Programming for Engineers in Python Recitation 1 Plan
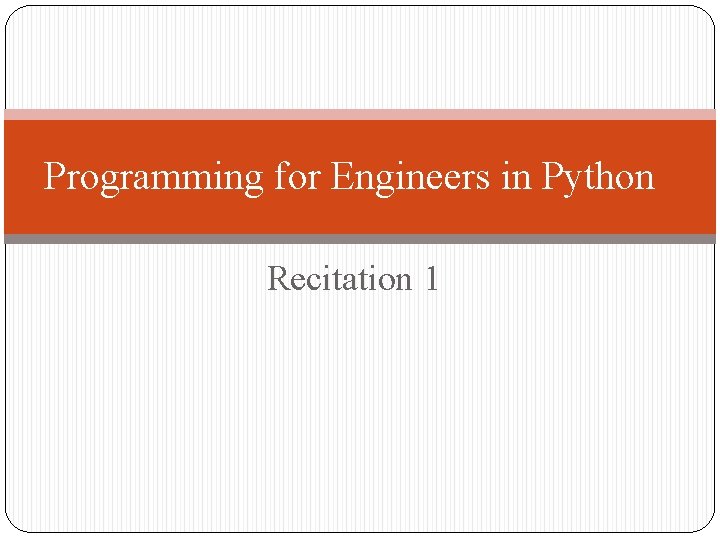
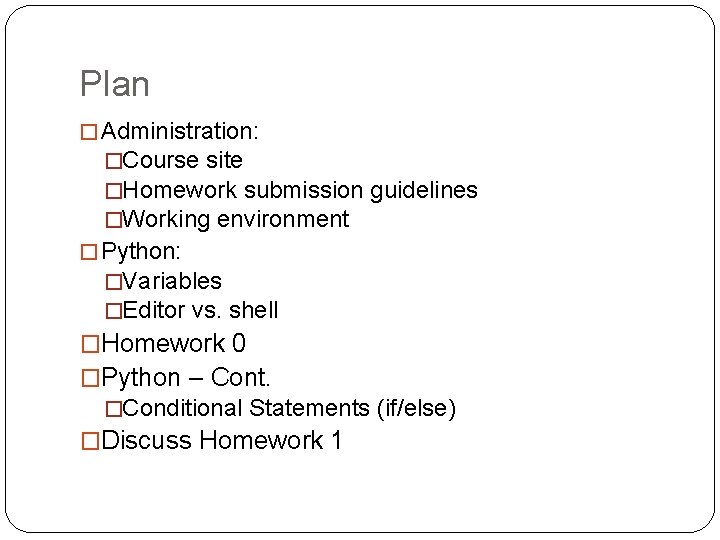
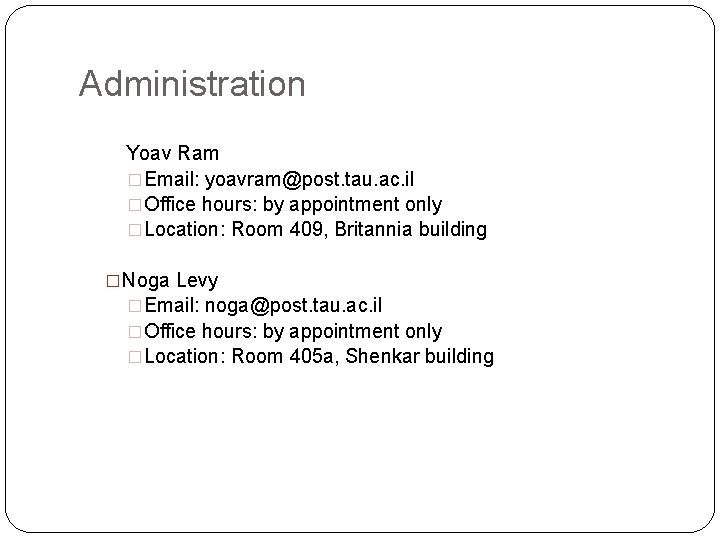
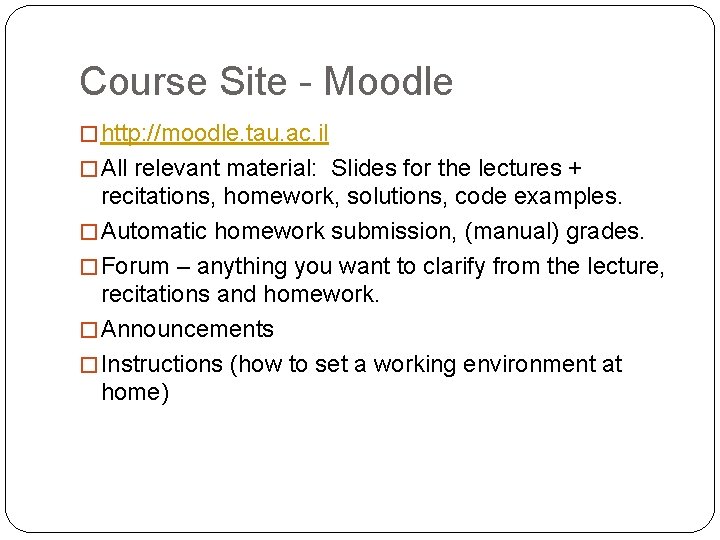
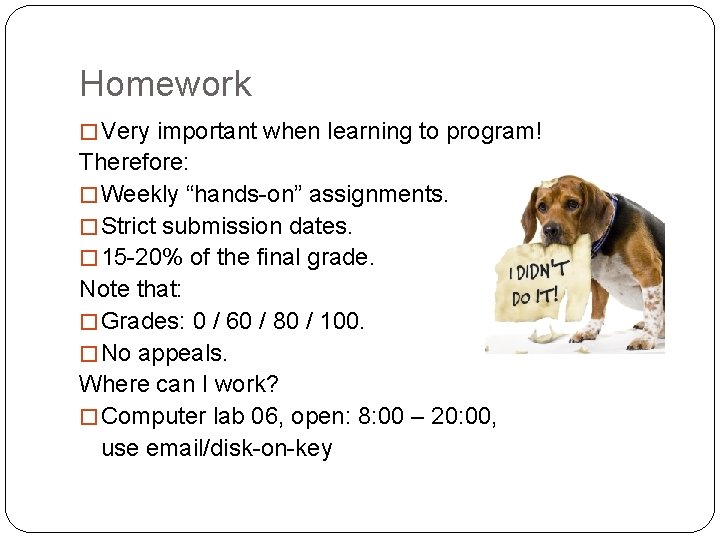
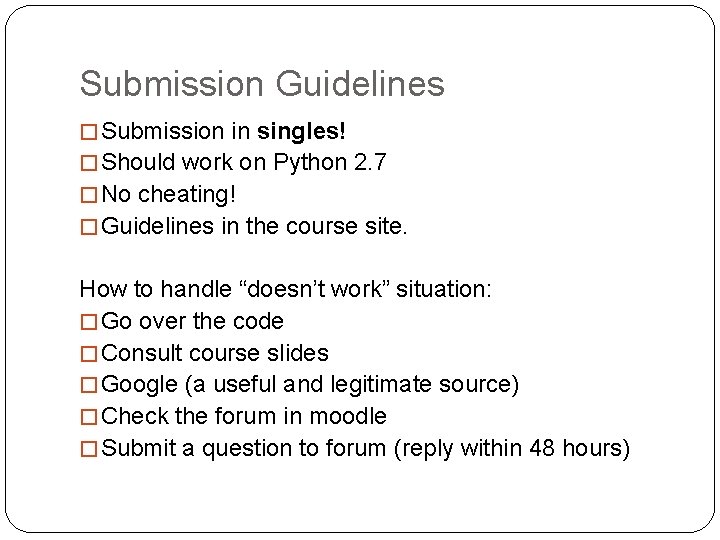
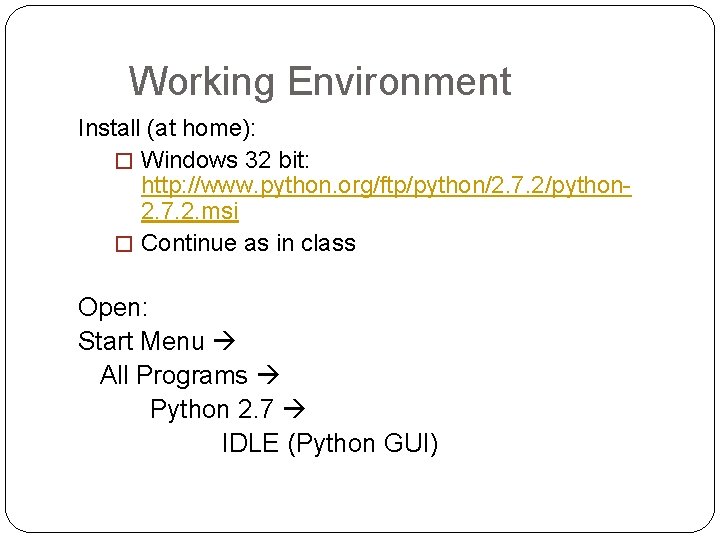
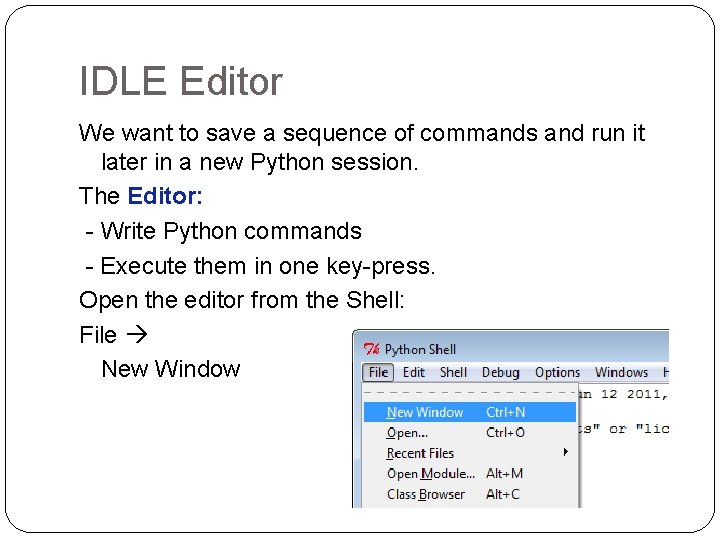
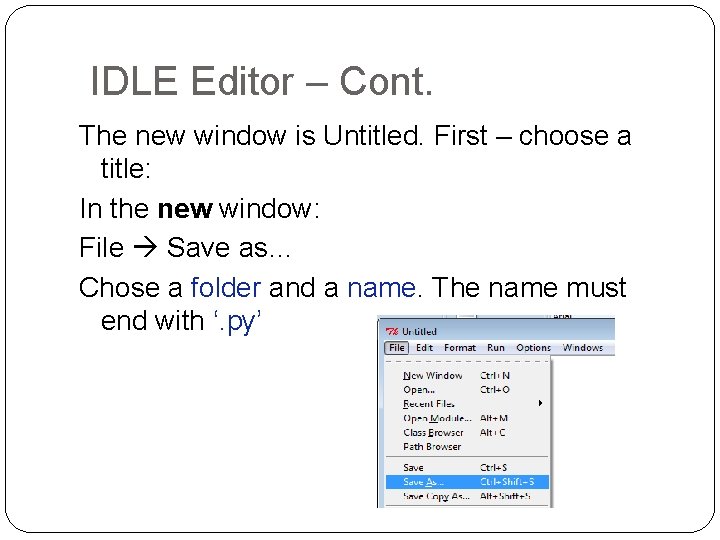
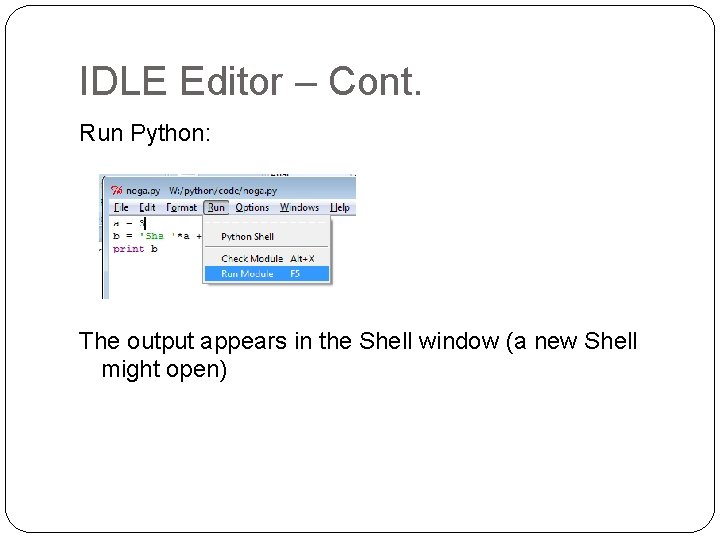
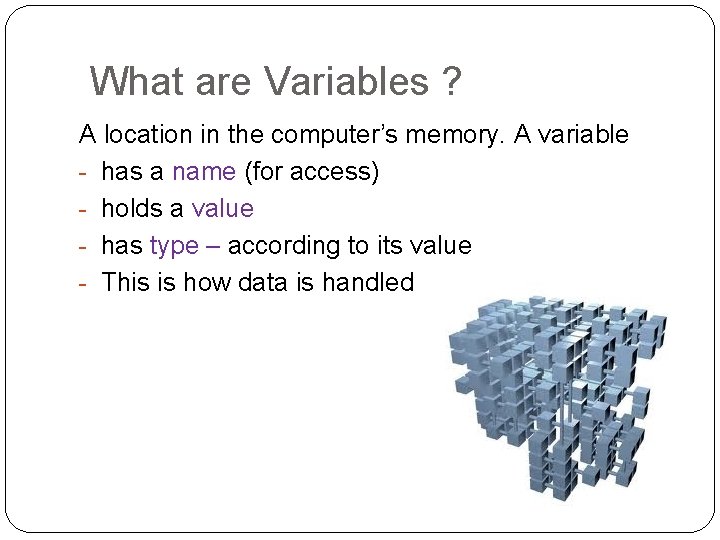
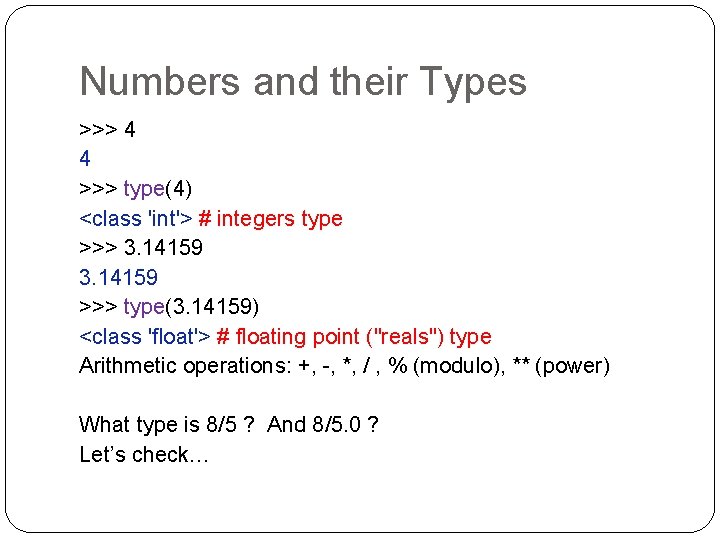
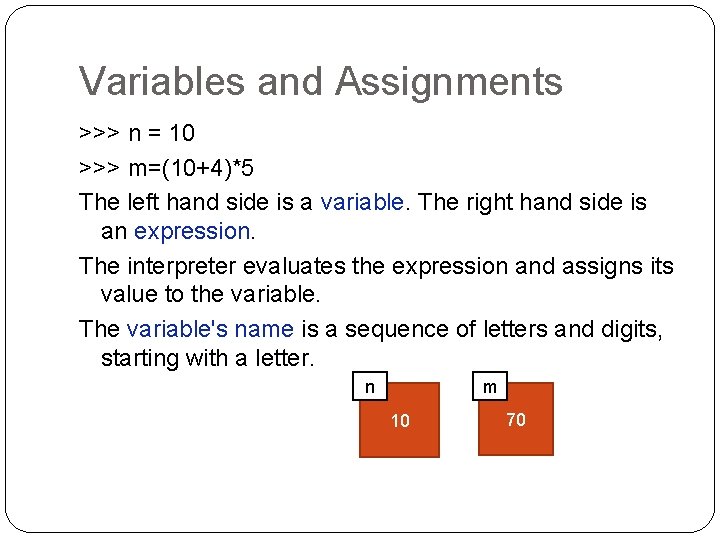
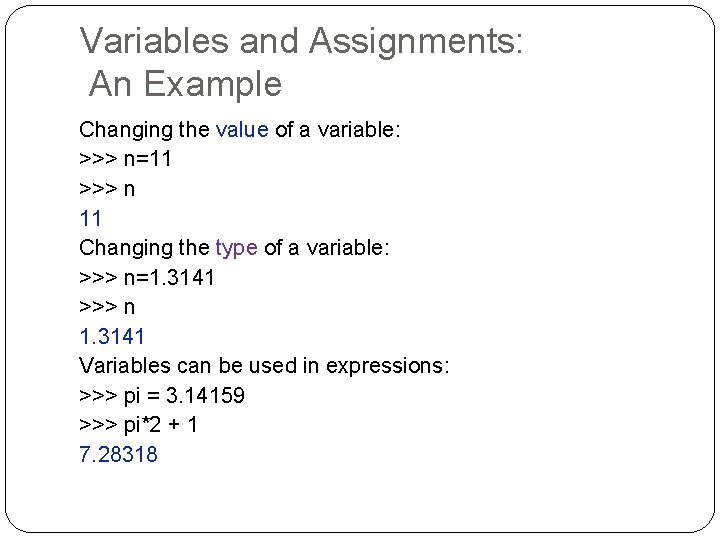
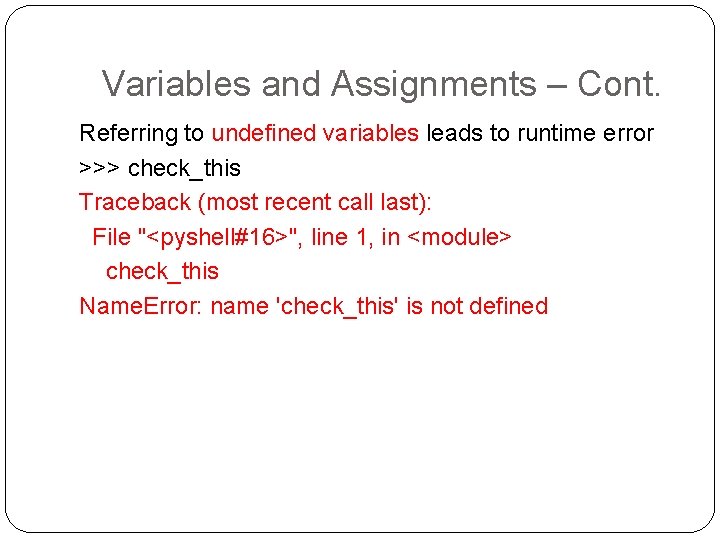
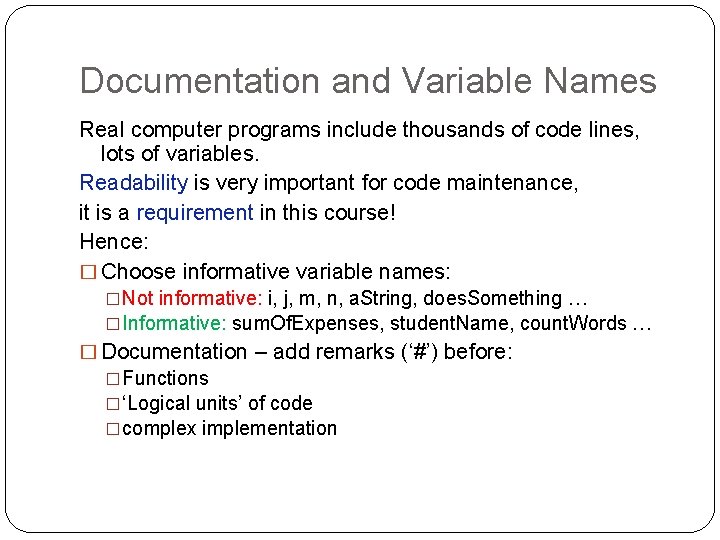
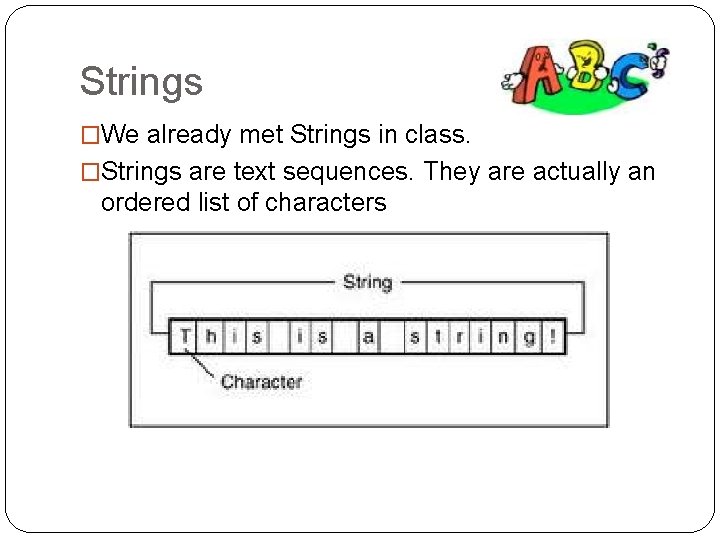
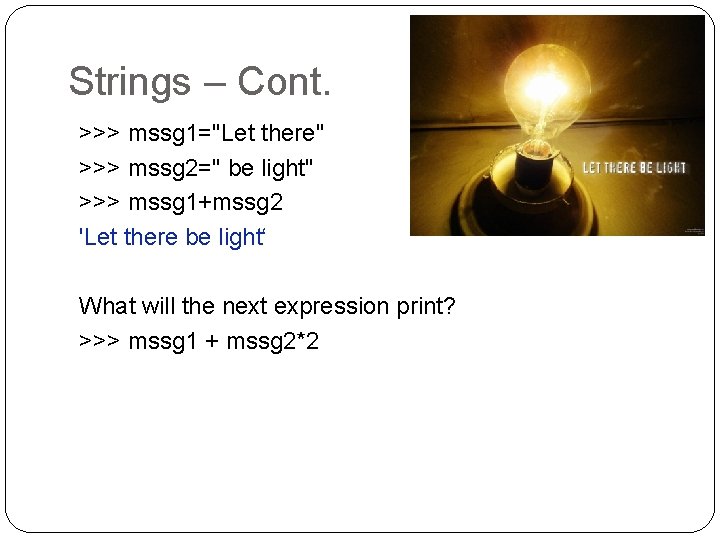
![Strings Access - Reminder >>> a=‘Hello’ >>> a[1: 3] 'el' >>> a[1: ] 'ello' Strings Access - Reminder >>> a=‘Hello’ >>> a[1: 3] 'el' >>> a[1: ] 'ello'](https://slidetodoc.com/presentation_image_h2/e86859fcbfb0d5dd6748a7cc8c4c7209/image-19.jpg)
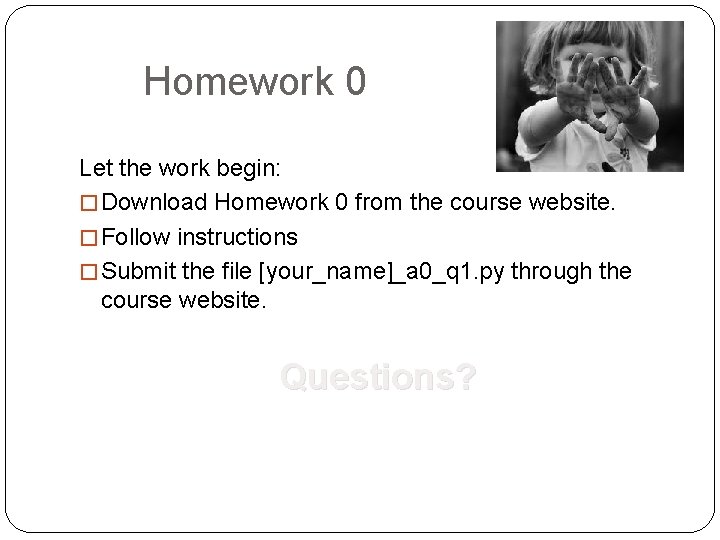
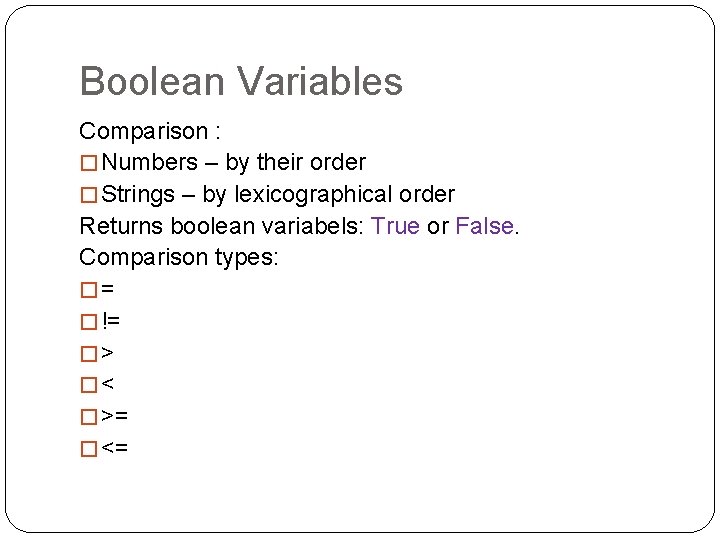
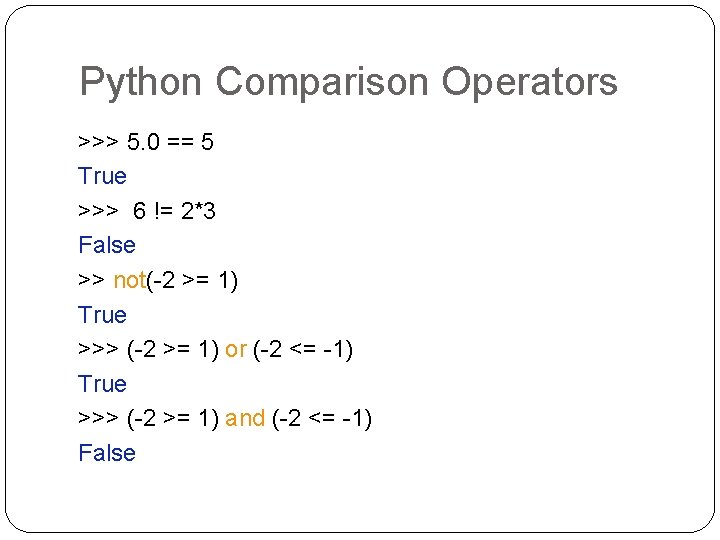
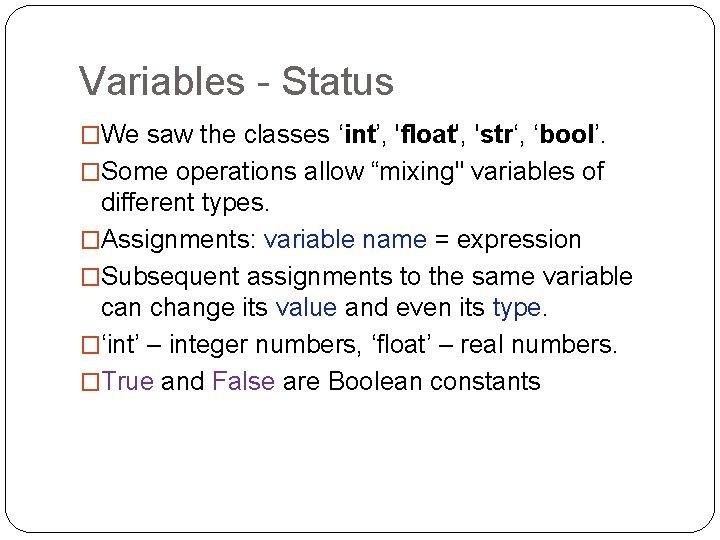
