File IO ICS 111 Introduction to Computer Science
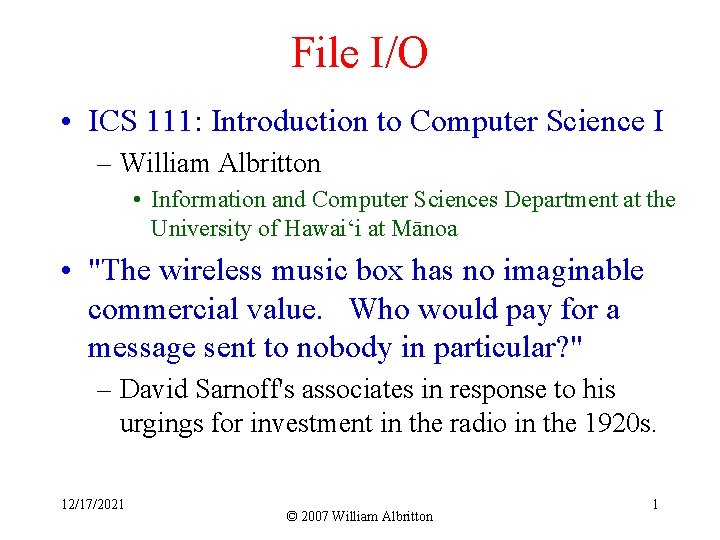
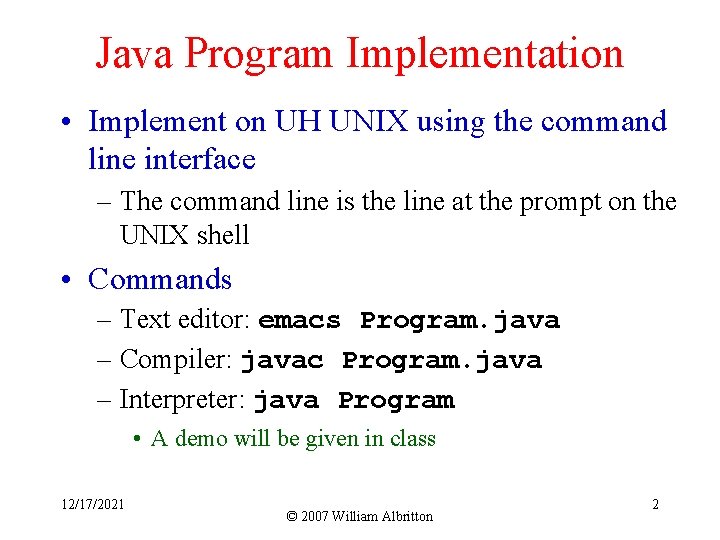
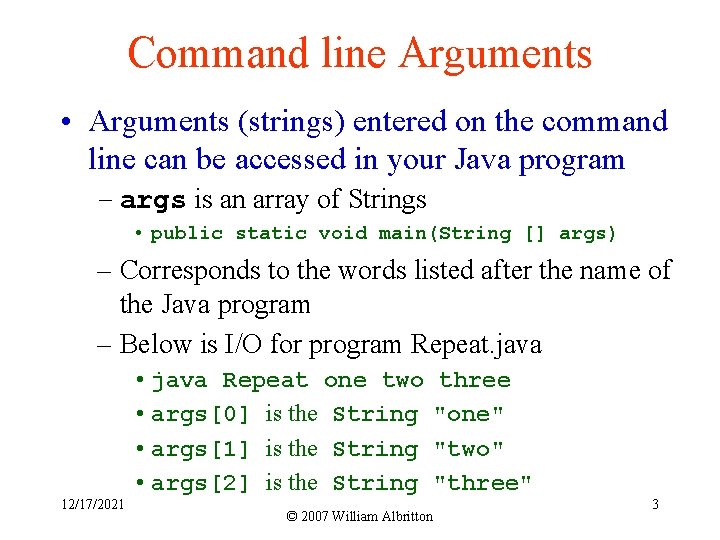
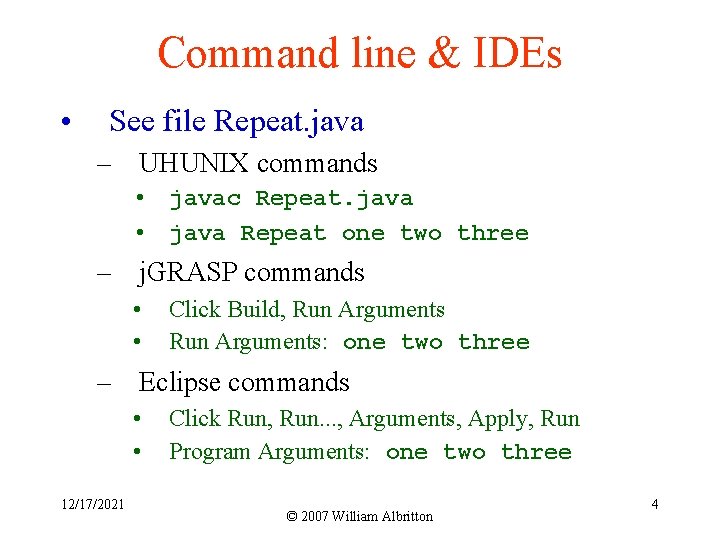
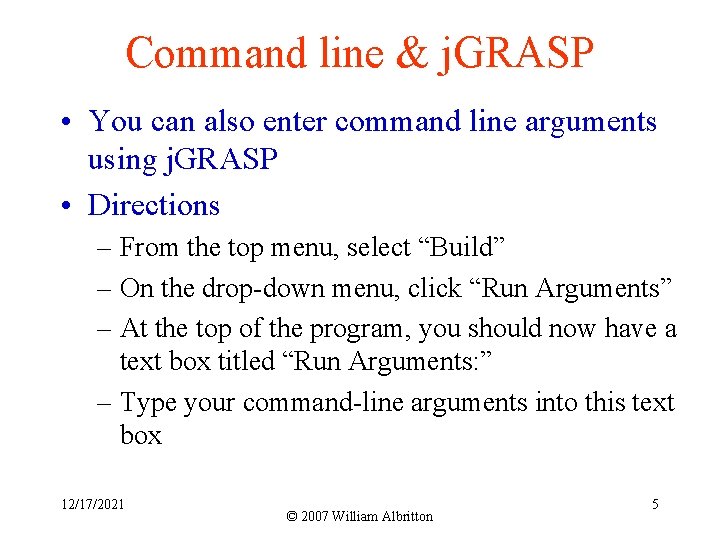
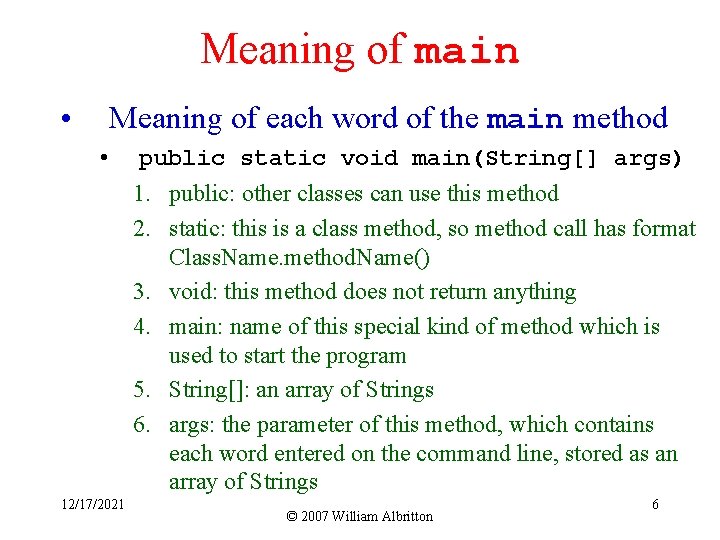
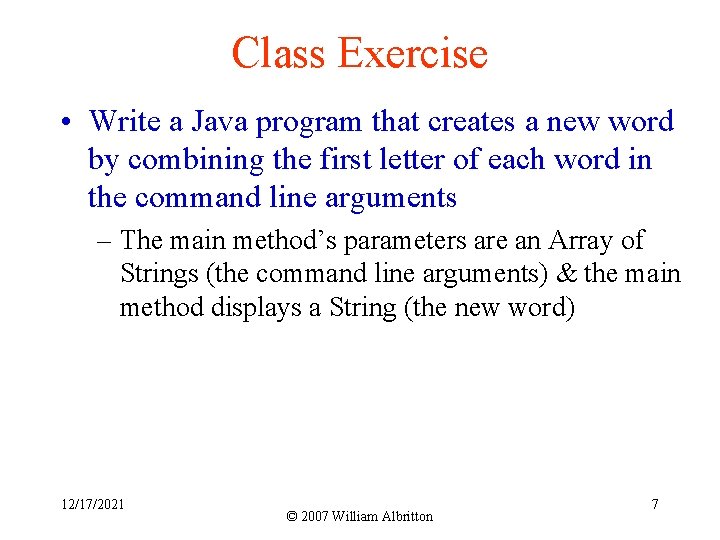
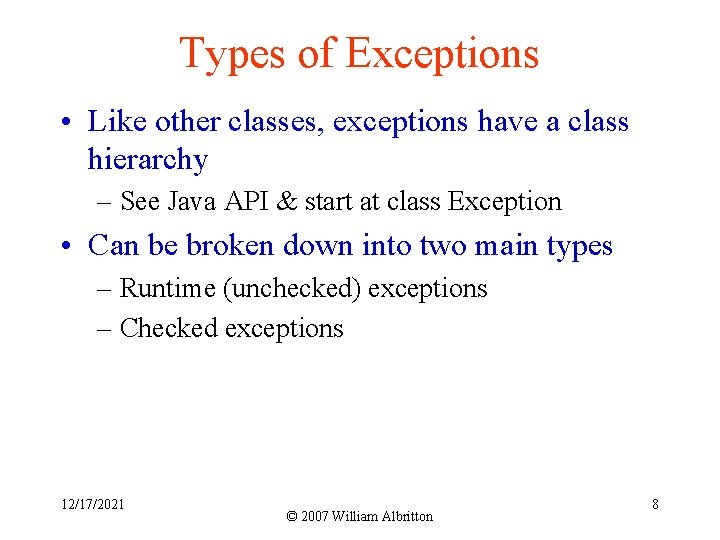
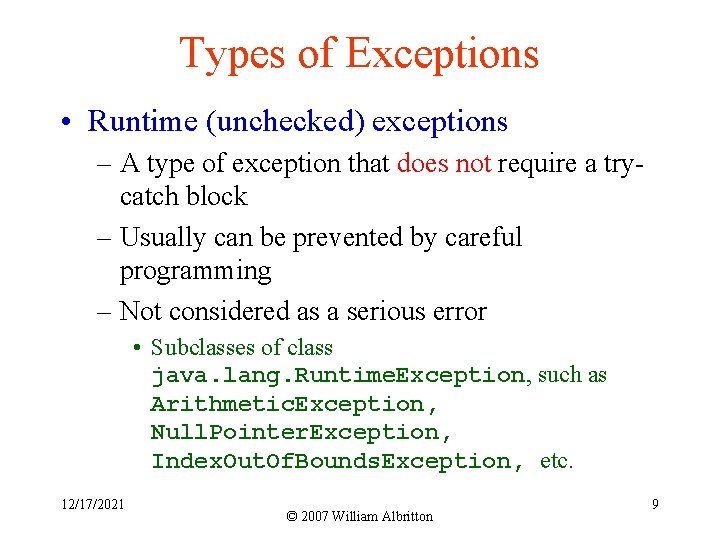
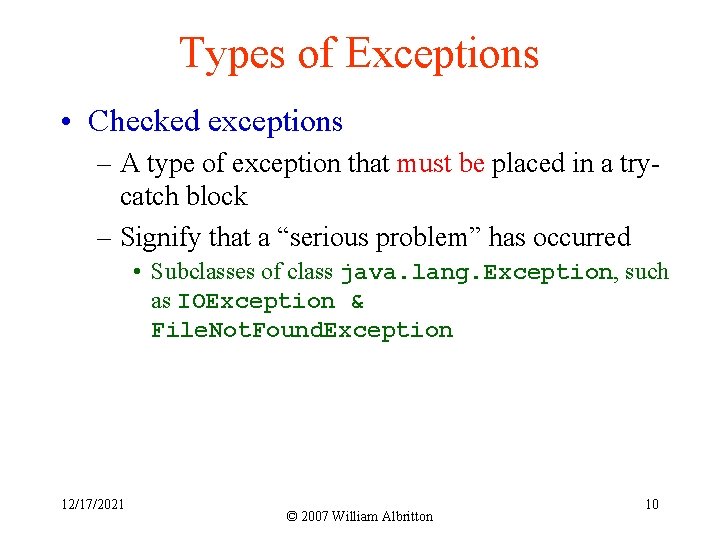
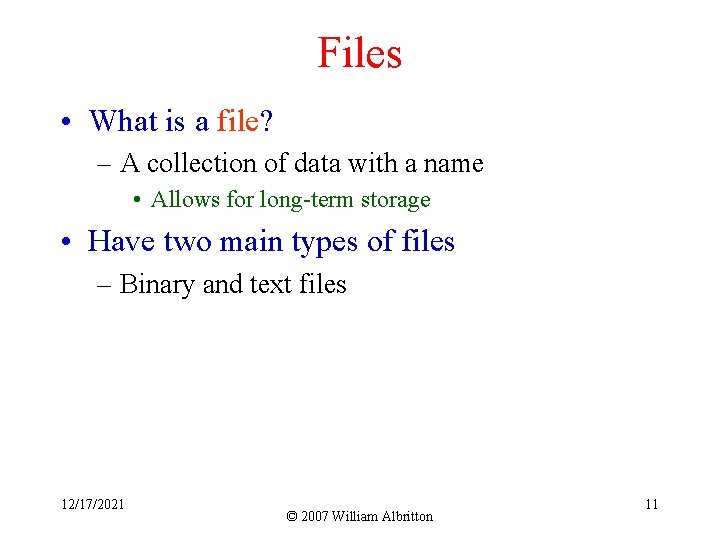
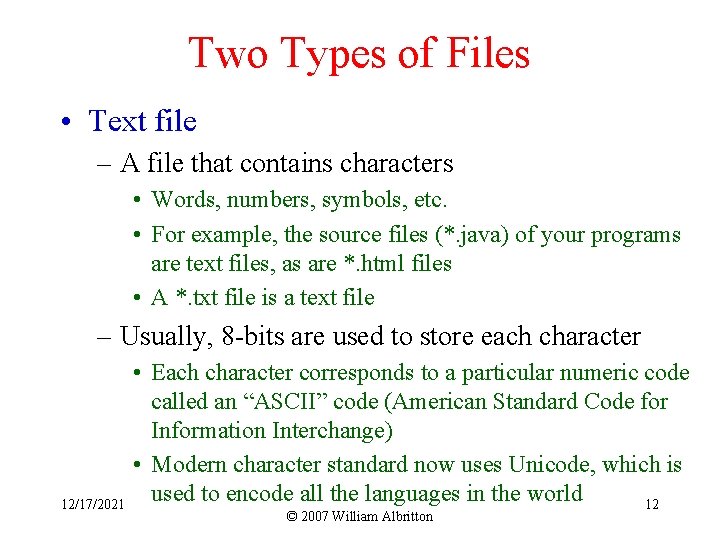
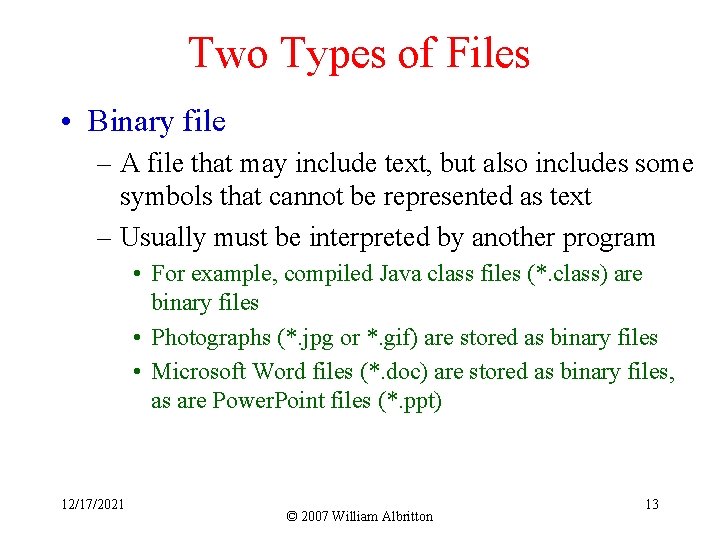
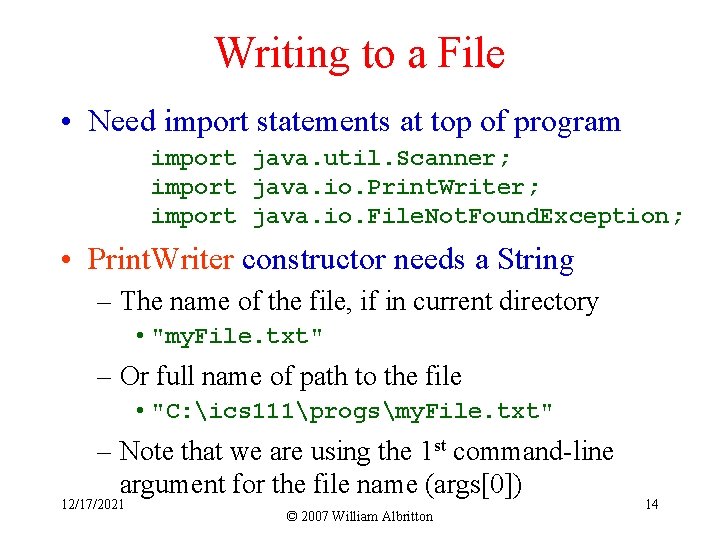
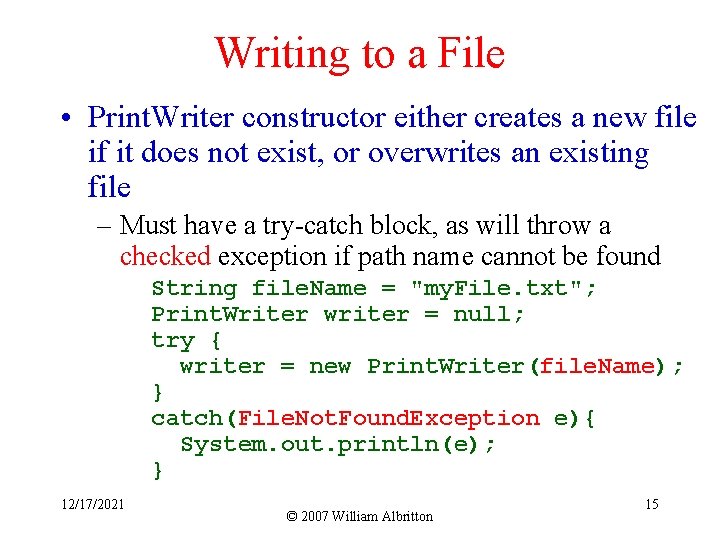
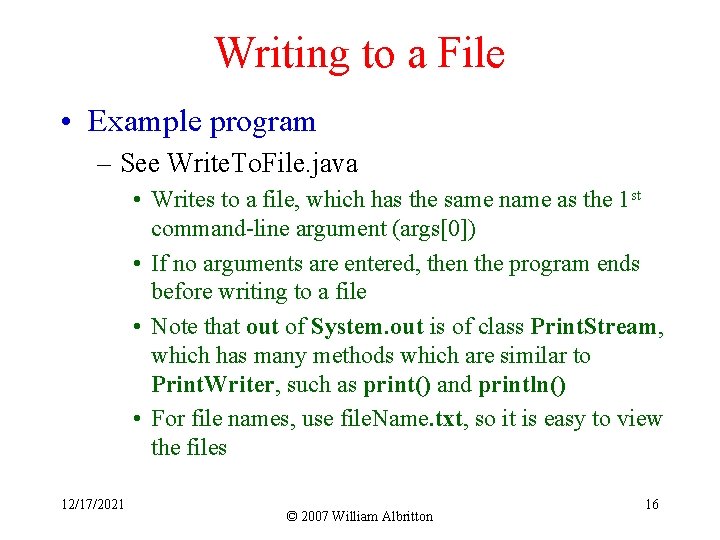
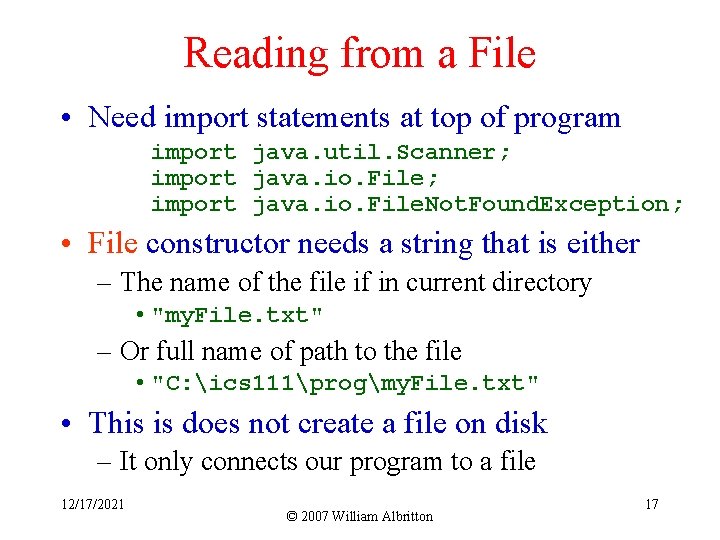
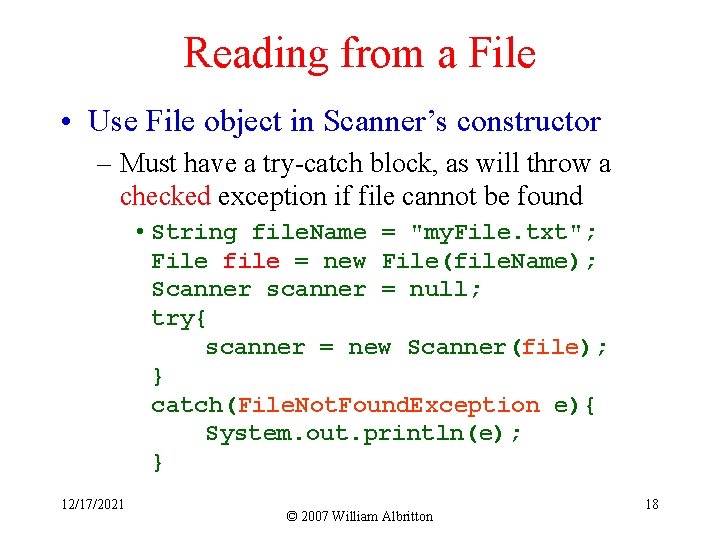
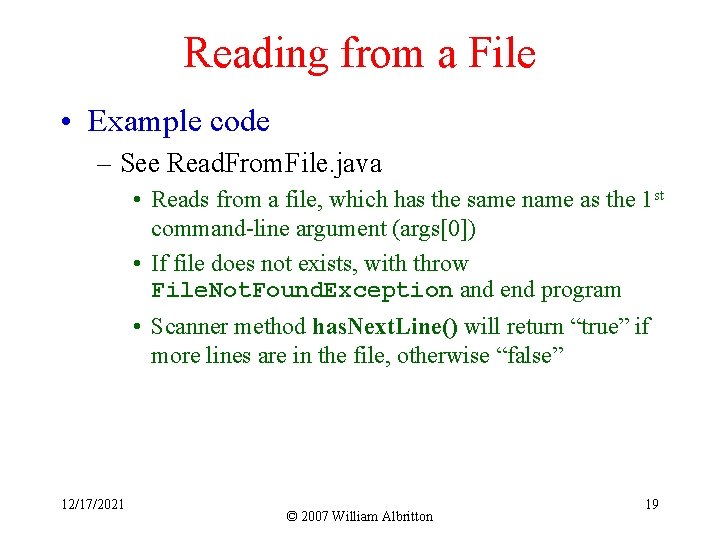
- Slides: 19
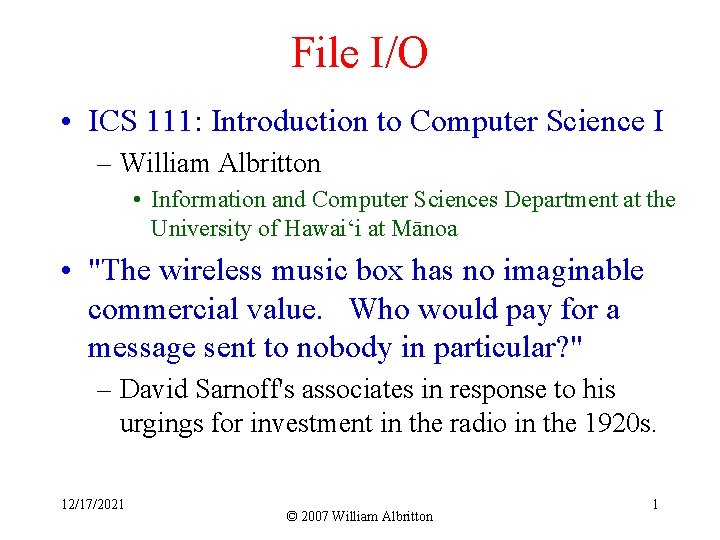
File I/O • ICS 111: Introduction to Computer Science I – William Albritton • Information and Computer Sciences Department at the University of Hawai‘i at Mānoa • "The wireless music box has no imaginable commercial value. Who would pay for a message