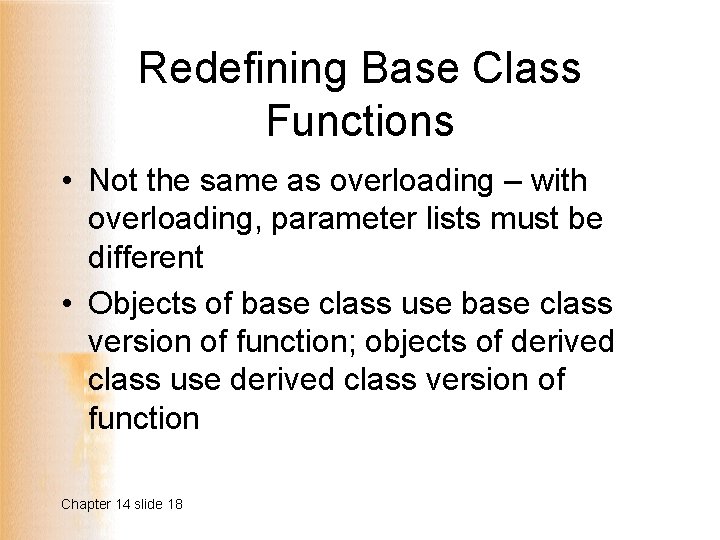
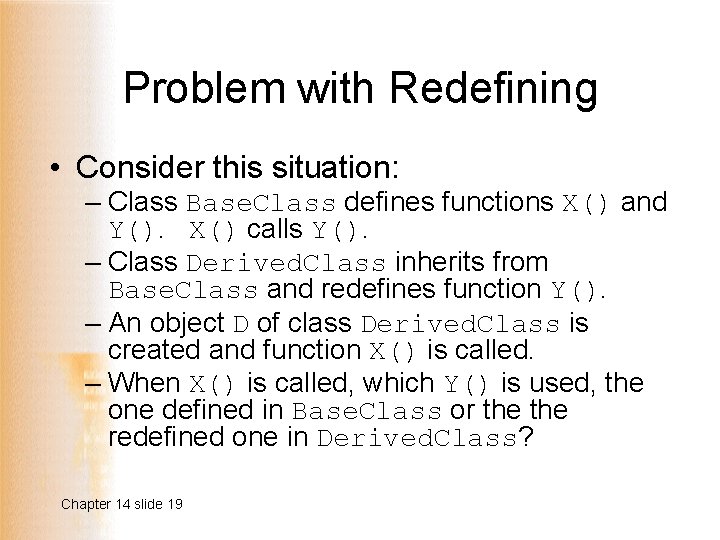
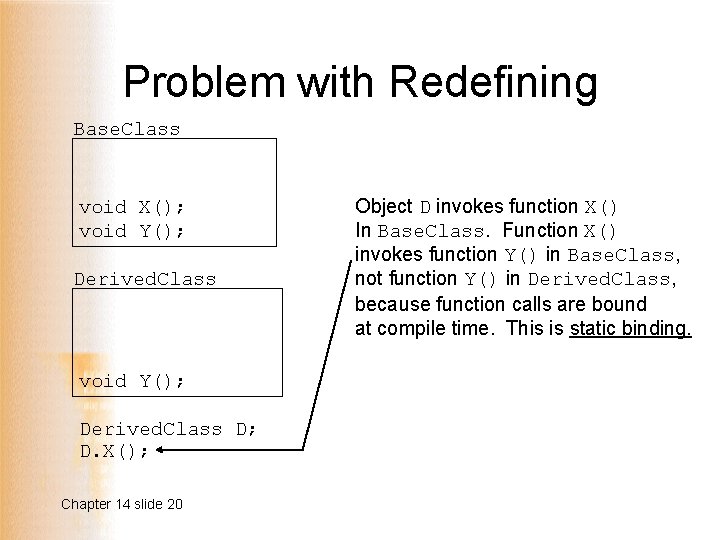
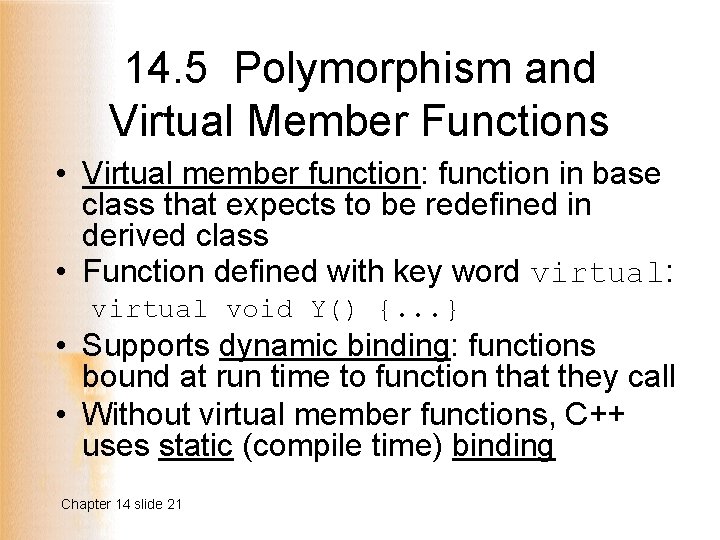
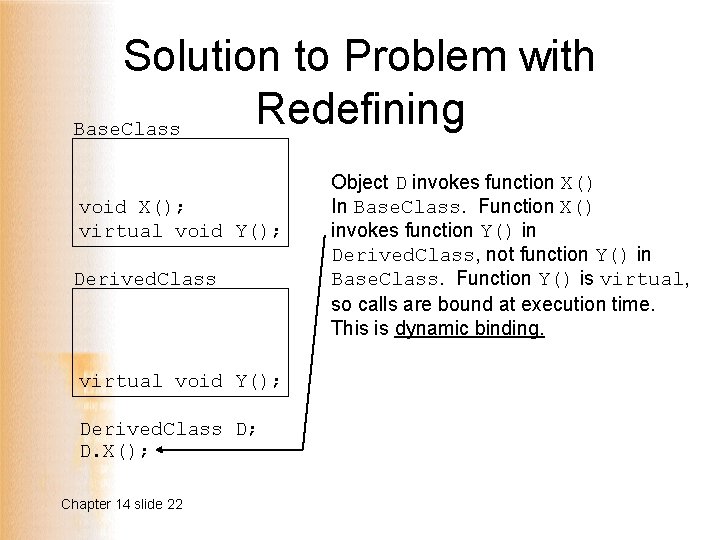
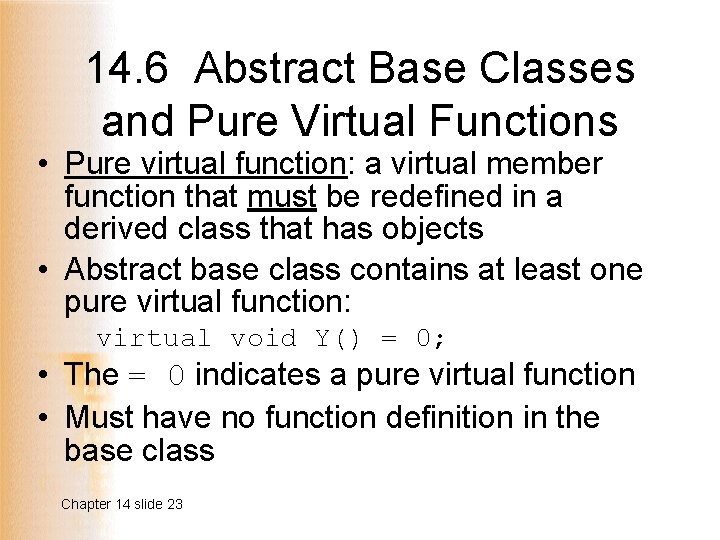
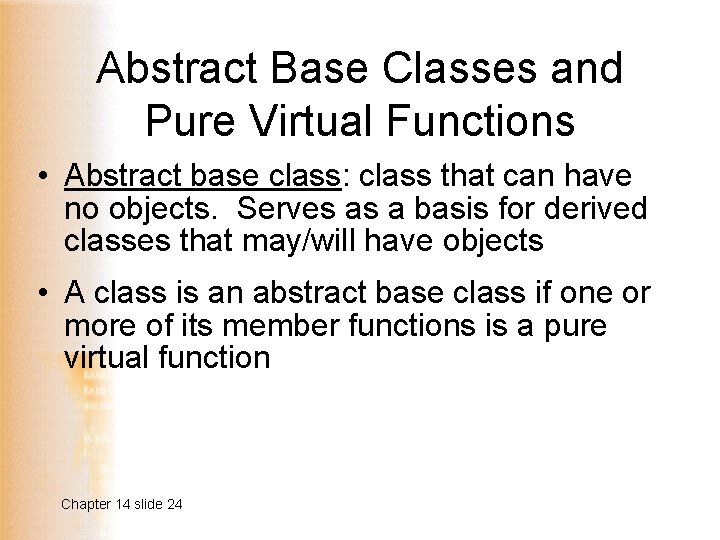
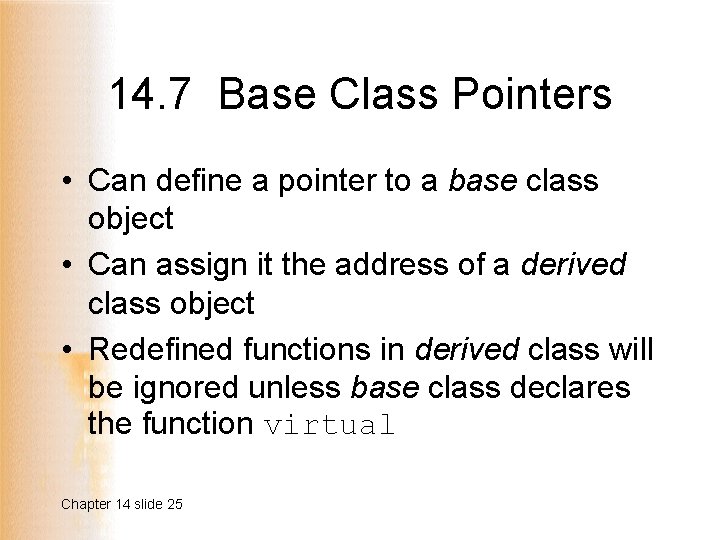
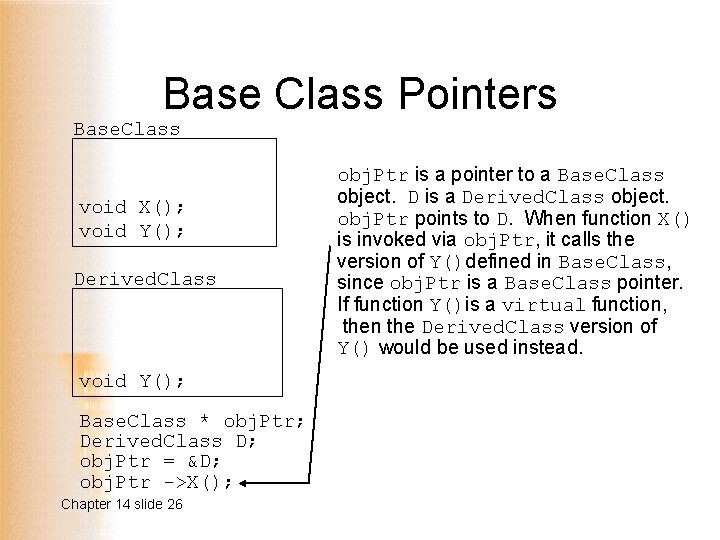
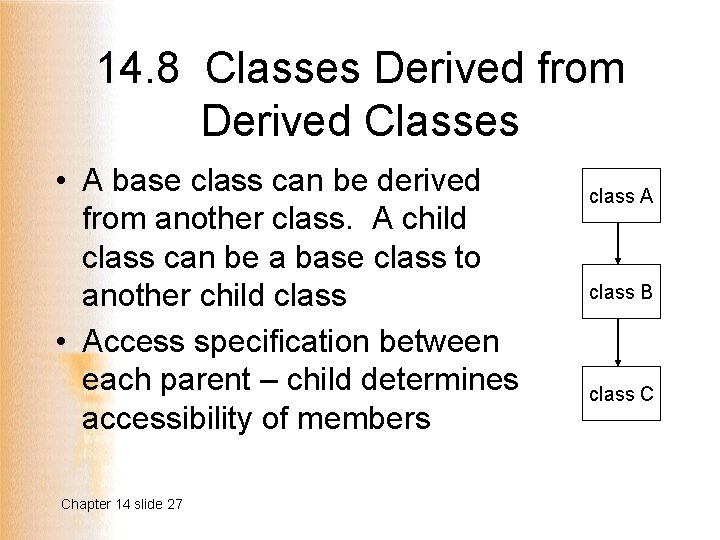
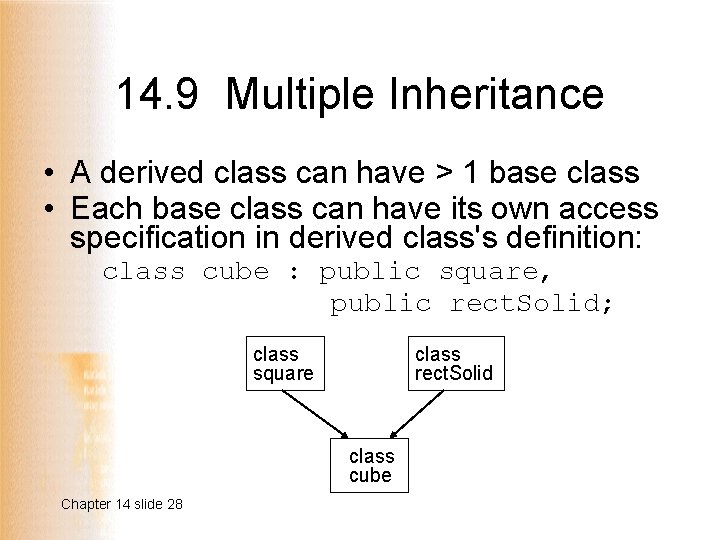
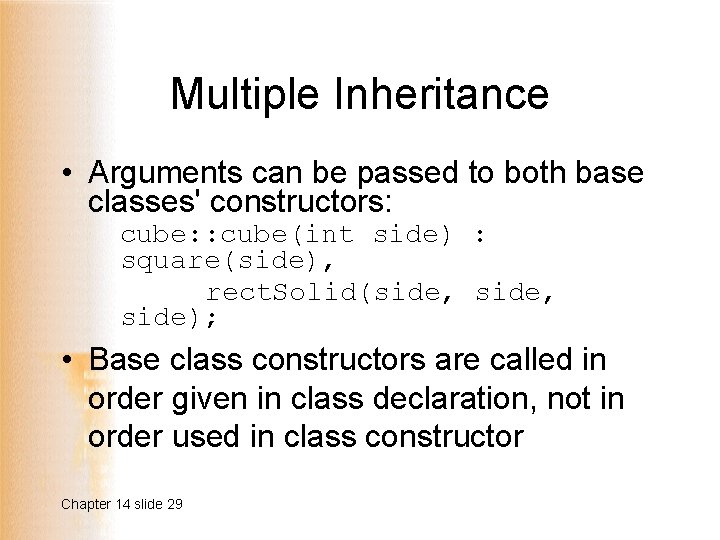
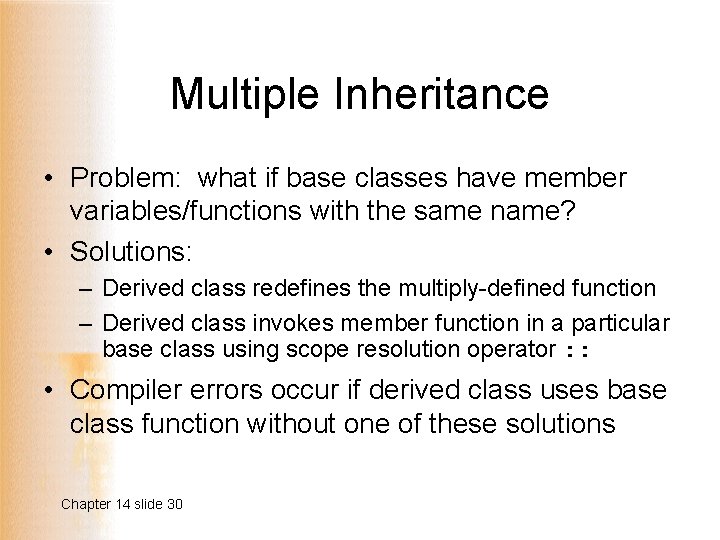
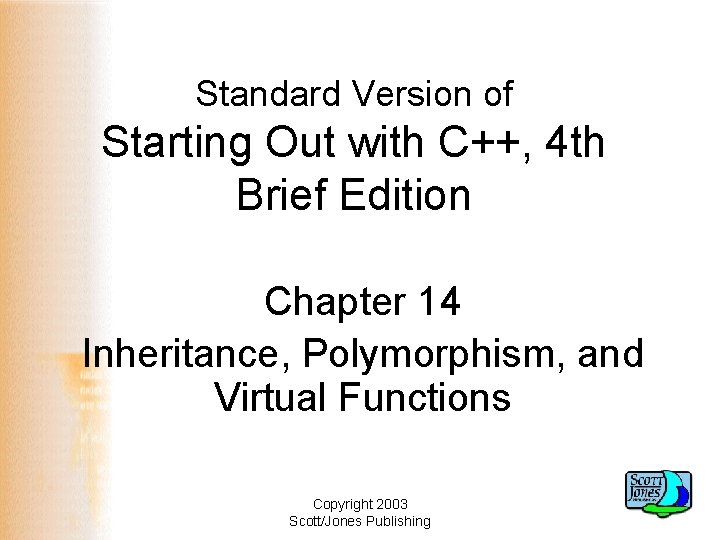
- Slides: 31
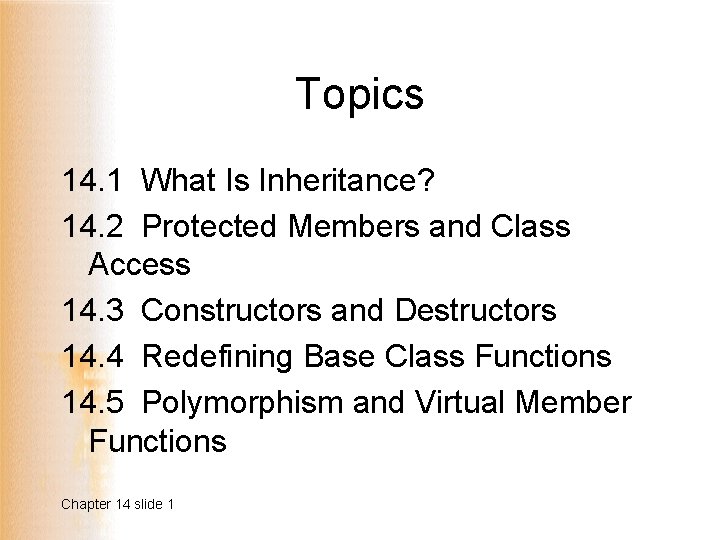
Topics 14. 1 What Is Inheritance? 14. 2 Protected Members and Class Access 14. 3 Constructors and Destructors 14. 4 Redefining Base Class Functions 14. 5 Polymorphism and Virtual Member Functions Chapter 14 slide 1
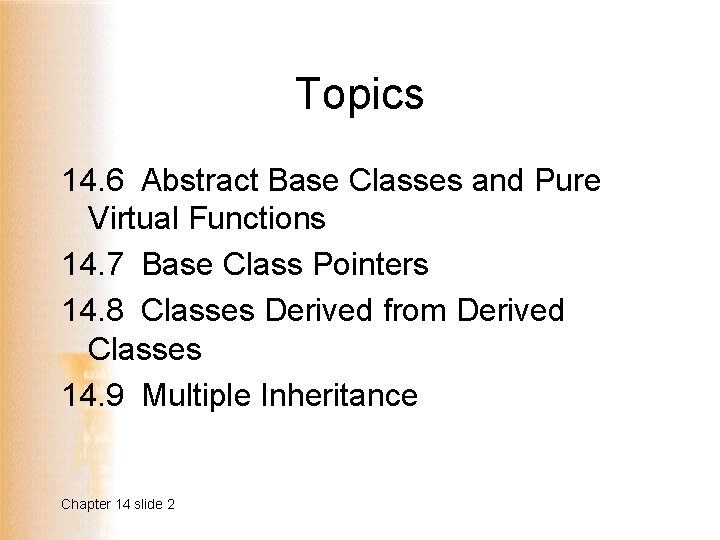
Topics 14. 6 Abstract Base Classes and Pure Virtual Functions 14. 7 Base Class Pointers 14. 8 Classes Derived from Derived Classes 14. 9 Multiple Inheritance Chapter 14 slide 2
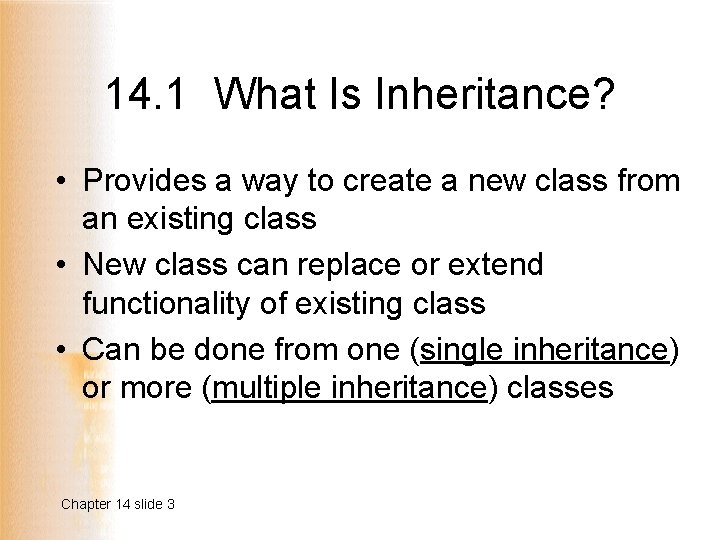
14. 1 What Is Inheritance? • Provides a way to create a new class from an existing class • New class can replace or extend functionality of existing class • Can be done from one (single inheritance) or more (multiple inheritance) classes Chapter 14 slide 3
