Python Programming 1 2008926 JauJi Lin Outline Python
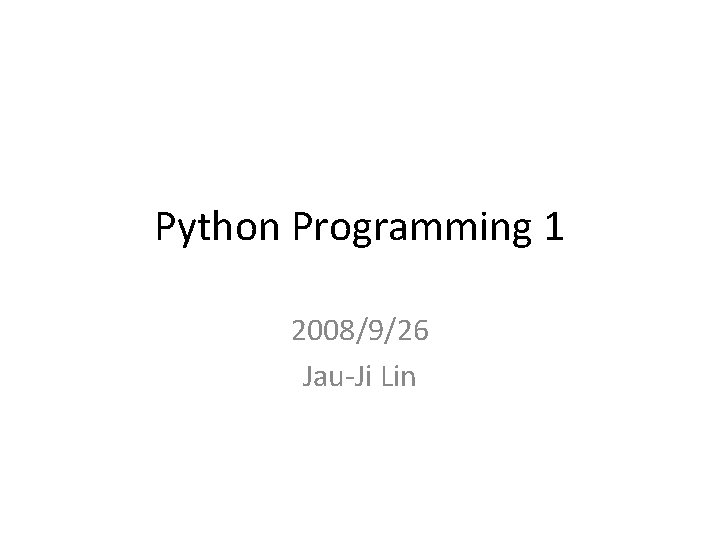
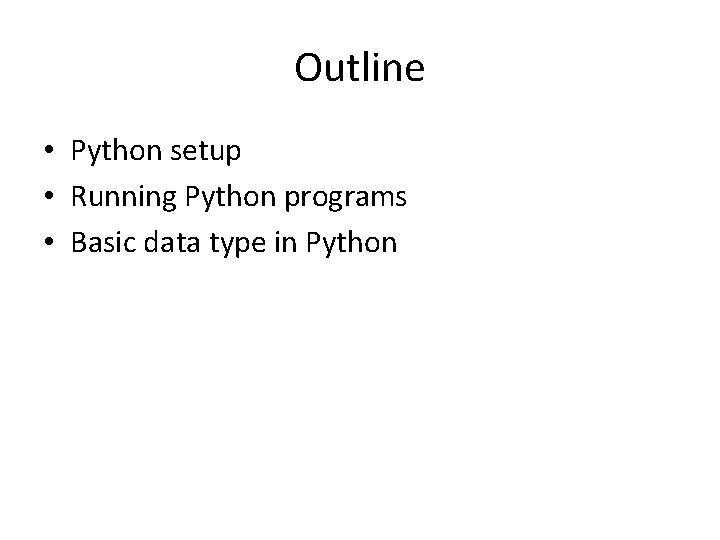
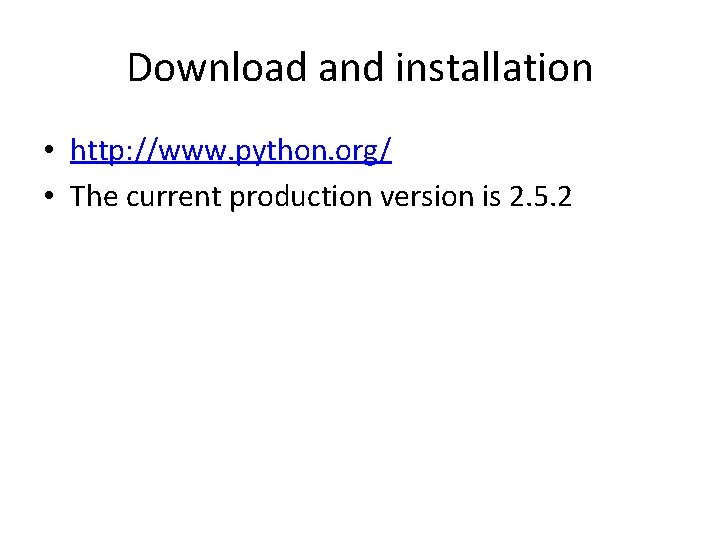
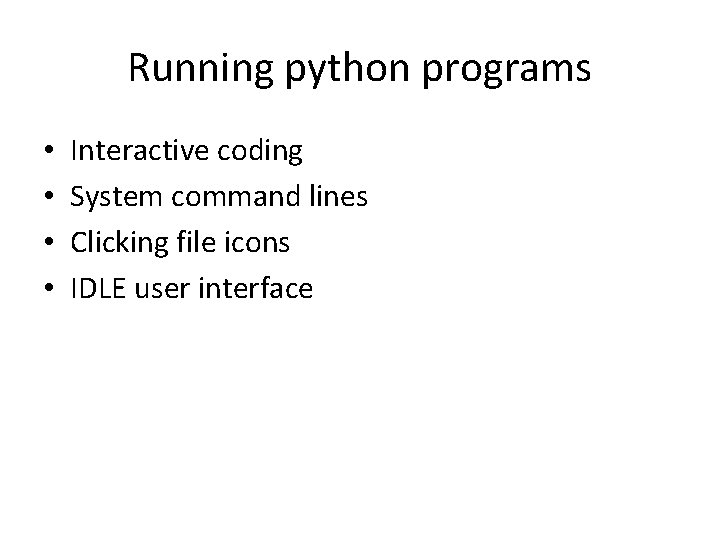
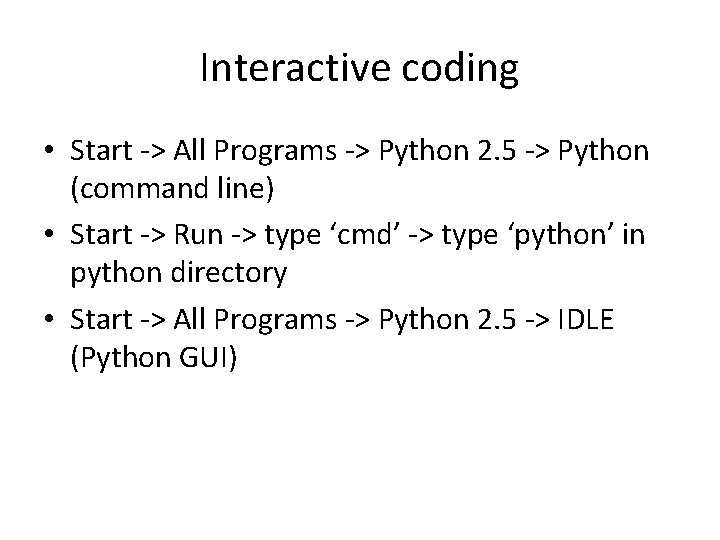
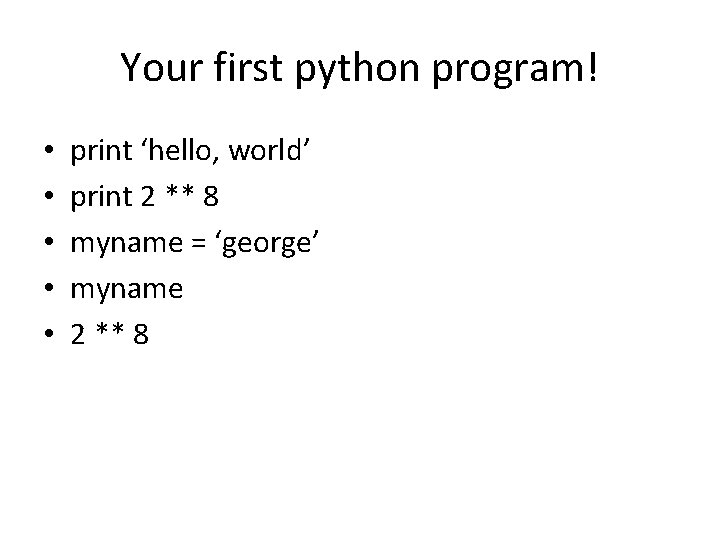
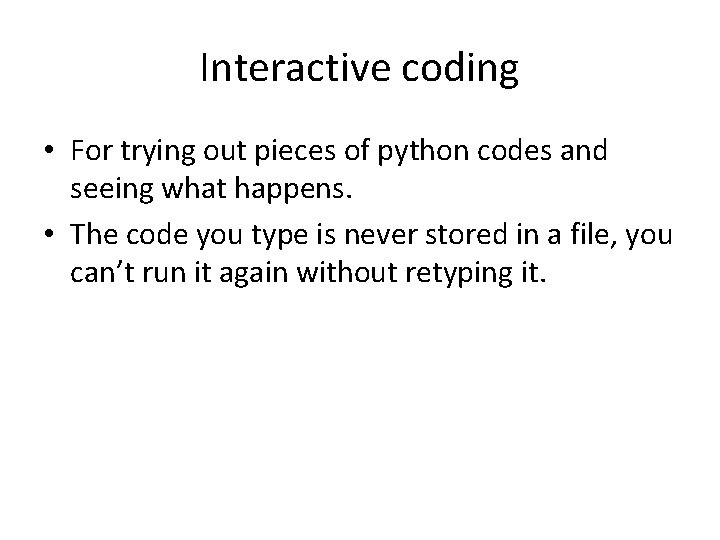
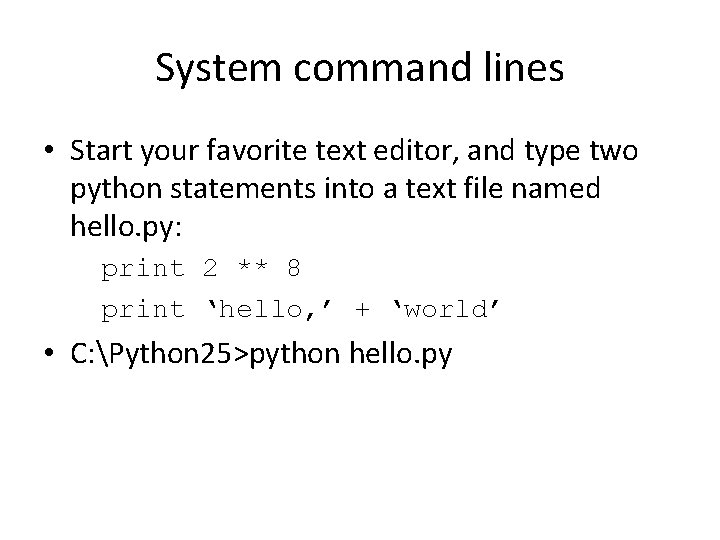
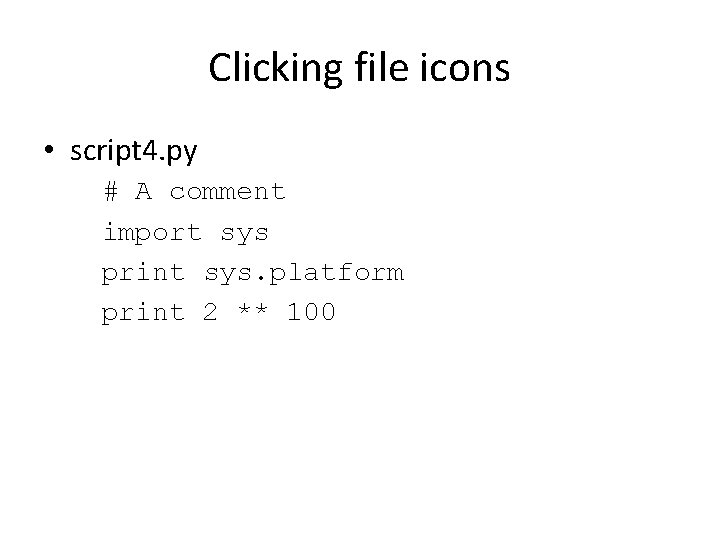
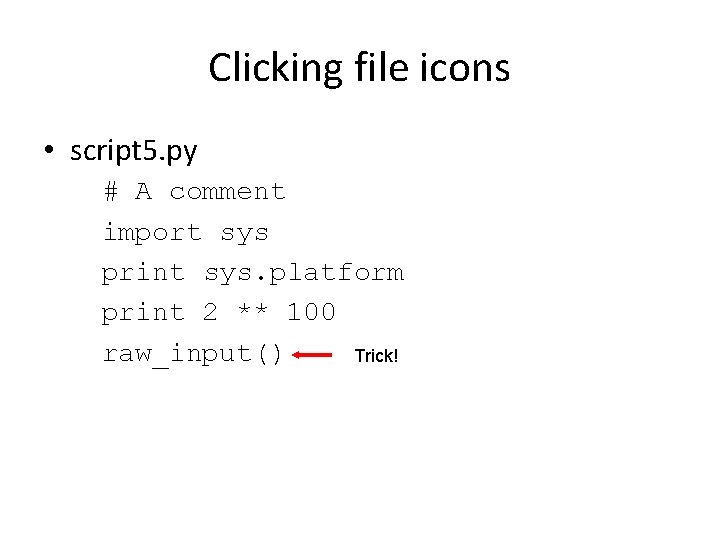
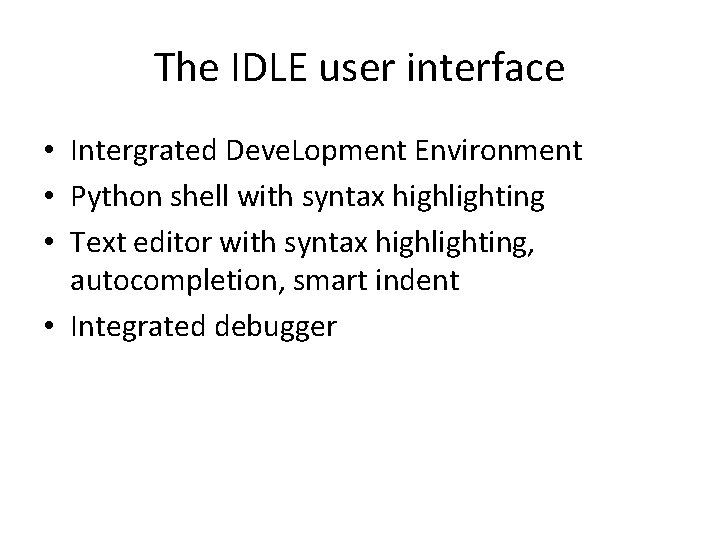
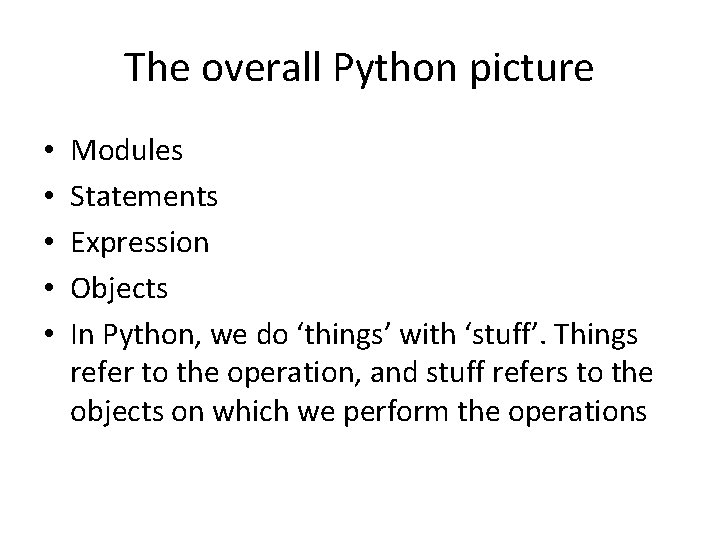
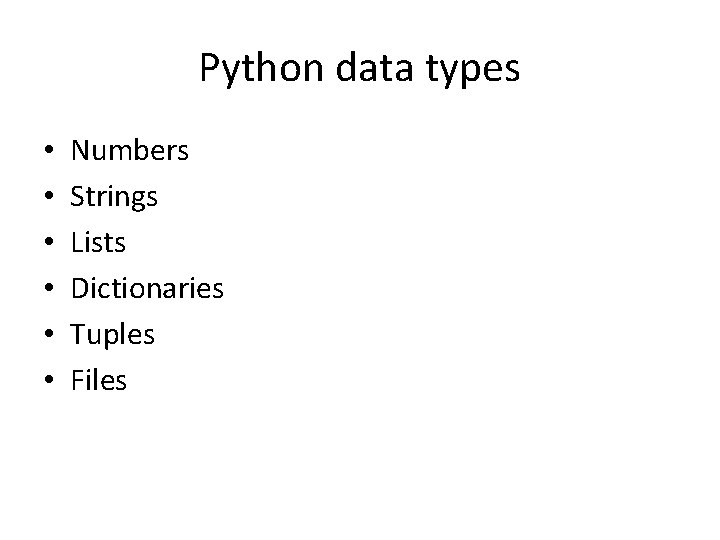
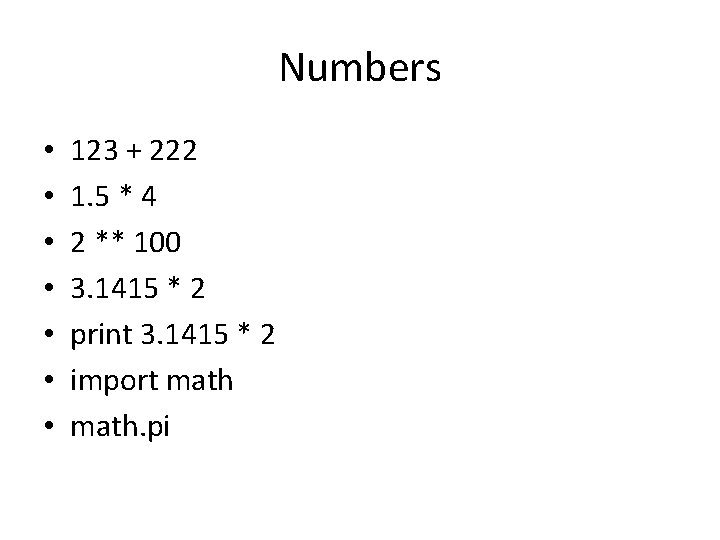
![Numbers • math. sqrt(85) • random() • random. choice([1, 2, 3, 4]) Numbers • math. sqrt(85) • random() • random. choice([1, 2, 3, 4])](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-15.jpg)