sent to nobody in particular? " – David Sarnoff's associates in response to his urgings for investment in the radio in the 1920 s. 12/17/2021 © 2007 William Albritton 1
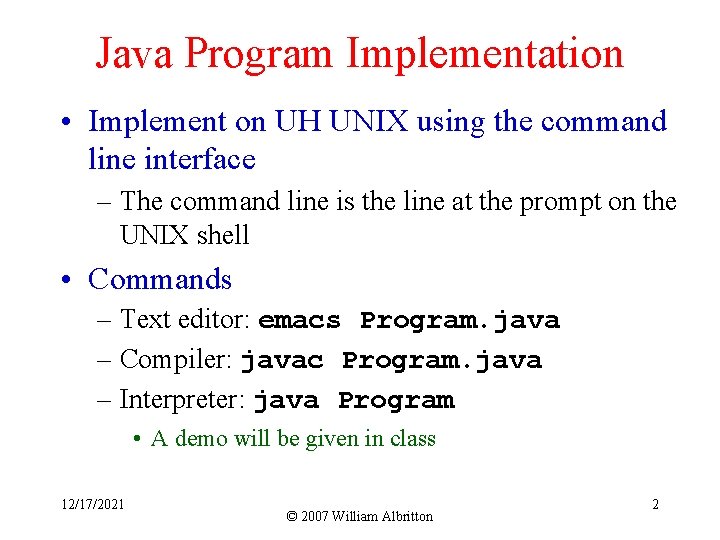
Java Program Implementation • Implement on UH UNIX using the command line interface – The command line is the line at the prompt on the UNIX shell • Commands – Text editor: emacs Program. java – Compiler: javac Program. java – Interpreter: java Program • A demo will be given in class 12/17/2021 © 2007 William Albritton 2
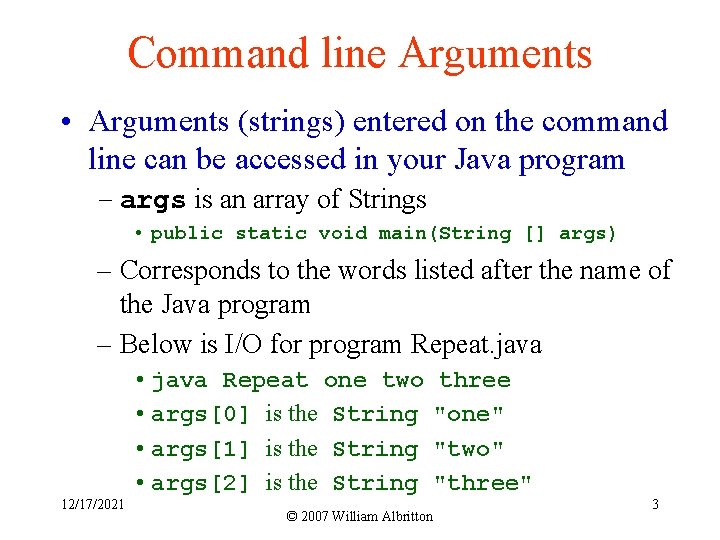
Command line Arguments • Arguments (strings) entered on the command line can be accessed in your Java program – args is an array of Strings • public static void main(String [] args) – Corresponds to the words listed after the name of the Java program – Below is I/O for program Repeat. java 12/17/2021 • java Repeat one two • args[0] is the String • args[1] is the String • args[2] is the String three "one" "two" "three" © 2007 William Albritton 3
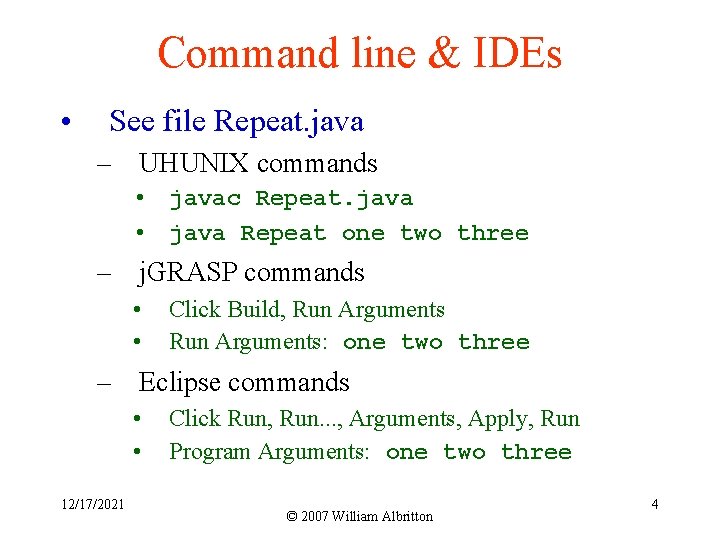