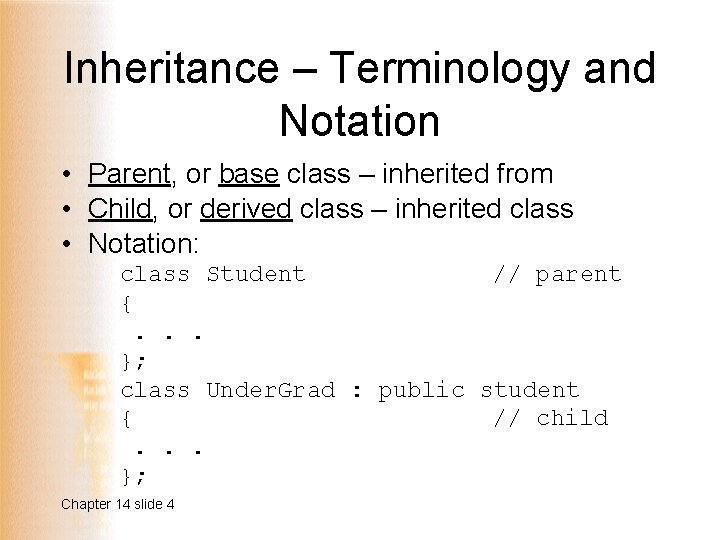
Inheritance – Terminology and Notation • Parent, or base class – inherited from • Child, or derived class – inherited class • Notation: class Student // parent {. . . }; class Under. Grad : public student { // child. . . }; Chapter 14 slide 4
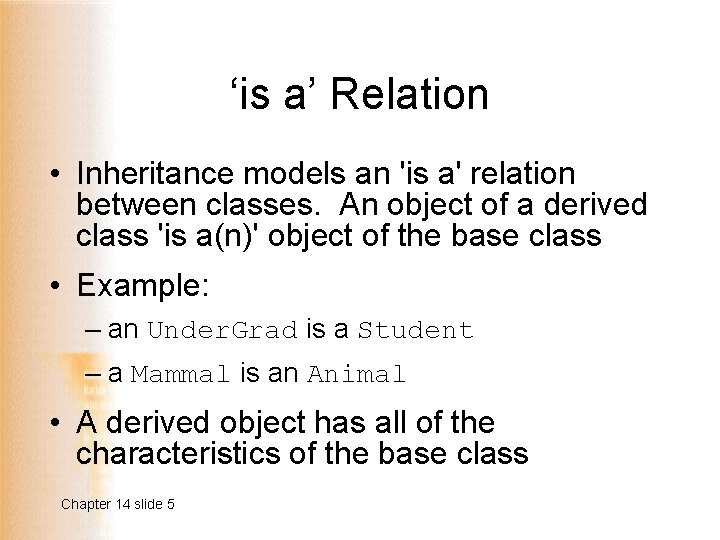
‘is a’ Relation • Inheritance models an 'is a' relation between classes. An object of a derived class 'is a(n)' object of the base class • Example: – an Under. Grad is a Student – a Mammal is an Animal • A derived object has all of the characteristics of the base class Chapter 14 slide 5
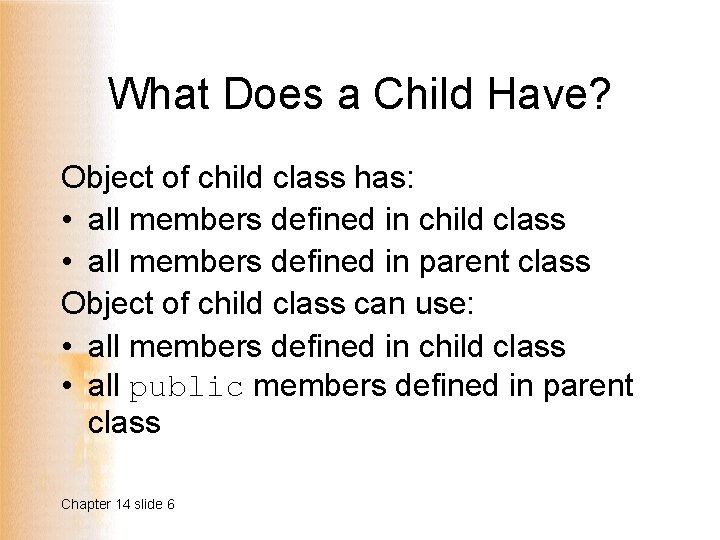
What Does a Child Have? Object of child class has: • all members defined in child class • all members defined in parent class Object of child class can use: • all members defined in child class • all public members defined in parent class Chapter 14 slide 6
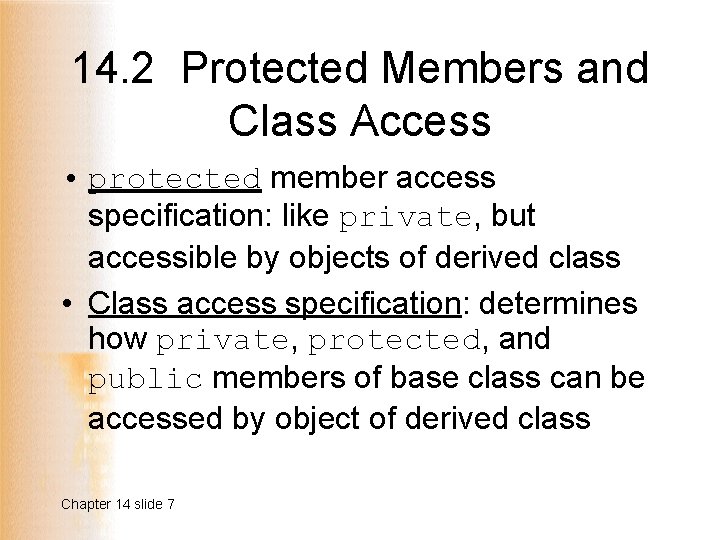
14. 2 Protected Members and Class Access • protected member access specification: like private, but accessible by objects of derived class • Class access specification: determines how private, protected, and public members of base class can be accessed by object of derived class Chapter 14 slide 7
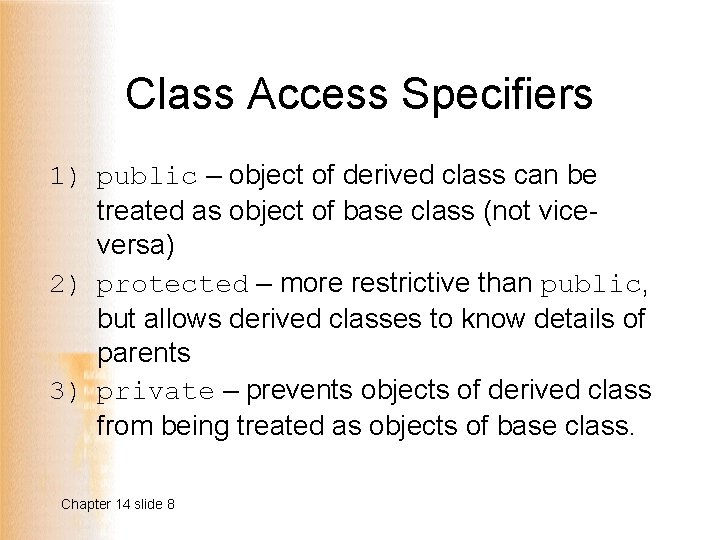