![Strings • • S = ‘spam’ len(S) S[0] S[1] S[-2] S S[1: 3] Strings • • S = ‘spam’ len(S) S[0] S[1] S[-2] S S[1: 3]](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-16.jpg)
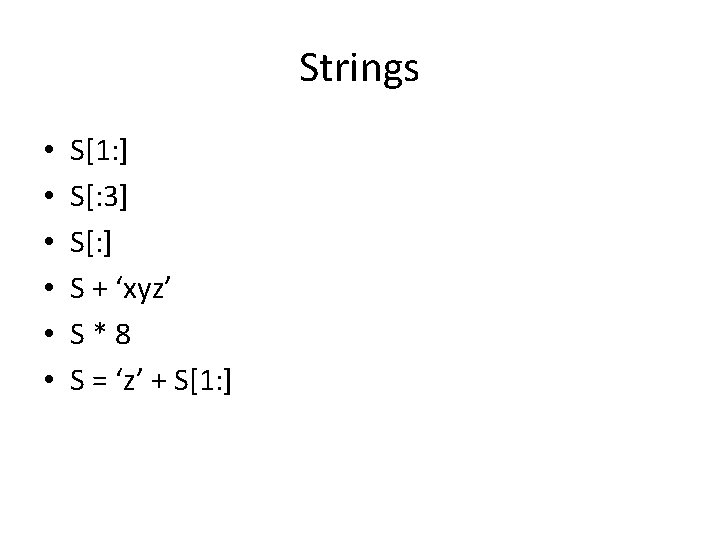
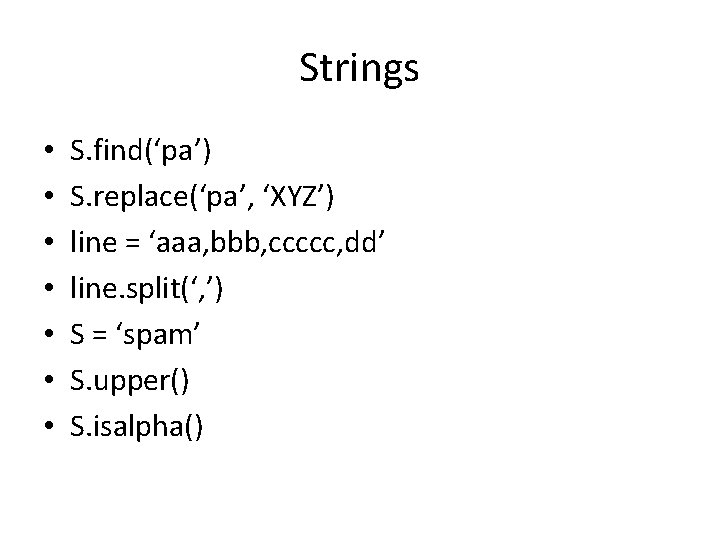
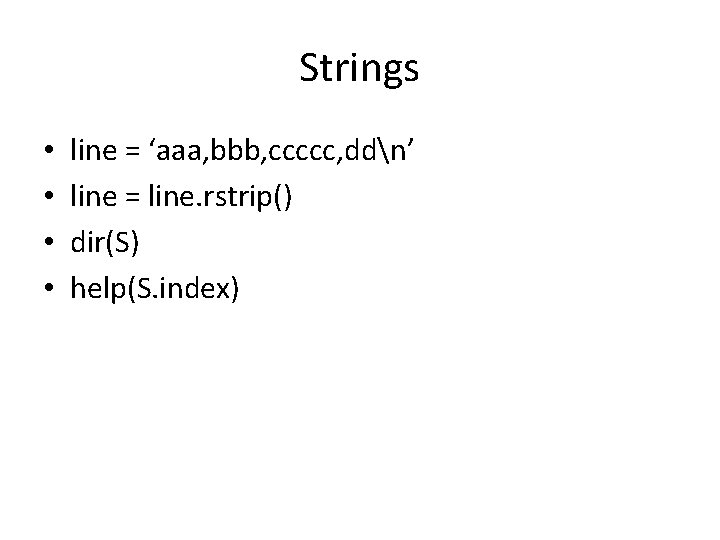
![Lists • • L = [123, 'spam', 1. 23] len(L) L[0] L[: -1] L Lists • • L = [123, 'spam', 1. 23] len(L) L[0] L[: -1] L](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-20.jpg)
![Lists • • • M = [‘bb’, ‘cc’, ‘aa’] M. sort() M. reverse() L[99] Lists • • • M = [‘bb’, ‘cc’, ‘aa’] M. sort() M. reverse() L[99]](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-21.jpg)