Command line & IDEs • See file Repeat. java – UHUNIX commands • javac Repeat. java • java Repeat one two three – j. GRASP commands • • Click Build, Run Arguments: one two three – Eclipse commands • • 12/17/2021 Click Run, Run. . . , Arguments, Apply, Run Program Arguments: one two three © 2007 William Albritton 4
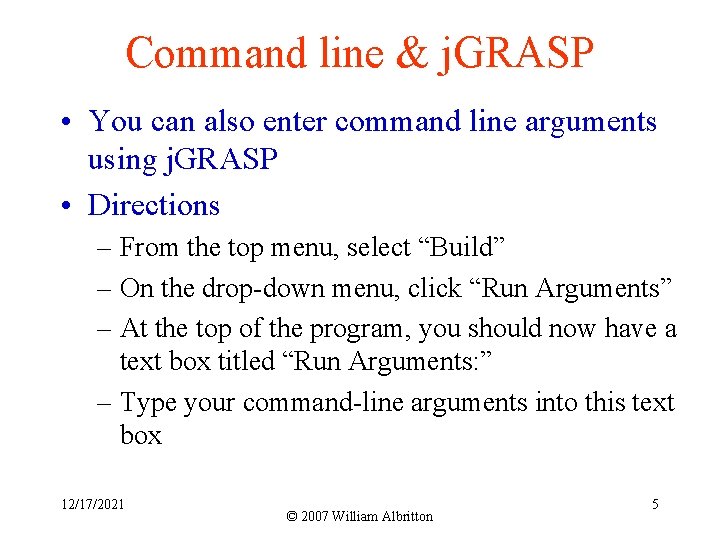
Command line & j. GRASP • You can also enter command line arguments using j. GRASP • Directions – From the top menu, select “Build” – On the drop-down menu, click “Run Arguments” – At the top of the program, you should now have a text box titled “Run Arguments: ” – Type your command-line arguments into this text box 12/17/2021 © 2007 William Albritton 5
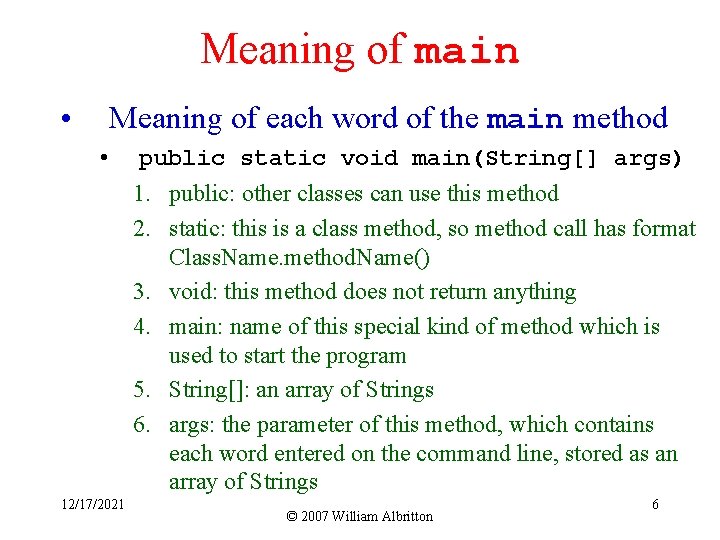
Meaning of main • Meaning of each word of the main method • public static void main(String[] args) 1. public: other classes can use this method 2. static: this is a class method, so method call has format Class. Name. method. Name() 3. void: this method does not return anything 4. main: name of this special kind of method which is used to start the program 5. String[]: an array of Strings 6. args: the parameter of this method, which contains each word entered on the command line, stored as an array of Strings 12/17/2021 © 2007 William Albritton 6
