Methods Parameters ICS 111 Introduction to Computer Science
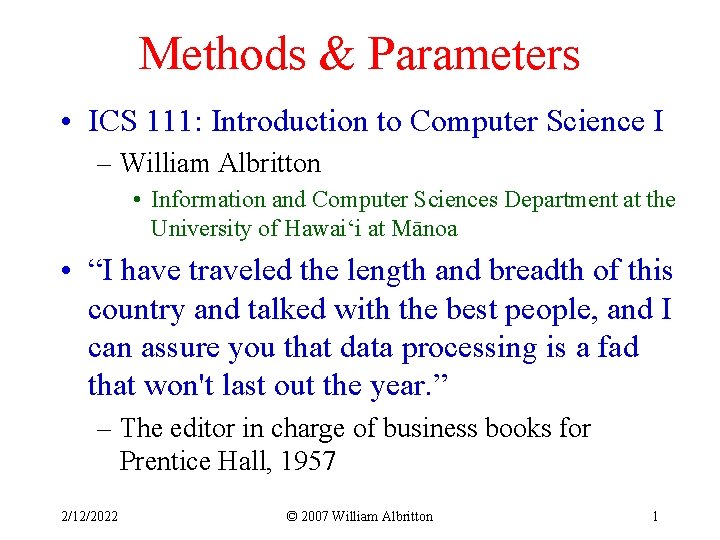
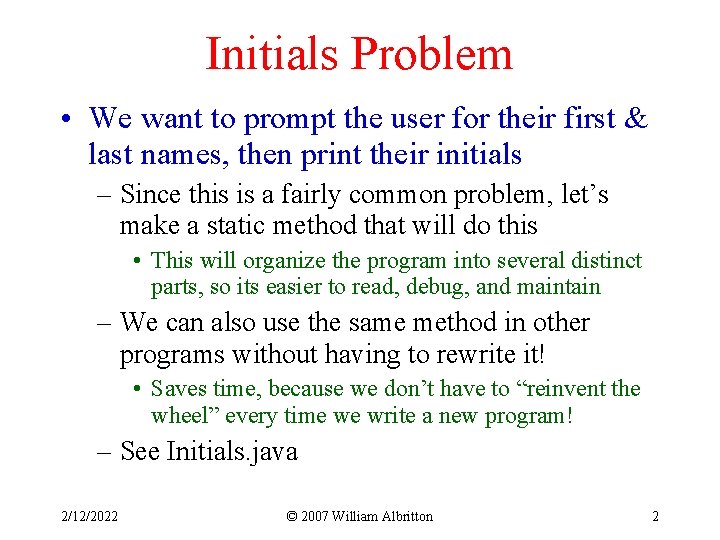
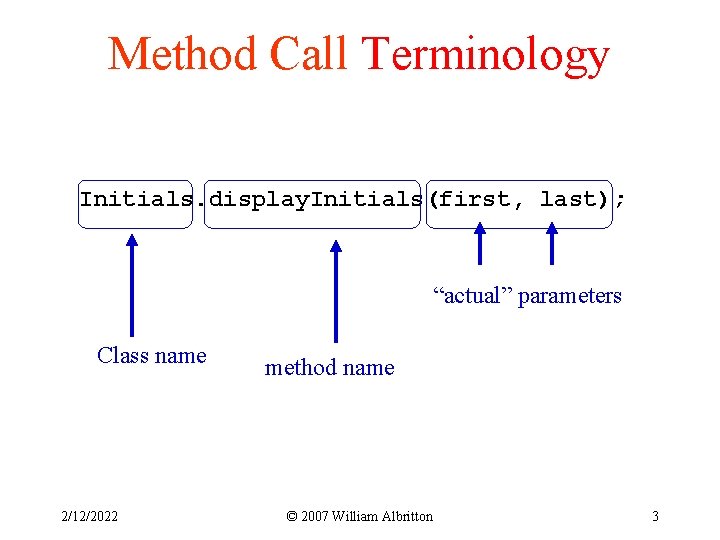
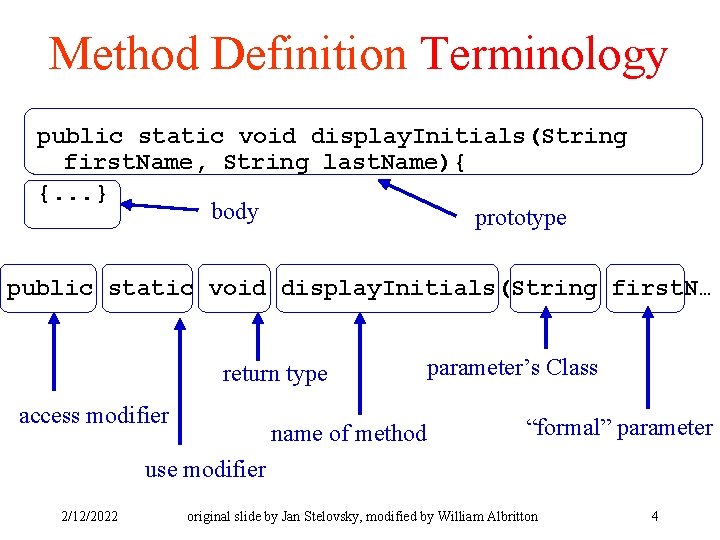
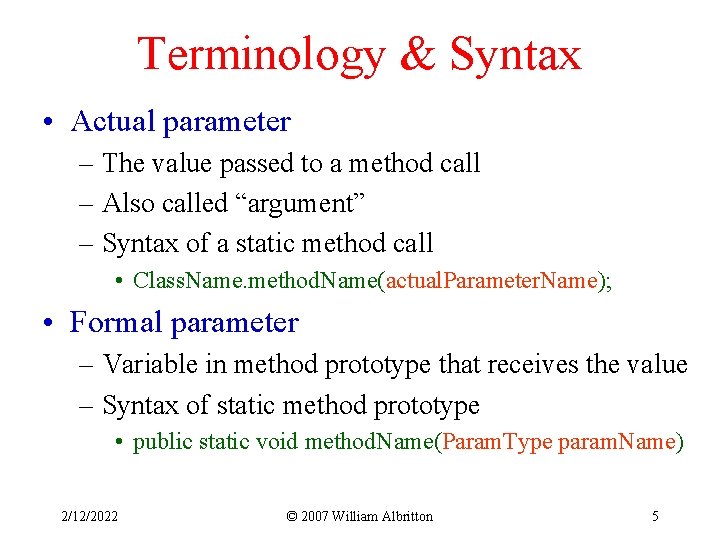
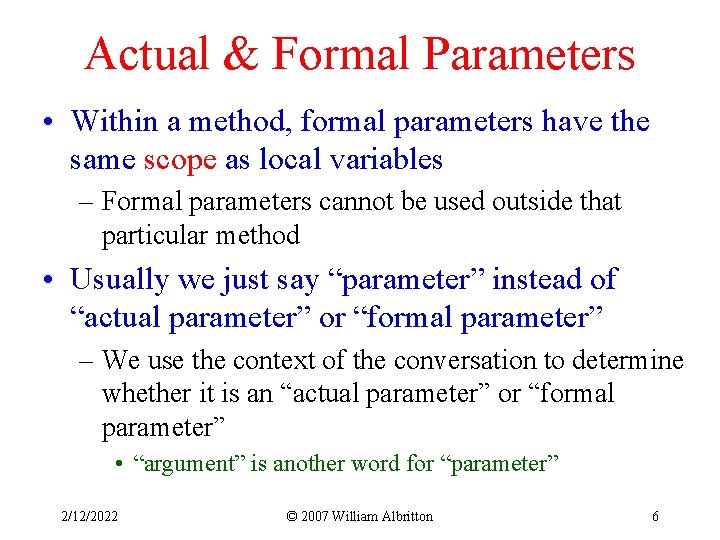
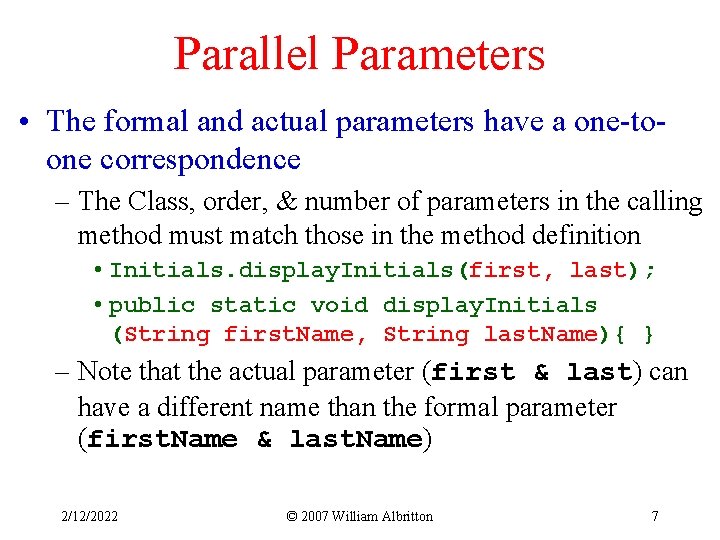
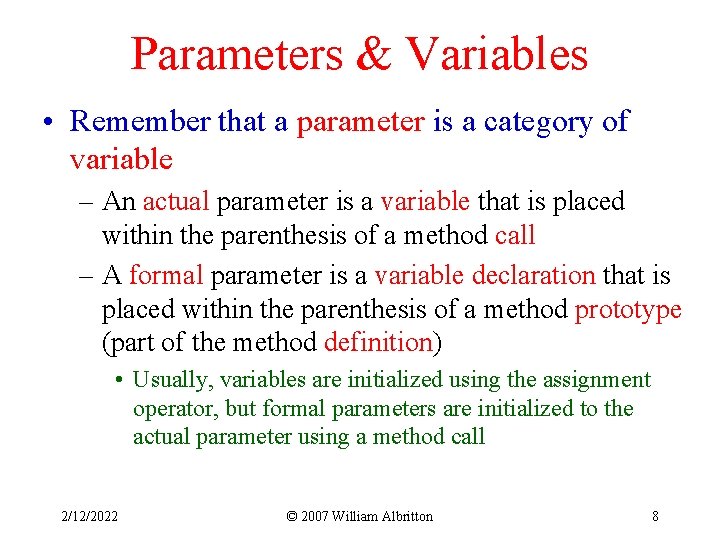
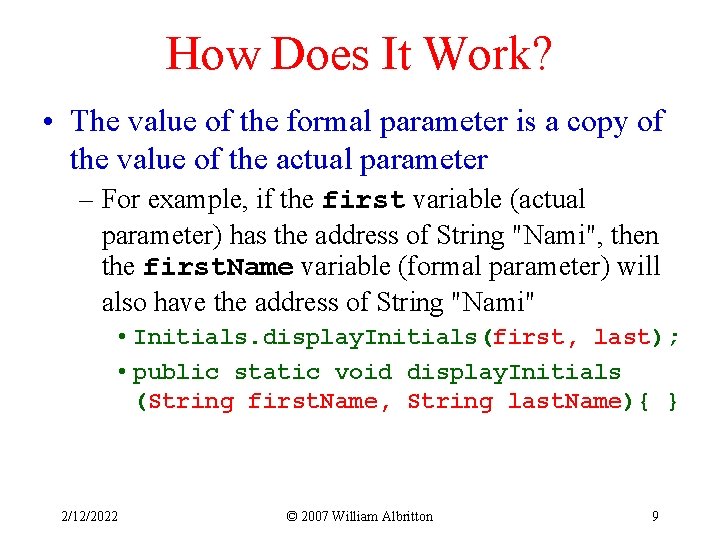
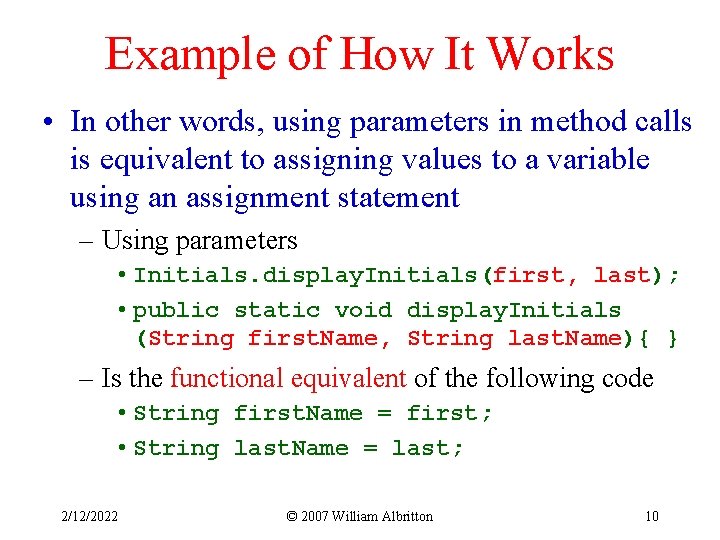
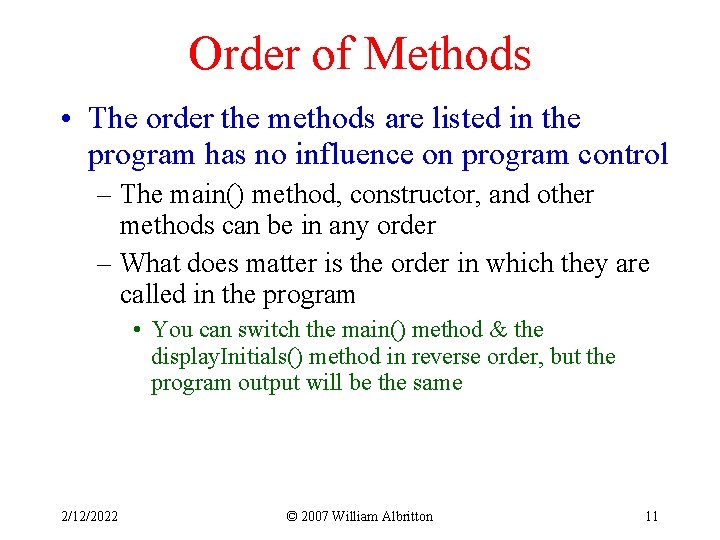
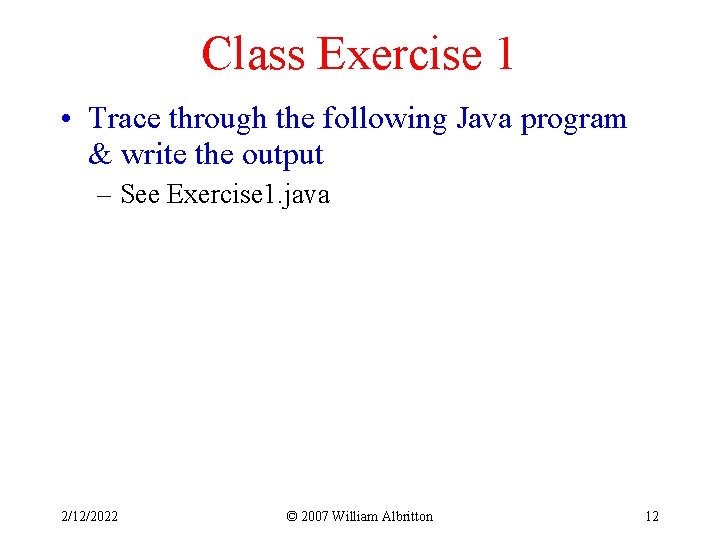
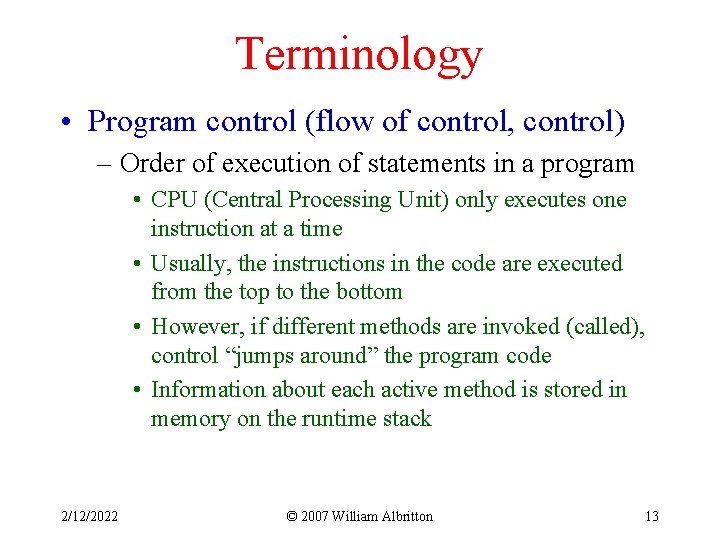
![Program Control main() method main(String [] args){ } method 1(); other methods method 1(){ Program Control main() method main(String [] args){ } method 1(); other methods method 1(){](https://slidetodoc.com/presentation_image_h2/b0631efcf04634fd79ad41d9b5520862/image-14.jpg)
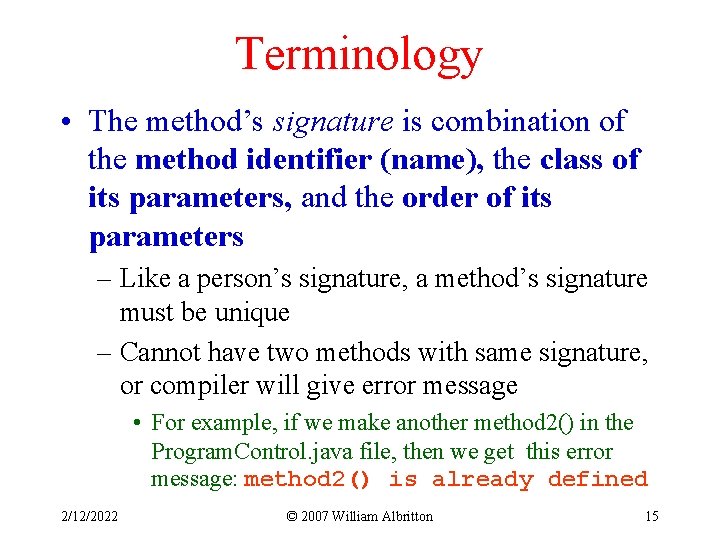
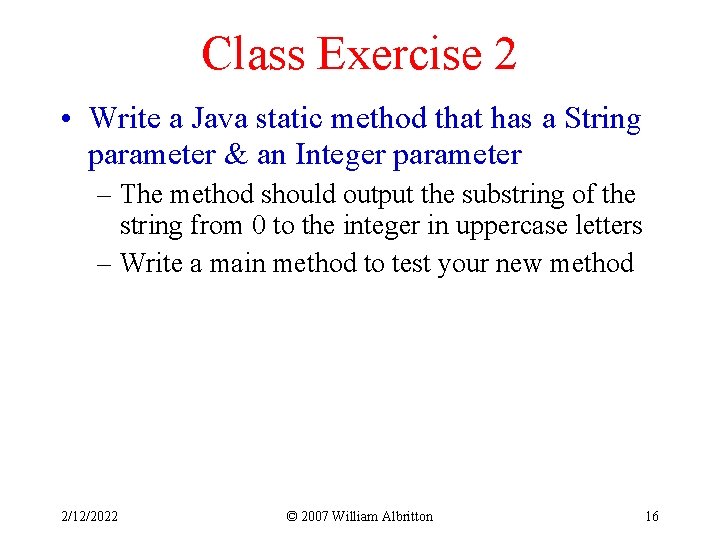
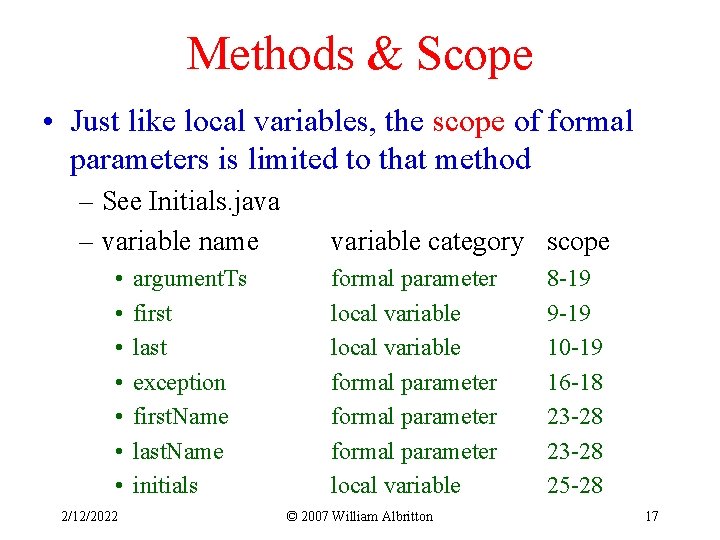
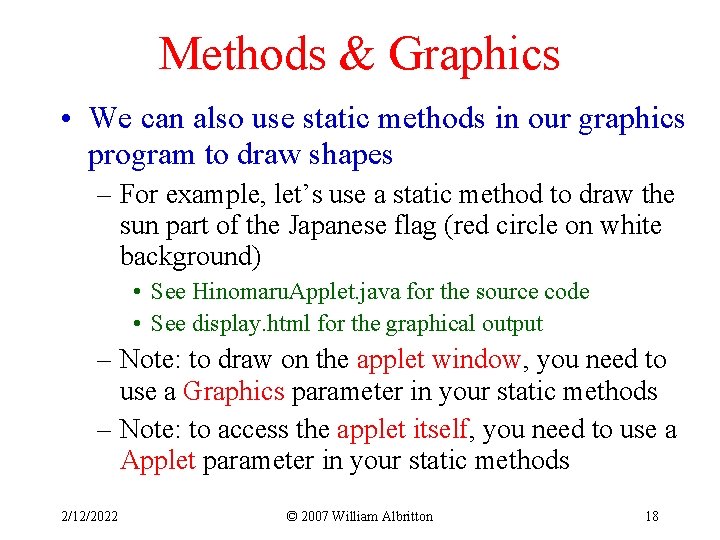
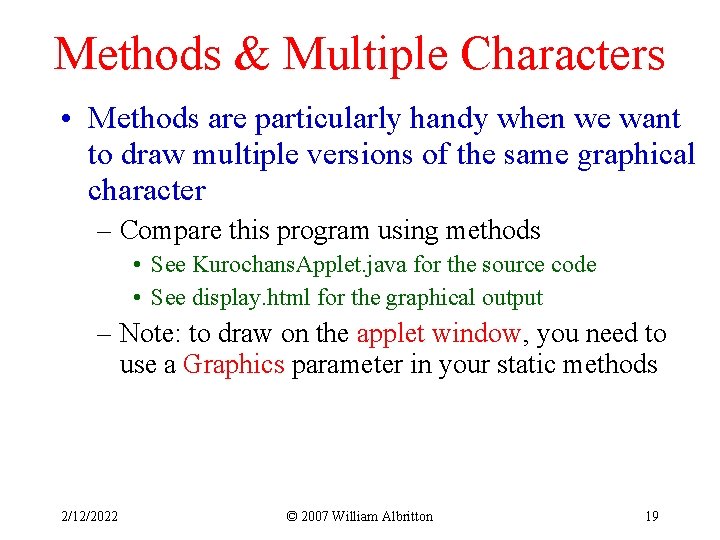
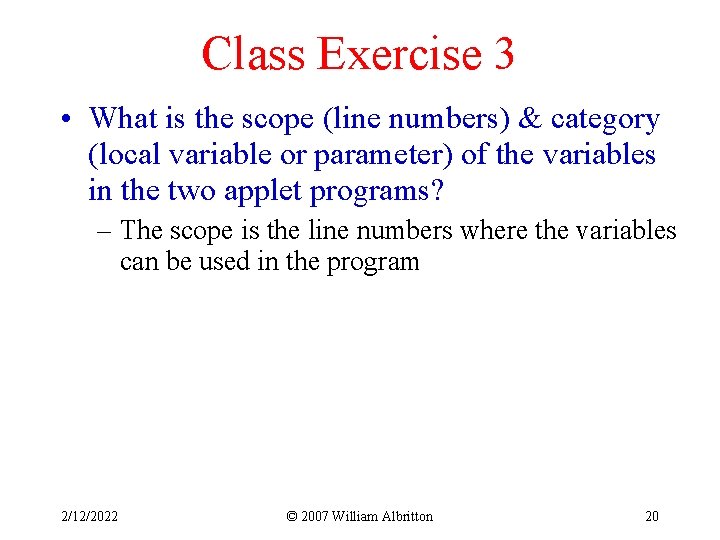
- Slides: 20
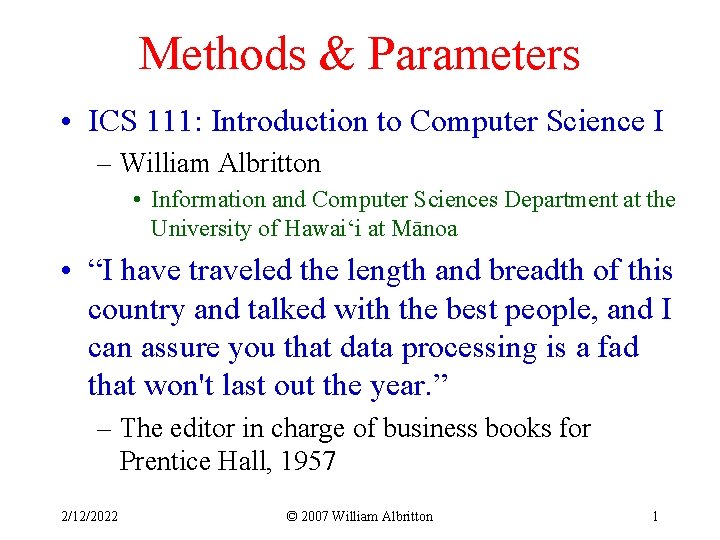
