PROGRAMMING IN HASKELL Type declarations and Modules let
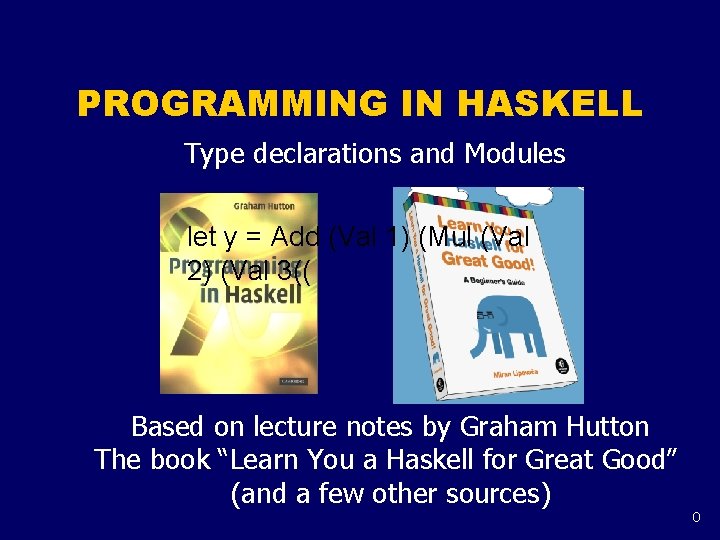
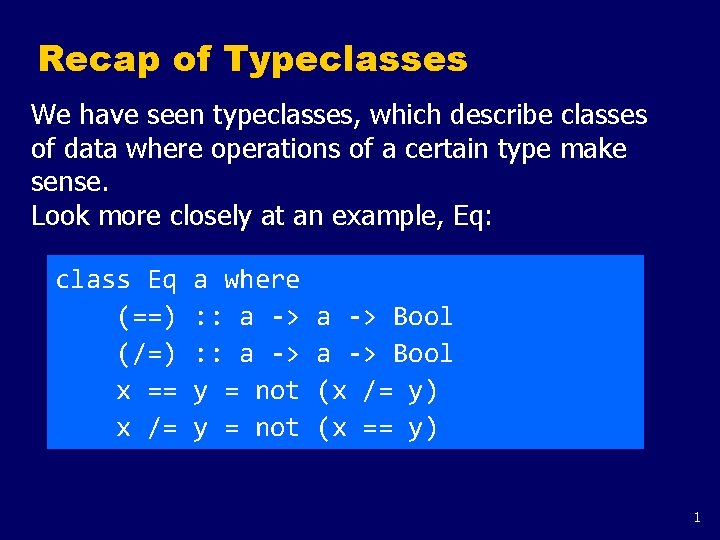
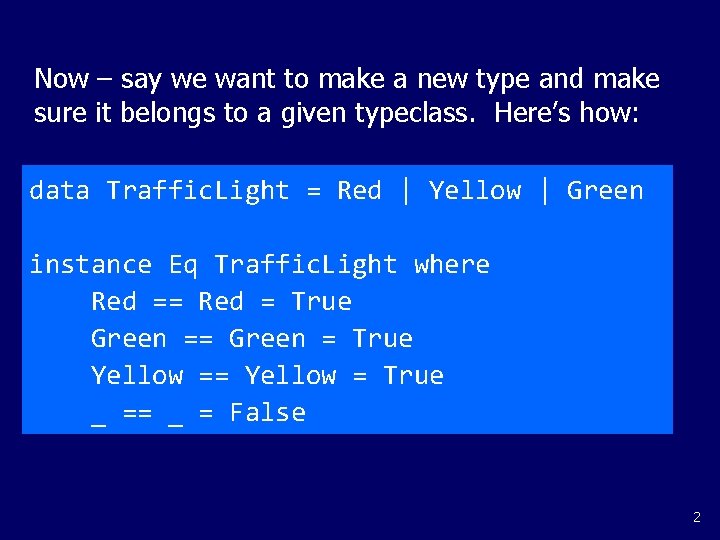
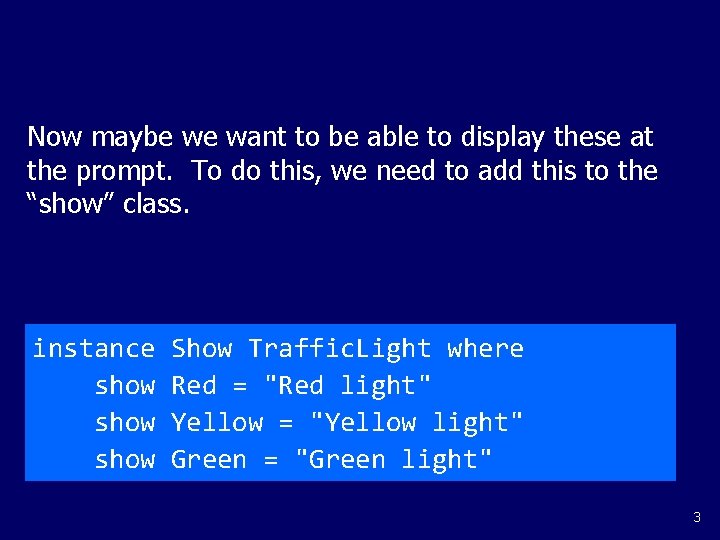
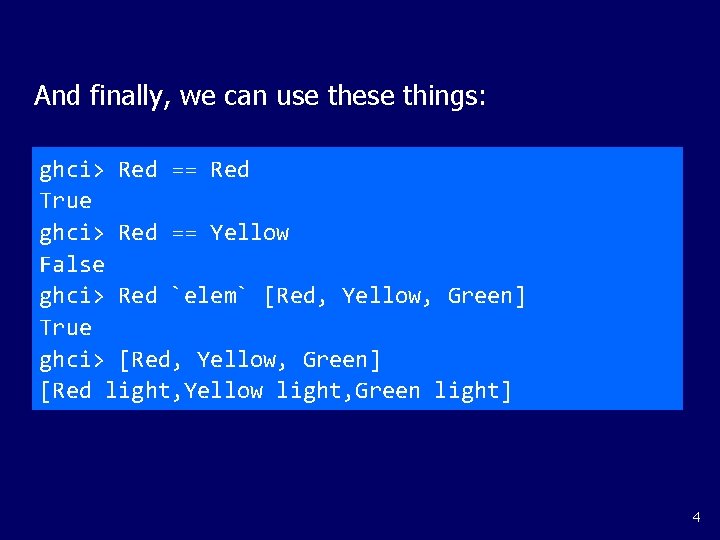
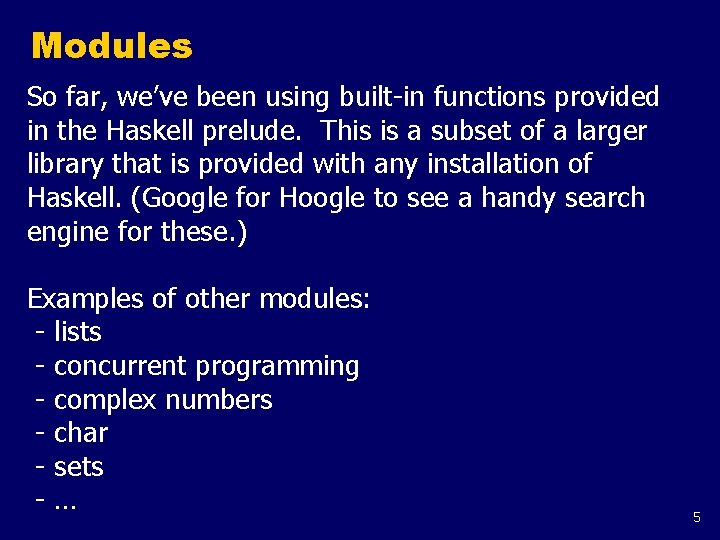
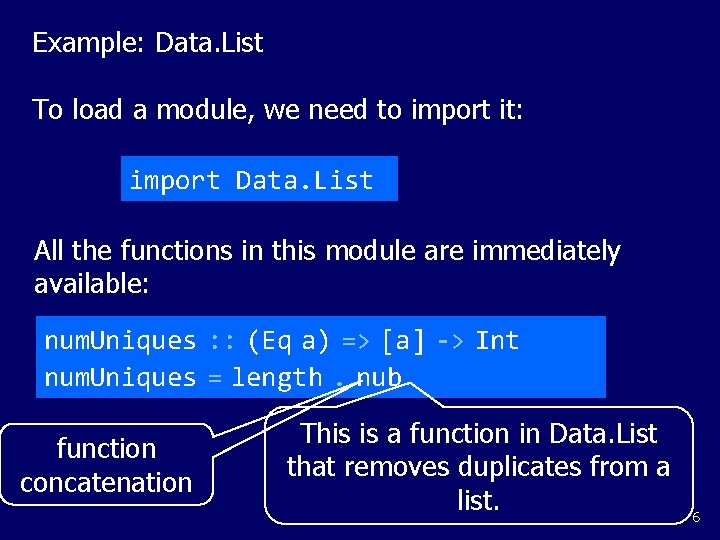
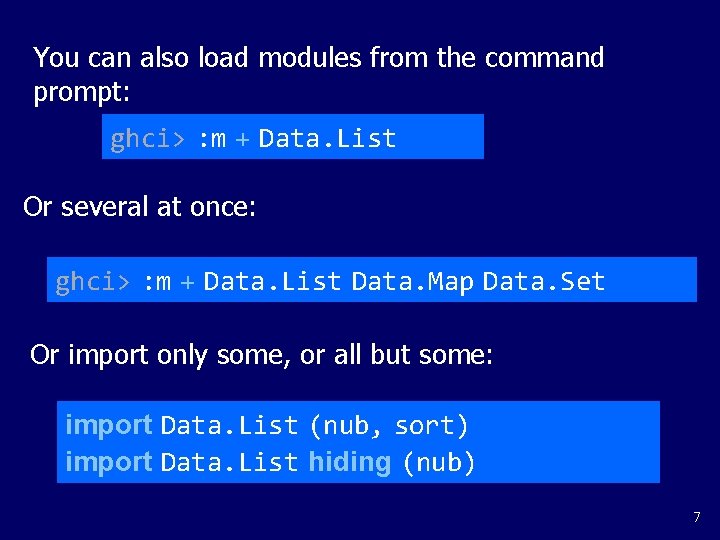
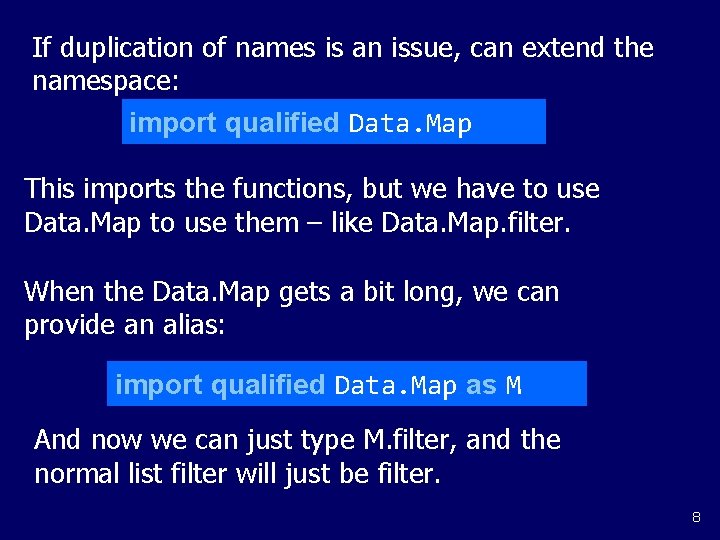
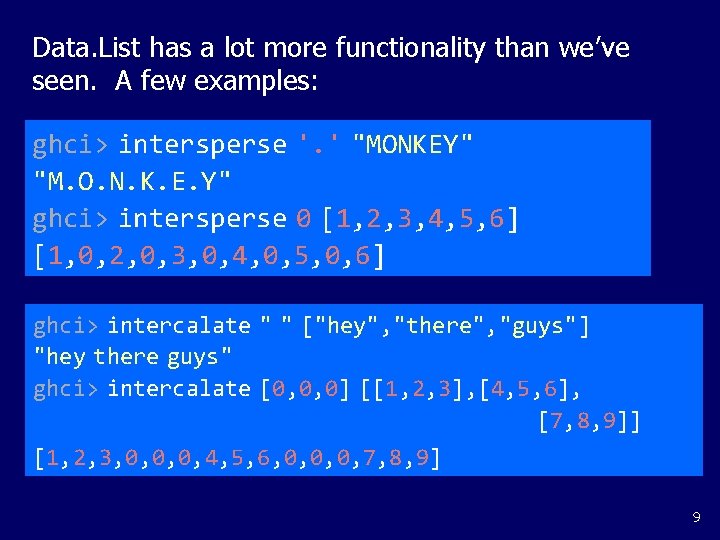
![And even more: ghci> transpose [[1, 2, 3], [4, 5, 6], [7, 8, 9]] And even more: ghci> transpose [[1, 2, 3], [4, 5, 6], [7, 8, 9]]](https://slidetodoc.com/presentation_image_h/d09f62bd6630f8887ce4bcf27a00ba05/image-11.jpg)
![And even more: ghci> and $ map (>4) [5, 6, 7, 8] True ghci> And even more: ghci> and $ map (>4) [5, 6, 7, 8] True ghci>](https://slidetodoc.com/presentation_image_h/d09f62bd6630f8887ce4bcf27a00ba05/image-12.jpg)
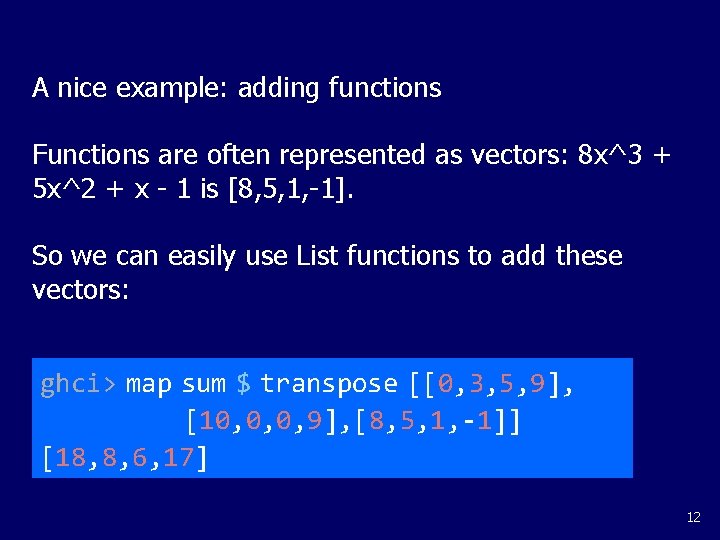
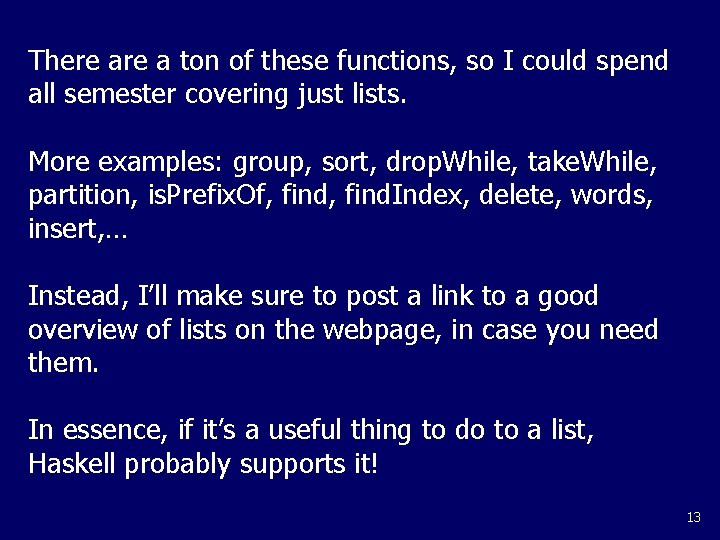
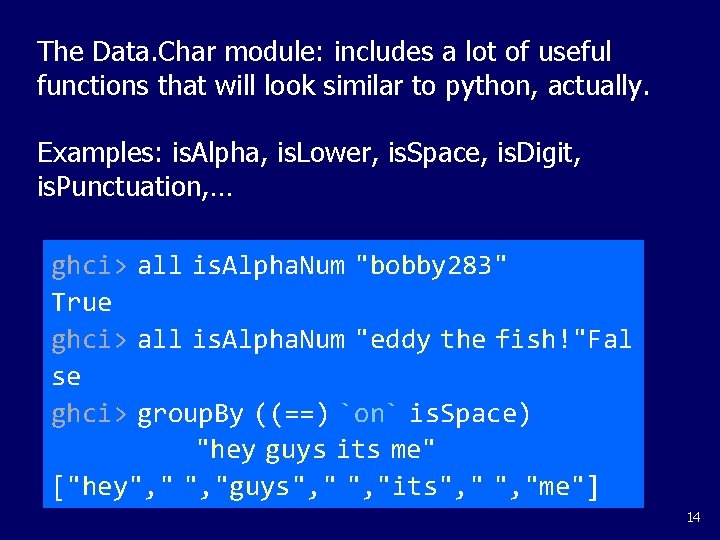
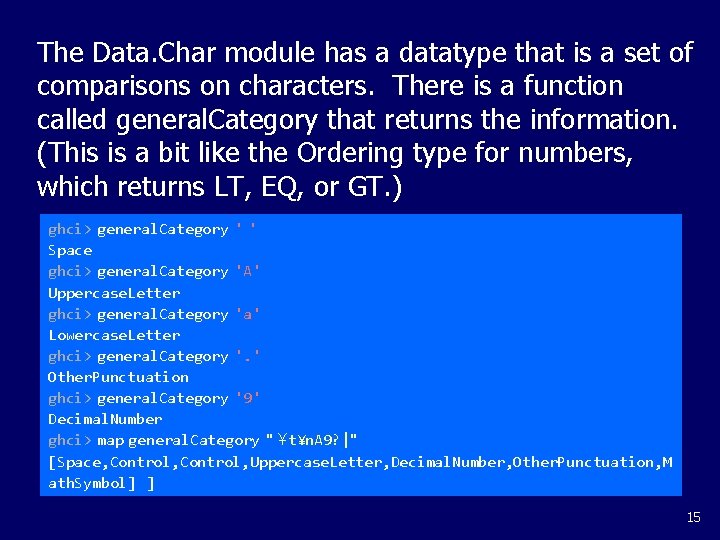
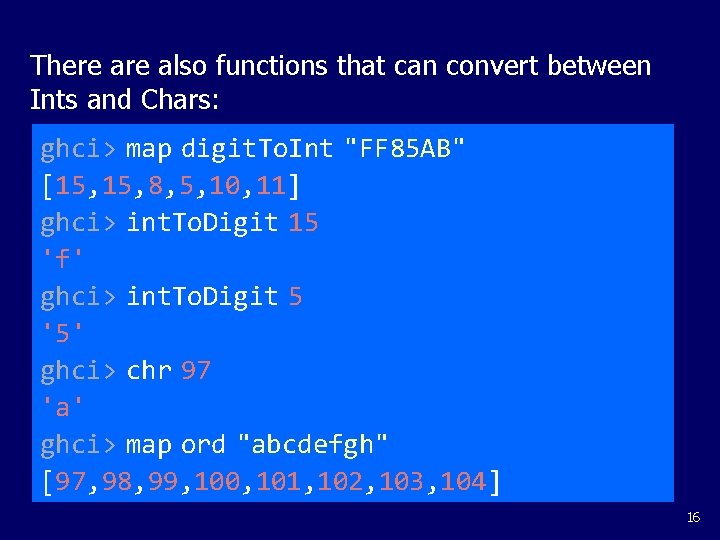
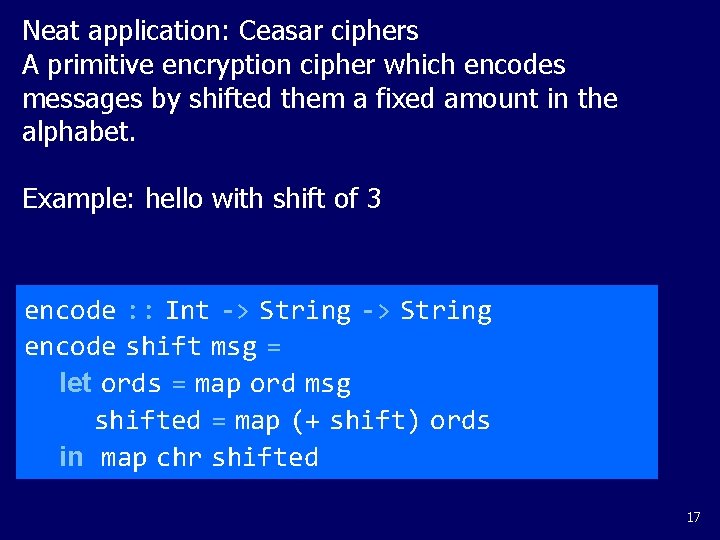
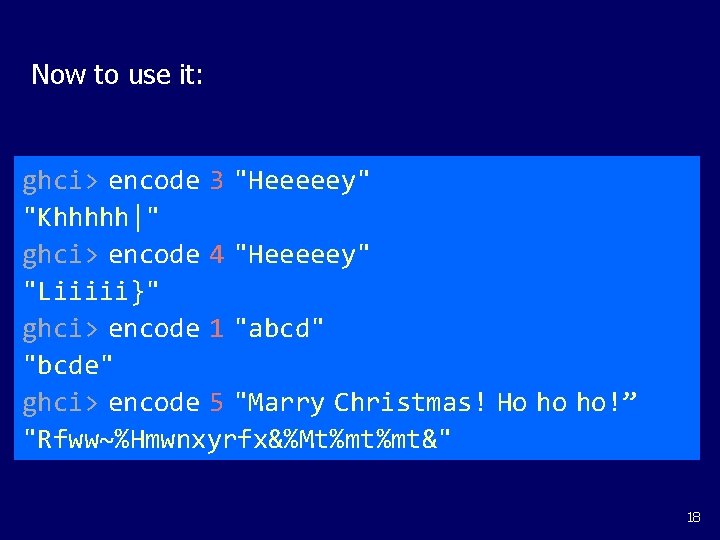
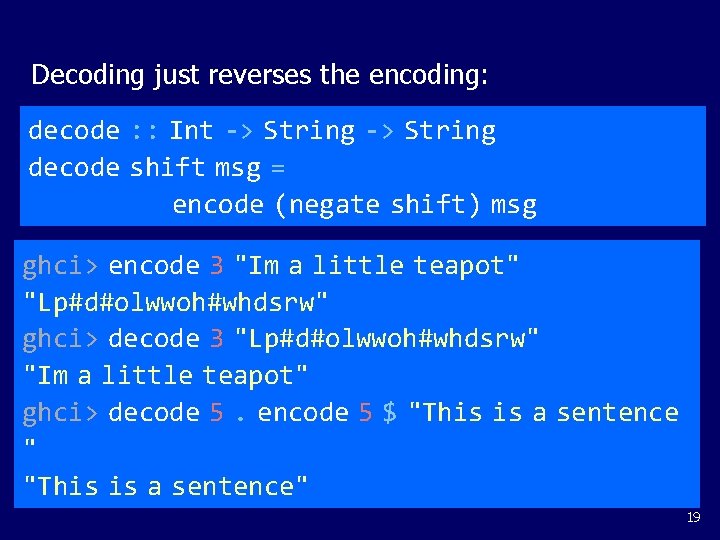
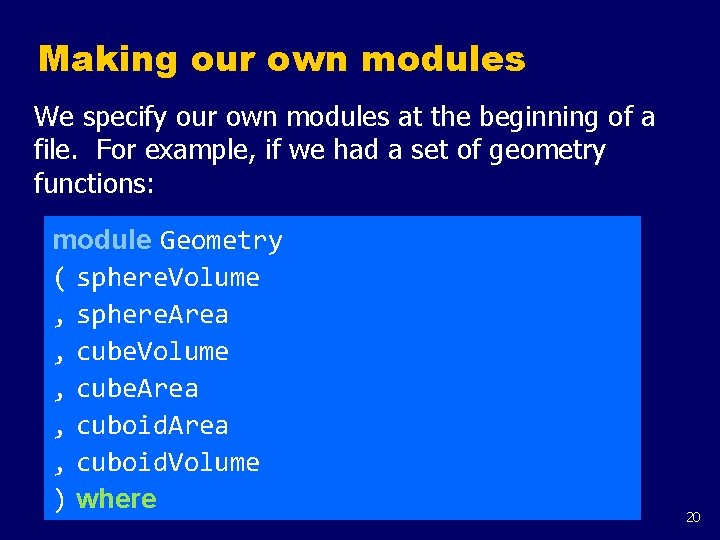
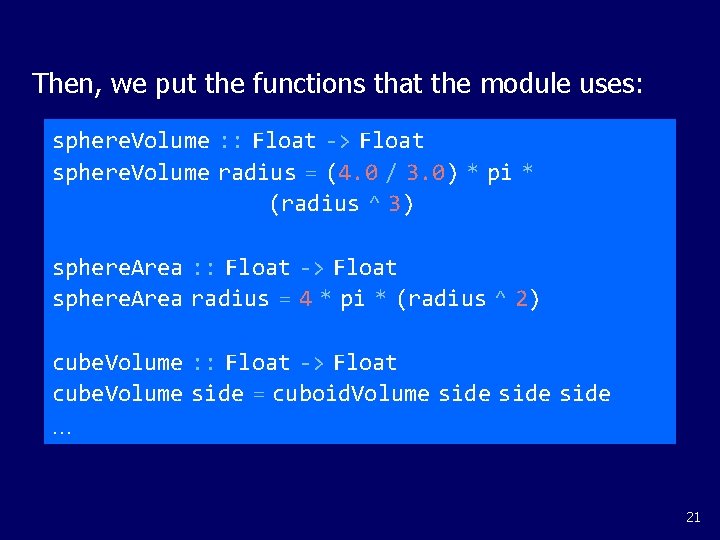
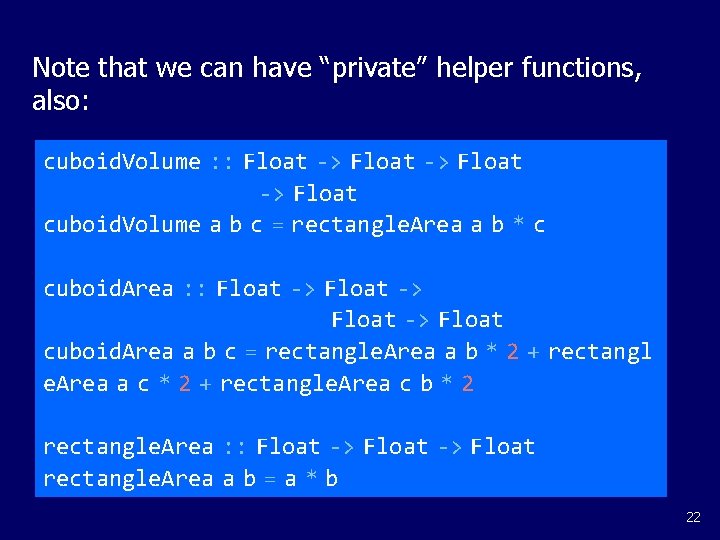
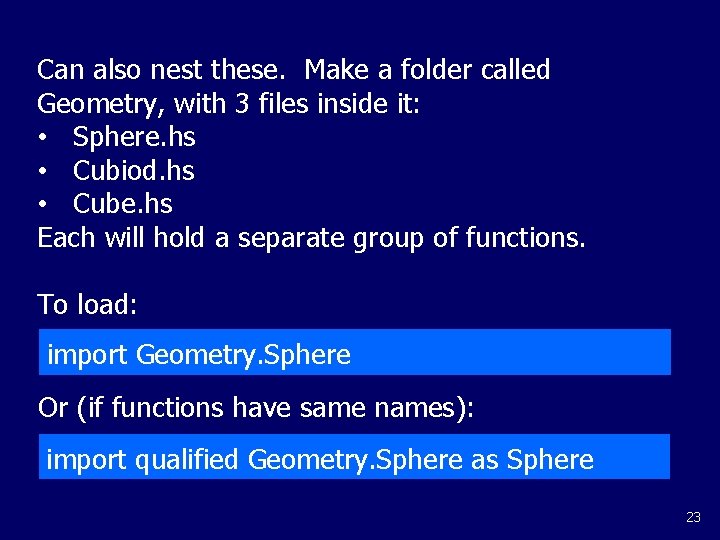
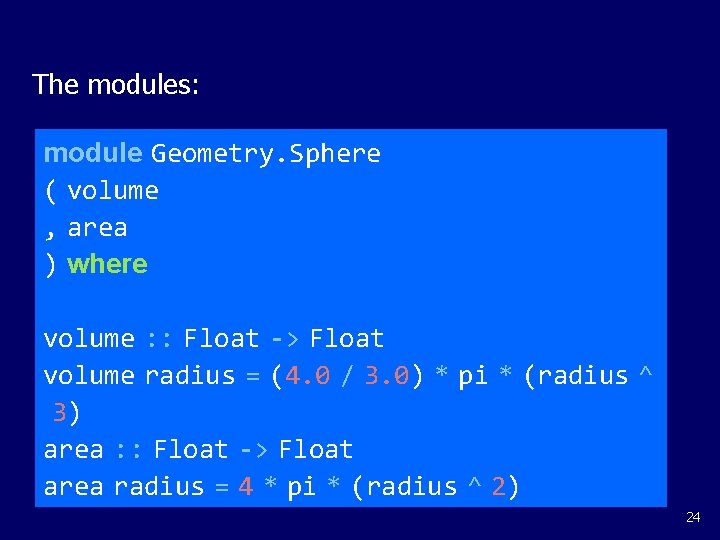
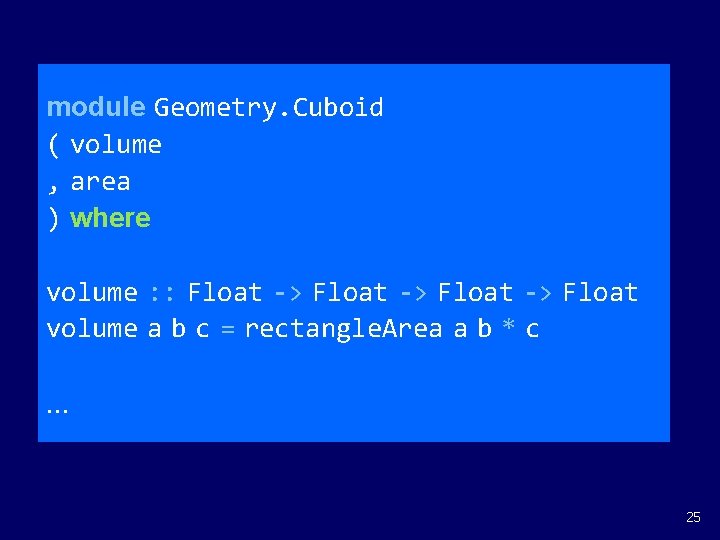
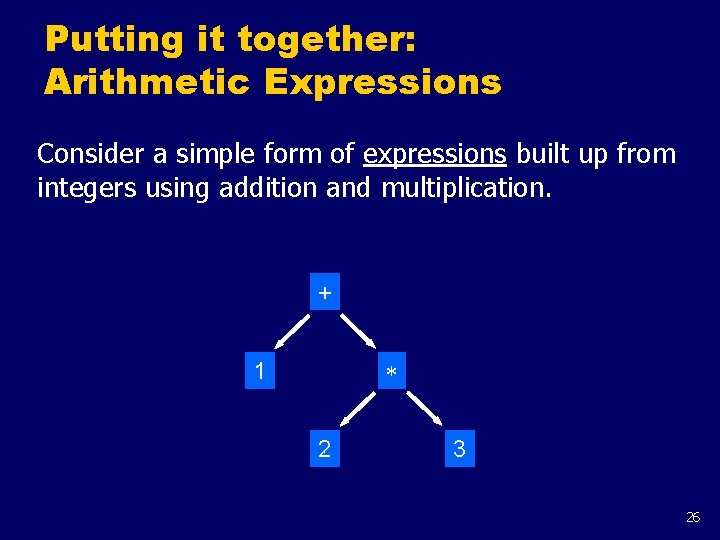
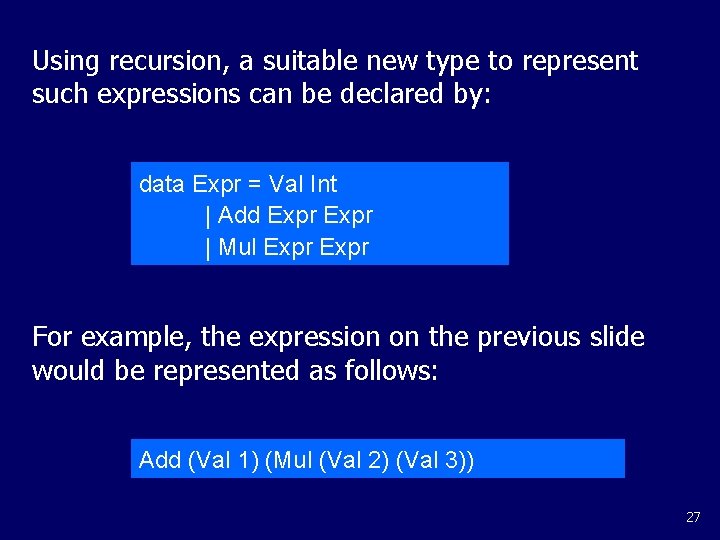
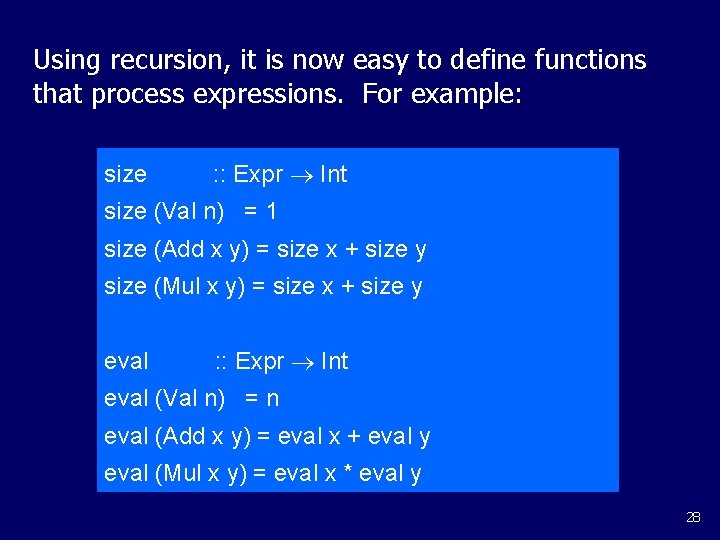
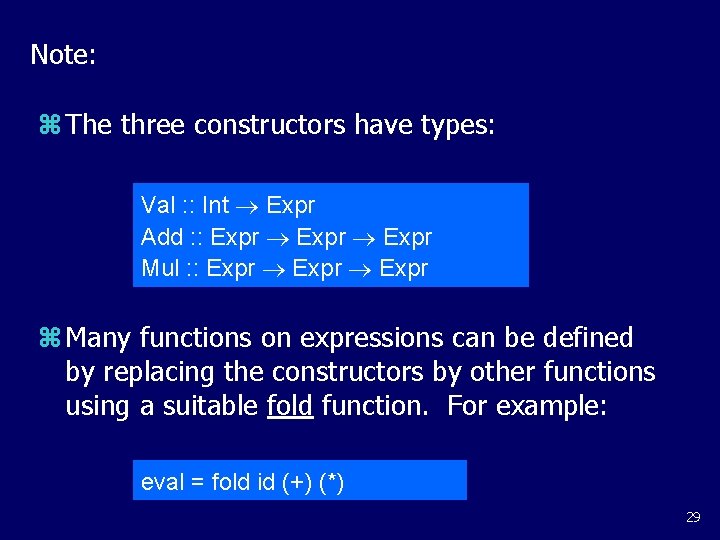
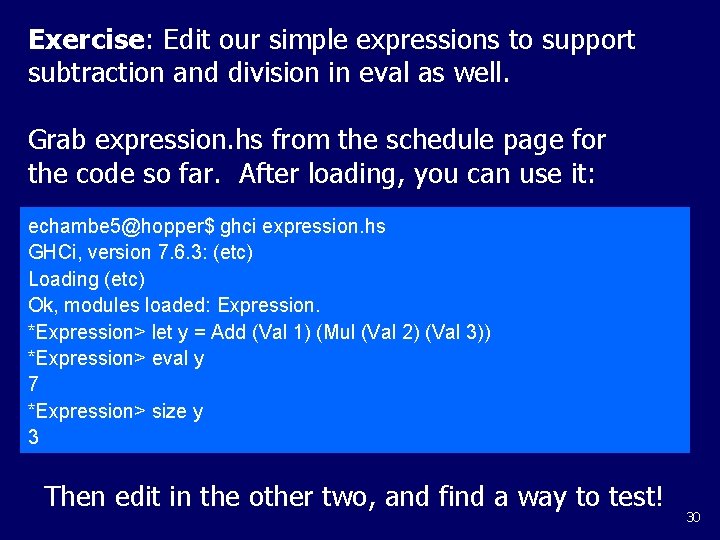
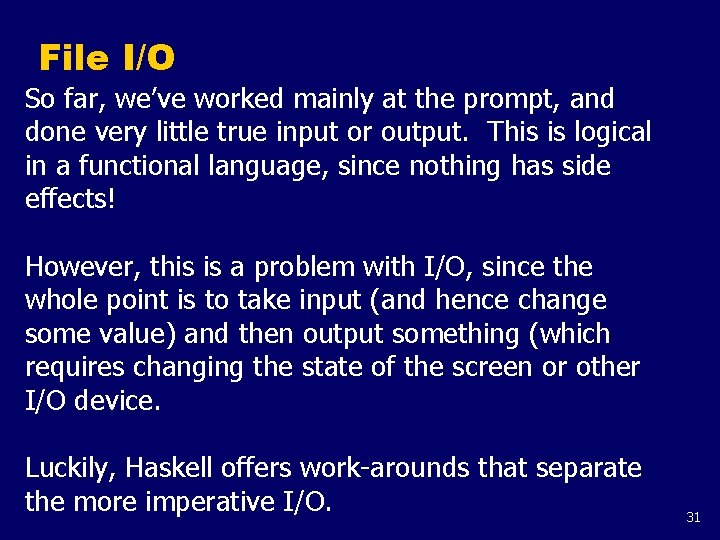
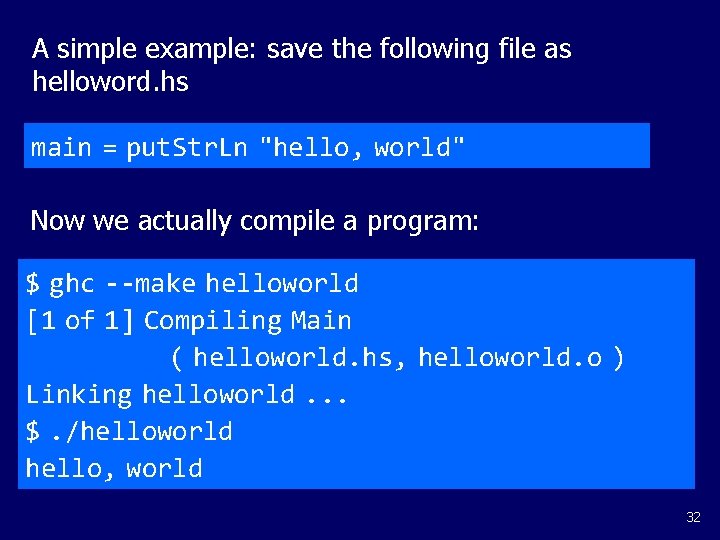
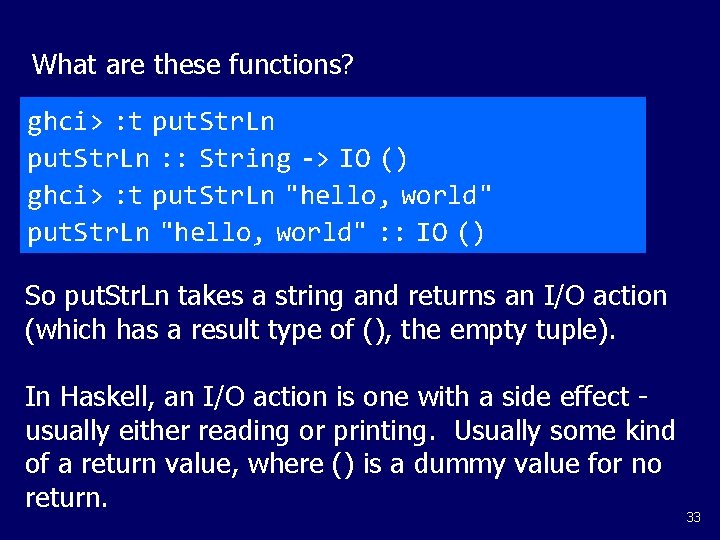
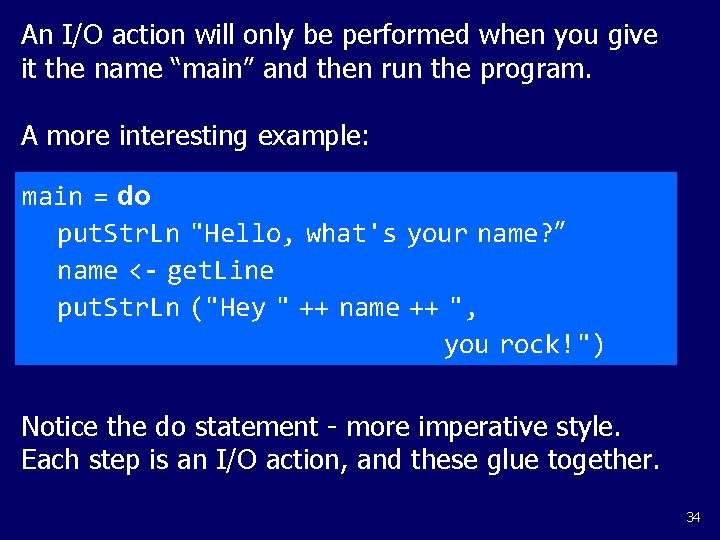
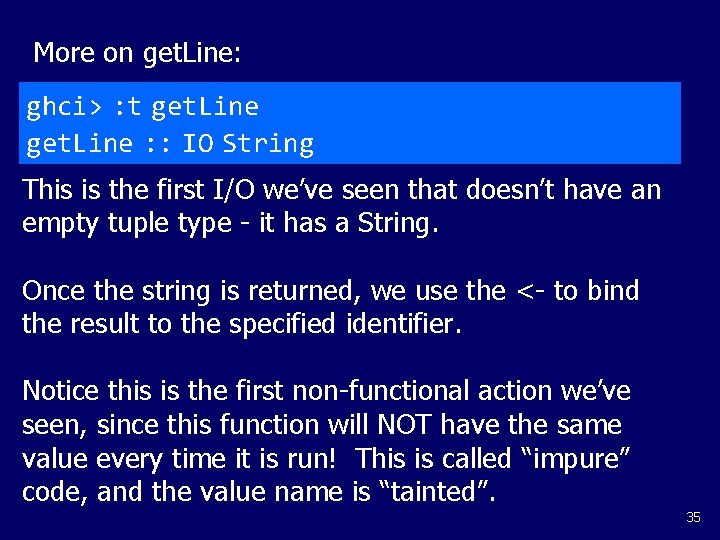
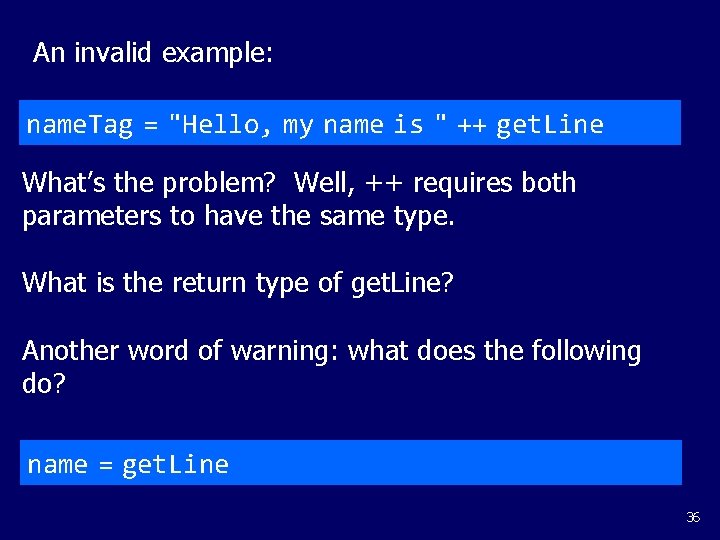
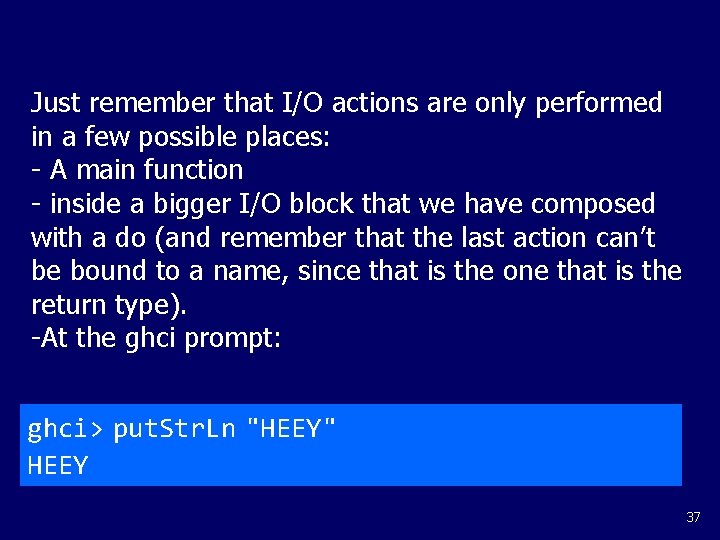
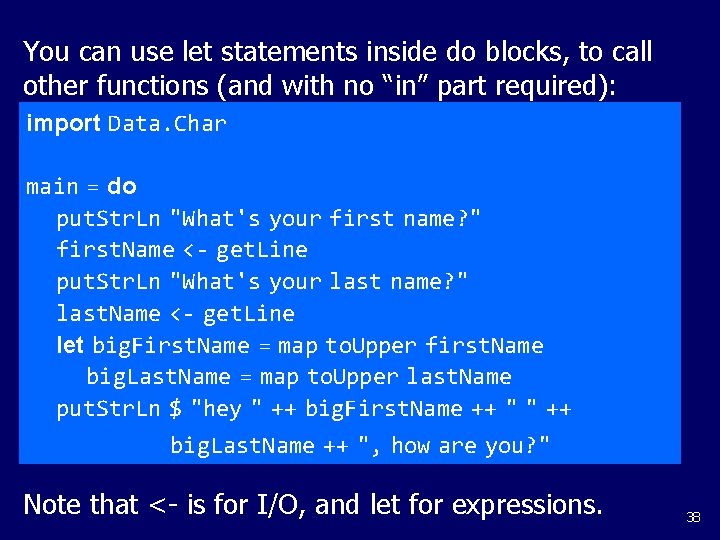
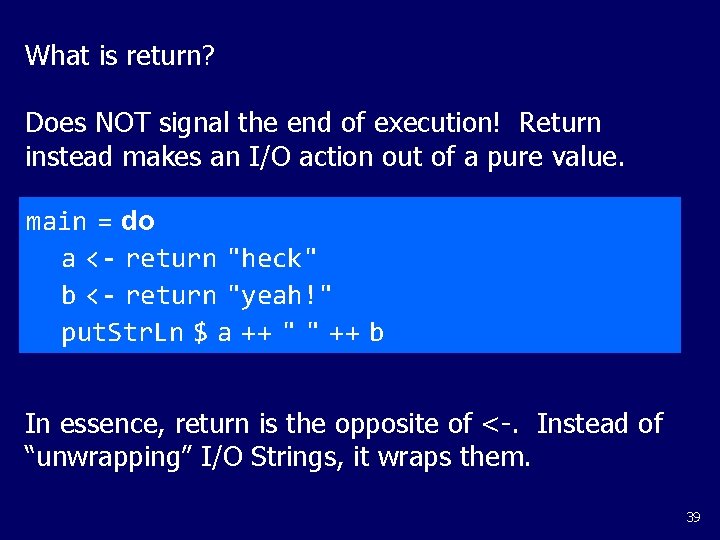
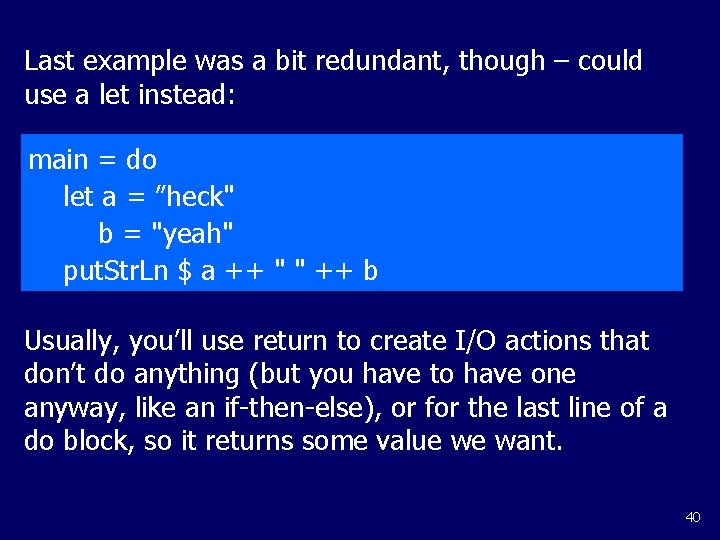
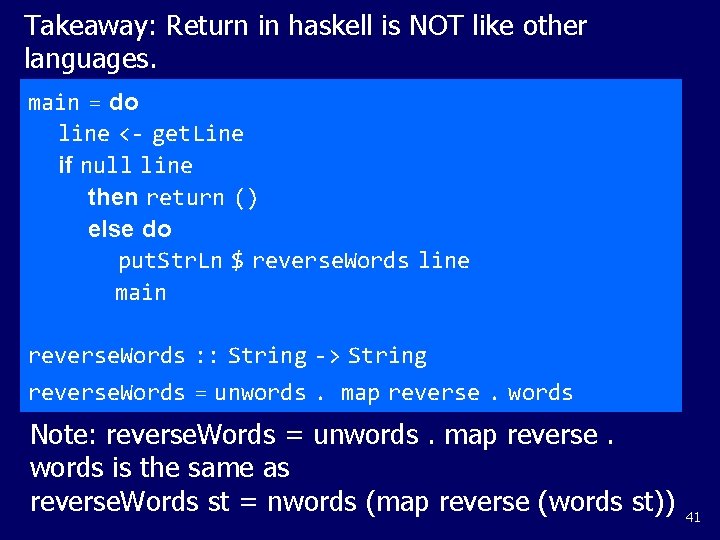
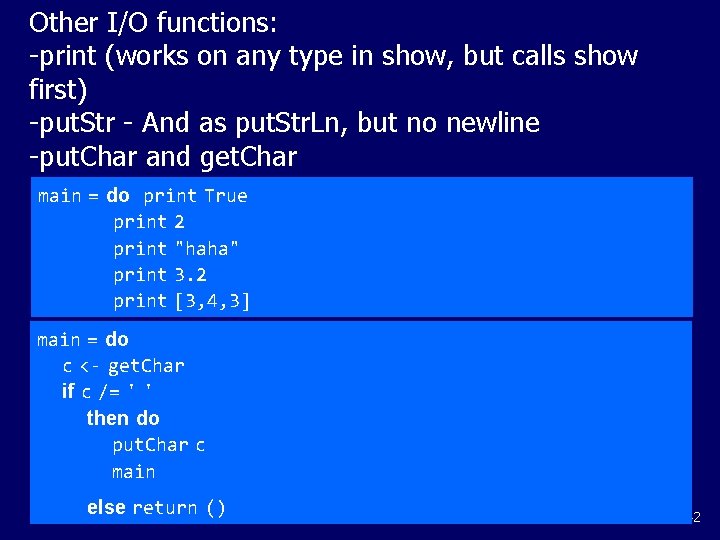
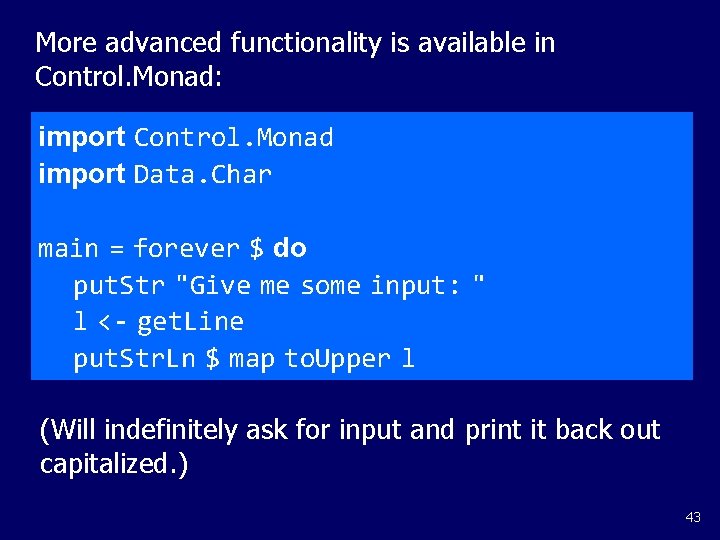
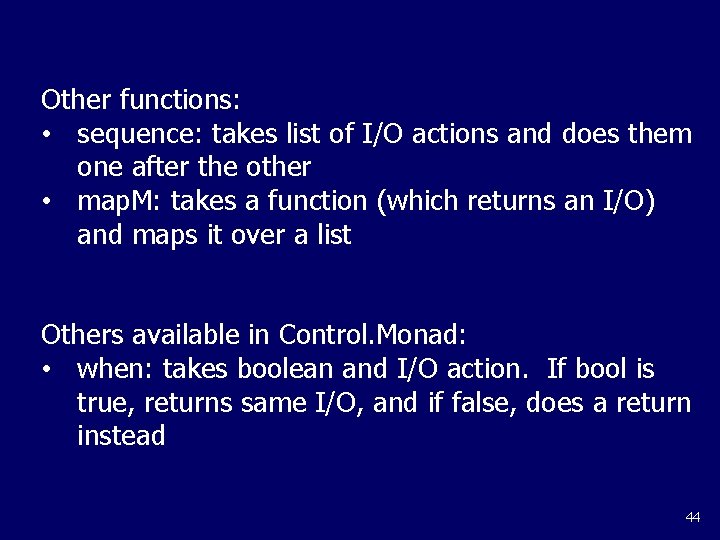
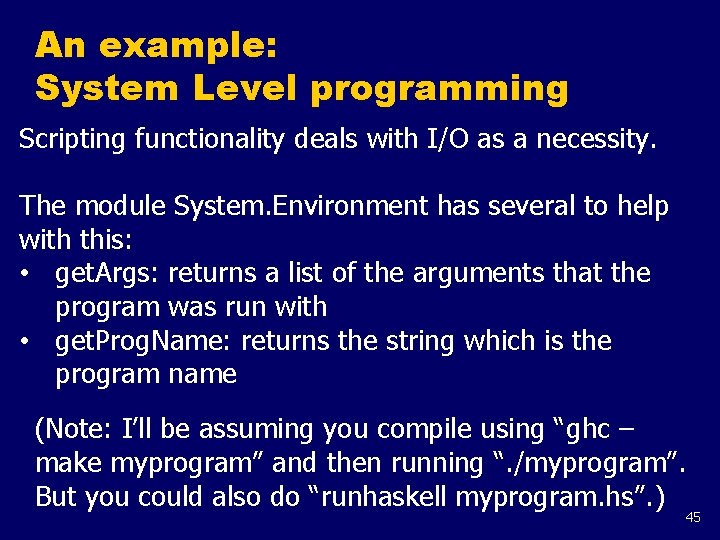
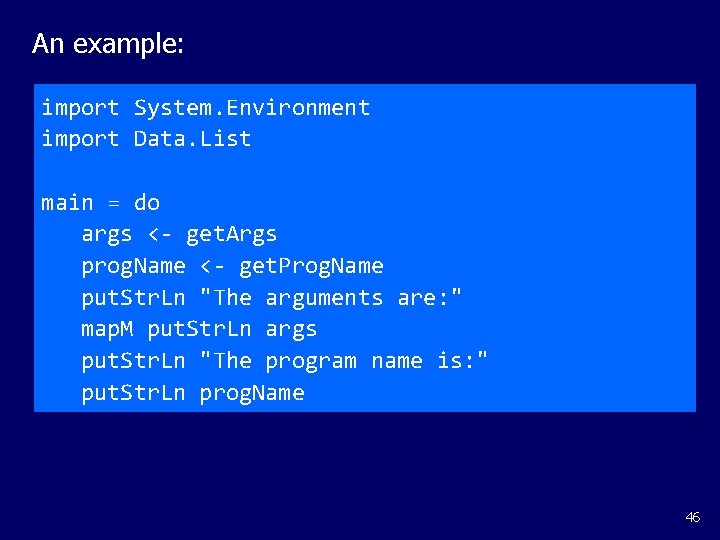
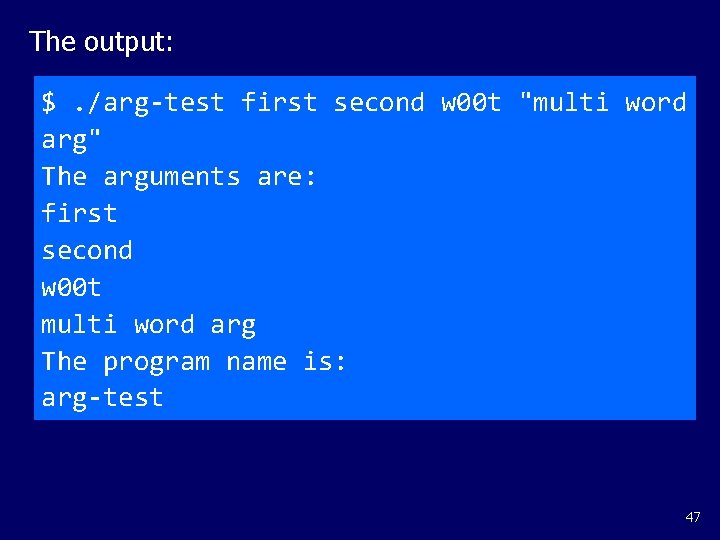
- Slides: 48
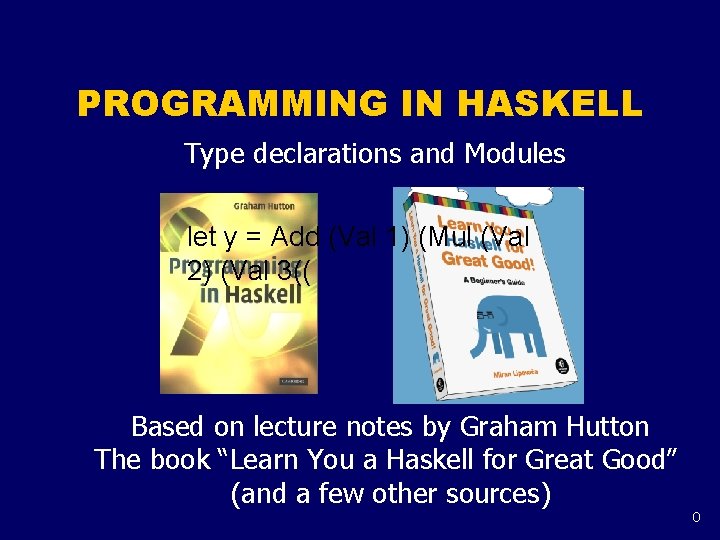
