react Internet course What is React React is
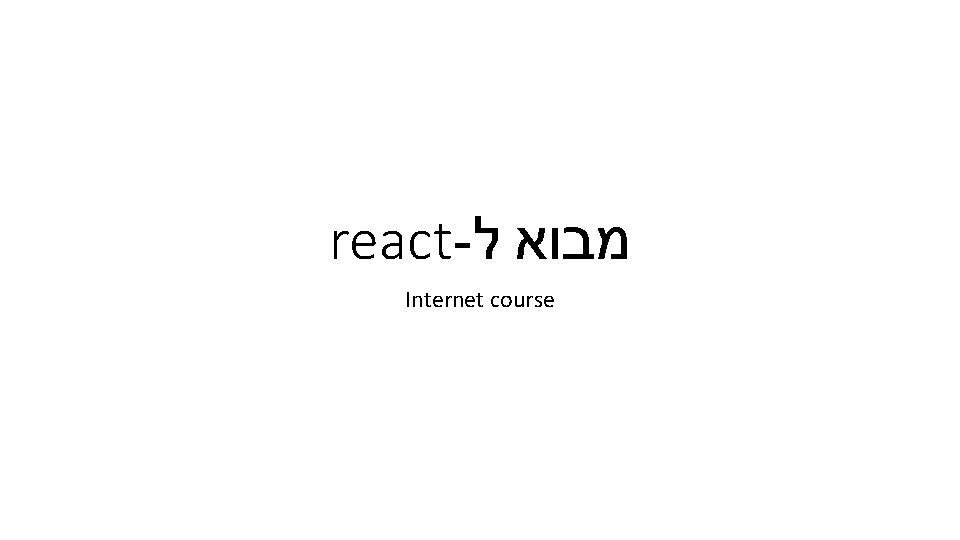
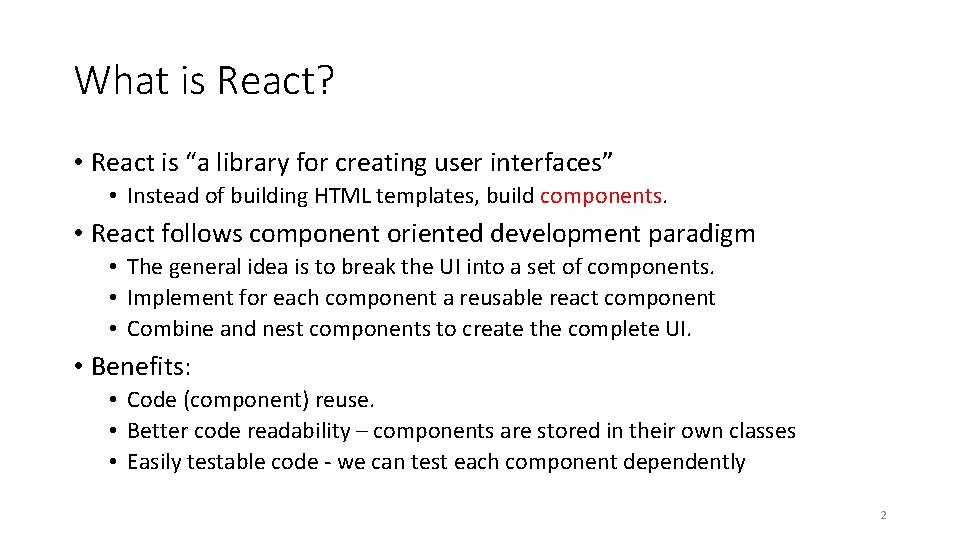
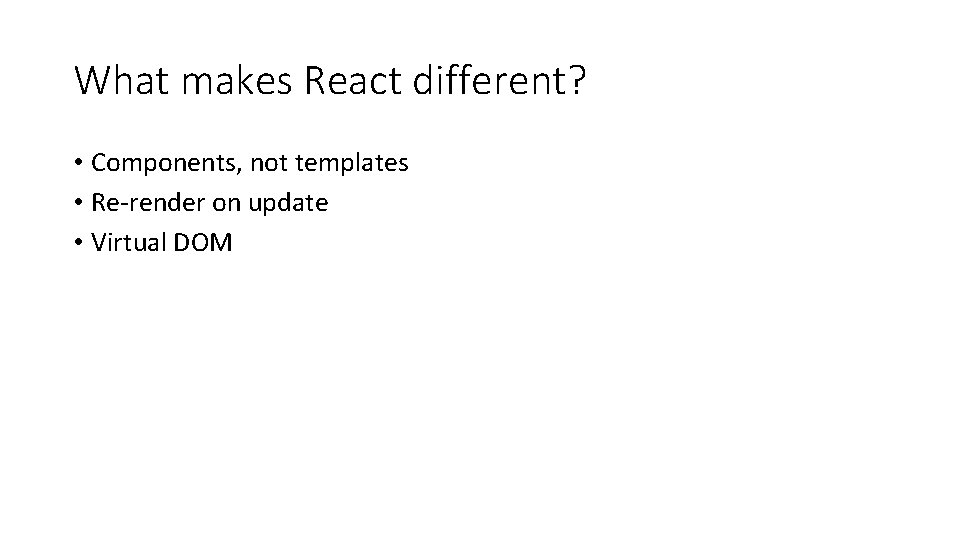
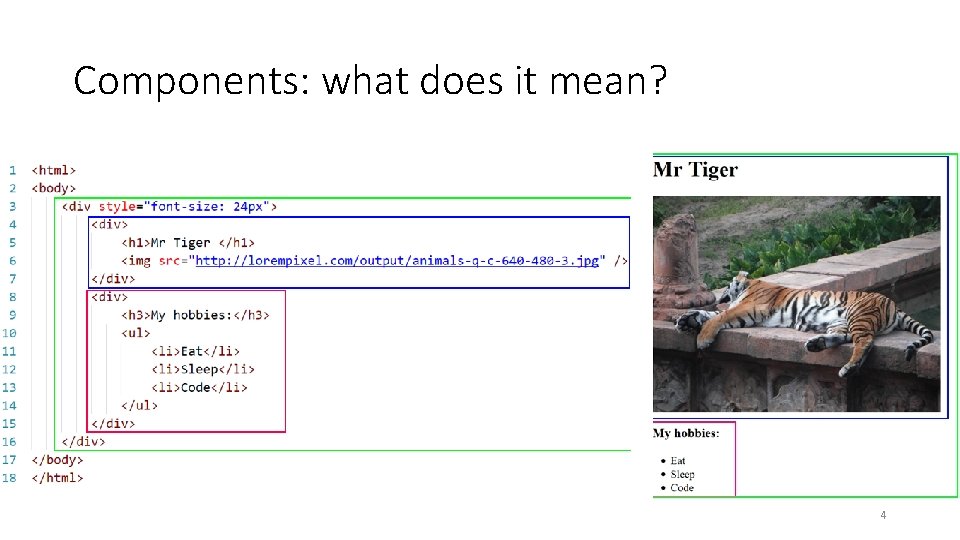
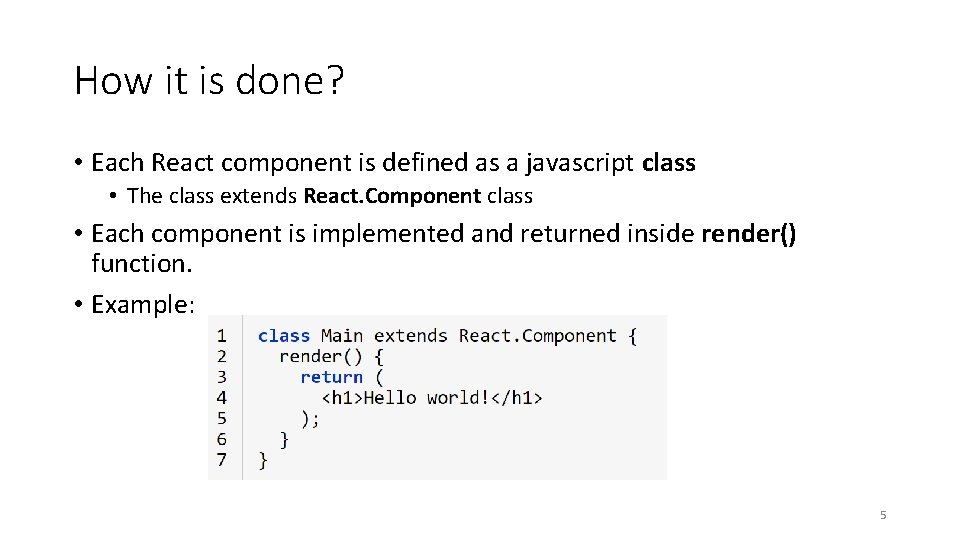
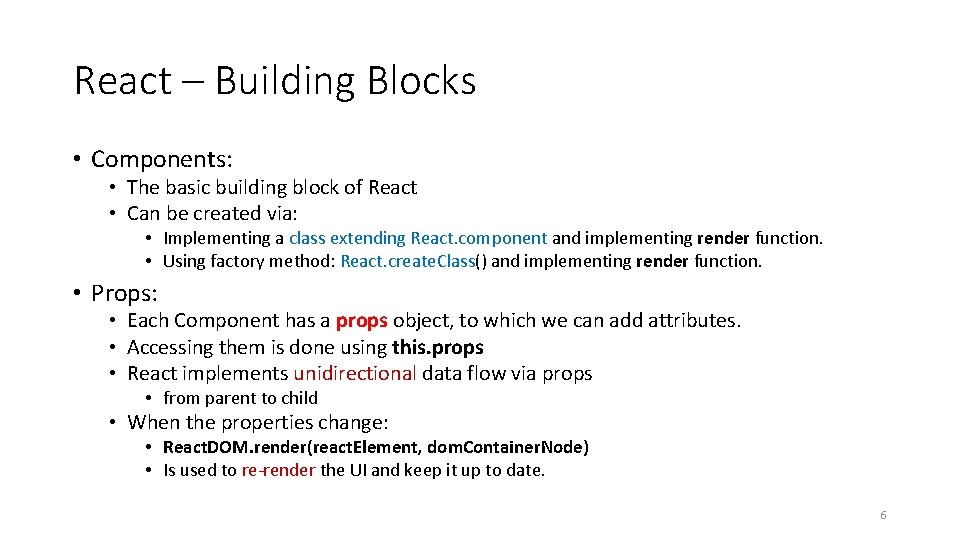
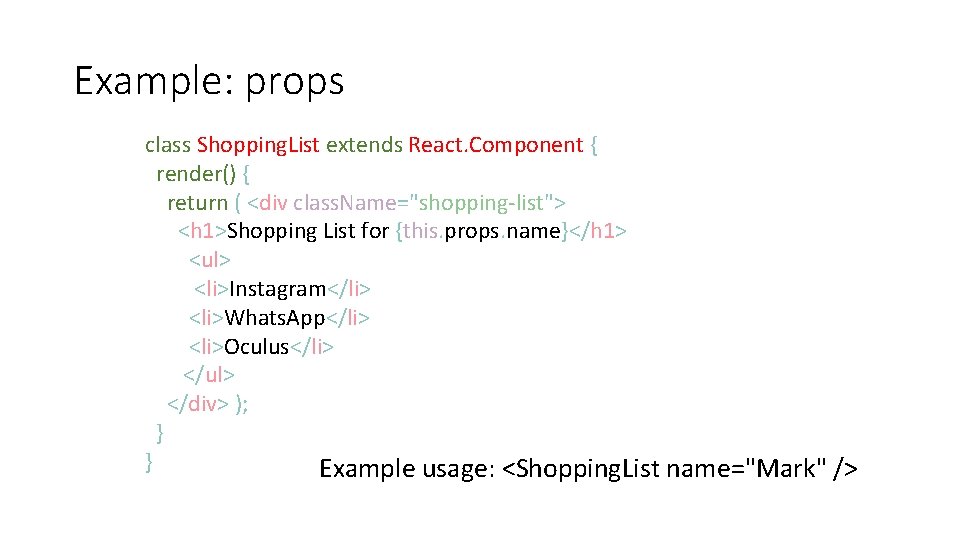
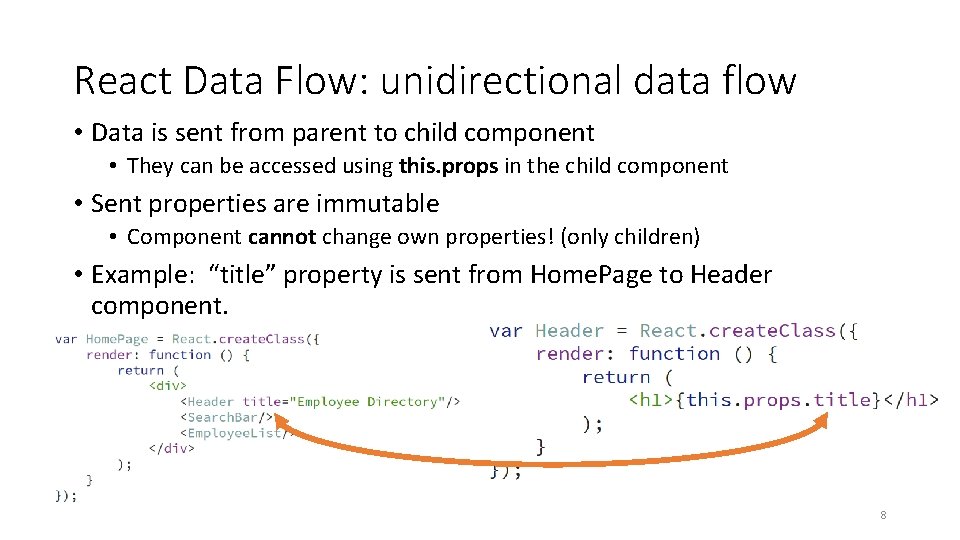
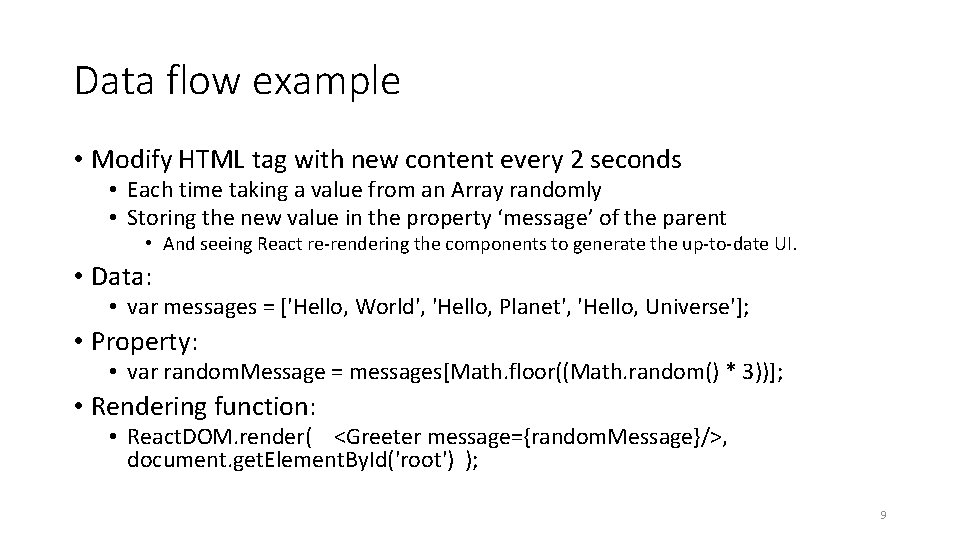
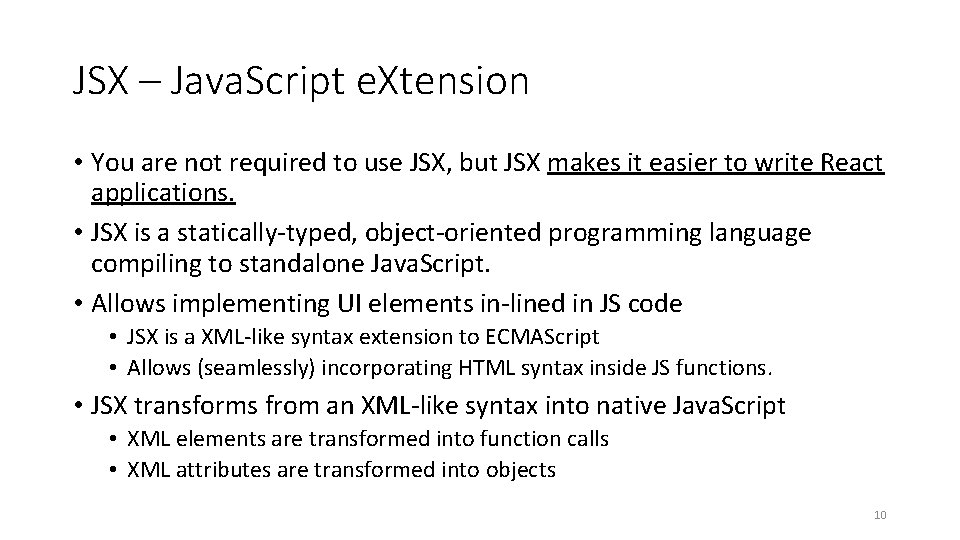
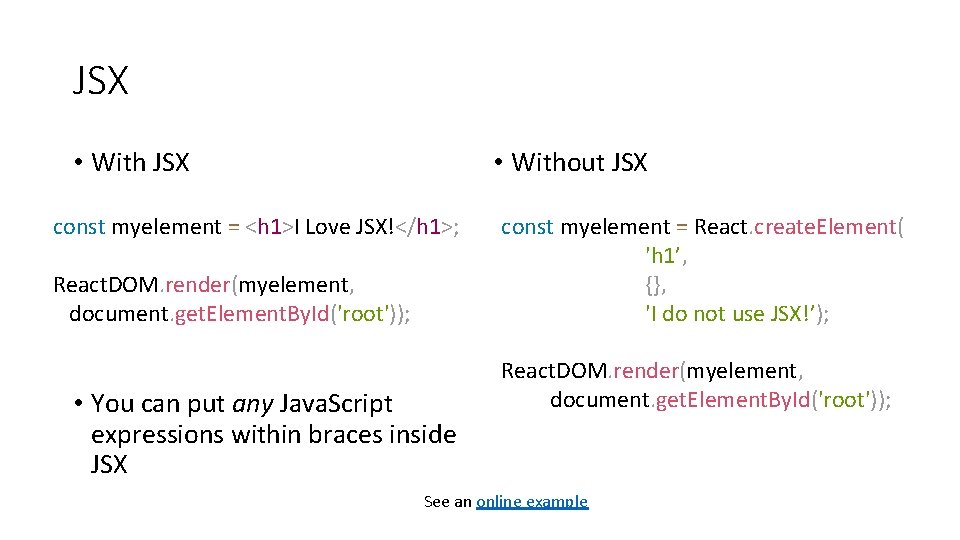
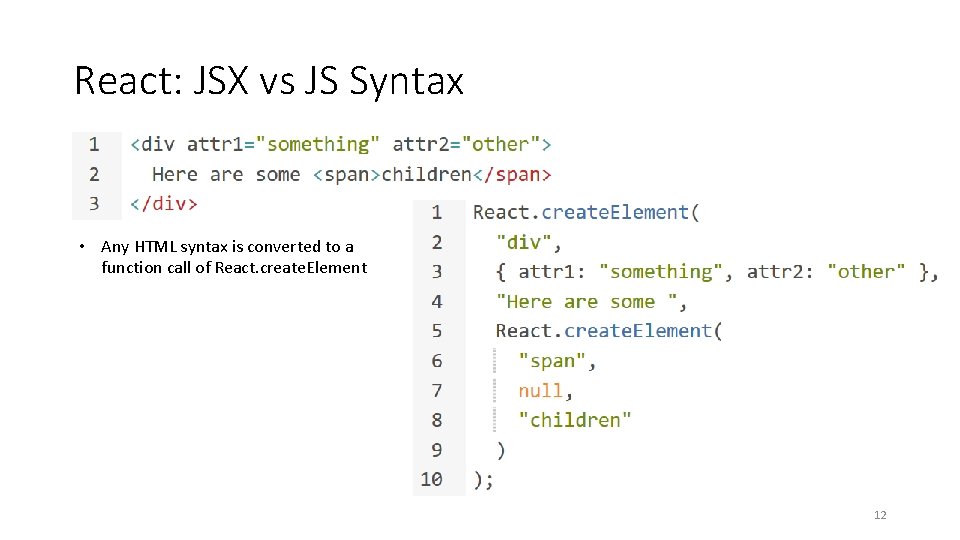
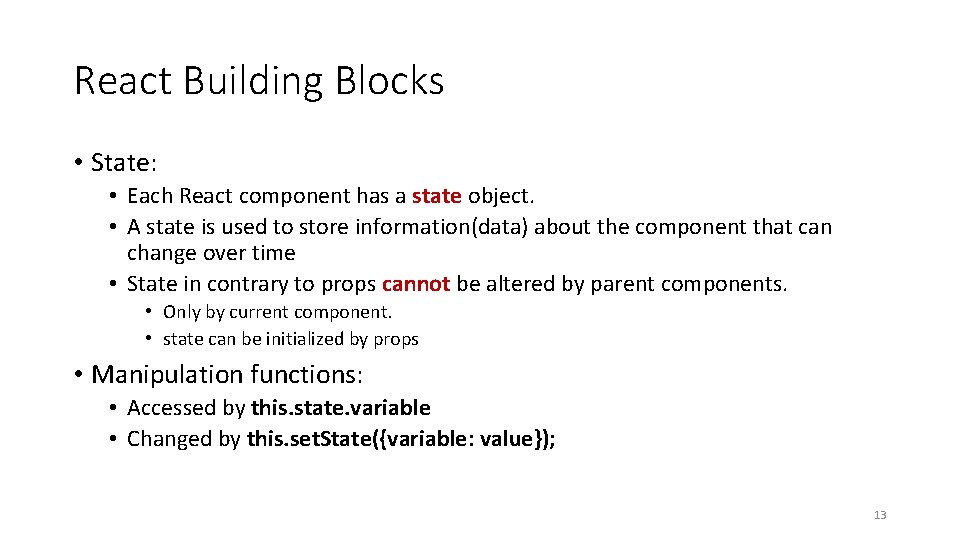
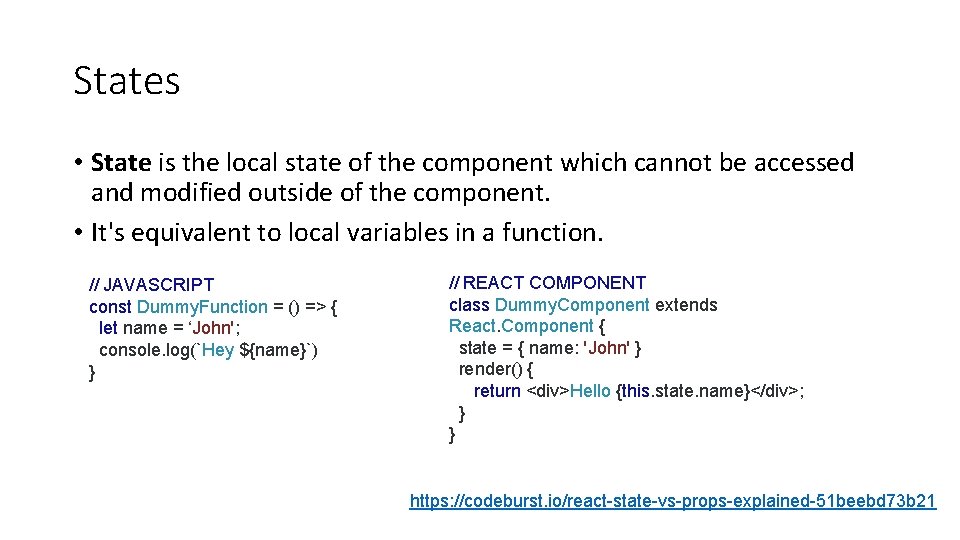
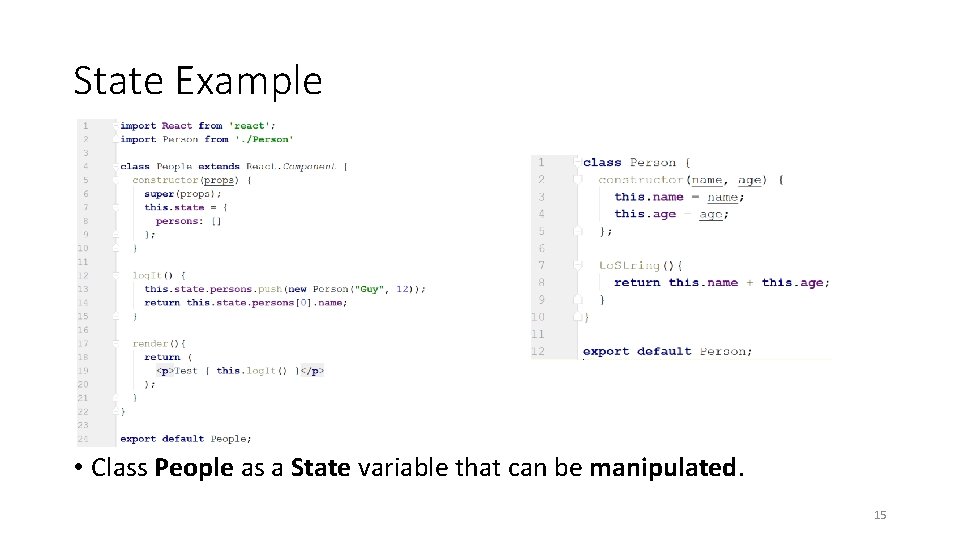
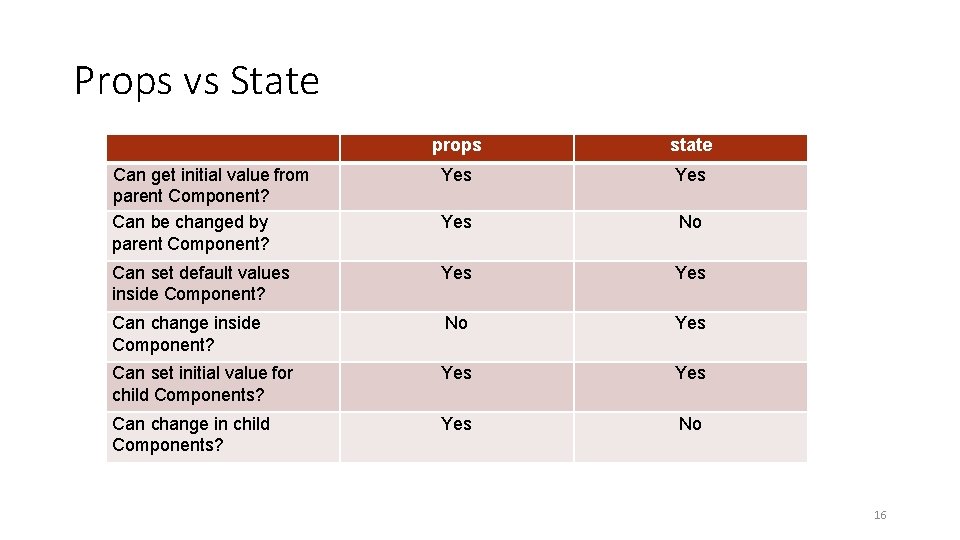
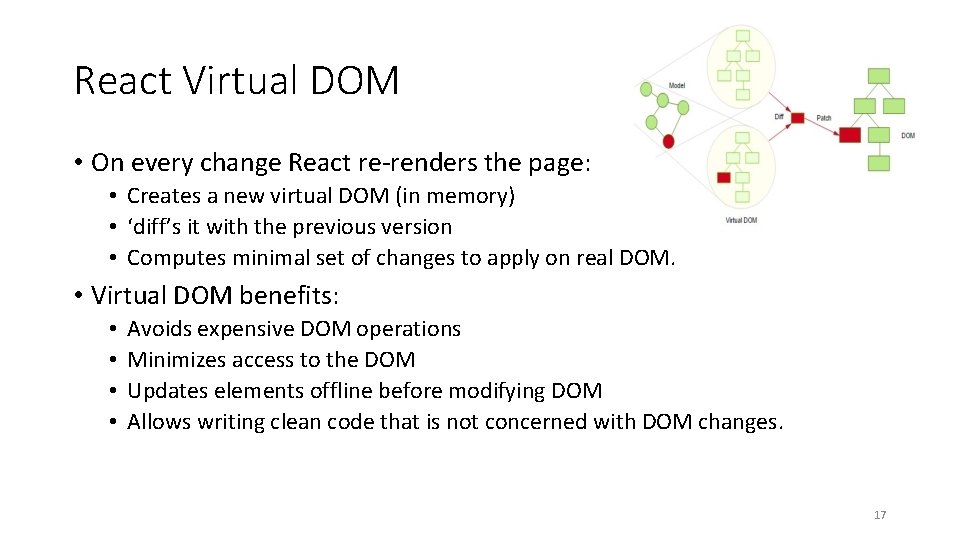
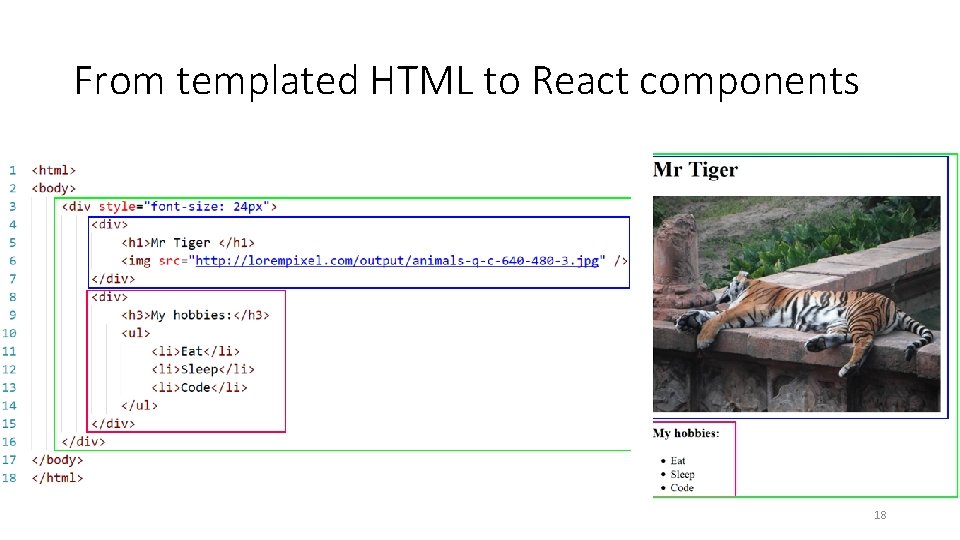
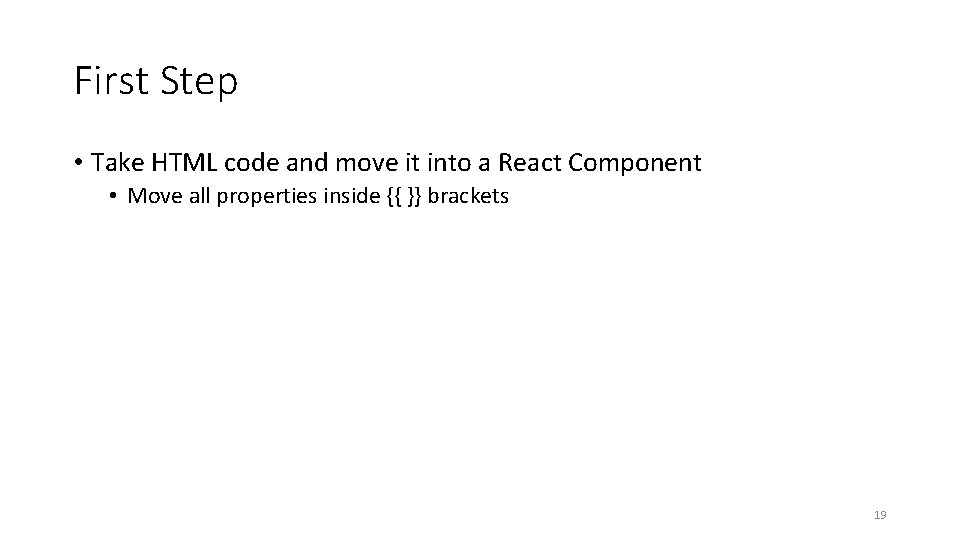
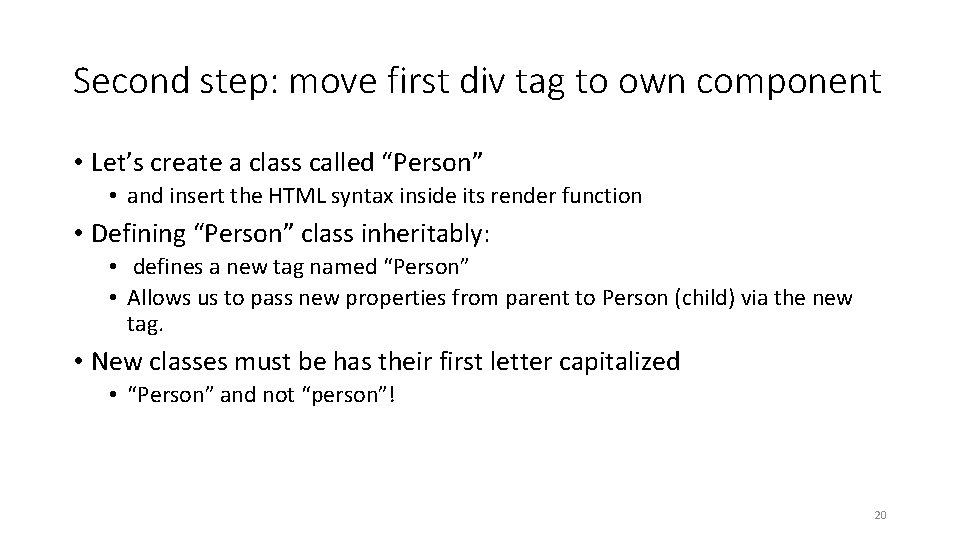
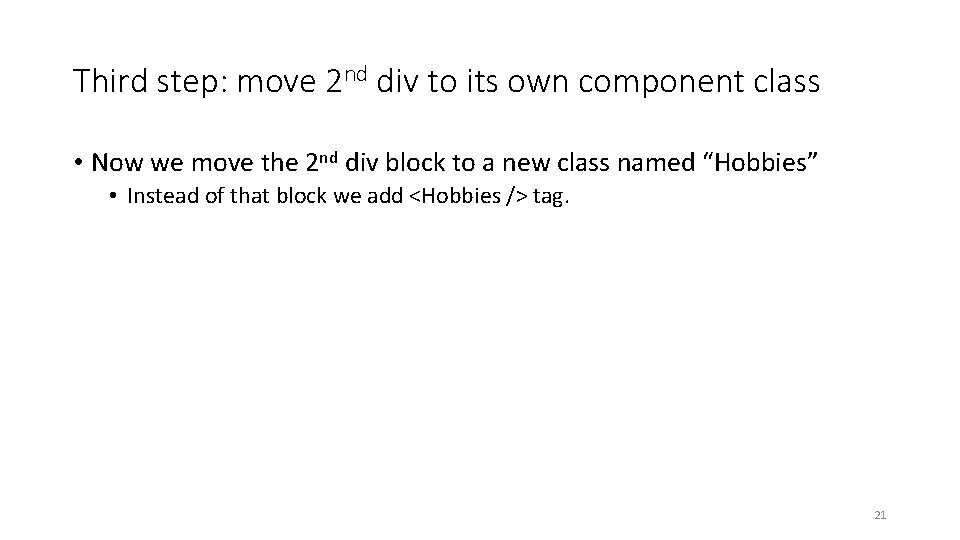
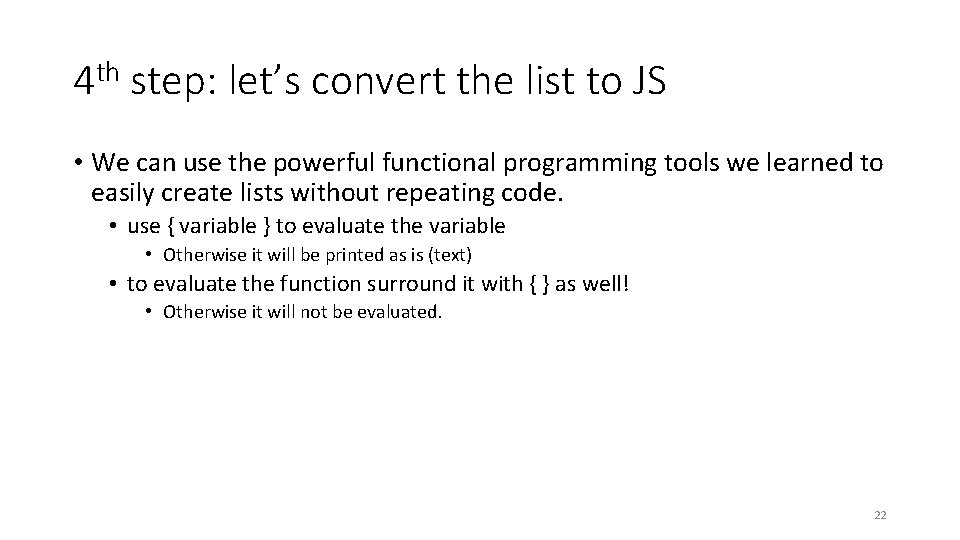
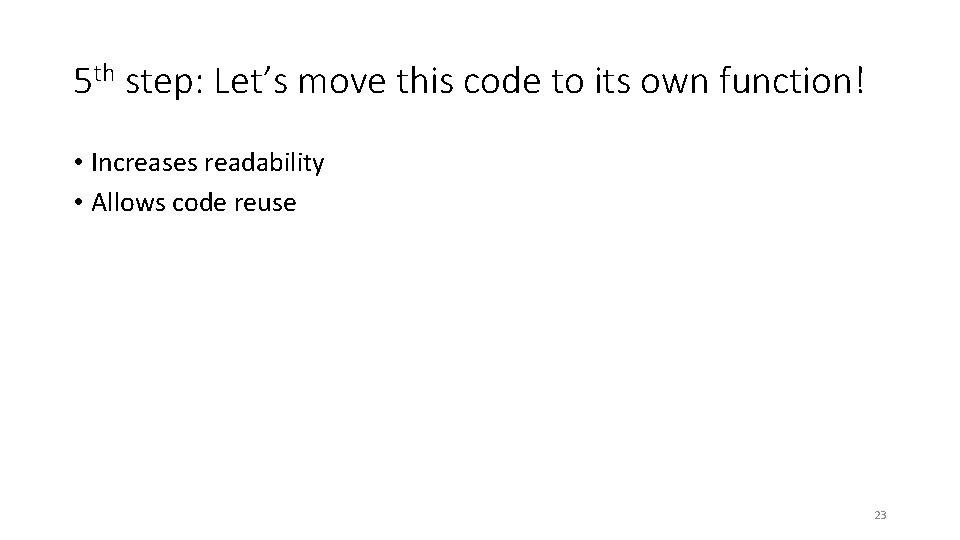
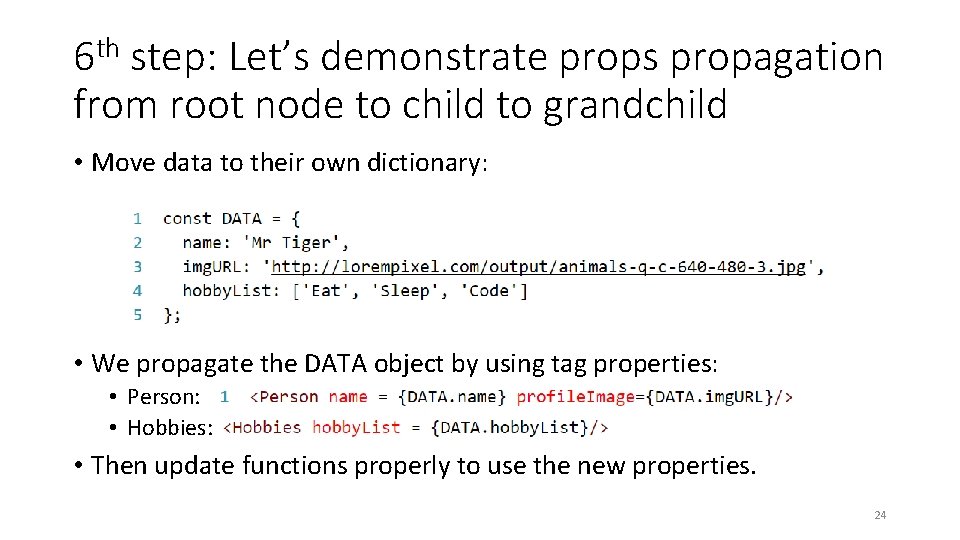
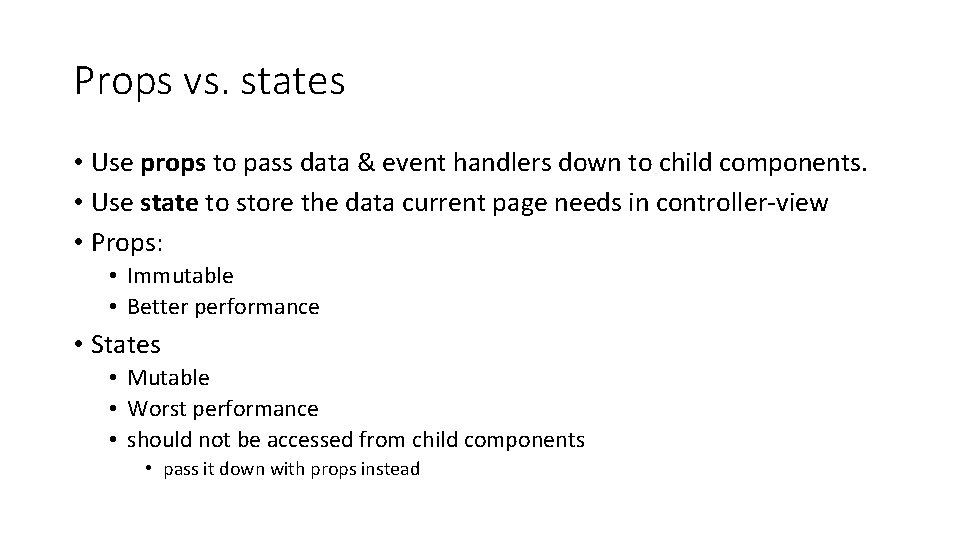
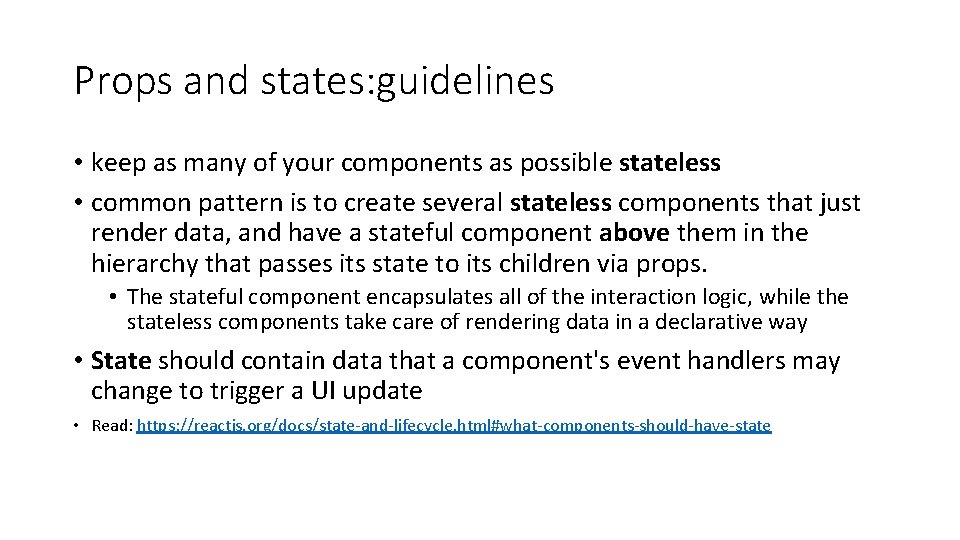
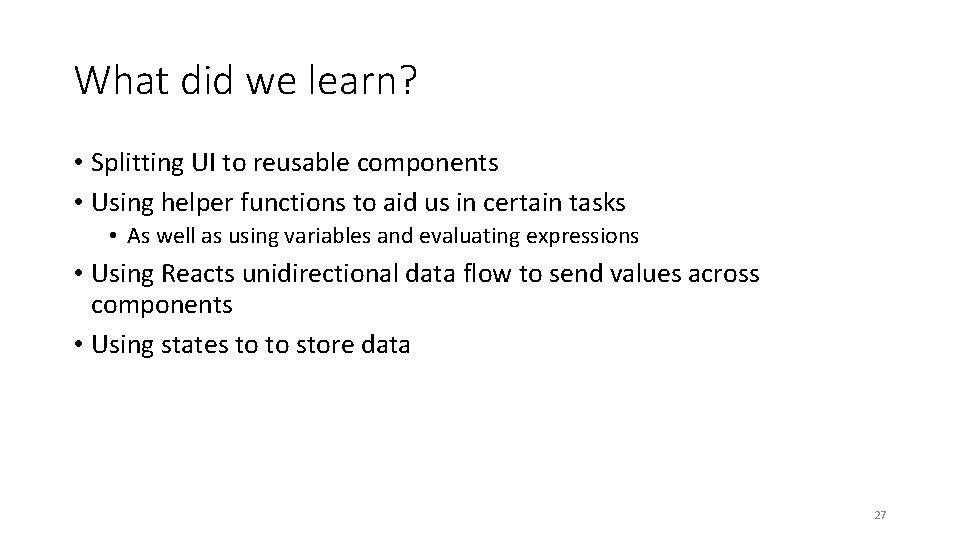
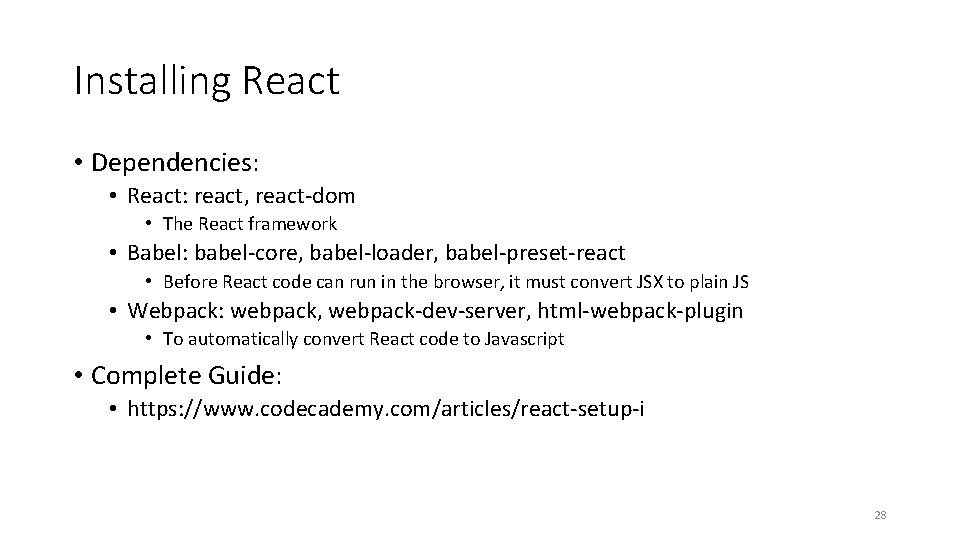
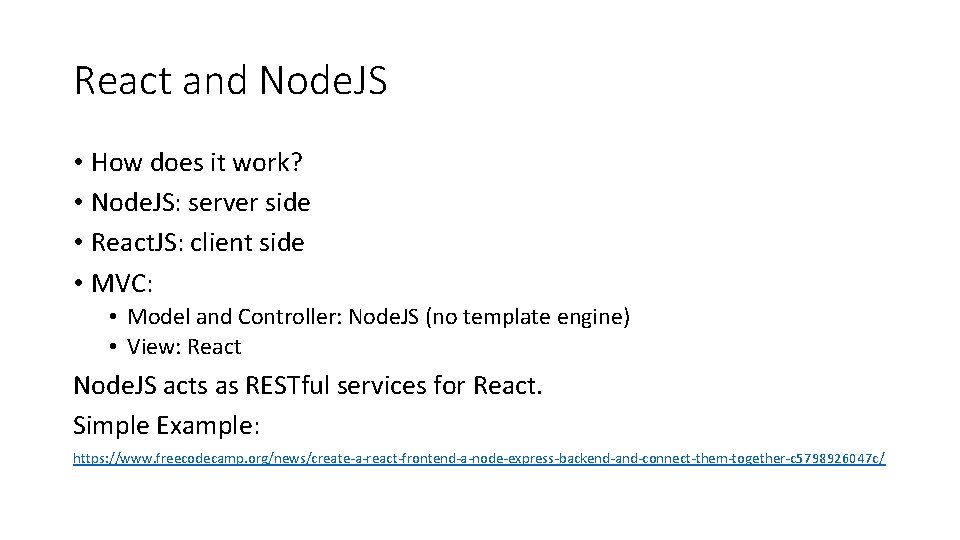
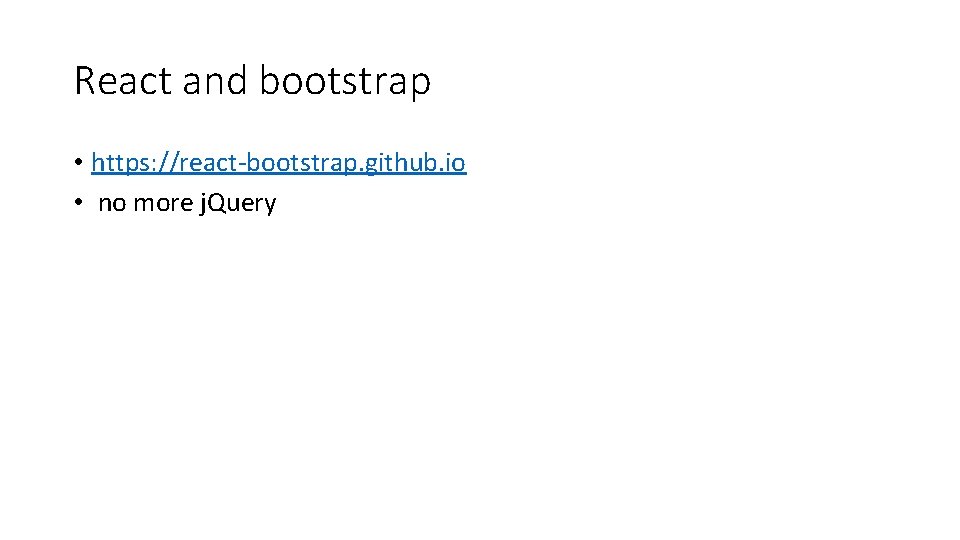
- Slides: 30
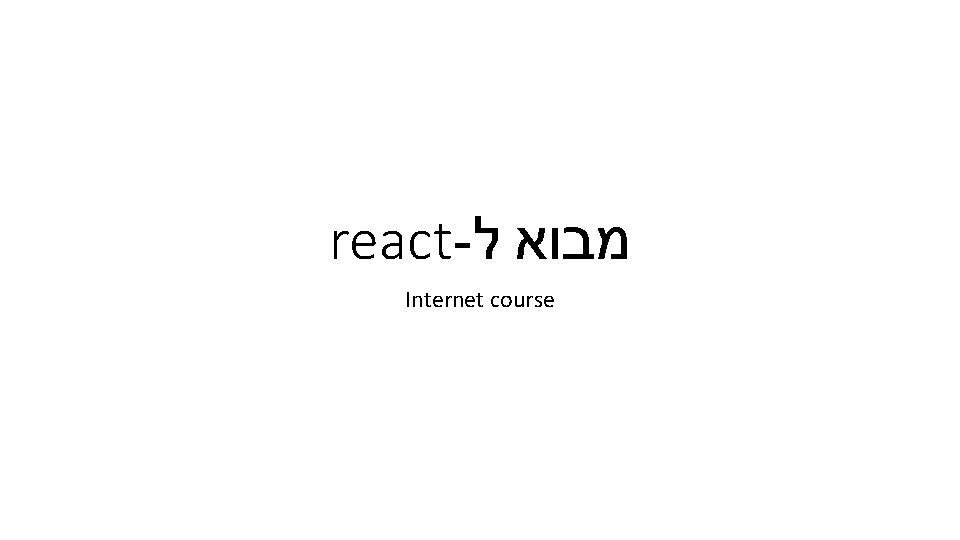
react- מבוא ל Internet course
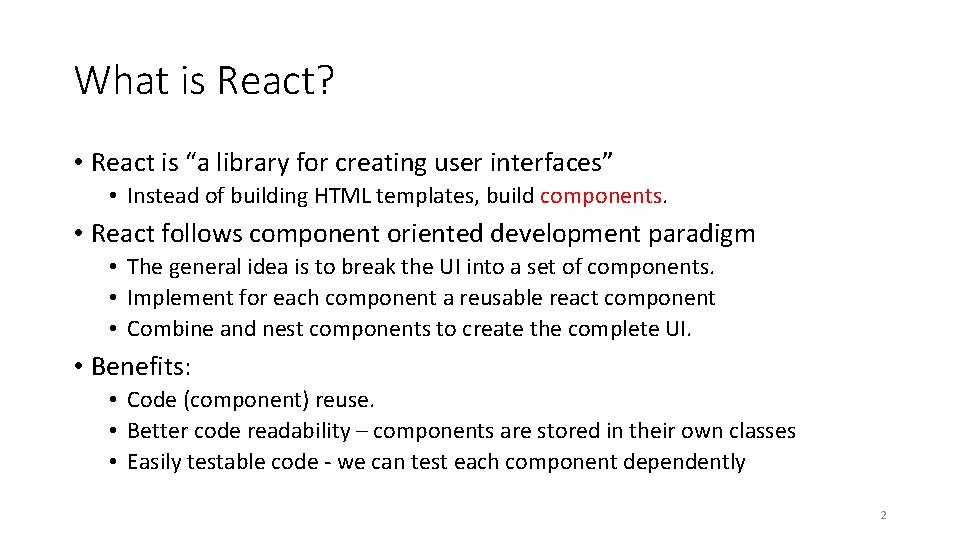
