React Native Navigation Screens Navigating React Navigation React
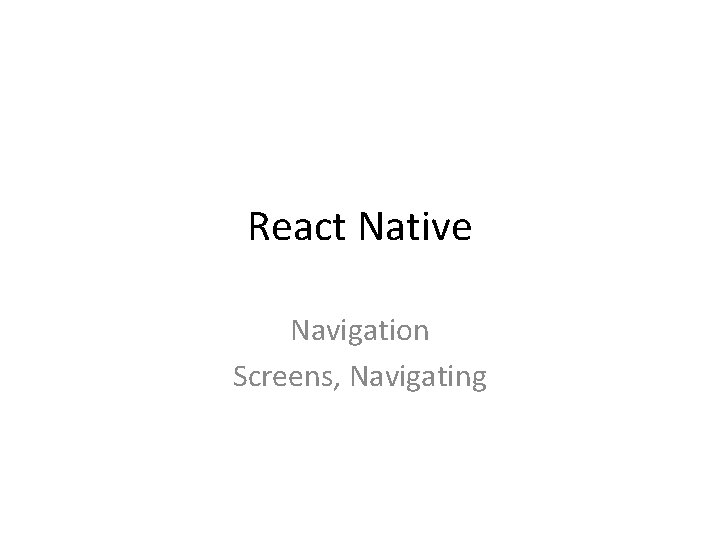
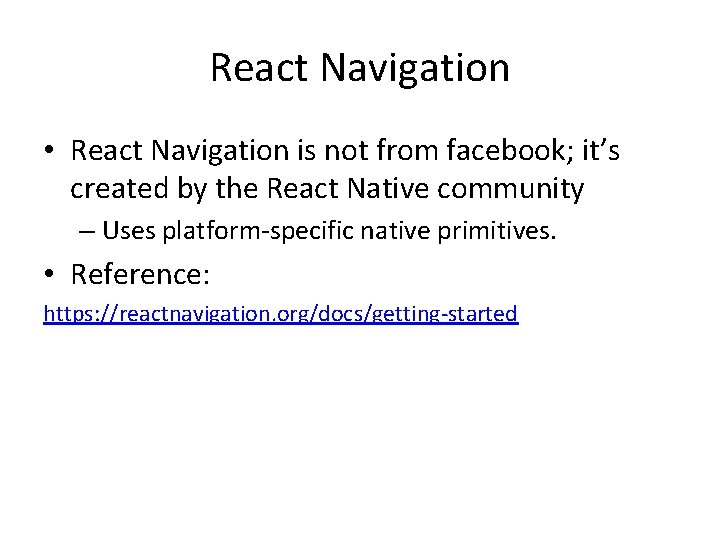
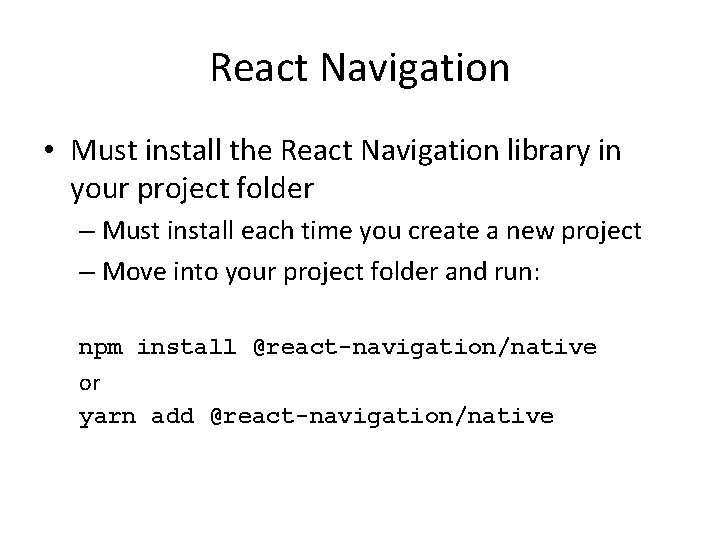
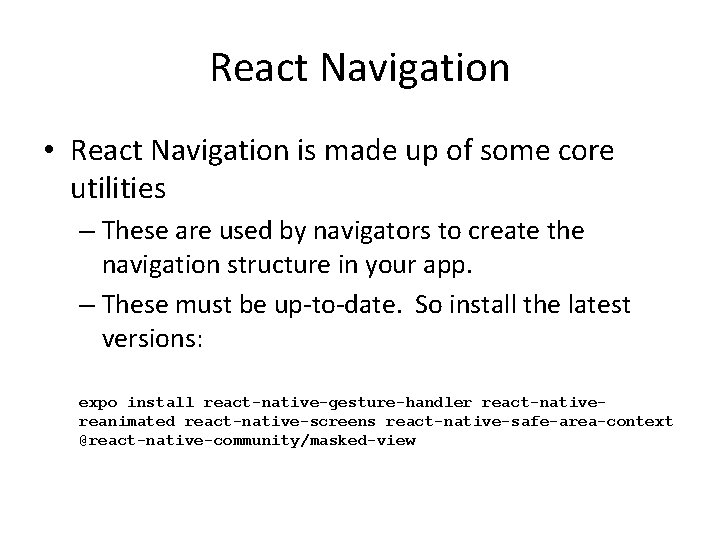
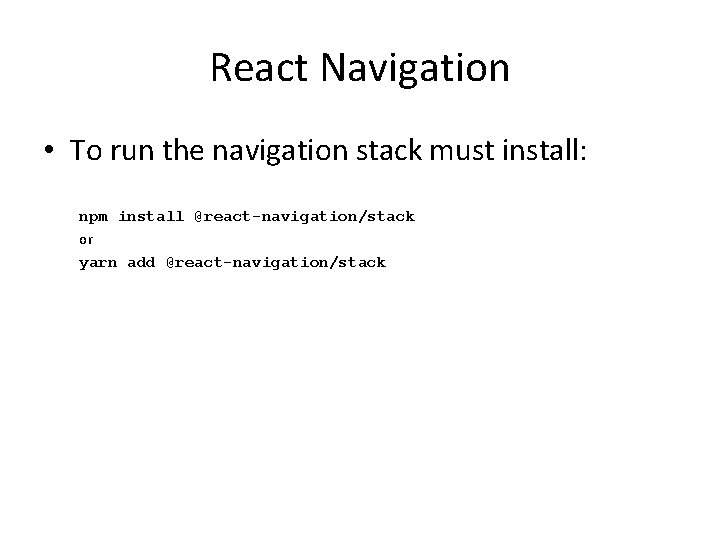
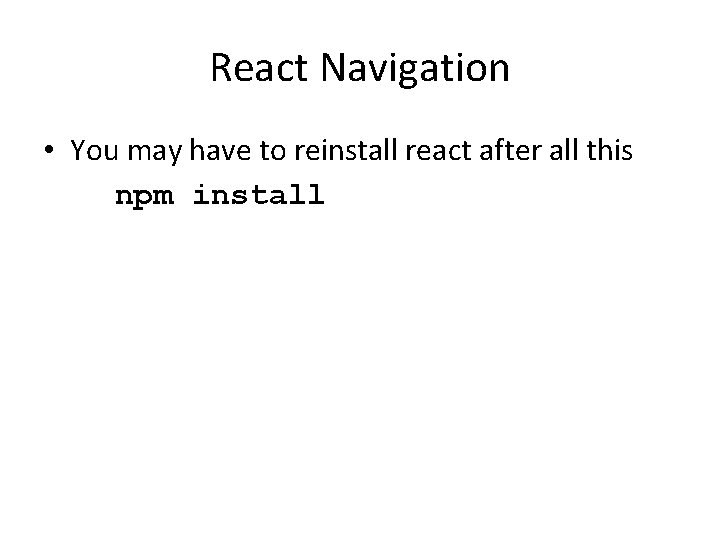
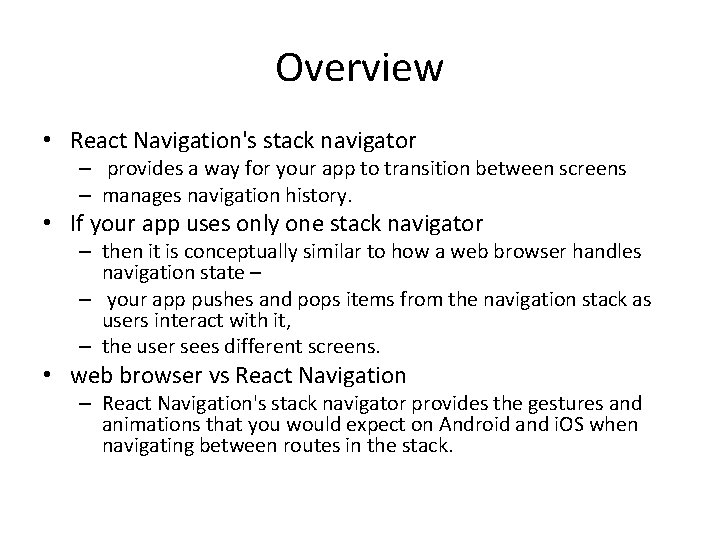
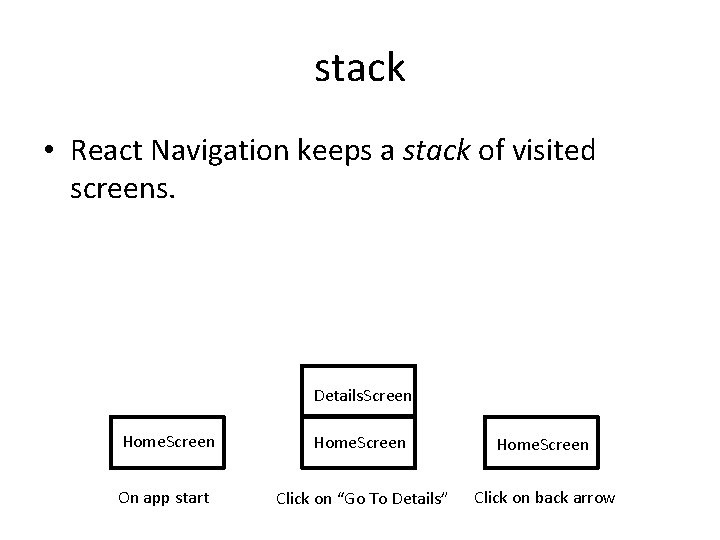
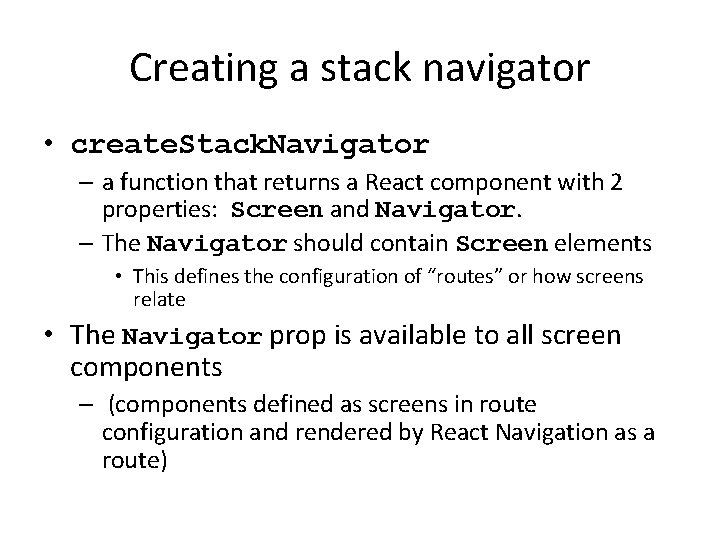
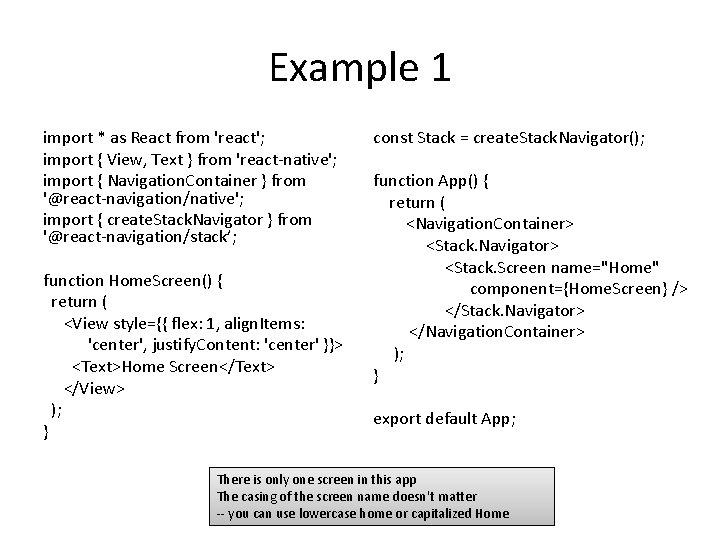
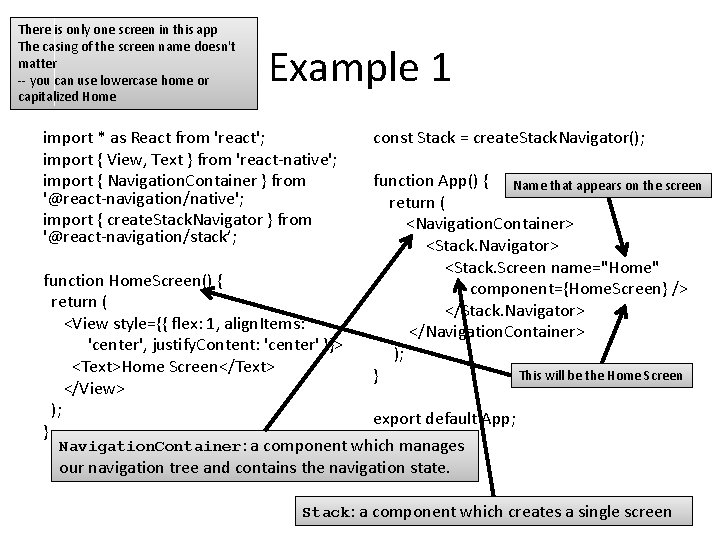
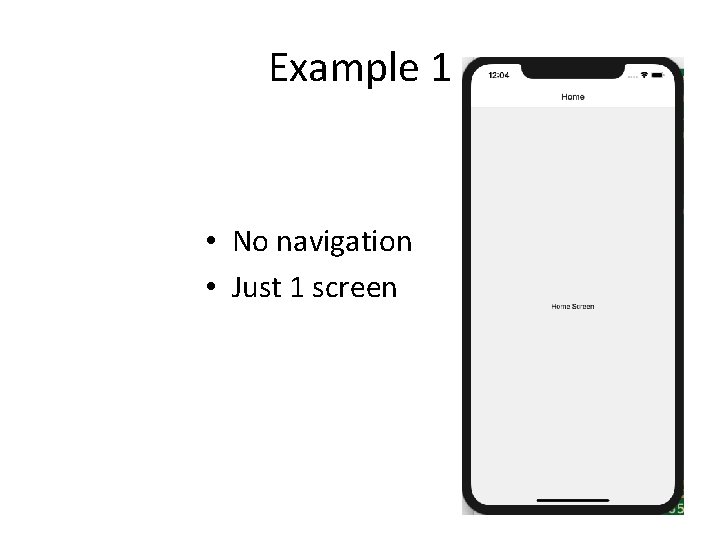
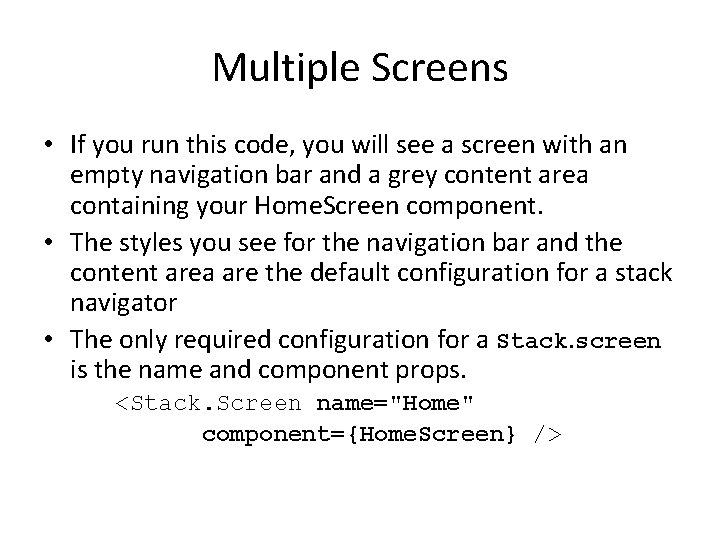
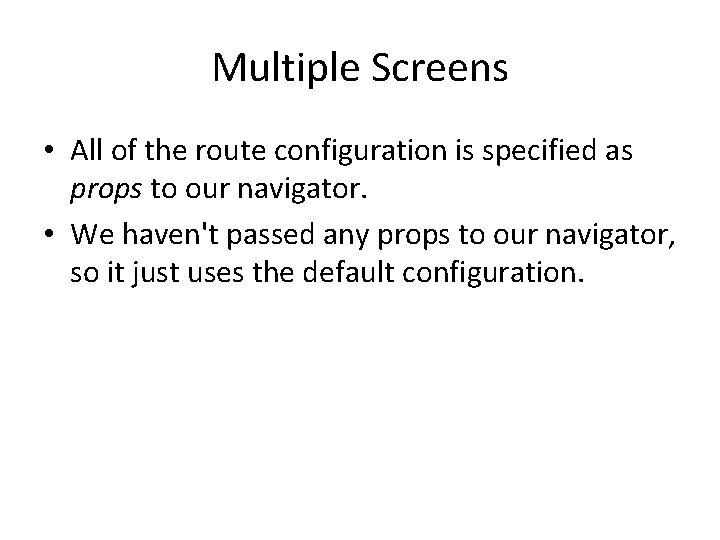
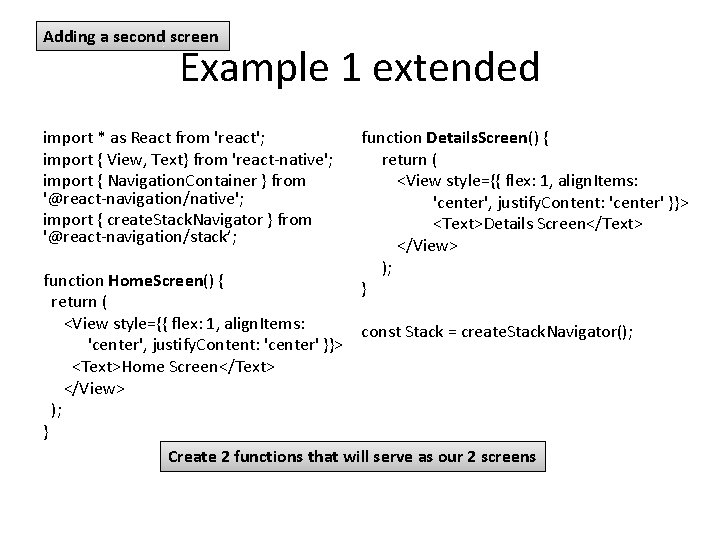
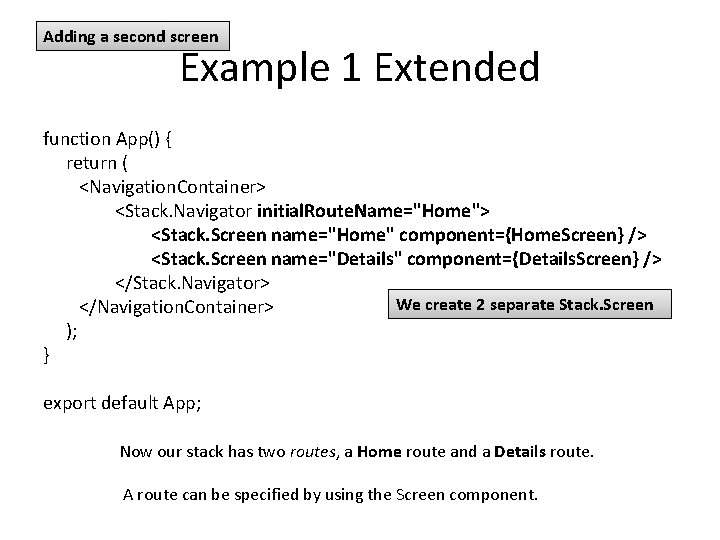
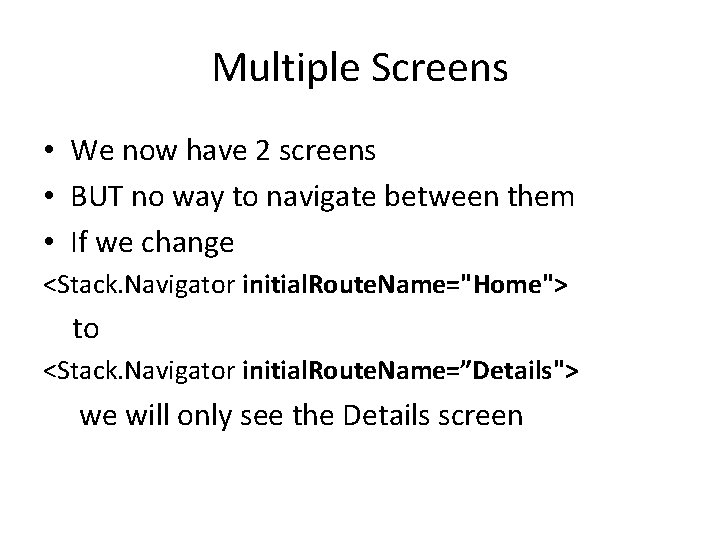
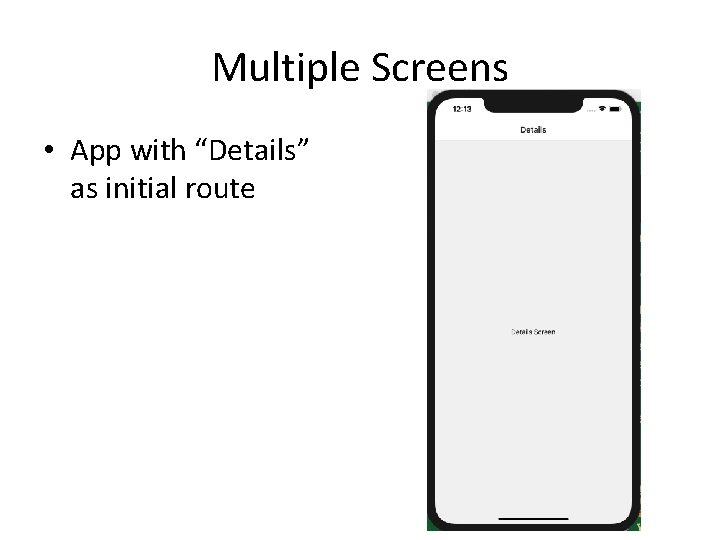
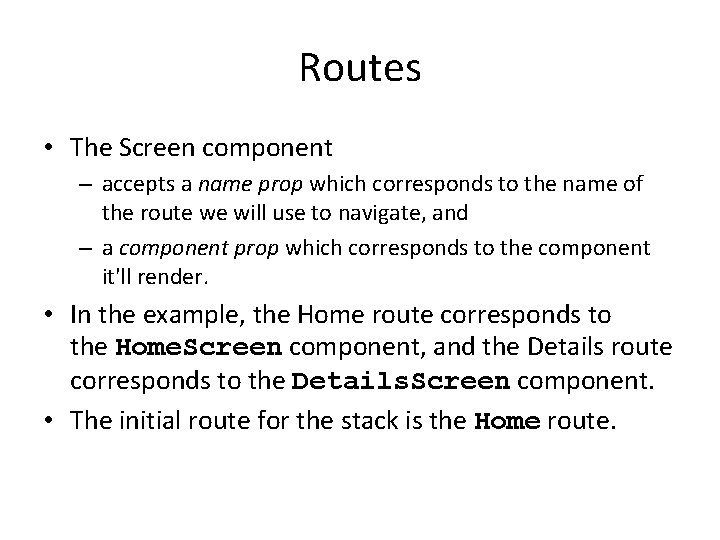
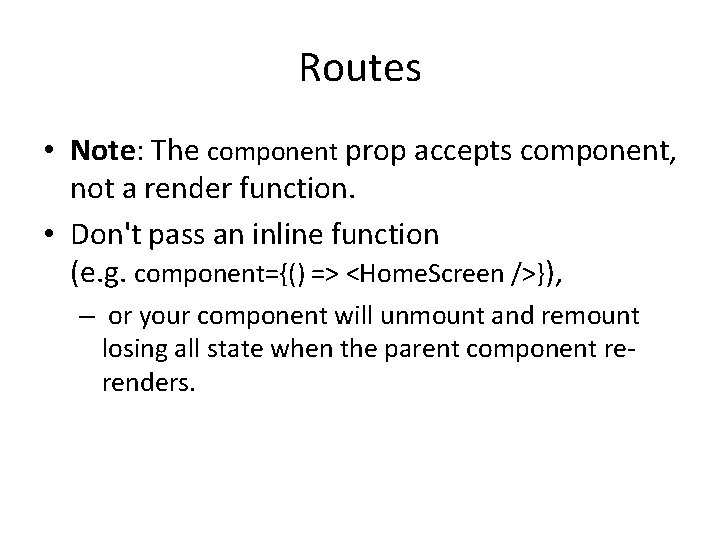
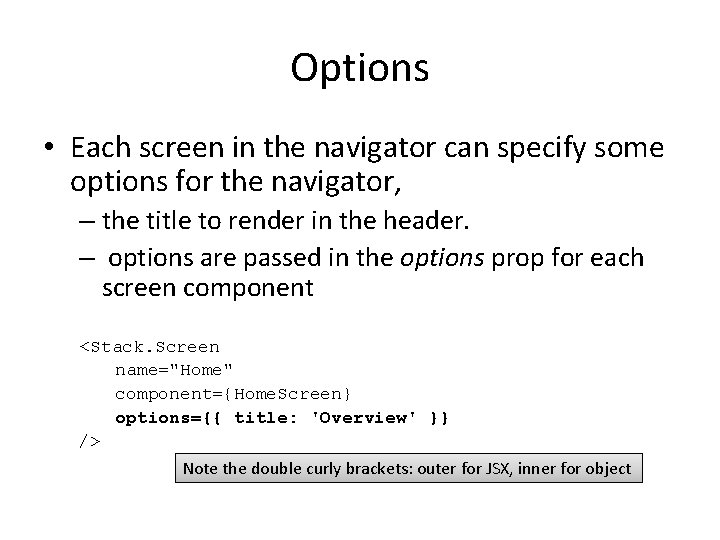
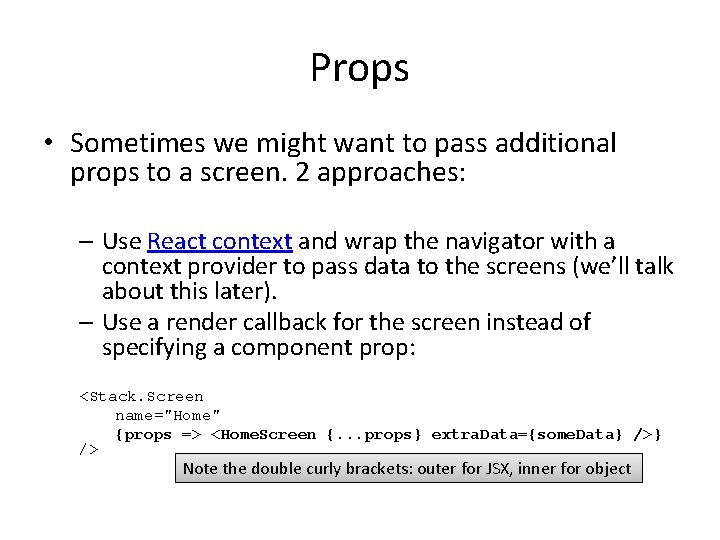
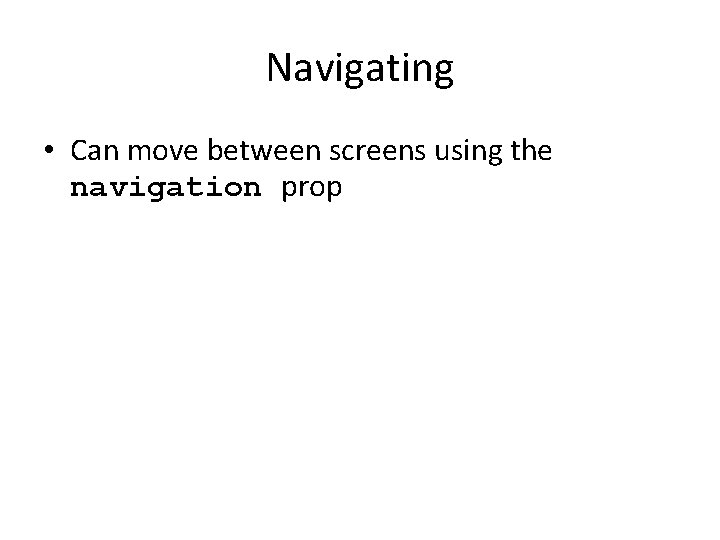
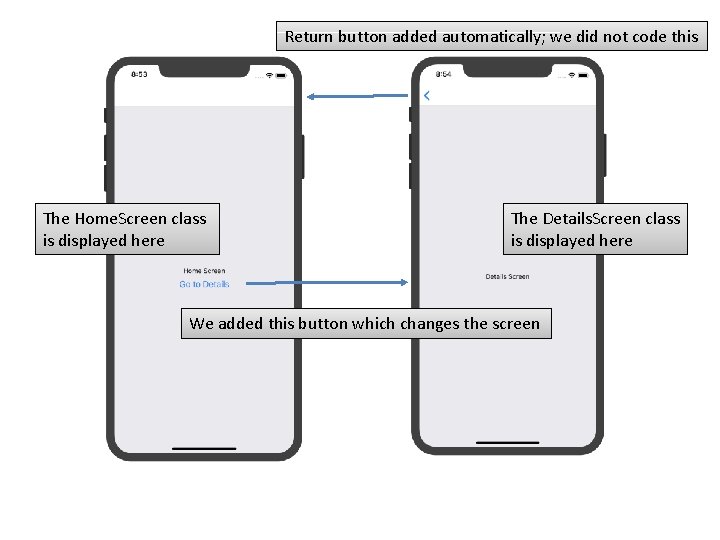
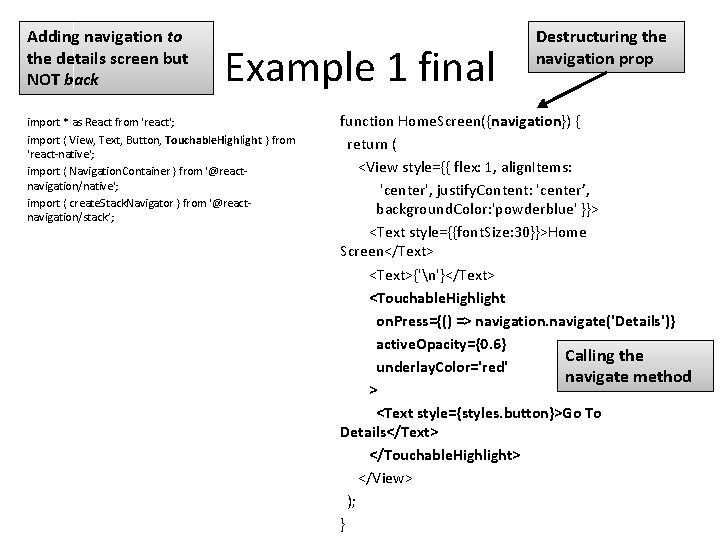
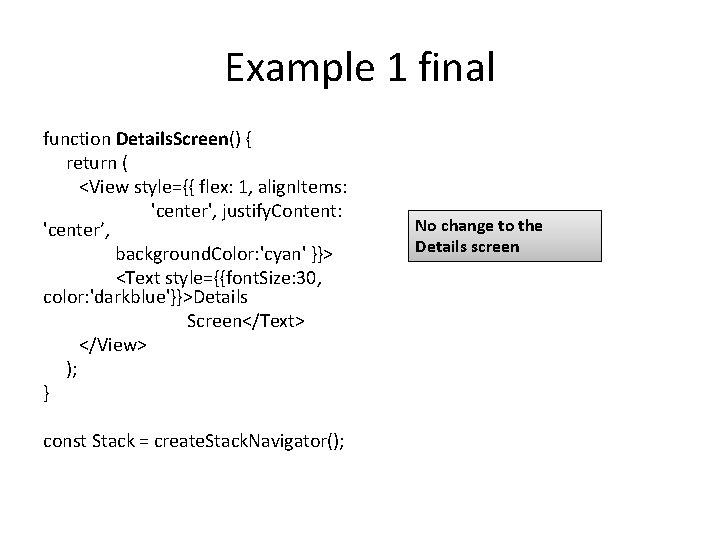
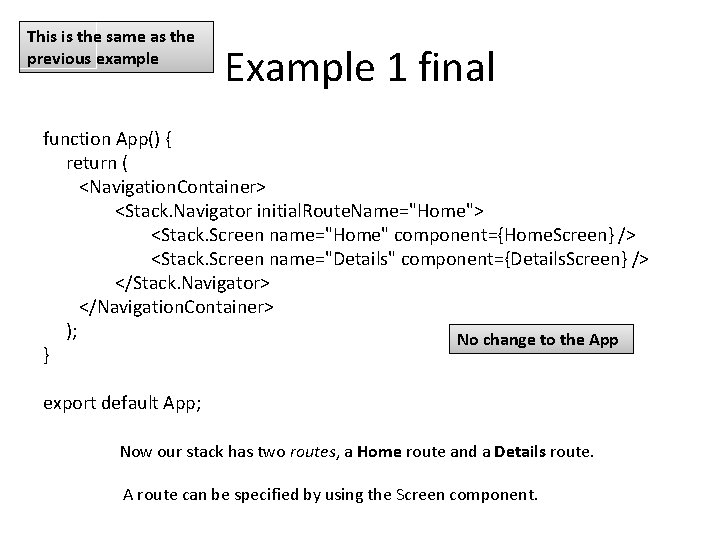
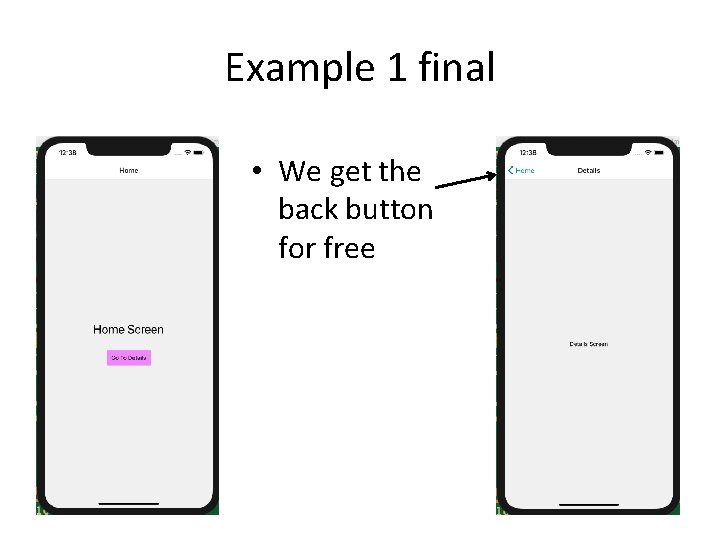
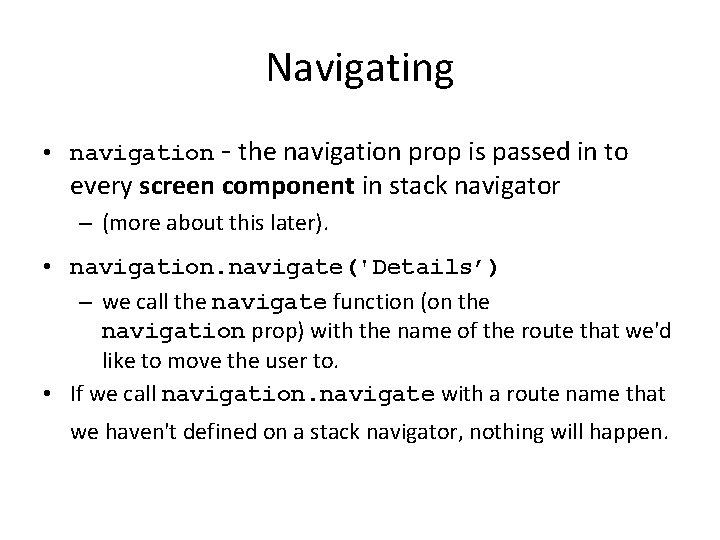
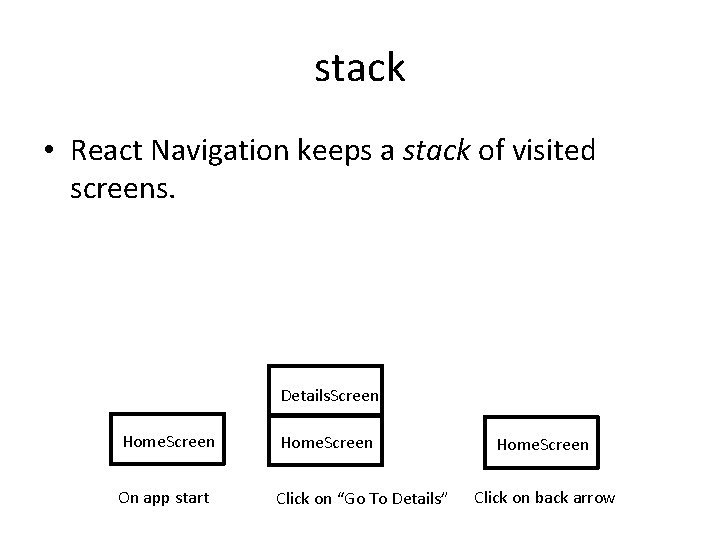
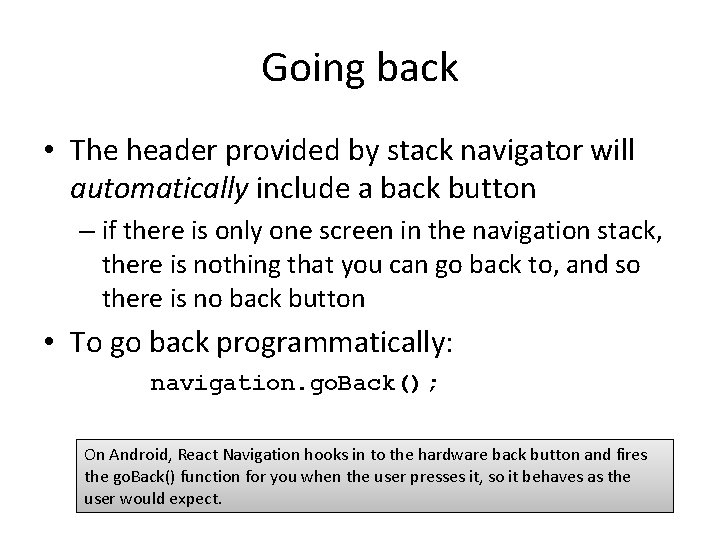
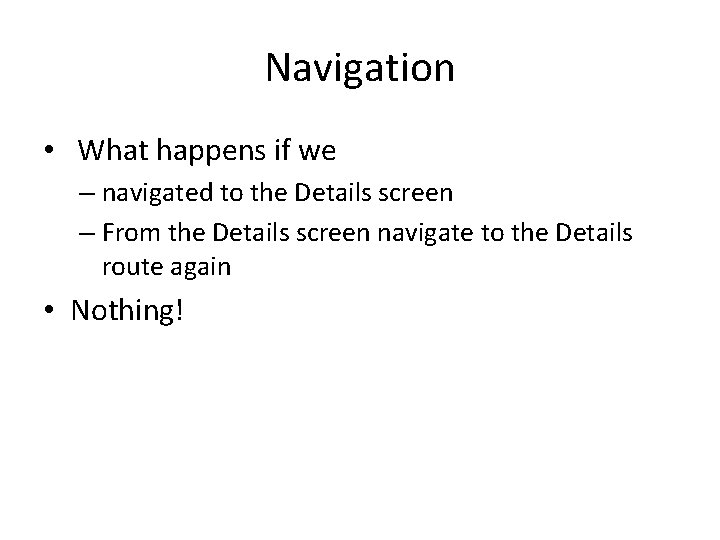
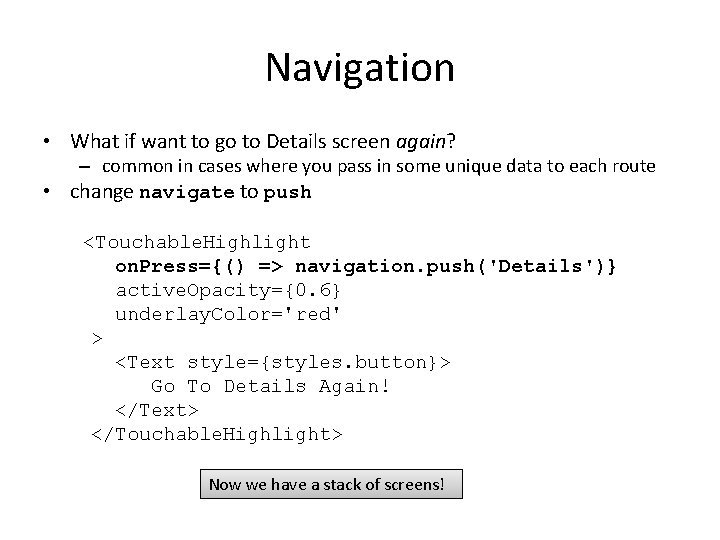
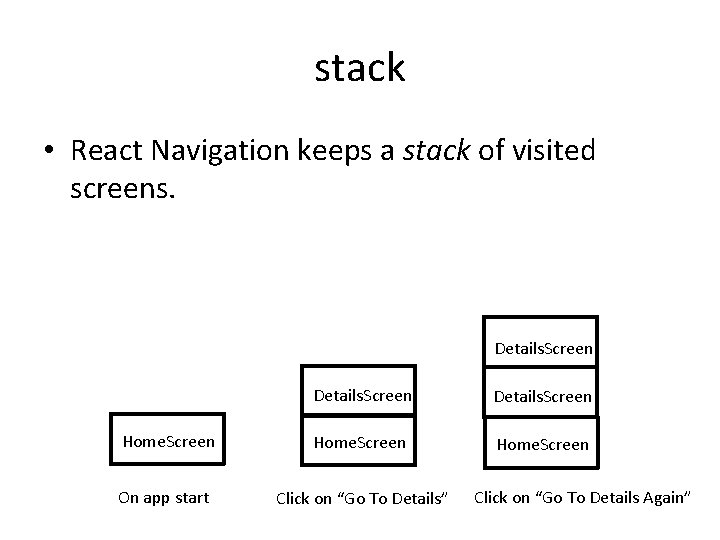
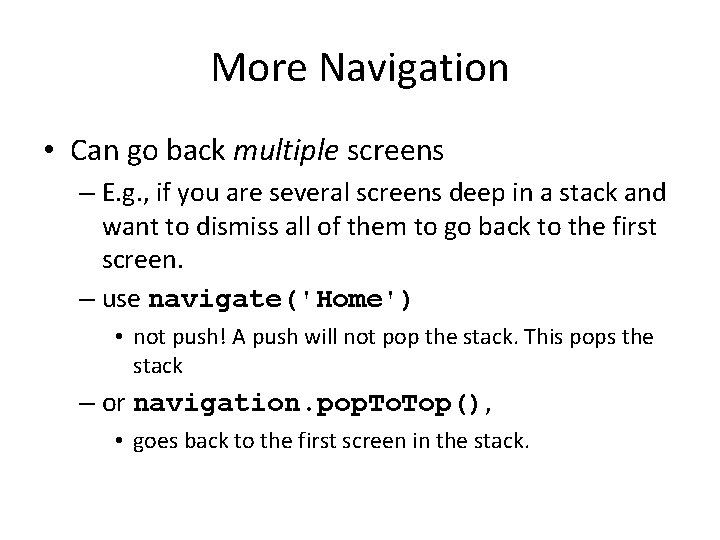