![Conditional Statements if <condition>: do something [else: do something else] Conditional Statements if <condition>: do something [else: do something else]](https://slidetodoc.com/presentation_image_h2/e86859fcbfb0d5dd6748a7cc8c4c7209/image-24.jpg)
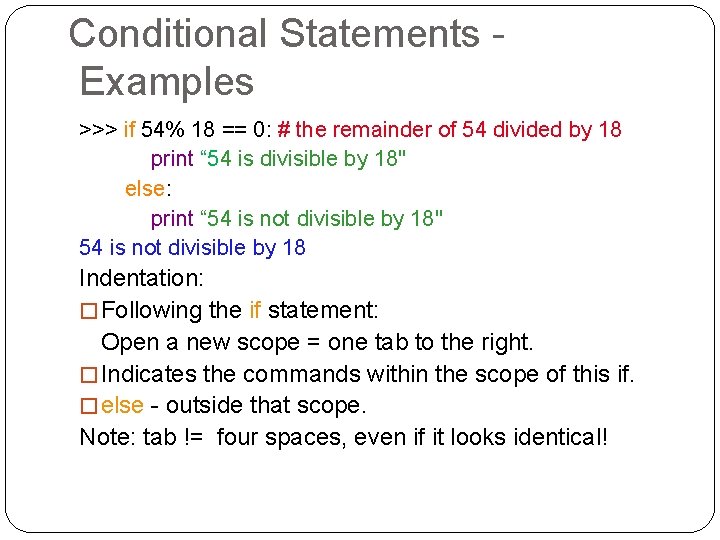
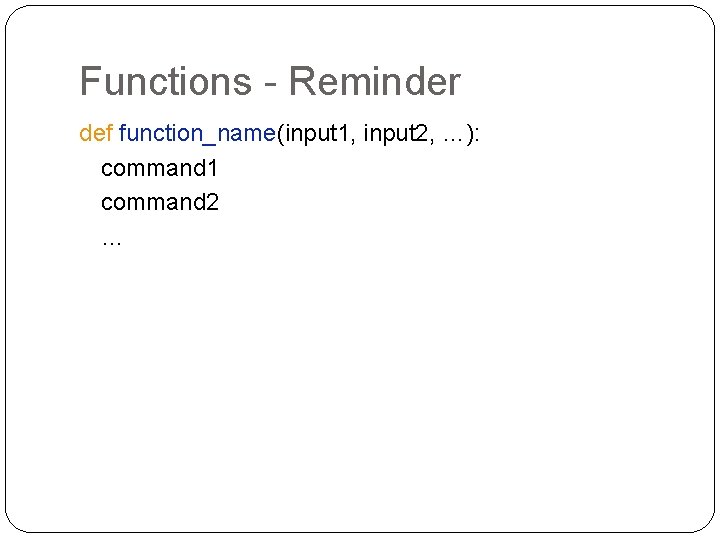
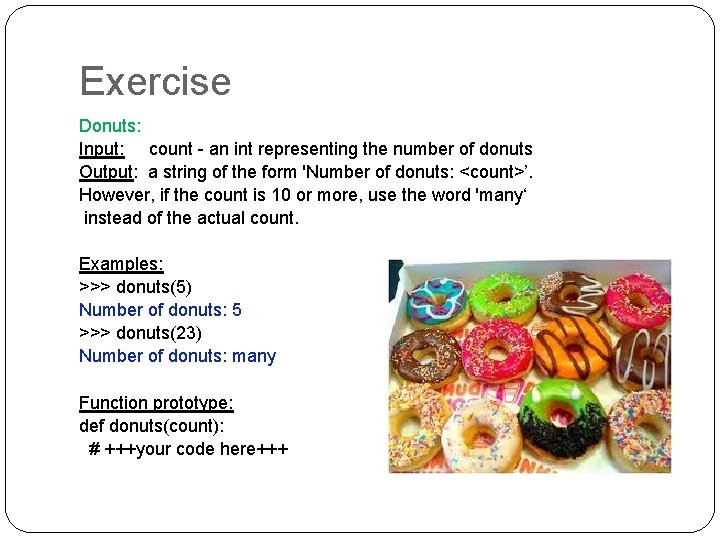
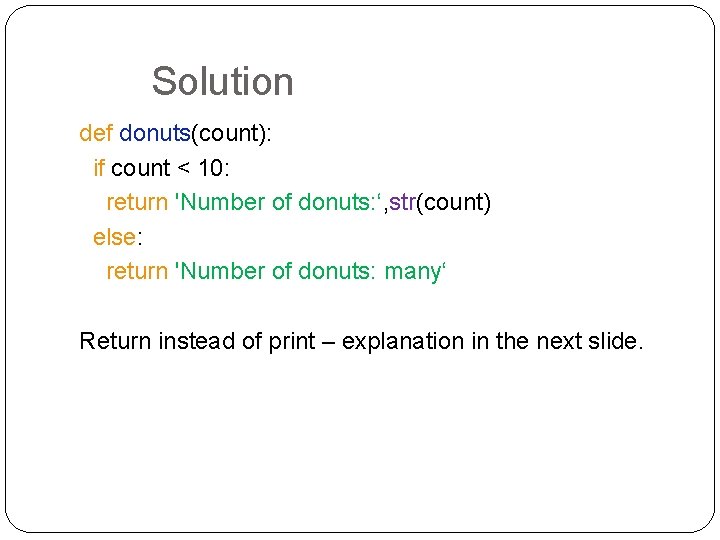
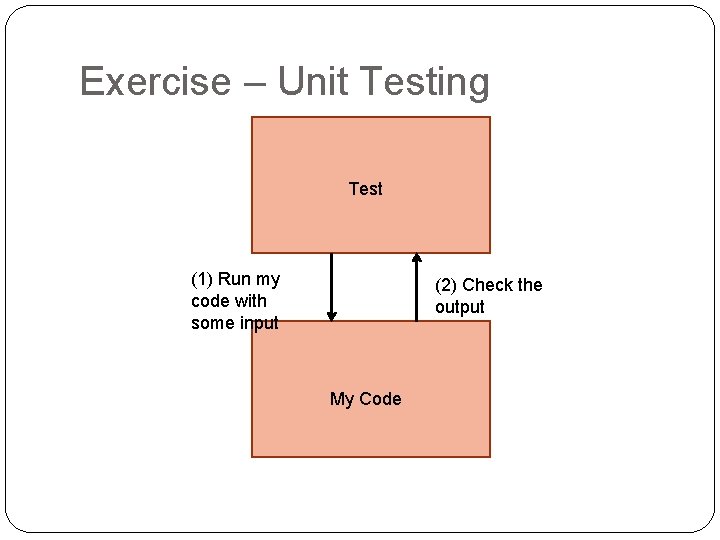
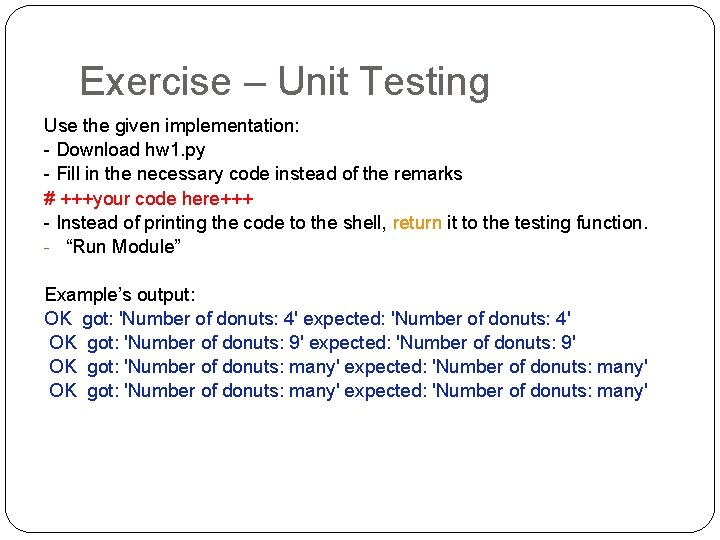
- Slides: 30
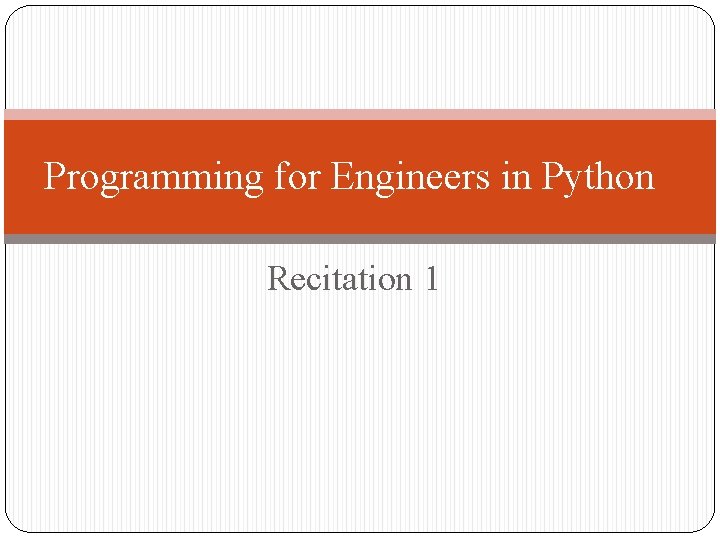
Programming for Engineers in Python Recitation 1
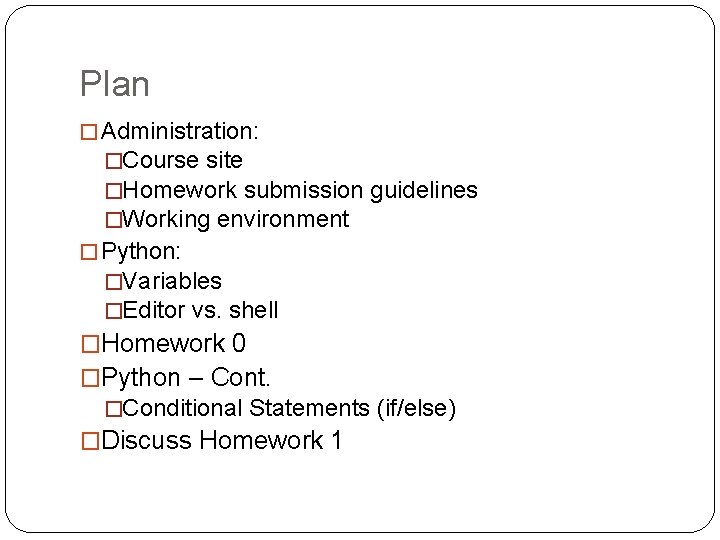
Plan � Administration: �Course site �Homework submission guidelines �Working environment � Python: �Variables �Editor vs. shell �Homework 0 �Python – Cont. �Conditional Statements (if/else) �Discuss Homework 1
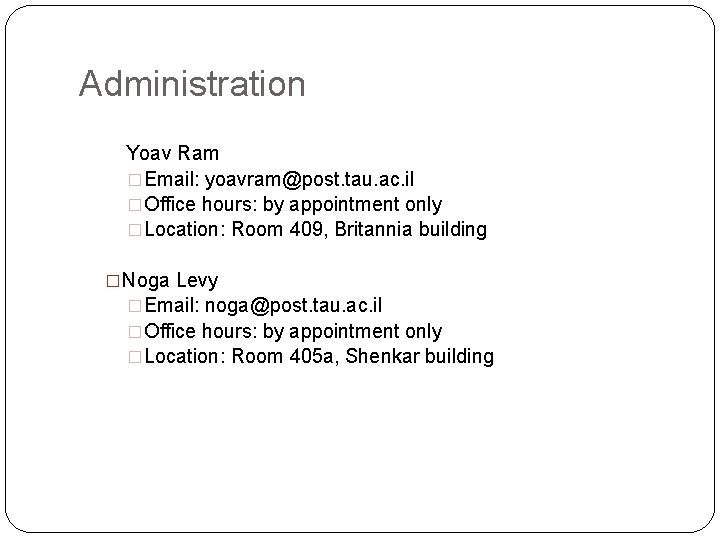
Administration Yoav Ram �Email: yoavram@post. tau. ac. il �Office hours: by appointment only �Location: Room 409, Britannia building �Noga Levy �Email: noga@post. tau. ac. il �Office hours: by appointment only �Location: Room 405 a, Shenkar building
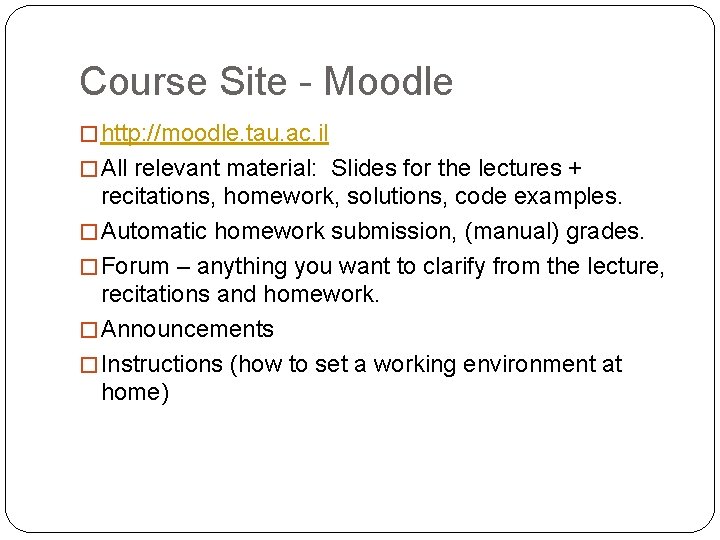