Methods & Parameters • ICS 111: Introduction to Computer Science I – William Albritton • Information and Computer Sciences Department at the University of Hawai‘i at Mānoa • “I have traveled the length and breadth of this country and talked with the best people, and I can assure you that data processing is a fad that won't last out the year. ” – The editor in charge of business books for Prentice Hall, 1957 2/12/2022 © 2007 William Albritton 1
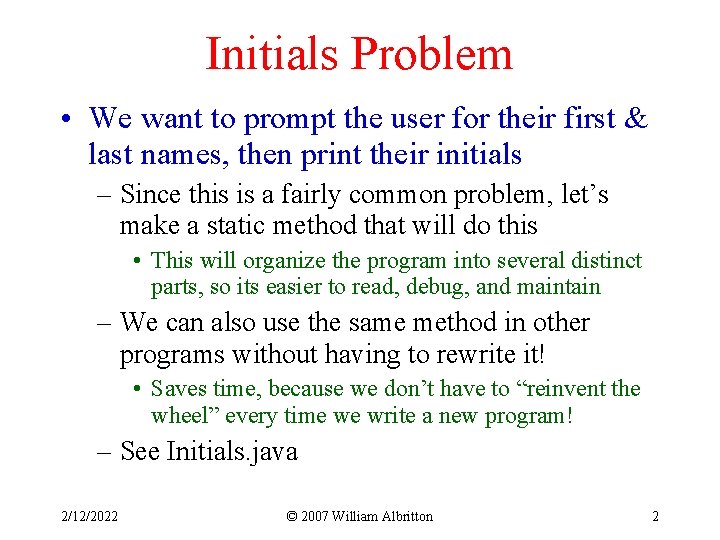
Initials Problem • We want to prompt the user for their first & last names, then print their initials – Since this is a fairly common problem, let’s make a static method that will do this • This will organize the program into several distinct parts, so its easier to read, debug, and maintain – We can also use the same method in other programs without having to rewrite it! • Saves time, because we don’t have to “reinvent the wheel” every time we write a new program! – See Initials. java 2/12/2022 © 2007 William Albritton 2
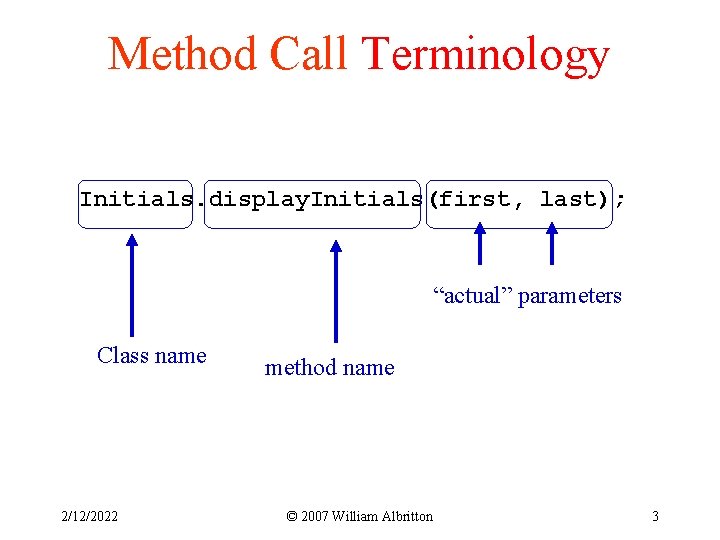
Method Call Terminology Initials. display. Initials(first, last); “actual” parameters Class name 2/12/2022 method name © 2007 William Albritton 3
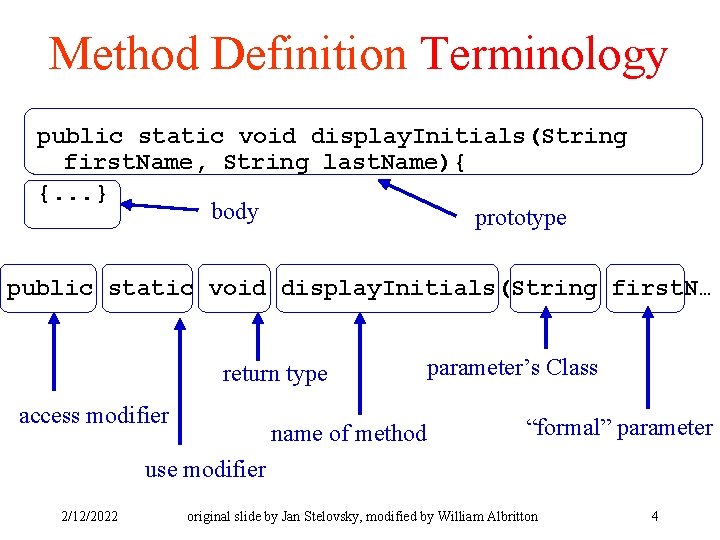
Method Definition Terminology public static void display. Initials(String first. Name, String last. Name){ {. . . } body prototype public static void display. Initials(String first. N… return type access modifier name of method parameter’s Class “formal” parameter use modifier 2/12/2022 original slide by Jan Stelovsky, modified by William Albritton 4
