Outline Administration Dr Scheme Functional vs Imperative Programming
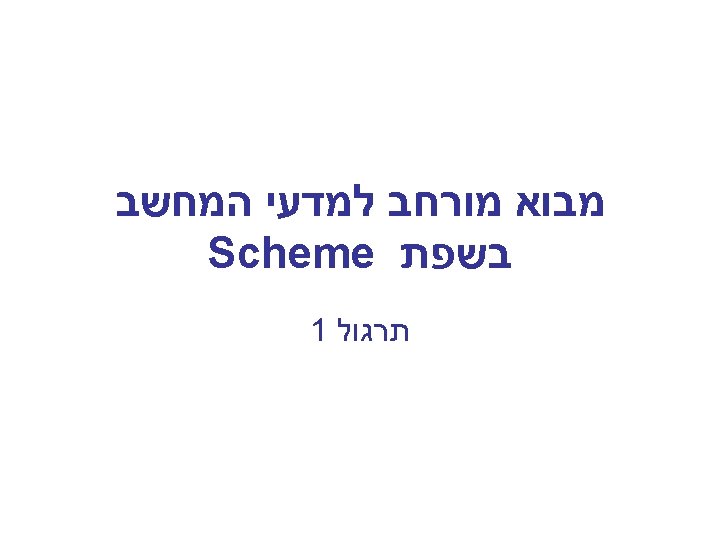
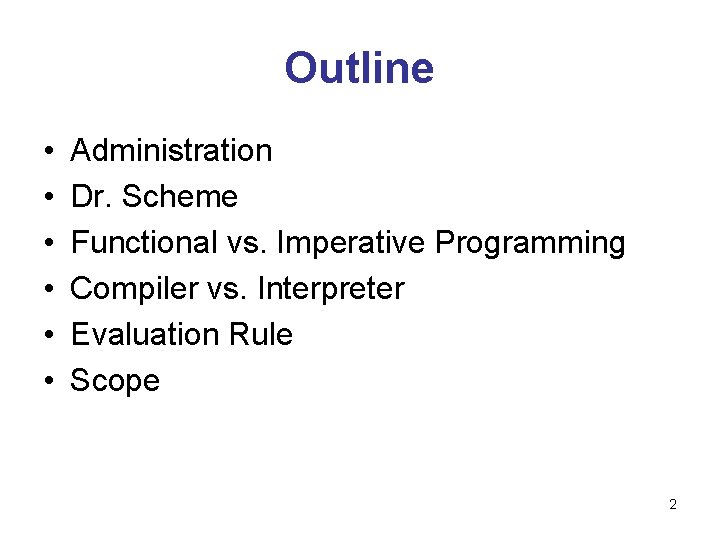
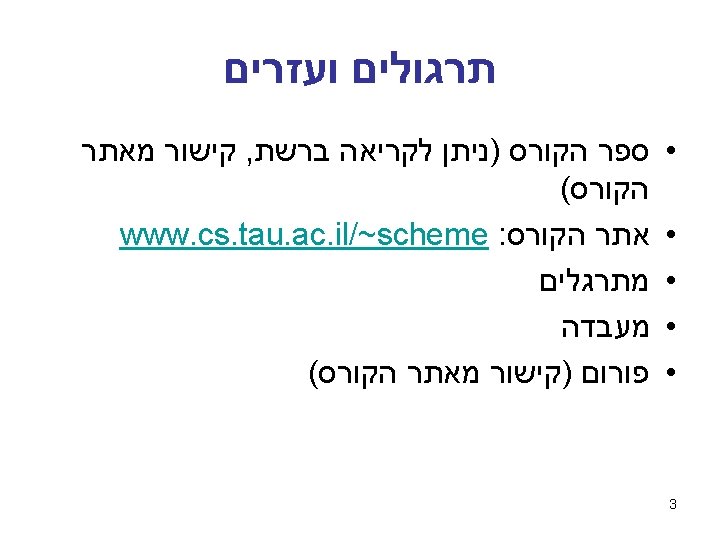
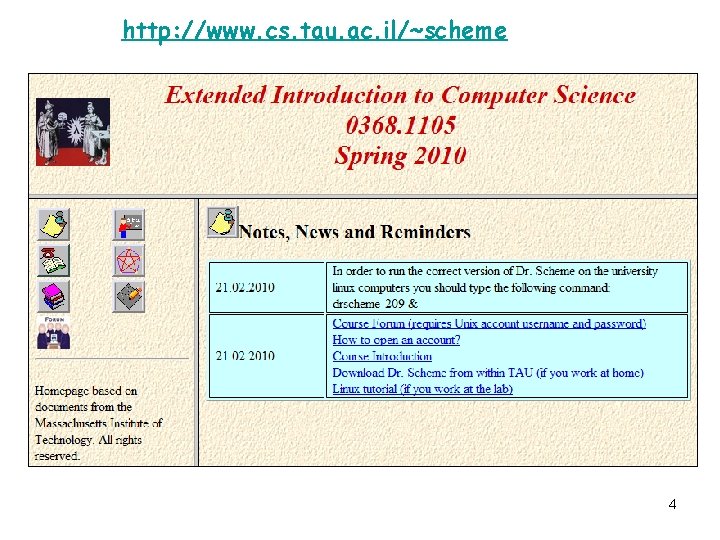
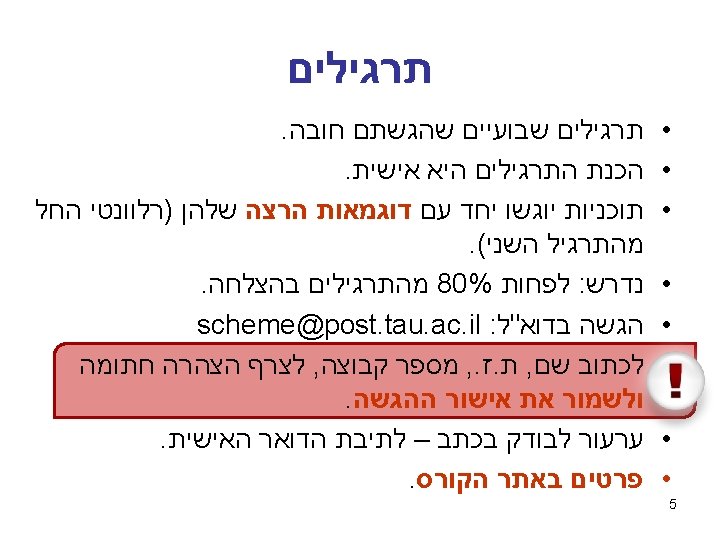
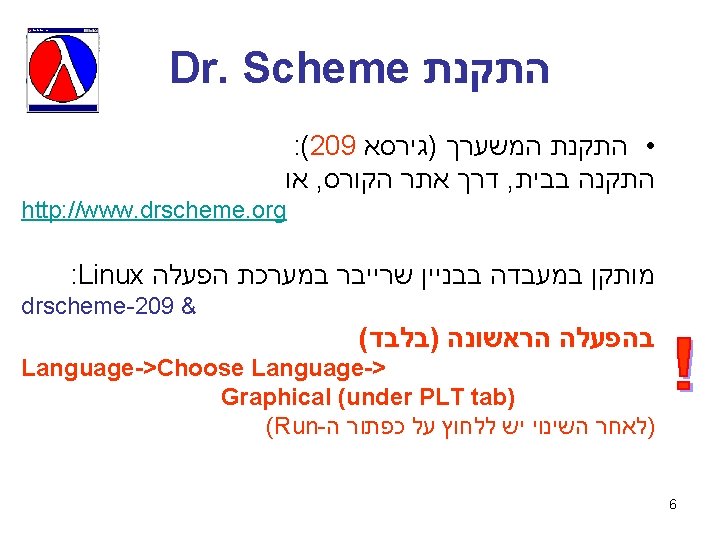
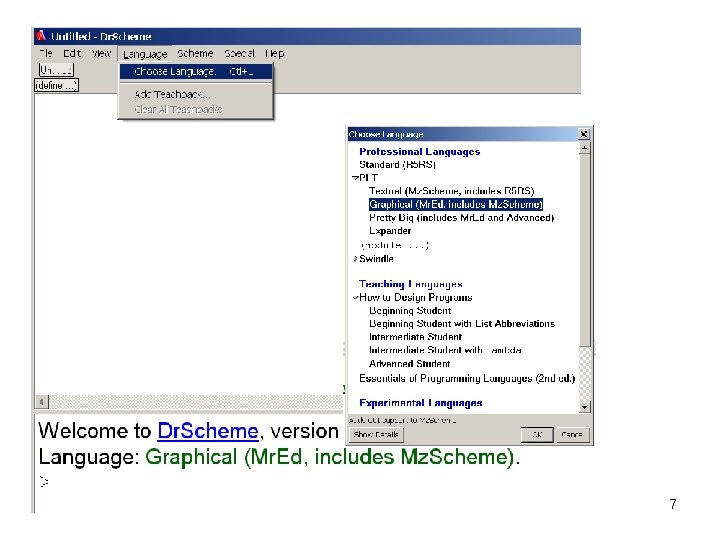
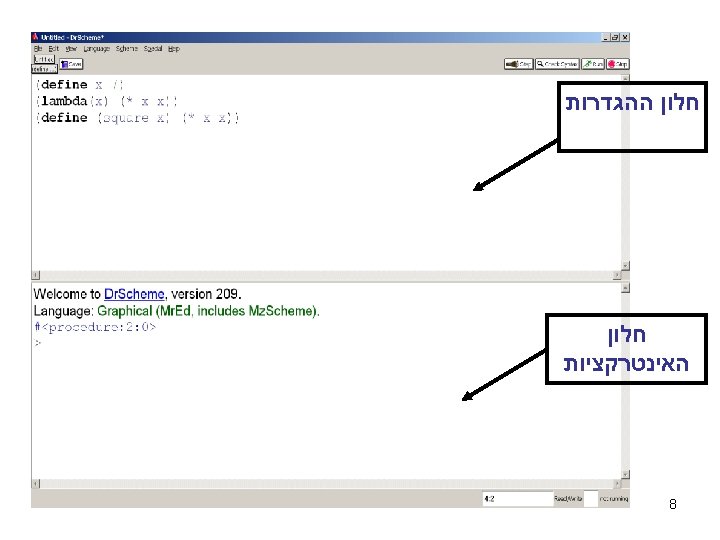
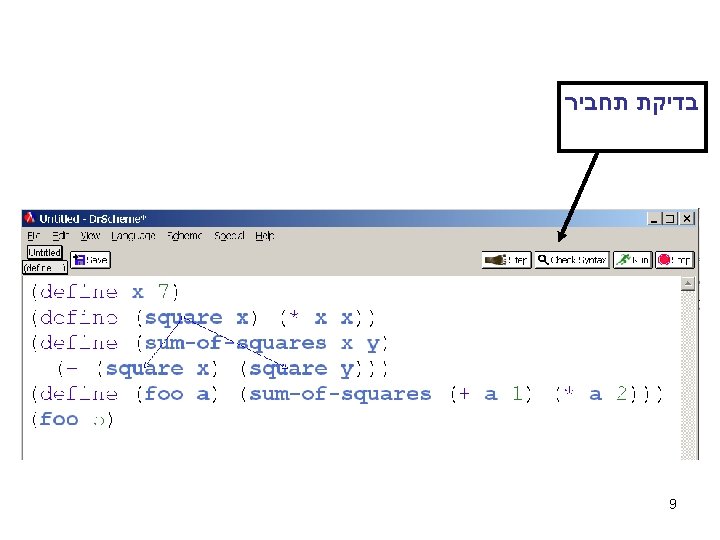
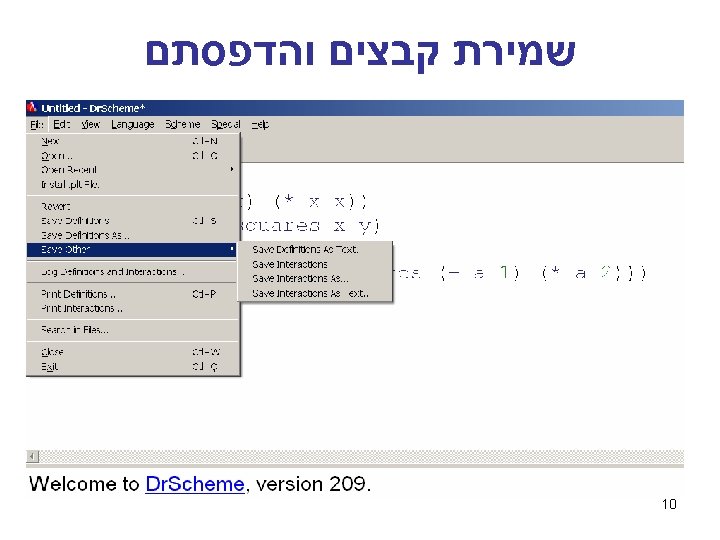
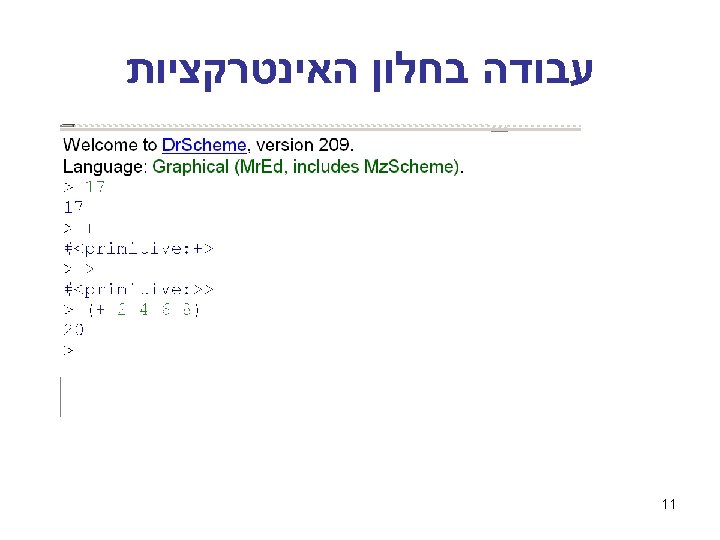
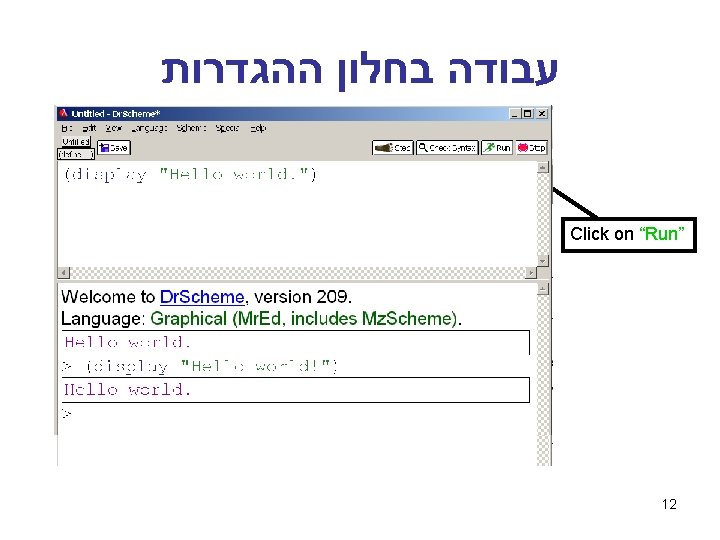
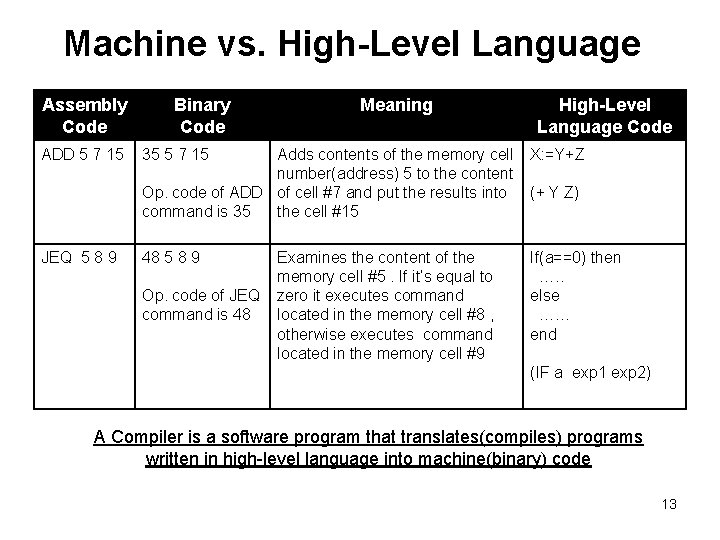
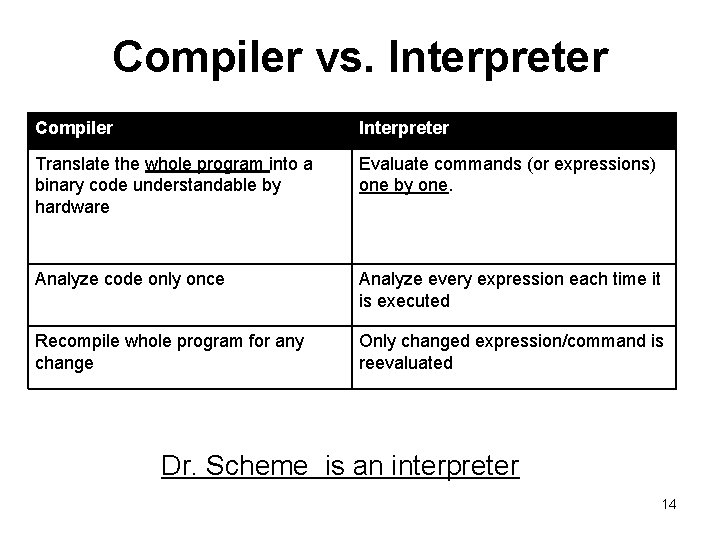
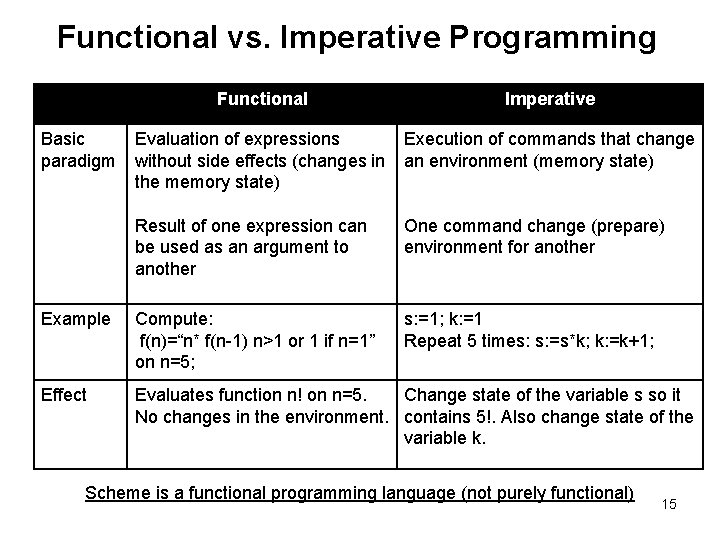
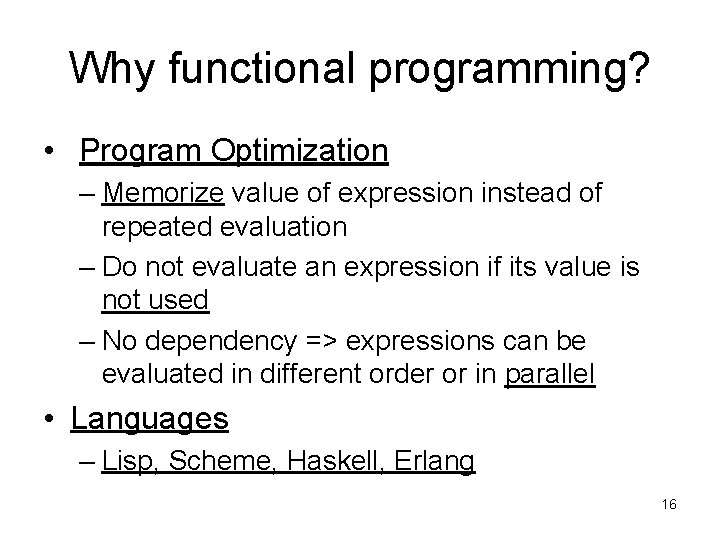
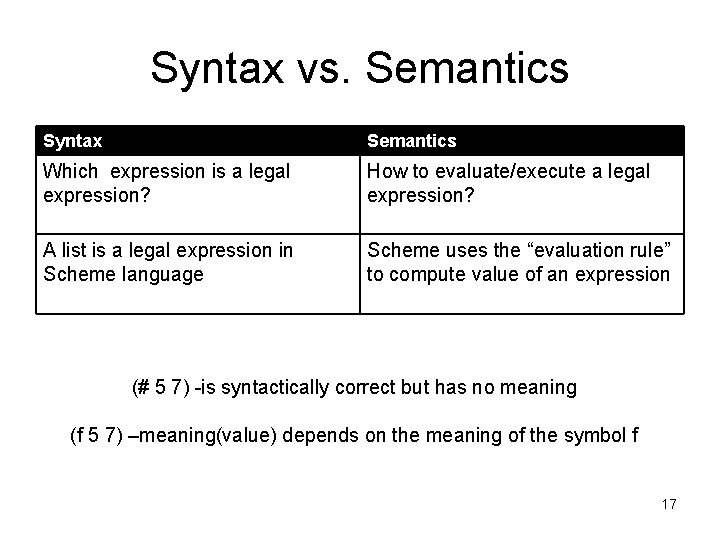
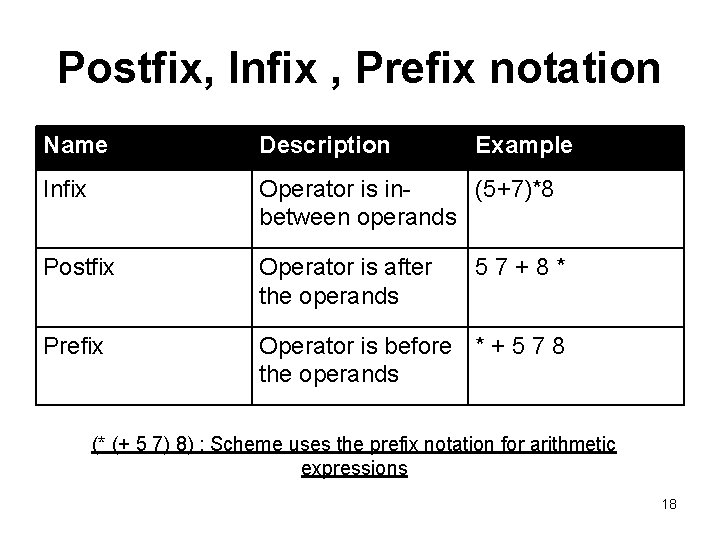
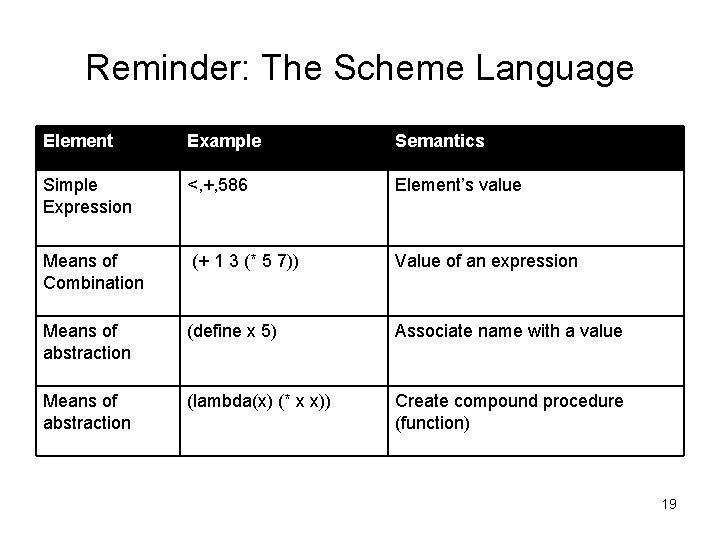
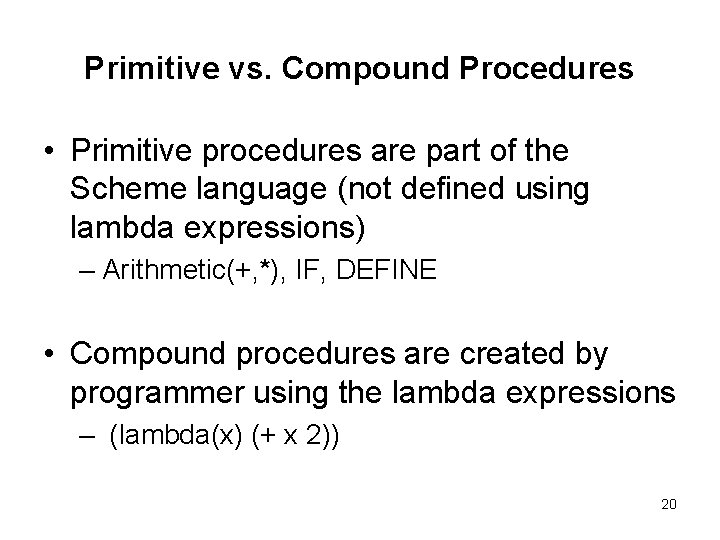
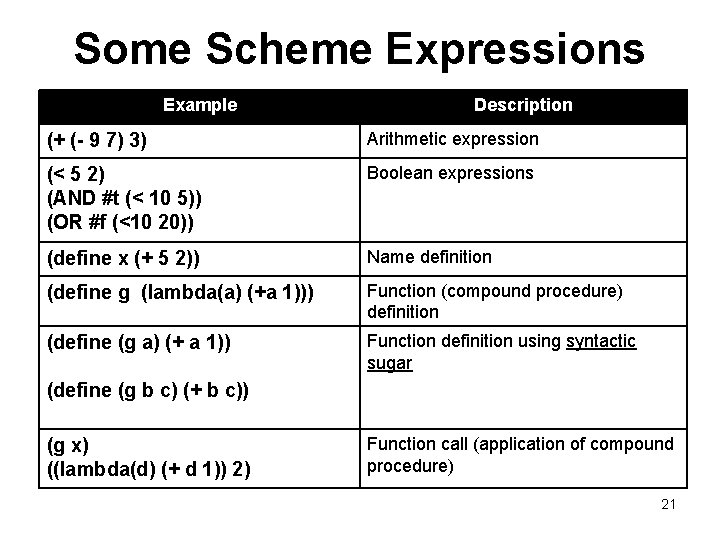
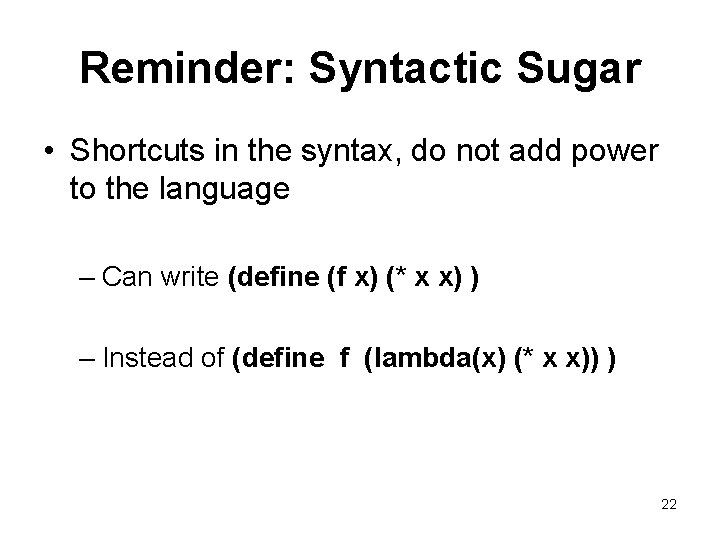
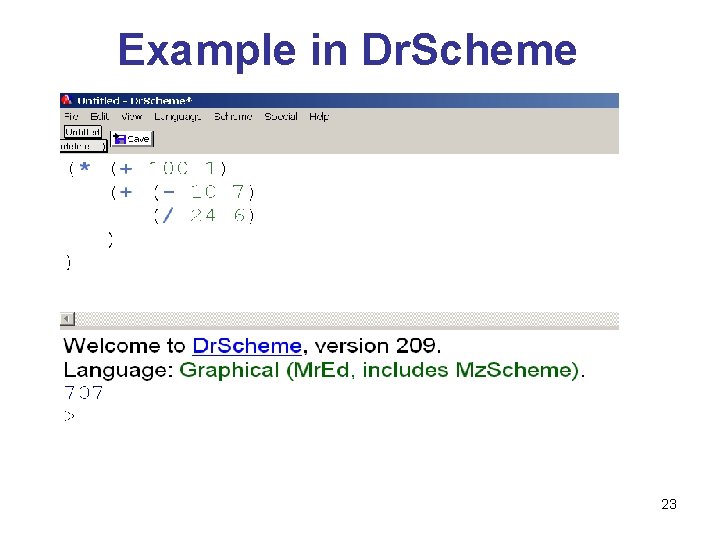
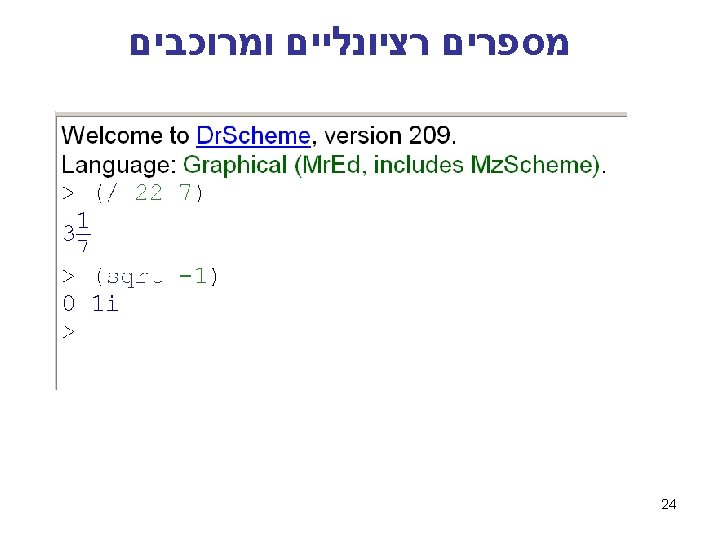
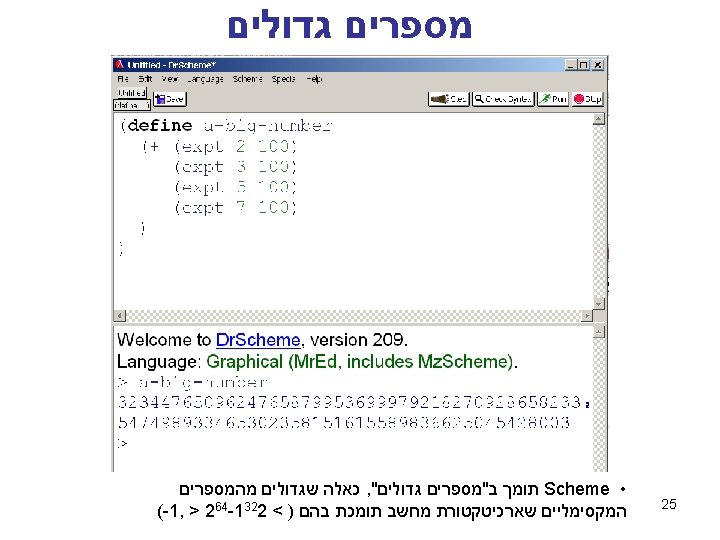
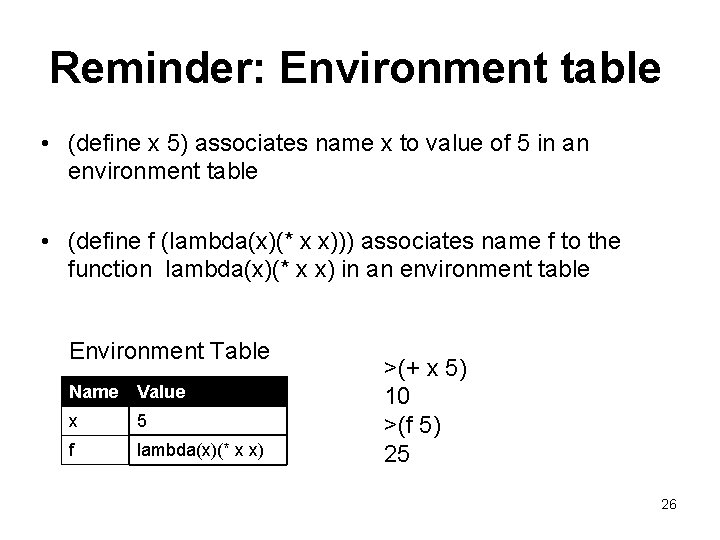
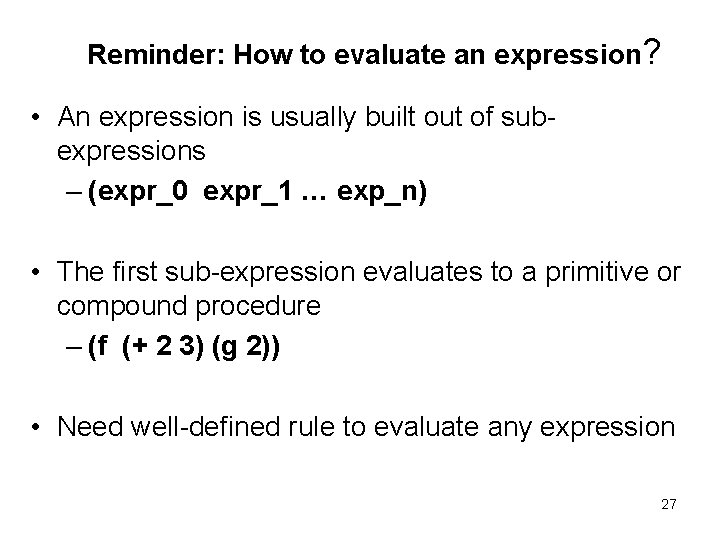
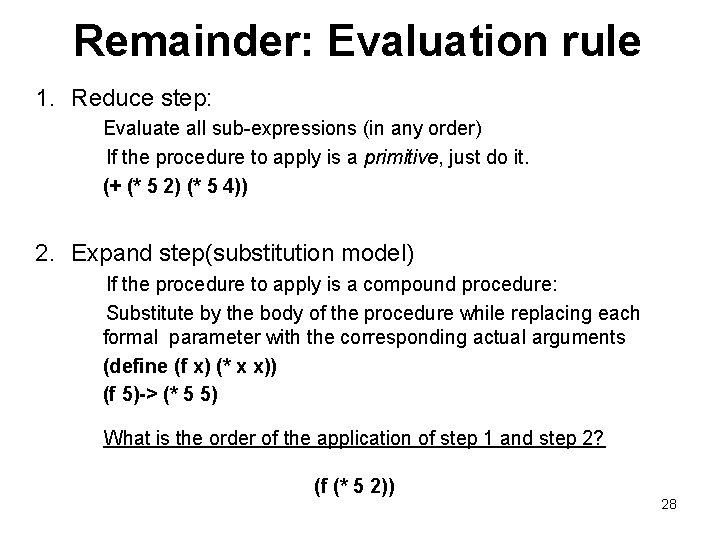
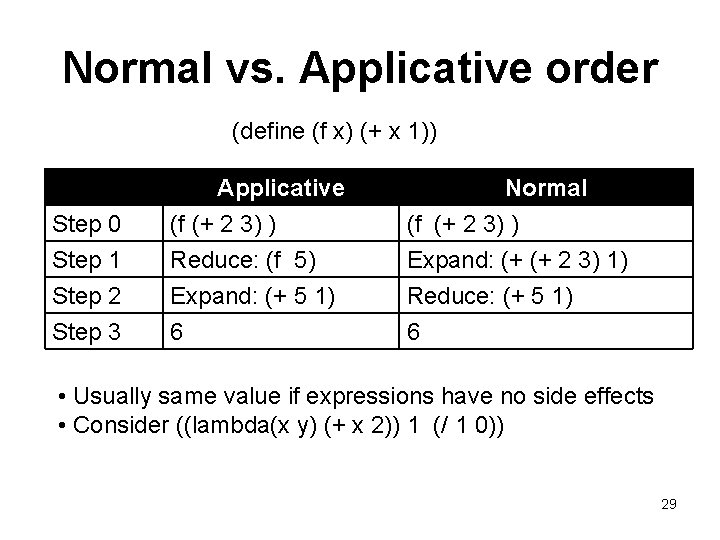
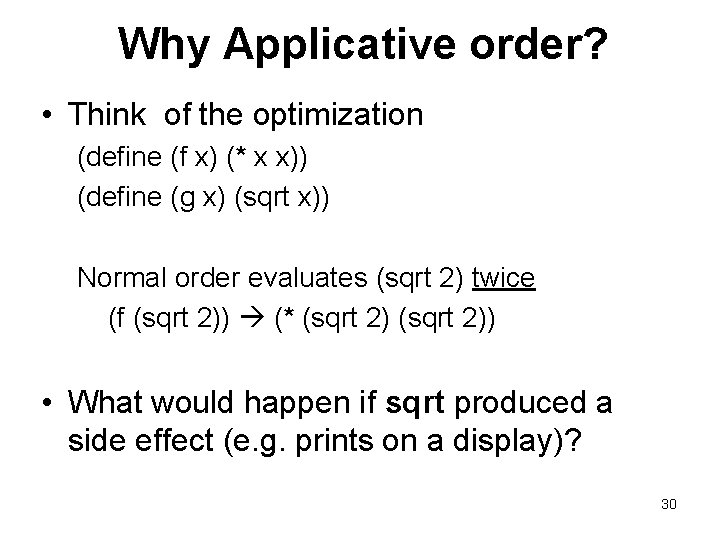
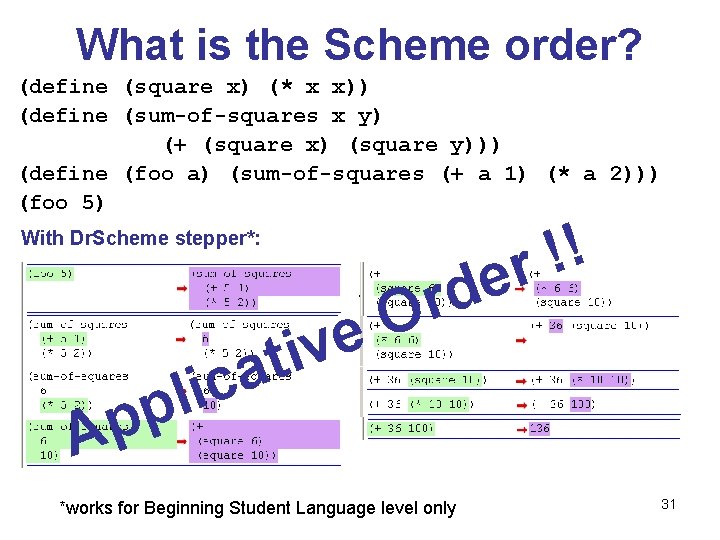
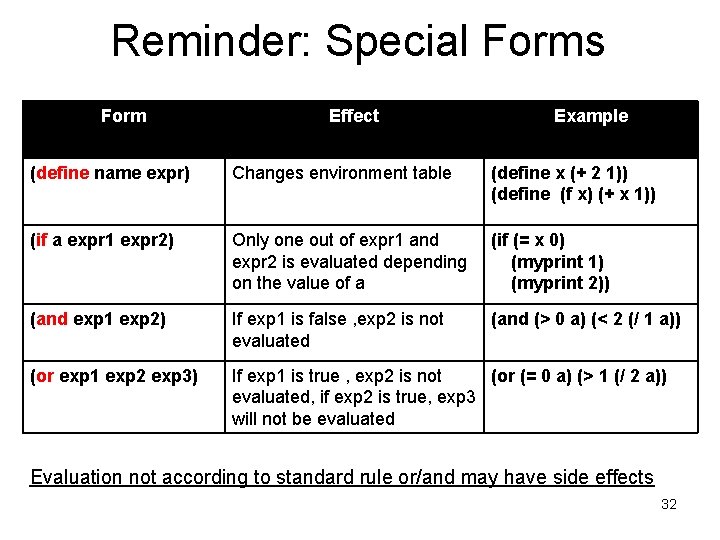
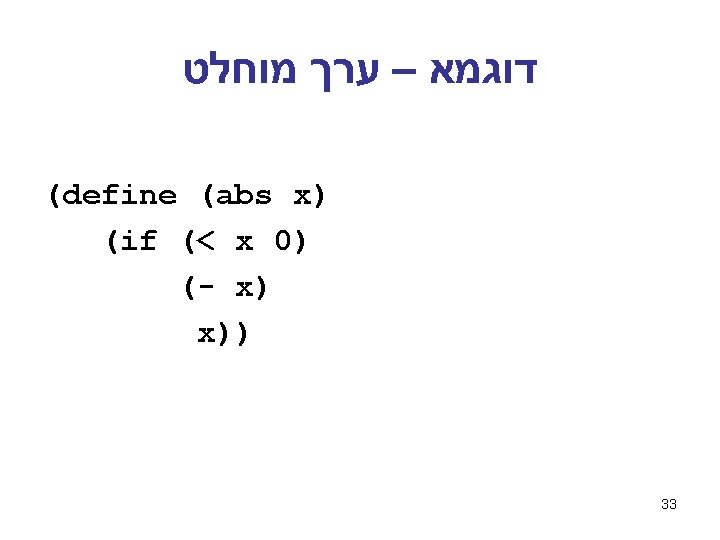
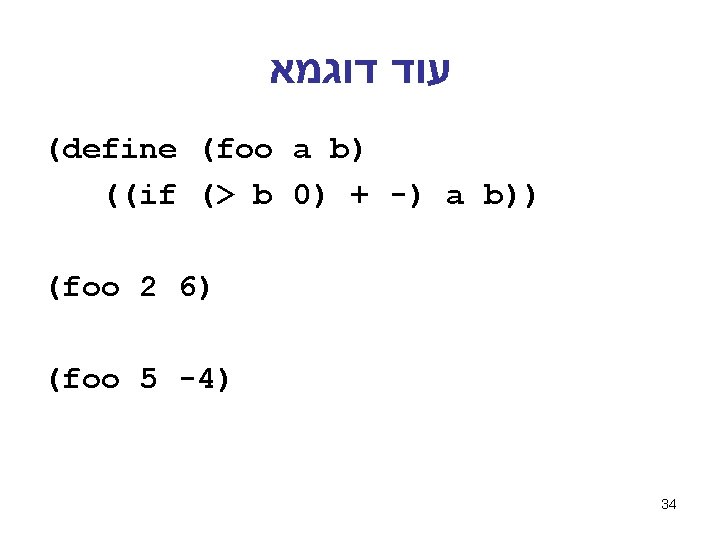
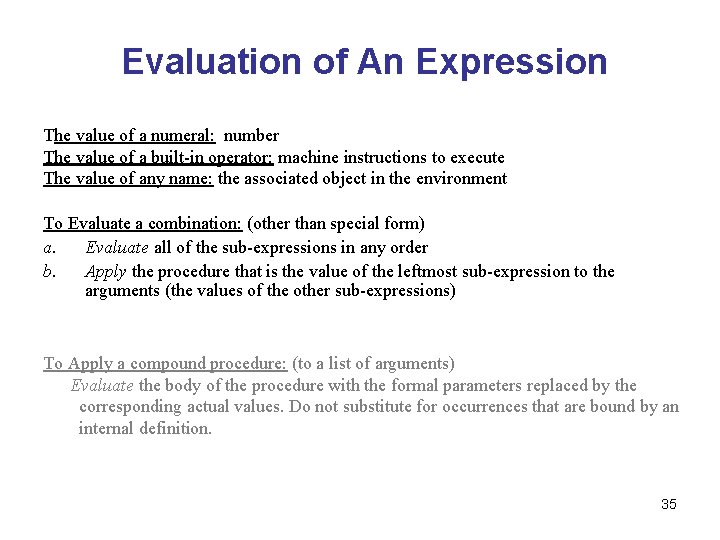
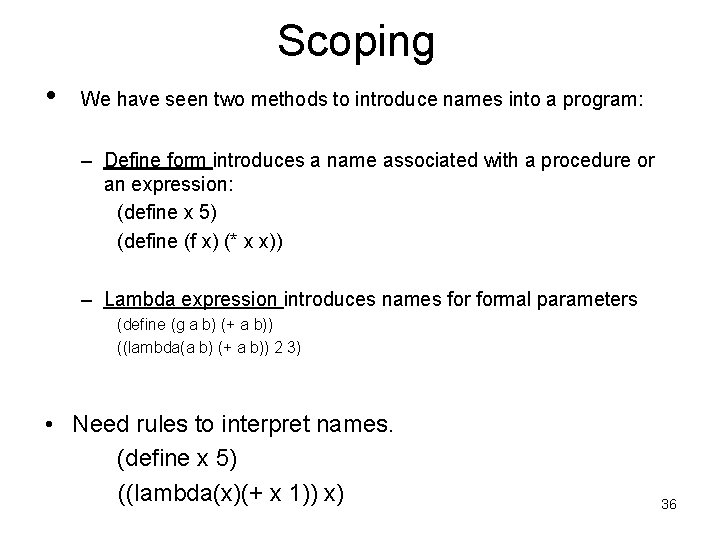
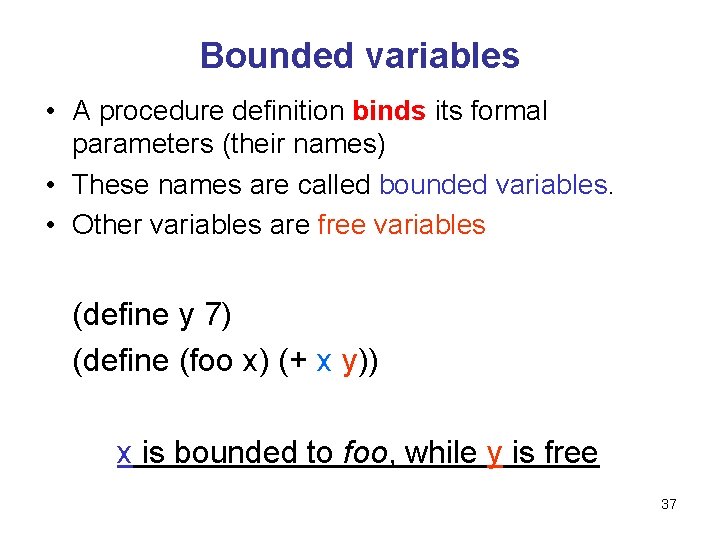
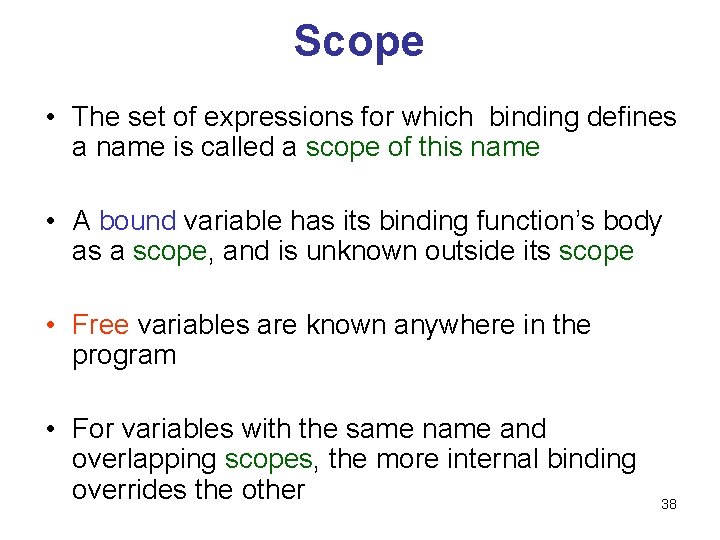
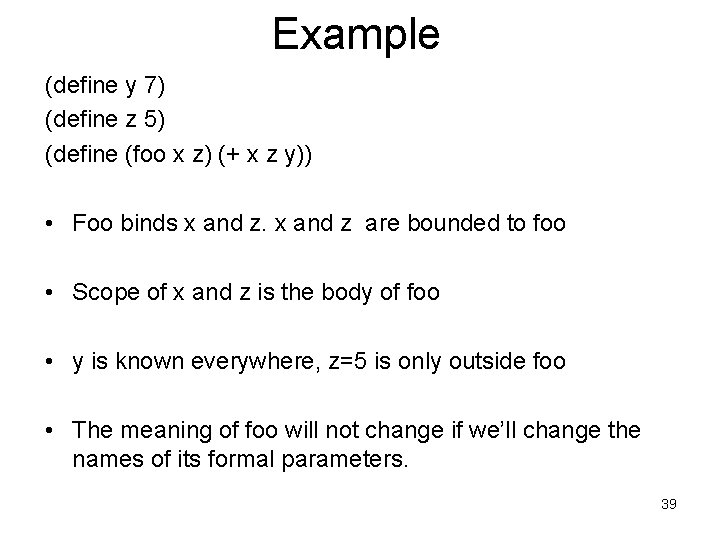
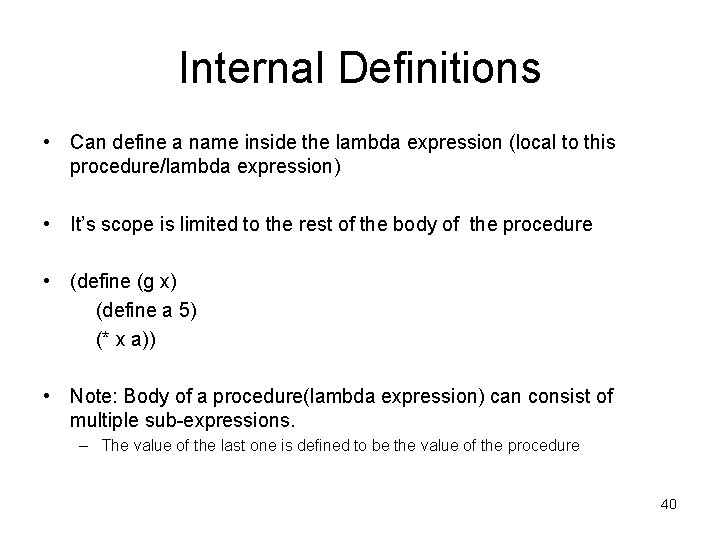
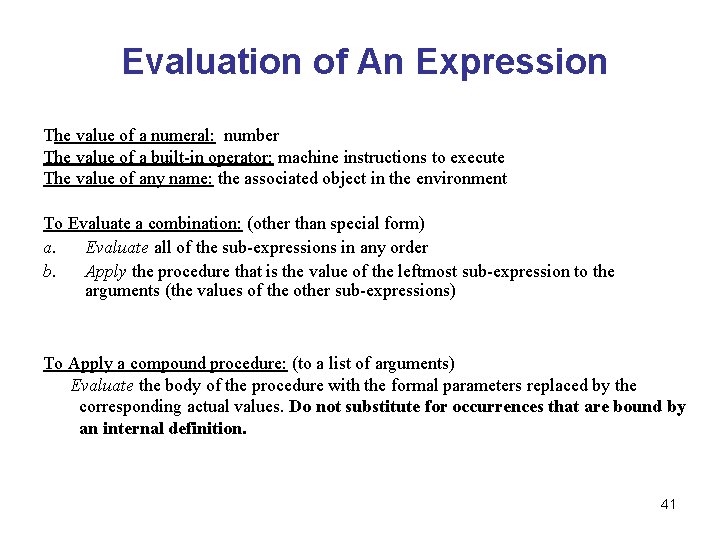
- Slides: 41
