Programming for Artists ART 315 Dr J R
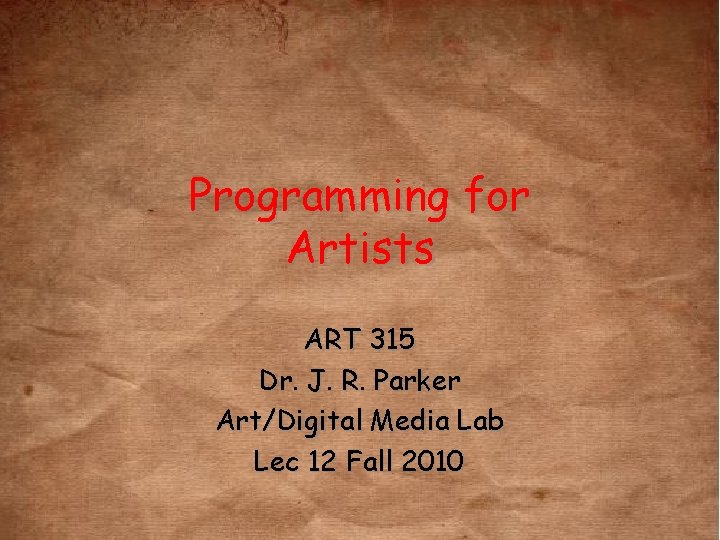
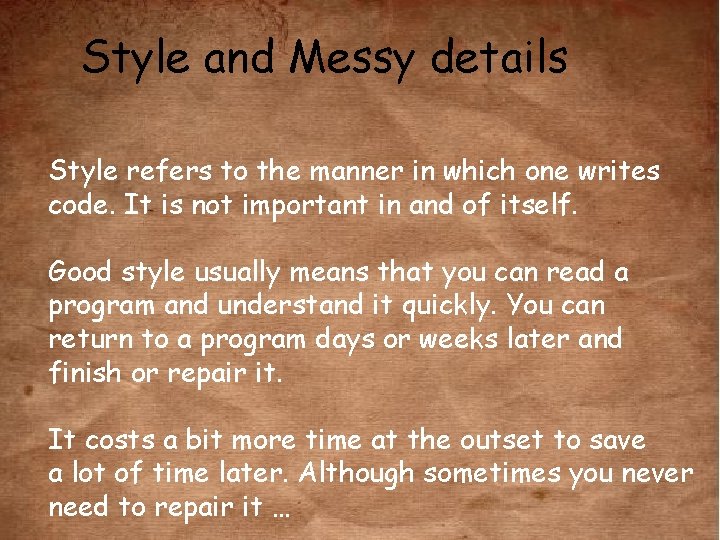
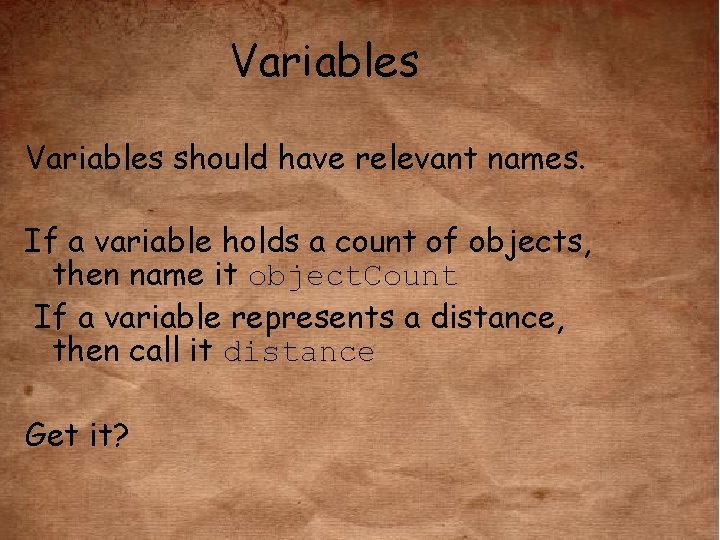
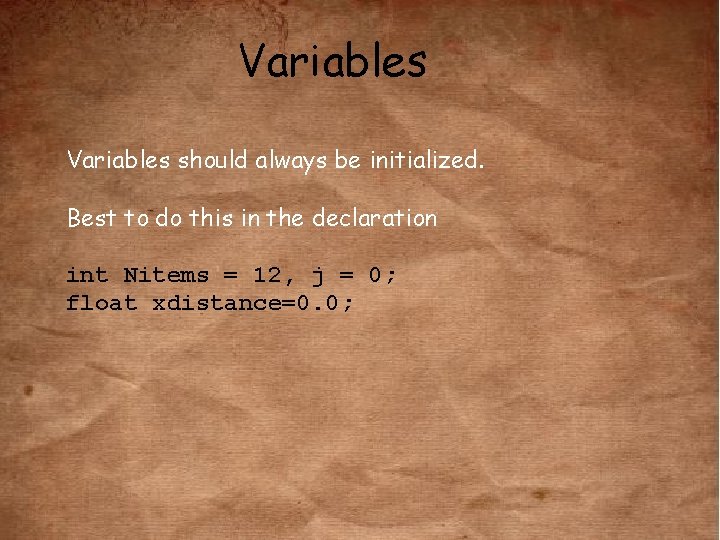
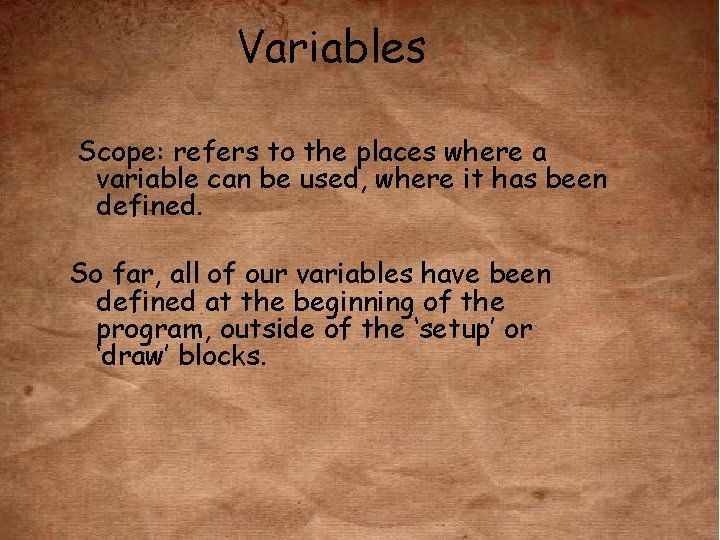
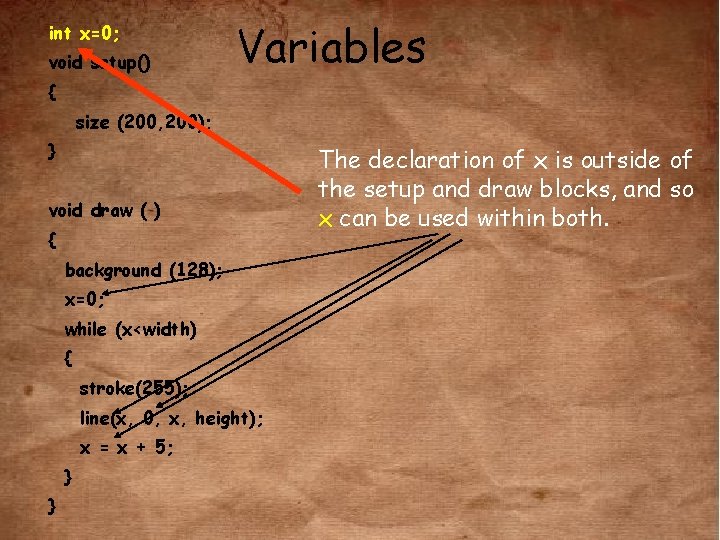
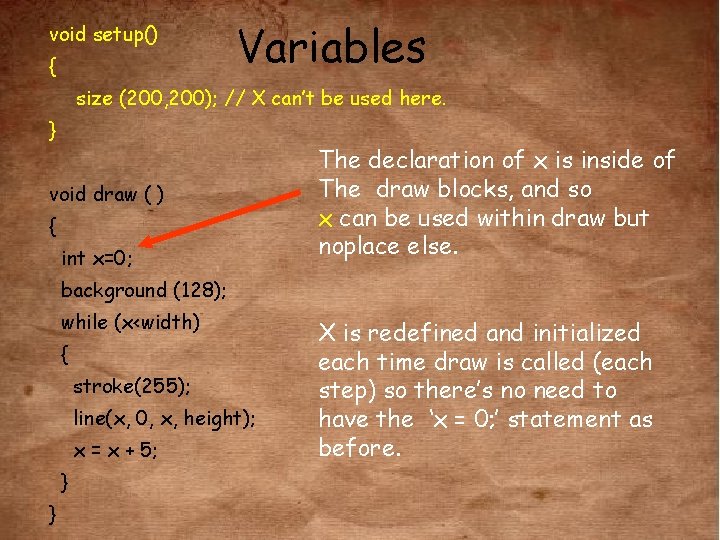
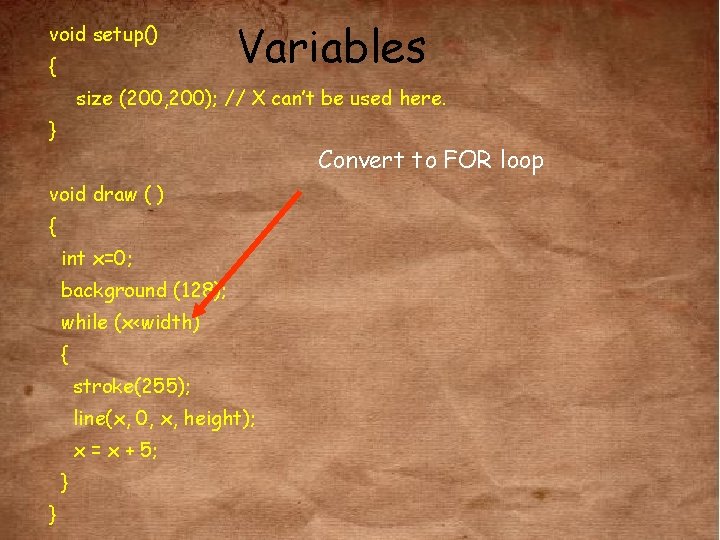
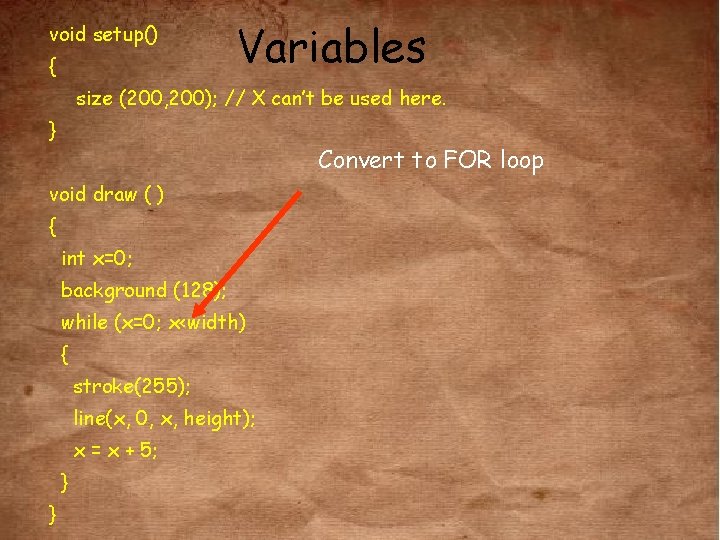
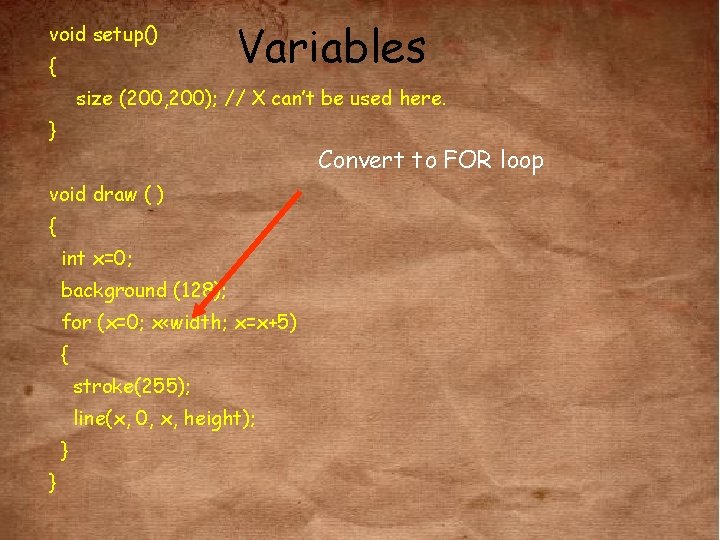
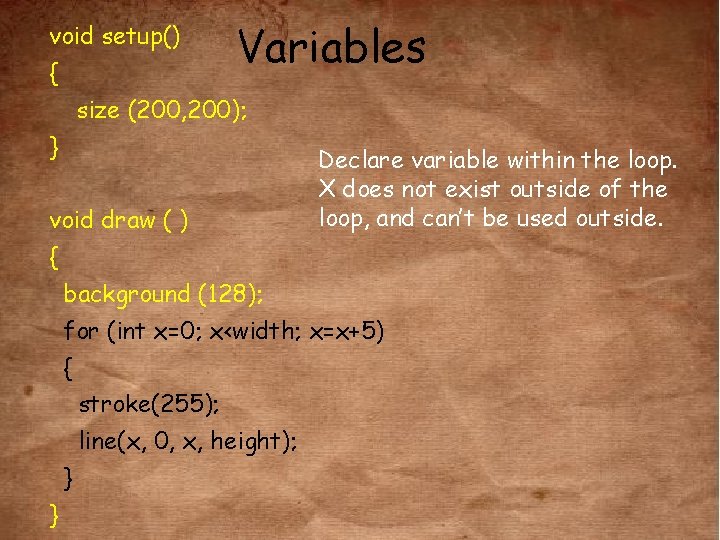
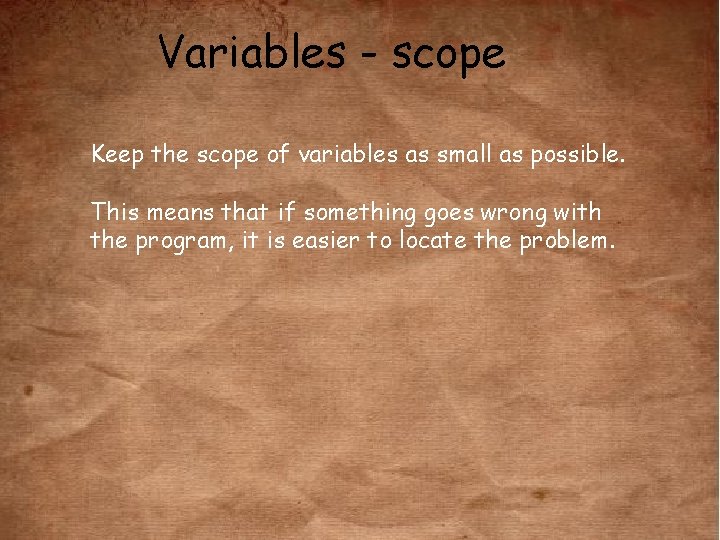