What is React? • React is “a library for creating user interfaces” • Instead of building HTML templates, build components. • React follows component oriented development paradigm • The general idea is to break the UI into a set of components. • Implement for each component a reusable react component • Combine and nest components to create the complete UI. • Benefits: • Code (component) reuse. • Better code readability – components are stored in their own classes • Easily testable code - we can test each component dependently 2
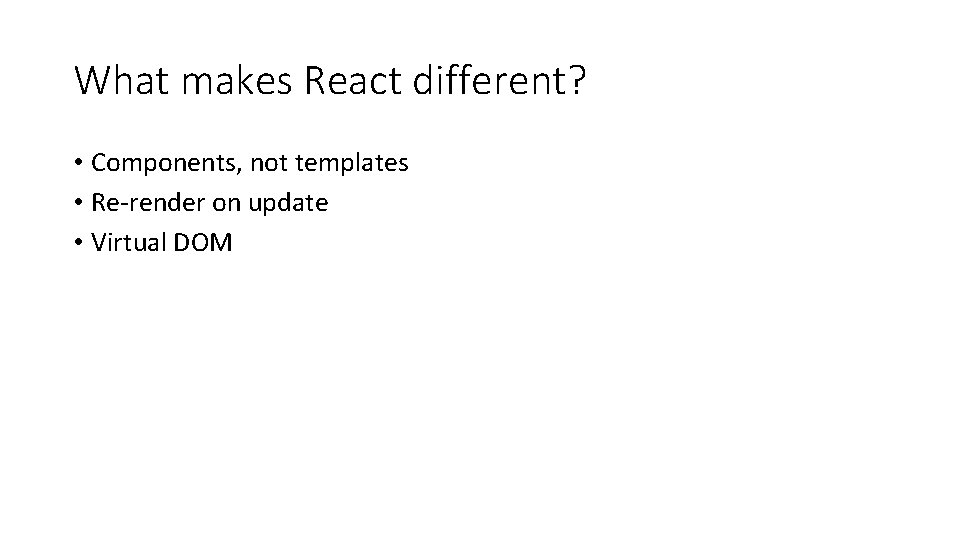
What makes React different? • Components, not templates • Re-render on update • Virtual DOM
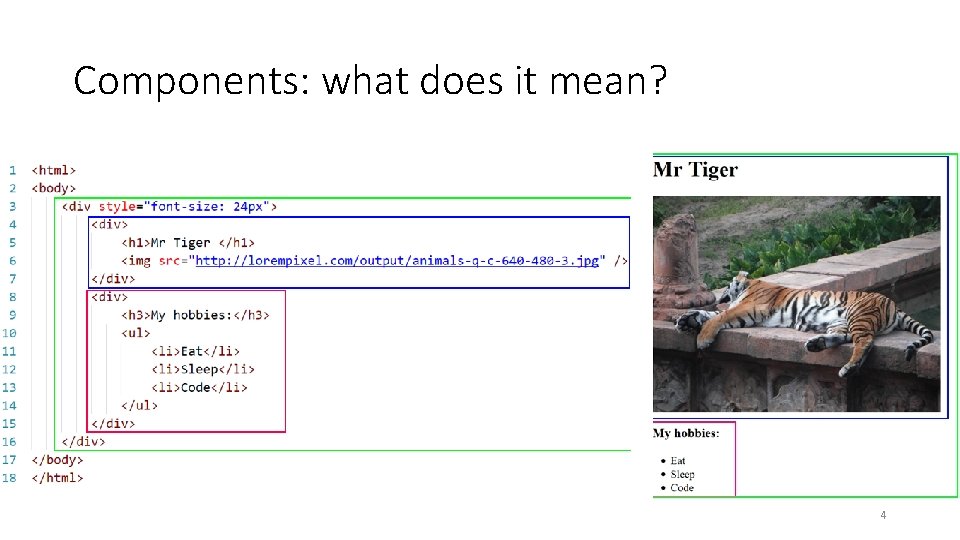
Components: what does it mean? 4
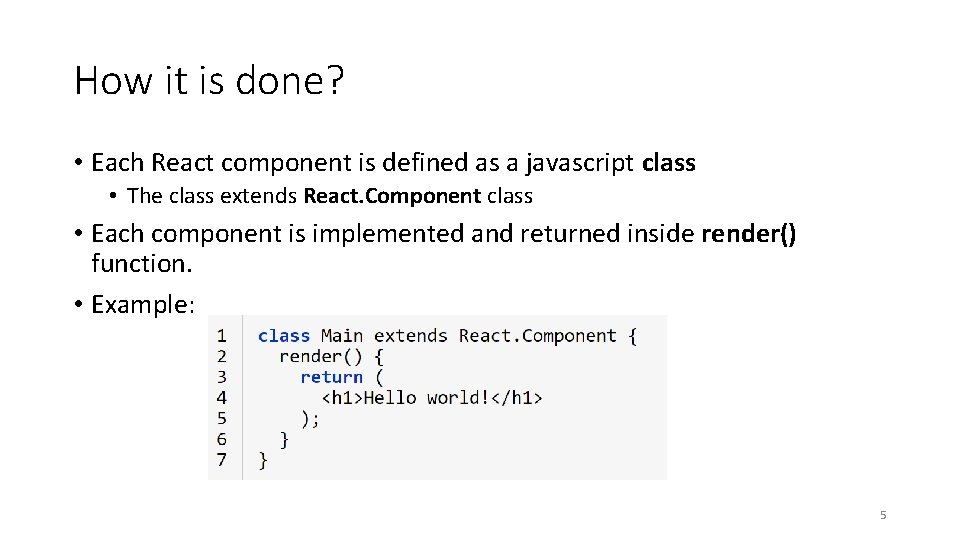
How it is done? • Each React component is defined as a javascript class • The class extends React. Component class • Each component is implemented and returned inside render() function. • Example: 5
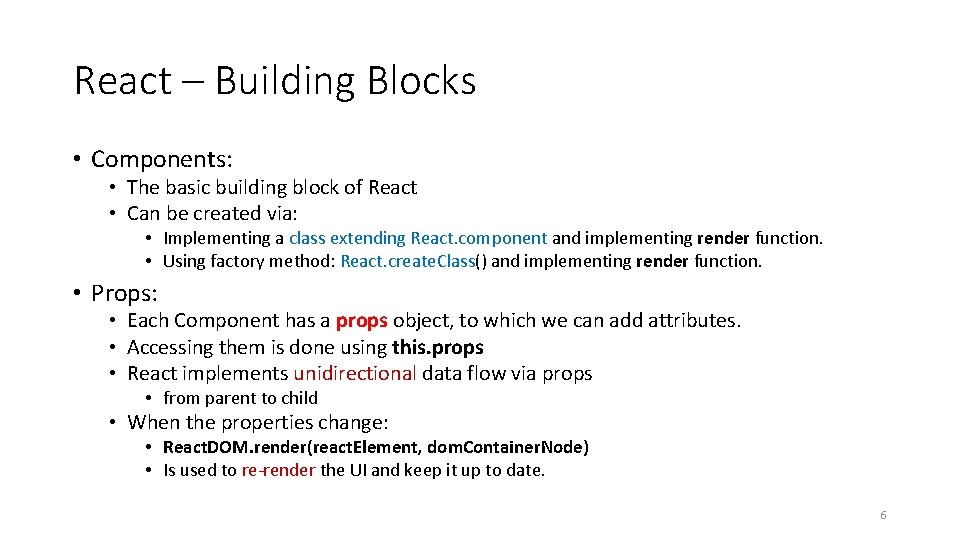
React – Building Blocks • Components: • The basic building block of React • Can be created via: • Implementing a class extending React. component and implementing render function. • Using factory method: React. create. Class() and implementing render function. • Props: • Each Component has a props object, to which we can add attributes. • Accessing them is done using this. props • React implements unidirectional data flow via props • from parent to child • When the properties change: • React. DOM. render(react. Element, dom. Container. Node) • Is used to re-render the UI and keep it up to date. 6
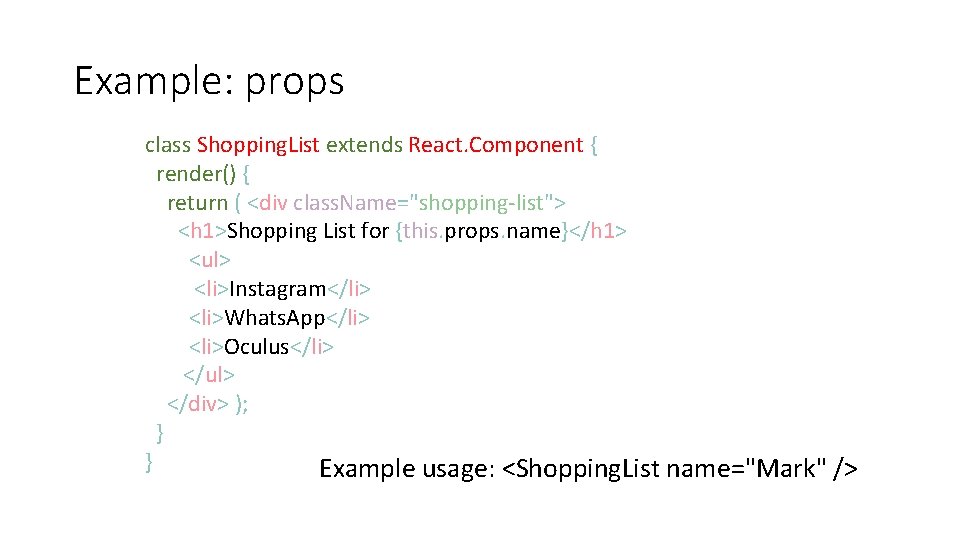