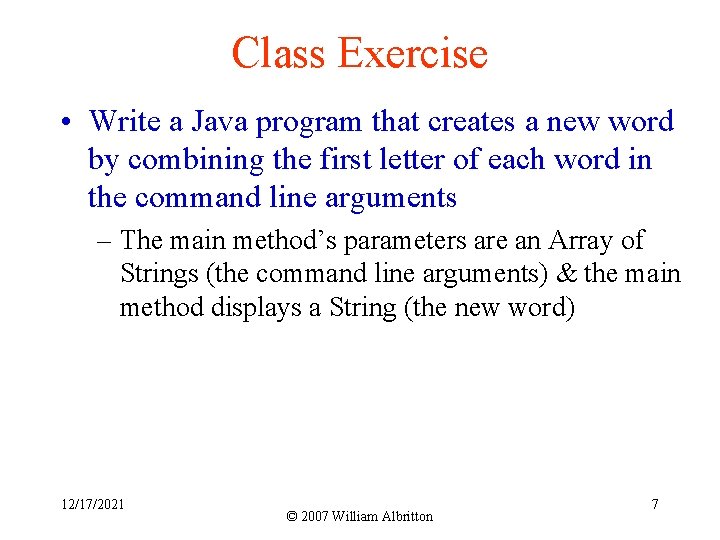
Class Exercise • Write a Java program that creates a new word by combining the first letter of each word in the command line arguments – The main method’s parameters are an Array of Strings (the command line arguments) & the main method displays a String (the new word) 12/17/2021 © 2007 William Albritton 7
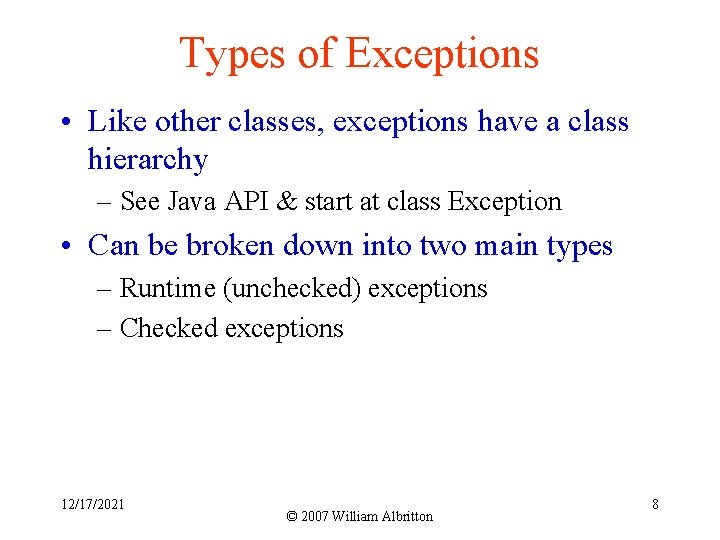
Types of Exceptions • Like other classes, exceptions have a class hierarchy – See Java API & start at class Exception • Can be broken down into two main types – Runtime (unchecked) exceptions – Checked exceptions 12/17/2021 © 2007 William Albritton 8
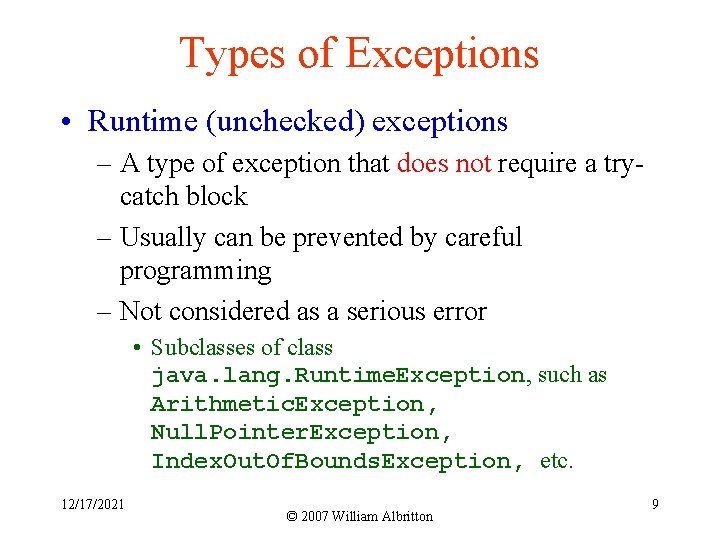
Types of Exceptions • Runtime (unchecked) exceptions – A type of exception that does not require a trycatch block – Usually can be prevented by careful programming – Not considered as a serious error • Subclasses of class java. lang. Runtime. Exception, such as Arithmetic. Exception, Null. Pointer. Exception, Index. Out. Of. Bounds. Exception, etc. 12/17/2021 © 2007 William Albritton 9