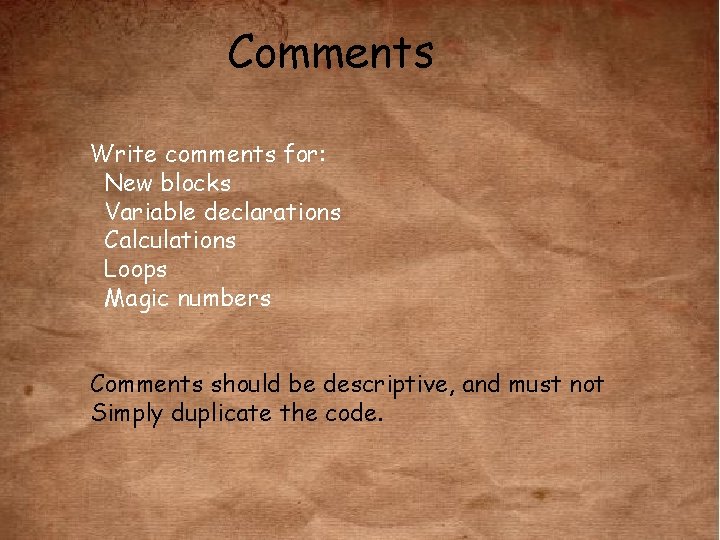
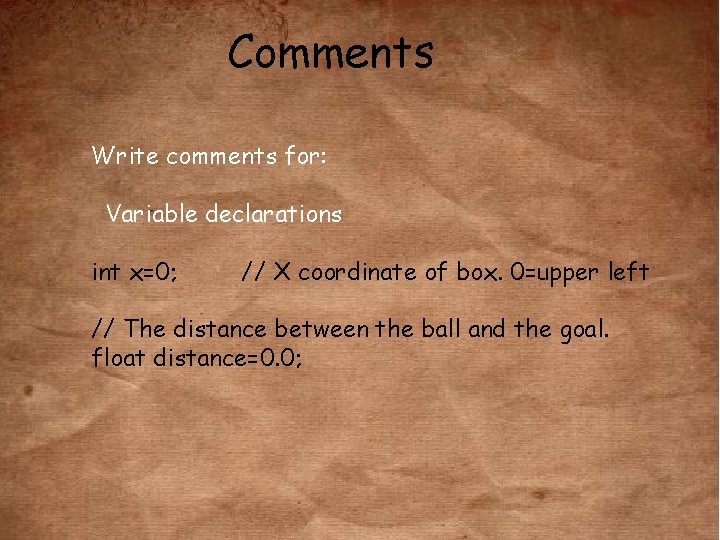
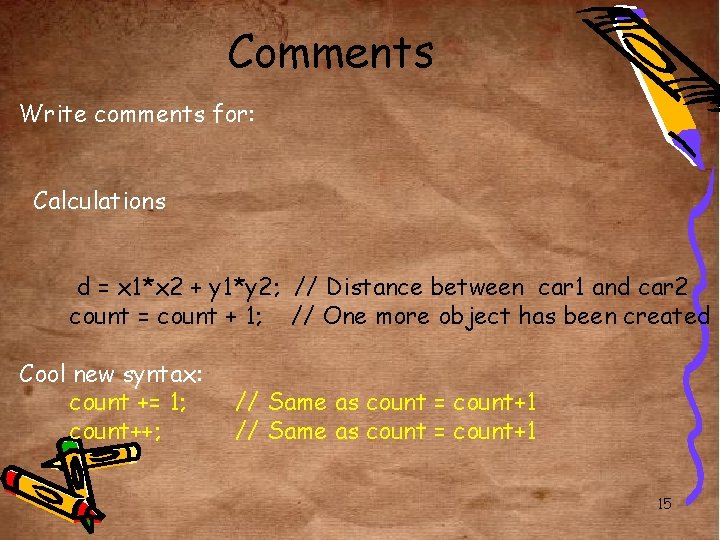
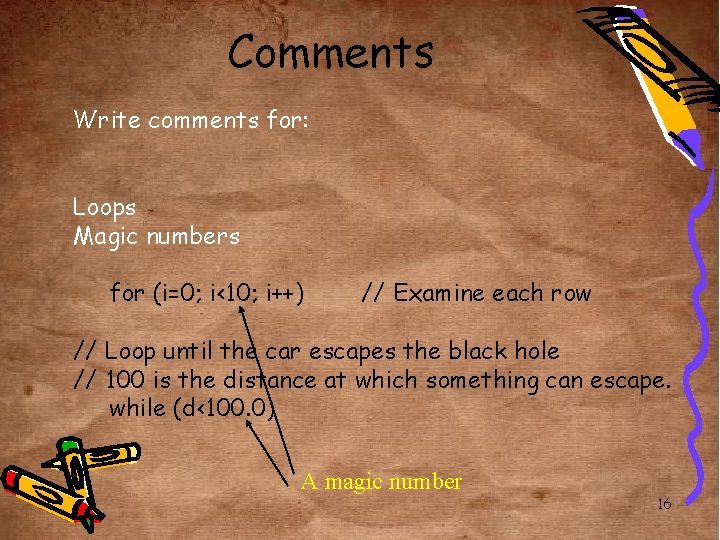
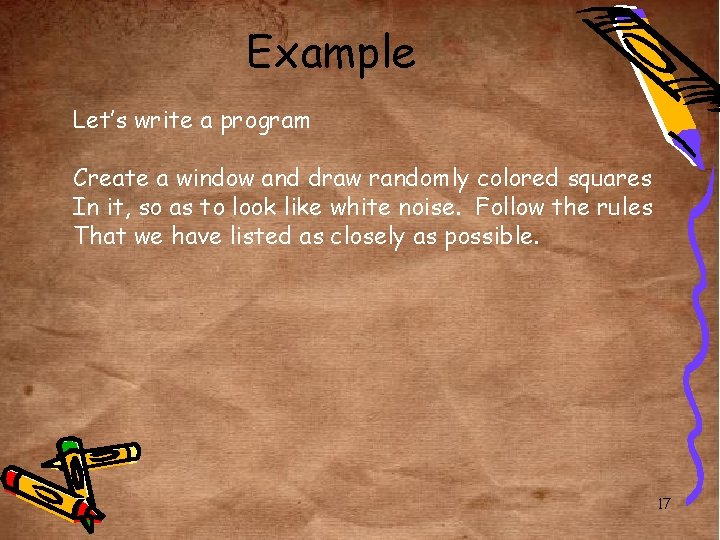
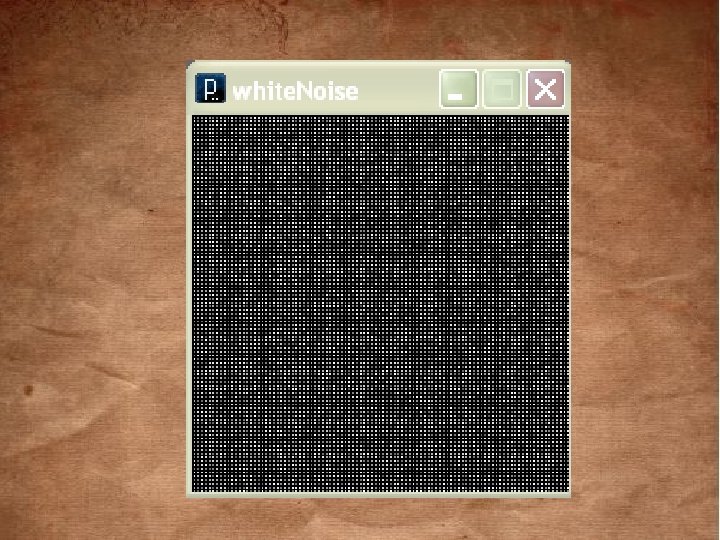
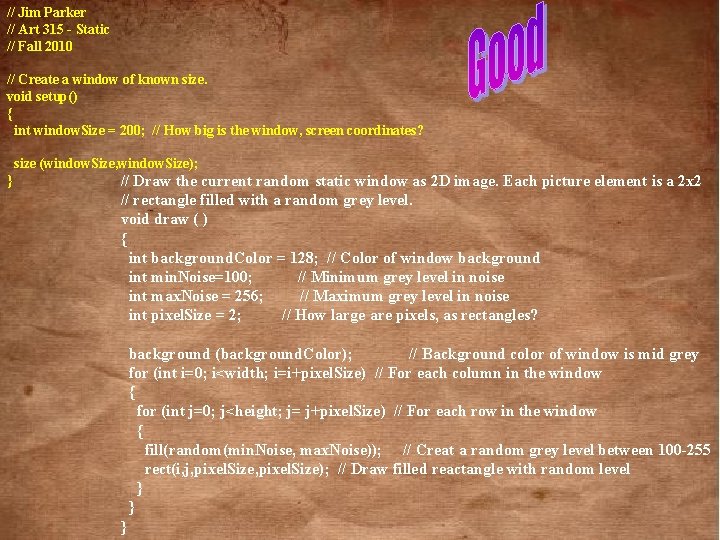
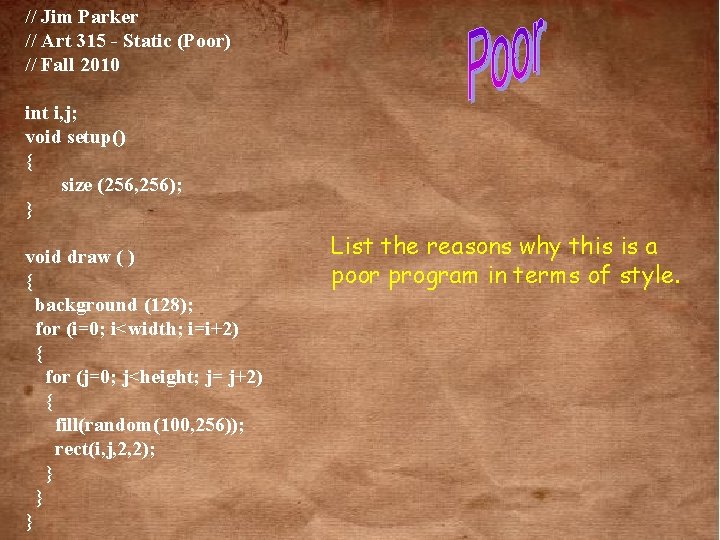
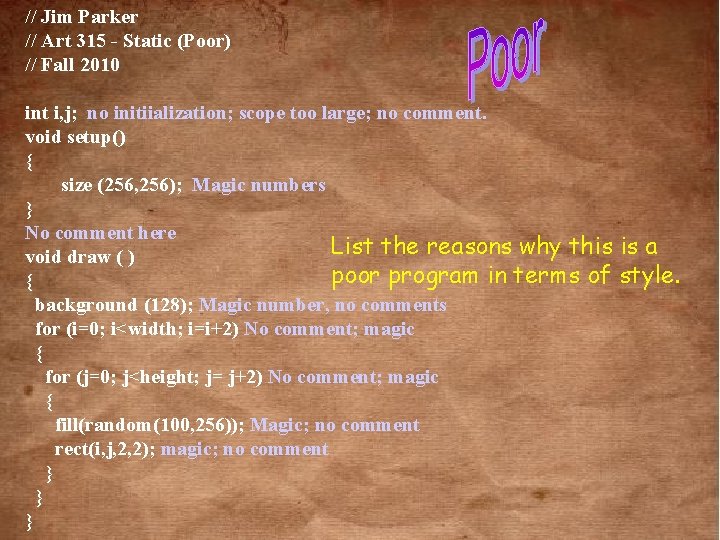
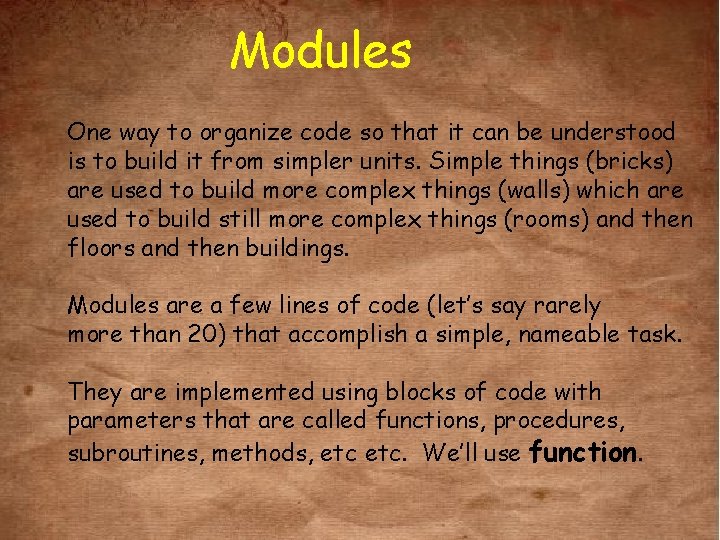
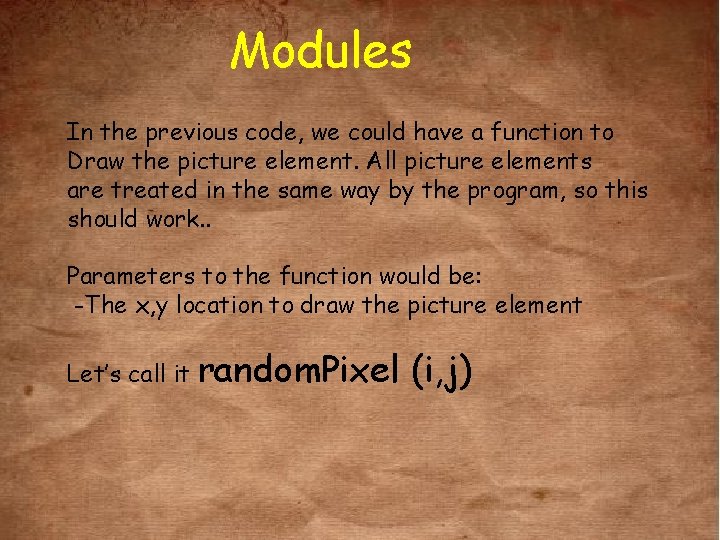
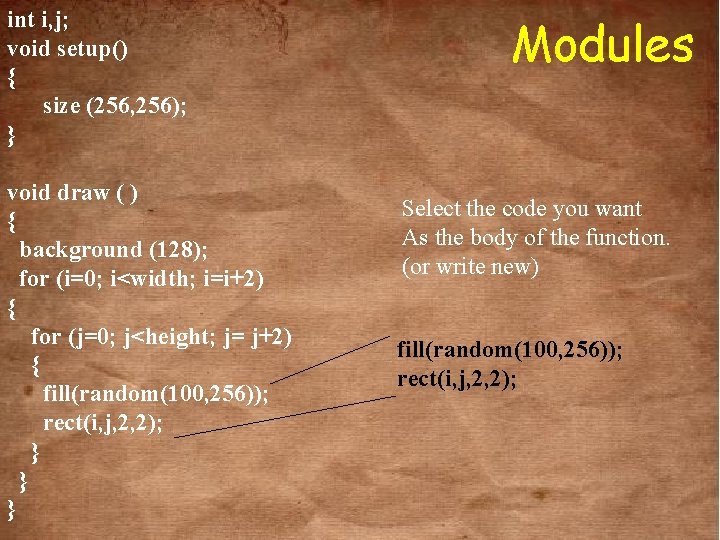
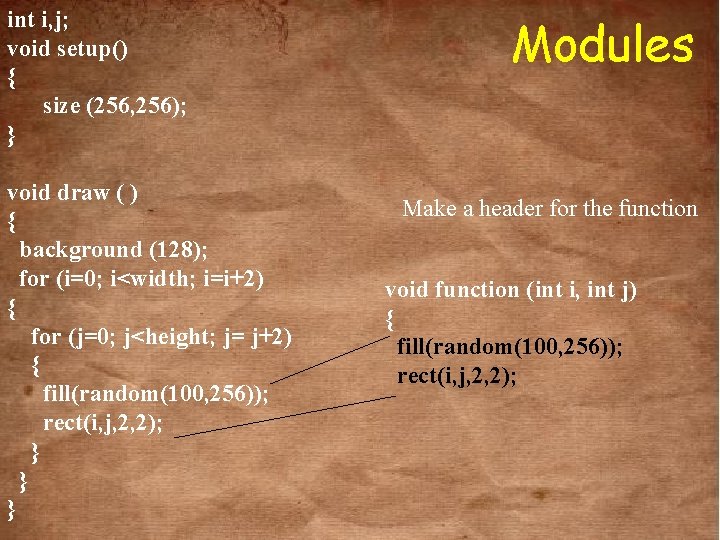