PROGRAMMING IN HASKELL Type declarations and Modules let y = Add (Val 1) (Mul (Val 2) (Val 3(( Based on lecture notes by Graham Hutton The book “Learn You a Haskell for Great Good” (and a few other sources) 0
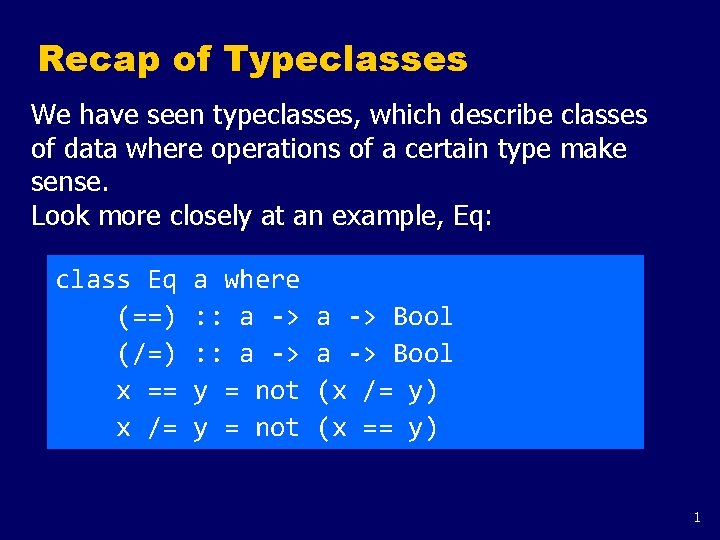
Recap of Typeclasses We have seen typeclasses, which describe classes of data where operations of a certain type make sense. Look more closely at an example, Eq: class Eq (==) (/=) x == x /= a where : : a -> y = not a -> Bool (x /= y) (x == y) 1
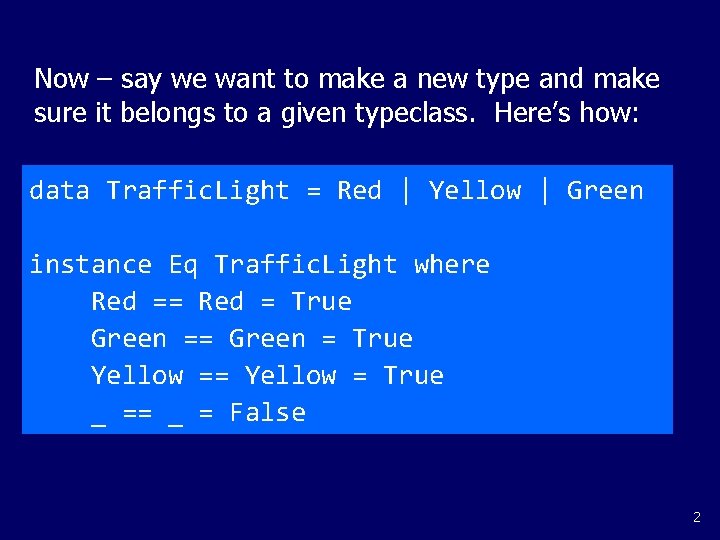
Now – say we want to make a new type and make sure it belongs to a given typeclass. Here’s how: data Traffic. Light = Red | Yellow | Green instance Eq Traffic. Light where Red == Red = True Green == Green = True Yellow == Yellow = True _ == _ = False 2
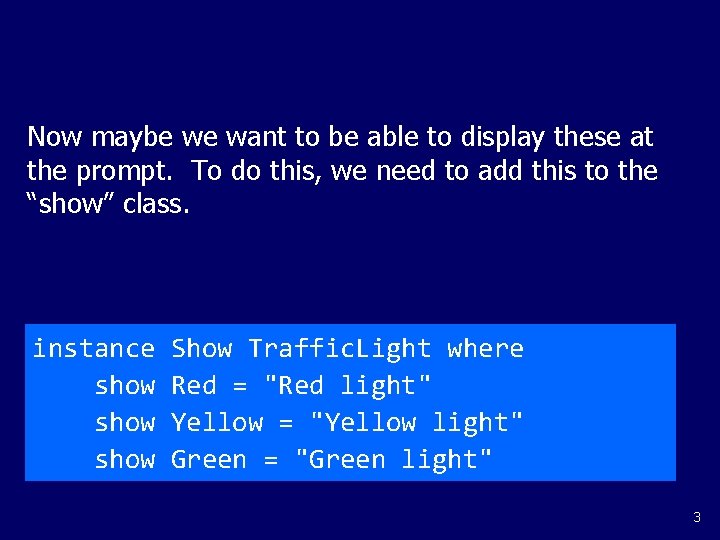
Now maybe we want to be able to display these at the prompt. To do this, we need to add this to the “show” class. instance show Show Traffic. Light where Red = "Red light" Yellow = "Yellow light" Green = "Green light" 3
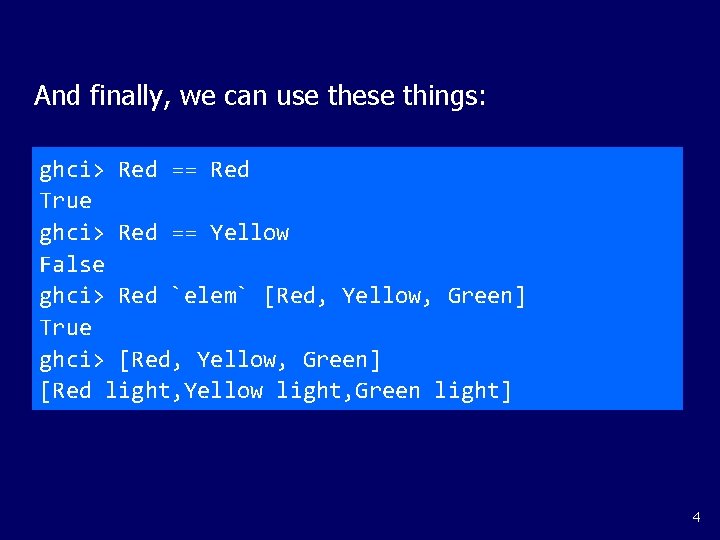
And finally, we can use these things: ghci> Red == Red True ghci> Red == Yellow False ghci> Red `elem` [Red, Yellow, Green] True ghci> [Red, Yellow, Green] [Red light, Yellow light, Green light] 4
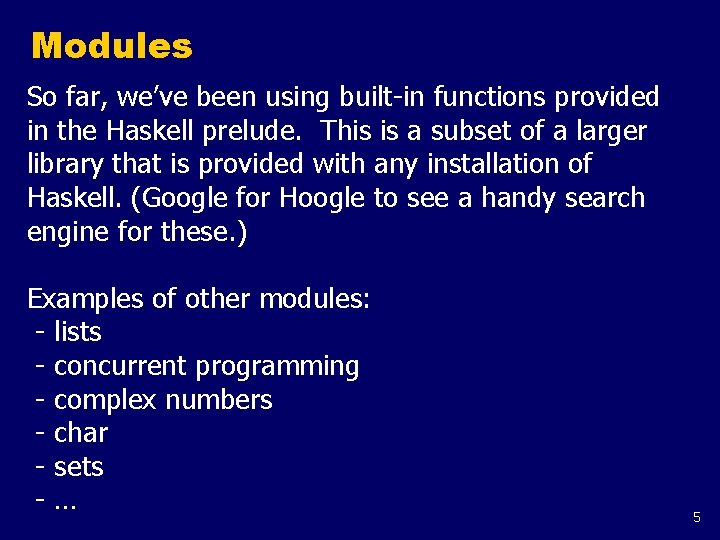
Modules So far, we’ve been using built-in functions provided in the Haskell prelude. This is a subset of a larger library that is provided with any installation of Haskell. (Google for Hoogle to see a handy search engine for these. ) Examples of other modules: - lists - concurrent programming - complex numbers - char - sets - … 5
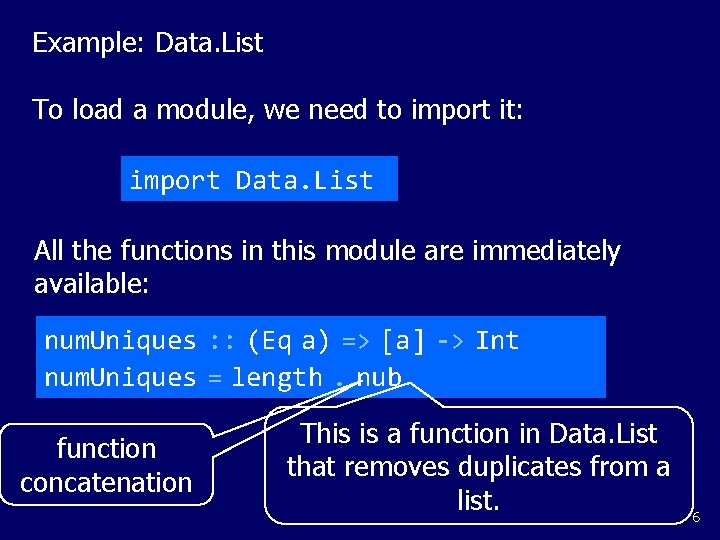
Example: Data. List To load a module, we need to import it: import Data. List All the functions in this module are immediately available: num. Uniques : : (Eq a) => [a] -> Int num. Uniques = length. nub function concatenation This is a function in Data. List that removes duplicates from a list. 6
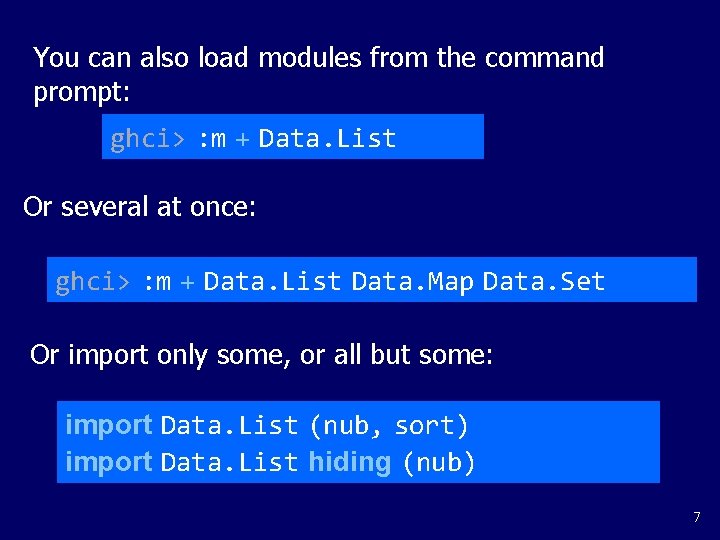
You can also load modules from the command prompt: ghci> : m + Data. List Or several at once: ghci> : m + Data. List Data. Map Data. Set Or import only some, or all but some: import Data. List (nub, sort) import Data. List hiding (nub) 7
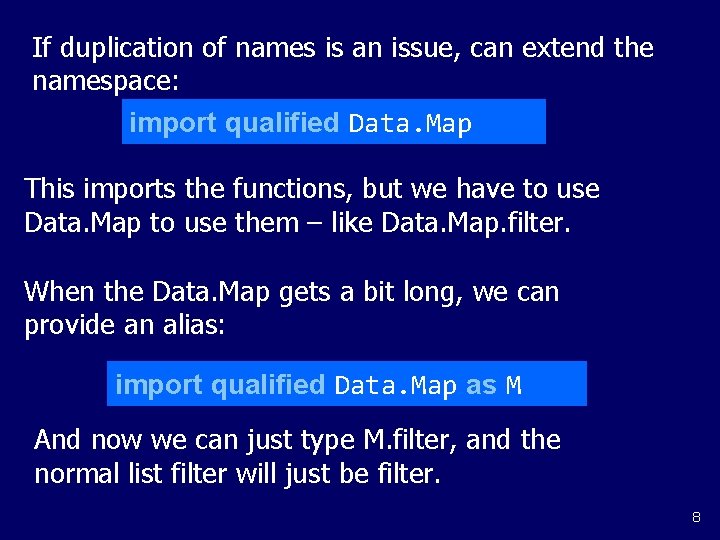
If duplication of names is an issue, can extend the namespace: import qualified Data. Map This imports the functions, but we have to use Data. Map to use them – like Data. Map. filter. When the Data. Map gets a bit long, we can provide an alias: import qualified Data. Map as M And now we can just type M. filter, and the normal list filter will just be filter. 8