Class Access Specifiers 1) public – object of derived class can be treated as object of base class (not viceversa) 2) protected – more restrictive than public, but allows derived classes to know details of parents 3) private – prevents objects of derived class from being treated as objects of base class. Chapter 14 slide 8
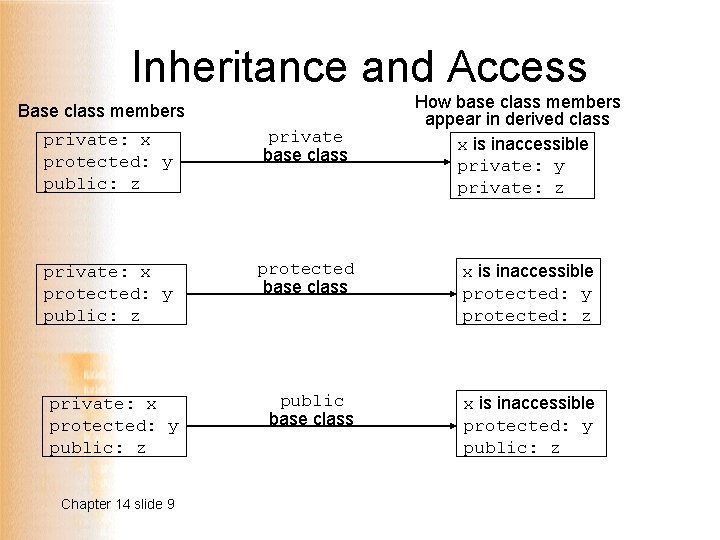
Inheritance and Access Base class members How base class members appear in derived class x is inaccessible private: y private: z private: x protected: y public: z private base class private: x protected: y public: z protected base class x is inaccessible protected: y protected: z public base class x is inaccessible protected: y public: z private: x protected: y public: z Chapter 14 slide 9
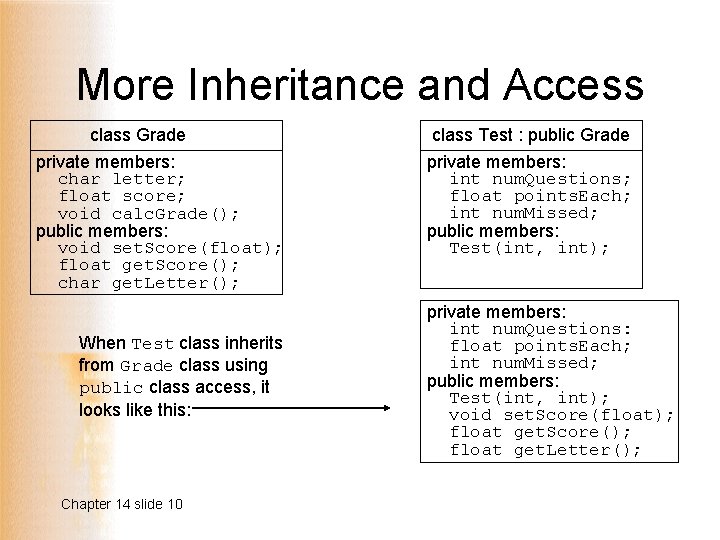
More Inheritance and Access class Grade private members: char letter; float score; void calc. Grade(); public members: void set. Score(float); float get. Score(); char get. Letter(); When Test class inherits from Grade class using public class access, it looks like this: Chapter 14 slide 10 class Test : public Grade private members: int num. Questions; float points. Each; int num. Missed; public members: Test(int, int); private members: int num. Questions: float points. Each; int num. Missed; public members: Test(int, int); void set. Score(float); float get. Score(); float get. Letter();
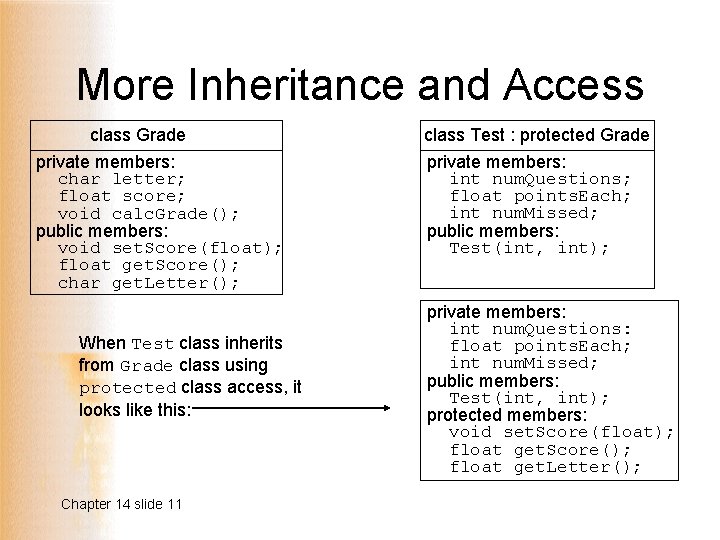
