Arrays ICS 111 Introduction to Computer Science I
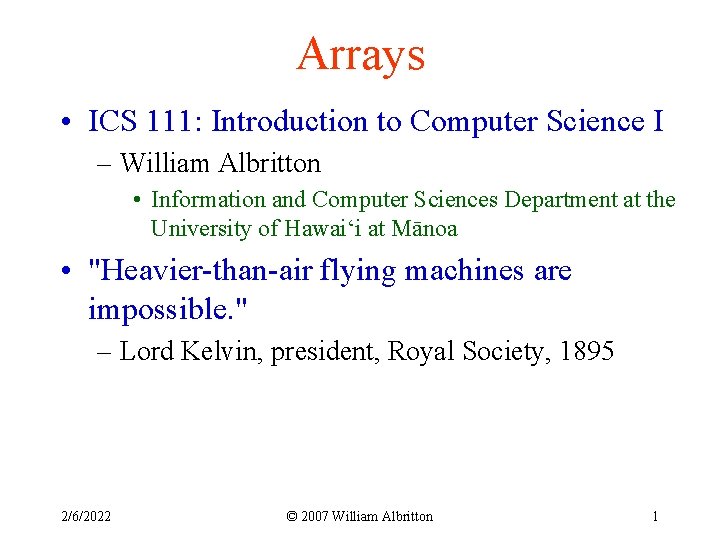
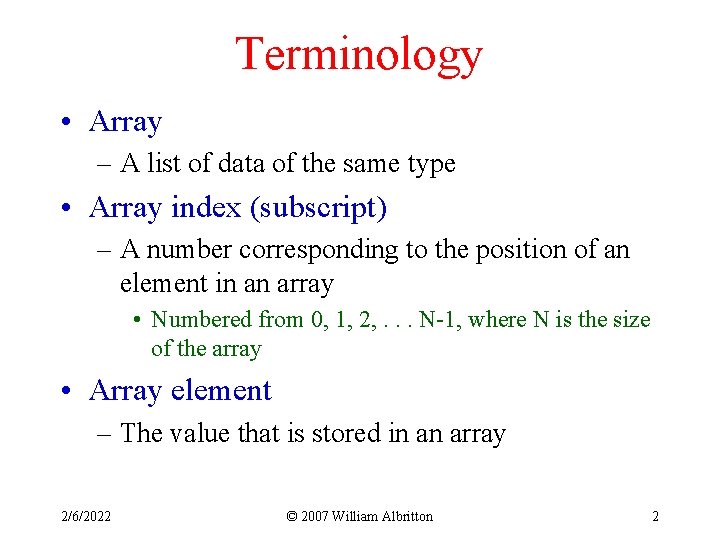
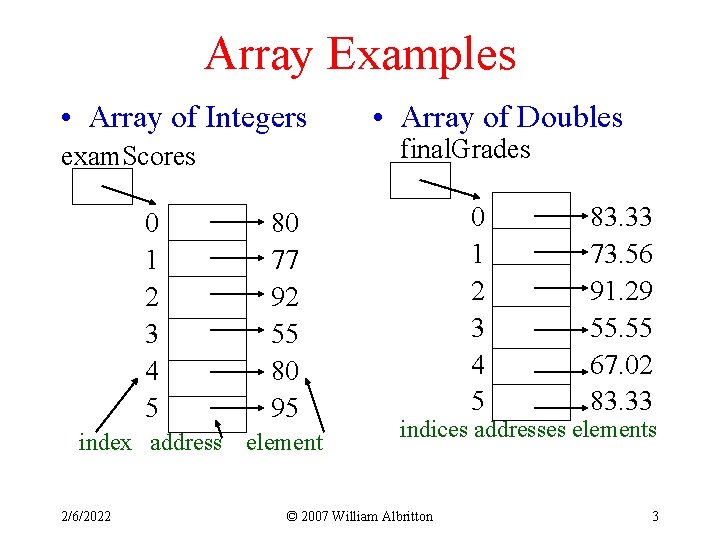
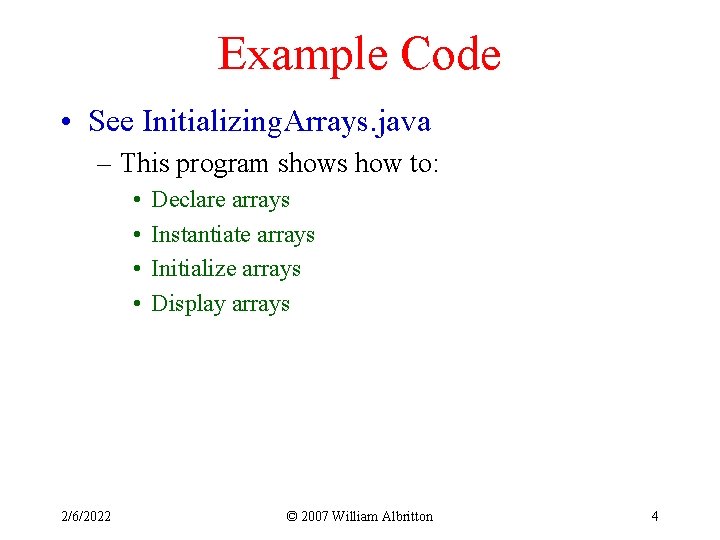
![Declaring Arrays • Syntax for an array declaration – Data. Type [] array. Name; Declaring Arrays • Syntax for an array declaration – Data. Type [] array. Name;](https://slidetodoc.com/presentation_image_h2/a6e266d1c2642b6cca1fc85e4bf71d5e/image-5.jpg)
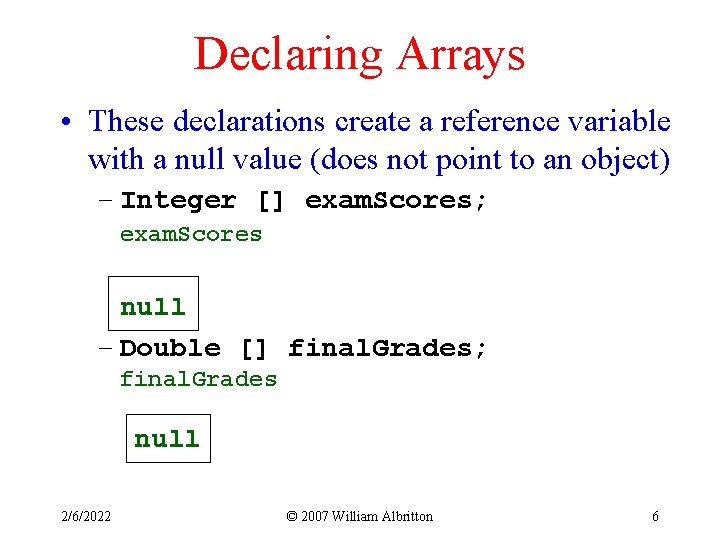
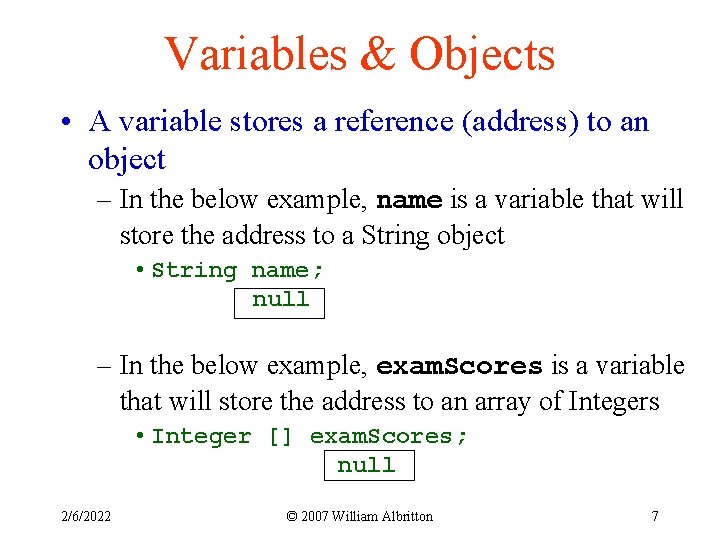
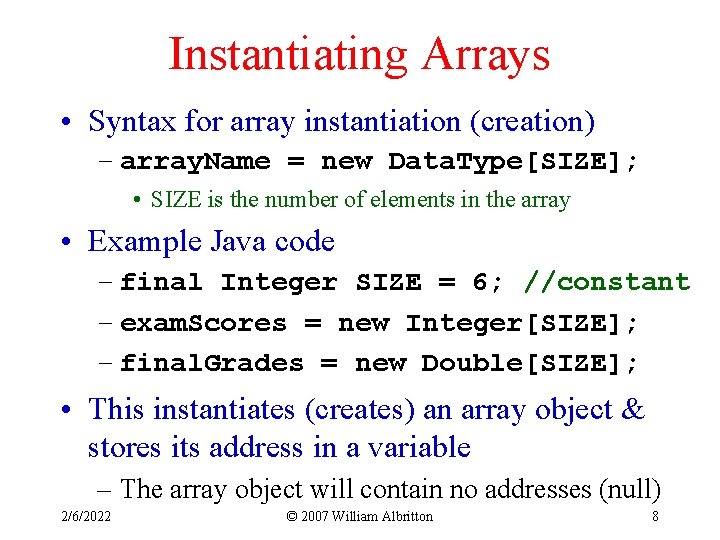
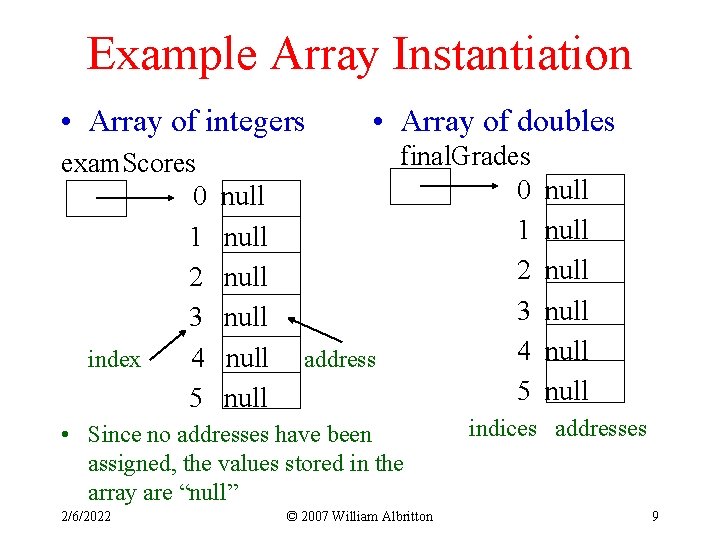
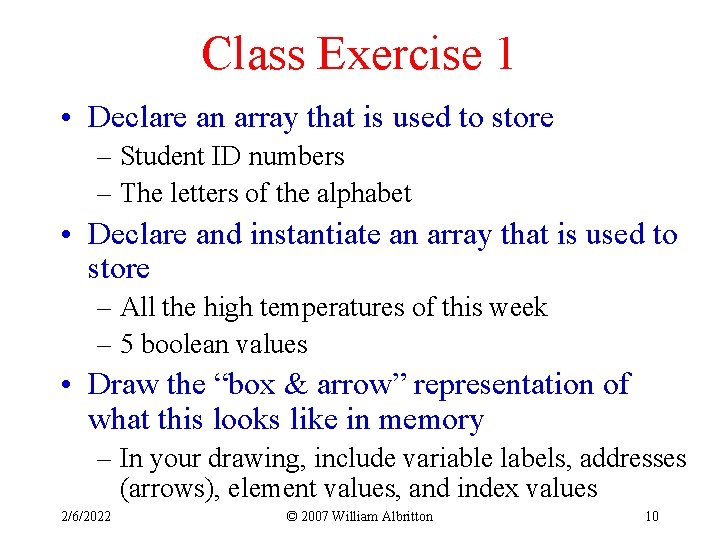
![Initializing Arrays • Syntax for initializing arrays – array. Name[i] = value; • Where Initializing Arrays • Syntax for initializing arrays – array. Name[i] = value; • Where](https://slidetodoc.com/presentation_image_h2/a6e266d1c2642b6cca1fc85e4bf71d5e/image-11.jpg)
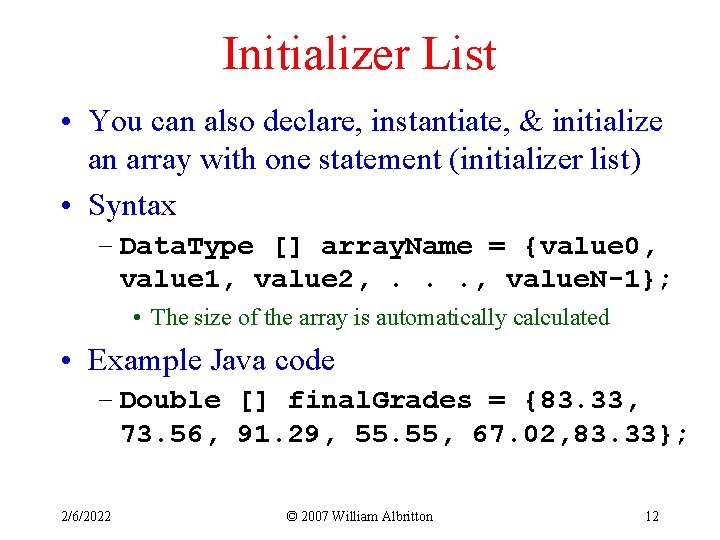
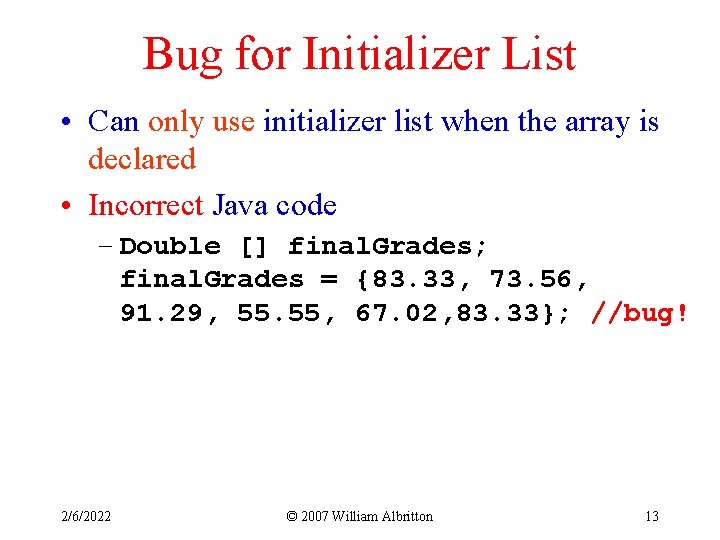
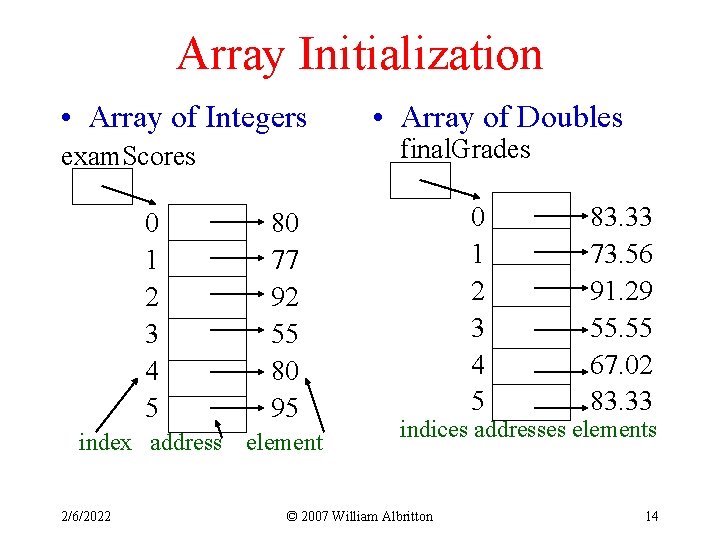
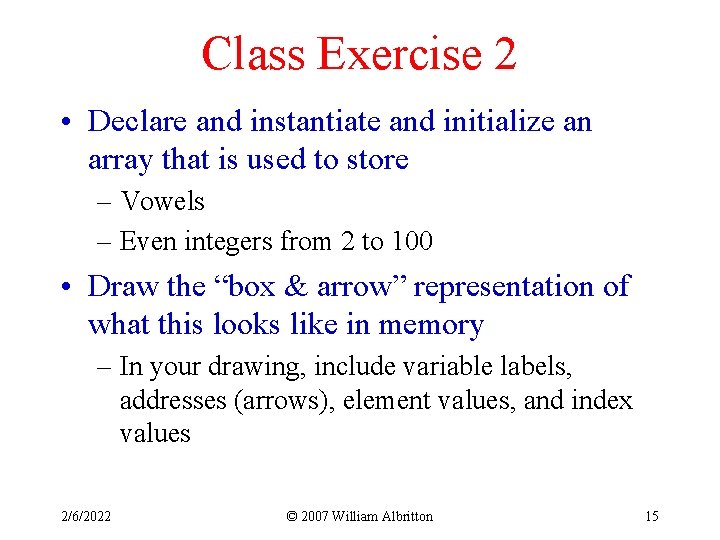