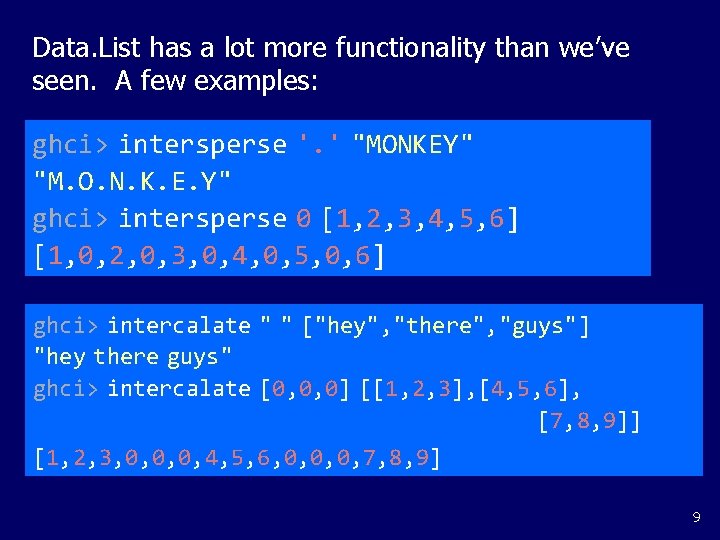
Data. List has a lot more functionality than we’ve seen. A few examples: ghci> intersperse '. ' "MONKEY" "M. O. N. K. E. Y" ghci> intersperse 0 [1, 2, 3, 4, 5, 6] [1, 0, 2, 0, 3, 0, 4, 0, 5, 0, 6] ghci> intercalate " " ["hey", "there", "guys"] "hey there guys" ghci> intercalate [0, 0, 0] [[1, 2, 3], [4, 5, 6], [7, 8, 9]] [1, 2, 3, 0, 0, 0, 4, 5, 6, 0, 0, 0, 7, 8, 9] 9
![And even more ghci transpose 1 2 3 4 5 6 7 8 9 And even more: ghci> transpose [[1, 2, 3], [4, 5, 6], [7, 8, 9]]](https://slidetodoc.com/presentation_image_h/d09f62bd6630f8887ce4bcf27a00ba05/image-11.jpg)
And even more: ghci> transpose [[1, 2, 3], [4, 5, 6], [7, 8, 9]] [[1, 4, 7], [2, 5, 8], [3, 6, 9]] ghci> transpose ["hey", "there", "guys"] [ "htg", "ehu", "yey", "rs", "e"] ghci> concat ["foo", "bar", "car"] "foobarcar" ghci> concat [[3, 4, 5], [2, 3, 4], [2, 1, 1]] [3, 4, 5, 2, 3, 4, 2, 1, 1] 10
![And even more ghci and map 4 5 6 7 8 True ghci And even more: ghci> and $ map (>4) [5, 6, 7, 8] True ghci>](https://slidetodoc.com/presentation_image_h/d09f62bd6630f8887ce4bcf27a00ba05/image-12.jpg)
And even more: ghci> and $ map (>4) [5, 6, 7, 8] True ghci> and $ map (==4) [4, 4, 4, 3, 4] False ghci> any (==4) [2, 3, 5, 6, 1, 4] True ghci> all (>4) [6, 9, 10] True 11
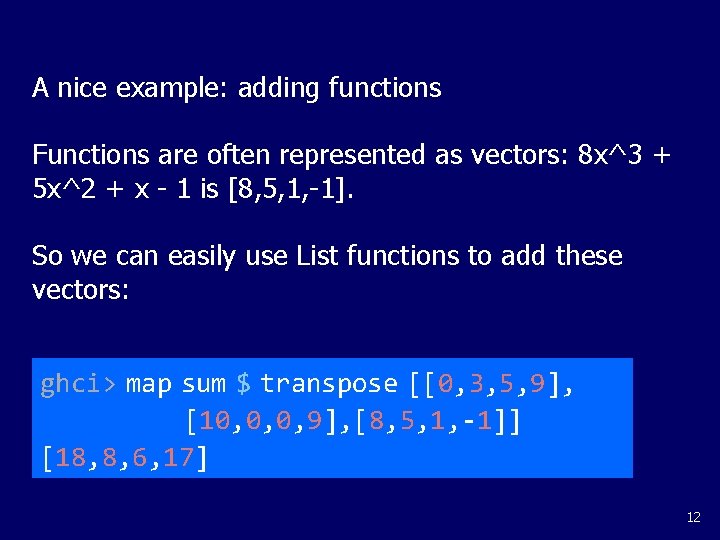
A nice example: adding functions Functions are often represented as vectors: 8 x^3 + 5 x^2 + x - 1 is [8, 5, 1, -1]. So we can easily use List functions to add these vectors: ghci> map sum $ transpose [[0, 3, 5, 9], [10, 0, 0, 9], [8, 5, 1, -1]] [18, 8, 6, 17] 12
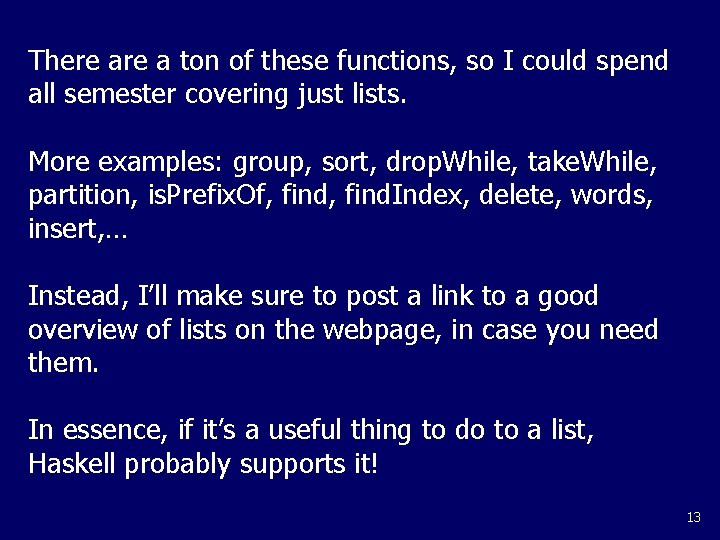
There a ton of these functions, so I could spend all semester covering just lists. More examples: group, sort, drop. While, take. While, partition, is. Prefix. Of, find. Index, delete, words, insert, … Instead, I’ll make sure to post a link to a good overview of lists on the webpage, in case you need them. In essence, if it’s a useful thing to do to a list, Haskell probably supports it! 13
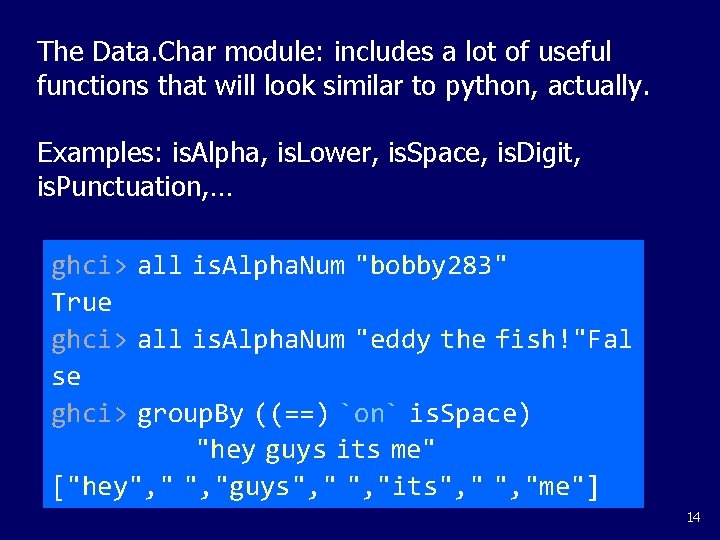
The Data. Char module: includes a lot of useful functions that will look similar to python, actually. Examples: is. Alpha, is. Lower, is. Space, is. Digit, is. Punctuation, … ghci> all is. Alpha. Num "bobby 283" True ghci> all is. Alpha. Num "eddy the fish!"Fal se ghci> group. By ((==) `on` is. Space) "hey guys its me" ["hey", "guys", "its", "me"] 14
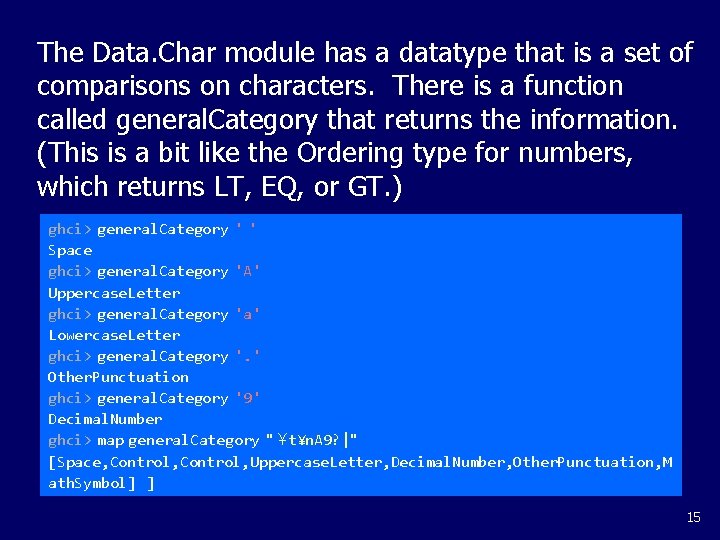
