React Native Lists 1 Overview React Native provides
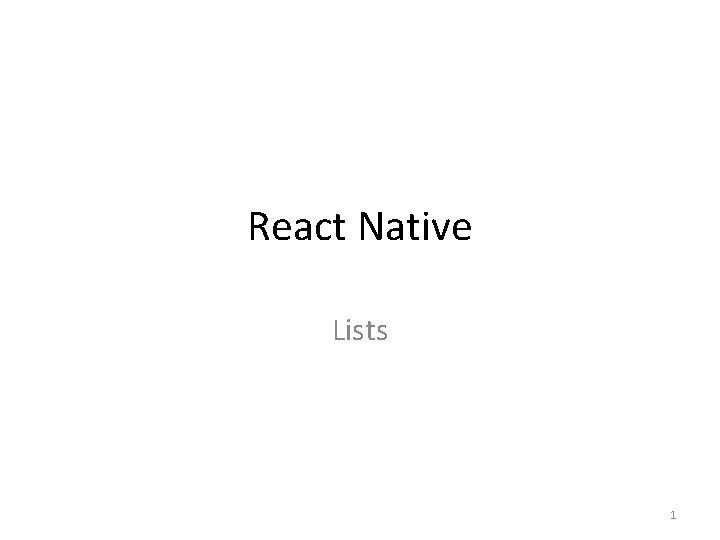
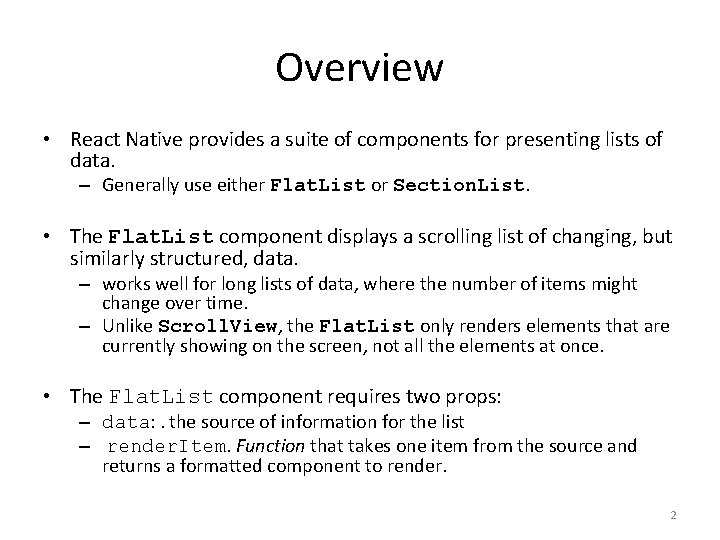
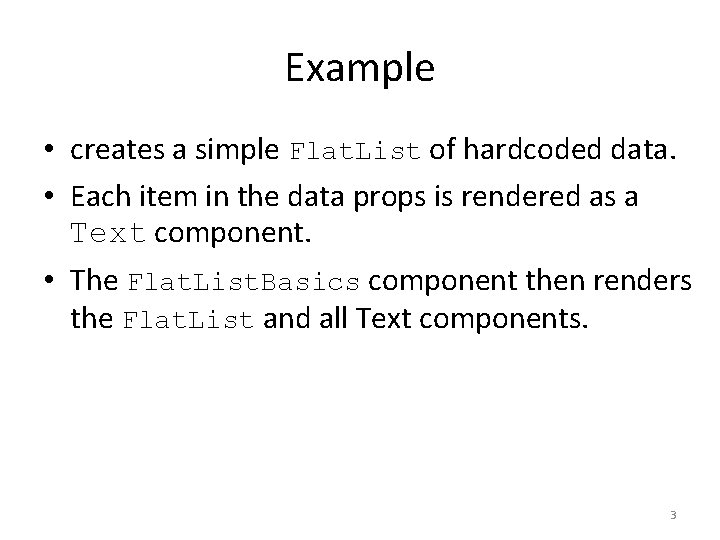
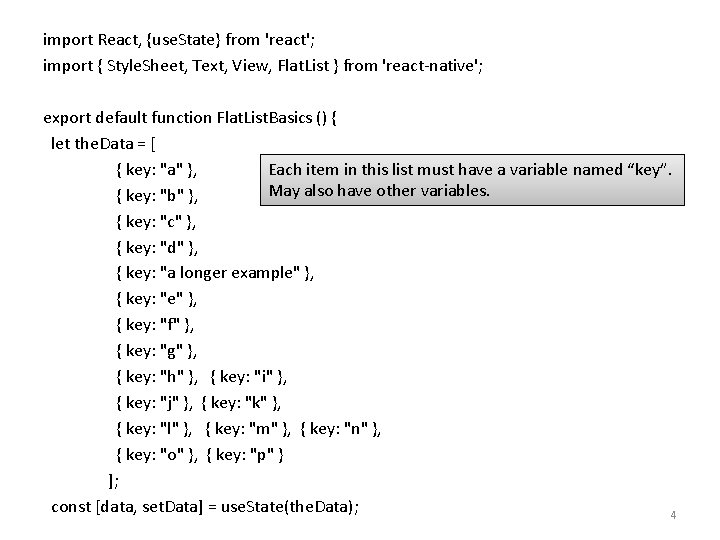
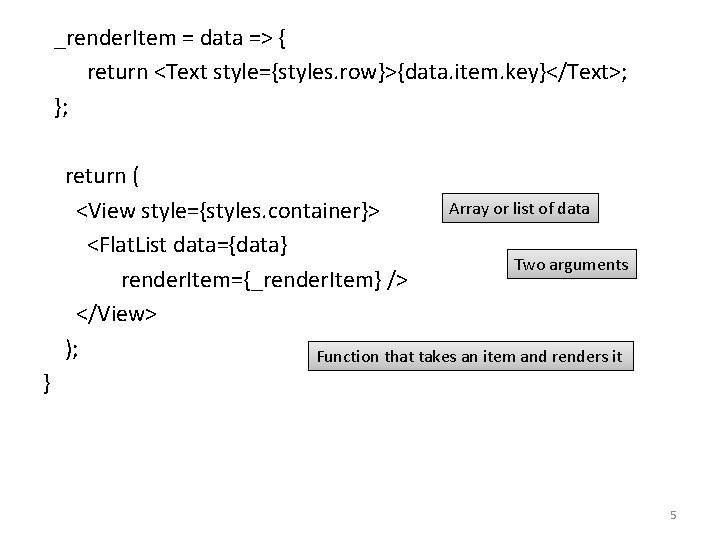
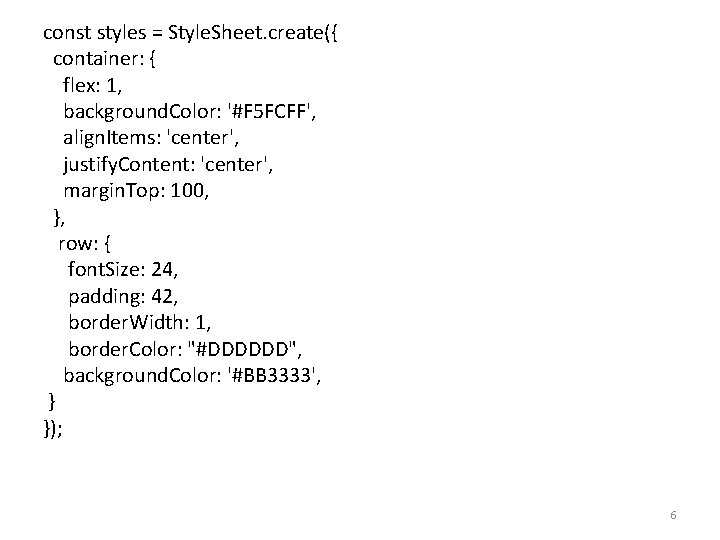
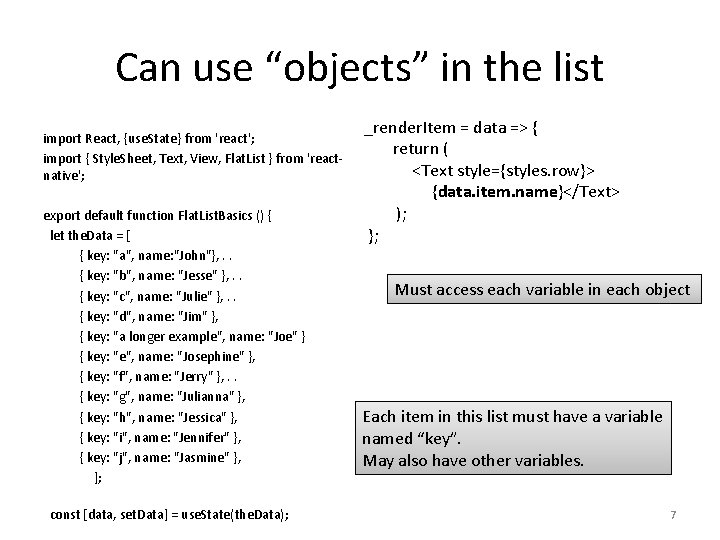
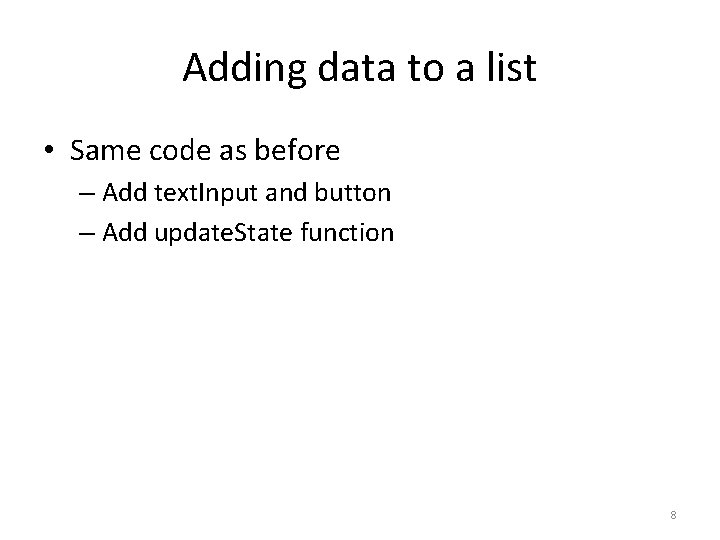
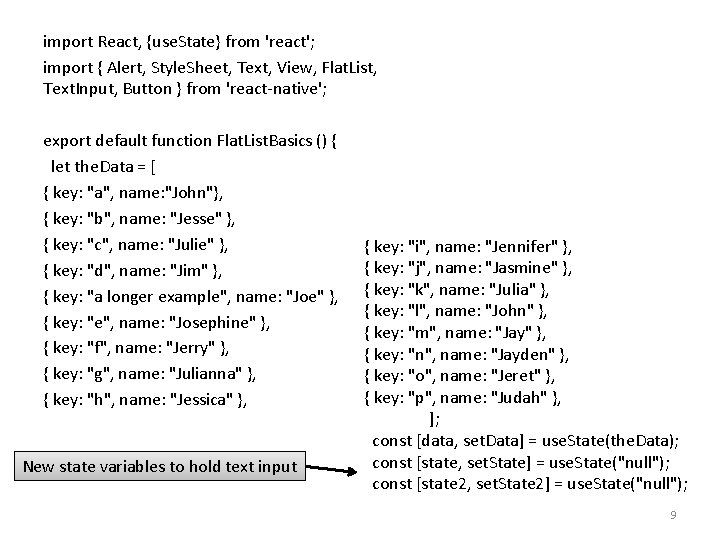
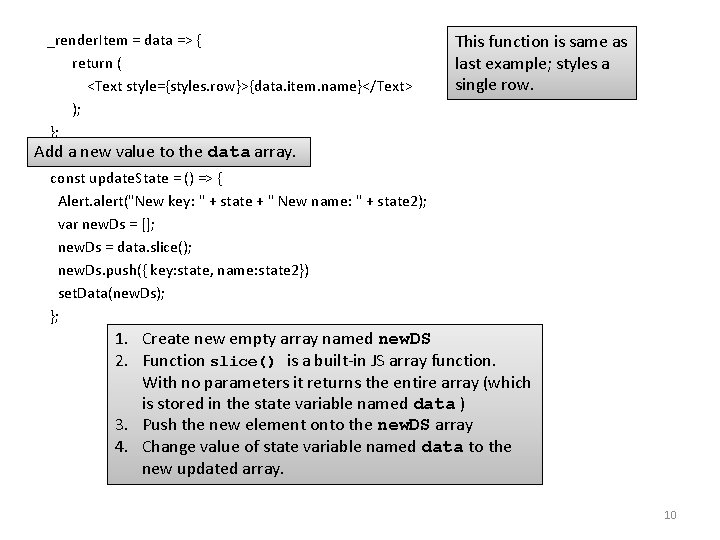
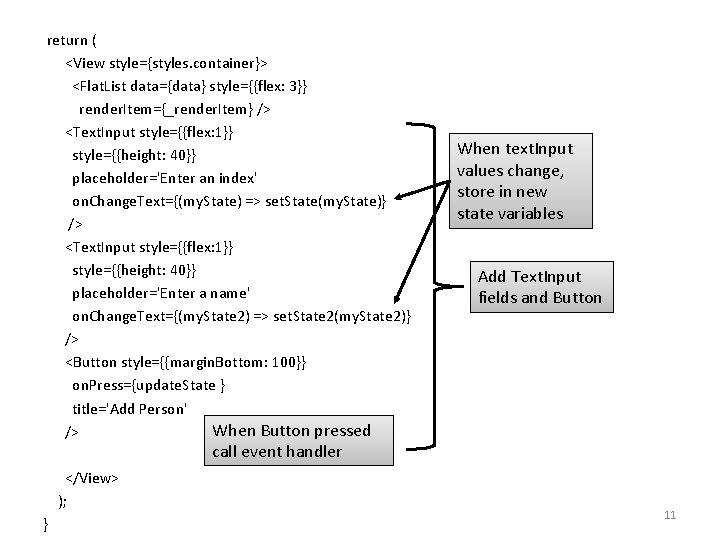
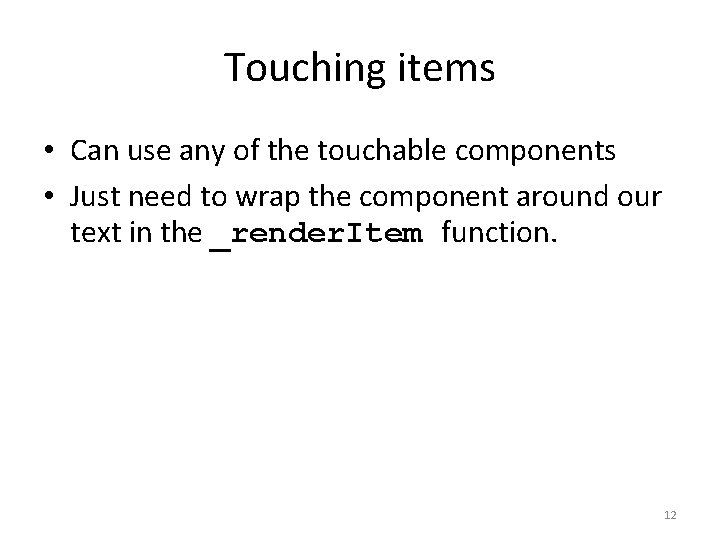
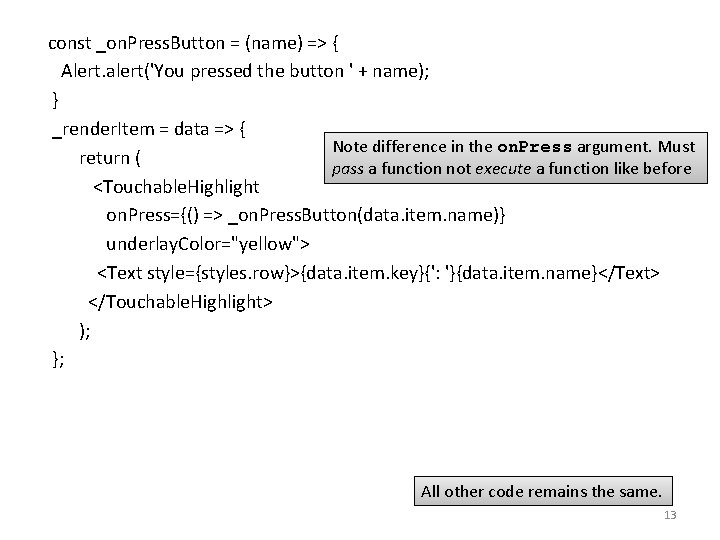
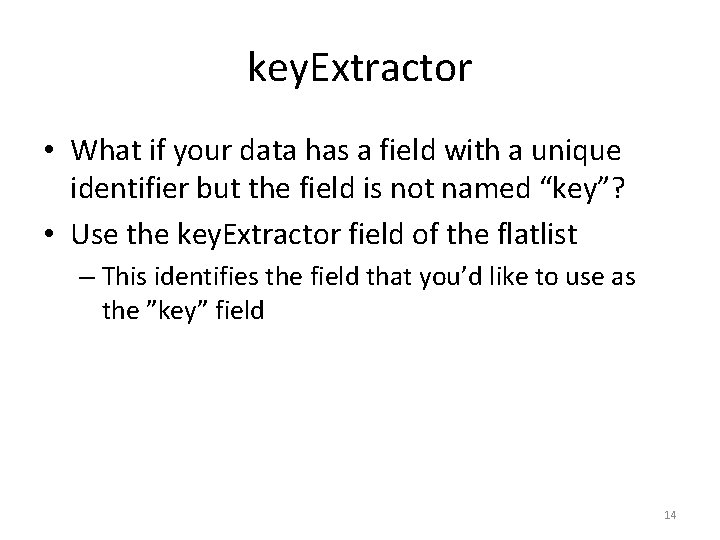
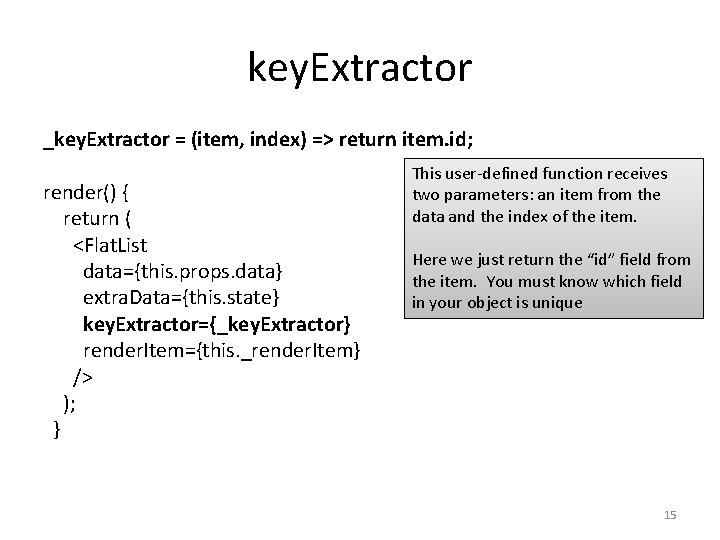
- Slides: 15
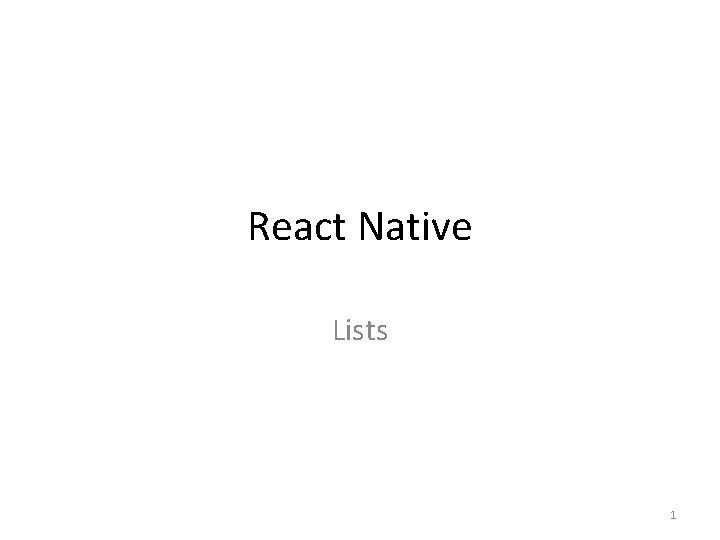
React Native Lists 1
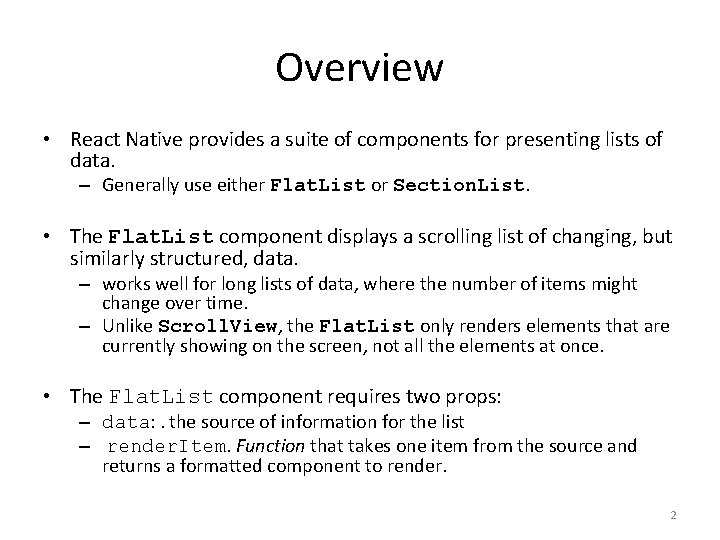
Overview • React Native provides a suite of components for presenting lists of data. – Generally use either Flat. List or Section. List. • The Flat. List component displays a scrolling