Example: props class Shopping. List extends React. Component { render() { return ( <div class. Name="shopping-list"> <h 1>Shopping List for {this. props. name}</h 1> <ul> <li>Instagram</li> <li>Whats. App</li> <li>Oculus</li> </ul> </div> ); } } Example usage: <Shopping. List name="Mark" />
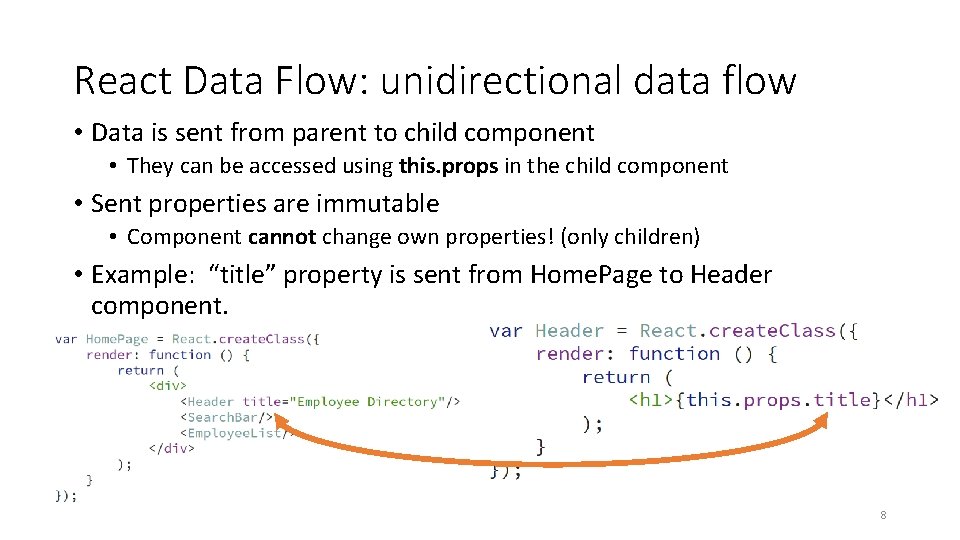
React Data Flow: unidirectional data flow • Data is sent from parent to child component • They can be accessed using this. props in the child component • Sent properties are immutable • Component cannot change own properties! (only children) • Example: “title” property is sent from Home. Page to Header component. 8
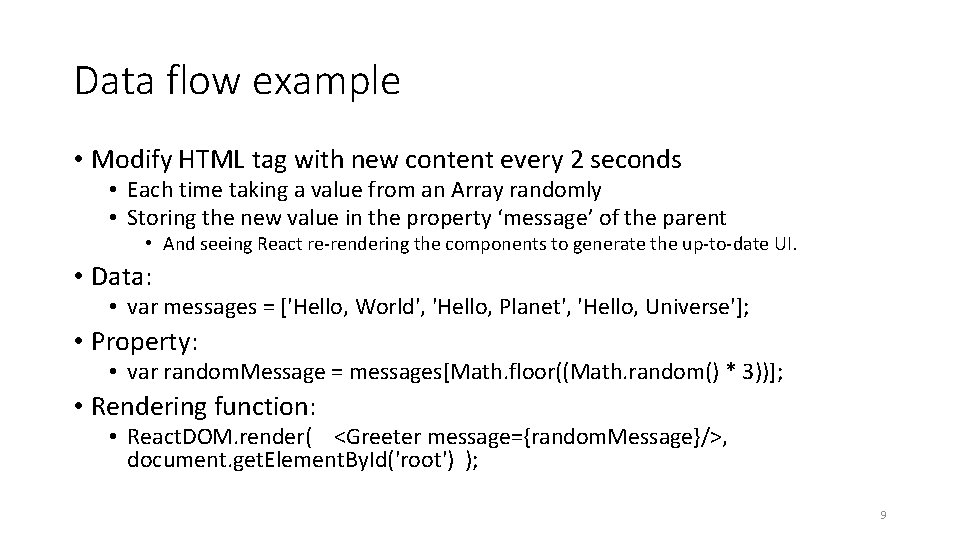
Data flow example • Modify HTML tag with new content every 2 seconds • Each time taking a value from an Array randomly • Storing the new value in the property ‘message’ of the parent • And seeing React re-rendering the components to generate the up-to-date UI. • Data: • var messages = ['Hello, World', 'Hello, Planet', 'Hello, Universe']; • Property: • var random. Message = messages[Math. floor((Math. random() * 3))]; • Rendering function: • React. DOM. render( <Greeter message={random. Message}/>, document. get. Element. By. Id('root') ); 9
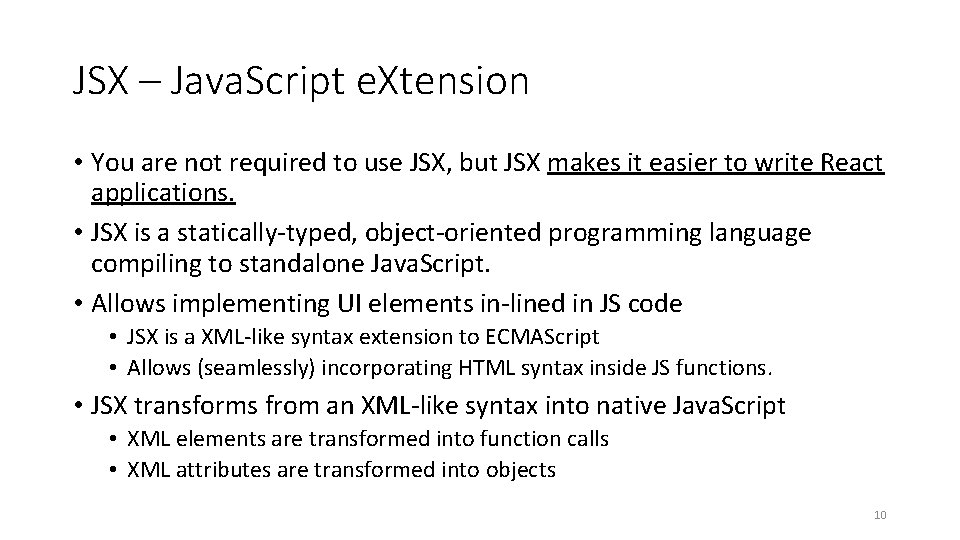
JSX – Java. Script e. Xtension • You are not required to use JSX, but JSX makes it easier to write React applications. • JSX is a statically-typed, object-oriented programming language compiling to standalone Java. Script. • Allows implementing UI elements