Course Site - Moodle � http: //moodle. tau. ac. il � All relevant material: Slides for the lectures + recitations, homework, solutions, code examples. � Automatic homework submission, (manual) grades. � Forum – anything you want to clarify from the lecture, recitations and homework. � Announcements � Instructions (how to set a working environment at home)
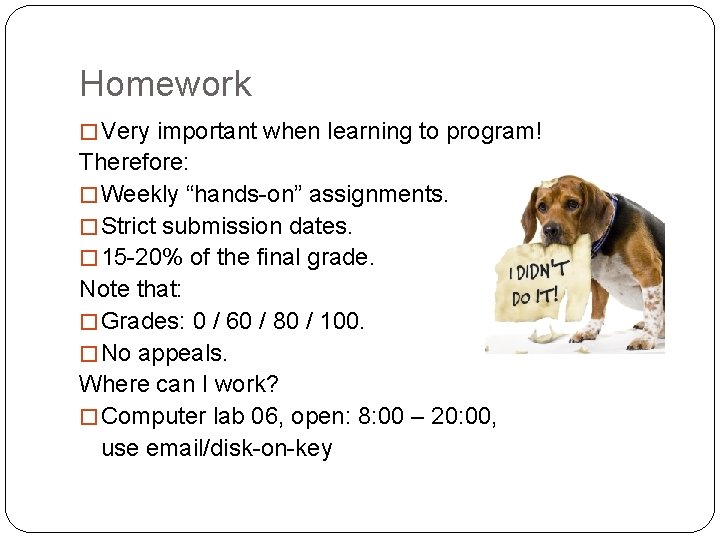
Homework � Very important when learning to program! Therefore: � Weekly “hands-on” assignments. � Strict submission dates. � 15 -20% of the final grade. Note that: � Grades: 0 / 60 / 80 / 100. � No appeals. Where can I work? � Computer lab 06, open: 8: 00 – 20: 00, use email/disk-on-key
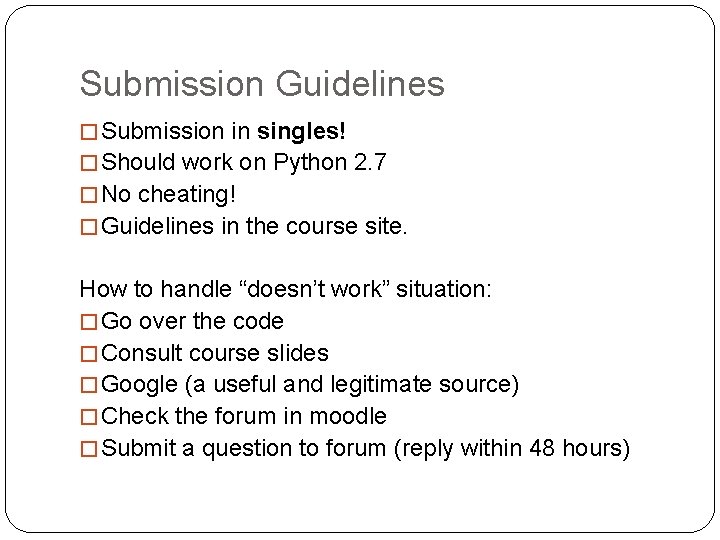
Submission Guidelines � Submission in singles! � Should work on Python 2. 7 � No cheating! � Guidelines in the course site. How to handle “doesn’t work” situation: � Go over the code � Consult course slides � Google (a useful and legitimate source) � Check the forum in moodle � Submit a question to forum (reply within 48 hours)
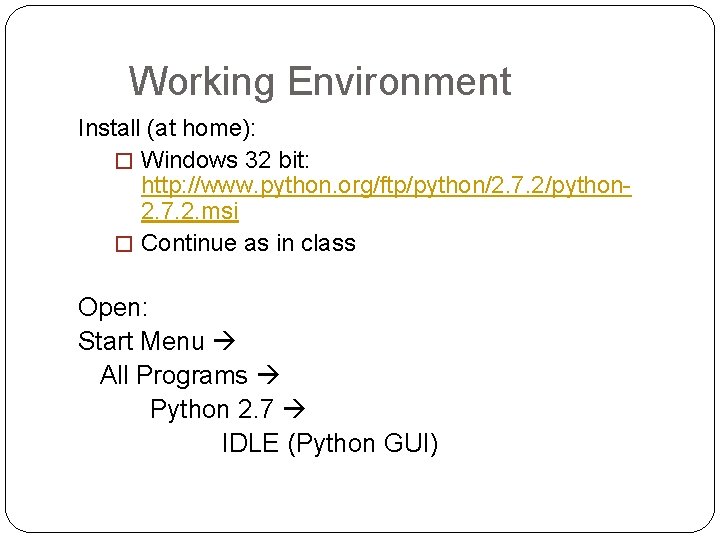
Working Environment Install (at home): � Windows 32 bit: http: //www. python. org/ftp/python/2. 7. 2/python 2. 7. 2. msi � Continue as in class Open: Start Menu All Programs Python 2. 7 IDLE (Python GUI)
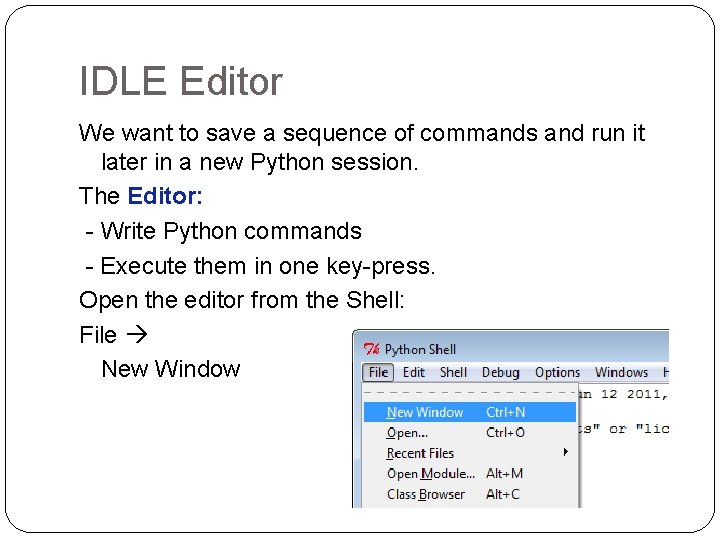