list of changing, but similarly structured, data. – works well for long lists of data, where the number of items might change over time. – Unlike Scroll. View, the Flat. List only renders elements that are currently showing on the screen, not all the elements at once. • The Flat. List component requires two props: – data: . the source of information for the list – render. Item. Function that takes one item from the source and returns a formatted component to render. 2
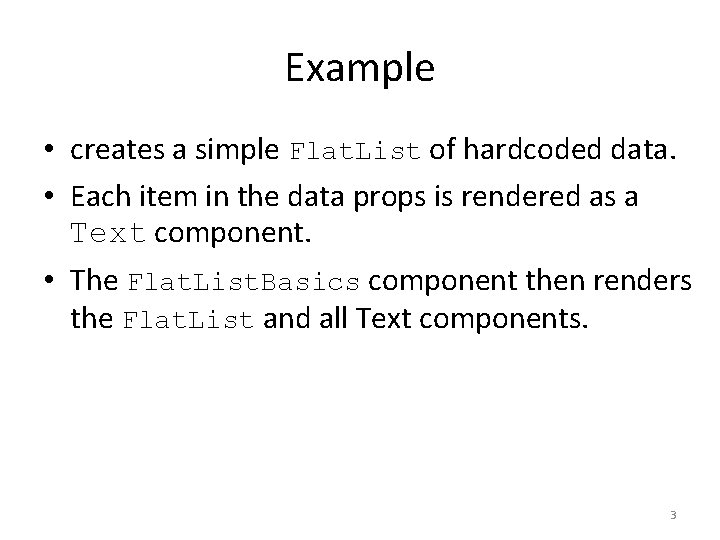
Example • creates a simple Flat. List of hardcoded data. • Each item in the data props is rendered as a Text component. • The Flat. List. Basics component then renders the Flat. List and all Text components. 3
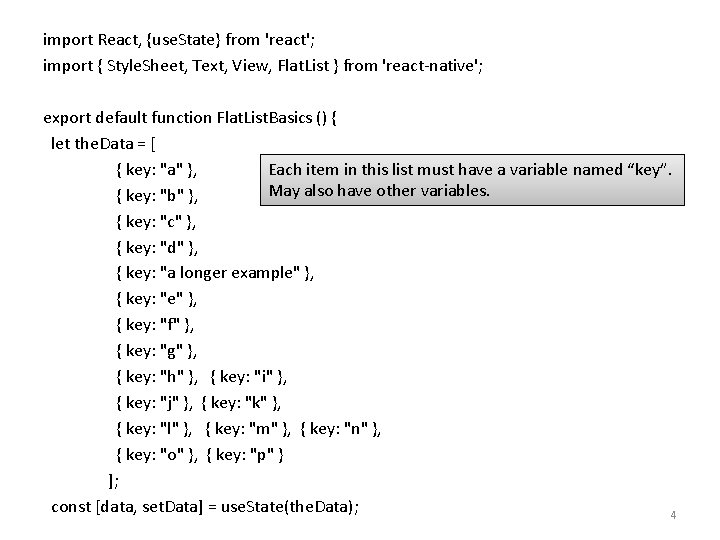
import React, {use. State} from 'react'; import { Style. Sheet, Text, View, Flat. List } from 'react-native'; export default function Flat. List. Basics () { let the. Data = [ { key: "a" }, Each item in this