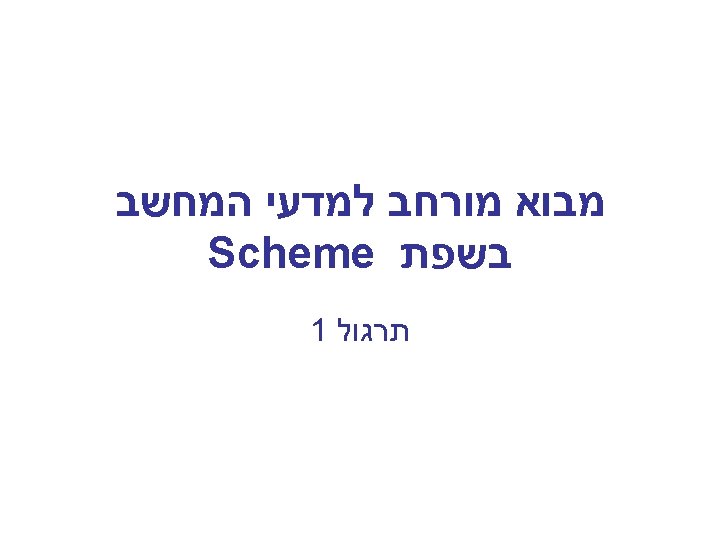
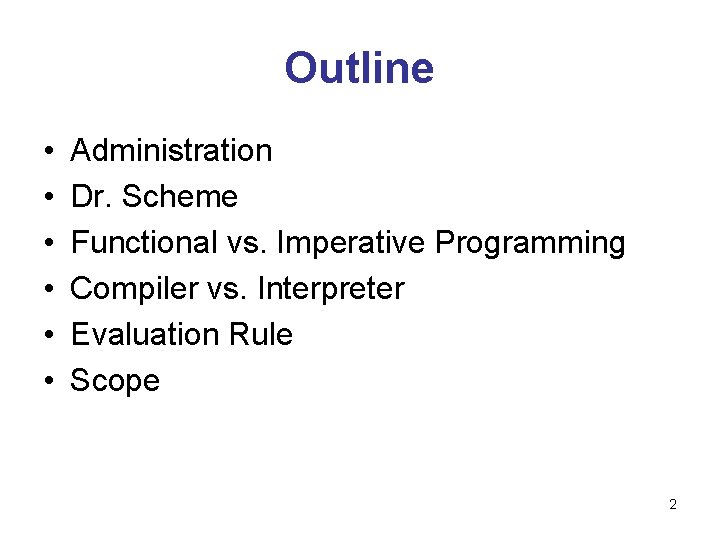
Outline • • • Administration Dr. Scheme Functional vs. Imperative Programming Compiler vs. Interpreter Evaluation Rule Scope 2
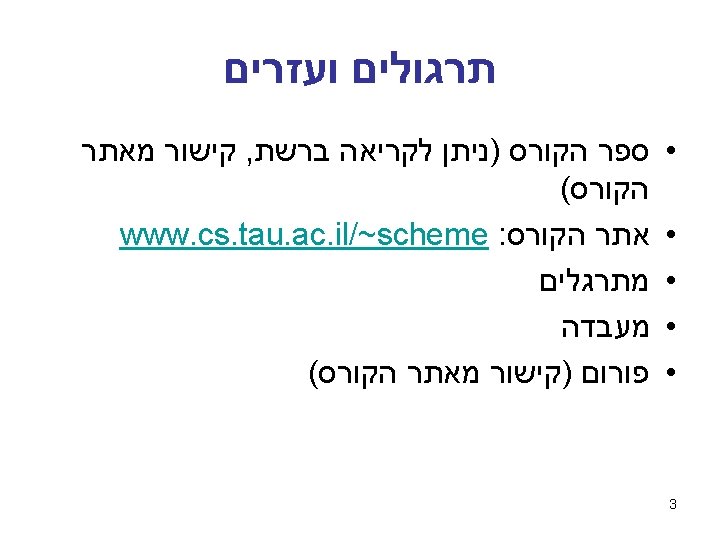
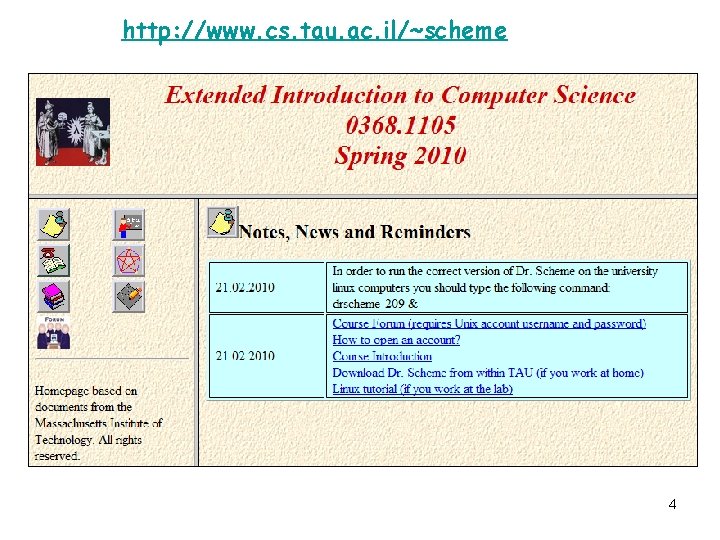
http: //www. cs. tau. ac. il/~scheme 4
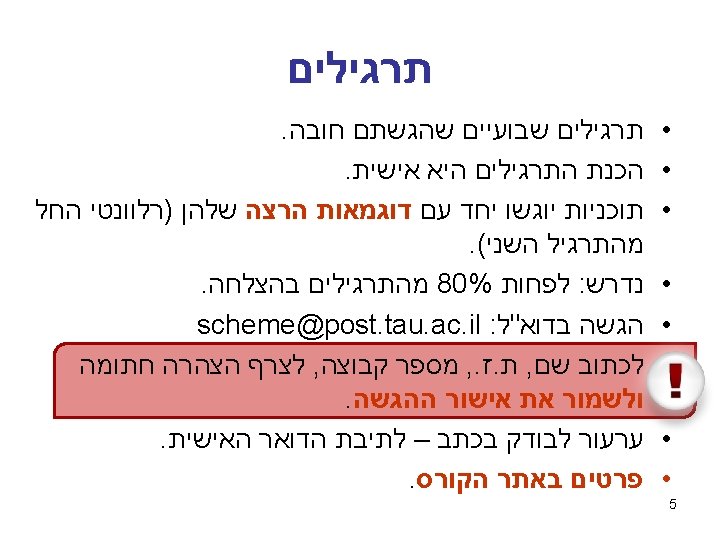
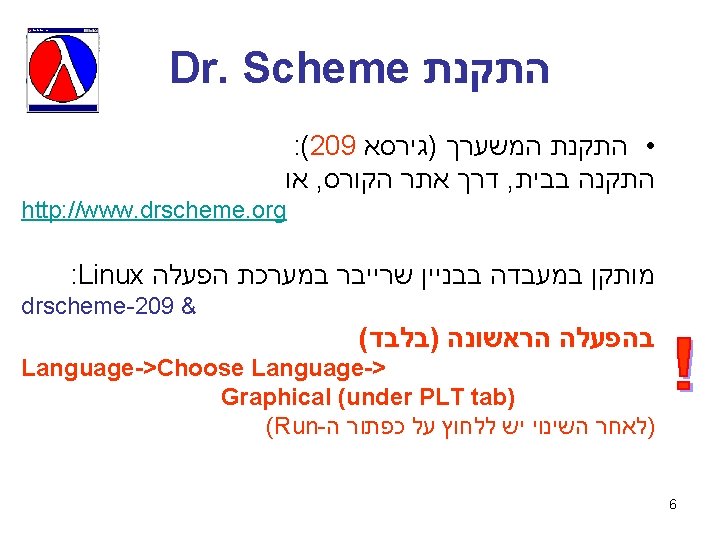
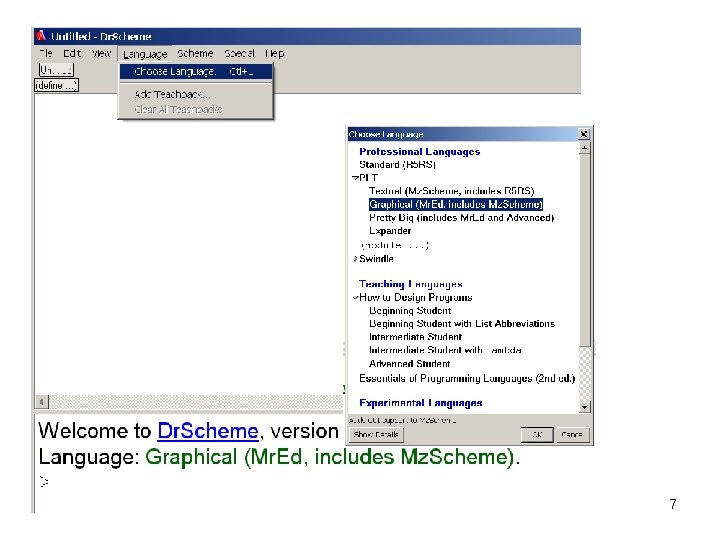
7
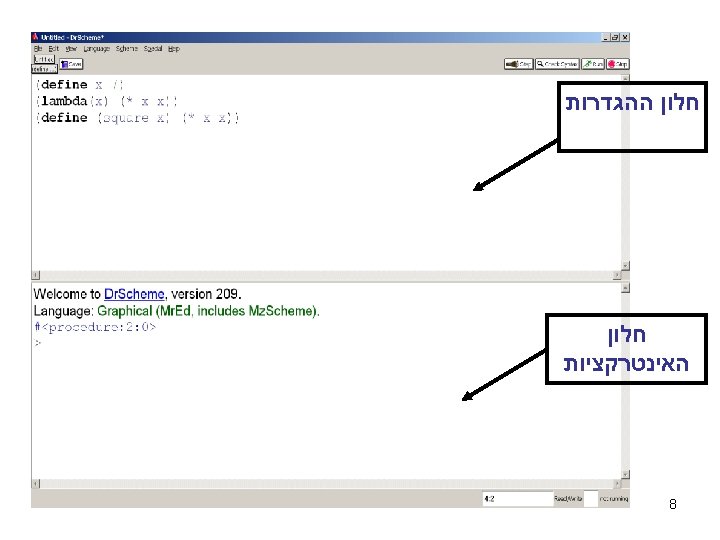
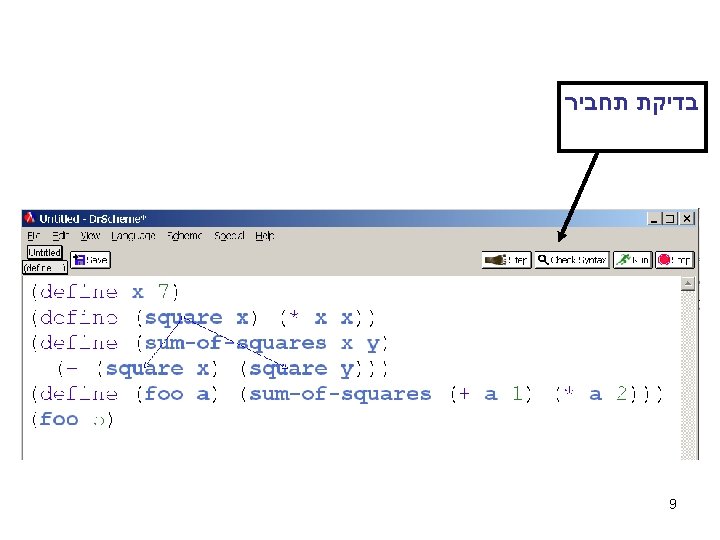
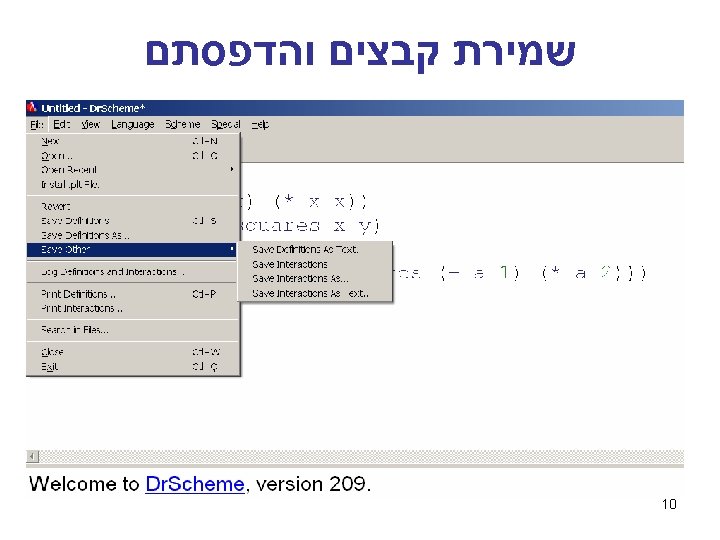
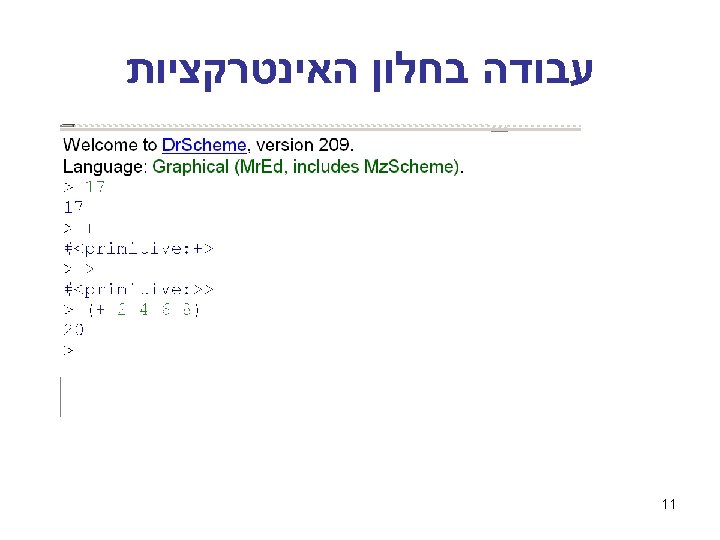
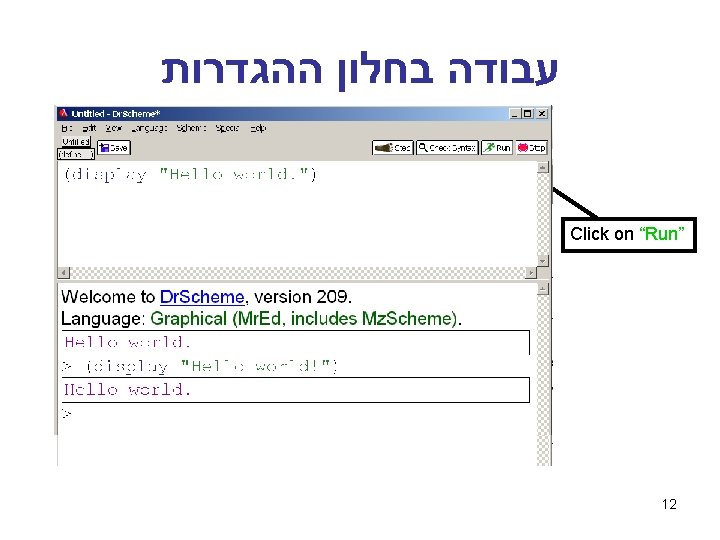
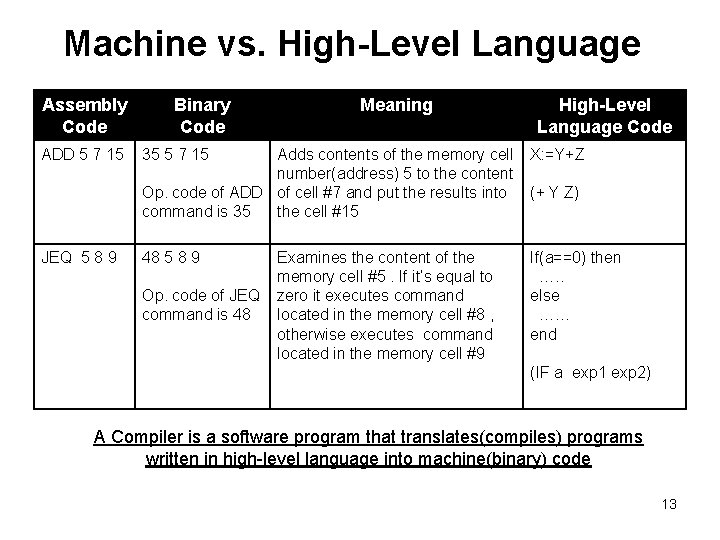
Machine vs. High-Level Language Assembly Code ADD 5 7 15 JEQ 5 8 9 Binary Code Meaning 35 5 7 15 High-Level Language Code Adds contents of the memory cell number(address) 5 to the content Op. code of ADD of cell #7 and put the results into command is 35 the cell #15 X: =Y+Z 48 5 8 9 If(a==0) then …. . else …… end Op. code of JEQ command is 48 Examines the content of the memory cell #5. If it’s equal to zero it executes command located in the memory cell #8 , otherwise executes command located in the memory cell #9 (+ Y Z) (IF a exp 1 exp 2) A Compiler is a software program that translates(compiles) programs written in high-level language into machine(binary) code 13
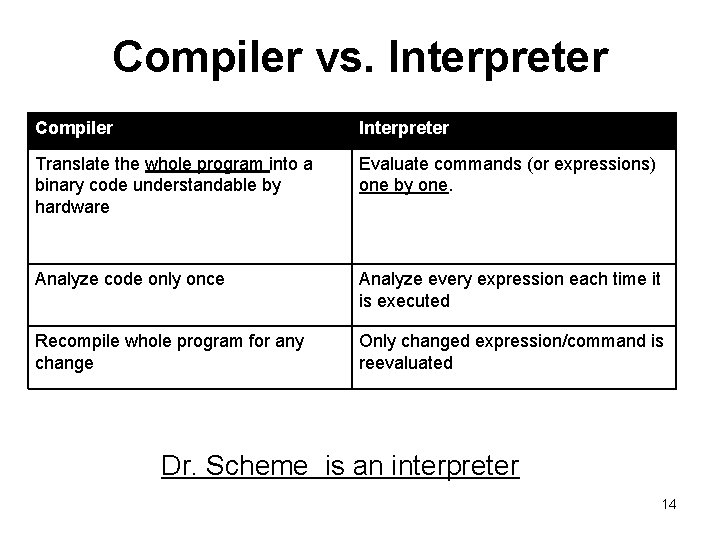
Compiler vs. Interpreter Compiler Interpreter Translate the whole program into a binary code understandable by hardware Evaluate commands (or expressions) one by one. Analyze code only once Analyze every expression each time it is executed Recompile whole program for any change Only changed expression/command is reevaluated Dr. Scheme is an interpreter 14