IDLE Editor We want to save a sequence of commands and run it later in a new Python session. The Editor: - Write Python commands - Execute them in one key-press. Open the editor from the Shell: File New Window
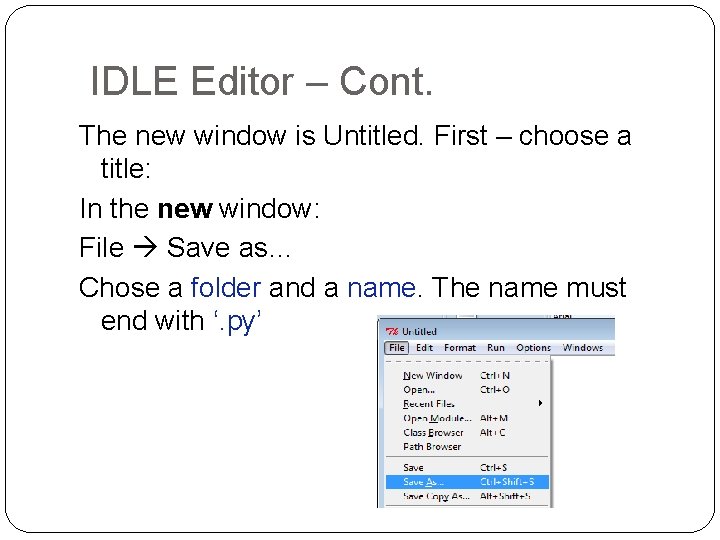
IDLE Editor – Cont. The new window is Untitled. First – choose a title: In the new window: File Save as… Chose a folder and a name. The name must end with ‘. py’
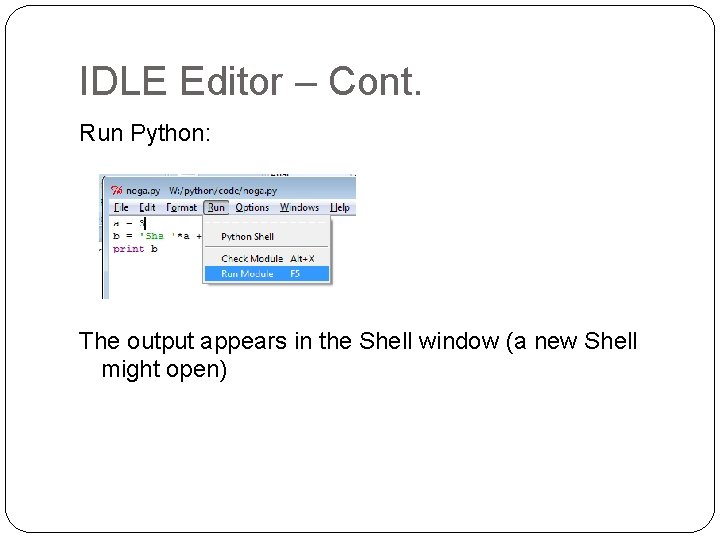
IDLE Editor – Cont. Run Python: The output appears in the Shell window (a new Shell might open)
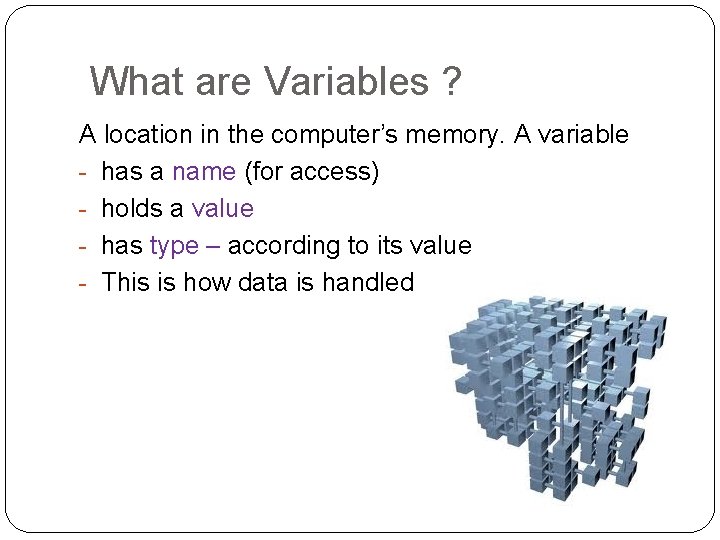
What are Variables ? A location in the computer’s memory. A variable - has a name (for access) - holds a value - has type – according to its value - This is how data is handled
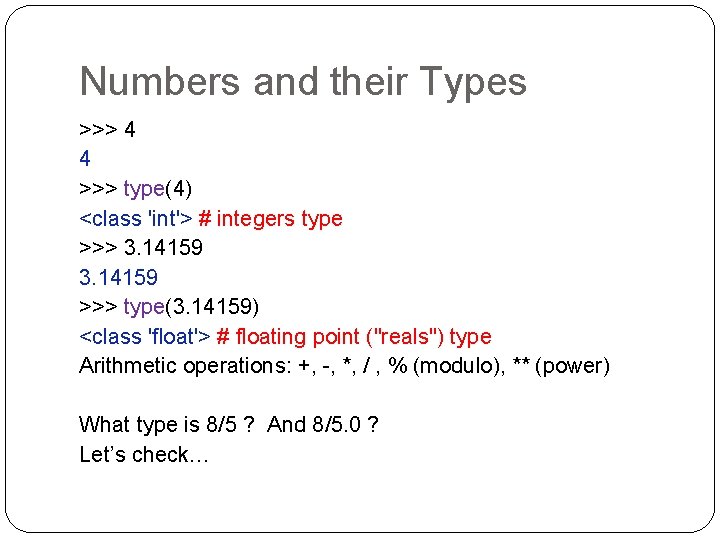
Numbers and their Types >>> 4 4 >>> type(4) <class 'int'> # integers type >>> 3. 14159 >>> type(3. 14159) <class 'float'> # floating point ("reals") type Arithmetic operations: +, -, *, / , % (modulo), ** (power) What type is 8/5 ? And 8/5. 0 ? Let’s check…
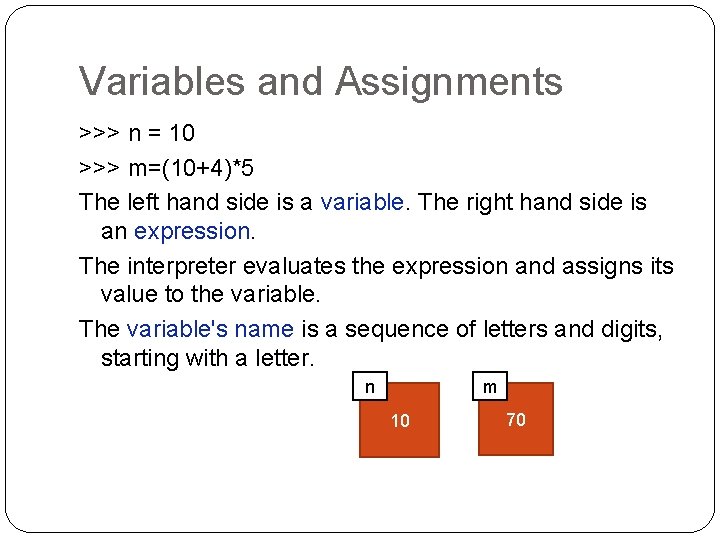
Variables and Assignments >>> n = 10 >>> m=(10+4)*5 The left hand side is a variable. The right hand side is an expression. The interpreter evaluates the expression and assigns its value to the variable. The variable's name is a sequence of letters and digits, starting with a letter. n m 10 70