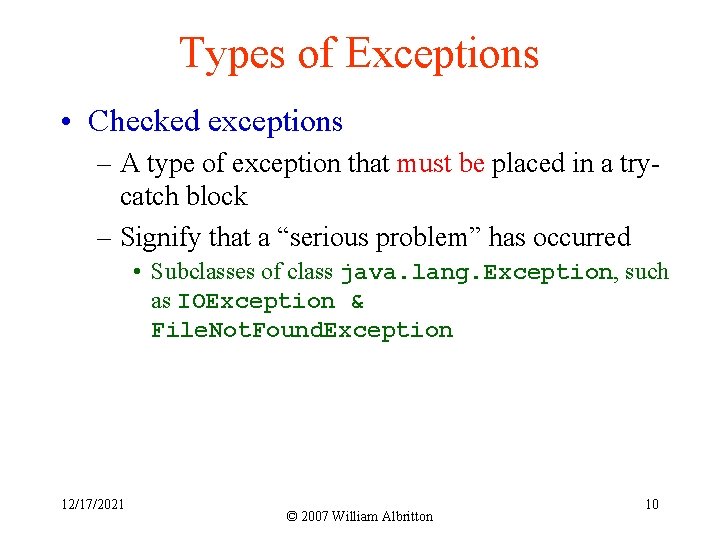
Types of Exceptions • Checked exceptions – A type of exception that must be placed in a trycatch block – Signify that a “serious problem” has occurred • Subclasses of class java. lang. Exception, such as IOException & File. Not. Found. Exception 12/17/2021 © 2007 William Albritton 10
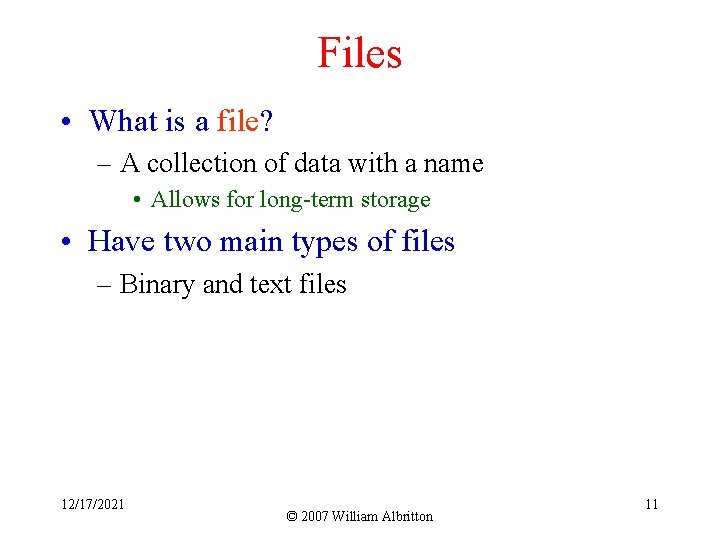
Files • What is a file? – A collection of data with a name • Allows for long-term storage • Have two main types of files – Binary and text files 12/17/2021 © 2007 William Albritton 11
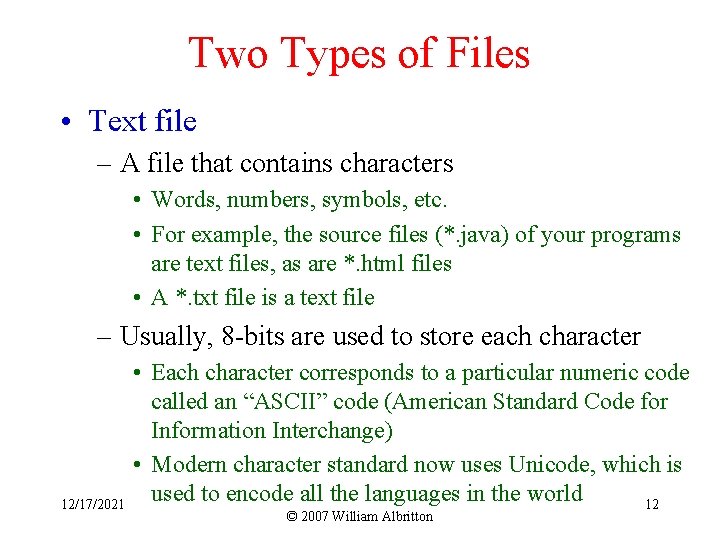
Two Types of Files • Text file – A file that contains characters • Words, numbers, symbols, etc. • For example, the source files (*. java) of your programs are text files, as are *. html files • A *. txt file is a text file – Usually, 8 -bits are used to store each character 12/17/2021 • Each character corresponds to a particular numeric code called an “ASCII” code (American Standard Code for Information Interchange) • Modern character standard now uses Unicode, which is used to encode all the languages in the world 12 © 2007 William Albritton