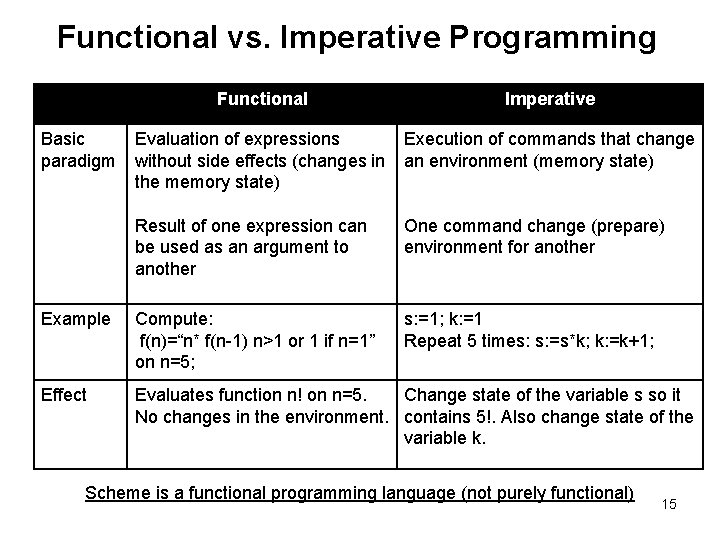
Functional vs. Imperative Programming Functional Imperative Evaluation of expressions without side effects (changes in the memory state) Execution of commands that change an environment (memory state) Result of one expression can be used as an argument to another One command change (prepare) environment for another Example Compute: f(n)=“n* f(n-1) n>1 or 1 if n=1” on n=5; s: =1; k: =1 Repeat 5 times: s: =s*k; k: =k+1; Effect Evaluates function n! on n=5. Change state of the variable s so it No changes in the environment. contains 5!. Also change state of the variable k. Basic paradigm Scheme is a functional programming language (not purely functional) 15
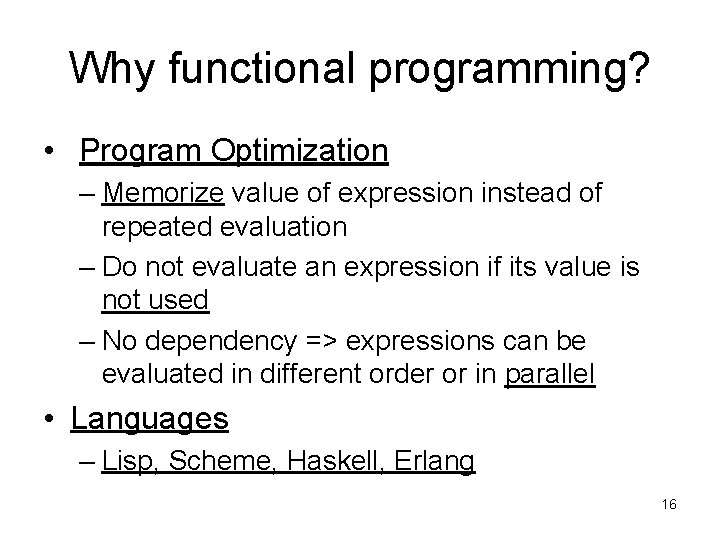
Why functional programming? • Program Optimization – Memorize value of expression instead of repeated evaluation – Do not evaluate an expression if its value is not used – No dependency => expressions can be evaluated in different order or in parallel • Languages – Lisp, Scheme, Haskell, Erlang 16
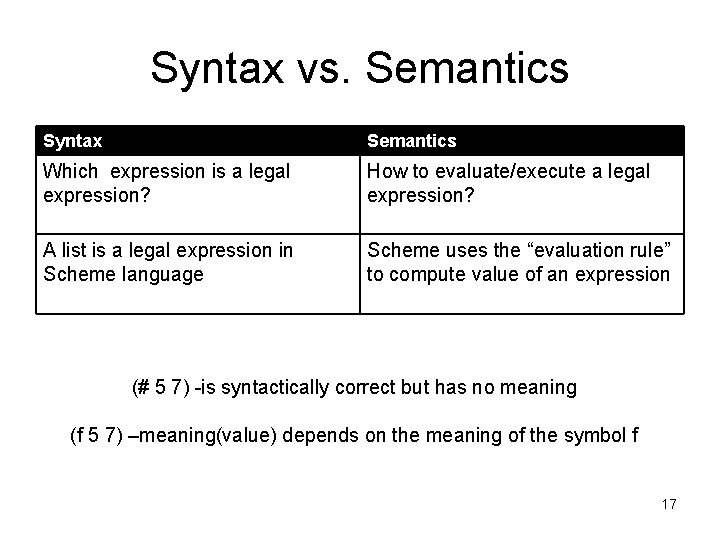
Syntax vs. Semantics Syntax Semantics Which expression is a legal expression? How to evaluate/execute a legal expression? A list is a legal expression in Scheme language Scheme uses the “evaluation rule” to compute value of an expression (# 5 7) -is syntactically correct but has no meaning (f 5 7) –meaning(value) depends on the meaning of the symbol f 17
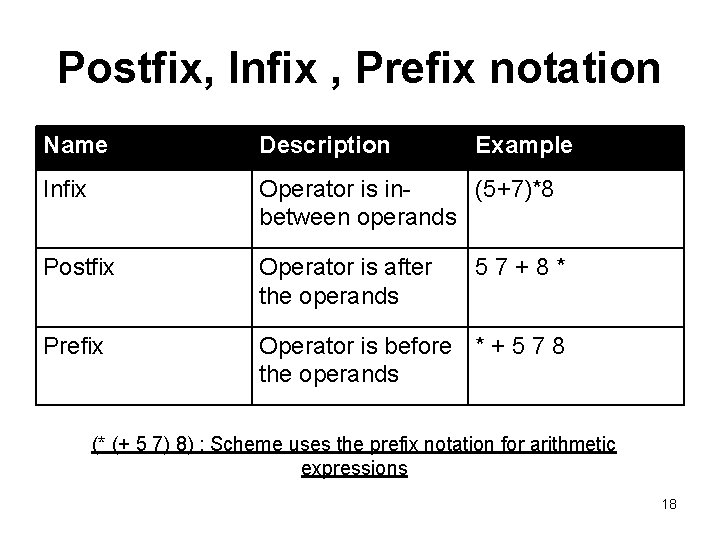
Postfix, Infix , Prefix notation Name Description Example Infix Operator is in(5+7)*8 between operands Postfix Operator is after the operands Prefix Operator is before * + 5 7 8 the operands 57+8* (* (+ 5 7) 8) : Scheme uses the prefix notation for arithmetic expressions 18