list must have a variable named “key”. May also have other variables. { key: "b" }, { key: "c" }, { key: "d" }, { key: "a longer example" }, { key: "f" }, { key: "g" }, { key: "h" }, { key: "i" }, { key: "j" }, { key: "k" }, { key: "l" }, { key: "m" }, { key: "n" }, { key: "o" }, { key: "p" } ]; const [data, set. Data] = use. State(the. Data); 4
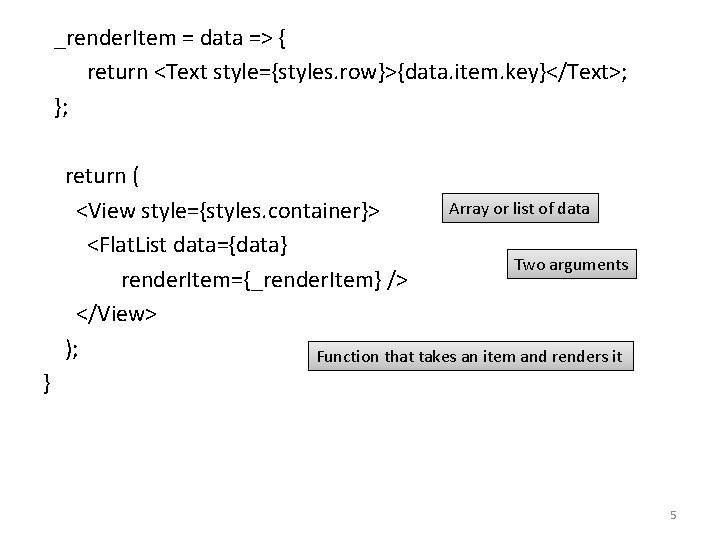
_render. Item = data => { return <Text style={styles. row}>{data. item. key}</Text>; }; return ( Array or list of data <View style={styles. container}> <Flat. List data={data} Two arguments render. Item={_render. Item} /> </View> ); Function that takes an item and renders it } 5
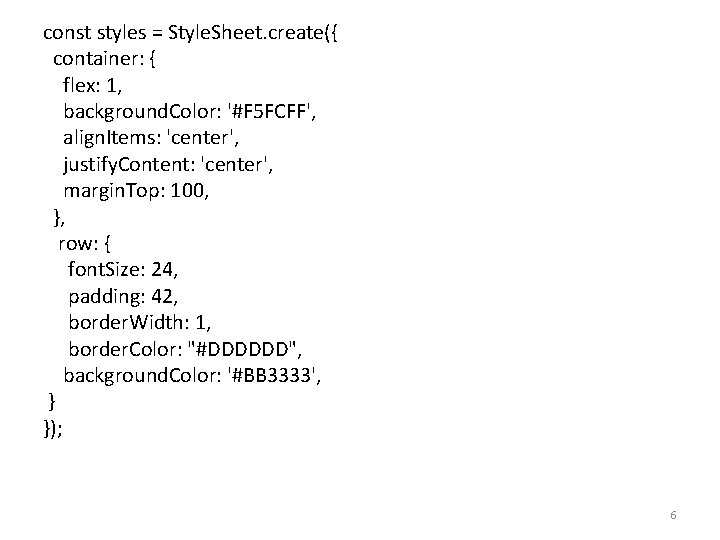
const styles = Style. Sheet. create({ container: { flex: 1, background. Color: '#F 5 FCFF', align. Items: 'center', justify. Content: 'center', margin. Top: 100, }, row: { font. Size: 24, padding: 42, border. Width: 1, border. Color: "#DDDDDD", background. Color: '#BB 3333', } }); 6