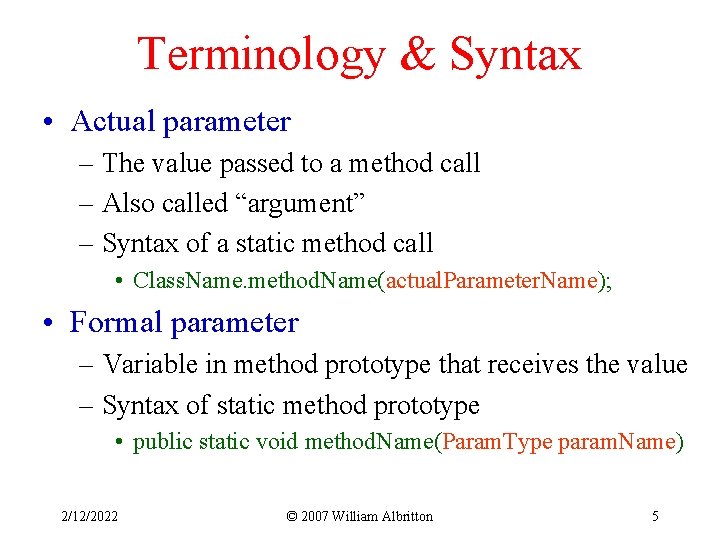
Terminology & Syntax • Actual parameter – The value passed to a method call – Also called “argument” – Syntax of a static method call • Class. Name. method. Name(actual. Parameter. Name); • Formal parameter – Variable in method prototype that receives the value – Syntax of static method prototype • public static void method. Name(Param. Type param. Name) 2/12/2022 © 2007 William Albritton 5
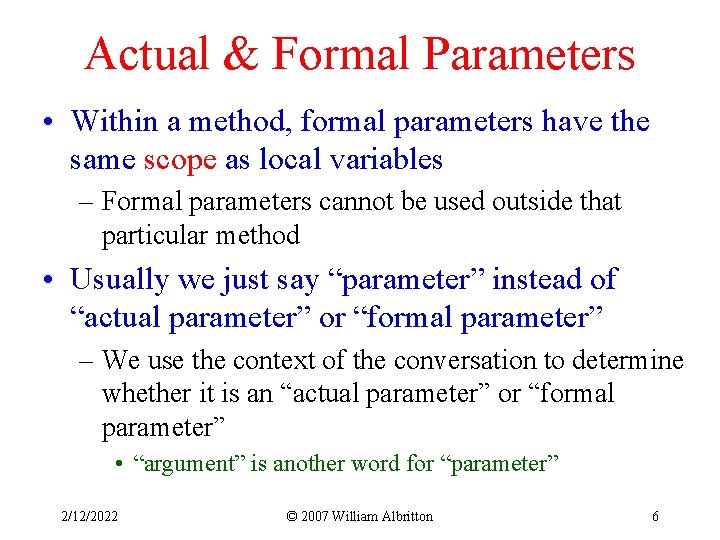
Actual & Formal Parameters • Within a method, formal parameters have the same scope as local variables – Formal parameters cannot be used outside that particular method • Usually we just say “parameter” instead of “actual parameter” or “formal parameter” – We use the context of the conversation to determine whether it is an “actual parameter” or “formal parameter” • “argument” is another word for “parameter” 2/12/2022 © 2007 William Albritton 6
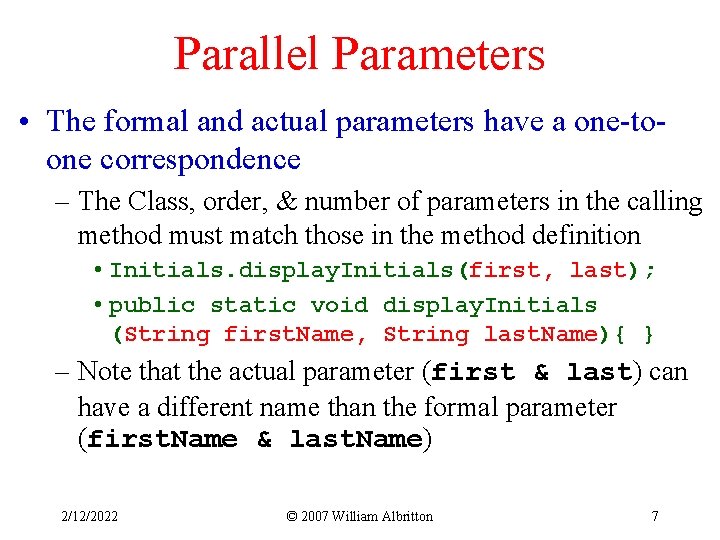
Parallel Parameters • The formal and actual parameters have a one-toone correspondence – The Class, order, & number of parameters in the calling method must match those in the method definition • Initials. display. Initials(first, last); • public