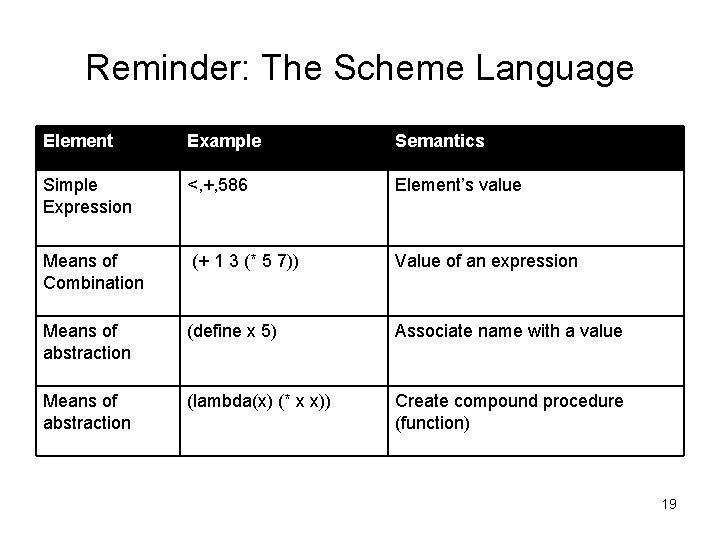
Reminder: The Scheme Language Element Example Semantics Simple Expression <, +, 586 Element’s value Means of Combination (+ 1 3 (* 5 7)) Value of an expression Means of abstraction (define x 5) Associate name with a value Means of abstraction (lambda(x) (* x x)) Create compound procedure (function) 19
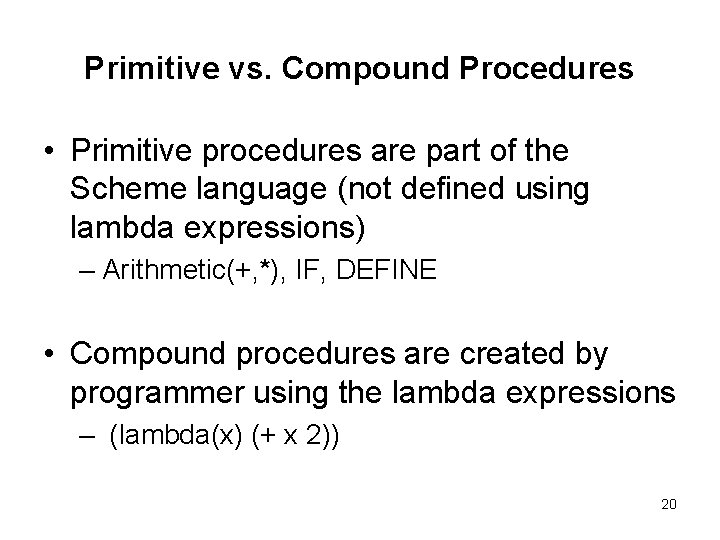
Primitive vs. Compound Procedures • Primitive procedures are part of the Scheme language (not defined using lambda expressions) – Arithmetic(+, *), IF, DEFINE • Compound procedures are created by programmer using the lambda expressions – (lambda(x) (+ x 2)) 20
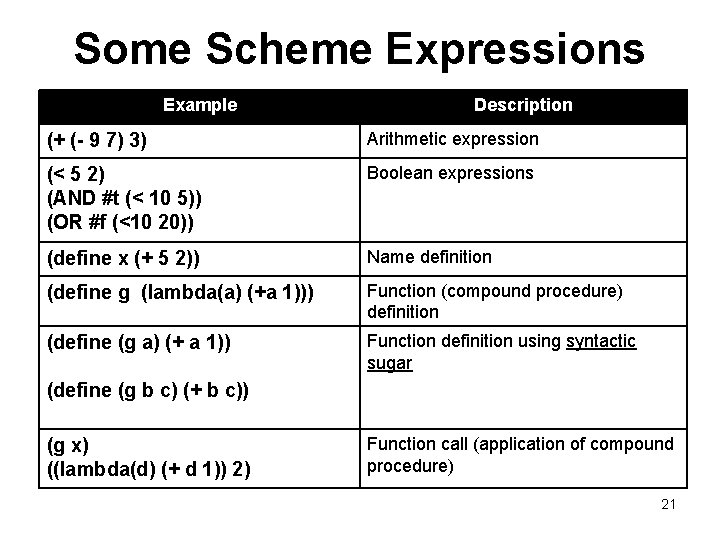
Some Scheme Expressions Example Description (+ (- 9 7) 3) Arithmetic expression (< 5 2) (AND #t (< 10 5)) (OR #f (<10 20)) Boolean expressions (define x (+ 5 2)) Name definition (define g (lambda(a) (+a 1))) Function (compound procedure) definition (define (g a) (+ a 1)) Function definition using syntactic sugar (define (g b c) (+ b c)) (g x) ((lambda(d) (+ d 1)) 2) Function call (application of compound procedure) 21
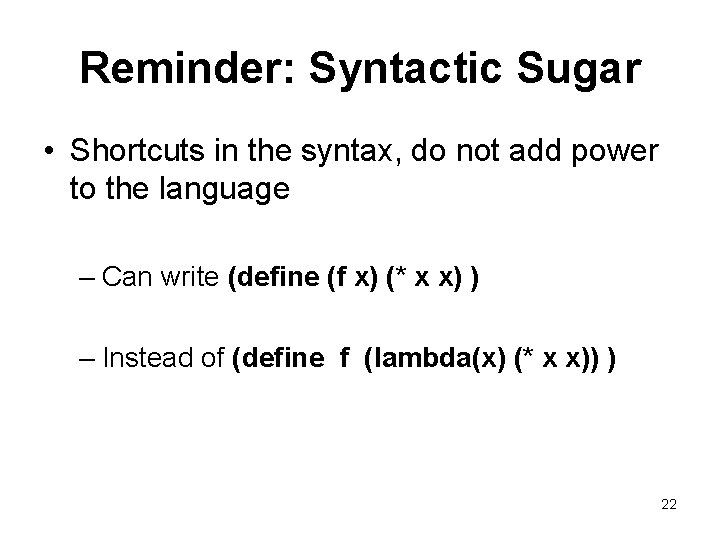
Reminder: Syntactic Sugar • Shortcuts in the syntax, do not add power to the language – Can write (define (f x) (* x x) ) – Instead of (define f (lambda(x) (* x x)) ) 22
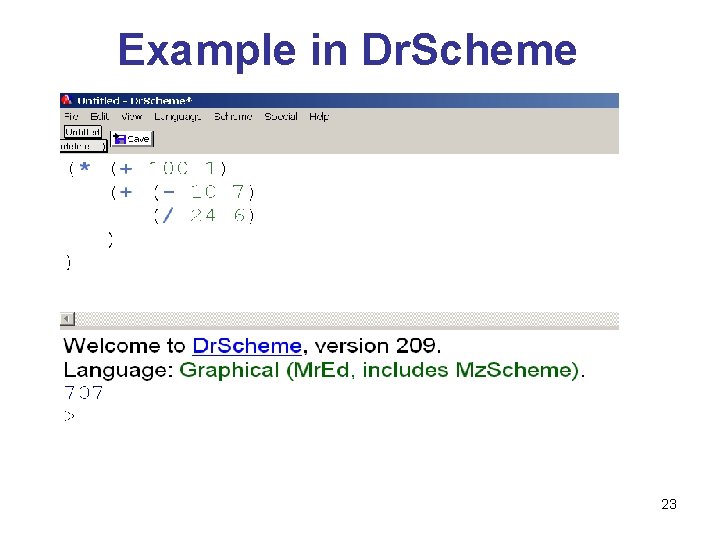
Example in Dr. Scheme 23
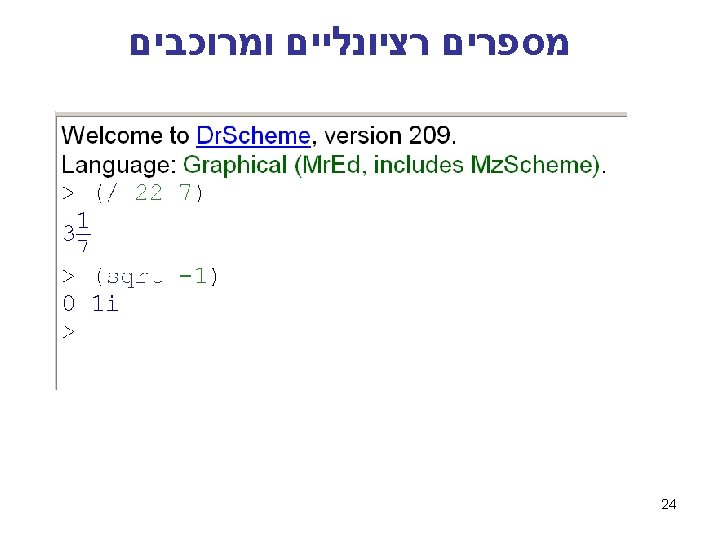
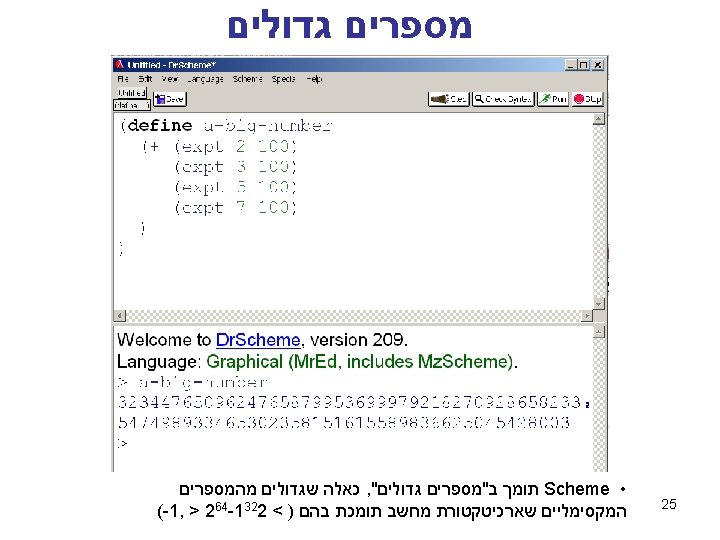
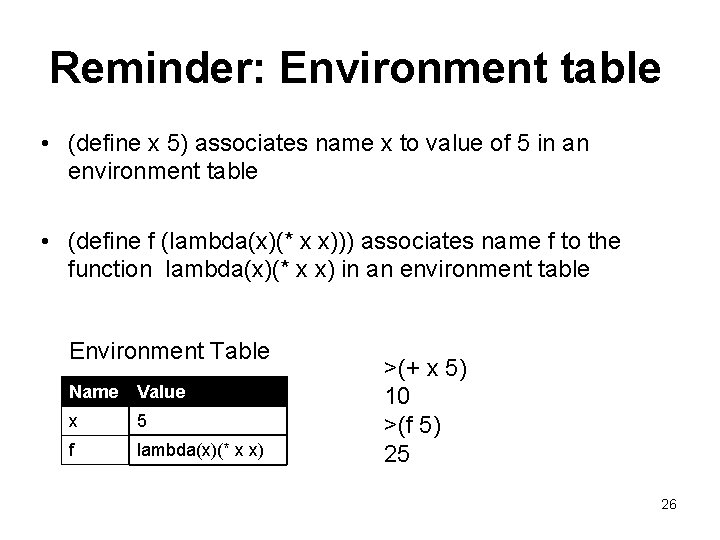
Reminder: Environment table • (define x 5) associates name x to value of 5 in an environment table • (define f (lambda(x)(* x x))) associates name f to the function lambda(x)(* x x) in an environment table Environment Table Name Value x 5 f lambda(x)(* x x) >(+ x 5) 10 >(f 5) 25 26