in-lined in JS code • JSX is a XML-like syntax extension to ECMAScript • Allows (seamlessly) incorporating HTML syntax inside JS functions. • JSX transforms from an XML-like syntax into native Java. Script • XML elements are transformed into function calls • XML attributes are transformed into objects 10
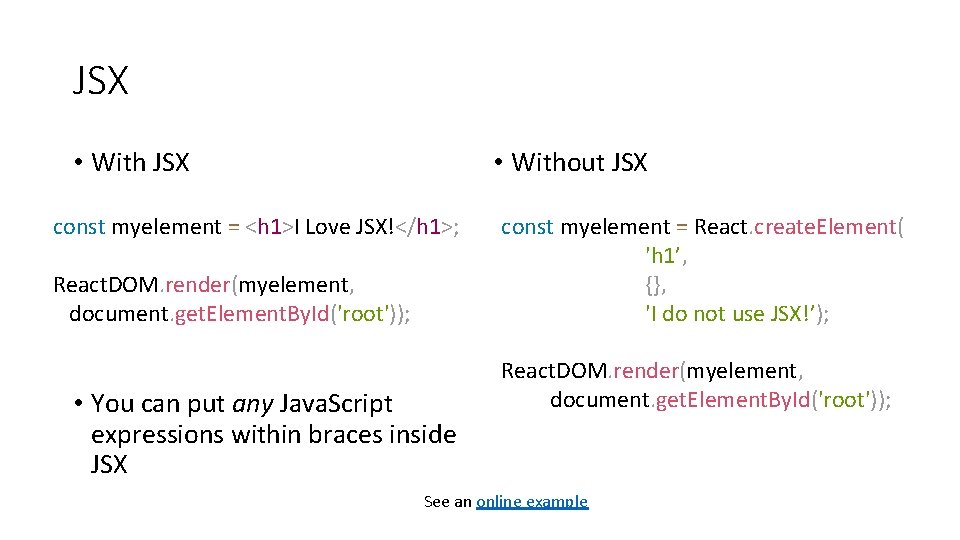
JSX • With JSX • Without JSX const myelement = <h 1>I Love JSX!</h 1>; React. DOM. render(myelement, document. get. Element. By. Id('root')); • You can put any Java. Script expressions within braces inside JSX const myelement = React. create. Element( 'h 1’, {}, 'I do not use JSX!’); React. DOM. render(myelement, document. get. Element. By. Id('root')); See an online example
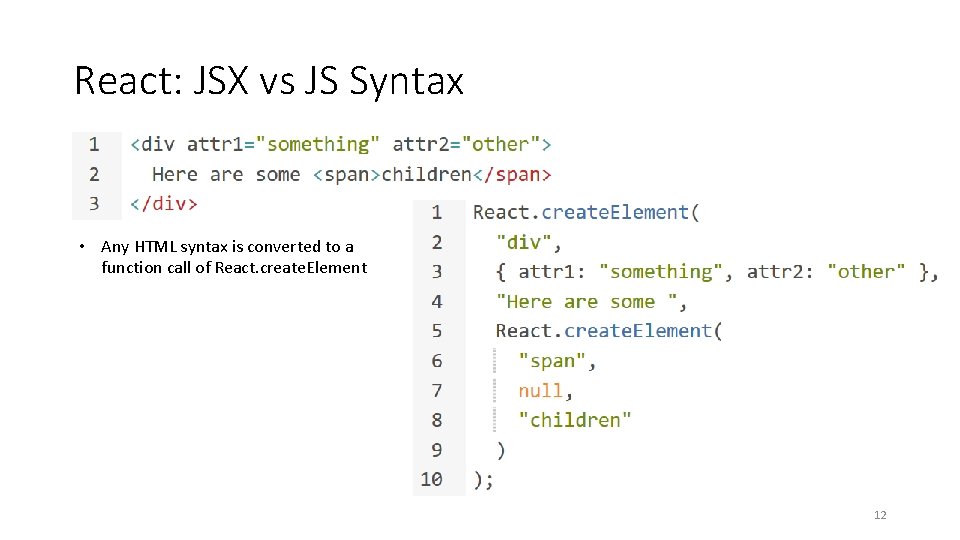
React: JSX vs JS Syntax • Any HTML syntax is converted to a function call of React. create. Element 12
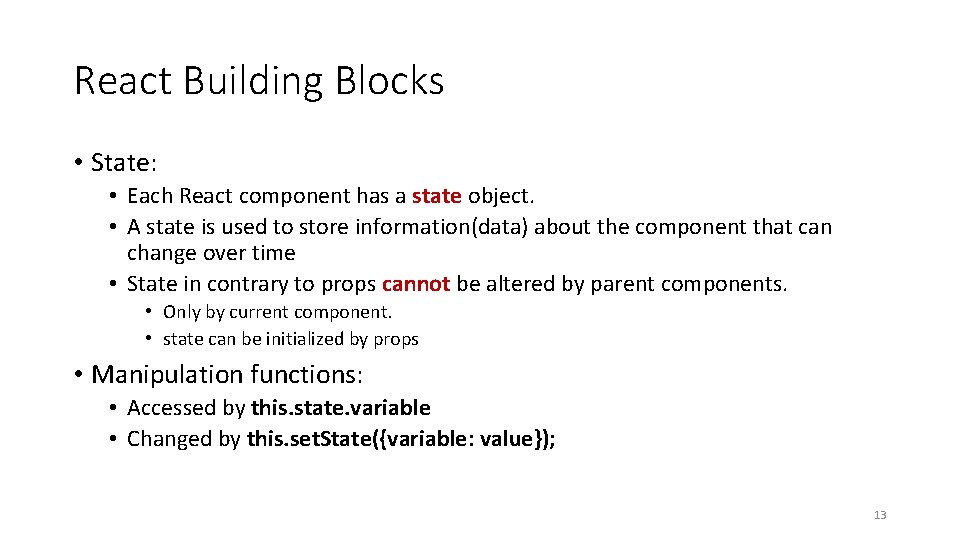
React Building Blocks • State: • Each React component has a state object. • A state is used to store information(data) about the component that can change over time • State in contrary to props cannot be altered by parent components. • Only by current component. • state can be initialized by props • Manipulation functions: • Accessed by this. state. variable • Changed by this. set. State({variable: value}); 13
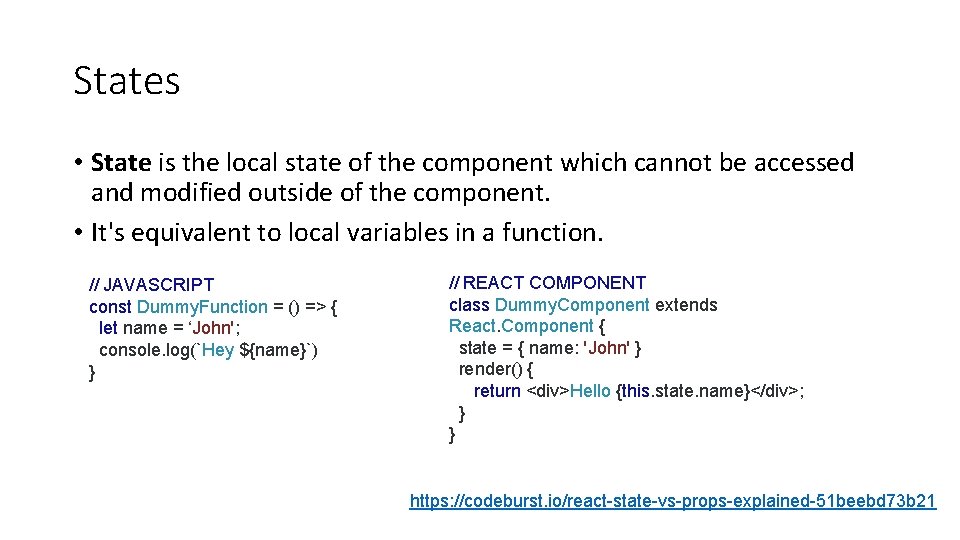