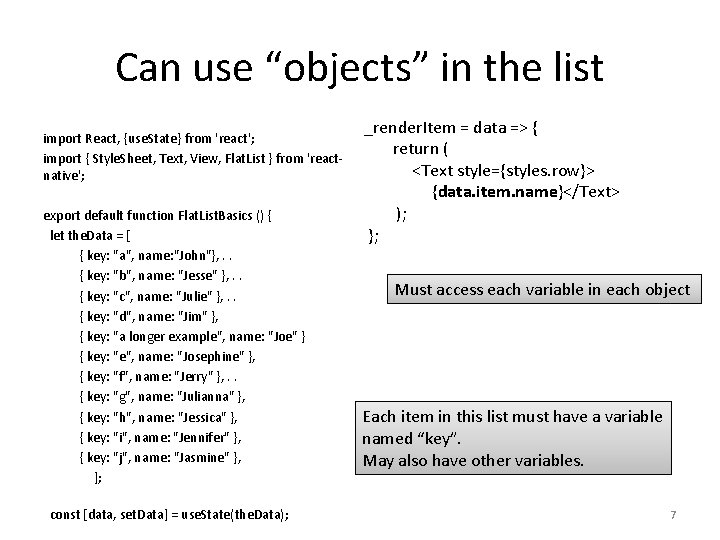
Can use “objects” in the list import React, {use. State} from 'react'; import { Style. Sheet, Text, View, Flat. List } from 'reactnative'; export default function Flat. List. Basics () { let the. Data = [ { key: "a", name: "John"}, . . { key: "b", name: "Jesse" }, . . { key: "c", name: "Julie" }, . . { key: "d", name: "Jim" }, { key: "a longer example", name: "Joe" } { key: "e", name: "Josephine" }, { key: "f", name: "Jerry" }, . . { key: "g", name: "Julianna" }, { key: "h", name: "Jessica" }, { key: "i", name: "Jennifer" }, { key: "j", name: "Jasmine" }, ]; const [data, set. Data] = use. State(the. Data); _render. Item = data => { return ( <Text style={styles. row}> {data. item. name}</Text> ); }; Must access each variable in each object Each item in this list must have a variable named “key”. May also have other variables. 7
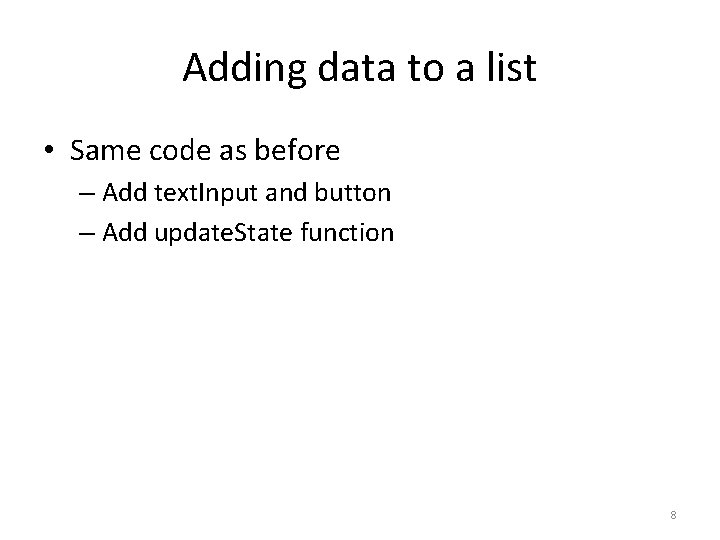