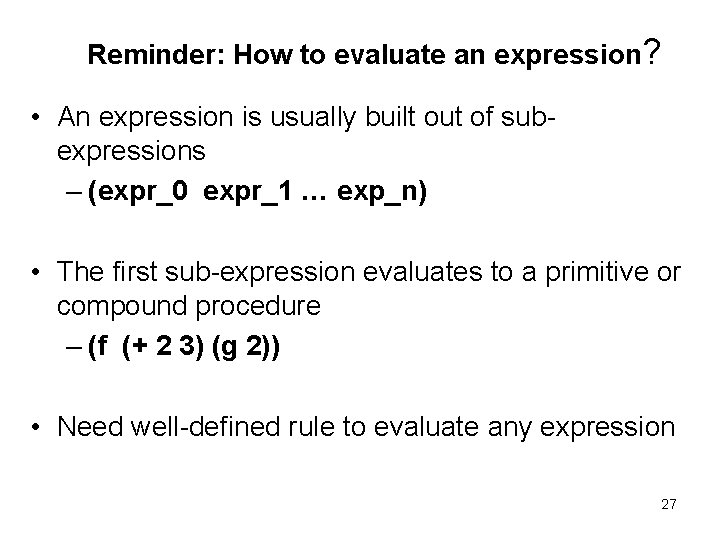
Reminder: How to evaluate an expression? • An expression is usually built out of subexpressions – (expr_0 expr_1 … exp_n) • The first sub-expression evaluates to a primitive or compound procedure – (f (+ 2 3) (g 2)) • Need well-defined rule to evaluate any expression 27
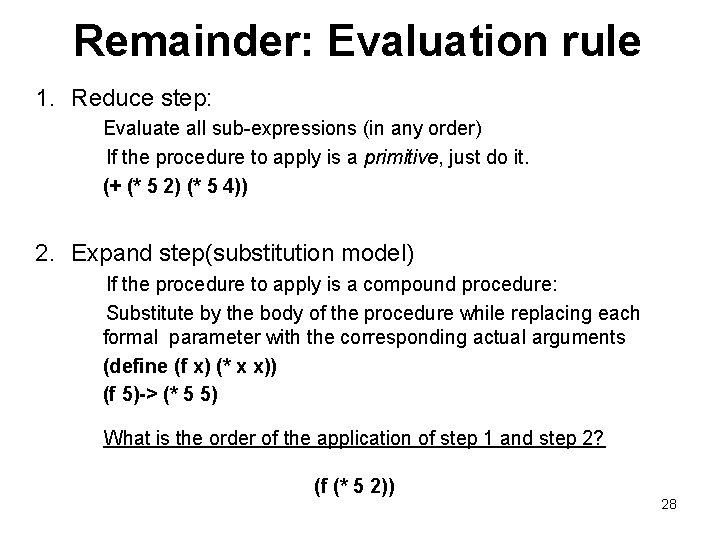
Remainder: Evaluation rule 1. Reduce step: Evaluate all sub-expressions (in any order) If the procedure to apply is a primitive, just do it. (+ (* 5 2) (* 5 4)) 2. Expand step(substitution model) If the procedure to apply is a compound procedure: Substitute by the body of the procedure while replacing each formal parameter with the corresponding actual arguments (define (f x) (* x x)) (f 5)-> (* 5 5) What is the order of the application of step 1 and step 2? (f (* 5 2)) 28
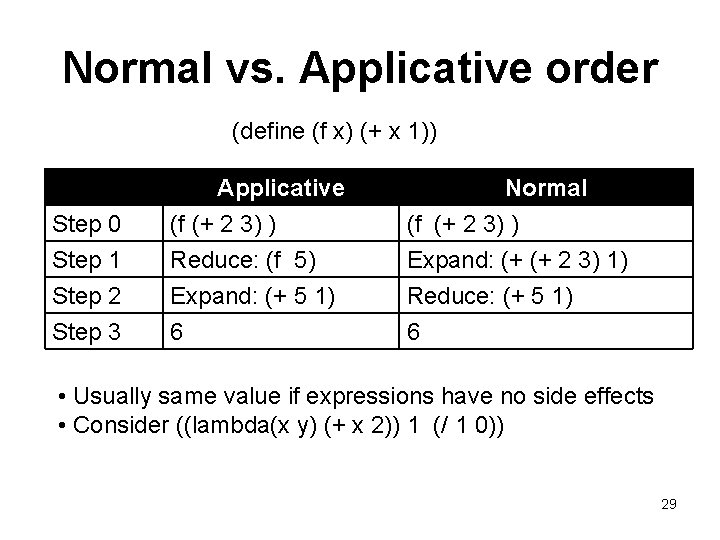
Normal vs. Applicative order (define (f x) (+ x 1)) Step 0 Step 1 Step 2 Applicative (f (+ 2 3) ) Reduce: (f 5) Expand: (+ 5 1) Normal (f (+ 2 3) ) Expand: (+ (+ 2 3) 1) Reduce: (+ 5 1) Step 3 6 6 • Usually same value if expressions have no side effects • Consider ((lambda(x y) (+ x 2)) 1 (/ 1 0)) 29
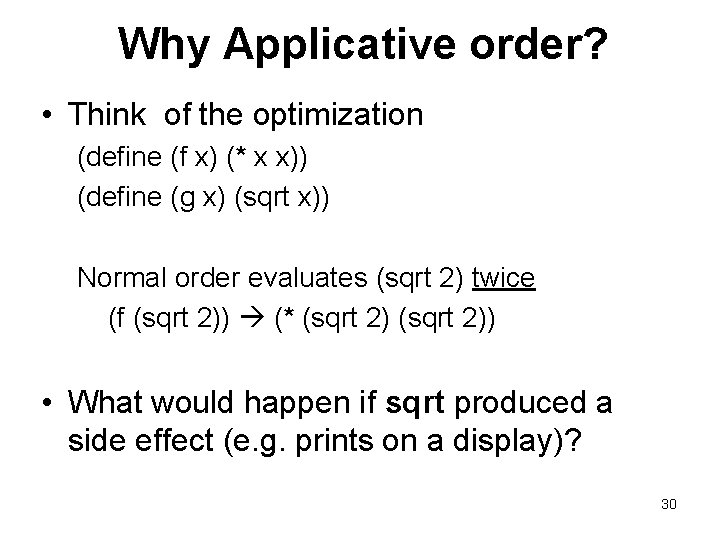
Why Applicative order? • Think of the optimization (define (f x) (* x x)) (define (g x) (sqrt x)) Normal order evaluates (sqrt 2) twice (f (sqrt 2)) (* (sqrt 2)) • What would happen if sqrt produced a side effect (e. g. prints on a display)? 30
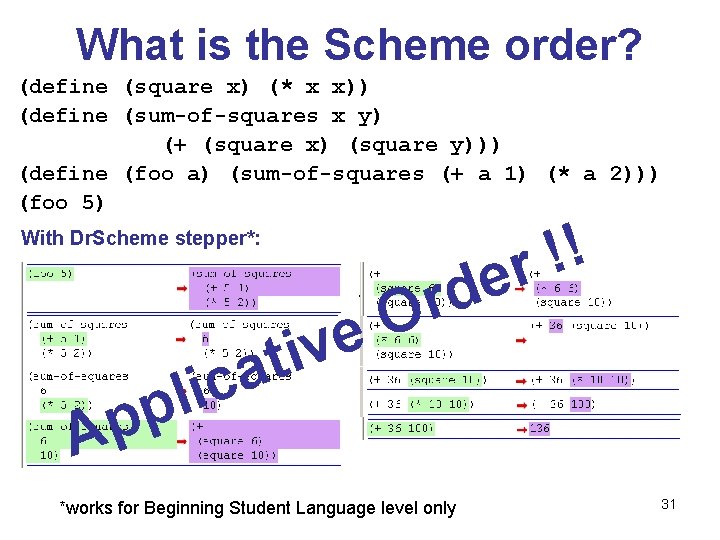
What is the Scheme order? (define (square x) (* x x)) (define (sum-of-squares x y) (+ (square x) (square y))) (define (foo a) (sum-of-squares (+ a 1) (* a 2))) (foo 5) ! ! er With Dr. Scheme stepper*: l pp i t a c i A d r O ve *works for Beginning Student Language level only 31