static void display. Initials (String first. Name, String last. Name){ } – Note that the actual parameter (first & last) can have a different name than the formal parameter (first. Name & last. Name) 2/12/2022 © 2007 William Albritton 7
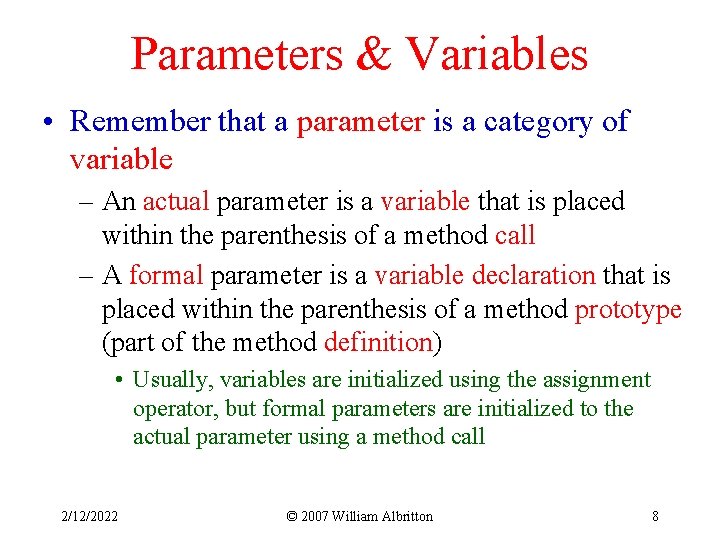
Parameters & Variables • Remember that a parameter is a category of variable – An actual parameter is a variable that is placed within the parenthesis of a method call – A formal parameter is a variable declaration that is placed within the parenthesis of a method prototype (part of the method definition) • Usually, variables are initialized using the assignment operator, but formal parameters are initialized to the actual parameter using a method call 2/12/2022 © 2007 William Albritton 8
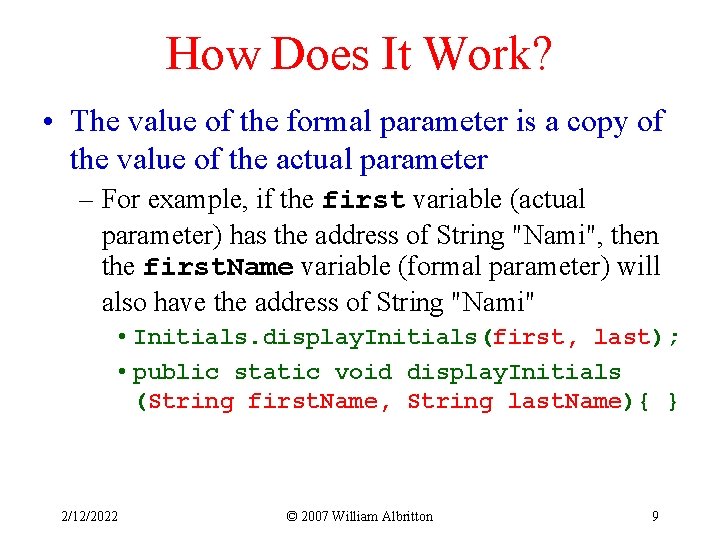
How Does It Work? • The value of the formal parameter is a copy of the value of the actual parameter – For example, if the first variable (actual parameter) has the address of String "Nami", then the first. Name variable (formal parameter) will also have the address of String "Nami" • Initials. display. Initials(first, last); • public static void display. Initials (String first. Name, String last. Name){ } 2/12/2022 © 2007 William Albritton 9
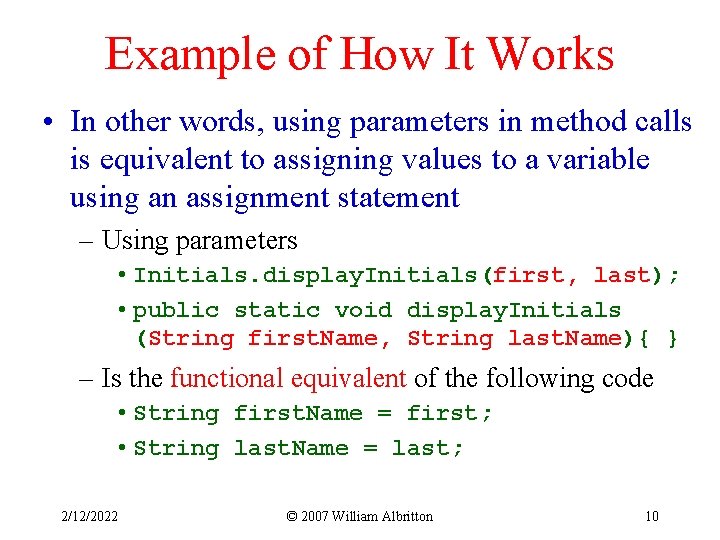