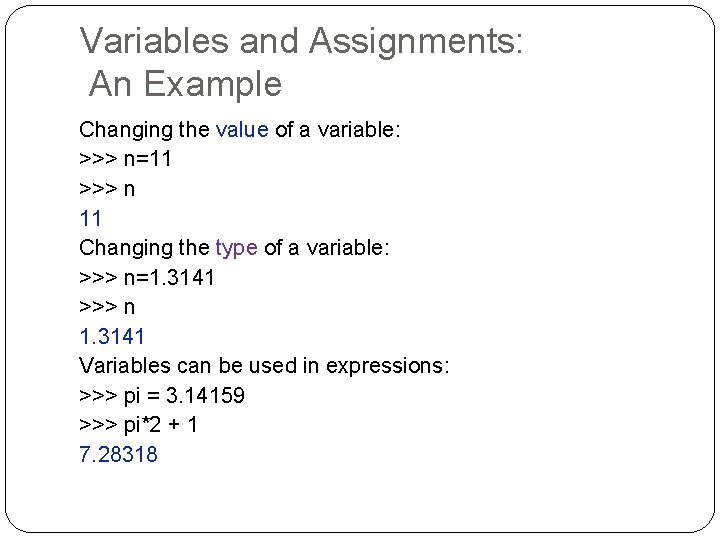
Variables and Assignments: An Example Changing the value of a variable: >>> n=11 >>> n 11 Changing the type of a variable: >>> n=1. 3141 >>> n 1. 3141 Variables can be used in expressions: >>> pi = 3. 14159 >>> pi*2 + 1 7. 28318
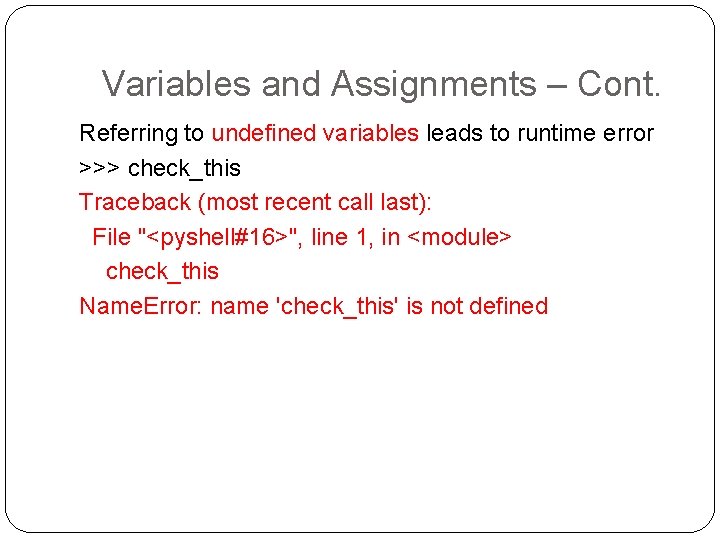
Variables and Assignments – Cont. Referring to undefined variables leads to runtime error >>> check_this Traceback (most recent call last): File "<pyshell#16>", line 1, in <module> check_this Name. Error: name 'check_this' is not defined
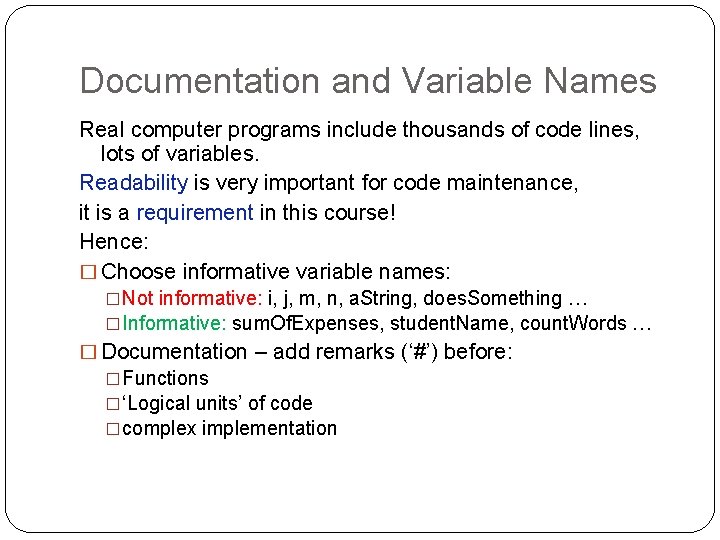
Documentation and Variable Names Real computer programs include thousands of code lines, lots of variables. Readability is very important for code maintenance, it is a requirement in this course! Hence: � Choose informative variable names: �Not informative: i, j, m, n, a. String, does. Something … �Informative: sum. Of. Expenses, student. Name, count. Words … � Documentation – add remarks (‘#’) before: �Functions �‘Logical units’ of code �complex implementation
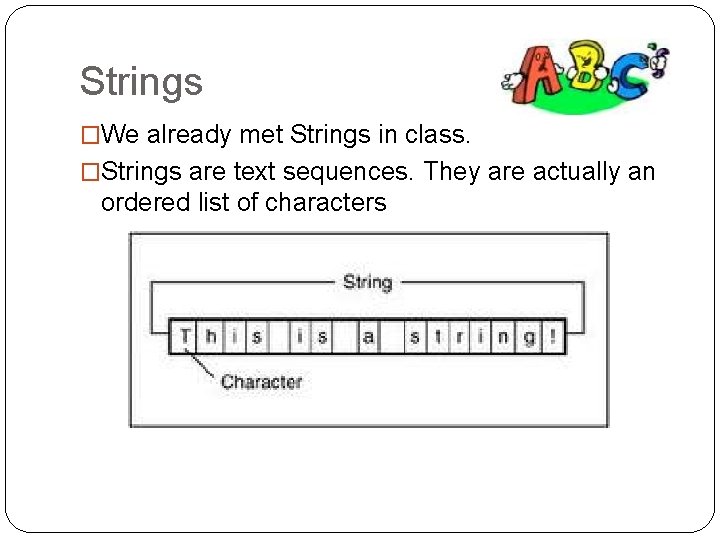