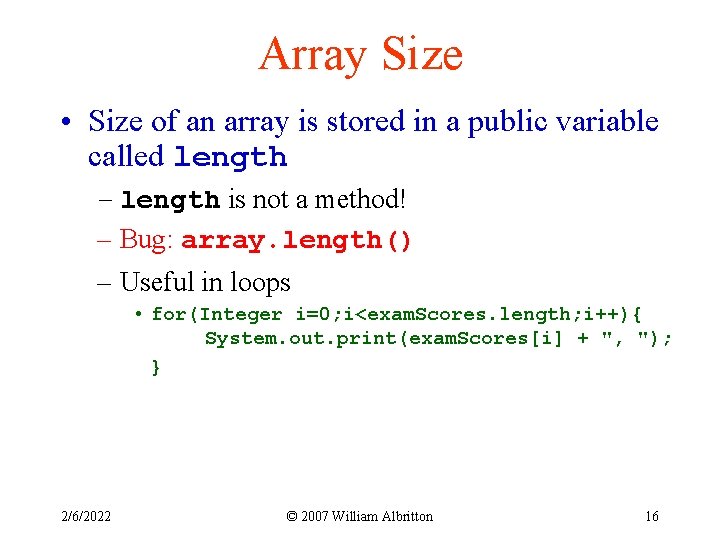
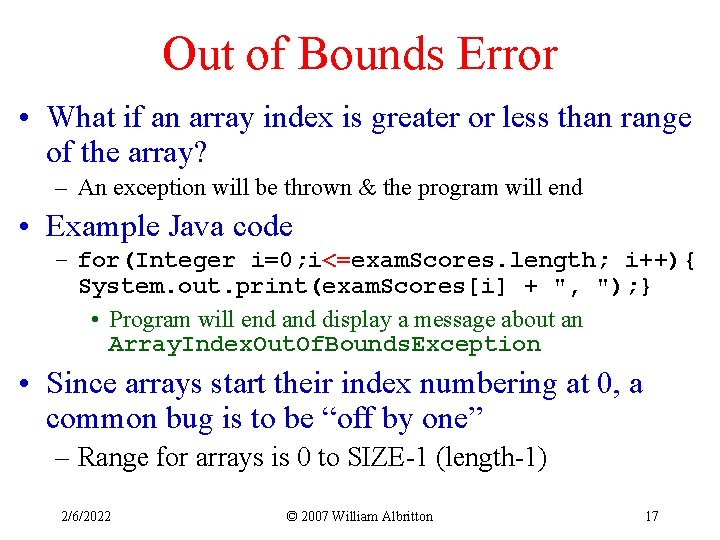
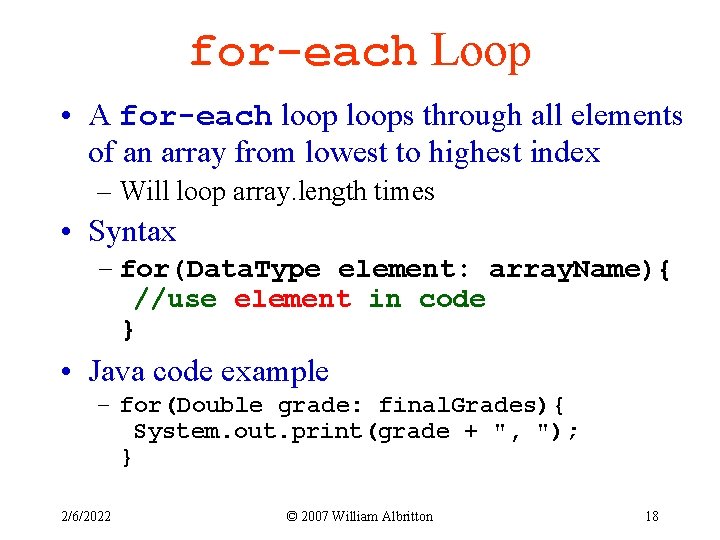
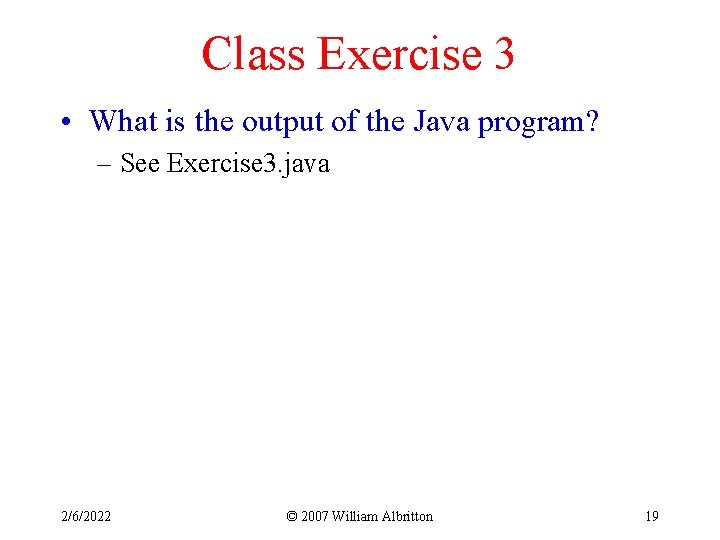
- Slides: 19
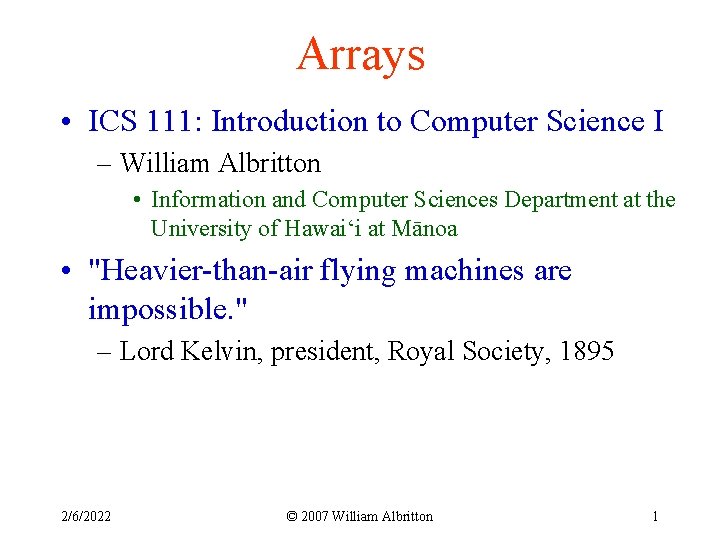
Arrays • ICS 111: Introduction to Computer Science I – William Albritton • Information and Computer Sciences Department at the University of Hawai‘i at Mānoa • "Heavier-than-air flying machines are impossible. " – Lord Kelvin, president, Royal Society, 1895 2/6/2022 © 2007 William Albritton 1
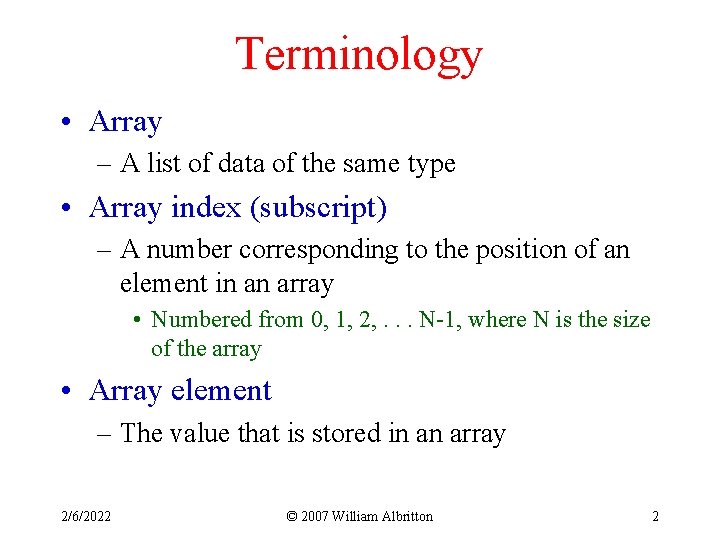
Terminology • Array – A list of data of the same type • Array index (subscript) – A number corresponding to the position of an element in an array • Numbered from 0, 1, 2, . . . N-1, where N is the size of the array • Array element – The value that is stored in an array 2/6/2022 © 2007 William Albritton 2
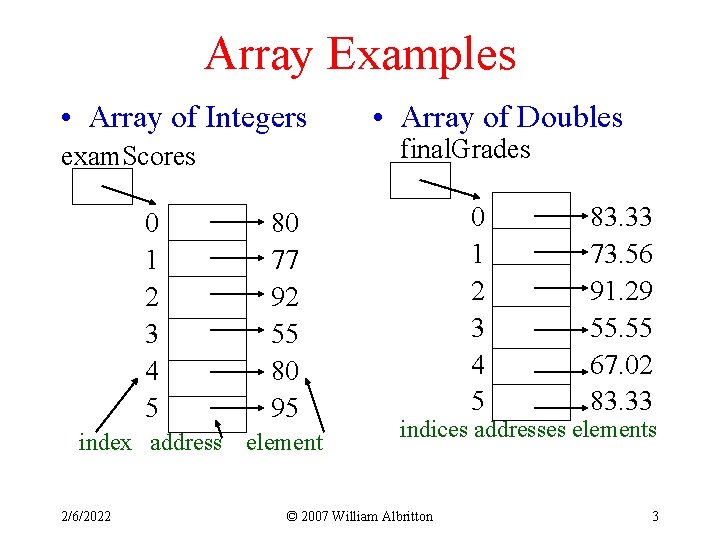
Array Examples • Array of Integers exam. Scores 0 1 2 3 4 5 80 77 92 55 80 95 index address element 2/6/2022 • Array of Doubles final. Grades 0 1 2 3 4 5 83. 33 73. 56 91. 29 55. 55 67. 02 83. 33 indices addresses elements © 2007 William Albritton 3