Example of How It Works • In other words, using parameters in method calls is equivalent to assigning values to a variable using an assignment statement – Using parameters • Initials. display. Initials(first, last); • public static void display. Initials (String first. Name, String last. Name){ } – Is the functional equivalent of the following code • String first. Name = first; • String last. Name = last; 2/12/2022 © 2007 William Albritton 10
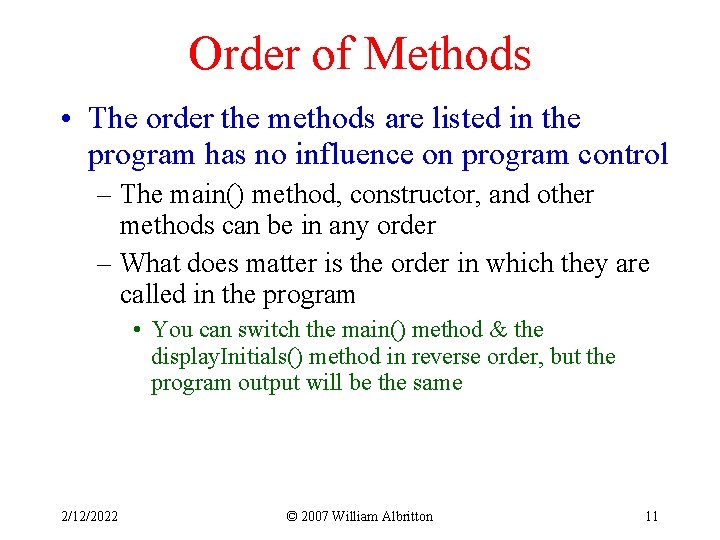
Order of Methods • The order the methods are listed in the program has no influence on program control – The main() method, constructor, and other methods can be in any order – What does matter is the order in which they are called in the program • You can switch the main() method & the display. Initials() method in reverse order, but the program output will be the same 2/12/2022 © 2007 William Albritton 11
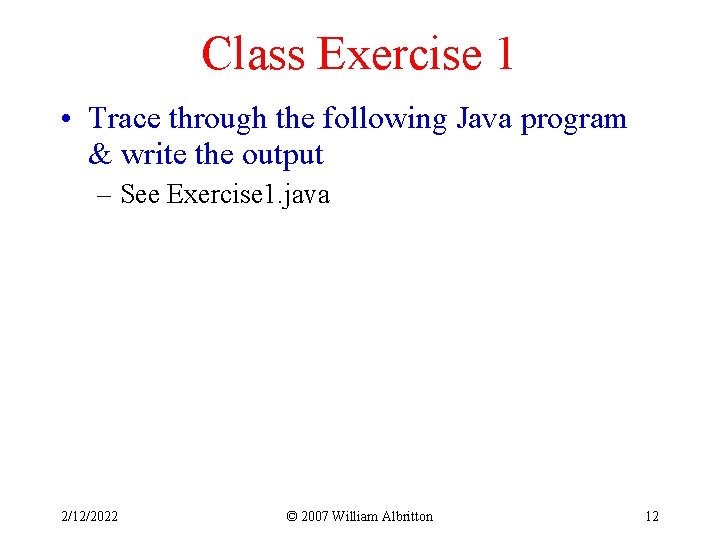
Class Exercise 1 • Trace through the following Java program & write the output – See Exercise 1. java 2/12/2022 © 2007 William Albritton 12
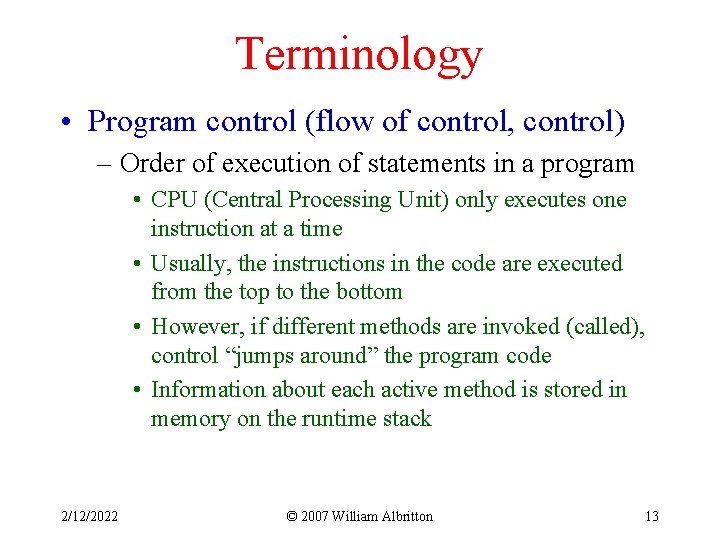