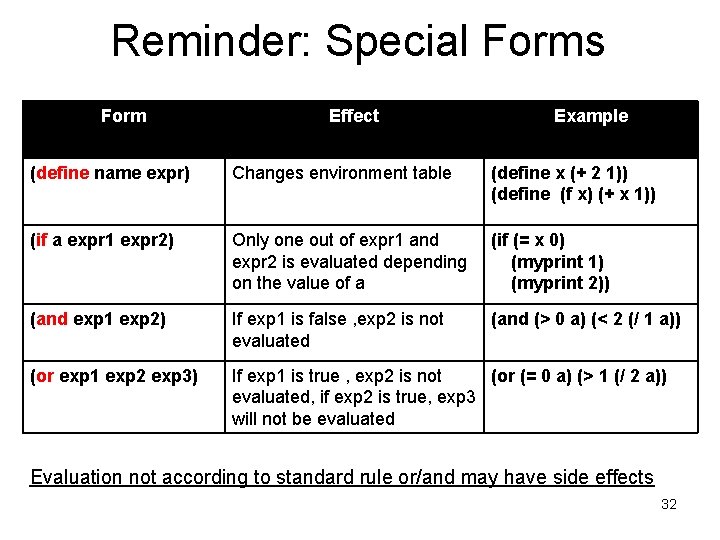
Reminder: Special Forms Form Effect Example (define name expr) Changes environment table (define x (+ 2 1)) (define (f x) (+ x 1)) (if a expr 1 expr 2) Only one out of expr 1 and expr 2 is evaluated depending on the value of a (if (= x 0) (myprint 1) (myprint 2)) (and exp 1 exp 2) If exp 1 is false , exp 2 is not evaluated (and (> 0 a) (< 2 (/ 1 a)) (or exp 1 exp 2 exp 3) If exp 1 is true , exp 2 is not (or (= 0 a) (> 1 (/ 2 a)) evaluated, if exp 2 is true, exp 3 will not be evaluated Evaluation not according to standard rule or/and may have side effects 32
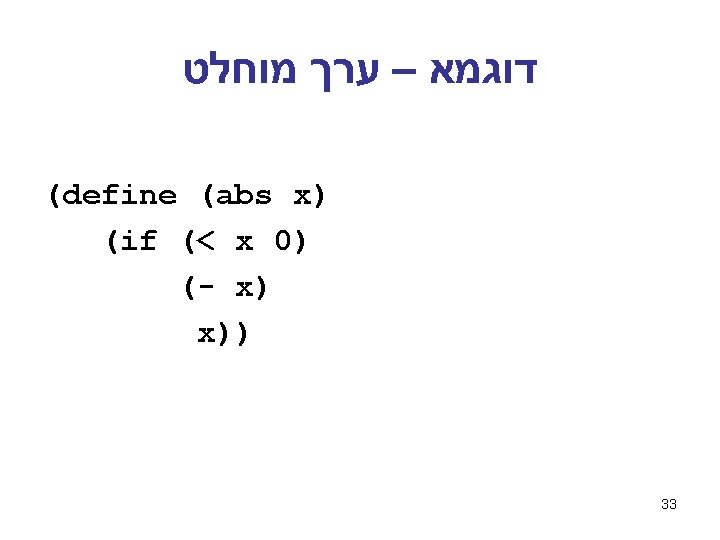
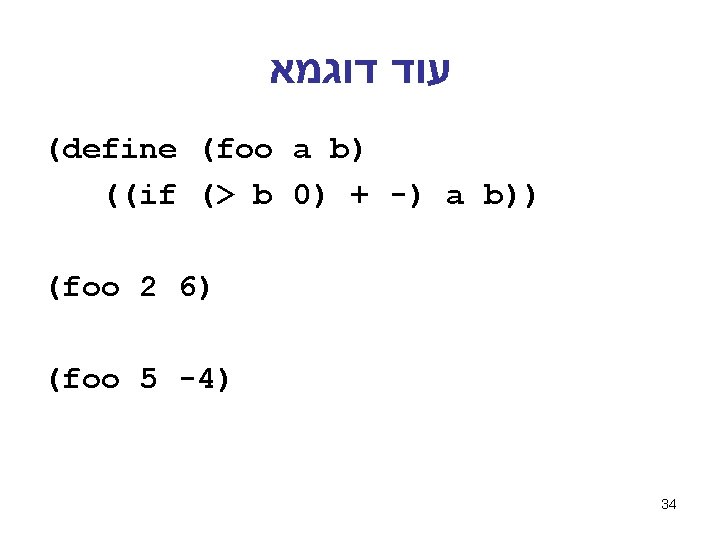
עוד דוגמא (define (foo a b) ((if (> b 0) + -) a b)) (foo 2 6) (foo 5 -4) 34
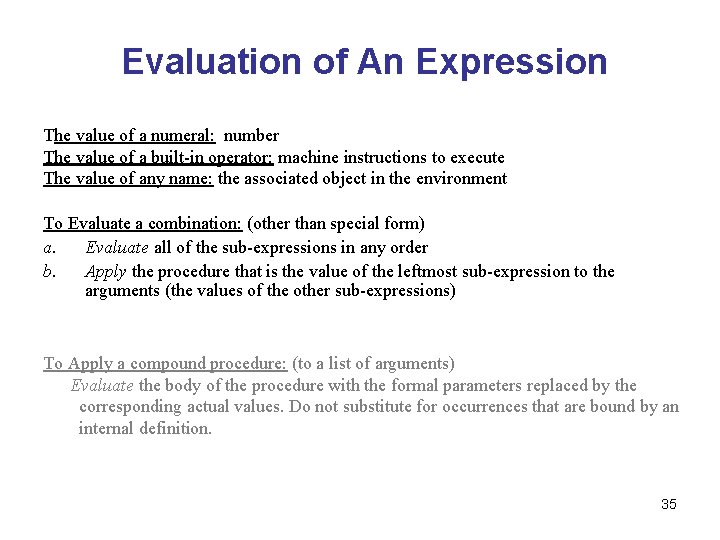
Evaluation of An Expression The value of a numeral: number The value of a built-in operator: machine instructions to execute The value of any name: the associated object in the environment To Evaluate a combination: (other than special form) a. Evaluate all of the sub-expressions in any order b. Apply the procedure that is the value of the leftmost sub-expression to the arguments (the values of the other sub-expressions) To Apply a compound procedure: (to a list of arguments) Evaluate the body of the procedure with the formal parameters replaced by the corresponding actual values. Do not substitute for occurrences that are bound by an internal definition. 35
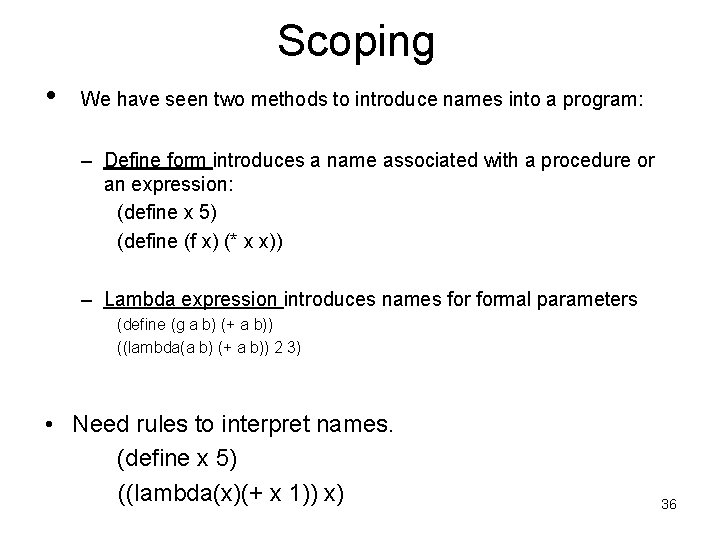
Scoping • We have seen two methods to introduce names into a program: – Define form introduces a name associated with a procedure or an expression: (define x 5) (define (f x) (* x x)) – Lambda expression introduces names formal parameters (define (g a b) (+ a b)) ((lambda(a b) (+ a b)) 2 3) • Need rules to interpret names. (define x 5) ((lambda(x)(+ x 1)) x) 36