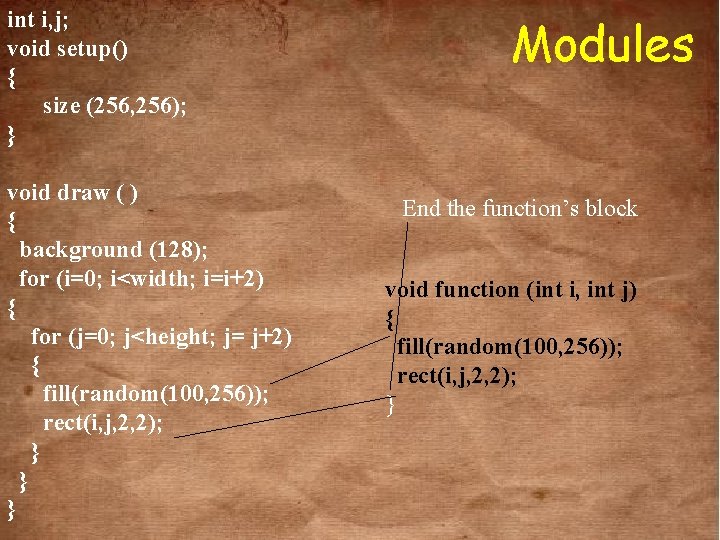
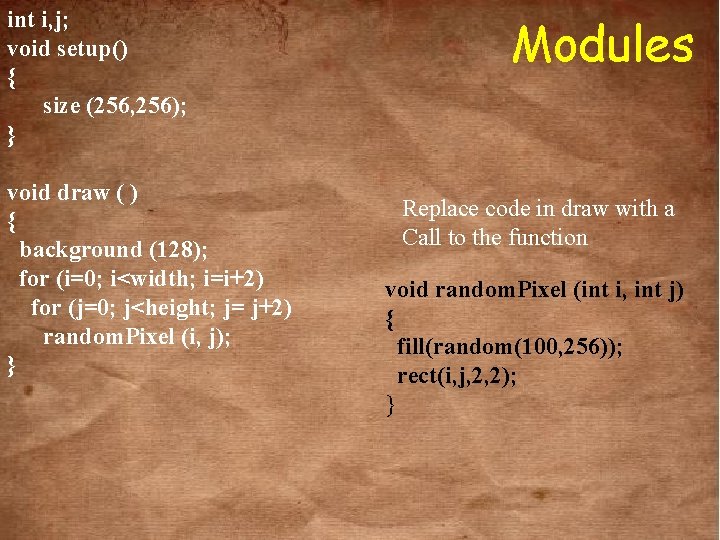
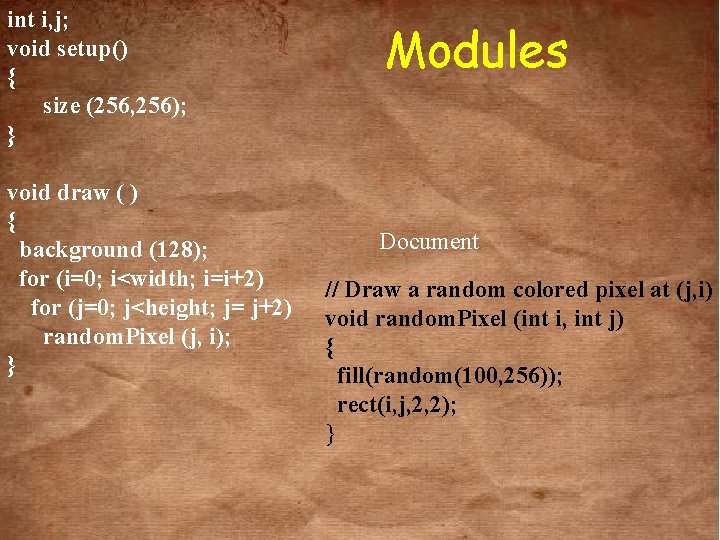
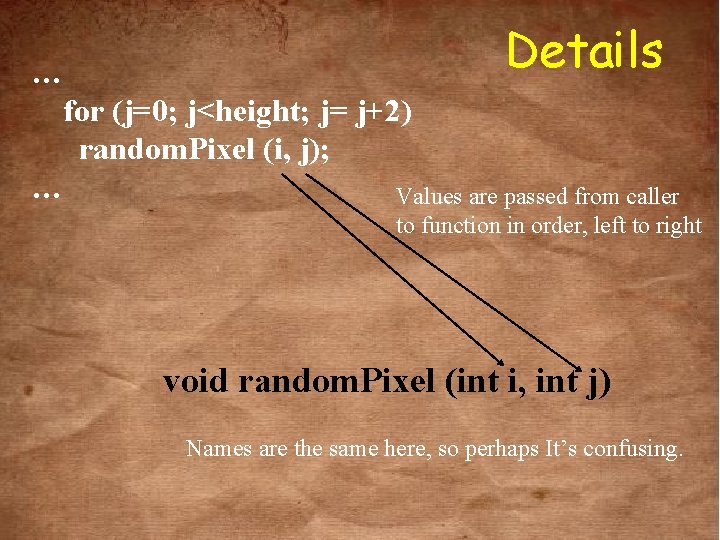
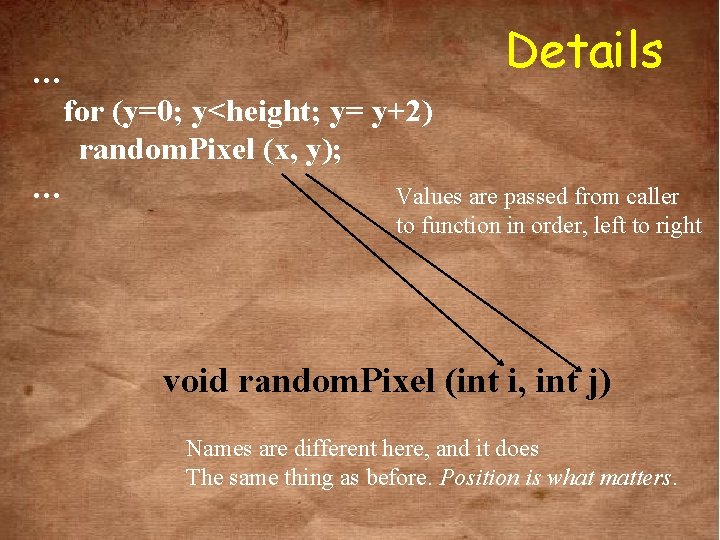
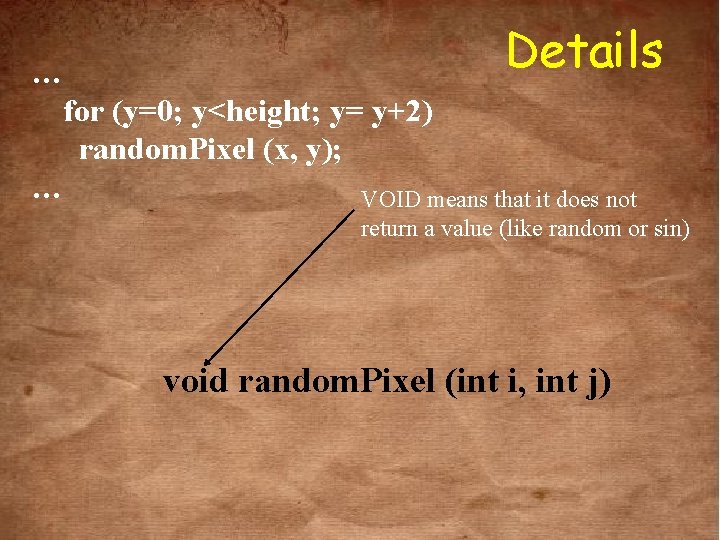
- Slides: 31
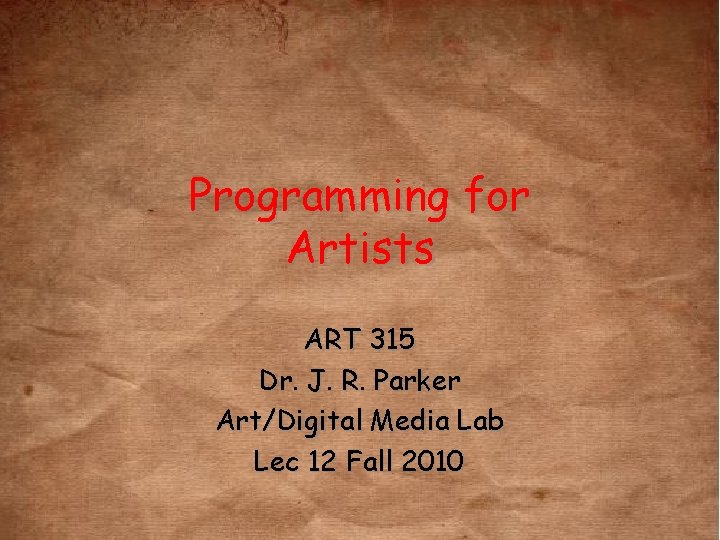
Programming for Artists ART 315 Dr. J. R. Parker Art/Digital Media Lab Lec 12 Fall 2010
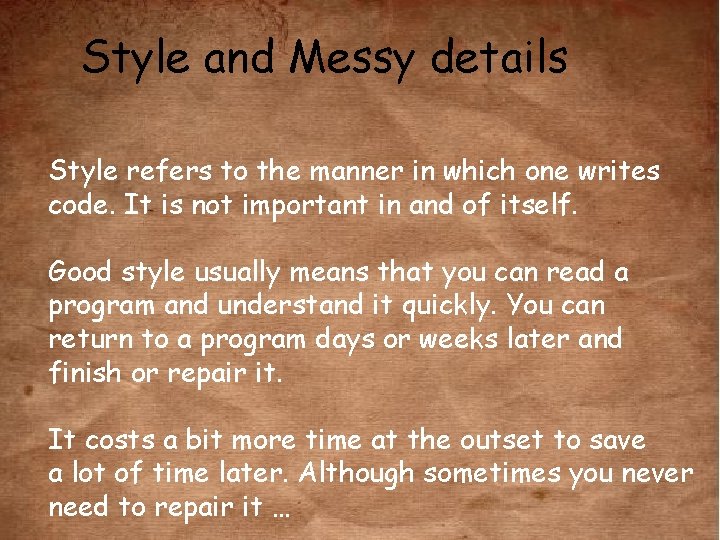