States • State is the local state of the component which cannot be accessed and modified outside of the component. • It's equivalent to local variables in a function. // JAVASCRIPT const Dummy. Function = () => { let name = ‘John'; console. log(`Hey ${name}`) } // REACT COMPONENT class Dummy. Component extends React. Component { state = { name: 'John' } render() { return <div>Hello {this. state. name}</div>; } } https: //codeburst. io/react-state-vs-props-explained-51 beebd 73 b 21
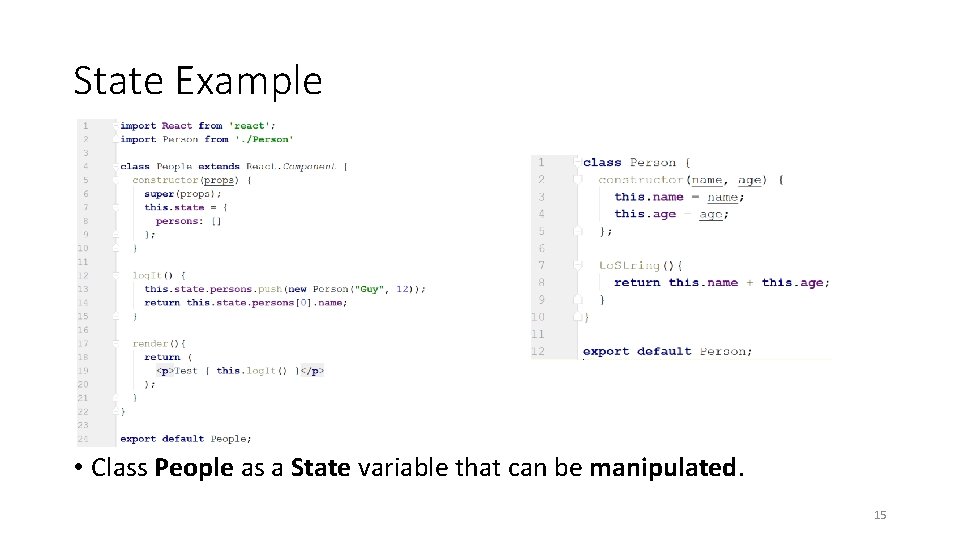
State Example • Class People as a State variable that can be manipulated. 15
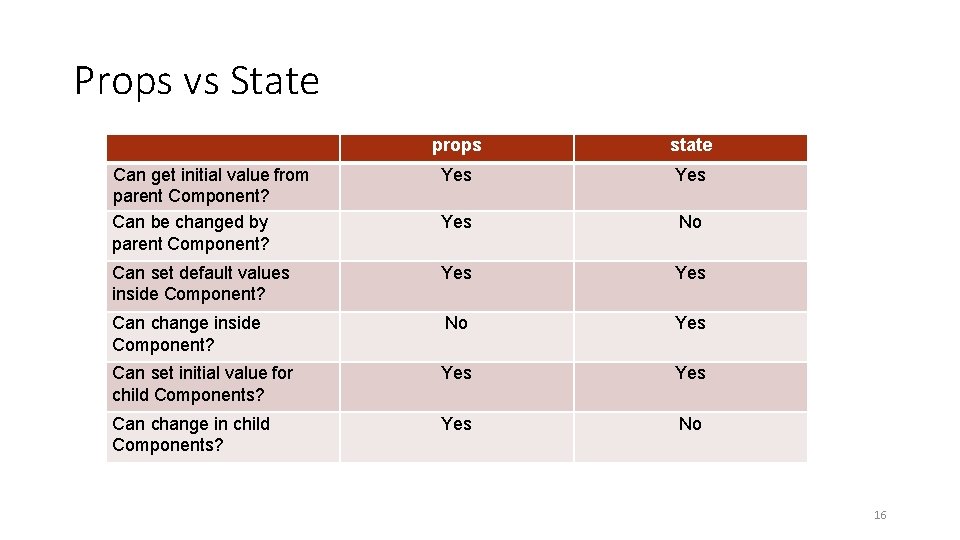
Props vs State props state Can get initial value from parent Component? Can be changed by parent Component? Yes Yes No Can set default values inside Component? Yes Can change inside Component? No Yes Can set initial value for child Components? Yes Can change in child Components? Yes No 16
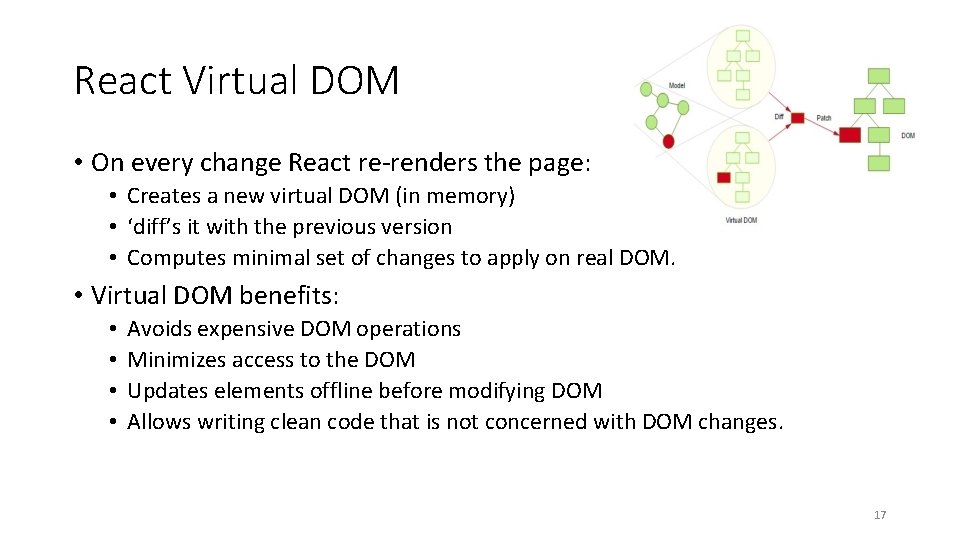
React Virtual DOM • On every change React re-renders the page: • Creates a new virtual DOM (in memory) • ‘diff’s it with the previous version • Computes minimal set of changes to apply on real DOM. • Virtual DOM benefits: • • Avoids expensive DOM operations Minimizes access to the DOM Updates elements offline before modifying DOM Allows writing clean code that is not concerned with DOM changes. 17
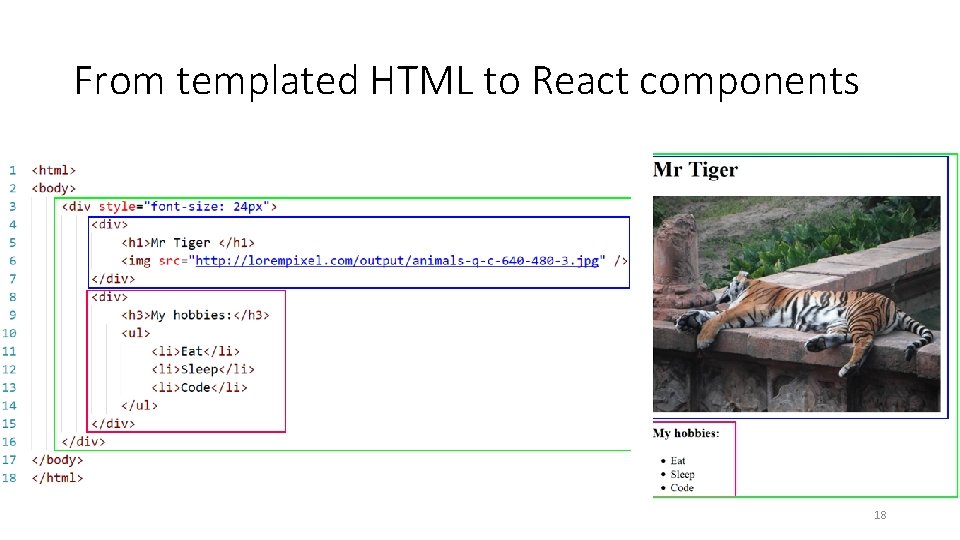
From templated HTML to React components 18
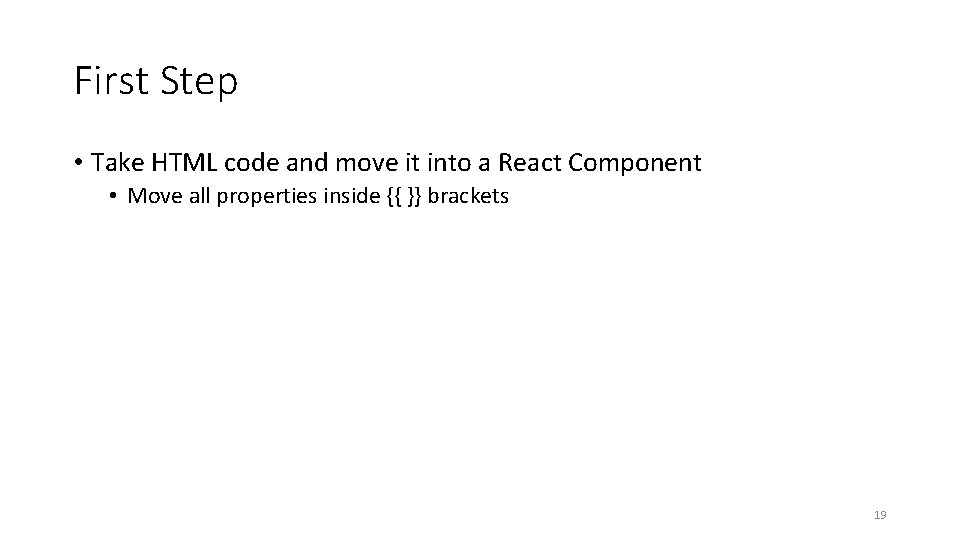
First Step • Take HTML code and move it into a React Component • Move all properties inside {{ }} brackets 19
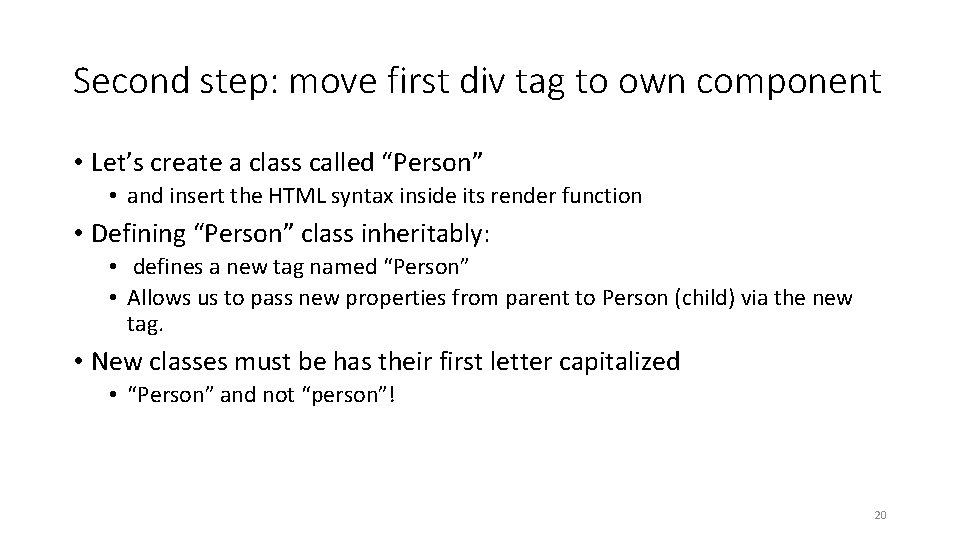