The Data. Char module has a datatype that is a set of comparisons on characters. There is a function called general. Category that returns the information. (This is a bit like the Ordering type for numbers, which returns LT, EQ, or GT. ) ghci> general. Category ' ' Space ghci> general. Category 'A' Uppercase. Letter ghci> general. Category 'a' Lowercase. Letter ghci> general. Category '. ' Other. Punctuation ghci> general. Category '9' Decimal. Number ghci> map general. Category " ¥t¥n. A 9? |" [Space, Control, Uppercase. Letter, Decimal. Number, Other. Punctuation, M ath. Symbol] ] 15
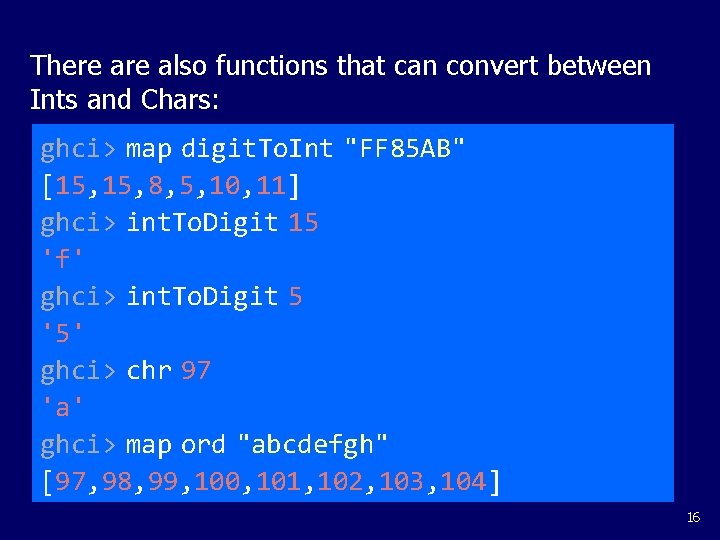
There also functions that can convert between Ints and Chars: ghci> map digit. To. Int "FF 85 AB" [15, 8, 5, 10, 11] ghci> int. To. Digit 15 'f' ghci> int. To. Digit 5 '5' ghci> chr 97 'a' ghci> map ord "abcdefgh" [97, 98, 99, 100, 101, 102, 103, 104] 16
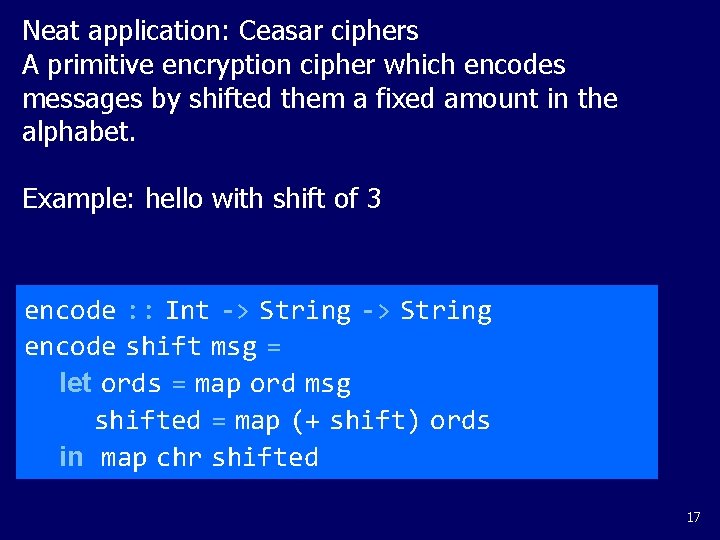
Neat application: Ceasar ciphers A primitive encryption cipher which encodes messages by shifted them a fixed amount in the alphabet. Example: hello with shift of 3 encode : : Int -> String encode shift msg = let ords = map ord msg shifted = map (+ shift) ords in map chr shifted 17
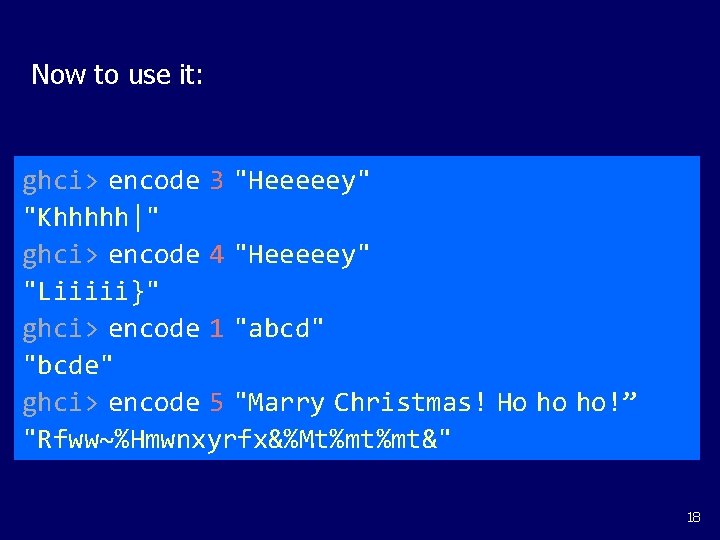
Now to use it: ghci> encode 3 "Heeeeey" "Khhhhh|" ghci> encode 4 "Heeeeey" "Liiiii}" ghci> encode 1 "abcd" "bcde" ghci> encode 5 "Marry Christmas! Ho ho ho!” "Rfww~%Hmwnxyrfx&%Mt%mt%mt&" 18
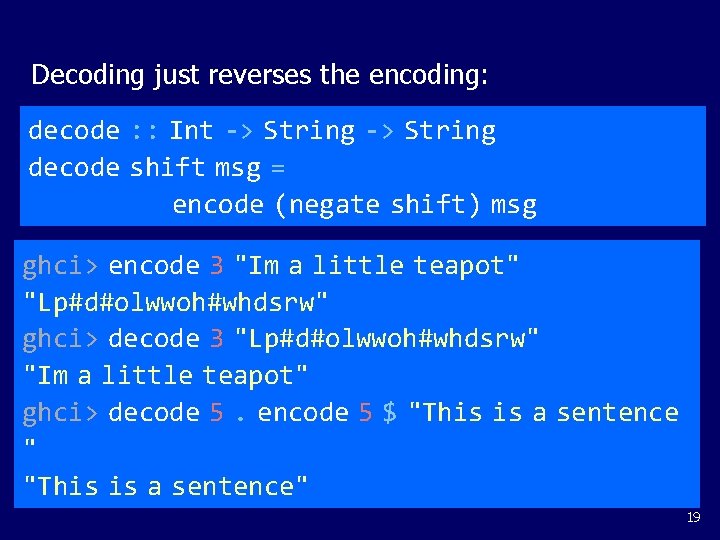
Decoding just reverses the encoding: decode : : Int -> String decode shift msg = encode (negate shift) msg ghci> encode 3 "Im a little teapot" "Lp#d#olwwoh#whdsrw" ghci> decode 3 "Lp#d#olwwoh#whdsrw" "Im a little teapot" ghci> decode 5. encode 5 $ "This is a sentence " "This is a sentence" 19
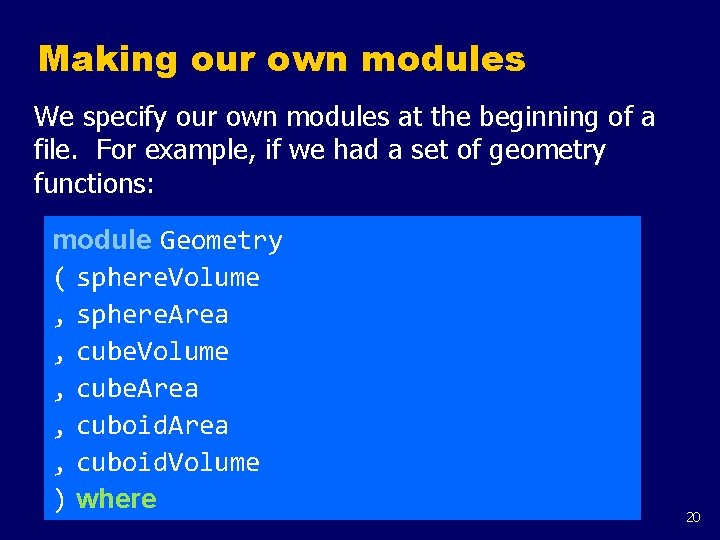
Making our own modules We specify our own modules at the beginning of a file. For example, if we had a set of geometry functions: module Geometry ( sphere. Volume , sphere. Area , cube. Volume , cube. Area , cuboid. Volume ) where 20
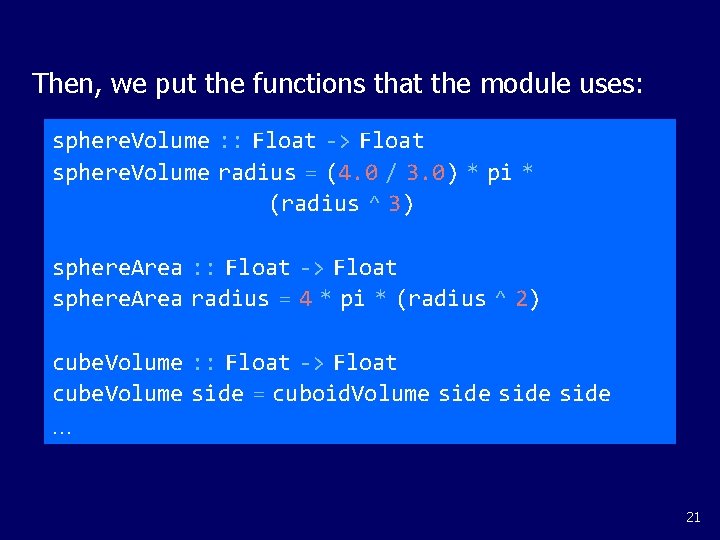
Then, we put the functions that the module uses: sphere. Volume : : Float -> Float sphere. Volume radius = (4. 0 / 3. 0) * pi * (radius ^ 3) sphere. Area : : Float -> Float sphere. Area radius = 4 * pi * (radius ^ 2) cube. Volume : : Float -> Float cube. Volume side = cuboid. Volume side … 21
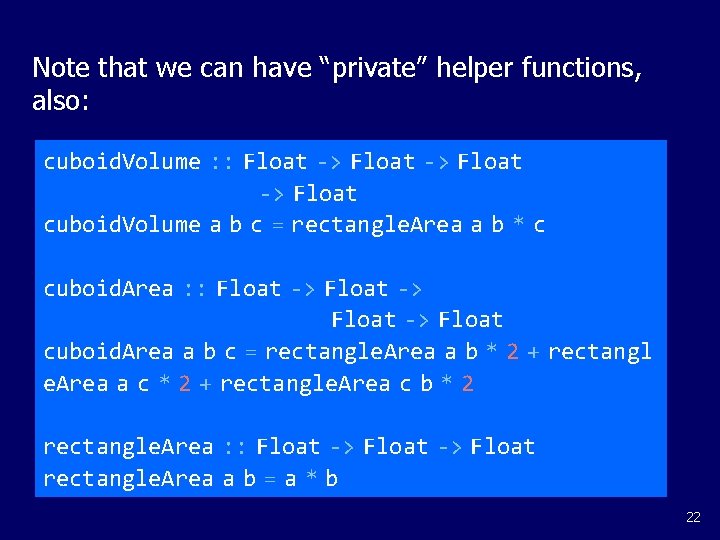