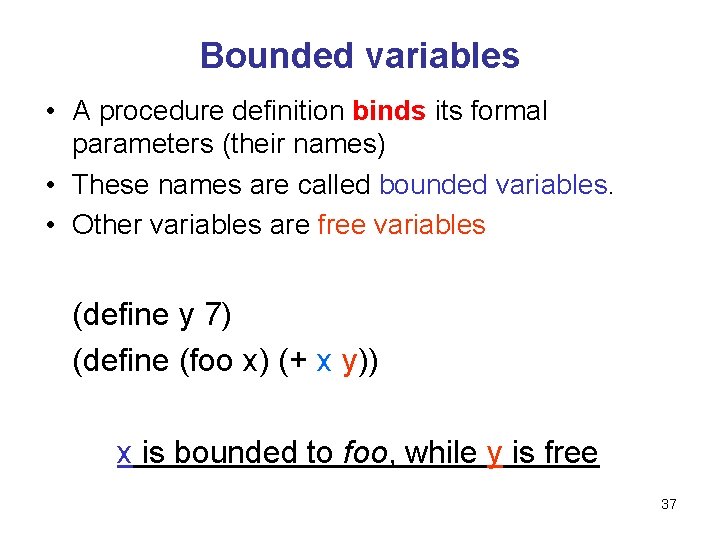
Bounded variables • A procedure definition binds its formal parameters (their names) • These names are called bounded variables. • Other variables are free variables (define y 7) (define (foo x) (+ x y)) x is bounded to foo, while y is free 37
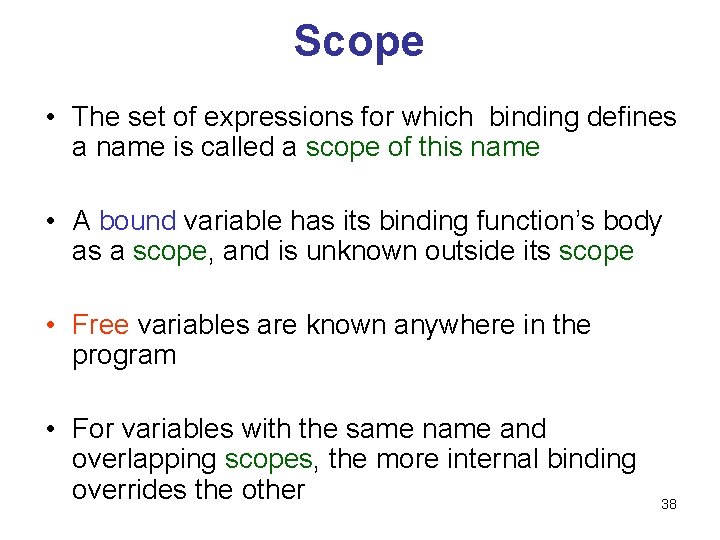
Scope • The set of expressions for which binding defines a name is called a scope of this name • A bound variable has its binding function’s body as a scope, and is unknown outside its scope • Free variables are known anywhere in the program • For variables with the same name and overlapping scopes, the more internal binding overrides the other 38
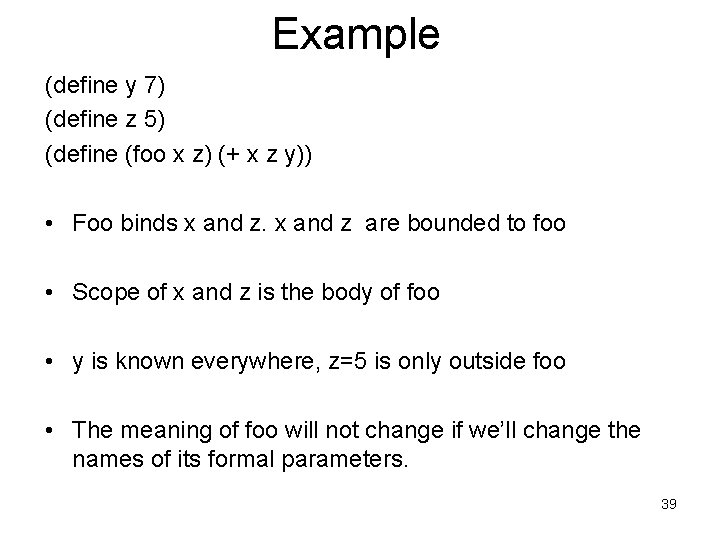
Example (define y 7) (define z 5) (define (foo x z) (+ x z y)) • Foo binds x and z are bounded to foo • Scope of x and z is the body of foo • y is known everywhere, z=5 is only outside foo • The meaning of foo will not change if we’ll change the names of its formal parameters. 39
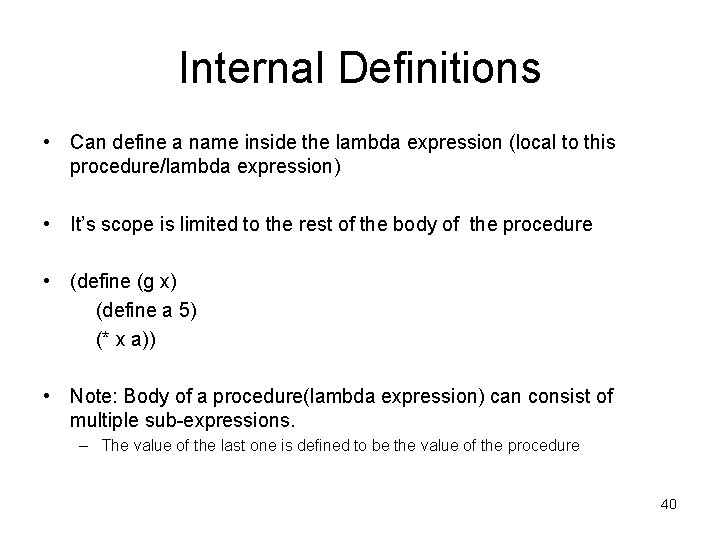
Internal Definitions • Can define a name inside the lambda expression (local to this procedure/lambda expression) • It’s scope is limited to the rest of the body of the procedure • (define (g x) (define a 5) (* x a)) • Note: Body of a procedure(lambda expression) can consist of multiple sub-expressions. – The value of the last one is defined to be the value of the procedure 40
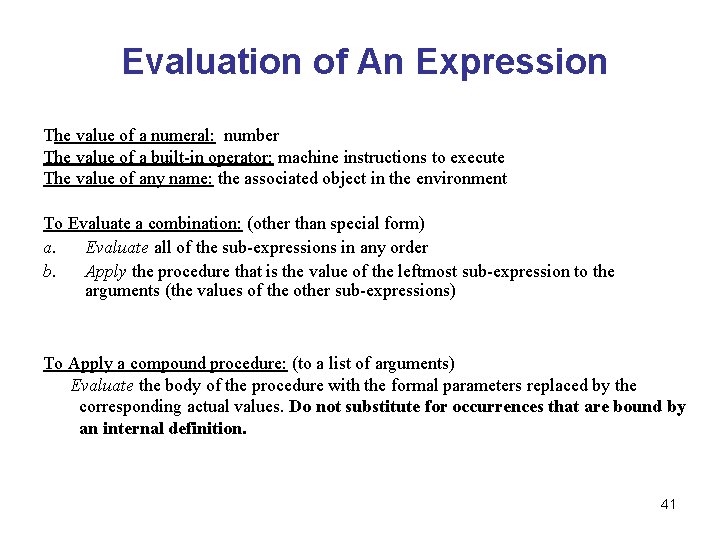