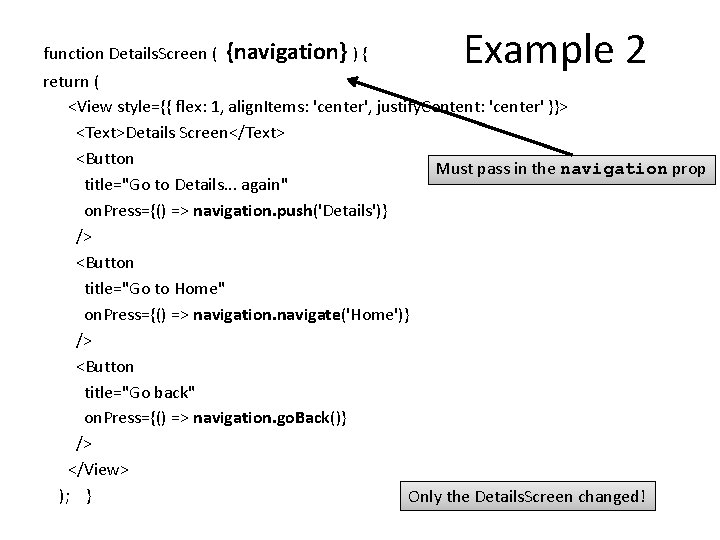
- Slides: 36
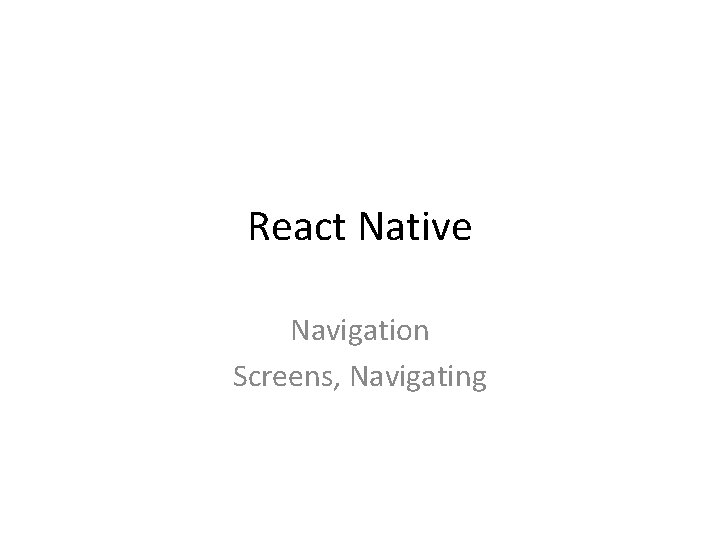
React Native Navigation Screens, Navigating
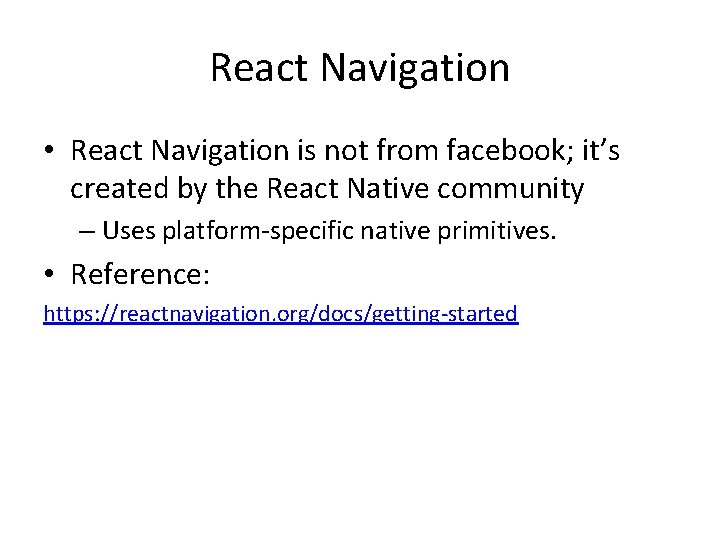
React Navigation • React Navigation is not from facebook; it’s created by the React Native community – Uses platform-specific native primitives. • Reference: https: //reactnavigation. org/docs/getting-started
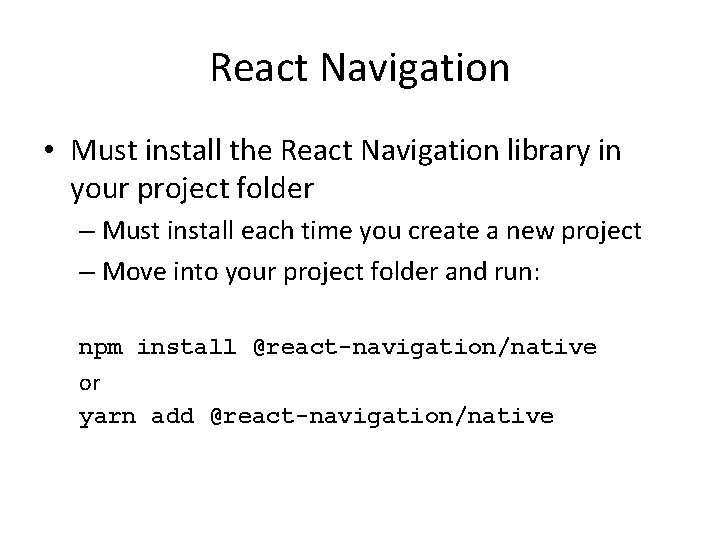
React Navigation • Must install the React Navigation library in your project folder – Must install each time you create a new project – Move into your project folder and run: npm install @react-navigation/native or yarn add @react-navigation/native
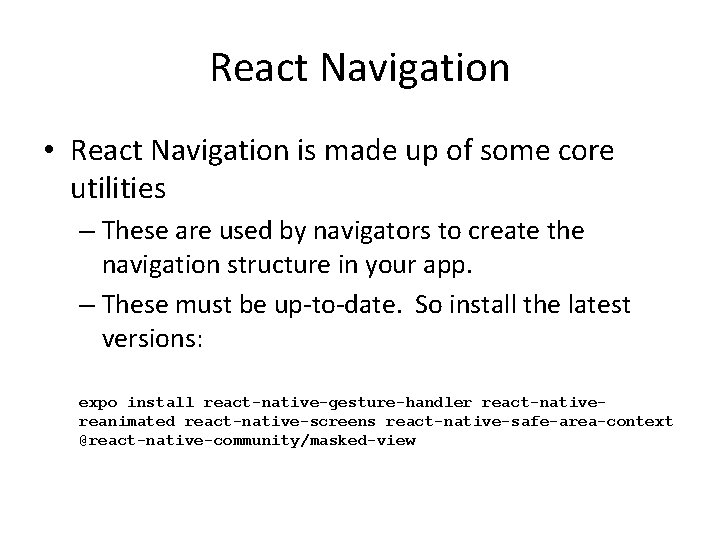
React Navigation • React Navigation is made up of some core utilities – These are used by navigators to create the navigation structure in your app. – These must be up-to-date. So install the latest versions: expo install react-native-gesture-handler react-nativereanimated react-native-screens react-native-safe-area-context @react-native-community/masked-view
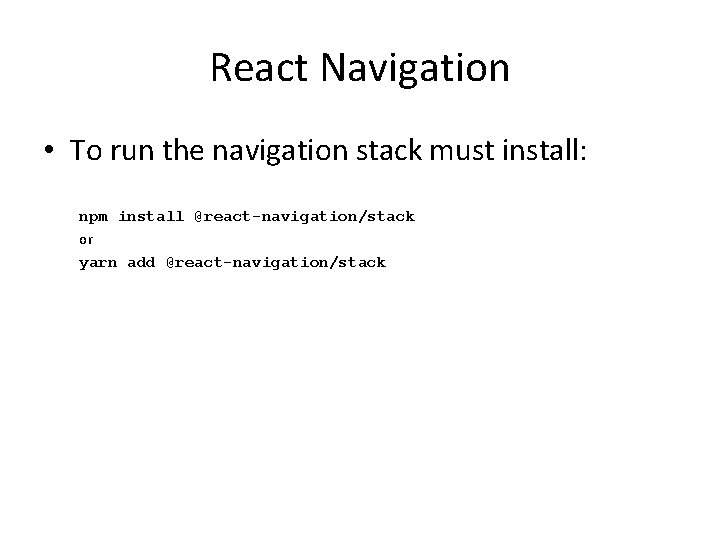
React Navigation • To run the navigation stack must install: npm install @react-navigation/stack or yarn add @react-navigation/stack
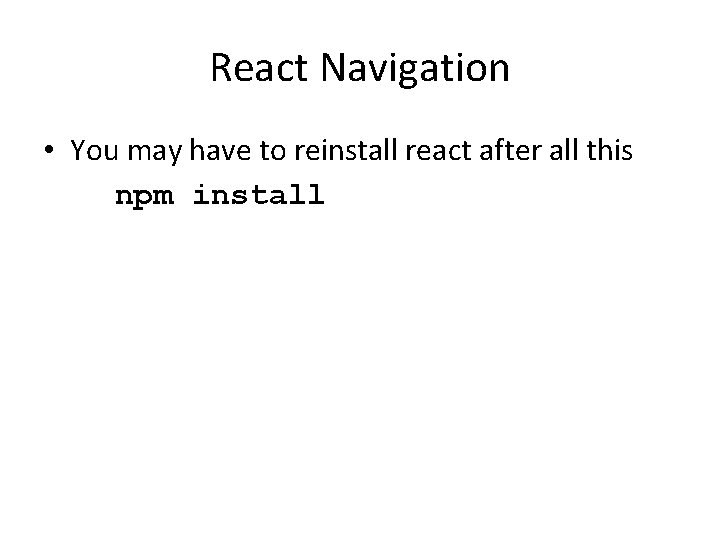
React Navigation • You may have to reinstall react after all this npm install
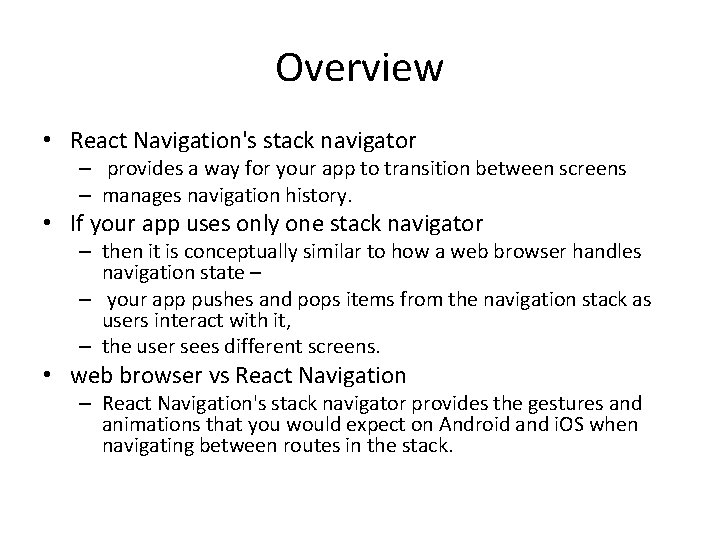