More Inheritance and Access class Grade private members: char letter; float score; void calc. Grade(); public members: void set. Score(float); float get. Score(); char get. Letter(); When Test class inherits from Grade class using protected class access, it looks like this: Chapter 14 slide 11 class Test : protected Grade private members: int num. Questions; float points. Each; int num. Missed; public members: Test(int, int); private members: int num. Questions: float points. Each; int num. Missed; public members: Test(int, int); protected members: void set. Score(float); float get. Score(); float get. Letter();
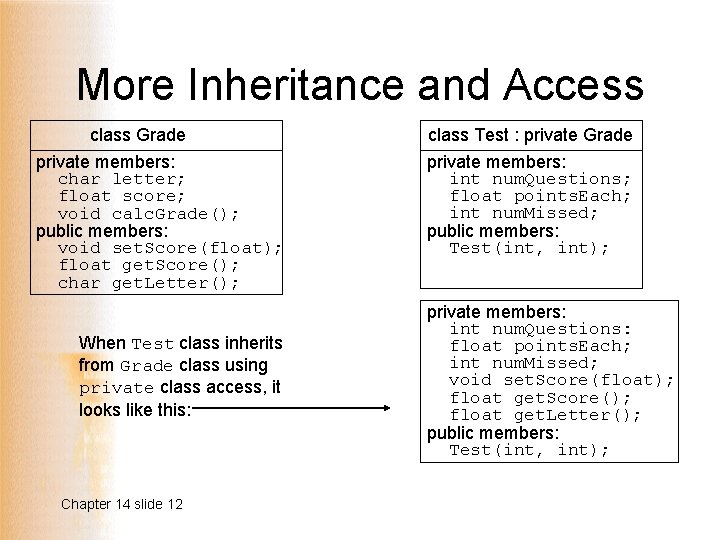
More Inheritance and Access class Grade private members: char letter; float score; void calc. Grade(); public members: void set. Score(float); float get. Score(); char get. Letter(); When Test class inherits from Grade class using private class access, it looks like this: Chapter 14 slide 12 class Test : private Grade private members: int num. Questions; float points. Each; int num. Missed; public members: Test(int, int); private members: int num. Questions: float points. Each; int num. Missed; void set. Score(float); float get. Score(); float get. Letter(); public members: Test(int, int);
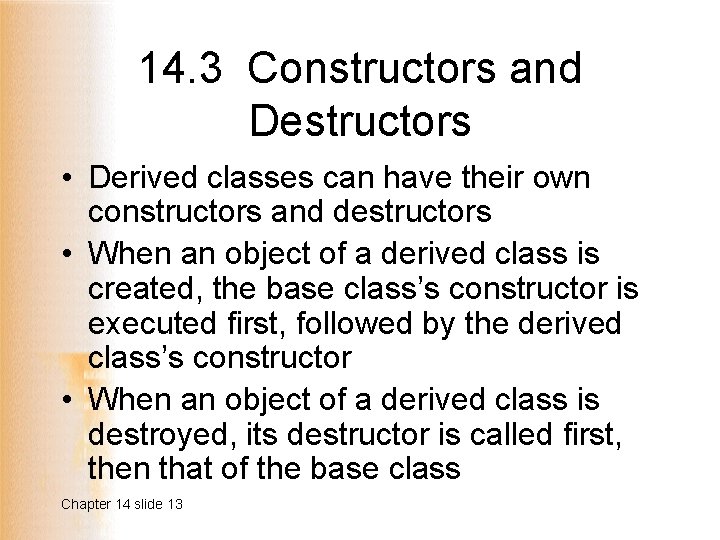