Adding data to a list • Same code as before – Add text. Input and button – Add update. State function 8
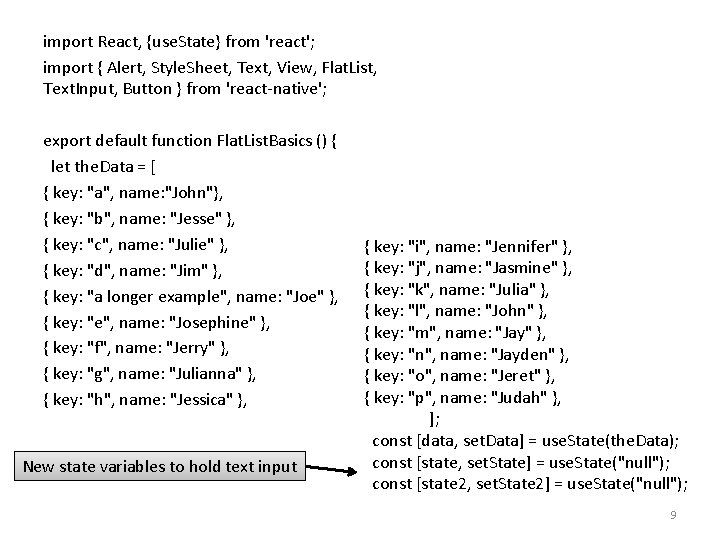
import React, {use. State} from 'react'; import { Alert, Style. Sheet, Text, View, Flat. List, Text. Input, Button } from 'react-native'; export default function Flat. List. Basics () { let the. Data = [ { key: "a", name: "John"}, { key: "b", name: "Jesse" }, { key: "c", name: "Julie" }, { key: "d", name: "Jim" }, { key: "a longer example", name: "Joe" }, { key: "e", name: "Josephine" }, { key: "f", name: "Jerry" }, { key: "g", name: "Julianna" }, { key: "h", name: "Jessica" }, New state variables to hold text input { key: "i", name: "Jennifer" }, { key: "j", name: "Jasmine" }, { key: "k", name: "Julia" }, { key: "l", name: "John" }, { key: "m", name: "Jay" }, { key: "n", name: "Jayden" }, { key: "o", name: "Jeret" }, { key: "p", name: "Judah" }, ]; const [data, set. Data] = use. State(the. Data); const [state, set. State] = use. State("null"); const [state 2, set. State 2] = use. State("null"); 9