![Lists • M = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] Lists • M = [ [1, 2, 3], [4, 5, 6], [7, 8, 9]](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-22.jpg)
![Lists • • [row[1] + 1 for row in M] [row[1] for row in Lists • • [row[1] + 1 for row in M] [row[1] for row in](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-23.jpg)
![Dictionaries • • D = {'food': 'Spam', 'quantity': 4, 'color': 'pink'} D[‘food’] D[‘quantity’] += Dictionaries • • D = {'food': 'Spam', 'quantity': 4, 'color': 'pink'} D[‘food’] D[‘quantity’] +=](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-24.jpg)
![Dictionaries • rec = {'name': {'first': 'Bob', 'last': 'Smith'}, 'job': ['dev', 'mgr'], 'age': 40. Dictionaries • rec = {'name': {'first': 'Bob', 'last': 'Smith'}, 'job': ['dev', 'mgr'], 'age': 40.](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-25.jpg)
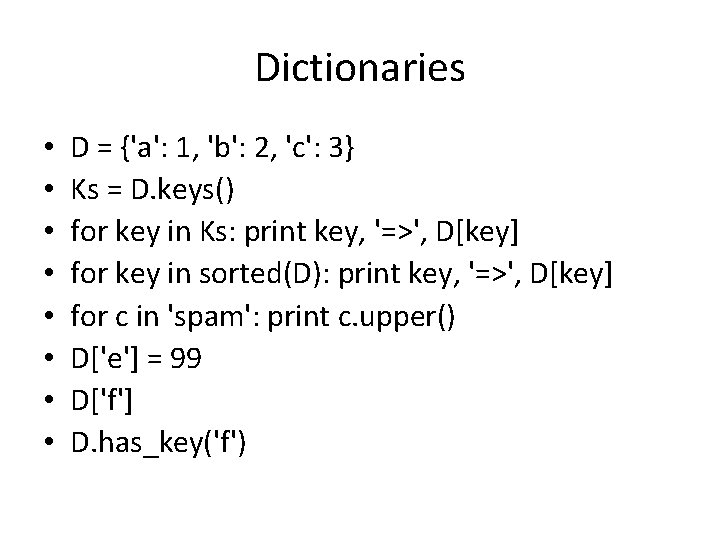
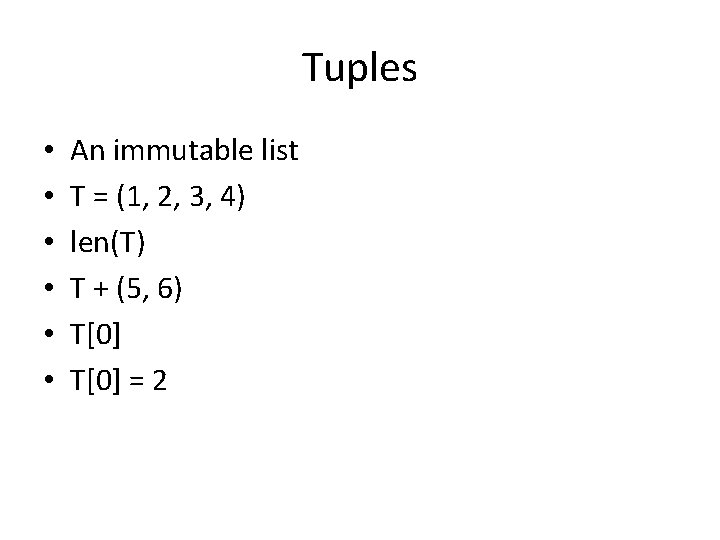
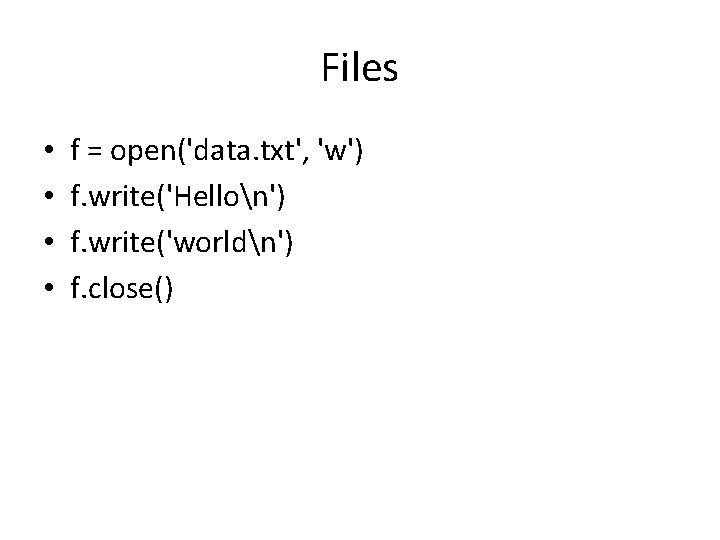
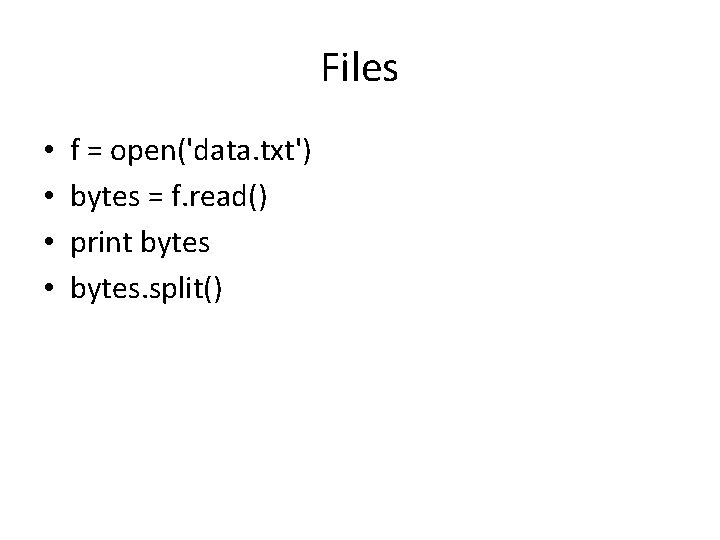
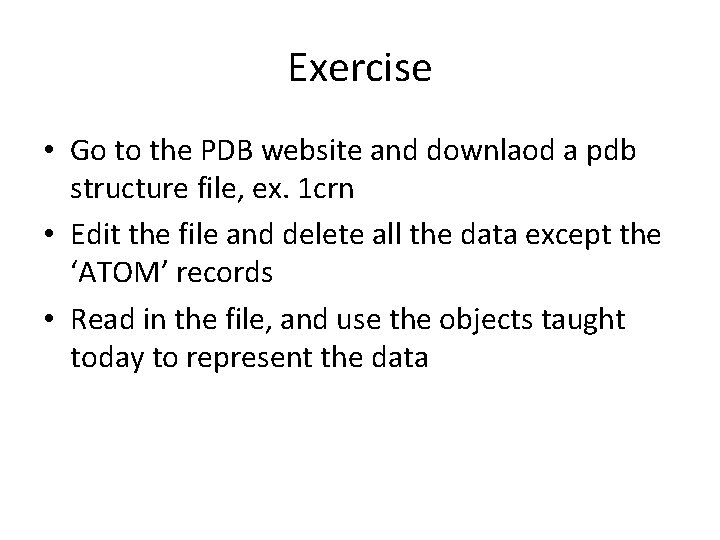
- Slides: 30
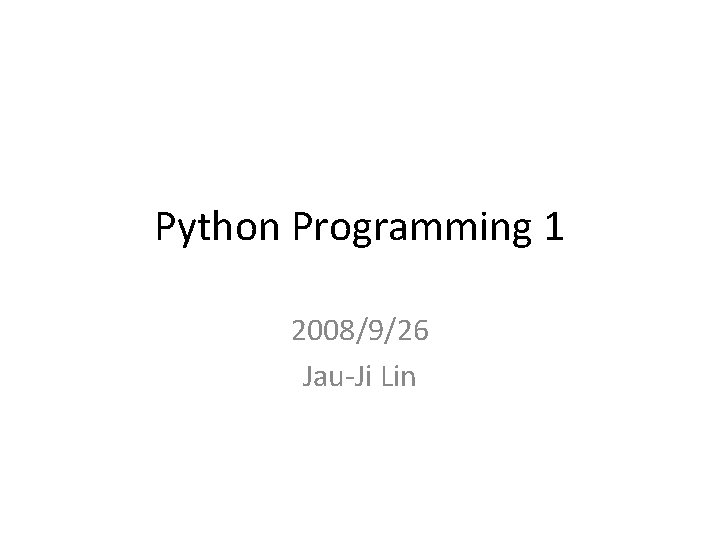
Python Programming 1 2008/9/26 Jau-Ji Lin
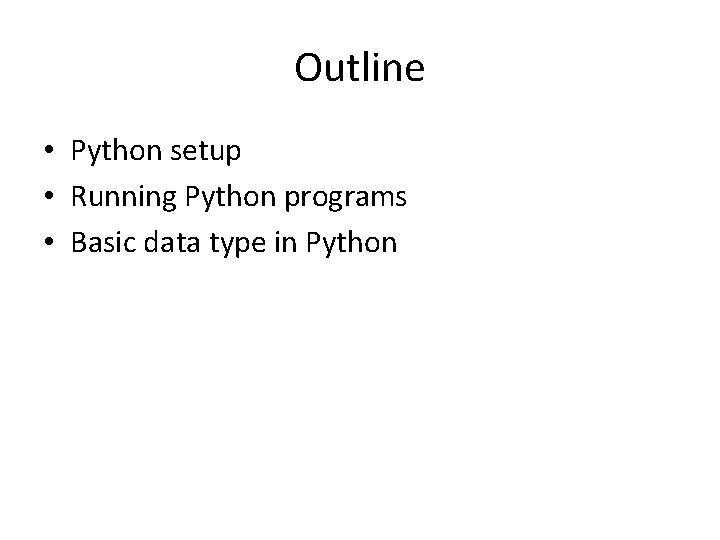
Outline • Python setup • Running Python programs • Basic data type in Python
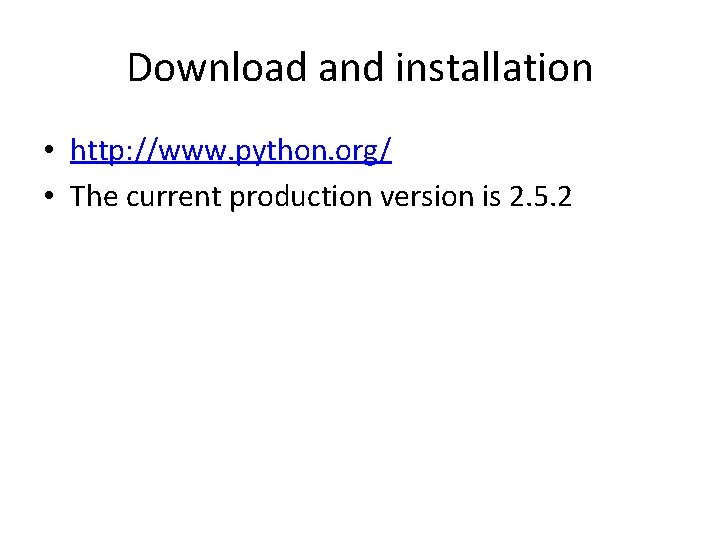
Download and installation • http: //www. python. org/ • The current production version is 2. 5. 2