Overview • React Navigation's stack navigator – provides a way for your app to transition between screens – manages navigation history. • If your app uses only one stack navigator – then it is conceptually similar to how a web browser handles navigation state – – your app pushes and pops items from the navigation stack as users interact with it, – the user sees different screens. • web browser vs React Navigation – React Navigation's stack navigator provides the gestures and animations that you would expect on Android and i. OS when navigating between routes in the stack.
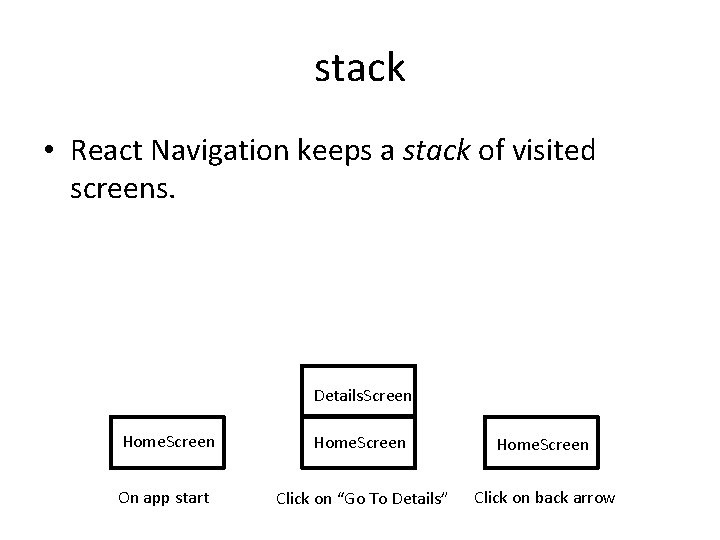
stack • React Navigation keeps a stack of visited screens. Details. Screen Home. Screen On app start Click on “Go To Details” Click on back arrow
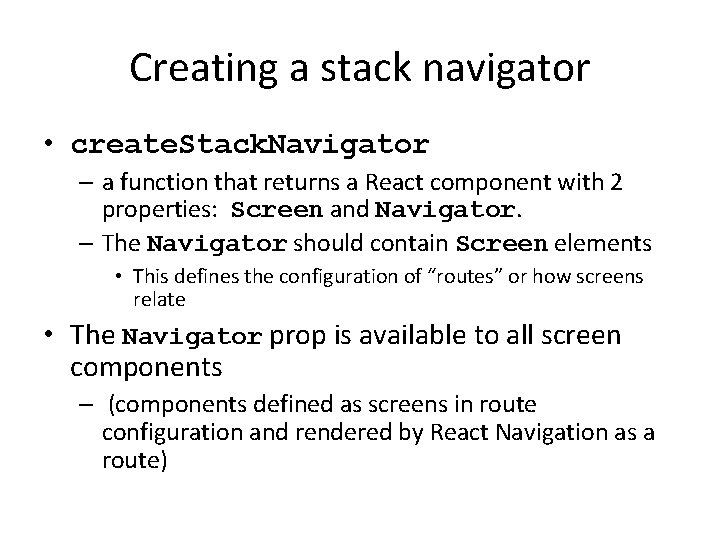
Creating a stack navigator • create. Stack. Navigator – a function that returns a React component with 2 properties: Screen and Navigator. – The Navigator should contain Screen elements • This defines the configuration of “routes” or how screens relate • The Navigator prop is available to all screen components – (components defined as screens in route configuration and rendered by React Navigation as a route)
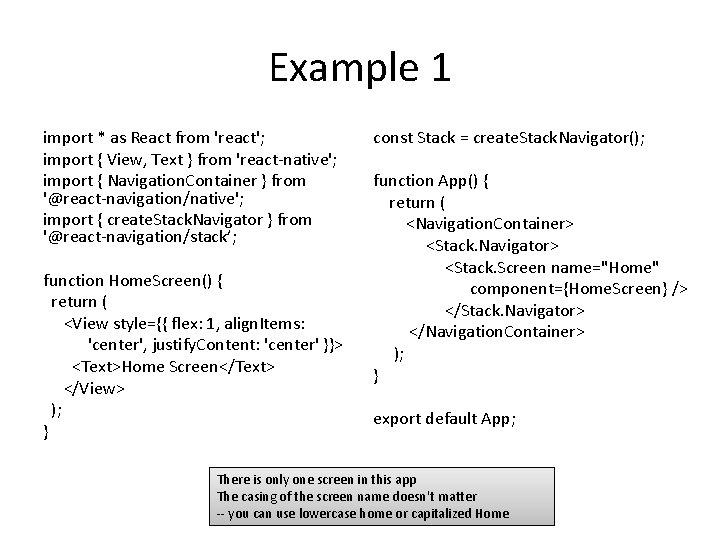
Example 1 import * as React from 'react'; import { View, Text } from 'react-native'; import { Navigation. Container } from '@react-navigation/native'; import { create. Stack. Navigator } from '@react-navigation/stack’; function Home. Screen() { return ( <View style={{ flex: 1, align. Items: 'center', justify. Content: 