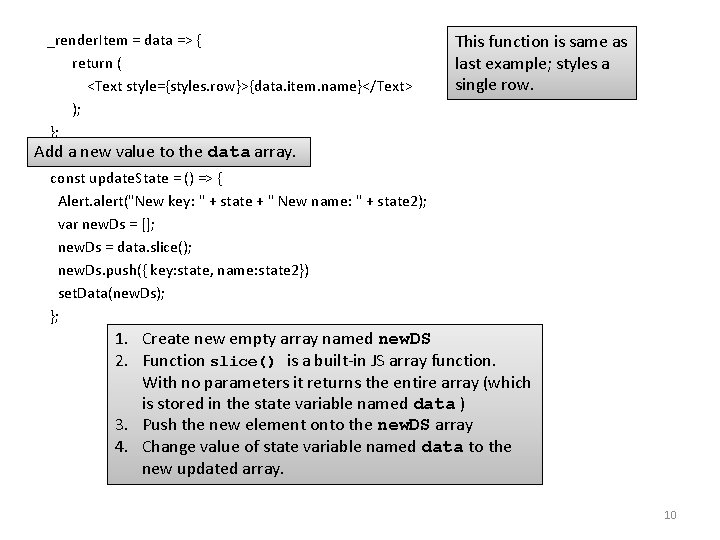
_render. Item = data => { return ( <Text style={styles. row}>{data. item. name}</Text> ); }; This function is same as last example; styles a single row. Add a new value to the data array. const update. State = () => { Alert. alert("New key: " + state + " New name: " + state 2); var new. Ds = []; new. Ds = data. slice(); new. Ds. push({ key: state, name: state 2}) set. Data(new. Ds); }; 1. Create new empty array named new. DS 2. Function slice() is a built-in JS array function. With no parameters it returns the entire array (which is stored in the state variable named data ) 3. Push the new element onto the new. DS array 4. Change value of state variable named data to the new updated array. 10
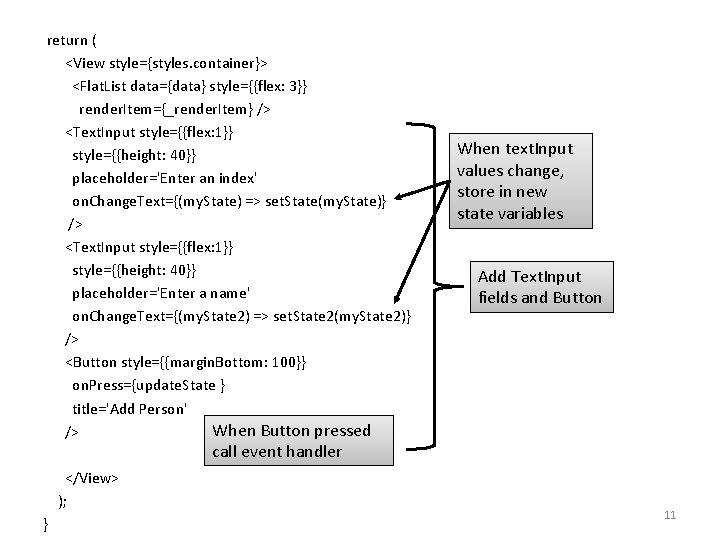