14. 3 Constructors and Destructors • Derived classes can have their own constructors and destructors • When an object of a derived class is created, the base class’s constructor is executed first, followed by the derived class’s constructor • When an object of a derived class is destroyed, its destructor is called first, then that of the base class Chapter 14 slide 13
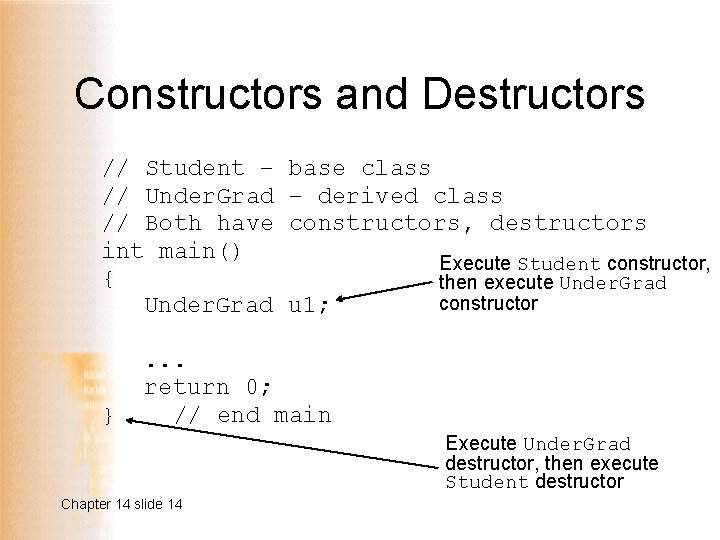
Constructors and Destructors // Student – // Under. Grad // Both have int main() { Under. Grad } base class – derived class constructors, destructors u 1; Execute Student constructor, then execute Under. Grad constructor . . . return 0; // end main Execute Under. Grad destructor, then execute Student destructor Chapter 14 slide 14
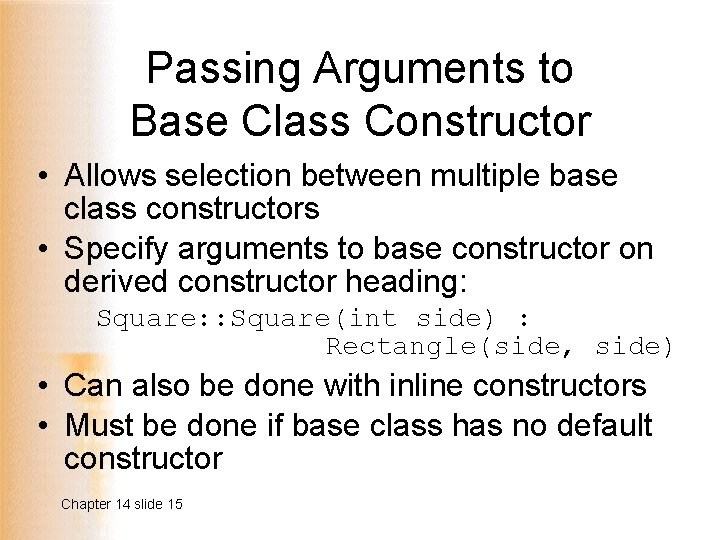
Passing Arguments to Base Class Constructor • Allows selection between multiple base class constructors • Specify arguments to base constructor on derived constructor heading: Square: : Square(int side) : Rectangle(side, side) • Can also be done with inline constructors • Must be done if base class has no default constructor Chapter 14 slide 15
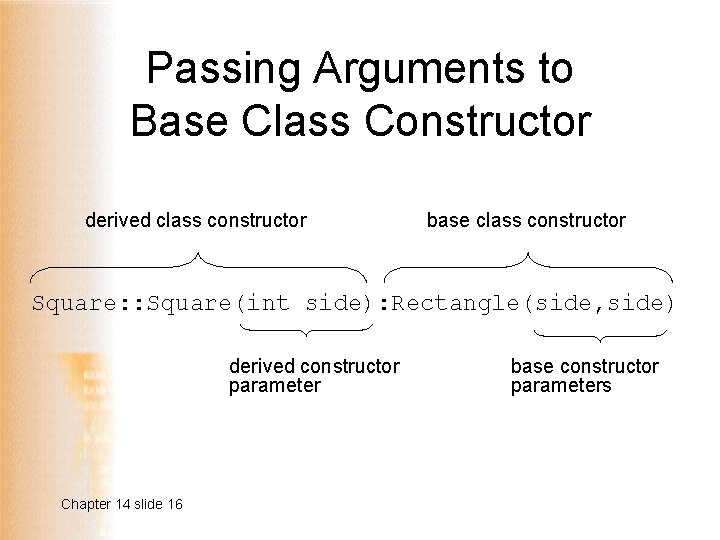
Passing Arguments to Base Class Constructor derived class constructor base class constructor Square: : Square(int side): Rectangle(side, side) derived constructor parameter Chapter 14 slide 16 base constructor parameters
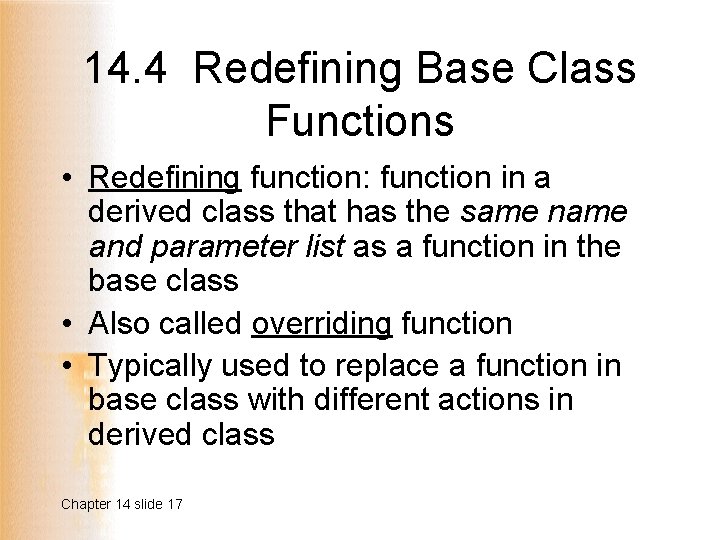