Evaluation of An Expression The value of a numeral: number The value of a built-in operator: machine instructions to execute The value of any name: the associated object in the environment To Evaluate a combination: (other than special form) a. Evaluate all of the sub-expressions in any order b. Apply the procedure that is the value of the leftmost sub-expression to the arguments (the values of the other sub-expressions) To Apply a compound procedure: (to a list of arguments) Evaluate the body of the procedure with the formal parameters replaced by the corresponding actual values. Do not substitute for occurrences that are bound by an internal definition. 41
Functional programming scheme
An imperative statement in system programming
Comparative programming languages
Forward reference table (frt) is arranged like
Assembly language programming
3 domain scheme and 5 kingdom scheme
Stata graph schemes download
Pyramid scheme vs ponzi scheme
Semi fixed space maintainer
Non functional plasma enzyme example
Enzymes
Functional and non functional
Scheme programming
Functional english course outline
Procedural vs functional
Fundamentals of functional programming language
Elm functional programming
Functional programming roadmap
Lisp functional programming
Typed functional programming
Fundamentals of functional programming language
Programming xkcd
Adt functional programming
Is lisp a functional programming language
Functional decomposition programming
Lisp functional programming
Functional programming
Evidence sandwich example
Ai2.appinventor.mit.edu emulator
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
System programming
Integer programming vs linear programming
Programing adalah
What is bossy verbs
Imperative declarative exclamatory
Kant first categorical imperative
Assembly language example
Reported speech order
Pronominal verbs passé composé interrogative
Actual parameters in c
Hypothetical imperative