Strings �We already met Strings in class. �Strings are text sequences. They are actually an ordered list of characters
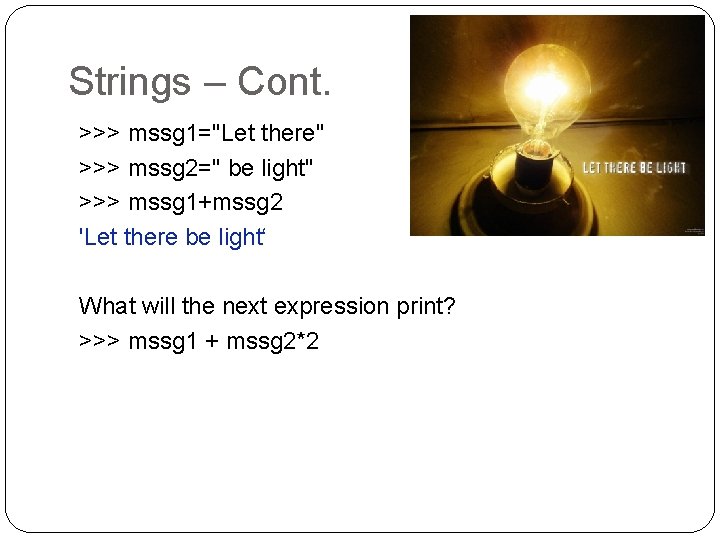
Strings – Cont. >>> mssg 1="Let there" >>> mssg 2=" be light" >>> mssg 1+mssg 2 'Let there be light‘ What will the next expression print? >>> mssg 1 + mssg 2*2
![Strings Access Reminder aHello a1 3 el a1 ello Strings Access - Reminder >>> a=‘Hello’ >>> a[1: 3] 'el' >>> a[1: ] 'ello'](https://slidetodoc.com/presentation_image_h2/e86859fcbfb0d5dd6748a7cc8c4c7209/image-19.jpg)
Strings Access - Reminder >>> a=‘Hello’ >>> a[1: 3] 'el' >>> a[1: ] 'ello' >>> a[-4: -2] 'el' >>> a[: -3] 'He' >>> a[-3: ] 'llo’ H e l l o 0 1 2 3 4 -5 -4 -3 -2 -1
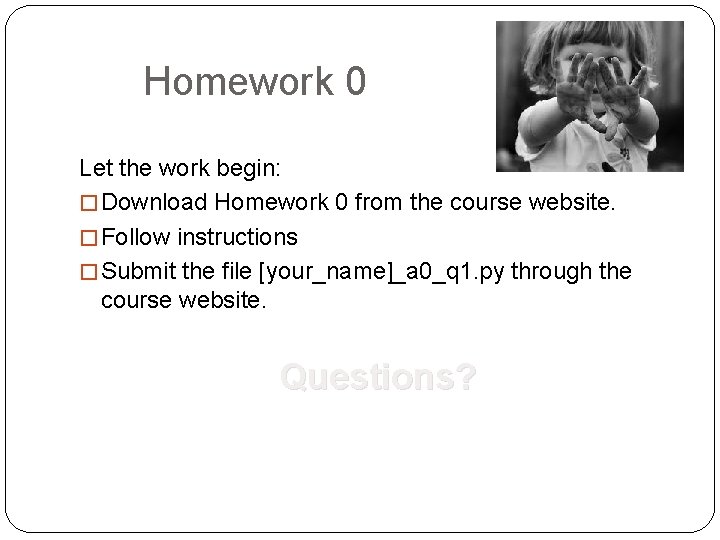
Homework 0 Let the work begin: � Download Homework 0 from the course website. � Follow instructions � Submit the file [your_name]_a 0_q 1. py through the course website. Questions?
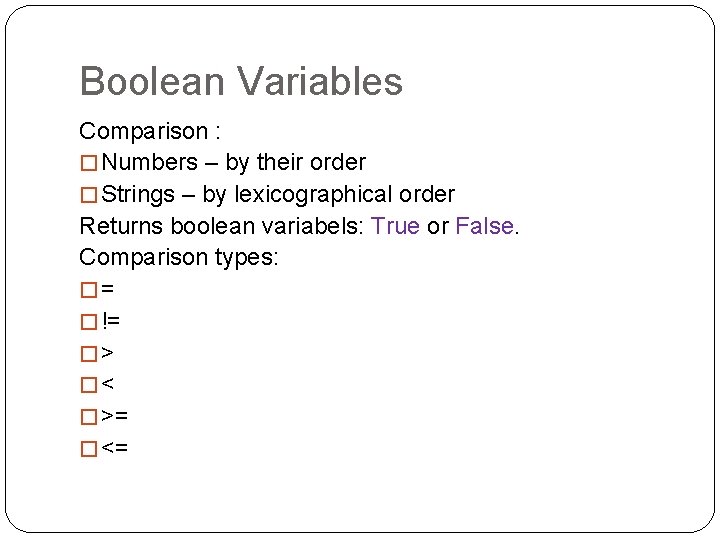
Boolean Variables Comparison : � Numbers – by their order � Strings – by lexicographical order Returns boolean variabels: True or False. Comparison types: �= � != �> �< � >= � <=
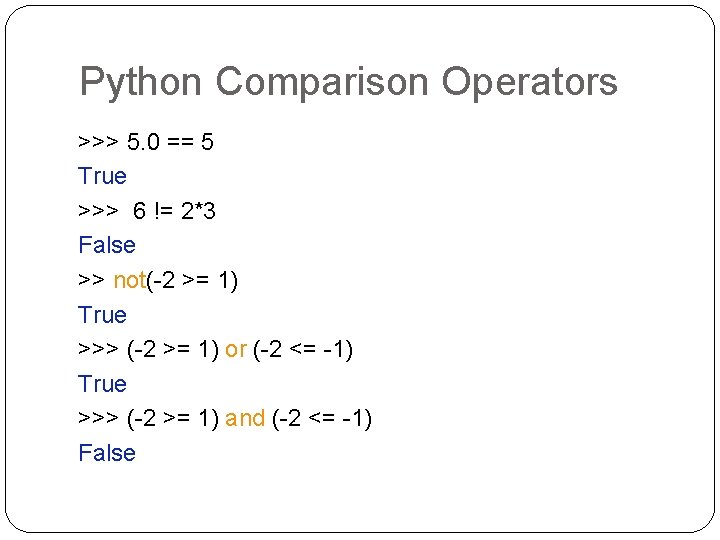