'center' }}> <Text>Home Screen</Text> </View> ); } const Stack = create. Stack. Navigator(); function App() { return ( <Navigation. Container> <Stack. Navigator> <Stack. Screen name="Home" component={Home. Screen} /> </Stack. Navigator> </Navigation. Container> ); } export default App; There is only one screen in this app The casing of the screen name doesn't matter -- you can use lowercase home or capitalized Home
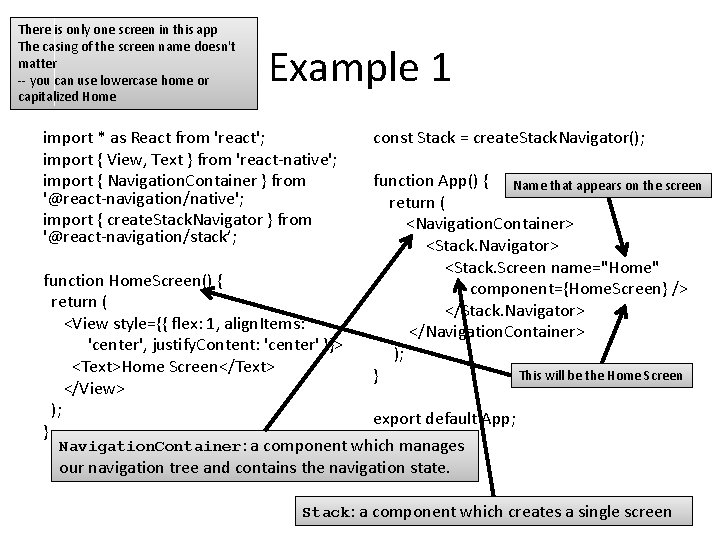
There is only one screen in this app The casing of the screen name doesn't matter -- you can use lowercase home or capitalized Home Example 1 import * as React from 'react'; import { View, Text } from 'react-native'; import { Navigation. Container } from '@react-navigation/native'; import { create. Stack. Navigator } from '@react-navigation/stack’; const Stack = create. Stack. Navigator(); function App() { Name that appears on the screen return ( <Navigation. Container> <Stack. Navigator> <Stack. Screen name="Home" component={Home. Screen} /> </Stack. Navigator> </Navigation. Container> ); This will be the Home Screen } function Home. Screen() { return ( <View style={{ flex: 1, align. Items: 'center', justify. Content: 'center' }}> <Text>Home Screen</Text> </View> ); export default App; } Navigation. Container: a component which manages our navigation tree and contains the navigation state. Stack: a component which creates a single screen
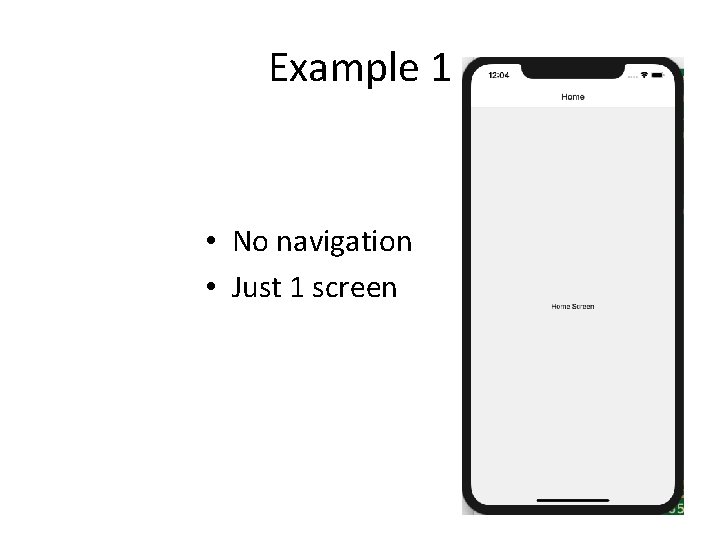
Example 1 • No navigation • Just 1 screen