14. 4 Redefining Base Class Functions • Redefining function: function in a derived class that has the same name and parameter list as a function in the base class • Also called overriding function • Typically used to replace a function in base class with different actions in derived class Chapter 14 slide 17
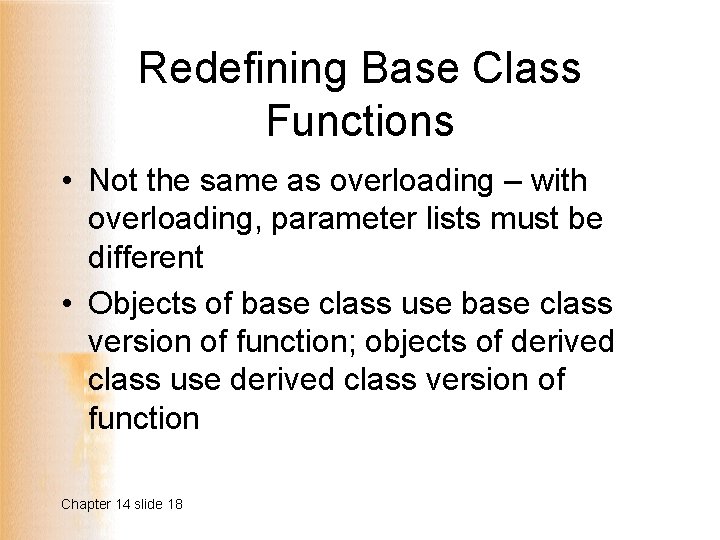
Redefining Base Class Functions • Not the same as overloading – with overloading, parameter lists must be different • Objects of base class use base class version of function; objects of derived class use derived class version of function Chapter 14 slide 18
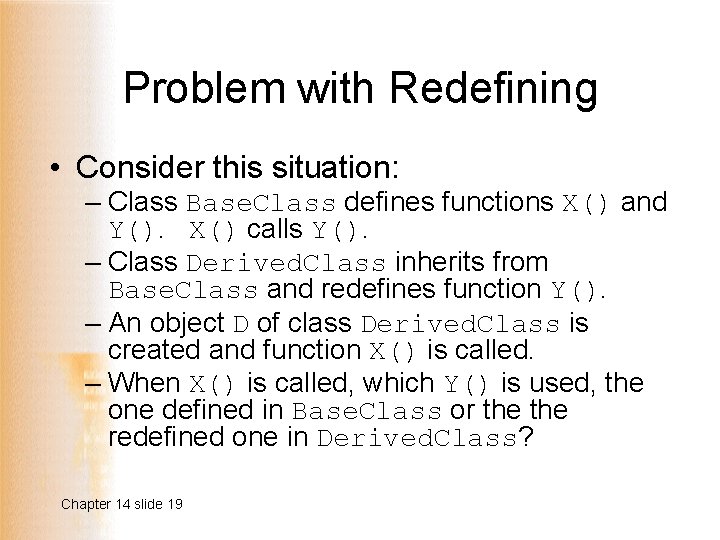
Problem with Redefining • Consider this situation: – Class Base. Class defines functions X() and Y(). X() calls Y(). – Class Derived. Class inherits from Base. Class and redefines function Y(). – An object D of class Derived. Class is created and function X() is called. – When X() is called, which Y() is used, the one defined in Base. Class or the redefined one in Derived. Class? Chapter 14 slide 19
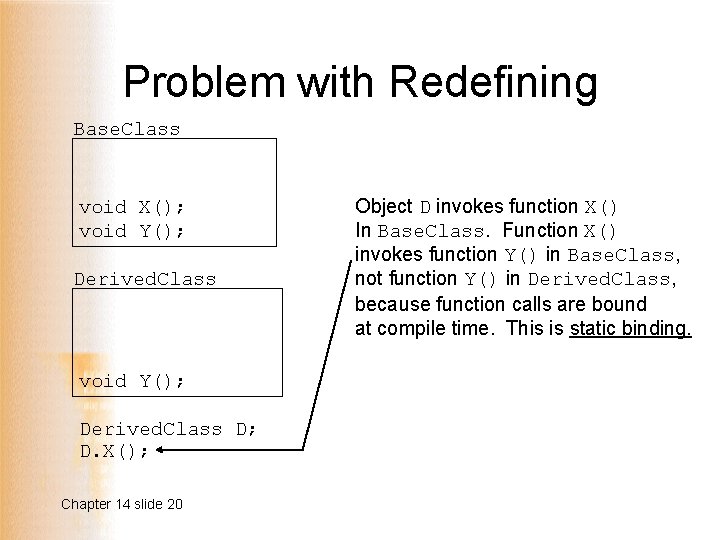
Problem with Redefining Base. Class void X(); void Y(); Derived. Class D; D. X(); Chapter 14 slide 20 Object D invokes function X() In Base. Class. Function X() invokes function Y() in Base. Class, not function Y() in Derived. Class, because function calls are bound at compile time. This is static binding.