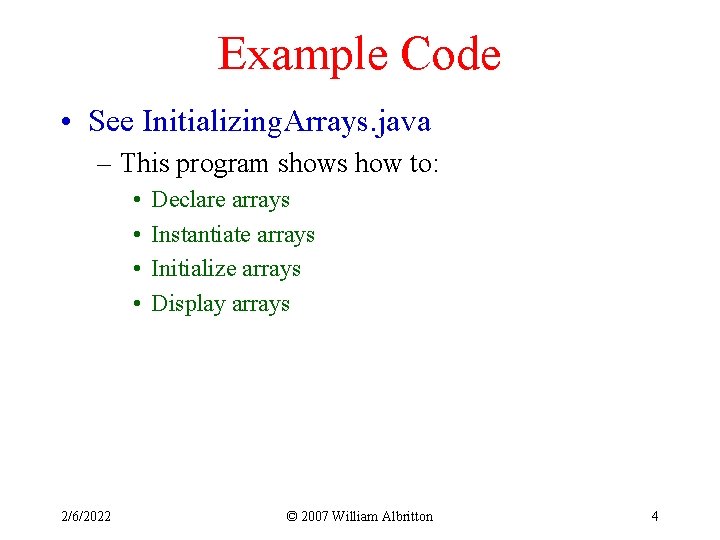
Example Code • See Initializing. Arrays. java – This program shows how to: • • 2/6/2022 Declare arrays Instantiate arrays Initialize arrays Display arrays © 2007 William Albritton 4
![Declaring Arrays Syntax for an array declaration Data Type array Name Declaring Arrays • Syntax for an array declaration – Data. Type [] array. Name;](https://slidetodoc.com/presentation_image_h2/a6e266d1c2642b6cca1fc85e4bf71d5e/image-5.jpg)
Declaring Arrays • Syntax for an array declaration – Data. Type [] array. Name; • Example Java code – Integer [] exam. Scores; – Double [] final. Grades; • A declaration creates a variable with the address to an array, but not the array itself – Since arrays are objects in Java, you must declare an array variable (which will later contain the address to the location of the array object) 2/6/2022 © 2007 William Albritton 5
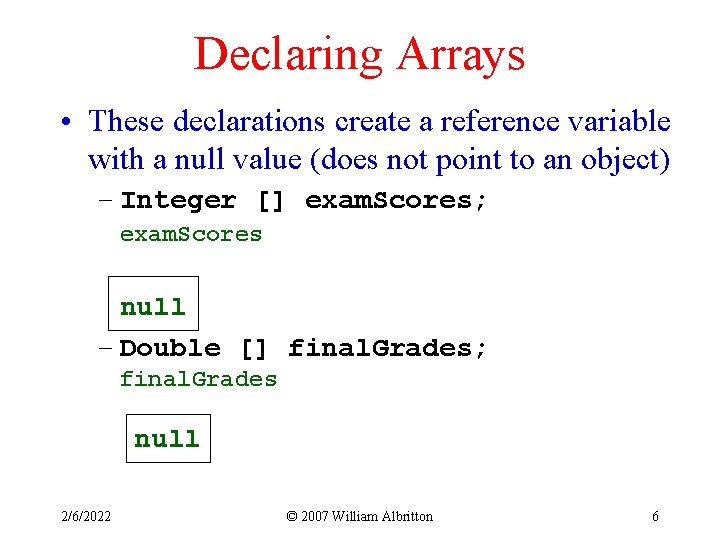
Declaring Arrays • These declarations create a reference variable with a null value (does not point to an object) – Integer [] exam. Scores; exam. Scores null – Double [] final. Grades; final. Grades null 2/6/2022 © 2007 William Albritton 6