Second step: move first div tag to own component • Let’s create a class called “Person” • and insert the HTML syntax inside its render function • Defining “Person” class inheritably: • defines a new tag named “Person” • Allows us to pass new properties from parent to Person (child) via the new tag. • New classes must be has their first letter capitalized • “Person” and not “person”! 20
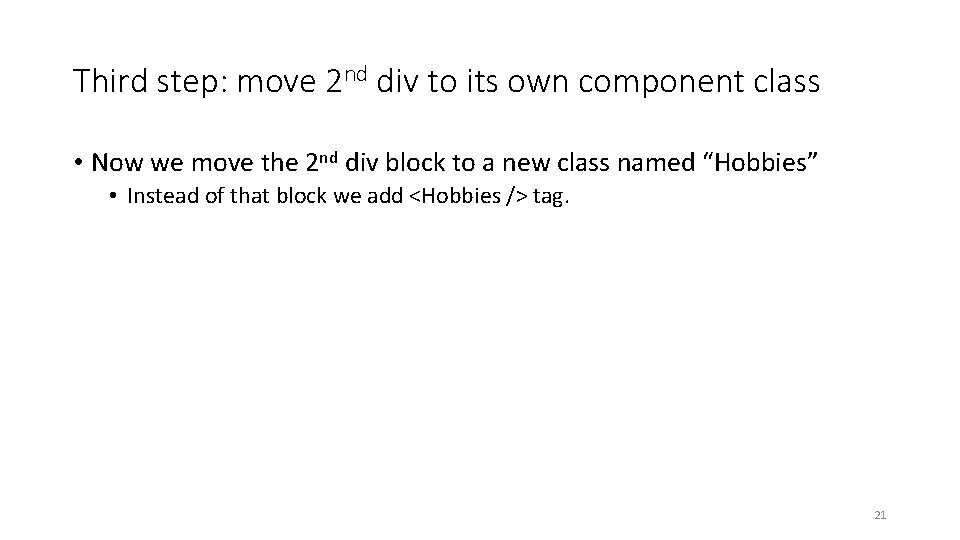
Third step: move 2 nd div to its own component class • Now we move the 2 nd div block to a new class named “Hobbies” • Instead of that block we add <Hobbies /> tag. 21
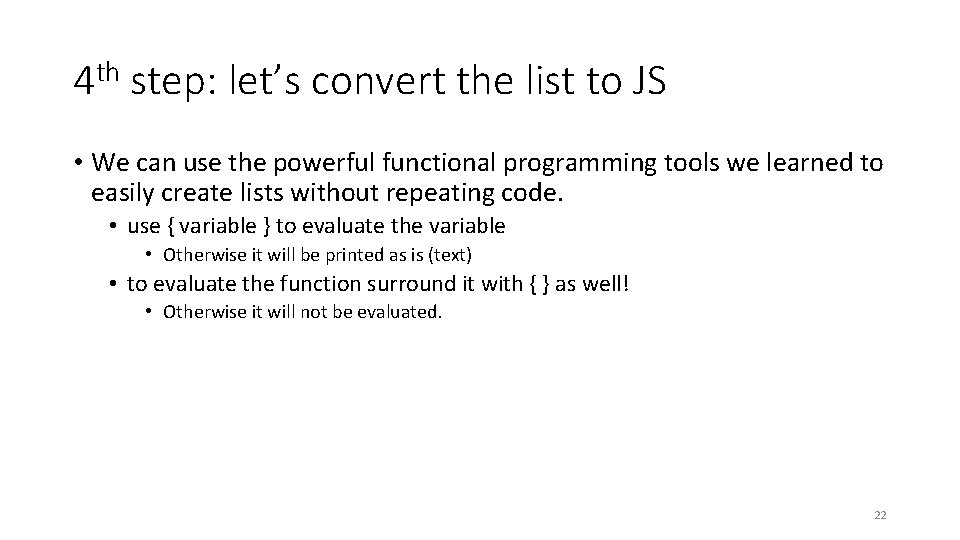
4 th step: let’s convert the list to JS • We can use the powerful functional programming tools we learned to easily create lists without repeating code. • use { variable } to evaluate the variable • Otherwise it will be printed as is (text) • to evaluate the function surround it with { } as well! • Otherwise it will not be evaluated. 22
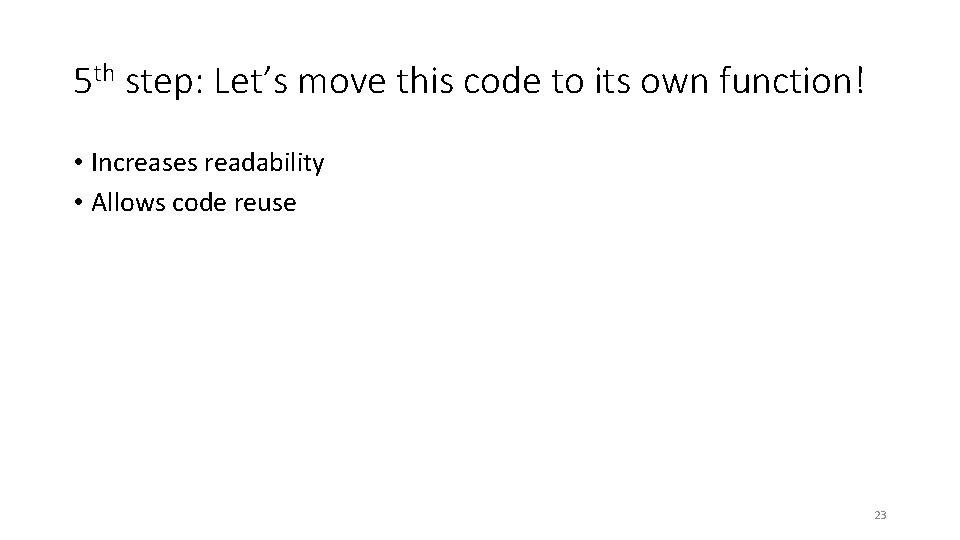
5 th step: Let’s move this code to its own function! • Increases readability • Allows code reuse 23
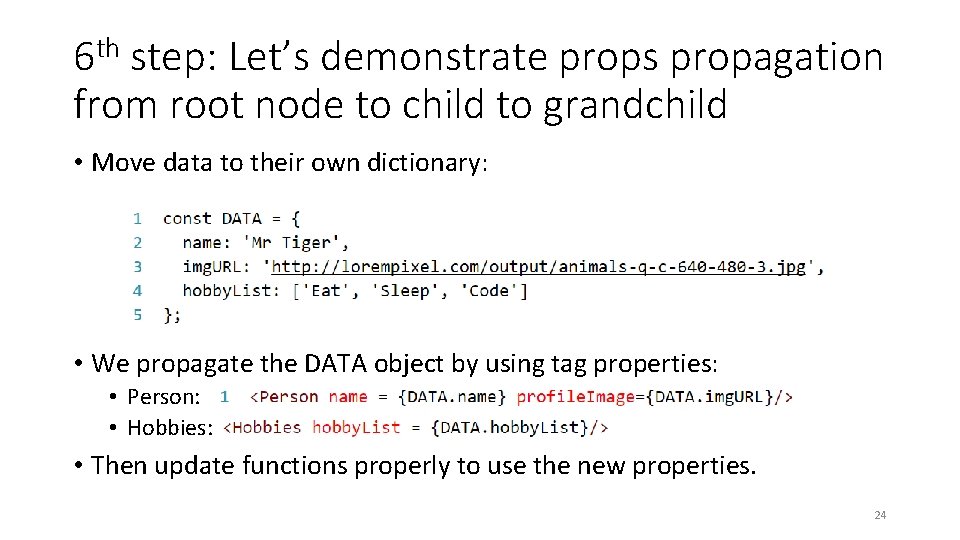
6 th step: Let’s demonstrate props propagation from root node to child to grandchild • Move data to their own dictionary: • We propagate the DATA object by using tag properties: • Person: • Hobbies: • Then update functions properly to use the new properties. 24
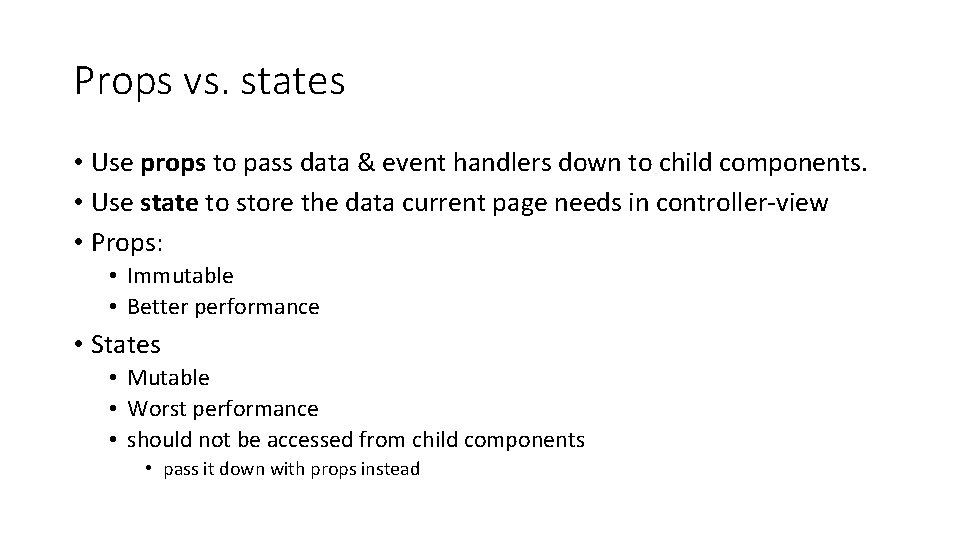