Style and Messy details Style refers to the manner in which one writes code. It is not important in and of itself. Good style usually means that you can read a program and understand it quickly. You can return to a program days or weeks later and finish or repair it. It costs a bit more time at the outset to save a lot of time later. Although sometimes you never need to repair it …
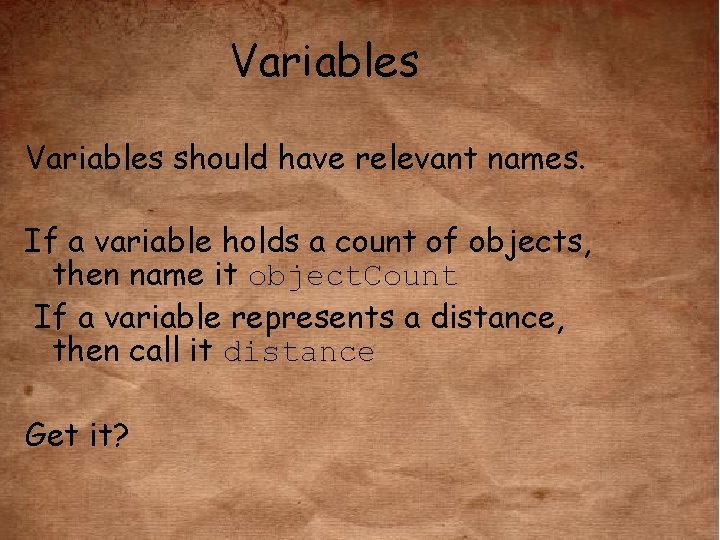
Variables should have relevant names. If a variable holds a count of objects, then name it object. Count If a variable represents a distance, then call it distance Get it?