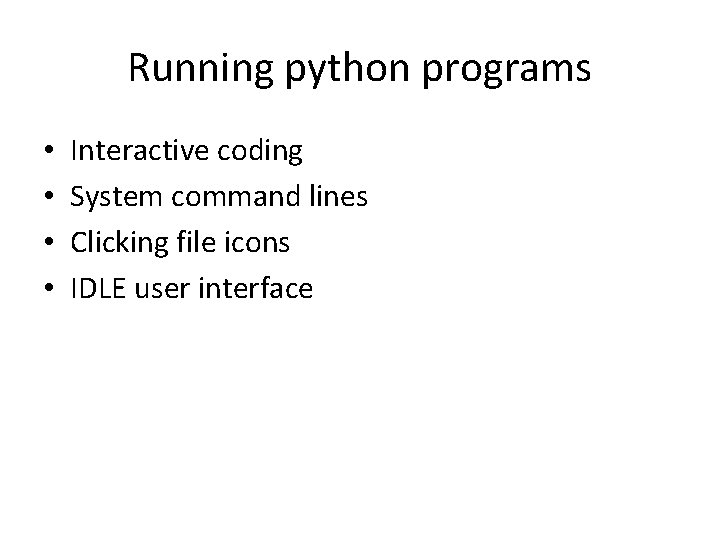
Running python programs • • Interactive coding System command lines Clicking file icons IDLE user interface
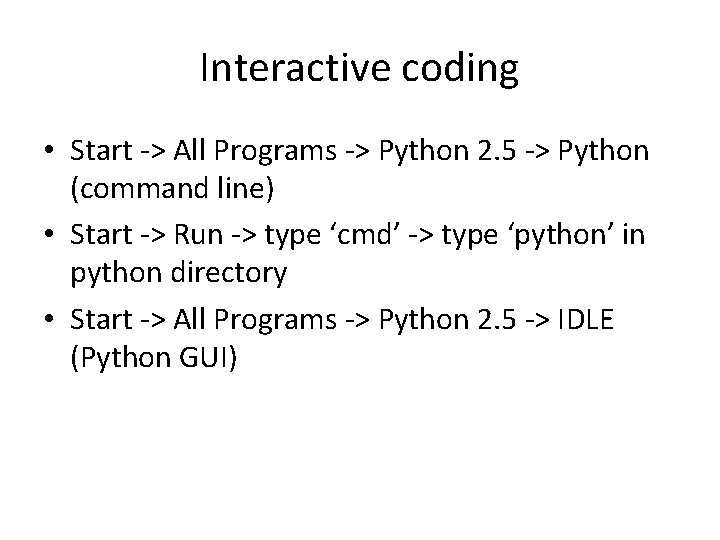
Interactive coding • Start -> All Programs -> Python 2. 5 -> Python (command line) • Start -> Run -> type ‘cmd’ -> type ‘python’ in python directory • Start -> All Programs -> Python 2. 5 -> IDLE (Python GUI)
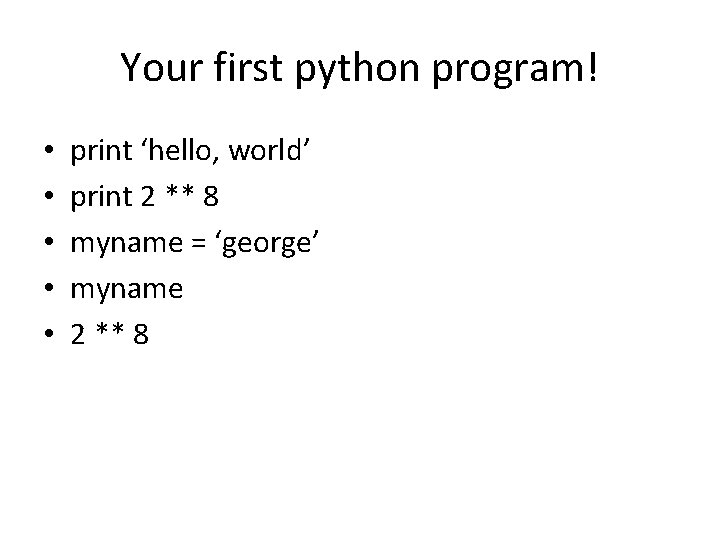
Your first python program! • • • print ‘hello, world’ print 2 ** 8 myname = ‘george’ myname 2 ** 8
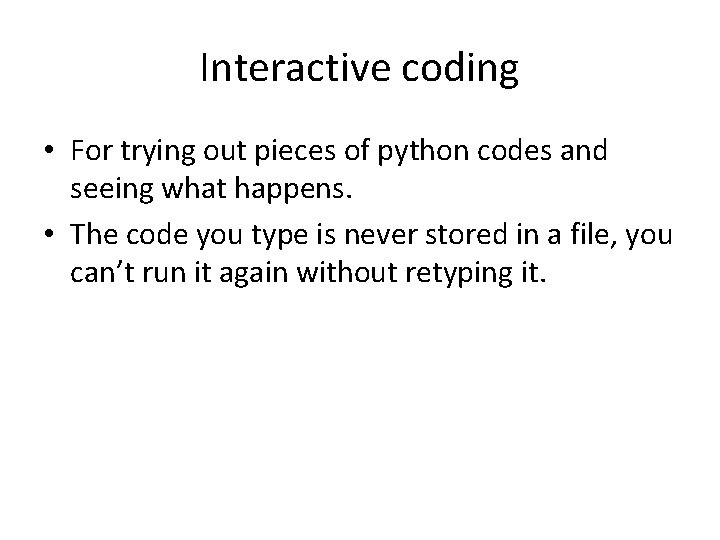
Interactive coding • For trying out pieces of python codes and seeing what happens. • The code you type is never stored in a file, you can’t run it again without retyping it.