return ( <View style={styles. container}> <Flat. List data={data} style={{flex: 3}} render. Item={_render. Item} /> <Text. Input style={{flex: 1}} style={{height: 40}} placeholder='Enter an index' on. Change. Text={(my. State) => set. State(my. State)} /> <Text. Input style={{flex: 1}} style={{height: 40}} placeholder='Enter a name' on. Change. Text={(my. State 2) => set. State 2(my. State 2)} /> <Button style={{margin. Bottom: 100}} on. Press={update. State } title='Add Person' When Button pressed /> When text. Input values change, store in new state variables Add Text. Input fields and Button call event handler </View> ); } 11
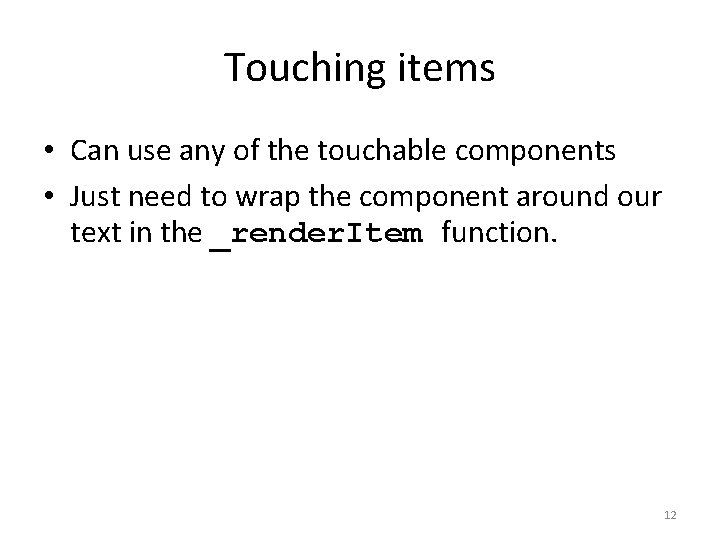
Touching items • Can use any of the touchable components • Just need to wrap the component around our text in the _render. Item function. 12
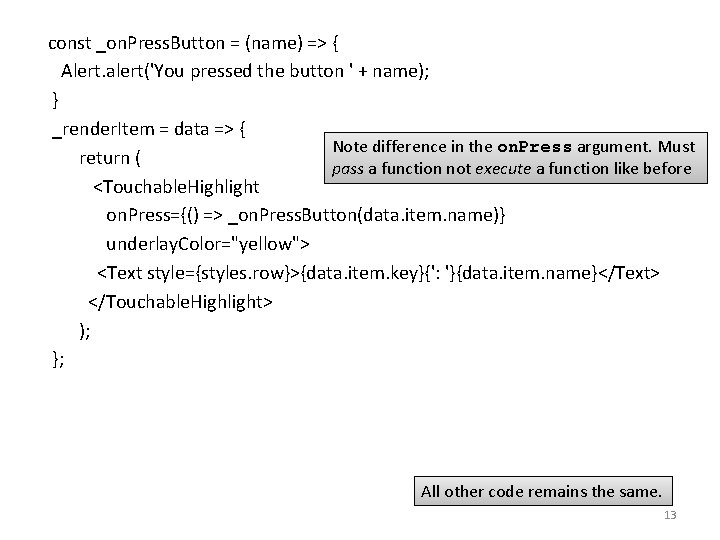
const _on. Press. Button = (name) => { Alert. alert('You pressed the button ' + name); } _render. Item = data => { Note difference in the on. Press argument. Must return ( pass a function not execute a function like before <Touchable. Highlight