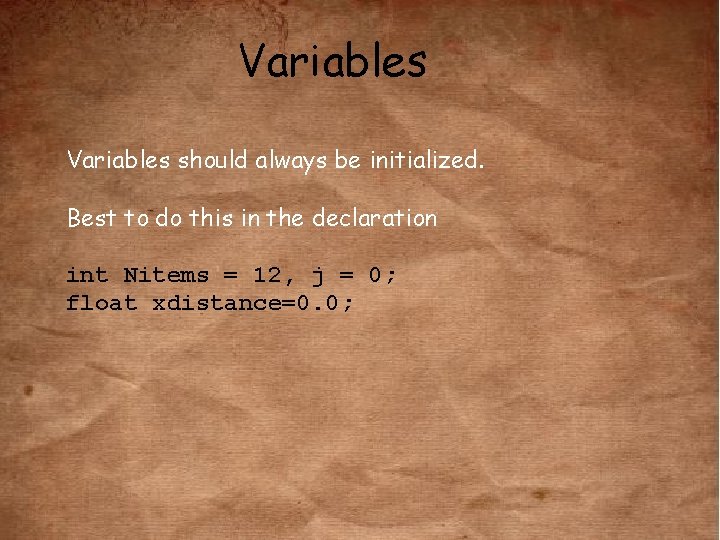
Variables should always be initialized. Best to do this in the declaration int Nitems = 12, j = 0; float xdistance=0. 0;
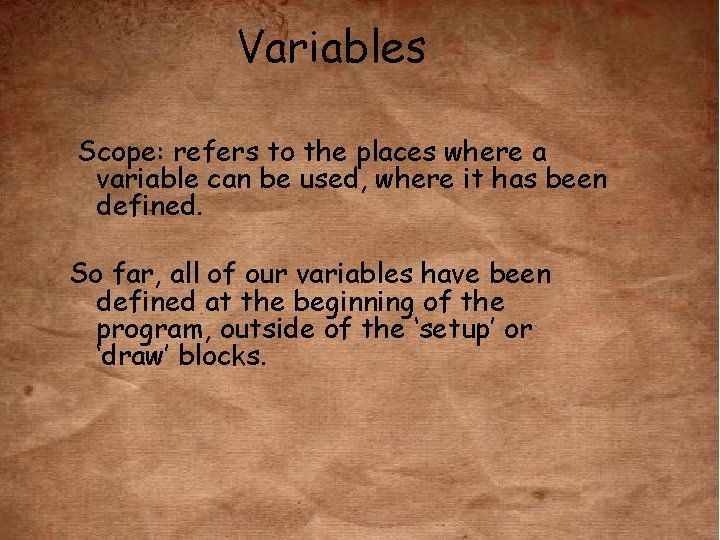
Variables Scope: refers to the places where a variable can be used, where it has been defined. So far, all of our variables have been defined at the beginning of the program, outside of the ‘setup’ or ‘draw’ blocks.
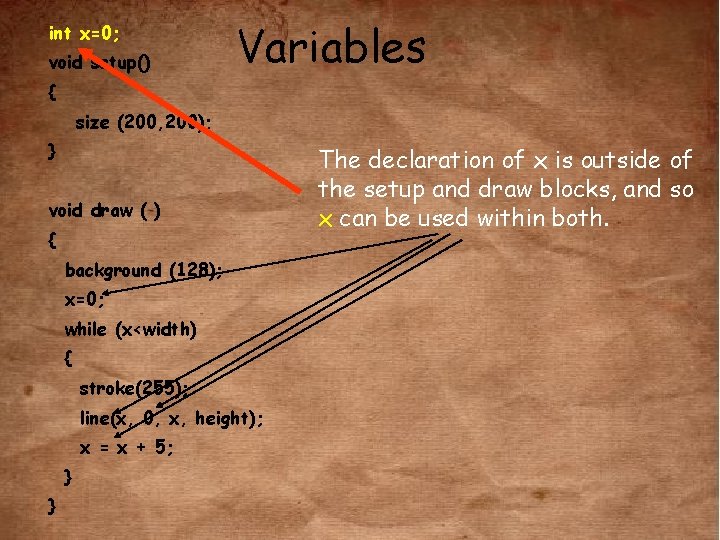
int x=0; void setup() Variables { size (200, 200); } void draw ( ) { background (128); x=0; while (x<width) { stroke(255); line(x, 0, x, height); x = x + 5; } } The declaration of x is outside of the setup and draw blocks, and so x can be used within both.
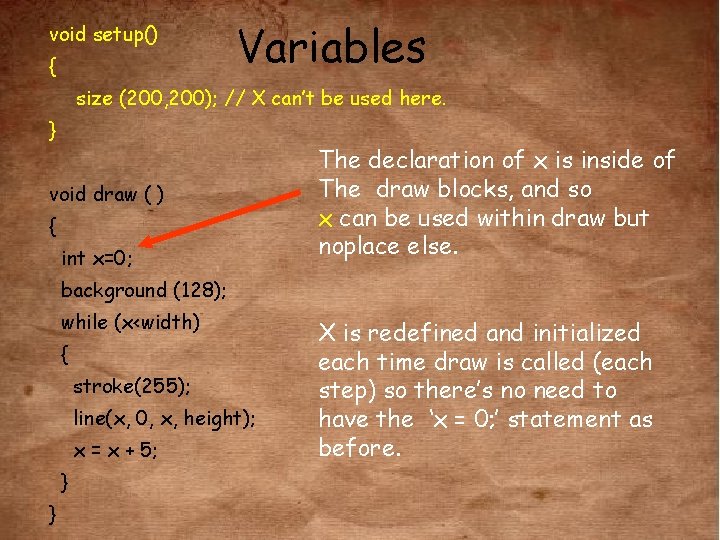
void setup() { Variables size (200, 200); // X can’t be used here. } void draw ( ) { int x=0; The declaration of x is inside of The draw blocks, and so x can be used within draw but noplace else. background (128); while (x<width) { stroke(255); line(x, 0, x, height); x = x + 5; } } X is redefined and initialized each time draw is called (each step) so there’s no need to have the ‘x = 0; ’ statement as before.
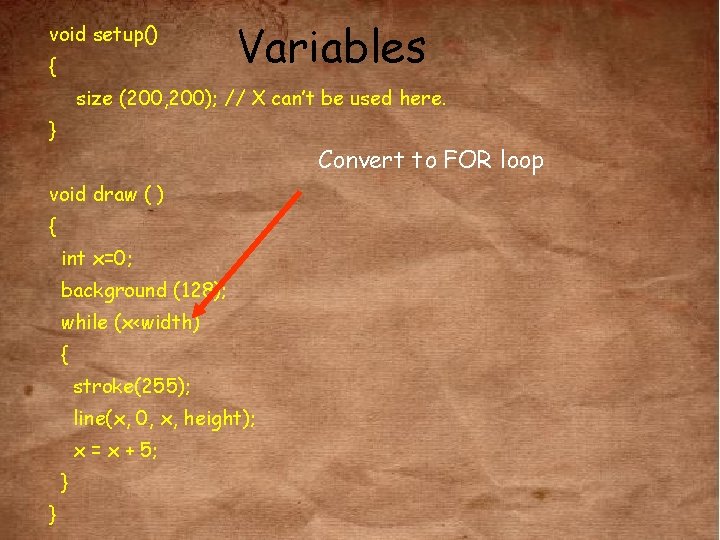
void setup() { Variables size (200, 200); // X can’t be used here. } Convert to FOR loop void draw ( ) { int x=0; background (128); while (x<width) { stroke(255); line(x, 0, x, height); x = x + 5; } }
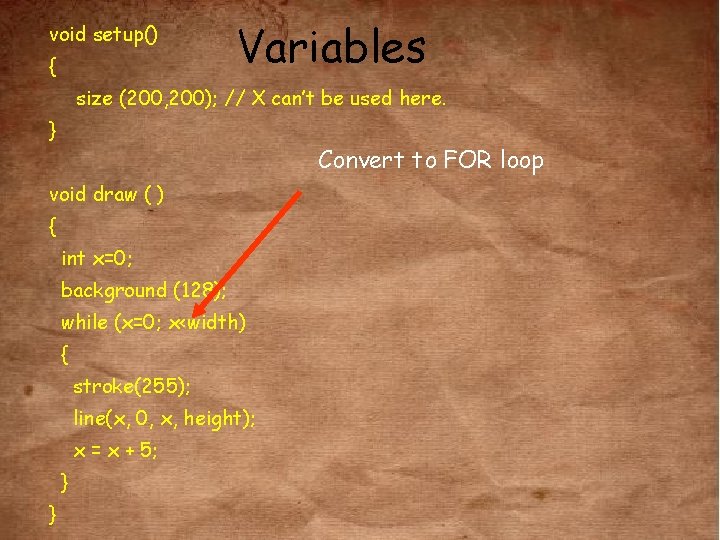
void setup() { Variables size (200, 200); // X can’t be used here. } Convert to FOR loop void draw ( ) { int x=0; background (128); while (x=0; x<width) { stroke(255); line(x, 0, x, height); x = x + 5; } }
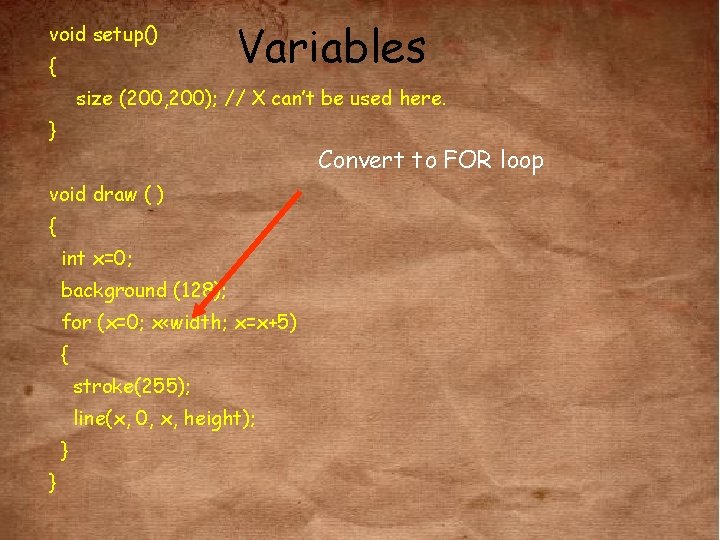
void setup() { Variables size (200, 200); // X can’t be used here. } Convert to FOR loop void draw ( ) { int x=0; background (128); for (x=0; x<width; x=x+5) { stroke(255); line(x, 0, x, height); } }
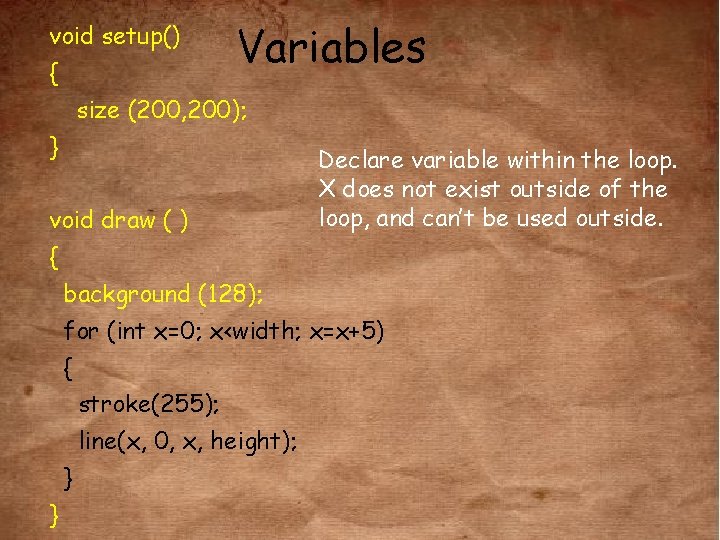
void setup() { Variables size (200, 200); } void draw ( ) Declare variable within the loop. X does not exist outside of the loop, and can’t be used outside. { background (128); for (int x=0; x<width; x=x+5) { stroke(255); line(x, 0, x, height); } }
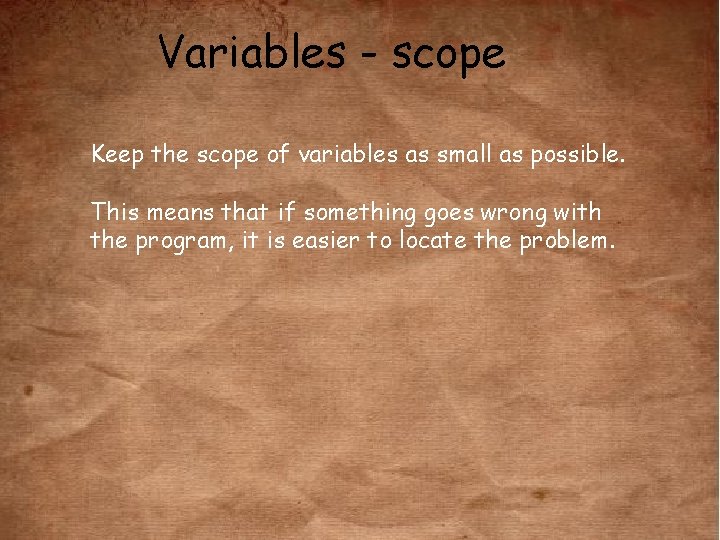
Variables - scope Keep the scope of variables as small as possible. This means that if something goes wrong with the program, it is easier to locate the problem.