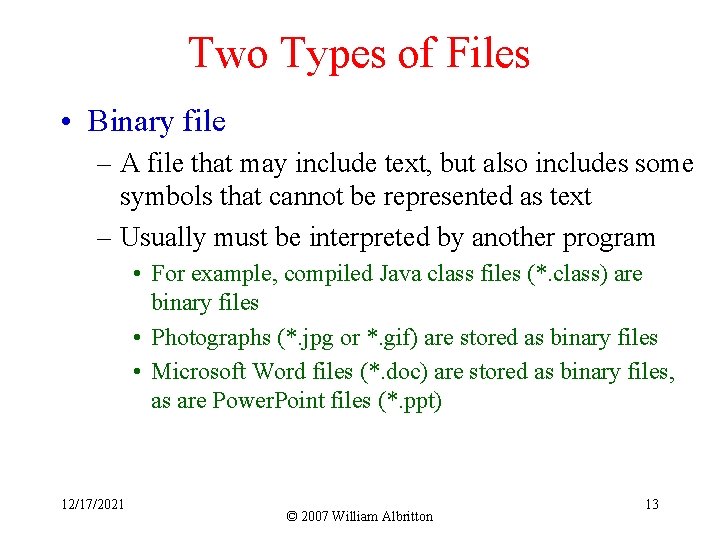
Two Types of Files • Binary file – A file that may include text, but also includes some symbols that cannot be represented as text – Usually must be interpreted by another program • For example, compiled Java class files (*. class) are binary files • Photographs (*. jpg or *. gif) are stored as binary files • Microsoft Word files (*. doc) are stored as binary files, as are Power. Point files (*. ppt) 12/17/2021 © 2007 William Albritton 13
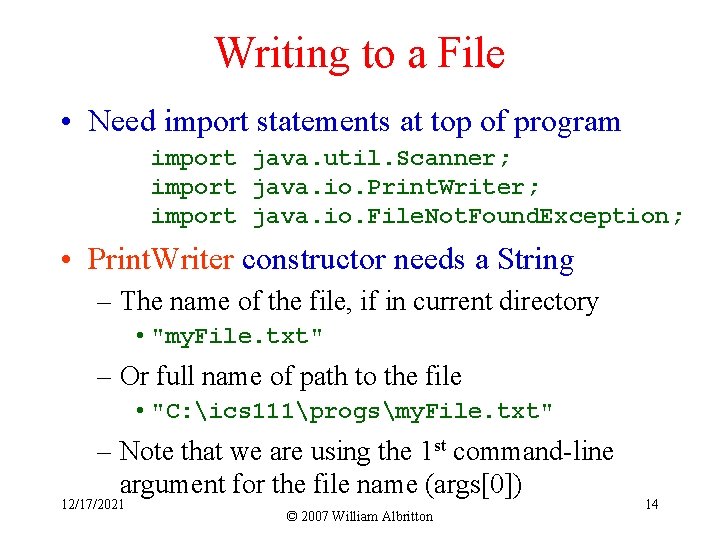
Writing to a File • Need import statements at top of program import java. util. Scanner; import java. io. Print. Writer; import java. io. File. Not. Found. Exception; • Print. Writer constructor needs a String – The name of the file, if in current directory • "my. File. txt" – Or full name of path to the file • "C: ics 111progsmy. File. txt" – Note that we are using the 1 st command-line argument for the file name (args[0]) 12/17/2021 © 2007 William Albritton 14
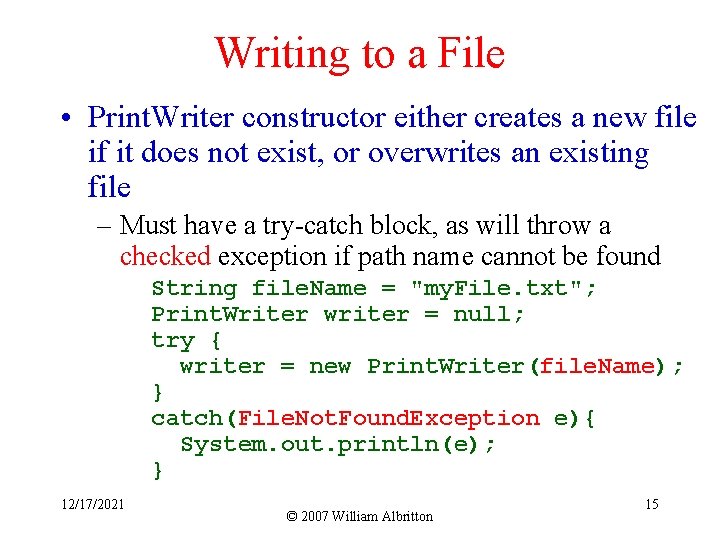