Note that we can have “private” helper functions, also: cuboid. Volume : : Float -> Float cuboid. Volume a b c = rectangle. Area a b * c cuboid. Area : : Float -> Float cuboid. Area a b c = rectangle. Area a b * 2 + rectangl e. Area a c * 2 + rectangle. Area c b * 2 rectangle. Area : : Float -> Float rectangle. Area a b = a * b 22
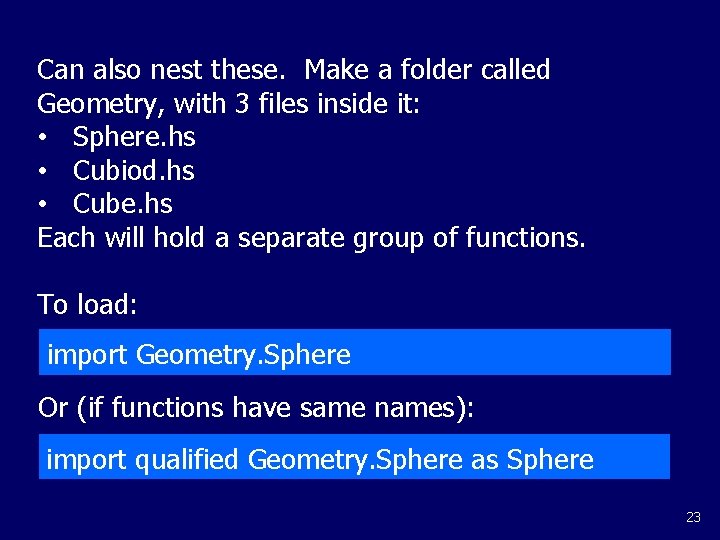
Can also nest these. Make a folder called Geometry, with 3 files inside it: • Sphere. hs • Cubiod. hs • Cube. hs Each will hold a separate group of functions. To load: import Geometry. Sphere Or (if functions have same names): import qualified Geometry. Sphere as Sphere 23
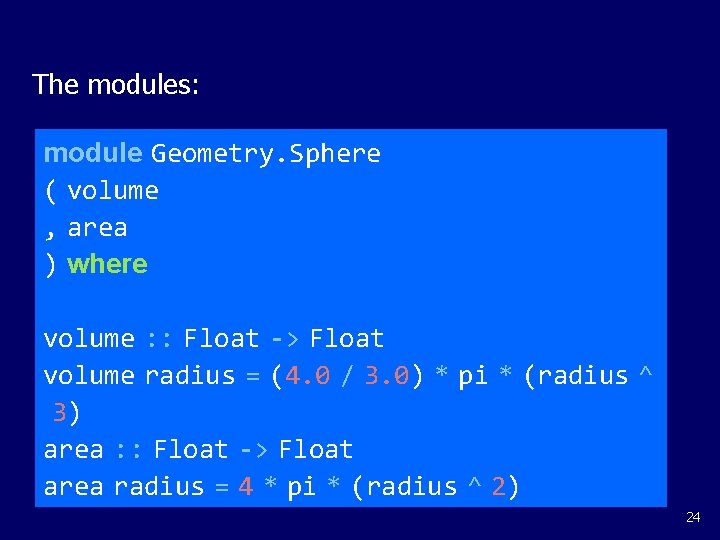
The modules: module Geometry. Sphere ( volume , area ) where volume : : Float -> Float volume radius = (4. 0 / 3. 0) * pi * (radius ^ 3) area : : Float -> Float area radius = 4 * pi * (radius ^ 2) 24
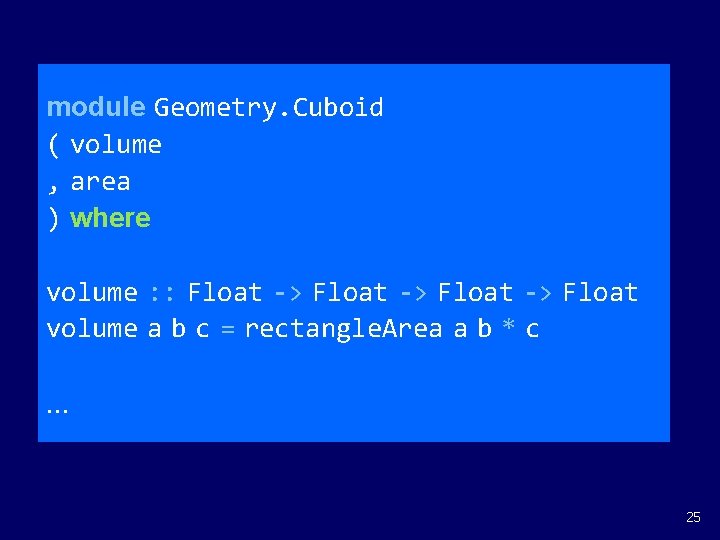
module Geometry. Cuboid ( volume , area ) where volume : : Float -> Float volume a b c = rectangle. Area a b * c … 25
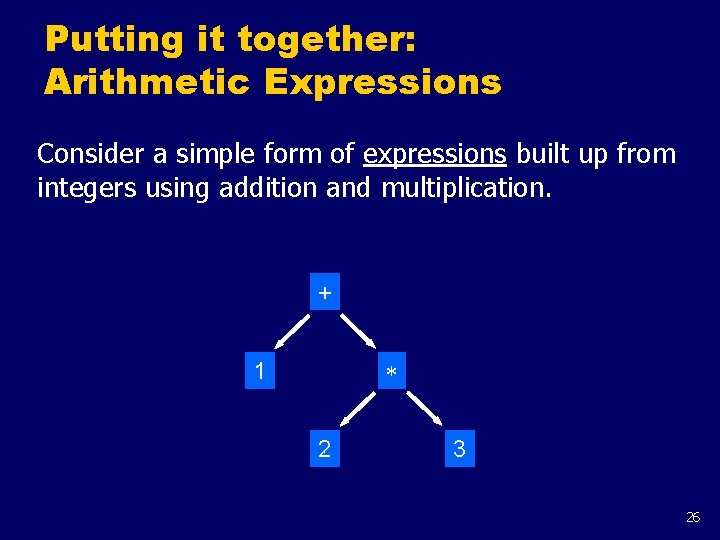
Putting it together: Arithmetic Expressions Consider a simple form of expressions built up from integers using addition and multiplication. + 1 2 3 26
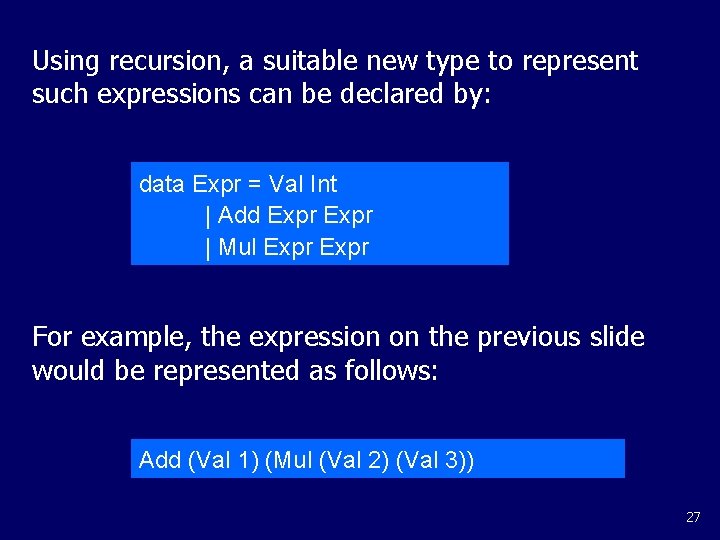
Using recursion, a suitable new type to represent such expressions can be declared by: data Expr = Val Int | Add Expr | Mul Expr For example, the expression on the previous slide would be represented as follows: Add (Val 1) (Mul (Val 2) (Val 3)) 27
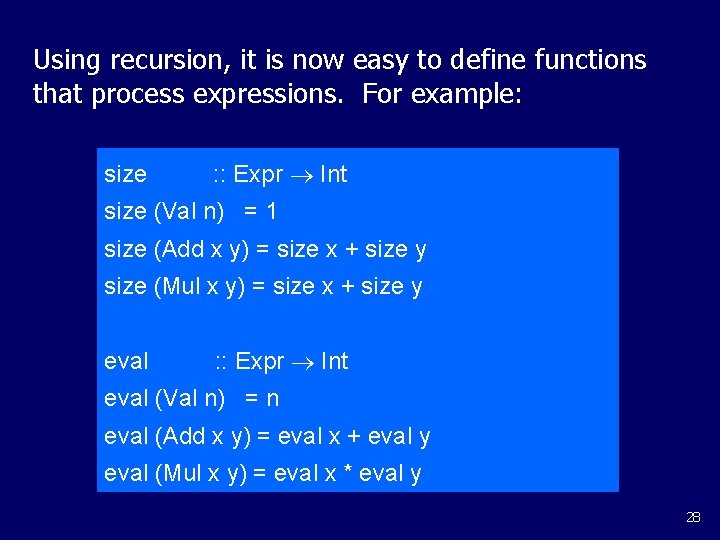
Using recursion, it is now easy to define functions that process expressions. For example: size : : Expr Int size (Val n) = 1 size (Add x y) = size x + size y size (Mul x y) = size x + size y eval : : Expr Int eval (Val n) = n eval (Add x y) = eval x + eval y eval (Mul x y) = eval x * eval y 28
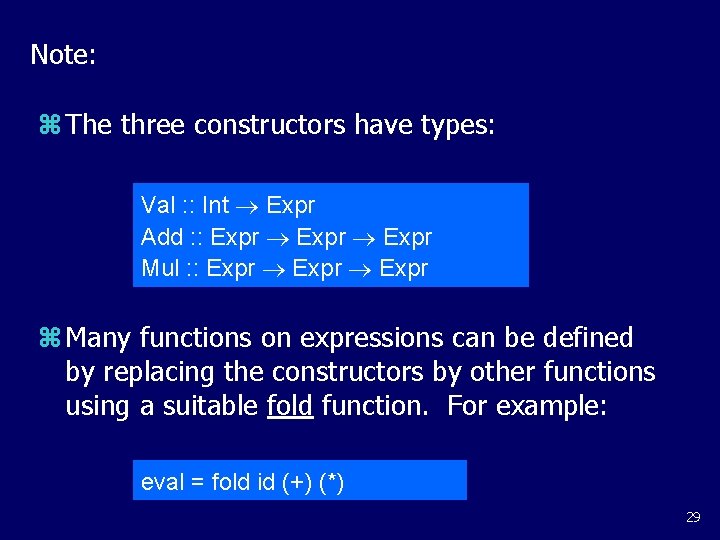
Note: z The three constructors have types: Val : : Int Expr Add : : Expr Mul : : Expr z Many functions on expressions can be defined by replacing the constructors by other functions using a suitable fold function. For example: eval = fold id (+) (*) 29
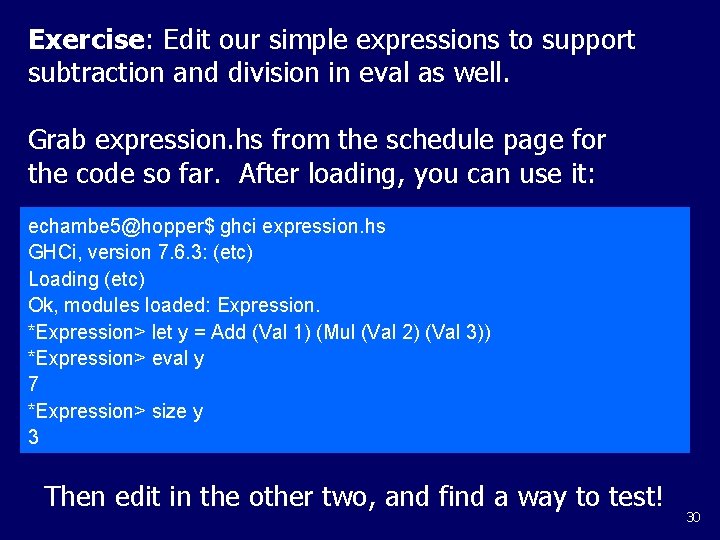
Exercise: Edit our simple expressions to support subtraction and division in eval as well. Grab expression. hs from the schedule page for the code so far. After loading, you can use it: echambe 5@hopper$ ghci expression. hs GHCi, version 7. 6. 3: (etc) Loading (etc) Ok, modules loaded: Expression. *Expression> let y = Add (Val 1) (Mul (Val 2) (Val 3)) *Expression> eval y 7 *Expression> size y 3 Then edit in the other two, and find a way to test! 30