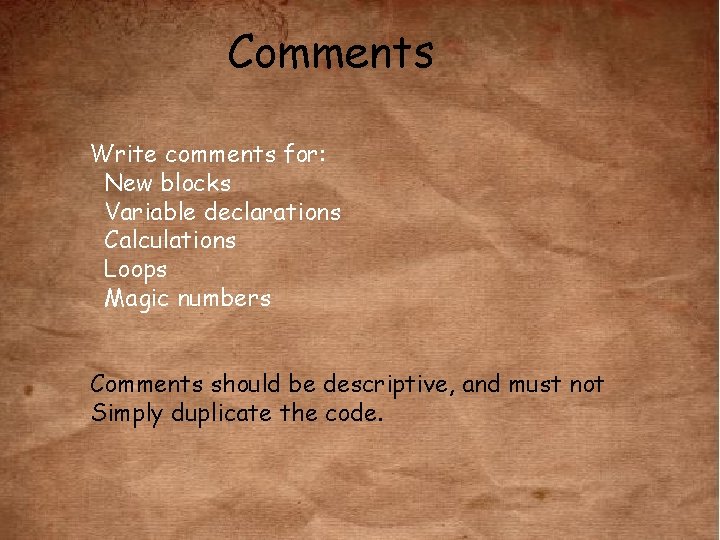
Comments Write comments for: New blocks Variable declarations Calculations Loops Magic numbers Comments should be descriptive, and must not Simply duplicate the code.
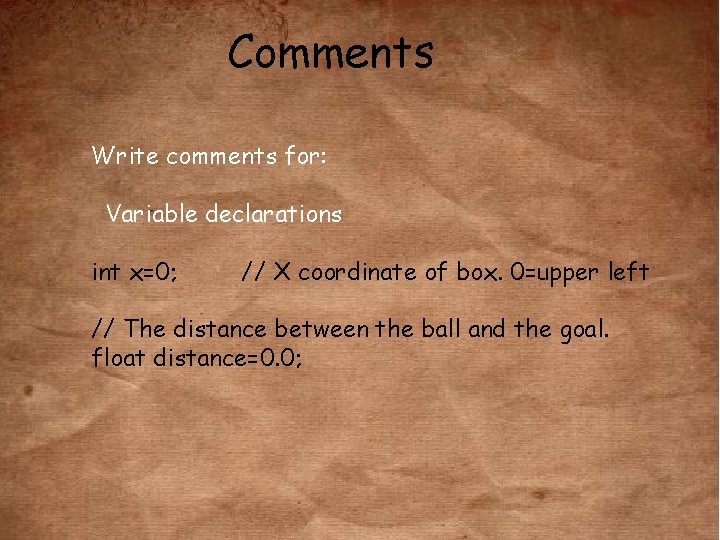
Comments Write comments for: Variable declarations int x=0; // X coordinate of box. 0=upper left // The distance between the ball and the goal. float distance=0. 0;
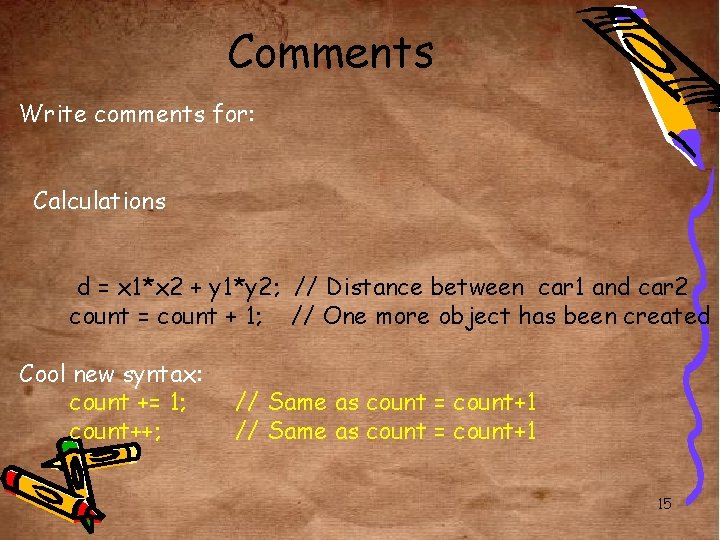
Comments Write comments for: Calculations d = x 1*x 2 + y 1*y 2; // Distance between car 1 and car 2 count = count + 1; // One more object has been created Cool new syntax: count += 1; count++; // Same as count = count+1 15
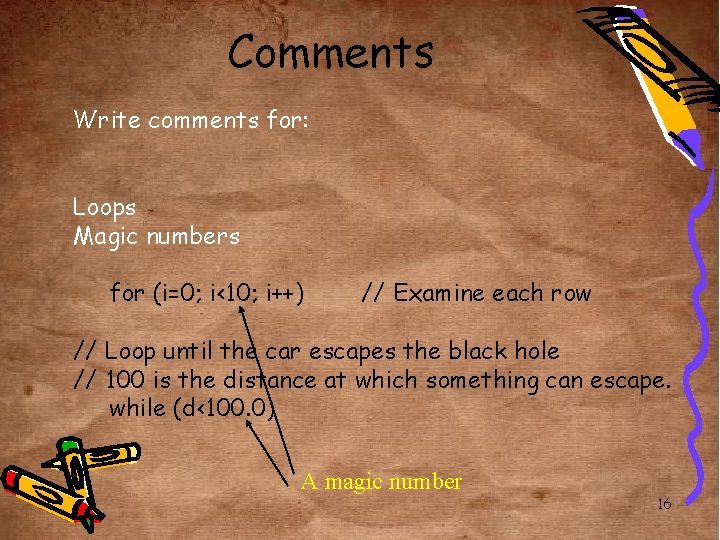
Comments Write comments for: Loops Magic numbers for (i=0; i<10; i++) // Examine each row // Loop until the car escapes the black hole // 100 is the distance at which something can escape. while (d<100. 0) A magic number 16
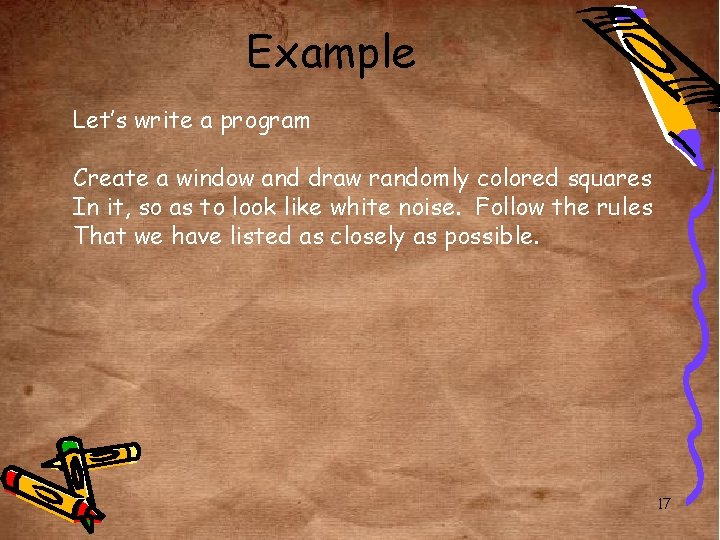
Example Let’s write a program Create a window and draw randomly colored squares In it, so as to look like white noise. Follow the rules That we have listed as closely as possible. 17
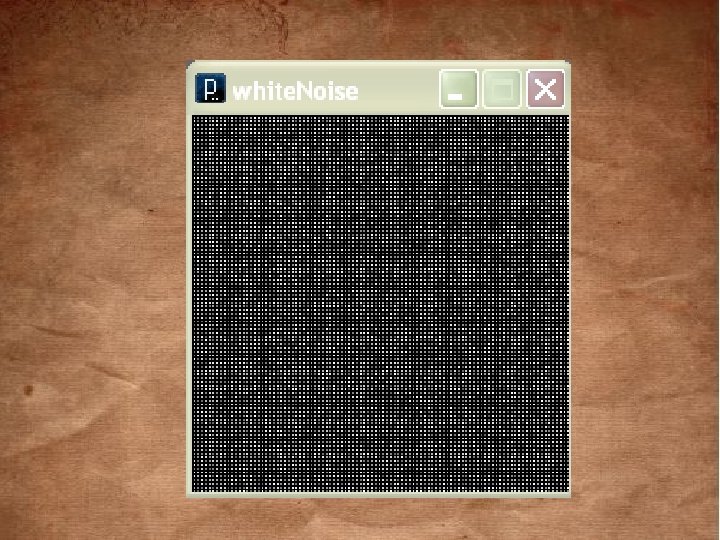
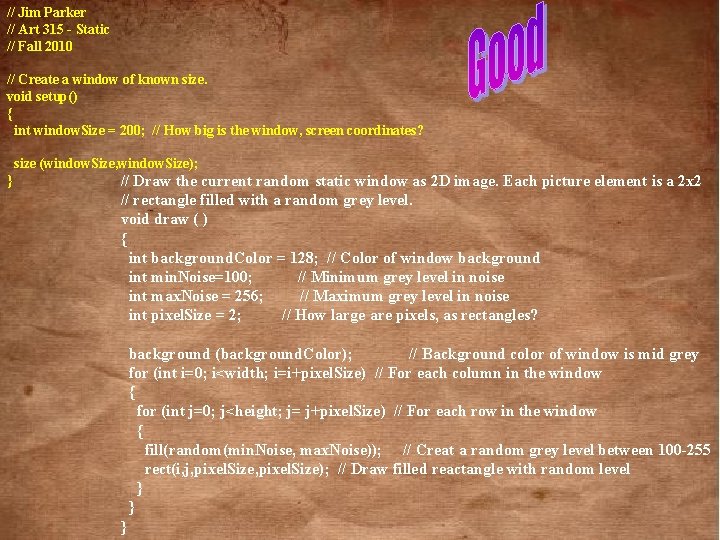
// Jim Parker // Art 315 - Static // Fall 2010 // Create a window of known size. void setup() { int window. Size = 200; // How big is the window, screen coordinates? size (window. Size, window. Size); } // Draw the current random static window as 2 D image. Each picture element is a 2 x 2 // rectangle filled with a random grey level. void draw ( ) { int background. Color = 128; // Color of window background int min. Noise=100; // Minimum grey level in noise int max. Noise = 256; // Maximum grey level in noise int pixel. Size = 2; // How large are pixels, as rectangles? background (background. Color); // Background color of window is mid grey for (int i=0; i<width; i=i+pixel. Size) // For each column in the window { for (int j=0; j<height; j= j+pixel. Size) // For each row in the window { fill(random(min. Noise, max. Noise)); // Creat a random grey level between 100 -255 rect(i, j, pixel. Size); // Draw filled reactangle with random level } } }
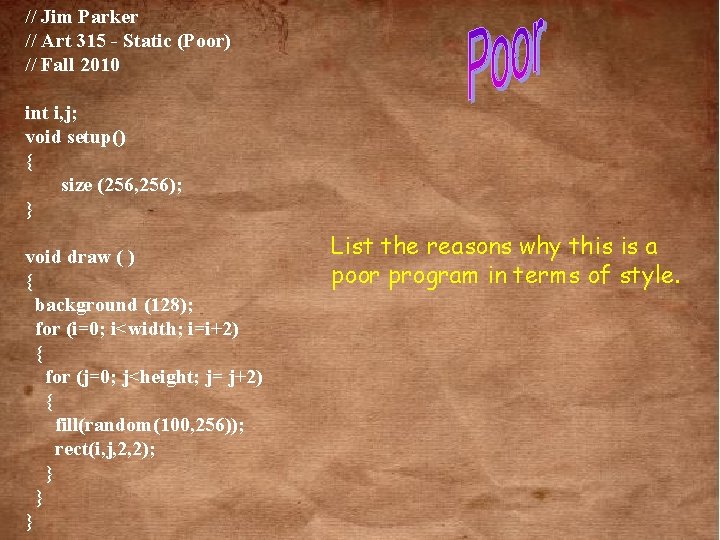