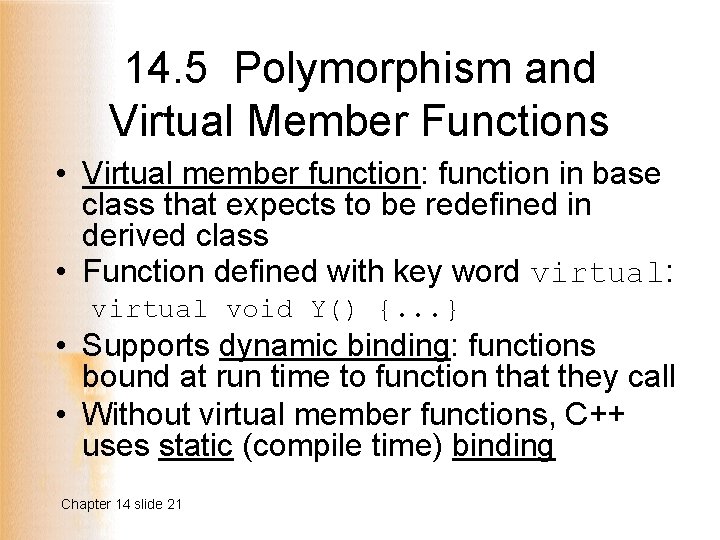
14. 5 Polymorphism and Virtual Member Functions • Virtual member function: function in base class that expects to be redefined in derived class • Function defined with key word virtual: virtual void Y() {. . . } • Supports dynamic binding: functions bound at run time to function that they call • Without virtual member functions, C++ uses static (compile time) binding Chapter 14 slide 21
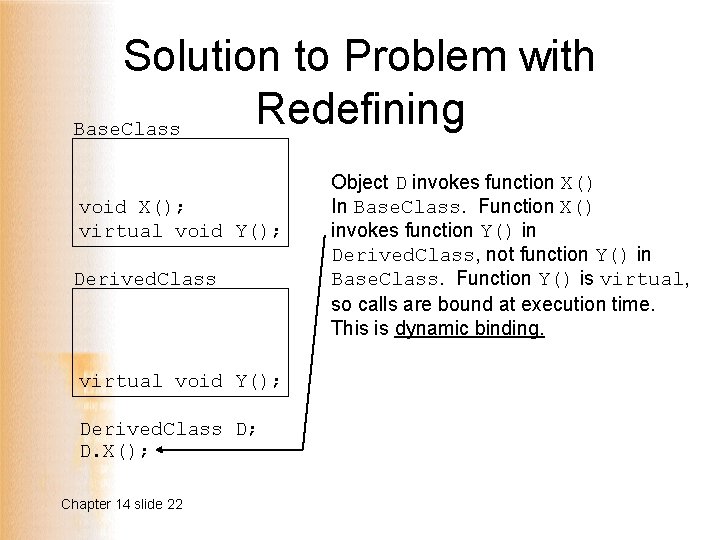
Solution to Problem with Redefining Base. Class void X(); virtual void Y(); Derived. Class D; D. X(); Chapter 14 slide 22 Object D invokes function X() In Base. Class. Function X() invokes function Y() in Derived. Class, not function Y() in Base. Class. Function Y() is virtual, so calls are bound at execution time. This is dynamic binding.
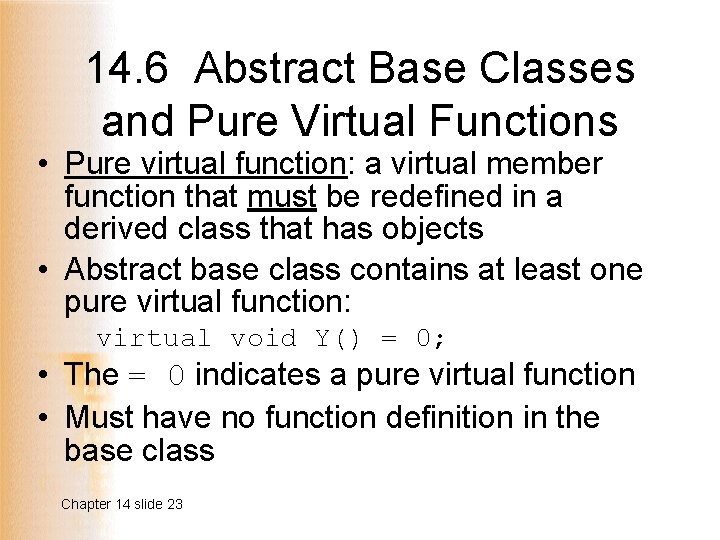
14. 6 Abstract Base Classes and Pure Virtual Functions • Pure virtual function: a virtual member function that must be redefined in a derived class that has objects • Abstract base class contains at least one pure virtual function: virtual void Y() = 0; • The = 0 indicates a pure virtual function • Must have no function definition in the base class Chapter 14 slide 23
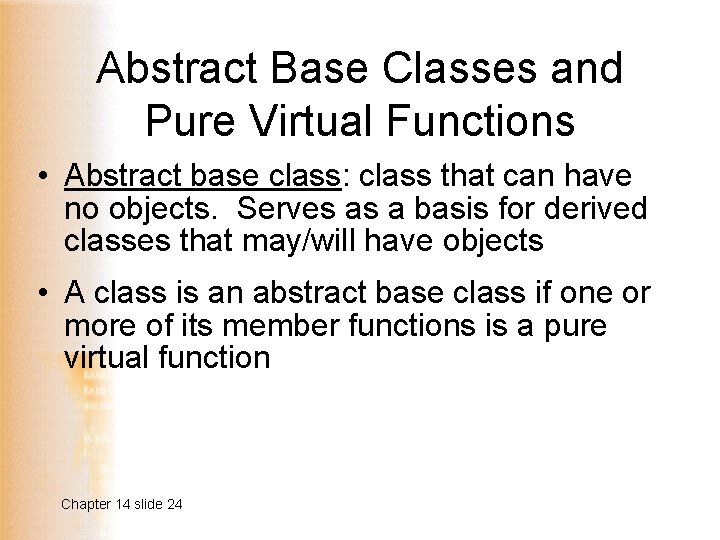
Abstract Base Classes and Pure Virtual Functions • Abstract base class: class that can have no objects. Serves as a basis for derived classes that may/will have objects • A class is an abstract base class if one or more of its member functions is a pure virtual function Chapter 14 slide 24
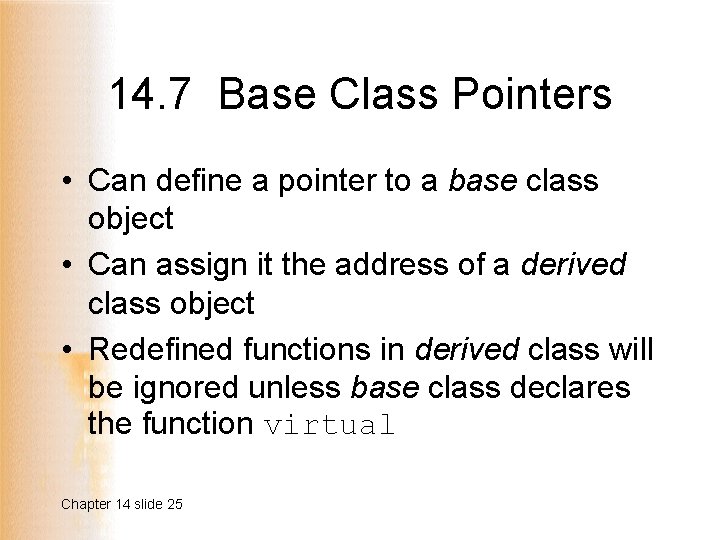
14. 7 Base Class Pointers • Can define a pointer to a base class object • Can assign it the address of a derived class object • Redefined functions in derived class will be ignored unless base class declares the function virtual Chapter 14 slide 25
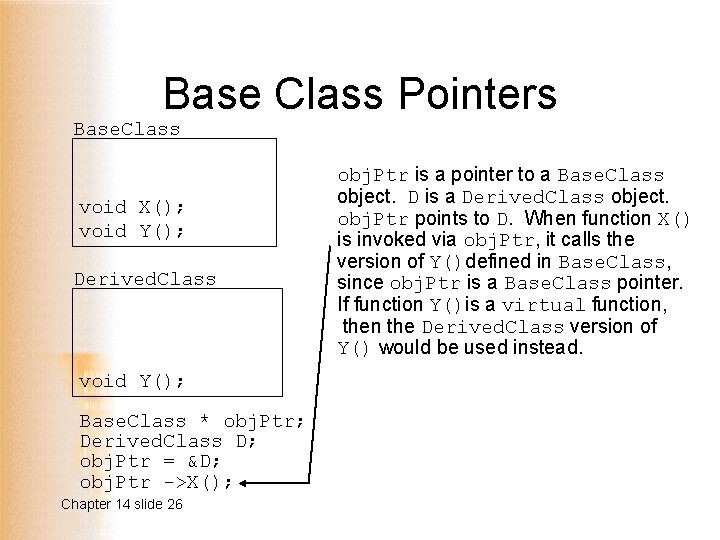
Base Class Pointers Base. Class void X(); void Y(); Derived. Class void Y(); Base. Class * obj. Ptr; Derived. Class D; obj. Ptr = &D; obj. Ptr ->X(); Chapter 14 slide 26 obj. Ptr is a pointer to a Base. Class object. D is a Derived. Class object. obj. Ptr points to D. When function X() is invoked via obj. Ptr, it calls the version of Y()defined in Base. Class, since obj. Ptr is a Base. Class pointer. If function Y()is a virtual function, then the Derived. Class version of Y() would be used instead.