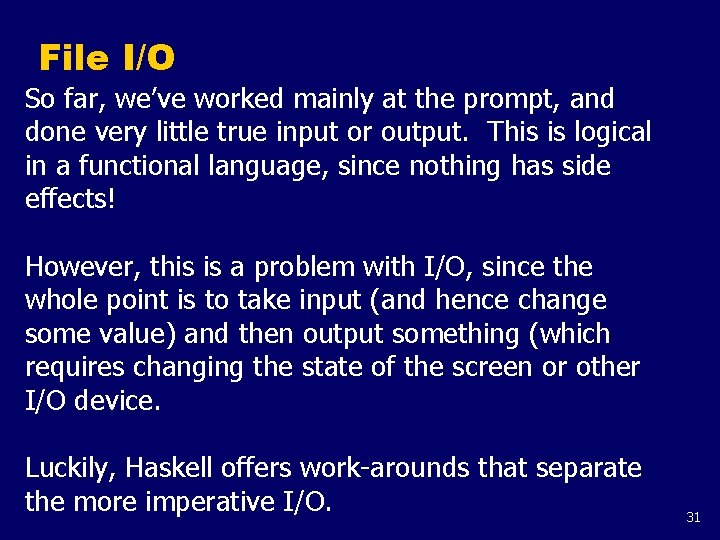
File I/O So far, we’ve worked mainly at the prompt, and done very little true input or output. This is logical in a functional language, since nothing has side effects! However, this is a problem with I/O, since the whole point is to take input (and hence change some value) and then output something (which requires changing the state of the screen or other I/O device. Luckily, Haskell offers work-arounds that separate the more imperative I/O. 31
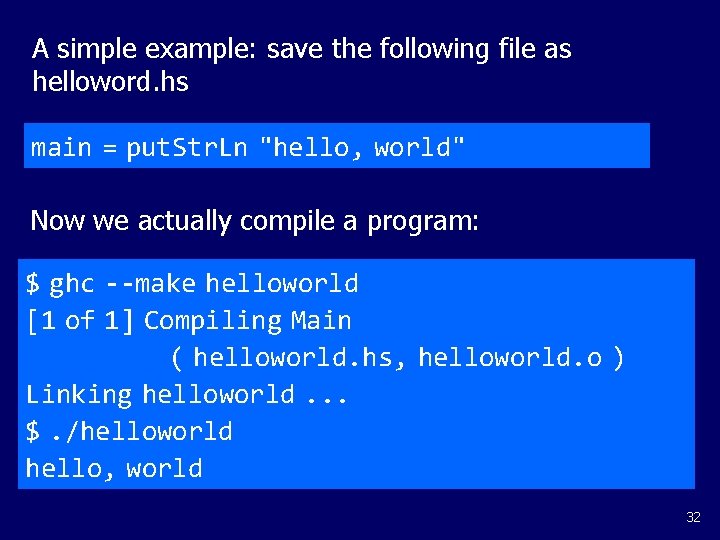
A simple example: save the following file as helloword. hs main = put. Str. Ln "hello, world" Now we actually compile a program: $ ghc --make helloworld [1 of 1] Compiling Main ( helloworld. hs, helloworld. o ) Linking helloworld. . . $. /helloworld hello, world 32
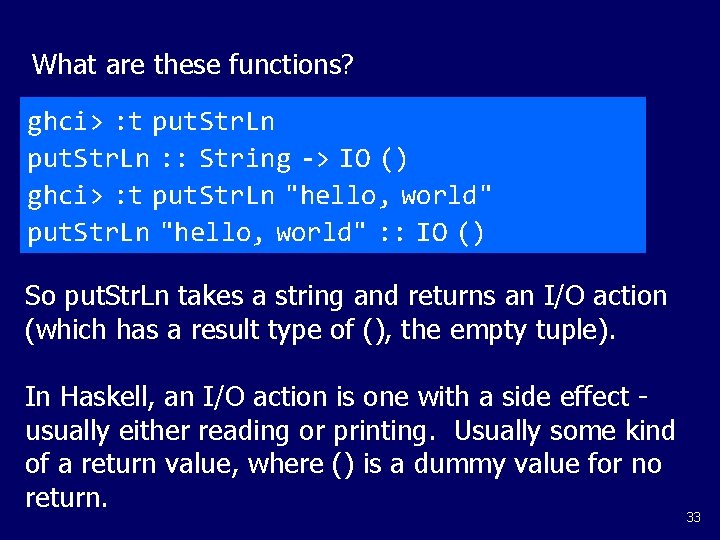
What are these functions? ghci> : t put. Str. Ln : : String -> IO () ghci> : t put. Str. Ln "hello, world" : : IO () So put. Str. Ln takes a string and returns an I/O action (which has a result type of (), the empty tuple). In Haskell, an I/O action is one with a side effect - usually either reading or printing. Usually some kind of a return value, where () is a dummy value for no return. 33
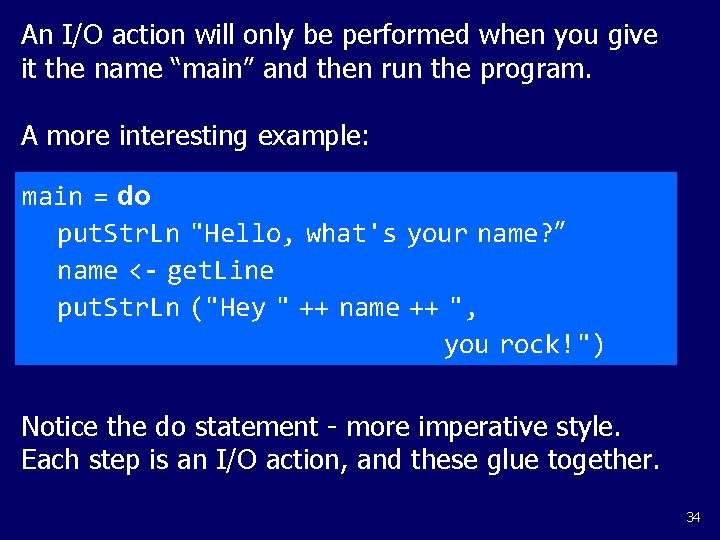
An I/O action will only be performed when you give it the name “main” and then run the program. A more interesting example: main = do put. Str. Ln "Hello, what's your name? ” name <- get. Line put. Str. Ln ("Hey " ++ name ++ ", you rock!") Notice the do statement - more imperative style. Each step is an I/O action, and these glue together. 34
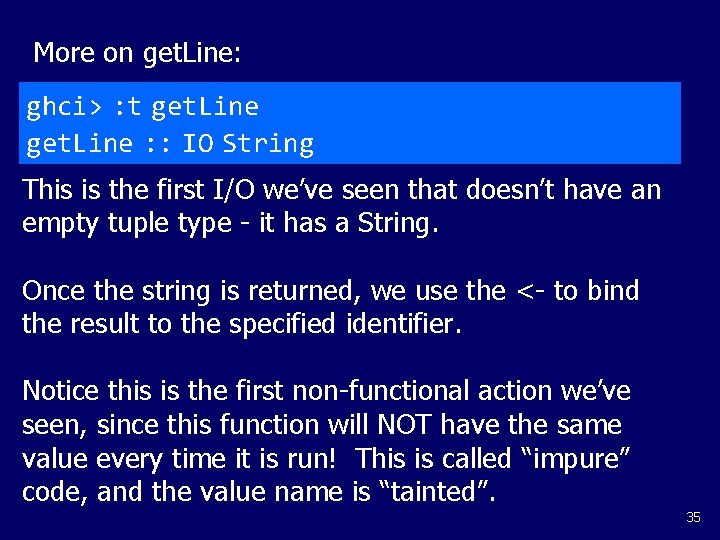
More on get. Line: ghci> : t get. Line : : IO String This is the first I/O we’ve seen that doesn’t have an empty tuple type - it has a String. Once the string is returned, we use the <- to bind the result to the specified identifier. Notice this is the first non-functional action we’ve seen, since this function will NOT have the same value every time it is run! This is called “impure” code, and the value name is “tainted”. 35
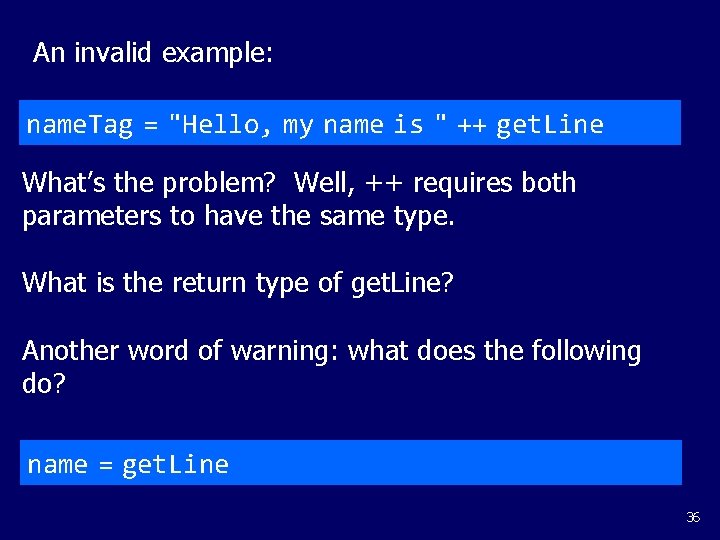
An invalid example: name. Tag = "Hello, my name is " ++ get. Line What’s the problem? Well, ++ requires both parameters to have the same type. What is the return type of get. Line? Another word of warning: what does the following do? name = get. Line 36
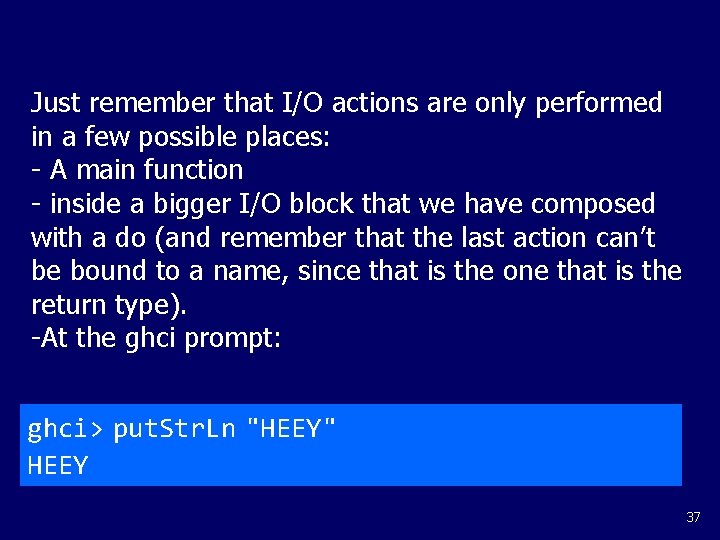