Terminology • Program control (flow of control, control) – Order of execution of statements in a program • CPU (Central Processing Unit) only executes one instruction at a time • Usually, the instructions in the code are executed from the top to the bottom • However, if different methods are invoked (called), control “jumps around” the program code • Information about each active method is stored in memory on the runtime stack 2/12/2022 © 2007 William Albritton 13
![Program Control main method mainString args method 1 other methods method 1 Program Control main() method main(String [] args){ } method 1(); other methods method 1(){](https://slidetodoc.com/presentation_image_h2/b0631efcf04634fd79ad41d9b5520862/image-14.jpg)
Program Control main() method main(String [] args){ } method 1(); other methods method 1(){ } method 2(); See Program. Control. java 2/12/2022 © 2007 William Albritton 14
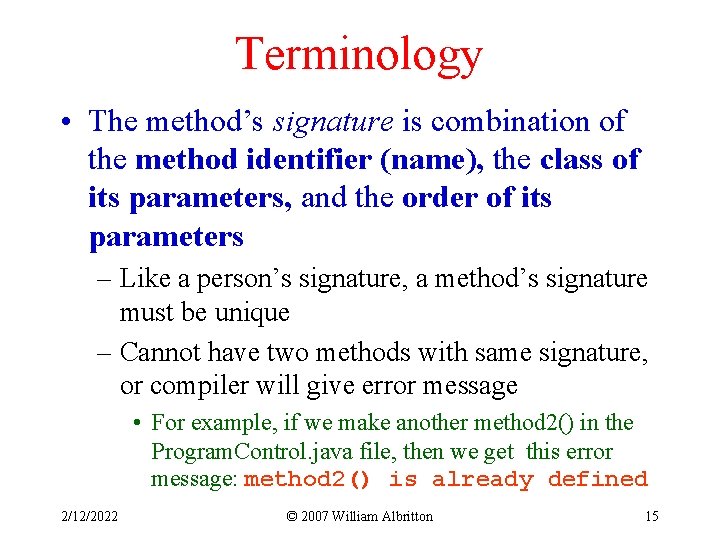
Terminology • The method’s signature is combination of the method identifier (name), the class of its parameters, and the order of its parameters – Like a person’s signature, a method’s signature must be unique – Cannot have two methods with same signature, or compiler will give error message • For example, if we make another method 2() in the Program. Control. java file, then we get this error message: method 2() is already defined 2/12/2022 © 2007 William Albritton 15
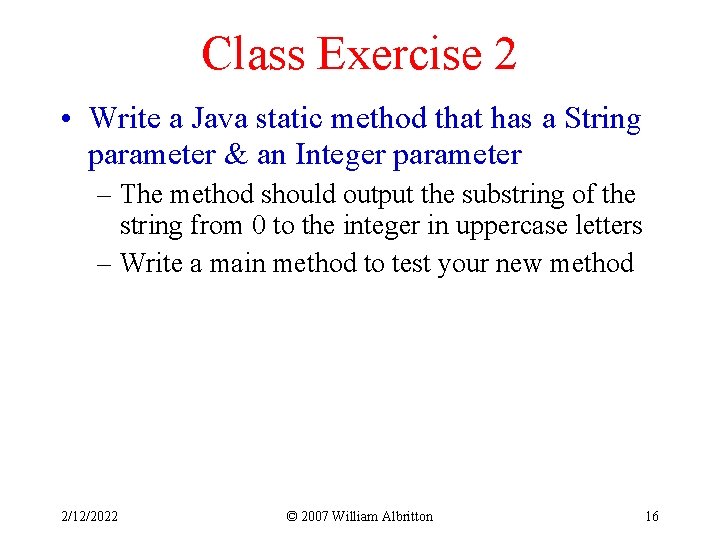