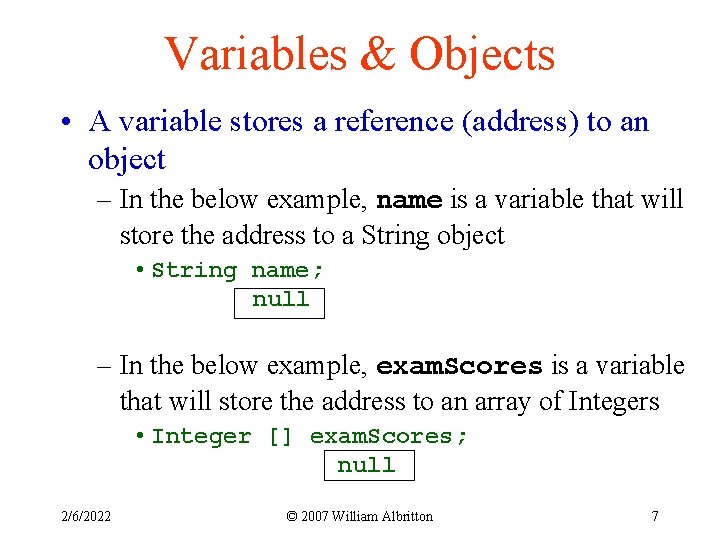
Variables & Objects • A variable stores a reference (address) to an object – In the below example, name is a variable that will store the address to a String object • String name; null – In the below example, exam. Scores is a variable that will store the address to an array of Integers • Integer [] exam. Scores; null 2/6/2022 © 2007 William Albritton 7
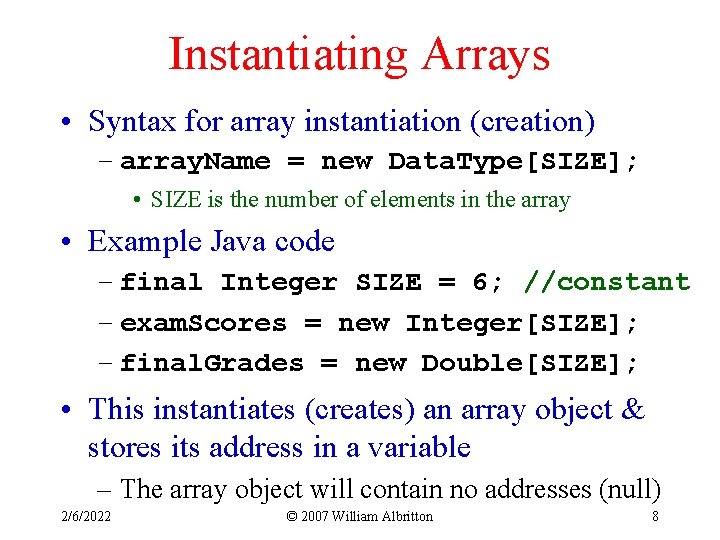
Instantiating Arrays • Syntax for array instantiation (creation) – array. Name = new Data. Type[SIZE]; • SIZE is the number of elements in the array • Example Java code – final Integer SIZE = 6; //constant – exam. Scores = new Integer[SIZE]; – final. Grades = new Double[SIZE]; • This instantiates (creates) an array object & stores its address in a variable – The array object will contain no addresses (null) 2/6/2022 © 2007 William Albritton 8
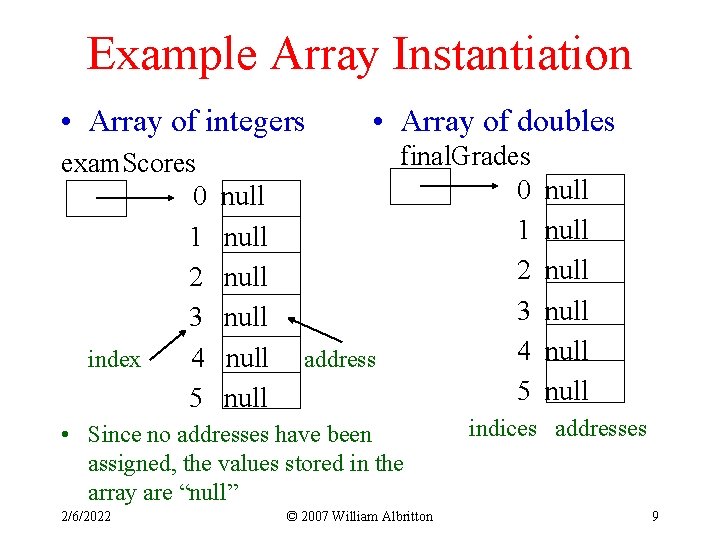