Just remember that I/O actions are only performed in a few possible places: - A main function - inside a bigger I/O block that we have composed with a do (and remember that the last action can’t be bound to a name, since that is the one that is the return type). -At the ghci prompt: ghci> put. Str. Ln "HEEY" HEEY 37
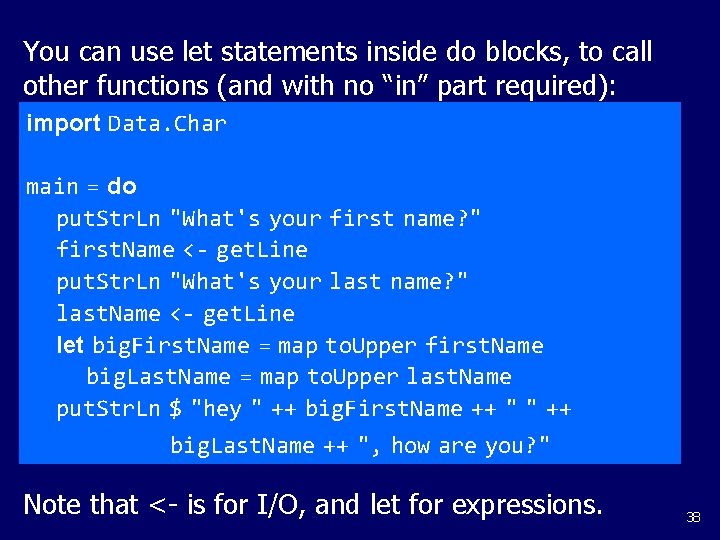
You can use let statements inside do blocks, to call other functions (and with no “in” part required): import Data. Char main = do put. Str. Ln "What's your first name? " first. Name <- get. Line put. Str. Ln "What's your last name? " last. Name <- get. Line let big. First. Name = map to. Upper first. Name big. Last. Name = map to. Upper last. Name put. Str. Ln $ "hey " ++ big. First. Name ++ " " ++ big. Last. Name ++ ", how are you? " Note that <- is for I/O, and let for expressions. 38
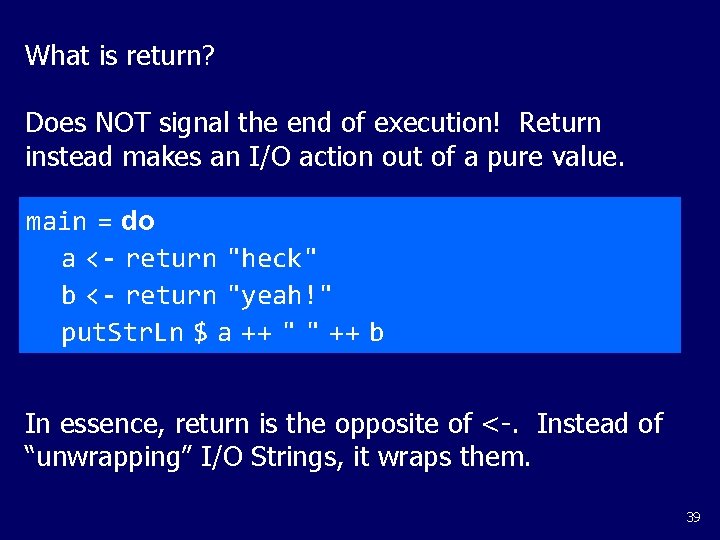
What is return? Does NOT signal the end of execution! Return instead makes an I/O action out of a pure value. main = do a <- return "heck" b <- return "yeah!" put. Str. Ln $ a ++ " " ++ b In essence, return is the opposite of <-. Instead of “unwrapping” I/O Strings, it wraps them. 39
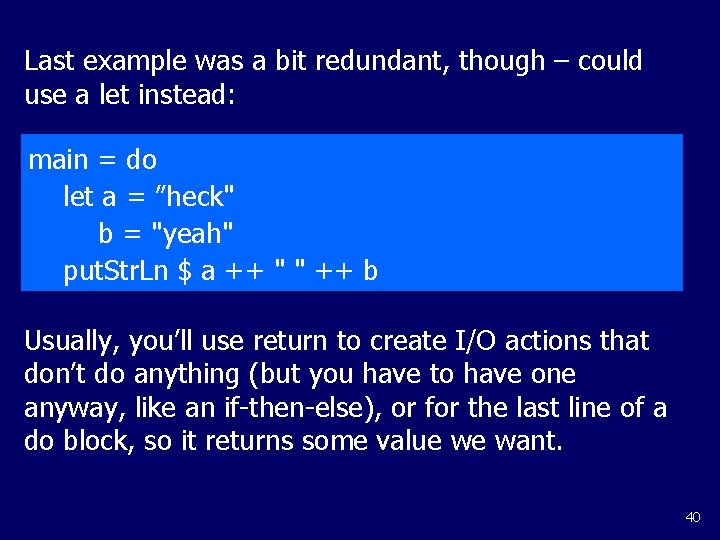
Last example was a bit redundant, though – could use a let instead: main = do let a = ”heck" b = "yeah" put. Str. Ln $ a ++ " " ++ b Usually, you’ll use return to create I/O actions that don’t do anything (but you have to have one anyway, like an if-then-else), or for the last line of a do block, so it returns some value we want. 40
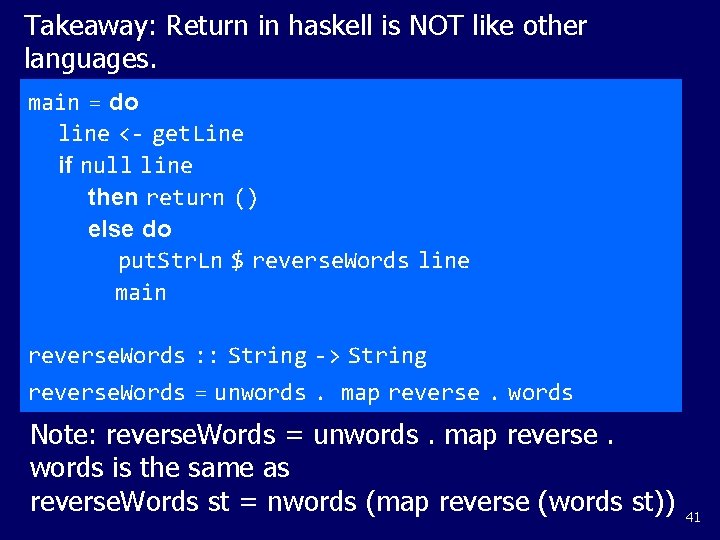
Takeaway: Return in haskell is NOT like other languages. main = do line <- get. Line if null line then return () else do put. Str. Ln $ reverse. Words line main reverse. Words : : String -> String reverse. Words = unwords. map reverse. words Note: reverse. Words = unwords. map reverse. words is the same as reverse. Words st = nwords (map reverse (words st)) 41
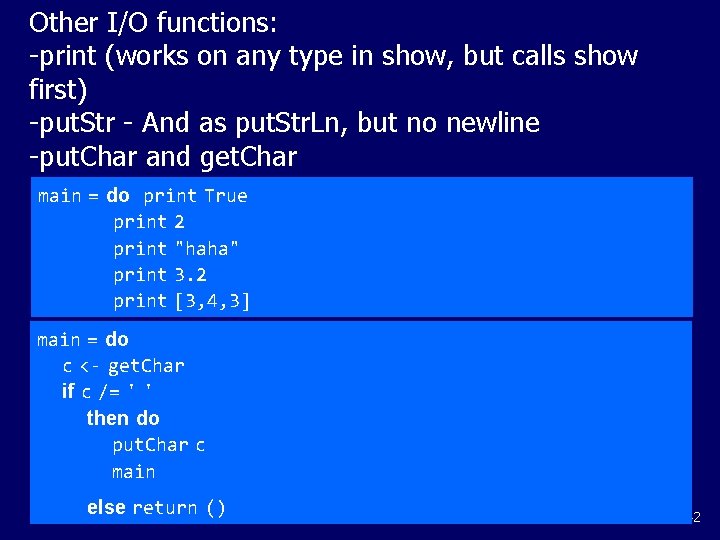
Other I/O functions: -print (works on any type in show, but calls show first) -put. Str - And as put. Str. Ln, but no newline -put. Char and get. Char main = do print True print 2 print "haha" print 3. 2 print [3, 4, 3] main = do c <- get. Char if c /= ' ' then do put. Char c main else return () 42
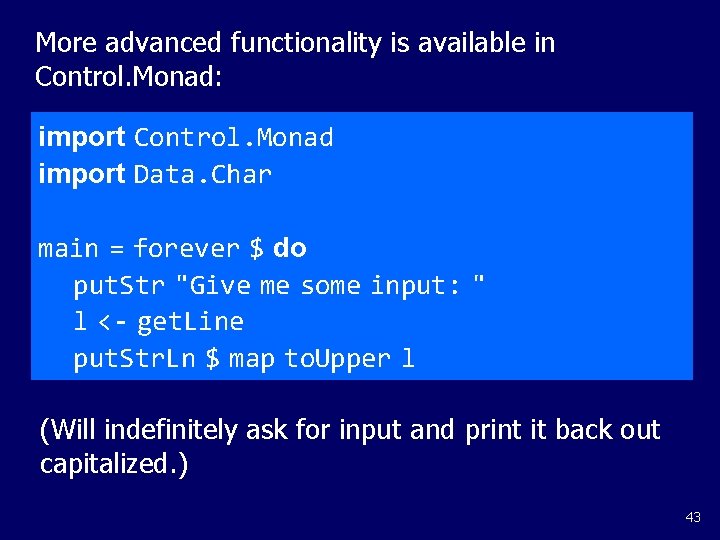
More advanced functionality is available in Control. Monad: import Control. Monad import Data. Char main = forever $ do put. Str "Give me some input: " l <- get. Line put. Str. Ln $ map to. Upper l (Will indefinitely ask for input and print it back out capitalized. ) 43