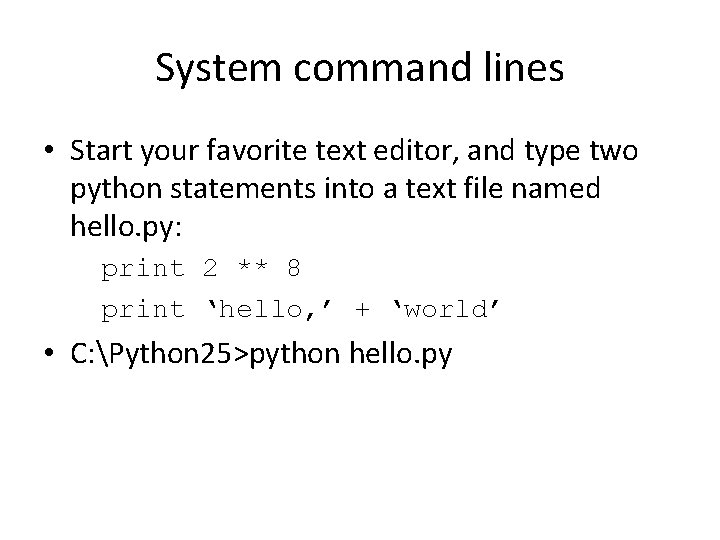
System command lines • Start your favorite text editor, and type two python statements into a text file named hello. py: print 2 ** 8 print ‘hello, ’ + ‘world’ • C: Python 25>python hello. py
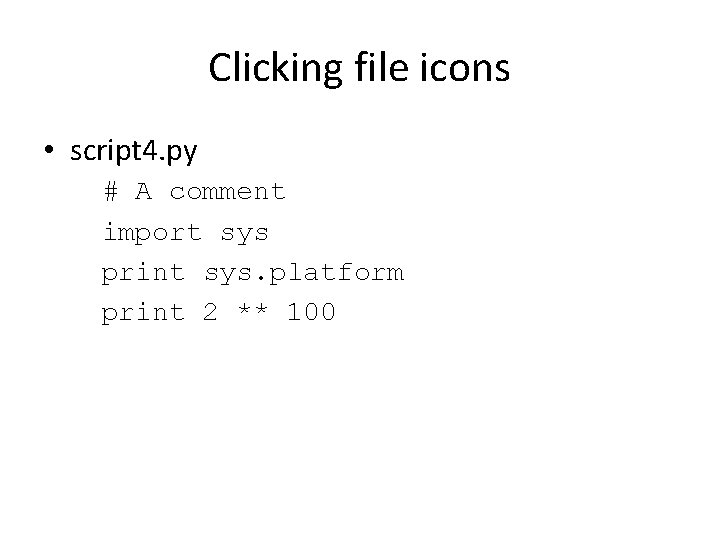
Clicking file icons • script 4. py # A comment import sys print sys. platform print 2 ** 100
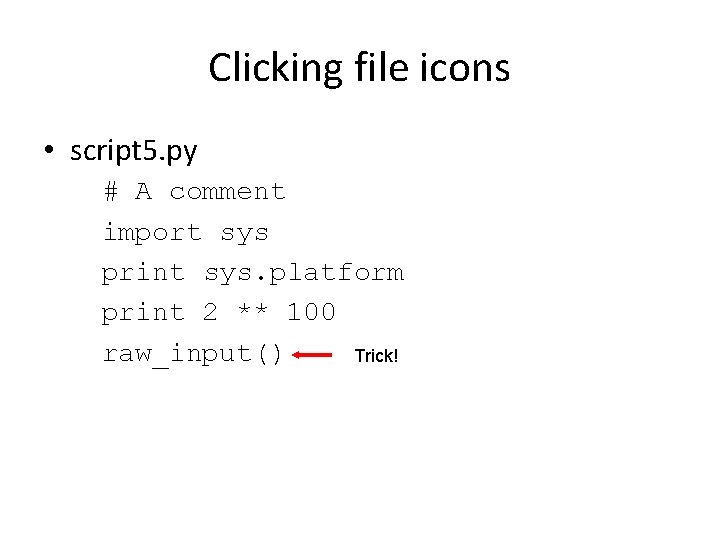
Clicking file icons • script 5. py # A comment import sys print sys. platform print 2 ** 100 raw_input() Trick!
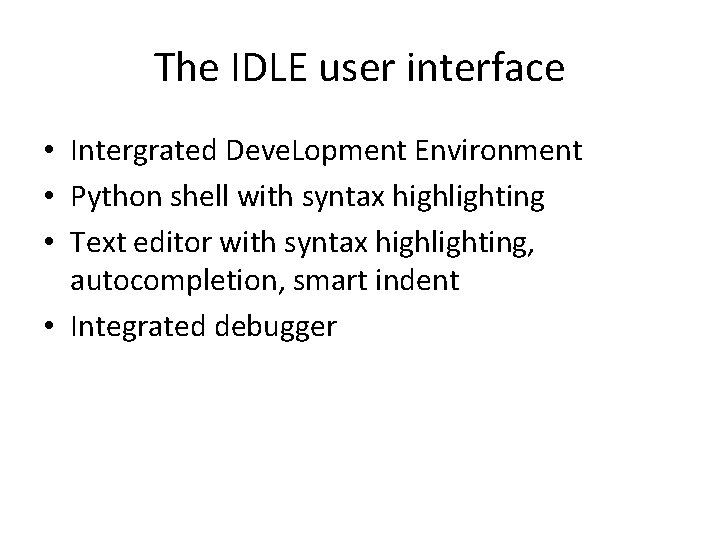
The IDLE user interface • Intergrated Deve. Lopment Environment • Python shell with syntax highlighting • Text editor with syntax highlighting, autocompletion, smart indent • Integrated debugger
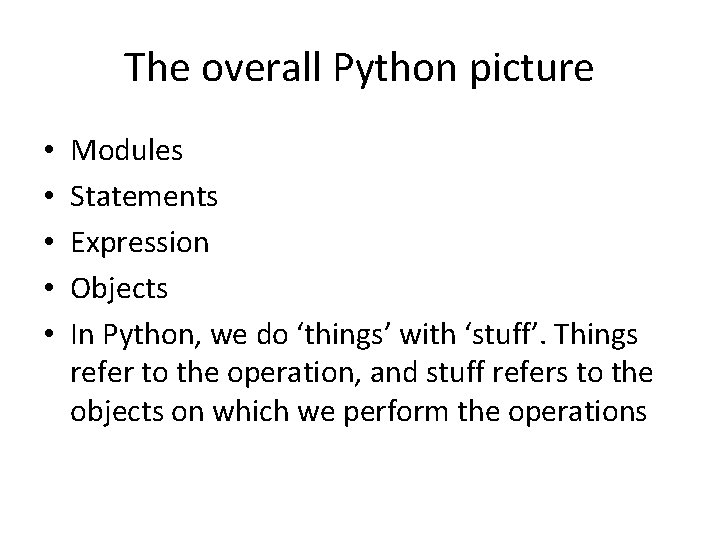
The overall Python picture • • • Modules Statements Expression Objects In Python, we do ‘things’ with ‘stuff’. Things refer to the operation, and stuff refers to the objects on which we perform the operations
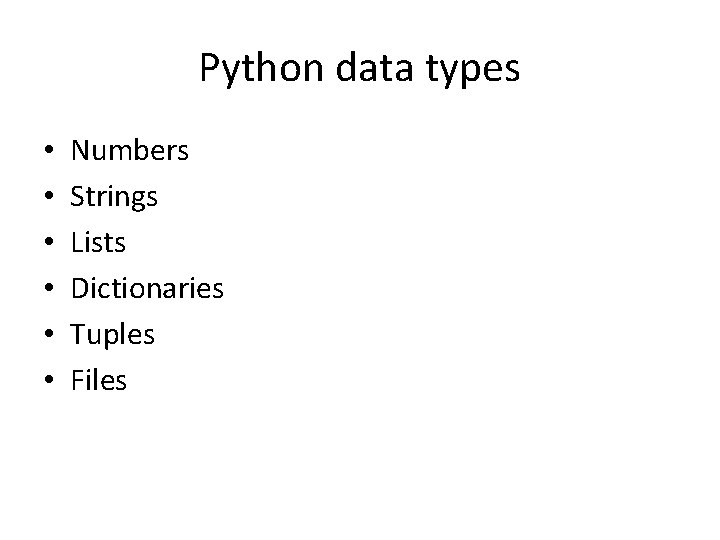
Python data types • • • Numbers Strings Lists Dictionaries Tuples Files
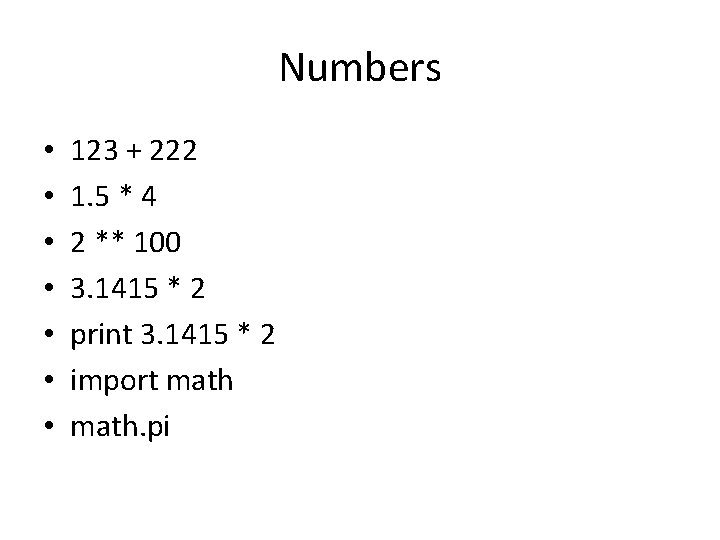
Numbers • • 123 + 222 1. 5 * 4 2 ** 100 3. 1415 * 2 print 3. 1415 * 2 import math. pi
![Numbers math sqrt85 random random choice1 2 3 4 Numbers • math. sqrt(85) • random() • random. choice([1, 2, 3, 4])](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-15.jpg)
Numbers • math. sqrt(85) • random() • random. choice([1, 2, 3, 4])
![Strings S spam lenS S0 S1 S2 S S1 3 Strings • • S = ‘spam’ len(S) S[0] S[1] S[-2] S S[1: 3]](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-16.jpg)
Strings • • S = ‘spam’ len(S) S[0] S[1] S[-2] S S[1: 3]
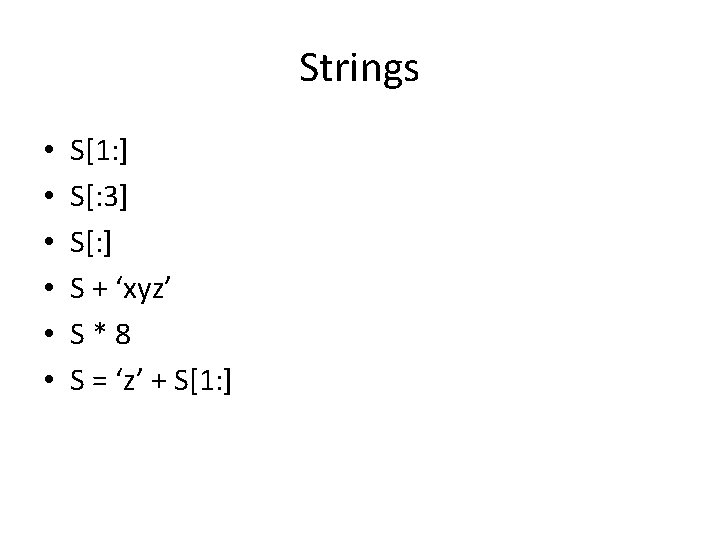
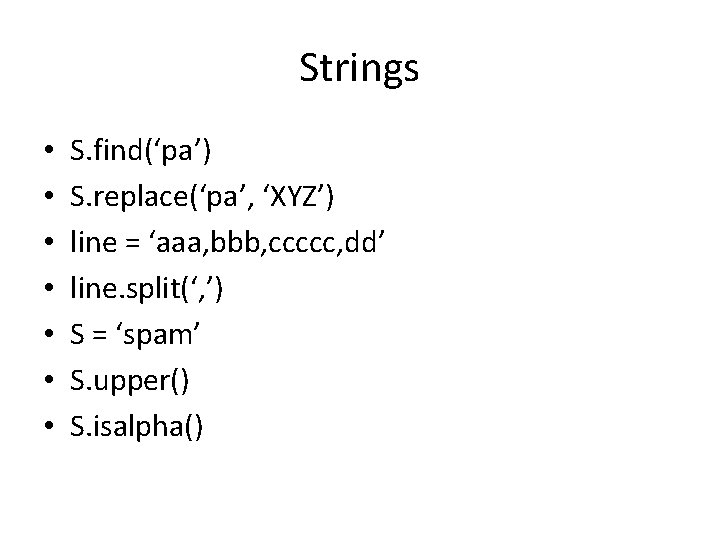
Strings • • S. find(‘pa’) S. replace(‘pa’, ‘XYZ’) line = ‘aaa, bbb, ccccc, dd’ line. split(‘, ’) S = ‘spam’ S. upper() S. isalpha()