Example Array Instantiation • Array of integers exam. Scores 0 1 2 3 index 4 5 null null • Array of doubles final. Grades 0 1 2 3 4 address 5 • Since no addresses have been assigned, the values stored in the array are “null” 2/6/2022 © 2007 William Albritton null null indices addresses 9
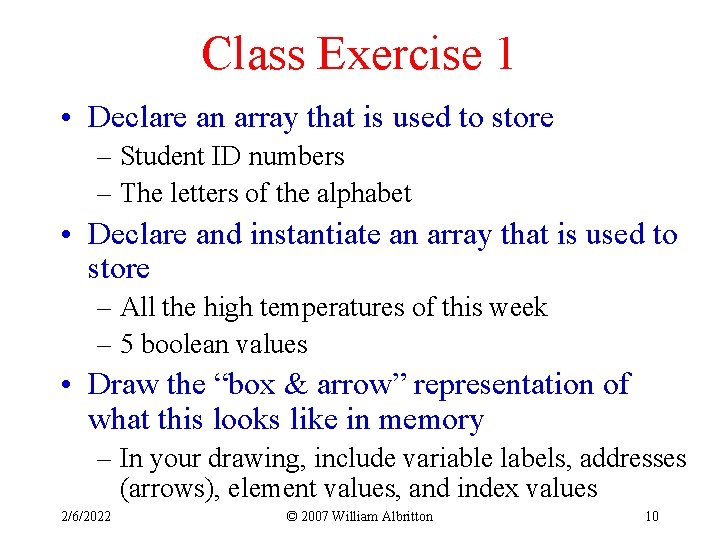
Class Exercise 1 • Declare an array that is used to store – Student ID numbers – The letters of the alphabet • Declare and instantiate an array that is used to store – All the high temperatures of this week – 5 boolean values • Draw the “box & arrow” representation of what this looks like in memory – In your drawing, include variable labels, addresses (arrows), element values, and index values 2/6/2022 © 2007 William Albritton 10
![Initializing Arrays Syntax for initializing arrays array Namei value Where Initializing Arrays • Syntax for initializing arrays – array. Name[i] = value; • Where](https://slidetodoc.com/presentation_image_h2/a6e266d1c2642b6cca1fc85e4bf71d5e/image-11.jpg)
Initializing Arrays • Syntax for initializing arrays – array. Name[i] = value; • Where i is the index of an element in the array • Example Java code – exam. Scores[0] – exam. Scores[1] – exam. Scores[2] – exam. Scores[3] – exam. Scores[4] – exam. Scores[5] 2/6/2022 = = = 80; 77; 92; 80; 63; 95; © 2007 William Albritton 11