Writing to a File • Print. Writer constructor either creates a new file if it does not exist, or overwrites an existing file – Must have a try-catch block, as will throw a checked exception if path name cannot be found String file. Name = "my. File. txt"; Print. Writer writer = null; try { writer = new Print. Writer(file. Name); } catch(File. Not. Found. Exception e){ System. out. println(e); } 12/17/2021 © 2007 William Albritton 15
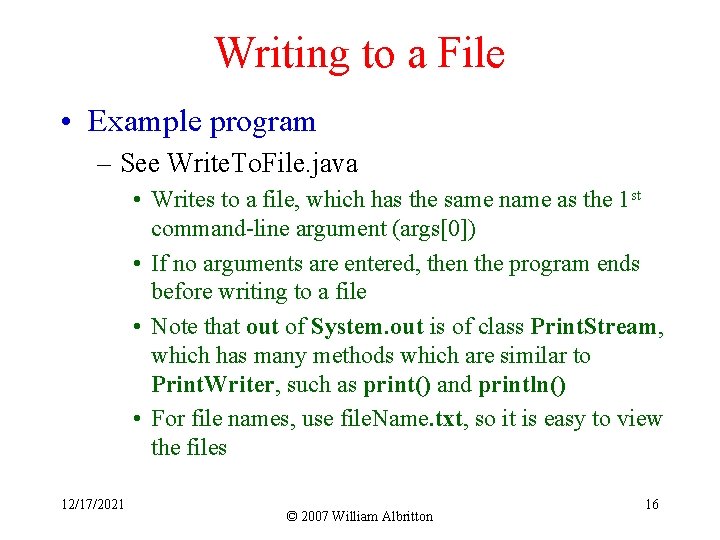
Writing to a File • Example program – See Write. To. File. java • Writes to a file, which has the same name as the 1 st command-line argument (args[0]) • If no arguments are entered, then the program ends before writing to a file • Note that out of System. out is of class Print. Stream, which has many methods which are similar to Print. Writer, such as print() and println() • For file names, use file. Name. txt, so it is easy to view the files 12/17/2021 © 2007 William Albritton 16
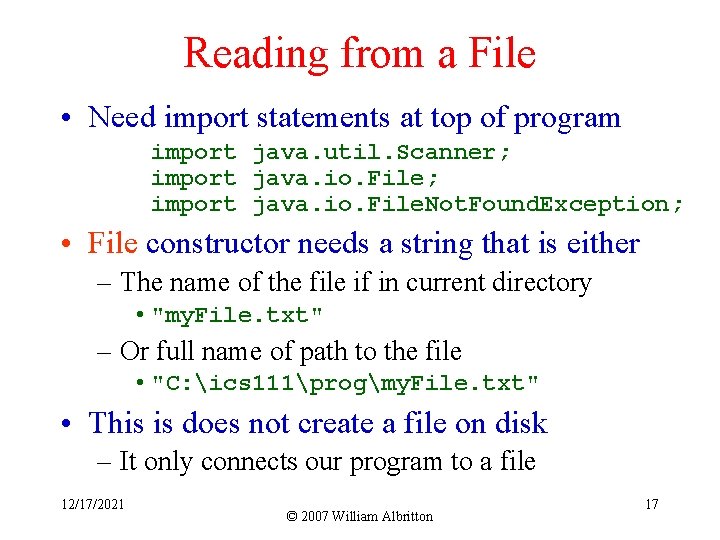