Class Exercise 2 • Write a Java static method that has a String parameter & an Integer parameter – The method should output the substring of the string from 0 to the integer in uppercase letters – Write a main method to test your new method 2/12/2022 © 2007 William Albritton 16
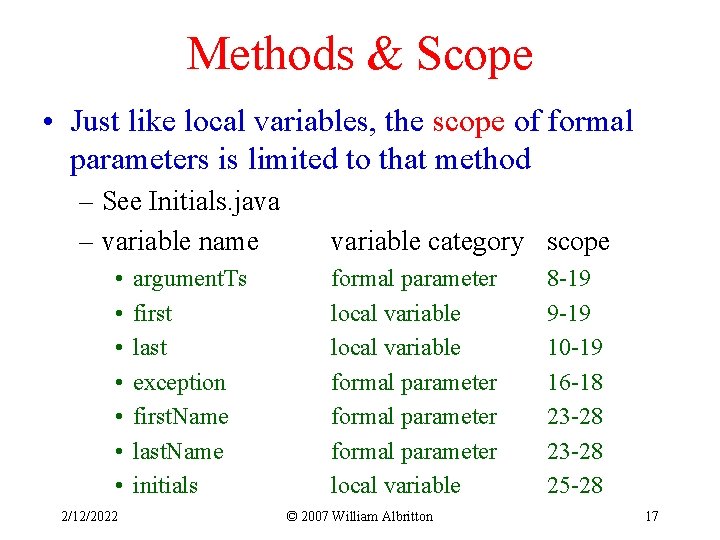
Methods & Scope • Just like local variables, the scope of formal parameters is limited to that method – See Initials. java – variable name • • 2/12/2022 argument. Ts first last exception first. Name last. Name initials variable category scope formal parameter local variable formal parameter local variable © 2007 William Albritton 8 -19 9 -19 10 -19 16 -18 23 -28 25 -28 17
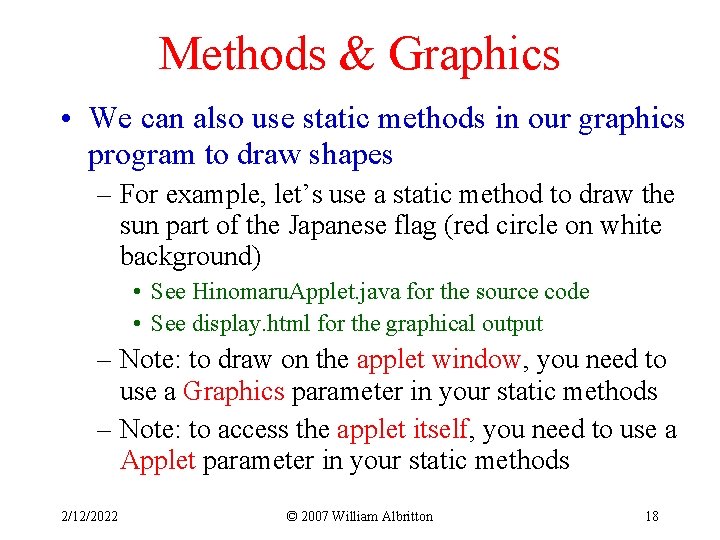
Methods & Graphics • We can also use static methods in our graphics program to draw shapes – For example, let’s use a static method to draw the sun part of the Japanese flag (red circle on white background) • See Hinomaru. Applet. java for the source code • See display. html for the graphical output – Note: to draw on the applet window, you need to use a Graphics parameter in your static methods – Note: to access the applet itself, you need to use a Applet parameter in your static methods 2/12/2022 © 2007 William Albritton 18
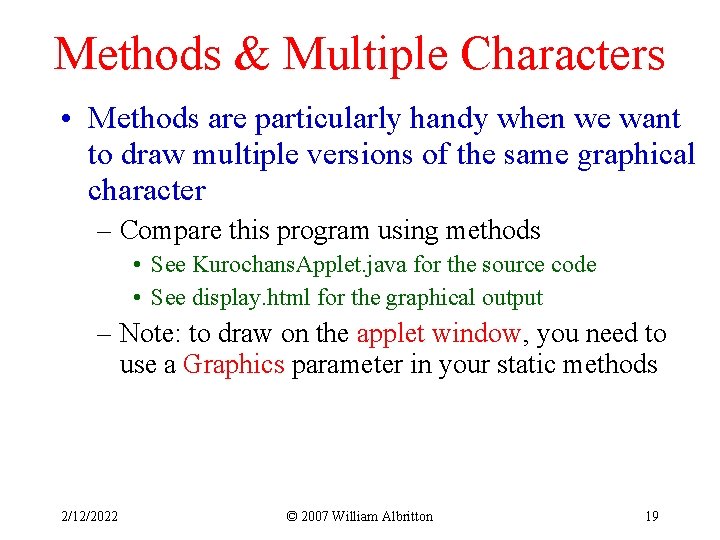