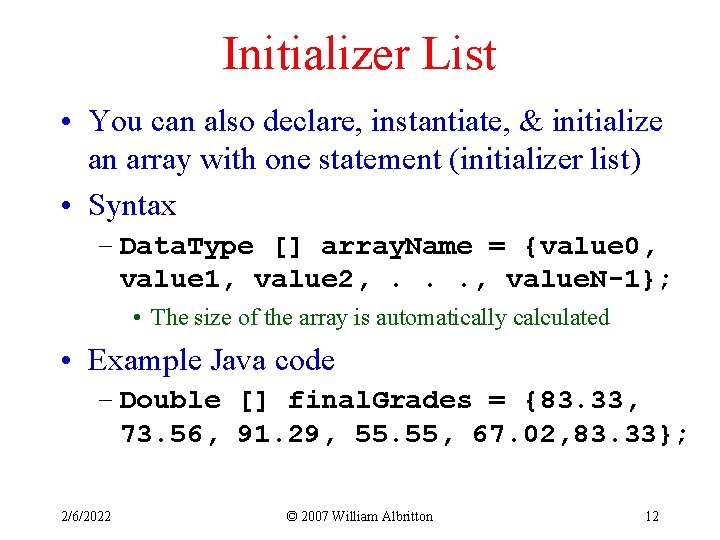
Initializer List • You can also declare, instantiate, & initialize an array with one statement (initializer list) • Syntax – Data. Type [] array. Name = {value 0, value 1, value 2, . . . , value. N-1}; • The size of the array is automatically calculated • Example Java code – Double [] final. Grades = {83. 33, 73. 56, 91. 29, 55. 55, 67. 02, 83. 33}; 2/6/2022 © 2007 William Albritton 12
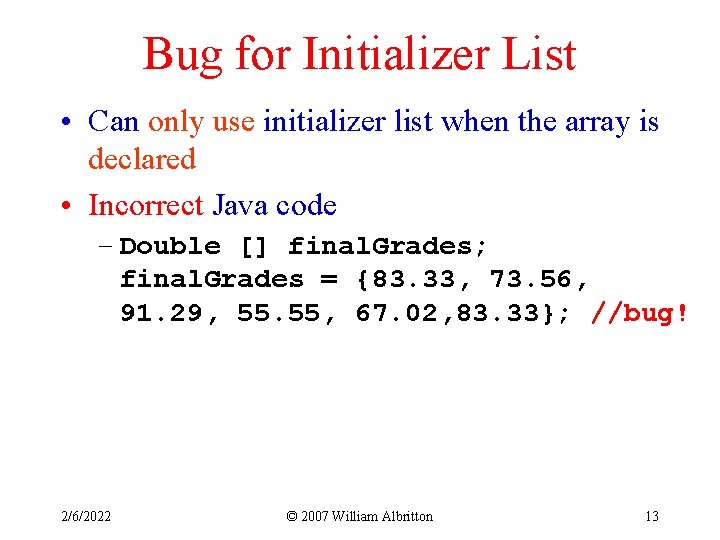
Bug for Initializer List • Can only use initializer list when the array is declared • Incorrect Java code – Double [] final. Grades; final. Grades = {83. 33, 73. 56, 91. 29, 55. 55, 67. 02, 83. 33}; //bug! 2/6/2022 © 2007 William Albritton 13
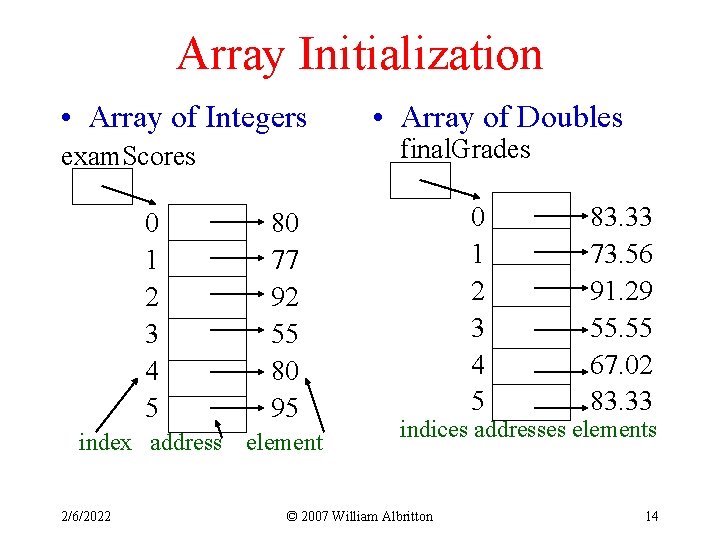
Array Initialization • Array of Integers exam. Scores 0 1 2 3 4 5 80 77 92 55 80 95 index address element 2/6/2022 • Array of Doubles final. Grades 0 1 2 3 4 5 83. 33 73. 56 91. 29 55. 55 67. 02 83. 33 indices addresses elements © 2007 William Albritton 14
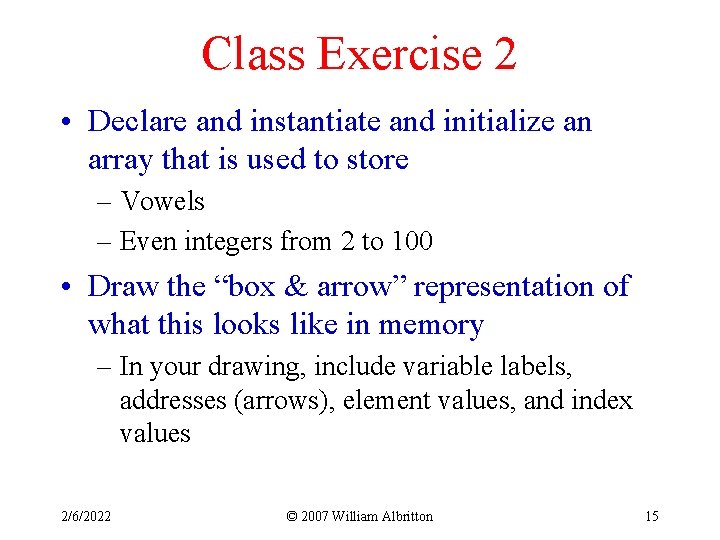