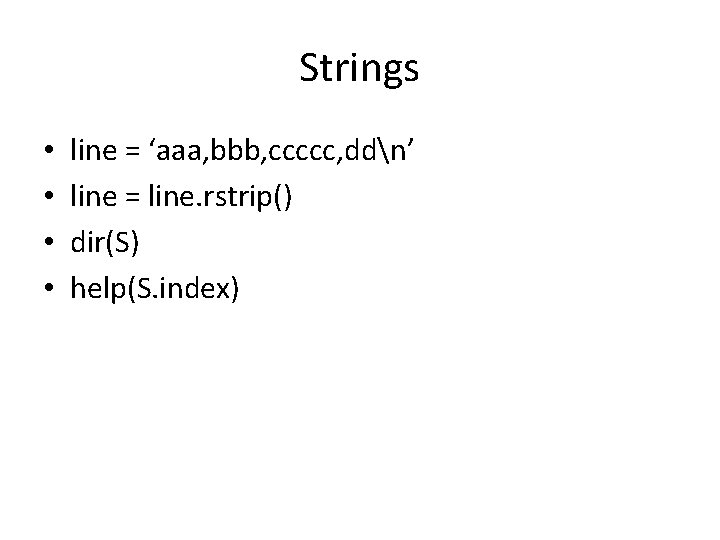
Strings • • line = ‘aaa, bbb, ccccc, ddn’ line = line. rstrip() dir(S) help(S. index)
![Lists L 123 spam 1 23 lenL L0 L 1 L Lists • • L = [123, 'spam', 1. 23] len(L) L[0] L[: -1] L](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-20.jpg)
Lists • • L = [123, 'spam', 1. 23] len(L) L[0] L[: -1] L + [4, 5, 6] L. append(‘NI’) L. pop(2)
![Lists M bb cc aa M sort M reverse L99 Lists • • • M = [‘bb’, ‘cc’, ‘aa’] M. sort() M. reverse() L[99]](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-21.jpg)
Lists • • • M = [‘bb’, ‘cc’, ‘aa’] M. sort() M. reverse() L[99] = 1
![Lists M 1 2 3 4 5 6 7 8 9 Lists • M = [ [1, 2, 3], [4, 5, 6], [7, 8, 9]](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-22.jpg)
Lists • M = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] • M[1][2] • col 2 = [row[1] for row in M]
![Lists row1 1 for row in M row1 for row in Lists • • [row[1] + 1 for row in M] [row[1] for row in](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-23.jpg)
Lists • • [row[1] + 1 for row in M] [row[1] for row in M if row[1] % 2 == 0] diag = [M[i][i] for i in [0, 1, 2]] doubles = [c * 2 for c in 'spam']
![Dictionaries D food Spam quantity 4 color pink Dfood Dquantity Dictionaries • • D = {'food': 'Spam', 'quantity': 4, 'color': 'pink'} D[‘food’] D[‘quantity’] +=](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-24.jpg)
Dictionaries • • D = {'food': 'Spam', 'quantity': 4, 'color': 'pink'} D[‘food’] D[‘quantity’] += 1 D = {} D[‘name’] = ‘Bob’ D[‘job’] = ‘dev’ D[‘age’] = 40
![Dictionaries rec name first Bob last Smith job dev mgr age 40 Dictionaries • rec = {'name': {'first': 'Bob', 'last': 'Smith'}, 'job': ['dev', 'mgr'], 'age': 40.](https://slidetodoc.com/presentation_image_h2/16ab90234e857cf636295e4cd0e8aa91/image-25.jpg)