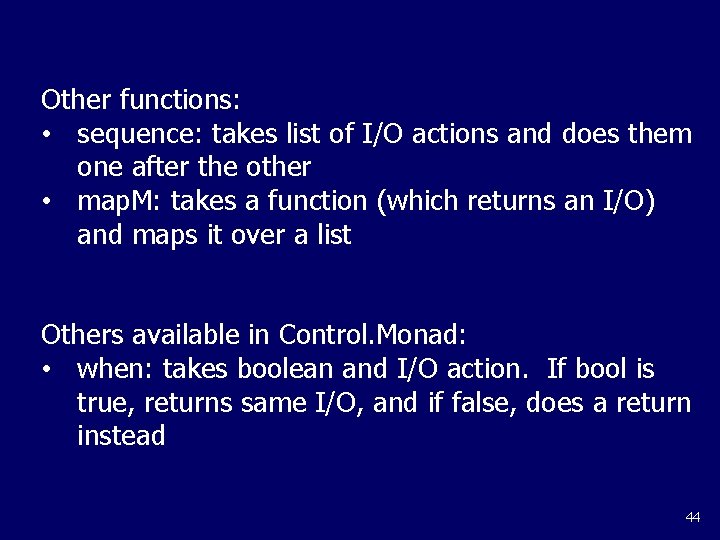
Other functions: • sequence: takes list of I/O actions and does them one after the other • map. M: takes a function (which returns an I/O) and maps it over a list Others available in Control. Monad: • when: takes boolean and I/O action. If bool is true, returns same I/O, and if false, does a return instead 44
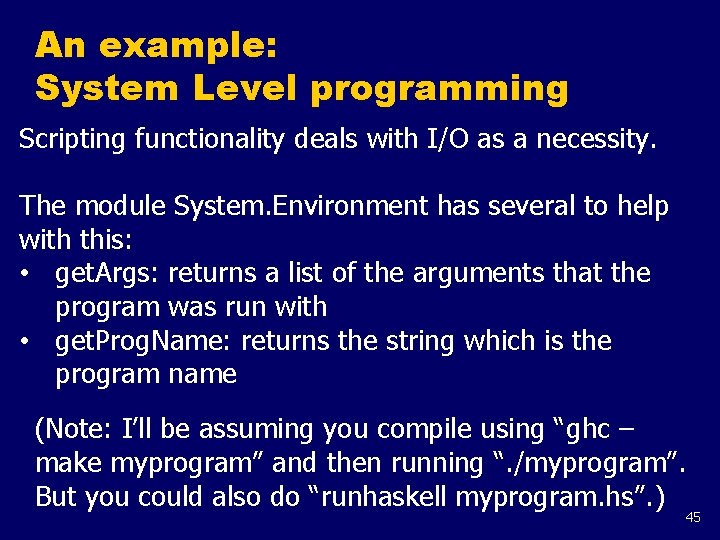
An example: System Level programming Scripting functionality deals with I/O as a necessity. The module System. Environment has several to help with this: • get. Args: returns a list of the arguments that the program was run with • get. Prog. Name: returns the string which is the program name (Note: I’ll be assuming you compile using “ghc – make myprogram” and then running “. /myprogram”. But you could also do “runhaskell myprogram. hs”. ) 45
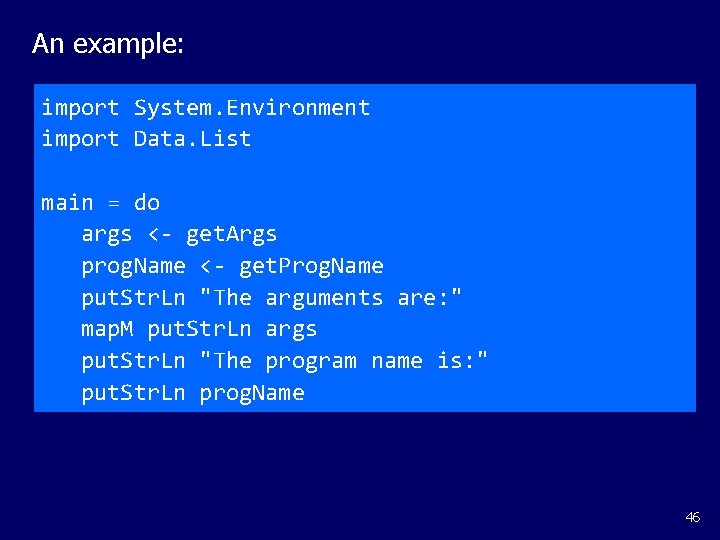
An example: import System. Environment import Data. List main = do args <- get. Args prog. Name <- get. Prog. Name put. Str. Ln "The arguments are: " map. M put. Str. Ln args put. Str. Ln "The program name is: " put. Str. Ln prog. Name 46
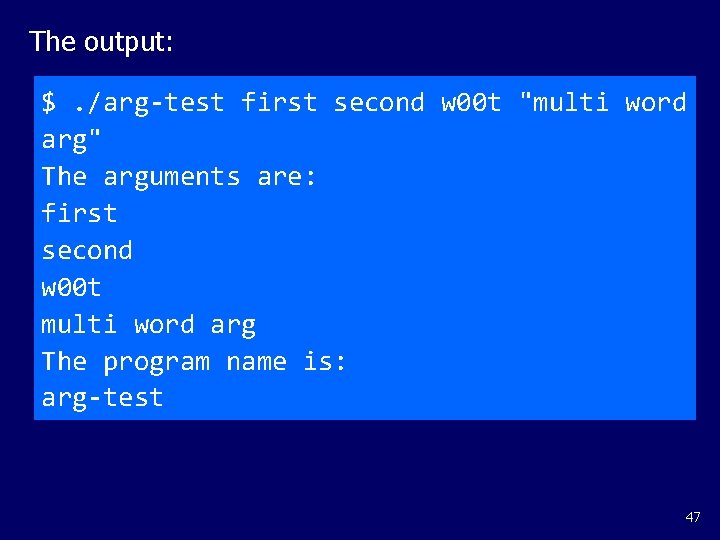
The output: $. /arg-test first second w 00 t "multi word arg" The arguments are: first second w 00 t multi word arg The program name is: arg-test 47
Types and declarations in intermediate code generation
Environmental labels and declarations
Comparing declarations venn diagram
Comparing declarations venn diagram
John 10:22-28
Haskell class hierarchy
Haskell type declaration
Indirect object examples
Let's go to my house
Mark 4:23-24
Perbedaan linear programming dan integer programming
Greedy vs dynamic
What is in system programming
Linear vs integer programming
Definisi linear
Generic subroutines and modules
Erp sales and marketing
Generic subroutines and modules
What is control abstraction
Generic subroutines and modules
All crm packages contain modules for prm and erm.
Pair
Haskell higher order functions
Haskell functor
Haskell reverse list recursively
Haskell list comprehension
Haskell map list
Haskell linguagem
Operadores logicos haskell
Formula do delta
Haskell adt
Haskell guards
Currying function technique
Visual studio haskell
Typed template haskell
Haskell odds
What is a monad haskell
Tips for haskell
Haskell cons
Fonksiyonel programlama
Haskell two dimensional array
Haskell microcontroller
Functor laws haskell
Haskell higher order functions
Haskell exercises solutions
Either haskell
Clojure haskell
Haskell bool
Haskell curry