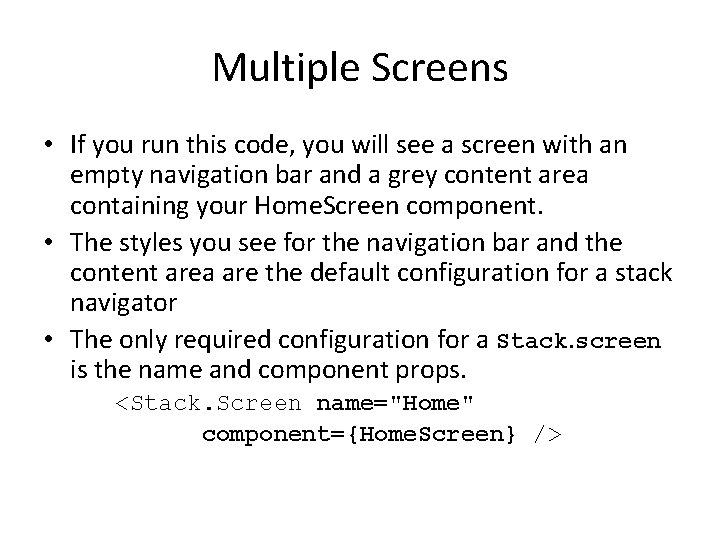
Multiple Screens • If you run this code, you will see a screen with an empty navigation bar and a grey content area containing your Home. Screen component. • The styles you see for the navigation bar and the content area are the default configuration for a stack navigator • The only required configuration for a Stack. screen is the name and component props. <Stack. Screen name="Home" component={Home. Screen} />
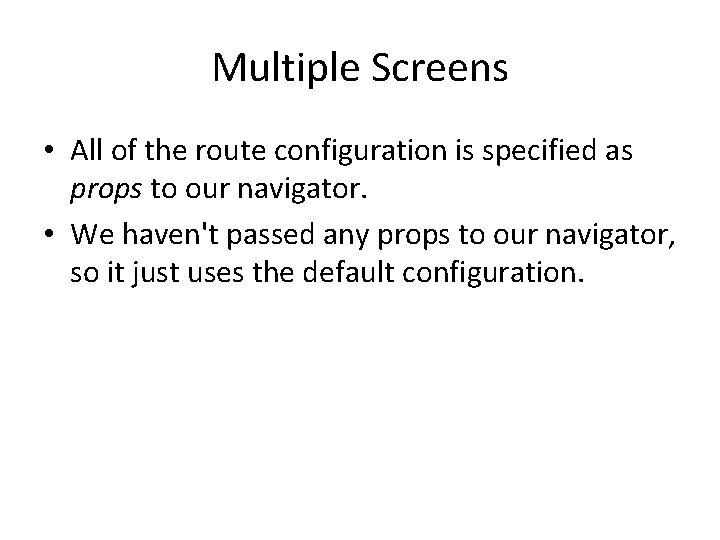
Multiple Screens • All of the route configuration is specified as props to our navigator. • We haven't passed any props to our navigator, so it just uses the default configuration.
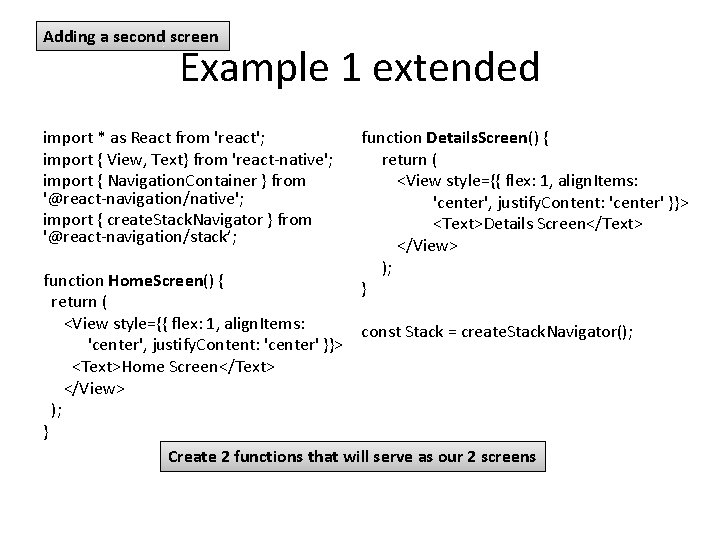
Adding a second screen Example 1 extended import * as React from 'react'; import { View, Text} from 'react-native'; import { Navigation. Container } from '@react-navigation/native'; import { create. Stack. Navigator } from '@react-navigation/stack’; function Details. Screen() { return ( <View style={{ flex: 1, align. Items: 'center', justify. Content: 'center' }}> <Text>Details Screen</Text> </View> ); } function Home. Screen() { return ( <View style={{ flex: 1, align. Items: const Stack = create. Stack. Navigator(); 'center', justify. Content: 'center' }}> <Text>Home Screen</Text> </View> ); } Create 2 functions that will serve as our 2 screens
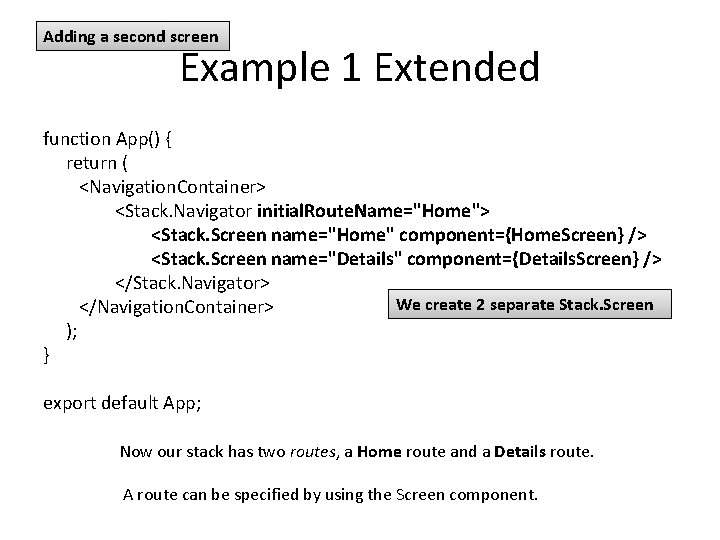