on. Press={() => _on. Press. Button(data. item. name)} underlay. Color="yellow"> <Text style={styles. row}>{data. item. key}{': '}{data. item. name}</Text> </Touchable. Highlight> ); }; All other code remains the same. 13
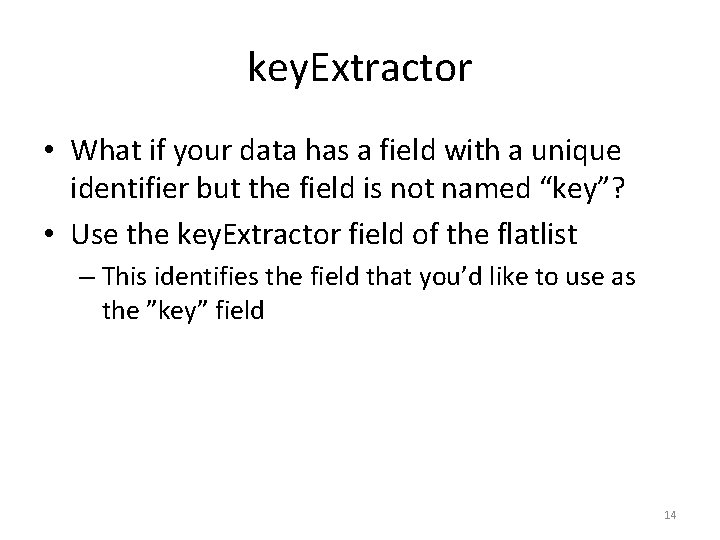
key. Extractor • What if your data has a field with a unique identifier but the field is not named “key”? • Use the key. Extractor field of the flatlist – This identifies the field that you’d like to use as the ”key” field 14
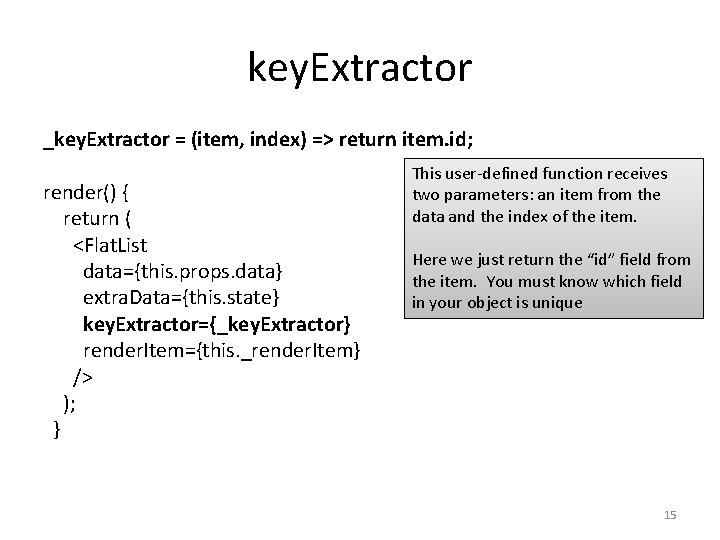
key. Extractor _key. Extractor = (item, index) => return item. id; render() { return ( <Flat. List data={this. props. data} extra. Data={this. state} key. Extractor={_key. Extractor} render. Item={this. _render. Item} /> ); } This user-defined function receives two parameters: an item from the data and the index of the item. Here we just return the “id” field from the item. You must know which field in your object is unique 15