Props vs. states • Use props to pass data & event handlers down to child components. • Use state to store the data current page needs in controller-view • Props: • Immutable • Better performance • States • Mutable • Worst performance • should not be accessed from child components • pass it down with props instead
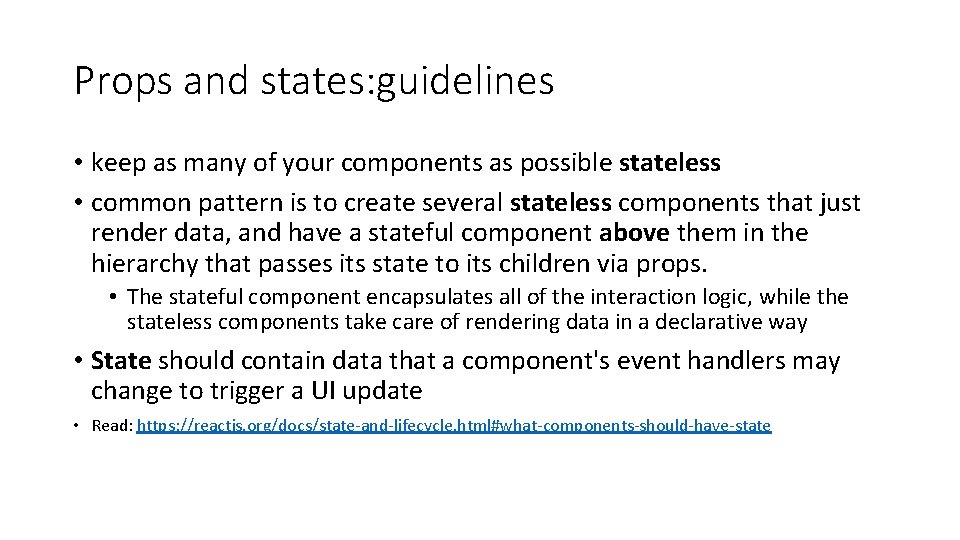
Props and states: guidelines • keep as many of your components as possible stateless • common pattern is to create several stateless components that just render data, and have a stateful component above them in the hierarchy that passes its state to its children via props. • The stateful component encapsulates all of the interaction logic, while the stateless components take care of rendering data in a declarative way • State should contain data that a component's event handlers may change to trigger a UI update • Read: https: //reactjs. org/docs/state-and-lifecycle. html#what-components-should-have-state
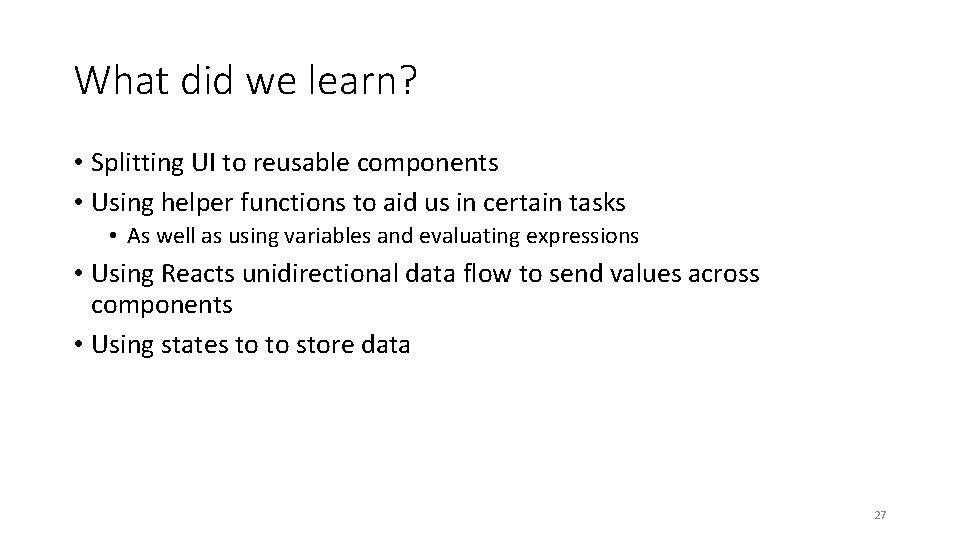
What did we learn? • Splitting UI to reusable components • Using helper functions to aid us in certain tasks • As well as using variables and evaluating expressions • Using Reacts unidirectional data flow to send values across components • Using states to to store data 27
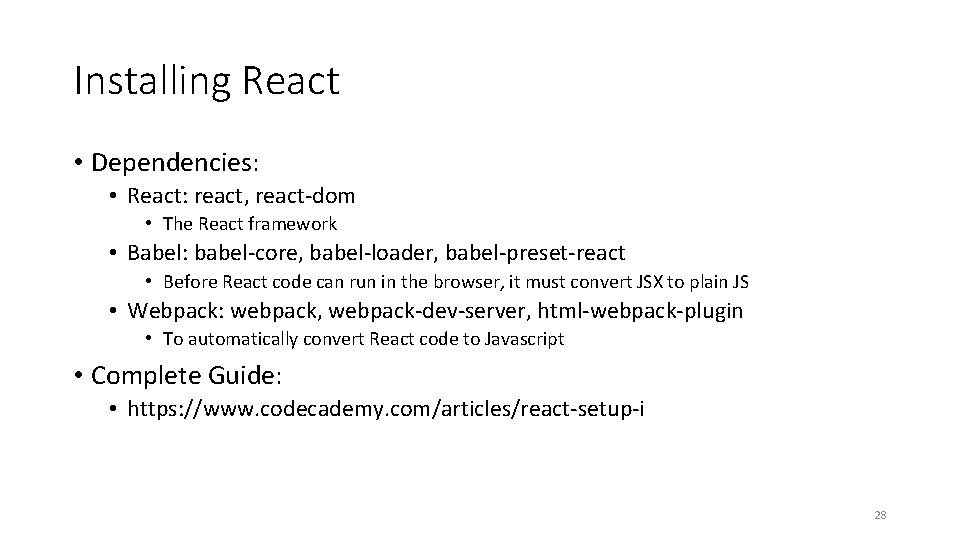
Installing React • Dependencies: • React: react, react-dom • The React framework • Babel: babel-core, babel-loader, babel-preset-react • Before React code can run in the browser, it must convert JSX to plain JS • Webpack: webpack, webpack-dev-server, html-webpack-plugin • To automatically convert React code to Javascript • Complete Guide: • https: //www. codecademy. com/articles/react-setup-i 28