Reading from a File • Need import statements at top of program import java. util. Scanner; import java. io. File. Not. Found. Exception; • File constructor needs a string that is either – The name of the file if in current directory • "my. File. txt" – Or full name of path to the file • "C: ics 111progmy. File. txt" • This is does not create a file on disk – It only connects our program to a file 12/17/2021 © 2007 William Albritton 17
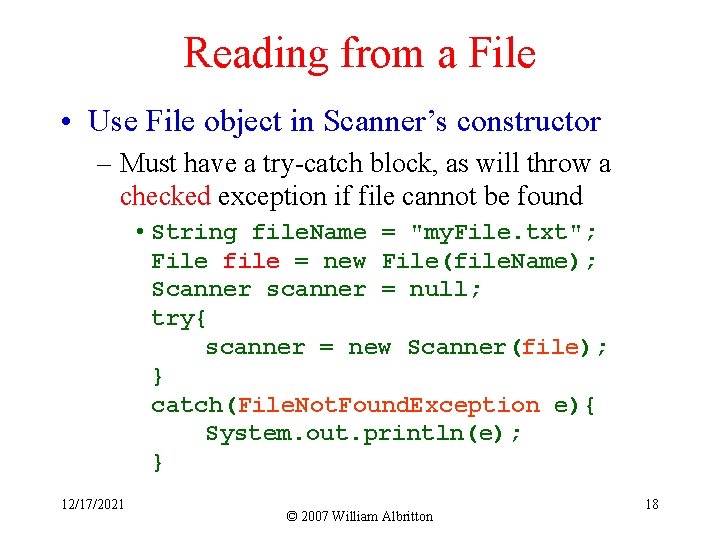
Reading from a File • Use File object in Scanner’s constructor – Must have a try-catch block, as will throw a checked exception if file cannot be found • String file. Name = "my. File. txt"; File file = new File(file. Name); Scanner scanner = null; try{ scanner = new Scanner(file); } catch(File. Not. Found. Exception e){ System. out. println(e); } 12/17/2021 © 2007 William Albritton 18
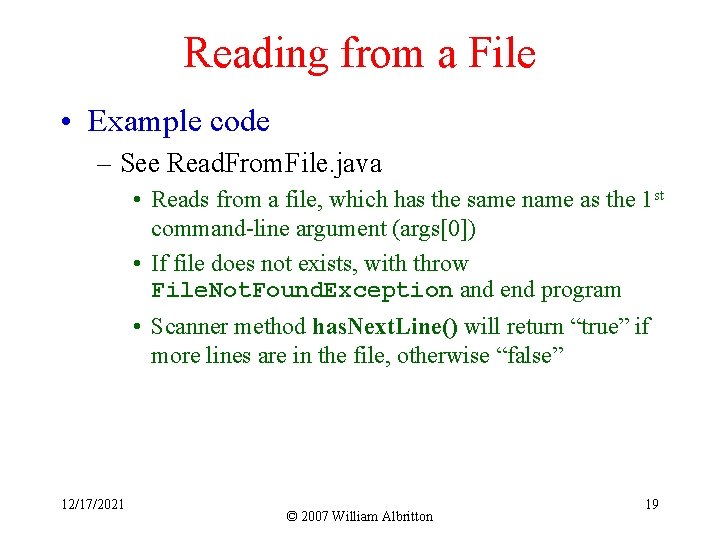
Reading from a File • Example code – See Read. From. File. java • Reads from a file, which has the same name as the 1 st command-line argument (args[0]) • If file does not exists, with throw File. Not. Found. Exception and end program • Scanner method has. Next. Line() will return “true” if more lines are in the file, otherwise “false” 12/17/2021 © 2007 William Albritton 19