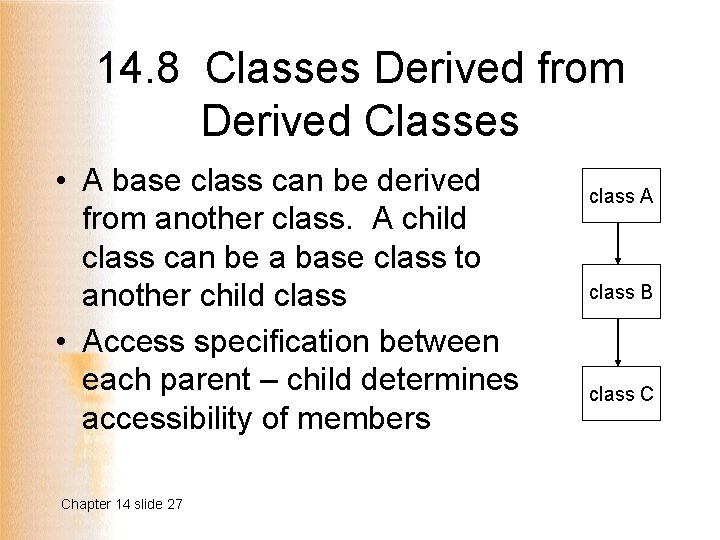
14. 8 Classes Derived from Derived Classes • A base class can be derived from another class. A child class can be a base class to another child class • Access specification between each parent – child determines accessibility of members Chapter 14 slide 27 class A class B class C
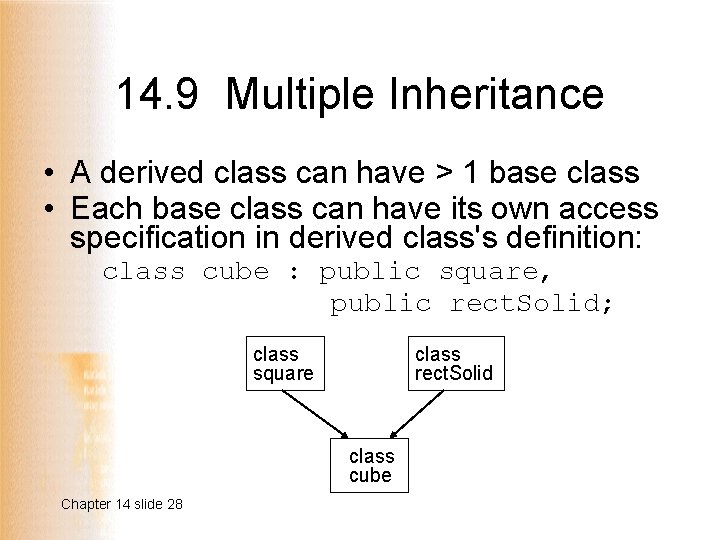
14. 9 Multiple Inheritance • A derived class can have > 1 base class • Each base class can have its own access specification in derived class's definition: class cube : public square, public rect. Solid; class square class rect. Solid class cube Chapter 14 slide 28
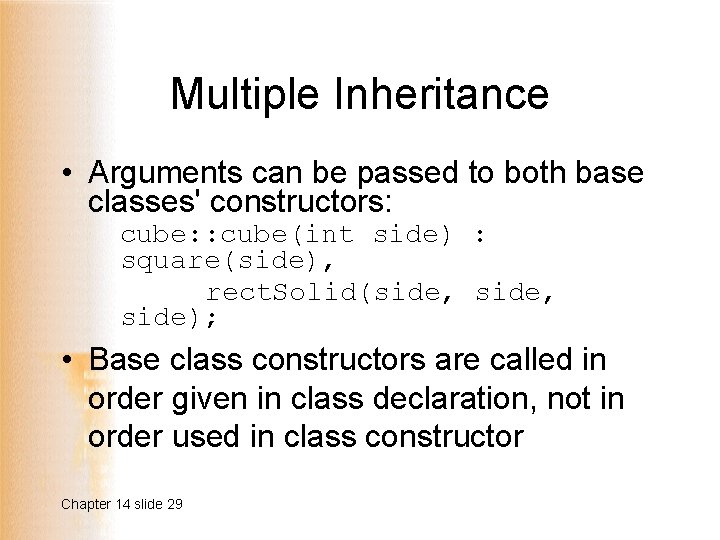
Multiple Inheritance • Arguments can be passed to both base classes' constructors: cube: : cube(int side) : square(side), rect. Solid(side, side); • Base class constructors are called in order given in class declaration, not in order used in class constructor Chapter 14 slide 29
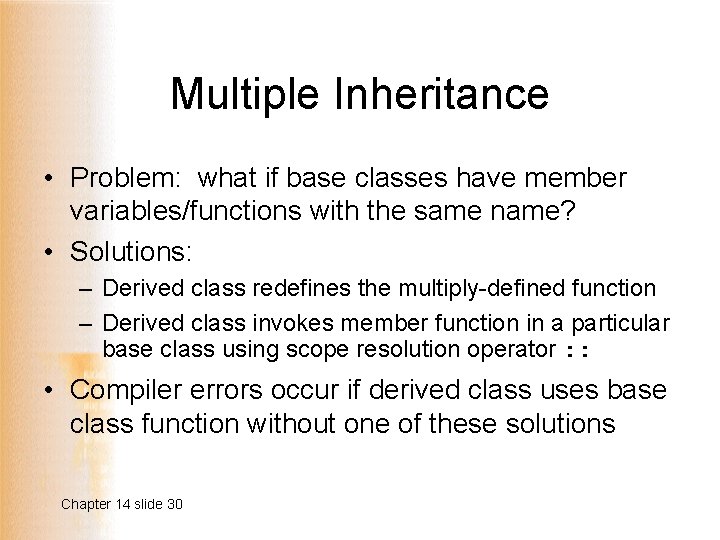
Multiple Inheritance • Problem: what if base classes have member variables/functions with the same name? • Solutions: – Derived class redefines the multiply-defined function – Derived class invokes member function in a particular base class using scope resolution operator : : • Compiler errors occur if derived class uses base class function without one of these solutions Chapter 14 slide 30
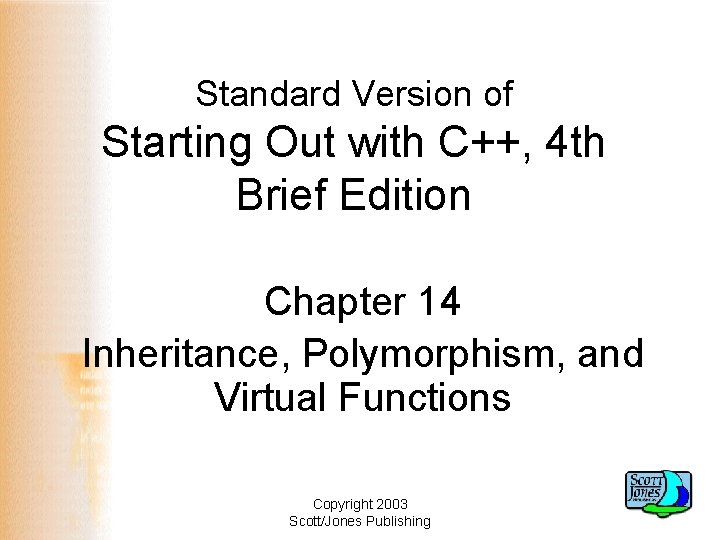
Standard Version of Starting Out with C++, 4 th Brief Edition Chapter 14 Inheritance, Polymorphism, and Virtual Functions Copyright 2003 Scott/Jones Publishing