Python Comparison Operators >>> 5. 0 == 5 True >>> 6 != 2*3 False >> not(-2 >= 1) True >>> (-2 >= 1) or (-2 <= -1) True >>> (-2 >= 1) and (-2 <= -1) False
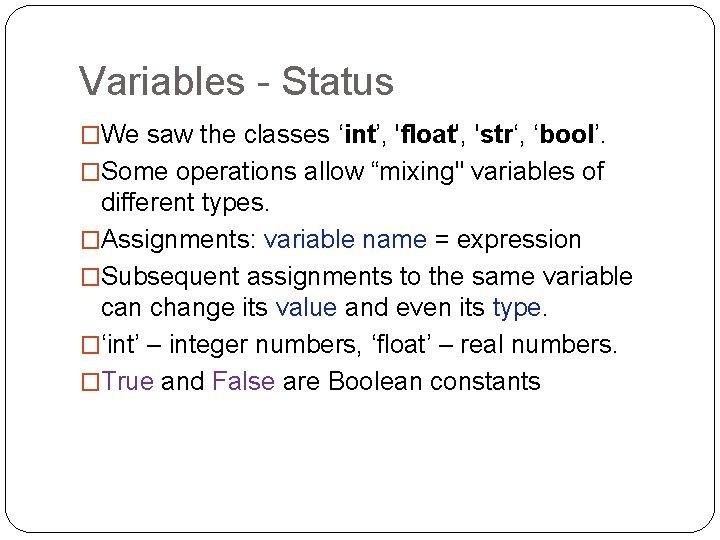
Variables - Status �We saw the classes ‘int’, 'float', 'str‘, ‘bool’. �Some operations allow “mixing" variables of different types. �Assignments: variable name = expression �Subsequent assignments to the same variable can change its value and even its type. �‘int’ – integer numbers, ‘float’ – real numbers. �True and False are Boolean constants
![Conditional Statements if condition do something else do something else Conditional Statements if <condition>: do something [else: do something else]](https://slidetodoc.com/presentation_image_h2/e86859fcbfb0d5dd6748a7cc8c4c7209/image-24.jpg)
Conditional Statements if <condition>: do something [else: do something else]
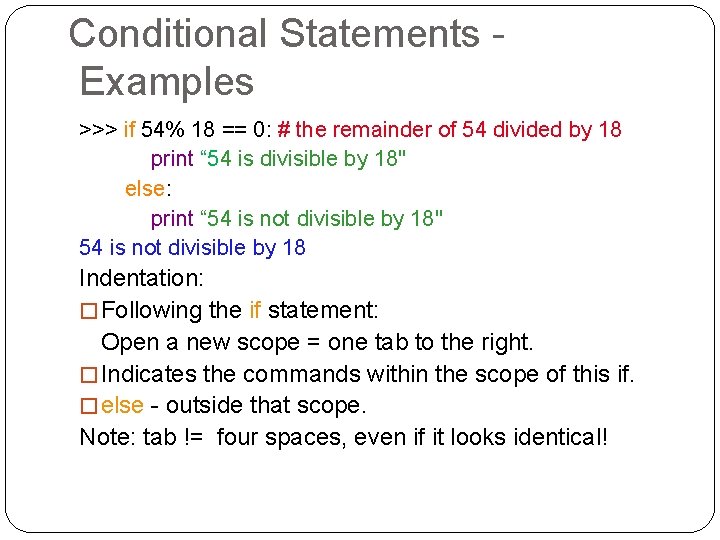
Conditional Statements Examples >>> if 54% 18 == 0: # the remainder of 54 divided by 18 print “ 54 is divisible by 18" else: print “ 54 is not divisible by 18" 54 is not divisible by 18 Indentation: � Following the if statement: Open a new scope = one tab to the right. � Indicates the commands within the scope of this if. � else - outside that scope. Note: tab != four spaces, even if it looks identical!