// Jim Parker // Art 315 - Static (Poor) // Fall 2010 int i, j; void setup() { size (256, 256); } void draw ( ) { background (128); for (i=0; i<width; i=i+2) { for (j=0; j<height; j= j+2) { fill(random(100, 256)); rect(i, j, 2, 2); } } } List the reasons why this is a poor program in terms of style.
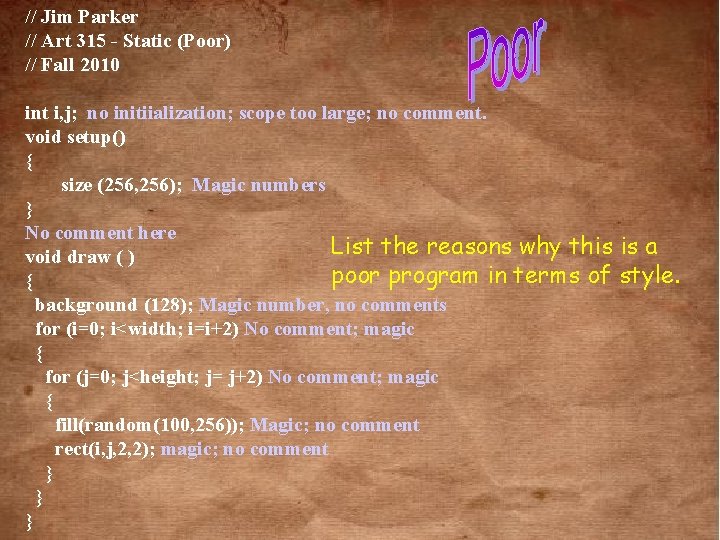
// Jim Parker // Art 315 - Static (Poor) // Fall 2010 int i, j; no initiialization; scope too large; no comment. void setup() { size (256, 256); Magic numbers } No comment here List the reasons why this void draw ( ) poor program in terms of { background (128); Magic number, no comments for (i=0; i<width; i=i+2) No comment; magic { for (j=0; j<height; j= j+2) No comment; magic { fill(random(100, 256)); Magic; no comment rect(i, j, 2, 2); magic; no comment } } } is a style.
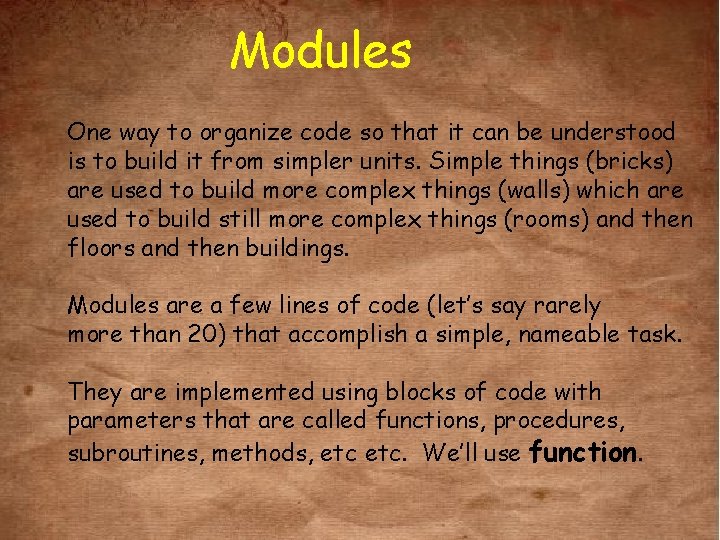
Modules One way to organize code so that it can be understood is to build it from simpler units. Simple things (bricks) are used to build more complex things (walls) which are used to build still more complex things (rooms) and then floors and then buildings. Modules are a few lines of code (let’s say rarely more than 20) that accomplish a simple, nameable task. They are implemented using blocks of code with parameters that are called functions, procedures, subroutines, methods, etc. We’ll use function.
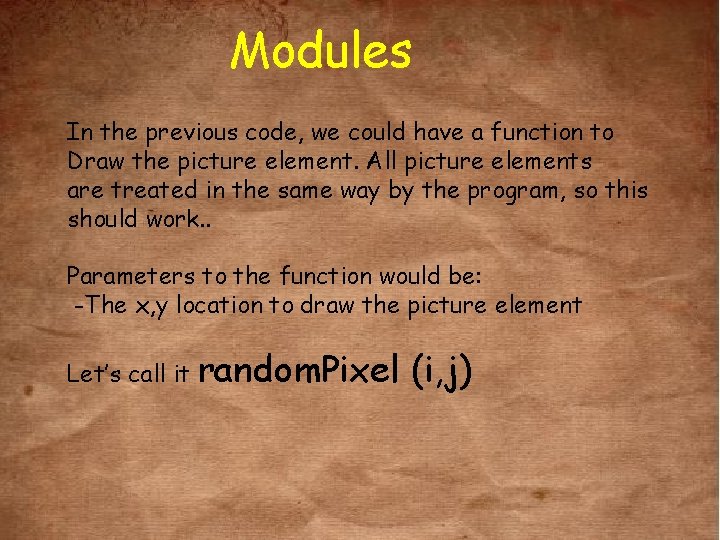
Modules In the previous code, we could have a function to Draw the picture element. All picture elements are treated in the same way by the program, so this should work. . Parameters to the function would be: -The x, y location to draw the picture element Let’s call it random. Pixel (i, j)
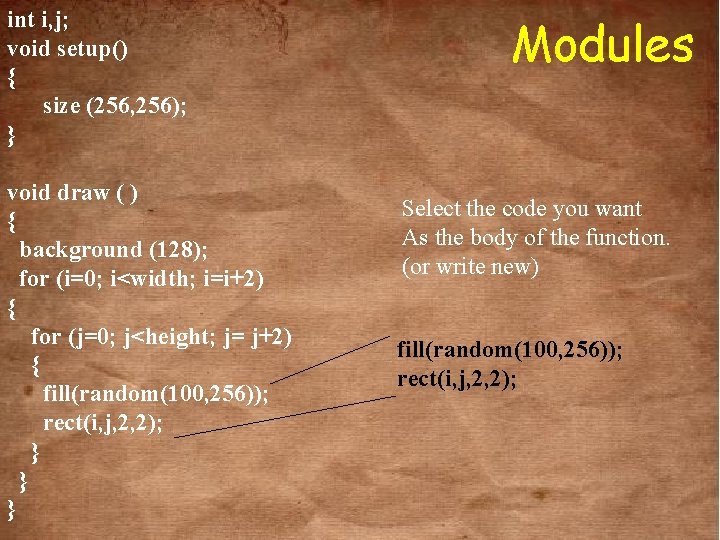
int i, j; void setup() { size (256, 256); } void draw ( ) { background (128); for (i=0; i<width; i=i+2) { for (j=0; j<height; j= j+2) { fill(random(100, 256)); rect(i, j, 2, 2); } } } Modules Select the code you want As the body of the function. (or write new) fill(random(100, 256)); rect(i, j, 2, 2);
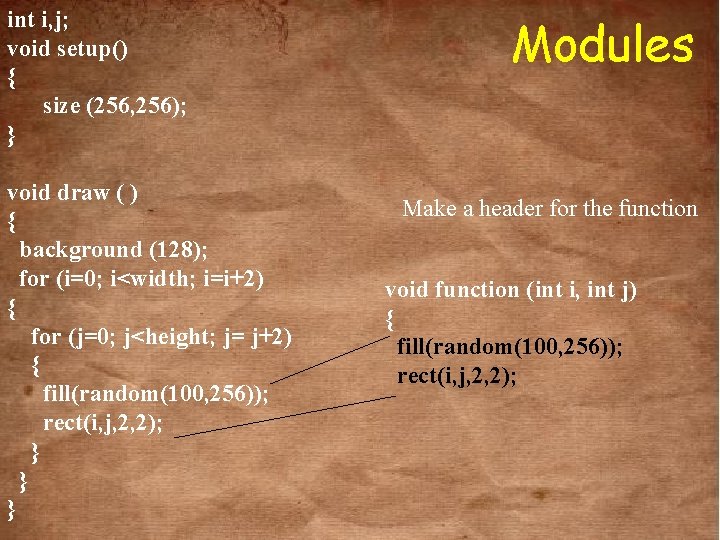
int i, j; void setup() { size (256, 256); } void draw ( ) { background (128); for (i=0; i<width; i=i+2) { for (j=0; j<height; j= j+2) { fill(random(100, 256)); rect(i, j, 2, 2); } } } Modules Make a header for the function void function (int i, int j) { fill(random(100, 256)); rect(i, j, 2, 2);
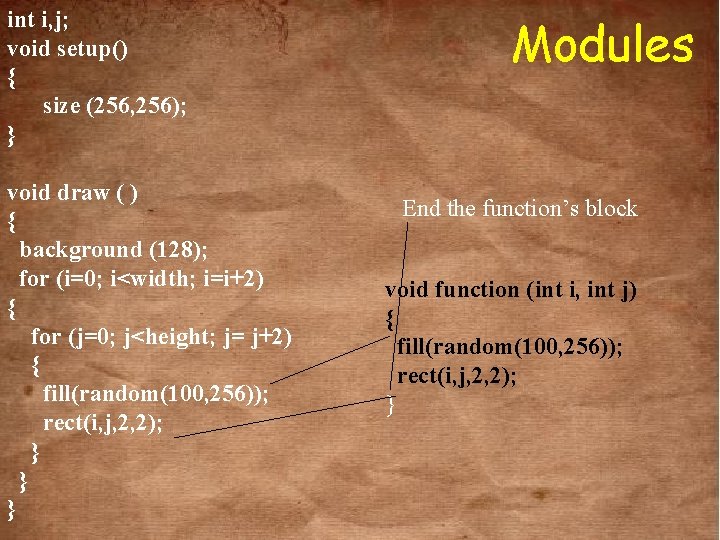