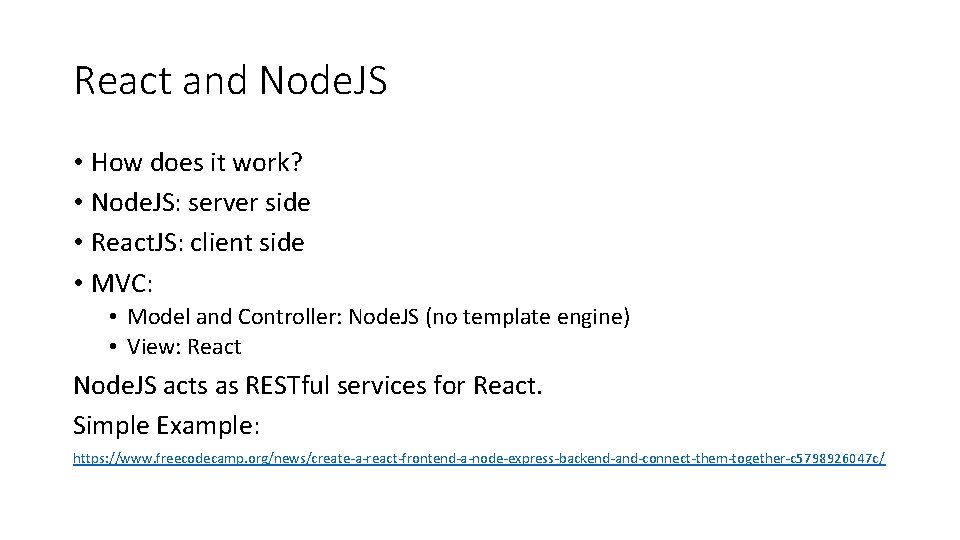
React and Node. JS • How does it work? • Node. JS: server side • React. JS: client side • MVC: • Model and Controller: Node. JS (no template engine) • View: React Node. JS acts as RESTful services for React. Simple Example: https: //www. freecodecamp. org/news/create-a-react-frontend-a-node-express-backend-and-connect-them-together-c 5798926047 c/
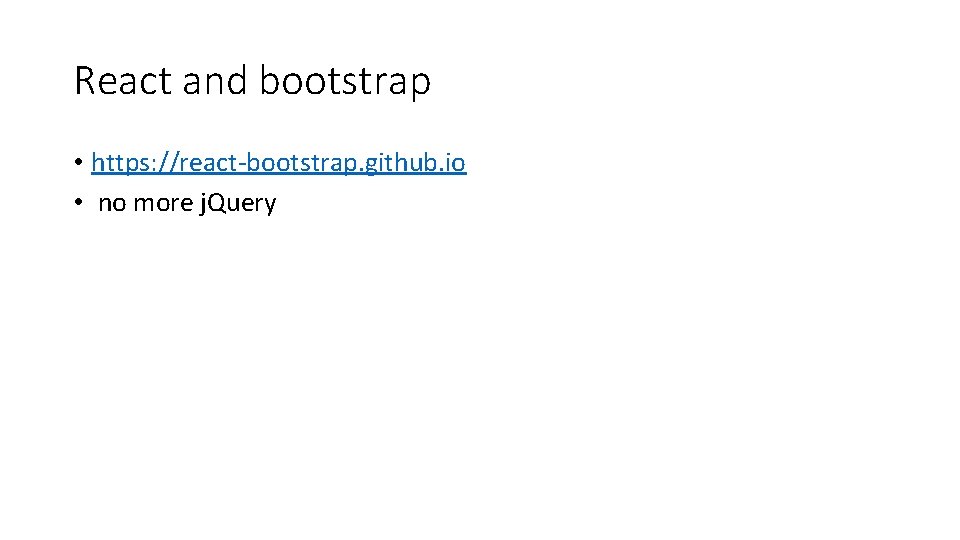
React and bootstrap • https: //react-bootstrap. github. io • no more j. Query
React traversy media
Sailor course brick
Course title and course number
Course interne moyenne externe
Internet engineering course
Internet or internet
Natural environment in marketing
Phenol and bromine
Daphne ovid
Great gatsby activities
Signals that tell your mind and body how to react.
React-native-animatable
How is janie able to tolerate her relationship
Firms react to unplanned inventory reductions by
Beryllium oxide reaction with water
React to indirect fire
Oedipus rex episode 2 summary
When does james gatz change his name why
Copper oxide hydrogen
Metals react with nonmetals to form ionic compounds by
What is king creon’s reaction to teiresias’ message?
Haloform structure
How does myrtle react to tom's arrival?
Act iv scene iv romeo and juliet
How did the northerners react to the novel?
How does ralph react when a boar comes charging
What condition threatens peeta's life?
Solid
React to cbrn attack
Eli mufisovski
R.e.a.c.t. task force