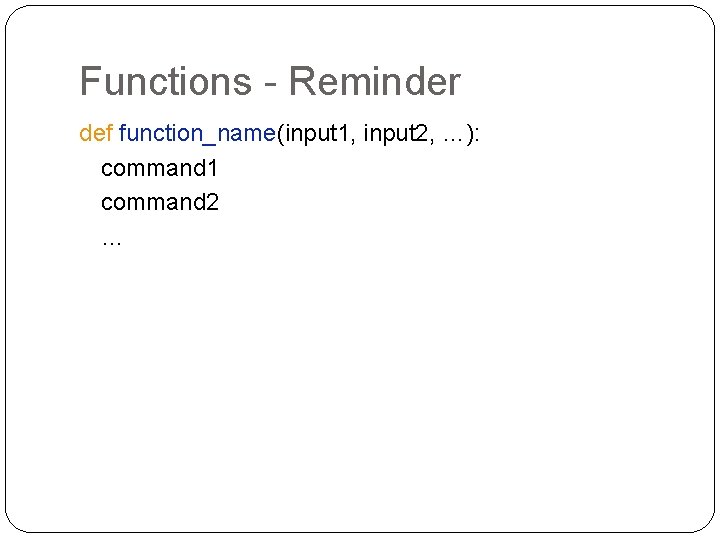
Functions - Reminder def function_name(input 1, input 2, …): command 1 command 2 …
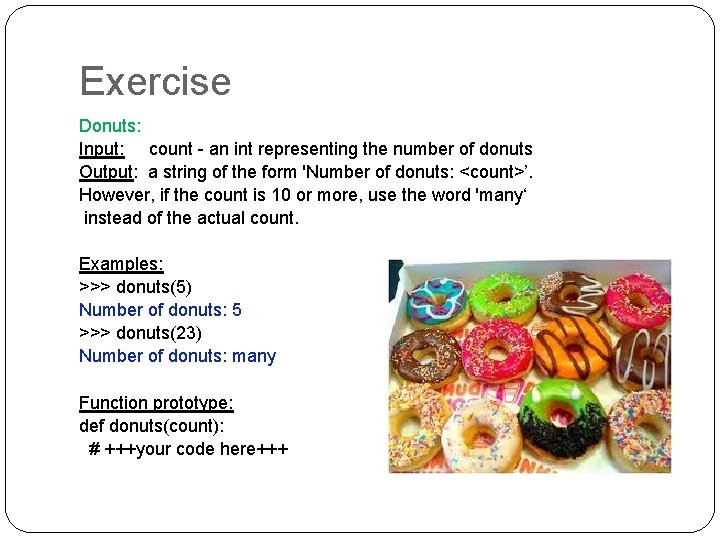
Exercise Donuts: Input: count - an int representing the number of donuts Output: a string of the form 'Number of donuts: <count>’. However, if the count is 10 or more, use the word 'many‘ instead of the actual count. Examples: >>> donuts(5) Number of donuts: 5 >>> donuts(23) Number of donuts: many Function prototype: def donuts(count): # +++your code here+++
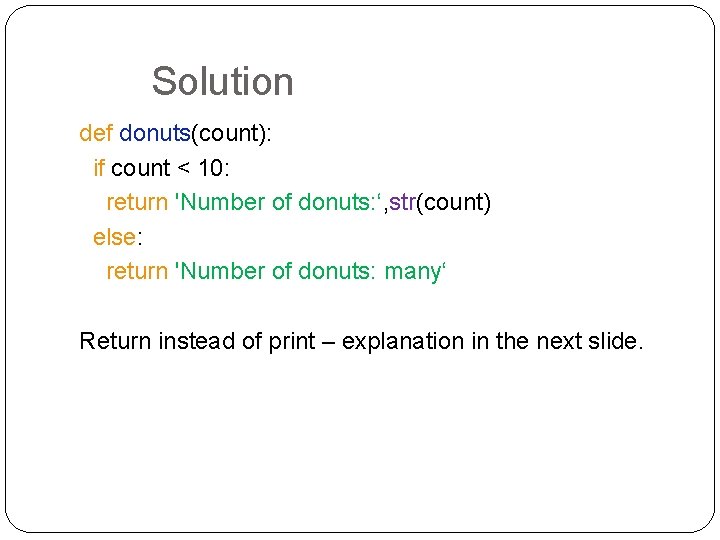
Solution def donuts(count): if count < 10: return 'Number of donuts: ‘, str(count) else: return 'Number of donuts: many‘ Return instead of print – explanation in the next slide.
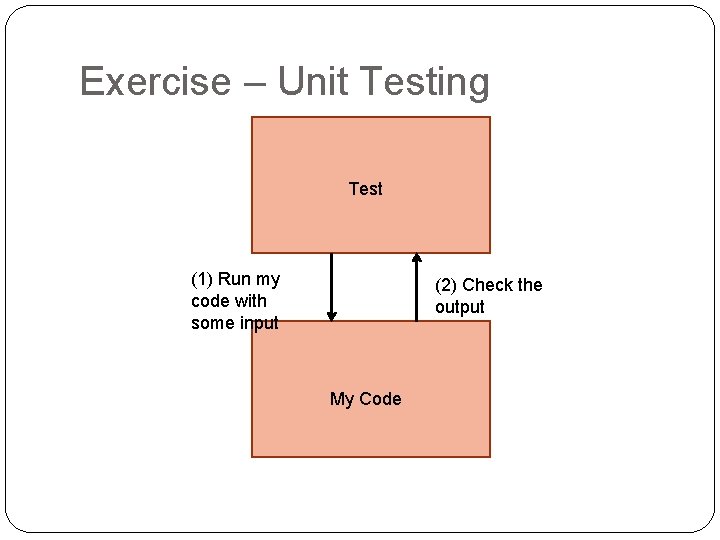
Exercise – Unit Testing Test (1) Run my code with some input (2) Check the output My Code
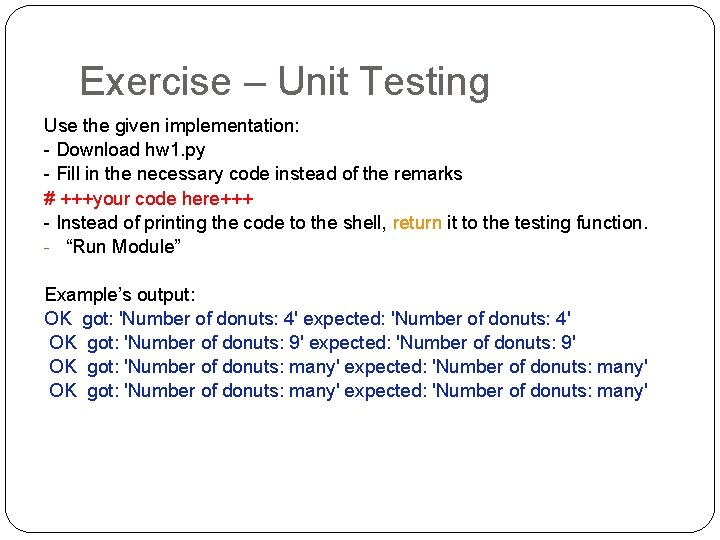
Exercise – Unit Testing Use the given implementation: - Download hw 1. py - Fill in the necessary code instead of the remarks # +++your code here+++ - Instead of printing the code to the shell, return it to the testing function. - “Run Module” Example’s output: OK got: 'Number of donuts: 4' expected: 'Number of donuts: 4' OK got: 'Number of donuts: 9' expected: 'Number of donuts: 9' OK got: 'Number of donuts: many' expected: 'Number of donuts: many'
Introduction to matlab for engineers
Passive recitation
Sajjdah
Impromptu speaking business
Poem recitation objectives
Tips for reciting poetry
What is meant by etiquette of recitation of the holy quran
Xvvvxv
What is performance marketing
Récitation les hiboux
Python procedural programming
Python chapter 5
Second order cone programming python
Python programming in context
Constraint programming python
Rapid gui programming with python and qt
Python programming in context
Cgi python
Pygaxy
Python programming
Programming essentials in python
Python blackjack oop
Python programming an introduction to computer science
Perbedaan linear programming dan integer programming
Greedy vs dynamic
System programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
Fspos
Novell typiska drag
Tack för att ni lyssnade bild