Class Exercise 2 • Declare and instantiate and initialize an array that is used to store – Vowels – Even integers from 2 to 100 • Draw the “box & arrow” representation of what this looks like in memory – In your drawing, include variable labels, addresses (arrows), element values, and index values 2/6/2022 © 2007 William Albritton 15
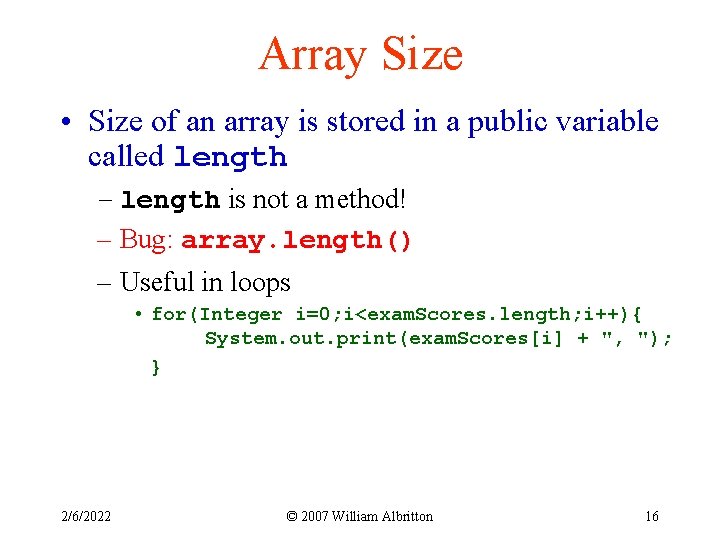
Array Size • Size of an array is stored in a public variable called length – length is not a method! – Bug: array. length() – Useful in loops • for(Integer i=0; i<exam. Scores. length; i++){ System. out. print(exam. Scores[i] + ", "); } 2/6/2022 © 2007 William Albritton 16
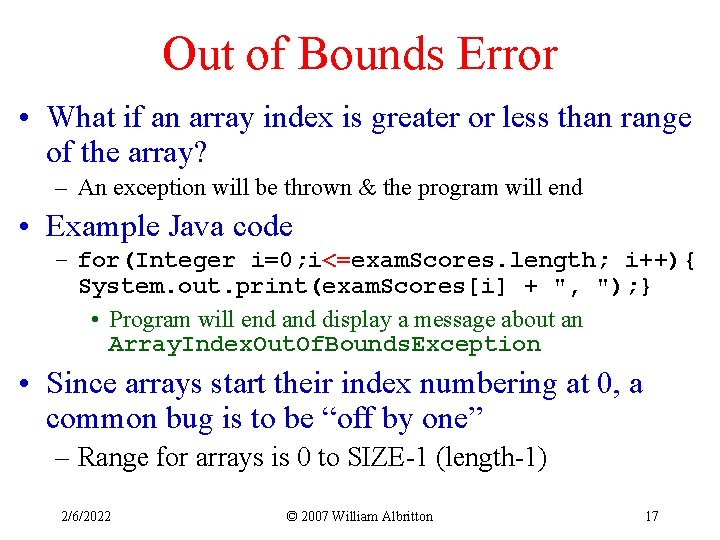
Out of Bounds Error • What if an array index is greater or less than range of the array? – An exception will be thrown & the program will end • Example Java code – for(Integer i=0; i<=exam. Scores. length; i++){ System. out. print(exam. Scores[i] + ", "); } • Program will end and display a message about an Array. Index. Out. Of. Bounds. Exception • Since arrays start their index numbering at 0, a common bug is to be “off by one” – Range for arrays is 0 to SIZE-1 (length-1) 2/6/2022 © 2007 William Albritton 17