Adding a second screen Example 1 Extended function App() { return ( <Navigation. Container> <Stack. Navigator initial. Route. Name="Home"> <Stack. Screen name="Home" component={Home. Screen} /> <Stack. Screen name="Details" component={Details. Screen} /> </Stack. Navigator> We create 2 separate Stack. Screen </Navigation. Container> ); } export default App; Now our stack has two routes, a Home route and a Details route. A route can be specified by using the Screen component.
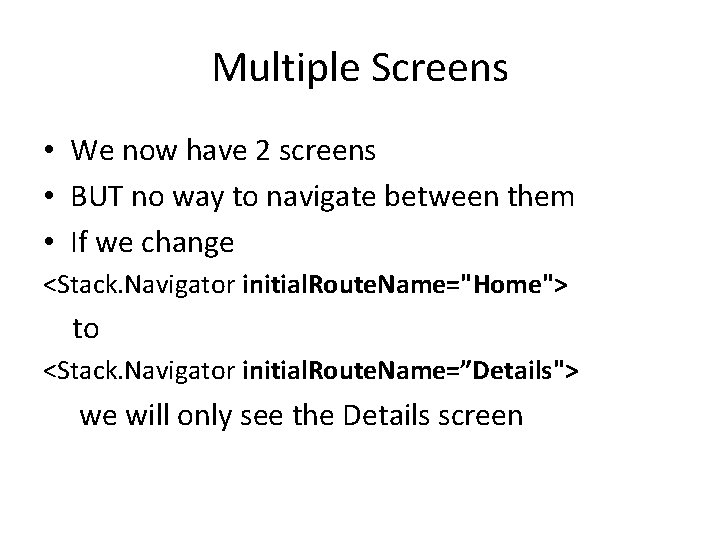
Multiple Screens • We now have 2 screens • BUT no way to navigate between them • If we change <Stack. Navigator initial. Route. Name="Home"> to <Stack. Navigator initial. Route. Name=”Details"> we will only see the Details screen
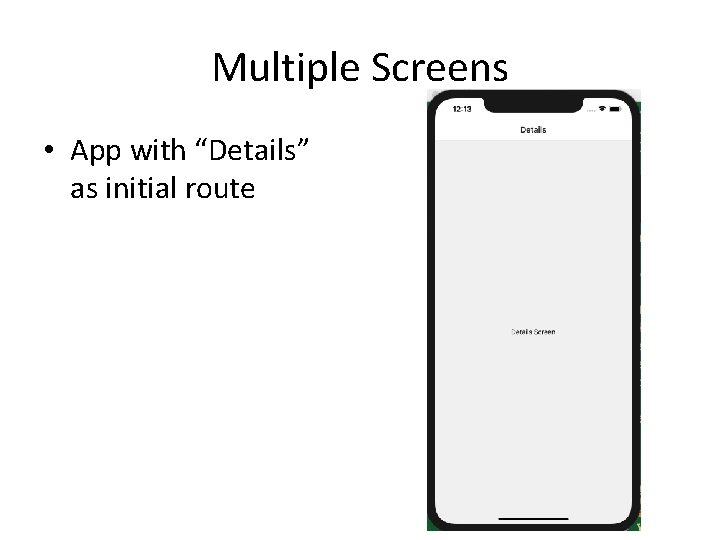
Multiple Screens • App with “Details” as initial route
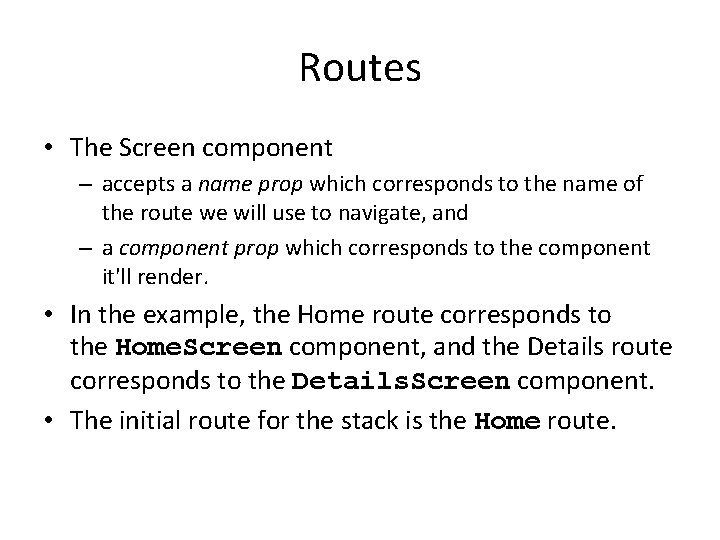
Routes • The Screen component – accepts a name prop which corresponds to the name of the route we will use to navigate, and – a component prop which corresponds to the component it'll render. • In the example, the Home route corresponds to the Home. Screen component, and the Details route corresponds to the Details. Screen component. • The initial route for the stack is the Home route.
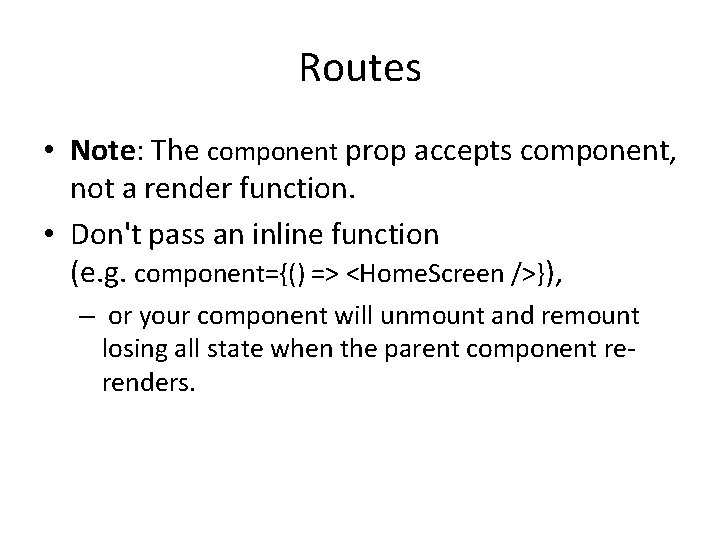
Routes • Note: The component prop accepts component, not a render function. • Don't pass an inline function (e. g. component={() => <Home. Screen />}), – or your component will unmount and remount losing all state when the parent component rerenders.
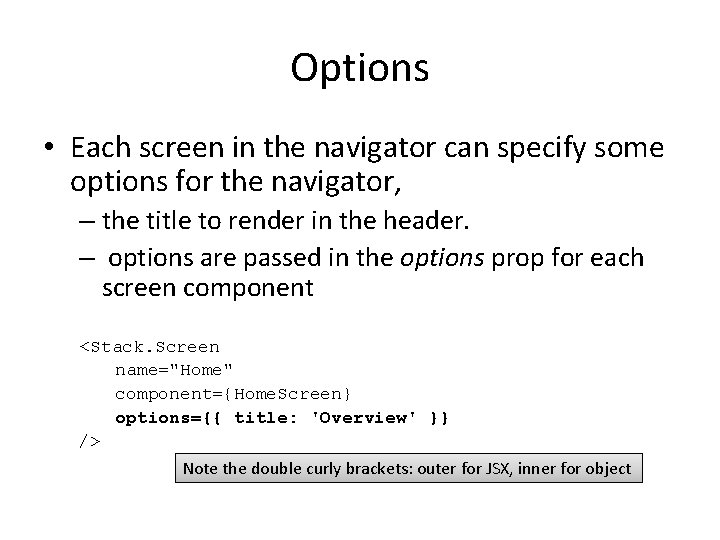
Options • Each screen in the navigator can specify some options for the navigator, – the title to render in the header. – options are passed in the options prop for each screen component <Stack. Screen name="Home" component={Home. Screen} options={{ title: 'Overview' }} /> Note the double curly brackets: outer for JSX, inner for object
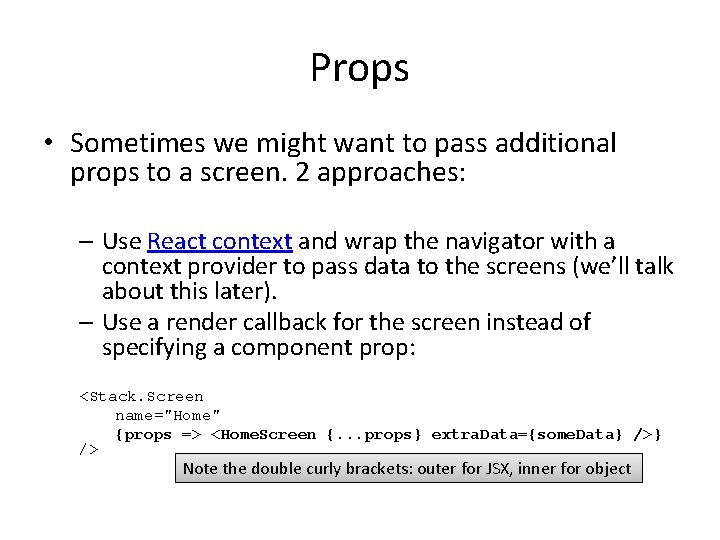
Props • Sometimes we might want to pass additional props to a screen. 2 approaches: – Use React context and wrap the navigator with a context provider to pass data to the screens (we’ll talk about this later). – Use a render callback for the screen instead of specifying a component prop: <Stack. Screen name="Home" {props => <Home. Screen {. . . props} extra. Data={some. Data} /> Note the double curly brackets: outer for JSX, inner for object
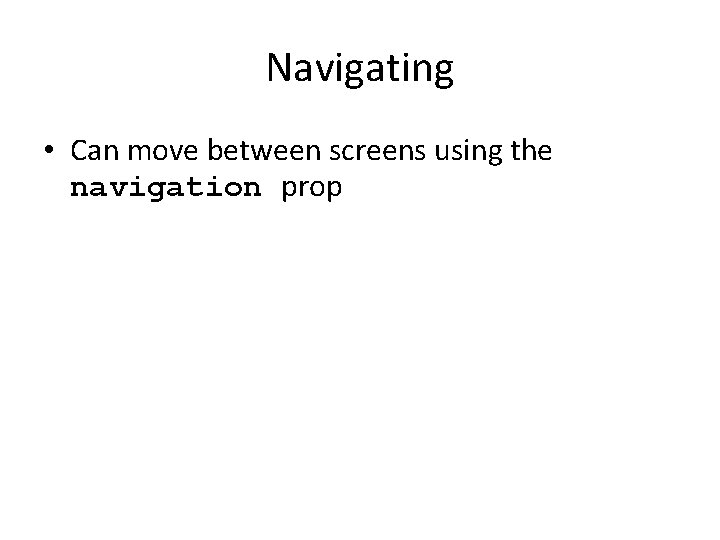
Navigating • Can move between screens using the navigation prop
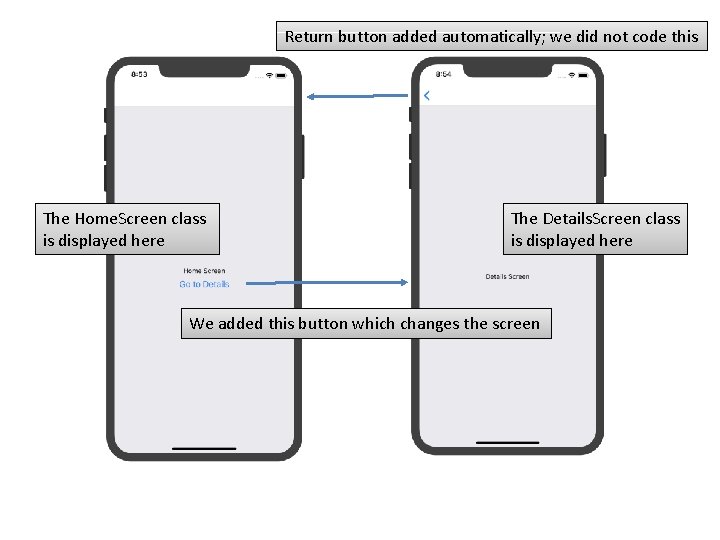
Return button added automatically; we did not code this The Home. Screen class is displayed here The Details. Screen class is displayed here We added this button which changes the screen
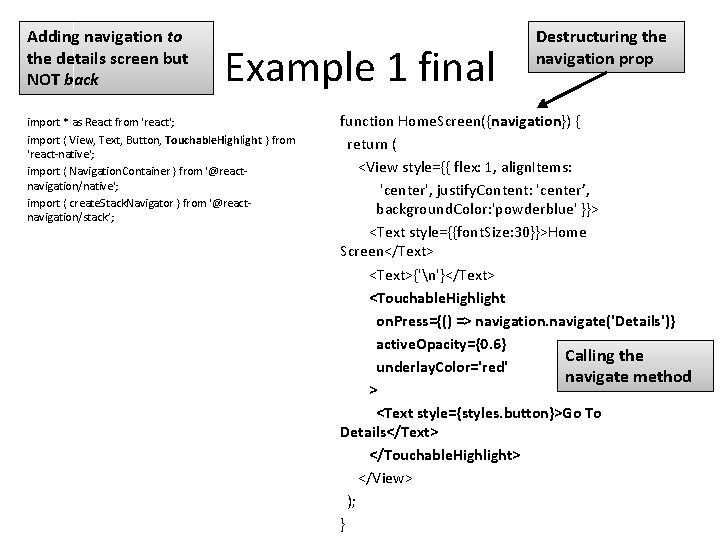
Adding navigation to the details screen but NOT back Example 1 final import * as React from 'react'; import { View, Text, Button, Touchable. Highlight } from 'react-native'; import { Navigation. Container } from '@reactnavigation/native'; import { create. Stack. Navigator } from '@reactnavigation/stack’; Destructuring the navigation prop function Home. Screen({navigation}) { return ( <View style={{ flex: 1, align. Items: 'center', justify. Content: 'center’, background. Color: 'powderblue' }}> <Text style={{font. Size: 30}}>Home Screen</Text> <Text>{'n'}</Text> <Touchable. Highlight on. Press={() => navigation. navigate('Details')} active. Opacity={0. 6} Calling the underlay. Color='red' navigate method > <Text style={styles. button}>Go To Details</Text> </Touchable. Highlight> </View> ); }
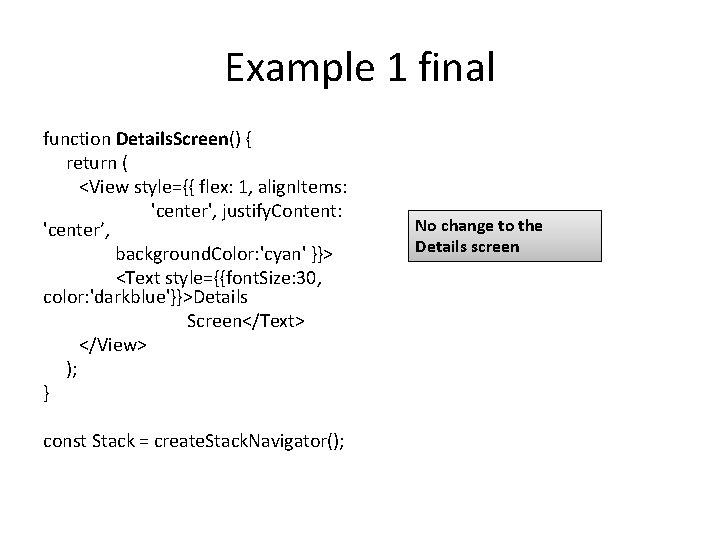
Example 1 final function Details. Screen() { return ( <View style={{ flex: 1, align. Items: 'center', justify. Content: 'center’, background. Color: 'cyan' }}> <Text style={{font. Size: 30, color: 'darkblue'}}>Details Screen</Text> </View> ); } const Stack = create. Stack. Navigator(); No change to the Details screen
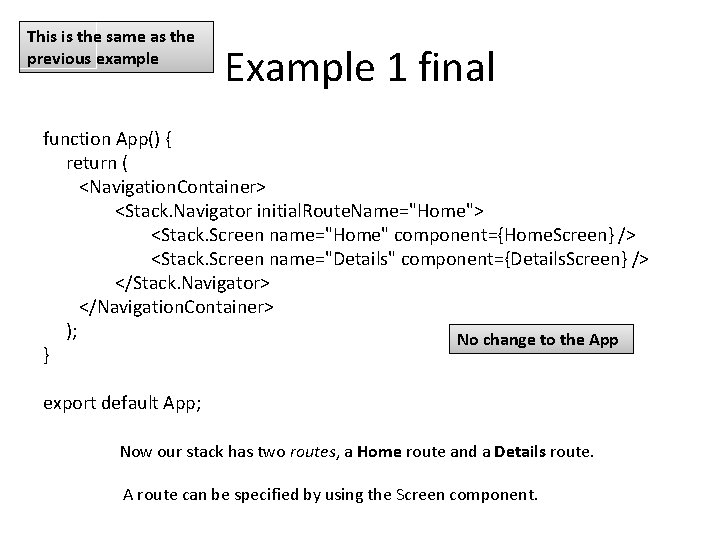
This is the same as the previous example Example 1 final function App() { return ( <Navigation. Container> <Stack. Navigator initial. Route. Name="Home"> <Stack. Screen name="Home" component={Home. Screen} /> <Stack. Screen name="Details" component={Details. Screen} /> </Stack. Navigator> </Navigation. Container> ); No change to the App } export default App; Now our stack has two routes, a Home route and a Details route. A route can be specified by using the Screen component.
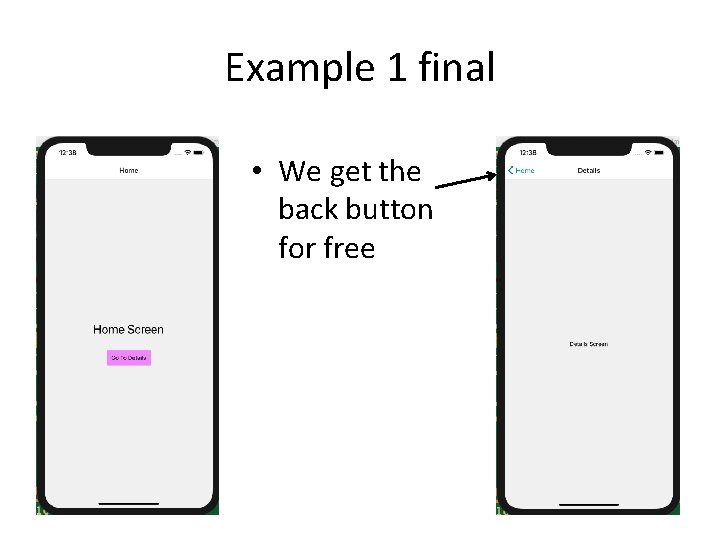
Example 1 final • We get the back button for free
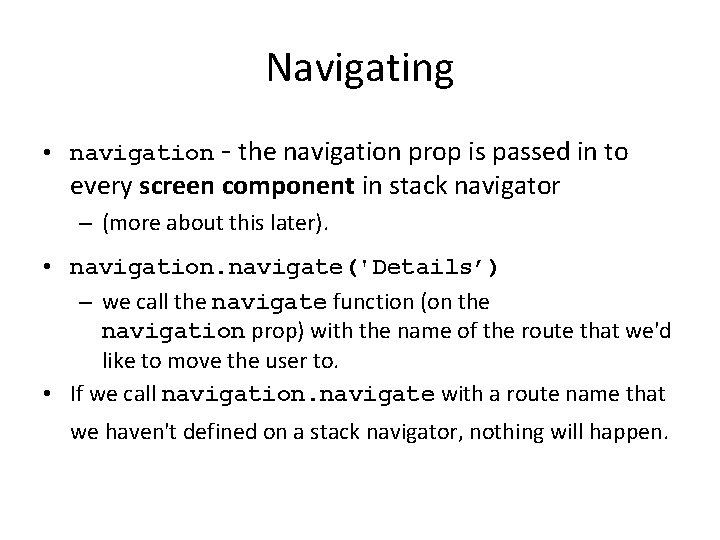
Navigating • navigation - the navigation prop is passed in to every screen component in stack navigator – (more about this later). • navigation. navigate('Details’) – we call the navigate function (on the navigation prop) with the name of the route that we'd like to move the user to. • If we call navigation. navigate with a route name that we haven't defined on a stack navigator, nothing will happen.
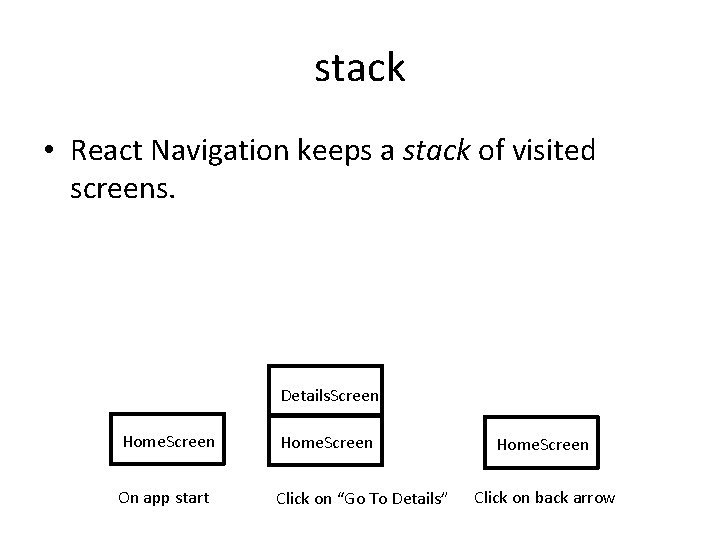
stack • React Navigation keeps a stack of visited screens. Details. Screen Home. Screen On app start Click on “Go To Details” Home. Screen Click on back arrow
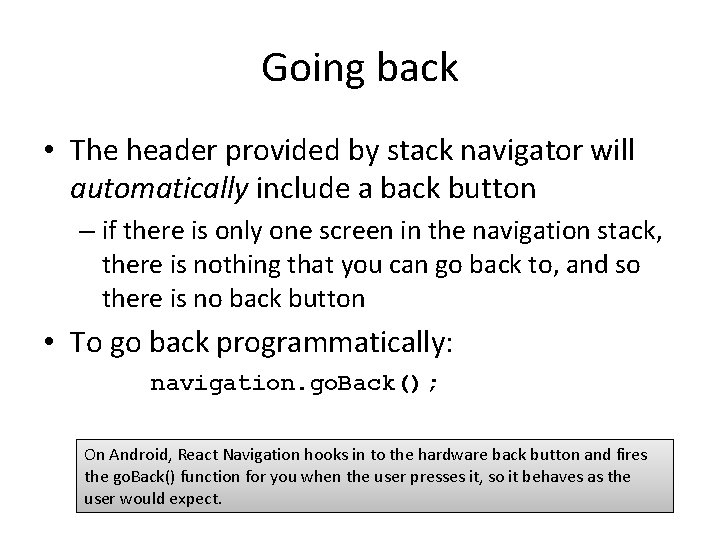
Going back • The header provided by stack navigator will automatically include a back button – if there is only one screen in the navigation stack, there is nothing that you can go back to, and so there is no back button • To go back programmatically: navigation. go. Back(); On Android, React Navigation hooks in to the hardware back button and fires the go. Back() function for you when the user presses it, so it behaves as the user would expect.
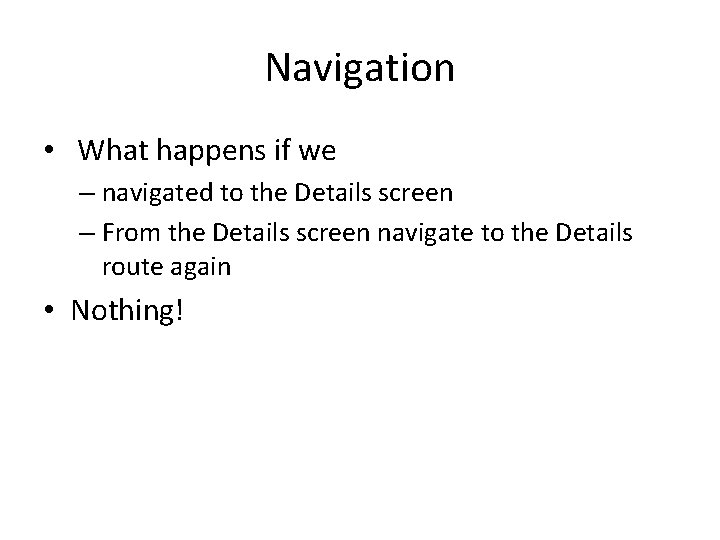
Navigation • What happens if we – navigated to the Details screen – From the Details screen navigate to the Details route again • Nothing!
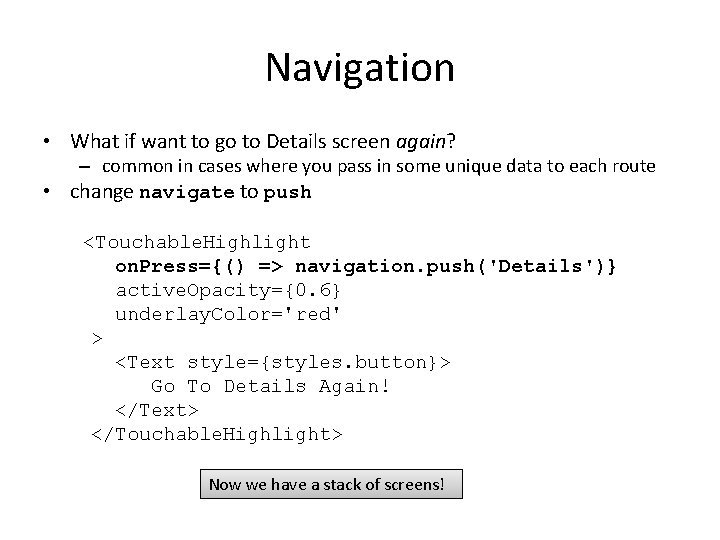
Navigation • What if want to go to Details screen again? – common in cases where you pass in some unique data to each route • change navigate to push <Touchable. Highlight on. Press={() => navigation. push('Details')} active. Opacity={0. 6} underlay. Color='red' > <Text style={styles. button}> Go To Details Again! </Text> </Touchable. Highlight> Now we have a stack of screens!
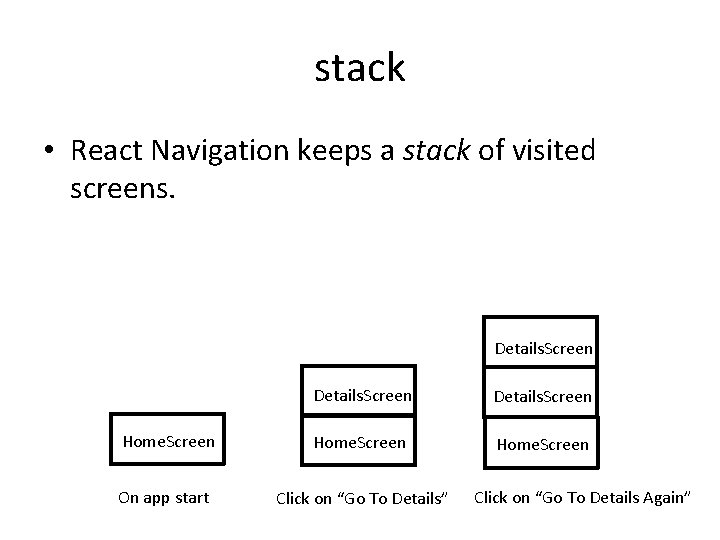
stack • React Navigation keeps a stack of visited screens. Details. Screen Home. Screen On app start Click on “Go To Details” Click on “Go To Details Again”
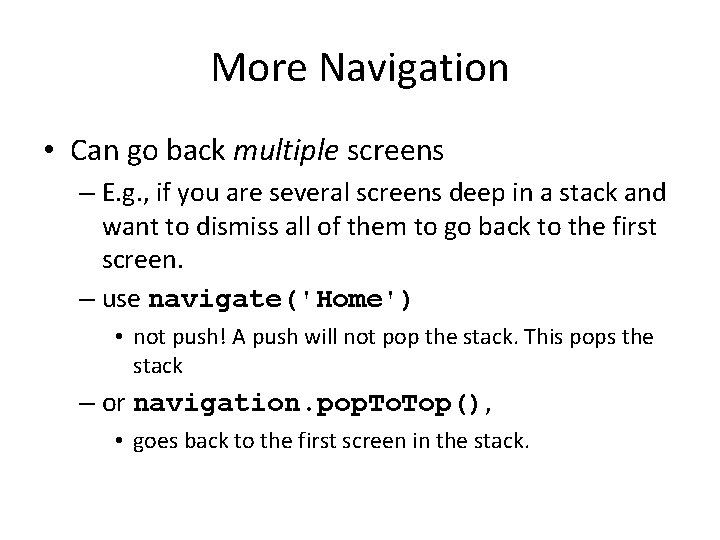
More Navigation • Can go back multiple screens – E. g. , if you are several screens deep in a stack and want to dismiss all of them to go back to the first screen. – use navigate('Home') • not push! A push will not pop the stack. This pops the stack – or navigation. pop. Top(), • goes back to the first screen in the stack.
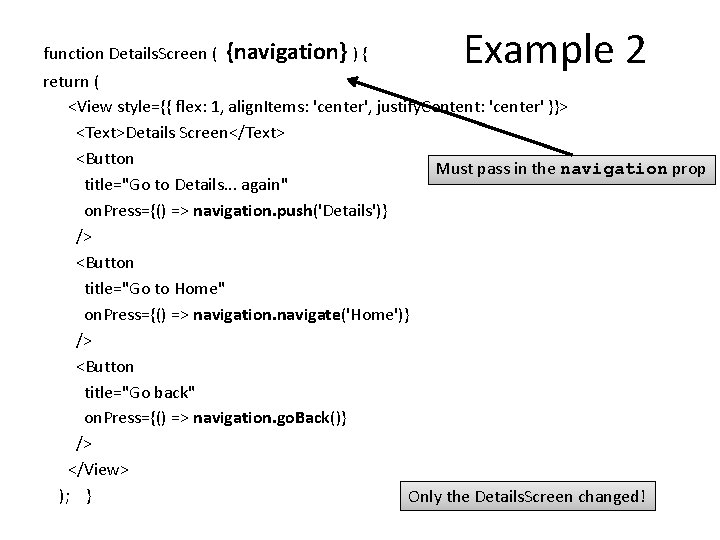
function Details. Screen ( {navigation} ) { Example 2 return ( <View style={{ flex: 1, align. Items: 'center', justify. Content: 'center' }}> <Text>Details Screen</Text> <Button Must pass in the navigation prop title="Go to Details. . . again" on. Press={() => navigation. push('Details')} /> <Button title="Go to Home" on. Press={() => navigation. navigate('Home')} /> <Button title="Go back" on. Press={() => navigation. go. Back()} /> </View> ); } Only the Details. Screen changed!