Dictionaries • rec = {'name': {'first': 'Bob', 'last': 'Smith'}, 'job': ['dev', 'mgr'], 'age': 40. 5} • rec['name']['last'] • rec['job'][-1] • rec['job']. append('janitor') • rec = 0
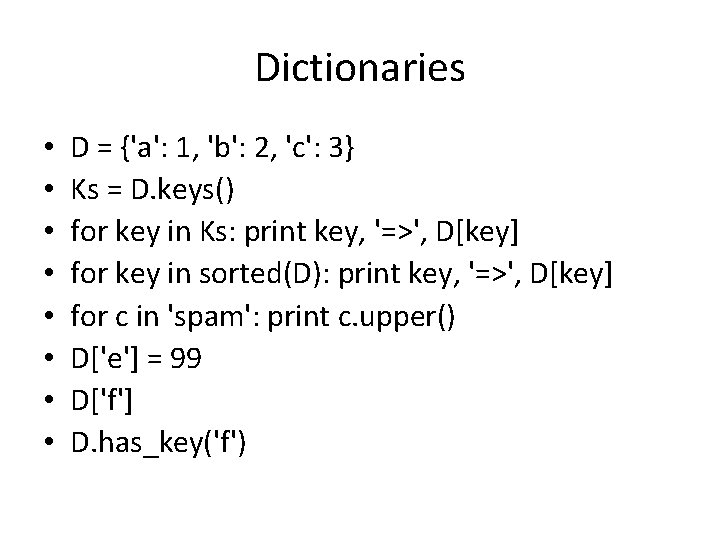
Dictionaries • • D = {'a': 1, 'b': 2, 'c': 3} Ks = D. keys() for key in Ks: print key, '=>', D[key] for key in sorted(D): print key, '=>', D[key] for c in 'spam': print c. upper() D['e'] = 99 D['f'] D. has_key('f')
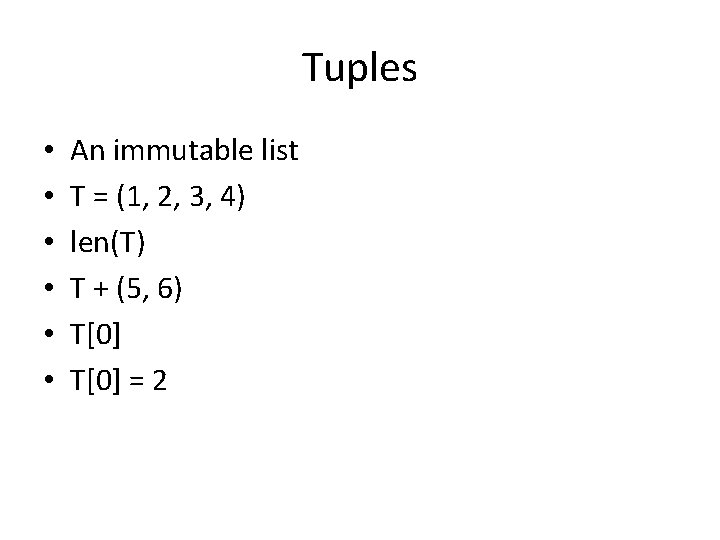
Tuples • • • An immutable list T = (1, 2, 3, 4) len(T) T + (5, 6) T[0] = 2
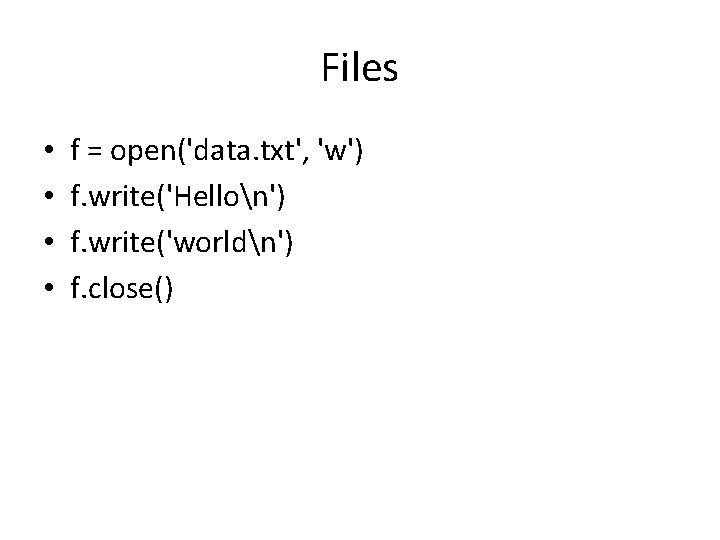
Files • • f = open('data. txt', 'w') f. write('Hellon') f. write('worldn') f. close()
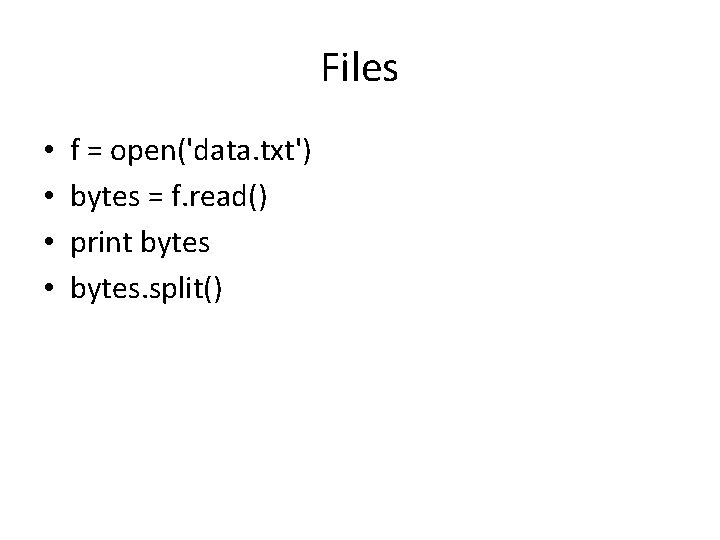
Files • • f = open('data. txt') bytes = f. read() print bytes. split()
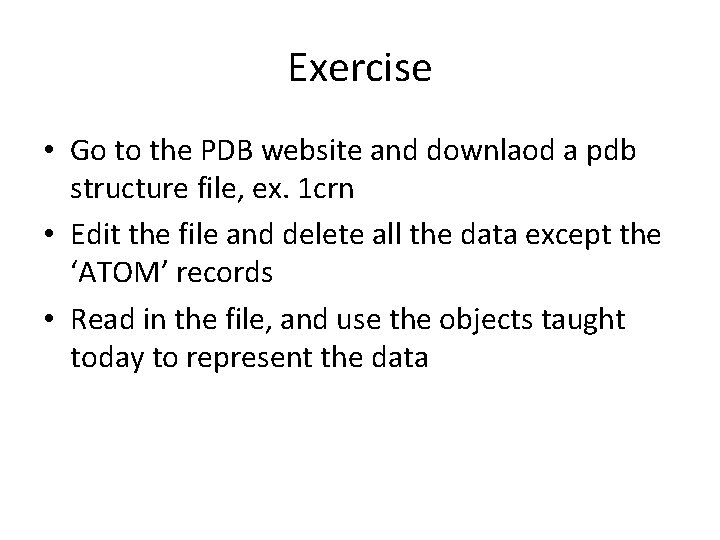
Exercise • Go to the PDB website and downlaod a pdb structure file, ex. 1 crn • Edit the file and delete all the data except the ‘ATOM’ records • Read in the file, and use the objects taught today to represent the data
What is sentence outline
Visual programming course outline
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
What is system programing
Integer programming vs linear programming
Programing adalah
Procedural programming python
Python chapter 5 programming exercises
Second order cone programming python
Python programming in context
Constraint programming python
Rapid gui programming with python and qt
Python programming in context
Python cgi programming examples
Audiolab python
Python programming
Programming essentials in python
Blackjack python object oriented
Python programming an introduction to computer science
Grad rebel slam
Va dơ lin
Meddi lin ne demek
Dr vivian lin
Modelo reciproco
Alison lin nci
De lin institute of technology
Kevin kelly cmu
Constance lin
Lin donn
Calvin lin ut austin