Methods & Multiple Characters • Methods are particularly handy when we want to draw multiple versions of the same graphical character – Compare this program using methods • See Kurochans. Applet. java for the source code • See display. html for the graphical output – Note: to draw on the applet window, you need to use a Graphics parameter in your static methods 2/12/2022 © 2007 William Albritton 19
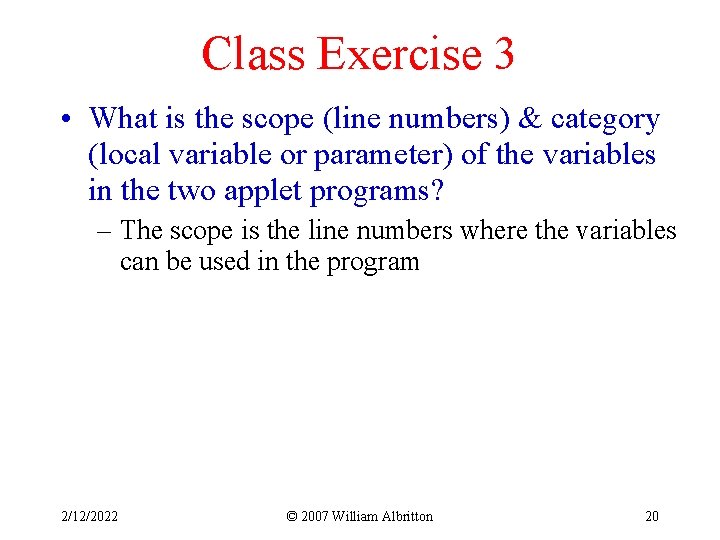
Class Exercise 3 • What is the scope (line numbers) & category (local variable or parameter) of the variables in the two applet programs? – The scope is the line numbers where the variables can be used in the program 2/12/2022 © 2007 William Albritton 20
011 101 001
Ics 111
Ics 111
Ics 111
Ics 400: advanced ics for complex incidents-aberdeen
Scientific methods in computer science
My favorite subject in school is science
It 111 introduction to computing
This is the midpoint inside the ellipse
K5 think central
Introduction to computer science midterm exam
Introduction to computer science midterm exam test
C++ code
Python programming an introduction to computer science
Wax pattern in dentistry
Qualitative analysis political science
What is science lesson 1
Research methods in exercise science
Dda algorithm advantages and disadvantages
Logical classification of input devices
Computer architecture performance evaluation methods