int i, j; void setup() { size (256, 256); } void draw ( ) { background (128); for (i=0; i<width; i=i+2) { for (j=0; j<height; j= j+2) { fill(random(100, 256)); rect(i, j, 2, 2); } } } Modules End the function’s block void function (int i, int j) { fill(random(100, 256)); rect(i, j, 2, 2); }
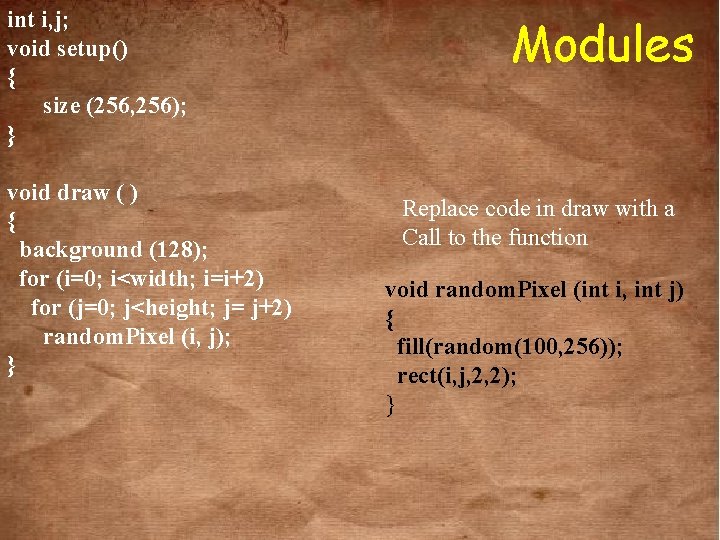
int i, j; void setup() { size (256, 256); } void draw ( ) { background (128); for (i=0; i<width; i=i+2) for (j=0; j<height; j= j+2) random. Pixel (i, j); } Modules Replace code in draw with a Call to the function void random. Pixel (int i, int j) { fill(random(100, 256)); rect(i, j, 2, 2); }
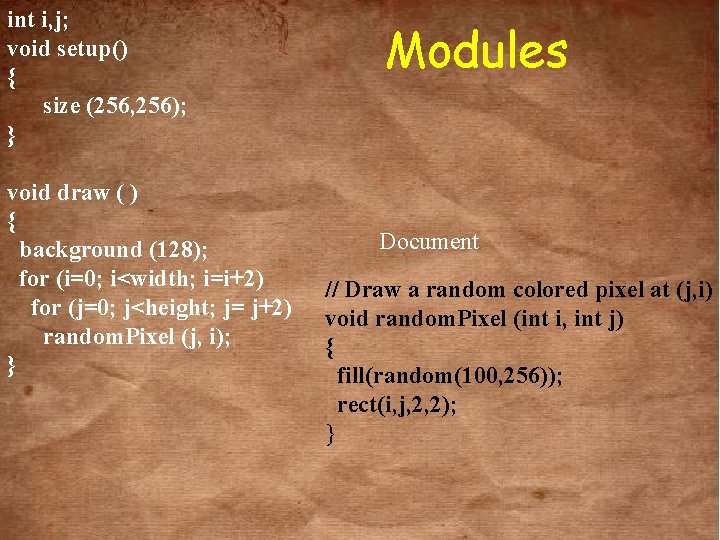
int i, j; void setup() { size (256, 256); } void draw ( ) { background (128); for (i=0; i<width; i=i+2) for (j=0; j<height; j= j+2) random. Pixel (j, i); } Modules Document // Draw a random colored pixel at (j, i) void random. Pixel (int i, int j) { fill(random(100, 256)); rect(i, j, 2, 2); }
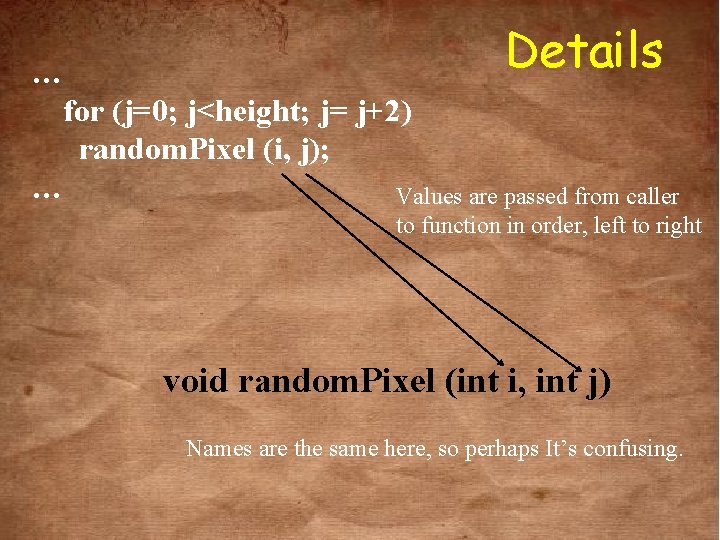
Details … for (j=0; j<height; j= j+2) random. Pixel (i, j); … Values are passed from caller to function in order, left to right void random. Pixel (int i, int j) Names are the same here, so perhaps It’s confusing.
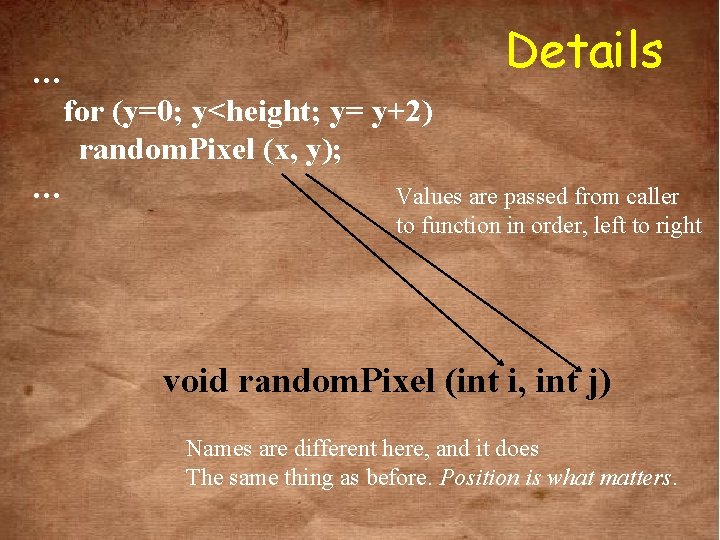
Details … for (y=0; y<height; y= y+2) random. Pixel (x, y); … Values are passed from caller to function in order, left to right void random. Pixel (int i, int j) Names are different here, and it does The same thing as before. Position is what matters.
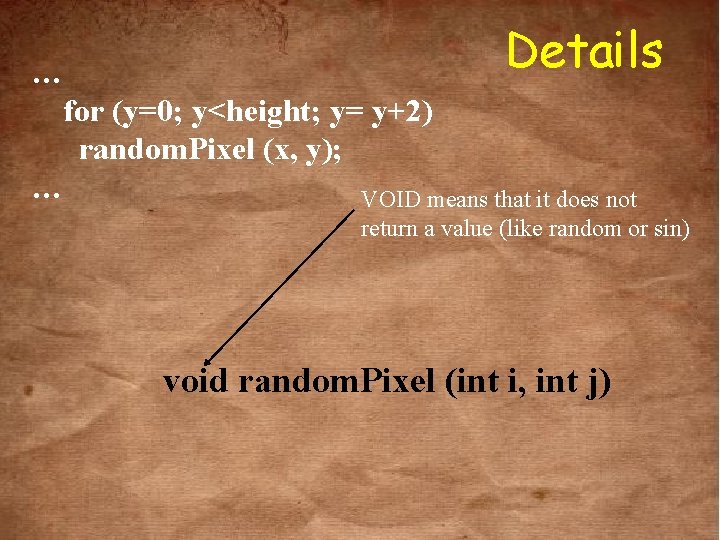
Details … for (y=0; y<height; y= y+2) random. Pixel (x, y); … VOID means that it does not return a value (like random or sin) void random. Pixel (int i, int j)
Principles of design radial balance
Physical function art
Op
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
What is in system programming
Linear vs integer programming
Definisi integer
Isa 315 flowchart
Isa 315 revised summary
Margin of safety can be expressed as
Find the exact value sin(225)
Contoh soal relatif prima
Nep 630
How to.find reference angle
Untuk sin 1050 berada pada kuadran
315
Economic 315
Mode of operation
Ee-315
Economic 315
Ba 315
Tentukan volume bola berikut a b c d e f
315 production
Scars of society
Metaphor in stereo hearts
Frank stella
Reflection art gcse
artists use this perspective to show objects face-on.
Where did latin rock originated
Krowehom
Muslim innovations and adaptations answers