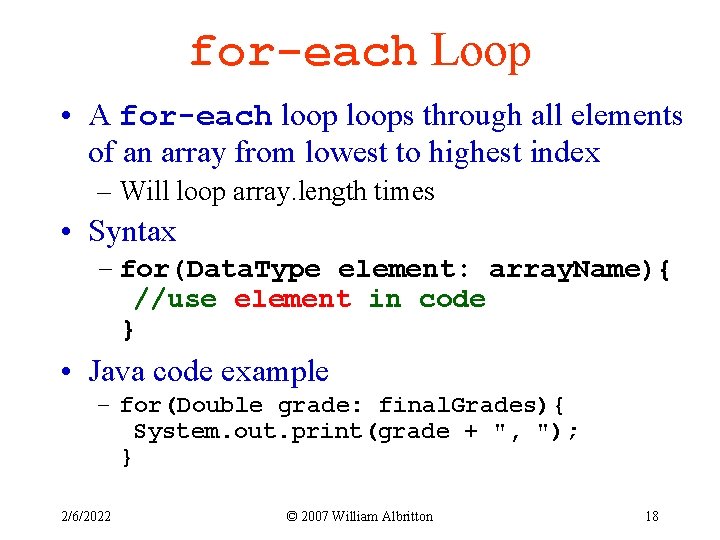
for-each Loop • A for-each loops through all elements of an array from lowest to highest index – Will loop array. length times • Syntax – for(Data. Type element: array. Name){ //use element in code } • Java code example – for(Double grade: final. Grades){ System. out. print(grade + ", "); } 2/6/2022 © 2007 William Albritton 18
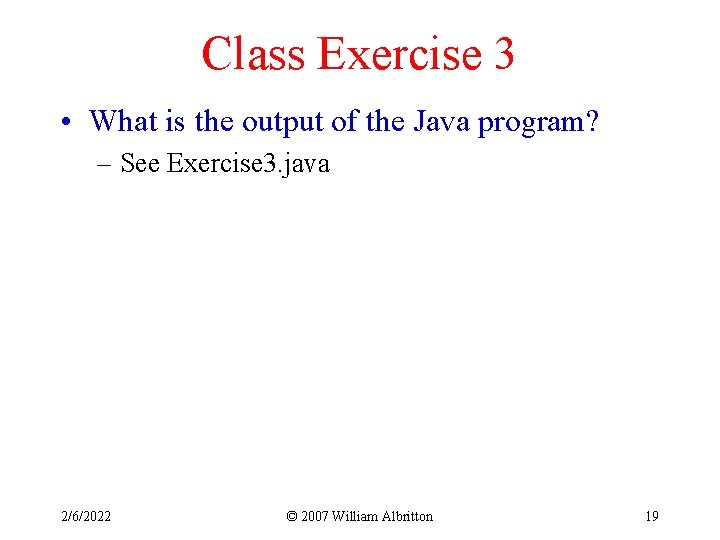
Class Exercise 3 • What is the output of the Java program? – See Exercise 3. java 2/6/2022 © 2007 William Albritton 19
Computer science arrays
011 101 001
Ics 111
Ics 33 midterm
Ics 111
Advanced incident management system
My favorite subject is music
It 111 introduction to computing
Think central k5
Introduction to computer science midterm exam
Introduction to computer science midterm exam test
C++ code
Python programming an introduction to computer science
Parallel arrays in data structure
Array of arrays c++
Ragged array
Partially filled arrays
C++ parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis